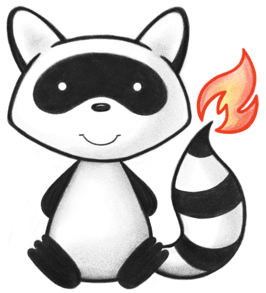
001package org.hl7.fhir.r5.liquid; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.exceptions.FHIRException; 007import org.hl7.fhir.r5.fhirpath.FHIRPathEngine; 008import org.hl7.fhir.r5.fhirpath.FHIRPathEngine.IEvaluationContext.FunctionDefinition; 009import org.hl7.fhir.r5.fhirpath.FHIRPathUtilityClasses.FunctionDetails; 010import org.hl7.fhir.r5.fhirpath.TypeDetails; 011import org.hl7.fhir.r5.fhirpath.ExpressionNode.CollectionStatus; 012import org.hl7.fhir.r5.model.Base; 013import org.hl7.fhir.r5.model.DateTimeType; 014import org.hl7.fhir.r5.model.IntegerType; 015import org.hl7.fhir.r5.model.StringType; 016import org.hl7.fhir.utilities.FhirPublication; 017import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 018import org.hl7.fhir.utilities.Utilities; 019 020import com.microsoft.schemas.office.visio.x2012.main.impl.FunctionDefTypeImpl; 021 022@MarkedToMoveToAdjunctPackage 023public class GlobalObject extends Base { 024 025 private DateTimeType dt; 026 private StringType pathToSpec; 027 028 public GlobalObject(DateTimeType td, StringType pathToSpec) { 029 super(); 030 this.dt = td; 031 this.pathToSpec = pathToSpec; 032 } 033 034 @Override 035 public String fhirType() { 036 return "GlobalObject"; 037 } 038 039 @Override 040 public String getIdBase() { 041 return null; 042 } 043 044 @Override 045 public void setIdBase(String value) { 046 throw new Error("Read only"); 047 } 048 049 @Override 050 public Base copy() { 051 return this; 052 } 053 054 @Override 055 public FhirPublication getFHIRPublicationVersion() { 056 return FhirPublication.R5; 057 } 058 059 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 060 if ("dateTime".equals(name)) { 061 return wrap(dt); 062 } else if ("path".equals(name)) { 063 return wrap(pathToSpec); 064 } else { 065 return super.getProperty(hash, name, checkValid); 066 } 067 } 068 069 private Base[] wrap(Base b) { 070 Base[] l = new Base[1]; 071 l[0] = b; 072 return l; 073 } 074 075 @Override 076 public List<Base> executeFunction(FHIRPathEngine engine, Object appContext, List<Base> focus, String functionName, List<List<Base>> parameters) { 077 return null; 078 } 079 080 public static class GlobalObjectRandomFunction extends FunctionDefinition { 081 082 @Override 083 public String name() { 084 return "random"; 085 } 086 087 @Override 088 public FunctionDetails details() { 089 return new FunctionDetails("Generate a Random Number", 1, 1); 090 } 091 092 @Override 093 public TypeDetails check(FHIRPathEngine engine, Object appContext, TypeDetails focus, List<TypeDetails> parameters) { 094 if (focus.hasType("GlobalObject")) { 095 return new TypeDetails(CollectionStatus.SINGLETON, "integer"); 096 } else { 097 return null; 098 } 099 } 100 101 @Override 102 public List<Base> execute(FHIRPathEngine engine, Object appContext, List<Base> focus, List<List<Base>> parameters) { 103 List<Base> list = new ArrayList<>(); 104 int scale = Utilities.parseInt(parameters.get(0).get(0).primitiveValue(), 100)+ 1; 105 list.add(new IntegerType((int)(Math.random() * scale))); 106 return list; 107 } 108 109 } 110}