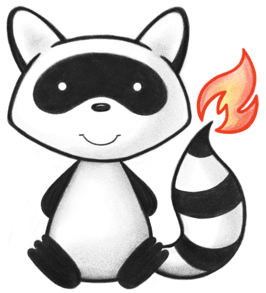
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 052 */ 053@ResourceDef(name="Account", profile="http://hl7.org/fhir/StructureDefinition/Account") 054public class Account extends DomainResource { 055 056 public enum AccountStatus { 057 /** 058 * This account is active and may be used. 059 */ 060 ACTIVE, 061 /** 062 * This account is inactive and should not be used to track financial information. 063 */ 064 INACTIVE, 065 /** 066 * This instance should not have been part of this patient's medical record. 067 */ 068 ENTEREDINERROR, 069 /** 070 * This account is on hold. 071 */ 072 ONHOLD, 073 /** 074 * The account status is unknown. 075 */ 076 UNKNOWN, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static AccountStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("active".equals(codeString)) 085 return ACTIVE; 086 if ("inactive".equals(codeString)) 087 return INACTIVE; 088 if ("entered-in-error".equals(codeString)) 089 return ENTEREDINERROR; 090 if ("on-hold".equals(codeString)) 091 return ONHOLD; 092 if ("unknown".equals(codeString)) 093 return UNKNOWN; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown AccountStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case ACTIVE: return "active"; 102 case INACTIVE: return "inactive"; 103 case ENTEREDINERROR: return "entered-in-error"; 104 case ONHOLD: return "on-hold"; 105 case UNKNOWN: return "unknown"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case ACTIVE: return "http://hl7.org/fhir/account-status"; 113 case INACTIVE: return "http://hl7.org/fhir/account-status"; 114 case ENTEREDINERROR: return "http://hl7.org/fhir/account-status"; 115 case ONHOLD: return "http://hl7.org/fhir/account-status"; 116 case UNKNOWN: return "http://hl7.org/fhir/account-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case ACTIVE: return "This account is active and may be used."; 124 case INACTIVE: return "This account is inactive and should not be used to track financial information."; 125 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 126 case ONHOLD: return "This account is on hold."; 127 case UNKNOWN: return "The account status is unknown."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case ACTIVE: return "Active"; 135 case INACTIVE: return "Inactive"; 136 case ENTEREDINERROR: return "Entered in error"; 137 case ONHOLD: return "On Hold"; 138 case UNKNOWN: return "Unknown"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class AccountStatusEnumFactory implements EnumFactory<AccountStatus> { 146 public AccountStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("active".equals(codeString)) 151 return AccountStatus.ACTIVE; 152 if ("inactive".equals(codeString)) 153 return AccountStatus.INACTIVE; 154 if ("entered-in-error".equals(codeString)) 155 return AccountStatus.ENTEREDINERROR; 156 if ("on-hold".equals(codeString)) 157 return AccountStatus.ONHOLD; 158 if ("unknown".equals(codeString)) 159 return AccountStatus.UNKNOWN; 160 throw new IllegalArgumentException("Unknown AccountStatus code '"+codeString+"'"); 161 } 162 public Enumeration<AccountStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<AccountStatus>(this, AccountStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<AccountStatus>(this, AccountStatus.NULL, code); 170 if ("active".equals(codeString)) 171 return new Enumeration<AccountStatus>(this, AccountStatus.ACTIVE, code); 172 if ("inactive".equals(codeString)) 173 return new Enumeration<AccountStatus>(this, AccountStatus.INACTIVE, code); 174 if ("entered-in-error".equals(codeString)) 175 return new Enumeration<AccountStatus>(this, AccountStatus.ENTEREDINERROR, code); 176 if ("on-hold".equals(codeString)) 177 return new Enumeration<AccountStatus>(this, AccountStatus.ONHOLD, code); 178 if ("unknown".equals(codeString)) 179 return new Enumeration<AccountStatus>(this, AccountStatus.UNKNOWN, code); 180 throw new FHIRException("Unknown AccountStatus code '"+codeString+"'"); 181 } 182 public String toCode(AccountStatus code) { 183 if (code == AccountStatus.NULL) 184 return null; 185 if (code == AccountStatus.ACTIVE) 186 return "active"; 187 if (code == AccountStatus.INACTIVE) 188 return "inactive"; 189 if (code == AccountStatus.ENTEREDINERROR) 190 return "entered-in-error"; 191 if (code == AccountStatus.ONHOLD) 192 return "on-hold"; 193 if (code == AccountStatus.UNKNOWN) 194 return "unknown"; 195 return "?"; 196 } 197 public String toSystem(AccountStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class CoverageComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay). 206 207A coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing. 208 */ 209 @Child(name = "coverage", type = {Coverage.class}, order=1, min=1, max=1, modifier=false, summary=true) 210 @Description(shortDefinition="The party(s), such as insurances, that may contribute to the payment of this account", formalDefinition="The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing." ) 211 protected Reference coverage; 212 213 /** 214 * The priority of the coverage in the context of this account. 215 */ 216 @Child(name = "priority", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 217 @Description(shortDefinition="The priority of the coverage in the context of this account", formalDefinition="The priority of the coverage in the context of this account." ) 218 protected PositiveIntType priority; 219 220 private static final long serialVersionUID = 1695665065L; 221 222 /** 223 * Constructor 224 */ 225 public CoverageComponent() { 226 super(); 227 } 228 229 /** 230 * Constructor 231 */ 232 public CoverageComponent(Reference coverage) { 233 super(); 234 this.setCoverage(coverage); 235 } 236 237 /** 238 * @return {@link #coverage} (The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay). 239 240A coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 241 */ 242 public Reference getCoverage() { 243 if (this.coverage == null) 244 if (Configuration.errorOnAutoCreate()) 245 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 246 else if (Configuration.doAutoCreate()) 247 this.coverage = new Reference(); // cc 248 return this.coverage; 249 } 250 251 public boolean hasCoverage() { 252 return this.coverage != null && !this.coverage.isEmpty(); 253 } 254 255 /** 256 * @param value {@link #coverage} (The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay). 257 258A coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.) 259 */ 260 public CoverageComponent setCoverage(Reference value) { 261 this.coverage = value; 262 return this; 263 } 264 265 /** 266 * @return {@link #priority} (The priority of the coverage in the context of this account.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 267 */ 268 public PositiveIntType getPriorityElement() { 269 if (this.priority == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create CoverageComponent.priority"); 272 else if (Configuration.doAutoCreate()) 273 this.priority = new PositiveIntType(); // bb 274 return this.priority; 275 } 276 277 public boolean hasPriorityElement() { 278 return this.priority != null && !this.priority.isEmpty(); 279 } 280 281 public boolean hasPriority() { 282 return this.priority != null && !this.priority.isEmpty(); 283 } 284 285 /** 286 * @param value {@link #priority} (The priority of the coverage in the context of this account.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 287 */ 288 public CoverageComponent setPriorityElement(PositiveIntType value) { 289 this.priority = value; 290 return this; 291 } 292 293 /** 294 * @return The priority of the coverage in the context of this account. 295 */ 296 public int getPriority() { 297 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 298 } 299 300 /** 301 * @param value The priority of the coverage in the context of this account. 302 */ 303 public CoverageComponent setPriority(int value) { 304 if (this.priority == null) 305 this.priority = new PositiveIntType(); 306 this.priority.setValue(value); 307 return this; 308 } 309 310 protected void listChildren(List<Property> children) { 311 super.listChildren(children); 312 children.add(new Property("coverage", "Reference(Coverage)", "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 0, 1, coverage)); 313 children.add(new Property("priority", "positiveInt", "The priority of the coverage in the context of this account.", 0, 1, priority)); 314 } 315 316 @Override 317 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 318 switch (_hash) { 319 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "The party(s) that contribute to payment (or part of) of the charges applied to this account (including self-pay).\n\nA coverage may only be responsible for specific types of charges, and the sequence of the coverages in the account could be important when processing billing.", 0, 1, coverage); 320 case -1165461084: /*priority*/ return new Property("priority", "positiveInt", "The priority of the coverage in the context of this account.", 0, 1, priority); 321 default: return super.getNamedProperty(_hash, _name, _checkValid); 322 } 323 324 } 325 326 @Override 327 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 328 switch (hash) { 329 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 330 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // PositiveIntType 331 default: return super.getProperty(hash, name, checkValid); 332 } 333 334 } 335 336 @Override 337 public Base setProperty(int hash, String name, Base value) throws FHIRException { 338 switch (hash) { 339 case -351767064: // coverage 340 this.coverage = TypeConvertor.castToReference(value); // Reference 341 return value; 342 case -1165461084: // priority 343 this.priority = TypeConvertor.castToPositiveInt(value); // PositiveIntType 344 return value; 345 default: return super.setProperty(hash, name, value); 346 } 347 348 } 349 350 @Override 351 public Base setProperty(String name, Base value) throws FHIRException { 352 if (name.equals("coverage")) { 353 this.coverage = TypeConvertor.castToReference(value); // Reference 354 } else if (name.equals("priority")) { 355 this.priority = TypeConvertor.castToPositiveInt(value); // PositiveIntType 356 } else 357 return super.setProperty(name, value); 358 return value; 359 } 360 361 @Override 362 public void removeChild(String name, Base value) throws FHIRException { 363 if (name.equals("coverage")) { 364 this.coverage = null; 365 } else if (name.equals("priority")) { 366 this.priority = null; 367 } else 368 super.removeChild(name, value); 369 370 } 371 372 @Override 373 public Base makeProperty(int hash, String name) throws FHIRException { 374 switch (hash) { 375 case -351767064: return getCoverage(); 376 case -1165461084: return getPriorityElement(); 377 default: return super.makeProperty(hash, name); 378 } 379 380 } 381 382 @Override 383 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 384 switch (hash) { 385 case -351767064: /*coverage*/ return new String[] {"Reference"}; 386 case -1165461084: /*priority*/ return new String[] {"positiveInt"}; 387 default: return super.getTypesForProperty(hash, name); 388 } 389 390 } 391 392 @Override 393 public Base addChild(String name) throws FHIRException { 394 if (name.equals("coverage")) { 395 this.coverage = new Reference(); 396 return this.coverage; 397 } 398 else if (name.equals("priority")) { 399 throw new FHIRException("Cannot call addChild on a singleton property Account.coverage.priority"); 400 } 401 else 402 return super.addChild(name); 403 } 404 405 public CoverageComponent copy() { 406 CoverageComponent dst = new CoverageComponent(); 407 copyValues(dst); 408 return dst; 409 } 410 411 public void copyValues(CoverageComponent dst) { 412 super.copyValues(dst); 413 dst.coverage = coverage == null ? null : coverage.copy(); 414 dst.priority = priority == null ? null : priority.copy(); 415 } 416 417 @Override 418 public boolean equalsDeep(Base other_) { 419 if (!super.equalsDeep(other_)) 420 return false; 421 if (!(other_ instanceof CoverageComponent)) 422 return false; 423 CoverageComponent o = (CoverageComponent) other_; 424 return compareDeep(coverage, o.coverage, true) && compareDeep(priority, o.priority, true); 425 } 426 427 @Override 428 public boolean equalsShallow(Base other_) { 429 if (!super.equalsShallow(other_)) 430 return false; 431 if (!(other_ instanceof CoverageComponent)) 432 return false; 433 CoverageComponent o = (CoverageComponent) other_; 434 return compareValues(priority, o.priority, true); 435 } 436 437 public boolean isEmpty() { 438 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, priority); 439 } 440 441 public String fhirType() { 442 return "Account.coverage"; 443 444 } 445 446 } 447 448 @Block() 449 public static class GuarantorComponent extends BackboneElement implements IBaseBackboneElement { 450 /** 451 * The entity who is responsible. 452 */ 453 @Child(name = "party", type = {Patient.class, RelatedPerson.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=false) 454 @Description(shortDefinition="Responsible entity", formalDefinition="The entity who is responsible." ) 455 protected Reference party; 456 457 /** 458 * A guarantor may be placed on credit hold or otherwise have their role temporarily suspended. 459 */ 460 @Child(name = "onHold", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 461 @Description(shortDefinition="Credit or other hold applied", formalDefinition="A guarantor may be placed on credit hold or otherwise have their role temporarily suspended." ) 462 protected BooleanType onHold; 463 464 /** 465 * The timeframe during which the guarantor accepts responsibility for the account. 466 */ 467 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 468 @Description(shortDefinition="Guarantee account during", formalDefinition="The timeframe during which the guarantor accepts responsibility for the account." ) 469 protected Period period; 470 471 private static final long serialVersionUID = -523056773L; 472 473 /** 474 * Constructor 475 */ 476 public GuarantorComponent() { 477 super(); 478 } 479 480 /** 481 * Constructor 482 */ 483 public GuarantorComponent(Reference party) { 484 super(); 485 this.setParty(party); 486 } 487 488 /** 489 * @return {@link #party} (The entity who is responsible.) 490 */ 491 public Reference getParty() { 492 if (this.party == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create GuarantorComponent.party"); 495 else if (Configuration.doAutoCreate()) 496 this.party = new Reference(); // cc 497 return this.party; 498 } 499 500 public boolean hasParty() { 501 return this.party != null && !this.party.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #party} (The entity who is responsible.) 506 */ 507 public GuarantorComponent setParty(Reference value) { 508 this.party = value; 509 return this; 510 } 511 512 /** 513 * @return {@link #onHold} (A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.). This is the underlying object with id, value and extensions. The accessor "getOnHold" gives direct access to the value 514 */ 515 public BooleanType getOnHoldElement() { 516 if (this.onHold == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create GuarantorComponent.onHold"); 519 else if (Configuration.doAutoCreate()) 520 this.onHold = new BooleanType(); // bb 521 return this.onHold; 522 } 523 524 public boolean hasOnHoldElement() { 525 return this.onHold != null && !this.onHold.isEmpty(); 526 } 527 528 public boolean hasOnHold() { 529 return this.onHold != null && !this.onHold.isEmpty(); 530 } 531 532 /** 533 * @param value {@link #onHold} (A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.). This is the underlying object with id, value and extensions. The accessor "getOnHold" gives direct access to the value 534 */ 535 public GuarantorComponent setOnHoldElement(BooleanType value) { 536 this.onHold = value; 537 return this; 538 } 539 540 /** 541 * @return A guarantor may be placed on credit hold or otherwise have their role temporarily suspended. 542 */ 543 public boolean getOnHold() { 544 return this.onHold == null || this.onHold.isEmpty() ? false : this.onHold.getValue(); 545 } 546 547 /** 548 * @param value A guarantor may be placed on credit hold or otherwise have their role temporarily suspended. 549 */ 550 public GuarantorComponent setOnHold(boolean value) { 551 if (this.onHold == null) 552 this.onHold = new BooleanType(); 553 this.onHold.setValue(value); 554 return this; 555 } 556 557 /** 558 * @return {@link #period} (The timeframe during which the guarantor accepts responsibility for the account.) 559 */ 560 public Period getPeriod() { 561 if (this.period == null) 562 if (Configuration.errorOnAutoCreate()) 563 throw new Error("Attempt to auto-create GuarantorComponent.period"); 564 else if (Configuration.doAutoCreate()) 565 this.period = new Period(); // cc 566 return this.period; 567 } 568 569 public boolean hasPeriod() { 570 return this.period != null && !this.period.isEmpty(); 571 } 572 573 /** 574 * @param value {@link #period} (The timeframe during which the guarantor accepts responsibility for the account.) 575 */ 576 public GuarantorComponent setPeriod(Period value) { 577 this.period = value; 578 return this; 579 } 580 581 protected void listChildren(List<Property> children) { 582 super.listChildren(children); 583 children.add(new Property("party", "Reference(Patient|RelatedPerson|Organization)", "The entity who is responsible.", 0, 1, party)); 584 children.add(new Property("onHold", "boolean", "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, onHold)); 585 children.add(new Property("period", "Period", "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period)); 586 } 587 588 @Override 589 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 590 switch (_hash) { 591 case 106437350: /*party*/ return new Property("party", "Reference(Patient|RelatedPerson|Organization)", "The entity who is responsible.", 0, 1, party); 592 case -1013289154: /*onHold*/ return new Property("onHold", "boolean", "A guarantor may be placed on credit hold or otherwise have their role temporarily suspended.", 0, 1, onHold); 593 case -991726143: /*period*/ return new Property("period", "Period", "The timeframe during which the guarantor accepts responsibility for the account.", 0, 1, period); 594 default: return super.getNamedProperty(_hash, _name, _checkValid); 595 } 596 597 } 598 599 @Override 600 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 601 switch (hash) { 602 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 603 case -1013289154: /*onHold*/ return this.onHold == null ? new Base[0] : new Base[] {this.onHold}; // BooleanType 604 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 605 default: return super.getProperty(hash, name, checkValid); 606 } 607 608 } 609 610 @Override 611 public Base setProperty(int hash, String name, Base value) throws FHIRException { 612 switch (hash) { 613 case 106437350: // party 614 this.party = TypeConvertor.castToReference(value); // Reference 615 return value; 616 case -1013289154: // onHold 617 this.onHold = TypeConvertor.castToBoolean(value); // BooleanType 618 return value; 619 case -991726143: // period 620 this.period = TypeConvertor.castToPeriod(value); // Period 621 return value; 622 default: return super.setProperty(hash, name, value); 623 } 624 625 } 626 627 @Override 628 public Base setProperty(String name, Base value) throws FHIRException { 629 if (name.equals("party")) { 630 this.party = TypeConvertor.castToReference(value); // Reference 631 } else if (name.equals("onHold")) { 632 this.onHold = TypeConvertor.castToBoolean(value); // BooleanType 633 } else if (name.equals("period")) { 634 this.period = TypeConvertor.castToPeriod(value); // Period 635 } else 636 return super.setProperty(name, value); 637 return value; 638 } 639 640 @Override 641 public void removeChild(String name, Base value) throws FHIRException { 642 if (name.equals("party")) { 643 this.party = null; 644 } else if (name.equals("onHold")) { 645 this.onHold = null; 646 } else if (name.equals("period")) { 647 this.period = null; 648 } else 649 super.removeChild(name, value); 650 651 } 652 653 @Override 654 public Base makeProperty(int hash, String name) throws FHIRException { 655 switch (hash) { 656 case 106437350: return getParty(); 657 case -1013289154: return getOnHoldElement(); 658 case -991726143: return getPeriod(); 659 default: return super.makeProperty(hash, name); 660 } 661 662 } 663 664 @Override 665 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 666 switch (hash) { 667 case 106437350: /*party*/ return new String[] {"Reference"}; 668 case -1013289154: /*onHold*/ return new String[] {"boolean"}; 669 case -991726143: /*period*/ return new String[] {"Period"}; 670 default: return super.getTypesForProperty(hash, name); 671 } 672 673 } 674 675 @Override 676 public Base addChild(String name) throws FHIRException { 677 if (name.equals("party")) { 678 this.party = new Reference(); 679 return this.party; 680 } 681 else if (name.equals("onHold")) { 682 throw new FHIRException("Cannot call addChild on a singleton property Account.guarantor.onHold"); 683 } 684 else if (name.equals("period")) { 685 this.period = new Period(); 686 return this.period; 687 } 688 else 689 return super.addChild(name); 690 } 691 692 public GuarantorComponent copy() { 693 GuarantorComponent dst = new GuarantorComponent(); 694 copyValues(dst); 695 return dst; 696 } 697 698 public void copyValues(GuarantorComponent dst) { 699 super.copyValues(dst); 700 dst.party = party == null ? null : party.copy(); 701 dst.onHold = onHold == null ? null : onHold.copy(); 702 dst.period = period == null ? null : period.copy(); 703 } 704 705 @Override 706 public boolean equalsDeep(Base other_) { 707 if (!super.equalsDeep(other_)) 708 return false; 709 if (!(other_ instanceof GuarantorComponent)) 710 return false; 711 GuarantorComponent o = (GuarantorComponent) other_; 712 return compareDeep(party, o.party, true) && compareDeep(onHold, o.onHold, true) && compareDeep(period, o.period, true) 713 ; 714 } 715 716 @Override 717 public boolean equalsShallow(Base other_) { 718 if (!super.equalsShallow(other_)) 719 return false; 720 if (!(other_ instanceof GuarantorComponent)) 721 return false; 722 GuarantorComponent o = (GuarantorComponent) other_; 723 return compareValues(onHold, o.onHold, true); 724 } 725 726 public boolean isEmpty() { 727 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(party, onHold, period); 728 } 729 730 public String fhirType() { 731 return "Account.guarantor"; 732 733 } 734 735 } 736 737 @Block() 738 public static class AccountDiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 739 /** 740 * Ranking of the diagnosis (for each type). 741 */ 742 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 743 @Description(shortDefinition="Ranking of the diagnosis (for each type)", formalDefinition="Ranking of the diagnosis (for each type)." ) 744 protected PositiveIntType sequence; 745 746 /** 747 * The diagnosis relevant to the account. 748 */ 749 @Child(name = "condition", type = {CodeableReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 750 @Description(shortDefinition="The diagnosis relevant to the account", formalDefinition="The diagnosis relevant to the account." ) 751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 752 protected CodeableReference condition; 753 754 /** 755 * Ranking of the diagnosis (for each type). 756 */ 757 @Child(name = "dateOfDiagnosis", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 758 @Description(shortDefinition="Date of the diagnosis (when coded diagnosis)", formalDefinition="Ranking of the diagnosis (for each type)." ) 759 protected DateTimeType dateOfDiagnosis; 760 761 /** 762 * Type that this diagnosis has relevant to the account (e.g. admission, billing, discharge ?). 763 */ 764 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 765 @Description(shortDefinition="Type that this diagnosis has relevant to the account (e.g. admission, billing, discharge ?)", formalDefinition="Type that this diagnosis has relevant to the account (e.g. admission, billing, discharge ?)." ) 766 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diagnosis-use") 767 protected List<CodeableConcept> type; 768 769 /** 770 * Was the Diagnosis present on Admission in the related Encounter. 771 */ 772 @Child(name = "onAdmission", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=false) 773 @Description(shortDefinition="Diagnosis present on Admission", formalDefinition="Was the Diagnosis present on Admission in the related Encounter." ) 774 protected BooleanType onAdmission; 775 776 /** 777 * The package code can be used to group diagnoses that may be priced or delivered as a single product. Such as DRGs. 778 */ 779 @Child(name = "packageCode", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 780 @Description(shortDefinition="Package Code specific for billing", formalDefinition="The package code can be used to group diagnoses that may be priced or delivered as a single product. Such as DRGs." ) 781 protected List<CodeableConcept> packageCode; 782 783 private static final long serialVersionUID = 57125500L; 784 785 /** 786 * Constructor 787 */ 788 public AccountDiagnosisComponent() { 789 super(); 790 } 791 792 /** 793 * Constructor 794 */ 795 public AccountDiagnosisComponent(CodeableReference condition) { 796 super(); 797 this.setCondition(condition); 798 } 799 800 /** 801 * @return {@link #sequence} (Ranking of the diagnosis (for each type).). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 802 */ 803 public PositiveIntType getSequenceElement() { 804 if (this.sequence == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create AccountDiagnosisComponent.sequence"); 807 else if (Configuration.doAutoCreate()) 808 this.sequence = new PositiveIntType(); // bb 809 return this.sequence; 810 } 811 812 public boolean hasSequenceElement() { 813 return this.sequence != null && !this.sequence.isEmpty(); 814 } 815 816 public boolean hasSequence() { 817 return this.sequence != null && !this.sequence.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #sequence} (Ranking of the diagnosis (for each type).). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 822 */ 823 public AccountDiagnosisComponent setSequenceElement(PositiveIntType value) { 824 this.sequence = value; 825 return this; 826 } 827 828 /** 829 * @return Ranking of the diagnosis (for each type). 830 */ 831 public int getSequence() { 832 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 833 } 834 835 /** 836 * @param value Ranking of the diagnosis (for each type). 837 */ 838 public AccountDiagnosisComponent setSequence(int value) { 839 if (this.sequence == null) 840 this.sequence = new PositiveIntType(); 841 this.sequence.setValue(value); 842 return this; 843 } 844 845 /** 846 * @return {@link #condition} (The diagnosis relevant to the account.) 847 */ 848 public CodeableReference getCondition() { 849 if (this.condition == null) 850 if (Configuration.errorOnAutoCreate()) 851 throw new Error("Attempt to auto-create AccountDiagnosisComponent.condition"); 852 else if (Configuration.doAutoCreate()) 853 this.condition = new CodeableReference(); // cc 854 return this.condition; 855 } 856 857 public boolean hasCondition() { 858 return this.condition != null && !this.condition.isEmpty(); 859 } 860 861 /** 862 * @param value {@link #condition} (The diagnosis relevant to the account.) 863 */ 864 public AccountDiagnosisComponent setCondition(CodeableReference value) { 865 this.condition = value; 866 return this; 867 } 868 869 /** 870 * @return {@link #dateOfDiagnosis} (Ranking of the diagnosis (for each type).). This is the underlying object with id, value and extensions. The accessor "getDateOfDiagnosis" gives direct access to the value 871 */ 872 public DateTimeType getDateOfDiagnosisElement() { 873 if (this.dateOfDiagnosis == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create AccountDiagnosisComponent.dateOfDiagnosis"); 876 else if (Configuration.doAutoCreate()) 877 this.dateOfDiagnosis = new DateTimeType(); // bb 878 return this.dateOfDiagnosis; 879 } 880 881 public boolean hasDateOfDiagnosisElement() { 882 return this.dateOfDiagnosis != null && !this.dateOfDiagnosis.isEmpty(); 883 } 884 885 public boolean hasDateOfDiagnosis() { 886 return this.dateOfDiagnosis != null && !this.dateOfDiagnosis.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #dateOfDiagnosis} (Ranking of the diagnosis (for each type).). This is the underlying object with id, value and extensions. The accessor "getDateOfDiagnosis" gives direct access to the value 891 */ 892 public AccountDiagnosisComponent setDateOfDiagnosisElement(DateTimeType value) { 893 this.dateOfDiagnosis = value; 894 return this; 895 } 896 897 /** 898 * @return Ranking of the diagnosis (for each type). 899 */ 900 public Date getDateOfDiagnosis() { 901 return this.dateOfDiagnosis == null ? null : this.dateOfDiagnosis.getValue(); 902 } 903 904 /** 905 * @param value Ranking of the diagnosis (for each type). 906 */ 907 public AccountDiagnosisComponent setDateOfDiagnosis(Date value) { 908 if (value == null) 909 this.dateOfDiagnosis = null; 910 else { 911 if (this.dateOfDiagnosis == null) 912 this.dateOfDiagnosis = new DateTimeType(); 913 this.dateOfDiagnosis.setValue(value); 914 } 915 return this; 916 } 917 918 /** 919 * @return {@link #type} (Type that this diagnosis has relevant to the account (e.g. admission, billing, discharge ?).) 920 */ 921 public List<CodeableConcept> getType() { 922 if (this.type == null) 923 this.type = new ArrayList<CodeableConcept>(); 924 return this.type; 925 } 926 927 /** 928 * @return Returns a reference to <code>this</code> for easy method chaining 929 */ 930 public AccountDiagnosisComponent setType(List<CodeableConcept> theType) { 931 this.type = theType; 932 return this; 933 } 934 935 public boolean hasType() { 936 if (this.type == null) 937 return false; 938 for (CodeableConcept item : this.type) 939 if (!item.isEmpty()) 940 return true; 941 return false; 942 } 943 944 public CodeableConcept addType() { //3 945 CodeableConcept t = new CodeableConcept(); 946 if (this.type == null) 947 this.type = new ArrayList<CodeableConcept>(); 948 this.type.add(t); 949 return t; 950 } 951 952 public AccountDiagnosisComponent addType(CodeableConcept t) { //3 953 if (t == null) 954 return this; 955 if (this.type == null) 956 this.type = new ArrayList<CodeableConcept>(); 957 this.type.add(t); 958 return this; 959 } 960 961 /** 962 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 963 */ 964 public CodeableConcept getTypeFirstRep() { 965 if (getType().isEmpty()) { 966 addType(); 967 } 968 return getType().get(0); 969 } 970 971 /** 972 * @return {@link #onAdmission} (Was the Diagnosis present on Admission in the related Encounter.). This is the underlying object with id, value and extensions. The accessor "getOnAdmission" gives direct access to the value 973 */ 974 public BooleanType getOnAdmissionElement() { 975 if (this.onAdmission == null) 976 if (Configuration.errorOnAutoCreate()) 977 throw new Error("Attempt to auto-create AccountDiagnosisComponent.onAdmission"); 978 else if (Configuration.doAutoCreate()) 979 this.onAdmission = new BooleanType(); // bb 980 return this.onAdmission; 981 } 982 983 public boolean hasOnAdmissionElement() { 984 return this.onAdmission != null && !this.onAdmission.isEmpty(); 985 } 986 987 public boolean hasOnAdmission() { 988 return this.onAdmission != null && !this.onAdmission.isEmpty(); 989 } 990 991 /** 992 * @param value {@link #onAdmission} (Was the Diagnosis present on Admission in the related Encounter.). This is the underlying object with id, value and extensions. The accessor "getOnAdmission" gives direct access to the value 993 */ 994 public AccountDiagnosisComponent setOnAdmissionElement(BooleanType value) { 995 this.onAdmission = value; 996 return this; 997 } 998 999 /** 1000 * @return Was the Diagnosis present on Admission in the related Encounter. 1001 */ 1002 public boolean getOnAdmission() { 1003 return this.onAdmission == null || this.onAdmission.isEmpty() ? false : this.onAdmission.getValue(); 1004 } 1005 1006 /** 1007 * @param value Was the Diagnosis present on Admission in the related Encounter. 1008 */ 1009 public AccountDiagnosisComponent setOnAdmission(boolean value) { 1010 if (this.onAdmission == null) 1011 this.onAdmission = new BooleanType(); 1012 this.onAdmission.setValue(value); 1013 return this; 1014 } 1015 1016 /** 1017 * @return {@link #packageCode} (The package code can be used to group diagnoses that may be priced or delivered as a single product. Such as DRGs.) 1018 */ 1019 public List<CodeableConcept> getPackageCode() { 1020 if (this.packageCode == null) 1021 this.packageCode = new ArrayList<CodeableConcept>(); 1022 return this.packageCode; 1023 } 1024 1025 /** 1026 * @return Returns a reference to <code>this</code> for easy method chaining 1027 */ 1028 public AccountDiagnosisComponent setPackageCode(List<CodeableConcept> thePackageCode) { 1029 this.packageCode = thePackageCode; 1030 return this; 1031 } 1032 1033 public boolean hasPackageCode() { 1034 if (this.packageCode == null) 1035 return false; 1036 for (CodeableConcept item : this.packageCode) 1037 if (!item.isEmpty()) 1038 return true; 1039 return false; 1040 } 1041 1042 public CodeableConcept addPackageCode() { //3 1043 CodeableConcept t = new CodeableConcept(); 1044 if (this.packageCode == null) 1045 this.packageCode = new ArrayList<CodeableConcept>(); 1046 this.packageCode.add(t); 1047 return t; 1048 } 1049 1050 public AccountDiagnosisComponent addPackageCode(CodeableConcept t) { //3 1051 if (t == null) 1052 return this; 1053 if (this.packageCode == null) 1054 this.packageCode = new ArrayList<CodeableConcept>(); 1055 this.packageCode.add(t); 1056 return this; 1057 } 1058 1059 /** 1060 * @return The first repetition of repeating field {@link #packageCode}, creating it if it does not already exist {3} 1061 */ 1062 public CodeableConcept getPackageCodeFirstRep() { 1063 if (getPackageCode().isEmpty()) { 1064 addPackageCode(); 1065 } 1066 return getPackageCode().get(0); 1067 } 1068 1069 protected void listChildren(List<Property> children) { 1070 super.listChildren(children); 1071 children.add(new Property("sequence", "positiveInt", "Ranking of the diagnosis (for each type).", 0, 1, sequence)); 1072 children.add(new Property("condition", "CodeableReference(Condition)", "The diagnosis relevant to the account.", 0, 1, condition)); 1073 children.add(new Property("dateOfDiagnosis", "dateTime", "Ranking of the diagnosis (for each type).", 0, 1, dateOfDiagnosis)); 1074 children.add(new Property("type", "CodeableConcept", "Type that this diagnosis has relevant to the account (e.g. admission, billing, discharge ?).", 0, java.lang.Integer.MAX_VALUE, type)); 1075 children.add(new Property("onAdmission", "boolean", "Was the Diagnosis present on Admission in the related Encounter.", 0, 1, onAdmission)); 1076 children.add(new Property("packageCode", "CodeableConcept", "The package code can be used to group diagnoses that may be priced or delivered as a single product. Such as DRGs.", 0, java.lang.Integer.MAX_VALUE, packageCode)); 1077 } 1078 1079 @Override 1080 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1081 switch (_hash) { 1082 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Ranking of the diagnosis (for each type).", 0, 1, sequence); 1083 case -861311717: /*condition*/ return new Property("condition", "CodeableReference(Condition)", "The diagnosis relevant to the account.", 0, 1, condition); 1084 case -774562228: /*dateOfDiagnosis*/ return new Property("dateOfDiagnosis", "dateTime", "Ranking of the diagnosis (for each type).", 0, 1, dateOfDiagnosis); 1085 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type that this diagnosis has relevant to the account (e.g. admission, billing, discharge ?).", 0, java.lang.Integer.MAX_VALUE, type); 1086 case -3386134: /*onAdmission*/ return new Property("onAdmission", "boolean", "Was the Diagnosis present on Admission in the related Encounter.", 0, 1, onAdmission); 1087 case 908444499: /*packageCode*/ return new Property("packageCode", "CodeableConcept", "The package code can be used to group diagnoses that may be priced or delivered as a single product. Such as DRGs.", 0, java.lang.Integer.MAX_VALUE, packageCode); 1088 default: return super.getNamedProperty(_hash, _name, _checkValid); 1089 } 1090 1091 } 1092 1093 @Override 1094 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1095 switch (hash) { 1096 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1097 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // CodeableReference 1098 case -774562228: /*dateOfDiagnosis*/ return this.dateOfDiagnosis == null ? new Base[0] : new Base[] {this.dateOfDiagnosis}; // DateTimeType 1099 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1100 case -3386134: /*onAdmission*/ return this.onAdmission == null ? new Base[0] : new Base[] {this.onAdmission}; // BooleanType 1101 case 908444499: /*packageCode*/ return this.packageCode == null ? new Base[0] : this.packageCode.toArray(new Base[this.packageCode.size()]); // CodeableConcept 1102 default: return super.getProperty(hash, name, checkValid); 1103 } 1104 1105 } 1106 1107 @Override 1108 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1109 switch (hash) { 1110 case 1349547969: // sequence 1111 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1112 return value; 1113 case -861311717: // condition 1114 this.condition = TypeConvertor.castToCodeableReference(value); // CodeableReference 1115 return value; 1116 case -774562228: // dateOfDiagnosis 1117 this.dateOfDiagnosis = TypeConvertor.castToDateTime(value); // DateTimeType 1118 return value; 1119 case 3575610: // type 1120 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1121 return value; 1122 case -3386134: // onAdmission 1123 this.onAdmission = TypeConvertor.castToBoolean(value); // BooleanType 1124 return value; 1125 case 908444499: // packageCode 1126 this.getPackageCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1127 return value; 1128 default: return super.setProperty(hash, name, value); 1129 } 1130 1131 } 1132 1133 @Override 1134 public Base setProperty(String name, Base value) throws FHIRException { 1135 if (name.equals("sequence")) { 1136 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1137 } else if (name.equals("condition")) { 1138 this.condition = TypeConvertor.castToCodeableReference(value); // CodeableReference 1139 } else if (name.equals("dateOfDiagnosis")) { 1140 this.dateOfDiagnosis = TypeConvertor.castToDateTime(value); // DateTimeType 1141 } else if (name.equals("type")) { 1142 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1143 } else if (name.equals("onAdmission")) { 1144 this.onAdmission = TypeConvertor.castToBoolean(value); // BooleanType 1145 } else if (name.equals("packageCode")) { 1146 this.getPackageCode().add(TypeConvertor.castToCodeableConcept(value)); 1147 } else 1148 return super.setProperty(name, value); 1149 return value; 1150 } 1151 1152 @Override 1153 public void removeChild(String name, Base value) throws FHIRException { 1154 if (name.equals("sequence")) { 1155 this.sequence = null; 1156 } else if (name.equals("condition")) { 1157 this.condition = null; 1158 } else if (name.equals("dateOfDiagnosis")) { 1159 this.dateOfDiagnosis = null; 1160 } else if (name.equals("type")) { 1161 this.getType().remove(value); 1162 } else if (name.equals("onAdmission")) { 1163 this.onAdmission = null; 1164 } else if (name.equals("packageCode")) { 1165 this.getPackageCode().remove(value); 1166 } else 1167 super.removeChild(name, value); 1168 1169 } 1170 1171 @Override 1172 public Base makeProperty(int hash, String name) throws FHIRException { 1173 switch (hash) { 1174 case 1349547969: return getSequenceElement(); 1175 case -861311717: return getCondition(); 1176 case -774562228: return getDateOfDiagnosisElement(); 1177 case 3575610: return addType(); 1178 case -3386134: return getOnAdmissionElement(); 1179 case 908444499: return addPackageCode(); 1180 default: return super.makeProperty(hash, name); 1181 } 1182 1183 } 1184 1185 @Override 1186 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1187 switch (hash) { 1188 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1189 case -861311717: /*condition*/ return new String[] {"CodeableReference"}; 1190 case -774562228: /*dateOfDiagnosis*/ return new String[] {"dateTime"}; 1191 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1192 case -3386134: /*onAdmission*/ return new String[] {"boolean"}; 1193 case 908444499: /*packageCode*/ return new String[] {"CodeableConcept"}; 1194 default: return super.getTypesForProperty(hash, name); 1195 } 1196 1197 } 1198 1199 @Override 1200 public Base addChild(String name) throws FHIRException { 1201 if (name.equals("sequence")) { 1202 throw new FHIRException("Cannot call addChild on a singleton property Account.diagnosis.sequence"); 1203 } 1204 else if (name.equals("condition")) { 1205 this.condition = new CodeableReference(); 1206 return this.condition; 1207 } 1208 else if (name.equals("dateOfDiagnosis")) { 1209 throw new FHIRException("Cannot call addChild on a singleton property Account.diagnosis.dateOfDiagnosis"); 1210 } 1211 else if (name.equals("type")) { 1212 return addType(); 1213 } 1214 else if (name.equals("onAdmission")) { 1215 throw new FHIRException("Cannot call addChild on a singleton property Account.diagnosis.onAdmission"); 1216 } 1217 else if (name.equals("packageCode")) { 1218 return addPackageCode(); 1219 } 1220 else 1221 return super.addChild(name); 1222 } 1223 1224 public AccountDiagnosisComponent copy() { 1225 AccountDiagnosisComponent dst = new AccountDiagnosisComponent(); 1226 copyValues(dst); 1227 return dst; 1228 } 1229 1230 public void copyValues(AccountDiagnosisComponent dst) { 1231 super.copyValues(dst); 1232 dst.sequence = sequence == null ? null : sequence.copy(); 1233 dst.condition = condition == null ? null : condition.copy(); 1234 dst.dateOfDiagnosis = dateOfDiagnosis == null ? null : dateOfDiagnosis.copy(); 1235 if (type != null) { 1236 dst.type = new ArrayList<CodeableConcept>(); 1237 for (CodeableConcept i : type) 1238 dst.type.add(i.copy()); 1239 }; 1240 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 1241 if (packageCode != null) { 1242 dst.packageCode = new ArrayList<CodeableConcept>(); 1243 for (CodeableConcept i : packageCode) 1244 dst.packageCode.add(i.copy()); 1245 }; 1246 } 1247 1248 @Override 1249 public boolean equalsDeep(Base other_) { 1250 if (!super.equalsDeep(other_)) 1251 return false; 1252 if (!(other_ instanceof AccountDiagnosisComponent)) 1253 return false; 1254 AccountDiagnosisComponent o = (AccountDiagnosisComponent) other_; 1255 return compareDeep(sequence, o.sequence, true) && compareDeep(condition, o.condition, true) && compareDeep(dateOfDiagnosis, o.dateOfDiagnosis, true) 1256 && compareDeep(type, o.type, true) && compareDeep(onAdmission, o.onAdmission, true) && compareDeep(packageCode, o.packageCode, true) 1257 ; 1258 } 1259 1260 @Override 1261 public boolean equalsShallow(Base other_) { 1262 if (!super.equalsShallow(other_)) 1263 return false; 1264 if (!(other_ instanceof AccountDiagnosisComponent)) 1265 return false; 1266 AccountDiagnosisComponent o = (AccountDiagnosisComponent) other_; 1267 return compareValues(sequence, o.sequence, true) && compareValues(dateOfDiagnosis, o.dateOfDiagnosis, true) 1268 && compareValues(onAdmission, o.onAdmission, true); 1269 } 1270 1271 public boolean isEmpty() { 1272 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, condition, dateOfDiagnosis 1273 , type, onAdmission, packageCode); 1274 } 1275 1276 public String fhirType() { 1277 return "Account.diagnosis"; 1278 1279 } 1280 1281 } 1282 1283 @Block() 1284 public static class AccountProcedureComponent extends BackboneElement implements IBaseBackboneElement { 1285 /** 1286 * Ranking of the procedure (for each type). 1287 */ 1288 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="Ranking of the procedure (for each type)", formalDefinition="Ranking of the procedure (for each type)." ) 1290 protected PositiveIntType sequence; 1291 1292 /** 1293 * The procedure relevant to the account. 1294 */ 1295 @Child(name = "code", type = {CodeableReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 1296 @Description(shortDefinition="The procedure relevant to the account", formalDefinition="The procedure relevant to the account." ) 1297 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 1298 protected CodeableReference code; 1299 1300 /** 1301 * Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used. 1302 */ 1303 @Child(name = "dateOfService", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1304 @Description(shortDefinition="Date of the procedure (when coded procedure)", formalDefinition="Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used." ) 1305 protected DateTimeType dateOfService; 1306 1307 /** 1308 * How this procedure value should be used in charging the account. 1309 */ 1310 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1311 @Description(shortDefinition="How this procedure value should be used in charging the account", formalDefinition="How this procedure value should be used in charging the account." ) 1312 protected List<CodeableConcept> type; 1313 1314 /** 1315 * The package code can be used to group procedures that may be priced or delivered as a single product. Such as DRGs. 1316 */ 1317 @Child(name = "packageCode", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1318 @Description(shortDefinition="Package Code specific for billing", formalDefinition="The package code can be used to group procedures that may be priced or delivered as a single product. Such as DRGs." ) 1319 protected List<CodeableConcept> packageCode; 1320 1321 /** 1322 * Any devices that were associated with the procedure relevant to the account. 1323 */ 1324 @Child(name = "device", type = {Device.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1325 @Description(shortDefinition="Any devices that were associated with the procedure", formalDefinition="Any devices that were associated with the procedure relevant to the account." ) 1326 protected List<Reference> device; 1327 1328 private static final long serialVersionUID = -797201673L; 1329 1330 /** 1331 * Constructor 1332 */ 1333 public AccountProcedureComponent() { 1334 super(); 1335 } 1336 1337 /** 1338 * Constructor 1339 */ 1340 public AccountProcedureComponent(CodeableReference code) { 1341 super(); 1342 this.setCode(code); 1343 } 1344 1345 /** 1346 * @return {@link #sequence} (Ranking of the procedure (for each type).). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1347 */ 1348 public PositiveIntType getSequenceElement() { 1349 if (this.sequence == null) 1350 if (Configuration.errorOnAutoCreate()) 1351 throw new Error("Attempt to auto-create AccountProcedureComponent.sequence"); 1352 else if (Configuration.doAutoCreate()) 1353 this.sequence = new PositiveIntType(); // bb 1354 return this.sequence; 1355 } 1356 1357 public boolean hasSequenceElement() { 1358 return this.sequence != null && !this.sequence.isEmpty(); 1359 } 1360 1361 public boolean hasSequence() { 1362 return this.sequence != null && !this.sequence.isEmpty(); 1363 } 1364 1365 /** 1366 * @param value {@link #sequence} (Ranking of the procedure (for each type).). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1367 */ 1368 public AccountProcedureComponent setSequenceElement(PositiveIntType value) { 1369 this.sequence = value; 1370 return this; 1371 } 1372 1373 /** 1374 * @return Ranking of the procedure (for each type). 1375 */ 1376 public int getSequence() { 1377 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1378 } 1379 1380 /** 1381 * @param value Ranking of the procedure (for each type). 1382 */ 1383 public AccountProcedureComponent setSequence(int value) { 1384 if (this.sequence == null) 1385 this.sequence = new PositiveIntType(); 1386 this.sequence.setValue(value); 1387 return this; 1388 } 1389 1390 /** 1391 * @return {@link #code} (The procedure relevant to the account.) 1392 */ 1393 public CodeableReference getCode() { 1394 if (this.code == null) 1395 if (Configuration.errorOnAutoCreate()) 1396 throw new Error("Attempt to auto-create AccountProcedureComponent.code"); 1397 else if (Configuration.doAutoCreate()) 1398 this.code = new CodeableReference(); // cc 1399 return this.code; 1400 } 1401 1402 public boolean hasCode() { 1403 return this.code != null && !this.code.isEmpty(); 1404 } 1405 1406 /** 1407 * @param value {@link #code} (The procedure relevant to the account.) 1408 */ 1409 public AccountProcedureComponent setCode(CodeableReference value) { 1410 this.code = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #dateOfService} (Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used.). This is the underlying object with id, value and extensions. The accessor "getDateOfService" gives direct access to the value 1416 */ 1417 public DateTimeType getDateOfServiceElement() { 1418 if (this.dateOfService == null) 1419 if (Configuration.errorOnAutoCreate()) 1420 throw new Error("Attempt to auto-create AccountProcedureComponent.dateOfService"); 1421 else if (Configuration.doAutoCreate()) 1422 this.dateOfService = new DateTimeType(); // bb 1423 return this.dateOfService; 1424 } 1425 1426 public boolean hasDateOfServiceElement() { 1427 return this.dateOfService != null && !this.dateOfService.isEmpty(); 1428 } 1429 1430 public boolean hasDateOfService() { 1431 return this.dateOfService != null && !this.dateOfService.isEmpty(); 1432 } 1433 1434 /** 1435 * @param value {@link #dateOfService} (Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used.). This is the underlying object with id, value and extensions. The accessor "getDateOfService" gives direct access to the value 1436 */ 1437 public AccountProcedureComponent setDateOfServiceElement(DateTimeType value) { 1438 this.dateOfService = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used. 1444 */ 1445 public Date getDateOfService() { 1446 return this.dateOfService == null ? null : this.dateOfService.getValue(); 1447 } 1448 1449 /** 1450 * @param value Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used. 1451 */ 1452 public AccountProcedureComponent setDateOfService(Date value) { 1453 if (value == null) 1454 this.dateOfService = null; 1455 else { 1456 if (this.dateOfService == null) 1457 this.dateOfService = new DateTimeType(); 1458 this.dateOfService.setValue(value); 1459 } 1460 return this; 1461 } 1462 1463 /** 1464 * @return {@link #type} (How this procedure value should be used in charging the account.) 1465 */ 1466 public List<CodeableConcept> getType() { 1467 if (this.type == null) 1468 this.type = new ArrayList<CodeableConcept>(); 1469 return this.type; 1470 } 1471 1472 /** 1473 * @return Returns a reference to <code>this</code> for easy method chaining 1474 */ 1475 public AccountProcedureComponent setType(List<CodeableConcept> theType) { 1476 this.type = theType; 1477 return this; 1478 } 1479 1480 public boolean hasType() { 1481 if (this.type == null) 1482 return false; 1483 for (CodeableConcept item : this.type) 1484 if (!item.isEmpty()) 1485 return true; 1486 return false; 1487 } 1488 1489 public CodeableConcept addType() { //3 1490 CodeableConcept t = new CodeableConcept(); 1491 if (this.type == null) 1492 this.type = new ArrayList<CodeableConcept>(); 1493 this.type.add(t); 1494 return t; 1495 } 1496 1497 public AccountProcedureComponent addType(CodeableConcept t) { //3 1498 if (t == null) 1499 return this; 1500 if (this.type == null) 1501 this.type = new ArrayList<CodeableConcept>(); 1502 this.type.add(t); 1503 return this; 1504 } 1505 1506 /** 1507 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1508 */ 1509 public CodeableConcept getTypeFirstRep() { 1510 if (getType().isEmpty()) { 1511 addType(); 1512 } 1513 return getType().get(0); 1514 } 1515 1516 /** 1517 * @return {@link #packageCode} (The package code can be used to group procedures that may be priced or delivered as a single product. Such as DRGs.) 1518 */ 1519 public List<CodeableConcept> getPackageCode() { 1520 if (this.packageCode == null) 1521 this.packageCode = new ArrayList<CodeableConcept>(); 1522 return this.packageCode; 1523 } 1524 1525 /** 1526 * @return Returns a reference to <code>this</code> for easy method chaining 1527 */ 1528 public AccountProcedureComponent setPackageCode(List<CodeableConcept> thePackageCode) { 1529 this.packageCode = thePackageCode; 1530 return this; 1531 } 1532 1533 public boolean hasPackageCode() { 1534 if (this.packageCode == null) 1535 return false; 1536 for (CodeableConcept item : this.packageCode) 1537 if (!item.isEmpty()) 1538 return true; 1539 return false; 1540 } 1541 1542 public CodeableConcept addPackageCode() { //3 1543 CodeableConcept t = new CodeableConcept(); 1544 if (this.packageCode == null) 1545 this.packageCode = new ArrayList<CodeableConcept>(); 1546 this.packageCode.add(t); 1547 return t; 1548 } 1549 1550 public AccountProcedureComponent addPackageCode(CodeableConcept t) { //3 1551 if (t == null) 1552 return this; 1553 if (this.packageCode == null) 1554 this.packageCode = new ArrayList<CodeableConcept>(); 1555 this.packageCode.add(t); 1556 return this; 1557 } 1558 1559 /** 1560 * @return The first repetition of repeating field {@link #packageCode}, creating it if it does not already exist {3} 1561 */ 1562 public CodeableConcept getPackageCodeFirstRep() { 1563 if (getPackageCode().isEmpty()) { 1564 addPackageCode(); 1565 } 1566 return getPackageCode().get(0); 1567 } 1568 1569 /** 1570 * @return {@link #device} (Any devices that were associated with the procedure relevant to the account.) 1571 */ 1572 public List<Reference> getDevice() { 1573 if (this.device == null) 1574 this.device = new ArrayList<Reference>(); 1575 return this.device; 1576 } 1577 1578 /** 1579 * @return Returns a reference to <code>this</code> for easy method chaining 1580 */ 1581 public AccountProcedureComponent setDevice(List<Reference> theDevice) { 1582 this.device = theDevice; 1583 return this; 1584 } 1585 1586 public boolean hasDevice() { 1587 if (this.device == null) 1588 return false; 1589 for (Reference item : this.device) 1590 if (!item.isEmpty()) 1591 return true; 1592 return false; 1593 } 1594 1595 public Reference addDevice() { //3 1596 Reference t = new Reference(); 1597 if (this.device == null) 1598 this.device = new ArrayList<Reference>(); 1599 this.device.add(t); 1600 return t; 1601 } 1602 1603 public AccountProcedureComponent addDevice(Reference t) { //3 1604 if (t == null) 1605 return this; 1606 if (this.device == null) 1607 this.device = new ArrayList<Reference>(); 1608 this.device.add(t); 1609 return this; 1610 } 1611 1612 /** 1613 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 1614 */ 1615 public Reference getDeviceFirstRep() { 1616 if (getDevice().isEmpty()) { 1617 addDevice(); 1618 } 1619 return getDevice().get(0); 1620 } 1621 1622 protected void listChildren(List<Property> children) { 1623 super.listChildren(children); 1624 children.add(new Property("sequence", "positiveInt", "Ranking of the procedure (for each type).", 0, 1, sequence)); 1625 children.add(new Property("code", "CodeableReference(Procedure)", "The procedure relevant to the account.", 0, 1, code)); 1626 children.add(new Property("dateOfService", "dateTime", "Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used.", 0, 1, dateOfService)); 1627 children.add(new Property("type", "CodeableConcept", "How this procedure value should be used in charging the account.", 0, java.lang.Integer.MAX_VALUE, type)); 1628 children.add(new Property("packageCode", "CodeableConcept", "The package code can be used to group procedures that may be priced or delivered as a single product. Such as DRGs.", 0, java.lang.Integer.MAX_VALUE, packageCode)); 1629 children.add(new Property("device", "Reference(Device)", "Any devices that were associated with the procedure relevant to the account.", 0, java.lang.Integer.MAX_VALUE, device)); 1630 } 1631 1632 @Override 1633 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1634 switch (_hash) { 1635 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Ranking of the procedure (for each type).", 0, 1, sequence); 1636 case 3059181: /*code*/ return new Property("code", "CodeableReference(Procedure)", "The procedure relevant to the account.", 0, 1, code); 1637 case -328510256: /*dateOfService*/ return new Property("dateOfService", "dateTime", "Date of the procedure when using a coded procedure. If using a reference to a procedure, then the date on the procedure should be used.", 0, 1, dateOfService); 1638 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "How this procedure value should be used in charging the account.", 0, java.lang.Integer.MAX_VALUE, type); 1639 case 908444499: /*packageCode*/ return new Property("packageCode", "CodeableConcept", "The package code can be used to group procedures that may be priced or delivered as a single product. Such as DRGs.", 0, java.lang.Integer.MAX_VALUE, packageCode); 1640 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "Any devices that were associated with the procedure relevant to the account.", 0, java.lang.Integer.MAX_VALUE, device); 1641 default: return super.getNamedProperty(_hash, _name, _checkValid); 1642 } 1643 1644 } 1645 1646 @Override 1647 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1648 switch (hash) { 1649 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1650 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableReference 1651 case -328510256: /*dateOfService*/ return this.dateOfService == null ? new Base[0] : new Base[] {this.dateOfService}; // DateTimeType 1652 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1653 case 908444499: /*packageCode*/ return this.packageCode == null ? new Base[0] : this.packageCode.toArray(new Base[this.packageCode.size()]); // CodeableConcept 1654 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 1655 default: return super.getProperty(hash, name, checkValid); 1656 } 1657 1658 } 1659 1660 @Override 1661 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1662 switch (hash) { 1663 case 1349547969: // sequence 1664 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1665 return value; 1666 case 3059181: // code 1667 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 1668 return value; 1669 case -328510256: // dateOfService 1670 this.dateOfService = TypeConvertor.castToDateTime(value); // DateTimeType 1671 return value; 1672 case 3575610: // type 1673 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1674 return value; 1675 case 908444499: // packageCode 1676 this.getPackageCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1677 return value; 1678 case -1335157162: // device 1679 this.getDevice().add(TypeConvertor.castToReference(value)); // Reference 1680 return value; 1681 default: return super.setProperty(hash, name, value); 1682 } 1683 1684 } 1685 1686 @Override 1687 public Base setProperty(String name, Base value) throws FHIRException { 1688 if (name.equals("sequence")) { 1689 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1690 } else if (name.equals("code")) { 1691 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 1692 } else if (name.equals("dateOfService")) { 1693 this.dateOfService = TypeConvertor.castToDateTime(value); // DateTimeType 1694 } else if (name.equals("type")) { 1695 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1696 } else if (name.equals("packageCode")) { 1697 this.getPackageCode().add(TypeConvertor.castToCodeableConcept(value)); 1698 } else if (name.equals("device")) { 1699 this.getDevice().add(TypeConvertor.castToReference(value)); 1700 } else 1701 return super.setProperty(name, value); 1702 return value; 1703 } 1704 1705 @Override 1706 public void removeChild(String name, Base value) throws FHIRException { 1707 if (name.equals("sequence")) { 1708 this.sequence = null; 1709 } else if (name.equals("code")) { 1710 this.code = null; 1711 } else if (name.equals("dateOfService")) { 1712 this.dateOfService = null; 1713 } else if (name.equals("type")) { 1714 this.getType().remove(value); 1715 } else if (name.equals("packageCode")) { 1716 this.getPackageCode().remove(value); 1717 } else if (name.equals("device")) { 1718 this.getDevice().remove(value); 1719 } else 1720 super.removeChild(name, value); 1721 1722 } 1723 1724 @Override 1725 public Base makeProperty(int hash, String name) throws FHIRException { 1726 switch (hash) { 1727 case 1349547969: return getSequenceElement(); 1728 case 3059181: return getCode(); 1729 case -328510256: return getDateOfServiceElement(); 1730 case 3575610: return addType(); 1731 case 908444499: return addPackageCode(); 1732 case -1335157162: return addDevice(); 1733 default: return super.makeProperty(hash, name); 1734 } 1735 1736 } 1737 1738 @Override 1739 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1740 switch (hash) { 1741 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1742 case 3059181: /*code*/ return new String[] {"CodeableReference"}; 1743 case -328510256: /*dateOfService*/ return new String[] {"dateTime"}; 1744 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1745 case 908444499: /*packageCode*/ return new String[] {"CodeableConcept"}; 1746 case -1335157162: /*device*/ return new String[] {"Reference"}; 1747 default: return super.getTypesForProperty(hash, name); 1748 } 1749 1750 } 1751 1752 @Override 1753 public Base addChild(String name) throws FHIRException { 1754 if (name.equals("sequence")) { 1755 throw new FHIRException("Cannot call addChild on a singleton property Account.procedure.sequence"); 1756 } 1757 else if (name.equals("code")) { 1758 this.code = new CodeableReference(); 1759 return this.code; 1760 } 1761 else if (name.equals("dateOfService")) { 1762 throw new FHIRException("Cannot call addChild on a singleton property Account.procedure.dateOfService"); 1763 } 1764 else if (name.equals("type")) { 1765 return addType(); 1766 } 1767 else if (name.equals("packageCode")) { 1768 return addPackageCode(); 1769 } 1770 else if (name.equals("device")) { 1771 return addDevice(); 1772 } 1773 else 1774 return super.addChild(name); 1775 } 1776 1777 public AccountProcedureComponent copy() { 1778 AccountProcedureComponent dst = new AccountProcedureComponent(); 1779 copyValues(dst); 1780 return dst; 1781 } 1782 1783 public void copyValues(AccountProcedureComponent dst) { 1784 super.copyValues(dst); 1785 dst.sequence = sequence == null ? null : sequence.copy(); 1786 dst.code = code == null ? null : code.copy(); 1787 dst.dateOfService = dateOfService == null ? null : dateOfService.copy(); 1788 if (type != null) { 1789 dst.type = new ArrayList<CodeableConcept>(); 1790 for (CodeableConcept i : type) 1791 dst.type.add(i.copy()); 1792 }; 1793 if (packageCode != null) { 1794 dst.packageCode = new ArrayList<CodeableConcept>(); 1795 for (CodeableConcept i : packageCode) 1796 dst.packageCode.add(i.copy()); 1797 }; 1798 if (device != null) { 1799 dst.device = new ArrayList<Reference>(); 1800 for (Reference i : device) 1801 dst.device.add(i.copy()); 1802 }; 1803 } 1804 1805 @Override 1806 public boolean equalsDeep(Base other_) { 1807 if (!super.equalsDeep(other_)) 1808 return false; 1809 if (!(other_ instanceof AccountProcedureComponent)) 1810 return false; 1811 AccountProcedureComponent o = (AccountProcedureComponent) other_; 1812 return compareDeep(sequence, o.sequence, true) && compareDeep(code, o.code, true) && compareDeep(dateOfService, o.dateOfService, true) 1813 && compareDeep(type, o.type, true) && compareDeep(packageCode, o.packageCode, true) && compareDeep(device, o.device, true) 1814 ; 1815 } 1816 1817 @Override 1818 public boolean equalsShallow(Base other_) { 1819 if (!super.equalsShallow(other_)) 1820 return false; 1821 if (!(other_ instanceof AccountProcedureComponent)) 1822 return false; 1823 AccountProcedureComponent o = (AccountProcedureComponent) other_; 1824 return compareValues(sequence, o.sequence, true) && compareValues(dateOfService, o.dateOfService, true) 1825 ; 1826 } 1827 1828 public boolean isEmpty() { 1829 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, code, dateOfService 1830 , type, packageCode, device); 1831 } 1832 1833 public String fhirType() { 1834 return "Account.procedure"; 1835 1836 } 1837 1838 } 1839 1840 @Block() 1841 public static class AccountRelatedAccountComponent extends BackboneElement implements IBaseBackboneElement { 1842 /** 1843 * Relationship of the associated Account. 1844 */ 1845 @Child(name = "relationship", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1846 @Description(shortDefinition="Relationship of the associated Account", formalDefinition="Relationship of the associated Account." ) 1847 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-relationship") 1848 protected CodeableConcept relationship; 1849 1850 /** 1851 * Reference to an associated Account. 1852 */ 1853 @Child(name = "account", type = {Account.class}, order=2, min=1, max=1, modifier=false, summary=false) 1854 @Description(shortDefinition="Reference to an associated Account", formalDefinition="Reference to an associated Account." ) 1855 protected Reference account; 1856 1857 private static final long serialVersionUID = 1586291361L; 1858 1859 /** 1860 * Constructor 1861 */ 1862 public AccountRelatedAccountComponent() { 1863 super(); 1864 } 1865 1866 /** 1867 * Constructor 1868 */ 1869 public AccountRelatedAccountComponent(Reference account) { 1870 super(); 1871 this.setAccount(account); 1872 } 1873 1874 /** 1875 * @return {@link #relationship} (Relationship of the associated Account.) 1876 */ 1877 public CodeableConcept getRelationship() { 1878 if (this.relationship == null) 1879 if (Configuration.errorOnAutoCreate()) 1880 throw new Error("Attempt to auto-create AccountRelatedAccountComponent.relationship"); 1881 else if (Configuration.doAutoCreate()) 1882 this.relationship = new CodeableConcept(); // cc 1883 return this.relationship; 1884 } 1885 1886 public boolean hasRelationship() { 1887 return this.relationship != null && !this.relationship.isEmpty(); 1888 } 1889 1890 /** 1891 * @param value {@link #relationship} (Relationship of the associated Account.) 1892 */ 1893 public AccountRelatedAccountComponent setRelationship(CodeableConcept value) { 1894 this.relationship = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return {@link #account} (Reference to an associated Account.) 1900 */ 1901 public Reference getAccount() { 1902 if (this.account == null) 1903 if (Configuration.errorOnAutoCreate()) 1904 throw new Error("Attempt to auto-create AccountRelatedAccountComponent.account"); 1905 else if (Configuration.doAutoCreate()) 1906 this.account = new Reference(); // cc 1907 return this.account; 1908 } 1909 1910 public boolean hasAccount() { 1911 return this.account != null && !this.account.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #account} (Reference to an associated Account.) 1916 */ 1917 public AccountRelatedAccountComponent setAccount(Reference value) { 1918 this.account = value; 1919 return this; 1920 } 1921 1922 protected void listChildren(List<Property> children) { 1923 super.listChildren(children); 1924 children.add(new Property("relationship", "CodeableConcept", "Relationship of the associated Account.", 0, 1, relationship)); 1925 children.add(new Property("account", "Reference(Account)", "Reference to an associated Account.", 0, 1, account)); 1926 } 1927 1928 @Override 1929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1930 switch (_hash) { 1931 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "Relationship of the associated Account.", 0, 1, relationship); 1932 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "Reference to an associated Account.", 0, 1, account); 1933 default: return super.getNamedProperty(_hash, _name, _checkValid); 1934 } 1935 1936 } 1937 1938 @Override 1939 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1940 switch (hash) { 1941 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 1942 case -1177318867: /*account*/ return this.account == null ? new Base[0] : new Base[] {this.account}; // Reference 1943 default: return super.getProperty(hash, name, checkValid); 1944 } 1945 1946 } 1947 1948 @Override 1949 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1950 switch (hash) { 1951 case -261851592: // relationship 1952 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1953 return value; 1954 case -1177318867: // account 1955 this.account = TypeConvertor.castToReference(value); // Reference 1956 return value; 1957 default: return super.setProperty(hash, name, value); 1958 } 1959 1960 } 1961 1962 @Override 1963 public Base setProperty(String name, Base value) throws FHIRException { 1964 if (name.equals("relationship")) { 1965 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1966 } else if (name.equals("account")) { 1967 this.account = TypeConvertor.castToReference(value); // Reference 1968 } else 1969 return super.setProperty(name, value); 1970 return value; 1971 } 1972 1973 @Override 1974 public void removeChild(String name, Base value) throws FHIRException { 1975 if (name.equals("relationship")) { 1976 this.relationship = null; 1977 } else if (name.equals("account")) { 1978 this.account = null; 1979 } else 1980 super.removeChild(name, value); 1981 1982 } 1983 1984 @Override 1985 public Base makeProperty(int hash, String name) throws FHIRException { 1986 switch (hash) { 1987 case -261851592: return getRelationship(); 1988 case -1177318867: return getAccount(); 1989 default: return super.makeProperty(hash, name); 1990 } 1991 1992 } 1993 1994 @Override 1995 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1996 switch (hash) { 1997 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 1998 case -1177318867: /*account*/ return new String[] {"Reference"}; 1999 default: return super.getTypesForProperty(hash, name); 2000 } 2001 2002 } 2003 2004 @Override 2005 public Base addChild(String name) throws FHIRException { 2006 if (name.equals("relationship")) { 2007 this.relationship = new CodeableConcept(); 2008 return this.relationship; 2009 } 2010 else if (name.equals("account")) { 2011 this.account = new Reference(); 2012 return this.account; 2013 } 2014 else 2015 return super.addChild(name); 2016 } 2017 2018 public AccountRelatedAccountComponent copy() { 2019 AccountRelatedAccountComponent dst = new AccountRelatedAccountComponent(); 2020 copyValues(dst); 2021 return dst; 2022 } 2023 2024 public void copyValues(AccountRelatedAccountComponent dst) { 2025 super.copyValues(dst); 2026 dst.relationship = relationship == null ? null : relationship.copy(); 2027 dst.account = account == null ? null : account.copy(); 2028 } 2029 2030 @Override 2031 public boolean equalsDeep(Base other_) { 2032 if (!super.equalsDeep(other_)) 2033 return false; 2034 if (!(other_ instanceof AccountRelatedAccountComponent)) 2035 return false; 2036 AccountRelatedAccountComponent o = (AccountRelatedAccountComponent) other_; 2037 return compareDeep(relationship, o.relationship, true) && compareDeep(account, o.account, true) 2038 ; 2039 } 2040 2041 @Override 2042 public boolean equalsShallow(Base other_) { 2043 if (!super.equalsShallow(other_)) 2044 return false; 2045 if (!(other_ instanceof AccountRelatedAccountComponent)) 2046 return false; 2047 AccountRelatedAccountComponent o = (AccountRelatedAccountComponent) other_; 2048 return true; 2049 } 2050 2051 public boolean isEmpty() { 2052 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relationship, account); 2053 } 2054 2055 public String fhirType() { 2056 return "Account.relatedAccount"; 2057 2058 } 2059 2060 } 2061 2062 @Block() 2063 public static class AccountBalanceComponent extends BackboneElement implements IBaseBackboneElement { 2064 /** 2065 * Who is expected to pay this part of the balance. 2066 */ 2067 @Child(name = "aggregate", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 2068 @Description(shortDefinition="Who is expected to pay this part of the balance", formalDefinition="Who is expected to pay this part of the balance." ) 2069 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-aggregate") 2070 protected CodeableConcept aggregate; 2071 2072 /** 2073 * The term of the account balances - The balance value is the amount that was outstanding for this age. 2074 */ 2075 @Child(name = "term", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2076 @Description(shortDefinition="current | 30 | 60 | 90 | 120", formalDefinition="The term of the account balances - The balance value is the amount that was outstanding for this age." ) 2077 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-balance-term") 2078 protected CodeableConcept term; 2079 2080 /** 2081 * The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process). 2082 */ 2083 @Child(name = "estimate", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2084 @Description(shortDefinition="Estimated balance", formalDefinition="The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process)." ) 2085 protected BooleanType estimate; 2086 2087 /** 2088 * The actual balance value calculated for the age defined in the term property. 2089 */ 2090 @Child(name = "amount", type = {Money.class}, order=4, min=1, max=1, modifier=false, summary=false) 2091 @Description(shortDefinition="Calculated amount", formalDefinition="The actual balance value calculated for the age defined in the term property." ) 2092 protected Money amount; 2093 2094 private static final long serialVersionUID = -338990145L; 2095 2096 /** 2097 * Constructor 2098 */ 2099 public AccountBalanceComponent() { 2100 super(); 2101 } 2102 2103 /** 2104 * Constructor 2105 */ 2106 public AccountBalanceComponent(Money amount) { 2107 super(); 2108 this.setAmount(amount); 2109 } 2110 2111 /** 2112 * @return {@link #aggregate} (Who is expected to pay this part of the balance.) 2113 */ 2114 public CodeableConcept getAggregate() { 2115 if (this.aggregate == null) 2116 if (Configuration.errorOnAutoCreate()) 2117 throw new Error("Attempt to auto-create AccountBalanceComponent.aggregate"); 2118 else if (Configuration.doAutoCreate()) 2119 this.aggregate = new CodeableConcept(); // cc 2120 return this.aggregate; 2121 } 2122 2123 public boolean hasAggregate() { 2124 return this.aggregate != null && !this.aggregate.isEmpty(); 2125 } 2126 2127 /** 2128 * @param value {@link #aggregate} (Who is expected to pay this part of the balance.) 2129 */ 2130 public AccountBalanceComponent setAggregate(CodeableConcept value) { 2131 this.aggregate = value; 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #term} (The term of the account balances - The balance value is the amount that was outstanding for this age.) 2137 */ 2138 public CodeableConcept getTerm() { 2139 if (this.term == null) 2140 if (Configuration.errorOnAutoCreate()) 2141 throw new Error("Attempt to auto-create AccountBalanceComponent.term"); 2142 else if (Configuration.doAutoCreate()) 2143 this.term = new CodeableConcept(); // cc 2144 return this.term; 2145 } 2146 2147 public boolean hasTerm() { 2148 return this.term != null && !this.term.isEmpty(); 2149 } 2150 2151 /** 2152 * @param value {@link #term} (The term of the account balances - The balance value is the amount that was outstanding for this age.) 2153 */ 2154 public AccountBalanceComponent setTerm(CodeableConcept value) { 2155 this.term = value; 2156 return this; 2157 } 2158 2159 /** 2160 * @return {@link #estimate} (The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process).). This is the underlying object with id, value and extensions. The accessor "getEstimate" gives direct access to the value 2161 */ 2162 public BooleanType getEstimateElement() { 2163 if (this.estimate == null) 2164 if (Configuration.errorOnAutoCreate()) 2165 throw new Error("Attempt to auto-create AccountBalanceComponent.estimate"); 2166 else if (Configuration.doAutoCreate()) 2167 this.estimate = new BooleanType(); // bb 2168 return this.estimate; 2169 } 2170 2171 public boolean hasEstimateElement() { 2172 return this.estimate != null && !this.estimate.isEmpty(); 2173 } 2174 2175 public boolean hasEstimate() { 2176 return this.estimate != null && !this.estimate.isEmpty(); 2177 } 2178 2179 /** 2180 * @param value {@link #estimate} (The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process).). This is the underlying object with id, value and extensions. The accessor "getEstimate" gives direct access to the value 2181 */ 2182 public AccountBalanceComponent setEstimateElement(BooleanType value) { 2183 this.estimate = value; 2184 return this; 2185 } 2186 2187 /** 2188 * @return The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process). 2189 */ 2190 public boolean getEstimate() { 2191 return this.estimate == null || this.estimate.isEmpty() ? false : this.estimate.getValue(); 2192 } 2193 2194 /** 2195 * @param value The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process). 2196 */ 2197 public AccountBalanceComponent setEstimate(boolean value) { 2198 if (this.estimate == null) 2199 this.estimate = new BooleanType(); 2200 this.estimate.setValue(value); 2201 return this; 2202 } 2203 2204 /** 2205 * @return {@link #amount} (The actual balance value calculated for the age defined in the term property.) 2206 */ 2207 public Money getAmount() { 2208 if (this.amount == null) 2209 if (Configuration.errorOnAutoCreate()) 2210 throw new Error("Attempt to auto-create AccountBalanceComponent.amount"); 2211 else if (Configuration.doAutoCreate()) 2212 this.amount = new Money(); // cc 2213 return this.amount; 2214 } 2215 2216 public boolean hasAmount() { 2217 return this.amount != null && !this.amount.isEmpty(); 2218 } 2219 2220 /** 2221 * @param value {@link #amount} (The actual balance value calculated for the age defined in the term property.) 2222 */ 2223 public AccountBalanceComponent setAmount(Money value) { 2224 this.amount = value; 2225 return this; 2226 } 2227 2228 protected void listChildren(List<Property> children) { 2229 super.listChildren(children); 2230 children.add(new Property("aggregate", "CodeableConcept", "Who is expected to pay this part of the balance.", 0, 1, aggregate)); 2231 children.add(new Property("term", "CodeableConcept", "The term of the account balances - The balance value is the amount that was outstanding for this age.", 0, 1, term)); 2232 children.add(new Property("estimate", "boolean", "The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process).", 0, 1, estimate)); 2233 children.add(new Property("amount", "Money", "The actual balance value calculated for the age defined in the term property.", 0, 1, amount)); 2234 } 2235 2236 @Override 2237 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2238 switch (_hash) { 2239 case 175177151: /*aggregate*/ return new Property("aggregate", "CodeableConcept", "Who is expected to pay this part of the balance.", 0, 1, aggregate); 2240 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term of the account balances - The balance value is the amount that was outstanding for this age.", 0, 1, term); 2241 case -1959779032: /*estimate*/ return new Property("estimate", "boolean", "The amount is only an estimated value - this is likely common for `current` term balances, but not with known terms (that were generated by a backend process).", 0, 1, estimate); 2242 case -1413853096: /*amount*/ return new Property("amount", "Money", "The actual balance value calculated for the age defined in the term property.", 0, 1, amount); 2243 default: return super.getNamedProperty(_hash, _name, _checkValid); 2244 } 2245 2246 } 2247 2248 @Override 2249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2250 switch (hash) { 2251 case 175177151: /*aggregate*/ return this.aggregate == null ? new Base[0] : new Base[] {this.aggregate}; // CodeableConcept 2252 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 2253 case -1959779032: /*estimate*/ return this.estimate == null ? new Base[0] : new Base[] {this.estimate}; // BooleanType 2254 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 2255 default: return super.getProperty(hash, name, checkValid); 2256 } 2257 2258 } 2259 2260 @Override 2261 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2262 switch (hash) { 2263 case 175177151: // aggregate 2264 this.aggregate = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2265 return value; 2266 case 3556460: // term 2267 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2268 return value; 2269 case -1959779032: // estimate 2270 this.estimate = TypeConvertor.castToBoolean(value); // BooleanType 2271 return value; 2272 case -1413853096: // amount 2273 this.amount = TypeConvertor.castToMoney(value); // Money 2274 return value; 2275 default: return super.setProperty(hash, name, value); 2276 } 2277 2278 } 2279 2280 @Override 2281 public Base setProperty(String name, Base value) throws FHIRException { 2282 if (name.equals("aggregate")) { 2283 this.aggregate = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2284 } else if (name.equals("term")) { 2285 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2286 } else if (name.equals("estimate")) { 2287 this.estimate = TypeConvertor.castToBoolean(value); // BooleanType 2288 } else if (name.equals("amount")) { 2289 this.amount = TypeConvertor.castToMoney(value); // Money 2290 } else 2291 return super.setProperty(name, value); 2292 return value; 2293 } 2294 2295 @Override 2296 public void removeChild(String name, Base value) throws FHIRException { 2297 if (name.equals("aggregate")) { 2298 this.aggregate = null; 2299 } else if (name.equals("term")) { 2300 this.term = null; 2301 } else if (name.equals("estimate")) { 2302 this.estimate = null; 2303 } else if (name.equals("amount")) { 2304 this.amount = null; 2305 } else 2306 super.removeChild(name, value); 2307 2308 } 2309 2310 @Override 2311 public Base makeProperty(int hash, String name) throws FHIRException { 2312 switch (hash) { 2313 case 175177151: return getAggregate(); 2314 case 3556460: return getTerm(); 2315 case -1959779032: return getEstimateElement(); 2316 case -1413853096: return getAmount(); 2317 default: return super.makeProperty(hash, name); 2318 } 2319 2320 } 2321 2322 @Override 2323 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2324 switch (hash) { 2325 case 175177151: /*aggregate*/ return new String[] {"CodeableConcept"}; 2326 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 2327 case -1959779032: /*estimate*/ return new String[] {"boolean"}; 2328 case -1413853096: /*amount*/ return new String[] {"Money"}; 2329 default: return super.getTypesForProperty(hash, name); 2330 } 2331 2332 } 2333 2334 @Override 2335 public Base addChild(String name) throws FHIRException { 2336 if (name.equals("aggregate")) { 2337 this.aggregate = new CodeableConcept(); 2338 return this.aggregate; 2339 } 2340 else if (name.equals("term")) { 2341 this.term = new CodeableConcept(); 2342 return this.term; 2343 } 2344 else if (name.equals("estimate")) { 2345 throw new FHIRException("Cannot call addChild on a singleton property Account.balance.estimate"); 2346 } 2347 else if (name.equals("amount")) { 2348 this.amount = new Money(); 2349 return this.amount; 2350 } 2351 else 2352 return super.addChild(name); 2353 } 2354 2355 public AccountBalanceComponent copy() { 2356 AccountBalanceComponent dst = new AccountBalanceComponent(); 2357 copyValues(dst); 2358 return dst; 2359 } 2360 2361 public void copyValues(AccountBalanceComponent dst) { 2362 super.copyValues(dst); 2363 dst.aggregate = aggregate == null ? null : aggregate.copy(); 2364 dst.term = term == null ? null : term.copy(); 2365 dst.estimate = estimate == null ? null : estimate.copy(); 2366 dst.amount = amount == null ? null : amount.copy(); 2367 } 2368 2369 @Override 2370 public boolean equalsDeep(Base other_) { 2371 if (!super.equalsDeep(other_)) 2372 return false; 2373 if (!(other_ instanceof AccountBalanceComponent)) 2374 return false; 2375 AccountBalanceComponent o = (AccountBalanceComponent) other_; 2376 return compareDeep(aggregate, o.aggregate, true) && compareDeep(term, o.term, true) && compareDeep(estimate, o.estimate, true) 2377 && compareDeep(amount, o.amount, true); 2378 } 2379 2380 @Override 2381 public boolean equalsShallow(Base other_) { 2382 if (!super.equalsShallow(other_)) 2383 return false; 2384 if (!(other_ instanceof AccountBalanceComponent)) 2385 return false; 2386 AccountBalanceComponent o = (AccountBalanceComponent) other_; 2387 return compareValues(estimate, o.estimate, true); 2388 } 2389 2390 public boolean isEmpty() { 2391 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(aggregate, term, estimate 2392 , amount); 2393 } 2394 2395 public String fhirType() { 2396 return "Account.balance"; 2397 2398 } 2399 2400 } 2401 2402 /** 2403 * Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number). 2404 */ 2405 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2406 @Description(shortDefinition="Account number", formalDefinition="Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number)." ) 2407 protected List<Identifier> identifier; 2408 2409 /** 2410 * Indicates whether the account is presently used/usable or not. 2411 */ 2412 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2413 @Description(shortDefinition="active | inactive | entered-in-error | on-hold | unknown", formalDefinition="Indicates whether the account is presently used/usable or not." ) 2414 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-status") 2415 protected Enumeration<AccountStatus> status; 2416 2417 /** 2418 * The BillingStatus tracks the lifecycle of the account through the billing process. It indicates how transactions are treated when they are allocated to the account. 2419 */ 2420 @Child(name = "billingStatus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2421 @Description(shortDefinition="Tracks the lifecycle of the account through the billing process", formalDefinition="The BillingStatus tracks the lifecycle of the account through the billing process. It indicates how transactions are treated when they are allocated to the account." ) 2422 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-billing-status") 2423 protected CodeableConcept billingStatus; 2424 2425 /** 2426 * Categorizes the account for reporting and searching purposes. 2427 */ 2428 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 2429 @Description(shortDefinition="E.g. patient, expense, depreciation", formalDefinition="Categorizes the account for reporting and searching purposes." ) 2430 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/account-type") 2431 protected CodeableConcept type; 2432 2433 /** 2434 * Name used for the account when displaying it to humans in reports, etc. 2435 */ 2436 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2437 @Description(shortDefinition="Human-readable label", formalDefinition="Name used for the account when displaying it to humans in reports, etc." ) 2438 protected StringType name; 2439 2440 /** 2441 * Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account. 2442 */ 2443 @Child(name = "subject", type = {Patient.class, Device.class, Practitioner.class, PractitionerRole.class, Location.class, HealthcareService.class, Organization.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2444 @Description(shortDefinition="The entity that caused the expenses", formalDefinition="Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account." ) 2445 protected List<Reference> subject; 2446 2447 /** 2448 * The date range of services associated with this account. 2449 */ 2450 @Child(name = "servicePeriod", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 2451 @Description(shortDefinition="Transaction window", formalDefinition="The date range of services associated with this account." ) 2452 protected Period servicePeriod; 2453 2454 /** 2455 * The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account. 2456 */ 2457 @Child(name = "coverage", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2458 @Description(shortDefinition="The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account", formalDefinition="The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account." ) 2459 protected List<CoverageComponent> coverage; 2460 2461 /** 2462 * Indicates the service area, hospital, department, etc. with responsibility for managing the Account. 2463 */ 2464 @Child(name = "owner", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=true) 2465 @Description(shortDefinition="Entity managing the Account", formalDefinition="Indicates the service area, hospital, department, etc. with responsibility for managing the Account." ) 2466 protected Reference owner; 2467 2468 /** 2469 * Provides additional information about what the account tracks and how it is used. 2470 */ 2471 @Child(name = "description", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2472 @Description(shortDefinition="Explanation of purpose/use", formalDefinition="Provides additional information about what the account tracks and how it is used." ) 2473 protected MarkdownType description; 2474 2475 /** 2476 * The parties responsible for balancing the account if other payment options fall short. 2477 */ 2478 @Child(name = "guarantor", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2479 @Description(shortDefinition="The parties ultimately responsible for balancing the Account", formalDefinition="The parties responsible for balancing the account if other payment options fall short." ) 2480 protected List<GuarantorComponent> guarantor; 2481 2482 /** 2483 * When using an account for billing a specific Encounter the set of diagnoses that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s). 2484 */ 2485 @Child(name = "diagnosis", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2486 @Description(shortDefinition="The list of diagnoses relevant to this account", formalDefinition="When using an account for billing a specific Encounter the set of diagnoses that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s)." ) 2487 protected List<AccountDiagnosisComponent> diagnosis; 2488 2489 /** 2490 * When using an account for billing a specific Encounter the set of procedures that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s). 2491 */ 2492 @Child(name = "procedure", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2493 @Description(shortDefinition="The list of procedures relevant to this account", formalDefinition="When using an account for billing a specific Encounter the set of procedures that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s)." ) 2494 protected List<AccountProcedureComponent> procedure; 2495 2496 /** 2497 * Other associated accounts related to this account. 2498 */ 2499 @Child(name = "relatedAccount", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2500 @Description(shortDefinition="Other associated accounts related to this account", formalDefinition="Other associated accounts related to this account." ) 2501 protected List<AccountRelatedAccountComponent> relatedAccount; 2502 2503 /** 2504 * The default currency for the account. 2505 */ 2506 @Child(name = "currency", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 2507 @Description(shortDefinition="The base or default currency", formalDefinition="The default currency for the account." ) 2508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/currencies") 2509 protected CodeableConcept currency; 2510 2511 /** 2512 * The calculated account balances - these are calculated and processed by the finance system. 2513 2514The balances with a `term` that is not current are usually generated/updated by an invoicing or similar process. 2515 */ 2516 @Child(name = "balance", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2517 @Description(shortDefinition="Calculated account balance(s)", formalDefinition="The calculated account balances - these are calculated and processed by the finance system.\r\rThe balances with a `term` that is not current are usually generated/updated by an invoicing or similar process." ) 2518 protected List<AccountBalanceComponent> balance; 2519 2520 /** 2521 * Time the balance amount was calculated. 2522 */ 2523 @Child(name = "calculatedAt", type = {InstantType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2524 @Description(shortDefinition="Time the balance amount was calculated", formalDefinition="Time the balance amount was calculated." ) 2525 protected InstantType calculatedAt; 2526 2527 private static final long serialVersionUID = -924752626L; 2528 2529 /** 2530 * Constructor 2531 */ 2532 public Account() { 2533 super(); 2534 } 2535 2536 /** 2537 * Constructor 2538 */ 2539 public Account(AccountStatus status) { 2540 super(); 2541 this.setStatus(status); 2542 } 2543 2544 /** 2545 * @return {@link #identifier} (Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).) 2546 */ 2547 public List<Identifier> getIdentifier() { 2548 if (this.identifier == null) 2549 this.identifier = new ArrayList<Identifier>(); 2550 return this.identifier; 2551 } 2552 2553 /** 2554 * @return Returns a reference to <code>this</code> for easy method chaining 2555 */ 2556 public Account setIdentifier(List<Identifier> theIdentifier) { 2557 this.identifier = theIdentifier; 2558 return this; 2559 } 2560 2561 public boolean hasIdentifier() { 2562 if (this.identifier == null) 2563 return false; 2564 for (Identifier item : this.identifier) 2565 if (!item.isEmpty()) 2566 return true; 2567 return false; 2568 } 2569 2570 public Identifier addIdentifier() { //3 2571 Identifier t = new Identifier(); 2572 if (this.identifier == null) 2573 this.identifier = new ArrayList<Identifier>(); 2574 this.identifier.add(t); 2575 return t; 2576 } 2577 2578 public Account addIdentifier(Identifier t) { //3 2579 if (t == null) 2580 return this; 2581 if (this.identifier == null) 2582 this.identifier = new ArrayList<Identifier>(); 2583 this.identifier.add(t); 2584 return this; 2585 } 2586 2587 /** 2588 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2589 */ 2590 public Identifier getIdentifierFirstRep() { 2591 if (getIdentifier().isEmpty()) { 2592 addIdentifier(); 2593 } 2594 return getIdentifier().get(0); 2595 } 2596 2597 /** 2598 * @return {@link #status} (Indicates whether the account is presently used/usable or not.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2599 */ 2600 public Enumeration<AccountStatus> getStatusElement() { 2601 if (this.status == null) 2602 if (Configuration.errorOnAutoCreate()) 2603 throw new Error("Attempt to auto-create Account.status"); 2604 else if (Configuration.doAutoCreate()) 2605 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); // bb 2606 return this.status; 2607 } 2608 2609 public boolean hasStatusElement() { 2610 return this.status != null && !this.status.isEmpty(); 2611 } 2612 2613 public boolean hasStatus() { 2614 return this.status != null && !this.status.isEmpty(); 2615 } 2616 2617 /** 2618 * @param value {@link #status} (Indicates whether the account is presently used/usable or not.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2619 */ 2620 public Account setStatusElement(Enumeration<AccountStatus> value) { 2621 this.status = value; 2622 return this; 2623 } 2624 2625 /** 2626 * @return Indicates whether the account is presently used/usable or not. 2627 */ 2628 public AccountStatus getStatus() { 2629 return this.status == null ? null : this.status.getValue(); 2630 } 2631 2632 /** 2633 * @param value Indicates whether the account is presently used/usable or not. 2634 */ 2635 public Account setStatus(AccountStatus value) { 2636 if (this.status == null) 2637 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); 2638 this.status.setValue(value); 2639 return this; 2640 } 2641 2642 /** 2643 * @return {@link #billingStatus} (The BillingStatus tracks the lifecycle of the account through the billing process. It indicates how transactions are treated when they are allocated to the account.) 2644 */ 2645 public CodeableConcept getBillingStatus() { 2646 if (this.billingStatus == null) 2647 if (Configuration.errorOnAutoCreate()) 2648 throw new Error("Attempt to auto-create Account.billingStatus"); 2649 else if (Configuration.doAutoCreate()) 2650 this.billingStatus = new CodeableConcept(); // cc 2651 return this.billingStatus; 2652 } 2653 2654 public boolean hasBillingStatus() { 2655 return this.billingStatus != null && !this.billingStatus.isEmpty(); 2656 } 2657 2658 /** 2659 * @param value {@link #billingStatus} (The BillingStatus tracks the lifecycle of the account through the billing process. It indicates how transactions are treated when they are allocated to the account.) 2660 */ 2661 public Account setBillingStatus(CodeableConcept value) { 2662 this.billingStatus = value; 2663 return this; 2664 } 2665 2666 /** 2667 * @return {@link #type} (Categorizes the account for reporting and searching purposes.) 2668 */ 2669 public CodeableConcept getType() { 2670 if (this.type == null) 2671 if (Configuration.errorOnAutoCreate()) 2672 throw new Error("Attempt to auto-create Account.type"); 2673 else if (Configuration.doAutoCreate()) 2674 this.type = new CodeableConcept(); // cc 2675 return this.type; 2676 } 2677 2678 public boolean hasType() { 2679 return this.type != null && !this.type.isEmpty(); 2680 } 2681 2682 /** 2683 * @param value {@link #type} (Categorizes the account for reporting and searching purposes.) 2684 */ 2685 public Account setType(CodeableConcept value) { 2686 this.type = value; 2687 return this; 2688 } 2689 2690 /** 2691 * @return {@link #name} (Name used for the account when displaying it to humans in reports, etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2692 */ 2693 public StringType getNameElement() { 2694 if (this.name == null) 2695 if (Configuration.errorOnAutoCreate()) 2696 throw new Error("Attempt to auto-create Account.name"); 2697 else if (Configuration.doAutoCreate()) 2698 this.name = new StringType(); // bb 2699 return this.name; 2700 } 2701 2702 public boolean hasNameElement() { 2703 return this.name != null && !this.name.isEmpty(); 2704 } 2705 2706 public boolean hasName() { 2707 return this.name != null && !this.name.isEmpty(); 2708 } 2709 2710 /** 2711 * @param value {@link #name} (Name used for the account when displaying it to humans in reports, etc.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2712 */ 2713 public Account setNameElement(StringType value) { 2714 this.name = value; 2715 return this; 2716 } 2717 2718 /** 2719 * @return Name used for the account when displaying it to humans in reports, etc. 2720 */ 2721 public String getName() { 2722 return this.name == null ? null : this.name.getValue(); 2723 } 2724 2725 /** 2726 * @param value Name used for the account when displaying it to humans in reports, etc. 2727 */ 2728 public Account setName(String value) { 2729 if (Utilities.noString(value)) 2730 this.name = null; 2731 else { 2732 if (this.name == null) 2733 this.name = new StringType(); 2734 this.name.setValue(value); 2735 } 2736 return this; 2737 } 2738 2739 /** 2740 * @return {@link #subject} (Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.) 2741 */ 2742 public List<Reference> getSubject() { 2743 if (this.subject == null) 2744 this.subject = new ArrayList<Reference>(); 2745 return this.subject; 2746 } 2747 2748 /** 2749 * @return Returns a reference to <code>this</code> for easy method chaining 2750 */ 2751 public Account setSubject(List<Reference> theSubject) { 2752 this.subject = theSubject; 2753 return this; 2754 } 2755 2756 public boolean hasSubject() { 2757 if (this.subject == null) 2758 return false; 2759 for (Reference item : this.subject) 2760 if (!item.isEmpty()) 2761 return true; 2762 return false; 2763 } 2764 2765 public Reference addSubject() { //3 2766 Reference t = new Reference(); 2767 if (this.subject == null) 2768 this.subject = new ArrayList<Reference>(); 2769 this.subject.add(t); 2770 return t; 2771 } 2772 2773 public Account addSubject(Reference t) { //3 2774 if (t == null) 2775 return this; 2776 if (this.subject == null) 2777 this.subject = new ArrayList<Reference>(); 2778 this.subject.add(t); 2779 return this; 2780 } 2781 2782 /** 2783 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 2784 */ 2785 public Reference getSubjectFirstRep() { 2786 if (getSubject().isEmpty()) { 2787 addSubject(); 2788 } 2789 return getSubject().get(0); 2790 } 2791 2792 /** 2793 * @return {@link #servicePeriod} (The date range of services associated with this account.) 2794 */ 2795 public Period getServicePeriod() { 2796 if (this.servicePeriod == null) 2797 if (Configuration.errorOnAutoCreate()) 2798 throw new Error("Attempt to auto-create Account.servicePeriod"); 2799 else if (Configuration.doAutoCreate()) 2800 this.servicePeriod = new Period(); // cc 2801 return this.servicePeriod; 2802 } 2803 2804 public boolean hasServicePeriod() { 2805 return this.servicePeriod != null && !this.servicePeriod.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #servicePeriod} (The date range of services associated with this account.) 2810 */ 2811 public Account setServicePeriod(Period value) { 2812 this.servicePeriod = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return {@link #coverage} (The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.) 2818 */ 2819 public List<CoverageComponent> getCoverage() { 2820 if (this.coverage == null) 2821 this.coverage = new ArrayList<CoverageComponent>(); 2822 return this.coverage; 2823 } 2824 2825 /** 2826 * @return Returns a reference to <code>this</code> for easy method chaining 2827 */ 2828 public Account setCoverage(List<CoverageComponent> theCoverage) { 2829 this.coverage = theCoverage; 2830 return this; 2831 } 2832 2833 public boolean hasCoverage() { 2834 if (this.coverage == null) 2835 return false; 2836 for (CoverageComponent item : this.coverage) 2837 if (!item.isEmpty()) 2838 return true; 2839 return false; 2840 } 2841 2842 public CoverageComponent addCoverage() { //3 2843 CoverageComponent t = new CoverageComponent(); 2844 if (this.coverage == null) 2845 this.coverage = new ArrayList<CoverageComponent>(); 2846 this.coverage.add(t); 2847 return t; 2848 } 2849 2850 public Account addCoverage(CoverageComponent t) { //3 2851 if (t == null) 2852 return this; 2853 if (this.coverage == null) 2854 this.coverage = new ArrayList<CoverageComponent>(); 2855 this.coverage.add(t); 2856 return this; 2857 } 2858 2859 /** 2860 * @return The first repetition of repeating field {@link #coverage}, creating it if it does not already exist {3} 2861 */ 2862 public CoverageComponent getCoverageFirstRep() { 2863 if (getCoverage().isEmpty()) { 2864 addCoverage(); 2865 } 2866 return getCoverage().get(0); 2867 } 2868 2869 /** 2870 * @return {@link #owner} (Indicates the service area, hospital, department, etc. with responsibility for managing the Account.) 2871 */ 2872 public Reference getOwner() { 2873 if (this.owner == null) 2874 if (Configuration.errorOnAutoCreate()) 2875 throw new Error("Attempt to auto-create Account.owner"); 2876 else if (Configuration.doAutoCreate()) 2877 this.owner = new Reference(); // cc 2878 return this.owner; 2879 } 2880 2881 public boolean hasOwner() { 2882 return this.owner != null && !this.owner.isEmpty(); 2883 } 2884 2885 /** 2886 * @param value {@link #owner} (Indicates the service area, hospital, department, etc. with responsibility for managing the Account.) 2887 */ 2888 public Account setOwner(Reference value) { 2889 this.owner = value; 2890 return this; 2891 } 2892 2893 /** 2894 * @return {@link #description} (Provides additional information about what the account tracks and how it is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2895 */ 2896 public MarkdownType getDescriptionElement() { 2897 if (this.description == null) 2898 if (Configuration.errorOnAutoCreate()) 2899 throw new Error("Attempt to auto-create Account.description"); 2900 else if (Configuration.doAutoCreate()) 2901 this.description = new MarkdownType(); // bb 2902 return this.description; 2903 } 2904 2905 public boolean hasDescriptionElement() { 2906 return this.description != null && !this.description.isEmpty(); 2907 } 2908 2909 public boolean hasDescription() { 2910 return this.description != null && !this.description.isEmpty(); 2911 } 2912 2913 /** 2914 * @param value {@link #description} (Provides additional information about what the account tracks and how it is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2915 */ 2916 public Account setDescriptionElement(MarkdownType value) { 2917 this.description = value; 2918 return this; 2919 } 2920 2921 /** 2922 * @return Provides additional information about what the account tracks and how it is used. 2923 */ 2924 public String getDescription() { 2925 return this.description == null ? null : this.description.getValue(); 2926 } 2927 2928 /** 2929 * @param value Provides additional information about what the account tracks and how it is used. 2930 */ 2931 public Account setDescription(String value) { 2932 if (Utilities.noString(value)) 2933 this.description = null; 2934 else { 2935 if (this.description == null) 2936 this.description = new MarkdownType(); 2937 this.description.setValue(value); 2938 } 2939 return this; 2940 } 2941 2942 /** 2943 * @return {@link #guarantor} (The parties responsible for balancing the account if other payment options fall short.) 2944 */ 2945 public List<GuarantorComponent> getGuarantor() { 2946 if (this.guarantor == null) 2947 this.guarantor = new ArrayList<GuarantorComponent>(); 2948 return this.guarantor; 2949 } 2950 2951 /** 2952 * @return Returns a reference to <code>this</code> for easy method chaining 2953 */ 2954 public Account setGuarantor(List<GuarantorComponent> theGuarantor) { 2955 this.guarantor = theGuarantor; 2956 return this; 2957 } 2958 2959 public boolean hasGuarantor() { 2960 if (this.guarantor == null) 2961 return false; 2962 for (GuarantorComponent item : this.guarantor) 2963 if (!item.isEmpty()) 2964 return true; 2965 return false; 2966 } 2967 2968 public GuarantorComponent addGuarantor() { //3 2969 GuarantorComponent t = new GuarantorComponent(); 2970 if (this.guarantor == null) 2971 this.guarantor = new ArrayList<GuarantorComponent>(); 2972 this.guarantor.add(t); 2973 return t; 2974 } 2975 2976 public Account addGuarantor(GuarantorComponent t) { //3 2977 if (t == null) 2978 return this; 2979 if (this.guarantor == null) 2980 this.guarantor = new ArrayList<GuarantorComponent>(); 2981 this.guarantor.add(t); 2982 return this; 2983 } 2984 2985 /** 2986 * @return The first repetition of repeating field {@link #guarantor}, creating it if it does not already exist {3} 2987 */ 2988 public GuarantorComponent getGuarantorFirstRep() { 2989 if (getGuarantor().isEmpty()) { 2990 addGuarantor(); 2991 } 2992 return getGuarantor().get(0); 2993 } 2994 2995 /** 2996 * @return {@link #diagnosis} (When using an account for billing a specific Encounter the set of diagnoses that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s).) 2997 */ 2998 public List<AccountDiagnosisComponent> getDiagnosis() { 2999 if (this.diagnosis == null) 3000 this.diagnosis = new ArrayList<AccountDiagnosisComponent>(); 3001 return this.diagnosis; 3002 } 3003 3004 /** 3005 * @return Returns a reference to <code>this</code> for easy method chaining 3006 */ 3007 public Account setDiagnosis(List<AccountDiagnosisComponent> theDiagnosis) { 3008 this.diagnosis = theDiagnosis; 3009 return this; 3010 } 3011 3012 public boolean hasDiagnosis() { 3013 if (this.diagnosis == null) 3014 return false; 3015 for (AccountDiagnosisComponent item : this.diagnosis) 3016 if (!item.isEmpty()) 3017 return true; 3018 return false; 3019 } 3020 3021 public AccountDiagnosisComponent addDiagnosis() { //3 3022 AccountDiagnosisComponent t = new AccountDiagnosisComponent(); 3023 if (this.diagnosis == null) 3024 this.diagnosis = new ArrayList<AccountDiagnosisComponent>(); 3025 this.diagnosis.add(t); 3026 return t; 3027 } 3028 3029 public Account addDiagnosis(AccountDiagnosisComponent t) { //3 3030 if (t == null) 3031 return this; 3032 if (this.diagnosis == null) 3033 this.diagnosis = new ArrayList<AccountDiagnosisComponent>(); 3034 this.diagnosis.add(t); 3035 return this; 3036 } 3037 3038 /** 3039 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 3040 */ 3041 public AccountDiagnosisComponent getDiagnosisFirstRep() { 3042 if (getDiagnosis().isEmpty()) { 3043 addDiagnosis(); 3044 } 3045 return getDiagnosis().get(0); 3046 } 3047 3048 /** 3049 * @return {@link #procedure} (When using an account for billing a specific Encounter the set of procedures that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s).) 3050 */ 3051 public List<AccountProcedureComponent> getProcedure() { 3052 if (this.procedure == null) 3053 this.procedure = new ArrayList<AccountProcedureComponent>(); 3054 return this.procedure; 3055 } 3056 3057 /** 3058 * @return Returns a reference to <code>this</code> for easy method chaining 3059 */ 3060 public Account setProcedure(List<AccountProcedureComponent> theProcedure) { 3061 this.procedure = theProcedure; 3062 return this; 3063 } 3064 3065 public boolean hasProcedure() { 3066 if (this.procedure == null) 3067 return false; 3068 for (AccountProcedureComponent item : this.procedure) 3069 if (!item.isEmpty()) 3070 return true; 3071 return false; 3072 } 3073 3074 public AccountProcedureComponent addProcedure() { //3 3075 AccountProcedureComponent t = new AccountProcedureComponent(); 3076 if (this.procedure == null) 3077 this.procedure = new ArrayList<AccountProcedureComponent>(); 3078 this.procedure.add(t); 3079 return t; 3080 } 3081 3082 public Account addProcedure(AccountProcedureComponent t) { //3 3083 if (t == null) 3084 return this; 3085 if (this.procedure == null) 3086 this.procedure = new ArrayList<AccountProcedureComponent>(); 3087 this.procedure.add(t); 3088 return this; 3089 } 3090 3091 /** 3092 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 3093 */ 3094 public AccountProcedureComponent getProcedureFirstRep() { 3095 if (getProcedure().isEmpty()) { 3096 addProcedure(); 3097 } 3098 return getProcedure().get(0); 3099 } 3100 3101 /** 3102 * @return {@link #relatedAccount} (Other associated accounts related to this account.) 3103 */ 3104 public List<AccountRelatedAccountComponent> getRelatedAccount() { 3105 if (this.relatedAccount == null) 3106 this.relatedAccount = new ArrayList<AccountRelatedAccountComponent>(); 3107 return this.relatedAccount; 3108 } 3109 3110 /** 3111 * @return Returns a reference to <code>this</code> for easy method chaining 3112 */ 3113 public Account setRelatedAccount(List<AccountRelatedAccountComponent> theRelatedAccount) { 3114 this.relatedAccount = theRelatedAccount; 3115 return this; 3116 } 3117 3118 public boolean hasRelatedAccount() { 3119 if (this.relatedAccount == null) 3120 return false; 3121 for (AccountRelatedAccountComponent item : this.relatedAccount) 3122 if (!item.isEmpty()) 3123 return true; 3124 return false; 3125 } 3126 3127 public AccountRelatedAccountComponent addRelatedAccount() { //3 3128 AccountRelatedAccountComponent t = new AccountRelatedAccountComponent(); 3129 if (this.relatedAccount == null) 3130 this.relatedAccount = new ArrayList<AccountRelatedAccountComponent>(); 3131 this.relatedAccount.add(t); 3132 return t; 3133 } 3134 3135 public Account addRelatedAccount(AccountRelatedAccountComponent t) { //3 3136 if (t == null) 3137 return this; 3138 if (this.relatedAccount == null) 3139 this.relatedAccount = new ArrayList<AccountRelatedAccountComponent>(); 3140 this.relatedAccount.add(t); 3141 return this; 3142 } 3143 3144 /** 3145 * @return The first repetition of repeating field {@link #relatedAccount}, creating it if it does not already exist {3} 3146 */ 3147 public AccountRelatedAccountComponent getRelatedAccountFirstRep() { 3148 if (getRelatedAccount().isEmpty()) { 3149 addRelatedAccount(); 3150 } 3151 return getRelatedAccount().get(0); 3152 } 3153 3154 /** 3155 * @return {@link #currency} (The default currency for the account.) 3156 */ 3157 public CodeableConcept getCurrency() { 3158 if (this.currency == null) 3159 if (Configuration.errorOnAutoCreate()) 3160 throw new Error("Attempt to auto-create Account.currency"); 3161 else if (Configuration.doAutoCreate()) 3162 this.currency = new CodeableConcept(); // cc 3163 return this.currency; 3164 } 3165 3166 public boolean hasCurrency() { 3167 return this.currency != null && !this.currency.isEmpty(); 3168 } 3169 3170 /** 3171 * @param value {@link #currency} (The default currency for the account.) 3172 */ 3173 public Account setCurrency(CodeableConcept value) { 3174 this.currency = value; 3175 return this; 3176 } 3177 3178 /** 3179 * @return {@link #balance} (The calculated account balances - these are calculated and processed by the finance system. 3180 3181The balances with a `term` that is not current are usually generated/updated by an invoicing or similar process.) 3182 */ 3183 public List<AccountBalanceComponent> getBalance() { 3184 if (this.balance == null) 3185 this.balance = new ArrayList<AccountBalanceComponent>(); 3186 return this.balance; 3187 } 3188 3189 /** 3190 * @return Returns a reference to <code>this</code> for easy method chaining 3191 */ 3192 public Account setBalance(List<AccountBalanceComponent> theBalance) { 3193 this.balance = theBalance; 3194 return this; 3195 } 3196 3197 public boolean hasBalance() { 3198 if (this.balance == null) 3199 return false; 3200 for (AccountBalanceComponent item : this.balance) 3201 if (!item.isEmpty()) 3202 return true; 3203 return false; 3204 } 3205 3206 public AccountBalanceComponent addBalance() { //3 3207 AccountBalanceComponent t = new AccountBalanceComponent(); 3208 if (this.balance == null) 3209 this.balance = new ArrayList<AccountBalanceComponent>(); 3210 this.balance.add(t); 3211 return t; 3212 } 3213 3214 public Account addBalance(AccountBalanceComponent t) { //3 3215 if (t == null) 3216 return this; 3217 if (this.balance == null) 3218 this.balance = new ArrayList<AccountBalanceComponent>(); 3219 this.balance.add(t); 3220 return this; 3221 } 3222 3223 /** 3224 * @return The first repetition of repeating field {@link #balance}, creating it if it does not already exist {3} 3225 */ 3226 public AccountBalanceComponent getBalanceFirstRep() { 3227 if (getBalance().isEmpty()) { 3228 addBalance(); 3229 } 3230 return getBalance().get(0); 3231 } 3232 3233 /** 3234 * @return {@link #calculatedAt} (Time the balance amount was calculated.). This is the underlying object with id, value and extensions. The accessor "getCalculatedAt" gives direct access to the value 3235 */ 3236 public InstantType getCalculatedAtElement() { 3237 if (this.calculatedAt == null) 3238 if (Configuration.errorOnAutoCreate()) 3239 throw new Error("Attempt to auto-create Account.calculatedAt"); 3240 else if (Configuration.doAutoCreate()) 3241 this.calculatedAt = new InstantType(); // bb 3242 return this.calculatedAt; 3243 } 3244 3245 public boolean hasCalculatedAtElement() { 3246 return this.calculatedAt != null && !this.calculatedAt.isEmpty(); 3247 } 3248 3249 public boolean hasCalculatedAt() { 3250 return this.calculatedAt != null && !this.calculatedAt.isEmpty(); 3251 } 3252 3253 /** 3254 * @param value {@link #calculatedAt} (Time the balance amount was calculated.). This is the underlying object with id, value and extensions. The accessor "getCalculatedAt" gives direct access to the value 3255 */ 3256 public Account setCalculatedAtElement(InstantType value) { 3257 this.calculatedAt = value; 3258 return this; 3259 } 3260 3261 /** 3262 * @return Time the balance amount was calculated. 3263 */ 3264 public Date getCalculatedAt() { 3265 return this.calculatedAt == null ? null : this.calculatedAt.getValue(); 3266 } 3267 3268 /** 3269 * @param value Time the balance amount was calculated. 3270 */ 3271 public Account setCalculatedAt(Date value) { 3272 if (value == null) 3273 this.calculatedAt = null; 3274 else { 3275 if (this.calculatedAt == null) 3276 this.calculatedAt = new InstantType(); 3277 this.calculatedAt.setValue(value); 3278 } 3279 return this; 3280 } 3281 3282 protected void listChildren(List<Property> children) { 3283 super.listChildren(children); 3284 children.add(new Property("identifier", "Identifier", "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).", 0, java.lang.Integer.MAX_VALUE, identifier)); 3285 children.add(new Property("status", "code", "Indicates whether the account is presently used/usable or not.", 0, 1, status)); 3286 children.add(new Property("billingStatus", "CodeableConcept", "The BillingStatus tracks the lifecycle of the account through the billing process. It indicates how transactions are treated when they are allocated to the account.", 0, 1, billingStatus)); 3287 children.add(new Property("type", "CodeableConcept", "Categorizes the account for reporting and searching purposes.", 0, 1, type)); 3288 children.add(new Property("name", "string", "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name)); 3289 children.add(new Property("subject", "Reference(Patient|Device|Practitioner|PractitionerRole|Location|HealthcareService|Organization)", "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.", 0, java.lang.Integer.MAX_VALUE, subject)); 3290 children.add(new Property("servicePeriod", "Period", "The date range of services associated with this account.", 0, 1, servicePeriod)); 3291 children.add(new Property("coverage", "", "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 0, java.lang.Integer.MAX_VALUE, coverage)); 3292 children.add(new Property("owner", "Reference(Organization)", "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.", 0, 1, owner)); 3293 children.add(new Property("description", "markdown", "Provides additional information about what the account tracks and how it is used.", 0, 1, description)); 3294 children.add(new Property("guarantor", "", "The parties responsible for balancing the account if other payment options fall short.", 0, java.lang.Integer.MAX_VALUE, guarantor)); 3295 children.add(new Property("diagnosis", "", "When using an account for billing a specific Encounter the set of diagnoses that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s).", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 3296 children.add(new Property("procedure", "", "When using an account for billing a specific Encounter the set of procedures that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s).", 0, java.lang.Integer.MAX_VALUE, procedure)); 3297 children.add(new Property("relatedAccount", "", "Other associated accounts related to this account.", 0, java.lang.Integer.MAX_VALUE, relatedAccount)); 3298 children.add(new Property("currency", "CodeableConcept", "The default currency for the account.", 0, 1, currency)); 3299 children.add(new Property("balance", "", "The calculated account balances - these are calculated and processed by the finance system.\r\rThe balances with a `term` that is not current are usually generated/updated by an invoicing or similar process.", 0, java.lang.Integer.MAX_VALUE, balance)); 3300 children.add(new Property("calculatedAt", "instant", "Time the balance amount was calculated.", 0, 1, calculatedAt)); 3301 } 3302 3303 @Override 3304 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3305 switch (_hash) { 3306 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier used to reference the account. Might or might not be intended for human use (e.g. credit card number).", 0, java.lang.Integer.MAX_VALUE, identifier); 3307 case -892481550: /*status*/ return new Property("status", "code", "Indicates whether the account is presently used/usable or not.", 0, 1, status); 3308 case -1524378035: /*billingStatus*/ return new Property("billingStatus", "CodeableConcept", "The BillingStatus tracks the lifecycle of the account through the billing process. It indicates how transactions are treated when they are allocated to the account.", 0, 1, billingStatus); 3309 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorizes the account for reporting and searching purposes.", 0, 1, type); 3310 case 3373707: /*name*/ return new Property("name", "string", "Name used for the account when displaying it to humans in reports, etc.", 0, 1, name); 3311 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Device|Practitioner|PractitionerRole|Location|HealthcareService|Organization)", "Identifies the entity which incurs the expenses. While the immediate recipients of services or goods might be entities related to the subject, the expenses were ultimately incurred by the subject of the Account.", 0, java.lang.Integer.MAX_VALUE, subject); 3312 case 2129104086: /*servicePeriod*/ return new Property("servicePeriod", "Period", "The date range of services associated with this account.", 0, 1, servicePeriod); 3313 case -351767064: /*coverage*/ return new Property("coverage", "", "The party(s) that are responsible for covering the payment of this account, and what order should they be applied to the account.", 0, java.lang.Integer.MAX_VALUE, coverage); 3314 case 106164915: /*owner*/ return new Property("owner", "Reference(Organization)", "Indicates the service area, hospital, department, etc. with responsibility for managing the Account.", 0, 1, owner); 3315 case -1724546052: /*description*/ return new Property("description", "markdown", "Provides additional information about what the account tracks and how it is used.", 0, 1, description); 3316 case -188629045: /*guarantor*/ return new Property("guarantor", "", "The parties responsible for balancing the account if other payment options fall short.", 0, java.lang.Integer.MAX_VALUE, guarantor); 3317 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "When using an account for billing a specific Encounter the set of diagnoses that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s).", 0, java.lang.Integer.MAX_VALUE, diagnosis); 3318 case -1095204141: /*procedure*/ return new Property("procedure", "", "When using an account for billing a specific Encounter the set of procedures that are relevant for billing are stored here on the account where they are able to be sequenced appropriately prior to processing to produce claim(s).", 0, java.lang.Integer.MAX_VALUE, procedure); 3319 case 962039682: /*relatedAccount*/ return new Property("relatedAccount", "", "Other associated accounts related to this account.", 0, java.lang.Integer.MAX_VALUE, relatedAccount); 3320 case 575402001: /*currency*/ return new Property("currency", "CodeableConcept", "The default currency for the account.", 0, 1, currency); 3321 case -339185956: /*balance*/ return new Property("balance", "", "The calculated account balances - these are calculated and processed by the finance system.\r\rThe balances with a `term` that is not current are usually generated/updated by an invoicing or similar process.", 0, java.lang.Integer.MAX_VALUE, balance); 3322 case 1089469073: /*calculatedAt*/ return new Property("calculatedAt", "instant", "Time the balance amount was calculated.", 0, 1, calculatedAt); 3323 default: return super.getNamedProperty(_hash, _name, _checkValid); 3324 } 3325 3326 } 3327 3328 @Override 3329 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3330 switch (hash) { 3331 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3332 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<AccountStatus> 3333 case -1524378035: /*billingStatus*/ return this.billingStatus == null ? new Base[0] : new Base[] {this.billingStatus}; // CodeableConcept 3334 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3335 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3336 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 3337 case 2129104086: /*servicePeriod*/ return this.servicePeriod == null ? new Base[0] : new Base[] {this.servicePeriod}; // Period 3338 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // CoverageComponent 3339 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 3340 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3341 case -188629045: /*guarantor*/ return this.guarantor == null ? new Base[0] : this.guarantor.toArray(new Base[this.guarantor.size()]); // GuarantorComponent 3342 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // AccountDiagnosisComponent 3343 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // AccountProcedureComponent 3344 case 962039682: /*relatedAccount*/ return this.relatedAccount == null ? new Base[0] : this.relatedAccount.toArray(new Base[this.relatedAccount.size()]); // AccountRelatedAccountComponent 3345 case 575402001: /*currency*/ return this.currency == null ? new Base[0] : new Base[] {this.currency}; // CodeableConcept 3346 case -339185956: /*balance*/ return this.balance == null ? new Base[0] : this.balance.toArray(new Base[this.balance.size()]); // AccountBalanceComponent 3347 case 1089469073: /*calculatedAt*/ return this.calculatedAt == null ? new Base[0] : new Base[] {this.calculatedAt}; // InstantType 3348 default: return super.getProperty(hash, name, checkValid); 3349 } 3350 3351 } 3352 3353 @Override 3354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3355 switch (hash) { 3356 case -1618432855: // identifier 3357 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3358 return value; 3359 case -892481550: // status 3360 value = new AccountStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3361 this.status = (Enumeration) value; // Enumeration<AccountStatus> 3362 return value; 3363 case -1524378035: // billingStatus 3364 this.billingStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3365 return value; 3366 case 3575610: // type 3367 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3368 return value; 3369 case 3373707: // name 3370 this.name = TypeConvertor.castToString(value); // StringType 3371 return value; 3372 case -1867885268: // subject 3373 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 3374 return value; 3375 case 2129104086: // servicePeriod 3376 this.servicePeriod = TypeConvertor.castToPeriod(value); // Period 3377 return value; 3378 case -351767064: // coverage 3379 this.getCoverage().add((CoverageComponent) value); // CoverageComponent 3380 return value; 3381 case 106164915: // owner 3382 this.owner = TypeConvertor.castToReference(value); // Reference 3383 return value; 3384 case -1724546052: // description 3385 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3386 return value; 3387 case -188629045: // guarantor 3388 this.getGuarantor().add((GuarantorComponent) value); // GuarantorComponent 3389 return value; 3390 case 1196993265: // diagnosis 3391 this.getDiagnosis().add((AccountDiagnosisComponent) value); // AccountDiagnosisComponent 3392 return value; 3393 case -1095204141: // procedure 3394 this.getProcedure().add((AccountProcedureComponent) value); // AccountProcedureComponent 3395 return value; 3396 case 962039682: // relatedAccount 3397 this.getRelatedAccount().add((AccountRelatedAccountComponent) value); // AccountRelatedAccountComponent 3398 return value; 3399 case 575402001: // currency 3400 this.currency = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3401 return value; 3402 case -339185956: // balance 3403 this.getBalance().add((AccountBalanceComponent) value); // AccountBalanceComponent 3404 return value; 3405 case 1089469073: // calculatedAt 3406 this.calculatedAt = TypeConvertor.castToInstant(value); // InstantType 3407 return value; 3408 default: return super.setProperty(hash, name, value); 3409 } 3410 3411 } 3412 3413 @Override 3414 public Base setProperty(String name, Base value) throws FHIRException { 3415 if (name.equals("identifier")) { 3416 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3417 } else if (name.equals("status")) { 3418 value = new AccountStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3419 this.status = (Enumeration) value; // Enumeration<AccountStatus> 3420 } else if (name.equals("billingStatus")) { 3421 this.billingStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3422 } else if (name.equals("type")) { 3423 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3424 } else if (name.equals("name")) { 3425 this.name = TypeConvertor.castToString(value); // StringType 3426 } else if (name.equals("subject")) { 3427 this.getSubject().add(TypeConvertor.castToReference(value)); 3428 } else if (name.equals("servicePeriod")) { 3429 this.servicePeriod = TypeConvertor.castToPeriod(value); // Period 3430 } else if (name.equals("coverage")) { 3431 this.getCoverage().add((CoverageComponent) value); 3432 } else if (name.equals("owner")) { 3433 this.owner = TypeConvertor.castToReference(value); // Reference 3434 } else if (name.equals("description")) { 3435 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3436 } else if (name.equals("guarantor")) { 3437 this.getGuarantor().add((GuarantorComponent) value); 3438 } else if (name.equals("diagnosis")) { 3439 this.getDiagnosis().add((AccountDiagnosisComponent) value); 3440 } else if (name.equals("procedure")) { 3441 this.getProcedure().add((AccountProcedureComponent) value); 3442 } else if (name.equals("relatedAccount")) { 3443 this.getRelatedAccount().add((AccountRelatedAccountComponent) value); 3444 } else if (name.equals("currency")) { 3445 this.currency = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3446 } else if (name.equals("balance")) { 3447 this.getBalance().add((AccountBalanceComponent) value); 3448 } else if (name.equals("calculatedAt")) { 3449 this.calculatedAt = TypeConvertor.castToInstant(value); // InstantType 3450 } else 3451 return super.setProperty(name, value); 3452 return value; 3453 } 3454 3455 @Override 3456 public void removeChild(String name, Base value) throws FHIRException { 3457 if (name.equals("identifier")) { 3458 this.getIdentifier().remove(value); 3459 } else if (name.equals("status")) { 3460 value = new AccountStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3461 this.status = (Enumeration) value; // Enumeration<AccountStatus> 3462 } else if (name.equals("billingStatus")) { 3463 this.billingStatus = null; 3464 } else if (name.equals("type")) { 3465 this.type = null; 3466 } else if (name.equals("name")) { 3467 this.name = null; 3468 } else if (name.equals("subject")) { 3469 this.getSubject().remove(value); 3470 } else if (name.equals("servicePeriod")) { 3471 this.servicePeriod = null; 3472 } else if (name.equals("coverage")) { 3473 this.getCoverage().remove((CoverageComponent) value); 3474 } else if (name.equals("owner")) { 3475 this.owner = null; 3476 } else if (name.equals("description")) { 3477 this.description = null; 3478 } else if (name.equals("guarantor")) { 3479 this.getGuarantor().remove((GuarantorComponent) value); 3480 } else if (name.equals("diagnosis")) { 3481 this.getDiagnosis().remove((AccountDiagnosisComponent) value); 3482 } else if (name.equals("procedure")) { 3483 this.getProcedure().remove((AccountProcedureComponent) value); 3484 } else if (name.equals("relatedAccount")) { 3485 this.getRelatedAccount().remove((AccountRelatedAccountComponent) value); 3486 } else if (name.equals("currency")) { 3487 this.currency = null; 3488 } else if (name.equals("balance")) { 3489 this.getBalance().remove((AccountBalanceComponent) value); 3490 } else if (name.equals("calculatedAt")) { 3491 this.calculatedAt = null; 3492 } else 3493 super.removeChild(name, value); 3494 3495 } 3496 3497 @Override 3498 public Base makeProperty(int hash, String name) throws FHIRException { 3499 switch (hash) { 3500 case -1618432855: return addIdentifier(); 3501 case -892481550: return getStatusElement(); 3502 case -1524378035: return getBillingStatus(); 3503 case 3575610: return getType(); 3504 case 3373707: return getNameElement(); 3505 case -1867885268: return addSubject(); 3506 case 2129104086: return getServicePeriod(); 3507 case -351767064: return addCoverage(); 3508 case 106164915: return getOwner(); 3509 case -1724546052: return getDescriptionElement(); 3510 case -188629045: return addGuarantor(); 3511 case 1196993265: return addDiagnosis(); 3512 case -1095204141: return addProcedure(); 3513 case 962039682: return addRelatedAccount(); 3514 case 575402001: return getCurrency(); 3515 case -339185956: return addBalance(); 3516 case 1089469073: return getCalculatedAtElement(); 3517 default: return super.makeProperty(hash, name); 3518 } 3519 3520 } 3521 3522 @Override 3523 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3524 switch (hash) { 3525 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3526 case -892481550: /*status*/ return new String[] {"code"}; 3527 case -1524378035: /*billingStatus*/ return new String[] {"CodeableConcept"}; 3528 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3529 case 3373707: /*name*/ return new String[] {"string"}; 3530 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3531 case 2129104086: /*servicePeriod*/ return new String[] {"Period"}; 3532 case -351767064: /*coverage*/ return new String[] {}; 3533 case 106164915: /*owner*/ return new String[] {"Reference"}; 3534 case -1724546052: /*description*/ return new String[] {"markdown"}; 3535 case -188629045: /*guarantor*/ return new String[] {}; 3536 case 1196993265: /*diagnosis*/ return new String[] {}; 3537 case -1095204141: /*procedure*/ return new String[] {}; 3538 case 962039682: /*relatedAccount*/ return new String[] {}; 3539 case 575402001: /*currency*/ return new String[] {"CodeableConcept"}; 3540 case -339185956: /*balance*/ return new String[] {}; 3541 case 1089469073: /*calculatedAt*/ return new String[] {"instant"}; 3542 default: return super.getTypesForProperty(hash, name); 3543 } 3544 3545 } 3546 3547 @Override 3548 public Base addChild(String name) throws FHIRException { 3549 if (name.equals("identifier")) { 3550 return addIdentifier(); 3551 } 3552 else if (name.equals("status")) { 3553 throw new FHIRException("Cannot call addChild on a singleton property Account.status"); 3554 } 3555 else if (name.equals("billingStatus")) { 3556 this.billingStatus = new CodeableConcept(); 3557 return this.billingStatus; 3558 } 3559 else if (name.equals("type")) { 3560 this.type = new CodeableConcept(); 3561 return this.type; 3562 } 3563 else if (name.equals("name")) { 3564 throw new FHIRException("Cannot call addChild on a singleton property Account.name"); 3565 } 3566 else if (name.equals("subject")) { 3567 return addSubject(); 3568 } 3569 else if (name.equals("servicePeriod")) { 3570 this.servicePeriod = new Period(); 3571 return this.servicePeriod; 3572 } 3573 else if (name.equals("coverage")) { 3574 return addCoverage(); 3575 } 3576 else if (name.equals("owner")) { 3577 this.owner = new Reference(); 3578 return this.owner; 3579 } 3580 else if (name.equals("description")) { 3581 throw new FHIRException("Cannot call addChild on a singleton property Account.description"); 3582 } 3583 else if (name.equals("guarantor")) { 3584 return addGuarantor(); 3585 } 3586 else if (name.equals("diagnosis")) { 3587 return addDiagnosis(); 3588 } 3589 else if (name.equals("procedure")) { 3590 return addProcedure(); 3591 } 3592 else if (name.equals("relatedAccount")) { 3593 return addRelatedAccount(); 3594 } 3595 else if (name.equals("currency")) { 3596 this.currency = new CodeableConcept(); 3597 return this.currency; 3598 } 3599 else if (name.equals("balance")) { 3600 return addBalance(); 3601 } 3602 else if (name.equals("calculatedAt")) { 3603 throw new FHIRException("Cannot call addChild on a singleton property Account.calculatedAt"); 3604 } 3605 else 3606 return super.addChild(name); 3607 } 3608 3609 public String fhirType() { 3610 return "Account"; 3611 3612 } 3613 3614 public Account copy() { 3615 Account dst = new Account(); 3616 copyValues(dst); 3617 return dst; 3618 } 3619 3620 public void copyValues(Account dst) { 3621 super.copyValues(dst); 3622 if (identifier != null) { 3623 dst.identifier = new ArrayList<Identifier>(); 3624 for (Identifier i : identifier) 3625 dst.identifier.add(i.copy()); 3626 }; 3627 dst.status = status == null ? null : status.copy(); 3628 dst.billingStatus = billingStatus == null ? null : billingStatus.copy(); 3629 dst.type = type == null ? null : type.copy(); 3630 dst.name = name == null ? null : name.copy(); 3631 if (subject != null) { 3632 dst.subject = new ArrayList<Reference>(); 3633 for (Reference i : subject) 3634 dst.subject.add(i.copy()); 3635 }; 3636 dst.servicePeriod = servicePeriod == null ? null : servicePeriod.copy(); 3637 if (coverage != null) { 3638 dst.coverage = new ArrayList<CoverageComponent>(); 3639 for (CoverageComponent i : coverage) 3640 dst.coverage.add(i.copy()); 3641 }; 3642 dst.owner = owner == null ? null : owner.copy(); 3643 dst.description = description == null ? null : description.copy(); 3644 if (guarantor != null) { 3645 dst.guarantor = new ArrayList<GuarantorComponent>(); 3646 for (GuarantorComponent i : guarantor) 3647 dst.guarantor.add(i.copy()); 3648 }; 3649 if (diagnosis != null) { 3650 dst.diagnosis = new ArrayList<AccountDiagnosisComponent>(); 3651 for (AccountDiagnosisComponent i : diagnosis) 3652 dst.diagnosis.add(i.copy()); 3653 }; 3654 if (procedure != null) { 3655 dst.procedure = new ArrayList<AccountProcedureComponent>(); 3656 for (AccountProcedureComponent i : procedure) 3657 dst.procedure.add(i.copy()); 3658 }; 3659 if (relatedAccount != null) { 3660 dst.relatedAccount = new ArrayList<AccountRelatedAccountComponent>(); 3661 for (AccountRelatedAccountComponent i : relatedAccount) 3662 dst.relatedAccount.add(i.copy()); 3663 }; 3664 dst.currency = currency == null ? null : currency.copy(); 3665 if (balance != null) { 3666 dst.balance = new ArrayList<AccountBalanceComponent>(); 3667 for (AccountBalanceComponent i : balance) 3668 dst.balance.add(i.copy()); 3669 }; 3670 dst.calculatedAt = calculatedAt == null ? null : calculatedAt.copy(); 3671 } 3672 3673 protected Account typedCopy() { 3674 return copy(); 3675 } 3676 3677 @Override 3678 public boolean equalsDeep(Base other_) { 3679 if (!super.equalsDeep(other_)) 3680 return false; 3681 if (!(other_ instanceof Account)) 3682 return false; 3683 Account o = (Account) other_; 3684 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(billingStatus, o.billingStatus, true) 3685 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) 3686 && compareDeep(servicePeriod, o.servicePeriod, true) && compareDeep(coverage, o.coverage, true) 3687 && compareDeep(owner, o.owner, true) && compareDeep(description, o.description, true) && compareDeep(guarantor, o.guarantor, true) 3688 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) && compareDeep(relatedAccount, o.relatedAccount, true) 3689 && compareDeep(currency, o.currency, true) && compareDeep(balance, o.balance, true) && compareDeep(calculatedAt, o.calculatedAt, true) 3690 ; 3691 } 3692 3693 @Override 3694 public boolean equalsShallow(Base other_) { 3695 if (!super.equalsShallow(other_)) 3696 return false; 3697 if (!(other_ instanceof Account)) 3698 return false; 3699 Account o = (Account) other_; 3700 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 3701 && compareValues(calculatedAt, o.calculatedAt, true); 3702 } 3703 3704 public boolean isEmpty() { 3705 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, billingStatus 3706 , type, name, subject, servicePeriod, coverage, owner, description, guarantor 3707 , diagnosis, procedure, relatedAccount, currency, balance, calculatedAt); 3708 } 3709 3710 @Override 3711 public ResourceType getResourceType() { 3712 return ResourceType.Account; 3713 } 3714 3715 /** 3716 * Search parameter: <b>guarantor</b> 3717 * <p> 3718 * Description: <b>The parties ultimately responsible for balancing the Account</b><br> 3719 * Type: <b>reference</b><br> 3720 * Path: <b>Account.guarantor.party</b><br> 3721 * </p> 3722 */ 3723 @SearchParamDefinition(name="guarantor", path="Account.guarantor.party", description="The parties ultimately responsible for balancing the Account", type="reference", target={Organization.class, Patient.class, RelatedPerson.class } ) 3724 public static final String SP_GUARANTOR = "guarantor"; 3725 /** 3726 * <b>Fluent Client</b> search parameter constant for <b>guarantor</b> 3727 * <p> 3728 * Description: <b>The parties ultimately responsible for balancing the Account</b><br> 3729 * Type: <b>reference</b><br> 3730 * Path: <b>Account.guarantor.party</b><br> 3731 * </p> 3732 */ 3733 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GUARANTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GUARANTOR); 3734 3735/** 3736 * Constant for fluent queries to be used to add include statements. Specifies 3737 * the path value of "<b>Account:guarantor</b>". 3738 */ 3739 public static final ca.uhn.fhir.model.api.Include INCLUDE_GUARANTOR = new ca.uhn.fhir.model.api.Include("Account:guarantor").toLocked(); 3740 3741 /** 3742 * Search parameter: <b>name</b> 3743 * <p> 3744 * Description: <b>Human-readable label</b><br> 3745 * Type: <b>string</b><br> 3746 * Path: <b>Account.name</b><br> 3747 * </p> 3748 */ 3749 @SearchParamDefinition(name="name", path="Account.name", description="Human-readable label", type="string" ) 3750 public static final String SP_NAME = "name"; 3751 /** 3752 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3753 * <p> 3754 * Description: <b>Human-readable label</b><br> 3755 * Type: <b>string</b><br> 3756 * Path: <b>Account.name</b><br> 3757 * </p> 3758 */ 3759 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3760 3761 /** 3762 * Search parameter: <b>owner</b> 3763 * <p> 3764 * Description: <b>Entity managing the Account</b><br> 3765 * Type: <b>reference</b><br> 3766 * Path: <b>Account.owner</b><br> 3767 * </p> 3768 */ 3769 @SearchParamDefinition(name="owner", path="Account.owner", description="Entity managing the Account", type="reference", target={Organization.class } ) 3770 public static final String SP_OWNER = "owner"; 3771 /** 3772 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 3773 * <p> 3774 * Description: <b>Entity managing the Account</b><br> 3775 * Type: <b>reference</b><br> 3776 * Path: <b>Account.owner</b><br> 3777 * </p> 3778 */ 3779 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OWNER); 3780 3781/** 3782 * Constant for fluent queries to be used to add include statements. Specifies 3783 * the path value of "<b>Account:owner</b>". 3784 */ 3785 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Account:owner").toLocked(); 3786 3787 /** 3788 * Search parameter: <b>period</b> 3789 * <p> 3790 * Description: <b>Transaction window</b><br> 3791 * Type: <b>date</b><br> 3792 * Path: <b>Account.servicePeriod</b><br> 3793 * </p> 3794 */ 3795 @SearchParamDefinition(name="period", path="Account.servicePeriod", description="Transaction window", type="date" ) 3796 public static final String SP_PERIOD = "period"; 3797 /** 3798 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3799 * <p> 3800 * Description: <b>Transaction window</b><br> 3801 * Type: <b>date</b><br> 3802 * Path: <b>Account.servicePeriod</b><br> 3803 * </p> 3804 */ 3805 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 3806 3807 /** 3808 * Search parameter: <b>relatedaccount</b> 3809 * <p> 3810 * Description: <b>Parent and other related accounts</b><br> 3811 * Type: <b>reference</b><br> 3812 * Path: <b>Account.relatedAccount.account</b><br> 3813 * </p> 3814 */ 3815 @SearchParamDefinition(name="relatedaccount", path="Account.relatedAccount.account", description="Parent and other related accounts", type="reference", target={Account.class } ) 3816 public static final String SP_RELATEDACCOUNT = "relatedaccount"; 3817 /** 3818 * <b>Fluent Client</b> search parameter constant for <b>relatedaccount</b> 3819 * <p> 3820 * Description: <b>Parent and other related accounts</b><br> 3821 * Type: <b>reference</b><br> 3822 * Path: <b>Account.relatedAccount.account</b><br> 3823 * </p> 3824 */ 3825 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATEDACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATEDACCOUNT); 3826 3827/** 3828 * Constant for fluent queries to be used to add include statements. Specifies 3829 * the path value of "<b>Account:relatedaccount</b>". 3830 */ 3831 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATEDACCOUNT = new ca.uhn.fhir.model.api.Include("Account:relatedaccount").toLocked(); 3832 3833 /** 3834 * Search parameter: <b>status</b> 3835 * <p> 3836 * Description: <b>active | inactive | entered-in-error | on-hold | unknown</b><br> 3837 * Type: <b>token</b><br> 3838 * Path: <b>Account.status</b><br> 3839 * </p> 3840 */ 3841 @SearchParamDefinition(name="status", path="Account.status", description="active | inactive | entered-in-error | on-hold | unknown", type="token" ) 3842 public static final String SP_STATUS = "status"; 3843 /** 3844 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3845 * <p> 3846 * Description: <b>active | inactive | entered-in-error | on-hold | unknown</b><br> 3847 * Type: <b>token</b><br> 3848 * Path: <b>Account.status</b><br> 3849 * </p> 3850 */ 3851 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3852 3853 /** 3854 * Search parameter: <b>subject</b> 3855 * <p> 3856 * Description: <b>The entity that caused the expenses</b><br> 3857 * Type: <b>reference</b><br> 3858 * Path: <b>Account.subject</b><br> 3859 * </p> 3860 */ 3861 @SearchParamDefinition(name="subject", path="Account.subject", description="The entity that caused the expenses", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class } ) 3862 public static final String SP_SUBJECT = "subject"; 3863 /** 3864 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3865 * <p> 3866 * Description: <b>The entity that caused the expenses</b><br> 3867 * Type: <b>reference</b><br> 3868 * Path: <b>Account.subject</b><br> 3869 * </p> 3870 */ 3871 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3872 3873/** 3874 * Constant for fluent queries to be used to add include statements. Specifies 3875 * the path value of "<b>Account:subject</b>". 3876 */ 3877 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Account:subject").toLocked(); 3878 3879 /** 3880 * Search parameter: <b>identifier</b> 3881 * <p> 3882 * Description: <b>Multiple Resources: 3883 3884* [Account](account.html): Account number 3885* [AdverseEvent](adverseevent.html): Business identifier for the event 3886* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3887* [Appointment](appointment.html): An Identifier of the Appointment 3888* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3889* [Basic](basic.html): Business identifier 3890* [BodyStructure](bodystructure.html): Bodystructure identifier 3891* [CarePlan](careplan.html): External Ids for this plan 3892* [CareTeam](careteam.html): External Ids for this team 3893* [ChargeItem](chargeitem.html): Business Identifier for item 3894* [Claim](claim.html): The primary identifier of the financial resource 3895* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3896* [ClinicalImpression](clinicalimpression.html): Business identifier 3897* [Communication](communication.html): Unique identifier 3898* [CommunicationRequest](communicationrequest.html): Unique identifier 3899* [Composition](composition.html): Version-independent identifier for the Composition 3900* [Condition](condition.html): A unique identifier of the condition record 3901* [Consent](consent.html): Identifier for this record (external references) 3902* [Contract](contract.html): The identity of the contract 3903* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3904* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3905* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3906* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3907* [DeviceRequest](devicerequest.html): Business identifier for request/order 3908* [DeviceUsage](deviceusage.html): Search by identifier 3909* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3910* [DocumentReference](documentreference.html): Identifier of the attachment binary 3911* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3912* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3913* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3914* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3915* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3916* [Flag](flag.html): Business identifier 3917* [Goal](goal.html): External Ids for this goal 3918* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3919* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3920* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3921* [Immunization](immunization.html): Business identifier 3922* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3923* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3924* [Invoice](invoice.html): Business Identifier for item 3925* [List](list.html): Business identifier 3926* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3927* [Medication](medication.html): Returns medications with this external identifier 3928* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3929* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3930* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3931* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3932* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3933* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3934* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3935* [Observation](observation.html): The unique id for a particular observation 3936* [Person](person.html): A person Identifier 3937* [Procedure](procedure.html): A unique identifier for a procedure 3938* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3939* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3940* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3941* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3942* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3943* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3944* [Specimen](specimen.html): The unique identifier associated with the specimen 3945* [SupplyDelivery](supplydelivery.html): External identifier 3946* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3947* [Task](task.html): Search for a task instance by its business identifier 3948* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3949</b><br> 3950 * Type: <b>token</b><br> 3951 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3952 * </p> 3953 */ 3954 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3955 public static final String SP_IDENTIFIER = "identifier"; 3956 /** 3957 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3958 * <p> 3959 * Description: <b>Multiple Resources: 3960 3961* [Account](account.html): Account number 3962* [AdverseEvent](adverseevent.html): Business identifier for the event 3963* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3964* [Appointment](appointment.html): An Identifier of the Appointment 3965* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3966* [Basic](basic.html): Business identifier 3967* [BodyStructure](bodystructure.html): Bodystructure identifier 3968* [CarePlan](careplan.html): External Ids for this plan 3969* [CareTeam](careteam.html): External Ids for this team 3970* [ChargeItem](chargeitem.html): Business Identifier for item 3971* [Claim](claim.html): The primary identifier of the financial resource 3972* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3973* [ClinicalImpression](clinicalimpression.html): Business identifier 3974* [Communication](communication.html): Unique identifier 3975* [CommunicationRequest](communicationrequest.html): Unique identifier 3976* [Composition](composition.html): Version-independent identifier for the Composition 3977* [Condition](condition.html): A unique identifier of the condition record 3978* [Consent](consent.html): Identifier for this record (external references) 3979* [Contract](contract.html): The identity of the contract 3980* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3981* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3982* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3983* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3984* [DeviceRequest](devicerequest.html): Business identifier for request/order 3985* [DeviceUsage](deviceusage.html): Search by identifier 3986* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3987* [DocumentReference](documentreference.html): Identifier of the attachment binary 3988* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3989* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3990* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3991* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3992* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3993* [Flag](flag.html): Business identifier 3994* [Goal](goal.html): External Ids for this goal 3995* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3996* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3997* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3998* [Immunization](immunization.html): Business identifier 3999* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4000* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4001* [Invoice](invoice.html): Business Identifier for item 4002* [List](list.html): Business identifier 4003* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4004* [Medication](medication.html): Returns medications with this external identifier 4005* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4006* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4007* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4008* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4009* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4010* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4011* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4012* [Observation](observation.html): The unique id for a particular observation 4013* [Person](person.html): A person Identifier 4014* [Procedure](procedure.html): A unique identifier for a procedure 4015* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4016* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4017* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4018* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4019* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4020* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4021* [Specimen](specimen.html): The unique identifier associated with the specimen 4022* [SupplyDelivery](supplydelivery.html): External identifier 4023* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4024* [Task](task.html): Search for a task instance by its business identifier 4025* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4026</b><br> 4027 * Type: <b>token</b><br> 4028 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4029 * </p> 4030 */ 4031 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4032 4033 /** 4034 * Search parameter: <b>patient</b> 4035 * <p> 4036 * Description: <b>Multiple Resources: 4037 4038* [Account](account.html): The entity that caused the expenses 4039* [AdverseEvent](adverseevent.html): Subject impacted by event 4040* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4041* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4042* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4043* [AuditEvent](auditevent.html): Where the activity involved patient data 4044* [Basic](basic.html): Identifies the focus of this resource 4045* [BodyStructure](bodystructure.html): Who this is about 4046* [CarePlan](careplan.html): Who the care plan is for 4047* [CareTeam](careteam.html): Who care team is for 4048* [ChargeItem](chargeitem.html): Individual service was done for/to 4049* [Claim](claim.html): Patient receiving the products or services 4050* [ClaimResponse](claimresponse.html): The subject of care 4051* [ClinicalImpression](clinicalimpression.html): Patient assessed 4052* [Communication](communication.html): Focus of message 4053* [CommunicationRequest](communicationrequest.html): Focus of message 4054* [Composition](composition.html): Who and/or what the composition is about 4055* [Condition](condition.html): Who has the condition? 4056* [Consent](consent.html): Who the consent applies to 4057* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4058* [Coverage](coverage.html): Retrieve coverages for a patient 4059* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4060* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4061* [DetectedIssue](detectedissue.html): Associated patient 4062* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4063* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4064* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4065* [DocumentReference](documentreference.html): Who/what is the subject of the document 4066* [Encounter](encounter.html): The patient present at the encounter 4067* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4068* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4069* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4070* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4071* [Flag](flag.html): The identity of a subject to list flags for 4072* [Goal](goal.html): Who this goal is intended for 4073* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4074* [ImagingSelection](imagingselection.html): Who the study is about 4075* [ImagingStudy](imagingstudy.html): Who the study is about 4076* [Immunization](immunization.html): The patient for the vaccination record 4077* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4078* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4079* [Invoice](invoice.html): Recipient(s) of goods and services 4080* [List](list.html): If all resources have the same subject 4081* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4082* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4083* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4084* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4085* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4086* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4087* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4088* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4089* [Observation](observation.html): The subject that the observation is about (if patient) 4090* [Person](person.html): The Person links to this Patient 4091* [Procedure](procedure.html): Search by subject - a patient 4092* [Provenance](provenance.html): Where the activity involved patient data 4093* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4094* [RelatedPerson](relatedperson.html): The patient this related person is related to 4095* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4096* [ResearchSubject](researchsubject.html): Who or what is part of study 4097* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4098* [ServiceRequest](servicerequest.html): Search by subject - a patient 4099* [Specimen](specimen.html): The patient the specimen comes from 4100* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4101* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4102* [Task](task.html): Search by patient 4103* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4104</b><br> 4105 * Type: <b>reference</b><br> 4106 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4107 * </p> 4108 */ 4109 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4110 public static final String SP_PATIENT = "patient"; 4111 /** 4112 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4113 * <p> 4114 * Description: <b>Multiple Resources: 4115 4116* [Account](account.html): The entity that caused the expenses 4117* [AdverseEvent](adverseevent.html): Subject impacted by event 4118* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4119* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4120* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4121* [AuditEvent](auditevent.html): Where the activity involved patient data 4122* [Basic](basic.html): Identifies the focus of this resource 4123* [BodyStructure](bodystructure.html): Who this is about 4124* [CarePlan](careplan.html): Who the care plan is for 4125* [CareTeam](careteam.html): Who care team is for 4126* [ChargeItem](chargeitem.html): Individual service was done for/to 4127* [Claim](claim.html): Patient receiving the products or services 4128* [ClaimResponse](claimresponse.html): The subject of care 4129* [ClinicalImpression](clinicalimpression.html): Patient assessed 4130* [Communication](communication.html): Focus of message 4131* [CommunicationRequest](communicationrequest.html): Focus of message 4132* [Composition](composition.html): Who and/or what the composition is about 4133* [Condition](condition.html): Who has the condition? 4134* [Consent](consent.html): Who the consent applies to 4135* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4136* [Coverage](coverage.html): Retrieve coverages for a patient 4137* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4138* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4139* [DetectedIssue](detectedissue.html): Associated patient 4140* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4141* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4142* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4143* [DocumentReference](documentreference.html): Who/what is the subject of the document 4144* [Encounter](encounter.html): The patient present at the encounter 4145* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4146* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4147* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4148* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4149* [Flag](flag.html): The identity of a subject to list flags for 4150* [Goal](goal.html): Who this goal is intended for 4151* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4152* [ImagingSelection](imagingselection.html): Who the study is about 4153* [ImagingStudy](imagingstudy.html): Who the study is about 4154* [Immunization](immunization.html): The patient for the vaccination record 4155* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4156* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4157* [Invoice](invoice.html): Recipient(s) of goods and services 4158* [List](list.html): If all resources have the same subject 4159* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4160* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4161* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4162* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4163* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4164* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4165* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4166* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4167* [Observation](observation.html): The subject that the observation is about (if patient) 4168* [Person](person.html): The Person links to this Patient 4169* [Procedure](procedure.html): Search by subject - a patient 4170* [Provenance](provenance.html): Where the activity involved patient data 4171* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4172* [RelatedPerson](relatedperson.html): The patient this related person is related to 4173* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4174* [ResearchSubject](researchsubject.html): Who or what is part of study 4175* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4176* [ServiceRequest](servicerequest.html): Search by subject - a patient 4177* [Specimen](specimen.html): The patient the specimen comes from 4178* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4179* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4180* [Task](task.html): Search by patient 4181* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4182</b><br> 4183 * Type: <b>reference</b><br> 4184 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4185 * </p> 4186 */ 4187 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4188 4189/** 4190 * Constant for fluent queries to be used to add include statements. Specifies 4191 * the path value of "<b>Account:patient</b>". 4192 */ 4193 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Account:patient").toLocked(); 4194 4195 /** 4196 * Search parameter: <b>type</b> 4197 * <p> 4198 * Description: <b>Multiple Resources: 4199 4200* [Account](account.html): E.g. patient, expense, depreciation 4201* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 4202* [Composition](composition.html): Kind of composition (LOINC if possible) 4203* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 4204* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 4205* [Encounter](encounter.html): Specific type of encounter 4206* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 4207* [Invoice](invoice.html): Type of Invoice 4208* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 4209* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 4210* [Specimen](specimen.html): The specimen type 4211</b><br> 4212 * Type: <b>token</b><br> 4213 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 4214 * </p> 4215 */ 4216 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 4217 public static final String SP_TYPE = "type"; 4218 /** 4219 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4220 * <p> 4221 * Description: <b>Multiple Resources: 4222 4223* [Account](account.html): E.g. patient, expense, depreciation 4224* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 4225* [Composition](composition.html): Kind of composition (LOINC if possible) 4226* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 4227* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 4228* [Encounter](encounter.html): Specific type of encounter 4229* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 4230* [Invoice](invoice.html): Type of Invoice 4231* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 4232* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 4233* [Specimen](specimen.html): The specimen type 4234</b><br> 4235 * Type: <b>token</b><br> 4236 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 4237 * </p> 4238 */ 4239 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4240 4241 4242} 4243