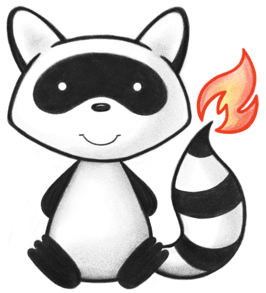
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 052 */ 053@ResourceDef(name="ActivityDefinition", profile="http://hl7.org/fhir/StructureDefinition/ActivityDefinition") 054public class ActivityDefinition extends MetadataResource { 055 056 public enum RequestResourceTypes { 057 /** 058 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 059 */ 060 APPOINTMENT, 061 /** 062 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 063 */ 064 APPOINTMENTRESPONSE, 065 /** 066 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 067 */ 068 CAREPLAN, 069 /** 070 * A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement. 071 */ 072 CLAIM, 073 /** 074 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 075 */ 076 COMMUNICATIONREQUEST, 077 /** 078 * The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 079 */ 080 COVERAGEELIGIBILITYREQUEST, 081 /** 082 * Represents a request a device to be provided to a specific patient. The device may be an implantable device to be subsequently implanted, or an external assistive device, such as a walker, to be delivered and subsequently be used. 083 */ 084 DEVICEREQUEST, 085 /** 086 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 087 */ 088 ENROLLMENTREQUEST, 089 /** 090 * A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification. 091 */ 092 IMMUNIZATIONRECOMMENDATION, 093 /** 094 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 095 */ 096 MEDICATIONREQUEST, 097 /** 098 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 099 */ 100 NUTRITIONORDER, 101 /** 102 * A set of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\". 103 */ 104 REQUESTORCHESTRATION, 105 /** 106 * A record of a request for service such as diagnostic investigations, treatments, or operations to be performed. 107 */ 108 SERVICEREQUEST, 109 /** 110 * A record of a non-patient specific request for a medication, substance, device, certain types of biologically derived product, and nutrition product used in the healthcare setting. 111 */ 112 SUPPLYREQUEST, 113 /** 114 * A task to be performed. 115 */ 116 TASK, 117 /** 118 * Record of transport. 119 */ 120 TRANSPORT, 121 /** 122 * An authorization for the provision of glasses and/or contact lenses to a patient. 123 */ 124 VISIONPRESCRIPTION, 125 /** 126 * added to help the parsers with the generic types 127 */ 128 NULL; 129 public static RequestResourceTypes fromCode(String codeString) throws FHIRException { 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("Appointment".equals(codeString)) 133 return APPOINTMENT; 134 if ("AppointmentResponse".equals(codeString)) 135 return APPOINTMENTRESPONSE; 136 if ("CarePlan".equals(codeString)) 137 return CAREPLAN; 138 if ("Claim".equals(codeString)) 139 return CLAIM; 140 if ("CommunicationRequest".equals(codeString)) 141 return COMMUNICATIONREQUEST; 142 if ("CoverageEligibilityRequest".equals(codeString)) 143 return COVERAGEELIGIBILITYREQUEST; 144 if ("DeviceRequest".equals(codeString)) 145 return DEVICEREQUEST; 146 if ("EnrollmentRequest".equals(codeString)) 147 return ENROLLMENTREQUEST; 148 if ("ImmunizationRecommendation".equals(codeString)) 149 return IMMUNIZATIONRECOMMENDATION; 150 if ("MedicationRequest".equals(codeString)) 151 return MEDICATIONREQUEST; 152 if ("NutritionOrder".equals(codeString)) 153 return NUTRITIONORDER; 154 if ("RequestOrchestration".equals(codeString)) 155 return REQUESTORCHESTRATION; 156 if ("ServiceRequest".equals(codeString)) 157 return SERVICEREQUEST; 158 if ("SupplyRequest".equals(codeString)) 159 return SUPPLYREQUEST; 160 if ("Task".equals(codeString)) 161 return TASK; 162 if ("Transport".equals(codeString)) 163 return TRANSPORT; 164 if ("VisionPrescription".equals(codeString)) 165 return VISIONPRESCRIPTION; 166 if (Configuration.isAcceptInvalidEnums()) 167 return null; 168 else 169 throw new FHIRException("Unknown RequestResourceTypes code '"+codeString+"'"); 170 } 171 public String toCode() { 172 switch (this) { 173 case APPOINTMENT: return "Appointment"; 174 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 175 case CAREPLAN: return "CarePlan"; 176 case CLAIM: return "Claim"; 177 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 178 case COVERAGEELIGIBILITYREQUEST: return "CoverageEligibilityRequest"; 179 case DEVICEREQUEST: return "DeviceRequest"; 180 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 181 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 182 case MEDICATIONREQUEST: return "MedicationRequest"; 183 case NUTRITIONORDER: return "NutritionOrder"; 184 case REQUESTORCHESTRATION: return "RequestOrchestration"; 185 case SERVICEREQUEST: return "ServiceRequest"; 186 case SUPPLYREQUEST: return "SupplyRequest"; 187 case TASK: return "Task"; 188 case TRANSPORT: return "Transport"; 189 case VISIONPRESCRIPTION: return "VisionPrescription"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case APPOINTMENT: return "http://hl7.org/fhir/fhir-types"; 197 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/fhir-types"; 198 case CAREPLAN: return "http://hl7.org/fhir/fhir-types"; 199 case CLAIM: return "http://hl7.org/fhir/fhir-types"; 200 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/fhir-types"; 201 case COVERAGEELIGIBILITYREQUEST: return "http://hl7.org/fhir/fhir-types"; 202 case DEVICEREQUEST: return "http://hl7.org/fhir/fhir-types"; 203 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/fhir-types"; 204 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/fhir-types"; 205 case MEDICATIONREQUEST: return "http://hl7.org/fhir/fhir-types"; 206 case NUTRITIONORDER: return "http://hl7.org/fhir/fhir-types"; 207 case REQUESTORCHESTRATION: return "http://hl7.org/fhir/fhir-types"; 208 case SERVICEREQUEST: return "http://hl7.org/fhir/fhir-types"; 209 case SUPPLYREQUEST: return "http://hl7.org/fhir/fhir-types"; 210 case TASK: return "http://hl7.org/fhir/fhir-types"; 211 case TRANSPORT: return "http://hl7.org/fhir/fhir-types"; 212 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/fhir-types"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getDefinition() { 218 switch (this) { 219 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 220 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 221 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 222 case CLAIM: return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 223 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 224 case COVERAGEELIGIBILITYREQUEST: return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 225 case DEVICEREQUEST: return "Represents a request a device to be provided to a specific patient. The device may be an implantable device to be subsequently implanted, or an external assistive device, such as a walker, to be delivered and subsequently be used."; 226 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 227 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 228 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 229 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 230 case REQUESTORCHESTRATION: return "A set of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 231 case SERVICEREQUEST: return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 232 case SUPPLYREQUEST: return "A record of a non-patient specific request for a medication, substance, device, certain types of biologically derived product, and nutrition product used in the healthcare setting."; 233 case TASK: return "A task to be performed."; 234 case TRANSPORT: return "Record of transport."; 235 case VISIONPRESCRIPTION: return "An authorization for the provision of glasses and/or contact lenses to a patient."; 236 case NULL: return null; 237 default: return "?"; 238 } 239 } 240 public String getDisplay() { 241 switch (this) { 242 case APPOINTMENT: return "Appointment"; 243 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 244 case CAREPLAN: return "CarePlan"; 245 case CLAIM: return "Claim"; 246 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 247 case COVERAGEELIGIBILITYREQUEST: return "CoverageEligibilityRequest"; 248 case DEVICEREQUEST: return "DeviceRequest"; 249 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 250 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 251 case MEDICATIONREQUEST: return "MedicationRequest"; 252 case NUTRITIONORDER: return "NutritionOrder"; 253 case REQUESTORCHESTRATION: return "RequestOrchestration"; 254 case SERVICEREQUEST: return "ServiceRequest"; 255 case SUPPLYREQUEST: return "SupplyRequest"; 256 case TASK: return "Task"; 257 case TRANSPORT: return "Transport"; 258 case VISIONPRESCRIPTION: return "VisionPrescription"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 } 264 265 public static class RequestResourceTypesEnumFactory implements EnumFactory<RequestResourceTypes> { 266 public RequestResourceTypes fromCode(String codeString) throws IllegalArgumentException { 267 if (codeString == null || "".equals(codeString)) 268 if (codeString == null || "".equals(codeString)) 269 return null; 270 if ("Appointment".equals(codeString)) 271 return RequestResourceTypes.APPOINTMENT; 272 if ("AppointmentResponse".equals(codeString)) 273 return RequestResourceTypes.APPOINTMENTRESPONSE; 274 if ("CarePlan".equals(codeString)) 275 return RequestResourceTypes.CAREPLAN; 276 if ("Claim".equals(codeString)) 277 return RequestResourceTypes.CLAIM; 278 if ("CommunicationRequest".equals(codeString)) 279 return RequestResourceTypes.COMMUNICATIONREQUEST; 280 if ("CoverageEligibilityRequest".equals(codeString)) 281 return RequestResourceTypes.COVERAGEELIGIBILITYREQUEST; 282 if ("DeviceRequest".equals(codeString)) 283 return RequestResourceTypes.DEVICEREQUEST; 284 if ("EnrollmentRequest".equals(codeString)) 285 return RequestResourceTypes.ENROLLMENTREQUEST; 286 if ("ImmunizationRecommendation".equals(codeString)) 287 return RequestResourceTypes.IMMUNIZATIONRECOMMENDATION; 288 if ("MedicationRequest".equals(codeString)) 289 return RequestResourceTypes.MEDICATIONREQUEST; 290 if ("NutritionOrder".equals(codeString)) 291 return RequestResourceTypes.NUTRITIONORDER; 292 if ("RequestOrchestration".equals(codeString)) 293 return RequestResourceTypes.REQUESTORCHESTRATION; 294 if ("ServiceRequest".equals(codeString)) 295 return RequestResourceTypes.SERVICEREQUEST; 296 if ("SupplyRequest".equals(codeString)) 297 return RequestResourceTypes.SUPPLYREQUEST; 298 if ("Task".equals(codeString)) 299 return RequestResourceTypes.TASK; 300 if ("Transport".equals(codeString)) 301 return RequestResourceTypes.TRANSPORT; 302 if ("VisionPrescription".equals(codeString)) 303 return RequestResourceTypes.VISIONPRESCRIPTION; 304 throw new IllegalArgumentException("Unknown RequestResourceTypes code '"+codeString+"'"); 305 } 306 public Enumeration<RequestResourceTypes> fromType(PrimitiveType<?> code) throws FHIRException { 307 if (code == null) 308 return null; 309 if (code.isEmpty()) 310 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.NULL, code); 311 String codeString = ((PrimitiveType) code).asStringValue(); 312 if (codeString == null || "".equals(codeString)) 313 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.NULL, code); 314 if ("Appointment".equals(codeString)) 315 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.APPOINTMENT, code); 316 if ("AppointmentResponse".equals(codeString)) 317 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.APPOINTMENTRESPONSE, code); 318 if ("CarePlan".equals(codeString)) 319 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.CAREPLAN, code); 320 if ("Claim".equals(codeString)) 321 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.CLAIM, code); 322 if ("CommunicationRequest".equals(codeString)) 323 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.COMMUNICATIONREQUEST, code); 324 if ("CoverageEligibilityRequest".equals(codeString)) 325 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.COVERAGEELIGIBILITYREQUEST, code); 326 if ("DeviceRequest".equals(codeString)) 327 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.DEVICEREQUEST, code); 328 if ("EnrollmentRequest".equals(codeString)) 329 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.ENROLLMENTREQUEST, code); 330 if ("ImmunizationRecommendation".equals(codeString)) 331 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.IMMUNIZATIONRECOMMENDATION, code); 332 if ("MedicationRequest".equals(codeString)) 333 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.MEDICATIONREQUEST, code); 334 if ("NutritionOrder".equals(codeString)) 335 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.NUTRITIONORDER, code); 336 if ("RequestOrchestration".equals(codeString)) 337 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.REQUESTORCHESTRATION, code); 338 if ("ServiceRequest".equals(codeString)) 339 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.SERVICEREQUEST, code); 340 if ("SupplyRequest".equals(codeString)) 341 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.SUPPLYREQUEST, code); 342 if ("Task".equals(codeString)) 343 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.TASK, code); 344 if ("Transport".equals(codeString)) 345 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.TRANSPORT, code); 346 if ("VisionPrescription".equals(codeString)) 347 return new Enumeration<RequestResourceTypes>(this, RequestResourceTypes.VISIONPRESCRIPTION, code); 348 throw new FHIRException("Unknown RequestResourceTypes code '"+codeString+"'"); 349 } 350 public String toCode(RequestResourceTypes code) { 351 if (code == RequestResourceTypes.NULL) 352 return null; 353 if (code == RequestResourceTypes.APPOINTMENT) 354 return "Appointment"; 355 if (code == RequestResourceTypes.APPOINTMENTRESPONSE) 356 return "AppointmentResponse"; 357 if (code == RequestResourceTypes.CAREPLAN) 358 return "CarePlan"; 359 if (code == RequestResourceTypes.CLAIM) 360 return "Claim"; 361 if (code == RequestResourceTypes.COMMUNICATIONREQUEST) 362 return "CommunicationRequest"; 363 if (code == RequestResourceTypes.COVERAGEELIGIBILITYREQUEST) 364 return "CoverageEligibilityRequest"; 365 if (code == RequestResourceTypes.DEVICEREQUEST) 366 return "DeviceRequest"; 367 if (code == RequestResourceTypes.ENROLLMENTREQUEST) 368 return "EnrollmentRequest"; 369 if (code == RequestResourceTypes.IMMUNIZATIONRECOMMENDATION) 370 return "ImmunizationRecommendation"; 371 if (code == RequestResourceTypes.MEDICATIONREQUEST) 372 return "MedicationRequest"; 373 if (code == RequestResourceTypes.NUTRITIONORDER) 374 return "NutritionOrder"; 375 if (code == RequestResourceTypes.REQUESTORCHESTRATION) 376 return "RequestOrchestration"; 377 if (code == RequestResourceTypes.SERVICEREQUEST) 378 return "ServiceRequest"; 379 if (code == RequestResourceTypes.SUPPLYREQUEST) 380 return "SupplyRequest"; 381 if (code == RequestResourceTypes.TASK) 382 return "Task"; 383 if (code == RequestResourceTypes.TRANSPORT) 384 return "Transport"; 385 if (code == RequestResourceTypes.VISIONPRESCRIPTION) 386 return "VisionPrescription"; 387 return "?"; 388 } 389 public String toSystem(RequestResourceTypes code) { 390 return code.getSystem(); 391 } 392 } 393 394 @Block() 395 public static class ActivityDefinitionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 396 /** 397 * The type of participant in the action. 398 */ 399 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 400 @Description(shortDefinition="careteam | device | group | healthcareservice | location | organization | patient | practitioner | practitionerrole | relatedperson", formalDefinition="The type of participant in the action." ) 401 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-type") 402 protected Enumeration<ActionParticipantType> type; 403 404 /** 405 * The type of participant in the action. 406 */ 407 @Child(name = "typeCanonical", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 408 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 409 protected CanonicalType typeCanonical; 410 411 /** 412 * The type of participant in the action. 413 */ 414 @Child(name = "typeReference", type = {CareTeam.class, Device.class, DeviceDefinition.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 415 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 416 protected Reference typeReference; 417 418 /** 419 * The role the participant should play in performing the described action. 420 */ 421 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 422 @Description(shortDefinition="E.g. Nurse, Surgeon, Parent, etc", formalDefinition="The role the participant should play in performing the described action." ) 423 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/action-participant-role") 424 protected CodeableConcept role; 425 426 /** 427 * Indicates how the actor will be involved in the action - author, reviewer, witness, etc. 428 */ 429 @Child(name = "function", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 430 @Description(shortDefinition="E.g. Author, Reviewer, Witness, etc", formalDefinition="Indicates how the actor will be involved in the action - author, reviewer, witness, etc." ) 431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-function") 432 protected CodeableConcept function; 433 434 private static final long serialVersionUID = 468446682L; 435 436 /** 437 * Constructor 438 */ 439 public ActivityDefinitionParticipantComponent() { 440 super(); 441 } 442 443 /** 444 * @return {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 445 */ 446 public Enumeration<ActionParticipantType> getTypeElement() { 447 if (this.type == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.type"); 450 else if (Configuration.doAutoCreate()) 451 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 452 return this.type; 453 } 454 455 public boolean hasTypeElement() { 456 return this.type != null && !this.type.isEmpty(); 457 } 458 459 public boolean hasType() { 460 return this.type != null && !this.type.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 465 */ 466 public ActivityDefinitionParticipantComponent setTypeElement(Enumeration<ActionParticipantType> value) { 467 this.type = value; 468 return this; 469 } 470 471 /** 472 * @return The type of participant in the action. 473 */ 474 public ActionParticipantType getType() { 475 return this.type == null ? null : this.type.getValue(); 476 } 477 478 /** 479 * @param value The type of participant in the action. 480 */ 481 public ActivityDefinitionParticipantComponent setType(ActionParticipantType value) { 482 if (value == null) 483 this.type = null; 484 else { 485 if (this.type == null) 486 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 487 this.type.setValue(value); 488 } 489 return this; 490 } 491 492 /** 493 * @return {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 494 */ 495 public CanonicalType getTypeCanonicalElement() { 496 if (this.typeCanonical == null) 497 if (Configuration.errorOnAutoCreate()) 498 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.typeCanonical"); 499 else if (Configuration.doAutoCreate()) 500 this.typeCanonical = new CanonicalType(); // bb 501 return this.typeCanonical; 502 } 503 504 public boolean hasTypeCanonicalElement() { 505 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 506 } 507 508 public boolean hasTypeCanonical() { 509 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 510 } 511 512 /** 513 * @param value {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 514 */ 515 public ActivityDefinitionParticipantComponent setTypeCanonicalElement(CanonicalType value) { 516 this.typeCanonical = value; 517 return this; 518 } 519 520 /** 521 * @return The type of participant in the action. 522 */ 523 public String getTypeCanonical() { 524 return this.typeCanonical == null ? null : this.typeCanonical.getValue(); 525 } 526 527 /** 528 * @param value The type of participant in the action. 529 */ 530 public ActivityDefinitionParticipantComponent setTypeCanonical(String value) { 531 if (Utilities.noString(value)) 532 this.typeCanonical = null; 533 else { 534 if (this.typeCanonical == null) 535 this.typeCanonical = new CanonicalType(); 536 this.typeCanonical.setValue(value); 537 } 538 return this; 539 } 540 541 /** 542 * @return {@link #typeReference} (The type of participant in the action.) 543 */ 544 public Reference getTypeReference() { 545 if (this.typeReference == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.typeReference"); 548 else if (Configuration.doAutoCreate()) 549 this.typeReference = new Reference(); // cc 550 return this.typeReference; 551 } 552 553 public boolean hasTypeReference() { 554 return this.typeReference != null && !this.typeReference.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #typeReference} (The type of participant in the action.) 559 */ 560 public ActivityDefinitionParticipantComponent setTypeReference(Reference value) { 561 this.typeReference = value; 562 return this; 563 } 564 565 /** 566 * @return {@link #role} (The role the participant should play in performing the described action.) 567 */ 568 public CodeableConcept getRole() { 569 if (this.role == null) 570 if (Configuration.errorOnAutoCreate()) 571 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.role"); 572 else if (Configuration.doAutoCreate()) 573 this.role = new CodeableConcept(); // cc 574 return this.role; 575 } 576 577 public boolean hasRole() { 578 return this.role != null && !this.role.isEmpty(); 579 } 580 581 /** 582 * @param value {@link #role} (The role the participant should play in performing the described action.) 583 */ 584 public ActivityDefinitionParticipantComponent setRole(CodeableConcept value) { 585 this.role = value; 586 return this; 587 } 588 589 /** 590 * @return {@link #function} (Indicates how the actor will be involved in the action - author, reviewer, witness, etc.) 591 */ 592 public CodeableConcept getFunction() { 593 if (this.function == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.function"); 596 else if (Configuration.doAutoCreate()) 597 this.function = new CodeableConcept(); // cc 598 return this.function; 599 } 600 601 public boolean hasFunction() { 602 return this.function != null && !this.function.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #function} (Indicates how the actor will be involved in the action - author, reviewer, witness, etc.) 607 */ 608 public ActivityDefinitionParticipantComponent setFunction(CodeableConcept value) { 609 this.function = value; 610 return this; 611 } 612 613 protected void listChildren(List<Property> children) { 614 super.listChildren(children); 615 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 616 children.add(new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical)); 617 children.add(new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference)); 618 children.add(new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role)); 619 children.add(new Property("function", "CodeableConcept", "Indicates how the actor will be involved in the action - author, reviewer, witness, etc.", 0, 1, function)); 620 } 621 622 @Override 623 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 624 switch (_hash) { 625 case 3575610: /*type*/ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 626 case -466635046: /*typeCanonical*/ return new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical); 627 case 2074825009: /*typeReference*/ return new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference); 628 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role); 629 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Indicates how the actor will be involved in the action - author, reviewer, witness, etc.", 0, 1, function); 630 default: return super.getNamedProperty(_hash, _name, _checkValid); 631 } 632 633 } 634 635 @Override 636 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 637 switch (hash) { 638 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ActionParticipantType> 639 case -466635046: /*typeCanonical*/ return this.typeCanonical == null ? new Base[0] : new Base[] {this.typeCanonical}; // CanonicalType 640 case 2074825009: /*typeReference*/ return this.typeReference == null ? new Base[0] : new Base[] {this.typeReference}; // Reference 641 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 642 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 643 default: return super.getProperty(hash, name, checkValid); 644 } 645 646 } 647 648 @Override 649 public Base setProperty(int hash, String name, Base value) throws FHIRException { 650 switch (hash) { 651 case 3575610: // type 652 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 653 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 654 return value; 655 case -466635046: // typeCanonical 656 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 657 return value; 658 case 2074825009: // typeReference 659 this.typeReference = TypeConvertor.castToReference(value); // Reference 660 return value; 661 case 3506294: // role 662 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 663 return value; 664 case 1380938712: // function 665 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 666 return value; 667 default: return super.setProperty(hash, name, value); 668 } 669 670 } 671 672 @Override 673 public Base setProperty(String name, Base value) throws FHIRException { 674 if (name.equals("type")) { 675 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 676 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 677 } else if (name.equals("typeCanonical")) { 678 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 679 } else if (name.equals("typeReference")) { 680 this.typeReference = TypeConvertor.castToReference(value); // Reference 681 } else if (name.equals("role")) { 682 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 683 } else if (name.equals("function")) { 684 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 685 } else 686 return super.setProperty(name, value); 687 return value; 688 } 689 690 @Override 691 public void removeChild(String name, Base value) throws FHIRException { 692 if (name.equals("type")) { 693 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 694 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 695 } else if (name.equals("typeCanonical")) { 696 this.typeCanonical = null; 697 } else if (name.equals("typeReference")) { 698 this.typeReference = null; 699 } else if (name.equals("role")) { 700 this.role = null; 701 } else if (name.equals("function")) { 702 this.function = null; 703 } else 704 super.removeChild(name, value); 705 706 } 707 708 @Override 709 public Base makeProperty(int hash, String name) throws FHIRException { 710 switch (hash) { 711 case 3575610: return getTypeElement(); 712 case -466635046: return getTypeCanonicalElement(); 713 case 2074825009: return getTypeReference(); 714 case 3506294: return getRole(); 715 case 1380938712: return getFunction(); 716 default: return super.makeProperty(hash, name); 717 } 718 719 } 720 721 @Override 722 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 723 switch (hash) { 724 case 3575610: /*type*/ return new String[] {"code"}; 725 case -466635046: /*typeCanonical*/ return new String[] {"canonical"}; 726 case 2074825009: /*typeReference*/ return new String[] {"Reference"}; 727 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 728 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 729 default: return super.getTypesForProperty(hash, name); 730 } 731 732 } 733 734 @Override 735 public Base addChild(String name) throws FHIRException { 736 if (name.equals("type")) { 737 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.participant.type"); 738 } 739 else if (name.equals("typeCanonical")) { 740 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.participant.typeCanonical"); 741 } 742 else if (name.equals("typeReference")) { 743 this.typeReference = new Reference(); 744 return this.typeReference; 745 } 746 else if (name.equals("role")) { 747 this.role = new CodeableConcept(); 748 return this.role; 749 } 750 else if (name.equals("function")) { 751 this.function = new CodeableConcept(); 752 return this.function; 753 } 754 else 755 return super.addChild(name); 756 } 757 758 public ActivityDefinitionParticipantComponent copy() { 759 ActivityDefinitionParticipantComponent dst = new ActivityDefinitionParticipantComponent(); 760 copyValues(dst); 761 return dst; 762 } 763 764 public void copyValues(ActivityDefinitionParticipantComponent dst) { 765 super.copyValues(dst); 766 dst.type = type == null ? null : type.copy(); 767 dst.typeCanonical = typeCanonical == null ? null : typeCanonical.copy(); 768 dst.typeReference = typeReference == null ? null : typeReference.copy(); 769 dst.role = role == null ? null : role.copy(); 770 dst.function = function == null ? null : function.copy(); 771 } 772 773 @Override 774 public boolean equalsDeep(Base other_) { 775 if (!super.equalsDeep(other_)) 776 return false; 777 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 778 return false; 779 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 780 return compareDeep(type, o.type, true) && compareDeep(typeCanonical, o.typeCanonical, true) && compareDeep(typeReference, o.typeReference, true) 781 && compareDeep(role, o.role, true) && compareDeep(function, o.function, true); 782 } 783 784 @Override 785 public boolean equalsShallow(Base other_) { 786 if (!super.equalsShallow(other_)) 787 return false; 788 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 789 return false; 790 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 791 return compareValues(type, o.type, true) && compareValues(typeCanonical, o.typeCanonical, true); 792 } 793 794 public boolean isEmpty() { 795 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, typeCanonical, typeReference 796 , role, function); 797 } 798 799 public String fhirType() { 800 return "ActivityDefinition.participant"; 801 802 } 803 804 } 805 806 @Block() 807 public static class ActivityDefinitionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 808 /** 809 * The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 810 */ 811 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 812 @Description(shortDefinition="The path to the element to be set dynamically", formalDefinition="The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details)." ) 813 protected StringType path; 814 815 /** 816 * An expression specifying the value of the customized element. 817 */ 818 @Child(name = "expression", type = {Expression.class}, order=2, min=1, max=1, modifier=false, summary=false) 819 @Description(shortDefinition="An expression that provides the dynamic value for the customization", formalDefinition="An expression specifying the value of the customized element." ) 820 protected Expression expression; 821 822 private static final long serialVersionUID = 1064529082L; 823 824 /** 825 * Constructor 826 */ 827 public ActivityDefinitionDynamicValueComponent() { 828 super(); 829 } 830 831 /** 832 * Constructor 833 */ 834 public ActivityDefinitionDynamicValueComponent(String path, Expression expression) { 835 super(); 836 this.setPath(path); 837 this.setExpression(expression); 838 } 839 840 /** 841 * @return {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 842 */ 843 public StringType getPathElement() { 844 if (this.path == null) 845 if (Configuration.errorOnAutoCreate()) 846 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.path"); 847 else if (Configuration.doAutoCreate()) 848 this.path = new StringType(); // bb 849 return this.path; 850 } 851 852 public boolean hasPathElement() { 853 return this.path != null && !this.path.isEmpty(); 854 } 855 856 public boolean hasPath() { 857 return this.path != null && !this.path.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 862 */ 863 public ActivityDefinitionDynamicValueComponent setPathElement(StringType value) { 864 this.path = value; 865 return this; 866 } 867 868 /** 869 * @return The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 870 */ 871 public String getPath() { 872 return this.path == null ? null : this.path.getValue(); 873 } 874 875 /** 876 * @param value The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 877 */ 878 public ActivityDefinitionDynamicValueComponent setPath(String value) { 879 if (this.path == null) 880 this.path = new StringType(); 881 this.path.setValue(value); 882 return this; 883 } 884 885 /** 886 * @return {@link #expression} (An expression specifying the value of the customized element.) 887 */ 888 public Expression getExpression() { 889 if (this.expression == null) 890 if (Configuration.errorOnAutoCreate()) 891 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.expression"); 892 else if (Configuration.doAutoCreate()) 893 this.expression = new Expression(); // cc 894 return this.expression; 895 } 896 897 public boolean hasExpression() { 898 return this.expression != null && !this.expression.isEmpty(); 899 } 900 901 /** 902 * @param value {@link #expression} (An expression specifying the value of the customized element.) 903 */ 904 public ActivityDefinitionDynamicValueComponent setExpression(Expression value) { 905 this.expression = value; 906 return this; 907 } 908 909 protected void listChildren(List<Property> children) { 910 super.listChildren(children); 911 children.add(new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, 1, path)); 912 children.add(new Property("expression", "Expression", "An expression specifying the value of the customized element.", 0, 1, expression)); 913 } 914 915 @Override 916 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 917 switch (_hash) { 918 case 3433509: /*path*/ return new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, 1, path); 919 case -1795452264: /*expression*/ return new Property("expression", "Expression", "An expression specifying the value of the customized element.", 0, 1, expression); 920 default: return super.getNamedProperty(_hash, _name, _checkValid); 921 } 922 923 } 924 925 @Override 926 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 927 switch (hash) { 928 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 929 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 930 default: return super.getProperty(hash, name, checkValid); 931 } 932 933 } 934 935 @Override 936 public Base setProperty(int hash, String name, Base value) throws FHIRException { 937 switch (hash) { 938 case 3433509: // path 939 this.path = TypeConvertor.castToString(value); // StringType 940 return value; 941 case -1795452264: // expression 942 this.expression = TypeConvertor.castToExpression(value); // Expression 943 return value; 944 default: return super.setProperty(hash, name, value); 945 } 946 947 } 948 949 @Override 950 public Base setProperty(String name, Base value) throws FHIRException { 951 if (name.equals("path")) { 952 this.path = TypeConvertor.castToString(value); // StringType 953 } else if (name.equals("expression")) { 954 this.expression = TypeConvertor.castToExpression(value); // Expression 955 } else 956 return super.setProperty(name, value); 957 return value; 958 } 959 960 @Override 961 public void removeChild(String name, Base value) throws FHIRException { 962 if (name.equals("path")) { 963 this.path = null; 964 } else if (name.equals("expression")) { 965 this.expression = null; 966 } else 967 super.removeChild(name, value); 968 969 } 970 971 @Override 972 public Base makeProperty(int hash, String name) throws FHIRException { 973 switch (hash) { 974 case 3433509: return getPathElement(); 975 case -1795452264: return getExpression(); 976 default: return super.makeProperty(hash, name); 977 } 978 979 } 980 981 @Override 982 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 983 switch (hash) { 984 case 3433509: /*path*/ return new String[] {"string"}; 985 case -1795452264: /*expression*/ return new String[] {"Expression"}; 986 default: return super.getTypesForProperty(hash, name); 987 } 988 989 } 990 991 @Override 992 public Base addChild(String name) throws FHIRException { 993 if (name.equals("path")) { 994 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.dynamicValue.path"); 995 } 996 else if (name.equals("expression")) { 997 this.expression = new Expression(); 998 return this.expression; 999 } 1000 else 1001 return super.addChild(name); 1002 } 1003 1004 public ActivityDefinitionDynamicValueComponent copy() { 1005 ActivityDefinitionDynamicValueComponent dst = new ActivityDefinitionDynamicValueComponent(); 1006 copyValues(dst); 1007 return dst; 1008 } 1009 1010 public void copyValues(ActivityDefinitionDynamicValueComponent dst) { 1011 super.copyValues(dst); 1012 dst.path = path == null ? null : path.copy(); 1013 dst.expression = expression == null ? null : expression.copy(); 1014 } 1015 1016 @Override 1017 public boolean equalsDeep(Base other_) { 1018 if (!super.equalsDeep(other_)) 1019 return false; 1020 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 1021 return false; 1022 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 1023 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 1024 } 1025 1026 @Override 1027 public boolean equalsShallow(Base other_) { 1028 if (!super.equalsShallow(other_)) 1029 return false; 1030 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 1031 return false; 1032 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 1033 return compareValues(path, o.path, true); 1034 } 1035 1036 public boolean isEmpty() { 1037 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 1038 } 1039 1040 public String fhirType() { 1041 return "ActivityDefinition.dynamicValue"; 1042 1043 } 1044 1045 } 1046 1047 /** 1048 * An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers. 1049 */ 1050 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1051 @Description(shortDefinition="Canonical identifier for this activity definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers." ) 1052 protected UriType url; 1053 1054 /** 1055 * A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1056 */ 1057 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1058 @Description(shortDefinition="Additional identifier for the activity definition", formalDefinition="A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1059 protected List<Identifier> identifier; 1060 1061 /** 1062 * The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 1063 */ 1064 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1065 @Description(shortDefinition="Business version of the activity definition", formalDefinition="The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets." ) 1066 protected StringType version; 1067 1068 /** 1069 * Indicates the mechanism used to compare versions to determine which is more current. 1070 */ 1071 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1072 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1074 protected DataType versionAlgorithm; 1075 1076 /** 1077 * A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1078 */ 1079 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1080 @Description(shortDefinition="Name for this activity definition (computer friendly)", formalDefinition="A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1081 protected StringType name; 1082 1083 /** 1084 * A short, descriptive, user-friendly title for the activity definition. 1085 */ 1086 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1087 @Description(shortDefinition="Name for this activity definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the activity definition." ) 1088 protected StringType title; 1089 1090 /** 1091 * An explanatory or alternate title for the activity definition giving additional information about its content. 1092 */ 1093 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1094 @Description(shortDefinition="Subordinate title of the activity definition", formalDefinition="An explanatory or alternate title for the activity definition giving additional information about its content." ) 1095 protected StringType subtitle; 1096 1097 /** 1098 * The status of this activity definition. Enables tracking the life-cycle of the content. 1099 */ 1100 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 1101 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this activity definition. Enables tracking the life-cycle of the content." ) 1102 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1103 protected Enumeration<PublicationStatus> status; 1104 1105 /** 1106 * A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1107 */ 1108 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1109 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1110 protected BooleanType experimental; 1111 1112 /** 1113 * A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource. 1114 */ 1115 @Child(name = "subject", type = {CodeableConcept.class, Group.class, MedicinalProductDefinition.class, SubstanceDefinition.class, AdministrableProductDefinition.class, ManufacturedItemDefinition.class, PackagedProductDefinition.class, CanonicalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1116 @Description(shortDefinition="Type of individual the activity definition is intended for", formalDefinition="A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource." ) 1117 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 1118 protected DataType subject; 1119 1120 /** 1121 * The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes. 1122 */ 1123 @Child(name = "date", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1124 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes." ) 1125 protected DateTimeType date; 1126 1127 /** 1128 * The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition. 1129 */ 1130 @Child(name = "publisher", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1131 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition." ) 1132 protected StringType publisher; 1133 1134 /** 1135 * Contact details to assist a user in finding and communicating with the publisher. 1136 */ 1137 @Child(name = "contact", type = {ContactDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1138 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1139 protected List<ContactDetail> contact; 1140 1141 /** 1142 * A free text natural language description of the activity definition from a consumer's perspective. 1143 */ 1144 @Child(name = "description", type = {MarkdownType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1145 @Description(shortDefinition="Natural language description of the activity definition", formalDefinition="A free text natural language description of the activity definition from a consumer's perspective." ) 1146 protected MarkdownType description; 1147 1148 /** 1149 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances. 1150 */ 1151 @Child(name = "useContext", type = {UsageContext.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1152 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances." ) 1153 protected List<UsageContext> useContext; 1154 1155 /** 1156 * A legal or geographic region in which the activity definition is intended to be used. 1157 */ 1158 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1159 @Description(shortDefinition="Intended jurisdiction for activity definition (if applicable)", formalDefinition="A legal or geographic region in which the activity definition is intended to be used." ) 1160 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1161 protected List<CodeableConcept> jurisdiction; 1162 1163 /** 1164 * Explanation of why this activity definition is needed and why it has been designed as it has. 1165 */ 1166 @Child(name = "purpose", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1167 @Description(shortDefinition="Why this activity definition is defined", formalDefinition="Explanation of why this activity definition is needed and why it has been designed as it has." ) 1168 protected MarkdownType purpose; 1169 1170 /** 1171 * A detailed description of how the activity definition is used from a clinical perspective. 1172 */ 1173 @Child(name = "usage", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1174 @Description(shortDefinition="Describes the clinical usage of the activity definition", formalDefinition="A detailed description of how the activity definition is used from a clinical perspective." ) 1175 protected MarkdownType usage; 1176 1177 /** 1178 * A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition. 1179 */ 1180 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 1181 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition." ) 1182 protected MarkdownType copyright; 1183 1184 /** 1185 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1186 */ 1187 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1188 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1189 protected StringType copyrightLabel; 1190 1191 /** 1192 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1193 */ 1194 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 1195 @Description(shortDefinition="When the activity definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1196 protected DateType approvalDate; 1197 1198 /** 1199 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1200 */ 1201 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1202 @Description(shortDefinition="When the activity definition was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 1203 protected DateType lastReviewDate; 1204 1205 /** 1206 * The period during which the activity definition content was or is planned to be in active use. 1207 */ 1208 @Child(name = "effectivePeriod", type = {Period.class}, order=22, min=0, max=1, modifier=false, summary=true) 1209 @Description(shortDefinition="When the activity definition is expected to be used", formalDefinition="The period during which the activity definition content was or is planned to be in active use." ) 1210 protected Period effectivePeriod; 1211 1212 /** 1213 * Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching. 1214 */ 1215 @Child(name = "topic", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1216 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching." ) 1217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 1218 protected List<CodeableConcept> topic; 1219 1220 /** 1221 * An individiual or organization primarily involved in the creation and maintenance of the content. 1222 */ 1223 @Child(name = "author", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1224 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 1225 protected List<ContactDetail> author; 1226 1227 /** 1228 * An individual or organization primarily responsible for internal coherence of the content. 1229 */ 1230 @Child(name = "editor", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1231 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 1232 protected List<ContactDetail> editor; 1233 1234 /** 1235 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 1236 */ 1237 @Child(name = "reviewer", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1238 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 1239 protected List<ContactDetail> reviewer; 1240 1241 /** 1242 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 1243 */ 1244 @Child(name = "endorser", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1245 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 1246 protected List<ContactDetail> endorser; 1247 1248 /** 1249 * Related artifacts such as additional documentation, justification, or bibliographic references. 1250 */ 1251 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1252 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 1253 protected List<RelatedArtifact> relatedArtifact; 1254 1255 /** 1256 * A reference to a Library resource containing any formal logic used by the activity definition. 1257 */ 1258 @Child(name = "library", type = {CanonicalType.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1259 @Description(shortDefinition="Logic used by the activity definition", formalDefinition="A reference to a Library resource containing any formal logic used by the activity definition." ) 1260 protected List<CanonicalType> library; 1261 1262 /** 1263 * A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. 1264 */ 1265 @Child(name = "kind", type = {CodeType.class}, order=30, min=0, max=1, modifier=false, summary=true) 1266 @Description(shortDefinition="Kind of resource", formalDefinition="A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest." ) 1267 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-resource-types") 1268 protected Enumeration<RequestResourceTypes> kind; 1269 1270 /** 1271 * A profile to which the target of the activity definition is expected to conform. 1272 */ 1273 @Child(name = "profile", type = {CanonicalType.class}, order=31, min=0, max=1, modifier=false, summary=false) 1274 @Description(shortDefinition="What profile the resource needs to conform to", formalDefinition="A profile to which the target of the activity definition is expected to conform." ) 1275 protected CanonicalType profile; 1276 1277 /** 1278 * Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter. 1279 */ 1280 @Child(name = "code", type = {CodeableConcept.class}, order=32, min=0, max=1, modifier=false, summary=true) 1281 @Description(shortDefinition="Detail type of activity", formalDefinition="Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter." ) 1282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 1283 protected CodeableConcept code; 1284 1285 /** 1286 * Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain. 1287 */ 1288 @Child(name = "intent", type = {CodeType.class}, order=33, min=0, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain." ) 1290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 1291 protected Enumeration<RequestIntent> intent; 1292 1293 /** 1294 * Indicates how quickly the activity should be addressed with respect to other requests. 1295 */ 1296 @Child(name = "priority", type = {CodeType.class}, order=34, min=0, max=1, modifier=false, summary=false) 1297 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the activity should be addressed with respect to other requests." ) 1298 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 1299 protected Enumeration<RequestPriority> priority; 1300 1301 /** 1302 * Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action. 1303 */ 1304 @Child(name = "doNotPerform", type = {BooleanType.class}, order=35, min=0, max=1, modifier=true, summary=true) 1305 @Description(shortDefinition="True if the activity should not be performed", formalDefinition="Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action." ) 1306 protected BooleanType doNotPerform; 1307 1308 /** 1309 * The timing or frequency upon which the described activity is to occur. 1310 */ 1311 @Child(name = "timing", type = {Timing.class, Age.class, Range.class, Duration.class}, order=36, min=0, max=1, modifier=false, summary=false) 1312 @Description(shortDefinition="When activity is to occur", formalDefinition="The timing or frequency upon which the described activity is to occur." ) 1313 protected DataType timing; 1314 1315 /** 1316 * If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc. 1317 */ 1318 @Child(name = "asNeeded", type = {BooleanType.class, CodeableConcept.class}, order=37, min=0, max=1, modifier=false, summary=true) 1319 @Description(shortDefinition="Preconditions for service", formalDefinition="If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc." ) 1320 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 1321 protected DataType asNeeded; 1322 1323 /** 1324 * Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc. 1325 */ 1326 @Child(name = "location", type = {CodeableReference.class}, order=38, min=0, max=1, modifier=false, summary=false) 1327 @Description(shortDefinition="Where it should happen", formalDefinition="Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc." ) 1328 protected CodeableReference location; 1329 1330 /** 1331 * Indicates who should participate in performing the action described. 1332 */ 1333 @Child(name = "participant", type = {}, order=39, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1334 @Description(shortDefinition="Who should participate in the action", formalDefinition="Indicates who should participate in performing the action described." ) 1335 protected List<ActivityDefinitionParticipantComponent> participant; 1336 1337 /** 1338 * Identifies the food, drug or other product being consumed or supplied in the activity. 1339 */ 1340 @Child(name = "product", type = {Medication.class, Ingredient.class, Substance.class, SubstanceDefinition.class, CodeableConcept.class}, order=40, min=0, max=1, modifier=false, summary=false) 1341 @Description(shortDefinition="What's administered/supplied", formalDefinition="Identifies the food, drug or other product being consumed or supplied in the activity." ) 1342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 1343 protected DataType product; 1344 1345 /** 1346 * Identifies the quantity expected to be consumed at once (per dose, per meal, etc.). 1347 */ 1348 @Child(name = "quantity", type = {Quantity.class}, order=41, min=0, max=1, modifier=false, summary=false) 1349 @Description(shortDefinition="How much is administered/consumed/supplied", formalDefinition="Identifies the quantity expected to be consumed at once (per dose, per meal, etc.)." ) 1350 protected Quantity quantity; 1351 1352 /** 1353 * Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources. 1354 */ 1355 @Child(name = "dosage", type = {Dosage.class}, order=42, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1356 @Description(shortDefinition="Detailed dosage instructions", formalDefinition="Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources." ) 1357 protected List<Dosage> dosage; 1358 1359 /** 1360 * Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites). 1361 */ 1362 @Child(name = "bodySite", type = {CodeableConcept.class}, order=43, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1363 @Description(shortDefinition="What part of body to perform on", formalDefinition="Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites)." ) 1364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1365 protected List<CodeableConcept> bodySite; 1366 1367 /** 1368 * Defines specimen requirements for the action to be performed, such as required specimens for a lab test. 1369 */ 1370 @Child(name = "specimenRequirement", type = {CanonicalType.class}, order=44, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1371 @Description(shortDefinition="What specimens are required to perform this action", formalDefinition="Defines specimen requirements for the action to be performed, such as required specimens for a lab test." ) 1372 protected List<CanonicalType> specimenRequirement; 1373 1374 /** 1375 * Defines observation requirements for the action to be performed, such as body weight or surface area. 1376 */ 1377 @Child(name = "observationRequirement", type = {CanonicalType.class}, order=45, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1378 @Description(shortDefinition="What observations are required to perform this action", formalDefinition="Defines observation requirements for the action to be performed, such as body weight or surface area." ) 1379 protected List<CanonicalType> observationRequirement; 1380 1381 /** 1382 * Defines the observations that are expected to be produced by the action. 1383 */ 1384 @Child(name = "observationResultRequirement", type = {CanonicalType.class}, order=46, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1385 @Description(shortDefinition="What observations must be produced by this action", formalDefinition="Defines the observations that are expected to be produced by the action." ) 1386 protected List<CanonicalType> observationResultRequirement; 1387 1388 /** 1389 * A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 1390 */ 1391 @Child(name = "transform", type = {CanonicalType.class}, order=47, min=0, max=1, modifier=false, summary=false) 1392 @Description(shortDefinition="Transform to apply the template", formalDefinition="A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input." ) 1393 protected CanonicalType transform; 1394 1395 /** 1396 * Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result. 1397 */ 1398 @Child(name = "dynamicValue", type = {}, order=48, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1399 @Description(shortDefinition="Dynamic aspects of the definition", formalDefinition="Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result." ) 1400 protected List<ActivityDefinitionDynamicValueComponent> dynamicValue; 1401 1402 private static final long serialVersionUID = -1134510373L; 1403 1404 /** 1405 * Constructor 1406 */ 1407 public ActivityDefinition() { 1408 super(); 1409 } 1410 1411 /** 1412 * Constructor 1413 */ 1414 public ActivityDefinition(PublicationStatus status) { 1415 super(); 1416 this.setStatus(status); 1417 } 1418 1419 /** 1420 * @return {@link #url} (An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1421 */ 1422 public UriType getUrlElement() { 1423 if (this.url == null) 1424 if (Configuration.errorOnAutoCreate()) 1425 throw new Error("Attempt to auto-create ActivityDefinition.url"); 1426 else if (Configuration.doAutoCreate()) 1427 this.url = new UriType(); // bb 1428 return this.url; 1429 } 1430 1431 public boolean hasUrlElement() { 1432 return this.url != null && !this.url.isEmpty(); 1433 } 1434 1435 public boolean hasUrl() { 1436 return this.url != null && !this.url.isEmpty(); 1437 } 1438 1439 /** 1440 * @param value {@link #url} (An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1441 */ 1442 public ActivityDefinition setUrlElement(UriType value) { 1443 this.url = value; 1444 return this; 1445 } 1446 1447 /** 1448 * @return An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers. 1449 */ 1450 public String getUrl() { 1451 return this.url == null ? null : this.url.getValue(); 1452 } 1453 1454 /** 1455 * @param value An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers. 1456 */ 1457 public ActivityDefinition setUrl(String value) { 1458 if (Utilities.noString(value)) 1459 this.url = null; 1460 else { 1461 if (this.url == null) 1462 this.url = new UriType(); 1463 this.url.setValue(value); 1464 } 1465 return this; 1466 } 1467 1468 /** 1469 * @return {@link #identifier} (A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1470 */ 1471 public List<Identifier> getIdentifier() { 1472 if (this.identifier == null) 1473 this.identifier = new ArrayList<Identifier>(); 1474 return this.identifier; 1475 } 1476 1477 /** 1478 * @return Returns a reference to <code>this</code> for easy method chaining 1479 */ 1480 public ActivityDefinition setIdentifier(List<Identifier> theIdentifier) { 1481 this.identifier = theIdentifier; 1482 return this; 1483 } 1484 1485 public boolean hasIdentifier() { 1486 if (this.identifier == null) 1487 return false; 1488 for (Identifier item : this.identifier) 1489 if (!item.isEmpty()) 1490 return true; 1491 return false; 1492 } 1493 1494 public Identifier addIdentifier() { //3 1495 Identifier t = new Identifier(); 1496 if (this.identifier == null) 1497 this.identifier = new ArrayList<Identifier>(); 1498 this.identifier.add(t); 1499 return t; 1500 } 1501 1502 public ActivityDefinition addIdentifier(Identifier t) { //3 1503 if (t == null) 1504 return this; 1505 if (this.identifier == null) 1506 this.identifier = new ArrayList<Identifier>(); 1507 this.identifier.add(t); 1508 return this; 1509 } 1510 1511 /** 1512 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1513 */ 1514 public Identifier getIdentifierFirstRep() { 1515 if (getIdentifier().isEmpty()) { 1516 addIdentifier(); 1517 } 1518 return getIdentifier().get(0); 1519 } 1520 1521 /** 1522 * @return {@link #version} (The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1523 */ 1524 public StringType getVersionElement() { 1525 if (this.version == null) 1526 if (Configuration.errorOnAutoCreate()) 1527 throw new Error("Attempt to auto-create ActivityDefinition.version"); 1528 else if (Configuration.doAutoCreate()) 1529 this.version = new StringType(); // bb 1530 return this.version; 1531 } 1532 1533 public boolean hasVersionElement() { 1534 return this.version != null && !this.version.isEmpty(); 1535 } 1536 1537 public boolean hasVersion() { 1538 return this.version != null && !this.version.isEmpty(); 1539 } 1540 1541 /** 1542 * @param value {@link #version} (The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1543 */ 1544 public ActivityDefinition setVersionElement(StringType value) { 1545 this.version = value; 1546 return this; 1547 } 1548 1549 /** 1550 * @return The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 1551 */ 1552 public String getVersion() { 1553 return this.version == null ? null : this.version.getValue(); 1554 } 1555 1556 /** 1557 * @param value The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 1558 */ 1559 public ActivityDefinition setVersion(String value) { 1560 if (Utilities.noString(value)) 1561 this.version = null; 1562 else { 1563 if (this.version == null) 1564 this.version = new StringType(); 1565 this.version.setValue(value); 1566 } 1567 return this; 1568 } 1569 1570 /** 1571 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1572 */ 1573 public DataType getVersionAlgorithm() { 1574 return this.versionAlgorithm; 1575 } 1576 1577 /** 1578 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1579 */ 1580 public StringType getVersionAlgorithmStringType() throws FHIRException { 1581 if (this.versionAlgorithm == null) 1582 this.versionAlgorithm = new StringType(); 1583 if (!(this.versionAlgorithm instanceof StringType)) 1584 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1585 return (StringType) this.versionAlgorithm; 1586 } 1587 1588 public boolean hasVersionAlgorithmStringType() { 1589 return this.versionAlgorithm instanceof StringType; 1590 } 1591 1592 /** 1593 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1594 */ 1595 public Coding getVersionAlgorithmCoding() throws FHIRException { 1596 if (this.versionAlgorithm == null) 1597 this.versionAlgorithm = new Coding(); 1598 if (!(this.versionAlgorithm instanceof Coding)) 1599 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1600 return (Coding) this.versionAlgorithm; 1601 } 1602 1603 public boolean hasVersionAlgorithmCoding() { 1604 return this.versionAlgorithm instanceof Coding; 1605 } 1606 1607 public boolean hasVersionAlgorithm() { 1608 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1609 } 1610 1611 /** 1612 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1613 */ 1614 public ActivityDefinition setVersionAlgorithm(DataType value) { 1615 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1616 throw new FHIRException("Not the right type for ActivityDefinition.versionAlgorithm[x]: "+value.fhirType()); 1617 this.versionAlgorithm = value; 1618 return this; 1619 } 1620 1621 /** 1622 * @return {@link #name} (A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1623 */ 1624 public StringType getNameElement() { 1625 if (this.name == null) 1626 if (Configuration.errorOnAutoCreate()) 1627 throw new Error("Attempt to auto-create ActivityDefinition.name"); 1628 else if (Configuration.doAutoCreate()) 1629 this.name = new StringType(); // bb 1630 return this.name; 1631 } 1632 1633 public boolean hasNameElement() { 1634 return this.name != null && !this.name.isEmpty(); 1635 } 1636 1637 public boolean hasName() { 1638 return this.name != null && !this.name.isEmpty(); 1639 } 1640 1641 /** 1642 * @param value {@link #name} (A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1643 */ 1644 public ActivityDefinition setNameElement(StringType value) { 1645 this.name = value; 1646 return this; 1647 } 1648 1649 /** 1650 * @return A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1651 */ 1652 public String getName() { 1653 return this.name == null ? null : this.name.getValue(); 1654 } 1655 1656 /** 1657 * @param value A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1658 */ 1659 public ActivityDefinition setName(String value) { 1660 if (Utilities.noString(value)) 1661 this.name = null; 1662 else { 1663 if (this.name == null) 1664 this.name = new StringType(); 1665 this.name.setValue(value); 1666 } 1667 return this; 1668 } 1669 1670 /** 1671 * @return {@link #title} (A short, descriptive, user-friendly title for the activity definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1672 */ 1673 public StringType getTitleElement() { 1674 if (this.title == null) 1675 if (Configuration.errorOnAutoCreate()) 1676 throw new Error("Attempt to auto-create ActivityDefinition.title"); 1677 else if (Configuration.doAutoCreate()) 1678 this.title = new StringType(); // bb 1679 return this.title; 1680 } 1681 1682 public boolean hasTitleElement() { 1683 return this.title != null && !this.title.isEmpty(); 1684 } 1685 1686 public boolean hasTitle() { 1687 return this.title != null && !this.title.isEmpty(); 1688 } 1689 1690 /** 1691 * @param value {@link #title} (A short, descriptive, user-friendly title for the activity definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1692 */ 1693 public ActivityDefinition setTitleElement(StringType value) { 1694 this.title = value; 1695 return this; 1696 } 1697 1698 /** 1699 * @return A short, descriptive, user-friendly title for the activity definition. 1700 */ 1701 public String getTitle() { 1702 return this.title == null ? null : this.title.getValue(); 1703 } 1704 1705 /** 1706 * @param value A short, descriptive, user-friendly title for the activity definition. 1707 */ 1708 public ActivityDefinition setTitle(String value) { 1709 if (Utilities.noString(value)) 1710 this.title = null; 1711 else { 1712 if (this.title == null) 1713 this.title = new StringType(); 1714 this.title.setValue(value); 1715 } 1716 return this; 1717 } 1718 1719 /** 1720 * @return {@link #subtitle} (An explanatory or alternate title for the activity definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 1721 */ 1722 public StringType getSubtitleElement() { 1723 if (this.subtitle == null) 1724 if (Configuration.errorOnAutoCreate()) 1725 throw new Error("Attempt to auto-create ActivityDefinition.subtitle"); 1726 else if (Configuration.doAutoCreate()) 1727 this.subtitle = new StringType(); // bb 1728 return this.subtitle; 1729 } 1730 1731 public boolean hasSubtitleElement() { 1732 return this.subtitle != null && !this.subtitle.isEmpty(); 1733 } 1734 1735 public boolean hasSubtitle() { 1736 return this.subtitle != null && !this.subtitle.isEmpty(); 1737 } 1738 1739 /** 1740 * @param value {@link #subtitle} (An explanatory or alternate title for the activity definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 1741 */ 1742 public ActivityDefinition setSubtitleElement(StringType value) { 1743 this.subtitle = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return An explanatory or alternate title for the activity definition giving additional information about its content. 1749 */ 1750 public String getSubtitle() { 1751 return this.subtitle == null ? null : this.subtitle.getValue(); 1752 } 1753 1754 /** 1755 * @param value An explanatory or alternate title for the activity definition giving additional information about its content. 1756 */ 1757 public ActivityDefinition setSubtitle(String value) { 1758 if (Utilities.noString(value)) 1759 this.subtitle = null; 1760 else { 1761 if (this.subtitle == null) 1762 this.subtitle = new StringType(); 1763 this.subtitle.setValue(value); 1764 } 1765 return this; 1766 } 1767 1768 /** 1769 * @return {@link #status} (The status of this activity definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1770 */ 1771 public Enumeration<PublicationStatus> getStatusElement() { 1772 if (this.status == null) 1773 if (Configuration.errorOnAutoCreate()) 1774 throw new Error("Attempt to auto-create ActivityDefinition.status"); 1775 else if (Configuration.doAutoCreate()) 1776 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1777 return this.status; 1778 } 1779 1780 public boolean hasStatusElement() { 1781 return this.status != null && !this.status.isEmpty(); 1782 } 1783 1784 public boolean hasStatus() { 1785 return this.status != null && !this.status.isEmpty(); 1786 } 1787 1788 /** 1789 * @param value {@link #status} (The status of this activity definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1790 */ 1791 public ActivityDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1792 this.status = value; 1793 return this; 1794 } 1795 1796 /** 1797 * @return The status of this activity definition. Enables tracking the life-cycle of the content. 1798 */ 1799 public PublicationStatus getStatus() { 1800 return this.status == null ? null : this.status.getValue(); 1801 } 1802 1803 /** 1804 * @param value The status of this activity definition. Enables tracking the life-cycle of the content. 1805 */ 1806 public ActivityDefinition setStatus(PublicationStatus value) { 1807 if (this.status == null) 1808 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1809 this.status.setValue(value); 1810 return this; 1811 } 1812 1813 /** 1814 * @return {@link #experimental} (A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1815 */ 1816 public BooleanType getExperimentalElement() { 1817 if (this.experimental == null) 1818 if (Configuration.errorOnAutoCreate()) 1819 throw new Error("Attempt to auto-create ActivityDefinition.experimental"); 1820 else if (Configuration.doAutoCreate()) 1821 this.experimental = new BooleanType(); // bb 1822 return this.experimental; 1823 } 1824 1825 public boolean hasExperimentalElement() { 1826 return this.experimental != null && !this.experimental.isEmpty(); 1827 } 1828 1829 public boolean hasExperimental() { 1830 return this.experimental != null && !this.experimental.isEmpty(); 1831 } 1832 1833 /** 1834 * @param value {@link #experimental} (A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1835 */ 1836 public ActivityDefinition setExperimentalElement(BooleanType value) { 1837 this.experimental = value; 1838 return this; 1839 } 1840 1841 /** 1842 * @return A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1843 */ 1844 public boolean getExperimental() { 1845 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1846 } 1847 1848 /** 1849 * @param value A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1850 */ 1851 public ActivityDefinition setExperimental(boolean value) { 1852 if (this.experimental == null) 1853 this.experimental = new BooleanType(); 1854 this.experimental.setValue(value); 1855 return this; 1856 } 1857 1858 /** 1859 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 1860 */ 1861 public DataType getSubject() { 1862 return this.subject; 1863 } 1864 1865 /** 1866 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 1867 */ 1868 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 1869 if (this.subject == null) 1870 this.subject = new CodeableConcept(); 1871 if (!(this.subject instanceof CodeableConcept)) 1872 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 1873 return (CodeableConcept) this.subject; 1874 } 1875 1876 public boolean hasSubjectCodeableConcept() { 1877 return this.subject instanceof CodeableConcept; 1878 } 1879 1880 /** 1881 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 1882 */ 1883 public Reference getSubjectReference() throws FHIRException { 1884 if (this.subject == null) 1885 this.subject = new Reference(); 1886 if (!(this.subject instanceof Reference)) 1887 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 1888 return (Reference) this.subject; 1889 } 1890 1891 public boolean hasSubjectReference() { 1892 return this.subject instanceof Reference; 1893 } 1894 1895 /** 1896 * @return {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 1897 */ 1898 public CanonicalType getSubjectCanonicalType() throws FHIRException { 1899 if (this.subject == null) 1900 this.subject = new CanonicalType(); 1901 if (!(this.subject instanceof CanonicalType)) 1902 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.subject.getClass().getName()+" was encountered"); 1903 return (CanonicalType) this.subject; 1904 } 1905 1906 public boolean hasSubjectCanonicalType() { 1907 return this.subject instanceof CanonicalType; 1908 } 1909 1910 public boolean hasSubject() { 1911 return this.subject != null && !this.subject.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #subject} (A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.) 1916 */ 1917 public ActivityDefinition setSubject(DataType value) { 1918 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference || value instanceof CanonicalType)) 1919 throw new FHIRException("Not the right type for ActivityDefinition.subject[x]: "+value.fhirType()); 1920 this.subject = value; 1921 return this; 1922 } 1923 1924 /** 1925 * @return {@link #date} (The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1926 */ 1927 public DateTimeType getDateElement() { 1928 if (this.date == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create ActivityDefinition.date"); 1931 else if (Configuration.doAutoCreate()) 1932 this.date = new DateTimeType(); // bb 1933 return this.date; 1934 } 1935 1936 public boolean hasDateElement() { 1937 return this.date != null && !this.date.isEmpty(); 1938 } 1939 1940 public boolean hasDate() { 1941 return this.date != null && !this.date.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #date} (The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1946 */ 1947 public ActivityDefinition setDateElement(DateTimeType value) { 1948 this.date = value; 1949 return this; 1950 } 1951 1952 /** 1953 * @return The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes. 1954 */ 1955 public Date getDate() { 1956 return this.date == null ? null : this.date.getValue(); 1957 } 1958 1959 /** 1960 * @param value The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes. 1961 */ 1962 public ActivityDefinition setDate(Date value) { 1963 if (value == null) 1964 this.date = null; 1965 else { 1966 if (this.date == null) 1967 this.date = new DateTimeType(); 1968 this.date.setValue(value); 1969 } 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1975 */ 1976 public StringType getPublisherElement() { 1977 if (this.publisher == null) 1978 if (Configuration.errorOnAutoCreate()) 1979 throw new Error("Attempt to auto-create ActivityDefinition.publisher"); 1980 else if (Configuration.doAutoCreate()) 1981 this.publisher = new StringType(); // bb 1982 return this.publisher; 1983 } 1984 1985 public boolean hasPublisherElement() { 1986 return this.publisher != null && !this.publisher.isEmpty(); 1987 } 1988 1989 public boolean hasPublisher() { 1990 return this.publisher != null && !this.publisher.isEmpty(); 1991 } 1992 1993 /** 1994 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1995 */ 1996 public ActivityDefinition setPublisherElement(StringType value) { 1997 this.publisher = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition. 2003 */ 2004 public String getPublisher() { 2005 return this.publisher == null ? null : this.publisher.getValue(); 2006 } 2007 2008 /** 2009 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition. 2010 */ 2011 public ActivityDefinition setPublisher(String value) { 2012 if (Utilities.noString(value)) 2013 this.publisher = null; 2014 else { 2015 if (this.publisher == null) 2016 this.publisher = new StringType(); 2017 this.publisher.setValue(value); 2018 } 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2024 */ 2025 public List<ContactDetail> getContact() { 2026 if (this.contact == null) 2027 this.contact = new ArrayList<ContactDetail>(); 2028 return this.contact; 2029 } 2030 2031 /** 2032 * @return Returns a reference to <code>this</code> for easy method chaining 2033 */ 2034 public ActivityDefinition setContact(List<ContactDetail> theContact) { 2035 this.contact = theContact; 2036 return this; 2037 } 2038 2039 public boolean hasContact() { 2040 if (this.contact == null) 2041 return false; 2042 for (ContactDetail item : this.contact) 2043 if (!item.isEmpty()) 2044 return true; 2045 return false; 2046 } 2047 2048 public ContactDetail addContact() { //3 2049 ContactDetail t = new ContactDetail(); 2050 if (this.contact == null) 2051 this.contact = new ArrayList<ContactDetail>(); 2052 this.contact.add(t); 2053 return t; 2054 } 2055 2056 public ActivityDefinition addContact(ContactDetail t) { //3 2057 if (t == null) 2058 return this; 2059 if (this.contact == null) 2060 this.contact = new ArrayList<ContactDetail>(); 2061 this.contact.add(t); 2062 return this; 2063 } 2064 2065 /** 2066 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2067 */ 2068 public ContactDetail getContactFirstRep() { 2069 if (getContact().isEmpty()) { 2070 addContact(); 2071 } 2072 return getContact().get(0); 2073 } 2074 2075 /** 2076 * @return {@link #description} (A free text natural language description of the activity definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2077 */ 2078 public MarkdownType getDescriptionElement() { 2079 if (this.description == null) 2080 if (Configuration.errorOnAutoCreate()) 2081 throw new Error("Attempt to auto-create ActivityDefinition.description"); 2082 else if (Configuration.doAutoCreate()) 2083 this.description = new MarkdownType(); // bb 2084 return this.description; 2085 } 2086 2087 public boolean hasDescriptionElement() { 2088 return this.description != null && !this.description.isEmpty(); 2089 } 2090 2091 public boolean hasDescription() { 2092 return this.description != null && !this.description.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #description} (A free text natural language description of the activity definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2097 */ 2098 public ActivityDefinition setDescriptionElement(MarkdownType value) { 2099 this.description = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return A free text natural language description of the activity definition from a consumer's perspective. 2105 */ 2106 public String getDescription() { 2107 return this.description == null ? null : this.description.getValue(); 2108 } 2109 2110 /** 2111 * @param value A free text natural language description of the activity definition from a consumer's perspective. 2112 */ 2113 public ActivityDefinition setDescription(String value) { 2114 if (Utilities.noString(value)) 2115 this.description = null; 2116 else { 2117 if (this.description == null) 2118 this.description = new MarkdownType(); 2119 this.description.setValue(value); 2120 } 2121 return this; 2122 } 2123 2124 /** 2125 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.) 2126 */ 2127 public List<UsageContext> getUseContext() { 2128 if (this.useContext == null) 2129 this.useContext = new ArrayList<UsageContext>(); 2130 return this.useContext; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public ActivityDefinition setUseContext(List<UsageContext> theUseContext) { 2137 this.useContext = theUseContext; 2138 return this; 2139 } 2140 2141 public boolean hasUseContext() { 2142 if (this.useContext == null) 2143 return false; 2144 for (UsageContext item : this.useContext) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 public UsageContext addUseContext() { //3 2151 UsageContext t = new UsageContext(); 2152 if (this.useContext == null) 2153 this.useContext = new ArrayList<UsageContext>(); 2154 this.useContext.add(t); 2155 return t; 2156 } 2157 2158 public ActivityDefinition addUseContext(UsageContext t) { //3 2159 if (t == null) 2160 return this; 2161 if (this.useContext == null) 2162 this.useContext = new ArrayList<UsageContext>(); 2163 this.useContext.add(t); 2164 return this; 2165 } 2166 2167 /** 2168 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2169 */ 2170 public UsageContext getUseContextFirstRep() { 2171 if (getUseContext().isEmpty()) { 2172 addUseContext(); 2173 } 2174 return getUseContext().get(0); 2175 } 2176 2177 /** 2178 * @return {@link #jurisdiction} (A legal or geographic region in which the activity definition is intended to be used.) 2179 */ 2180 public List<CodeableConcept> getJurisdiction() { 2181 if (this.jurisdiction == null) 2182 this.jurisdiction = new ArrayList<CodeableConcept>(); 2183 return this.jurisdiction; 2184 } 2185 2186 /** 2187 * @return Returns a reference to <code>this</code> for easy method chaining 2188 */ 2189 public ActivityDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2190 this.jurisdiction = theJurisdiction; 2191 return this; 2192 } 2193 2194 public boolean hasJurisdiction() { 2195 if (this.jurisdiction == null) 2196 return false; 2197 for (CodeableConcept item : this.jurisdiction) 2198 if (!item.isEmpty()) 2199 return true; 2200 return false; 2201 } 2202 2203 public CodeableConcept addJurisdiction() { //3 2204 CodeableConcept t = new CodeableConcept(); 2205 if (this.jurisdiction == null) 2206 this.jurisdiction = new ArrayList<CodeableConcept>(); 2207 this.jurisdiction.add(t); 2208 return t; 2209 } 2210 2211 public ActivityDefinition addJurisdiction(CodeableConcept t) { //3 2212 if (t == null) 2213 return this; 2214 if (this.jurisdiction == null) 2215 this.jurisdiction = new ArrayList<CodeableConcept>(); 2216 this.jurisdiction.add(t); 2217 return this; 2218 } 2219 2220 /** 2221 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2222 */ 2223 public CodeableConcept getJurisdictionFirstRep() { 2224 if (getJurisdiction().isEmpty()) { 2225 addJurisdiction(); 2226 } 2227 return getJurisdiction().get(0); 2228 } 2229 2230 /** 2231 * @return {@link #purpose} (Explanation of why this activity definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2232 */ 2233 public MarkdownType getPurposeElement() { 2234 if (this.purpose == null) 2235 if (Configuration.errorOnAutoCreate()) 2236 throw new Error("Attempt to auto-create ActivityDefinition.purpose"); 2237 else if (Configuration.doAutoCreate()) 2238 this.purpose = new MarkdownType(); // bb 2239 return this.purpose; 2240 } 2241 2242 public boolean hasPurposeElement() { 2243 return this.purpose != null && !this.purpose.isEmpty(); 2244 } 2245 2246 public boolean hasPurpose() { 2247 return this.purpose != null && !this.purpose.isEmpty(); 2248 } 2249 2250 /** 2251 * @param value {@link #purpose} (Explanation of why this activity definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2252 */ 2253 public ActivityDefinition setPurposeElement(MarkdownType value) { 2254 this.purpose = value; 2255 return this; 2256 } 2257 2258 /** 2259 * @return Explanation of why this activity definition is needed and why it has been designed as it has. 2260 */ 2261 public String getPurpose() { 2262 return this.purpose == null ? null : this.purpose.getValue(); 2263 } 2264 2265 /** 2266 * @param value Explanation of why this activity definition is needed and why it has been designed as it has. 2267 */ 2268 public ActivityDefinition setPurpose(String value) { 2269 if (Utilities.noString(value)) 2270 this.purpose = null; 2271 else { 2272 if (this.purpose == null) 2273 this.purpose = new MarkdownType(); 2274 this.purpose.setValue(value); 2275 } 2276 return this; 2277 } 2278 2279 /** 2280 * @return {@link #usage} (A detailed description of how the activity definition is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2281 */ 2282 public MarkdownType getUsageElement() { 2283 if (this.usage == null) 2284 if (Configuration.errorOnAutoCreate()) 2285 throw new Error("Attempt to auto-create ActivityDefinition.usage"); 2286 else if (Configuration.doAutoCreate()) 2287 this.usage = new MarkdownType(); // bb 2288 return this.usage; 2289 } 2290 2291 public boolean hasUsageElement() { 2292 return this.usage != null && !this.usage.isEmpty(); 2293 } 2294 2295 public boolean hasUsage() { 2296 return this.usage != null && !this.usage.isEmpty(); 2297 } 2298 2299 /** 2300 * @param value {@link #usage} (A detailed description of how the activity definition is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2301 */ 2302 public ActivityDefinition setUsageElement(MarkdownType value) { 2303 this.usage = value; 2304 return this; 2305 } 2306 2307 /** 2308 * @return A detailed description of how the activity definition is used from a clinical perspective. 2309 */ 2310 public String getUsage() { 2311 return this.usage == null ? null : this.usage.getValue(); 2312 } 2313 2314 /** 2315 * @param value A detailed description of how the activity definition is used from a clinical perspective. 2316 */ 2317 public ActivityDefinition setUsage(String value) { 2318 if (Utilities.noString(value)) 2319 this.usage = null; 2320 else { 2321 if (this.usage == null) 2322 this.usage = new MarkdownType(); 2323 this.usage.setValue(value); 2324 } 2325 return this; 2326 } 2327 2328 /** 2329 * @return {@link #copyright} (A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2330 */ 2331 public MarkdownType getCopyrightElement() { 2332 if (this.copyright == null) 2333 if (Configuration.errorOnAutoCreate()) 2334 throw new Error("Attempt to auto-create ActivityDefinition.copyright"); 2335 else if (Configuration.doAutoCreate()) 2336 this.copyright = new MarkdownType(); // bb 2337 return this.copyright; 2338 } 2339 2340 public boolean hasCopyrightElement() { 2341 return this.copyright != null && !this.copyright.isEmpty(); 2342 } 2343 2344 public boolean hasCopyright() { 2345 return this.copyright != null && !this.copyright.isEmpty(); 2346 } 2347 2348 /** 2349 * @param value {@link #copyright} (A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2350 */ 2351 public ActivityDefinition setCopyrightElement(MarkdownType value) { 2352 this.copyright = value; 2353 return this; 2354 } 2355 2356 /** 2357 * @return A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition. 2358 */ 2359 public String getCopyright() { 2360 return this.copyright == null ? null : this.copyright.getValue(); 2361 } 2362 2363 /** 2364 * @param value A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition. 2365 */ 2366 public ActivityDefinition setCopyright(String value) { 2367 if (Utilities.noString(value)) 2368 this.copyright = null; 2369 else { 2370 if (this.copyright == null) 2371 this.copyright = new MarkdownType(); 2372 this.copyright.setValue(value); 2373 } 2374 return this; 2375 } 2376 2377 /** 2378 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2379 */ 2380 public StringType getCopyrightLabelElement() { 2381 if (this.copyrightLabel == null) 2382 if (Configuration.errorOnAutoCreate()) 2383 throw new Error("Attempt to auto-create ActivityDefinition.copyrightLabel"); 2384 else if (Configuration.doAutoCreate()) 2385 this.copyrightLabel = new StringType(); // bb 2386 return this.copyrightLabel; 2387 } 2388 2389 public boolean hasCopyrightLabelElement() { 2390 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2391 } 2392 2393 public boolean hasCopyrightLabel() { 2394 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2395 } 2396 2397 /** 2398 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2399 */ 2400 public ActivityDefinition setCopyrightLabelElement(StringType value) { 2401 this.copyrightLabel = value; 2402 return this; 2403 } 2404 2405 /** 2406 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2407 */ 2408 public String getCopyrightLabel() { 2409 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2410 } 2411 2412 /** 2413 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2414 */ 2415 public ActivityDefinition setCopyrightLabel(String value) { 2416 if (Utilities.noString(value)) 2417 this.copyrightLabel = null; 2418 else { 2419 if (this.copyrightLabel == null) 2420 this.copyrightLabel = new StringType(); 2421 this.copyrightLabel.setValue(value); 2422 } 2423 return this; 2424 } 2425 2426 /** 2427 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2428 */ 2429 public DateType getApprovalDateElement() { 2430 if (this.approvalDate == null) 2431 if (Configuration.errorOnAutoCreate()) 2432 throw new Error("Attempt to auto-create ActivityDefinition.approvalDate"); 2433 else if (Configuration.doAutoCreate()) 2434 this.approvalDate = new DateType(); // bb 2435 return this.approvalDate; 2436 } 2437 2438 public boolean hasApprovalDateElement() { 2439 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2440 } 2441 2442 public boolean hasApprovalDate() { 2443 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2444 } 2445 2446 /** 2447 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2448 */ 2449 public ActivityDefinition setApprovalDateElement(DateType value) { 2450 this.approvalDate = value; 2451 return this; 2452 } 2453 2454 /** 2455 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2456 */ 2457 public Date getApprovalDate() { 2458 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2459 } 2460 2461 /** 2462 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2463 */ 2464 public ActivityDefinition setApprovalDate(Date value) { 2465 if (value == null) 2466 this.approvalDate = null; 2467 else { 2468 if (this.approvalDate == null) 2469 this.approvalDate = new DateType(); 2470 this.approvalDate.setValue(value); 2471 } 2472 return this; 2473 } 2474 2475 /** 2476 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2477 */ 2478 public DateType getLastReviewDateElement() { 2479 if (this.lastReviewDate == null) 2480 if (Configuration.errorOnAutoCreate()) 2481 throw new Error("Attempt to auto-create ActivityDefinition.lastReviewDate"); 2482 else if (Configuration.doAutoCreate()) 2483 this.lastReviewDate = new DateType(); // bb 2484 return this.lastReviewDate; 2485 } 2486 2487 public boolean hasLastReviewDateElement() { 2488 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2489 } 2490 2491 public boolean hasLastReviewDate() { 2492 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2493 } 2494 2495 /** 2496 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2497 */ 2498 public ActivityDefinition setLastReviewDateElement(DateType value) { 2499 this.lastReviewDate = value; 2500 return this; 2501 } 2502 2503 /** 2504 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2505 */ 2506 public Date getLastReviewDate() { 2507 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2508 } 2509 2510 /** 2511 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2512 */ 2513 public ActivityDefinition setLastReviewDate(Date value) { 2514 if (value == null) 2515 this.lastReviewDate = null; 2516 else { 2517 if (this.lastReviewDate == null) 2518 this.lastReviewDate = new DateType(); 2519 this.lastReviewDate.setValue(value); 2520 } 2521 return this; 2522 } 2523 2524 /** 2525 * @return {@link #effectivePeriod} (The period during which the activity definition content was or is planned to be in active use.) 2526 */ 2527 public Period getEffectivePeriod() { 2528 if (this.effectivePeriod == null) 2529 if (Configuration.errorOnAutoCreate()) 2530 throw new Error("Attempt to auto-create ActivityDefinition.effectivePeriod"); 2531 else if (Configuration.doAutoCreate()) 2532 this.effectivePeriod = new Period(); // cc 2533 return this.effectivePeriod; 2534 } 2535 2536 public boolean hasEffectivePeriod() { 2537 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2538 } 2539 2540 /** 2541 * @param value {@link #effectivePeriod} (The period during which the activity definition content was or is planned to be in active use.) 2542 */ 2543 public ActivityDefinition setEffectivePeriod(Period value) { 2544 this.effectivePeriod = value; 2545 return this; 2546 } 2547 2548 /** 2549 * @return {@link #topic} (Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.) 2550 */ 2551 public List<CodeableConcept> getTopic() { 2552 if (this.topic == null) 2553 this.topic = new ArrayList<CodeableConcept>(); 2554 return this.topic; 2555 } 2556 2557 /** 2558 * @return Returns a reference to <code>this</code> for easy method chaining 2559 */ 2560 public ActivityDefinition setTopic(List<CodeableConcept> theTopic) { 2561 this.topic = theTopic; 2562 return this; 2563 } 2564 2565 public boolean hasTopic() { 2566 if (this.topic == null) 2567 return false; 2568 for (CodeableConcept item : this.topic) 2569 if (!item.isEmpty()) 2570 return true; 2571 return false; 2572 } 2573 2574 public CodeableConcept addTopic() { //3 2575 CodeableConcept t = new CodeableConcept(); 2576 if (this.topic == null) 2577 this.topic = new ArrayList<CodeableConcept>(); 2578 this.topic.add(t); 2579 return t; 2580 } 2581 2582 public ActivityDefinition addTopic(CodeableConcept t) { //3 2583 if (t == null) 2584 return this; 2585 if (this.topic == null) 2586 this.topic = new ArrayList<CodeableConcept>(); 2587 this.topic.add(t); 2588 return this; 2589 } 2590 2591 /** 2592 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 2593 */ 2594 public CodeableConcept getTopicFirstRep() { 2595 if (getTopic().isEmpty()) { 2596 addTopic(); 2597 } 2598 return getTopic().get(0); 2599 } 2600 2601 /** 2602 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 2603 */ 2604 public List<ContactDetail> getAuthor() { 2605 if (this.author == null) 2606 this.author = new ArrayList<ContactDetail>(); 2607 return this.author; 2608 } 2609 2610 /** 2611 * @return Returns a reference to <code>this</code> for easy method chaining 2612 */ 2613 public ActivityDefinition setAuthor(List<ContactDetail> theAuthor) { 2614 this.author = theAuthor; 2615 return this; 2616 } 2617 2618 public boolean hasAuthor() { 2619 if (this.author == null) 2620 return false; 2621 for (ContactDetail item : this.author) 2622 if (!item.isEmpty()) 2623 return true; 2624 return false; 2625 } 2626 2627 public ContactDetail addAuthor() { //3 2628 ContactDetail t = new ContactDetail(); 2629 if (this.author == null) 2630 this.author = new ArrayList<ContactDetail>(); 2631 this.author.add(t); 2632 return t; 2633 } 2634 2635 public ActivityDefinition addAuthor(ContactDetail t) { //3 2636 if (t == null) 2637 return this; 2638 if (this.author == null) 2639 this.author = new ArrayList<ContactDetail>(); 2640 this.author.add(t); 2641 return this; 2642 } 2643 2644 /** 2645 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 2646 */ 2647 public ContactDetail getAuthorFirstRep() { 2648 if (getAuthor().isEmpty()) { 2649 addAuthor(); 2650 } 2651 return getAuthor().get(0); 2652 } 2653 2654 /** 2655 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 2656 */ 2657 public List<ContactDetail> getEditor() { 2658 if (this.editor == null) 2659 this.editor = new ArrayList<ContactDetail>(); 2660 return this.editor; 2661 } 2662 2663 /** 2664 * @return Returns a reference to <code>this</code> for easy method chaining 2665 */ 2666 public ActivityDefinition setEditor(List<ContactDetail> theEditor) { 2667 this.editor = theEditor; 2668 return this; 2669 } 2670 2671 public boolean hasEditor() { 2672 if (this.editor == null) 2673 return false; 2674 for (ContactDetail item : this.editor) 2675 if (!item.isEmpty()) 2676 return true; 2677 return false; 2678 } 2679 2680 public ContactDetail addEditor() { //3 2681 ContactDetail t = new ContactDetail(); 2682 if (this.editor == null) 2683 this.editor = new ArrayList<ContactDetail>(); 2684 this.editor.add(t); 2685 return t; 2686 } 2687 2688 public ActivityDefinition addEditor(ContactDetail t) { //3 2689 if (t == null) 2690 return this; 2691 if (this.editor == null) 2692 this.editor = new ArrayList<ContactDetail>(); 2693 this.editor.add(t); 2694 return this; 2695 } 2696 2697 /** 2698 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 2699 */ 2700 public ContactDetail getEditorFirstRep() { 2701 if (getEditor().isEmpty()) { 2702 addEditor(); 2703 } 2704 return getEditor().get(0); 2705 } 2706 2707 /** 2708 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 2709 */ 2710 public List<ContactDetail> getReviewer() { 2711 if (this.reviewer == null) 2712 this.reviewer = new ArrayList<ContactDetail>(); 2713 return this.reviewer; 2714 } 2715 2716 /** 2717 * @return Returns a reference to <code>this</code> for easy method chaining 2718 */ 2719 public ActivityDefinition setReviewer(List<ContactDetail> theReviewer) { 2720 this.reviewer = theReviewer; 2721 return this; 2722 } 2723 2724 public boolean hasReviewer() { 2725 if (this.reviewer == null) 2726 return false; 2727 for (ContactDetail item : this.reviewer) 2728 if (!item.isEmpty()) 2729 return true; 2730 return false; 2731 } 2732 2733 public ContactDetail addReviewer() { //3 2734 ContactDetail t = new ContactDetail(); 2735 if (this.reviewer == null) 2736 this.reviewer = new ArrayList<ContactDetail>(); 2737 this.reviewer.add(t); 2738 return t; 2739 } 2740 2741 public ActivityDefinition addReviewer(ContactDetail t) { //3 2742 if (t == null) 2743 return this; 2744 if (this.reviewer == null) 2745 this.reviewer = new ArrayList<ContactDetail>(); 2746 this.reviewer.add(t); 2747 return this; 2748 } 2749 2750 /** 2751 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 2752 */ 2753 public ContactDetail getReviewerFirstRep() { 2754 if (getReviewer().isEmpty()) { 2755 addReviewer(); 2756 } 2757 return getReviewer().get(0); 2758 } 2759 2760 /** 2761 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 2762 */ 2763 public List<ContactDetail> getEndorser() { 2764 if (this.endorser == null) 2765 this.endorser = new ArrayList<ContactDetail>(); 2766 return this.endorser; 2767 } 2768 2769 /** 2770 * @return Returns a reference to <code>this</code> for easy method chaining 2771 */ 2772 public ActivityDefinition setEndorser(List<ContactDetail> theEndorser) { 2773 this.endorser = theEndorser; 2774 return this; 2775 } 2776 2777 public boolean hasEndorser() { 2778 if (this.endorser == null) 2779 return false; 2780 for (ContactDetail item : this.endorser) 2781 if (!item.isEmpty()) 2782 return true; 2783 return false; 2784 } 2785 2786 public ContactDetail addEndorser() { //3 2787 ContactDetail t = new ContactDetail(); 2788 if (this.endorser == null) 2789 this.endorser = new ArrayList<ContactDetail>(); 2790 this.endorser.add(t); 2791 return t; 2792 } 2793 2794 public ActivityDefinition addEndorser(ContactDetail t) { //3 2795 if (t == null) 2796 return this; 2797 if (this.endorser == null) 2798 this.endorser = new ArrayList<ContactDetail>(); 2799 this.endorser.add(t); 2800 return this; 2801 } 2802 2803 /** 2804 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 2805 */ 2806 public ContactDetail getEndorserFirstRep() { 2807 if (getEndorser().isEmpty()) { 2808 addEndorser(); 2809 } 2810 return getEndorser().get(0); 2811 } 2812 2813 /** 2814 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 2815 */ 2816 public List<RelatedArtifact> getRelatedArtifact() { 2817 if (this.relatedArtifact == null) 2818 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2819 return this.relatedArtifact; 2820 } 2821 2822 /** 2823 * @return Returns a reference to <code>this</code> for easy method chaining 2824 */ 2825 public ActivityDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2826 this.relatedArtifact = theRelatedArtifact; 2827 return this; 2828 } 2829 2830 public boolean hasRelatedArtifact() { 2831 if (this.relatedArtifact == null) 2832 return false; 2833 for (RelatedArtifact item : this.relatedArtifact) 2834 if (!item.isEmpty()) 2835 return true; 2836 return false; 2837 } 2838 2839 public RelatedArtifact addRelatedArtifact() { //3 2840 RelatedArtifact t = new RelatedArtifact(); 2841 if (this.relatedArtifact == null) 2842 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2843 this.relatedArtifact.add(t); 2844 return t; 2845 } 2846 2847 public ActivityDefinition addRelatedArtifact(RelatedArtifact t) { //3 2848 if (t == null) 2849 return this; 2850 if (this.relatedArtifact == null) 2851 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2852 this.relatedArtifact.add(t); 2853 return this; 2854 } 2855 2856 /** 2857 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 2858 */ 2859 public RelatedArtifact getRelatedArtifactFirstRep() { 2860 if (getRelatedArtifact().isEmpty()) { 2861 addRelatedArtifact(); 2862 } 2863 return getRelatedArtifact().get(0); 2864 } 2865 2866 /** 2867 * @return {@link #library} (A reference to a Library resource containing any formal logic used by the activity definition.) 2868 */ 2869 public List<CanonicalType> getLibrary() { 2870 if (this.library == null) 2871 this.library = new ArrayList<CanonicalType>(); 2872 return this.library; 2873 } 2874 2875 /** 2876 * @return Returns a reference to <code>this</code> for easy method chaining 2877 */ 2878 public ActivityDefinition setLibrary(List<CanonicalType> theLibrary) { 2879 this.library = theLibrary; 2880 return this; 2881 } 2882 2883 public boolean hasLibrary() { 2884 if (this.library == null) 2885 return false; 2886 for (CanonicalType item : this.library) 2887 if (!item.isEmpty()) 2888 return true; 2889 return false; 2890 } 2891 2892 /** 2893 * @return {@link #library} (A reference to a Library resource containing any formal logic used by the activity definition.) 2894 */ 2895 public CanonicalType addLibraryElement() {//2 2896 CanonicalType t = new CanonicalType(); 2897 if (this.library == null) 2898 this.library = new ArrayList<CanonicalType>(); 2899 this.library.add(t); 2900 return t; 2901 } 2902 2903 /** 2904 * @param value {@link #library} (A reference to a Library resource containing any formal logic used by the activity definition.) 2905 */ 2906 public ActivityDefinition addLibrary(String value) { //1 2907 CanonicalType t = new CanonicalType(); 2908 t.setValue(value); 2909 if (this.library == null) 2910 this.library = new ArrayList<CanonicalType>(); 2911 this.library.add(t); 2912 return this; 2913 } 2914 2915 /** 2916 * @param value {@link #library} (A reference to a Library resource containing any formal logic used by the activity definition.) 2917 */ 2918 public boolean hasLibrary(String value) { 2919 if (this.library == null) 2920 return false; 2921 for (CanonicalType v : this.library) 2922 if (v.getValue().equals(value)) // canonical 2923 return true; 2924 return false; 2925 } 2926 2927 /** 2928 * @return {@link #kind} (A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2929 */ 2930 public Enumeration<RequestResourceTypes> getKindElement() { 2931 if (this.kind == null) 2932 if (Configuration.errorOnAutoCreate()) 2933 throw new Error("Attempt to auto-create ActivityDefinition.kind"); 2934 else if (Configuration.doAutoCreate()) 2935 this.kind = new Enumeration<RequestResourceTypes>(new RequestResourceTypesEnumFactory()); // bb 2936 return this.kind; 2937 } 2938 2939 public boolean hasKindElement() { 2940 return this.kind != null && !this.kind.isEmpty(); 2941 } 2942 2943 public boolean hasKind() { 2944 return this.kind != null && !this.kind.isEmpty(); 2945 } 2946 2947 /** 2948 * @param value {@link #kind} (A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2949 */ 2950 public ActivityDefinition setKindElement(Enumeration<RequestResourceTypes> value) { 2951 this.kind = value; 2952 return this; 2953 } 2954 2955 /** 2956 * @return A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. 2957 */ 2958 public RequestResourceTypes getKind() { 2959 return this.kind == null ? null : this.kind.getValue(); 2960 } 2961 2962 /** 2963 * @param value A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. 2964 */ 2965 public ActivityDefinition setKind(RequestResourceTypes value) { 2966 if (value == null) 2967 this.kind = null; 2968 else { 2969 if (this.kind == null) 2970 this.kind = new Enumeration<RequestResourceTypes>(new RequestResourceTypesEnumFactory()); 2971 this.kind.setValue(value); 2972 } 2973 return this; 2974 } 2975 2976 /** 2977 * @return {@link #profile} (A profile to which the target of the activity definition is expected to conform.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 2978 */ 2979 public CanonicalType getProfileElement() { 2980 if (this.profile == null) 2981 if (Configuration.errorOnAutoCreate()) 2982 throw new Error("Attempt to auto-create ActivityDefinition.profile"); 2983 else if (Configuration.doAutoCreate()) 2984 this.profile = new CanonicalType(); // bb 2985 return this.profile; 2986 } 2987 2988 public boolean hasProfileElement() { 2989 return this.profile != null && !this.profile.isEmpty(); 2990 } 2991 2992 public boolean hasProfile() { 2993 return this.profile != null && !this.profile.isEmpty(); 2994 } 2995 2996 /** 2997 * @param value {@link #profile} (A profile to which the target of the activity definition is expected to conform.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 2998 */ 2999 public ActivityDefinition setProfileElement(CanonicalType value) { 3000 this.profile = value; 3001 return this; 3002 } 3003 3004 /** 3005 * @return A profile to which the target of the activity definition is expected to conform. 3006 */ 3007 public String getProfile() { 3008 return this.profile == null ? null : this.profile.getValue(); 3009 } 3010 3011 /** 3012 * @param value A profile to which the target of the activity definition is expected to conform. 3013 */ 3014 public ActivityDefinition setProfile(String value) { 3015 if (Utilities.noString(value)) 3016 this.profile = null; 3017 else { 3018 if (this.profile == null) 3019 this.profile = new CanonicalType(); 3020 this.profile.setValue(value); 3021 } 3022 return this; 3023 } 3024 3025 /** 3026 * @return {@link #code} (Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.) 3027 */ 3028 public CodeableConcept getCode() { 3029 if (this.code == null) 3030 if (Configuration.errorOnAutoCreate()) 3031 throw new Error("Attempt to auto-create ActivityDefinition.code"); 3032 else if (Configuration.doAutoCreate()) 3033 this.code = new CodeableConcept(); // cc 3034 return this.code; 3035 } 3036 3037 public boolean hasCode() { 3038 return this.code != null && !this.code.isEmpty(); 3039 } 3040 3041 /** 3042 * @param value {@link #code} (Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.) 3043 */ 3044 public ActivityDefinition setCode(CodeableConcept value) { 3045 this.code = value; 3046 return this; 3047 } 3048 3049 /** 3050 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 3051 */ 3052 public Enumeration<RequestIntent> getIntentElement() { 3053 if (this.intent == null) 3054 if (Configuration.errorOnAutoCreate()) 3055 throw new Error("Attempt to auto-create ActivityDefinition.intent"); 3056 else if (Configuration.doAutoCreate()) 3057 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 3058 return this.intent; 3059 } 3060 3061 public boolean hasIntentElement() { 3062 return this.intent != null && !this.intent.isEmpty(); 3063 } 3064 3065 public boolean hasIntent() { 3066 return this.intent != null && !this.intent.isEmpty(); 3067 } 3068 3069 /** 3070 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 3071 */ 3072 public ActivityDefinition setIntentElement(Enumeration<RequestIntent> value) { 3073 this.intent = value; 3074 return this; 3075 } 3076 3077 /** 3078 * @return Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain. 3079 */ 3080 public RequestIntent getIntent() { 3081 return this.intent == null ? null : this.intent.getValue(); 3082 } 3083 3084 /** 3085 * @param value Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain. 3086 */ 3087 public ActivityDefinition setIntent(RequestIntent value) { 3088 if (value == null) 3089 this.intent = null; 3090 else { 3091 if (this.intent == null) 3092 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 3093 this.intent.setValue(value); 3094 } 3095 return this; 3096 } 3097 3098 /** 3099 * @return {@link #priority} (Indicates how quickly the activity should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 3100 */ 3101 public Enumeration<RequestPriority> getPriorityElement() { 3102 if (this.priority == null) 3103 if (Configuration.errorOnAutoCreate()) 3104 throw new Error("Attempt to auto-create ActivityDefinition.priority"); 3105 else if (Configuration.doAutoCreate()) 3106 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 3107 return this.priority; 3108 } 3109 3110 public boolean hasPriorityElement() { 3111 return this.priority != null && !this.priority.isEmpty(); 3112 } 3113 3114 public boolean hasPriority() { 3115 return this.priority != null && !this.priority.isEmpty(); 3116 } 3117 3118 /** 3119 * @param value {@link #priority} (Indicates how quickly the activity should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 3120 */ 3121 public ActivityDefinition setPriorityElement(Enumeration<RequestPriority> value) { 3122 this.priority = value; 3123 return this; 3124 } 3125 3126 /** 3127 * @return Indicates how quickly the activity should be addressed with respect to other requests. 3128 */ 3129 public RequestPriority getPriority() { 3130 return this.priority == null ? null : this.priority.getValue(); 3131 } 3132 3133 /** 3134 * @param value Indicates how quickly the activity should be addressed with respect to other requests. 3135 */ 3136 public ActivityDefinition setPriority(RequestPriority value) { 3137 if (value == null) 3138 this.priority = null; 3139 else { 3140 if (this.priority == null) 3141 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 3142 this.priority.setValue(value); 3143 } 3144 return this; 3145 } 3146 3147 /** 3148 * @return {@link #doNotPerform} (Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 3149 */ 3150 public BooleanType getDoNotPerformElement() { 3151 if (this.doNotPerform == null) 3152 if (Configuration.errorOnAutoCreate()) 3153 throw new Error("Attempt to auto-create ActivityDefinition.doNotPerform"); 3154 else if (Configuration.doAutoCreate()) 3155 this.doNotPerform = new BooleanType(); // bb 3156 return this.doNotPerform; 3157 } 3158 3159 public boolean hasDoNotPerformElement() { 3160 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 3161 } 3162 3163 public boolean hasDoNotPerform() { 3164 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 3165 } 3166 3167 /** 3168 * @param value {@link #doNotPerform} (Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 3169 */ 3170 public ActivityDefinition setDoNotPerformElement(BooleanType value) { 3171 this.doNotPerform = value; 3172 return this; 3173 } 3174 3175 /** 3176 * @return Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action. 3177 */ 3178 public boolean getDoNotPerform() { 3179 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 3180 } 3181 3182 /** 3183 * @param value Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action. 3184 */ 3185 public ActivityDefinition setDoNotPerform(boolean value) { 3186 if (this.doNotPerform == null) 3187 this.doNotPerform = new BooleanType(); 3188 this.doNotPerform.setValue(value); 3189 return this; 3190 } 3191 3192 /** 3193 * @return {@link #timing} (The timing or frequency upon which the described activity is to occur.) 3194 */ 3195 public DataType getTiming() { 3196 return this.timing; 3197 } 3198 3199 /** 3200 * @return {@link #timing} (The timing or frequency upon which the described activity is to occur.) 3201 */ 3202 public Timing getTimingTiming() throws FHIRException { 3203 if (this.timing == null) 3204 this.timing = new Timing(); 3205 if (!(this.timing instanceof Timing)) 3206 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 3207 return (Timing) this.timing; 3208 } 3209 3210 public boolean hasTimingTiming() { 3211 return this.timing instanceof Timing; 3212 } 3213 3214 /** 3215 * @return {@link #timing} (The timing or frequency upon which the described activity is to occur.) 3216 */ 3217 public Age getTimingAge() throws FHIRException { 3218 if (this.timing == null) 3219 this.timing = new Age(); 3220 if (!(this.timing instanceof Age)) 3221 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.timing.getClass().getName()+" was encountered"); 3222 return (Age) this.timing; 3223 } 3224 3225 public boolean hasTimingAge() { 3226 return this.timing instanceof Age; 3227 } 3228 3229 /** 3230 * @return {@link #timing} (The timing or frequency upon which the described activity is to occur.) 3231 */ 3232 public Range getTimingRange() throws FHIRException { 3233 if (this.timing == null) 3234 this.timing = new Range(); 3235 if (!(this.timing instanceof Range)) 3236 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 3237 return (Range) this.timing; 3238 } 3239 3240 public boolean hasTimingRange() { 3241 return this.timing instanceof Range; 3242 } 3243 3244 /** 3245 * @return {@link #timing} (The timing or frequency upon which the described activity is to occur.) 3246 */ 3247 public Duration getTimingDuration() throws FHIRException { 3248 if (this.timing == null) 3249 this.timing = new Duration(); 3250 if (!(this.timing instanceof Duration)) 3251 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.timing.getClass().getName()+" was encountered"); 3252 return (Duration) this.timing; 3253 } 3254 3255 public boolean hasTimingDuration() { 3256 return this.timing instanceof Duration; 3257 } 3258 3259 public boolean hasTiming() { 3260 return this.timing != null && !this.timing.isEmpty(); 3261 } 3262 3263 /** 3264 * @param value {@link #timing} (The timing or frequency upon which the described activity is to occur.) 3265 */ 3266 public ActivityDefinition setTiming(DataType value) { 3267 if (value != null && !(value instanceof Timing || value instanceof Age || value instanceof Range || value instanceof Duration)) 3268 throw new FHIRException("Not the right type for ActivityDefinition.timing[x]: "+value.fhirType()); 3269 this.timing = value; 3270 return this; 3271 } 3272 3273 /** 3274 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 3275 */ 3276 public DataType getAsNeeded() { 3277 return this.asNeeded; 3278 } 3279 3280 /** 3281 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 3282 */ 3283 public BooleanType getAsNeededBooleanType() throws FHIRException { 3284 if (this.asNeeded == null) 3285 this.asNeeded = new BooleanType(); 3286 if (!(this.asNeeded instanceof BooleanType)) 3287 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 3288 return (BooleanType) this.asNeeded; 3289 } 3290 3291 public boolean hasAsNeededBooleanType() { 3292 return this.asNeeded instanceof BooleanType; 3293 } 3294 3295 /** 3296 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 3297 */ 3298 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 3299 if (this.asNeeded == null) 3300 this.asNeeded = new CodeableConcept(); 3301 if (!(this.asNeeded instanceof CodeableConcept)) 3302 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 3303 return (CodeableConcept) this.asNeeded; 3304 } 3305 3306 public boolean hasAsNeededCodeableConcept() { 3307 return this.asNeeded instanceof CodeableConcept; 3308 } 3309 3310 public boolean hasAsNeeded() { 3311 return this.asNeeded != null && !this.asNeeded.isEmpty(); 3312 } 3313 3314 /** 3315 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 3316 */ 3317 public ActivityDefinition setAsNeeded(DataType value) { 3318 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 3319 throw new FHIRException("Not the right type for ActivityDefinition.asNeeded[x]: "+value.fhirType()); 3320 this.asNeeded = value; 3321 return this; 3322 } 3323 3324 /** 3325 * @return {@link #location} (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 3326 */ 3327 public CodeableReference getLocation() { 3328 if (this.location == null) 3329 if (Configuration.errorOnAutoCreate()) 3330 throw new Error("Attempt to auto-create ActivityDefinition.location"); 3331 else if (Configuration.doAutoCreate()) 3332 this.location = new CodeableReference(); // cc 3333 return this.location; 3334 } 3335 3336 public boolean hasLocation() { 3337 return this.location != null && !this.location.isEmpty(); 3338 } 3339 3340 /** 3341 * @param value {@link #location} (Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.) 3342 */ 3343 public ActivityDefinition setLocation(CodeableReference value) { 3344 this.location = value; 3345 return this; 3346 } 3347 3348 /** 3349 * @return {@link #participant} (Indicates who should participate in performing the action described.) 3350 */ 3351 public List<ActivityDefinitionParticipantComponent> getParticipant() { 3352 if (this.participant == null) 3353 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 3354 return this.participant; 3355 } 3356 3357 /** 3358 * @return Returns a reference to <code>this</code> for easy method chaining 3359 */ 3360 public ActivityDefinition setParticipant(List<ActivityDefinitionParticipantComponent> theParticipant) { 3361 this.participant = theParticipant; 3362 return this; 3363 } 3364 3365 public boolean hasParticipant() { 3366 if (this.participant == null) 3367 return false; 3368 for (ActivityDefinitionParticipantComponent item : this.participant) 3369 if (!item.isEmpty()) 3370 return true; 3371 return false; 3372 } 3373 3374 public ActivityDefinitionParticipantComponent addParticipant() { //3 3375 ActivityDefinitionParticipantComponent t = new ActivityDefinitionParticipantComponent(); 3376 if (this.participant == null) 3377 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 3378 this.participant.add(t); 3379 return t; 3380 } 3381 3382 public ActivityDefinition addParticipant(ActivityDefinitionParticipantComponent t) { //3 3383 if (t == null) 3384 return this; 3385 if (this.participant == null) 3386 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 3387 this.participant.add(t); 3388 return this; 3389 } 3390 3391 /** 3392 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 3393 */ 3394 public ActivityDefinitionParticipantComponent getParticipantFirstRep() { 3395 if (getParticipant().isEmpty()) { 3396 addParticipant(); 3397 } 3398 return getParticipant().get(0); 3399 } 3400 3401 /** 3402 * @return {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 3403 */ 3404 public DataType getProduct() { 3405 return this.product; 3406 } 3407 3408 /** 3409 * @return {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 3410 */ 3411 public Reference getProductReference() throws FHIRException { 3412 if (this.product == null) 3413 this.product = new Reference(); 3414 if (!(this.product instanceof Reference)) 3415 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.product.getClass().getName()+" was encountered"); 3416 return (Reference) this.product; 3417 } 3418 3419 public boolean hasProductReference() { 3420 return this.product instanceof Reference; 3421 } 3422 3423 /** 3424 * @return {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 3425 */ 3426 public CodeableConcept getProductCodeableConcept() throws FHIRException { 3427 if (this.product == null) 3428 this.product = new CodeableConcept(); 3429 if (!(this.product instanceof CodeableConcept)) 3430 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.product.getClass().getName()+" was encountered"); 3431 return (CodeableConcept) this.product; 3432 } 3433 3434 public boolean hasProductCodeableConcept() { 3435 return this.product instanceof CodeableConcept; 3436 } 3437 3438 public boolean hasProduct() { 3439 return this.product != null && !this.product.isEmpty(); 3440 } 3441 3442 /** 3443 * @param value {@link #product} (Identifies the food, drug or other product being consumed or supplied in the activity.) 3444 */ 3445 public ActivityDefinition setProduct(DataType value) { 3446 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 3447 throw new FHIRException("Not the right type for ActivityDefinition.product[x]: "+value.fhirType()); 3448 this.product = value; 3449 return this; 3450 } 3451 3452 /** 3453 * @return {@link #quantity} (Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).) 3454 */ 3455 public Quantity getQuantity() { 3456 if (this.quantity == null) 3457 if (Configuration.errorOnAutoCreate()) 3458 throw new Error("Attempt to auto-create ActivityDefinition.quantity"); 3459 else if (Configuration.doAutoCreate()) 3460 this.quantity = new Quantity(); // cc 3461 return this.quantity; 3462 } 3463 3464 public boolean hasQuantity() { 3465 return this.quantity != null && !this.quantity.isEmpty(); 3466 } 3467 3468 /** 3469 * @param value {@link #quantity} (Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).) 3470 */ 3471 public ActivityDefinition setQuantity(Quantity value) { 3472 this.quantity = value; 3473 return this; 3474 } 3475 3476 /** 3477 * @return {@link #dosage} (Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.) 3478 */ 3479 public List<Dosage> getDosage() { 3480 if (this.dosage == null) 3481 this.dosage = new ArrayList<Dosage>(); 3482 return this.dosage; 3483 } 3484 3485 /** 3486 * @return Returns a reference to <code>this</code> for easy method chaining 3487 */ 3488 public ActivityDefinition setDosage(List<Dosage> theDosage) { 3489 this.dosage = theDosage; 3490 return this; 3491 } 3492 3493 public boolean hasDosage() { 3494 if (this.dosage == null) 3495 return false; 3496 for (Dosage item : this.dosage) 3497 if (!item.isEmpty()) 3498 return true; 3499 return false; 3500 } 3501 3502 public Dosage addDosage() { //3 3503 Dosage t = new Dosage(); 3504 if (this.dosage == null) 3505 this.dosage = new ArrayList<Dosage>(); 3506 this.dosage.add(t); 3507 return t; 3508 } 3509 3510 public ActivityDefinition addDosage(Dosage t) { //3 3511 if (t == null) 3512 return this; 3513 if (this.dosage == null) 3514 this.dosage = new ArrayList<Dosage>(); 3515 this.dosage.add(t); 3516 return this; 3517 } 3518 3519 /** 3520 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 3521 */ 3522 public Dosage getDosageFirstRep() { 3523 if (getDosage().isEmpty()) { 3524 addDosage(); 3525 } 3526 return getDosage().get(0); 3527 } 3528 3529 /** 3530 * @return {@link #bodySite} (Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).) 3531 */ 3532 public List<CodeableConcept> getBodySite() { 3533 if (this.bodySite == null) 3534 this.bodySite = new ArrayList<CodeableConcept>(); 3535 return this.bodySite; 3536 } 3537 3538 /** 3539 * @return Returns a reference to <code>this</code> for easy method chaining 3540 */ 3541 public ActivityDefinition setBodySite(List<CodeableConcept> theBodySite) { 3542 this.bodySite = theBodySite; 3543 return this; 3544 } 3545 3546 public boolean hasBodySite() { 3547 if (this.bodySite == null) 3548 return false; 3549 for (CodeableConcept item : this.bodySite) 3550 if (!item.isEmpty()) 3551 return true; 3552 return false; 3553 } 3554 3555 public CodeableConcept addBodySite() { //3 3556 CodeableConcept t = new CodeableConcept(); 3557 if (this.bodySite == null) 3558 this.bodySite = new ArrayList<CodeableConcept>(); 3559 this.bodySite.add(t); 3560 return t; 3561 } 3562 3563 public ActivityDefinition addBodySite(CodeableConcept t) { //3 3564 if (t == null) 3565 return this; 3566 if (this.bodySite == null) 3567 this.bodySite = new ArrayList<CodeableConcept>(); 3568 this.bodySite.add(t); 3569 return this; 3570 } 3571 3572 /** 3573 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 3574 */ 3575 public CodeableConcept getBodySiteFirstRep() { 3576 if (getBodySite().isEmpty()) { 3577 addBodySite(); 3578 } 3579 return getBodySite().get(0); 3580 } 3581 3582 /** 3583 * @return {@link #specimenRequirement} (Defines specimen requirements for the action to be performed, such as required specimens for a lab test.) 3584 */ 3585 public List<CanonicalType> getSpecimenRequirement() { 3586 if (this.specimenRequirement == null) 3587 this.specimenRequirement = new ArrayList<CanonicalType>(); 3588 return this.specimenRequirement; 3589 } 3590 3591 /** 3592 * @return Returns a reference to <code>this</code> for easy method chaining 3593 */ 3594 public ActivityDefinition setSpecimenRequirement(List<CanonicalType> theSpecimenRequirement) { 3595 this.specimenRequirement = theSpecimenRequirement; 3596 return this; 3597 } 3598 3599 public boolean hasSpecimenRequirement() { 3600 if (this.specimenRequirement == null) 3601 return false; 3602 for (CanonicalType item : this.specimenRequirement) 3603 if (!item.isEmpty()) 3604 return true; 3605 return false; 3606 } 3607 3608 /** 3609 * @return {@link #specimenRequirement} (Defines specimen requirements for the action to be performed, such as required specimens for a lab test.) 3610 */ 3611 public CanonicalType addSpecimenRequirementElement() {//2 3612 CanonicalType t = new CanonicalType(); 3613 if (this.specimenRequirement == null) 3614 this.specimenRequirement = new ArrayList<CanonicalType>(); 3615 this.specimenRequirement.add(t); 3616 return t; 3617 } 3618 3619 /** 3620 * @param value {@link #specimenRequirement} (Defines specimen requirements for the action to be performed, such as required specimens for a lab test.) 3621 */ 3622 public ActivityDefinition addSpecimenRequirement(String value) { //1 3623 CanonicalType t = new CanonicalType(); 3624 t.setValue(value); 3625 if (this.specimenRequirement == null) 3626 this.specimenRequirement = new ArrayList<CanonicalType>(); 3627 this.specimenRequirement.add(t); 3628 return this; 3629 } 3630 3631 /** 3632 * @param value {@link #specimenRequirement} (Defines specimen requirements for the action to be performed, such as required specimens for a lab test.) 3633 */ 3634 public boolean hasSpecimenRequirement(String value) { 3635 if (this.specimenRequirement == null) 3636 return false; 3637 for (CanonicalType v : this.specimenRequirement) 3638 if (v.getValue().equals(value)) // canonical 3639 return true; 3640 return false; 3641 } 3642 3643 /** 3644 * @return {@link #observationRequirement} (Defines observation requirements for the action to be performed, such as body weight or surface area.) 3645 */ 3646 public List<CanonicalType> getObservationRequirement() { 3647 if (this.observationRequirement == null) 3648 this.observationRequirement = new ArrayList<CanonicalType>(); 3649 return this.observationRequirement; 3650 } 3651 3652 /** 3653 * @return Returns a reference to <code>this</code> for easy method chaining 3654 */ 3655 public ActivityDefinition setObservationRequirement(List<CanonicalType> theObservationRequirement) { 3656 this.observationRequirement = theObservationRequirement; 3657 return this; 3658 } 3659 3660 public boolean hasObservationRequirement() { 3661 if (this.observationRequirement == null) 3662 return false; 3663 for (CanonicalType item : this.observationRequirement) 3664 if (!item.isEmpty()) 3665 return true; 3666 return false; 3667 } 3668 3669 /** 3670 * @return {@link #observationRequirement} (Defines observation requirements for the action to be performed, such as body weight or surface area.) 3671 */ 3672 public CanonicalType addObservationRequirementElement() {//2 3673 CanonicalType t = new CanonicalType(); 3674 if (this.observationRequirement == null) 3675 this.observationRequirement = new ArrayList<CanonicalType>(); 3676 this.observationRequirement.add(t); 3677 return t; 3678 } 3679 3680 /** 3681 * @param value {@link #observationRequirement} (Defines observation requirements for the action to be performed, such as body weight or surface area.) 3682 */ 3683 public ActivityDefinition addObservationRequirement(String value) { //1 3684 CanonicalType t = new CanonicalType(); 3685 t.setValue(value); 3686 if (this.observationRequirement == null) 3687 this.observationRequirement = new ArrayList<CanonicalType>(); 3688 this.observationRequirement.add(t); 3689 return this; 3690 } 3691 3692 /** 3693 * @param value {@link #observationRequirement} (Defines observation requirements for the action to be performed, such as body weight or surface area.) 3694 */ 3695 public boolean hasObservationRequirement(String value) { 3696 if (this.observationRequirement == null) 3697 return false; 3698 for (CanonicalType v : this.observationRequirement) 3699 if (v.getValue().equals(value)) // canonical 3700 return true; 3701 return false; 3702 } 3703 3704 /** 3705 * @return {@link #observationResultRequirement} (Defines the observations that are expected to be produced by the action.) 3706 */ 3707 public List<CanonicalType> getObservationResultRequirement() { 3708 if (this.observationResultRequirement == null) 3709 this.observationResultRequirement = new ArrayList<CanonicalType>(); 3710 return this.observationResultRequirement; 3711 } 3712 3713 /** 3714 * @return Returns a reference to <code>this</code> for easy method chaining 3715 */ 3716 public ActivityDefinition setObservationResultRequirement(List<CanonicalType> theObservationResultRequirement) { 3717 this.observationResultRequirement = theObservationResultRequirement; 3718 return this; 3719 } 3720 3721 public boolean hasObservationResultRequirement() { 3722 if (this.observationResultRequirement == null) 3723 return false; 3724 for (CanonicalType item : this.observationResultRequirement) 3725 if (!item.isEmpty()) 3726 return true; 3727 return false; 3728 } 3729 3730 /** 3731 * @return {@link #observationResultRequirement} (Defines the observations that are expected to be produced by the action.) 3732 */ 3733 public CanonicalType addObservationResultRequirementElement() {//2 3734 CanonicalType t = new CanonicalType(); 3735 if (this.observationResultRequirement == null) 3736 this.observationResultRequirement = new ArrayList<CanonicalType>(); 3737 this.observationResultRequirement.add(t); 3738 return t; 3739 } 3740 3741 /** 3742 * @param value {@link #observationResultRequirement} (Defines the observations that are expected to be produced by the action.) 3743 */ 3744 public ActivityDefinition addObservationResultRequirement(String value) { //1 3745 CanonicalType t = new CanonicalType(); 3746 t.setValue(value); 3747 if (this.observationResultRequirement == null) 3748 this.observationResultRequirement = new ArrayList<CanonicalType>(); 3749 this.observationResultRequirement.add(t); 3750 return this; 3751 } 3752 3753 /** 3754 * @param value {@link #observationResultRequirement} (Defines the observations that are expected to be produced by the action.) 3755 */ 3756 public boolean hasObservationResultRequirement(String value) { 3757 if (this.observationResultRequirement == null) 3758 return false; 3759 for (CanonicalType v : this.observationResultRequirement) 3760 if (v.getValue().equals(value)) // canonical 3761 return true; 3762 return false; 3763 } 3764 3765 /** 3766 * @return {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 3767 */ 3768 public CanonicalType getTransformElement() { 3769 if (this.transform == null) 3770 if (Configuration.errorOnAutoCreate()) 3771 throw new Error("Attempt to auto-create ActivityDefinition.transform"); 3772 else if (Configuration.doAutoCreate()) 3773 this.transform = new CanonicalType(); // bb 3774 return this.transform; 3775 } 3776 3777 public boolean hasTransformElement() { 3778 return this.transform != null && !this.transform.isEmpty(); 3779 } 3780 3781 public boolean hasTransform() { 3782 return this.transform != null && !this.transform.isEmpty(); 3783 } 3784 3785 /** 3786 * @param value {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 3787 */ 3788 public ActivityDefinition setTransformElement(CanonicalType value) { 3789 this.transform = value; 3790 return this; 3791 } 3792 3793 /** 3794 * @return A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 3795 */ 3796 public String getTransform() { 3797 return this.transform == null ? null : this.transform.getValue(); 3798 } 3799 3800 /** 3801 * @param value A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 3802 */ 3803 public ActivityDefinition setTransform(String value) { 3804 if (Utilities.noString(value)) 3805 this.transform = null; 3806 else { 3807 if (this.transform == null) 3808 this.transform = new CanonicalType(); 3809 this.transform.setValue(value); 3810 } 3811 return this; 3812 } 3813 3814 /** 3815 * @return {@link #dynamicValue} (Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.) 3816 */ 3817 public List<ActivityDefinitionDynamicValueComponent> getDynamicValue() { 3818 if (this.dynamicValue == null) 3819 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 3820 return this.dynamicValue; 3821 } 3822 3823 /** 3824 * @return Returns a reference to <code>this</code> for easy method chaining 3825 */ 3826 public ActivityDefinition setDynamicValue(List<ActivityDefinitionDynamicValueComponent> theDynamicValue) { 3827 this.dynamicValue = theDynamicValue; 3828 return this; 3829 } 3830 3831 public boolean hasDynamicValue() { 3832 if (this.dynamicValue == null) 3833 return false; 3834 for (ActivityDefinitionDynamicValueComponent item : this.dynamicValue) 3835 if (!item.isEmpty()) 3836 return true; 3837 return false; 3838 } 3839 3840 public ActivityDefinitionDynamicValueComponent addDynamicValue() { //3 3841 ActivityDefinitionDynamicValueComponent t = new ActivityDefinitionDynamicValueComponent(); 3842 if (this.dynamicValue == null) 3843 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 3844 this.dynamicValue.add(t); 3845 return t; 3846 } 3847 3848 public ActivityDefinition addDynamicValue(ActivityDefinitionDynamicValueComponent t) { //3 3849 if (t == null) 3850 return this; 3851 if (this.dynamicValue == null) 3852 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 3853 this.dynamicValue.add(t); 3854 return this; 3855 } 3856 3857 /** 3858 * @return The first repetition of repeating field {@link #dynamicValue}, creating it if it does not already exist {3} 3859 */ 3860 public ActivityDefinitionDynamicValueComponent getDynamicValueFirstRep() { 3861 if (getDynamicValue().isEmpty()) { 3862 addDynamicValue(); 3863 } 3864 return getDynamicValue().get(0); 3865 } 3866 3867 protected void listChildren(List<Property> children) { 3868 super.listChildren(children); 3869 children.add(new Property("url", "uri", "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.", 0, 1, url)); 3870 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3871 children.add(new Property("version", "string", "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version)); 3872 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3873 children.add(new Property("name", "string", "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3874 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title)); 3875 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the activity definition giving additional information about its content.", 0, 1, subtitle)); 3876 children.add(new Property("status", "code", "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3877 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3878 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)|canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject)); 3879 children.add(new Property("date", "dateTime", "The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 0, 1, date)); 3880 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition.", 0, 1, publisher)); 3881 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3882 children.add(new Property("description", "markdown", "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, description)); 3883 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3884 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the activity definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3885 children.add(new Property("purpose", "markdown", "Explanation of why this activity definition is needed and why it has been designed as it has.", 0, 1, purpose)); 3886 children.add(new Property("usage", "markdown", "A detailed description of how the activity definition is used from a clinical perspective.", 0, 1, usage)); 3887 children.add(new Property("copyright", "markdown", "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 0, 1, copyright)); 3888 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3889 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3890 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 3891 children.add(new Property("effectivePeriod", "Period", "The period during which the activity definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 3892 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 3893 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 3894 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 3895 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 3896 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 3897 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 3898 children.add(new Property("library", "canonical(Library)", "A reference to a Library resource containing any formal logic used by the activity definition.", 0, java.lang.Integer.MAX_VALUE, library)); 3899 children.add(new Property("kind", "code", "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.", 0, 1, kind)); 3900 children.add(new Property("profile", "canonical(StructureDefinition)", "A profile to which the target of the activity definition is expected to conform.", 0, 1, profile)); 3901 children.add(new Property("code", "CodeableConcept", "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 1, code)); 3902 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.", 0, 1, intent)); 3903 children.add(new Property("priority", "code", "Indicates how quickly the activity should be addressed with respect to other requests.", 0, 1, priority)); 3904 children.add(new Property("doNotPerform", "boolean", "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.", 0, 1, doNotPerform)); 3905 children.add(new Property("timing[x]", "Timing|Age|Range|Duration", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing)); 3906 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded)); 3907 children.add(new Property("location", "CodeableReference(Location)", "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location)); 3908 children.add(new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant)); 3909 children.add(new Property("product[x]", "Reference(Medication|Ingredient|Substance|SubstanceDefinition)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product)); 3910 children.add(new Property("quantity", "Quantity", "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity)); 3911 children.add(new Property("dosage", "Dosage", "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 0, java.lang.Integer.MAX_VALUE, dosage)); 3912 children.add(new Property("bodySite", "CodeableConcept", "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, java.lang.Integer.MAX_VALUE, bodySite)); 3913 children.add(new Property("specimenRequirement", "canonical(SpecimenDefinition)", "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.", 0, java.lang.Integer.MAX_VALUE, specimenRequirement)); 3914 children.add(new Property("observationRequirement", "canonical(ObservationDefinition)", "Defines observation requirements for the action to be performed, such as body weight or surface area.", 0, java.lang.Integer.MAX_VALUE, observationRequirement)); 3915 children.add(new Property("observationResultRequirement", "canonical(ObservationDefinition)", "Defines the observations that are expected to be produced by the action.", 0, java.lang.Integer.MAX_VALUE, observationResultRequirement)); 3916 children.add(new Property("transform", "canonical(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform)); 3917 children.add(new Property("dynamicValue", "", "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 3918 } 3919 3920 @Override 3921 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3922 switch (_hash) { 3923 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.", 0, 1, url); 3924 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3925 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version); 3926 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3927 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3928 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3929 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3930 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3931 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title); 3932 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the activity definition giving additional information about its content.", 0, 1, subtitle); 3933 case -892481550: /*status*/ return new Property("status", "code", "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3934 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3935 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)|canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3936 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)|canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3937 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3938 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group|MedicinalProductDefinition|SubstanceDefinition|AdministrableProductDefinition|ManufacturedItemDefinition|PackagedProductDefinition)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3939 case -1768521432: /*subjectCanonical*/ return new Property("subject[x]", "canonical(EvidenceVariable)", "A code, group definition, or canonical reference that describes or identifies the intended subject of the activity being defined. Canonical references are allowed to support the definition of protocols for drug and substance quality specifications, and is allowed to reference a MedicinalProductDefinition, SubstanceDefinition, AdministrableProductDefinition, ManufacturedItemDefinition, or PackagedProductDefinition resource.", 0, 1, subject); 3940 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the activity definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 0, 1, date); 3941 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the activity definition.", 0, 1, publisher); 3942 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3943 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, description); 3944 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3945 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the activity definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3946 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this activity definition is needed and why it has been designed as it has.", 0, 1, purpose); 3947 case 111574433: /*usage*/ return new Property("usage", "markdown", "A detailed description of how the activity definition is used from a clinical perspective.", 0, 1, usage); 3948 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 0, 1, copyright); 3949 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3950 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 3951 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 3952 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the activity definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 3953 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 3954 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 3955 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 3956 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 3957 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 3958 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 3959 case 166208699: /*library*/ return new Property("library", "canonical(Library)", "A reference to a Library resource containing any formal logic used by the activity definition.", 0, java.lang.Integer.MAX_VALUE, library); 3960 case 3292052: /*kind*/ return new Property("kind", "code", "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest.", 0, 1, kind); 3961 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A profile to which the target of the activity definition is expected to conform.", 0, 1, profile); 3962 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 1, code); 3963 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.", 0, 1, intent); 3964 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the activity should be addressed with respect to other requests.", 0, 1, priority); 3965 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.", 0, 1, doNotPerform); 3966 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Timing|Age|Range|Duration", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing); 3967 case -873664438: /*timing*/ return new Property("timing[x]", "Timing|Age|Range|Duration", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing); 3968 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing); 3969 case 164607061: /*timingAge*/ return new Property("timing[x]", "Age", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing); 3970 case -710871277: /*timingRange*/ return new Property("timing[x]", "Range", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing); 3971 case -1327253506: /*timingDuration*/ return new Property("timing[x]", "Duration", "The timing or frequency upon which the described activity is to occur.", 0, 1, timing); 3972 case -544329575: /*asNeeded[x]*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 3973 case -1432923513: /*asNeeded*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 3974 case -591717471: /*asNeededBoolean*/ return new Property("asNeeded[x]", "boolean", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 3975 case 1556420122: /*asNeededCodeableConcept*/ return new Property("asNeeded[x]", "CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 3976 case 1901043637: /*location*/ return new Property("location", "CodeableReference(Location)", "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location); 3977 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, participant); 3978 case 1753005361: /*product[x]*/ return new Property("product[x]", "Reference(Medication|Ingredient|Substance|SubstanceDefinition)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 3979 case -309474065: /*product*/ return new Property("product[x]", "Reference(Medication|Ingredient|Substance|SubstanceDefinition)|CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 3980 case -669667556: /*productReference*/ return new Property("product[x]", "Reference(Medication|Ingredient|Substance|SubstanceDefinition)", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 3981 case 906854066: /*productCodeableConcept*/ return new Property("product[x]", "CodeableConcept", "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 3982 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity); 3983 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 0, java.lang.Integer.MAX_VALUE, dosage); 3984 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, java.lang.Integer.MAX_VALUE, bodySite); 3985 case 1498467355: /*specimenRequirement*/ return new Property("specimenRequirement", "canonical(SpecimenDefinition)", "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.", 0, java.lang.Integer.MAX_VALUE, specimenRequirement); 3986 case 362354807: /*observationRequirement*/ return new Property("observationRequirement", "canonical(ObservationDefinition)", "Defines observation requirements for the action to be performed, such as body weight or surface area.", 0, java.lang.Integer.MAX_VALUE, observationRequirement); 3987 case 395230490: /*observationResultRequirement*/ return new Property("observationResultRequirement", "canonical(ObservationDefinition)", "Defines the observations that are expected to be produced by the action.", 0, java.lang.Integer.MAX_VALUE, observationResultRequirement); 3988 case 1052666732: /*transform*/ return new Property("transform", "canonical(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform); 3989 case 572625010: /*dynamicValue*/ return new Property("dynamicValue", "", "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue); 3990 default: return super.getNamedProperty(_hash, _name, _checkValid); 3991 } 3992 3993 } 3994 3995 @Override 3996 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3997 switch (hash) { 3998 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3999 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4000 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4001 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4002 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4003 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4004 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 4005 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4006 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4007 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 4008 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4009 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4010 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4011 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4012 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4013 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4014 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4015 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // MarkdownType 4016 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4017 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4018 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4019 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4020 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4021 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 4022 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4023 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4024 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4025 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4026 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4027 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 4028 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<RequestResourceTypes> 4029 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 4030 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 4031 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 4032 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 4033 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 4034 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 4035 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // DataType 4036 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // CodeableReference 4037 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // ActivityDefinitionParticipantComponent 4038 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // DataType 4039 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4040 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 4041 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 4042 case 1498467355: /*specimenRequirement*/ return this.specimenRequirement == null ? new Base[0] : this.specimenRequirement.toArray(new Base[this.specimenRequirement.size()]); // CanonicalType 4043 case 362354807: /*observationRequirement*/ return this.observationRequirement == null ? new Base[0] : this.observationRequirement.toArray(new Base[this.observationRequirement.size()]); // CanonicalType 4044 case 395230490: /*observationResultRequirement*/ return this.observationResultRequirement == null ? new Base[0] : this.observationResultRequirement.toArray(new Base[this.observationResultRequirement.size()]); // CanonicalType 4045 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // CanonicalType 4046 case 572625010: /*dynamicValue*/ return this.dynamicValue == null ? new Base[0] : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // ActivityDefinitionDynamicValueComponent 4047 default: return super.getProperty(hash, name, checkValid); 4048 } 4049 4050 } 4051 4052 @Override 4053 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4054 switch (hash) { 4055 case 116079: // url 4056 this.url = TypeConvertor.castToUri(value); // UriType 4057 return value; 4058 case -1618432855: // identifier 4059 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4060 return value; 4061 case 351608024: // version 4062 this.version = TypeConvertor.castToString(value); // StringType 4063 return value; 4064 case 1508158071: // versionAlgorithm 4065 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4066 return value; 4067 case 3373707: // name 4068 this.name = TypeConvertor.castToString(value); // StringType 4069 return value; 4070 case 110371416: // title 4071 this.title = TypeConvertor.castToString(value); // StringType 4072 return value; 4073 case -2060497896: // subtitle 4074 this.subtitle = TypeConvertor.castToString(value); // StringType 4075 return value; 4076 case -892481550: // status 4077 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4078 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4079 return value; 4080 case -404562712: // experimental 4081 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4082 return value; 4083 case -1867885268: // subject 4084 this.subject = TypeConvertor.castToType(value); // DataType 4085 return value; 4086 case 3076014: // date 4087 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4088 return value; 4089 case 1447404028: // publisher 4090 this.publisher = TypeConvertor.castToString(value); // StringType 4091 return value; 4092 case 951526432: // contact 4093 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4094 return value; 4095 case -1724546052: // description 4096 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4097 return value; 4098 case -669707736: // useContext 4099 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4100 return value; 4101 case -507075711: // jurisdiction 4102 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4103 return value; 4104 case -220463842: // purpose 4105 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4106 return value; 4107 case 111574433: // usage 4108 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 4109 return value; 4110 case 1522889671: // copyright 4111 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4112 return value; 4113 case 765157229: // copyrightLabel 4114 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4115 return value; 4116 case 223539345: // approvalDate 4117 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4118 return value; 4119 case -1687512484: // lastReviewDate 4120 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4121 return value; 4122 case -403934648: // effectivePeriod 4123 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4124 return value; 4125 case 110546223: // topic 4126 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4127 return value; 4128 case -1406328437: // author 4129 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4130 return value; 4131 case -1307827859: // editor 4132 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4133 return value; 4134 case -261190139: // reviewer 4135 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4136 return value; 4137 case 1740277666: // endorser 4138 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4139 return value; 4140 case 666807069: // relatedArtifact 4141 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4142 return value; 4143 case 166208699: // library 4144 this.getLibrary().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4145 return value; 4146 case 3292052: // kind 4147 value = new RequestResourceTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 4148 this.kind = (Enumeration) value; // Enumeration<RequestResourceTypes> 4149 return value; 4150 case -309425751: // profile 4151 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 4152 return value; 4153 case 3059181: // code 4154 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4155 return value; 4156 case -1183762788: // intent 4157 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 4158 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4159 return value; 4160 case -1165461084: // priority 4161 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4162 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4163 return value; 4164 case -1788508167: // doNotPerform 4165 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 4166 return value; 4167 case -873664438: // timing 4168 this.timing = TypeConvertor.castToType(value); // DataType 4169 return value; 4170 case -1432923513: // asNeeded 4171 this.asNeeded = TypeConvertor.castToType(value); // DataType 4172 return value; 4173 case 1901043637: // location 4174 this.location = TypeConvertor.castToCodeableReference(value); // CodeableReference 4175 return value; 4176 case 767422259: // participant 4177 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); // ActivityDefinitionParticipantComponent 4178 return value; 4179 case -309474065: // product 4180 this.product = TypeConvertor.castToType(value); // DataType 4181 return value; 4182 case -1285004149: // quantity 4183 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4184 return value; 4185 case -1326018889: // dosage 4186 this.getDosage().add(TypeConvertor.castToDosage(value)); // Dosage 4187 return value; 4188 case 1702620169: // bodySite 4189 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4190 return value; 4191 case 1498467355: // specimenRequirement 4192 this.getSpecimenRequirement().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4193 return value; 4194 case 362354807: // observationRequirement 4195 this.getObservationRequirement().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4196 return value; 4197 case 395230490: // observationResultRequirement 4198 this.getObservationResultRequirement().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4199 return value; 4200 case 1052666732: // transform 4201 this.transform = TypeConvertor.castToCanonical(value); // CanonicalType 4202 return value; 4203 case 572625010: // dynamicValue 4204 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); // ActivityDefinitionDynamicValueComponent 4205 return value; 4206 default: return super.setProperty(hash, name, value); 4207 } 4208 4209 } 4210 4211 @Override 4212 public Base setProperty(String name, Base value) throws FHIRException { 4213 if (name.equals("url")) { 4214 this.url = TypeConvertor.castToUri(value); // UriType 4215 } else if (name.equals("identifier")) { 4216 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4217 } else if (name.equals("version")) { 4218 this.version = TypeConvertor.castToString(value); // StringType 4219 } else if (name.equals("versionAlgorithm[x]")) { 4220 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4221 } else if (name.equals("name")) { 4222 this.name = TypeConvertor.castToString(value); // StringType 4223 } else if (name.equals("title")) { 4224 this.title = TypeConvertor.castToString(value); // StringType 4225 } else if (name.equals("subtitle")) { 4226 this.subtitle = TypeConvertor.castToString(value); // StringType 4227 } else if (name.equals("status")) { 4228 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4229 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4230 } else if (name.equals("experimental")) { 4231 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4232 } else if (name.equals("subject[x]")) { 4233 this.subject = TypeConvertor.castToType(value); // DataType 4234 } else if (name.equals("date")) { 4235 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4236 } else if (name.equals("publisher")) { 4237 this.publisher = TypeConvertor.castToString(value); // StringType 4238 } else if (name.equals("contact")) { 4239 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4240 } else if (name.equals("description")) { 4241 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4242 } else if (name.equals("useContext")) { 4243 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4244 } else if (name.equals("jurisdiction")) { 4245 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4246 } else if (name.equals("purpose")) { 4247 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4248 } else if (name.equals("usage")) { 4249 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 4250 } else if (name.equals("copyright")) { 4251 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4252 } else if (name.equals("copyrightLabel")) { 4253 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4254 } else if (name.equals("approvalDate")) { 4255 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4256 } else if (name.equals("lastReviewDate")) { 4257 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4258 } else if (name.equals("effectivePeriod")) { 4259 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4260 } else if (name.equals("topic")) { 4261 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 4262 } else if (name.equals("author")) { 4263 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 4264 } else if (name.equals("editor")) { 4265 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 4266 } else if (name.equals("reviewer")) { 4267 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 4268 } else if (name.equals("endorser")) { 4269 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 4270 } else if (name.equals("relatedArtifact")) { 4271 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4272 } else if (name.equals("library")) { 4273 this.getLibrary().add(TypeConvertor.castToCanonical(value)); 4274 } else if (name.equals("kind")) { 4275 value = new RequestResourceTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 4276 this.kind = (Enumeration) value; // Enumeration<RequestResourceTypes> 4277 } else if (name.equals("profile")) { 4278 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 4279 } else if (name.equals("code")) { 4280 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4281 } else if (name.equals("intent")) { 4282 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 4283 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4284 } else if (name.equals("priority")) { 4285 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4286 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4287 } else if (name.equals("doNotPerform")) { 4288 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 4289 } else if (name.equals("timing[x]")) { 4290 this.timing = TypeConvertor.castToType(value); // DataType 4291 } else if (name.equals("asNeeded[x]")) { 4292 this.asNeeded = TypeConvertor.castToType(value); // DataType 4293 } else if (name.equals("location")) { 4294 this.location = TypeConvertor.castToCodeableReference(value); // CodeableReference 4295 } else if (name.equals("participant")) { 4296 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); 4297 } else if (name.equals("product[x]")) { 4298 this.product = TypeConvertor.castToType(value); // DataType 4299 } else if (name.equals("quantity")) { 4300 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4301 } else if (name.equals("dosage")) { 4302 this.getDosage().add(TypeConvertor.castToDosage(value)); 4303 } else if (name.equals("bodySite")) { 4304 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); 4305 } else if (name.equals("specimenRequirement")) { 4306 this.getSpecimenRequirement().add(TypeConvertor.castToCanonical(value)); 4307 } else if (name.equals("observationRequirement")) { 4308 this.getObservationRequirement().add(TypeConvertor.castToCanonical(value)); 4309 } else if (name.equals("observationResultRequirement")) { 4310 this.getObservationResultRequirement().add(TypeConvertor.castToCanonical(value)); 4311 } else if (name.equals("transform")) { 4312 this.transform = TypeConvertor.castToCanonical(value); // CanonicalType 4313 } else if (name.equals("dynamicValue")) { 4314 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); 4315 } else 4316 return super.setProperty(name, value); 4317 return value; 4318 } 4319 4320 @Override 4321 public void removeChild(String name, Base value) throws FHIRException { 4322 if (name.equals("url")) { 4323 this.url = null; 4324 } else if (name.equals("identifier")) { 4325 this.getIdentifier().remove(value); 4326 } else if (name.equals("version")) { 4327 this.version = null; 4328 } else if (name.equals("versionAlgorithm[x]")) { 4329 this.versionAlgorithm = null; 4330 } else if (name.equals("name")) { 4331 this.name = null; 4332 } else if (name.equals("title")) { 4333 this.title = null; 4334 } else if (name.equals("subtitle")) { 4335 this.subtitle = null; 4336 } else if (name.equals("status")) { 4337 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4338 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4339 } else if (name.equals("experimental")) { 4340 this.experimental = null; 4341 } else if (name.equals("subject[x]")) { 4342 this.subject = null; 4343 } else if (name.equals("date")) { 4344 this.date = null; 4345 } else if (name.equals("publisher")) { 4346 this.publisher = null; 4347 } else if (name.equals("contact")) { 4348 this.getContact().remove(value); 4349 } else if (name.equals("description")) { 4350 this.description = null; 4351 } else if (name.equals("useContext")) { 4352 this.getUseContext().remove(value); 4353 } else if (name.equals("jurisdiction")) { 4354 this.getJurisdiction().remove(value); 4355 } else if (name.equals("purpose")) { 4356 this.purpose = null; 4357 } else if (name.equals("usage")) { 4358 this.usage = null; 4359 } else if (name.equals("copyright")) { 4360 this.copyright = null; 4361 } else if (name.equals("copyrightLabel")) { 4362 this.copyrightLabel = null; 4363 } else if (name.equals("approvalDate")) { 4364 this.approvalDate = null; 4365 } else if (name.equals("lastReviewDate")) { 4366 this.lastReviewDate = null; 4367 } else if (name.equals("effectivePeriod")) { 4368 this.effectivePeriod = null; 4369 } else if (name.equals("topic")) { 4370 this.getTopic().remove(value); 4371 } else if (name.equals("author")) { 4372 this.getAuthor().remove(value); 4373 } else if (name.equals("editor")) { 4374 this.getEditor().remove(value); 4375 } else if (name.equals("reviewer")) { 4376 this.getReviewer().remove(value); 4377 } else if (name.equals("endorser")) { 4378 this.getEndorser().remove(value); 4379 } else if (name.equals("relatedArtifact")) { 4380 this.getRelatedArtifact().remove(value); 4381 } else if (name.equals("library")) { 4382 this.getLibrary().remove(value); 4383 } else if (name.equals("kind")) { 4384 value = new RequestResourceTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 4385 this.kind = (Enumeration) value; // Enumeration<RequestResourceTypes> 4386 } else if (name.equals("profile")) { 4387 this.profile = null; 4388 } else if (name.equals("code")) { 4389 this.code = null; 4390 } else if (name.equals("intent")) { 4391 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 4392 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 4393 } else if (name.equals("priority")) { 4394 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4395 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4396 } else if (name.equals("doNotPerform")) { 4397 this.doNotPerform = null; 4398 } else if (name.equals("timing[x]")) { 4399 this.timing = null; 4400 } else if (name.equals("asNeeded[x]")) { 4401 this.asNeeded = null; 4402 } else if (name.equals("location")) { 4403 this.location = null; 4404 } else if (name.equals("participant")) { 4405 this.getParticipant().remove((ActivityDefinitionParticipantComponent) value); 4406 } else if (name.equals("product[x]")) { 4407 this.product = null; 4408 } else if (name.equals("quantity")) { 4409 this.quantity = null; 4410 } else if (name.equals("dosage")) { 4411 this.getDosage().remove(value); 4412 } else if (name.equals("bodySite")) { 4413 this.getBodySite().remove(value); 4414 } else if (name.equals("specimenRequirement")) { 4415 this.getSpecimenRequirement().remove(value); 4416 } else if (name.equals("observationRequirement")) { 4417 this.getObservationRequirement().remove(value); 4418 } else if (name.equals("observationResultRequirement")) { 4419 this.getObservationResultRequirement().remove(value); 4420 } else if (name.equals("transform")) { 4421 this.transform = null; 4422 } else if (name.equals("dynamicValue")) { 4423 this.getDynamicValue().remove((ActivityDefinitionDynamicValueComponent) value); 4424 } else 4425 super.removeChild(name, value); 4426 4427 } 4428 4429 @Override 4430 public Base makeProperty(int hash, String name) throws FHIRException { 4431 switch (hash) { 4432 case 116079: return getUrlElement(); 4433 case -1618432855: return addIdentifier(); 4434 case 351608024: return getVersionElement(); 4435 case -115699031: return getVersionAlgorithm(); 4436 case 1508158071: return getVersionAlgorithm(); 4437 case 3373707: return getNameElement(); 4438 case 110371416: return getTitleElement(); 4439 case -2060497896: return getSubtitleElement(); 4440 case -892481550: return getStatusElement(); 4441 case -404562712: return getExperimentalElement(); 4442 case -573640748: return getSubject(); 4443 case -1867885268: return getSubject(); 4444 case 3076014: return getDateElement(); 4445 case 1447404028: return getPublisherElement(); 4446 case 951526432: return addContact(); 4447 case -1724546052: return getDescriptionElement(); 4448 case -669707736: return addUseContext(); 4449 case -507075711: return addJurisdiction(); 4450 case -220463842: return getPurposeElement(); 4451 case 111574433: return getUsageElement(); 4452 case 1522889671: return getCopyrightElement(); 4453 case 765157229: return getCopyrightLabelElement(); 4454 case 223539345: return getApprovalDateElement(); 4455 case -1687512484: return getLastReviewDateElement(); 4456 case -403934648: return getEffectivePeriod(); 4457 case 110546223: return addTopic(); 4458 case -1406328437: return addAuthor(); 4459 case -1307827859: return addEditor(); 4460 case -261190139: return addReviewer(); 4461 case 1740277666: return addEndorser(); 4462 case 666807069: return addRelatedArtifact(); 4463 case 166208699: return addLibraryElement(); 4464 case 3292052: return getKindElement(); 4465 case -309425751: return getProfileElement(); 4466 case 3059181: return getCode(); 4467 case -1183762788: return getIntentElement(); 4468 case -1165461084: return getPriorityElement(); 4469 case -1788508167: return getDoNotPerformElement(); 4470 case 164632566: return getTiming(); 4471 case -873664438: return getTiming(); 4472 case -544329575: return getAsNeeded(); 4473 case -1432923513: return getAsNeeded(); 4474 case 1901043637: return getLocation(); 4475 case 767422259: return addParticipant(); 4476 case 1753005361: return getProduct(); 4477 case -309474065: return getProduct(); 4478 case -1285004149: return getQuantity(); 4479 case -1326018889: return addDosage(); 4480 case 1702620169: return addBodySite(); 4481 case 1498467355: return addSpecimenRequirementElement(); 4482 case 362354807: return addObservationRequirementElement(); 4483 case 395230490: return addObservationResultRequirementElement(); 4484 case 1052666732: return getTransformElement(); 4485 case 572625010: return addDynamicValue(); 4486 default: return super.makeProperty(hash, name); 4487 } 4488 4489 } 4490 4491 @Override 4492 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4493 switch (hash) { 4494 case 116079: /*url*/ return new String[] {"uri"}; 4495 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4496 case 351608024: /*version*/ return new String[] {"string"}; 4497 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4498 case 3373707: /*name*/ return new String[] {"string"}; 4499 case 110371416: /*title*/ return new String[] {"string"}; 4500 case -2060497896: /*subtitle*/ return new String[] {"string"}; 4501 case -892481550: /*status*/ return new String[] {"code"}; 4502 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4503 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference", "canonical"}; 4504 case 3076014: /*date*/ return new String[] {"dateTime"}; 4505 case 1447404028: /*publisher*/ return new String[] {"string"}; 4506 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4507 case -1724546052: /*description*/ return new String[] {"markdown"}; 4508 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4509 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4510 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4511 case 111574433: /*usage*/ return new String[] {"markdown"}; 4512 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4513 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4514 case 223539345: /*approvalDate*/ return new String[] {"date"}; 4515 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 4516 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4517 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 4518 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 4519 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 4520 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 4521 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 4522 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 4523 case 166208699: /*library*/ return new String[] {"canonical"}; 4524 case 3292052: /*kind*/ return new String[] {"code"}; 4525 case -309425751: /*profile*/ return new String[] {"canonical"}; 4526 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 4527 case -1183762788: /*intent*/ return new String[] {"code"}; 4528 case -1165461084: /*priority*/ return new String[] {"code"}; 4529 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 4530 case -873664438: /*timing*/ return new String[] {"Timing", "Age", "Range", "Duration"}; 4531 case -1432923513: /*asNeeded*/ return new String[] {"boolean", "CodeableConcept"}; 4532 case 1901043637: /*location*/ return new String[] {"CodeableReference"}; 4533 case 767422259: /*participant*/ return new String[] {}; 4534 case -309474065: /*product*/ return new String[] {"Reference", "CodeableConcept"}; 4535 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 4536 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 4537 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4538 case 1498467355: /*specimenRequirement*/ return new String[] {"canonical"}; 4539 case 362354807: /*observationRequirement*/ return new String[] {"canonical"}; 4540 case 395230490: /*observationResultRequirement*/ return new String[] {"canonical"}; 4541 case 1052666732: /*transform*/ return new String[] {"canonical"}; 4542 case 572625010: /*dynamicValue*/ return new String[] {}; 4543 default: return super.getTypesForProperty(hash, name); 4544 } 4545 4546 } 4547 4548 @Override 4549 public Base addChild(String name) throws FHIRException { 4550 if (name.equals("url")) { 4551 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.url"); 4552 } 4553 else if (name.equals("identifier")) { 4554 return addIdentifier(); 4555 } 4556 else if (name.equals("version")) { 4557 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.version"); 4558 } 4559 else if (name.equals("versionAlgorithmString")) { 4560 this.versionAlgorithm = new StringType(); 4561 return this.versionAlgorithm; 4562 } 4563 else if (name.equals("versionAlgorithmCoding")) { 4564 this.versionAlgorithm = new Coding(); 4565 return this.versionAlgorithm; 4566 } 4567 else if (name.equals("name")) { 4568 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.name"); 4569 } 4570 else if (name.equals("title")) { 4571 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.title"); 4572 } 4573 else if (name.equals("subtitle")) { 4574 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.subtitle"); 4575 } 4576 else if (name.equals("status")) { 4577 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.status"); 4578 } 4579 else if (name.equals("experimental")) { 4580 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.experimental"); 4581 } 4582 else if (name.equals("subjectCodeableConcept")) { 4583 this.subject = new CodeableConcept(); 4584 return this.subject; 4585 } 4586 else if (name.equals("subjectReference")) { 4587 this.subject = new Reference(); 4588 return this.subject; 4589 } 4590 else if (name.equals("subjectCanonical")) { 4591 this.subject = new CanonicalType(); 4592 return this.subject; 4593 } 4594 else if (name.equals("date")) { 4595 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.date"); 4596 } 4597 else if (name.equals("publisher")) { 4598 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.publisher"); 4599 } 4600 else if (name.equals("contact")) { 4601 return addContact(); 4602 } 4603 else if (name.equals("description")) { 4604 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.description"); 4605 } 4606 else if (name.equals("useContext")) { 4607 return addUseContext(); 4608 } 4609 else if (name.equals("jurisdiction")) { 4610 return addJurisdiction(); 4611 } 4612 else if (name.equals("purpose")) { 4613 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.purpose"); 4614 } 4615 else if (name.equals("usage")) { 4616 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.usage"); 4617 } 4618 else if (name.equals("copyright")) { 4619 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.copyright"); 4620 } 4621 else if (name.equals("copyrightLabel")) { 4622 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.copyrightLabel"); 4623 } 4624 else if (name.equals("approvalDate")) { 4625 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.approvalDate"); 4626 } 4627 else if (name.equals("lastReviewDate")) { 4628 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.lastReviewDate"); 4629 } 4630 else if (name.equals("effectivePeriod")) { 4631 this.effectivePeriod = new Period(); 4632 return this.effectivePeriod; 4633 } 4634 else if (name.equals("topic")) { 4635 return addTopic(); 4636 } 4637 else if (name.equals("author")) { 4638 return addAuthor(); 4639 } 4640 else if (name.equals("editor")) { 4641 return addEditor(); 4642 } 4643 else if (name.equals("reviewer")) { 4644 return addReviewer(); 4645 } 4646 else if (name.equals("endorser")) { 4647 return addEndorser(); 4648 } 4649 else if (name.equals("relatedArtifact")) { 4650 return addRelatedArtifact(); 4651 } 4652 else if (name.equals("library")) { 4653 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.library"); 4654 } 4655 else if (name.equals("kind")) { 4656 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.kind"); 4657 } 4658 else if (name.equals("profile")) { 4659 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.profile"); 4660 } 4661 else if (name.equals("code")) { 4662 this.code = new CodeableConcept(); 4663 return this.code; 4664 } 4665 else if (name.equals("intent")) { 4666 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.intent"); 4667 } 4668 else if (name.equals("priority")) { 4669 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.priority"); 4670 } 4671 else if (name.equals("doNotPerform")) { 4672 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.doNotPerform"); 4673 } 4674 else if (name.equals("timingTiming")) { 4675 this.timing = new Timing(); 4676 return this.timing; 4677 } 4678 else if (name.equals("timingAge")) { 4679 this.timing = new Age(); 4680 return this.timing; 4681 } 4682 else if (name.equals("timingRange")) { 4683 this.timing = new Range(); 4684 return this.timing; 4685 } 4686 else if (name.equals("timingDuration")) { 4687 this.timing = new Duration(); 4688 return this.timing; 4689 } 4690 else if (name.equals("asNeededBoolean")) { 4691 this.asNeeded = new BooleanType(); 4692 return this.asNeeded; 4693 } 4694 else if (name.equals("asNeededCodeableConcept")) { 4695 this.asNeeded = new CodeableConcept(); 4696 return this.asNeeded; 4697 } 4698 else if (name.equals("location")) { 4699 this.location = new CodeableReference(); 4700 return this.location; 4701 } 4702 else if (name.equals("participant")) { 4703 return addParticipant(); 4704 } 4705 else if (name.equals("productReference")) { 4706 this.product = new Reference(); 4707 return this.product; 4708 } 4709 else if (name.equals("productCodeableConcept")) { 4710 this.product = new CodeableConcept(); 4711 return this.product; 4712 } 4713 else if (name.equals("quantity")) { 4714 this.quantity = new Quantity(); 4715 return this.quantity; 4716 } 4717 else if (name.equals("dosage")) { 4718 return addDosage(); 4719 } 4720 else if (name.equals("bodySite")) { 4721 return addBodySite(); 4722 } 4723 else if (name.equals("specimenRequirement")) { 4724 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.specimenRequirement"); 4725 } 4726 else if (name.equals("observationRequirement")) { 4727 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.observationRequirement"); 4728 } 4729 else if (name.equals("observationResultRequirement")) { 4730 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.observationResultRequirement"); 4731 } 4732 else if (name.equals("transform")) { 4733 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.transform"); 4734 } 4735 else if (name.equals("dynamicValue")) { 4736 return addDynamicValue(); 4737 } 4738 else 4739 return super.addChild(name); 4740 } 4741 4742 public String fhirType() { 4743 return "ActivityDefinition"; 4744 4745 } 4746 4747 public ActivityDefinition copy() { 4748 ActivityDefinition dst = new ActivityDefinition(); 4749 copyValues(dst); 4750 return dst; 4751 } 4752 4753 public void copyValues(ActivityDefinition dst) { 4754 super.copyValues(dst); 4755 dst.url = url == null ? null : url.copy(); 4756 if (identifier != null) { 4757 dst.identifier = new ArrayList<Identifier>(); 4758 for (Identifier i : identifier) 4759 dst.identifier.add(i.copy()); 4760 }; 4761 dst.version = version == null ? null : version.copy(); 4762 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4763 dst.name = name == null ? null : name.copy(); 4764 dst.title = title == null ? null : title.copy(); 4765 dst.subtitle = subtitle == null ? null : subtitle.copy(); 4766 dst.status = status == null ? null : status.copy(); 4767 dst.experimental = experimental == null ? null : experimental.copy(); 4768 dst.subject = subject == null ? null : subject.copy(); 4769 dst.date = date == null ? null : date.copy(); 4770 dst.publisher = publisher == null ? null : publisher.copy(); 4771 if (contact != null) { 4772 dst.contact = new ArrayList<ContactDetail>(); 4773 for (ContactDetail i : contact) 4774 dst.contact.add(i.copy()); 4775 }; 4776 dst.description = description == null ? null : description.copy(); 4777 if (useContext != null) { 4778 dst.useContext = new ArrayList<UsageContext>(); 4779 for (UsageContext i : useContext) 4780 dst.useContext.add(i.copy()); 4781 }; 4782 if (jurisdiction != null) { 4783 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4784 for (CodeableConcept i : jurisdiction) 4785 dst.jurisdiction.add(i.copy()); 4786 }; 4787 dst.purpose = purpose == null ? null : purpose.copy(); 4788 dst.usage = usage == null ? null : usage.copy(); 4789 dst.copyright = copyright == null ? null : copyright.copy(); 4790 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4791 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4792 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4793 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4794 if (topic != null) { 4795 dst.topic = new ArrayList<CodeableConcept>(); 4796 for (CodeableConcept i : topic) 4797 dst.topic.add(i.copy()); 4798 }; 4799 if (author != null) { 4800 dst.author = new ArrayList<ContactDetail>(); 4801 for (ContactDetail i : author) 4802 dst.author.add(i.copy()); 4803 }; 4804 if (editor != null) { 4805 dst.editor = new ArrayList<ContactDetail>(); 4806 for (ContactDetail i : editor) 4807 dst.editor.add(i.copy()); 4808 }; 4809 if (reviewer != null) { 4810 dst.reviewer = new ArrayList<ContactDetail>(); 4811 for (ContactDetail i : reviewer) 4812 dst.reviewer.add(i.copy()); 4813 }; 4814 if (endorser != null) { 4815 dst.endorser = new ArrayList<ContactDetail>(); 4816 for (ContactDetail i : endorser) 4817 dst.endorser.add(i.copy()); 4818 }; 4819 if (relatedArtifact != null) { 4820 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4821 for (RelatedArtifact i : relatedArtifact) 4822 dst.relatedArtifact.add(i.copy()); 4823 }; 4824 if (library != null) { 4825 dst.library = new ArrayList<CanonicalType>(); 4826 for (CanonicalType i : library) 4827 dst.library.add(i.copy()); 4828 }; 4829 dst.kind = kind == null ? null : kind.copy(); 4830 dst.profile = profile == null ? null : profile.copy(); 4831 dst.code = code == null ? null : code.copy(); 4832 dst.intent = intent == null ? null : intent.copy(); 4833 dst.priority = priority == null ? null : priority.copy(); 4834 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 4835 dst.timing = timing == null ? null : timing.copy(); 4836 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 4837 dst.location = location == null ? null : location.copy(); 4838 if (participant != null) { 4839 dst.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4840 for (ActivityDefinitionParticipantComponent i : participant) 4841 dst.participant.add(i.copy()); 4842 }; 4843 dst.product = product == null ? null : product.copy(); 4844 dst.quantity = quantity == null ? null : quantity.copy(); 4845 if (dosage != null) { 4846 dst.dosage = new ArrayList<Dosage>(); 4847 for (Dosage i : dosage) 4848 dst.dosage.add(i.copy()); 4849 }; 4850 if (bodySite != null) { 4851 dst.bodySite = new ArrayList<CodeableConcept>(); 4852 for (CodeableConcept i : bodySite) 4853 dst.bodySite.add(i.copy()); 4854 }; 4855 if (specimenRequirement != null) { 4856 dst.specimenRequirement = new ArrayList<CanonicalType>(); 4857 for (CanonicalType i : specimenRequirement) 4858 dst.specimenRequirement.add(i.copy()); 4859 }; 4860 if (observationRequirement != null) { 4861 dst.observationRequirement = new ArrayList<CanonicalType>(); 4862 for (CanonicalType i : observationRequirement) 4863 dst.observationRequirement.add(i.copy()); 4864 }; 4865 if (observationResultRequirement != null) { 4866 dst.observationResultRequirement = new ArrayList<CanonicalType>(); 4867 for (CanonicalType i : observationResultRequirement) 4868 dst.observationResultRequirement.add(i.copy()); 4869 }; 4870 dst.transform = transform == null ? null : transform.copy(); 4871 if (dynamicValue != null) { 4872 dst.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4873 for (ActivityDefinitionDynamicValueComponent i : dynamicValue) 4874 dst.dynamicValue.add(i.copy()); 4875 }; 4876 } 4877 4878 protected ActivityDefinition typedCopy() { 4879 return copy(); 4880 } 4881 4882 @Override 4883 public boolean equalsDeep(Base other_) { 4884 if (!super.equalsDeep(other_)) 4885 return false; 4886 if (!(other_ instanceof ActivityDefinition)) 4887 return false; 4888 ActivityDefinition o = (ActivityDefinition) other_; 4889 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4890 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4891 && compareDeep(subtitle, o.subtitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 4892 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) 4893 && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4894 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 4895 && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 4896 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 4897 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 4898 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 4899 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 4900 && compareDeep(kind, o.kind, true) && compareDeep(profile, o.profile, true) && compareDeep(code, o.code, true) 4901 && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) && compareDeep(doNotPerform, o.doNotPerform, true) 4902 && compareDeep(timing, o.timing, true) && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(location, o.location, true) 4903 && compareDeep(participant, o.participant, true) && compareDeep(product, o.product, true) && compareDeep(quantity, o.quantity, true) 4904 && compareDeep(dosage, o.dosage, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(specimenRequirement, o.specimenRequirement, true) 4905 && compareDeep(observationRequirement, o.observationRequirement, true) && compareDeep(observationResultRequirement, o.observationResultRequirement, true) 4906 && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true) 4907 ; 4908 } 4909 4910 @Override 4911 public boolean equalsShallow(Base other_) { 4912 if (!super.equalsShallow(other_)) 4913 return false; 4914 if (!(other_ instanceof ActivityDefinition)) 4915 return false; 4916 ActivityDefinition o = (ActivityDefinition) other_; 4917 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4918 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 4919 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 4920 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) 4921 && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 4922 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 4923 && compareValues(library, o.library, true) && compareValues(kind, o.kind, true) && compareValues(profile, o.profile, true) 4924 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) && compareValues(doNotPerform, o.doNotPerform, true) 4925 && compareValues(specimenRequirement, o.specimenRequirement, true) && compareValues(observationRequirement, o.observationRequirement, true) 4926 && compareValues(observationResultRequirement, o.observationResultRequirement, true) && compareValues(transform, o.transform, true) 4927 ; 4928 } 4929 4930 public boolean isEmpty() { 4931 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4932 , versionAlgorithm, name, title, subtitle, status, experimental, subject, date 4933 , publisher, contact, description, useContext, jurisdiction, purpose, usage, copyright 4934 , copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor 4935 , reviewer, endorser, relatedArtifact, library, kind, profile, code, intent 4936 , priority, doNotPerform, timing, asNeeded, location, participant, product, quantity 4937 , dosage, bodySite, specimenRequirement, observationRequirement, observationResultRequirement 4938 , transform, dynamicValue); 4939 } 4940 4941 @Override 4942 public ResourceType getResourceType() { 4943 return ResourceType.ActivityDefinition; 4944 } 4945 4946 /** 4947 * Search parameter: <b>kind</b> 4948 * <p> 4949 * Description: <b>The kind of activity definition</b><br> 4950 * Type: <b>token</b><br> 4951 * Path: <b>ActivityDefinition.kind</b><br> 4952 * </p> 4953 */ 4954 @SearchParamDefinition(name="kind", path="ActivityDefinition.kind", description="The kind of activity definition", type="token" ) 4955 public static final String SP_KIND = "kind"; 4956 /** 4957 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 4958 * <p> 4959 * Description: <b>The kind of activity definition</b><br> 4960 * Type: <b>token</b><br> 4961 * Path: <b>ActivityDefinition.kind</b><br> 4962 * </p> 4963 */ 4964 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 4965 4966 /** 4967 * Search parameter: <b>context-quantity</b> 4968 * <p> 4969 * Description: <b>Multiple Resources: 4970 4971* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4972* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4973* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4974* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4975* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4976* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4977* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4978* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4979* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4980* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4981* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4982* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4983* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4984* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4985* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4986* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4987* [Library](library.html): A quantity- or range-valued use context assigned to the library 4988* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4989* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4990* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4991* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4992* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4993* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4994* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4995* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4996* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4997* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4998* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4999* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5000* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5001</b><br> 5002 * Type: <b>quantity</b><br> 5003 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5004 * </p> 5005 */ 5006 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5007 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5008 /** 5009 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5010 * <p> 5011 * Description: <b>Multiple Resources: 5012 5013* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5014* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5015* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5016* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5017* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5018* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5019* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5020* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5021* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5022* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5023* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5024* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5025* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5026* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5027* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5028* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5029* [Library](library.html): A quantity- or range-valued use context assigned to the library 5030* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5031* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5032* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5033* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5034* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5035* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5036* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5037* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5038* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5039* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5040* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5041* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5042* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5043</b><br> 5044 * Type: <b>quantity</b><br> 5045 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5046 * </p> 5047 */ 5048 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5049 5050 /** 5051 * Search parameter: <b>context-type-quantity</b> 5052 * <p> 5053 * Description: <b>Multiple Resources: 5054 5055* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5056* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5057* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5058* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5059* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5060* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5061* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5062* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5063* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5064* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5065* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5066* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5067* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5068* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5069* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5070* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5071* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5072* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5073* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5074* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5075* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5076* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5077* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5078* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5079* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5080* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5081* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5082* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5083* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5084* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5085</b><br> 5086 * Type: <b>composite</b><br> 5087 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5088 * </p> 5089 */ 5090 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5091 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5092 /** 5093 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5094 * <p> 5095 * Description: <b>Multiple Resources: 5096 5097* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5098* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5099* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5100* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5101* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5102* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5103* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5104* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5105* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5106* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5107* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5108* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5109* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5110* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5111* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5112* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5113* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5114* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5115* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5116* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5117* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5118* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5119* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5120* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5121* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5122* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5123* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5124* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5125* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5126* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5127</b><br> 5128 * Type: <b>composite</b><br> 5129 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5130 * </p> 5131 */ 5132 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5133 5134 /** 5135 * Search parameter: <b>context-type-value</b> 5136 * <p> 5137 * Description: <b>Multiple Resources: 5138 5139* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5140* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5141* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5142* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5143* [Citation](citation.html): A use context type and value assigned to the citation 5144* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5145* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5146* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5147* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5148* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5149* [Evidence](evidence.html): A use context type and value assigned to the evidence 5150* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5151* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5152* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5153* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5154* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5155* [Library](library.html): A use context type and value assigned to the library 5156* [Measure](measure.html): A use context type and value assigned to the measure 5157* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5158* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5159* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5160* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5161* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5162* [Requirements](requirements.html): A use context type and value assigned to the requirements 5163* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5164* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5165* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5166* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5167* [TestScript](testscript.html): A use context type and value assigned to the test script 5168* [ValueSet](valueset.html): A use context type and value assigned to the value set 5169</b><br> 5170 * Type: <b>composite</b><br> 5171 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5172 * </p> 5173 */ 5174 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 5175 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5176 /** 5177 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5178 * <p> 5179 * Description: <b>Multiple Resources: 5180 5181* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5182* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5183* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5184* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5185* [Citation](citation.html): A use context type and value assigned to the citation 5186* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5187* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5188* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5189* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5190* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5191* [Evidence](evidence.html): A use context type and value assigned to the evidence 5192* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5193* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5194* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5195* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5196* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5197* [Library](library.html): A use context type and value assigned to the library 5198* [Measure](measure.html): A use context type and value assigned to the measure 5199* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5200* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5201* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5202* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5203* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5204* [Requirements](requirements.html): A use context type and value assigned to the requirements 5205* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5206* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5207* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5208* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5209* [TestScript](testscript.html): A use context type and value assigned to the test script 5210* [ValueSet](valueset.html): A use context type and value assigned to the value set 5211</b><br> 5212 * Type: <b>composite</b><br> 5213 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5214 * </p> 5215 */ 5216 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 5217 5218 /** 5219 * Search parameter: <b>context-type</b> 5220 * <p> 5221 * Description: <b>Multiple Resources: 5222 5223* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5224* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5225* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5226* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5227* [Citation](citation.html): A type of use context assigned to the citation 5228* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5229* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5230* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5231* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5232* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5233* [Evidence](evidence.html): A type of use context assigned to the evidence 5234* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5235* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5236* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5237* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5238* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5239* [Library](library.html): A type of use context assigned to the library 5240* [Measure](measure.html): A type of use context assigned to the measure 5241* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5242* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5243* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5244* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5245* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5246* [Requirements](requirements.html): A type of use context assigned to the requirements 5247* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5248* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5249* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5250* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5251* [TestScript](testscript.html): A type of use context assigned to the test script 5252* [ValueSet](valueset.html): A type of use context assigned to the value set 5253</b><br> 5254 * Type: <b>token</b><br> 5255 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5256 * </p> 5257 */ 5258 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 5259 public static final String SP_CONTEXT_TYPE = "context-type"; 5260 /** 5261 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5262 * <p> 5263 * Description: <b>Multiple Resources: 5264 5265* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5266* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5267* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5268* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5269* [Citation](citation.html): A type of use context assigned to the citation 5270* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5271* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5272* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5273* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5274* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5275* [Evidence](evidence.html): A type of use context assigned to the evidence 5276* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5277* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5278* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5279* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5280* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5281* [Library](library.html): A type of use context assigned to the library 5282* [Measure](measure.html): A type of use context assigned to the measure 5283* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5284* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5285* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5286* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5287* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5288* [Requirements](requirements.html): A type of use context assigned to the requirements 5289* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5290* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5291* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5292* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5293* [TestScript](testscript.html): A type of use context assigned to the test script 5294* [ValueSet](valueset.html): A type of use context assigned to the value set 5295</b><br> 5296 * Type: <b>token</b><br> 5297 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5298 * </p> 5299 */ 5300 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 5301 5302 /** 5303 * Search parameter: <b>context</b> 5304 * <p> 5305 * Description: <b>Multiple Resources: 5306 5307* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5308* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5309* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5310* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5311* [Citation](citation.html): A use context assigned to the citation 5312* [CodeSystem](codesystem.html): A use context assigned to the code system 5313* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5314* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5315* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5316* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5317* [Evidence](evidence.html): A use context assigned to the evidence 5318* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5319* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5320* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5321* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5322* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5323* [Library](library.html): A use context assigned to the library 5324* [Measure](measure.html): A use context assigned to the measure 5325* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5326* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5327* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5328* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5329* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5330* [Requirements](requirements.html): A use context assigned to the requirements 5331* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5332* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5333* [StructureMap](structuremap.html): A use context assigned to the structure map 5334* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5335* [TestScript](testscript.html): A use context assigned to the test script 5336* [ValueSet](valueset.html): A use context assigned to the value set 5337</b><br> 5338 * Type: <b>token</b><br> 5339 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5340 * </p> 5341 */ 5342 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 5343 public static final String SP_CONTEXT = "context"; 5344 /** 5345 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5346 * <p> 5347 * Description: <b>Multiple Resources: 5348 5349* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5350* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5351* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5352* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5353* [Citation](citation.html): A use context assigned to the citation 5354* [CodeSystem](codesystem.html): A use context assigned to the code system 5355* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5356* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5357* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5358* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5359* [Evidence](evidence.html): A use context assigned to the evidence 5360* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5361* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5362* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5363* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5364* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5365* [Library](library.html): A use context assigned to the library 5366* [Measure](measure.html): A use context assigned to the measure 5367* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5368* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5369* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5370* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5371* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5372* [Requirements](requirements.html): A use context assigned to the requirements 5373* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5374* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5375* [StructureMap](structuremap.html): A use context assigned to the structure map 5376* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5377* [TestScript](testscript.html): A use context assigned to the test script 5378* [ValueSet](valueset.html): A use context assigned to the value set 5379</b><br> 5380 * Type: <b>token</b><br> 5381 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5382 * </p> 5383 */ 5384 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 5385 5386 /** 5387 * Search parameter: <b>date</b> 5388 * <p> 5389 * Description: <b>Multiple Resources: 5390 5391* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5392* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5393* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5394* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5395* [Citation](citation.html): The citation publication date 5396* [CodeSystem](codesystem.html): The code system publication date 5397* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5398* [ConceptMap](conceptmap.html): The concept map publication date 5399* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5400* [EventDefinition](eventdefinition.html): The event definition publication date 5401* [Evidence](evidence.html): The evidence publication date 5402* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5403* [ExampleScenario](examplescenario.html): The example scenario publication date 5404* [GraphDefinition](graphdefinition.html): The graph definition publication date 5405* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5406* [Library](library.html): The library publication date 5407* [Measure](measure.html): The measure publication date 5408* [MessageDefinition](messagedefinition.html): The message definition publication date 5409* [NamingSystem](namingsystem.html): The naming system publication date 5410* [OperationDefinition](operationdefinition.html): The operation definition publication date 5411* [PlanDefinition](plandefinition.html): The plan definition publication date 5412* [Questionnaire](questionnaire.html): The questionnaire publication date 5413* [Requirements](requirements.html): The requirements publication date 5414* [SearchParameter](searchparameter.html): The search parameter publication date 5415* [StructureDefinition](structuredefinition.html): The structure definition publication date 5416* [StructureMap](structuremap.html): The structure map publication date 5417* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5418* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5419* [TestScript](testscript.html): The test script publication date 5420* [ValueSet](valueset.html): The value set publication date 5421</b><br> 5422 * Type: <b>date</b><br> 5423 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5424 * </p> 5425 */ 5426 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 5427 public static final String SP_DATE = "date"; 5428 /** 5429 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5430 * <p> 5431 * Description: <b>Multiple Resources: 5432 5433* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5434* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5435* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5436* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5437* [Citation](citation.html): The citation publication date 5438* [CodeSystem](codesystem.html): The code system publication date 5439* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5440* [ConceptMap](conceptmap.html): The concept map publication date 5441* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5442* [EventDefinition](eventdefinition.html): The event definition publication date 5443* [Evidence](evidence.html): The evidence publication date 5444* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5445* [ExampleScenario](examplescenario.html): The example scenario publication date 5446* [GraphDefinition](graphdefinition.html): The graph definition publication date 5447* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5448* [Library](library.html): The library publication date 5449* [Measure](measure.html): The measure publication date 5450* [MessageDefinition](messagedefinition.html): The message definition publication date 5451* [NamingSystem](namingsystem.html): The naming system publication date 5452* [OperationDefinition](operationdefinition.html): The operation definition publication date 5453* [PlanDefinition](plandefinition.html): The plan definition publication date 5454* [Questionnaire](questionnaire.html): The questionnaire publication date 5455* [Requirements](requirements.html): The requirements publication date 5456* [SearchParameter](searchparameter.html): The search parameter publication date 5457* [StructureDefinition](structuredefinition.html): The structure definition publication date 5458* [StructureMap](structuremap.html): The structure map publication date 5459* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5460* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5461* [TestScript](testscript.html): The test script publication date 5462* [ValueSet](valueset.html): The value set publication date 5463</b><br> 5464 * Type: <b>date</b><br> 5465 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5466 * </p> 5467 */ 5468 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5469 5470 /** 5471 * Search parameter: <b>description</b> 5472 * <p> 5473 * Description: <b>Multiple Resources: 5474 5475* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5476* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5477* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5478* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5479* [Citation](citation.html): The description of the citation 5480* [CodeSystem](codesystem.html): The description of the code system 5481* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5482* [ConceptMap](conceptmap.html): The description of the concept map 5483* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5484* [EventDefinition](eventdefinition.html): The description of the event definition 5485* [Evidence](evidence.html): The description of the evidence 5486* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5487* [GraphDefinition](graphdefinition.html): The description of the graph definition 5488* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5489* [Library](library.html): The description of the library 5490* [Measure](measure.html): The description of the measure 5491* [MessageDefinition](messagedefinition.html): The description of the message definition 5492* [NamingSystem](namingsystem.html): The description of the naming system 5493* [OperationDefinition](operationdefinition.html): The description of the operation definition 5494* [PlanDefinition](plandefinition.html): The description of the plan definition 5495* [Questionnaire](questionnaire.html): The description of the questionnaire 5496* [Requirements](requirements.html): The description of the requirements 5497* [SearchParameter](searchparameter.html): The description of the search parameter 5498* [StructureDefinition](structuredefinition.html): The description of the structure definition 5499* [StructureMap](structuremap.html): The description of the structure map 5500* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5501* [TestScript](testscript.html): The description of the test script 5502* [ValueSet](valueset.html): The description of the value set 5503</b><br> 5504 * Type: <b>string</b><br> 5505 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5506 * </p> 5507 */ 5508 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 5509 public static final String SP_DESCRIPTION = "description"; 5510 /** 5511 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5512 * <p> 5513 * Description: <b>Multiple Resources: 5514 5515* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5516* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5517* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5518* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5519* [Citation](citation.html): The description of the citation 5520* [CodeSystem](codesystem.html): The description of the code system 5521* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5522* [ConceptMap](conceptmap.html): The description of the concept map 5523* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5524* [EventDefinition](eventdefinition.html): The description of the event definition 5525* [Evidence](evidence.html): The description of the evidence 5526* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5527* [GraphDefinition](graphdefinition.html): The description of the graph definition 5528* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5529* [Library](library.html): The description of the library 5530* [Measure](measure.html): The description of the measure 5531* [MessageDefinition](messagedefinition.html): The description of the message definition 5532* [NamingSystem](namingsystem.html): The description of the naming system 5533* [OperationDefinition](operationdefinition.html): The description of the operation definition 5534* [PlanDefinition](plandefinition.html): The description of the plan definition 5535* [Questionnaire](questionnaire.html): The description of the questionnaire 5536* [Requirements](requirements.html): The description of the requirements 5537* [SearchParameter](searchparameter.html): The description of the search parameter 5538* [StructureDefinition](structuredefinition.html): The description of the structure definition 5539* [StructureMap](structuremap.html): The description of the structure map 5540* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5541* [TestScript](testscript.html): The description of the test script 5542* [ValueSet](valueset.html): The description of the value set 5543</b><br> 5544 * Type: <b>string</b><br> 5545 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5546 * </p> 5547 */ 5548 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5549 5550 /** 5551 * Search parameter: <b>identifier</b> 5552 * <p> 5553 * Description: <b>Multiple Resources: 5554 5555* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5556* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5557* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5558* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5559* [Citation](citation.html): External identifier for the citation 5560* [CodeSystem](codesystem.html): External identifier for the code system 5561* [ConceptMap](conceptmap.html): External identifier for the concept map 5562* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5563* [EventDefinition](eventdefinition.html): External identifier for the event definition 5564* [Evidence](evidence.html): External identifier for the evidence 5565* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5566* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5567* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5568* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5569* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5570* [Library](library.html): External identifier for the library 5571* [Measure](measure.html): External identifier for the measure 5572* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5573* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5574* [NamingSystem](namingsystem.html): External identifier for the naming system 5575* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5576* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5577* [PlanDefinition](plandefinition.html): External identifier for the plan definition 5578* [Questionnaire](questionnaire.html): External identifier for the questionnaire 5579* [Requirements](requirements.html): External identifier for the requirements 5580* [SearchParameter](searchparameter.html): External identifier for the search parameter 5581* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5582* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5583* [StructureMap](structuremap.html): External identifier for the structure map 5584* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5585* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5586* [TestPlan](testplan.html): An identifier for the test plan 5587* [TestScript](testscript.html): External identifier for the test script 5588* [ValueSet](valueset.html): External identifier for the value set 5589</b><br> 5590 * Type: <b>token</b><br> 5591 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5592 * </p> 5593 */ 5594 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 5595 public static final String SP_IDENTIFIER = "identifier"; 5596 /** 5597 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5598 * <p> 5599 * Description: <b>Multiple Resources: 5600 5601* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5602* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5603* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5604* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5605* [Citation](citation.html): External identifier for the citation 5606* [CodeSystem](codesystem.html): External identifier for the code system 5607* [ConceptMap](conceptmap.html): External identifier for the concept map 5608* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5609* [EventDefinition](eventdefinition.html): External identifier for the event definition 5610* [Evidence](evidence.html): External identifier for the evidence 5611* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5612* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5613* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5614* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5615* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5616* [Library](library.html): External identifier for the library 5617* [Measure](measure.html): External identifier for the measure 5618* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5619* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5620* [NamingSystem](namingsystem.html): External identifier for the naming system 5621* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5622* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5623* [PlanDefinition](plandefinition.html): External identifier for the plan definition 5624* [Questionnaire](questionnaire.html): External identifier for the questionnaire 5625* [Requirements](requirements.html): External identifier for the requirements 5626* [SearchParameter](searchparameter.html): External identifier for the search parameter 5627* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5628* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5629* [StructureMap](structuremap.html): External identifier for the structure map 5630* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5631* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5632* [TestPlan](testplan.html): An identifier for the test plan 5633* [TestScript](testscript.html): External identifier for the test script 5634* [ValueSet](valueset.html): External identifier for the value set 5635</b><br> 5636 * Type: <b>token</b><br> 5637 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5638 * </p> 5639 */ 5640 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5641 5642 /** 5643 * Search parameter: <b>jurisdiction</b> 5644 * <p> 5645 * Description: <b>Multiple Resources: 5646 5647* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 5648* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 5649* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 5650* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 5651* [Citation](citation.html): Intended jurisdiction for the citation 5652* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 5653* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 5654* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 5655* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 5656* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 5657* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 5658* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 5659* [Library](library.html): Intended jurisdiction for the library 5660* [Measure](measure.html): Intended jurisdiction for the measure 5661* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 5662* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 5663* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 5664* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 5665* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 5666* [Requirements](requirements.html): Intended jurisdiction for the requirements 5667* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 5668* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 5669* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 5670* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 5671* [TestScript](testscript.html): Intended jurisdiction for the test script 5672* [ValueSet](valueset.html): Intended jurisdiction for the value set 5673</b><br> 5674 * Type: <b>token</b><br> 5675 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 5676 * </p> 5677 */ 5678 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 5679 public static final String SP_JURISDICTION = "jurisdiction"; 5680 /** 5681 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5682 * <p> 5683 * Description: <b>Multiple Resources: 5684 5685* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 5686* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 5687* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 5688* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 5689* [Citation](citation.html): Intended jurisdiction for the citation 5690* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 5691* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 5692* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 5693* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 5694* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 5695* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 5696* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 5697* [Library](library.html): Intended jurisdiction for the library 5698* [Measure](measure.html): Intended jurisdiction for the measure 5699* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 5700* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 5701* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 5702* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 5703* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 5704* [Requirements](requirements.html): Intended jurisdiction for the requirements 5705* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 5706* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 5707* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 5708* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 5709* [TestScript](testscript.html): Intended jurisdiction for the test script 5710* [ValueSet](valueset.html): Intended jurisdiction for the value set 5711</b><br> 5712 * Type: <b>token</b><br> 5713 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 5714 * </p> 5715 */ 5716 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 5717 5718 /** 5719 * Search parameter: <b>name</b> 5720 * <p> 5721 * Description: <b>Multiple Resources: 5722 5723* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 5724* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 5725* [Citation](citation.html): Computationally friendly name of the citation 5726* [CodeSystem](codesystem.html): Computationally friendly name of the code system 5727* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 5728* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 5729* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 5730* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 5731* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 5732* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 5733* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 5734* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 5735* [Library](library.html): Computationally friendly name of the library 5736* [Measure](measure.html): Computationally friendly name of the measure 5737* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 5738* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 5739* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 5740* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 5741* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 5742* [Requirements](requirements.html): Computationally friendly name of the requirements 5743* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 5744* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 5745* [StructureMap](structuremap.html): Computationally friendly name of the structure map 5746* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 5747* [TestScript](testscript.html): Computationally friendly name of the test script 5748* [ValueSet](valueset.html): Computationally friendly name of the value set 5749</b><br> 5750 * Type: <b>string</b><br> 5751 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 5752 * </p> 5753 */ 5754 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 5755 public static final String SP_NAME = "name"; 5756 /** 5757 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5758 * <p> 5759 * Description: <b>Multiple Resources: 5760 5761* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 5762* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 5763* [Citation](citation.html): Computationally friendly name of the citation 5764* [CodeSystem](codesystem.html): Computationally friendly name of the code system 5765* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 5766* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 5767* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 5768* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 5769* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 5770* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 5771* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 5772* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 5773* [Library](library.html): Computationally friendly name of the library 5774* [Measure](measure.html): Computationally friendly name of the measure 5775* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 5776* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 5777* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 5778* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 5779* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 5780* [Requirements](requirements.html): Computationally friendly name of the requirements 5781* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 5782* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 5783* [StructureMap](structuremap.html): Computationally friendly name of the structure map 5784* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 5785* [TestScript](testscript.html): Computationally friendly name of the test script 5786* [ValueSet](valueset.html): Computationally friendly name of the value set 5787</b><br> 5788 * Type: <b>string</b><br> 5789 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 5790 * </p> 5791 */ 5792 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 5793 5794 /** 5795 * Search parameter: <b>publisher</b> 5796 * <p> 5797 * Description: <b>Multiple Resources: 5798 5799* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5800* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5801* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5802* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5803* [Citation](citation.html): Name of the publisher of the citation 5804* [CodeSystem](codesystem.html): Name of the publisher of the code system 5805* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5806* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5807* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5808* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5809* [Evidence](evidence.html): Name of the publisher of the evidence 5810* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5811* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5812* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5813* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5814* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5815* [Library](library.html): Name of the publisher of the library 5816* [Measure](measure.html): Name of the publisher of the measure 5817* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5818* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5819* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5820* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5821* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5822* [Requirements](requirements.html): Name of the publisher of the requirements 5823* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5824* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5825* [StructureMap](structuremap.html): Name of the publisher of the structure map 5826* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5827* [TestScript](testscript.html): Name of the publisher of the test script 5828* [ValueSet](valueset.html): Name of the publisher of the value set 5829</b><br> 5830 * Type: <b>string</b><br> 5831 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5832 * </p> 5833 */ 5834 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 5835 public static final String SP_PUBLISHER = "publisher"; 5836 /** 5837 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5838 * <p> 5839 * Description: <b>Multiple Resources: 5840 5841* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5842* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5843* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5844* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5845* [Citation](citation.html): Name of the publisher of the citation 5846* [CodeSystem](codesystem.html): Name of the publisher of the code system 5847* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5848* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5849* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5850* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5851* [Evidence](evidence.html): Name of the publisher of the evidence 5852* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5853* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5854* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5855* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5856* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5857* [Library](library.html): Name of the publisher of the library 5858* [Measure](measure.html): Name of the publisher of the measure 5859* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5860* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5861* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5862* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5863* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5864* [Requirements](requirements.html): Name of the publisher of the requirements 5865* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5866* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5867* [StructureMap](structuremap.html): Name of the publisher of the structure map 5868* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5869* [TestScript](testscript.html): Name of the publisher of the test script 5870* [ValueSet](valueset.html): Name of the publisher of the value set 5871</b><br> 5872 * Type: <b>string</b><br> 5873 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5874 * </p> 5875 */ 5876 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5877 5878 /** 5879 * Search parameter: <b>status</b> 5880 * <p> 5881 * Description: <b>Multiple Resources: 5882 5883* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5884* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5885* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5886* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5887* [Citation](citation.html): The current status of the citation 5888* [CodeSystem](codesystem.html): The current status of the code system 5889* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5890* [ConceptMap](conceptmap.html): The current status of the concept map 5891* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5892* [EventDefinition](eventdefinition.html): The current status of the event definition 5893* [Evidence](evidence.html): The current status of the evidence 5894* [EvidenceReport](evidencereport.html): The current status of the evidence report 5895* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5896* [ExampleScenario](examplescenario.html): The current status of the example scenario 5897* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5898* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5899* [Library](library.html): The current status of the library 5900* [Measure](measure.html): The current status of the measure 5901* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5902* [MessageDefinition](messagedefinition.html): The current status of the message definition 5903* [NamingSystem](namingsystem.html): The current status of the naming system 5904* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5905* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5906* [PlanDefinition](plandefinition.html): The current status of the plan definition 5907* [Questionnaire](questionnaire.html): The current status of the questionnaire 5908* [Requirements](requirements.html): The current status of the requirements 5909* [SearchParameter](searchparameter.html): The current status of the search parameter 5910* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5911* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5912* [StructureMap](structuremap.html): The current status of the structure map 5913* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5914* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5915* [TestPlan](testplan.html): The current status of the test plan 5916* [TestScript](testscript.html): The current status of the test script 5917* [ValueSet](valueset.html): The current status of the value set 5918</b><br> 5919 * Type: <b>token</b><br> 5920 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5921 * </p> 5922 */ 5923 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 5924 public static final String SP_STATUS = "status"; 5925 /** 5926 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5927 * <p> 5928 * Description: <b>Multiple Resources: 5929 5930* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5931* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5932* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5933* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5934* [Citation](citation.html): The current status of the citation 5935* [CodeSystem](codesystem.html): The current status of the code system 5936* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5937* [ConceptMap](conceptmap.html): The current status of the concept map 5938* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5939* [EventDefinition](eventdefinition.html): The current status of the event definition 5940* [Evidence](evidence.html): The current status of the evidence 5941* [EvidenceReport](evidencereport.html): The current status of the evidence report 5942* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5943* [ExampleScenario](examplescenario.html): The current status of the example scenario 5944* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5945* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5946* [Library](library.html): The current status of the library 5947* [Measure](measure.html): The current status of the measure 5948* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5949* [MessageDefinition](messagedefinition.html): The current status of the message definition 5950* [NamingSystem](namingsystem.html): The current status of the naming system 5951* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5952* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5953* [PlanDefinition](plandefinition.html): The current status of the plan definition 5954* [Questionnaire](questionnaire.html): The current status of the questionnaire 5955* [Requirements](requirements.html): The current status of the requirements 5956* [SearchParameter](searchparameter.html): The current status of the search parameter 5957* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5958* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5959* [StructureMap](structuremap.html): The current status of the structure map 5960* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5961* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5962* [TestPlan](testplan.html): The current status of the test plan 5963* [TestScript](testscript.html): The current status of the test script 5964* [ValueSet](valueset.html): The current status of the value set 5965</b><br> 5966 * Type: <b>token</b><br> 5967 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5968 * </p> 5969 */ 5970 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5971 5972 /** 5973 * Search parameter: <b>title</b> 5974 * <p> 5975 * Description: <b>Multiple Resources: 5976 5977* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5978* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5979* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5980* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5981* [Citation](citation.html): The human-friendly name of the citation 5982* [CodeSystem](codesystem.html): The human-friendly name of the code system 5983* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5984* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5985* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5986* [Evidence](evidence.html): The human-friendly name of the evidence 5987* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5988* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5989* [Library](library.html): The human-friendly name of the library 5990* [Measure](measure.html): The human-friendly name of the measure 5991* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5992* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5993* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5994* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5995* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5996* [Requirements](requirements.html): The human-friendly name of the requirements 5997* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5998* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5999* [StructureMap](structuremap.html): The human-friendly name of the structure map 6000* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6001* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6002* [TestScript](testscript.html): The human-friendly name of the test script 6003* [ValueSet](valueset.html): The human-friendly name of the value set 6004</b><br> 6005 * Type: <b>string</b><br> 6006 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6007 * </p> 6008 */ 6009 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 6010 public static final String SP_TITLE = "title"; 6011 /** 6012 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6013 * <p> 6014 * Description: <b>Multiple Resources: 6015 6016* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6017* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6018* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6019* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6020* [Citation](citation.html): The human-friendly name of the citation 6021* [CodeSystem](codesystem.html): The human-friendly name of the code system 6022* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6023* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6024* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6025* [Evidence](evidence.html): The human-friendly name of the evidence 6026* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6027* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6028* [Library](library.html): The human-friendly name of the library 6029* [Measure](measure.html): The human-friendly name of the measure 6030* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6031* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6032* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6033* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6034* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6035* [Requirements](requirements.html): The human-friendly name of the requirements 6036* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6037* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6038* [StructureMap](structuremap.html): The human-friendly name of the structure map 6039* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6040* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6041* [TestScript](testscript.html): The human-friendly name of the test script 6042* [ValueSet](valueset.html): The human-friendly name of the value set 6043</b><br> 6044 * Type: <b>string</b><br> 6045 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6046 * </p> 6047 */ 6048 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6049 6050 /** 6051 * Search parameter: <b>url</b> 6052 * <p> 6053 * Description: <b>Multiple Resources: 6054 6055* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6056* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6057* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6058* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6059* [Citation](citation.html): The uri that identifies the citation 6060* [CodeSystem](codesystem.html): The uri that identifies the code system 6061* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6062* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6063* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6064* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6065* [Evidence](evidence.html): The uri that identifies the evidence 6066* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6067* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6068* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6069* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6070* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6071* [Library](library.html): The uri that identifies the library 6072* [Measure](measure.html): The uri that identifies the measure 6073* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6074* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6075* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6076* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6077* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6078* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6079* [Requirements](requirements.html): The uri that identifies the requirements 6080* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6081* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6082* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6083* [StructureMap](structuremap.html): The uri that identifies the structure map 6084* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6085* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6086* [TestPlan](testplan.html): The uri that identifies the test plan 6087* [TestScript](testscript.html): The uri that identifies the test script 6088* [ValueSet](valueset.html): The uri that identifies the value set 6089</b><br> 6090 * Type: <b>uri</b><br> 6091 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6092 * </p> 6093 */ 6094 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6095 public static final String SP_URL = "url"; 6096 /** 6097 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6098 * <p> 6099 * Description: <b>Multiple Resources: 6100 6101* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6102* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6103* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6104* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6105* [Citation](citation.html): The uri that identifies the citation 6106* [CodeSystem](codesystem.html): The uri that identifies the code system 6107* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6108* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6109* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6110* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6111* [Evidence](evidence.html): The uri that identifies the evidence 6112* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6113* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6114* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6115* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6116* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6117* [Library](library.html): The uri that identifies the library 6118* [Measure](measure.html): The uri that identifies the measure 6119* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6120* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6121* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6122* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6123* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6124* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6125* [Requirements](requirements.html): The uri that identifies the requirements 6126* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6127* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6128* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6129* [StructureMap](structuremap.html): The uri that identifies the structure map 6130* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6131* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6132* [TestPlan](testplan.html): The uri that identifies the test plan 6133* [TestScript](testscript.html): The uri that identifies the test script 6134* [ValueSet](valueset.html): The uri that identifies the value set 6135</b><br> 6136 * Type: <b>uri</b><br> 6137 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6138 * </p> 6139 */ 6140 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6141 6142 /** 6143 * Search parameter: <b>version</b> 6144 * <p> 6145 * Description: <b>Multiple Resources: 6146 6147* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6148* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6149* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6150* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6151* [Citation](citation.html): The business version of the citation 6152* [CodeSystem](codesystem.html): The business version of the code system 6153* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6154* [ConceptMap](conceptmap.html): The business version of the concept map 6155* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6156* [EventDefinition](eventdefinition.html): The business version of the event definition 6157* [Evidence](evidence.html): The business version of the evidence 6158* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6159* [ExampleScenario](examplescenario.html): The business version of the example scenario 6160* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6161* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6162* [Library](library.html): The business version of the library 6163* [Measure](measure.html): The business version of the measure 6164* [MessageDefinition](messagedefinition.html): The business version of the message definition 6165* [NamingSystem](namingsystem.html): The business version of the naming system 6166* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6167* [PlanDefinition](plandefinition.html): The business version of the plan definition 6168* [Questionnaire](questionnaire.html): The business version of the questionnaire 6169* [Requirements](requirements.html): The business version of the requirements 6170* [SearchParameter](searchparameter.html): The business version of the search parameter 6171* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6172* [StructureMap](structuremap.html): The business version of the structure map 6173* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6174* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6175* [TestScript](testscript.html): The business version of the test script 6176* [ValueSet](valueset.html): The business version of the value set 6177</b><br> 6178 * Type: <b>token</b><br> 6179 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6180 * </p> 6181 */ 6182 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 6183 public static final String SP_VERSION = "version"; 6184 /** 6185 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6186 * <p> 6187 * Description: <b>Multiple Resources: 6188 6189* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6190* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6191* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6192* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6193* [Citation](citation.html): The business version of the citation 6194* [CodeSystem](codesystem.html): The business version of the code system 6195* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6196* [ConceptMap](conceptmap.html): The business version of the concept map 6197* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6198* [EventDefinition](eventdefinition.html): The business version of the event definition 6199* [Evidence](evidence.html): The business version of the evidence 6200* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6201* [ExampleScenario](examplescenario.html): The business version of the example scenario 6202* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6203* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6204* [Library](library.html): The business version of the library 6205* [Measure](measure.html): The business version of the measure 6206* [MessageDefinition](messagedefinition.html): The business version of the message definition 6207* [NamingSystem](namingsystem.html): The business version of the naming system 6208* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6209* [PlanDefinition](plandefinition.html): The business version of the plan definition 6210* [Questionnaire](questionnaire.html): The business version of the questionnaire 6211* [Requirements](requirements.html): The business version of the requirements 6212* [SearchParameter](searchparameter.html): The business version of the search parameter 6213* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6214* [StructureMap](structuremap.html): The business version of the structure map 6215* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6216* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6217* [TestScript](testscript.html): The business version of the test script 6218* [ValueSet](valueset.html): The business version of the value set 6219</b><br> 6220 * Type: <b>token</b><br> 6221 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6222 * </p> 6223 */ 6224 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6225 6226 /** 6227 * Search parameter: <b>composed-of</b> 6228 * <p> 6229 * Description: <b>Multiple Resources: 6230 6231* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6232* [EventDefinition](eventdefinition.html): What resource is being referenced 6233* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6234* [Library](library.html): What resource is being referenced 6235* [Measure](measure.html): What resource is being referenced 6236* [PlanDefinition](plandefinition.html): What resource is being referenced 6237</b><br> 6238 * Type: <b>reference</b><br> 6239 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 6240 * </p> 6241 */ 6242 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6243 public static final String SP_COMPOSED_OF = "composed-of"; 6244 /** 6245 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 6246 * <p> 6247 * Description: <b>Multiple Resources: 6248 6249* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6250* [EventDefinition](eventdefinition.html): What resource is being referenced 6251* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6252* [Library](library.html): What resource is being referenced 6253* [Measure](measure.html): What resource is being referenced 6254* [PlanDefinition](plandefinition.html): What resource is being referenced 6255</b><br> 6256 * Type: <b>reference</b><br> 6257 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 6258 * </p> 6259 */ 6260 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 6261 6262/** 6263 * Constant for fluent queries to be used to add include statements. Specifies 6264 * the path value of "<b>ActivityDefinition:composed-of</b>". 6265 */ 6266 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("ActivityDefinition:composed-of").toLocked(); 6267 6268 /** 6269 * Search parameter: <b>depends-on</b> 6270 * <p> 6271 * Description: <b>Multiple Resources: 6272 6273* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6274* [EventDefinition](eventdefinition.html): What resource is being referenced 6275* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6276* [Library](library.html): What resource is being referenced 6277* [Measure](measure.html): What resource is being referenced 6278* [PlanDefinition](plandefinition.html): What resource is being referenced 6279</b><br> 6280 * Type: <b>reference</b><br> 6281 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 6282 * </p> 6283 */ 6284 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6285 public static final String SP_DEPENDS_ON = "depends-on"; 6286 /** 6287 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 6288 * <p> 6289 * Description: <b>Multiple Resources: 6290 6291* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6292* [EventDefinition](eventdefinition.html): What resource is being referenced 6293* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6294* [Library](library.html): What resource is being referenced 6295* [Measure](measure.html): What resource is being referenced 6296* [PlanDefinition](plandefinition.html): What resource is being referenced 6297</b><br> 6298 * Type: <b>reference</b><br> 6299 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 6300 * </p> 6301 */ 6302 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 6303 6304/** 6305 * Constant for fluent queries to be used to add include statements. Specifies 6306 * the path value of "<b>ActivityDefinition:depends-on</b>". 6307 */ 6308 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("ActivityDefinition:depends-on").toLocked(); 6309 6310 /** 6311 * Search parameter: <b>derived-from</b> 6312 * <p> 6313 * Description: <b>Multiple Resources: 6314 6315* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6316* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6317* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6318* [EventDefinition](eventdefinition.html): What resource is being referenced 6319* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6320* [Library](library.html): What resource is being referenced 6321* [Measure](measure.html): What resource is being referenced 6322* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6323* [PlanDefinition](plandefinition.html): What resource is being referenced 6324* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6325</b><br> 6326 * Type: <b>reference</b><br> 6327 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6328 * </p> 6329 */ 6330 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6331 public static final String SP_DERIVED_FROM = "derived-from"; 6332 /** 6333 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 6334 * <p> 6335 * Description: <b>Multiple Resources: 6336 6337* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6338* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6339* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6340* [EventDefinition](eventdefinition.html): What resource is being referenced 6341* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6342* [Library](library.html): What resource is being referenced 6343* [Measure](measure.html): What resource is being referenced 6344* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6345* [PlanDefinition](plandefinition.html): What resource is being referenced 6346* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6347</b><br> 6348 * Type: <b>reference</b><br> 6349 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6350 * </p> 6351 */ 6352 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 6353 6354/** 6355 * Constant for fluent queries to be used to add include statements. Specifies 6356 * the path value of "<b>ActivityDefinition:derived-from</b>". 6357 */ 6358 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ActivityDefinition:derived-from").toLocked(); 6359 6360 /** 6361 * Search parameter: <b>effective</b> 6362 * <p> 6363 * Description: <b>Multiple Resources: 6364 6365* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 6366* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 6367* [Citation](citation.html): The time during which the citation is intended to be in use 6368* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 6369* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 6370* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 6371* [Library](library.html): The time during which the library is intended to be in use 6372* [Measure](measure.html): The time during which the measure is intended to be in use 6373* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 6374* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 6375* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 6376* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 6377</b><br> 6378 * Type: <b>date</b><br> 6379 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 6380 * </p> 6381 */ 6382 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 6383 public static final String SP_EFFECTIVE = "effective"; 6384 /** 6385 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6386 * <p> 6387 * Description: <b>Multiple Resources: 6388 6389* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 6390* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 6391* [Citation](citation.html): The time during which the citation is intended to be in use 6392* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 6393* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 6394* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 6395* [Library](library.html): The time during which the library is intended to be in use 6396* [Measure](measure.html): The time during which the measure is intended to be in use 6397* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 6398* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 6399* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 6400* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 6401</b><br> 6402 * Type: <b>date</b><br> 6403 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 6404 * </p> 6405 */ 6406 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 6407 6408 /** 6409 * Search parameter: <b>predecessor</b> 6410 * <p> 6411 * Description: <b>Multiple Resources: 6412 6413* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6414* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6415* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6416* [EventDefinition](eventdefinition.html): What resource is being referenced 6417* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6418* [Library](library.html): What resource is being referenced 6419* [Measure](measure.html): What resource is being referenced 6420* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6421* [PlanDefinition](plandefinition.html): What resource is being referenced 6422* [ValueSet](valueset.html): The predecessor of the ValueSet 6423</b><br> 6424 * Type: <b>reference</b><br> 6425 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6426 * </p> 6427 */ 6428 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6429 public static final String SP_PREDECESSOR = "predecessor"; 6430 /** 6431 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6432 * <p> 6433 * Description: <b>Multiple Resources: 6434 6435* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6436* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6437* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6438* [EventDefinition](eventdefinition.html): What resource is being referenced 6439* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6440* [Library](library.html): What resource is being referenced 6441* [Measure](measure.html): What resource is being referenced 6442* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6443* [PlanDefinition](plandefinition.html): What resource is being referenced 6444* [ValueSet](valueset.html): The predecessor of the ValueSet 6445</b><br> 6446 * Type: <b>reference</b><br> 6447 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6448 * </p> 6449 */ 6450 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 6451 6452/** 6453 * Constant for fluent queries to be used to add include statements. Specifies 6454 * the path value of "<b>ActivityDefinition:predecessor</b>". 6455 */ 6456 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("ActivityDefinition:predecessor").toLocked(); 6457 6458 /** 6459 * Search parameter: <b>successor</b> 6460 * <p> 6461 * Description: <b>Multiple Resources: 6462 6463* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6464* [EventDefinition](eventdefinition.html): What resource is being referenced 6465* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6466* [Library](library.html): What resource is being referenced 6467* [Measure](measure.html): What resource is being referenced 6468* [PlanDefinition](plandefinition.html): What resource is being referenced 6469</b><br> 6470 * Type: <b>reference</b><br> 6471 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 6472 * </p> 6473 */ 6474 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6475 public static final String SP_SUCCESSOR = "successor"; 6476 /** 6477 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 6478 * <p> 6479 * Description: <b>Multiple Resources: 6480 6481* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6482* [EventDefinition](eventdefinition.html): What resource is being referenced 6483* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6484* [Library](library.html): What resource is being referenced 6485* [Measure](measure.html): What resource is being referenced 6486* [PlanDefinition](plandefinition.html): What resource is being referenced 6487</b><br> 6488 * Type: <b>reference</b><br> 6489 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 6490 * </p> 6491 */ 6492 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 6493 6494/** 6495 * Constant for fluent queries to be used to add include statements. Specifies 6496 * the path value of "<b>ActivityDefinition:successor</b>". 6497 */ 6498 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("ActivityDefinition:successor").toLocked(); 6499 6500 /** 6501 * Search parameter: <b>topic</b> 6502 * <p> 6503 * Description: <b>Multiple Resources: 6504 6505* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6506* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6507* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6508* [EventDefinition](eventdefinition.html): Topics associated with the module 6509* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6510* [Library](library.html): Topics associated with the module 6511* [Measure](measure.html): Topics associated with the measure 6512* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6513* [PlanDefinition](plandefinition.html): Topics associated with the module 6514* [ValueSet](valueset.html): Topics associated with the ValueSet 6515</b><br> 6516 * Type: <b>token</b><br> 6517 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6518 * </p> 6519 */ 6520 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 6521 public static final String SP_TOPIC = "topic"; 6522 /** 6523 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 6524 * <p> 6525 * Description: <b>Multiple Resources: 6526 6527* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6528* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6529* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6530* [EventDefinition](eventdefinition.html): Topics associated with the module 6531* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6532* [Library](library.html): Topics associated with the module 6533* [Measure](measure.html): Topics associated with the measure 6534* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6535* [PlanDefinition](plandefinition.html): Topics associated with the module 6536* [ValueSet](valueset.html): Topics associated with the ValueSet 6537</b><br> 6538 * Type: <b>token</b><br> 6539 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6540 * </p> 6541 */ 6542 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 6543 6544 6545} 6546