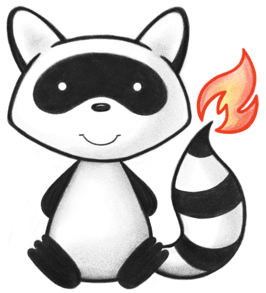
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The ActorDefinition resource is used to describe an actor - a human or an application that plays a role in data exchange, and that may have obligations associated with the role the actor plays. 052 */ 053@ResourceDef(name="ActorDefinition", profile="http://hl7.org/fhir/StructureDefinition/ActorDefinition") 054public class ActorDefinition extends CanonicalResource { 055 056 /** 057 * An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers. 058 */ 059 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Canonical identifier for this actor definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers." ) 061 protected UriType url; 062 063 /** 064 * A formal identifier that is used to identify this actor definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 065 */ 066 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 067 @Description(shortDefinition="Additional identifier for the actor definition (business identifier)", formalDefinition="A formal identifier that is used to identify this actor definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 068 protected List<Identifier> identifier; 069 070 /** 071 * The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 072 */ 073 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Business version of the actor definition", formalDefinition="The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 075 protected StringType version; 076 077 /** 078 * Indicates the mechanism used to compare versions to determine which is more current. 079 */ 080 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 083 protected DataType versionAlgorithm; 084 085 /** 086 * A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 087 */ 088 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Name for this actor definition (computer friendly)", formalDefinition="A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 090 protected StringType name; 091 092 /** 093 * A short, descriptive, user-friendly title for the actor definition. 094 */ 095 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 096 @Description(shortDefinition="Name for this actor definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the actor definition." ) 097 protected StringType title; 098 099 /** 100 * The status of this actor definition. Enables tracking the life-cycle of the content. 101 */ 102 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 103 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this actor definition. Enables tracking the life-cycle of the content." ) 104 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 105 protected Enumeration<PublicationStatus> status; 106 107 /** 108 * A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 109 */ 110 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 111 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 112 protected BooleanType experimental; 113 114 /** 115 * The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes. 116 */ 117 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 118 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes." ) 119 protected DateTimeType date; 120 121 /** 122 * The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition. 123 */ 124 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 125 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition." ) 126 protected StringType publisher; 127 128 /** 129 * Contact details to assist a user in finding and communicating with the publisher. 130 */ 131 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 132 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 133 protected List<ContactDetail> contact; 134 135 /** 136 * A free text natural language description of the actor. 137 */ 138 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 139 @Description(shortDefinition="Natural language description of the actor", formalDefinition="A free text natural language description of the actor." ) 140 protected MarkdownType description; 141 142 /** 143 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate actor definition instances. 144 */ 145 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 146 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate actor definition instances." ) 147 protected List<UsageContext> useContext; 148 149 /** 150 * A legal or geographic region in which the actor definition is intended to be used. 151 */ 152 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 153 @Description(shortDefinition="Intended jurisdiction for actor definition (if applicable)", formalDefinition="A legal or geographic region in which the actor definition is intended to be used." ) 154 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 155 protected List<CodeableConcept> jurisdiction; 156 157 /** 158 * Explanation of why this actor definition is needed and why it has been designed as it has. 159 */ 160 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 161 @Description(shortDefinition="Why this actor definition is defined", formalDefinition="Explanation of why this actor definition is needed and why it has been designed as it has." ) 162 protected MarkdownType purpose; 163 164 /** 165 * A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition. 166 */ 167 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 168 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition." ) 169 protected MarkdownType copyright; 170 171 /** 172 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 173 */ 174 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 175 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 176 protected StringType copyrightLabel; 177 178 /** 179 * Whether the actor represents a human or an appliction. 180 */ 181 @Child(name = "type", type = {CodeType.class}, order=17, min=1, max=1, modifier=false, summary=true) 182 @Description(shortDefinition="person | system", formalDefinition="Whether the actor represents a human or an appliction." ) 183 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/examplescenario-actor-type") 184 protected Enumeration<ExampleScenarioActorType> type; 185 186 /** 187 * Documentation about the functionality of the actor. 188 */ 189 @Child(name = "documentation", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Functionality associated with the actor", formalDefinition="Documentation about the functionality of the actor." ) 191 protected MarkdownType documentation; 192 193 /** 194 * A reference to additional documentation about the actor, but description and documentation. 195 */ 196 @Child(name = "reference", type = {UrlType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 197 @Description(shortDefinition="Reference to more information about the actor", formalDefinition="A reference to additional documentation about the actor, but description and documentation." ) 198 protected List<UrlType> reference; 199 200 /** 201 * The capability statement for the actor (if the concept is applicable). 202 */ 203 @Child(name = "capabilities", type = {CanonicalType.class}, order=20, min=0, max=1, modifier=false, summary=false) 204 @Description(shortDefinition="CapabilityStatement for the actor (if applicable)", formalDefinition="The capability statement for the actor (if the concept is applicable)." ) 205 protected CanonicalType capabilities; 206 207 /** 208 * A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG. 209 */ 210 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 211 @Description(shortDefinition="Definition of this actor in another context / IG", formalDefinition="A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG." ) 212 protected List<CanonicalType> derivedFrom; 213 214 private static final long serialVersionUID = -493373068L; 215 216 /** 217 * Constructor 218 */ 219 public ActorDefinition() { 220 super(); 221 } 222 223 /** 224 * Constructor 225 */ 226 public ActorDefinition(PublicationStatus status, ExampleScenarioActorType type) { 227 super(); 228 this.setStatus(status); 229 this.setType(type); 230 } 231 232 /** 233 * @return {@link #url} (An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 234 */ 235 public UriType getUrlElement() { 236 if (this.url == null) 237 if (Configuration.errorOnAutoCreate()) 238 throw new Error("Attempt to auto-create ActorDefinition.url"); 239 else if (Configuration.doAutoCreate()) 240 this.url = new UriType(); // bb 241 return this.url; 242 } 243 244 public boolean hasUrlElement() { 245 return this.url != null && !this.url.isEmpty(); 246 } 247 248 public boolean hasUrl() { 249 return this.url != null && !this.url.isEmpty(); 250 } 251 252 /** 253 * @param value {@link #url} (An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 254 */ 255 public ActorDefinition setUrlElement(UriType value) { 256 this.url = value; 257 return this; 258 } 259 260 /** 261 * @return An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers. 262 */ 263 public String getUrl() { 264 return this.url == null ? null : this.url.getValue(); 265 } 266 267 /** 268 * @param value An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers. 269 */ 270 public ActorDefinition setUrl(String value) { 271 if (Utilities.noString(value)) 272 this.url = null; 273 else { 274 if (this.url == null) 275 this.url = new UriType(); 276 this.url.setValue(value); 277 } 278 return this; 279 } 280 281 /** 282 * @return {@link #identifier} (A formal identifier that is used to identify this actor definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 283 */ 284 public List<Identifier> getIdentifier() { 285 if (this.identifier == null) 286 this.identifier = new ArrayList<Identifier>(); 287 return this.identifier; 288 } 289 290 /** 291 * @return Returns a reference to <code>this</code> for easy method chaining 292 */ 293 public ActorDefinition setIdentifier(List<Identifier> theIdentifier) { 294 this.identifier = theIdentifier; 295 return this; 296 } 297 298 public boolean hasIdentifier() { 299 if (this.identifier == null) 300 return false; 301 for (Identifier item : this.identifier) 302 if (!item.isEmpty()) 303 return true; 304 return false; 305 } 306 307 public Identifier addIdentifier() { //3 308 Identifier t = new Identifier(); 309 if (this.identifier == null) 310 this.identifier = new ArrayList<Identifier>(); 311 this.identifier.add(t); 312 return t; 313 } 314 315 public ActorDefinition addIdentifier(Identifier t) { //3 316 if (t == null) 317 return this; 318 if (this.identifier == null) 319 this.identifier = new ArrayList<Identifier>(); 320 this.identifier.add(t); 321 return this; 322 } 323 324 /** 325 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 326 */ 327 public Identifier getIdentifierFirstRep() { 328 if (getIdentifier().isEmpty()) { 329 addIdentifier(); 330 } 331 return getIdentifier().get(0); 332 } 333 334 /** 335 * @return {@link #version} (The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 336 */ 337 public StringType getVersionElement() { 338 if (this.version == null) 339 if (Configuration.errorOnAutoCreate()) 340 throw new Error("Attempt to auto-create ActorDefinition.version"); 341 else if (Configuration.doAutoCreate()) 342 this.version = new StringType(); // bb 343 return this.version; 344 } 345 346 public boolean hasVersionElement() { 347 return this.version != null && !this.version.isEmpty(); 348 } 349 350 public boolean hasVersion() { 351 return this.version != null && !this.version.isEmpty(); 352 } 353 354 /** 355 * @param value {@link #version} (The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 356 */ 357 public ActorDefinition setVersionElement(StringType value) { 358 this.version = value; 359 return this; 360 } 361 362 /** 363 * @return The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 364 */ 365 public String getVersion() { 366 return this.version == null ? null : this.version.getValue(); 367 } 368 369 /** 370 * @param value The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 371 */ 372 public ActorDefinition setVersion(String value) { 373 if (Utilities.noString(value)) 374 this.version = null; 375 else { 376 if (this.version == null) 377 this.version = new StringType(); 378 this.version.setValue(value); 379 } 380 return this; 381 } 382 383 /** 384 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 385 */ 386 public DataType getVersionAlgorithm() { 387 return this.versionAlgorithm; 388 } 389 390 /** 391 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 392 */ 393 public StringType getVersionAlgorithmStringType() throws FHIRException { 394 if (this.versionAlgorithm == null) 395 this.versionAlgorithm = new StringType(); 396 if (!(this.versionAlgorithm instanceof StringType)) 397 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 398 return (StringType) this.versionAlgorithm; 399 } 400 401 public boolean hasVersionAlgorithmStringType() { 402 return this != null && this.versionAlgorithm instanceof StringType; 403 } 404 405 /** 406 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 407 */ 408 public Coding getVersionAlgorithmCoding() throws FHIRException { 409 if (this.versionAlgorithm == null) 410 this.versionAlgorithm = new Coding(); 411 if (!(this.versionAlgorithm instanceof Coding)) 412 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 413 return (Coding) this.versionAlgorithm; 414 } 415 416 public boolean hasVersionAlgorithmCoding() { 417 return this != null && this.versionAlgorithm instanceof Coding; 418 } 419 420 public boolean hasVersionAlgorithm() { 421 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 426 */ 427 public ActorDefinition setVersionAlgorithm(DataType value) { 428 if (value != null && !(value instanceof StringType || value instanceof Coding)) 429 throw new FHIRException("Not the right type for ActorDefinition.versionAlgorithm[x]: "+value.fhirType()); 430 this.versionAlgorithm = value; 431 return this; 432 } 433 434 /** 435 * @return {@link #name} (A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 436 */ 437 public StringType getNameElement() { 438 if (this.name == null) 439 if (Configuration.errorOnAutoCreate()) 440 throw new Error("Attempt to auto-create ActorDefinition.name"); 441 else if (Configuration.doAutoCreate()) 442 this.name = new StringType(); // bb 443 return this.name; 444 } 445 446 public boolean hasNameElement() { 447 return this.name != null && !this.name.isEmpty(); 448 } 449 450 public boolean hasName() { 451 return this.name != null && !this.name.isEmpty(); 452 } 453 454 /** 455 * @param value {@link #name} (A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 456 */ 457 public ActorDefinition setNameElement(StringType value) { 458 this.name = value; 459 return this; 460 } 461 462 /** 463 * @return A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 464 */ 465 public String getName() { 466 return this.name == null ? null : this.name.getValue(); 467 } 468 469 /** 470 * @param value A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 471 */ 472 public ActorDefinition setName(String value) { 473 if (Utilities.noString(value)) 474 this.name = null; 475 else { 476 if (this.name == null) 477 this.name = new StringType(); 478 this.name.setValue(value); 479 } 480 return this; 481 } 482 483 /** 484 * @return {@link #title} (A short, descriptive, user-friendly title for the actor definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 485 */ 486 public StringType getTitleElement() { 487 if (this.title == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create ActorDefinition.title"); 490 else if (Configuration.doAutoCreate()) 491 this.title = new StringType(); // bb 492 return this.title; 493 } 494 495 public boolean hasTitleElement() { 496 return this.title != null && !this.title.isEmpty(); 497 } 498 499 public boolean hasTitle() { 500 return this.title != null && !this.title.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #title} (A short, descriptive, user-friendly title for the actor definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 505 */ 506 public ActorDefinition setTitleElement(StringType value) { 507 this.title = value; 508 return this; 509 } 510 511 /** 512 * @return A short, descriptive, user-friendly title for the actor definition. 513 */ 514 public String getTitle() { 515 return this.title == null ? null : this.title.getValue(); 516 } 517 518 /** 519 * @param value A short, descriptive, user-friendly title for the actor definition. 520 */ 521 public ActorDefinition setTitle(String value) { 522 if (Utilities.noString(value)) 523 this.title = null; 524 else { 525 if (this.title == null) 526 this.title = new StringType(); 527 this.title.setValue(value); 528 } 529 return this; 530 } 531 532 /** 533 * @return {@link #status} (The status of this actor definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 534 */ 535 public Enumeration<PublicationStatus> getStatusElement() { 536 if (this.status == null) 537 if (Configuration.errorOnAutoCreate()) 538 throw new Error("Attempt to auto-create ActorDefinition.status"); 539 else if (Configuration.doAutoCreate()) 540 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 541 return this.status; 542 } 543 544 public boolean hasStatusElement() { 545 return this.status != null && !this.status.isEmpty(); 546 } 547 548 public boolean hasStatus() { 549 return this.status != null && !this.status.isEmpty(); 550 } 551 552 /** 553 * @param value {@link #status} (The status of this actor definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 554 */ 555 public ActorDefinition setStatusElement(Enumeration<PublicationStatus> value) { 556 this.status = value; 557 return this; 558 } 559 560 /** 561 * @return The status of this actor definition. Enables tracking the life-cycle of the content. 562 */ 563 public PublicationStatus getStatus() { 564 return this.status == null ? null : this.status.getValue(); 565 } 566 567 /** 568 * @param value The status of this actor definition. Enables tracking the life-cycle of the content. 569 */ 570 public ActorDefinition setStatus(PublicationStatus value) { 571 if (this.status == null) 572 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 573 this.status.setValue(value); 574 return this; 575 } 576 577 /** 578 * @return {@link #experimental} (A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 579 */ 580 public BooleanType getExperimentalElement() { 581 if (this.experimental == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create ActorDefinition.experimental"); 584 else if (Configuration.doAutoCreate()) 585 this.experimental = new BooleanType(); // bb 586 return this.experimental; 587 } 588 589 public boolean hasExperimentalElement() { 590 return this.experimental != null && !this.experimental.isEmpty(); 591 } 592 593 public boolean hasExperimental() { 594 return this.experimental != null && !this.experimental.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #experimental} (A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 599 */ 600 public ActorDefinition setExperimentalElement(BooleanType value) { 601 this.experimental = value; 602 return this; 603 } 604 605 /** 606 * @return A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 607 */ 608 public boolean getExperimental() { 609 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 610 } 611 612 /** 613 * @param value A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 614 */ 615 public ActorDefinition setExperimental(boolean value) { 616 if (this.experimental == null) 617 this.experimental = new BooleanType(); 618 this.experimental.setValue(value); 619 return this; 620 } 621 622 /** 623 * @return {@link #date} (The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 624 */ 625 public DateTimeType getDateElement() { 626 if (this.date == null) 627 if (Configuration.errorOnAutoCreate()) 628 throw new Error("Attempt to auto-create ActorDefinition.date"); 629 else if (Configuration.doAutoCreate()) 630 this.date = new DateTimeType(); // bb 631 return this.date; 632 } 633 634 public boolean hasDateElement() { 635 return this.date != null && !this.date.isEmpty(); 636 } 637 638 public boolean hasDate() { 639 return this.date != null && !this.date.isEmpty(); 640 } 641 642 /** 643 * @param value {@link #date} (The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 644 */ 645 public ActorDefinition setDateElement(DateTimeType value) { 646 this.date = value; 647 return this; 648 } 649 650 /** 651 * @return The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes. 652 */ 653 public Date getDate() { 654 return this.date == null ? null : this.date.getValue(); 655 } 656 657 /** 658 * @param value The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes. 659 */ 660 public ActorDefinition setDate(Date value) { 661 if (value == null) 662 this.date = null; 663 else { 664 if (this.date == null) 665 this.date = new DateTimeType(); 666 this.date.setValue(value); 667 } 668 return this; 669 } 670 671 /** 672 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 673 */ 674 public StringType getPublisherElement() { 675 if (this.publisher == null) 676 if (Configuration.errorOnAutoCreate()) 677 throw new Error("Attempt to auto-create ActorDefinition.publisher"); 678 else if (Configuration.doAutoCreate()) 679 this.publisher = new StringType(); // bb 680 return this.publisher; 681 } 682 683 public boolean hasPublisherElement() { 684 return this.publisher != null && !this.publisher.isEmpty(); 685 } 686 687 public boolean hasPublisher() { 688 return this.publisher != null && !this.publisher.isEmpty(); 689 } 690 691 /** 692 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 693 */ 694 public ActorDefinition setPublisherElement(StringType value) { 695 this.publisher = value; 696 return this; 697 } 698 699 /** 700 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition. 701 */ 702 public String getPublisher() { 703 return this.publisher == null ? null : this.publisher.getValue(); 704 } 705 706 /** 707 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition. 708 */ 709 public ActorDefinition setPublisher(String value) { 710 if (Utilities.noString(value)) 711 this.publisher = null; 712 else { 713 if (this.publisher == null) 714 this.publisher = new StringType(); 715 this.publisher.setValue(value); 716 } 717 return this; 718 } 719 720 /** 721 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 722 */ 723 public List<ContactDetail> getContact() { 724 if (this.contact == null) 725 this.contact = new ArrayList<ContactDetail>(); 726 return this.contact; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public ActorDefinition setContact(List<ContactDetail> theContact) { 733 this.contact = theContact; 734 return this; 735 } 736 737 public boolean hasContact() { 738 if (this.contact == null) 739 return false; 740 for (ContactDetail item : this.contact) 741 if (!item.isEmpty()) 742 return true; 743 return false; 744 } 745 746 public ContactDetail addContact() { //3 747 ContactDetail t = new ContactDetail(); 748 if (this.contact == null) 749 this.contact = new ArrayList<ContactDetail>(); 750 this.contact.add(t); 751 return t; 752 } 753 754 public ActorDefinition addContact(ContactDetail t) { //3 755 if (t == null) 756 return this; 757 if (this.contact == null) 758 this.contact = new ArrayList<ContactDetail>(); 759 this.contact.add(t); 760 return this; 761 } 762 763 /** 764 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 765 */ 766 public ContactDetail getContactFirstRep() { 767 if (getContact().isEmpty()) { 768 addContact(); 769 } 770 return getContact().get(0); 771 } 772 773 /** 774 * @return {@link #description} (A free text natural language description of the actor.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 775 */ 776 public MarkdownType getDescriptionElement() { 777 if (this.description == null) 778 if (Configuration.errorOnAutoCreate()) 779 throw new Error("Attempt to auto-create ActorDefinition.description"); 780 else if (Configuration.doAutoCreate()) 781 this.description = new MarkdownType(); // bb 782 return this.description; 783 } 784 785 public boolean hasDescriptionElement() { 786 return this.description != null && !this.description.isEmpty(); 787 } 788 789 public boolean hasDescription() { 790 return this.description != null && !this.description.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #description} (A free text natural language description of the actor.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 795 */ 796 public ActorDefinition setDescriptionElement(MarkdownType value) { 797 this.description = value; 798 return this; 799 } 800 801 /** 802 * @return A free text natural language description of the actor. 803 */ 804 public String getDescription() { 805 return this.description == null ? null : this.description.getValue(); 806 } 807 808 /** 809 * @param value A free text natural language description of the actor. 810 */ 811 public ActorDefinition setDescription(String value) { 812 if (Utilities.noString(value)) 813 this.description = null; 814 else { 815 if (this.description == null) 816 this.description = new MarkdownType(); 817 this.description.setValue(value); 818 } 819 return this; 820 } 821 822 /** 823 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate actor definition instances.) 824 */ 825 public List<UsageContext> getUseContext() { 826 if (this.useContext == null) 827 this.useContext = new ArrayList<UsageContext>(); 828 return this.useContext; 829 } 830 831 /** 832 * @return Returns a reference to <code>this</code> for easy method chaining 833 */ 834 public ActorDefinition setUseContext(List<UsageContext> theUseContext) { 835 this.useContext = theUseContext; 836 return this; 837 } 838 839 public boolean hasUseContext() { 840 if (this.useContext == null) 841 return false; 842 for (UsageContext item : this.useContext) 843 if (!item.isEmpty()) 844 return true; 845 return false; 846 } 847 848 public UsageContext addUseContext() { //3 849 UsageContext t = new UsageContext(); 850 if (this.useContext == null) 851 this.useContext = new ArrayList<UsageContext>(); 852 this.useContext.add(t); 853 return t; 854 } 855 856 public ActorDefinition addUseContext(UsageContext t) { //3 857 if (t == null) 858 return this; 859 if (this.useContext == null) 860 this.useContext = new ArrayList<UsageContext>(); 861 this.useContext.add(t); 862 return this; 863 } 864 865 /** 866 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 867 */ 868 public UsageContext getUseContextFirstRep() { 869 if (getUseContext().isEmpty()) { 870 addUseContext(); 871 } 872 return getUseContext().get(0); 873 } 874 875 /** 876 * @return {@link #jurisdiction} (A legal or geographic region in which the actor definition is intended to be used.) 877 */ 878 public List<CodeableConcept> getJurisdiction() { 879 if (this.jurisdiction == null) 880 this.jurisdiction = new ArrayList<CodeableConcept>(); 881 return this.jurisdiction; 882 } 883 884 /** 885 * @return Returns a reference to <code>this</code> for easy method chaining 886 */ 887 public ActorDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 888 this.jurisdiction = theJurisdiction; 889 return this; 890 } 891 892 public boolean hasJurisdiction() { 893 if (this.jurisdiction == null) 894 return false; 895 for (CodeableConcept item : this.jurisdiction) 896 if (!item.isEmpty()) 897 return true; 898 return false; 899 } 900 901 public CodeableConcept addJurisdiction() { //3 902 CodeableConcept t = new CodeableConcept(); 903 if (this.jurisdiction == null) 904 this.jurisdiction = new ArrayList<CodeableConcept>(); 905 this.jurisdiction.add(t); 906 return t; 907 } 908 909 public ActorDefinition addJurisdiction(CodeableConcept t) { //3 910 if (t == null) 911 return this; 912 if (this.jurisdiction == null) 913 this.jurisdiction = new ArrayList<CodeableConcept>(); 914 this.jurisdiction.add(t); 915 return this; 916 } 917 918 /** 919 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 920 */ 921 public CodeableConcept getJurisdictionFirstRep() { 922 if (getJurisdiction().isEmpty()) { 923 addJurisdiction(); 924 } 925 return getJurisdiction().get(0); 926 } 927 928 /** 929 * @return {@link #purpose} (Explanation of why this actor definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 930 */ 931 public MarkdownType getPurposeElement() { 932 if (this.purpose == null) 933 if (Configuration.errorOnAutoCreate()) 934 throw new Error("Attempt to auto-create ActorDefinition.purpose"); 935 else if (Configuration.doAutoCreate()) 936 this.purpose = new MarkdownType(); // bb 937 return this.purpose; 938 } 939 940 public boolean hasPurposeElement() { 941 return this.purpose != null && !this.purpose.isEmpty(); 942 } 943 944 public boolean hasPurpose() { 945 return this.purpose != null && !this.purpose.isEmpty(); 946 } 947 948 /** 949 * @param value {@link #purpose} (Explanation of why this actor definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 950 */ 951 public ActorDefinition setPurposeElement(MarkdownType value) { 952 this.purpose = value; 953 return this; 954 } 955 956 /** 957 * @return Explanation of why this actor definition is needed and why it has been designed as it has. 958 */ 959 public String getPurpose() { 960 return this.purpose == null ? null : this.purpose.getValue(); 961 } 962 963 /** 964 * @param value Explanation of why this actor definition is needed and why it has been designed as it has. 965 */ 966 public ActorDefinition setPurpose(String value) { 967 if (Utilities.noString(value)) 968 this.purpose = null; 969 else { 970 if (this.purpose == null) 971 this.purpose = new MarkdownType(); 972 this.purpose.setValue(value); 973 } 974 return this; 975 } 976 977 /** 978 * @return {@link #copyright} (A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 979 */ 980 public MarkdownType getCopyrightElement() { 981 if (this.copyright == null) 982 if (Configuration.errorOnAutoCreate()) 983 throw new Error("Attempt to auto-create ActorDefinition.copyright"); 984 else if (Configuration.doAutoCreate()) 985 this.copyright = new MarkdownType(); // bb 986 return this.copyright; 987 } 988 989 public boolean hasCopyrightElement() { 990 return this.copyright != null && !this.copyright.isEmpty(); 991 } 992 993 public boolean hasCopyright() { 994 return this.copyright != null && !this.copyright.isEmpty(); 995 } 996 997 /** 998 * @param value {@link #copyright} (A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 999 */ 1000 public ActorDefinition setCopyrightElement(MarkdownType value) { 1001 this.copyright = value; 1002 return this; 1003 } 1004 1005 /** 1006 * @return A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition. 1007 */ 1008 public String getCopyright() { 1009 return this.copyright == null ? null : this.copyright.getValue(); 1010 } 1011 1012 /** 1013 * @param value A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition. 1014 */ 1015 public ActorDefinition setCopyright(String value) { 1016 if (Utilities.noString(value)) 1017 this.copyright = null; 1018 else { 1019 if (this.copyright == null) 1020 this.copyright = new MarkdownType(); 1021 this.copyright.setValue(value); 1022 } 1023 return this; 1024 } 1025 1026 /** 1027 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1028 */ 1029 public StringType getCopyrightLabelElement() { 1030 if (this.copyrightLabel == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create ActorDefinition.copyrightLabel"); 1033 else if (Configuration.doAutoCreate()) 1034 this.copyrightLabel = new StringType(); // bb 1035 return this.copyrightLabel; 1036 } 1037 1038 public boolean hasCopyrightLabelElement() { 1039 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1040 } 1041 1042 public boolean hasCopyrightLabel() { 1043 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1048 */ 1049 public ActorDefinition setCopyrightLabelElement(StringType value) { 1050 this.copyrightLabel = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1056 */ 1057 public String getCopyrightLabel() { 1058 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1059 } 1060 1061 /** 1062 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1063 */ 1064 public ActorDefinition setCopyrightLabel(String value) { 1065 if (Utilities.noString(value)) 1066 this.copyrightLabel = null; 1067 else { 1068 if (this.copyrightLabel == null) 1069 this.copyrightLabel = new StringType(); 1070 this.copyrightLabel.setValue(value); 1071 } 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #type} (Whether the actor represents a human or an appliction.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1077 */ 1078 public Enumeration<ExampleScenarioActorType> getTypeElement() { 1079 if (this.type == null) 1080 if (Configuration.errorOnAutoCreate()) 1081 throw new Error("Attempt to auto-create ActorDefinition.type"); 1082 else if (Configuration.doAutoCreate()) 1083 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); // bb 1084 return this.type; 1085 } 1086 1087 public boolean hasTypeElement() { 1088 return this.type != null && !this.type.isEmpty(); 1089 } 1090 1091 public boolean hasType() { 1092 return this.type != null && !this.type.isEmpty(); 1093 } 1094 1095 /** 1096 * @param value {@link #type} (Whether the actor represents a human or an appliction.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1097 */ 1098 public ActorDefinition setTypeElement(Enumeration<ExampleScenarioActorType> value) { 1099 this.type = value; 1100 return this; 1101 } 1102 1103 /** 1104 * @return Whether the actor represents a human or an appliction. 1105 */ 1106 public ExampleScenarioActorType getType() { 1107 return this.type == null ? null : this.type.getValue(); 1108 } 1109 1110 /** 1111 * @param value Whether the actor represents a human or an appliction. 1112 */ 1113 public ActorDefinition setType(ExampleScenarioActorType value) { 1114 if (this.type == null) 1115 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); 1116 this.type.setValue(value); 1117 return this; 1118 } 1119 1120 /** 1121 * @return {@link #documentation} (Documentation about the functionality of the actor.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1122 */ 1123 public MarkdownType getDocumentationElement() { 1124 if (this.documentation == null) 1125 if (Configuration.errorOnAutoCreate()) 1126 throw new Error("Attempt to auto-create ActorDefinition.documentation"); 1127 else if (Configuration.doAutoCreate()) 1128 this.documentation = new MarkdownType(); // bb 1129 return this.documentation; 1130 } 1131 1132 public boolean hasDocumentationElement() { 1133 return this.documentation != null && !this.documentation.isEmpty(); 1134 } 1135 1136 public boolean hasDocumentation() { 1137 return this.documentation != null && !this.documentation.isEmpty(); 1138 } 1139 1140 /** 1141 * @param value {@link #documentation} (Documentation about the functionality of the actor.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1142 */ 1143 public ActorDefinition setDocumentationElement(MarkdownType value) { 1144 this.documentation = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return Documentation about the functionality of the actor. 1150 */ 1151 public String getDocumentation() { 1152 return this.documentation == null ? null : this.documentation.getValue(); 1153 } 1154 1155 /** 1156 * @param value Documentation about the functionality of the actor. 1157 */ 1158 public ActorDefinition setDocumentation(String value) { 1159 if (Utilities.noString(value)) 1160 this.documentation = null; 1161 else { 1162 if (this.documentation == null) 1163 this.documentation = new MarkdownType(); 1164 this.documentation.setValue(value); 1165 } 1166 return this; 1167 } 1168 1169 /** 1170 * @return {@link #reference} (A reference to additional documentation about the actor, but description and documentation.) 1171 */ 1172 public List<UrlType> getReference() { 1173 if (this.reference == null) 1174 this.reference = new ArrayList<UrlType>(); 1175 return this.reference; 1176 } 1177 1178 /** 1179 * @return Returns a reference to <code>this</code> for easy method chaining 1180 */ 1181 public ActorDefinition setReference(List<UrlType> theReference) { 1182 this.reference = theReference; 1183 return this; 1184 } 1185 1186 public boolean hasReference() { 1187 if (this.reference == null) 1188 return false; 1189 for (UrlType item : this.reference) 1190 if (!item.isEmpty()) 1191 return true; 1192 return false; 1193 } 1194 1195 /** 1196 * @return {@link #reference} (A reference to additional documentation about the actor, but description and documentation.) 1197 */ 1198 public UrlType addReferenceElement() {//2 1199 UrlType t = new UrlType(); 1200 if (this.reference == null) 1201 this.reference = new ArrayList<UrlType>(); 1202 this.reference.add(t); 1203 return t; 1204 } 1205 1206 /** 1207 * @param value {@link #reference} (A reference to additional documentation about the actor, but description and documentation.) 1208 */ 1209 public ActorDefinition addReference(String value) { //1 1210 UrlType t = new UrlType(); 1211 t.setValue(value); 1212 if (this.reference == null) 1213 this.reference = new ArrayList<UrlType>(); 1214 this.reference.add(t); 1215 return this; 1216 } 1217 1218 /** 1219 * @param value {@link #reference} (A reference to additional documentation about the actor, but description and documentation.) 1220 */ 1221 public boolean hasReference(String value) { 1222 if (this.reference == null) 1223 return false; 1224 for (UrlType v : this.reference) 1225 if (v.getValue().equals(value)) // url 1226 return true; 1227 return false; 1228 } 1229 1230 /** 1231 * @return {@link #capabilities} (The capability statement for the actor (if the concept is applicable).). This is the underlying object with id, value and extensions. The accessor "getCapabilities" gives direct access to the value 1232 */ 1233 public CanonicalType getCapabilitiesElement() { 1234 if (this.capabilities == null) 1235 if (Configuration.errorOnAutoCreate()) 1236 throw new Error("Attempt to auto-create ActorDefinition.capabilities"); 1237 else if (Configuration.doAutoCreate()) 1238 this.capabilities = new CanonicalType(); // bb 1239 return this.capabilities; 1240 } 1241 1242 public boolean hasCapabilitiesElement() { 1243 return this.capabilities != null && !this.capabilities.isEmpty(); 1244 } 1245 1246 public boolean hasCapabilities() { 1247 return this.capabilities != null && !this.capabilities.isEmpty(); 1248 } 1249 1250 /** 1251 * @param value {@link #capabilities} (The capability statement for the actor (if the concept is applicable).). This is the underlying object with id, value and extensions. The accessor "getCapabilities" gives direct access to the value 1252 */ 1253 public ActorDefinition setCapabilitiesElement(CanonicalType value) { 1254 this.capabilities = value; 1255 return this; 1256 } 1257 1258 /** 1259 * @return The capability statement for the actor (if the concept is applicable). 1260 */ 1261 public String getCapabilities() { 1262 return this.capabilities == null ? null : this.capabilities.getValue(); 1263 } 1264 1265 /** 1266 * @param value The capability statement for the actor (if the concept is applicable). 1267 */ 1268 public ActorDefinition setCapabilities(String value) { 1269 if (Utilities.noString(value)) 1270 this.capabilities = null; 1271 else { 1272 if (this.capabilities == null) 1273 this.capabilities = new CanonicalType(); 1274 this.capabilities.setValue(value); 1275 } 1276 return this; 1277 } 1278 1279 /** 1280 * @return {@link #derivedFrom} (A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG.) 1281 */ 1282 public List<CanonicalType> getDerivedFrom() { 1283 if (this.derivedFrom == null) 1284 this.derivedFrom = new ArrayList<CanonicalType>(); 1285 return this.derivedFrom; 1286 } 1287 1288 /** 1289 * @return Returns a reference to <code>this</code> for easy method chaining 1290 */ 1291 public ActorDefinition setDerivedFrom(List<CanonicalType> theDerivedFrom) { 1292 this.derivedFrom = theDerivedFrom; 1293 return this; 1294 } 1295 1296 public boolean hasDerivedFrom() { 1297 if (this.derivedFrom == null) 1298 return false; 1299 for (CanonicalType item : this.derivedFrom) 1300 if (!item.isEmpty()) 1301 return true; 1302 return false; 1303 } 1304 1305 /** 1306 * @return {@link #derivedFrom} (A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG.) 1307 */ 1308 public CanonicalType addDerivedFromElement() {//2 1309 CanonicalType t = new CanonicalType(); 1310 if (this.derivedFrom == null) 1311 this.derivedFrom = new ArrayList<CanonicalType>(); 1312 this.derivedFrom.add(t); 1313 return t; 1314 } 1315 1316 /** 1317 * @param value {@link #derivedFrom} (A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG.) 1318 */ 1319 public ActorDefinition addDerivedFrom(String value) { //1 1320 CanonicalType t = new CanonicalType(); 1321 t.setValue(value); 1322 if (this.derivedFrom == null) 1323 this.derivedFrom = new ArrayList<CanonicalType>(); 1324 this.derivedFrom.add(t); 1325 return this; 1326 } 1327 1328 /** 1329 * @param value {@link #derivedFrom} (A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG.) 1330 */ 1331 public boolean hasDerivedFrom(String value) { 1332 if (this.derivedFrom == null) 1333 return false; 1334 for (CanonicalType v : this.derivedFrom) 1335 if (v.getValue().equals(value)) // canonical 1336 return true; 1337 return false; 1338 } 1339 1340 protected void listChildren(List<Property> children) { 1341 super.listChildren(children); 1342 children.add(new Property("url", "uri", "An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers.", 0, 1, url)); 1343 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this actor definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1344 children.add(new Property("version", "string", "The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 1345 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 1346 children.add(new Property("name", "string", "A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1347 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the actor definition.", 0, 1, title)); 1348 children.add(new Property("status", "code", "The status of this actor definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1349 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 1350 children.add(new Property("date", "dateTime", "The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes.", 0, 1, date)); 1351 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition.", 0, 1, publisher)); 1352 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1353 children.add(new Property("description", "markdown", "A free text natural language description of the actor.", 0, 1, description)); 1354 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate actor definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1355 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the actor definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1356 children.add(new Property("purpose", "markdown", "Explanation of why this actor definition is needed and why it has been designed as it has.", 0, 1, purpose)); 1357 children.add(new Property("copyright", "markdown", "A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition.", 0, 1, copyright)); 1358 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 1359 children.add(new Property("type", "code", "Whether the actor represents a human or an appliction.", 0, 1, type)); 1360 children.add(new Property("documentation", "markdown", "Documentation about the functionality of the actor.", 0, 1, documentation)); 1361 children.add(new Property("reference", "url", "A reference to additional documentation about the actor, but description and documentation.", 0, java.lang.Integer.MAX_VALUE, reference)); 1362 children.add(new Property("capabilities", "canonical(CapabilityStatement)", "The capability statement for the actor (if the concept is applicable).", 0, 1, capabilities)); 1363 children.add(new Property("derivedFrom", "canonical(ActorDefinition)", "A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1364 } 1365 1366 @Override 1367 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1368 switch (_hash) { 1369 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this actor definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this actor definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the actor definition is stored on different servers.", 0, 1, url); 1370 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this actor definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 1371 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the actor definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the actor definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 1372 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1373 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1374 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1375 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1376 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the actor definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1377 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the actor definition.", 0, 1, title); 1378 case -892481550: /*status*/ return new Property("status", "code", "The status of this actor definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1379 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this actor definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 1380 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the actor definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the actor definition changes.", 0, 1, date); 1381 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the actor definition.", 0, 1, publisher); 1382 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1383 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the actor.", 0, 1, description); 1384 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate actor definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1385 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the actor definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1386 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this actor definition is needed and why it has been designed as it has.", 0, 1, purpose); 1387 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the actor definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the actor definition.", 0, 1, copyright); 1388 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 1389 case 3575610: /*type*/ return new Property("type", "code", "Whether the actor represents a human or an appliction.", 0, 1, type); 1390 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Documentation about the functionality of the actor.", 0, 1, documentation); 1391 case -925155509: /*reference*/ return new Property("reference", "url", "A reference to additional documentation about the actor, but description and documentation.", 0, java.lang.Integer.MAX_VALUE, reference); 1392 case -1487597642: /*capabilities*/ return new Property("capabilities", "canonical(CapabilityStatement)", "The capability statement for the actor (if the concept is applicable).", 0, 1, capabilities); 1393 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(ActorDefinition)", "A url that identifies the definition of this actor in another IG (which IG must be listed in the dependencies). This actor inherits all the obligations etc. as defined in the other IG.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1394 default: return super.getNamedProperty(_hash, _name, _checkValid); 1395 } 1396 1397 } 1398 1399 @Override 1400 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1401 switch (hash) { 1402 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1403 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1404 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1405 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 1406 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1407 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1408 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1409 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1410 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1411 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1412 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1413 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1414 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1415 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1416 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 1417 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 1418 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 1419 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ExampleScenarioActorType> 1420 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 1421 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // UrlType 1422 case -1487597642: /*capabilities*/ return this.capabilities == null ? new Base[0] : new Base[] {this.capabilities}; // CanonicalType 1423 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 1424 default: return super.getProperty(hash, name, checkValid); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1431 switch (hash) { 1432 case 116079: // url 1433 this.url = TypeConvertor.castToUri(value); // UriType 1434 return value; 1435 case -1618432855: // identifier 1436 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1437 return value; 1438 case 351608024: // version 1439 this.version = TypeConvertor.castToString(value); // StringType 1440 return value; 1441 case 1508158071: // versionAlgorithm 1442 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 1443 return value; 1444 case 3373707: // name 1445 this.name = TypeConvertor.castToString(value); // StringType 1446 return value; 1447 case 110371416: // title 1448 this.title = TypeConvertor.castToString(value); // StringType 1449 return value; 1450 case -892481550: // status 1451 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1452 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1453 return value; 1454 case -404562712: // experimental 1455 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 1456 return value; 1457 case 3076014: // date 1458 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1459 return value; 1460 case 1447404028: // publisher 1461 this.publisher = TypeConvertor.castToString(value); // StringType 1462 return value; 1463 case 951526432: // contact 1464 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1465 return value; 1466 case -1724546052: // description 1467 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1468 return value; 1469 case -669707736: // useContext 1470 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 1471 return value; 1472 case -507075711: // jurisdiction 1473 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1474 return value; 1475 case -220463842: // purpose 1476 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 1477 return value; 1478 case 1522889671: // copyright 1479 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 1480 return value; 1481 case 765157229: // copyrightLabel 1482 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 1483 return value; 1484 case 3575610: // type 1485 value = new ExampleScenarioActorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1486 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 1487 return value; 1488 case 1587405498: // documentation 1489 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 1490 return value; 1491 case -925155509: // reference 1492 this.getReference().add(TypeConvertor.castToUrl(value)); // UrlType 1493 return value; 1494 case -1487597642: // capabilities 1495 this.capabilities = TypeConvertor.castToCanonical(value); // CanonicalType 1496 return value; 1497 case 1077922663: // derivedFrom 1498 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1499 return value; 1500 default: return super.setProperty(hash, name, value); 1501 } 1502 1503 } 1504 1505 @Override 1506 public Base setProperty(String name, Base value) throws FHIRException { 1507 if (name.equals("url")) { 1508 this.url = TypeConvertor.castToUri(value); // UriType 1509 } else if (name.equals("identifier")) { 1510 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1511 } else if (name.equals("version")) { 1512 this.version = TypeConvertor.castToString(value); // StringType 1513 } else if (name.equals("versionAlgorithm[x]")) { 1514 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 1515 } else if (name.equals("name")) { 1516 this.name = TypeConvertor.castToString(value); // StringType 1517 } else if (name.equals("title")) { 1518 this.title = TypeConvertor.castToString(value); // StringType 1519 } else if (name.equals("status")) { 1520 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1521 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1522 } else if (name.equals("experimental")) { 1523 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 1524 } else if (name.equals("date")) { 1525 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1526 } else if (name.equals("publisher")) { 1527 this.publisher = TypeConvertor.castToString(value); // StringType 1528 } else if (name.equals("contact")) { 1529 this.getContact().add(TypeConvertor.castToContactDetail(value)); 1530 } else if (name.equals("description")) { 1531 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1532 } else if (name.equals("useContext")) { 1533 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 1534 } else if (name.equals("jurisdiction")) { 1535 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 1536 } else if (name.equals("purpose")) { 1537 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 1538 } else if (name.equals("copyright")) { 1539 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 1540 } else if (name.equals("copyrightLabel")) { 1541 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 1542 } else if (name.equals("type")) { 1543 value = new ExampleScenarioActorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1544 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 1545 } else if (name.equals("documentation")) { 1546 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 1547 } else if (name.equals("reference")) { 1548 this.getReference().add(TypeConvertor.castToUrl(value)); 1549 } else if (name.equals("capabilities")) { 1550 this.capabilities = TypeConvertor.castToCanonical(value); // CanonicalType 1551 } else if (name.equals("derivedFrom")) { 1552 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); 1553 } else 1554 return super.setProperty(name, value); 1555 return value; 1556 } 1557 1558 @Override 1559 public void removeChild(String name, Base value) throws FHIRException { 1560 if (name.equals("url")) { 1561 this.url = null; 1562 } else if (name.equals("identifier")) { 1563 this.getIdentifier().remove(value); 1564 } else if (name.equals("version")) { 1565 this.version = null; 1566 } else if (name.equals("versionAlgorithm[x]")) { 1567 this.versionAlgorithm = null; 1568 } else if (name.equals("name")) { 1569 this.name = null; 1570 } else if (name.equals("title")) { 1571 this.title = null; 1572 } else if (name.equals("status")) { 1573 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1574 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1575 } else if (name.equals("experimental")) { 1576 this.experimental = null; 1577 } else if (name.equals("date")) { 1578 this.date = null; 1579 } else if (name.equals("publisher")) { 1580 this.publisher = null; 1581 } else if (name.equals("contact")) { 1582 this.getContact().remove(value); 1583 } else if (name.equals("description")) { 1584 this.description = null; 1585 } else if (name.equals("useContext")) { 1586 this.getUseContext().remove(value); 1587 } else if (name.equals("jurisdiction")) { 1588 this.getJurisdiction().remove(value); 1589 } else if (name.equals("purpose")) { 1590 this.purpose = null; 1591 } else if (name.equals("copyright")) { 1592 this.copyright = null; 1593 } else if (name.equals("copyrightLabel")) { 1594 this.copyrightLabel = null; 1595 } else if (name.equals("type")) { 1596 value = new ExampleScenarioActorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1597 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 1598 } else if (name.equals("documentation")) { 1599 this.documentation = null; 1600 } else if (name.equals("reference")) { 1601 this.getReference().remove(value); 1602 } else if (name.equals("capabilities")) { 1603 this.capabilities = null; 1604 } else if (name.equals("derivedFrom")) { 1605 this.getDerivedFrom().remove(value); 1606 } else 1607 super.removeChild(name, value); 1608 1609 } 1610 1611 @Override 1612 public Base makeProperty(int hash, String name) throws FHIRException { 1613 switch (hash) { 1614 case 116079: return getUrlElement(); 1615 case -1618432855: return addIdentifier(); 1616 case 351608024: return getVersionElement(); 1617 case -115699031: return getVersionAlgorithm(); 1618 case 1508158071: return getVersionAlgorithm(); 1619 case 3373707: return getNameElement(); 1620 case 110371416: return getTitleElement(); 1621 case -892481550: return getStatusElement(); 1622 case -404562712: return getExperimentalElement(); 1623 case 3076014: return getDateElement(); 1624 case 1447404028: return getPublisherElement(); 1625 case 951526432: return addContact(); 1626 case -1724546052: return getDescriptionElement(); 1627 case -669707736: return addUseContext(); 1628 case -507075711: return addJurisdiction(); 1629 case -220463842: return getPurposeElement(); 1630 case 1522889671: return getCopyrightElement(); 1631 case 765157229: return getCopyrightLabelElement(); 1632 case 3575610: return getTypeElement(); 1633 case 1587405498: return getDocumentationElement(); 1634 case -925155509: return addReferenceElement(); 1635 case -1487597642: return getCapabilitiesElement(); 1636 case 1077922663: return addDerivedFromElement(); 1637 default: return super.makeProperty(hash, name); 1638 } 1639 1640 } 1641 1642 @Override 1643 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1644 switch (hash) { 1645 case 116079: /*url*/ return new String[] {"uri"}; 1646 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1647 case 351608024: /*version*/ return new String[] {"string"}; 1648 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 1649 case 3373707: /*name*/ return new String[] {"string"}; 1650 case 110371416: /*title*/ return new String[] {"string"}; 1651 case -892481550: /*status*/ return new String[] {"code"}; 1652 case -404562712: /*experimental*/ return new String[] {"boolean"}; 1653 case 3076014: /*date*/ return new String[] {"dateTime"}; 1654 case 1447404028: /*publisher*/ return new String[] {"string"}; 1655 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1656 case -1724546052: /*description*/ return new String[] {"markdown"}; 1657 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1658 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1659 case -220463842: /*purpose*/ return new String[] {"markdown"}; 1660 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 1661 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 1662 case 3575610: /*type*/ return new String[] {"code"}; 1663 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 1664 case -925155509: /*reference*/ return new String[] {"url"}; 1665 case -1487597642: /*capabilities*/ return new String[] {"canonical"}; 1666 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 1667 default: return super.getTypesForProperty(hash, name); 1668 } 1669 1670 } 1671 1672 @Override 1673 public Base addChild(String name) throws FHIRException { 1674 if (name.equals("url")) { 1675 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.url"); 1676 } 1677 else if (name.equals("identifier")) { 1678 return addIdentifier(); 1679 } 1680 else if (name.equals("version")) { 1681 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.version"); 1682 } 1683 else if (name.equals("versionAlgorithmString")) { 1684 this.versionAlgorithm = new StringType(); 1685 return this.versionAlgorithm; 1686 } 1687 else if (name.equals("versionAlgorithmCoding")) { 1688 this.versionAlgorithm = new Coding(); 1689 return this.versionAlgorithm; 1690 } 1691 else if (name.equals("name")) { 1692 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.name"); 1693 } 1694 else if (name.equals("title")) { 1695 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.title"); 1696 } 1697 else if (name.equals("status")) { 1698 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.status"); 1699 } 1700 else if (name.equals("experimental")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.experimental"); 1702 } 1703 else if (name.equals("date")) { 1704 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.date"); 1705 } 1706 else if (name.equals("publisher")) { 1707 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.publisher"); 1708 } 1709 else if (name.equals("contact")) { 1710 return addContact(); 1711 } 1712 else if (name.equals("description")) { 1713 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.description"); 1714 } 1715 else if (name.equals("useContext")) { 1716 return addUseContext(); 1717 } 1718 else if (name.equals("jurisdiction")) { 1719 return addJurisdiction(); 1720 } 1721 else if (name.equals("purpose")) { 1722 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.purpose"); 1723 } 1724 else if (name.equals("copyright")) { 1725 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.copyright"); 1726 } 1727 else if (name.equals("copyrightLabel")) { 1728 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.copyrightLabel"); 1729 } 1730 else if (name.equals("type")) { 1731 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.type"); 1732 } 1733 else if (name.equals("documentation")) { 1734 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.documentation"); 1735 } 1736 else if (name.equals("reference")) { 1737 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.reference"); 1738 } 1739 else if (name.equals("capabilities")) { 1740 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.capabilities"); 1741 } 1742 else if (name.equals("derivedFrom")) { 1743 throw new FHIRException("Cannot call addChild on a singleton property ActorDefinition.derivedFrom"); 1744 } 1745 else 1746 return super.addChild(name); 1747 } 1748 1749 public String fhirType() { 1750 return "ActorDefinition"; 1751 1752 } 1753 1754 public ActorDefinition copy() { 1755 ActorDefinition dst = new ActorDefinition(); 1756 copyValues(dst); 1757 return dst; 1758 } 1759 1760 public void copyValues(ActorDefinition dst) { 1761 super.copyValues(dst); 1762 dst.url = url == null ? null : url.copy(); 1763 if (identifier != null) { 1764 dst.identifier = new ArrayList<Identifier>(); 1765 for (Identifier i : identifier) 1766 dst.identifier.add(i.copy()); 1767 }; 1768 dst.version = version == null ? null : version.copy(); 1769 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 1770 dst.name = name == null ? null : name.copy(); 1771 dst.title = title == null ? null : title.copy(); 1772 dst.status = status == null ? null : status.copy(); 1773 dst.experimental = experimental == null ? null : experimental.copy(); 1774 dst.date = date == null ? null : date.copy(); 1775 dst.publisher = publisher == null ? null : publisher.copy(); 1776 if (contact != null) { 1777 dst.contact = new ArrayList<ContactDetail>(); 1778 for (ContactDetail i : contact) 1779 dst.contact.add(i.copy()); 1780 }; 1781 dst.description = description == null ? null : description.copy(); 1782 if (useContext != null) { 1783 dst.useContext = new ArrayList<UsageContext>(); 1784 for (UsageContext i : useContext) 1785 dst.useContext.add(i.copy()); 1786 }; 1787 if (jurisdiction != null) { 1788 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1789 for (CodeableConcept i : jurisdiction) 1790 dst.jurisdiction.add(i.copy()); 1791 }; 1792 dst.purpose = purpose == null ? null : purpose.copy(); 1793 dst.copyright = copyright == null ? null : copyright.copy(); 1794 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 1795 dst.type = type == null ? null : type.copy(); 1796 dst.documentation = documentation == null ? null : documentation.copy(); 1797 if (reference != null) { 1798 dst.reference = new ArrayList<UrlType>(); 1799 for (UrlType i : reference) 1800 dst.reference.add(i.copy()); 1801 }; 1802 dst.capabilities = capabilities == null ? null : capabilities.copy(); 1803 if (derivedFrom != null) { 1804 dst.derivedFrom = new ArrayList<CanonicalType>(); 1805 for (CanonicalType i : derivedFrom) 1806 dst.derivedFrom.add(i.copy()); 1807 }; 1808 } 1809 1810 protected ActorDefinition typedCopy() { 1811 return copy(); 1812 } 1813 1814 @Override 1815 public boolean equalsDeep(Base other_) { 1816 if (!super.equalsDeep(other_)) 1817 return false; 1818 if (!(other_ instanceof ActorDefinition)) 1819 return false; 1820 ActorDefinition o = (ActorDefinition) other_; 1821 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 1822 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 1823 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 1824 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 1825 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 1826 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 1827 && compareDeep(type, o.type, true) && compareDeep(documentation, o.documentation, true) && compareDeep(reference, o.reference, true) 1828 && compareDeep(capabilities, o.capabilities, true) && compareDeep(derivedFrom, o.derivedFrom, true) 1829 ; 1830 } 1831 1832 @Override 1833 public boolean equalsShallow(Base other_) { 1834 if (!super.equalsShallow(other_)) 1835 return false; 1836 if (!(other_ instanceof ActorDefinition)) 1837 return false; 1838 ActorDefinition o = (ActorDefinition) other_; 1839 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 1840 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 1841 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 1842 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 1843 && compareValues(type, o.type, true) && compareValues(documentation, o.documentation, true) && compareValues(reference, o.reference, true) 1844 && compareValues(capabilities, o.capabilities, true) && compareValues(derivedFrom, o.derivedFrom, true) 1845 ; 1846 } 1847 1848 public boolean isEmpty() { 1849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 1850 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 1851 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, type 1852 , documentation, reference, capabilities, derivedFrom); 1853 } 1854 1855 @Override 1856 public ResourceType getResourceType() { 1857 return ResourceType.ActorDefinition; 1858 } 1859 1860 /** 1861 * Search parameter: <b>type</b> 1862 * <p> 1863 * Description: <b>The type of actor</b><br> 1864 * Type: <b>token</b><br> 1865 * Path: <b>ActorDefinition.type</b><br> 1866 * </p> 1867 */ 1868 @SearchParamDefinition(name="type", path="ActorDefinition.type", description="The type of actor", type="token" ) 1869 public static final String SP_TYPE = "type"; 1870 /** 1871 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1872 * <p> 1873 * Description: <b>The type of actor</b><br> 1874 * Type: <b>token</b><br> 1875 * Path: <b>ActorDefinition.type</b><br> 1876 * </p> 1877 */ 1878 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1879 1880 /** 1881 * Search parameter: <b>context-quantity</b> 1882 * <p> 1883 * Description: <b>Multiple Resources: 1884 1885* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 1886* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 1887* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 1888* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 1889* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 1890* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 1891* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 1892* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 1893* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 1894* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 1895* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 1896* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 1897* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 1898* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 1899* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 1900* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 1901* [Library](library.html): A quantity- or range-valued use context assigned to the library 1902* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 1903* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 1904* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 1905* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 1906* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 1907* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 1908* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 1909* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 1910* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 1911* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 1912* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 1913* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 1914* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 1915</b><br> 1916 * Type: <b>quantity</b><br> 1917 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 1918 * </p> 1919 */ 1920 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 1921 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 1922 /** 1923 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 1924 * <p> 1925 * Description: <b>Multiple Resources: 1926 1927* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 1928* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 1929* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 1930* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 1931* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 1932* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 1933* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 1934* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 1935* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 1936* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 1937* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 1938* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 1939* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 1940* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 1941* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 1942* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 1943* [Library](library.html): A quantity- or range-valued use context assigned to the library 1944* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 1945* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 1946* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 1947* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 1948* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 1949* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 1950* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 1951* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 1952* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 1953* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 1954* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 1955* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 1956* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 1957</b><br> 1958 * Type: <b>quantity</b><br> 1959 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 1960 * </p> 1961 */ 1962 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 1963 1964 /** 1965 * Search parameter: <b>context-type-quantity</b> 1966 * <p> 1967 * Description: <b>Multiple Resources: 1968 1969* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 1970* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 1971* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 1972* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 1973* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 1974* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 1975* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 1976* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 1977* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 1978* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 1979* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 1980* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 1981* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 1982* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 1983* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 1984* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 1985* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 1986* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 1987* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 1988* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 1989* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 1990* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 1991* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 1992* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 1993* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 1994* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 1995* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 1996* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 1997* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 1998* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 1999</b><br> 2000 * Type: <b>composite</b><br> 2001 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2002 * </p> 2003 */ 2004 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2005 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2006 /** 2007 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2008 * <p> 2009 * Description: <b>Multiple Resources: 2010 2011* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2012* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2013* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2014* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2015* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2016* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2017* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2018* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2019* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2020* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2021* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2022* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2023* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2024* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2025* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2026* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2027* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2028* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2029* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2030* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2031* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2032* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2033* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2034* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2035* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2036* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2037* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2038* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2039* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2040* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2041</b><br> 2042 * Type: <b>composite</b><br> 2043 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2044 * </p> 2045 */ 2046 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 2047 2048 /** 2049 * Search parameter: <b>context-type-value</b> 2050 * <p> 2051 * Description: <b>Multiple Resources: 2052 2053* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2054* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2055* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2056* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2057* [Citation](citation.html): A use context type and value assigned to the citation 2058* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2059* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2060* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2061* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2062* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2063* [Evidence](evidence.html): A use context type and value assigned to the evidence 2064* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2065* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2066* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2067* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2068* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2069* [Library](library.html): A use context type and value assigned to the library 2070* [Measure](measure.html): A use context type and value assigned to the measure 2071* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2072* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2073* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2074* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2075* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2076* [Requirements](requirements.html): A use context type and value assigned to the requirements 2077* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2078* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2079* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2080* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2081* [TestScript](testscript.html): A use context type and value assigned to the test script 2082* [ValueSet](valueset.html): A use context type and value assigned to the value set 2083</b><br> 2084 * Type: <b>composite</b><br> 2085 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2086 * </p> 2087 */ 2088 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 2089 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2090 /** 2091 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2092 * <p> 2093 * Description: <b>Multiple Resources: 2094 2095* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2096* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2097* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2098* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2099* [Citation](citation.html): A use context type and value assigned to the citation 2100* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2101* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2102* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2103* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2104* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2105* [Evidence](evidence.html): A use context type and value assigned to the evidence 2106* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2107* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2108* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2109* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2110* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2111* [Library](library.html): A use context type and value assigned to the library 2112* [Measure](measure.html): A use context type and value assigned to the measure 2113* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2114* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2115* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2116* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2117* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2118* [Requirements](requirements.html): A use context type and value assigned to the requirements 2119* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2120* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2121* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2122* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2123* [TestScript](testscript.html): A use context type and value assigned to the test script 2124* [ValueSet](valueset.html): A use context type and value assigned to the value set 2125</b><br> 2126 * Type: <b>composite</b><br> 2127 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2128 * </p> 2129 */ 2130 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 2131 2132 /** 2133 * Search parameter: <b>context-type</b> 2134 * <p> 2135 * Description: <b>Multiple Resources: 2136 2137* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2138* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2139* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2140* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2141* [Citation](citation.html): A type of use context assigned to the citation 2142* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2143* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2144* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2145* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2146* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2147* [Evidence](evidence.html): A type of use context assigned to the evidence 2148* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2149* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2150* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2151* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2152* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2153* [Library](library.html): A type of use context assigned to the library 2154* [Measure](measure.html): A type of use context assigned to the measure 2155* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2156* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2157* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2158* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2159* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2160* [Requirements](requirements.html): A type of use context assigned to the requirements 2161* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2162* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2163* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2164* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2165* [TestScript](testscript.html): A type of use context assigned to the test script 2166* [ValueSet](valueset.html): A type of use context assigned to the value set 2167</b><br> 2168 * Type: <b>token</b><br> 2169 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2170 * </p> 2171 */ 2172 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 2173 public static final String SP_CONTEXT_TYPE = "context-type"; 2174 /** 2175 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2176 * <p> 2177 * Description: <b>Multiple Resources: 2178 2179* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2180* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2181* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2182* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2183* [Citation](citation.html): A type of use context assigned to the citation 2184* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2185* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2186* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2187* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2188* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2189* [Evidence](evidence.html): A type of use context assigned to the evidence 2190* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2191* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2192* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2193* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2194* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2195* [Library](library.html): A type of use context assigned to the library 2196* [Measure](measure.html): A type of use context assigned to the measure 2197* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2198* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2199* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2200* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2201* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2202* [Requirements](requirements.html): A type of use context assigned to the requirements 2203* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2204* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2205* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2206* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2207* [TestScript](testscript.html): A type of use context assigned to the test script 2208* [ValueSet](valueset.html): A type of use context assigned to the value set 2209</b><br> 2210 * Type: <b>token</b><br> 2211 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2212 * </p> 2213 */ 2214 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 2215 2216 /** 2217 * Search parameter: <b>context</b> 2218 * <p> 2219 * Description: <b>Multiple Resources: 2220 2221* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 2222* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 2223* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 2224* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 2225* [Citation](citation.html): A use context assigned to the citation 2226* [CodeSystem](codesystem.html): A use context assigned to the code system 2227* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 2228* [ConceptMap](conceptmap.html): A use context assigned to the concept map 2229* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 2230* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 2231* [Evidence](evidence.html): A use context assigned to the evidence 2232* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 2233* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 2234* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 2235* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 2236* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 2237* [Library](library.html): A use context assigned to the library 2238* [Measure](measure.html): A use context assigned to the measure 2239* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 2240* [NamingSystem](namingsystem.html): A use context assigned to the naming system 2241* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 2242* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 2243* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 2244* [Requirements](requirements.html): A use context assigned to the requirements 2245* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 2246* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 2247* [StructureMap](structuremap.html): A use context assigned to the structure map 2248* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 2249* [TestScript](testscript.html): A use context assigned to the test script 2250* [ValueSet](valueset.html): A use context assigned to the value set 2251</b><br> 2252 * Type: <b>token</b><br> 2253 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 2257 public static final String SP_CONTEXT = "context"; 2258 /** 2259 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2260 * <p> 2261 * Description: <b>Multiple Resources: 2262 2263* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 2264* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 2265* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 2266* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 2267* [Citation](citation.html): A use context assigned to the citation 2268* [CodeSystem](codesystem.html): A use context assigned to the code system 2269* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 2270* [ConceptMap](conceptmap.html): A use context assigned to the concept map 2271* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 2272* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 2273* [Evidence](evidence.html): A use context assigned to the evidence 2274* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 2275* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 2276* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 2277* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 2278* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 2279* [Library](library.html): A use context assigned to the library 2280* [Measure](measure.html): A use context assigned to the measure 2281* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 2282* [NamingSystem](namingsystem.html): A use context assigned to the naming system 2283* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 2284* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 2285* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 2286* [Requirements](requirements.html): A use context assigned to the requirements 2287* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 2288* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 2289* [StructureMap](structuremap.html): A use context assigned to the structure map 2290* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 2291* [TestScript](testscript.html): A use context assigned to the test script 2292* [ValueSet](valueset.html): A use context assigned to the value set 2293</b><br> 2294 * Type: <b>token</b><br> 2295 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 2296 * </p> 2297 */ 2298 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 2299 2300 /** 2301 * Search parameter: <b>date</b> 2302 * <p> 2303 * Description: <b>Multiple Resources: 2304 2305* [ActivityDefinition](activitydefinition.html): The activity definition publication date 2306* [ActorDefinition](actordefinition.html): The Actor Definition publication date 2307* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 2308* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 2309* [Citation](citation.html): The citation publication date 2310* [CodeSystem](codesystem.html): The code system publication date 2311* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 2312* [ConceptMap](conceptmap.html): The concept map publication date 2313* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 2314* [EventDefinition](eventdefinition.html): The event definition publication date 2315* [Evidence](evidence.html): The evidence publication date 2316* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 2317* [ExampleScenario](examplescenario.html): The example scenario publication date 2318* [GraphDefinition](graphdefinition.html): The graph definition publication date 2319* [ImplementationGuide](implementationguide.html): The implementation guide publication date 2320* [Library](library.html): The library publication date 2321* [Measure](measure.html): The measure publication date 2322* [MessageDefinition](messagedefinition.html): The message definition publication date 2323* [NamingSystem](namingsystem.html): The naming system publication date 2324* [OperationDefinition](operationdefinition.html): The operation definition publication date 2325* [PlanDefinition](plandefinition.html): The plan definition publication date 2326* [Questionnaire](questionnaire.html): The questionnaire publication date 2327* [Requirements](requirements.html): The requirements publication date 2328* [SearchParameter](searchparameter.html): The search parameter publication date 2329* [StructureDefinition](structuredefinition.html): The structure definition publication date 2330* [StructureMap](structuremap.html): The structure map publication date 2331* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 2332* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 2333* [TestScript](testscript.html): The test script publication date 2334* [ValueSet](valueset.html): The value set publication date 2335</b><br> 2336 * Type: <b>date</b><br> 2337 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 2338 * </p> 2339 */ 2340 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 2341 public static final String SP_DATE = "date"; 2342 /** 2343 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2344 * <p> 2345 * Description: <b>Multiple Resources: 2346 2347* [ActivityDefinition](activitydefinition.html): The activity definition publication date 2348* [ActorDefinition](actordefinition.html): The Actor Definition publication date 2349* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 2350* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 2351* [Citation](citation.html): The citation publication date 2352* [CodeSystem](codesystem.html): The code system publication date 2353* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 2354* [ConceptMap](conceptmap.html): The concept map publication date 2355* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 2356* [EventDefinition](eventdefinition.html): The event definition publication date 2357* [Evidence](evidence.html): The evidence publication date 2358* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 2359* [ExampleScenario](examplescenario.html): The example scenario publication date 2360* [GraphDefinition](graphdefinition.html): The graph definition publication date 2361* [ImplementationGuide](implementationguide.html): The implementation guide publication date 2362* [Library](library.html): The library publication date 2363* [Measure](measure.html): The measure publication date 2364* [MessageDefinition](messagedefinition.html): The message definition publication date 2365* [NamingSystem](namingsystem.html): The naming system publication date 2366* [OperationDefinition](operationdefinition.html): The operation definition publication date 2367* [PlanDefinition](plandefinition.html): The plan definition publication date 2368* [Questionnaire](questionnaire.html): The questionnaire publication date 2369* [Requirements](requirements.html): The requirements publication date 2370* [SearchParameter](searchparameter.html): The search parameter publication date 2371* [StructureDefinition](structuredefinition.html): The structure definition publication date 2372* [StructureMap](structuremap.html): The structure map publication date 2373* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 2374* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 2375* [TestScript](testscript.html): The test script publication date 2376* [ValueSet](valueset.html): The value set publication date 2377</b><br> 2378 * Type: <b>date</b><br> 2379 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 2380 * </p> 2381 */ 2382 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2383 2384 /** 2385 * Search parameter: <b>description</b> 2386 * <p> 2387 * Description: <b>Multiple Resources: 2388 2389* [ActivityDefinition](activitydefinition.html): The description of the activity definition 2390* [ActorDefinition](actordefinition.html): The description of the Actor Definition 2391* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 2392* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 2393* [Citation](citation.html): The description of the citation 2394* [CodeSystem](codesystem.html): The description of the code system 2395* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 2396* [ConceptMap](conceptmap.html): The description of the concept map 2397* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 2398* [EventDefinition](eventdefinition.html): The description of the event definition 2399* [Evidence](evidence.html): The description of the evidence 2400* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 2401* [GraphDefinition](graphdefinition.html): The description of the graph definition 2402* [ImplementationGuide](implementationguide.html): The description of the implementation guide 2403* [Library](library.html): The description of the library 2404* [Measure](measure.html): The description of the measure 2405* [MessageDefinition](messagedefinition.html): The description of the message definition 2406* [NamingSystem](namingsystem.html): The description of the naming system 2407* [OperationDefinition](operationdefinition.html): The description of the operation definition 2408* [PlanDefinition](plandefinition.html): The description of the plan definition 2409* [Questionnaire](questionnaire.html): The description of the questionnaire 2410* [Requirements](requirements.html): The description of the requirements 2411* [SearchParameter](searchparameter.html): The description of the search parameter 2412* [StructureDefinition](structuredefinition.html): The description of the structure definition 2413* [StructureMap](structuremap.html): The description of the structure map 2414* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 2415* [TestScript](testscript.html): The description of the test script 2416* [ValueSet](valueset.html): The description of the value set 2417</b><br> 2418 * Type: <b>string</b><br> 2419 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 2420 * </p> 2421 */ 2422 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 2423 public static final String SP_DESCRIPTION = "description"; 2424 /** 2425 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2426 * <p> 2427 * Description: <b>Multiple Resources: 2428 2429* [ActivityDefinition](activitydefinition.html): The description of the activity definition 2430* [ActorDefinition](actordefinition.html): The description of the Actor Definition 2431* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 2432* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 2433* [Citation](citation.html): The description of the citation 2434* [CodeSystem](codesystem.html): The description of the code system 2435* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 2436* [ConceptMap](conceptmap.html): The description of the concept map 2437* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 2438* [EventDefinition](eventdefinition.html): The description of the event definition 2439* [Evidence](evidence.html): The description of the evidence 2440* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 2441* [GraphDefinition](graphdefinition.html): The description of the graph definition 2442* [ImplementationGuide](implementationguide.html): The description of the implementation guide 2443* [Library](library.html): The description of the library 2444* [Measure](measure.html): The description of the measure 2445* [MessageDefinition](messagedefinition.html): The description of the message definition 2446* [NamingSystem](namingsystem.html): The description of the naming system 2447* [OperationDefinition](operationdefinition.html): The description of the operation definition 2448* [PlanDefinition](plandefinition.html): The description of the plan definition 2449* [Questionnaire](questionnaire.html): The description of the questionnaire 2450* [Requirements](requirements.html): The description of the requirements 2451* [SearchParameter](searchparameter.html): The description of the search parameter 2452* [StructureDefinition](structuredefinition.html): The description of the structure definition 2453* [StructureMap](structuremap.html): The description of the structure map 2454* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 2455* [TestScript](testscript.html): The description of the test script 2456* [ValueSet](valueset.html): The description of the value set 2457</b><br> 2458 * Type: <b>string</b><br> 2459 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 2460 * </p> 2461 */ 2462 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2463 2464 /** 2465 * Search parameter: <b>identifier</b> 2466 * <p> 2467 * Description: <b>Multiple Resources: 2468 2469* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 2470* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 2471* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 2472* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 2473* [Citation](citation.html): External identifier for the citation 2474* [CodeSystem](codesystem.html): External identifier for the code system 2475* [ConceptMap](conceptmap.html): External identifier for the concept map 2476* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 2477* [EventDefinition](eventdefinition.html): External identifier for the event definition 2478* [Evidence](evidence.html): External identifier for the evidence 2479* [EvidenceReport](evidencereport.html): External identifier for the evidence report 2480* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 2481* [ExampleScenario](examplescenario.html): External identifier for the example scenario 2482* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 2483* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 2484* [Library](library.html): External identifier for the library 2485* [Measure](measure.html): External identifier for the measure 2486* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 2487* [MessageDefinition](messagedefinition.html): External identifier for the message definition 2488* [NamingSystem](namingsystem.html): External identifier for the naming system 2489* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 2490* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 2491* [PlanDefinition](plandefinition.html): External identifier for the plan definition 2492* [Questionnaire](questionnaire.html): External identifier for the questionnaire 2493* [Requirements](requirements.html): External identifier for the requirements 2494* [SearchParameter](searchparameter.html): External identifier for the search parameter 2495* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 2496* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 2497* [StructureMap](structuremap.html): External identifier for the structure map 2498* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 2499* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 2500* [TestPlan](testplan.html): An identifier for the test plan 2501* [TestScript](testscript.html): External identifier for the test script 2502* [ValueSet](valueset.html): External identifier for the value set 2503</b><br> 2504 * Type: <b>token</b><br> 2505 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 2506 * </p> 2507 */ 2508 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 2509 public static final String SP_IDENTIFIER = "identifier"; 2510 /** 2511 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2512 * <p> 2513 * Description: <b>Multiple Resources: 2514 2515* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 2516* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 2517* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 2518* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 2519* [Citation](citation.html): External identifier for the citation 2520* [CodeSystem](codesystem.html): External identifier for the code system 2521* [ConceptMap](conceptmap.html): External identifier for the concept map 2522* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 2523* [EventDefinition](eventdefinition.html): External identifier for the event definition 2524* [Evidence](evidence.html): External identifier for the evidence 2525* [EvidenceReport](evidencereport.html): External identifier for the evidence report 2526* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 2527* [ExampleScenario](examplescenario.html): External identifier for the example scenario 2528* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 2529* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 2530* [Library](library.html): External identifier for the library 2531* [Measure](measure.html): External identifier for the measure 2532* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 2533* [MessageDefinition](messagedefinition.html): External identifier for the message definition 2534* [NamingSystem](namingsystem.html): External identifier for the naming system 2535* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 2536* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 2537* [PlanDefinition](plandefinition.html): External identifier for the plan definition 2538* [Questionnaire](questionnaire.html): External identifier for the questionnaire 2539* [Requirements](requirements.html): External identifier for the requirements 2540* [SearchParameter](searchparameter.html): External identifier for the search parameter 2541* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 2542* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 2543* [StructureMap](structuremap.html): External identifier for the structure map 2544* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 2545* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 2546* [TestPlan](testplan.html): An identifier for the test plan 2547* [TestScript](testscript.html): External identifier for the test script 2548* [ValueSet](valueset.html): External identifier for the value set 2549</b><br> 2550 * Type: <b>token</b><br> 2551 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 2552 * </p> 2553 */ 2554 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2555 2556 /** 2557 * Search parameter: <b>jurisdiction</b> 2558 * <p> 2559 * Description: <b>Multiple Resources: 2560 2561* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 2562* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 2563* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 2564* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 2565* [Citation](citation.html): Intended jurisdiction for the citation 2566* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 2567* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 2568* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 2569* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 2570* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 2571* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 2572* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 2573* [Library](library.html): Intended jurisdiction for the library 2574* [Measure](measure.html): Intended jurisdiction for the measure 2575* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 2576* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 2577* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 2578* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 2579* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 2580* [Requirements](requirements.html): Intended jurisdiction for the requirements 2581* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 2582* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 2583* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 2584* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 2585* [TestScript](testscript.html): Intended jurisdiction for the test script 2586* [ValueSet](valueset.html): Intended jurisdiction for the value set 2587</b><br> 2588 * Type: <b>token</b><br> 2589 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 2590 * </p> 2591 */ 2592 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 2593 public static final String SP_JURISDICTION = "jurisdiction"; 2594 /** 2595 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2596 * <p> 2597 * Description: <b>Multiple Resources: 2598 2599* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 2600* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 2601* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 2602* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 2603* [Citation](citation.html): Intended jurisdiction for the citation 2604* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 2605* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 2606* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 2607* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 2608* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 2609* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 2610* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 2611* [Library](library.html): Intended jurisdiction for the library 2612* [Measure](measure.html): Intended jurisdiction for the measure 2613* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 2614* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 2615* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 2616* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 2617* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 2618* [Requirements](requirements.html): Intended jurisdiction for the requirements 2619* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 2620* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 2621* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 2622* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 2623* [TestScript](testscript.html): Intended jurisdiction for the test script 2624* [ValueSet](valueset.html): Intended jurisdiction for the value set 2625</b><br> 2626 * Type: <b>token</b><br> 2627 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 2628 * </p> 2629 */ 2630 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2631 2632 /** 2633 * Search parameter: <b>publisher</b> 2634 * <p> 2635 * Description: <b>Multiple Resources: 2636 2637* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 2638* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 2639* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 2640* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 2641* [Citation](citation.html): Name of the publisher of the citation 2642* [CodeSystem](codesystem.html): Name of the publisher of the code system 2643* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 2644* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 2645* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 2646* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 2647* [Evidence](evidence.html): Name of the publisher of the evidence 2648* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 2649* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 2650* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 2651* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 2652* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 2653* [Library](library.html): Name of the publisher of the library 2654* [Measure](measure.html): Name of the publisher of the measure 2655* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 2656* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 2657* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 2658* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 2659* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 2660* [Requirements](requirements.html): Name of the publisher of the requirements 2661* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 2662* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 2663* [StructureMap](structuremap.html): Name of the publisher of the structure map 2664* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 2665* [TestScript](testscript.html): Name of the publisher of the test script 2666* [ValueSet](valueset.html): Name of the publisher of the value set 2667</b><br> 2668 * Type: <b>string</b><br> 2669 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 2670 * </p> 2671 */ 2672 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 2673 public static final String SP_PUBLISHER = "publisher"; 2674 /** 2675 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2676 * <p> 2677 * Description: <b>Multiple Resources: 2678 2679* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 2680* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 2681* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 2682* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 2683* [Citation](citation.html): Name of the publisher of the citation 2684* [CodeSystem](codesystem.html): Name of the publisher of the code system 2685* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 2686* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 2687* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 2688* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 2689* [Evidence](evidence.html): Name of the publisher of the evidence 2690* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 2691* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 2692* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 2693* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 2694* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 2695* [Library](library.html): Name of the publisher of the library 2696* [Measure](measure.html): Name of the publisher of the measure 2697* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 2698* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 2699* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 2700* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 2701* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 2702* [Requirements](requirements.html): Name of the publisher of the requirements 2703* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 2704* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 2705* [StructureMap](structuremap.html): Name of the publisher of the structure map 2706* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 2707* [TestScript](testscript.html): Name of the publisher of the test script 2708* [ValueSet](valueset.html): Name of the publisher of the value set 2709</b><br> 2710 * Type: <b>string</b><br> 2711 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 2712 * </p> 2713 */ 2714 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2715 2716 /** 2717 * Search parameter: <b>status</b> 2718 * <p> 2719 * Description: <b>Multiple Resources: 2720 2721* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 2722* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 2723* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 2724* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 2725* [Citation](citation.html): The current status of the citation 2726* [CodeSystem](codesystem.html): The current status of the code system 2727* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 2728* [ConceptMap](conceptmap.html): The current status of the concept map 2729* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 2730* [EventDefinition](eventdefinition.html): The current status of the event definition 2731* [Evidence](evidence.html): The current status of the evidence 2732* [EvidenceReport](evidencereport.html): The current status of the evidence report 2733* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 2734* [ExampleScenario](examplescenario.html): The current status of the example scenario 2735* [GraphDefinition](graphdefinition.html): The current status of the graph definition 2736* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 2737* [Library](library.html): The current status of the library 2738* [Measure](measure.html): The current status of the measure 2739* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 2740* [MessageDefinition](messagedefinition.html): The current status of the message definition 2741* [NamingSystem](namingsystem.html): The current status of the naming system 2742* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 2743* [OperationDefinition](operationdefinition.html): The current status of the operation definition 2744* [PlanDefinition](plandefinition.html): The current status of the plan definition 2745* [Questionnaire](questionnaire.html): The current status of the questionnaire 2746* [Requirements](requirements.html): The current status of the requirements 2747* [SearchParameter](searchparameter.html): The current status of the search parameter 2748* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 2749* [StructureDefinition](structuredefinition.html): The current status of the structure definition 2750* [StructureMap](structuremap.html): The current status of the structure map 2751* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 2752* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 2753* [TestPlan](testplan.html): The current status of the test plan 2754* [TestScript](testscript.html): The current status of the test script 2755* [ValueSet](valueset.html): The current status of the value set 2756</b><br> 2757 * Type: <b>token</b><br> 2758 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 2759 * </p> 2760 */ 2761 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 2762 public static final String SP_STATUS = "status"; 2763 /** 2764 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2765 * <p> 2766 * Description: <b>Multiple Resources: 2767 2768* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 2769* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 2770* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 2771* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 2772* [Citation](citation.html): The current status of the citation 2773* [CodeSystem](codesystem.html): The current status of the code system 2774* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 2775* [ConceptMap](conceptmap.html): The current status of the concept map 2776* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 2777* [EventDefinition](eventdefinition.html): The current status of the event definition 2778* [Evidence](evidence.html): The current status of the evidence 2779* [EvidenceReport](evidencereport.html): The current status of the evidence report 2780* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 2781* [ExampleScenario](examplescenario.html): The current status of the example scenario 2782* [GraphDefinition](graphdefinition.html): The current status of the graph definition 2783* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 2784* [Library](library.html): The current status of the library 2785* [Measure](measure.html): The current status of the measure 2786* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 2787* [MessageDefinition](messagedefinition.html): The current status of the message definition 2788* [NamingSystem](namingsystem.html): The current status of the naming system 2789* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 2790* [OperationDefinition](operationdefinition.html): The current status of the operation definition 2791* [PlanDefinition](plandefinition.html): The current status of the plan definition 2792* [Questionnaire](questionnaire.html): The current status of the questionnaire 2793* [Requirements](requirements.html): The current status of the requirements 2794* [SearchParameter](searchparameter.html): The current status of the search parameter 2795* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 2796* [StructureDefinition](structuredefinition.html): The current status of the structure definition 2797* [StructureMap](structuremap.html): The current status of the structure map 2798* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 2799* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 2800* [TestPlan](testplan.html): The current status of the test plan 2801* [TestScript](testscript.html): The current status of the test script 2802* [ValueSet](valueset.html): The current status of the value set 2803</b><br> 2804 * Type: <b>token</b><br> 2805 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 2806 * </p> 2807 */ 2808 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2809 2810 /** 2811 * Search parameter: <b>title</b> 2812 * <p> 2813 * Description: <b>Multiple Resources: 2814 2815* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 2816* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 2817* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 2818* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 2819* [Citation](citation.html): The human-friendly name of the citation 2820* [CodeSystem](codesystem.html): The human-friendly name of the code system 2821* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 2822* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 2823* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 2824* [Evidence](evidence.html): The human-friendly name of the evidence 2825* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 2826* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 2827* [Library](library.html): The human-friendly name of the library 2828* [Measure](measure.html): The human-friendly name of the measure 2829* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 2830* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 2831* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 2832* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 2833* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 2834* [Requirements](requirements.html): The human-friendly name of the requirements 2835* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 2836* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 2837* [StructureMap](structuremap.html): The human-friendly name of the structure map 2838* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 2839* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 2840* [TestScript](testscript.html): The human-friendly name of the test script 2841* [ValueSet](valueset.html): The human-friendly name of the value set 2842</b><br> 2843 * Type: <b>string</b><br> 2844 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 2845 * </p> 2846 */ 2847 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 2848 public static final String SP_TITLE = "title"; 2849 /** 2850 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2851 * <p> 2852 * Description: <b>Multiple Resources: 2853 2854* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 2855* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 2856* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 2857* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 2858* [Citation](citation.html): The human-friendly name of the citation 2859* [CodeSystem](codesystem.html): The human-friendly name of the code system 2860* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 2861* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 2862* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 2863* [Evidence](evidence.html): The human-friendly name of the evidence 2864* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 2865* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 2866* [Library](library.html): The human-friendly name of the library 2867* [Measure](measure.html): The human-friendly name of the measure 2868* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 2869* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 2870* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 2871* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 2872* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 2873* [Requirements](requirements.html): The human-friendly name of the requirements 2874* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 2875* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 2876* [StructureMap](structuremap.html): The human-friendly name of the structure map 2877* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 2878* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 2879* [TestScript](testscript.html): The human-friendly name of the test script 2880* [ValueSet](valueset.html): The human-friendly name of the value set 2881</b><br> 2882 * Type: <b>string</b><br> 2883 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 2884 * </p> 2885 */ 2886 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 2887 2888 /** 2889 * Search parameter: <b>url</b> 2890 * <p> 2891 * Description: <b>Multiple Resources: 2892 2893* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 2894* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 2895* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 2896* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 2897* [Citation](citation.html): The uri that identifies the citation 2898* [CodeSystem](codesystem.html): The uri that identifies the code system 2899* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 2900* [ConceptMap](conceptmap.html): The URI that identifies the concept map 2901* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 2902* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 2903* [Evidence](evidence.html): The uri that identifies the evidence 2904* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 2905* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 2906* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 2907* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 2908* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 2909* [Library](library.html): The uri that identifies the library 2910* [Measure](measure.html): The uri that identifies the measure 2911* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 2912* [NamingSystem](namingsystem.html): The uri that identifies the naming system 2913* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 2914* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 2915* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 2916* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 2917* [Requirements](requirements.html): The uri that identifies the requirements 2918* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 2919* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 2920* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 2921* [StructureMap](structuremap.html): The uri that identifies the structure map 2922* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 2923* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 2924* [TestPlan](testplan.html): The uri that identifies the test plan 2925* [TestScript](testscript.html): The uri that identifies the test script 2926* [ValueSet](valueset.html): The uri that identifies the value set 2927</b><br> 2928 * Type: <b>uri</b><br> 2929 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 2930 * </p> 2931 */ 2932 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 2933 public static final String SP_URL = "url"; 2934 /** 2935 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2936 * <p> 2937 * Description: <b>Multiple Resources: 2938 2939* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 2940* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 2941* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 2942* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 2943* [Citation](citation.html): The uri that identifies the citation 2944* [CodeSystem](codesystem.html): The uri that identifies the code system 2945* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 2946* [ConceptMap](conceptmap.html): The URI that identifies the concept map 2947* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 2948* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 2949* [Evidence](evidence.html): The uri that identifies the evidence 2950* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 2951* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 2952* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 2953* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 2954* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 2955* [Library](library.html): The uri that identifies the library 2956* [Measure](measure.html): The uri that identifies the measure 2957* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 2958* [NamingSystem](namingsystem.html): The uri that identifies the naming system 2959* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 2960* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 2961* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 2962* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 2963* [Requirements](requirements.html): The uri that identifies the requirements 2964* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 2965* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 2966* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 2967* [StructureMap](structuremap.html): The uri that identifies the structure map 2968* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 2969* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 2970* [TestPlan](testplan.html): The uri that identifies the test plan 2971* [TestScript](testscript.html): The uri that identifies the test script 2972* [ValueSet](valueset.html): The uri that identifies the value set 2973</b><br> 2974 * Type: <b>uri</b><br> 2975 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 2976 * </p> 2977 */ 2978 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2979 2980 /** 2981 * Search parameter: <b>version</b> 2982 * <p> 2983 * Description: <b>Multiple Resources: 2984 2985* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 2986* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 2987* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 2988* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 2989* [Citation](citation.html): The business version of the citation 2990* [CodeSystem](codesystem.html): The business version of the code system 2991* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 2992* [ConceptMap](conceptmap.html): The business version of the concept map 2993* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 2994* [EventDefinition](eventdefinition.html): The business version of the event definition 2995* [Evidence](evidence.html): The business version of the evidence 2996* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 2997* [ExampleScenario](examplescenario.html): The business version of the example scenario 2998* [GraphDefinition](graphdefinition.html): The business version of the graph definition 2999* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3000* [Library](library.html): The business version of the library 3001* [Measure](measure.html): The business version of the measure 3002* [MessageDefinition](messagedefinition.html): The business version of the message definition 3003* [NamingSystem](namingsystem.html): The business version of the naming system 3004* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3005* [PlanDefinition](plandefinition.html): The business version of the plan definition 3006* [Questionnaire](questionnaire.html): The business version of the questionnaire 3007* [Requirements](requirements.html): The business version of the requirements 3008* [SearchParameter](searchparameter.html): The business version of the search parameter 3009* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3010* [StructureMap](structuremap.html): The business version of the structure map 3011* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3012* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3013* [TestScript](testscript.html): The business version of the test script 3014* [ValueSet](valueset.html): The business version of the value set 3015</b><br> 3016 * Type: <b>token</b><br> 3017 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3018 * </p> 3019 */ 3020 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 3021 public static final String SP_VERSION = "version"; 3022 /** 3023 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3024 * <p> 3025 * Description: <b>Multiple Resources: 3026 3027* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3028* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3029* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3030* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3031* [Citation](citation.html): The business version of the citation 3032* [CodeSystem](codesystem.html): The business version of the code system 3033* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3034* [ConceptMap](conceptmap.html): The business version of the concept map 3035* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3036* [EventDefinition](eventdefinition.html): The business version of the event definition 3037* [Evidence](evidence.html): The business version of the evidence 3038* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3039* [ExampleScenario](examplescenario.html): The business version of the example scenario 3040* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3041* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3042* [Library](library.html): The business version of the library 3043* [Measure](measure.html): The business version of the measure 3044* [MessageDefinition](messagedefinition.html): The business version of the message definition 3045* [NamingSystem](namingsystem.html): The business version of the naming system 3046* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3047* [PlanDefinition](plandefinition.html): The business version of the plan definition 3048* [Questionnaire](questionnaire.html): The business version of the questionnaire 3049* [Requirements](requirements.html): The business version of the requirements 3050* [SearchParameter](searchparameter.html): The business version of the search parameter 3051* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3052* [StructureMap](structuremap.html): The business version of the structure map 3053* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3054* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3055* [TestScript](testscript.html): The business version of the test script 3056* [ValueSet](valueset.html): The business version of the value set 3057</b><br> 3058 * Type: <b>token</b><br> 3059 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3060 * </p> 3061 */ 3062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3063 3064 3065} 3066