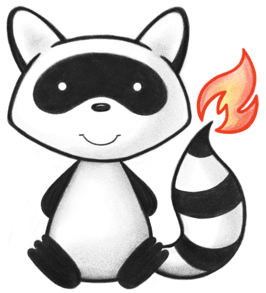
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Address Type: An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 050The ISO21090-codedString may be used to provide a coded representation of the contents of strings in an Address. 051 */ 052@DatatypeDef(name="Address") 053public class Address extends DataType implements ICompositeType { 054 055 public enum AddressType { 056 /** 057 * Mailing addresses - PO Boxes and care-of addresses. 058 */ 059 POSTAL, 060 /** 061 * A physical address that can be visited. 062 */ 063 PHYSICAL, 064 /** 065 * An address that is both physical and postal. 066 */ 067 BOTH, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static AddressType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("postal".equals(codeString)) 076 return POSTAL; 077 if ("physical".equals(codeString)) 078 return PHYSICAL; 079 if ("both".equals(codeString)) 080 return BOTH; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown AddressType code '"+codeString+"'"); 085 } 086 public String toCode() { 087 switch (this) { 088 case POSTAL: return "postal"; 089 case PHYSICAL: return "physical"; 090 case BOTH: return "both"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getSystem() { 096 switch (this) { 097 case POSTAL: return "http://hl7.org/fhir/address-type"; 098 case PHYSICAL: return "http://hl7.org/fhir/address-type"; 099 case BOTH: return "http://hl7.org/fhir/address-type"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getDefinition() { 105 switch (this) { 106 case POSTAL: return "Mailing addresses - PO Boxes and care-of addresses."; 107 case PHYSICAL: return "A physical address that can be visited."; 108 case BOTH: return "An address that is both physical and postal."; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDisplay() { 114 switch (this) { 115 case POSTAL: return "Postal"; 116 case PHYSICAL: return "Physical"; 117 case BOTH: return "Postal & Physical"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 } 123 124 public static class AddressTypeEnumFactory implements EnumFactory<AddressType> { 125 public AddressType fromCode(String codeString) throws IllegalArgumentException { 126 if (codeString == null || "".equals(codeString)) 127 if (codeString == null || "".equals(codeString)) 128 return null; 129 if ("postal".equals(codeString)) 130 return AddressType.POSTAL; 131 if ("physical".equals(codeString)) 132 return AddressType.PHYSICAL; 133 if ("both".equals(codeString)) 134 return AddressType.BOTH; 135 throw new IllegalArgumentException("Unknown AddressType code '"+codeString+"'"); 136 } 137 public Enumeration<AddressType> fromType(PrimitiveType<?> code) throws FHIRException { 138 if (code == null) 139 return null; 140 if (code.isEmpty()) 141 return new Enumeration<AddressType>(this, AddressType.NULL, code); 142 String codeString = ((PrimitiveType) code).asStringValue(); 143 if (codeString == null || "".equals(codeString)) 144 return new Enumeration<AddressType>(this, AddressType.NULL, code); 145 if ("postal".equals(codeString)) 146 return new Enumeration<AddressType>(this, AddressType.POSTAL, code); 147 if ("physical".equals(codeString)) 148 return new Enumeration<AddressType>(this, AddressType.PHYSICAL, code); 149 if ("both".equals(codeString)) 150 return new Enumeration<AddressType>(this, AddressType.BOTH, code); 151 throw new FHIRException("Unknown AddressType code '"+codeString+"'"); 152 } 153 public String toCode(AddressType code) { 154 if (code == AddressType.NULL) 155 return null; 156 if (code == AddressType.POSTAL) 157 return "postal"; 158 if (code == AddressType.PHYSICAL) 159 return "physical"; 160 if (code == AddressType.BOTH) 161 return "both"; 162 return "?"; 163 } 164 public String toSystem(AddressType code) { 165 return code.getSystem(); 166 } 167 } 168 169 public enum AddressUse { 170 /** 171 * A communication address at a home. 172 */ 173 HOME, 174 /** 175 * An office address. First choice for business related contacts during business hours. 176 */ 177 WORK, 178 /** 179 * A temporary address. The period can provide more detailed information. 180 */ 181 TEMP, 182 /** 183 * This address is no longer in use (or was never correct but retained for records). 184 */ 185 OLD, 186 /** 187 * An address to be used to send bills, invoices, receipts etc. 188 */ 189 BILLING, 190 /** 191 * added to help the parsers with the generic types 192 */ 193 NULL; 194 public static AddressUse fromCode(String codeString) throws FHIRException { 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("home".equals(codeString)) 198 return HOME; 199 if ("work".equals(codeString)) 200 return WORK; 201 if ("temp".equals(codeString)) 202 return TEMP; 203 if ("old".equals(codeString)) 204 return OLD; 205 if ("billing".equals(codeString)) 206 return BILLING; 207 if (Configuration.isAcceptInvalidEnums()) 208 return null; 209 else 210 throw new FHIRException("Unknown AddressUse code '"+codeString+"'"); 211 } 212 public String toCode() { 213 switch (this) { 214 case HOME: return "home"; 215 case WORK: return "work"; 216 case TEMP: return "temp"; 217 case OLD: return "old"; 218 case BILLING: return "billing"; 219 case NULL: return null; 220 default: return "?"; 221 } 222 } 223 public String getSystem() { 224 switch (this) { 225 case HOME: return "http://hl7.org/fhir/address-use"; 226 case WORK: return "http://hl7.org/fhir/address-use"; 227 case TEMP: return "http://hl7.org/fhir/address-use"; 228 case OLD: return "http://hl7.org/fhir/address-use"; 229 case BILLING: return "http://hl7.org/fhir/address-use"; 230 case NULL: return null; 231 default: return "?"; 232 } 233 } 234 public String getDefinition() { 235 switch (this) { 236 case HOME: return "A communication address at a home."; 237 case WORK: return "An office address. First choice for business related contacts during business hours."; 238 case TEMP: return "A temporary address. The period can provide more detailed information."; 239 case OLD: return "This address is no longer in use (or was never correct but retained for records)."; 240 case BILLING: return "An address to be used to send bills, invoices, receipts etc."; 241 case NULL: return null; 242 default: return "?"; 243 } 244 } 245 public String getDisplay() { 246 switch (this) { 247 case HOME: return "Home"; 248 case WORK: return "Work"; 249 case TEMP: return "Temporary"; 250 case OLD: return "Old / Incorrect"; 251 case BILLING: return "Billing"; 252 case NULL: return null; 253 default: return "?"; 254 } 255 } 256 } 257 258 public static class AddressUseEnumFactory implements EnumFactory<AddressUse> { 259 public AddressUse fromCode(String codeString) throws IllegalArgumentException { 260 if (codeString == null || "".equals(codeString)) 261 if (codeString == null || "".equals(codeString)) 262 return null; 263 if ("home".equals(codeString)) 264 return AddressUse.HOME; 265 if ("work".equals(codeString)) 266 return AddressUse.WORK; 267 if ("temp".equals(codeString)) 268 return AddressUse.TEMP; 269 if ("old".equals(codeString)) 270 return AddressUse.OLD; 271 if ("billing".equals(codeString)) 272 return AddressUse.BILLING; 273 throw new IllegalArgumentException("Unknown AddressUse code '"+codeString+"'"); 274 } 275 public Enumeration<AddressUse> fromType(PrimitiveType<?> code) throws FHIRException { 276 if (code == null) 277 return null; 278 if (code.isEmpty()) 279 return new Enumeration<AddressUse>(this, AddressUse.NULL, code); 280 String codeString = ((PrimitiveType) code).asStringValue(); 281 if (codeString == null || "".equals(codeString)) 282 return new Enumeration<AddressUse>(this, AddressUse.NULL, code); 283 if ("home".equals(codeString)) 284 return new Enumeration<AddressUse>(this, AddressUse.HOME, code); 285 if ("work".equals(codeString)) 286 return new Enumeration<AddressUse>(this, AddressUse.WORK, code); 287 if ("temp".equals(codeString)) 288 return new Enumeration<AddressUse>(this, AddressUse.TEMP, code); 289 if ("old".equals(codeString)) 290 return new Enumeration<AddressUse>(this, AddressUse.OLD, code); 291 if ("billing".equals(codeString)) 292 return new Enumeration<AddressUse>(this, AddressUse.BILLING, code); 293 throw new FHIRException("Unknown AddressUse code '"+codeString+"'"); 294 } 295 public String toCode(AddressUse code) { 296 if (code == AddressUse.NULL) 297 return null; 298 if (code == AddressUse.HOME) 299 return "home"; 300 if (code == AddressUse.WORK) 301 return "work"; 302 if (code == AddressUse.TEMP) 303 return "temp"; 304 if (code == AddressUse.OLD) 305 return "old"; 306 if (code == AddressUse.BILLING) 307 return "billing"; 308 return "?"; 309 } 310 public String toSystem(AddressUse code) { 311 return code.getSystem(); 312 } 313 } 314 315 /** 316 * The purpose of this address. 317 */ 318 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 319 @Description(shortDefinition="home | work | temp | old | billing - purpose of this address", formalDefinition="The purpose of this address." ) 320 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/address-use") 321 protected Enumeration<AddressUse> use; 322 323 /** 324 * Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 325 */ 326 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 327 @Description(shortDefinition="postal | physical | both", formalDefinition="Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both." ) 328 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/address-type") 329 protected Enumeration<AddressType> type; 330 331 /** 332 * Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts. 333 */ 334 @Child(name = "text", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 335 @Description(shortDefinition="Text representation of the address", formalDefinition="Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts." ) 336 protected StringType text; 337 338 /** 339 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information. 340 */ 341 @Child(name = "line", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 342 @Description(shortDefinition="Street name, number, direction & P.O. Box etc.", formalDefinition="This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information." ) 343 protected List<StringType> line; 344 345 /** 346 * The name of the city, town, suburb, village or other community or delivery center. 347 */ 348 @Child(name = "city", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 349 @Description(shortDefinition="Name of city, town etc.", formalDefinition="The name of the city, town, suburb, village or other community or delivery center." ) 350 protected StringType city; 351 352 /** 353 * The name of the administrative area (county). 354 */ 355 @Child(name = "district", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 356 @Description(shortDefinition="District name (aka county)", formalDefinition="The name of the administrative area (county)." ) 357 protected StringType district; 358 359 /** 360 * Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes). 361 */ 362 @Child(name = "state", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 363 @Description(shortDefinition="Sub-unit of country (abbreviations ok)", formalDefinition="Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes)." ) 364 protected StringType state; 365 366 /** 367 * A postal code designating a region defined by the postal service. 368 */ 369 @Child(name = "postalCode", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 370 @Description(shortDefinition="Postal code for area", formalDefinition="A postal code designating a region defined by the postal service." ) 371 protected StringType postalCode; 372 373 /** 374 * Country - a nation as commonly understood or generally accepted. 375 */ 376 @Child(name = "country", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 377 @Description(shortDefinition="Country (e.g. may be ISO 3166 2 or 3 letter code)", formalDefinition="Country - a nation as commonly understood or generally accepted." ) 378 protected StringType country; 379 380 /** 381 * Time period when address was/is in use. 382 */ 383 @Child(name = "period", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 384 @Description(shortDefinition="Time period when address was/is in use", formalDefinition="Time period when address was/is in use." ) 385 protected Period period; 386 387 private static final long serialVersionUID = 561490318L; 388 389 /** 390 * Constructor 391 */ 392 public Address() { 393 super(); 394 } 395 396 /** 397 * @return {@link #use} (The purpose of this address.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 398 */ 399 public Enumeration<AddressUse> getUseElement() { 400 if (this.use == null) 401 if (Configuration.errorOnAutoCreate()) 402 throw new Error("Attempt to auto-create Address.use"); 403 else if (Configuration.doAutoCreate()) 404 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); // bb 405 return this.use; 406 } 407 408 public boolean hasUseElement() { 409 return this.use != null && !this.use.isEmpty(); 410 } 411 412 public boolean hasUse() { 413 return this.use != null && !this.use.isEmpty(); 414 } 415 416 /** 417 * @param value {@link #use} (The purpose of this address.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 418 */ 419 public Address setUseElement(Enumeration<AddressUse> value) { 420 this.use = value; 421 return this; 422 } 423 424 /** 425 * @return The purpose of this address. 426 */ 427 public AddressUse getUse() { 428 return this.use == null ? null : this.use.getValue(); 429 } 430 431 /** 432 * @param value The purpose of this address. 433 */ 434 public Address setUse(AddressUse value) { 435 if (value == null) 436 this.use = null; 437 else { 438 if (this.use == null) 439 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); 440 this.use.setValue(value); 441 } 442 return this; 443 } 444 445 /** 446 * @return {@link #type} (Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 447 */ 448 public Enumeration<AddressType> getTypeElement() { 449 if (this.type == null) 450 if (Configuration.errorOnAutoCreate()) 451 throw new Error("Attempt to auto-create Address.type"); 452 else if (Configuration.doAutoCreate()) 453 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); // bb 454 return this.type; 455 } 456 457 public boolean hasTypeElement() { 458 return this.type != null && !this.type.isEmpty(); 459 } 460 461 public boolean hasType() { 462 return this.type != null && !this.type.isEmpty(); 463 } 464 465 /** 466 * @param value {@link #type} (Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 467 */ 468 public Address setTypeElement(Enumeration<AddressType> value) { 469 this.type = value; 470 return this; 471 } 472 473 /** 474 * @return Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 475 */ 476 public AddressType getType() { 477 return this.type == null ? null : this.type.getValue(); 478 } 479 480 /** 481 * @param value Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 482 */ 483 public Address setType(AddressType value) { 484 if (value == null) 485 this.type = null; 486 else { 487 if (this.type == null) 488 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); 489 this.type.setValue(value); 490 } 491 return this; 492 } 493 494 /** 495 * @return {@link #text} (Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 496 */ 497 public StringType getTextElement() { 498 if (this.text == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create Address.text"); 501 else if (Configuration.doAutoCreate()) 502 this.text = new StringType(); // bb 503 return this.text; 504 } 505 506 public boolean hasTextElement() { 507 return this.text != null && !this.text.isEmpty(); 508 } 509 510 public boolean hasText() { 511 return this.text != null && !this.text.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #text} (Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 516 */ 517 public Address setTextElement(StringType value) { 518 this.text = value; 519 return this; 520 } 521 522 /** 523 * @return Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts. 524 */ 525 public String getText() { 526 return this.text == null ? null : this.text.getValue(); 527 } 528 529 /** 530 * @param value Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts. 531 */ 532 public Address setText(String value) { 533 if (Utilities.noString(value)) 534 this.text = null; 535 else { 536 if (this.text == null) 537 this.text = new StringType(); 538 this.text.setValue(value); 539 } 540 return this; 541 } 542 543 /** 544 * @return {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 545 */ 546 public List<StringType> getLine() { 547 if (this.line == null) 548 this.line = new ArrayList<StringType>(); 549 return this.line; 550 } 551 552 /** 553 * @return Returns a reference to <code>this</code> for easy method chaining 554 */ 555 public Address setLine(List<StringType> theLine) { 556 this.line = theLine; 557 return this; 558 } 559 560 public boolean hasLine() { 561 if (this.line == null) 562 return false; 563 for (StringType item : this.line) 564 if (!item.isEmpty()) 565 return true; 566 return false; 567 } 568 569 /** 570 * @return {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 571 */ 572 public StringType addLineElement() {//2 573 StringType t = new StringType(); 574 if (this.line == null) 575 this.line = new ArrayList<StringType>(); 576 this.line.add(t); 577 return t; 578 } 579 580 /** 581 * @param value {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 582 */ 583 public Address addLine(String value) { //1 584 StringType t = new StringType(); 585 t.setValue(value); 586 if (this.line == null) 587 this.line = new ArrayList<StringType>(); 588 this.line.add(t); 589 return this; 590 } 591 592 /** 593 * @param value {@link #line} (This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.) 594 */ 595 public boolean hasLine(String value) { 596 if (this.line == null) 597 return false; 598 for (StringType v : this.line) 599 if (v.getValue().equals(value)) // string 600 return true; 601 return false; 602 } 603 604 /** 605 * @return {@link #city} (The name of the city, town, suburb, village or other community or delivery center.). This is the underlying object with id, value and extensions. The accessor "getCity" gives direct access to the value 606 */ 607 public StringType getCityElement() { 608 if (this.city == null) 609 if (Configuration.errorOnAutoCreate()) 610 throw new Error("Attempt to auto-create Address.city"); 611 else if (Configuration.doAutoCreate()) 612 this.city = new StringType(); // bb 613 return this.city; 614 } 615 616 public boolean hasCityElement() { 617 return this.city != null && !this.city.isEmpty(); 618 } 619 620 public boolean hasCity() { 621 return this.city != null && !this.city.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #city} (The name of the city, town, suburb, village or other community or delivery center.). This is the underlying object with id, value and extensions. The accessor "getCity" gives direct access to the value 626 */ 627 public Address setCityElement(StringType value) { 628 this.city = value; 629 return this; 630 } 631 632 /** 633 * @return The name of the city, town, suburb, village or other community or delivery center. 634 */ 635 public String getCity() { 636 return this.city == null ? null : this.city.getValue(); 637 } 638 639 /** 640 * @param value The name of the city, town, suburb, village or other community or delivery center. 641 */ 642 public Address setCity(String value) { 643 if (Utilities.noString(value)) 644 this.city = null; 645 else { 646 if (this.city == null) 647 this.city = new StringType(); 648 this.city.setValue(value); 649 } 650 return this; 651 } 652 653 /** 654 * @return {@link #district} (The name of the administrative area (county).). This is the underlying object with id, value and extensions. The accessor "getDistrict" gives direct access to the value 655 */ 656 public StringType getDistrictElement() { 657 if (this.district == null) 658 if (Configuration.errorOnAutoCreate()) 659 throw new Error("Attempt to auto-create Address.district"); 660 else if (Configuration.doAutoCreate()) 661 this.district = new StringType(); // bb 662 return this.district; 663 } 664 665 public boolean hasDistrictElement() { 666 return this.district != null && !this.district.isEmpty(); 667 } 668 669 public boolean hasDistrict() { 670 return this.district != null && !this.district.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #district} (The name of the administrative area (county).). This is the underlying object with id, value and extensions. The accessor "getDistrict" gives direct access to the value 675 */ 676 public Address setDistrictElement(StringType value) { 677 this.district = value; 678 return this; 679 } 680 681 /** 682 * @return The name of the administrative area (county). 683 */ 684 public String getDistrict() { 685 return this.district == null ? null : this.district.getValue(); 686 } 687 688 /** 689 * @param value The name of the administrative area (county). 690 */ 691 public Address setDistrict(String value) { 692 if (Utilities.noString(value)) 693 this.district = null; 694 else { 695 if (this.district == null) 696 this.district = new StringType(); 697 this.district.setValue(value); 698 } 699 return this; 700 } 701 702 /** 703 * @return {@link #state} (Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 704 */ 705 public StringType getStateElement() { 706 if (this.state == null) 707 if (Configuration.errorOnAutoCreate()) 708 throw new Error("Attempt to auto-create Address.state"); 709 else if (Configuration.doAutoCreate()) 710 this.state = new StringType(); // bb 711 return this.state; 712 } 713 714 public boolean hasStateElement() { 715 return this.state != null && !this.state.isEmpty(); 716 } 717 718 public boolean hasState() { 719 return this.state != null && !this.state.isEmpty(); 720 } 721 722 /** 723 * @param value {@link #state} (Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 724 */ 725 public Address setStateElement(StringType value) { 726 this.state = value; 727 return this; 728 } 729 730 /** 731 * @return Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes). 732 */ 733 public String getState() { 734 return this.state == null ? null : this.state.getValue(); 735 } 736 737 /** 738 * @param value Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes). 739 */ 740 public Address setState(String value) { 741 if (Utilities.noString(value)) 742 this.state = null; 743 else { 744 if (this.state == null) 745 this.state = new StringType(); 746 this.state.setValue(value); 747 } 748 return this; 749 } 750 751 /** 752 * @return {@link #postalCode} (A postal code designating a region defined by the postal service.). This is the underlying object with id, value and extensions. The accessor "getPostalCode" gives direct access to the value 753 */ 754 public StringType getPostalCodeElement() { 755 if (this.postalCode == null) 756 if (Configuration.errorOnAutoCreate()) 757 throw new Error("Attempt to auto-create Address.postalCode"); 758 else if (Configuration.doAutoCreate()) 759 this.postalCode = new StringType(); // bb 760 return this.postalCode; 761 } 762 763 public boolean hasPostalCodeElement() { 764 return this.postalCode != null && !this.postalCode.isEmpty(); 765 } 766 767 public boolean hasPostalCode() { 768 return this.postalCode != null && !this.postalCode.isEmpty(); 769 } 770 771 /** 772 * @param value {@link #postalCode} (A postal code designating a region defined by the postal service.). This is the underlying object with id, value and extensions. The accessor "getPostalCode" gives direct access to the value 773 */ 774 public Address setPostalCodeElement(StringType value) { 775 this.postalCode = value; 776 return this; 777 } 778 779 /** 780 * @return A postal code designating a region defined by the postal service. 781 */ 782 public String getPostalCode() { 783 return this.postalCode == null ? null : this.postalCode.getValue(); 784 } 785 786 /** 787 * @param value A postal code designating a region defined by the postal service. 788 */ 789 public Address setPostalCode(String value) { 790 if (Utilities.noString(value)) 791 this.postalCode = null; 792 else { 793 if (this.postalCode == null) 794 this.postalCode = new StringType(); 795 this.postalCode.setValue(value); 796 } 797 return this; 798 } 799 800 /** 801 * @return {@link #country} (Country - a nation as commonly understood or generally accepted.). This is the underlying object with id, value and extensions. The accessor "getCountry" gives direct access to the value 802 */ 803 public StringType getCountryElement() { 804 if (this.country == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create Address.country"); 807 else if (Configuration.doAutoCreate()) 808 this.country = new StringType(); // bb 809 return this.country; 810 } 811 812 public boolean hasCountryElement() { 813 return this.country != null && !this.country.isEmpty(); 814 } 815 816 public boolean hasCountry() { 817 return this.country != null && !this.country.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #country} (Country - a nation as commonly understood or generally accepted.). This is the underlying object with id, value and extensions. The accessor "getCountry" gives direct access to the value 822 */ 823 public Address setCountryElement(StringType value) { 824 this.country = value; 825 return this; 826 } 827 828 /** 829 * @return Country - a nation as commonly understood or generally accepted. 830 */ 831 public String getCountry() { 832 return this.country == null ? null : this.country.getValue(); 833 } 834 835 /** 836 * @param value Country - a nation as commonly understood or generally accepted. 837 */ 838 public Address setCountry(String value) { 839 if (Utilities.noString(value)) 840 this.country = null; 841 else { 842 if (this.country == null) 843 this.country = new StringType(); 844 this.country.setValue(value); 845 } 846 return this; 847 } 848 849 /** 850 * @return {@link #period} (Time period when address was/is in use.) 851 */ 852 public Period getPeriod() { 853 if (this.period == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create Address.period"); 856 else if (Configuration.doAutoCreate()) 857 this.period = new Period(); // cc 858 return this.period; 859 } 860 861 public boolean hasPeriod() { 862 return this.period != null && !this.period.isEmpty(); 863 } 864 865 /** 866 * @param value {@link #period} (Time period when address was/is in use.) 867 */ 868 public Address setPeriod(Period value) { 869 this.period = value; 870 return this; 871 } 872 873 protected void listChildren(List<Property> children) { 874 super.listChildren(children); 875 children.add(new Property("use", "code", "The purpose of this address.", 0, 1, use)); 876 children.add(new Property("type", "code", "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 0, 1, type)); 877 children.add(new Property("text", "string", "Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.", 0, 1, text)); 878 children.add(new Property("line", "string", "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 0, java.lang.Integer.MAX_VALUE, line)); 879 children.add(new Property("city", "string", "The name of the city, town, suburb, village or other community or delivery center.", 0, 1, city)); 880 children.add(new Property("district", "string", "The name of the administrative area (county).", 0, 1, district)); 881 children.add(new Property("state", "string", "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).", 0, 1, state)); 882 children.add(new Property("postalCode", "string", "A postal code designating a region defined by the postal service.", 0, 1, postalCode)); 883 children.add(new Property("country", "string", "Country - a nation as commonly understood or generally accepted.", 0, 1, country)); 884 children.add(new Property("period", "Period", "Time period when address was/is in use.", 0, 1, period)); 885 } 886 887 @Override 888 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 889 switch (_hash) { 890 case 116103: /*use*/ return new Property("use", "code", "The purpose of this address.", 0, 1, use); 891 case 3575610: /*type*/ return new Property("type", "code", "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 0, 1, type); 892 case 3556653: /*text*/ return new Property("text", "string", "Specifies the entire address as it should be displayed e.g. on a postal label. This may be provided instead of or as well as the specific parts.", 0, 1, text); 893 case 3321844: /*line*/ return new Property("line", "string", "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 0, java.lang.Integer.MAX_VALUE, line); 894 case 3053931: /*city*/ return new Property("city", "string", "The name of the city, town, suburb, village or other community or delivery center.", 0, 1, city); 895 case 288961422: /*district*/ return new Property("district", "string", "The name of the administrative area (county).", 0, 1, district); 896 case 109757585: /*state*/ return new Property("state", "string", "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (e.g. US 2 letter state codes).", 0, 1, state); 897 case 2011152728: /*postalCode*/ return new Property("postalCode", "string", "A postal code designating a region defined by the postal service.", 0, 1, postalCode); 898 case 957831062: /*country*/ return new Property("country", "string", "Country - a nation as commonly understood or generally accepted.", 0, 1, country); 899 case -991726143: /*period*/ return new Property("period", "Period", "Time period when address was/is in use.", 0, 1, period); 900 default: return super.getNamedProperty(_hash, _name, _checkValid); 901 } 902 903 } 904 905 @Override 906 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 907 switch (hash) { 908 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<AddressUse> 909 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<AddressType> 910 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 911 case 3321844: /*line*/ return this.line == null ? new Base[0] : this.line.toArray(new Base[this.line.size()]); // StringType 912 case 3053931: /*city*/ return this.city == null ? new Base[0] : new Base[] {this.city}; // StringType 913 case 288961422: /*district*/ return this.district == null ? new Base[0] : new Base[] {this.district}; // StringType 914 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // StringType 915 case 2011152728: /*postalCode*/ return this.postalCode == null ? new Base[0] : new Base[] {this.postalCode}; // StringType 916 case 957831062: /*country*/ return this.country == null ? new Base[0] : new Base[] {this.country}; // StringType 917 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 918 default: return super.getProperty(hash, name, checkValid); 919 } 920 921 } 922 923 @Override 924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 925 switch (hash) { 926 case 116103: // use 927 value = new AddressUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 928 this.use = (Enumeration) value; // Enumeration<AddressUse> 929 return value; 930 case 3575610: // type 931 value = new AddressTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 932 this.type = (Enumeration) value; // Enumeration<AddressType> 933 return value; 934 case 3556653: // text 935 this.text = TypeConvertor.castToString(value); // StringType 936 return value; 937 case 3321844: // line 938 this.getLine().add(TypeConvertor.castToString(value)); // StringType 939 return value; 940 case 3053931: // city 941 this.city = TypeConvertor.castToString(value); // StringType 942 return value; 943 case 288961422: // district 944 this.district = TypeConvertor.castToString(value); // StringType 945 return value; 946 case 109757585: // state 947 this.state = TypeConvertor.castToString(value); // StringType 948 return value; 949 case 2011152728: // postalCode 950 this.postalCode = TypeConvertor.castToString(value); // StringType 951 return value; 952 case 957831062: // country 953 this.country = TypeConvertor.castToString(value); // StringType 954 return value; 955 case -991726143: // period 956 this.period = TypeConvertor.castToPeriod(value); // Period 957 return value; 958 default: return super.setProperty(hash, name, value); 959 } 960 961 } 962 963 @Override 964 public Base setProperty(String name, Base value) throws FHIRException { 965 if (name.equals("use")) { 966 value = new AddressUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 967 this.use = (Enumeration) value; // Enumeration<AddressUse> 968 } else if (name.equals("type")) { 969 value = new AddressTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 970 this.type = (Enumeration) value; // Enumeration<AddressType> 971 } else if (name.equals("text")) { 972 this.text = TypeConvertor.castToString(value); // StringType 973 } else if (name.equals("line")) { 974 this.getLine().add(TypeConvertor.castToString(value)); 975 } else if (name.equals("city")) { 976 this.city = TypeConvertor.castToString(value); // StringType 977 } else if (name.equals("district")) { 978 this.district = TypeConvertor.castToString(value); // StringType 979 } else if (name.equals("state")) { 980 this.state = TypeConvertor.castToString(value); // StringType 981 } else if (name.equals("postalCode")) { 982 this.postalCode = TypeConvertor.castToString(value); // StringType 983 } else if (name.equals("country")) { 984 this.country = TypeConvertor.castToString(value); // StringType 985 } else if (name.equals("period")) { 986 this.period = TypeConvertor.castToPeriod(value); // Period 987 } else 988 return super.setProperty(name, value); 989 return value; 990 } 991 992 @Override 993 public void removeChild(String name, Base value) throws FHIRException { 994 if (name.equals("use")) { 995 value = new AddressUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 996 this.use = (Enumeration) value; // Enumeration<AddressUse> 997 } else if (name.equals("type")) { 998 value = new AddressTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 999 this.type = (Enumeration) value; // Enumeration<AddressType> 1000 } else if (name.equals("text")) { 1001 this.text = null; 1002 } else if (name.equals("line")) { 1003 this.getLine().remove(value); 1004 } else if (name.equals("city")) { 1005 this.city = null; 1006 } else if (name.equals("district")) { 1007 this.district = null; 1008 } else if (name.equals("state")) { 1009 this.state = null; 1010 } else if (name.equals("postalCode")) { 1011 this.postalCode = null; 1012 } else if (name.equals("country")) { 1013 this.country = null; 1014 } else if (name.equals("period")) { 1015 this.period = null; 1016 } else 1017 super.removeChild(name, value); 1018 1019 } 1020 1021 @Override 1022 public Base makeProperty(int hash, String name) throws FHIRException { 1023 switch (hash) { 1024 case 116103: return getUseElement(); 1025 case 3575610: return getTypeElement(); 1026 case 3556653: return getTextElement(); 1027 case 3321844: return addLineElement(); 1028 case 3053931: return getCityElement(); 1029 case 288961422: return getDistrictElement(); 1030 case 109757585: return getStateElement(); 1031 case 2011152728: return getPostalCodeElement(); 1032 case 957831062: return getCountryElement(); 1033 case -991726143: return getPeriod(); 1034 default: return super.makeProperty(hash, name); 1035 } 1036 1037 } 1038 1039 @Override 1040 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1041 switch (hash) { 1042 case 116103: /*use*/ return new String[] {"code"}; 1043 case 3575610: /*type*/ return new String[] {"code"}; 1044 case 3556653: /*text*/ return new String[] {"string"}; 1045 case 3321844: /*line*/ return new String[] {"string"}; 1046 case 3053931: /*city*/ return new String[] {"string"}; 1047 case 288961422: /*district*/ return new String[] {"string"}; 1048 case 109757585: /*state*/ return new String[] {"string"}; 1049 case 2011152728: /*postalCode*/ return new String[] {"string"}; 1050 case 957831062: /*country*/ return new String[] {"string"}; 1051 case -991726143: /*period*/ return new String[] {"Period"}; 1052 default: return super.getTypesForProperty(hash, name); 1053 } 1054 1055 } 1056 1057 @Override 1058 public Base addChild(String name) throws FHIRException { 1059 if (name.equals("use")) { 1060 throw new FHIRException("Cannot call addChild on a singleton property Address.use"); 1061 } 1062 else if (name.equals("type")) { 1063 throw new FHIRException("Cannot call addChild on a singleton property Address.type"); 1064 } 1065 else if (name.equals("text")) { 1066 throw new FHIRException("Cannot call addChild on a singleton property Address.text"); 1067 } 1068 else if (name.equals("line")) { 1069 throw new FHIRException("Cannot call addChild on a singleton property Address.line"); 1070 } 1071 else if (name.equals("city")) { 1072 throw new FHIRException("Cannot call addChild on a singleton property Address.city"); 1073 } 1074 else if (name.equals("district")) { 1075 throw new FHIRException("Cannot call addChild on a singleton property Address.district"); 1076 } 1077 else if (name.equals("state")) { 1078 throw new FHIRException("Cannot call addChild on a singleton property Address.state"); 1079 } 1080 else if (name.equals("postalCode")) { 1081 throw new FHIRException("Cannot call addChild on a singleton property Address.postalCode"); 1082 } 1083 else if (name.equals("country")) { 1084 throw new FHIRException("Cannot call addChild on a singleton property Address.country"); 1085 } 1086 else if (name.equals("period")) { 1087 this.period = new Period(); 1088 return this.period; 1089 } 1090 else 1091 return super.addChild(name); 1092 } 1093 1094 public String fhirType() { 1095 return "Address"; 1096 1097 } 1098 1099 public Address copy() { 1100 Address dst = new Address(); 1101 copyValues(dst); 1102 return dst; 1103 } 1104 1105 public void copyValues(Address dst) { 1106 super.copyValues(dst); 1107 dst.use = use == null ? null : use.copy(); 1108 dst.type = type == null ? null : type.copy(); 1109 dst.text = text == null ? null : text.copy(); 1110 if (line != null) { 1111 dst.line = new ArrayList<StringType>(); 1112 for (StringType i : line) 1113 dst.line.add(i.copy()); 1114 }; 1115 dst.city = city == null ? null : city.copy(); 1116 dst.district = district == null ? null : district.copy(); 1117 dst.state = state == null ? null : state.copy(); 1118 dst.postalCode = postalCode == null ? null : postalCode.copy(); 1119 dst.country = country == null ? null : country.copy(); 1120 dst.period = period == null ? null : period.copy(); 1121 } 1122 1123 protected Address typedCopy() { 1124 return copy(); 1125 } 1126 1127 @Override 1128 public boolean equalsDeep(Base other_) { 1129 if (!super.equalsDeep(other_)) 1130 return false; 1131 if (!(other_ instanceof Address)) 1132 return false; 1133 Address o = (Address) other_; 1134 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 1135 && compareDeep(line, o.line, true) && compareDeep(city, o.city, true) && compareDeep(district, o.district, true) 1136 && compareDeep(state, o.state, true) && compareDeep(postalCode, o.postalCode, true) && compareDeep(country, o.country, true) 1137 && compareDeep(period, o.period, true); 1138 } 1139 1140 @Override 1141 public boolean equalsShallow(Base other_) { 1142 if (!super.equalsShallow(other_)) 1143 return false; 1144 if (!(other_ instanceof Address)) 1145 return false; 1146 Address o = (Address) other_; 1147 return compareValues(use, o.use, true) && compareValues(type, o.type, true) && compareValues(text, o.text, true) 1148 && compareValues(line, o.line, true) && compareValues(city, o.city, true) && compareValues(district, o.district, true) 1149 && compareValues(state, o.state, true) && compareValues(postalCode, o.postalCode, true) && compareValues(country, o.country, true) 1150 ; 1151 } 1152 1153 public boolean isEmpty() { 1154 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, type, text, line 1155 , city, district, state, postalCode, country, period); 1156 } 1157 1158 1159} 1160