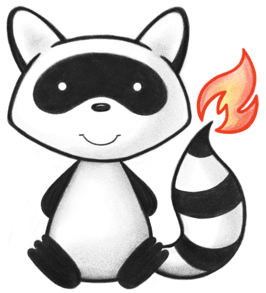
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A medicinal product in the final form which is suitable for administering to a patient (after any mixing of multiple components, dissolution etc. has been performed). 052 */ 053@ResourceDef(name="AdministrableProductDefinition", profile="http://hl7.org/fhir/StructureDefinition/AdministrableProductDefinition") 054public class AdministrableProductDefinition extends DomainResource { 055 056 @Block() 057 public static class AdministrableProductDefinitionPropertyComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A code expressing the type of characteristic. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="A code expressing the type of characteristic", formalDefinition="A code expressing the type of characteristic." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-characteristic-codes") 064 protected CodeableConcept type; 065 066 /** 067 * A value for the characteristic. 068 */ 069 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, DateType.class, BooleanType.class, MarkdownType.class, Attachment.class, Binary.class}, order=2, min=0, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="A value for the characteristic", formalDefinition="A value for the characteristic." ) 071 protected DataType value; 072 073 /** 074 * The status of characteristic e.g. assigned or pending. 075 */ 076 @Child(name = "status", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 077 @Description(shortDefinition="The status of characteristic e.g. assigned or pending", formalDefinition="The status of characteristic e.g. assigned or pending." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 079 protected CodeableConcept status; 080 081 private static final long serialVersionUID = -872048207L; 082 083 /** 084 * Constructor 085 */ 086 public AdministrableProductDefinitionPropertyComponent() { 087 super(); 088 } 089 090 /** 091 * Constructor 092 */ 093 public AdministrableProductDefinitionPropertyComponent(CodeableConcept type) { 094 super(); 095 this.setType(type); 096 } 097 098 /** 099 * @return {@link #type} (A code expressing the type of characteristic.) 100 */ 101 public CodeableConcept getType() { 102 if (this.type == null) 103 if (Configuration.errorOnAutoCreate()) 104 throw new Error("Attempt to auto-create AdministrableProductDefinitionPropertyComponent.type"); 105 else if (Configuration.doAutoCreate()) 106 this.type = new CodeableConcept(); // cc 107 return this.type; 108 } 109 110 public boolean hasType() { 111 return this.type != null && !this.type.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #type} (A code expressing the type of characteristic.) 116 */ 117 public AdministrableProductDefinitionPropertyComponent setType(CodeableConcept value) { 118 this.type = value; 119 return this; 120 } 121 122 /** 123 * @return {@link #value} (A value for the characteristic.) 124 */ 125 public DataType getValue() { 126 return this.value; 127 } 128 129 /** 130 * @return {@link #value} (A value for the characteristic.) 131 */ 132 public CodeableConcept getValueCodeableConcept() throws FHIRException { 133 if (this.value == null) 134 this.value = new CodeableConcept(); 135 if (!(this.value instanceof CodeableConcept)) 136 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 137 return (CodeableConcept) this.value; 138 } 139 140 public boolean hasValueCodeableConcept() { 141 return this.value instanceof CodeableConcept; 142 } 143 144 /** 145 * @return {@link #value} (A value for the characteristic.) 146 */ 147 public Quantity getValueQuantity() throws FHIRException { 148 if (this.value == null) 149 this.value = new Quantity(); 150 if (!(this.value instanceof Quantity)) 151 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 152 return (Quantity) this.value; 153 } 154 155 public boolean hasValueQuantity() { 156 return this.value instanceof Quantity; 157 } 158 159 /** 160 * @return {@link #value} (A value for the characteristic.) 161 */ 162 public DateType getValueDateType() throws FHIRException { 163 if (this.value == null) 164 this.value = new DateType(); 165 if (!(this.value instanceof DateType)) 166 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 167 return (DateType) this.value; 168 } 169 170 public boolean hasValueDateType() { 171 return this.value instanceof DateType; 172 } 173 174 /** 175 * @return {@link #value} (A value for the characteristic.) 176 */ 177 public BooleanType getValueBooleanType() throws FHIRException { 178 if (this.value == null) 179 this.value = new BooleanType(); 180 if (!(this.value instanceof BooleanType)) 181 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 182 return (BooleanType) this.value; 183 } 184 185 public boolean hasValueBooleanType() { 186 return this.value instanceof BooleanType; 187 } 188 189 /** 190 * @return {@link #value} (A value for the characteristic.) 191 */ 192 public MarkdownType getValueMarkdownType() throws FHIRException { 193 if (this.value == null) 194 this.value = new MarkdownType(); 195 if (!(this.value instanceof MarkdownType)) 196 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 197 return (MarkdownType) this.value; 198 } 199 200 public boolean hasValueMarkdownType() { 201 return this.value instanceof MarkdownType; 202 } 203 204 /** 205 * @return {@link #value} (A value for the characteristic.) 206 */ 207 public Attachment getValueAttachment() throws FHIRException { 208 if (this.value == null) 209 this.value = new Attachment(); 210 if (!(this.value instanceof Attachment)) 211 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 212 return (Attachment) this.value; 213 } 214 215 public boolean hasValueAttachment() { 216 return this.value instanceof Attachment; 217 } 218 219 /** 220 * @return {@link #value} (A value for the characteristic.) 221 */ 222 public Reference getValueReference() throws FHIRException { 223 if (this.value == null) 224 this.value = new Reference(); 225 if (!(this.value instanceof Reference)) 226 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 227 return (Reference) this.value; 228 } 229 230 public boolean hasValueReference() { 231 return this.value instanceof Reference; 232 } 233 234 public boolean hasValue() { 235 return this.value != null && !this.value.isEmpty(); 236 } 237 238 /** 239 * @param value {@link #value} (A value for the characteristic.) 240 */ 241 public AdministrableProductDefinitionPropertyComponent setValue(DataType value) { 242 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof DateType || value instanceof BooleanType || value instanceof MarkdownType || value instanceof Attachment || value instanceof Reference)) 243 throw new FHIRException("Not the right type for AdministrableProductDefinition.property.value[x]: "+value.fhirType()); 244 this.value = value; 245 return this; 246 } 247 248 /** 249 * @return {@link #status} (The status of characteristic e.g. assigned or pending.) 250 */ 251 public CodeableConcept getStatus() { 252 if (this.status == null) 253 if (Configuration.errorOnAutoCreate()) 254 throw new Error("Attempt to auto-create AdministrableProductDefinitionPropertyComponent.status"); 255 else if (Configuration.doAutoCreate()) 256 this.status = new CodeableConcept(); // cc 257 return this.status; 258 } 259 260 public boolean hasStatus() { 261 return this.status != null && !this.status.isEmpty(); 262 } 263 264 /** 265 * @param value {@link #status} (The status of characteristic e.g. assigned or pending.) 266 */ 267 public AdministrableProductDefinitionPropertyComponent setStatus(CodeableConcept value) { 268 this.status = value; 269 return this; 270 } 271 272 protected void listChildren(List<Property> children) { 273 super.listChildren(children); 274 children.add(new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type)); 275 children.add(new Property("value[x]", "CodeableConcept|Quantity|date|boolean|markdown|Attachment|Reference(Binary)", "A value for the characteristic.", 0, 1, value)); 276 children.add(new Property("status", "CodeableConcept", "The status of characteristic e.g. assigned or pending.", 0, 1, status)); 277 } 278 279 @Override 280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 281 switch (_hash) { 282 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type); 283 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|markdown|Attachment|Reference(Binary)", "A value for the characteristic.", 0, 1, value); 284 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|markdown|Attachment|Reference(Binary)", "A value for the characteristic.", 0, 1, value); 285 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "A value for the characteristic.", 0, 1, value); 286 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "A value for the characteristic.", 0, 1, value); 287 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "A value for the characteristic.", 0, 1, value); 288 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "A value for the characteristic.", 0, 1, value); 289 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "A value for the characteristic.", 0, 1, value); 290 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "A value for the characteristic.", 0, 1, value); 291 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Binary)", "A value for the characteristic.", 0, 1, value); 292 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status of characteristic e.g. assigned or pending.", 0, 1, status); 293 default: return super.getNamedProperty(_hash, _name, _checkValid); 294 } 295 296 } 297 298 @Override 299 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 300 switch (hash) { 301 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 302 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 303 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 304 default: return super.getProperty(hash, name, checkValid); 305 } 306 307 } 308 309 @Override 310 public Base setProperty(int hash, String name, Base value) throws FHIRException { 311 switch (hash) { 312 case 3575610: // type 313 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 314 return value; 315 case 111972721: // value 316 this.value = TypeConvertor.castToType(value); // DataType 317 return value; 318 case -892481550: // status 319 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 320 return value; 321 default: return super.setProperty(hash, name, value); 322 } 323 324 } 325 326 @Override 327 public Base setProperty(String name, Base value) throws FHIRException { 328 if (name.equals("type")) { 329 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 330 } else if (name.equals("value[x]")) { 331 this.value = TypeConvertor.castToType(value); // DataType 332 } else if (name.equals("status")) { 333 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 334 } else 335 return super.setProperty(name, value); 336 return value; 337 } 338 339 @Override 340 public void removeChild(String name, Base value) throws FHIRException { 341 if (name.equals("type")) { 342 this.type = null; 343 } else if (name.equals("value[x]")) { 344 this.value = null; 345 } else if (name.equals("status")) { 346 this.status = null; 347 } else 348 super.removeChild(name, value); 349 350 } 351 352 @Override 353 public Base makeProperty(int hash, String name) throws FHIRException { 354 switch (hash) { 355 case 3575610: return getType(); 356 case -1410166417: return getValue(); 357 case 111972721: return getValue(); 358 case -892481550: return getStatus(); 359 default: return super.makeProperty(hash, name); 360 } 361 362 } 363 364 @Override 365 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 366 switch (hash) { 367 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 368 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "date", "boolean", "markdown", "Attachment", "Reference"}; 369 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 370 default: return super.getTypesForProperty(hash, name); 371 } 372 373 } 374 375 @Override 376 public Base addChild(String name) throws FHIRException { 377 if (name.equals("type")) { 378 this.type = new CodeableConcept(); 379 return this.type; 380 } 381 else if (name.equals("valueCodeableConcept")) { 382 this.value = new CodeableConcept(); 383 return this.value; 384 } 385 else if (name.equals("valueQuantity")) { 386 this.value = new Quantity(); 387 return this.value; 388 } 389 else if (name.equals("valueDate")) { 390 this.value = new DateType(); 391 return this.value; 392 } 393 else if (name.equals("valueBoolean")) { 394 this.value = new BooleanType(); 395 return this.value; 396 } 397 else if (name.equals("valueMarkdown")) { 398 this.value = new MarkdownType(); 399 return this.value; 400 } 401 else if (name.equals("valueAttachment")) { 402 this.value = new Attachment(); 403 return this.value; 404 } 405 else if (name.equals("valueReference")) { 406 this.value = new Reference(); 407 return this.value; 408 } 409 else if (name.equals("status")) { 410 this.status = new CodeableConcept(); 411 return this.status; 412 } 413 else 414 return super.addChild(name); 415 } 416 417 public AdministrableProductDefinitionPropertyComponent copy() { 418 AdministrableProductDefinitionPropertyComponent dst = new AdministrableProductDefinitionPropertyComponent(); 419 copyValues(dst); 420 return dst; 421 } 422 423 public void copyValues(AdministrableProductDefinitionPropertyComponent dst) { 424 super.copyValues(dst); 425 dst.type = type == null ? null : type.copy(); 426 dst.value = value == null ? null : value.copy(); 427 dst.status = status == null ? null : status.copy(); 428 } 429 430 @Override 431 public boolean equalsDeep(Base other_) { 432 if (!super.equalsDeep(other_)) 433 return false; 434 if (!(other_ instanceof AdministrableProductDefinitionPropertyComponent)) 435 return false; 436 AdministrableProductDefinitionPropertyComponent o = (AdministrableProductDefinitionPropertyComponent) other_; 437 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(status, o.status, true) 438 ; 439 } 440 441 @Override 442 public boolean equalsShallow(Base other_) { 443 if (!super.equalsShallow(other_)) 444 return false; 445 if (!(other_ instanceof AdministrableProductDefinitionPropertyComponent)) 446 return false; 447 AdministrableProductDefinitionPropertyComponent o = (AdministrableProductDefinitionPropertyComponent) other_; 448 return true; 449 } 450 451 public boolean isEmpty() { 452 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, status); 453 } 454 455 public String fhirType() { 456 return "AdministrableProductDefinition.property"; 457 458 } 459 460 } 461 462 @Block() 463 public static class AdministrableProductDefinitionRouteOfAdministrationComponent extends BackboneElement implements IBaseBackboneElement { 464 /** 465 * Coded expression for the route. 466 */ 467 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 468 @Description(shortDefinition="Coded expression for the route", formalDefinition="Coded expression for the route." ) 469 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 470 protected CodeableConcept code; 471 472 /** 473 * The first dose (dose quantity) administered can be specified for the product, using a numerical value and its unit of measurement. 474 */ 475 @Child(name = "firstDose", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 476 @Description(shortDefinition="The first dose (dose quantity) administered can be specified for the product", formalDefinition="The first dose (dose quantity) administered can be specified for the product, using a numerical value and its unit of measurement." ) 477 protected Quantity firstDose; 478 479 /** 480 * The maximum single dose that can be administered, specified using a numerical value and its unit of measurement. 481 */ 482 @Child(name = "maxSingleDose", type = {Quantity.class}, order=3, min=0, max=1, modifier=false, summary=true) 483 @Description(shortDefinition="The maximum single dose that can be administered", formalDefinition="The maximum single dose that can be administered, specified using a numerical value and its unit of measurement." ) 484 protected Quantity maxSingleDose; 485 486 /** 487 * The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered. 488 */ 489 @Child(name = "maxDosePerDay", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 490 @Description(shortDefinition="The maximum dose quantity to be administered in any one 24-h period", formalDefinition="The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered." ) 491 protected Quantity maxDosePerDay; 492 493 /** 494 * The maximum dose per treatment period that can be administered. 495 */ 496 @Child(name = "maxDosePerTreatmentPeriod", type = {Ratio.class}, order=5, min=0, max=1, modifier=false, summary=true) 497 @Description(shortDefinition="The maximum dose per treatment period that can be administered", formalDefinition="The maximum dose per treatment period that can be administered." ) 498 protected Ratio maxDosePerTreatmentPeriod; 499 500 /** 501 * The maximum treatment period during which the product can be administered. 502 */ 503 @Child(name = "maxTreatmentPeriod", type = {Duration.class}, order=6, min=0, max=1, modifier=false, summary=true) 504 @Description(shortDefinition="The maximum treatment period during which the product can be administered", formalDefinition="The maximum treatment period during which the product can be administered." ) 505 protected Duration maxTreatmentPeriod; 506 507 /** 508 * A species for which this route applies. 509 */ 510 @Child(name = "targetSpecies", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 511 @Description(shortDefinition="A species for which this route applies", formalDefinition="A species for which this route applies." ) 512 protected List<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent> targetSpecies; 513 514 private static final long serialVersionUID = 322274730L; 515 516 /** 517 * Constructor 518 */ 519 public AdministrableProductDefinitionRouteOfAdministrationComponent() { 520 super(); 521 } 522 523 /** 524 * Constructor 525 */ 526 public AdministrableProductDefinitionRouteOfAdministrationComponent(CodeableConcept code) { 527 super(); 528 this.setCode(code); 529 } 530 531 /** 532 * @return {@link #code} (Coded expression for the route.) 533 */ 534 public CodeableConcept getCode() { 535 if (this.code == null) 536 if (Configuration.errorOnAutoCreate()) 537 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationComponent.code"); 538 else if (Configuration.doAutoCreate()) 539 this.code = new CodeableConcept(); // cc 540 return this.code; 541 } 542 543 public boolean hasCode() { 544 return this.code != null && !this.code.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #code} (Coded expression for the route.) 549 */ 550 public AdministrableProductDefinitionRouteOfAdministrationComponent setCode(CodeableConcept value) { 551 this.code = value; 552 return this; 553 } 554 555 /** 556 * @return {@link #firstDose} (The first dose (dose quantity) administered can be specified for the product, using a numerical value and its unit of measurement.) 557 */ 558 public Quantity getFirstDose() { 559 if (this.firstDose == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationComponent.firstDose"); 562 else if (Configuration.doAutoCreate()) 563 this.firstDose = new Quantity(); // cc 564 return this.firstDose; 565 } 566 567 public boolean hasFirstDose() { 568 return this.firstDose != null && !this.firstDose.isEmpty(); 569 } 570 571 /** 572 * @param value {@link #firstDose} (The first dose (dose quantity) administered can be specified for the product, using a numerical value and its unit of measurement.) 573 */ 574 public AdministrableProductDefinitionRouteOfAdministrationComponent setFirstDose(Quantity value) { 575 this.firstDose = value; 576 return this; 577 } 578 579 /** 580 * @return {@link #maxSingleDose} (The maximum single dose that can be administered, specified using a numerical value and its unit of measurement.) 581 */ 582 public Quantity getMaxSingleDose() { 583 if (this.maxSingleDose == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationComponent.maxSingleDose"); 586 else if (Configuration.doAutoCreate()) 587 this.maxSingleDose = new Quantity(); // cc 588 return this.maxSingleDose; 589 } 590 591 public boolean hasMaxSingleDose() { 592 return this.maxSingleDose != null && !this.maxSingleDose.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #maxSingleDose} (The maximum single dose that can be administered, specified using a numerical value and its unit of measurement.) 597 */ 598 public AdministrableProductDefinitionRouteOfAdministrationComponent setMaxSingleDose(Quantity value) { 599 this.maxSingleDose = value; 600 return this; 601 } 602 603 /** 604 * @return {@link #maxDosePerDay} (The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered.) 605 */ 606 public Quantity getMaxDosePerDay() { 607 if (this.maxDosePerDay == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationComponent.maxDosePerDay"); 610 else if (Configuration.doAutoCreate()) 611 this.maxDosePerDay = new Quantity(); // cc 612 return this.maxDosePerDay; 613 } 614 615 public boolean hasMaxDosePerDay() { 616 return this.maxDosePerDay != null && !this.maxDosePerDay.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #maxDosePerDay} (The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered.) 621 */ 622 public AdministrableProductDefinitionRouteOfAdministrationComponent setMaxDosePerDay(Quantity value) { 623 this.maxDosePerDay = value; 624 return this; 625 } 626 627 /** 628 * @return {@link #maxDosePerTreatmentPeriod} (The maximum dose per treatment period that can be administered.) 629 */ 630 public Ratio getMaxDosePerTreatmentPeriod() { 631 if (this.maxDosePerTreatmentPeriod == null) 632 if (Configuration.errorOnAutoCreate()) 633 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationComponent.maxDosePerTreatmentPeriod"); 634 else if (Configuration.doAutoCreate()) 635 this.maxDosePerTreatmentPeriod = new Ratio(); // cc 636 return this.maxDosePerTreatmentPeriod; 637 } 638 639 public boolean hasMaxDosePerTreatmentPeriod() { 640 return this.maxDosePerTreatmentPeriod != null && !this.maxDosePerTreatmentPeriod.isEmpty(); 641 } 642 643 /** 644 * @param value {@link #maxDosePerTreatmentPeriod} (The maximum dose per treatment period that can be administered.) 645 */ 646 public AdministrableProductDefinitionRouteOfAdministrationComponent setMaxDosePerTreatmentPeriod(Ratio value) { 647 this.maxDosePerTreatmentPeriod = value; 648 return this; 649 } 650 651 /** 652 * @return {@link #maxTreatmentPeriod} (The maximum treatment period during which the product can be administered.) 653 */ 654 public Duration getMaxTreatmentPeriod() { 655 if (this.maxTreatmentPeriod == null) 656 if (Configuration.errorOnAutoCreate()) 657 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationComponent.maxTreatmentPeriod"); 658 else if (Configuration.doAutoCreate()) 659 this.maxTreatmentPeriod = new Duration(); // cc 660 return this.maxTreatmentPeriod; 661 } 662 663 public boolean hasMaxTreatmentPeriod() { 664 return this.maxTreatmentPeriod != null && !this.maxTreatmentPeriod.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #maxTreatmentPeriod} (The maximum treatment period during which the product can be administered.) 669 */ 670 public AdministrableProductDefinitionRouteOfAdministrationComponent setMaxTreatmentPeriod(Duration value) { 671 this.maxTreatmentPeriod = value; 672 return this; 673 } 674 675 /** 676 * @return {@link #targetSpecies} (A species for which this route applies.) 677 */ 678 public List<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent> getTargetSpecies() { 679 if (this.targetSpecies == null) 680 this.targetSpecies = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent>(); 681 return this.targetSpecies; 682 } 683 684 /** 685 * @return Returns a reference to <code>this</code> for easy method chaining 686 */ 687 public AdministrableProductDefinitionRouteOfAdministrationComponent setTargetSpecies(List<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent> theTargetSpecies) { 688 this.targetSpecies = theTargetSpecies; 689 return this; 690 } 691 692 public boolean hasTargetSpecies() { 693 if (this.targetSpecies == null) 694 return false; 695 for (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent item : this.targetSpecies) 696 if (!item.isEmpty()) 697 return true; 698 return false; 699 } 700 701 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent addTargetSpecies() { //3 702 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent t = new AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent(); 703 if (this.targetSpecies == null) 704 this.targetSpecies = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent>(); 705 this.targetSpecies.add(t); 706 return t; 707 } 708 709 public AdministrableProductDefinitionRouteOfAdministrationComponent addTargetSpecies(AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent t) { //3 710 if (t == null) 711 return this; 712 if (this.targetSpecies == null) 713 this.targetSpecies = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent>(); 714 this.targetSpecies.add(t); 715 return this; 716 } 717 718 /** 719 * @return The first repetition of repeating field {@link #targetSpecies}, creating it if it does not already exist {3} 720 */ 721 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent getTargetSpeciesFirstRep() { 722 if (getTargetSpecies().isEmpty()) { 723 addTargetSpecies(); 724 } 725 return getTargetSpecies().get(0); 726 } 727 728 protected void listChildren(List<Property> children) { 729 super.listChildren(children); 730 children.add(new Property("code", "CodeableConcept", "Coded expression for the route.", 0, 1, code)); 731 children.add(new Property("firstDose", "Quantity", "The first dose (dose quantity) administered can be specified for the product, using a numerical value and its unit of measurement.", 0, 1, firstDose)); 732 children.add(new Property("maxSingleDose", "Quantity", "The maximum single dose that can be administered, specified using a numerical value and its unit of measurement.", 0, 1, maxSingleDose)); 733 children.add(new Property("maxDosePerDay", "Quantity", "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered.", 0, 1, maxDosePerDay)); 734 children.add(new Property("maxDosePerTreatmentPeriod", "Ratio", "The maximum dose per treatment period that can be administered.", 0, 1, maxDosePerTreatmentPeriod)); 735 children.add(new Property("maxTreatmentPeriod", "Duration", "The maximum treatment period during which the product can be administered.", 0, 1, maxTreatmentPeriod)); 736 children.add(new Property("targetSpecies", "", "A species for which this route applies.", 0, java.lang.Integer.MAX_VALUE, targetSpecies)); 737 } 738 739 @Override 740 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 741 switch (_hash) { 742 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded expression for the route.", 0, 1, code); 743 case 132551405: /*firstDose*/ return new Property("firstDose", "Quantity", "The first dose (dose quantity) administered can be specified for the product, using a numerical value and its unit of measurement.", 0, 1, firstDose); 744 case -259207927: /*maxSingleDose*/ return new Property("maxSingleDose", "Quantity", "The maximum single dose that can be administered, specified using a numerical value and its unit of measurement.", 0, 1, maxSingleDose); 745 case -2017475520: /*maxDosePerDay*/ return new Property("maxDosePerDay", "Quantity", "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered.", 0, 1, maxDosePerDay); 746 case -608040195: /*maxDosePerTreatmentPeriod*/ return new Property("maxDosePerTreatmentPeriod", "Ratio", "The maximum dose per treatment period that can be administered.", 0, 1, maxDosePerTreatmentPeriod); 747 case 920698453: /*maxTreatmentPeriod*/ return new Property("maxTreatmentPeriod", "Duration", "The maximum treatment period during which the product can be administered.", 0, 1, maxTreatmentPeriod); 748 case 295481963: /*targetSpecies*/ return new Property("targetSpecies", "", "A species for which this route applies.", 0, java.lang.Integer.MAX_VALUE, targetSpecies); 749 default: return super.getNamedProperty(_hash, _name, _checkValid); 750 } 751 752 } 753 754 @Override 755 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 756 switch (hash) { 757 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 758 case 132551405: /*firstDose*/ return this.firstDose == null ? new Base[0] : new Base[] {this.firstDose}; // Quantity 759 case -259207927: /*maxSingleDose*/ return this.maxSingleDose == null ? new Base[0] : new Base[] {this.maxSingleDose}; // Quantity 760 case -2017475520: /*maxDosePerDay*/ return this.maxDosePerDay == null ? new Base[0] : new Base[] {this.maxDosePerDay}; // Quantity 761 case -608040195: /*maxDosePerTreatmentPeriod*/ return this.maxDosePerTreatmentPeriod == null ? new Base[0] : new Base[] {this.maxDosePerTreatmentPeriod}; // Ratio 762 case 920698453: /*maxTreatmentPeriod*/ return this.maxTreatmentPeriod == null ? new Base[0] : new Base[] {this.maxTreatmentPeriod}; // Duration 763 case 295481963: /*targetSpecies*/ return this.targetSpecies == null ? new Base[0] : this.targetSpecies.toArray(new Base[this.targetSpecies.size()]); // AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent 764 default: return super.getProperty(hash, name, checkValid); 765 } 766 767 } 768 769 @Override 770 public Base setProperty(int hash, String name, Base value) throws FHIRException { 771 switch (hash) { 772 case 3059181: // code 773 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 774 return value; 775 case 132551405: // firstDose 776 this.firstDose = TypeConvertor.castToQuantity(value); // Quantity 777 return value; 778 case -259207927: // maxSingleDose 779 this.maxSingleDose = TypeConvertor.castToQuantity(value); // Quantity 780 return value; 781 case -2017475520: // maxDosePerDay 782 this.maxDosePerDay = TypeConvertor.castToQuantity(value); // Quantity 783 return value; 784 case -608040195: // maxDosePerTreatmentPeriod 785 this.maxDosePerTreatmentPeriod = TypeConvertor.castToRatio(value); // Ratio 786 return value; 787 case 920698453: // maxTreatmentPeriod 788 this.maxTreatmentPeriod = TypeConvertor.castToDuration(value); // Duration 789 return value; 790 case 295481963: // targetSpecies 791 this.getTargetSpecies().add((AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent) value); // AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent 792 return value; 793 default: return super.setProperty(hash, name, value); 794 } 795 796 } 797 798 @Override 799 public Base setProperty(String name, Base value) throws FHIRException { 800 if (name.equals("code")) { 801 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 802 } else if (name.equals("firstDose")) { 803 this.firstDose = TypeConvertor.castToQuantity(value); // Quantity 804 } else if (name.equals("maxSingleDose")) { 805 this.maxSingleDose = TypeConvertor.castToQuantity(value); // Quantity 806 } else if (name.equals("maxDosePerDay")) { 807 this.maxDosePerDay = TypeConvertor.castToQuantity(value); // Quantity 808 } else if (name.equals("maxDosePerTreatmentPeriod")) { 809 this.maxDosePerTreatmentPeriod = TypeConvertor.castToRatio(value); // Ratio 810 } else if (name.equals("maxTreatmentPeriod")) { 811 this.maxTreatmentPeriod = TypeConvertor.castToDuration(value); // Duration 812 } else if (name.equals("targetSpecies")) { 813 this.getTargetSpecies().add((AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent) value); 814 } else 815 return super.setProperty(name, value); 816 return value; 817 } 818 819 @Override 820 public void removeChild(String name, Base value) throws FHIRException { 821 if (name.equals("code")) { 822 this.code = null; 823 } else if (name.equals("firstDose")) { 824 this.firstDose = null; 825 } else if (name.equals("maxSingleDose")) { 826 this.maxSingleDose = null; 827 } else if (name.equals("maxDosePerDay")) { 828 this.maxDosePerDay = null; 829 } else if (name.equals("maxDosePerTreatmentPeriod")) { 830 this.maxDosePerTreatmentPeriod = null; 831 } else if (name.equals("maxTreatmentPeriod")) { 832 this.maxTreatmentPeriod = null; 833 } else if (name.equals("targetSpecies")) { 834 this.getTargetSpecies().remove((AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent) value); 835 } else 836 super.removeChild(name, value); 837 838 } 839 840 @Override 841 public Base makeProperty(int hash, String name) throws FHIRException { 842 switch (hash) { 843 case 3059181: return getCode(); 844 case 132551405: return getFirstDose(); 845 case -259207927: return getMaxSingleDose(); 846 case -2017475520: return getMaxDosePerDay(); 847 case -608040195: return getMaxDosePerTreatmentPeriod(); 848 case 920698453: return getMaxTreatmentPeriod(); 849 case 295481963: return addTargetSpecies(); 850 default: return super.makeProperty(hash, name); 851 } 852 853 } 854 855 @Override 856 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 857 switch (hash) { 858 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 859 case 132551405: /*firstDose*/ return new String[] {"Quantity"}; 860 case -259207927: /*maxSingleDose*/ return new String[] {"Quantity"}; 861 case -2017475520: /*maxDosePerDay*/ return new String[] {"Quantity"}; 862 case -608040195: /*maxDosePerTreatmentPeriod*/ return new String[] {"Ratio"}; 863 case 920698453: /*maxTreatmentPeriod*/ return new String[] {"Duration"}; 864 case 295481963: /*targetSpecies*/ return new String[] {}; 865 default: return super.getTypesForProperty(hash, name); 866 } 867 868 } 869 870 @Override 871 public Base addChild(String name) throws FHIRException { 872 if (name.equals("code")) { 873 this.code = new CodeableConcept(); 874 return this.code; 875 } 876 else if (name.equals("firstDose")) { 877 this.firstDose = new Quantity(); 878 return this.firstDose; 879 } 880 else if (name.equals("maxSingleDose")) { 881 this.maxSingleDose = new Quantity(); 882 return this.maxSingleDose; 883 } 884 else if (name.equals("maxDosePerDay")) { 885 this.maxDosePerDay = new Quantity(); 886 return this.maxDosePerDay; 887 } 888 else if (name.equals("maxDosePerTreatmentPeriod")) { 889 this.maxDosePerTreatmentPeriod = new Ratio(); 890 return this.maxDosePerTreatmentPeriod; 891 } 892 else if (name.equals("maxTreatmentPeriod")) { 893 this.maxTreatmentPeriod = new Duration(); 894 return this.maxTreatmentPeriod; 895 } 896 else if (name.equals("targetSpecies")) { 897 return addTargetSpecies(); 898 } 899 else 900 return super.addChild(name); 901 } 902 903 public AdministrableProductDefinitionRouteOfAdministrationComponent copy() { 904 AdministrableProductDefinitionRouteOfAdministrationComponent dst = new AdministrableProductDefinitionRouteOfAdministrationComponent(); 905 copyValues(dst); 906 return dst; 907 } 908 909 public void copyValues(AdministrableProductDefinitionRouteOfAdministrationComponent dst) { 910 super.copyValues(dst); 911 dst.code = code == null ? null : code.copy(); 912 dst.firstDose = firstDose == null ? null : firstDose.copy(); 913 dst.maxSingleDose = maxSingleDose == null ? null : maxSingleDose.copy(); 914 dst.maxDosePerDay = maxDosePerDay == null ? null : maxDosePerDay.copy(); 915 dst.maxDosePerTreatmentPeriod = maxDosePerTreatmentPeriod == null ? null : maxDosePerTreatmentPeriod.copy(); 916 dst.maxTreatmentPeriod = maxTreatmentPeriod == null ? null : maxTreatmentPeriod.copy(); 917 if (targetSpecies != null) { 918 dst.targetSpecies = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent>(); 919 for (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent i : targetSpecies) 920 dst.targetSpecies.add(i.copy()); 921 }; 922 } 923 924 @Override 925 public boolean equalsDeep(Base other_) { 926 if (!super.equalsDeep(other_)) 927 return false; 928 if (!(other_ instanceof AdministrableProductDefinitionRouteOfAdministrationComponent)) 929 return false; 930 AdministrableProductDefinitionRouteOfAdministrationComponent o = (AdministrableProductDefinitionRouteOfAdministrationComponent) other_; 931 return compareDeep(code, o.code, true) && compareDeep(firstDose, o.firstDose, true) && compareDeep(maxSingleDose, o.maxSingleDose, true) 932 && compareDeep(maxDosePerDay, o.maxDosePerDay, true) && compareDeep(maxDosePerTreatmentPeriod, o.maxDosePerTreatmentPeriod, true) 933 && compareDeep(maxTreatmentPeriod, o.maxTreatmentPeriod, true) && compareDeep(targetSpecies, o.targetSpecies, true) 934 ; 935 } 936 937 @Override 938 public boolean equalsShallow(Base other_) { 939 if (!super.equalsShallow(other_)) 940 return false; 941 if (!(other_ instanceof AdministrableProductDefinitionRouteOfAdministrationComponent)) 942 return false; 943 AdministrableProductDefinitionRouteOfAdministrationComponent o = (AdministrableProductDefinitionRouteOfAdministrationComponent) other_; 944 return true; 945 } 946 947 public boolean isEmpty() { 948 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, firstDose, maxSingleDose 949 , maxDosePerDay, maxDosePerTreatmentPeriod, maxTreatmentPeriod, targetSpecies); 950 } 951 952 public String fhirType() { 953 return "AdministrableProductDefinition.routeOfAdministration"; 954 955 } 956 957 } 958 959 @Block() 960 public static class AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent extends BackboneElement implements IBaseBackboneElement { 961 /** 962 * Coded expression for the species. 963 */ 964 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 965 @Description(shortDefinition="Coded expression for the species", formalDefinition="Coded expression for the species." ) 966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/target-species") 967 protected CodeableConcept code; 968 969 /** 970 * A species specific time during which consumption of animal product is not appropriate. 971 */ 972 @Child(name = "withdrawalPeriod", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 973 @Description(shortDefinition="A species specific time during which consumption of animal product is not appropriate", formalDefinition="A species specific time during which consumption of animal product is not appropriate." ) 974 protected List<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> withdrawalPeriod; 975 976 private static final long serialVersionUID = -560311351L; 977 978 /** 979 * Constructor 980 */ 981 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent() { 982 super(); 983 } 984 985 /** 986 * Constructor 987 */ 988 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent(CodeableConcept code) { 989 super(); 990 this.setCode(code); 991 } 992 993 /** 994 * @return {@link #code} (Coded expression for the species.) 995 */ 996 public CodeableConcept getCode() { 997 if (this.code == null) 998 if (Configuration.errorOnAutoCreate()) 999 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent.code"); 1000 else if (Configuration.doAutoCreate()) 1001 this.code = new CodeableConcept(); // cc 1002 return this.code; 1003 } 1004 1005 public boolean hasCode() { 1006 return this.code != null && !this.code.isEmpty(); 1007 } 1008 1009 /** 1010 * @param value {@link #code} (Coded expression for the species.) 1011 */ 1012 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent setCode(CodeableConcept value) { 1013 this.code = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #withdrawalPeriod} (A species specific time during which consumption of animal product is not appropriate.) 1019 */ 1020 public List<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> getWithdrawalPeriod() { 1021 if (this.withdrawalPeriod == null) 1022 this.withdrawalPeriod = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 1023 return this.withdrawalPeriod; 1024 } 1025 1026 /** 1027 * @return Returns a reference to <code>this</code> for easy method chaining 1028 */ 1029 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent setWithdrawalPeriod(List<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> theWithdrawalPeriod) { 1030 this.withdrawalPeriod = theWithdrawalPeriod; 1031 return this; 1032 } 1033 1034 public boolean hasWithdrawalPeriod() { 1035 if (this.withdrawalPeriod == null) 1036 return false; 1037 for (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent item : this.withdrawalPeriod) 1038 if (!item.isEmpty()) 1039 return true; 1040 return false; 1041 } 1042 1043 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent addWithdrawalPeriod() { //3 1044 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent t = new AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(); 1045 if (this.withdrawalPeriod == null) 1046 this.withdrawalPeriod = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 1047 this.withdrawalPeriod.add(t); 1048 return t; 1049 } 1050 1051 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent addWithdrawalPeriod(AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent t) { //3 1052 if (t == null) 1053 return this; 1054 if (this.withdrawalPeriod == null) 1055 this.withdrawalPeriod = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 1056 this.withdrawalPeriod.add(t); 1057 return this; 1058 } 1059 1060 /** 1061 * @return The first repetition of repeating field {@link #withdrawalPeriod}, creating it if it does not already exist {3} 1062 */ 1063 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent getWithdrawalPeriodFirstRep() { 1064 if (getWithdrawalPeriod().isEmpty()) { 1065 addWithdrawalPeriod(); 1066 } 1067 return getWithdrawalPeriod().get(0); 1068 } 1069 1070 protected void listChildren(List<Property> children) { 1071 super.listChildren(children); 1072 children.add(new Property("code", "CodeableConcept", "Coded expression for the species.", 0, 1, code)); 1073 children.add(new Property("withdrawalPeriod", "", "A species specific time during which consumption of animal product is not appropriate.", 0, java.lang.Integer.MAX_VALUE, withdrawalPeriod)); 1074 } 1075 1076 @Override 1077 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1078 switch (_hash) { 1079 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded expression for the species.", 0, 1, code); 1080 case -98450730: /*withdrawalPeriod*/ return new Property("withdrawalPeriod", "", "A species specific time during which consumption of animal product is not appropriate.", 0, java.lang.Integer.MAX_VALUE, withdrawalPeriod); 1081 default: return super.getNamedProperty(_hash, _name, _checkValid); 1082 } 1083 1084 } 1085 1086 @Override 1087 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1088 switch (hash) { 1089 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1090 case -98450730: /*withdrawalPeriod*/ return this.withdrawalPeriod == null ? new Base[0] : this.withdrawalPeriod.toArray(new Base[this.withdrawalPeriod.size()]); // AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1091 default: return super.getProperty(hash, name, checkValid); 1092 } 1093 1094 } 1095 1096 @Override 1097 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1098 switch (hash) { 1099 case 3059181: // code 1100 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1101 return value; 1102 case -98450730: // withdrawalPeriod 1103 this.getWithdrawalPeriod().add((AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); // AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1104 return value; 1105 default: return super.setProperty(hash, name, value); 1106 } 1107 1108 } 1109 1110 @Override 1111 public Base setProperty(String name, Base value) throws FHIRException { 1112 if (name.equals("code")) { 1113 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1114 } else if (name.equals("withdrawalPeriod")) { 1115 this.getWithdrawalPeriod().add((AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); 1116 } else 1117 return super.setProperty(name, value); 1118 return value; 1119 } 1120 1121 @Override 1122 public void removeChild(String name, Base value) throws FHIRException { 1123 if (name.equals("code")) { 1124 this.code = null; 1125 } else if (name.equals("withdrawalPeriod")) { 1126 this.getWithdrawalPeriod().remove((AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); 1127 } else 1128 super.removeChild(name, value); 1129 1130 } 1131 1132 @Override 1133 public Base makeProperty(int hash, String name) throws FHIRException { 1134 switch (hash) { 1135 case 3059181: return getCode(); 1136 case -98450730: return addWithdrawalPeriod(); 1137 default: return super.makeProperty(hash, name); 1138 } 1139 1140 } 1141 1142 @Override 1143 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1144 switch (hash) { 1145 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1146 case -98450730: /*withdrawalPeriod*/ return new String[] {}; 1147 default: return super.getTypesForProperty(hash, name); 1148 } 1149 1150 } 1151 1152 @Override 1153 public Base addChild(String name) throws FHIRException { 1154 if (name.equals("code")) { 1155 this.code = new CodeableConcept(); 1156 return this.code; 1157 } 1158 else if (name.equals("withdrawalPeriod")) { 1159 return addWithdrawalPeriod(); 1160 } 1161 else 1162 return super.addChild(name); 1163 } 1164 1165 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent copy() { 1166 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent dst = new AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent(); 1167 copyValues(dst); 1168 return dst; 1169 } 1170 1171 public void copyValues(AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent dst) { 1172 super.copyValues(dst); 1173 dst.code = code == null ? null : code.copy(); 1174 if (withdrawalPeriod != null) { 1175 dst.withdrawalPeriod = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 1176 for (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent i : withdrawalPeriod) 1177 dst.withdrawalPeriod.add(i.copy()); 1178 }; 1179 } 1180 1181 @Override 1182 public boolean equalsDeep(Base other_) { 1183 if (!super.equalsDeep(other_)) 1184 return false; 1185 if (!(other_ instanceof AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent)) 1186 return false; 1187 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent o = (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent) other_; 1188 return compareDeep(code, o.code, true) && compareDeep(withdrawalPeriod, o.withdrawalPeriod, true) 1189 ; 1190 } 1191 1192 @Override 1193 public boolean equalsShallow(Base other_) { 1194 if (!super.equalsShallow(other_)) 1195 return false; 1196 if (!(other_ instanceof AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent)) 1197 return false; 1198 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent o = (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesComponent) other_; 1199 return true; 1200 } 1201 1202 public boolean isEmpty() { 1203 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, withdrawalPeriod); 1204 } 1205 1206 public String fhirType() { 1207 return "AdministrableProductDefinition.routeOfAdministration.targetSpecies"; 1208 1209 } 1210 1211 } 1212 1213 @Block() 1214 public static class AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent extends BackboneElement implements IBaseBackboneElement { 1215 /** 1216 * Coded expression for the type of tissue for which the withdrawal period applies, e.g. meat, milk. 1217 */ 1218 @Child(name = "tissue", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1219 @Description(shortDefinition="The type of tissue for which the withdrawal period applies, e.g. meat, milk", formalDefinition="Coded expression for the type of tissue for which the withdrawal period applies, e.g. meat, milk." ) 1220 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/animal-tissue-type") 1221 protected CodeableConcept tissue; 1222 1223 /** 1224 * A value for the time. 1225 */ 1226 @Child(name = "value", type = {Quantity.class}, order=2, min=1, max=1, modifier=false, summary=true) 1227 @Description(shortDefinition="A value for the time", formalDefinition="A value for the time." ) 1228 protected Quantity value; 1229 1230 /** 1231 * Extra information about the withdrawal period. 1232 */ 1233 @Child(name = "supportingInformation", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1234 @Description(shortDefinition="Extra information about the withdrawal period", formalDefinition="Extra information about the withdrawal period." ) 1235 protected StringType supportingInformation; 1236 1237 private static final long serialVersionUID = -1113691238L; 1238 1239 /** 1240 * Constructor 1241 */ 1242 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent() { 1243 super(); 1244 } 1245 1246 /** 1247 * Constructor 1248 */ 1249 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(CodeableConcept tissue, Quantity value) { 1250 super(); 1251 this.setTissue(tissue); 1252 this.setValue(value); 1253 } 1254 1255 /** 1256 * @return {@link #tissue} (Coded expression for the type of tissue for which the withdrawal period applies, e.g. meat, milk.) 1257 */ 1258 public CodeableConcept getTissue() { 1259 if (this.tissue == null) 1260 if (Configuration.errorOnAutoCreate()) 1261 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.tissue"); 1262 else if (Configuration.doAutoCreate()) 1263 this.tissue = new CodeableConcept(); // cc 1264 return this.tissue; 1265 } 1266 1267 public boolean hasTissue() { 1268 return this.tissue != null && !this.tissue.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #tissue} (Coded expression for the type of tissue for which the withdrawal period applies, e.g. meat, milk.) 1273 */ 1274 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setTissue(CodeableConcept value) { 1275 this.tissue = value; 1276 return this; 1277 } 1278 1279 /** 1280 * @return {@link #value} (A value for the time.) 1281 */ 1282 public Quantity getValue() { 1283 if (this.value == null) 1284 if (Configuration.errorOnAutoCreate()) 1285 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.value"); 1286 else if (Configuration.doAutoCreate()) 1287 this.value = new Quantity(); // cc 1288 return this.value; 1289 } 1290 1291 public boolean hasValue() { 1292 return this.value != null && !this.value.isEmpty(); 1293 } 1294 1295 /** 1296 * @param value {@link #value} (A value for the time.) 1297 */ 1298 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setValue(Quantity value) { 1299 this.value = value; 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #supportingInformation} (Extra information about the withdrawal period.). This is the underlying object with id, value and extensions. The accessor "getSupportingInformation" gives direct access to the value 1305 */ 1306 public StringType getSupportingInformationElement() { 1307 if (this.supportingInformation == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.supportingInformation"); 1310 else if (Configuration.doAutoCreate()) 1311 this.supportingInformation = new StringType(); // bb 1312 return this.supportingInformation; 1313 } 1314 1315 public boolean hasSupportingInformationElement() { 1316 return this.supportingInformation != null && !this.supportingInformation.isEmpty(); 1317 } 1318 1319 public boolean hasSupportingInformation() { 1320 return this.supportingInformation != null && !this.supportingInformation.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #supportingInformation} (Extra information about the withdrawal period.). This is the underlying object with id, value and extensions. The accessor "getSupportingInformation" gives direct access to the value 1325 */ 1326 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setSupportingInformationElement(StringType value) { 1327 this.supportingInformation = value; 1328 return this; 1329 } 1330 1331 /** 1332 * @return Extra information about the withdrawal period. 1333 */ 1334 public String getSupportingInformation() { 1335 return this.supportingInformation == null ? null : this.supportingInformation.getValue(); 1336 } 1337 1338 /** 1339 * @param value Extra information about the withdrawal period. 1340 */ 1341 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setSupportingInformation(String value) { 1342 if (Utilities.noString(value)) 1343 this.supportingInformation = null; 1344 else { 1345 if (this.supportingInformation == null) 1346 this.supportingInformation = new StringType(); 1347 this.supportingInformation.setValue(value); 1348 } 1349 return this; 1350 } 1351 1352 protected void listChildren(List<Property> children) { 1353 super.listChildren(children); 1354 children.add(new Property("tissue", "CodeableConcept", "Coded expression for the type of tissue for which the withdrawal period applies, e.g. meat, milk.", 0, 1, tissue)); 1355 children.add(new Property("value", "Quantity", "A value for the time.", 0, 1, value)); 1356 children.add(new Property("supportingInformation", "string", "Extra information about the withdrawal period.", 0, 1, supportingInformation)); 1357 } 1358 1359 @Override 1360 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1361 switch (_hash) { 1362 case -873475867: /*tissue*/ return new Property("tissue", "CodeableConcept", "Coded expression for the type of tissue for which the withdrawal period applies, e.g. meat, milk.", 0, 1, tissue); 1363 case 111972721: /*value*/ return new Property("value", "Quantity", "A value for the time.", 0, 1, value); 1364 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "string", "Extra information about the withdrawal period.", 0, 1, supportingInformation); 1365 default: return super.getNamedProperty(_hash, _name, _checkValid); 1366 } 1367 1368 } 1369 1370 @Override 1371 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1372 switch (hash) { 1373 case -873475867: /*tissue*/ return this.tissue == null ? new Base[0] : new Base[] {this.tissue}; // CodeableConcept 1374 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Quantity 1375 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : new Base[] {this.supportingInformation}; // StringType 1376 default: return super.getProperty(hash, name, checkValid); 1377 } 1378 1379 } 1380 1381 @Override 1382 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1383 switch (hash) { 1384 case -873475867: // tissue 1385 this.tissue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1386 return value; 1387 case 111972721: // value 1388 this.value = TypeConvertor.castToQuantity(value); // Quantity 1389 return value; 1390 case -1248768647: // supportingInformation 1391 this.supportingInformation = TypeConvertor.castToString(value); // StringType 1392 return value; 1393 default: return super.setProperty(hash, name, value); 1394 } 1395 1396 } 1397 1398 @Override 1399 public Base setProperty(String name, Base value) throws FHIRException { 1400 if (name.equals("tissue")) { 1401 this.tissue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1402 } else if (name.equals("value")) { 1403 this.value = TypeConvertor.castToQuantity(value); // Quantity 1404 } else if (name.equals("supportingInformation")) { 1405 this.supportingInformation = TypeConvertor.castToString(value); // StringType 1406 } else 1407 return super.setProperty(name, value); 1408 return value; 1409 } 1410 1411 @Override 1412 public void removeChild(String name, Base value) throws FHIRException { 1413 if (name.equals("tissue")) { 1414 this.tissue = null; 1415 } else if (name.equals("value")) { 1416 this.value = null; 1417 } else if (name.equals("supportingInformation")) { 1418 this.supportingInformation = null; 1419 } else 1420 super.removeChild(name, value); 1421 1422 } 1423 1424 @Override 1425 public Base makeProperty(int hash, String name) throws FHIRException { 1426 switch (hash) { 1427 case -873475867: return getTissue(); 1428 case 111972721: return getValue(); 1429 case -1248768647: return getSupportingInformationElement(); 1430 default: return super.makeProperty(hash, name); 1431 } 1432 1433 } 1434 1435 @Override 1436 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1437 switch (hash) { 1438 case -873475867: /*tissue*/ return new String[] {"CodeableConcept"}; 1439 case 111972721: /*value*/ return new String[] {"Quantity"}; 1440 case -1248768647: /*supportingInformation*/ return new String[] {"string"}; 1441 default: return super.getTypesForProperty(hash, name); 1442 } 1443 1444 } 1445 1446 @Override 1447 public Base addChild(String name) throws FHIRException { 1448 if (name.equals("tissue")) { 1449 this.tissue = new CodeableConcept(); 1450 return this.tissue; 1451 } 1452 else if (name.equals("value")) { 1453 this.value = new Quantity(); 1454 return this.value; 1455 } 1456 else if (name.equals("supportingInformation")) { 1457 throw new FHIRException("Cannot call addChild on a singleton property AdministrableProductDefinition.routeOfAdministration.targetSpecies.withdrawalPeriod.supportingInformation"); 1458 } 1459 else 1460 return super.addChild(name); 1461 } 1462 1463 public AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent copy() { 1464 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent dst = new AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(); 1465 copyValues(dst); 1466 return dst; 1467 } 1468 1469 public void copyValues(AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent dst) { 1470 super.copyValues(dst); 1471 dst.tissue = tissue == null ? null : tissue.copy(); 1472 dst.value = value == null ? null : value.copy(); 1473 dst.supportingInformation = supportingInformation == null ? null : supportingInformation.copy(); 1474 } 1475 1476 @Override 1477 public boolean equalsDeep(Base other_) { 1478 if (!super.equalsDeep(other_)) 1479 return false; 1480 if (!(other_ instanceof AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent)) 1481 return false; 1482 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent o = (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) other_; 1483 return compareDeep(tissue, o.tissue, true) && compareDeep(value, o.value, true) && compareDeep(supportingInformation, o.supportingInformation, true) 1484 ; 1485 } 1486 1487 @Override 1488 public boolean equalsShallow(Base other_) { 1489 if (!super.equalsShallow(other_)) 1490 return false; 1491 if (!(other_ instanceof AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent)) 1492 return false; 1493 AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent o = (AdministrableProductDefinitionRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) other_; 1494 return compareValues(supportingInformation, o.supportingInformation, true); 1495 } 1496 1497 public boolean isEmpty() { 1498 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(tissue, value, supportingInformation 1499 ); 1500 } 1501 1502 public String fhirType() { 1503 return "AdministrableProductDefinition.routeOfAdministration.targetSpecies.withdrawalPeriod"; 1504 1505 } 1506 1507 } 1508 1509 /** 1510 * An identifier for the administrable product. 1511 */ 1512 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1513 @Description(shortDefinition="An identifier for the administrable product", formalDefinition="An identifier for the administrable product." ) 1514 protected List<Identifier> identifier; 1515 1516 /** 1517 * The status of this administrable product. Enables tracking the life-cycle of the content. 1518 */ 1519 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1520 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this administrable product. Enables tracking the life-cycle of the content." ) 1521 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1522 protected Enumeration<PublicationStatus> status; 1523 1524 /** 1525 * References a product from which one or more of the constituent parts of that product can be prepared and used as described by this administrable product. If this administrable product describes the administration of a crushed tablet, the 'formOf' would be the product representing a distribution containing tablets and possibly also a cream. This is distinct from the 'producedFrom' which refers to the specific components of the product that are used in this preparation, rather than the product as a whole. 1526 */ 1527 @Child(name = "formOf", type = {MedicinalProductDefinition.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1528 @Description(shortDefinition="References a product from which one or more of the constituent parts of that product can be prepared and used as described by this administrable product", formalDefinition="References a product from which one or more of the constituent parts of that product can be prepared and used as described by this administrable product. If this administrable product describes the administration of a crushed tablet, the 'formOf' would be the product representing a distribution containing tablets and possibly also a cream. This is distinct from the 'producedFrom' which refers to the specific components of the product that are used in this preparation, rather than the product as a whole." ) 1529 protected List<Reference> formOf; 1530 1531 /** 1532 * The dose form of the final product after necessary reconstitution or processing. Contrasts to the manufactured dose form (see ManufacturedItemDefinition). If the manufactured form was 'powder for solution for injection', the administrable dose form could be 'solution for injection' (once mixed with another item having manufactured form 'solvent for solution for injection'). 1533 */ 1534 @Child(name = "administrableDoseForm", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1535 @Description(shortDefinition="The dose form of the final product after necessary reconstitution or processing", formalDefinition="The dose form of the final product after necessary reconstitution or processing. Contrasts to the manufactured dose form (see ManufacturedItemDefinition). If the manufactured form was 'powder for solution for injection', the administrable dose form could be 'solution for injection' (once mixed with another item having manufactured form 'solvent for solution for injection')." ) 1536 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrable-dose-form") 1537 protected CodeableConcept administrableDoseForm; 1538 1539 /** 1540 * The presentation type in which this item is given to a patient. e.g. for a spray - 'puff' (as in 'contains 100 mcg per puff'), or for a liquid - 'vial' (as in 'contains 5 ml per vial'). 1541 */ 1542 @Child(name = "unitOfPresentation", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1543 @Description(shortDefinition="The presentation type in which this item is given to a patient. e.g. for a spray - 'puff'", formalDefinition="The presentation type in which this item is given to a patient. e.g. for a spray - 'puff' (as in 'contains 100 mcg per puff'), or for a liquid - 'vial' (as in 'contains 5 ml per vial')." ) 1544 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/unit-of-presentation") 1545 protected CodeableConcept unitOfPresentation; 1546 1547 /** 1548 * Indicates the specific manufactured items that are part of the 'formOf' product that are used in the preparation of this specific administrable form. In some cases, an administrable form might use all of the items from the overall product (or there might only be one item), while in other cases, an administrable form might use only a subset of the items available in the overall product. For example, an administrable form might involve combining a liquid and a powder available as part of an overall product, but not involve applying the also supplied cream. 1549 */ 1550 @Child(name = "producedFrom", type = {ManufacturedItemDefinition.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1551 @Description(shortDefinition="Indicates the specific manufactured items that are part of the 'formOf' product that are used in the preparation of this specific administrable form", formalDefinition="Indicates the specific manufactured items that are part of the 'formOf' product that are used in the preparation of this specific administrable form. In some cases, an administrable form might use all of the items from the overall product (or there might only be one item), while in other cases, an administrable form might use only a subset of the items available in the overall product. For example, an administrable form might involve combining a liquid and a powder available as part of an overall product, but not involve applying the also supplied cream." ) 1552 protected List<Reference> producedFrom; 1553 1554 /** 1555 * The ingredients of this administrable medicinal product. This is only needed if the ingredients are not specified either using ManufacturedItemDefiniton (via AdministrableProductDefinition.producedFrom) to state which component items are used to make this, or using by incoming references from the Ingredient resource, to state in detail which substances exist within this. This element allows a basic coded ingredient to be used. 1556 */ 1557 @Child(name = "ingredient", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1558 @Description(shortDefinition="The ingredients of this administrable medicinal product. This is only needed if the ingredients are not specified either using ManufacturedItemDefiniton, or using by incoming references from the Ingredient resource", formalDefinition="The ingredients of this administrable medicinal product. This is only needed if the ingredients are not specified either using ManufacturedItemDefiniton (via AdministrableProductDefinition.producedFrom) to state which component items are used to make this, or using by incoming references from the Ingredient resource, to state in detail which substances exist within this. This element allows a basic coded ingredient to be used." ) 1559 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-codes") 1560 protected List<CodeableConcept> ingredient; 1561 1562 /** 1563 * A device that is integral to the medicinal product, in effect being considered as an "ingredient" of the medicinal product. This is not intended for devices that are just co-packaged. 1564 */ 1565 @Child(name = "device", type = {DeviceDefinition.class}, order=7, min=0, max=1, modifier=false, summary=true) 1566 @Description(shortDefinition="A device that is integral to the medicinal product, in effect being considered as an \"ingredient\" of the medicinal product", formalDefinition="A device that is integral to the medicinal product, in effect being considered as an \"ingredient\" of the medicinal product. This is not intended for devices that are just co-packaged." ) 1567 protected Reference device; 1568 1569 /** 1570 * A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere. 1571 */ 1572 @Child(name = "description", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1573 @Description(shortDefinition="A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed", formalDefinition="A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere." ) 1574 protected MarkdownType description; 1575 1576 /** 1577 * Characteristics e.g. a product's onset of action. 1578 */ 1579 @Child(name = "property", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1580 @Description(shortDefinition="Characteristics e.g. a product's onset of action", formalDefinition="Characteristics e.g. a product's onset of action." ) 1581 protected List<AdministrableProductDefinitionPropertyComponent> property; 1582 1583 /** 1584 * The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. RouteOfAdministration cannot be used when the 'formOf' product already uses MedicinalProductDefinition.route (and vice versa). 1585 */ 1586 @Child(name = "routeOfAdministration", type = {}, order=10, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1587 @Description(shortDefinition="The path by which the product is taken into or makes contact with the body", formalDefinition="The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. RouteOfAdministration cannot be used when the 'formOf' product already uses MedicinalProductDefinition.route (and vice versa)." ) 1588 protected List<AdministrableProductDefinitionRouteOfAdministrationComponent> routeOfAdministration; 1589 1590 private static final long serialVersionUID = -487805677L; 1591 1592 /** 1593 * Constructor 1594 */ 1595 public AdministrableProductDefinition() { 1596 super(); 1597 } 1598 1599 /** 1600 * Constructor 1601 */ 1602 public AdministrableProductDefinition(PublicationStatus status, AdministrableProductDefinitionRouteOfAdministrationComponent routeOfAdministration) { 1603 super(); 1604 this.setStatus(status); 1605 this.addRouteOfAdministration(routeOfAdministration); 1606 } 1607 1608 /** 1609 * @return {@link #identifier} (An identifier for the administrable product.) 1610 */ 1611 public List<Identifier> getIdentifier() { 1612 if (this.identifier == null) 1613 this.identifier = new ArrayList<Identifier>(); 1614 return this.identifier; 1615 } 1616 1617 /** 1618 * @return Returns a reference to <code>this</code> for easy method chaining 1619 */ 1620 public AdministrableProductDefinition setIdentifier(List<Identifier> theIdentifier) { 1621 this.identifier = theIdentifier; 1622 return this; 1623 } 1624 1625 public boolean hasIdentifier() { 1626 if (this.identifier == null) 1627 return false; 1628 for (Identifier item : this.identifier) 1629 if (!item.isEmpty()) 1630 return true; 1631 return false; 1632 } 1633 1634 public Identifier addIdentifier() { //3 1635 Identifier t = new Identifier(); 1636 if (this.identifier == null) 1637 this.identifier = new ArrayList<Identifier>(); 1638 this.identifier.add(t); 1639 return t; 1640 } 1641 1642 public AdministrableProductDefinition addIdentifier(Identifier t) { //3 1643 if (t == null) 1644 return this; 1645 if (this.identifier == null) 1646 this.identifier = new ArrayList<Identifier>(); 1647 this.identifier.add(t); 1648 return this; 1649 } 1650 1651 /** 1652 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1653 */ 1654 public Identifier getIdentifierFirstRep() { 1655 if (getIdentifier().isEmpty()) { 1656 addIdentifier(); 1657 } 1658 return getIdentifier().get(0); 1659 } 1660 1661 /** 1662 * @return {@link #status} (The status of this administrable product. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1663 */ 1664 public Enumeration<PublicationStatus> getStatusElement() { 1665 if (this.status == null) 1666 if (Configuration.errorOnAutoCreate()) 1667 throw new Error("Attempt to auto-create AdministrableProductDefinition.status"); 1668 else if (Configuration.doAutoCreate()) 1669 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1670 return this.status; 1671 } 1672 1673 public boolean hasStatusElement() { 1674 return this.status != null && !this.status.isEmpty(); 1675 } 1676 1677 public boolean hasStatus() { 1678 return this.status != null && !this.status.isEmpty(); 1679 } 1680 1681 /** 1682 * @param value {@link #status} (The status of this administrable product. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1683 */ 1684 public AdministrableProductDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1685 this.status = value; 1686 return this; 1687 } 1688 1689 /** 1690 * @return The status of this administrable product. Enables tracking the life-cycle of the content. 1691 */ 1692 public PublicationStatus getStatus() { 1693 return this.status == null ? null : this.status.getValue(); 1694 } 1695 1696 /** 1697 * @param value The status of this administrable product. Enables tracking the life-cycle of the content. 1698 */ 1699 public AdministrableProductDefinition setStatus(PublicationStatus value) { 1700 if (this.status == null) 1701 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1702 this.status.setValue(value); 1703 return this; 1704 } 1705 1706 /** 1707 * @return {@link #formOf} (References a product from which one or more of the constituent parts of that product can be prepared and used as described by this administrable product. If this administrable product describes the administration of a crushed tablet, the 'formOf' would be the product representing a distribution containing tablets and possibly also a cream. This is distinct from the 'producedFrom' which refers to the specific components of the product that are used in this preparation, rather than the product as a whole.) 1708 */ 1709 public List<Reference> getFormOf() { 1710 if (this.formOf == null) 1711 this.formOf = new ArrayList<Reference>(); 1712 return this.formOf; 1713 } 1714 1715 /** 1716 * @return Returns a reference to <code>this</code> for easy method chaining 1717 */ 1718 public AdministrableProductDefinition setFormOf(List<Reference> theFormOf) { 1719 this.formOf = theFormOf; 1720 return this; 1721 } 1722 1723 public boolean hasFormOf() { 1724 if (this.formOf == null) 1725 return false; 1726 for (Reference item : this.formOf) 1727 if (!item.isEmpty()) 1728 return true; 1729 return false; 1730 } 1731 1732 public Reference addFormOf() { //3 1733 Reference t = new Reference(); 1734 if (this.formOf == null) 1735 this.formOf = new ArrayList<Reference>(); 1736 this.formOf.add(t); 1737 return t; 1738 } 1739 1740 public AdministrableProductDefinition addFormOf(Reference t) { //3 1741 if (t == null) 1742 return this; 1743 if (this.formOf == null) 1744 this.formOf = new ArrayList<Reference>(); 1745 this.formOf.add(t); 1746 return this; 1747 } 1748 1749 /** 1750 * @return The first repetition of repeating field {@link #formOf}, creating it if it does not already exist {3} 1751 */ 1752 public Reference getFormOfFirstRep() { 1753 if (getFormOf().isEmpty()) { 1754 addFormOf(); 1755 } 1756 return getFormOf().get(0); 1757 } 1758 1759 /** 1760 * @return {@link #administrableDoseForm} (The dose form of the final product after necessary reconstitution or processing. Contrasts to the manufactured dose form (see ManufacturedItemDefinition). If the manufactured form was 'powder for solution for injection', the administrable dose form could be 'solution for injection' (once mixed with another item having manufactured form 'solvent for solution for injection').) 1761 */ 1762 public CodeableConcept getAdministrableDoseForm() { 1763 if (this.administrableDoseForm == null) 1764 if (Configuration.errorOnAutoCreate()) 1765 throw new Error("Attempt to auto-create AdministrableProductDefinition.administrableDoseForm"); 1766 else if (Configuration.doAutoCreate()) 1767 this.administrableDoseForm = new CodeableConcept(); // cc 1768 return this.administrableDoseForm; 1769 } 1770 1771 public boolean hasAdministrableDoseForm() { 1772 return this.administrableDoseForm != null && !this.administrableDoseForm.isEmpty(); 1773 } 1774 1775 /** 1776 * @param value {@link #administrableDoseForm} (The dose form of the final product after necessary reconstitution or processing. Contrasts to the manufactured dose form (see ManufacturedItemDefinition). If the manufactured form was 'powder for solution for injection', the administrable dose form could be 'solution for injection' (once mixed with another item having manufactured form 'solvent for solution for injection').) 1777 */ 1778 public AdministrableProductDefinition setAdministrableDoseForm(CodeableConcept value) { 1779 this.administrableDoseForm = value; 1780 return this; 1781 } 1782 1783 /** 1784 * @return {@link #unitOfPresentation} (The presentation type in which this item is given to a patient. e.g. for a spray - 'puff' (as in 'contains 100 mcg per puff'), or for a liquid - 'vial' (as in 'contains 5 ml per vial').) 1785 */ 1786 public CodeableConcept getUnitOfPresentation() { 1787 if (this.unitOfPresentation == null) 1788 if (Configuration.errorOnAutoCreate()) 1789 throw new Error("Attempt to auto-create AdministrableProductDefinition.unitOfPresentation"); 1790 else if (Configuration.doAutoCreate()) 1791 this.unitOfPresentation = new CodeableConcept(); // cc 1792 return this.unitOfPresentation; 1793 } 1794 1795 public boolean hasUnitOfPresentation() { 1796 return this.unitOfPresentation != null && !this.unitOfPresentation.isEmpty(); 1797 } 1798 1799 /** 1800 * @param value {@link #unitOfPresentation} (The presentation type in which this item is given to a patient. e.g. for a spray - 'puff' (as in 'contains 100 mcg per puff'), or for a liquid - 'vial' (as in 'contains 5 ml per vial').) 1801 */ 1802 public AdministrableProductDefinition setUnitOfPresentation(CodeableConcept value) { 1803 this.unitOfPresentation = value; 1804 return this; 1805 } 1806 1807 /** 1808 * @return {@link #producedFrom} (Indicates the specific manufactured items that are part of the 'formOf' product that are used in the preparation of this specific administrable form. In some cases, an administrable form might use all of the items from the overall product (or there might only be one item), while in other cases, an administrable form might use only a subset of the items available in the overall product. For example, an administrable form might involve combining a liquid and a powder available as part of an overall product, but not involve applying the also supplied cream.) 1809 */ 1810 public List<Reference> getProducedFrom() { 1811 if (this.producedFrom == null) 1812 this.producedFrom = new ArrayList<Reference>(); 1813 return this.producedFrom; 1814 } 1815 1816 /** 1817 * @return Returns a reference to <code>this</code> for easy method chaining 1818 */ 1819 public AdministrableProductDefinition setProducedFrom(List<Reference> theProducedFrom) { 1820 this.producedFrom = theProducedFrom; 1821 return this; 1822 } 1823 1824 public boolean hasProducedFrom() { 1825 if (this.producedFrom == null) 1826 return false; 1827 for (Reference item : this.producedFrom) 1828 if (!item.isEmpty()) 1829 return true; 1830 return false; 1831 } 1832 1833 public Reference addProducedFrom() { //3 1834 Reference t = new Reference(); 1835 if (this.producedFrom == null) 1836 this.producedFrom = new ArrayList<Reference>(); 1837 this.producedFrom.add(t); 1838 return t; 1839 } 1840 1841 public AdministrableProductDefinition addProducedFrom(Reference t) { //3 1842 if (t == null) 1843 return this; 1844 if (this.producedFrom == null) 1845 this.producedFrom = new ArrayList<Reference>(); 1846 this.producedFrom.add(t); 1847 return this; 1848 } 1849 1850 /** 1851 * @return The first repetition of repeating field {@link #producedFrom}, creating it if it does not already exist {3} 1852 */ 1853 public Reference getProducedFromFirstRep() { 1854 if (getProducedFrom().isEmpty()) { 1855 addProducedFrom(); 1856 } 1857 return getProducedFrom().get(0); 1858 } 1859 1860 /** 1861 * @return {@link #ingredient} (The ingredients of this administrable medicinal product. This is only needed if the ingredients are not specified either using ManufacturedItemDefiniton (via AdministrableProductDefinition.producedFrom) to state which component items are used to make this, or using by incoming references from the Ingredient resource, to state in detail which substances exist within this. This element allows a basic coded ingredient to be used.) 1862 */ 1863 public List<CodeableConcept> getIngredient() { 1864 if (this.ingredient == null) 1865 this.ingredient = new ArrayList<CodeableConcept>(); 1866 return this.ingredient; 1867 } 1868 1869 /** 1870 * @return Returns a reference to <code>this</code> for easy method chaining 1871 */ 1872 public AdministrableProductDefinition setIngredient(List<CodeableConcept> theIngredient) { 1873 this.ingredient = theIngredient; 1874 return this; 1875 } 1876 1877 public boolean hasIngredient() { 1878 if (this.ingredient == null) 1879 return false; 1880 for (CodeableConcept item : this.ingredient) 1881 if (!item.isEmpty()) 1882 return true; 1883 return false; 1884 } 1885 1886 public CodeableConcept addIngredient() { //3 1887 CodeableConcept t = new CodeableConcept(); 1888 if (this.ingredient == null) 1889 this.ingredient = new ArrayList<CodeableConcept>(); 1890 this.ingredient.add(t); 1891 return t; 1892 } 1893 1894 public AdministrableProductDefinition addIngredient(CodeableConcept t) { //3 1895 if (t == null) 1896 return this; 1897 if (this.ingredient == null) 1898 this.ingredient = new ArrayList<CodeableConcept>(); 1899 this.ingredient.add(t); 1900 return this; 1901 } 1902 1903 /** 1904 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 1905 */ 1906 public CodeableConcept getIngredientFirstRep() { 1907 if (getIngredient().isEmpty()) { 1908 addIngredient(); 1909 } 1910 return getIngredient().get(0); 1911 } 1912 1913 /** 1914 * @return {@link #device} (A device that is integral to the medicinal product, in effect being considered as an "ingredient" of the medicinal product. This is not intended for devices that are just co-packaged.) 1915 */ 1916 public Reference getDevice() { 1917 if (this.device == null) 1918 if (Configuration.errorOnAutoCreate()) 1919 throw new Error("Attempt to auto-create AdministrableProductDefinition.device"); 1920 else if (Configuration.doAutoCreate()) 1921 this.device = new Reference(); // cc 1922 return this.device; 1923 } 1924 1925 public boolean hasDevice() { 1926 return this.device != null && !this.device.isEmpty(); 1927 } 1928 1929 /** 1930 * @param value {@link #device} (A device that is integral to the medicinal product, in effect being considered as an "ingredient" of the medicinal product. This is not intended for devices that are just co-packaged.) 1931 */ 1932 public AdministrableProductDefinition setDevice(Reference value) { 1933 this.device = value; 1934 return this; 1935 } 1936 1937 /** 1938 * @return {@link #description} (A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1939 */ 1940 public MarkdownType getDescriptionElement() { 1941 if (this.description == null) 1942 if (Configuration.errorOnAutoCreate()) 1943 throw new Error("Attempt to auto-create AdministrableProductDefinition.description"); 1944 else if (Configuration.doAutoCreate()) 1945 this.description = new MarkdownType(); // bb 1946 return this.description; 1947 } 1948 1949 public boolean hasDescriptionElement() { 1950 return this.description != null && !this.description.isEmpty(); 1951 } 1952 1953 public boolean hasDescription() { 1954 return this.description != null && !this.description.isEmpty(); 1955 } 1956 1957 /** 1958 * @param value {@link #description} (A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1959 */ 1960 public AdministrableProductDefinition setDescriptionElement(MarkdownType value) { 1961 this.description = value; 1962 return this; 1963 } 1964 1965 /** 1966 * @return A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere. 1967 */ 1968 public String getDescription() { 1969 return this.description == null ? null : this.description.getValue(); 1970 } 1971 1972 /** 1973 * @param value A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere. 1974 */ 1975 public AdministrableProductDefinition setDescription(String value) { 1976 if (Utilities.noString(value)) 1977 this.description = null; 1978 else { 1979 if (this.description == null) 1980 this.description = new MarkdownType(); 1981 this.description.setValue(value); 1982 } 1983 return this; 1984 } 1985 1986 /** 1987 * @return {@link #property} (Characteristics e.g. a product's onset of action.) 1988 */ 1989 public List<AdministrableProductDefinitionPropertyComponent> getProperty() { 1990 if (this.property == null) 1991 this.property = new ArrayList<AdministrableProductDefinitionPropertyComponent>(); 1992 return this.property; 1993 } 1994 1995 /** 1996 * @return Returns a reference to <code>this</code> for easy method chaining 1997 */ 1998 public AdministrableProductDefinition setProperty(List<AdministrableProductDefinitionPropertyComponent> theProperty) { 1999 this.property = theProperty; 2000 return this; 2001 } 2002 2003 public boolean hasProperty() { 2004 if (this.property == null) 2005 return false; 2006 for (AdministrableProductDefinitionPropertyComponent item : this.property) 2007 if (!item.isEmpty()) 2008 return true; 2009 return false; 2010 } 2011 2012 public AdministrableProductDefinitionPropertyComponent addProperty() { //3 2013 AdministrableProductDefinitionPropertyComponent t = new AdministrableProductDefinitionPropertyComponent(); 2014 if (this.property == null) 2015 this.property = new ArrayList<AdministrableProductDefinitionPropertyComponent>(); 2016 this.property.add(t); 2017 return t; 2018 } 2019 2020 public AdministrableProductDefinition addProperty(AdministrableProductDefinitionPropertyComponent t) { //3 2021 if (t == null) 2022 return this; 2023 if (this.property == null) 2024 this.property = new ArrayList<AdministrableProductDefinitionPropertyComponent>(); 2025 this.property.add(t); 2026 return this; 2027 } 2028 2029 /** 2030 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 2031 */ 2032 public AdministrableProductDefinitionPropertyComponent getPropertyFirstRep() { 2033 if (getProperty().isEmpty()) { 2034 addProperty(); 2035 } 2036 return getProperty().get(0); 2037 } 2038 2039 /** 2040 * @return {@link #routeOfAdministration} (The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. RouteOfAdministration cannot be used when the 'formOf' product already uses MedicinalProductDefinition.route (and vice versa).) 2041 */ 2042 public List<AdministrableProductDefinitionRouteOfAdministrationComponent> getRouteOfAdministration() { 2043 if (this.routeOfAdministration == null) 2044 this.routeOfAdministration = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationComponent>(); 2045 return this.routeOfAdministration; 2046 } 2047 2048 /** 2049 * @return Returns a reference to <code>this</code> for easy method chaining 2050 */ 2051 public AdministrableProductDefinition setRouteOfAdministration(List<AdministrableProductDefinitionRouteOfAdministrationComponent> theRouteOfAdministration) { 2052 this.routeOfAdministration = theRouteOfAdministration; 2053 return this; 2054 } 2055 2056 public boolean hasRouteOfAdministration() { 2057 if (this.routeOfAdministration == null) 2058 return false; 2059 for (AdministrableProductDefinitionRouteOfAdministrationComponent item : this.routeOfAdministration) 2060 if (!item.isEmpty()) 2061 return true; 2062 return false; 2063 } 2064 2065 public AdministrableProductDefinitionRouteOfAdministrationComponent addRouteOfAdministration() { //3 2066 AdministrableProductDefinitionRouteOfAdministrationComponent t = new AdministrableProductDefinitionRouteOfAdministrationComponent(); 2067 if (this.routeOfAdministration == null) 2068 this.routeOfAdministration = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationComponent>(); 2069 this.routeOfAdministration.add(t); 2070 return t; 2071 } 2072 2073 public AdministrableProductDefinition addRouteOfAdministration(AdministrableProductDefinitionRouteOfAdministrationComponent t) { //3 2074 if (t == null) 2075 return this; 2076 if (this.routeOfAdministration == null) 2077 this.routeOfAdministration = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationComponent>(); 2078 this.routeOfAdministration.add(t); 2079 return this; 2080 } 2081 2082 /** 2083 * @return The first repetition of repeating field {@link #routeOfAdministration}, creating it if it does not already exist {3} 2084 */ 2085 public AdministrableProductDefinitionRouteOfAdministrationComponent getRouteOfAdministrationFirstRep() { 2086 if (getRouteOfAdministration().isEmpty()) { 2087 addRouteOfAdministration(); 2088 } 2089 return getRouteOfAdministration().get(0); 2090 } 2091 2092 protected void listChildren(List<Property> children) { 2093 super.listChildren(children); 2094 children.add(new Property("identifier", "Identifier", "An identifier for the administrable product.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2095 children.add(new Property("status", "code", "The status of this administrable product. Enables tracking the life-cycle of the content.", 0, 1, status)); 2096 children.add(new Property("formOf", "Reference(MedicinalProductDefinition)", "References a product from which one or more of the constituent parts of that product can be prepared and used as described by this administrable product. If this administrable product describes the administration of a crushed tablet, the 'formOf' would be the product representing a distribution containing tablets and possibly also a cream. This is distinct from the 'producedFrom' which refers to the specific components of the product that are used in this preparation, rather than the product as a whole.", 0, java.lang.Integer.MAX_VALUE, formOf)); 2097 children.add(new Property("administrableDoseForm", "CodeableConcept", "The dose form of the final product after necessary reconstitution or processing. Contrasts to the manufactured dose form (see ManufacturedItemDefinition). If the manufactured form was 'powder for solution for injection', the administrable dose form could be 'solution for injection' (once mixed with another item having manufactured form 'solvent for solution for injection').", 0, 1, administrableDoseForm)); 2098 children.add(new Property("unitOfPresentation", "CodeableConcept", "The presentation type in which this item is given to a patient. e.g. for a spray - 'puff' (as in 'contains 100 mcg per puff'), or for a liquid - 'vial' (as in 'contains 5 ml per vial').", 0, 1, unitOfPresentation)); 2099 children.add(new Property("producedFrom", "Reference(ManufacturedItemDefinition)", "Indicates the specific manufactured items that are part of the 'formOf' product that are used in the preparation of this specific administrable form. In some cases, an administrable form might use all of the items from the overall product (or there might only be one item), while in other cases, an administrable form might use only a subset of the items available in the overall product. For example, an administrable form might involve combining a liquid and a powder available as part of an overall product, but not involve applying the also supplied cream.", 0, java.lang.Integer.MAX_VALUE, producedFrom)); 2100 children.add(new Property("ingredient", "CodeableConcept", "The ingredients of this administrable medicinal product. This is only needed if the ingredients are not specified either using ManufacturedItemDefiniton (via AdministrableProductDefinition.producedFrom) to state which component items are used to make this, or using by incoming references from the Ingredient resource, to state in detail which substances exist within this. This element allows a basic coded ingredient to be used.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 2101 children.add(new Property("device", "Reference(DeviceDefinition)", "A device that is integral to the medicinal product, in effect being considered as an \"ingredient\" of the medicinal product. This is not intended for devices that are just co-packaged.", 0, 1, device)); 2102 children.add(new Property("description", "markdown", "A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere.", 0, 1, description)); 2103 children.add(new Property("property", "", "Characteristics e.g. a product's onset of action.", 0, java.lang.Integer.MAX_VALUE, property)); 2104 children.add(new Property("routeOfAdministration", "", "The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. RouteOfAdministration cannot be used when the 'formOf' product already uses MedicinalProductDefinition.route (and vice versa).", 0, java.lang.Integer.MAX_VALUE, routeOfAdministration)); 2105 } 2106 2107 @Override 2108 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2109 switch (_hash) { 2110 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier for the administrable product.", 0, java.lang.Integer.MAX_VALUE, identifier); 2111 case -892481550: /*status*/ return new Property("status", "code", "The status of this administrable product. Enables tracking the life-cycle of the content.", 0, 1, status); 2112 case -1268779589: /*formOf*/ return new Property("formOf", "Reference(MedicinalProductDefinition)", "References a product from which one or more of the constituent parts of that product can be prepared and used as described by this administrable product. If this administrable product describes the administration of a crushed tablet, the 'formOf' would be the product representing a distribution containing tablets and possibly also a cream. This is distinct from the 'producedFrom' which refers to the specific components of the product that are used in this preparation, rather than the product as a whole.", 0, java.lang.Integer.MAX_VALUE, formOf); 2113 case 1446105202: /*administrableDoseForm*/ return new Property("administrableDoseForm", "CodeableConcept", "The dose form of the final product after necessary reconstitution or processing. Contrasts to the manufactured dose form (see ManufacturedItemDefinition). If the manufactured form was 'powder for solution for injection', the administrable dose form could be 'solution for injection' (once mixed with another item having manufactured form 'solvent for solution for injection').", 0, 1, administrableDoseForm); 2114 case -1427765963: /*unitOfPresentation*/ return new Property("unitOfPresentation", "CodeableConcept", "The presentation type in which this item is given to a patient. e.g. for a spray - 'puff' (as in 'contains 100 mcg per puff'), or for a liquid - 'vial' (as in 'contains 5 ml per vial').", 0, 1, unitOfPresentation); 2115 case 588380494: /*producedFrom*/ return new Property("producedFrom", "Reference(ManufacturedItemDefinition)", "Indicates the specific manufactured items that are part of the 'formOf' product that are used in the preparation of this specific administrable form. In some cases, an administrable form might use all of the items from the overall product (or there might only be one item), while in other cases, an administrable form might use only a subset of the items available in the overall product. For example, an administrable form might involve combining a liquid and a powder available as part of an overall product, but not involve applying the also supplied cream.", 0, java.lang.Integer.MAX_VALUE, producedFrom); 2116 case -206409263: /*ingredient*/ return new Property("ingredient", "CodeableConcept", "The ingredients of this administrable medicinal product. This is only needed if the ingredients are not specified either using ManufacturedItemDefiniton (via AdministrableProductDefinition.producedFrom) to state which component items are used to make this, or using by incoming references from the Ingredient resource, to state in detail which substances exist within this. This element allows a basic coded ingredient to be used.", 0, java.lang.Integer.MAX_VALUE, ingredient); 2117 case -1335157162: /*device*/ return new Property("device", "Reference(DeviceDefinition)", "A device that is integral to the medicinal product, in effect being considered as an \"ingredient\" of the medicinal product. This is not intended for devices that are just co-packaged.", 0, 1, device); 2118 case -1724546052: /*description*/ return new Property("description", "markdown", "A general description of the product, when in its final form, suitable for administration e.g. effervescent blue liquid, to be swallowed. Intended to be used when the other structured properties of this resource are insufficient or cannot be supported. It is not intended to duplicate information already carried elswehere.", 0, 1, description); 2119 case -993141291: /*property*/ return new Property("property", "", "Characteristics e.g. a product's onset of action.", 0, java.lang.Integer.MAX_VALUE, property); 2120 case 1742084734: /*routeOfAdministration*/ return new Property("routeOfAdministration", "", "The path by which the product is taken into or makes contact with the body. In some regions this is referred to as the licenced or approved route. RouteOfAdministration cannot be used when the 'formOf' product already uses MedicinalProductDefinition.route (and vice versa).", 0, java.lang.Integer.MAX_VALUE, routeOfAdministration); 2121 default: return super.getNamedProperty(_hash, _name, _checkValid); 2122 } 2123 2124 } 2125 2126 @Override 2127 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2128 switch (hash) { 2129 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2130 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2131 case -1268779589: /*formOf*/ return this.formOf == null ? new Base[0] : this.formOf.toArray(new Base[this.formOf.size()]); // Reference 2132 case 1446105202: /*administrableDoseForm*/ return this.administrableDoseForm == null ? new Base[0] : new Base[] {this.administrableDoseForm}; // CodeableConcept 2133 case -1427765963: /*unitOfPresentation*/ return this.unitOfPresentation == null ? new Base[0] : new Base[] {this.unitOfPresentation}; // CodeableConcept 2134 case 588380494: /*producedFrom*/ return this.producedFrom == null ? new Base[0] : this.producedFrom.toArray(new Base[this.producedFrom.size()]); // Reference 2135 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // CodeableConcept 2136 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 2137 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2138 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // AdministrableProductDefinitionPropertyComponent 2139 case 1742084734: /*routeOfAdministration*/ return this.routeOfAdministration == null ? new Base[0] : this.routeOfAdministration.toArray(new Base[this.routeOfAdministration.size()]); // AdministrableProductDefinitionRouteOfAdministrationComponent 2140 default: return super.getProperty(hash, name, checkValid); 2141 } 2142 2143 } 2144 2145 @Override 2146 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2147 switch (hash) { 2148 case -1618432855: // identifier 2149 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2150 return value; 2151 case -892481550: // status 2152 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2153 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2154 return value; 2155 case -1268779589: // formOf 2156 this.getFormOf().add(TypeConvertor.castToReference(value)); // Reference 2157 return value; 2158 case 1446105202: // administrableDoseForm 2159 this.administrableDoseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2160 return value; 2161 case -1427765963: // unitOfPresentation 2162 this.unitOfPresentation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2163 return value; 2164 case 588380494: // producedFrom 2165 this.getProducedFrom().add(TypeConvertor.castToReference(value)); // Reference 2166 return value; 2167 case -206409263: // ingredient 2168 this.getIngredient().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2169 return value; 2170 case -1335157162: // device 2171 this.device = TypeConvertor.castToReference(value); // Reference 2172 return value; 2173 case -1724546052: // description 2174 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2175 return value; 2176 case -993141291: // property 2177 this.getProperty().add((AdministrableProductDefinitionPropertyComponent) value); // AdministrableProductDefinitionPropertyComponent 2178 return value; 2179 case 1742084734: // routeOfAdministration 2180 this.getRouteOfAdministration().add((AdministrableProductDefinitionRouteOfAdministrationComponent) value); // AdministrableProductDefinitionRouteOfAdministrationComponent 2181 return value; 2182 default: return super.setProperty(hash, name, value); 2183 } 2184 2185 } 2186 2187 @Override 2188 public Base setProperty(String name, Base value) throws FHIRException { 2189 if (name.equals("identifier")) { 2190 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2191 } else if (name.equals("status")) { 2192 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2193 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2194 } else if (name.equals("formOf")) { 2195 this.getFormOf().add(TypeConvertor.castToReference(value)); 2196 } else if (name.equals("administrableDoseForm")) { 2197 this.administrableDoseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2198 } else if (name.equals("unitOfPresentation")) { 2199 this.unitOfPresentation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2200 } else if (name.equals("producedFrom")) { 2201 this.getProducedFrom().add(TypeConvertor.castToReference(value)); 2202 } else if (name.equals("ingredient")) { 2203 this.getIngredient().add(TypeConvertor.castToCodeableConcept(value)); 2204 } else if (name.equals("device")) { 2205 this.device = TypeConvertor.castToReference(value); // Reference 2206 } else if (name.equals("description")) { 2207 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2208 } else if (name.equals("property")) { 2209 this.getProperty().add((AdministrableProductDefinitionPropertyComponent) value); 2210 } else if (name.equals("routeOfAdministration")) { 2211 this.getRouteOfAdministration().add((AdministrableProductDefinitionRouteOfAdministrationComponent) value); 2212 } else 2213 return super.setProperty(name, value); 2214 return value; 2215 } 2216 2217 @Override 2218 public void removeChild(String name, Base value) throws FHIRException { 2219 if (name.equals("identifier")) { 2220 this.getIdentifier().remove(value); 2221 } else if (name.equals("status")) { 2222 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2223 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2224 } else if (name.equals("formOf")) { 2225 this.getFormOf().remove(value); 2226 } else if (name.equals("administrableDoseForm")) { 2227 this.administrableDoseForm = null; 2228 } else if (name.equals("unitOfPresentation")) { 2229 this.unitOfPresentation = null; 2230 } else if (name.equals("producedFrom")) { 2231 this.getProducedFrom().remove(value); 2232 } else if (name.equals("ingredient")) { 2233 this.getIngredient().remove(value); 2234 } else if (name.equals("device")) { 2235 this.device = null; 2236 } else if (name.equals("description")) { 2237 this.description = null; 2238 } else if (name.equals("property")) { 2239 this.getProperty().remove((AdministrableProductDefinitionPropertyComponent) value); 2240 } else if (name.equals("routeOfAdministration")) { 2241 this.getRouteOfAdministration().remove((AdministrableProductDefinitionRouteOfAdministrationComponent) value); 2242 } else 2243 super.removeChild(name, value); 2244 2245 } 2246 2247 @Override 2248 public Base makeProperty(int hash, String name) throws FHIRException { 2249 switch (hash) { 2250 case -1618432855: return addIdentifier(); 2251 case -892481550: return getStatusElement(); 2252 case -1268779589: return addFormOf(); 2253 case 1446105202: return getAdministrableDoseForm(); 2254 case -1427765963: return getUnitOfPresentation(); 2255 case 588380494: return addProducedFrom(); 2256 case -206409263: return addIngredient(); 2257 case -1335157162: return getDevice(); 2258 case -1724546052: return getDescriptionElement(); 2259 case -993141291: return addProperty(); 2260 case 1742084734: return addRouteOfAdministration(); 2261 default: return super.makeProperty(hash, name); 2262 } 2263 2264 } 2265 2266 @Override 2267 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2268 switch (hash) { 2269 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2270 case -892481550: /*status*/ return new String[] {"code"}; 2271 case -1268779589: /*formOf*/ return new String[] {"Reference"}; 2272 case 1446105202: /*administrableDoseForm*/ return new String[] {"CodeableConcept"}; 2273 case -1427765963: /*unitOfPresentation*/ return new String[] {"CodeableConcept"}; 2274 case 588380494: /*producedFrom*/ return new String[] {"Reference"}; 2275 case -206409263: /*ingredient*/ return new String[] {"CodeableConcept"}; 2276 case -1335157162: /*device*/ return new String[] {"Reference"}; 2277 case -1724546052: /*description*/ return new String[] {"markdown"}; 2278 case -993141291: /*property*/ return new String[] {}; 2279 case 1742084734: /*routeOfAdministration*/ return new String[] {}; 2280 default: return super.getTypesForProperty(hash, name); 2281 } 2282 2283 } 2284 2285 @Override 2286 public Base addChild(String name) throws FHIRException { 2287 if (name.equals("identifier")) { 2288 return addIdentifier(); 2289 } 2290 else if (name.equals("status")) { 2291 throw new FHIRException("Cannot call addChild on a singleton property AdministrableProductDefinition.status"); 2292 } 2293 else if (name.equals("formOf")) { 2294 return addFormOf(); 2295 } 2296 else if (name.equals("administrableDoseForm")) { 2297 this.administrableDoseForm = new CodeableConcept(); 2298 return this.administrableDoseForm; 2299 } 2300 else if (name.equals("unitOfPresentation")) { 2301 this.unitOfPresentation = new CodeableConcept(); 2302 return this.unitOfPresentation; 2303 } 2304 else if (name.equals("producedFrom")) { 2305 return addProducedFrom(); 2306 } 2307 else if (name.equals("ingredient")) { 2308 return addIngredient(); 2309 } 2310 else if (name.equals("device")) { 2311 this.device = new Reference(); 2312 return this.device; 2313 } 2314 else if (name.equals("description")) { 2315 throw new FHIRException("Cannot call addChild on a singleton property AdministrableProductDefinition.description"); 2316 } 2317 else if (name.equals("property")) { 2318 return addProperty(); 2319 } 2320 else if (name.equals("routeOfAdministration")) { 2321 return addRouteOfAdministration(); 2322 } 2323 else 2324 return super.addChild(name); 2325 } 2326 2327 public String fhirType() { 2328 return "AdministrableProductDefinition"; 2329 2330 } 2331 2332 public AdministrableProductDefinition copy() { 2333 AdministrableProductDefinition dst = new AdministrableProductDefinition(); 2334 copyValues(dst); 2335 return dst; 2336 } 2337 2338 public void copyValues(AdministrableProductDefinition dst) { 2339 super.copyValues(dst); 2340 if (identifier != null) { 2341 dst.identifier = new ArrayList<Identifier>(); 2342 for (Identifier i : identifier) 2343 dst.identifier.add(i.copy()); 2344 }; 2345 dst.status = status == null ? null : status.copy(); 2346 if (formOf != null) { 2347 dst.formOf = new ArrayList<Reference>(); 2348 for (Reference i : formOf) 2349 dst.formOf.add(i.copy()); 2350 }; 2351 dst.administrableDoseForm = administrableDoseForm == null ? null : administrableDoseForm.copy(); 2352 dst.unitOfPresentation = unitOfPresentation == null ? null : unitOfPresentation.copy(); 2353 if (producedFrom != null) { 2354 dst.producedFrom = new ArrayList<Reference>(); 2355 for (Reference i : producedFrom) 2356 dst.producedFrom.add(i.copy()); 2357 }; 2358 if (ingredient != null) { 2359 dst.ingredient = new ArrayList<CodeableConcept>(); 2360 for (CodeableConcept i : ingredient) 2361 dst.ingredient.add(i.copy()); 2362 }; 2363 dst.device = device == null ? null : device.copy(); 2364 dst.description = description == null ? null : description.copy(); 2365 if (property != null) { 2366 dst.property = new ArrayList<AdministrableProductDefinitionPropertyComponent>(); 2367 for (AdministrableProductDefinitionPropertyComponent i : property) 2368 dst.property.add(i.copy()); 2369 }; 2370 if (routeOfAdministration != null) { 2371 dst.routeOfAdministration = new ArrayList<AdministrableProductDefinitionRouteOfAdministrationComponent>(); 2372 for (AdministrableProductDefinitionRouteOfAdministrationComponent i : routeOfAdministration) 2373 dst.routeOfAdministration.add(i.copy()); 2374 }; 2375 } 2376 2377 protected AdministrableProductDefinition typedCopy() { 2378 return copy(); 2379 } 2380 2381 @Override 2382 public boolean equalsDeep(Base other_) { 2383 if (!super.equalsDeep(other_)) 2384 return false; 2385 if (!(other_ instanceof AdministrableProductDefinition)) 2386 return false; 2387 AdministrableProductDefinition o = (AdministrableProductDefinition) other_; 2388 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(formOf, o.formOf, true) 2389 && compareDeep(administrableDoseForm, o.administrableDoseForm, true) && compareDeep(unitOfPresentation, o.unitOfPresentation, true) 2390 && compareDeep(producedFrom, o.producedFrom, true) && compareDeep(ingredient, o.ingredient, true) 2391 && compareDeep(device, o.device, true) && compareDeep(description, o.description, true) && compareDeep(property, o.property, true) 2392 && compareDeep(routeOfAdministration, o.routeOfAdministration, true); 2393 } 2394 2395 @Override 2396 public boolean equalsShallow(Base other_) { 2397 if (!super.equalsShallow(other_)) 2398 return false; 2399 if (!(other_ instanceof AdministrableProductDefinition)) 2400 return false; 2401 AdministrableProductDefinition o = (AdministrableProductDefinition) other_; 2402 return compareValues(status, o.status, true) && compareValues(description, o.description, true); 2403 } 2404 2405 public boolean isEmpty() { 2406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, formOf 2407 , administrableDoseForm, unitOfPresentation, producedFrom, ingredient, device, description 2408 , property, routeOfAdministration); 2409 } 2410 2411 @Override 2412 public ResourceType getResourceType() { 2413 return ResourceType.AdministrableProductDefinition; 2414 } 2415 2416 /** 2417 * Search parameter: <b>device</b> 2418 * <p> 2419 * Description: <b>A device that is integral to the medicinal product, in effect being considered as an "ingredient" of the medicinal product. This is not intended for devices that are just co-packaged</b><br> 2420 * Type: <b>reference</b><br> 2421 * Path: <b>AdministrableProductDefinition.device</b><br> 2422 * </p> 2423 */ 2424 @SearchParamDefinition(name="device", path="AdministrableProductDefinition.device", description="A device that is integral to the medicinal product, in effect being considered as an \"ingredient\" of the medicinal product. This is not intended for devices that are just co-packaged", type="reference", target={DeviceDefinition.class } ) 2425 public static final String SP_DEVICE = "device"; 2426 /** 2427 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2428 * <p> 2429 * Description: <b>A device that is integral to the medicinal product, in effect being considered as an "ingredient" of the medicinal product. This is not intended for devices that are just co-packaged</b><br> 2430 * Type: <b>reference</b><br> 2431 * Path: <b>AdministrableProductDefinition.device</b><br> 2432 * </p> 2433 */ 2434 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 2435 2436/** 2437 * Constant for fluent queries to be used to add include statements. Specifies 2438 * the path value of "<b>AdministrableProductDefinition:device</b>". 2439 */ 2440 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("AdministrableProductDefinition:device").toLocked(); 2441 2442 /** 2443 * Search parameter: <b>dose-form</b> 2444 * <p> 2445 * Description: <b>The administrable dose form, i.e. the dose form of the final product after necessary reconstitution or processing</b><br> 2446 * Type: <b>token</b><br> 2447 * Path: <b>AdministrableProductDefinition.administrableDoseForm</b><br> 2448 * </p> 2449 */ 2450 @SearchParamDefinition(name="dose-form", path="AdministrableProductDefinition.administrableDoseForm", description="The administrable dose form, i.e. the dose form of the final product after necessary reconstitution or processing", type="token" ) 2451 public static final String SP_DOSE_FORM = "dose-form"; 2452 /** 2453 * <b>Fluent Client</b> search parameter constant for <b>dose-form</b> 2454 * <p> 2455 * Description: <b>The administrable dose form, i.e. the dose form of the final product after necessary reconstitution or processing</b><br> 2456 * Type: <b>token</b><br> 2457 * Path: <b>AdministrableProductDefinition.administrableDoseForm</b><br> 2458 * </p> 2459 */ 2460 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSE_FORM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOSE_FORM); 2461 2462 /** 2463 * Search parameter: <b>form-of</b> 2464 * <p> 2465 * Description: <b>The medicinal product that this is an administrable form of. This is not a reference to the item(s) that make up this administrable form - it is the whole product</b><br> 2466 * Type: <b>reference</b><br> 2467 * Path: <b>AdministrableProductDefinition.formOf</b><br> 2468 * </p> 2469 */ 2470 @SearchParamDefinition(name="form-of", path="AdministrableProductDefinition.formOf", description="The medicinal product that this is an administrable form of. This is not a reference to the item(s) that make up this administrable form - it is the whole product", type="reference", target={MedicinalProductDefinition.class } ) 2471 public static final String SP_FORM_OF = "form-of"; 2472 /** 2473 * <b>Fluent Client</b> search parameter constant for <b>form-of</b> 2474 * <p> 2475 * Description: <b>The medicinal product that this is an administrable form of. This is not a reference to the item(s) that make up this administrable form - it is the whole product</b><br> 2476 * Type: <b>reference</b><br> 2477 * Path: <b>AdministrableProductDefinition.formOf</b><br> 2478 * </p> 2479 */ 2480 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FORM_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FORM_OF); 2481 2482/** 2483 * Constant for fluent queries to be used to add include statements. Specifies 2484 * the path value of "<b>AdministrableProductDefinition:form-of</b>". 2485 */ 2486 public static final ca.uhn.fhir.model.api.Include INCLUDE_FORM_OF = new ca.uhn.fhir.model.api.Include("AdministrableProductDefinition:form-of").toLocked(); 2487 2488 /** 2489 * Search parameter: <b>identifier</b> 2490 * <p> 2491 * Description: <b>An identifier for the administrable product</b><br> 2492 * Type: <b>token</b><br> 2493 * Path: <b>AdministrableProductDefinition.identifier</b><br> 2494 * </p> 2495 */ 2496 @SearchParamDefinition(name="identifier", path="AdministrableProductDefinition.identifier", description="An identifier for the administrable product", type="token" ) 2497 public static final String SP_IDENTIFIER = "identifier"; 2498 /** 2499 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2500 * <p> 2501 * Description: <b>An identifier for the administrable product</b><br> 2502 * Type: <b>token</b><br> 2503 * Path: <b>AdministrableProductDefinition.identifier</b><br> 2504 * </p> 2505 */ 2506 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2507 2508 /** 2509 * Search parameter: <b>ingredient</b> 2510 * <p> 2511 * Description: <b>The ingredients of this administrable medicinal product</b><br> 2512 * Type: <b>token</b><br> 2513 * Path: <b>AdministrableProductDefinition.ingredient</b><br> 2514 * </p> 2515 */ 2516 @SearchParamDefinition(name="ingredient", path="AdministrableProductDefinition.ingredient", description="The ingredients of this administrable medicinal product", type="token" ) 2517 public static final String SP_INGREDIENT = "ingredient"; 2518 /** 2519 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 2520 * <p> 2521 * Description: <b>The ingredients of this administrable medicinal product</b><br> 2522 * Type: <b>token</b><br> 2523 * Path: <b>AdministrableProductDefinition.ingredient</b><br> 2524 * </p> 2525 */ 2526 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT); 2527 2528 /** 2529 * Search parameter: <b>manufactured-item</b> 2530 * <p> 2531 * Description: <b>The manufactured item(s) that this administrable product is produced from. Either a single item, or several that are mixed before administration (e.g. a power item and a solution item). Note that these are not raw ingredients</b><br> 2532 * Type: <b>reference</b><br> 2533 * Path: <b>AdministrableProductDefinition.producedFrom</b><br> 2534 * </p> 2535 */ 2536 @SearchParamDefinition(name="manufactured-item", path="AdministrableProductDefinition.producedFrom", description="The manufactured item(s) that this administrable product is produced from. Either a single item, or several that are mixed before administration (e.g. a power item and a solution item). Note that these are not raw ingredients", type="reference", target={ManufacturedItemDefinition.class } ) 2537 public static final String SP_MANUFACTURED_ITEM = "manufactured-item"; 2538 /** 2539 * <b>Fluent Client</b> search parameter constant for <b>manufactured-item</b> 2540 * <p> 2541 * Description: <b>The manufactured item(s) that this administrable product is produced from. Either a single item, or several that are mixed before administration (e.g. a power item and a solution item). Note that these are not raw ingredients</b><br> 2542 * Type: <b>reference</b><br> 2543 * Path: <b>AdministrableProductDefinition.producedFrom</b><br> 2544 * </p> 2545 */ 2546 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURED_ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURED_ITEM); 2547 2548/** 2549 * Constant for fluent queries to be used to add include statements. Specifies 2550 * the path value of "<b>AdministrableProductDefinition:manufactured-item</b>". 2551 */ 2552 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURED_ITEM = new ca.uhn.fhir.model.api.Include("AdministrableProductDefinition:manufactured-item").toLocked(); 2553 2554 /** 2555 * Search parameter: <b>route</b> 2556 * <p> 2557 * Description: <b>Coded expression for the route</b><br> 2558 * Type: <b>token</b><br> 2559 * Path: <b>AdministrableProductDefinition.routeOfAdministration.code</b><br> 2560 * </p> 2561 */ 2562 @SearchParamDefinition(name="route", path="AdministrableProductDefinition.routeOfAdministration.code", description="Coded expression for the route", type="token" ) 2563 public static final String SP_ROUTE = "route"; 2564 /** 2565 * <b>Fluent Client</b> search parameter constant for <b>route</b> 2566 * <p> 2567 * Description: <b>Coded expression for the route</b><br> 2568 * Type: <b>token</b><br> 2569 * Path: <b>AdministrableProductDefinition.routeOfAdministration.code</b><br> 2570 * </p> 2571 */ 2572 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROUTE); 2573 2574 /** 2575 * Search parameter: <b>status</b> 2576 * <p> 2577 * Description: <b>The status of this administrable product. Enables tracking the life-cycle of the content.</b><br> 2578 * Type: <b>token</b><br> 2579 * Path: <b>AdministrableProductDefinition.status</b><br> 2580 * </p> 2581 */ 2582 @SearchParamDefinition(name="status", path="AdministrableProductDefinition.status", description="The status of this administrable product. Enables tracking the life-cycle of the content.", type="token" ) 2583 public static final String SP_STATUS = "status"; 2584 /** 2585 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2586 * <p> 2587 * Description: <b>The status of this administrable product. Enables tracking the life-cycle of the content.</b><br> 2588 * Type: <b>token</b><br> 2589 * Path: <b>AdministrableProductDefinition.status</b><br> 2590 * </p> 2591 */ 2592 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2593 2594 /** 2595 * Search parameter: <b>target-species</b> 2596 * <p> 2597 * Description: <b>Coded expression for the species</b><br> 2598 * Type: <b>token</b><br> 2599 * Path: <b>AdministrableProductDefinition.routeOfAdministration.targetSpecies.code</b><br> 2600 * </p> 2601 */ 2602 @SearchParamDefinition(name="target-species", path="AdministrableProductDefinition.routeOfAdministration.targetSpecies.code", description="Coded expression for the species", type="token" ) 2603 public static final String SP_TARGET_SPECIES = "target-species"; 2604 /** 2605 * <b>Fluent Client</b> search parameter constant for <b>target-species</b> 2606 * <p> 2607 * Description: <b>Coded expression for the species</b><br> 2608 * Type: <b>token</b><br> 2609 * Path: <b>AdministrableProductDefinition.routeOfAdministration.targetSpecies.code</b><br> 2610 * </p> 2611 */ 2612 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_SPECIES = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_SPECIES); 2613 2614 2615} 2616