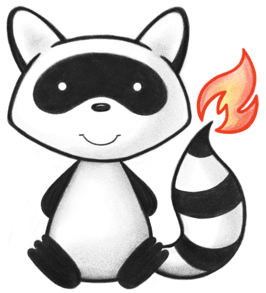
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An event (i.e. any change to current patient status) that may be related to unintended effects on a patient or research participant. The unintended effects may require additional monitoring, treatment, hospitalization, or may result in death. The AdverseEvent resource also extends to potential or avoided events that could have had such effects. There are two major domains where the AdverseEvent resource is expected to be used. One is in clinical care reported adverse events and the other is in reporting adverse events in clinical research trial management. Adverse events can be reported by healthcare providers, patients, caregivers or by medical products manufacturers. Given the differences between these two concepts, we recommend consulting the domain specific implementation guides when implementing the AdverseEvent Resource. The implementation guides include specific extensions, value sets and constraints. 052 */ 053@ResourceDef(name="AdverseEvent", profile="http://hl7.org/fhir/StructureDefinition/AdverseEvent") 054public class AdverseEvent extends DomainResource { 055 056 public enum AdverseEventActuality { 057 /** 058 * The adverse event actually happened regardless of whether anyone was affected or harmed. 059 */ 060 ACTUAL, 061 /** 062 * A potential adverse event. 063 */ 064 POTENTIAL, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static AdverseEventActuality fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("actual".equals(codeString)) 073 return ACTUAL; 074 if ("potential".equals(codeString)) 075 return POTENTIAL; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown AdverseEventActuality code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case ACTUAL: return "actual"; 084 case POTENTIAL: return "potential"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case ACTUAL: return "http://hl7.org/fhir/adverse-event-actuality"; 092 case POTENTIAL: return "http://hl7.org/fhir/adverse-event-actuality"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case ACTUAL: return "The adverse event actually happened regardless of whether anyone was affected or harmed."; 100 case POTENTIAL: return "A potential adverse event."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case ACTUAL: return "Adverse Event"; 108 case POTENTIAL: return "Potential Adverse Event"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class AdverseEventActualityEnumFactory implements EnumFactory<AdverseEventActuality> { 116 public AdverseEventActuality fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("actual".equals(codeString)) 121 return AdverseEventActuality.ACTUAL; 122 if ("potential".equals(codeString)) 123 return AdverseEventActuality.POTENTIAL; 124 throw new IllegalArgumentException("Unknown AdverseEventActuality code '"+codeString+"'"); 125 } 126 public Enumeration<AdverseEventActuality> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.NULL, code); 134 if ("actual".equals(codeString)) 135 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.ACTUAL, code); 136 if ("potential".equals(codeString)) 137 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.POTENTIAL, code); 138 throw new FHIRException("Unknown AdverseEventActuality code '"+codeString+"'"); 139 } 140 public String toCode(AdverseEventActuality code) { 141 if (code == AdverseEventActuality.ACTUAL) 142 return "actual"; 143 if (code == AdverseEventActuality.POTENTIAL) 144 return "potential"; 145 return "?"; 146 } 147 public String toSystem(AdverseEventActuality code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum AdverseEventStatus { 153 /** 154 * The event is currently occurring. 155 */ 156 INPROGRESS, 157 /** 158 * The event has now concluded. 159 */ 160 COMPLETED, 161 /** 162 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".). 163 */ 164 ENTEREDINERROR, 165 /** 166 * The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 167 */ 168 UNKNOWN, 169 /** 170 * added to help the parsers with the generic types 171 */ 172 NULL; 173 public static AdverseEventStatus fromCode(String codeString) throws FHIRException { 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("in-progress".equals(codeString)) 177 return INPROGRESS; 178 if ("completed".equals(codeString)) 179 return COMPLETED; 180 if ("entered-in-error".equals(codeString)) 181 return ENTEREDINERROR; 182 if ("unknown".equals(codeString)) 183 return UNKNOWN; 184 if (Configuration.isAcceptInvalidEnums()) 185 return null; 186 else 187 throw new FHIRException("Unknown AdverseEventStatus code '"+codeString+"'"); 188 } 189 public String toCode() { 190 switch (this) { 191 case INPROGRESS: return "in-progress"; 192 case COMPLETED: return "completed"; 193 case ENTEREDINERROR: return "entered-in-error"; 194 case UNKNOWN: return "unknown"; 195 case NULL: return null; 196 default: return "?"; 197 } 198 } 199 public String getSystem() { 200 switch (this) { 201 case INPROGRESS: return "http://hl7.org/fhir/event-status"; 202 case COMPLETED: return "http://hl7.org/fhir/event-status"; 203 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 204 case UNKNOWN: return "http://hl7.org/fhir/event-status"; 205 case NULL: return null; 206 default: return "?"; 207 } 208 } 209 public String getDefinition() { 210 switch (this) { 211 case INPROGRESS: return "The event is currently occurring."; 212 case COMPLETED: return "The event has now concluded."; 213 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 214 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getDisplay() { 220 switch (this) { 221 case INPROGRESS: return "In Progress"; 222 case COMPLETED: return "Completed"; 223 case ENTEREDINERROR: return "Entered in Error"; 224 case UNKNOWN: return "Unknown"; 225 case NULL: return null; 226 default: return "?"; 227 } 228 } 229 } 230 231 public static class AdverseEventStatusEnumFactory implements EnumFactory<AdverseEventStatus> { 232 public AdverseEventStatus fromCode(String codeString) throws IllegalArgumentException { 233 if (codeString == null || "".equals(codeString)) 234 if (codeString == null || "".equals(codeString)) 235 return null; 236 if ("in-progress".equals(codeString)) 237 return AdverseEventStatus.INPROGRESS; 238 if ("completed".equals(codeString)) 239 return AdverseEventStatus.COMPLETED; 240 if ("entered-in-error".equals(codeString)) 241 return AdverseEventStatus.ENTEREDINERROR; 242 if ("unknown".equals(codeString)) 243 return AdverseEventStatus.UNKNOWN; 244 throw new IllegalArgumentException("Unknown AdverseEventStatus code '"+codeString+"'"); 245 } 246 public Enumeration<AdverseEventStatus> fromType(PrimitiveType<?> code) throws FHIRException { 247 if (code == null) 248 return null; 249 if (code.isEmpty()) 250 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.NULL, code); 251 String codeString = ((PrimitiveType) code).asStringValue(); 252 if (codeString == null || "".equals(codeString)) 253 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.NULL, code); 254 if ("in-progress".equals(codeString)) 255 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.INPROGRESS, code); 256 if ("completed".equals(codeString)) 257 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.COMPLETED, code); 258 if ("entered-in-error".equals(codeString)) 259 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.ENTEREDINERROR, code); 260 if ("unknown".equals(codeString)) 261 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.UNKNOWN, code); 262 throw new FHIRException("Unknown AdverseEventStatus code '"+codeString+"'"); 263 } 264 public String toCode(AdverseEventStatus code) { 265 if (code == AdverseEventStatus.INPROGRESS) 266 return "in-progress"; 267 if (code == AdverseEventStatus.COMPLETED) 268 return "completed"; 269 if (code == AdverseEventStatus.ENTEREDINERROR) 270 return "entered-in-error"; 271 if (code == AdverseEventStatus.UNKNOWN) 272 return "unknown"; 273 return "?"; 274 } 275 public String toSystem(AdverseEventStatus code) { 276 return code.getSystem(); 277 } 278 } 279 280 @Block() 281 public static class AdverseEventParticipantComponent extends BackboneElement implements IBaseBackboneElement { 282 /** 283 * Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant. 284 */ 285 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 286 @Description(shortDefinition="Type of involvement", formalDefinition="Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant." ) 287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-participant-function") 288 protected CodeableConcept function; 289 290 /** 291 * Indicates who or what participated in the event. 292 */ 293 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class, ResearchSubject.class}, order=2, min=1, max=1, modifier=false, summary=true) 294 @Description(shortDefinition="Who was involved in the adverse event or the potential adverse event", formalDefinition="Indicates who or what participated in the event." ) 295 protected Reference actor; 296 297 private static final long serialVersionUID = -576943815L; 298 299 /** 300 * Constructor 301 */ 302 public AdverseEventParticipantComponent() { 303 super(); 304 } 305 306 /** 307 * Constructor 308 */ 309 public AdverseEventParticipantComponent(Reference actor) { 310 super(); 311 this.setActor(actor); 312 } 313 314 /** 315 * @return {@link #function} (Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.) 316 */ 317 public CodeableConcept getFunction() { 318 if (this.function == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create AdverseEventParticipantComponent.function"); 321 else if (Configuration.doAutoCreate()) 322 this.function = new CodeableConcept(); // cc 323 return this.function; 324 } 325 326 public boolean hasFunction() { 327 return this.function != null && !this.function.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #function} (Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.) 332 */ 333 public AdverseEventParticipantComponent setFunction(CodeableConcept value) { 334 this.function = value; 335 return this; 336 } 337 338 /** 339 * @return {@link #actor} (Indicates who or what participated in the event.) 340 */ 341 public Reference getActor() { 342 if (this.actor == null) 343 if (Configuration.errorOnAutoCreate()) 344 throw new Error("Attempt to auto-create AdverseEventParticipantComponent.actor"); 345 else if (Configuration.doAutoCreate()) 346 this.actor = new Reference(); // cc 347 return this.actor; 348 } 349 350 public boolean hasActor() { 351 return this.actor != null && !this.actor.isEmpty(); 352 } 353 354 /** 355 * @param value {@link #actor} (Indicates who or what participated in the event.) 356 */ 357 public AdverseEventParticipantComponent setActor(Reference value) { 358 this.actor = value; 359 return this; 360 } 361 362 protected void listChildren(List<Property> children) { 363 super.listChildren(children); 364 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.", 0, 1, function)); 365 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson|ResearchSubject)", "Indicates who or what participated in the event.", 0, 1, actor)); 366 } 367 368 @Override 369 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 370 switch (_hash) { 371 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.", 0, 1, function); 372 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson|ResearchSubject)", "Indicates who or what participated in the event.", 0, 1, actor); 373 default: return super.getNamedProperty(_hash, _name, _checkValid); 374 } 375 376 } 377 378 @Override 379 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 380 switch (hash) { 381 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 382 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 383 default: return super.getProperty(hash, name, checkValid); 384 } 385 386 } 387 388 @Override 389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 390 switch (hash) { 391 case 1380938712: // function 392 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 393 return value; 394 case 92645877: // actor 395 this.actor = TypeConvertor.castToReference(value); // Reference 396 return value; 397 default: return super.setProperty(hash, name, value); 398 } 399 400 } 401 402 @Override 403 public Base setProperty(String name, Base value) throws FHIRException { 404 if (name.equals("function")) { 405 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 406 } else if (name.equals("actor")) { 407 this.actor = TypeConvertor.castToReference(value); // Reference 408 } else 409 return super.setProperty(name, value); 410 return value; 411 } 412 413 @Override 414 public void removeChild(String name, Base value) throws FHIRException { 415 if (name.equals("function")) { 416 this.function = null; 417 } else if (name.equals("actor")) { 418 this.actor = null; 419 } else 420 super.removeChild(name, value); 421 422 } 423 424 @Override 425 public Base makeProperty(int hash, String name) throws FHIRException { 426 switch (hash) { 427 case 1380938712: return getFunction(); 428 case 92645877: return getActor(); 429 default: return super.makeProperty(hash, name); 430 } 431 432 } 433 434 @Override 435 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 436 switch (hash) { 437 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 438 case 92645877: /*actor*/ return new String[] {"Reference"}; 439 default: return super.getTypesForProperty(hash, name); 440 } 441 442 } 443 444 @Override 445 public Base addChild(String name) throws FHIRException { 446 if (name.equals("function")) { 447 this.function = new CodeableConcept(); 448 return this.function; 449 } 450 else if (name.equals("actor")) { 451 this.actor = new Reference(); 452 return this.actor; 453 } 454 else 455 return super.addChild(name); 456 } 457 458 public AdverseEventParticipantComponent copy() { 459 AdverseEventParticipantComponent dst = new AdverseEventParticipantComponent(); 460 copyValues(dst); 461 return dst; 462 } 463 464 public void copyValues(AdverseEventParticipantComponent dst) { 465 super.copyValues(dst); 466 dst.function = function == null ? null : function.copy(); 467 dst.actor = actor == null ? null : actor.copy(); 468 } 469 470 @Override 471 public boolean equalsDeep(Base other_) { 472 if (!super.equalsDeep(other_)) 473 return false; 474 if (!(other_ instanceof AdverseEventParticipantComponent)) 475 return false; 476 AdverseEventParticipantComponent o = (AdverseEventParticipantComponent) other_; 477 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 478 } 479 480 @Override 481 public boolean equalsShallow(Base other_) { 482 if (!super.equalsShallow(other_)) 483 return false; 484 if (!(other_ instanceof AdverseEventParticipantComponent)) 485 return false; 486 AdverseEventParticipantComponent o = (AdverseEventParticipantComponent) other_; 487 return true; 488 } 489 490 public boolean isEmpty() { 491 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 492 } 493 494 public String fhirType() { 495 return "AdverseEvent.participant"; 496 497 } 498 499 } 500 501 @Block() 502 public static class AdverseEventSuspectEntityComponent extends BackboneElement implements IBaseBackboneElement { 503 /** 504 * Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device. 505 */ 506 @Child(name = "instance", type = {CodeableConcept.class, Immunization.class, Procedure.class, Substance.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, Device.class, BiologicallyDerivedProduct.class, ResearchStudy.class}, order=1, min=1, max=1, modifier=false, summary=true) 507 @Description(shortDefinition="Refers to the specific entity that caused the adverse event", formalDefinition="Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device." ) 508 protected DataType instance; 509 510 /** 511 * Information on the possible cause of the event. 512 */ 513 @Child(name = "causality", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 514 @Description(shortDefinition="Information on the possible cause of the event", formalDefinition="Information on the possible cause of the event." ) 515 protected AdverseEventSuspectEntityCausalityComponent causality; 516 517 private static final long serialVersionUID = -1455097004L; 518 519 /** 520 * Constructor 521 */ 522 public AdverseEventSuspectEntityComponent() { 523 super(); 524 } 525 526 /** 527 * Constructor 528 */ 529 public AdverseEventSuspectEntityComponent(DataType instance) { 530 super(); 531 this.setInstance(instance); 532 } 533 534 /** 535 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 536 */ 537 public DataType getInstance() { 538 return this.instance; 539 } 540 541 /** 542 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 543 */ 544 public CodeableConcept getInstanceCodeableConcept() throws FHIRException { 545 if (this.instance == null) 546 this.instance = new CodeableConcept(); 547 if (!(this.instance instanceof CodeableConcept)) 548 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.instance.getClass().getName()+" was encountered"); 549 return (CodeableConcept) this.instance; 550 } 551 552 public boolean hasInstanceCodeableConcept() { 553 return this != null && this.instance instanceof CodeableConcept; 554 } 555 556 /** 557 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 558 */ 559 public Reference getInstanceReference() throws FHIRException { 560 if (this.instance == null) 561 this.instance = new Reference(); 562 if (!(this.instance instanceof Reference)) 563 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.instance.getClass().getName()+" was encountered"); 564 return (Reference) this.instance; 565 } 566 567 public boolean hasInstanceReference() { 568 return this != null && this.instance instanceof Reference; 569 } 570 571 public boolean hasInstance() { 572 return this.instance != null && !this.instance.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 577 */ 578 public AdverseEventSuspectEntityComponent setInstance(DataType value) { 579 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 580 throw new FHIRException("Not the right type for AdverseEvent.suspectEntity.instance[x]: "+value.fhirType()); 581 this.instance = value; 582 return this; 583 } 584 585 /** 586 * @return {@link #causality} (Information on the possible cause of the event.) 587 */ 588 public AdverseEventSuspectEntityCausalityComponent getCausality() { 589 if (this.causality == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causality"); 592 else if (Configuration.doAutoCreate()) 593 this.causality = new AdverseEventSuspectEntityCausalityComponent(); // cc 594 return this.causality; 595 } 596 597 public boolean hasCausality() { 598 return this.causality != null && !this.causality.isEmpty(); 599 } 600 601 /** 602 * @param value {@link #causality} (Information on the possible cause of the event.) 603 */ 604 public AdverseEventSuspectEntityComponent setCausality(AdverseEventSuspectEntityCausalityComponent value) { 605 this.causality = value; 606 return this; 607 } 608 609 protected void listChildren(List<Property> children) { 610 super.listChildren(children); 611 children.add(new Property("instance[x]", "CodeableConcept|Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance)); 612 children.add(new Property("causality", "", "Information on the possible cause of the event.", 0, 1, causality)); 613 } 614 615 @Override 616 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 617 switch (_hash) { 618 case -2101998645: /*instance[x]*/ return new Property("instance[x]", "CodeableConcept|Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 619 case 555127957: /*instance*/ return new Property("instance[x]", "CodeableConcept|Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 620 case 697546316: /*instanceCodeableConcept*/ return new Property("instance[x]", "CodeableConcept", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 621 case -1675877834: /*instanceReference*/ return new Property("instance[x]", "Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 622 case -1446450521: /*causality*/ return new Property("causality", "", "Information on the possible cause of the event.", 0, 1, causality); 623 default: return super.getNamedProperty(_hash, _name, _checkValid); 624 } 625 626 } 627 628 @Override 629 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 630 switch (hash) { 631 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // DataType 632 case -1446450521: /*causality*/ return this.causality == null ? new Base[0] : new Base[] {this.causality}; // AdverseEventSuspectEntityCausalityComponent 633 default: return super.getProperty(hash, name, checkValid); 634 } 635 636 } 637 638 @Override 639 public Base setProperty(int hash, String name, Base value) throws FHIRException { 640 switch (hash) { 641 case 555127957: // instance 642 this.instance = TypeConvertor.castToType(value); // DataType 643 return value; 644 case -1446450521: // causality 645 this.causality = (AdverseEventSuspectEntityCausalityComponent) value; // AdverseEventSuspectEntityCausalityComponent 646 return value; 647 default: return super.setProperty(hash, name, value); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(String name, Base value) throws FHIRException { 654 if (name.equals("instance[x]")) { 655 this.instance = TypeConvertor.castToType(value); // DataType 656 } else if (name.equals("causality")) { 657 this.causality = (AdverseEventSuspectEntityCausalityComponent) value; // AdverseEventSuspectEntityCausalityComponent 658 } else 659 return super.setProperty(name, value); 660 return value; 661 } 662 663 @Override 664 public void removeChild(String name, Base value) throws FHIRException { 665 if (name.equals("instance[x]")) { 666 this.instance = null; 667 } else if (name.equals("causality")) { 668 this.causality = (AdverseEventSuspectEntityCausalityComponent) value; // AdverseEventSuspectEntityCausalityComponent 669 } else 670 super.removeChild(name, value); 671 672 } 673 674 @Override 675 public Base makeProperty(int hash, String name) throws FHIRException { 676 switch (hash) { 677 case -2101998645: return getInstance(); 678 case 555127957: return getInstance(); 679 case -1446450521: return getCausality(); 680 default: return super.makeProperty(hash, name); 681 } 682 683 } 684 685 @Override 686 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 687 switch (hash) { 688 case 555127957: /*instance*/ return new String[] {"CodeableConcept", "Reference"}; 689 case -1446450521: /*causality*/ return new String[] {}; 690 default: return super.getTypesForProperty(hash, name); 691 } 692 693 } 694 695 @Override 696 public Base addChild(String name) throws FHIRException { 697 if (name.equals("instanceCodeableConcept")) { 698 this.instance = new CodeableConcept(); 699 return this.instance; 700 } 701 else if (name.equals("instanceReference")) { 702 this.instance = new Reference(); 703 return this.instance; 704 } 705 else if (name.equals("causality")) { 706 this.causality = new AdverseEventSuspectEntityCausalityComponent(); 707 return this.causality; 708 } 709 else 710 return super.addChild(name); 711 } 712 713 public AdverseEventSuspectEntityComponent copy() { 714 AdverseEventSuspectEntityComponent dst = new AdverseEventSuspectEntityComponent(); 715 copyValues(dst); 716 return dst; 717 } 718 719 public void copyValues(AdverseEventSuspectEntityComponent dst) { 720 super.copyValues(dst); 721 dst.instance = instance == null ? null : instance.copy(); 722 dst.causality = causality == null ? null : causality.copy(); 723 } 724 725 @Override 726 public boolean equalsDeep(Base other_) { 727 if (!super.equalsDeep(other_)) 728 return false; 729 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 730 return false; 731 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 732 return compareDeep(instance, o.instance, true) && compareDeep(causality, o.causality, true); 733 } 734 735 @Override 736 public boolean equalsShallow(Base other_) { 737 if (!super.equalsShallow(other_)) 738 return false; 739 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 740 return false; 741 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 742 return true; 743 } 744 745 public boolean isEmpty() { 746 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(instance, causality); 747 } 748 749 public String fhirType() { 750 return "AdverseEvent.suspectEntity"; 751 752 } 753 754 } 755 756 @Block() 757 public static class AdverseEventSuspectEntityCausalityComponent extends BackboneElement implements IBaseBackboneElement { 758 /** 759 * The method of evaluating the relatedness of the suspected entity to the event. 760 */ 761 @Child(name = "assessmentMethod", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 762 @Description(shortDefinition="Method of evaluating the relatedness of the suspected entity to the event", formalDefinition="The method of evaluating the relatedness of the suspected entity to the event." ) 763 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-method") 764 protected CodeableConcept assessmentMethod; 765 766 /** 767 * The result of the assessment regarding the relatedness of the suspected entity to the event. 768 */ 769 @Child(name = "entityRelatedness", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 770 @Description(shortDefinition="Result of the assessment regarding the relatedness of the suspected entity to the event", formalDefinition="The result of the assessment regarding the relatedness of the suspected entity to the event." ) 771 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-assess") 772 protected CodeableConcept entityRelatedness; 773 774 /** 775 * The author of the information on the possible cause of the event. 776 */ 777 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, ResearchSubject.class}, order=3, min=0, max=1, modifier=false, summary=true) 778 @Description(shortDefinition="Author of the information on the possible cause of the event", formalDefinition="The author of the information on the possible cause of the event." ) 779 protected Reference author; 780 781 private static final long serialVersionUID = 486112728L; 782 783 /** 784 * Constructor 785 */ 786 public AdverseEventSuspectEntityCausalityComponent() { 787 super(); 788 } 789 790 /** 791 * @return {@link #assessmentMethod} (The method of evaluating the relatedness of the suspected entity to the event.) 792 */ 793 public CodeableConcept getAssessmentMethod() { 794 if (this.assessmentMethod == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.assessmentMethod"); 797 else if (Configuration.doAutoCreate()) 798 this.assessmentMethod = new CodeableConcept(); // cc 799 return this.assessmentMethod; 800 } 801 802 public boolean hasAssessmentMethod() { 803 return this.assessmentMethod != null && !this.assessmentMethod.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #assessmentMethod} (The method of evaluating the relatedness of the suspected entity to the event.) 808 */ 809 public AdverseEventSuspectEntityCausalityComponent setAssessmentMethod(CodeableConcept value) { 810 this.assessmentMethod = value; 811 return this; 812 } 813 814 /** 815 * @return {@link #entityRelatedness} (The result of the assessment regarding the relatedness of the suspected entity to the event.) 816 */ 817 public CodeableConcept getEntityRelatedness() { 818 if (this.entityRelatedness == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.entityRelatedness"); 821 else if (Configuration.doAutoCreate()) 822 this.entityRelatedness = new CodeableConcept(); // cc 823 return this.entityRelatedness; 824 } 825 826 public boolean hasEntityRelatedness() { 827 return this.entityRelatedness != null && !this.entityRelatedness.isEmpty(); 828 } 829 830 /** 831 * @param value {@link #entityRelatedness} (The result of the assessment regarding the relatedness of the suspected entity to the event.) 832 */ 833 public AdverseEventSuspectEntityCausalityComponent setEntityRelatedness(CodeableConcept value) { 834 this.entityRelatedness = value; 835 return this; 836 } 837 838 /** 839 * @return {@link #author} (The author of the information on the possible cause of the event.) 840 */ 841 public Reference getAuthor() { 842 if (this.author == null) 843 if (Configuration.errorOnAutoCreate()) 844 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.author"); 845 else if (Configuration.doAutoCreate()) 846 this.author = new Reference(); // cc 847 return this.author; 848 } 849 850 public boolean hasAuthor() { 851 return this.author != null && !this.author.isEmpty(); 852 } 853 854 /** 855 * @param value {@link #author} (The author of the information on the possible cause of the event.) 856 */ 857 public AdverseEventSuspectEntityCausalityComponent setAuthor(Reference value) { 858 this.author = value; 859 return this; 860 } 861 862 protected void listChildren(List<Property> children) { 863 super.listChildren(children); 864 children.add(new Property("assessmentMethod", "CodeableConcept", "The method of evaluating the relatedness of the suspected entity to the event.", 0, 1, assessmentMethod)); 865 children.add(new Property("entityRelatedness", "CodeableConcept", "The result of the assessment regarding the relatedness of the suspected entity to the event.", 0, 1, entityRelatedness)); 866 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|ResearchSubject)", "The author of the information on the possible cause of the event.", 0, 1, author)); 867 } 868 869 @Override 870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 871 switch (_hash) { 872 case 1681283651: /*assessmentMethod*/ return new Property("assessmentMethod", "CodeableConcept", "The method of evaluating the relatedness of the suspected entity to the event.", 0, 1, assessmentMethod); 873 case 2000199967: /*entityRelatedness*/ return new Property("entityRelatedness", "CodeableConcept", "The result of the assessment regarding the relatedness of the suspected entity to the event.", 0, 1, entityRelatedness); 874 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|ResearchSubject)", "The author of the information on the possible cause of the event.", 0, 1, author); 875 default: return super.getNamedProperty(_hash, _name, _checkValid); 876 } 877 878 } 879 880 @Override 881 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 882 switch (hash) { 883 case 1681283651: /*assessmentMethod*/ return this.assessmentMethod == null ? new Base[0] : new Base[] {this.assessmentMethod}; // CodeableConcept 884 case 2000199967: /*entityRelatedness*/ return this.entityRelatedness == null ? new Base[0] : new Base[] {this.entityRelatedness}; // CodeableConcept 885 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 886 default: return super.getProperty(hash, name, checkValid); 887 } 888 889 } 890 891 @Override 892 public Base setProperty(int hash, String name, Base value) throws FHIRException { 893 switch (hash) { 894 case 1681283651: // assessmentMethod 895 this.assessmentMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 896 return value; 897 case 2000199967: // entityRelatedness 898 this.entityRelatedness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 899 return value; 900 case -1406328437: // author 901 this.author = TypeConvertor.castToReference(value); // Reference 902 return value; 903 default: return super.setProperty(hash, name, value); 904 } 905 906 } 907 908 @Override 909 public Base setProperty(String name, Base value) throws FHIRException { 910 if (name.equals("assessmentMethod")) { 911 this.assessmentMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 912 } else if (name.equals("entityRelatedness")) { 913 this.entityRelatedness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 914 } else if (name.equals("author")) { 915 this.author = TypeConvertor.castToReference(value); // Reference 916 } else 917 return super.setProperty(name, value); 918 return value; 919 } 920 921 @Override 922 public void removeChild(String name, Base value) throws FHIRException { 923 if (name.equals("assessmentMethod")) { 924 this.assessmentMethod = null; 925 } else if (name.equals("entityRelatedness")) { 926 this.entityRelatedness = null; 927 } else if (name.equals("author")) { 928 this.author = null; 929 } else 930 super.removeChild(name, value); 931 932 } 933 934 @Override 935 public Base makeProperty(int hash, String name) throws FHIRException { 936 switch (hash) { 937 case 1681283651: return getAssessmentMethod(); 938 case 2000199967: return getEntityRelatedness(); 939 case -1406328437: return getAuthor(); 940 default: return super.makeProperty(hash, name); 941 } 942 943 } 944 945 @Override 946 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 947 switch (hash) { 948 case 1681283651: /*assessmentMethod*/ return new String[] {"CodeableConcept"}; 949 case 2000199967: /*entityRelatedness*/ return new String[] {"CodeableConcept"}; 950 case -1406328437: /*author*/ return new String[] {"Reference"}; 951 default: return super.getTypesForProperty(hash, name); 952 } 953 954 } 955 956 @Override 957 public Base addChild(String name) throws FHIRException { 958 if (name.equals("assessmentMethod")) { 959 this.assessmentMethod = new CodeableConcept(); 960 return this.assessmentMethod; 961 } 962 else if (name.equals("entityRelatedness")) { 963 this.entityRelatedness = new CodeableConcept(); 964 return this.entityRelatedness; 965 } 966 else if (name.equals("author")) { 967 this.author = new Reference(); 968 return this.author; 969 } 970 else 971 return super.addChild(name); 972 } 973 974 public AdverseEventSuspectEntityCausalityComponent copy() { 975 AdverseEventSuspectEntityCausalityComponent dst = new AdverseEventSuspectEntityCausalityComponent(); 976 copyValues(dst); 977 return dst; 978 } 979 980 public void copyValues(AdverseEventSuspectEntityCausalityComponent dst) { 981 super.copyValues(dst); 982 dst.assessmentMethod = assessmentMethod == null ? null : assessmentMethod.copy(); 983 dst.entityRelatedness = entityRelatedness == null ? null : entityRelatedness.copy(); 984 dst.author = author == null ? null : author.copy(); 985 } 986 987 @Override 988 public boolean equalsDeep(Base other_) { 989 if (!super.equalsDeep(other_)) 990 return false; 991 if (!(other_ instanceof AdverseEventSuspectEntityCausalityComponent)) 992 return false; 993 AdverseEventSuspectEntityCausalityComponent o = (AdverseEventSuspectEntityCausalityComponent) other_; 994 return compareDeep(assessmentMethod, o.assessmentMethod, true) && compareDeep(entityRelatedness, o.entityRelatedness, true) 995 && compareDeep(author, o.author, true); 996 } 997 998 @Override 999 public boolean equalsShallow(Base other_) { 1000 if (!super.equalsShallow(other_)) 1001 return false; 1002 if (!(other_ instanceof AdverseEventSuspectEntityCausalityComponent)) 1003 return false; 1004 AdverseEventSuspectEntityCausalityComponent o = (AdverseEventSuspectEntityCausalityComponent) other_; 1005 return true; 1006 } 1007 1008 public boolean isEmpty() { 1009 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(assessmentMethod, entityRelatedness 1010 , author); 1011 } 1012 1013 public String fhirType() { 1014 return "AdverseEvent.suspectEntity.causality"; 1015 1016 } 1017 1018 } 1019 1020 @Block() 1021 public static class AdverseEventContributingFactorComponent extends BackboneElement implements IBaseBackboneElement { 1022 /** 1023 * The item that is suspected to have increased the probability or severity of the adverse event. 1024 */ 1025 @Child(name = "item", type = {Condition.class, Observation.class, AllergyIntolerance.class, FamilyMemberHistory.class, Immunization.class, Procedure.class, Device.class, DeviceUsage.class, DocumentReference.class, MedicationAdministration.class, MedicationStatement.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1026 @Description(shortDefinition="Item suspected to have increased the probability or severity of the adverse event", formalDefinition="The item that is suspected to have increased the probability or severity of the adverse event." ) 1027 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-contributing-factor") 1028 protected DataType item; 1029 1030 private static final long serialVersionUID = 1847936859L; 1031 1032 /** 1033 * Constructor 1034 */ 1035 public AdverseEventContributingFactorComponent() { 1036 super(); 1037 } 1038 1039 /** 1040 * Constructor 1041 */ 1042 public AdverseEventContributingFactorComponent(DataType item) { 1043 super(); 1044 this.setItem(item); 1045 } 1046 1047 /** 1048 * @return {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1049 */ 1050 public DataType getItem() { 1051 return this.item; 1052 } 1053 1054 /** 1055 * @return {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1056 */ 1057 public Reference getItemReference() throws FHIRException { 1058 if (this.item == null) 1059 this.item = new Reference(); 1060 if (!(this.item instanceof Reference)) 1061 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1062 return (Reference) this.item; 1063 } 1064 1065 public boolean hasItemReference() { 1066 return this != null && this.item instanceof Reference; 1067 } 1068 1069 /** 1070 * @return {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1071 */ 1072 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1073 if (this.item == null) 1074 this.item = new CodeableConcept(); 1075 if (!(this.item instanceof CodeableConcept)) 1076 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1077 return (CodeableConcept) this.item; 1078 } 1079 1080 public boolean hasItemCodeableConcept() { 1081 return this != null && this.item instanceof CodeableConcept; 1082 } 1083 1084 public boolean hasItem() { 1085 return this.item != null && !this.item.isEmpty(); 1086 } 1087 1088 /** 1089 * @param value {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1090 */ 1091 public AdverseEventContributingFactorComponent setItem(DataType value) { 1092 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1093 throw new FHIRException("Not the right type for AdverseEvent.contributingFactor.item[x]: "+value.fhirType()); 1094 this.item = value; 1095 return this; 1096 } 1097 1098 protected void listChildren(List<Property> children) { 1099 super.listChildren(children); 1100 children.add(new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)|CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item)); 1101 } 1102 1103 @Override 1104 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1105 switch (_hash) { 1106 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)|CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1107 case 3242771: /*item*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)|CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1108 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1109 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1110 default: return super.getNamedProperty(_hash, _name, _checkValid); 1111 } 1112 1113 } 1114 1115 @Override 1116 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1117 switch (hash) { 1118 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1119 default: return super.getProperty(hash, name, checkValid); 1120 } 1121 1122 } 1123 1124 @Override 1125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1126 switch (hash) { 1127 case 3242771: // item 1128 this.item = TypeConvertor.castToType(value); // DataType 1129 return value; 1130 default: return super.setProperty(hash, name, value); 1131 } 1132 1133 } 1134 1135 @Override 1136 public Base setProperty(String name, Base value) throws FHIRException { 1137 if (name.equals("item[x]")) { 1138 this.item = TypeConvertor.castToType(value); // DataType 1139 } else 1140 return super.setProperty(name, value); 1141 return value; 1142 } 1143 1144 @Override 1145 public void removeChild(String name, Base value) throws FHIRException { 1146 if (name.equals("item[x]")) { 1147 this.item = null; 1148 } else 1149 super.removeChild(name, value); 1150 1151 } 1152 1153 @Override 1154 public Base makeProperty(int hash, String name) throws FHIRException { 1155 switch (hash) { 1156 case 2116201613: return getItem(); 1157 case 3242771: return getItem(); 1158 default: return super.makeProperty(hash, name); 1159 } 1160 1161 } 1162 1163 @Override 1164 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1165 switch (hash) { 1166 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1167 default: return super.getTypesForProperty(hash, name); 1168 } 1169 1170 } 1171 1172 @Override 1173 public Base addChild(String name) throws FHIRException { 1174 if (name.equals("itemReference")) { 1175 this.item = new Reference(); 1176 return this.item; 1177 } 1178 else if (name.equals("itemCodeableConcept")) { 1179 this.item = new CodeableConcept(); 1180 return this.item; 1181 } 1182 else 1183 return super.addChild(name); 1184 } 1185 1186 public AdverseEventContributingFactorComponent copy() { 1187 AdverseEventContributingFactorComponent dst = new AdverseEventContributingFactorComponent(); 1188 copyValues(dst); 1189 return dst; 1190 } 1191 1192 public void copyValues(AdverseEventContributingFactorComponent dst) { 1193 super.copyValues(dst); 1194 dst.item = item == null ? null : item.copy(); 1195 } 1196 1197 @Override 1198 public boolean equalsDeep(Base other_) { 1199 if (!super.equalsDeep(other_)) 1200 return false; 1201 if (!(other_ instanceof AdverseEventContributingFactorComponent)) 1202 return false; 1203 AdverseEventContributingFactorComponent o = (AdverseEventContributingFactorComponent) other_; 1204 return compareDeep(item, o.item, true); 1205 } 1206 1207 @Override 1208 public boolean equalsShallow(Base other_) { 1209 if (!super.equalsShallow(other_)) 1210 return false; 1211 if (!(other_ instanceof AdverseEventContributingFactorComponent)) 1212 return false; 1213 AdverseEventContributingFactorComponent o = (AdverseEventContributingFactorComponent) other_; 1214 return true; 1215 } 1216 1217 public boolean isEmpty() { 1218 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1219 } 1220 1221 public String fhirType() { 1222 return "AdverseEvent.contributingFactor"; 1223 1224 } 1225 1226 } 1227 1228 @Block() 1229 public static class AdverseEventPreventiveActionComponent extends BackboneElement implements IBaseBackboneElement { 1230 /** 1231 * The action that contributed to avoiding the adverse event. 1232 */ 1233 @Child(name = "item", type = {Immunization.class, Procedure.class, DocumentReference.class, MedicationAdministration.class, MedicationRequest.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1234 @Description(shortDefinition="Action that contributed to avoiding the adverse event", formalDefinition="The action that contributed to avoiding the adverse event." ) 1235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-preventive-action") 1236 protected DataType item; 1237 1238 private static final long serialVersionUID = 1847936859L; 1239 1240 /** 1241 * Constructor 1242 */ 1243 public AdverseEventPreventiveActionComponent() { 1244 super(); 1245 } 1246 1247 /** 1248 * Constructor 1249 */ 1250 public AdverseEventPreventiveActionComponent(DataType item) { 1251 super(); 1252 this.setItem(item); 1253 } 1254 1255 /** 1256 * @return {@link #item} (The action that contributed to avoiding the adverse event.) 1257 */ 1258 public DataType getItem() { 1259 return this.item; 1260 } 1261 1262 /** 1263 * @return {@link #item} (The action that contributed to avoiding the adverse event.) 1264 */ 1265 public Reference getItemReference() throws FHIRException { 1266 if (this.item == null) 1267 this.item = new Reference(); 1268 if (!(this.item instanceof Reference)) 1269 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1270 return (Reference) this.item; 1271 } 1272 1273 public boolean hasItemReference() { 1274 return this != null && this.item instanceof Reference; 1275 } 1276 1277 /** 1278 * @return {@link #item} (The action that contributed to avoiding the adverse event.) 1279 */ 1280 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1281 if (this.item == null) 1282 this.item = new CodeableConcept(); 1283 if (!(this.item instanceof CodeableConcept)) 1284 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1285 return (CodeableConcept) this.item; 1286 } 1287 1288 public boolean hasItemCodeableConcept() { 1289 return this != null && this.item instanceof CodeableConcept; 1290 } 1291 1292 public boolean hasItem() { 1293 return this.item != null && !this.item.isEmpty(); 1294 } 1295 1296 /** 1297 * @param value {@link #item} (The action that contributed to avoiding the adverse event.) 1298 */ 1299 public AdverseEventPreventiveActionComponent setItem(DataType value) { 1300 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1301 throw new FHIRException("Not the right type for AdverseEvent.preventiveAction.item[x]: "+value.fhirType()); 1302 this.item = value; 1303 return this; 1304 } 1305 1306 protected void listChildren(List<Property> children) { 1307 super.listChildren(children); 1308 children.add(new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item)); 1309 } 1310 1311 @Override 1312 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1313 switch (_hash) { 1314 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1315 case 3242771: /*item*/ return new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1316 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1317 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1318 default: return super.getNamedProperty(_hash, _name, _checkValid); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1325 switch (hash) { 1326 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1327 default: return super.getProperty(hash, name, checkValid); 1328 } 1329 1330 } 1331 1332 @Override 1333 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1334 switch (hash) { 1335 case 3242771: // item 1336 this.item = TypeConvertor.castToType(value); // DataType 1337 return value; 1338 default: return super.setProperty(hash, name, value); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base setProperty(String name, Base value) throws FHIRException { 1345 if (name.equals("item[x]")) { 1346 this.item = TypeConvertor.castToType(value); // DataType 1347 } else 1348 return super.setProperty(name, value); 1349 return value; 1350 } 1351 1352 @Override 1353 public void removeChild(String name, Base value) throws FHIRException { 1354 if (name.equals("item[x]")) { 1355 this.item = null; 1356 } else 1357 super.removeChild(name, value); 1358 1359 } 1360 1361 @Override 1362 public Base makeProperty(int hash, String name) throws FHIRException { 1363 switch (hash) { 1364 case 2116201613: return getItem(); 1365 case 3242771: return getItem(); 1366 default: return super.makeProperty(hash, name); 1367 } 1368 1369 } 1370 1371 @Override 1372 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1373 switch (hash) { 1374 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1375 default: return super.getTypesForProperty(hash, name); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base addChild(String name) throws FHIRException { 1382 if (name.equals("itemReference")) { 1383 this.item = new Reference(); 1384 return this.item; 1385 } 1386 else if (name.equals("itemCodeableConcept")) { 1387 this.item = new CodeableConcept(); 1388 return this.item; 1389 } 1390 else 1391 return super.addChild(name); 1392 } 1393 1394 public AdverseEventPreventiveActionComponent copy() { 1395 AdverseEventPreventiveActionComponent dst = new AdverseEventPreventiveActionComponent(); 1396 copyValues(dst); 1397 return dst; 1398 } 1399 1400 public void copyValues(AdverseEventPreventiveActionComponent dst) { 1401 super.copyValues(dst); 1402 dst.item = item == null ? null : item.copy(); 1403 } 1404 1405 @Override 1406 public boolean equalsDeep(Base other_) { 1407 if (!super.equalsDeep(other_)) 1408 return false; 1409 if (!(other_ instanceof AdverseEventPreventiveActionComponent)) 1410 return false; 1411 AdverseEventPreventiveActionComponent o = (AdverseEventPreventiveActionComponent) other_; 1412 return compareDeep(item, o.item, true); 1413 } 1414 1415 @Override 1416 public boolean equalsShallow(Base other_) { 1417 if (!super.equalsShallow(other_)) 1418 return false; 1419 if (!(other_ instanceof AdverseEventPreventiveActionComponent)) 1420 return false; 1421 AdverseEventPreventiveActionComponent o = (AdverseEventPreventiveActionComponent) other_; 1422 return true; 1423 } 1424 1425 public boolean isEmpty() { 1426 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1427 } 1428 1429 public String fhirType() { 1430 return "AdverseEvent.preventiveAction"; 1431 1432 } 1433 1434 } 1435 1436 @Block() 1437 public static class AdverseEventMitigatingActionComponent extends BackboneElement implements IBaseBackboneElement { 1438 /** 1439 * The ameliorating action taken after the adverse event occured in order to reduce the extent of harm. 1440 */ 1441 @Child(name = "item", type = {Procedure.class, DocumentReference.class, MedicationAdministration.class, MedicationRequest.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1442 @Description(shortDefinition="Ameliorating action taken after the adverse event occured in order to reduce the extent of harm", formalDefinition="The ameliorating action taken after the adverse event occured in order to reduce the extent of harm." ) 1443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-mitigating-action") 1444 protected DataType item; 1445 1446 private static final long serialVersionUID = 1847936859L; 1447 1448 /** 1449 * Constructor 1450 */ 1451 public AdverseEventMitigatingActionComponent() { 1452 super(); 1453 } 1454 1455 /** 1456 * Constructor 1457 */ 1458 public AdverseEventMitigatingActionComponent(DataType item) { 1459 super(); 1460 this.setItem(item); 1461 } 1462 1463 /** 1464 * @return {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1465 */ 1466 public DataType getItem() { 1467 return this.item; 1468 } 1469 1470 /** 1471 * @return {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1472 */ 1473 public Reference getItemReference() throws FHIRException { 1474 if (this.item == null) 1475 this.item = new Reference(); 1476 if (!(this.item instanceof Reference)) 1477 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1478 return (Reference) this.item; 1479 } 1480 1481 public boolean hasItemReference() { 1482 return this != null && this.item instanceof Reference; 1483 } 1484 1485 /** 1486 * @return {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1487 */ 1488 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1489 if (this.item == null) 1490 this.item = new CodeableConcept(); 1491 if (!(this.item instanceof CodeableConcept)) 1492 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1493 return (CodeableConcept) this.item; 1494 } 1495 1496 public boolean hasItemCodeableConcept() { 1497 return this != null && this.item instanceof CodeableConcept; 1498 } 1499 1500 public boolean hasItem() { 1501 return this.item != null && !this.item.isEmpty(); 1502 } 1503 1504 /** 1505 * @param value {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1506 */ 1507 public AdverseEventMitigatingActionComponent setItem(DataType value) { 1508 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1509 throw new FHIRException("Not the right type for AdverseEvent.mitigatingAction.item[x]: "+value.fhirType()); 1510 this.item = value; 1511 return this; 1512 } 1513 1514 protected void listChildren(List<Property> children) { 1515 super.listChildren(children); 1516 children.add(new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item)); 1517 } 1518 1519 @Override 1520 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1521 switch (_hash) { 1522 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1523 case 3242771: /*item*/ return new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1524 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1525 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1526 default: return super.getNamedProperty(_hash, _name, _checkValid); 1527 } 1528 1529 } 1530 1531 @Override 1532 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1533 switch (hash) { 1534 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1535 default: return super.getProperty(hash, name, checkValid); 1536 } 1537 1538 } 1539 1540 @Override 1541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1542 switch (hash) { 1543 case 3242771: // item 1544 this.item = TypeConvertor.castToType(value); // DataType 1545 return value; 1546 default: return super.setProperty(hash, name, value); 1547 } 1548 1549 } 1550 1551 @Override 1552 public Base setProperty(String name, Base value) throws FHIRException { 1553 if (name.equals("item[x]")) { 1554 this.item = TypeConvertor.castToType(value); // DataType 1555 } else 1556 return super.setProperty(name, value); 1557 return value; 1558 } 1559 1560 @Override 1561 public void removeChild(String name, Base value) throws FHIRException { 1562 if (name.equals("item[x]")) { 1563 this.item = null; 1564 } else 1565 super.removeChild(name, value); 1566 1567 } 1568 1569 @Override 1570 public Base makeProperty(int hash, String name) throws FHIRException { 1571 switch (hash) { 1572 case 2116201613: return getItem(); 1573 case 3242771: return getItem(); 1574 default: return super.makeProperty(hash, name); 1575 } 1576 1577 } 1578 1579 @Override 1580 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1581 switch (hash) { 1582 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1583 default: return super.getTypesForProperty(hash, name); 1584 } 1585 1586 } 1587 1588 @Override 1589 public Base addChild(String name) throws FHIRException { 1590 if (name.equals("itemReference")) { 1591 this.item = new Reference(); 1592 return this.item; 1593 } 1594 else if (name.equals("itemCodeableConcept")) { 1595 this.item = new CodeableConcept(); 1596 return this.item; 1597 } 1598 else 1599 return super.addChild(name); 1600 } 1601 1602 public AdverseEventMitigatingActionComponent copy() { 1603 AdverseEventMitigatingActionComponent dst = new AdverseEventMitigatingActionComponent(); 1604 copyValues(dst); 1605 return dst; 1606 } 1607 1608 public void copyValues(AdverseEventMitigatingActionComponent dst) { 1609 super.copyValues(dst); 1610 dst.item = item == null ? null : item.copy(); 1611 } 1612 1613 @Override 1614 public boolean equalsDeep(Base other_) { 1615 if (!super.equalsDeep(other_)) 1616 return false; 1617 if (!(other_ instanceof AdverseEventMitigatingActionComponent)) 1618 return false; 1619 AdverseEventMitigatingActionComponent o = (AdverseEventMitigatingActionComponent) other_; 1620 return compareDeep(item, o.item, true); 1621 } 1622 1623 @Override 1624 public boolean equalsShallow(Base other_) { 1625 if (!super.equalsShallow(other_)) 1626 return false; 1627 if (!(other_ instanceof AdverseEventMitigatingActionComponent)) 1628 return false; 1629 AdverseEventMitigatingActionComponent o = (AdverseEventMitigatingActionComponent) other_; 1630 return true; 1631 } 1632 1633 public boolean isEmpty() { 1634 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1635 } 1636 1637 public String fhirType() { 1638 return "AdverseEvent.mitigatingAction"; 1639 1640 } 1641 1642 } 1643 1644 @Block() 1645 public static class AdverseEventSupportingInfoComponent extends BackboneElement implements IBaseBackboneElement { 1646 /** 1647 * Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action. 1648 */ 1649 @Child(name = "item", type = {Condition.class, Observation.class, AllergyIntolerance.class, FamilyMemberHistory.class, Immunization.class, Procedure.class, DocumentReference.class, MedicationAdministration.class, MedicationStatement.class, QuestionnaireResponse.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1650 @Description(shortDefinition="Subject medical history or document relevant to this adverse event", formalDefinition="Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action." ) 1651 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-supporting-info") 1652 protected DataType item; 1653 1654 private static final long serialVersionUID = 1847936859L; 1655 1656 /** 1657 * Constructor 1658 */ 1659 public AdverseEventSupportingInfoComponent() { 1660 super(); 1661 } 1662 1663 /** 1664 * Constructor 1665 */ 1666 public AdverseEventSupportingInfoComponent(DataType item) { 1667 super(); 1668 this.setItem(item); 1669 } 1670 1671 /** 1672 * @return {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1673 */ 1674 public DataType getItem() { 1675 return this.item; 1676 } 1677 1678 /** 1679 * @return {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1680 */ 1681 public Reference getItemReference() throws FHIRException { 1682 if (this.item == null) 1683 this.item = new Reference(); 1684 if (!(this.item instanceof Reference)) 1685 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1686 return (Reference) this.item; 1687 } 1688 1689 public boolean hasItemReference() { 1690 return this != null && this.item instanceof Reference; 1691 } 1692 1693 /** 1694 * @return {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1695 */ 1696 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1697 if (this.item == null) 1698 this.item = new CodeableConcept(); 1699 if (!(this.item instanceof CodeableConcept)) 1700 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1701 return (CodeableConcept) this.item; 1702 } 1703 1704 public boolean hasItemCodeableConcept() { 1705 return this != null && this.item instanceof CodeableConcept; 1706 } 1707 1708 public boolean hasItem() { 1709 return this.item != null && !this.item.isEmpty(); 1710 } 1711 1712 /** 1713 * @param value {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1714 */ 1715 public AdverseEventSupportingInfoComponent setItem(DataType value) { 1716 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1717 throw new FHIRException("Not the right type for AdverseEvent.supportingInfo.item[x]: "+value.fhirType()); 1718 this.item = value; 1719 return this; 1720 } 1721 1722 protected void listChildren(List<Property> children) { 1723 super.listChildren(children); 1724 children.add(new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)|CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item)); 1725 } 1726 1727 @Override 1728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1729 switch (_hash) { 1730 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)|CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1731 case 3242771: /*item*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)|CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1732 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1733 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1734 default: return super.getNamedProperty(_hash, _name, _checkValid); 1735 } 1736 1737 } 1738 1739 @Override 1740 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1741 switch (hash) { 1742 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1743 default: return super.getProperty(hash, name, checkValid); 1744 } 1745 1746 } 1747 1748 @Override 1749 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1750 switch (hash) { 1751 case 3242771: // item 1752 this.item = TypeConvertor.castToType(value); // DataType 1753 return value; 1754 default: return super.setProperty(hash, name, value); 1755 } 1756 1757 } 1758 1759 @Override 1760 public Base setProperty(String name, Base value) throws FHIRException { 1761 if (name.equals("item[x]")) { 1762 this.item = TypeConvertor.castToType(value); // DataType 1763 } else 1764 return super.setProperty(name, value); 1765 return value; 1766 } 1767 1768 @Override 1769 public void removeChild(String name, Base value) throws FHIRException { 1770 if (name.equals("item[x]")) { 1771 this.item = null; 1772 } else 1773 super.removeChild(name, value); 1774 1775 } 1776 1777 @Override 1778 public Base makeProperty(int hash, String name) throws FHIRException { 1779 switch (hash) { 1780 case 2116201613: return getItem(); 1781 case 3242771: return getItem(); 1782 default: return super.makeProperty(hash, name); 1783 } 1784 1785 } 1786 1787 @Override 1788 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1789 switch (hash) { 1790 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1791 default: return super.getTypesForProperty(hash, name); 1792 } 1793 1794 } 1795 1796 @Override 1797 public Base addChild(String name) throws FHIRException { 1798 if (name.equals("itemReference")) { 1799 this.item = new Reference(); 1800 return this.item; 1801 } 1802 else if (name.equals("itemCodeableConcept")) { 1803 this.item = new CodeableConcept(); 1804 return this.item; 1805 } 1806 else 1807 return super.addChild(name); 1808 } 1809 1810 public AdverseEventSupportingInfoComponent copy() { 1811 AdverseEventSupportingInfoComponent dst = new AdverseEventSupportingInfoComponent(); 1812 copyValues(dst); 1813 return dst; 1814 } 1815 1816 public void copyValues(AdverseEventSupportingInfoComponent dst) { 1817 super.copyValues(dst); 1818 dst.item = item == null ? null : item.copy(); 1819 } 1820 1821 @Override 1822 public boolean equalsDeep(Base other_) { 1823 if (!super.equalsDeep(other_)) 1824 return false; 1825 if (!(other_ instanceof AdverseEventSupportingInfoComponent)) 1826 return false; 1827 AdverseEventSupportingInfoComponent o = (AdverseEventSupportingInfoComponent) other_; 1828 return compareDeep(item, o.item, true); 1829 } 1830 1831 @Override 1832 public boolean equalsShallow(Base other_) { 1833 if (!super.equalsShallow(other_)) 1834 return false; 1835 if (!(other_ instanceof AdverseEventSupportingInfoComponent)) 1836 return false; 1837 AdverseEventSupportingInfoComponent o = (AdverseEventSupportingInfoComponent) other_; 1838 return true; 1839 } 1840 1841 public boolean isEmpty() { 1842 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1843 } 1844 1845 public String fhirType() { 1846 return "AdverseEvent.supportingInfo"; 1847 1848 } 1849 1850 } 1851 1852 /** 1853 * Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 1854 */ 1855 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1856 @Description(shortDefinition="Business identifier for the event", formalDefinition="Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 1857 protected List<Identifier> identifier; 1858 1859 /** 1860 * The current state of the adverse event or potential adverse event. 1861 */ 1862 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1863 @Description(shortDefinition="in-progress | completed | entered-in-error | unknown", formalDefinition="The current state of the adverse event or potential adverse event." ) 1864 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-status") 1865 protected Enumeration<AdverseEventStatus> status; 1866 1867 /** 1868 * Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely. 1869 */ 1870 @Child(name = "actuality", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1871 @Description(shortDefinition="actual | potential", formalDefinition="Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely." ) 1872 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-actuality") 1873 protected Enumeration<AdverseEventActuality> actuality; 1874 1875 /** 1876 * The overall type of event, intended for search and filtering purposes. 1877 */ 1878 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1879 @Description(shortDefinition="wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site", formalDefinition="The overall type of event, intended for search and filtering purposes." ) 1880 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-category") 1881 protected List<CodeableConcept> category; 1882 1883 /** 1884 * Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused. 1885 */ 1886 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1887 @Description(shortDefinition="Event or incident that occurred or was averted", formalDefinition="Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused." ) 1888 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-type") 1889 protected CodeableConcept code; 1890 1891 /** 1892 * This subject or group impacted by the event. 1893 */ 1894 @Child(name = "subject", type = {Patient.class, Group.class, Practitioner.class, RelatedPerson.class, ResearchSubject.class}, order=5, min=1, max=1, modifier=false, summary=true) 1895 @Description(shortDefinition="Subject impacted by event", formalDefinition="This subject or group impacted by the event." ) 1896 protected Reference subject; 1897 1898 /** 1899 * The Encounter associated with the start of the AdverseEvent. 1900 */ 1901 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 1902 @Description(shortDefinition="The Encounter associated with the start of the AdverseEvent", formalDefinition="The Encounter associated with the start of the AdverseEvent." ) 1903 protected Reference encounter; 1904 1905 /** 1906 * The date (and perhaps time) when the adverse event occurred. 1907 */ 1908 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 1909 @Description(shortDefinition="When the event occurred", formalDefinition="The date (and perhaps time) when the adverse event occurred." ) 1910 protected DataType occurrence; 1911 1912 /** 1913 * Estimated or actual date the AdverseEvent began, in the opinion of the reporter. 1914 */ 1915 @Child(name = "detected", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1916 @Description(shortDefinition="When the event was detected", formalDefinition="Estimated or actual date the AdverseEvent began, in the opinion of the reporter." ) 1917 protected DateTimeType detected; 1918 1919 /** 1920 * The date on which the existence of the AdverseEvent was first recorded. 1921 */ 1922 @Child(name = "recordedDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1923 @Description(shortDefinition="When the event was recorded", formalDefinition="The date on which the existence of the AdverseEvent was first recorded." ) 1924 protected DateTimeType recordedDate; 1925 1926 /** 1927 * Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall. 1928 */ 1929 @Child(name = "resultingEffect", type = {Condition.class, Observation.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1930 @Description(shortDefinition="Effect on the subject due to this event", formalDefinition="Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall." ) 1931 protected List<Reference> resultingEffect; 1932 1933 /** 1934 * The information about where the adverse event occurred. 1935 */ 1936 @Child(name = "location", type = {Location.class}, order=11, min=0, max=1, modifier=false, summary=true) 1937 @Description(shortDefinition="Location where adverse event occurred", formalDefinition="The information about where the adverse event occurred." ) 1938 protected Reference location; 1939 1940 /** 1941 * Assessment whether this event, or averted event, was of clinical importance. 1942 */ 1943 @Child(name = "seriousness", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 1944 @Description(shortDefinition="Seriousness or gravity of the event", formalDefinition="Assessment whether this event, or averted event, was of clinical importance." ) 1945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-seriousness") 1946 protected CodeableConcept seriousness; 1947 1948 /** 1949 * Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal. 1950 */ 1951 @Child(name = "outcome", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1952 @Description(shortDefinition="Type of outcome from the adverse event", formalDefinition="Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal." ) 1953 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-outcome") 1954 protected List<CodeableConcept> outcome; 1955 1956 /** 1957 * Information on who recorded the adverse event. May be the patient or a practitioner. 1958 */ 1959 @Child(name = "recorder", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, ResearchSubject.class}, order=14, min=0, max=1, modifier=false, summary=true) 1960 @Description(shortDefinition="Who recorded the adverse event", formalDefinition="Information on who recorded the adverse event. May be the patient or a practitioner." ) 1961 protected Reference recorder; 1962 1963 /** 1964 * Indicates who or what participated in the adverse event and how they were involved. 1965 */ 1966 @Child(name = "participant", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1967 @Description(shortDefinition="Who was involved in the adverse event or the potential adverse event and what they did", formalDefinition="Indicates who or what participated in the adverse event and how they were involved." ) 1968 protected List<AdverseEventParticipantComponent> participant; 1969 1970 /** 1971 * The research study that the subject is enrolled in. 1972 */ 1973 @Child(name = "study", type = {ResearchStudy.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1974 @Description(shortDefinition="Research study that the subject is enrolled in", formalDefinition="The research study that the subject is enrolled in." ) 1975 protected List<Reference> study; 1976 1977 /** 1978 * Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely. 1979 */ 1980 @Child(name = "expectedInResearchStudy", type = {BooleanType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1981 @Description(shortDefinition="Considered likely or probable or anticipated in the research study", formalDefinition="Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely." ) 1982 protected BooleanType expectedInResearchStudy; 1983 1984 /** 1985 * Describes the entity that is suspected to have caused the adverse event. 1986 */ 1987 @Child(name = "suspectEntity", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1988 @Description(shortDefinition="The suspected agent causing the adverse event", formalDefinition="Describes the entity that is suspected to have caused the adverse event." ) 1989 protected List<AdverseEventSuspectEntityComponent> suspectEntity; 1990 1991 /** 1992 * The contributing factors suspected to have increased the probability or severity of the adverse event. 1993 */ 1994 @Child(name = "contributingFactor", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1995 @Description(shortDefinition="Contributing factors suspected to have increased the probability or severity of the adverse event", formalDefinition="The contributing factors suspected to have increased the probability or severity of the adverse event." ) 1996 protected List<AdverseEventContributingFactorComponent> contributingFactor; 1997 1998 /** 1999 * Preventive actions that contributed to avoiding the adverse event. 2000 */ 2001 @Child(name = "preventiveAction", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2002 @Description(shortDefinition="Preventive actions that contributed to avoiding the adverse event", formalDefinition="Preventive actions that contributed to avoiding the adverse event." ) 2003 protected List<AdverseEventPreventiveActionComponent> preventiveAction; 2004 2005 /** 2006 * The ameliorating action taken after the adverse event occured in order to reduce the extent of harm. 2007 */ 2008 @Child(name = "mitigatingAction", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2009 @Description(shortDefinition="Ameliorating actions taken after the adverse event occured in order to reduce the extent of harm", formalDefinition="The ameliorating action taken after the adverse event occured in order to reduce the extent of harm." ) 2010 protected List<AdverseEventMitigatingActionComponent> mitigatingAction; 2011 2012 /** 2013 * Supporting information relevant to the event. 2014 */ 2015 @Child(name = "supportingInfo", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2016 @Description(shortDefinition="Supporting information relevant to the event", formalDefinition="Supporting information relevant to the event." ) 2017 protected List<AdverseEventSupportingInfoComponent> supportingInfo; 2018 2019 /** 2020 * Comments made about the adverse event by the performer, subject or other participants. 2021 */ 2022 @Child(name = "note", type = {Annotation.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2023 @Description(shortDefinition="Comment on adverse event", formalDefinition="Comments made about the adverse event by the performer, subject or other participants." ) 2024 protected List<Annotation> note; 2025 2026 private static final long serialVersionUID = -1861590732L; 2027 2028 /** 2029 * Constructor 2030 */ 2031 public AdverseEvent() { 2032 super(); 2033 } 2034 2035 /** 2036 * Constructor 2037 */ 2038 public AdverseEvent(AdverseEventStatus status, AdverseEventActuality actuality, Reference subject) { 2039 super(); 2040 this.setStatus(status); 2041 this.setActuality(actuality); 2042 this.setSubject(subject); 2043 } 2044 2045 /** 2046 * @return {@link #identifier} (Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 2047 */ 2048 public List<Identifier> getIdentifier() { 2049 if (this.identifier == null) 2050 this.identifier = new ArrayList<Identifier>(); 2051 return this.identifier; 2052 } 2053 2054 /** 2055 * @return Returns a reference to <code>this</code> for easy method chaining 2056 */ 2057 public AdverseEvent setIdentifier(List<Identifier> theIdentifier) { 2058 this.identifier = theIdentifier; 2059 return this; 2060 } 2061 2062 public boolean hasIdentifier() { 2063 if (this.identifier == null) 2064 return false; 2065 for (Identifier item : this.identifier) 2066 if (!item.isEmpty()) 2067 return true; 2068 return false; 2069 } 2070 2071 public Identifier addIdentifier() { //3 2072 Identifier t = new Identifier(); 2073 if (this.identifier == null) 2074 this.identifier = new ArrayList<Identifier>(); 2075 this.identifier.add(t); 2076 return t; 2077 } 2078 2079 public AdverseEvent addIdentifier(Identifier t) { //3 2080 if (t == null) 2081 return this; 2082 if (this.identifier == null) 2083 this.identifier = new ArrayList<Identifier>(); 2084 this.identifier.add(t); 2085 return this; 2086 } 2087 2088 /** 2089 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2090 */ 2091 public Identifier getIdentifierFirstRep() { 2092 if (getIdentifier().isEmpty()) { 2093 addIdentifier(); 2094 } 2095 return getIdentifier().get(0); 2096 } 2097 2098 /** 2099 * @return {@link #status} (The current state of the adverse event or potential adverse event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2100 */ 2101 public Enumeration<AdverseEventStatus> getStatusElement() { 2102 if (this.status == null) 2103 if (Configuration.errorOnAutoCreate()) 2104 throw new Error("Attempt to auto-create AdverseEvent.status"); 2105 else if (Configuration.doAutoCreate()) 2106 this.status = new Enumeration<AdverseEventStatus>(new AdverseEventStatusEnumFactory()); // bb 2107 return this.status; 2108 } 2109 2110 public boolean hasStatusElement() { 2111 return this.status != null && !this.status.isEmpty(); 2112 } 2113 2114 public boolean hasStatus() { 2115 return this.status != null && !this.status.isEmpty(); 2116 } 2117 2118 /** 2119 * @param value {@link #status} (The current state of the adverse event or potential adverse event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2120 */ 2121 public AdverseEvent setStatusElement(Enumeration<AdverseEventStatus> value) { 2122 this.status = value; 2123 return this; 2124 } 2125 2126 /** 2127 * @return The current state of the adverse event or potential adverse event. 2128 */ 2129 public AdverseEventStatus getStatus() { 2130 return this.status == null ? null : this.status.getValue(); 2131 } 2132 2133 /** 2134 * @param value The current state of the adverse event or potential adverse event. 2135 */ 2136 public AdverseEvent setStatus(AdverseEventStatus value) { 2137 if (this.status == null) 2138 this.status = new Enumeration<AdverseEventStatus>(new AdverseEventStatusEnumFactory()); 2139 this.status.setValue(value); 2140 return this; 2141 } 2142 2143 /** 2144 * @return {@link #actuality} (Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.). This is the underlying object with id, value and extensions. The accessor "getActuality" gives direct access to the value 2145 */ 2146 public Enumeration<AdverseEventActuality> getActualityElement() { 2147 if (this.actuality == null) 2148 if (Configuration.errorOnAutoCreate()) 2149 throw new Error("Attempt to auto-create AdverseEvent.actuality"); 2150 else if (Configuration.doAutoCreate()) 2151 this.actuality = new Enumeration<AdverseEventActuality>(new AdverseEventActualityEnumFactory()); // bb 2152 return this.actuality; 2153 } 2154 2155 public boolean hasActualityElement() { 2156 return this.actuality != null && !this.actuality.isEmpty(); 2157 } 2158 2159 public boolean hasActuality() { 2160 return this.actuality != null && !this.actuality.isEmpty(); 2161 } 2162 2163 /** 2164 * @param value {@link #actuality} (Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.). This is the underlying object with id, value and extensions. The accessor "getActuality" gives direct access to the value 2165 */ 2166 public AdverseEvent setActualityElement(Enumeration<AdverseEventActuality> value) { 2167 this.actuality = value; 2168 return this; 2169 } 2170 2171 /** 2172 * @return Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely. 2173 */ 2174 public AdverseEventActuality getActuality() { 2175 return this.actuality == null ? null : this.actuality.getValue(); 2176 } 2177 2178 /** 2179 * @param value Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely. 2180 */ 2181 public AdverseEvent setActuality(AdverseEventActuality value) { 2182 if (this.actuality == null) 2183 this.actuality = new Enumeration<AdverseEventActuality>(new AdverseEventActualityEnumFactory()); 2184 this.actuality.setValue(value); 2185 return this; 2186 } 2187 2188 /** 2189 * @return {@link #category} (The overall type of event, intended for search and filtering purposes.) 2190 */ 2191 public List<CodeableConcept> getCategory() { 2192 if (this.category == null) 2193 this.category = new ArrayList<CodeableConcept>(); 2194 return this.category; 2195 } 2196 2197 /** 2198 * @return Returns a reference to <code>this</code> for easy method chaining 2199 */ 2200 public AdverseEvent setCategory(List<CodeableConcept> theCategory) { 2201 this.category = theCategory; 2202 return this; 2203 } 2204 2205 public boolean hasCategory() { 2206 if (this.category == null) 2207 return false; 2208 for (CodeableConcept item : this.category) 2209 if (!item.isEmpty()) 2210 return true; 2211 return false; 2212 } 2213 2214 public CodeableConcept addCategory() { //3 2215 CodeableConcept t = new CodeableConcept(); 2216 if (this.category == null) 2217 this.category = new ArrayList<CodeableConcept>(); 2218 this.category.add(t); 2219 return t; 2220 } 2221 2222 public AdverseEvent addCategory(CodeableConcept t) { //3 2223 if (t == null) 2224 return this; 2225 if (this.category == null) 2226 this.category = new ArrayList<CodeableConcept>(); 2227 this.category.add(t); 2228 return this; 2229 } 2230 2231 /** 2232 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2233 */ 2234 public CodeableConcept getCategoryFirstRep() { 2235 if (getCategory().isEmpty()) { 2236 addCategory(); 2237 } 2238 return getCategory().get(0); 2239 } 2240 2241 /** 2242 * @return {@link #code} (Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.) 2243 */ 2244 public CodeableConcept getCode() { 2245 if (this.code == null) 2246 if (Configuration.errorOnAutoCreate()) 2247 throw new Error("Attempt to auto-create AdverseEvent.code"); 2248 else if (Configuration.doAutoCreate()) 2249 this.code = new CodeableConcept(); // cc 2250 return this.code; 2251 } 2252 2253 public boolean hasCode() { 2254 return this.code != null && !this.code.isEmpty(); 2255 } 2256 2257 /** 2258 * @param value {@link #code} (Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.) 2259 */ 2260 public AdverseEvent setCode(CodeableConcept value) { 2261 this.code = value; 2262 return this; 2263 } 2264 2265 /** 2266 * @return {@link #subject} (This subject or group impacted by the event.) 2267 */ 2268 public Reference getSubject() { 2269 if (this.subject == null) 2270 if (Configuration.errorOnAutoCreate()) 2271 throw new Error("Attempt to auto-create AdverseEvent.subject"); 2272 else if (Configuration.doAutoCreate()) 2273 this.subject = new Reference(); // cc 2274 return this.subject; 2275 } 2276 2277 public boolean hasSubject() { 2278 return this.subject != null && !this.subject.isEmpty(); 2279 } 2280 2281 /** 2282 * @param value {@link #subject} (This subject or group impacted by the event.) 2283 */ 2284 public AdverseEvent setSubject(Reference value) { 2285 this.subject = value; 2286 return this; 2287 } 2288 2289 /** 2290 * @return {@link #encounter} (The Encounter associated with the start of the AdverseEvent.) 2291 */ 2292 public Reference getEncounter() { 2293 if (this.encounter == null) 2294 if (Configuration.errorOnAutoCreate()) 2295 throw new Error("Attempt to auto-create AdverseEvent.encounter"); 2296 else if (Configuration.doAutoCreate()) 2297 this.encounter = new Reference(); // cc 2298 return this.encounter; 2299 } 2300 2301 public boolean hasEncounter() { 2302 return this.encounter != null && !this.encounter.isEmpty(); 2303 } 2304 2305 /** 2306 * @param value {@link #encounter} (The Encounter associated with the start of the AdverseEvent.) 2307 */ 2308 public AdverseEvent setEncounter(Reference value) { 2309 this.encounter = value; 2310 return this; 2311 } 2312 2313 /** 2314 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2315 */ 2316 public DataType getOccurrence() { 2317 return this.occurrence; 2318 } 2319 2320 /** 2321 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2322 */ 2323 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2324 if (this.occurrence == null) 2325 this.occurrence = new DateTimeType(); 2326 if (!(this.occurrence instanceof DateTimeType)) 2327 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2328 return (DateTimeType) this.occurrence; 2329 } 2330 2331 public boolean hasOccurrenceDateTimeType() { 2332 return this != null && this.occurrence instanceof DateTimeType; 2333 } 2334 2335 /** 2336 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2337 */ 2338 public Period getOccurrencePeriod() throws FHIRException { 2339 if (this.occurrence == null) 2340 this.occurrence = new Period(); 2341 if (!(this.occurrence instanceof Period)) 2342 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2343 return (Period) this.occurrence; 2344 } 2345 2346 public boolean hasOccurrencePeriod() { 2347 return this != null && this.occurrence instanceof Period; 2348 } 2349 2350 /** 2351 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2352 */ 2353 public Timing getOccurrenceTiming() throws FHIRException { 2354 if (this.occurrence == null) 2355 this.occurrence = new Timing(); 2356 if (!(this.occurrence instanceof Timing)) 2357 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2358 return (Timing) this.occurrence; 2359 } 2360 2361 public boolean hasOccurrenceTiming() { 2362 return this != null && this.occurrence instanceof Timing; 2363 } 2364 2365 public boolean hasOccurrence() { 2366 return this.occurrence != null && !this.occurrence.isEmpty(); 2367 } 2368 2369 /** 2370 * @param value {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2371 */ 2372 public AdverseEvent setOccurrence(DataType value) { 2373 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 2374 throw new FHIRException("Not the right type for AdverseEvent.occurrence[x]: "+value.fhirType()); 2375 this.occurrence = value; 2376 return this; 2377 } 2378 2379 /** 2380 * @return {@link #detected} (Estimated or actual date the AdverseEvent began, in the opinion of the reporter.). This is the underlying object with id, value and extensions. The accessor "getDetected" gives direct access to the value 2381 */ 2382 public DateTimeType getDetectedElement() { 2383 if (this.detected == null) 2384 if (Configuration.errorOnAutoCreate()) 2385 throw new Error("Attempt to auto-create AdverseEvent.detected"); 2386 else if (Configuration.doAutoCreate()) 2387 this.detected = new DateTimeType(); // bb 2388 return this.detected; 2389 } 2390 2391 public boolean hasDetectedElement() { 2392 return this.detected != null && !this.detected.isEmpty(); 2393 } 2394 2395 public boolean hasDetected() { 2396 return this.detected != null && !this.detected.isEmpty(); 2397 } 2398 2399 /** 2400 * @param value {@link #detected} (Estimated or actual date the AdverseEvent began, in the opinion of the reporter.). This is the underlying object with id, value and extensions. The accessor "getDetected" gives direct access to the value 2401 */ 2402 public AdverseEvent setDetectedElement(DateTimeType value) { 2403 this.detected = value; 2404 return this; 2405 } 2406 2407 /** 2408 * @return Estimated or actual date the AdverseEvent began, in the opinion of the reporter. 2409 */ 2410 public Date getDetected() { 2411 return this.detected == null ? null : this.detected.getValue(); 2412 } 2413 2414 /** 2415 * @param value Estimated or actual date the AdverseEvent began, in the opinion of the reporter. 2416 */ 2417 public AdverseEvent setDetected(Date value) { 2418 if (value == null) 2419 this.detected = null; 2420 else { 2421 if (this.detected == null) 2422 this.detected = new DateTimeType(); 2423 this.detected.setValue(value); 2424 } 2425 return this; 2426 } 2427 2428 /** 2429 * @return {@link #recordedDate} (The date on which the existence of the AdverseEvent was first recorded.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 2430 */ 2431 public DateTimeType getRecordedDateElement() { 2432 if (this.recordedDate == null) 2433 if (Configuration.errorOnAutoCreate()) 2434 throw new Error("Attempt to auto-create AdverseEvent.recordedDate"); 2435 else if (Configuration.doAutoCreate()) 2436 this.recordedDate = new DateTimeType(); // bb 2437 return this.recordedDate; 2438 } 2439 2440 public boolean hasRecordedDateElement() { 2441 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2442 } 2443 2444 public boolean hasRecordedDate() { 2445 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2446 } 2447 2448 /** 2449 * @param value {@link #recordedDate} (The date on which the existence of the AdverseEvent was first recorded.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 2450 */ 2451 public AdverseEvent setRecordedDateElement(DateTimeType value) { 2452 this.recordedDate = value; 2453 return this; 2454 } 2455 2456 /** 2457 * @return The date on which the existence of the AdverseEvent was first recorded. 2458 */ 2459 public Date getRecordedDate() { 2460 return this.recordedDate == null ? null : this.recordedDate.getValue(); 2461 } 2462 2463 /** 2464 * @param value The date on which the existence of the AdverseEvent was first recorded. 2465 */ 2466 public AdverseEvent setRecordedDate(Date value) { 2467 if (value == null) 2468 this.recordedDate = null; 2469 else { 2470 if (this.recordedDate == null) 2471 this.recordedDate = new DateTimeType(); 2472 this.recordedDate.setValue(value); 2473 } 2474 return this; 2475 } 2476 2477 /** 2478 * @return {@link #resultingEffect} (Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall.) 2479 */ 2480 public List<Reference> getResultingEffect() { 2481 if (this.resultingEffect == null) 2482 this.resultingEffect = new ArrayList<Reference>(); 2483 return this.resultingEffect; 2484 } 2485 2486 /** 2487 * @return Returns a reference to <code>this</code> for easy method chaining 2488 */ 2489 public AdverseEvent setResultingEffect(List<Reference> theResultingEffect) { 2490 this.resultingEffect = theResultingEffect; 2491 return this; 2492 } 2493 2494 public boolean hasResultingEffect() { 2495 if (this.resultingEffect == null) 2496 return false; 2497 for (Reference item : this.resultingEffect) 2498 if (!item.isEmpty()) 2499 return true; 2500 return false; 2501 } 2502 2503 public Reference addResultingEffect() { //3 2504 Reference t = new Reference(); 2505 if (this.resultingEffect == null) 2506 this.resultingEffect = new ArrayList<Reference>(); 2507 this.resultingEffect.add(t); 2508 return t; 2509 } 2510 2511 public AdverseEvent addResultingEffect(Reference t) { //3 2512 if (t == null) 2513 return this; 2514 if (this.resultingEffect == null) 2515 this.resultingEffect = new ArrayList<Reference>(); 2516 this.resultingEffect.add(t); 2517 return this; 2518 } 2519 2520 /** 2521 * @return The first repetition of repeating field {@link #resultingEffect}, creating it if it does not already exist {3} 2522 */ 2523 public Reference getResultingEffectFirstRep() { 2524 if (getResultingEffect().isEmpty()) { 2525 addResultingEffect(); 2526 } 2527 return getResultingEffect().get(0); 2528 } 2529 2530 /** 2531 * @return {@link #location} (The information about where the adverse event occurred.) 2532 */ 2533 public Reference getLocation() { 2534 if (this.location == null) 2535 if (Configuration.errorOnAutoCreate()) 2536 throw new Error("Attempt to auto-create AdverseEvent.location"); 2537 else if (Configuration.doAutoCreate()) 2538 this.location = new Reference(); // cc 2539 return this.location; 2540 } 2541 2542 public boolean hasLocation() { 2543 return this.location != null && !this.location.isEmpty(); 2544 } 2545 2546 /** 2547 * @param value {@link #location} (The information about where the adverse event occurred.) 2548 */ 2549 public AdverseEvent setLocation(Reference value) { 2550 this.location = value; 2551 return this; 2552 } 2553 2554 /** 2555 * @return {@link #seriousness} (Assessment whether this event, or averted event, was of clinical importance.) 2556 */ 2557 public CodeableConcept getSeriousness() { 2558 if (this.seriousness == null) 2559 if (Configuration.errorOnAutoCreate()) 2560 throw new Error("Attempt to auto-create AdverseEvent.seriousness"); 2561 else if (Configuration.doAutoCreate()) 2562 this.seriousness = new CodeableConcept(); // cc 2563 return this.seriousness; 2564 } 2565 2566 public boolean hasSeriousness() { 2567 return this.seriousness != null && !this.seriousness.isEmpty(); 2568 } 2569 2570 /** 2571 * @param value {@link #seriousness} (Assessment whether this event, or averted event, was of clinical importance.) 2572 */ 2573 public AdverseEvent setSeriousness(CodeableConcept value) { 2574 this.seriousness = value; 2575 return this; 2576 } 2577 2578 /** 2579 * @return {@link #outcome} (Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal.) 2580 */ 2581 public List<CodeableConcept> getOutcome() { 2582 if (this.outcome == null) 2583 this.outcome = new ArrayList<CodeableConcept>(); 2584 return this.outcome; 2585 } 2586 2587 /** 2588 * @return Returns a reference to <code>this</code> for easy method chaining 2589 */ 2590 public AdverseEvent setOutcome(List<CodeableConcept> theOutcome) { 2591 this.outcome = theOutcome; 2592 return this; 2593 } 2594 2595 public boolean hasOutcome() { 2596 if (this.outcome == null) 2597 return false; 2598 for (CodeableConcept item : this.outcome) 2599 if (!item.isEmpty()) 2600 return true; 2601 return false; 2602 } 2603 2604 public CodeableConcept addOutcome() { //3 2605 CodeableConcept t = new CodeableConcept(); 2606 if (this.outcome == null) 2607 this.outcome = new ArrayList<CodeableConcept>(); 2608 this.outcome.add(t); 2609 return t; 2610 } 2611 2612 public AdverseEvent addOutcome(CodeableConcept t) { //3 2613 if (t == null) 2614 return this; 2615 if (this.outcome == null) 2616 this.outcome = new ArrayList<CodeableConcept>(); 2617 this.outcome.add(t); 2618 return this; 2619 } 2620 2621 /** 2622 * @return The first repetition of repeating field {@link #outcome}, creating it if it does not already exist {3} 2623 */ 2624 public CodeableConcept getOutcomeFirstRep() { 2625 if (getOutcome().isEmpty()) { 2626 addOutcome(); 2627 } 2628 return getOutcome().get(0); 2629 } 2630 2631 /** 2632 * @return {@link #recorder} (Information on who recorded the adverse event. May be the patient or a practitioner.) 2633 */ 2634 public Reference getRecorder() { 2635 if (this.recorder == null) 2636 if (Configuration.errorOnAutoCreate()) 2637 throw new Error("Attempt to auto-create AdverseEvent.recorder"); 2638 else if (Configuration.doAutoCreate()) 2639 this.recorder = new Reference(); // cc 2640 return this.recorder; 2641 } 2642 2643 public boolean hasRecorder() { 2644 return this.recorder != null && !this.recorder.isEmpty(); 2645 } 2646 2647 /** 2648 * @param value {@link #recorder} (Information on who recorded the adverse event. May be the patient or a practitioner.) 2649 */ 2650 public AdverseEvent setRecorder(Reference value) { 2651 this.recorder = value; 2652 return this; 2653 } 2654 2655 /** 2656 * @return {@link #participant} (Indicates who or what participated in the adverse event and how they were involved.) 2657 */ 2658 public List<AdverseEventParticipantComponent> getParticipant() { 2659 if (this.participant == null) 2660 this.participant = new ArrayList<AdverseEventParticipantComponent>(); 2661 return this.participant; 2662 } 2663 2664 /** 2665 * @return Returns a reference to <code>this</code> for easy method chaining 2666 */ 2667 public AdverseEvent setParticipant(List<AdverseEventParticipantComponent> theParticipant) { 2668 this.participant = theParticipant; 2669 return this; 2670 } 2671 2672 public boolean hasParticipant() { 2673 if (this.participant == null) 2674 return false; 2675 for (AdverseEventParticipantComponent item : this.participant) 2676 if (!item.isEmpty()) 2677 return true; 2678 return false; 2679 } 2680 2681 public AdverseEventParticipantComponent addParticipant() { //3 2682 AdverseEventParticipantComponent t = new AdverseEventParticipantComponent(); 2683 if (this.participant == null) 2684 this.participant = new ArrayList<AdverseEventParticipantComponent>(); 2685 this.participant.add(t); 2686 return t; 2687 } 2688 2689 public AdverseEvent addParticipant(AdverseEventParticipantComponent t) { //3 2690 if (t == null) 2691 return this; 2692 if (this.participant == null) 2693 this.participant = new ArrayList<AdverseEventParticipantComponent>(); 2694 this.participant.add(t); 2695 return this; 2696 } 2697 2698 /** 2699 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 2700 */ 2701 public AdverseEventParticipantComponent getParticipantFirstRep() { 2702 if (getParticipant().isEmpty()) { 2703 addParticipant(); 2704 } 2705 return getParticipant().get(0); 2706 } 2707 2708 /** 2709 * @return {@link #study} (The research study that the subject is enrolled in.) 2710 */ 2711 public List<Reference> getStudy() { 2712 if (this.study == null) 2713 this.study = new ArrayList<Reference>(); 2714 return this.study; 2715 } 2716 2717 /** 2718 * @return Returns a reference to <code>this</code> for easy method chaining 2719 */ 2720 public AdverseEvent setStudy(List<Reference> theStudy) { 2721 this.study = theStudy; 2722 return this; 2723 } 2724 2725 public boolean hasStudy() { 2726 if (this.study == null) 2727 return false; 2728 for (Reference item : this.study) 2729 if (!item.isEmpty()) 2730 return true; 2731 return false; 2732 } 2733 2734 public Reference addStudy() { //3 2735 Reference t = new Reference(); 2736 if (this.study == null) 2737 this.study = new ArrayList<Reference>(); 2738 this.study.add(t); 2739 return t; 2740 } 2741 2742 public AdverseEvent addStudy(Reference t) { //3 2743 if (t == null) 2744 return this; 2745 if (this.study == null) 2746 this.study = new ArrayList<Reference>(); 2747 this.study.add(t); 2748 return this; 2749 } 2750 2751 /** 2752 * @return The first repetition of repeating field {@link #study}, creating it if it does not already exist {3} 2753 */ 2754 public Reference getStudyFirstRep() { 2755 if (getStudy().isEmpty()) { 2756 addStudy(); 2757 } 2758 return getStudy().get(0); 2759 } 2760 2761 /** 2762 * @return {@link #expectedInResearchStudy} (Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.). This is the underlying object with id, value and extensions. The accessor "getExpectedInResearchStudy" gives direct access to the value 2763 */ 2764 public BooleanType getExpectedInResearchStudyElement() { 2765 if (this.expectedInResearchStudy == null) 2766 if (Configuration.errorOnAutoCreate()) 2767 throw new Error("Attempt to auto-create AdverseEvent.expectedInResearchStudy"); 2768 else if (Configuration.doAutoCreate()) 2769 this.expectedInResearchStudy = new BooleanType(); // bb 2770 return this.expectedInResearchStudy; 2771 } 2772 2773 public boolean hasExpectedInResearchStudyElement() { 2774 return this.expectedInResearchStudy != null && !this.expectedInResearchStudy.isEmpty(); 2775 } 2776 2777 public boolean hasExpectedInResearchStudy() { 2778 return this.expectedInResearchStudy != null && !this.expectedInResearchStudy.isEmpty(); 2779 } 2780 2781 /** 2782 * @param value {@link #expectedInResearchStudy} (Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.). This is the underlying object with id, value and extensions. The accessor "getExpectedInResearchStudy" gives direct access to the value 2783 */ 2784 public AdverseEvent setExpectedInResearchStudyElement(BooleanType value) { 2785 this.expectedInResearchStudy = value; 2786 return this; 2787 } 2788 2789 /** 2790 * @return Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely. 2791 */ 2792 public boolean getExpectedInResearchStudy() { 2793 return this.expectedInResearchStudy == null || this.expectedInResearchStudy.isEmpty() ? false : this.expectedInResearchStudy.getValue(); 2794 } 2795 2796 /** 2797 * @param value Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely. 2798 */ 2799 public AdverseEvent setExpectedInResearchStudy(boolean value) { 2800 if (this.expectedInResearchStudy == null) 2801 this.expectedInResearchStudy = new BooleanType(); 2802 this.expectedInResearchStudy.setValue(value); 2803 return this; 2804 } 2805 2806 /** 2807 * @return {@link #suspectEntity} (Describes the entity that is suspected to have caused the adverse event.) 2808 */ 2809 public List<AdverseEventSuspectEntityComponent> getSuspectEntity() { 2810 if (this.suspectEntity == null) 2811 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2812 return this.suspectEntity; 2813 } 2814 2815 /** 2816 * @return Returns a reference to <code>this</code> for easy method chaining 2817 */ 2818 public AdverseEvent setSuspectEntity(List<AdverseEventSuspectEntityComponent> theSuspectEntity) { 2819 this.suspectEntity = theSuspectEntity; 2820 return this; 2821 } 2822 2823 public boolean hasSuspectEntity() { 2824 if (this.suspectEntity == null) 2825 return false; 2826 for (AdverseEventSuspectEntityComponent item : this.suspectEntity) 2827 if (!item.isEmpty()) 2828 return true; 2829 return false; 2830 } 2831 2832 public AdverseEventSuspectEntityComponent addSuspectEntity() { //3 2833 AdverseEventSuspectEntityComponent t = new AdverseEventSuspectEntityComponent(); 2834 if (this.suspectEntity == null) 2835 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2836 this.suspectEntity.add(t); 2837 return t; 2838 } 2839 2840 public AdverseEvent addSuspectEntity(AdverseEventSuspectEntityComponent t) { //3 2841 if (t == null) 2842 return this; 2843 if (this.suspectEntity == null) 2844 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2845 this.suspectEntity.add(t); 2846 return this; 2847 } 2848 2849 /** 2850 * @return The first repetition of repeating field {@link #suspectEntity}, creating it if it does not already exist {3} 2851 */ 2852 public AdverseEventSuspectEntityComponent getSuspectEntityFirstRep() { 2853 if (getSuspectEntity().isEmpty()) { 2854 addSuspectEntity(); 2855 } 2856 return getSuspectEntity().get(0); 2857 } 2858 2859 /** 2860 * @return {@link #contributingFactor} (The contributing factors suspected to have increased the probability or severity of the adverse event.) 2861 */ 2862 public List<AdverseEventContributingFactorComponent> getContributingFactor() { 2863 if (this.contributingFactor == null) 2864 this.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 2865 return this.contributingFactor; 2866 } 2867 2868 /** 2869 * @return Returns a reference to <code>this</code> for easy method chaining 2870 */ 2871 public AdverseEvent setContributingFactor(List<AdverseEventContributingFactorComponent> theContributingFactor) { 2872 this.contributingFactor = theContributingFactor; 2873 return this; 2874 } 2875 2876 public boolean hasContributingFactor() { 2877 if (this.contributingFactor == null) 2878 return false; 2879 for (AdverseEventContributingFactorComponent item : this.contributingFactor) 2880 if (!item.isEmpty()) 2881 return true; 2882 return false; 2883 } 2884 2885 public AdverseEventContributingFactorComponent addContributingFactor() { //3 2886 AdverseEventContributingFactorComponent t = new AdverseEventContributingFactorComponent(); 2887 if (this.contributingFactor == null) 2888 this.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 2889 this.contributingFactor.add(t); 2890 return t; 2891 } 2892 2893 public AdverseEvent addContributingFactor(AdverseEventContributingFactorComponent t) { //3 2894 if (t == null) 2895 return this; 2896 if (this.contributingFactor == null) 2897 this.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 2898 this.contributingFactor.add(t); 2899 return this; 2900 } 2901 2902 /** 2903 * @return The first repetition of repeating field {@link #contributingFactor}, creating it if it does not already exist {3} 2904 */ 2905 public AdverseEventContributingFactorComponent getContributingFactorFirstRep() { 2906 if (getContributingFactor().isEmpty()) { 2907 addContributingFactor(); 2908 } 2909 return getContributingFactor().get(0); 2910 } 2911 2912 /** 2913 * @return {@link #preventiveAction} (Preventive actions that contributed to avoiding the adverse event.) 2914 */ 2915 public List<AdverseEventPreventiveActionComponent> getPreventiveAction() { 2916 if (this.preventiveAction == null) 2917 this.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 2918 return this.preventiveAction; 2919 } 2920 2921 /** 2922 * @return Returns a reference to <code>this</code> for easy method chaining 2923 */ 2924 public AdverseEvent setPreventiveAction(List<AdverseEventPreventiveActionComponent> thePreventiveAction) { 2925 this.preventiveAction = thePreventiveAction; 2926 return this; 2927 } 2928 2929 public boolean hasPreventiveAction() { 2930 if (this.preventiveAction == null) 2931 return false; 2932 for (AdverseEventPreventiveActionComponent item : this.preventiveAction) 2933 if (!item.isEmpty()) 2934 return true; 2935 return false; 2936 } 2937 2938 public AdverseEventPreventiveActionComponent addPreventiveAction() { //3 2939 AdverseEventPreventiveActionComponent t = new AdverseEventPreventiveActionComponent(); 2940 if (this.preventiveAction == null) 2941 this.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 2942 this.preventiveAction.add(t); 2943 return t; 2944 } 2945 2946 public AdverseEvent addPreventiveAction(AdverseEventPreventiveActionComponent t) { //3 2947 if (t == null) 2948 return this; 2949 if (this.preventiveAction == null) 2950 this.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 2951 this.preventiveAction.add(t); 2952 return this; 2953 } 2954 2955 /** 2956 * @return The first repetition of repeating field {@link #preventiveAction}, creating it if it does not already exist {3} 2957 */ 2958 public AdverseEventPreventiveActionComponent getPreventiveActionFirstRep() { 2959 if (getPreventiveAction().isEmpty()) { 2960 addPreventiveAction(); 2961 } 2962 return getPreventiveAction().get(0); 2963 } 2964 2965 /** 2966 * @return {@link #mitigatingAction} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 2967 */ 2968 public List<AdverseEventMitigatingActionComponent> getMitigatingAction() { 2969 if (this.mitigatingAction == null) 2970 this.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 2971 return this.mitigatingAction; 2972 } 2973 2974 /** 2975 * @return Returns a reference to <code>this</code> for easy method chaining 2976 */ 2977 public AdverseEvent setMitigatingAction(List<AdverseEventMitigatingActionComponent> theMitigatingAction) { 2978 this.mitigatingAction = theMitigatingAction; 2979 return this; 2980 } 2981 2982 public boolean hasMitigatingAction() { 2983 if (this.mitigatingAction == null) 2984 return false; 2985 for (AdverseEventMitigatingActionComponent item : this.mitigatingAction) 2986 if (!item.isEmpty()) 2987 return true; 2988 return false; 2989 } 2990 2991 public AdverseEventMitigatingActionComponent addMitigatingAction() { //3 2992 AdverseEventMitigatingActionComponent t = new AdverseEventMitigatingActionComponent(); 2993 if (this.mitigatingAction == null) 2994 this.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 2995 this.mitigatingAction.add(t); 2996 return t; 2997 } 2998 2999 public AdverseEvent addMitigatingAction(AdverseEventMitigatingActionComponent t) { //3 3000 if (t == null) 3001 return this; 3002 if (this.mitigatingAction == null) 3003 this.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 3004 this.mitigatingAction.add(t); 3005 return this; 3006 } 3007 3008 /** 3009 * @return The first repetition of repeating field {@link #mitigatingAction}, creating it if it does not already exist {3} 3010 */ 3011 public AdverseEventMitigatingActionComponent getMitigatingActionFirstRep() { 3012 if (getMitigatingAction().isEmpty()) { 3013 addMitigatingAction(); 3014 } 3015 return getMitigatingAction().get(0); 3016 } 3017 3018 /** 3019 * @return {@link #supportingInfo} (Supporting information relevant to the event.) 3020 */ 3021 public List<AdverseEventSupportingInfoComponent> getSupportingInfo() { 3022 if (this.supportingInfo == null) 3023 this.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3024 return this.supportingInfo; 3025 } 3026 3027 /** 3028 * @return Returns a reference to <code>this</code> for easy method chaining 3029 */ 3030 public AdverseEvent setSupportingInfo(List<AdverseEventSupportingInfoComponent> theSupportingInfo) { 3031 this.supportingInfo = theSupportingInfo; 3032 return this; 3033 } 3034 3035 public boolean hasSupportingInfo() { 3036 if (this.supportingInfo == null) 3037 return false; 3038 for (AdverseEventSupportingInfoComponent item : this.supportingInfo) 3039 if (!item.isEmpty()) 3040 return true; 3041 return false; 3042 } 3043 3044 public AdverseEventSupportingInfoComponent addSupportingInfo() { //3 3045 AdverseEventSupportingInfoComponent t = new AdverseEventSupportingInfoComponent(); 3046 if (this.supportingInfo == null) 3047 this.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3048 this.supportingInfo.add(t); 3049 return t; 3050 } 3051 3052 public AdverseEvent addSupportingInfo(AdverseEventSupportingInfoComponent t) { //3 3053 if (t == null) 3054 return this; 3055 if (this.supportingInfo == null) 3056 this.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3057 this.supportingInfo.add(t); 3058 return this; 3059 } 3060 3061 /** 3062 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 3063 */ 3064 public AdverseEventSupportingInfoComponent getSupportingInfoFirstRep() { 3065 if (getSupportingInfo().isEmpty()) { 3066 addSupportingInfo(); 3067 } 3068 return getSupportingInfo().get(0); 3069 } 3070 3071 /** 3072 * @return {@link #note} (Comments made about the adverse event by the performer, subject or other participants.) 3073 */ 3074 public List<Annotation> getNote() { 3075 if (this.note == null) 3076 this.note = new ArrayList<Annotation>(); 3077 return this.note; 3078 } 3079 3080 /** 3081 * @return Returns a reference to <code>this</code> for easy method chaining 3082 */ 3083 public AdverseEvent setNote(List<Annotation> theNote) { 3084 this.note = theNote; 3085 return this; 3086 } 3087 3088 public boolean hasNote() { 3089 if (this.note == null) 3090 return false; 3091 for (Annotation item : this.note) 3092 if (!item.isEmpty()) 3093 return true; 3094 return false; 3095 } 3096 3097 public Annotation addNote() { //3 3098 Annotation t = new Annotation(); 3099 if (this.note == null) 3100 this.note = new ArrayList<Annotation>(); 3101 this.note.add(t); 3102 return t; 3103 } 3104 3105 public AdverseEvent addNote(Annotation t) { //3 3106 if (t == null) 3107 return this; 3108 if (this.note == null) 3109 this.note = new ArrayList<Annotation>(); 3110 this.note.add(t); 3111 return this; 3112 } 3113 3114 /** 3115 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3116 */ 3117 public Annotation getNoteFirstRep() { 3118 if (getNote().isEmpty()) { 3119 addNote(); 3120 } 3121 return getNote().get(0); 3122 } 3123 3124 protected void listChildren(List<Property> children) { 3125 super.listChildren(children); 3126 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3127 children.add(new Property("status", "code", "The current state of the adverse event or potential adverse event.", 0, 1, status)); 3128 children.add(new Property("actuality", "code", "Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.", 0, 1, actuality)); 3129 children.add(new Property("category", "CodeableConcept", "The overall type of event, intended for search and filtering purposes.", 0, java.lang.Integer.MAX_VALUE, category)); 3130 children.add(new Property("code", "CodeableConcept", "Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.", 0, 1, code)); 3131 children.add(new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|ResearchSubject)", "This subject or group impacted by the event.", 0, 1, subject)); 3132 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter associated with the start of the AdverseEvent.", 0, 1, encounter)); 3133 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence)); 3134 children.add(new Property("detected", "dateTime", "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.", 0, 1, detected)); 3135 children.add(new Property("recordedDate", "dateTime", "The date on which the existence of the AdverseEvent was first recorded.", 0, 1, recordedDate)); 3136 children.add(new Property("resultingEffect", "Reference(Condition|Observation)", "Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall.", 0, java.lang.Integer.MAX_VALUE, resultingEffect)); 3137 children.add(new Property("location", "Reference(Location)", "The information about where the adverse event occurred.", 0, 1, location)); 3138 children.add(new Property("seriousness", "CodeableConcept", "Assessment whether this event, or averted event, was of clinical importance.", 0, 1, seriousness)); 3139 children.add(new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal.", 0, java.lang.Integer.MAX_VALUE, outcome)); 3140 children.add(new Property("recorder", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|ResearchSubject)", "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder)); 3141 children.add(new Property("participant", "", "Indicates who or what participated in the adverse event and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant)); 3142 children.add(new Property("study", "Reference(ResearchStudy)", "The research study that the subject is enrolled in.", 0, java.lang.Integer.MAX_VALUE, study)); 3143 children.add(new Property("expectedInResearchStudy", "boolean", "Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.", 0, 1, expectedInResearchStudy)); 3144 children.add(new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, suspectEntity)); 3145 children.add(new Property("contributingFactor", "", "The contributing factors suspected to have increased the probability or severity of the adverse event.", 0, java.lang.Integer.MAX_VALUE, contributingFactor)); 3146 children.add(new Property("preventiveAction", "", "Preventive actions that contributed to avoiding the adverse event.", 0, java.lang.Integer.MAX_VALUE, preventiveAction)); 3147 children.add(new Property("mitigatingAction", "", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, java.lang.Integer.MAX_VALUE, mitigatingAction)); 3148 children.add(new Property("supportingInfo", "", "Supporting information relevant to the event.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 3149 children.add(new Property("note", "Annotation", "Comments made about the adverse event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 3150 } 3151 3152 @Override 3153 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3154 switch (_hash) { 3155 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3156 case -892481550: /*status*/ return new Property("status", "code", "The current state of the adverse event or potential adverse event.", 0, 1, status); 3157 case 528866400: /*actuality*/ return new Property("actuality", "code", "Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.", 0, 1, actuality); 3158 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The overall type of event, intended for search and filtering purposes.", 0, java.lang.Integer.MAX_VALUE, category); 3159 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.", 0, 1, code); 3160 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|ResearchSubject)", "This subject or group impacted by the event.", 0, 1, subject); 3161 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter associated with the start of the AdverseEvent.", 0, 1, encounter); 3162 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3163 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3164 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3165 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3166 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3167 case 1048254082: /*detected*/ return new Property("detected", "dateTime", "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.", 0, 1, detected); 3168 case -1952893826: /*recordedDate*/ return new Property("recordedDate", "dateTime", "The date on which the existence of the AdverseEvent was first recorded.", 0, 1, recordedDate); 3169 case -2113579882: /*resultingEffect*/ return new Property("resultingEffect", "Reference(Condition|Observation)", "Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall.", 0, java.lang.Integer.MAX_VALUE, resultingEffect); 3170 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The information about where the adverse event occurred.", 0, 1, location); 3171 case -1551003909: /*seriousness*/ return new Property("seriousness", "CodeableConcept", "Assessment whether this event, or averted event, was of clinical importance.", 0, 1, seriousness); 3172 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal.", 0, java.lang.Integer.MAX_VALUE, outcome); 3173 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|ResearchSubject)", "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder); 3174 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what participated in the adverse event and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant); 3175 case 109776329: /*study*/ return new Property("study", "Reference(ResearchStudy)", "The research study that the subject is enrolled in.", 0, java.lang.Integer.MAX_VALUE, study); 3176 case -1071467023: /*expectedInResearchStudy*/ return new Property("expectedInResearchStudy", "boolean", "Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.", 0, 1, expectedInResearchStudy); 3177 case -1957422662: /*suspectEntity*/ return new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, suspectEntity); 3178 case -219647527: /*contributingFactor*/ return new Property("contributingFactor", "", "The contributing factors suspected to have increased the probability or severity of the adverse event.", 0, java.lang.Integer.MAX_VALUE, contributingFactor); 3179 case 2052341334: /*preventiveAction*/ return new Property("preventiveAction", "", "Preventive actions that contributed to avoiding the adverse event.", 0, java.lang.Integer.MAX_VALUE, preventiveAction); 3180 case 1992862383: /*mitigatingAction*/ return new Property("mitigatingAction", "", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, java.lang.Integer.MAX_VALUE, mitigatingAction); 3181 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Supporting information relevant to the event.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 3182 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the adverse event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 3183 default: return super.getNamedProperty(_hash, _name, _checkValid); 3184 } 3185 3186 } 3187 3188 @Override 3189 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3190 switch (hash) { 3191 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3192 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<AdverseEventStatus> 3193 case 528866400: /*actuality*/ return this.actuality == null ? new Base[0] : new Base[] {this.actuality}; // Enumeration<AdverseEventActuality> 3194 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3195 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3196 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3197 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3198 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 3199 case 1048254082: /*detected*/ return this.detected == null ? new Base[0] : new Base[] {this.detected}; // DateTimeType 3200 case -1952893826: /*recordedDate*/ return this.recordedDate == null ? new Base[0] : new Base[] {this.recordedDate}; // DateTimeType 3201 case -2113579882: /*resultingEffect*/ return this.resultingEffect == null ? new Base[0] : this.resultingEffect.toArray(new Base[this.resultingEffect.size()]); // Reference 3202 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 3203 case -1551003909: /*seriousness*/ return this.seriousness == null ? new Base[0] : new Base[] {this.seriousness}; // CodeableConcept 3204 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : this.outcome.toArray(new Base[this.outcome.size()]); // CodeableConcept 3205 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 3206 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // AdverseEventParticipantComponent 3207 case 109776329: /*study*/ return this.study == null ? new Base[0] : this.study.toArray(new Base[this.study.size()]); // Reference 3208 case -1071467023: /*expectedInResearchStudy*/ return this.expectedInResearchStudy == null ? new Base[0] : new Base[] {this.expectedInResearchStudy}; // BooleanType 3209 case -1957422662: /*suspectEntity*/ return this.suspectEntity == null ? new Base[0] : this.suspectEntity.toArray(new Base[this.suspectEntity.size()]); // AdverseEventSuspectEntityComponent 3210 case -219647527: /*contributingFactor*/ return this.contributingFactor == null ? new Base[0] : this.contributingFactor.toArray(new Base[this.contributingFactor.size()]); // AdverseEventContributingFactorComponent 3211 case 2052341334: /*preventiveAction*/ return this.preventiveAction == null ? new Base[0] : this.preventiveAction.toArray(new Base[this.preventiveAction.size()]); // AdverseEventPreventiveActionComponent 3212 case 1992862383: /*mitigatingAction*/ return this.mitigatingAction == null ? new Base[0] : this.mitigatingAction.toArray(new Base[this.mitigatingAction.size()]); // AdverseEventMitigatingActionComponent 3213 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // AdverseEventSupportingInfoComponent 3214 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3215 default: return super.getProperty(hash, name, checkValid); 3216 } 3217 3218 } 3219 3220 @Override 3221 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3222 switch (hash) { 3223 case -1618432855: // identifier 3224 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3225 return value; 3226 case -892481550: // status 3227 value = new AdverseEventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3228 this.status = (Enumeration) value; // Enumeration<AdverseEventStatus> 3229 return value; 3230 case 528866400: // actuality 3231 value = new AdverseEventActualityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3232 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 3233 return value; 3234 case 50511102: // category 3235 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3236 return value; 3237 case 3059181: // code 3238 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3239 return value; 3240 case -1867885268: // subject 3241 this.subject = TypeConvertor.castToReference(value); // Reference 3242 return value; 3243 case 1524132147: // encounter 3244 this.encounter = TypeConvertor.castToReference(value); // Reference 3245 return value; 3246 case 1687874001: // occurrence 3247 this.occurrence = TypeConvertor.castToType(value); // DataType 3248 return value; 3249 case 1048254082: // detected 3250 this.detected = TypeConvertor.castToDateTime(value); // DateTimeType 3251 return value; 3252 case -1952893826: // recordedDate 3253 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 3254 return value; 3255 case -2113579882: // resultingEffect 3256 this.getResultingEffect().add(TypeConvertor.castToReference(value)); // Reference 3257 return value; 3258 case 1901043637: // location 3259 this.location = TypeConvertor.castToReference(value); // Reference 3260 return value; 3261 case -1551003909: // seriousness 3262 this.seriousness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3263 return value; 3264 case -1106507950: // outcome 3265 this.getOutcome().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3266 return value; 3267 case -799233858: // recorder 3268 this.recorder = TypeConvertor.castToReference(value); // Reference 3269 return value; 3270 case 767422259: // participant 3271 this.getParticipant().add((AdverseEventParticipantComponent) value); // AdverseEventParticipantComponent 3272 return value; 3273 case 109776329: // study 3274 this.getStudy().add(TypeConvertor.castToReference(value)); // Reference 3275 return value; 3276 case -1071467023: // expectedInResearchStudy 3277 this.expectedInResearchStudy = TypeConvertor.castToBoolean(value); // BooleanType 3278 return value; 3279 case -1957422662: // suspectEntity 3280 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); // AdverseEventSuspectEntityComponent 3281 return value; 3282 case -219647527: // contributingFactor 3283 this.getContributingFactor().add((AdverseEventContributingFactorComponent) value); // AdverseEventContributingFactorComponent 3284 return value; 3285 case 2052341334: // preventiveAction 3286 this.getPreventiveAction().add((AdverseEventPreventiveActionComponent) value); // AdverseEventPreventiveActionComponent 3287 return value; 3288 case 1992862383: // mitigatingAction 3289 this.getMitigatingAction().add((AdverseEventMitigatingActionComponent) value); // AdverseEventMitigatingActionComponent 3290 return value; 3291 case 1922406657: // supportingInfo 3292 this.getSupportingInfo().add((AdverseEventSupportingInfoComponent) value); // AdverseEventSupportingInfoComponent 3293 return value; 3294 case 3387378: // note 3295 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3296 return value; 3297 default: return super.setProperty(hash, name, value); 3298 } 3299 3300 } 3301 3302 @Override 3303 public Base setProperty(String name, Base value) throws FHIRException { 3304 if (name.equals("identifier")) { 3305 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3306 } else if (name.equals("status")) { 3307 value = new AdverseEventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3308 this.status = (Enumeration) value; // Enumeration<AdverseEventStatus> 3309 } else if (name.equals("actuality")) { 3310 value = new AdverseEventActualityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3311 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 3312 } else if (name.equals("category")) { 3313 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3314 } else if (name.equals("code")) { 3315 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3316 } else if (name.equals("subject")) { 3317 this.subject = TypeConvertor.castToReference(value); // Reference 3318 } else if (name.equals("encounter")) { 3319 this.encounter = TypeConvertor.castToReference(value); // Reference 3320 } else if (name.equals("occurrence[x]")) { 3321 this.occurrence = TypeConvertor.castToType(value); // DataType 3322 } else if (name.equals("detected")) { 3323 this.detected = TypeConvertor.castToDateTime(value); // DateTimeType 3324 } else if (name.equals("recordedDate")) { 3325 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 3326 } else if (name.equals("resultingEffect")) { 3327 this.getResultingEffect().add(TypeConvertor.castToReference(value)); 3328 } else if (name.equals("location")) { 3329 this.location = TypeConvertor.castToReference(value); // Reference 3330 } else if (name.equals("seriousness")) { 3331 this.seriousness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3332 } else if (name.equals("outcome")) { 3333 this.getOutcome().add(TypeConvertor.castToCodeableConcept(value)); 3334 } else if (name.equals("recorder")) { 3335 this.recorder = TypeConvertor.castToReference(value); // Reference 3336 } else if (name.equals("participant")) { 3337 this.getParticipant().add((AdverseEventParticipantComponent) value); 3338 } else if (name.equals("study")) { 3339 this.getStudy().add(TypeConvertor.castToReference(value)); 3340 } else if (name.equals("expectedInResearchStudy")) { 3341 this.expectedInResearchStudy = TypeConvertor.castToBoolean(value); // BooleanType 3342 } else if (name.equals("suspectEntity")) { 3343 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); 3344 } else if (name.equals("contributingFactor")) { 3345 this.getContributingFactor().add((AdverseEventContributingFactorComponent) value); 3346 } else if (name.equals("preventiveAction")) { 3347 this.getPreventiveAction().add((AdverseEventPreventiveActionComponent) value); 3348 } else if (name.equals("mitigatingAction")) { 3349 this.getMitigatingAction().add((AdverseEventMitigatingActionComponent) value); 3350 } else if (name.equals("supportingInfo")) { 3351 this.getSupportingInfo().add((AdverseEventSupportingInfoComponent) value); 3352 } else if (name.equals("note")) { 3353 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3354 } else 3355 return super.setProperty(name, value); 3356 return value; 3357 } 3358 3359 @Override 3360 public void removeChild(String name, Base value) throws FHIRException { 3361 if (name.equals("identifier")) { 3362 this.getIdentifier().remove(value); 3363 } else if (name.equals("status")) { 3364 value = new AdverseEventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3365 this.status = (Enumeration) value; // Enumeration<AdverseEventStatus> 3366 } else if (name.equals("actuality")) { 3367 value = new AdverseEventActualityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3368 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 3369 } else if (name.equals("category")) { 3370 this.getCategory().remove(value); 3371 } else if (name.equals("code")) { 3372 this.code = null; 3373 } else if (name.equals("subject")) { 3374 this.subject = null; 3375 } else if (name.equals("encounter")) { 3376 this.encounter = null; 3377 } else if (name.equals("occurrence[x]")) { 3378 this.occurrence = null; 3379 } else if (name.equals("detected")) { 3380 this.detected = null; 3381 } else if (name.equals("recordedDate")) { 3382 this.recordedDate = null; 3383 } else if (name.equals("resultingEffect")) { 3384 this.getResultingEffect().remove(value); 3385 } else if (name.equals("location")) { 3386 this.location = null; 3387 } else if (name.equals("seriousness")) { 3388 this.seriousness = null; 3389 } else if (name.equals("outcome")) { 3390 this.getOutcome().remove(value); 3391 } else if (name.equals("recorder")) { 3392 this.recorder = null; 3393 } else if (name.equals("participant")) { 3394 this.getParticipant().remove((AdverseEventParticipantComponent) value); 3395 } else if (name.equals("study")) { 3396 this.getStudy().remove(value); 3397 } else if (name.equals("expectedInResearchStudy")) { 3398 this.expectedInResearchStudy = null; 3399 } else if (name.equals("suspectEntity")) { 3400 this.getSuspectEntity().remove((AdverseEventSuspectEntityComponent) value); 3401 } else if (name.equals("contributingFactor")) { 3402 this.getContributingFactor().remove((AdverseEventContributingFactorComponent) value); 3403 } else if (name.equals("preventiveAction")) { 3404 this.getPreventiveAction().remove((AdverseEventPreventiveActionComponent) value); 3405 } else if (name.equals("mitigatingAction")) { 3406 this.getMitigatingAction().remove((AdverseEventMitigatingActionComponent) value); 3407 } else if (name.equals("supportingInfo")) { 3408 this.getSupportingInfo().remove((AdverseEventSupportingInfoComponent) value); 3409 } else if (name.equals("note")) { 3410 this.getNote().remove(value); 3411 } else 3412 super.removeChild(name, value); 3413 3414 } 3415 3416 @Override 3417 public Base makeProperty(int hash, String name) throws FHIRException { 3418 switch (hash) { 3419 case -1618432855: return addIdentifier(); 3420 case -892481550: return getStatusElement(); 3421 case 528866400: return getActualityElement(); 3422 case 50511102: return addCategory(); 3423 case 3059181: return getCode(); 3424 case -1867885268: return getSubject(); 3425 case 1524132147: return getEncounter(); 3426 case -2022646513: return getOccurrence(); 3427 case 1687874001: return getOccurrence(); 3428 case 1048254082: return getDetectedElement(); 3429 case -1952893826: return getRecordedDateElement(); 3430 case -2113579882: return addResultingEffect(); 3431 case 1901043637: return getLocation(); 3432 case -1551003909: return getSeriousness(); 3433 case -1106507950: return addOutcome(); 3434 case -799233858: return getRecorder(); 3435 case 767422259: return addParticipant(); 3436 case 109776329: return addStudy(); 3437 case -1071467023: return getExpectedInResearchStudyElement(); 3438 case -1957422662: return addSuspectEntity(); 3439 case -219647527: return addContributingFactor(); 3440 case 2052341334: return addPreventiveAction(); 3441 case 1992862383: return addMitigatingAction(); 3442 case 1922406657: return addSupportingInfo(); 3443 case 3387378: return addNote(); 3444 default: return super.makeProperty(hash, name); 3445 } 3446 3447 } 3448 3449 @Override 3450 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3451 switch (hash) { 3452 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3453 case -892481550: /*status*/ return new String[] {"code"}; 3454 case 528866400: /*actuality*/ return new String[] {"code"}; 3455 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3456 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3457 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3458 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3459 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 3460 case 1048254082: /*detected*/ return new String[] {"dateTime"}; 3461 case -1952893826: /*recordedDate*/ return new String[] {"dateTime"}; 3462 case -2113579882: /*resultingEffect*/ return new String[] {"Reference"}; 3463 case 1901043637: /*location*/ return new String[] {"Reference"}; 3464 case -1551003909: /*seriousness*/ return new String[] {"CodeableConcept"}; 3465 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 3466 case -799233858: /*recorder*/ return new String[] {"Reference"}; 3467 case 767422259: /*participant*/ return new String[] {}; 3468 case 109776329: /*study*/ return new String[] {"Reference"}; 3469 case -1071467023: /*expectedInResearchStudy*/ return new String[] {"boolean"}; 3470 case -1957422662: /*suspectEntity*/ return new String[] {}; 3471 case -219647527: /*contributingFactor*/ return new String[] {}; 3472 case 2052341334: /*preventiveAction*/ return new String[] {}; 3473 case 1992862383: /*mitigatingAction*/ return new String[] {}; 3474 case 1922406657: /*supportingInfo*/ return new String[] {}; 3475 case 3387378: /*note*/ return new String[] {"Annotation"}; 3476 default: return super.getTypesForProperty(hash, name); 3477 } 3478 3479 } 3480 3481 @Override 3482 public Base addChild(String name) throws FHIRException { 3483 if (name.equals("identifier")) { 3484 return addIdentifier(); 3485 } 3486 else if (name.equals("status")) { 3487 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.status"); 3488 } 3489 else if (name.equals("actuality")) { 3490 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.actuality"); 3491 } 3492 else if (name.equals("category")) { 3493 return addCategory(); 3494 } 3495 else if (name.equals("code")) { 3496 this.code = new CodeableConcept(); 3497 return this.code; 3498 } 3499 else if (name.equals("subject")) { 3500 this.subject = new Reference(); 3501 return this.subject; 3502 } 3503 else if (name.equals("encounter")) { 3504 this.encounter = new Reference(); 3505 return this.encounter; 3506 } 3507 else if (name.equals("occurrenceDateTime")) { 3508 this.occurrence = new DateTimeType(); 3509 return this.occurrence; 3510 } 3511 else if (name.equals("occurrencePeriod")) { 3512 this.occurrence = new Period(); 3513 return this.occurrence; 3514 } 3515 else if (name.equals("occurrenceTiming")) { 3516 this.occurrence = new Timing(); 3517 return this.occurrence; 3518 } 3519 else if (name.equals("detected")) { 3520 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.detected"); 3521 } 3522 else if (name.equals("recordedDate")) { 3523 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.recordedDate"); 3524 } 3525 else if (name.equals("resultingEffect")) { 3526 return addResultingEffect(); 3527 } 3528 else if (name.equals("location")) { 3529 this.location = new Reference(); 3530 return this.location; 3531 } 3532 else if (name.equals("seriousness")) { 3533 this.seriousness = new CodeableConcept(); 3534 return this.seriousness; 3535 } 3536 else if (name.equals("outcome")) { 3537 return addOutcome(); 3538 } 3539 else if (name.equals("recorder")) { 3540 this.recorder = new Reference(); 3541 return this.recorder; 3542 } 3543 else if (name.equals("participant")) { 3544 return addParticipant(); 3545 } 3546 else if (name.equals("study")) { 3547 return addStudy(); 3548 } 3549 else if (name.equals("expectedInResearchStudy")) { 3550 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.expectedInResearchStudy"); 3551 } 3552 else if (name.equals("suspectEntity")) { 3553 return addSuspectEntity(); 3554 } 3555 else if (name.equals("contributingFactor")) { 3556 return addContributingFactor(); 3557 } 3558 else if (name.equals("preventiveAction")) { 3559 return addPreventiveAction(); 3560 } 3561 else if (name.equals("mitigatingAction")) { 3562 return addMitigatingAction(); 3563 } 3564 else if (name.equals("supportingInfo")) { 3565 return addSupportingInfo(); 3566 } 3567 else if (name.equals("note")) { 3568 return addNote(); 3569 } 3570 else 3571 return super.addChild(name); 3572 } 3573 3574 public String fhirType() { 3575 return "AdverseEvent"; 3576 3577 } 3578 3579 public AdverseEvent copy() { 3580 AdverseEvent dst = new AdverseEvent(); 3581 copyValues(dst); 3582 return dst; 3583 } 3584 3585 public void copyValues(AdverseEvent dst) { 3586 super.copyValues(dst); 3587 if (identifier != null) { 3588 dst.identifier = new ArrayList<Identifier>(); 3589 for (Identifier i : identifier) 3590 dst.identifier.add(i.copy()); 3591 }; 3592 dst.status = status == null ? null : status.copy(); 3593 dst.actuality = actuality == null ? null : actuality.copy(); 3594 if (category != null) { 3595 dst.category = new ArrayList<CodeableConcept>(); 3596 for (CodeableConcept i : category) 3597 dst.category.add(i.copy()); 3598 }; 3599 dst.code = code == null ? null : code.copy(); 3600 dst.subject = subject == null ? null : subject.copy(); 3601 dst.encounter = encounter == null ? null : encounter.copy(); 3602 dst.occurrence = occurrence == null ? null : occurrence.copy(); 3603 dst.detected = detected == null ? null : detected.copy(); 3604 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 3605 if (resultingEffect != null) { 3606 dst.resultingEffect = new ArrayList<Reference>(); 3607 for (Reference i : resultingEffect) 3608 dst.resultingEffect.add(i.copy()); 3609 }; 3610 dst.location = location == null ? null : location.copy(); 3611 dst.seriousness = seriousness == null ? null : seriousness.copy(); 3612 if (outcome != null) { 3613 dst.outcome = new ArrayList<CodeableConcept>(); 3614 for (CodeableConcept i : outcome) 3615 dst.outcome.add(i.copy()); 3616 }; 3617 dst.recorder = recorder == null ? null : recorder.copy(); 3618 if (participant != null) { 3619 dst.participant = new ArrayList<AdverseEventParticipantComponent>(); 3620 for (AdverseEventParticipantComponent i : participant) 3621 dst.participant.add(i.copy()); 3622 }; 3623 if (study != null) { 3624 dst.study = new ArrayList<Reference>(); 3625 for (Reference i : study) 3626 dst.study.add(i.copy()); 3627 }; 3628 dst.expectedInResearchStudy = expectedInResearchStudy == null ? null : expectedInResearchStudy.copy(); 3629 if (suspectEntity != null) { 3630 dst.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 3631 for (AdverseEventSuspectEntityComponent i : suspectEntity) 3632 dst.suspectEntity.add(i.copy()); 3633 }; 3634 if (contributingFactor != null) { 3635 dst.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 3636 for (AdverseEventContributingFactorComponent i : contributingFactor) 3637 dst.contributingFactor.add(i.copy()); 3638 }; 3639 if (preventiveAction != null) { 3640 dst.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 3641 for (AdverseEventPreventiveActionComponent i : preventiveAction) 3642 dst.preventiveAction.add(i.copy()); 3643 }; 3644 if (mitigatingAction != null) { 3645 dst.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 3646 for (AdverseEventMitigatingActionComponent i : mitigatingAction) 3647 dst.mitigatingAction.add(i.copy()); 3648 }; 3649 if (supportingInfo != null) { 3650 dst.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3651 for (AdverseEventSupportingInfoComponent i : supportingInfo) 3652 dst.supportingInfo.add(i.copy()); 3653 }; 3654 if (note != null) { 3655 dst.note = new ArrayList<Annotation>(); 3656 for (Annotation i : note) 3657 dst.note.add(i.copy()); 3658 }; 3659 } 3660 3661 protected AdverseEvent typedCopy() { 3662 return copy(); 3663 } 3664 3665 @Override 3666 public boolean equalsDeep(Base other_) { 3667 if (!super.equalsDeep(other_)) 3668 return false; 3669 if (!(other_ instanceof AdverseEvent)) 3670 return false; 3671 AdverseEvent o = (AdverseEvent) other_; 3672 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(actuality, o.actuality, true) 3673 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 3674 && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) && compareDeep(detected, o.detected, true) 3675 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(resultingEffect, o.resultingEffect, true) 3676 && compareDeep(location, o.location, true) && compareDeep(seriousness, o.seriousness, true) && compareDeep(outcome, o.outcome, true) 3677 && compareDeep(recorder, o.recorder, true) && compareDeep(participant, o.participant, true) && compareDeep(study, o.study, true) 3678 && compareDeep(expectedInResearchStudy, o.expectedInResearchStudy, true) && compareDeep(suspectEntity, o.suspectEntity, true) 3679 && compareDeep(contributingFactor, o.contributingFactor, true) && compareDeep(preventiveAction, o.preventiveAction, true) 3680 && compareDeep(mitigatingAction, o.mitigatingAction, true) && compareDeep(supportingInfo, o.supportingInfo, true) 3681 && compareDeep(note, o.note, true); 3682 } 3683 3684 @Override 3685 public boolean equalsShallow(Base other_) { 3686 if (!super.equalsShallow(other_)) 3687 return false; 3688 if (!(other_ instanceof AdverseEvent)) 3689 return false; 3690 AdverseEvent o = (AdverseEvent) other_; 3691 return compareValues(status, o.status, true) && compareValues(actuality, o.actuality, true) && compareValues(detected, o.detected, true) 3692 && compareValues(recordedDate, o.recordedDate, true) && compareValues(expectedInResearchStudy, o.expectedInResearchStudy, true) 3693 ; 3694 } 3695 3696 public boolean isEmpty() { 3697 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, actuality 3698 , category, code, subject, encounter, occurrence, detected, recordedDate, resultingEffect 3699 , location, seriousness, outcome, recorder, participant, study, expectedInResearchStudy 3700 , suspectEntity, contributingFactor, preventiveAction, mitigatingAction, supportingInfo 3701 , note); 3702 } 3703 3704 @Override 3705 public ResourceType getResourceType() { 3706 return ResourceType.AdverseEvent; 3707 } 3708 3709 /** 3710 * Search parameter: <b>actuality</b> 3711 * <p> 3712 * Description: <b>actual | potential</b><br> 3713 * Type: <b>token</b><br> 3714 * Path: <b>AdverseEvent.actuality</b><br> 3715 * </p> 3716 */ 3717 @SearchParamDefinition(name="actuality", path="AdverseEvent.actuality", description="actual | potential", type="token" ) 3718 public static final String SP_ACTUALITY = "actuality"; 3719 /** 3720 * <b>Fluent Client</b> search parameter constant for <b>actuality</b> 3721 * <p> 3722 * Description: <b>actual | potential</b><br> 3723 * Type: <b>token</b><br> 3724 * Path: <b>AdverseEvent.actuality</b><br> 3725 * </p> 3726 */ 3727 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTUALITY); 3728 3729 /** 3730 * Search parameter: <b>category</b> 3731 * <p> 3732 * Description: <b>wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site</b><br> 3733 * Type: <b>token</b><br> 3734 * Path: <b>AdverseEvent.category</b><br> 3735 * </p> 3736 */ 3737 @SearchParamDefinition(name="category", path="AdverseEvent.category", description="wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site", type="token" ) 3738 public static final String SP_CATEGORY = "category"; 3739 /** 3740 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3741 * <p> 3742 * Description: <b>wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site</b><br> 3743 * Type: <b>token</b><br> 3744 * Path: <b>AdverseEvent.category</b><br> 3745 * </p> 3746 */ 3747 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3748 3749 /** 3750 * Search parameter: <b>location</b> 3751 * <p> 3752 * Description: <b>Location where adverse event occurred</b><br> 3753 * Type: <b>reference</b><br> 3754 * Path: <b>AdverseEvent.location</b><br> 3755 * </p> 3756 */ 3757 @SearchParamDefinition(name="location", path="AdverseEvent.location", description="Location where adverse event occurred", type="reference", target={Location.class } ) 3758 public static final String SP_LOCATION = "location"; 3759 /** 3760 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3761 * <p> 3762 * Description: <b>Location where adverse event occurred</b><br> 3763 * Type: <b>reference</b><br> 3764 * Path: <b>AdverseEvent.location</b><br> 3765 * </p> 3766 */ 3767 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 3768 3769/** 3770 * Constant for fluent queries to be used to add include statements. Specifies 3771 * the path value of "<b>AdverseEvent:location</b>". 3772 */ 3773 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("AdverseEvent:location").toLocked(); 3774 3775 /** 3776 * Search parameter: <b>recorder</b> 3777 * <p> 3778 * Description: <b>Who recorded the adverse event</b><br> 3779 * Type: <b>reference</b><br> 3780 * Path: <b>AdverseEvent.recorder</b><br> 3781 * </p> 3782 */ 3783 @SearchParamDefinition(name="recorder", path="AdverseEvent.recorder", description="Who recorded the adverse event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, ResearchSubject.class } ) 3784 public static final String SP_RECORDER = "recorder"; 3785 /** 3786 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 3787 * <p> 3788 * Description: <b>Who recorded the adverse event</b><br> 3789 * Type: <b>reference</b><br> 3790 * Path: <b>AdverseEvent.recorder</b><br> 3791 * </p> 3792 */ 3793 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECORDER); 3794 3795/** 3796 * Constant for fluent queries to be used to add include statements. Specifies 3797 * the path value of "<b>AdverseEvent:recorder</b>". 3798 */ 3799 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include("AdverseEvent:recorder").toLocked(); 3800 3801 /** 3802 * Search parameter: <b>resultingeffect</b> 3803 * <p> 3804 * Description: <b>Effect on the subject due to this event</b><br> 3805 * Type: <b>reference</b><br> 3806 * Path: <b>AdverseEvent.resultingEffect</b><br> 3807 * </p> 3808 */ 3809 @SearchParamDefinition(name="resultingeffect", path="AdverseEvent.resultingEffect", description="Effect on the subject due to this event", type="reference", target={Condition.class, Observation.class } ) 3810 public static final String SP_RESULTINGEFFECT = "resultingeffect"; 3811 /** 3812 * <b>Fluent Client</b> search parameter constant for <b>resultingeffect</b> 3813 * <p> 3814 * Description: <b>Effect on the subject due to this event</b><br> 3815 * Type: <b>reference</b><br> 3816 * Path: <b>AdverseEvent.resultingEffect</b><br> 3817 * </p> 3818 */ 3819 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULTINGEFFECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESULTINGEFFECT); 3820 3821/** 3822 * Constant for fluent queries to be used to add include statements. Specifies 3823 * the path value of "<b>AdverseEvent:resultingeffect</b>". 3824 */ 3825 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULTINGEFFECT = new ca.uhn.fhir.model.api.Include("AdverseEvent:resultingeffect").toLocked(); 3826 3827 /** 3828 * Search parameter: <b>seriousness</b> 3829 * <p> 3830 * Description: <b>Seriousness or gravity of the event</b><br> 3831 * Type: <b>token</b><br> 3832 * Path: <b>AdverseEvent.seriousness</b><br> 3833 * </p> 3834 */ 3835 @SearchParamDefinition(name="seriousness", path="AdverseEvent.seriousness", description="Seriousness or gravity of the event", type="token" ) 3836 public static final String SP_SERIOUSNESS = "seriousness"; 3837 /** 3838 * <b>Fluent Client</b> search parameter constant for <b>seriousness</b> 3839 * <p> 3840 * Description: <b>Seriousness or gravity of the event</b><br> 3841 * Type: <b>token</b><br> 3842 * Path: <b>AdverseEvent.seriousness</b><br> 3843 * </p> 3844 */ 3845 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIOUSNESS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIOUSNESS); 3846 3847 /** 3848 * Search parameter: <b>status</b> 3849 * <p> 3850 * Description: <b>in-progress | completed | entered-in-error | unknown</b><br> 3851 * Type: <b>token</b><br> 3852 * Path: <b>AdverseEvent.status</b><br> 3853 * </p> 3854 */ 3855 @SearchParamDefinition(name="status", path="AdverseEvent.status", description="in-progress | completed | entered-in-error | unknown", type="token" ) 3856 public static final String SP_STATUS = "status"; 3857 /** 3858 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3859 * <p> 3860 * Description: <b>in-progress | completed | entered-in-error | unknown</b><br> 3861 * Type: <b>token</b><br> 3862 * Path: <b>AdverseEvent.status</b><br> 3863 * </p> 3864 */ 3865 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3866 3867 /** 3868 * Search parameter: <b>study</b> 3869 * <p> 3870 * Description: <b>Research study that the subject is enrolled in</b><br> 3871 * Type: <b>reference</b><br> 3872 * Path: <b>AdverseEvent.study</b><br> 3873 * </p> 3874 */ 3875 @SearchParamDefinition(name="study", path="AdverseEvent.study", description="Research study that the subject is enrolled in", type="reference", target={ResearchStudy.class } ) 3876 public static final String SP_STUDY = "study"; 3877 /** 3878 * <b>Fluent Client</b> search parameter constant for <b>study</b> 3879 * <p> 3880 * Description: <b>Research study that the subject is enrolled in</b><br> 3881 * Type: <b>reference</b><br> 3882 * Path: <b>AdverseEvent.study</b><br> 3883 * </p> 3884 */ 3885 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_STUDY); 3886 3887/** 3888 * Constant for fluent queries to be used to add include statements. Specifies 3889 * the path value of "<b>AdverseEvent:study</b>". 3890 */ 3891 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include("AdverseEvent:study").toLocked(); 3892 3893 /** 3894 * Search parameter: <b>subject</b> 3895 * <p> 3896 * Description: <b>Subject impacted by event</b><br> 3897 * Type: <b>reference</b><br> 3898 * Path: <b>AdverseEvent.subject</b><br> 3899 * </p> 3900 */ 3901 @SearchParamDefinition(name="subject", path="AdverseEvent.subject", description="Subject impacted by event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class, Practitioner.class, RelatedPerson.class, ResearchSubject.class } ) 3902 public static final String SP_SUBJECT = "subject"; 3903 /** 3904 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3905 * <p> 3906 * Description: <b>Subject impacted by event</b><br> 3907 * Type: <b>reference</b><br> 3908 * Path: <b>AdverseEvent.subject</b><br> 3909 * </p> 3910 */ 3911 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3912 3913/** 3914 * Constant for fluent queries to be used to add include statements. Specifies 3915 * the path value of "<b>AdverseEvent:subject</b>". 3916 */ 3917 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("AdverseEvent:subject").toLocked(); 3918 3919 /** 3920 * Search parameter: <b>substance</b> 3921 * <p> 3922 * Description: <b>Refers to the specific entity that caused the adverse event</b><br> 3923 * Type: <b>reference</b><br> 3924 * Path: <b>(AdverseEvent.suspectEntity.instance as Reference)</b><br> 3925 * </p> 3926 */ 3927 @SearchParamDefinition(name="substance", path="(AdverseEvent.suspectEntity.instance as Reference)", description="Refers to the specific entity that caused the adverse event", type="reference", target={BiologicallyDerivedProduct.class, Device.class, Immunization.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, Procedure.class, ResearchStudy.class, Substance.class } ) 3928 public static final String SP_SUBSTANCE = "substance"; 3929 /** 3930 * <b>Fluent Client</b> search parameter constant for <b>substance</b> 3931 * <p> 3932 * Description: <b>Refers to the specific entity that caused the adverse event</b><br> 3933 * Type: <b>reference</b><br> 3934 * Path: <b>(AdverseEvent.suspectEntity.instance as Reference)</b><br> 3935 * </p> 3936 */ 3937 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE); 3938 3939/** 3940 * Constant for fluent queries to be used to add include statements. Specifies 3941 * the path value of "<b>AdverseEvent:substance</b>". 3942 */ 3943 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE = new ca.uhn.fhir.model.api.Include("AdverseEvent:substance").toLocked(); 3944 3945 /** 3946 * Search parameter: <b>code</b> 3947 * <p> 3948 * Description: <b>Multiple Resources: 3949 3950* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3951* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3952* [AuditEvent](auditevent.html): More specific code for the event 3953* [Basic](basic.html): Kind of Resource 3954* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3955* [Condition](condition.html): Code for the condition 3956* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3957* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3958* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3959* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3960* [ImagingSelection](imagingselection.html): The imaging selection status 3961* [List](list.html): What the purpose of this list is 3962* [Medication](medication.html): Returns medications for a specific code 3963* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3964* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3965* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3966* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3967* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3968* [Observation](observation.html): The code of the observation type 3969* [Procedure](procedure.html): A code to identify a procedure 3970* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3971* [Task](task.html): Search by task code 3972</b><br> 3973 * Type: <b>token</b><br> 3974 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3975 * </p> 3976 */ 3977 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3978 public static final String SP_CODE = "code"; 3979 /** 3980 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3981 * <p> 3982 * Description: <b>Multiple Resources: 3983 3984* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3985* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3986* [AuditEvent](auditevent.html): More specific code for the event 3987* [Basic](basic.html): Kind of Resource 3988* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3989* [Condition](condition.html): Code for the condition 3990* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3991* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3992* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3993* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3994* [ImagingSelection](imagingselection.html): The imaging selection status 3995* [List](list.html): What the purpose of this list is 3996* [Medication](medication.html): Returns medications for a specific code 3997* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3998* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3999* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4000* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4001* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4002* [Observation](observation.html): The code of the observation type 4003* [Procedure](procedure.html): A code to identify a procedure 4004* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4005* [Task](task.html): Search by task code 4006</b><br> 4007 * Type: <b>token</b><br> 4008 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4009 * </p> 4010 */ 4011 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4012 4013 /** 4014 * Search parameter: <b>date</b> 4015 * <p> 4016 * Description: <b>Multiple Resources: 4017 4018* [AdverseEvent](adverseevent.html): When the event occurred 4019* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4020* [Appointment](appointment.html): Appointment date/time. 4021* [AuditEvent](auditevent.html): Time when the event was recorded 4022* [CarePlan](careplan.html): Time period plan covers 4023* [CareTeam](careteam.html): A date within the coverage time period. 4024* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4025* [Composition](composition.html): Composition editing time 4026* [Consent](consent.html): When consent was agreed to 4027* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4028* [DocumentReference](documentreference.html): When this document reference was created 4029* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4030* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4031* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4032* [Flag](flag.html): Time period when flag is active 4033* [Immunization](immunization.html): Vaccination (non)-Administration Date 4034* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4035* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4036* [Invoice](invoice.html): Invoice date / posting date 4037* [List](list.html): When the list was prepared 4038* [MeasureReport](measurereport.html): The date of the measure report 4039* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4040* [Observation](observation.html): Clinically relevant time/time-period for observation 4041* [Procedure](procedure.html): When the procedure occurred or is occurring 4042* [ResearchSubject](researchsubject.html): Start and end of participation 4043* [RiskAssessment](riskassessment.html): When was assessment made? 4044* [SupplyRequest](supplyrequest.html): When the request was made 4045</b><br> 4046 * Type: <b>date</b><br> 4047 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4048 * </p> 4049 */ 4050 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4051 public static final String SP_DATE = "date"; 4052 /** 4053 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4054 * <p> 4055 * Description: <b>Multiple Resources: 4056 4057* [AdverseEvent](adverseevent.html): When the event occurred 4058* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4059* [Appointment](appointment.html): Appointment date/time. 4060* [AuditEvent](auditevent.html): Time when the event was recorded 4061* [CarePlan](careplan.html): Time period plan covers 4062* [CareTeam](careteam.html): A date within the coverage time period. 4063* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4064* [Composition](composition.html): Composition editing time 4065* [Consent](consent.html): When consent was agreed to 4066* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4067* [DocumentReference](documentreference.html): When this document reference was created 4068* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4069* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4070* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4071* [Flag](flag.html): Time period when flag is active 4072* [Immunization](immunization.html): Vaccination (non)-Administration Date 4073* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4074* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4075* [Invoice](invoice.html): Invoice date / posting date 4076* [List](list.html): When the list was prepared 4077* [MeasureReport](measurereport.html): The date of the measure report 4078* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4079* [Observation](observation.html): Clinically relevant time/time-period for observation 4080* [Procedure](procedure.html): When the procedure occurred or is occurring 4081* [ResearchSubject](researchsubject.html): Start and end of participation 4082* [RiskAssessment](riskassessment.html): When was assessment made? 4083* [SupplyRequest](supplyrequest.html): When the request was made 4084</b><br> 4085 * Type: <b>date</b><br> 4086 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4087 * </p> 4088 */ 4089 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4090 4091 /** 4092 * Search parameter: <b>identifier</b> 4093 * <p> 4094 * Description: <b>Multiple Resources: 4095 4096* [Account](account.html): Account number 4097* [AdverseEvent](adverseevent.html): Business identifier for the event 4098* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4099* [Appointment](appointment.html): An Identifier of the Appointment 4100* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4101* [Basic](basic.html): Business identifier 4102* [BodyStructure](bodystructure.html): Bodystructure identifier 4103* [CarePlan](careplan.html): External Ids for this plan 4104* [CareTeam](careteam.html): External Ids for this team 4105* [ChargeItem](chargeitem.html): Business Identifier for item 4106* [Claim](claim.html): The primary identifier of the financial resource 4107* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4108* [ClinicalImpression](clinicalimpression.html): Business identifier 4109* [Communication](communication.html): Unique identifier 4110* [CommunicationRequest](communicationrequest.html): Unique identifier 4111* [Composition](composition.html): Version-independent identifier for the Composition 4112* [Condition](condition.html): A unique identifier of the condition record 4113* [Consent](consent.html): Identifier for this record (external references) 4114* [Contract](contract.html): The identity of the contract 4115* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4116* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4117* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4118* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4119* [DeviceRequest](devicerequest.html): Business identifier for request/order 4120* [DeviceUsage](deviceusage.html): Search by identifier 4121* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4122* [DocumentReference](documentreference.html): Identifier of the attachment binary 4123* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4124* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4125* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4126* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4127* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4128* [Flag](flag.html): Business identifier 4129* [Goal](goal.html): External Ids for this goal 4130* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4131* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4132* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4133* [Immunization](immunization.html): Business identifier 4134* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4135* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4136* [Invoice](invoice.html): Business Identifier for item 4137* [List](list.html): Business identifier 4138* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4139* [Medication](medication.html): Returns medications with this external identifier 4140* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4141* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4142* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4143* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4144* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4145* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4146* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4147* [Observation](observation.html): The unique id for a particular observation 4148* [Person](person.html): A person Identifier 4149* [Procedure](procedure.html): A unique identifier for a procedure 4150* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4151* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4152* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4153* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4154* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4155* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4156* [Specimen](specimen.html): The unique identifier associated with the specimen 4157* [SupplyDelivery](supplydelivery.html): External identifier 4158* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4159* [Task](task.html): Search for a task instance by its business identifier 4160* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4161</b><br> 4162 * Type: <b>token</b><br> 4163 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4164 * </p> 4165 */ 4166 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4167 public static final String SP_IDENTIFIER = "identifier"; 4168 /** 4169 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4170 * <p> 4171 * Description: <b>Multiple Resources: 4172 4173* [Account](account.html): Account number 4174* [AdverseEvent](adverseevent.html): Business identifier for the event 4175* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4176* [Appointment](appointment.html): An Identifier of the Appointment 4177* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4178* [Basic](basic.html): Business identifier 4179* [BodyStructure](bodystructure.html): Bodystructure identifier 4180* [CarePlan](careplan.html): External Ids for this plan 4181* [CareTeam](careteam.html): External Ids for this team 4182* [ChargeItem](chargeitem.html): Business Identifier for item 4183* [Claim](claim.html): The primary identifier of the financial resource 4184* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4185* [ClinicalImpression](clinicalimpression.html): Business identifier 4186* [Communication](communication.html): Unique identifier 4187* [CommunicationRequest](communicationrequest.html): Unique identifier 4188* [Composition](composition.html): Version-independent identifier for the Composition 4189* [Condition](condition.html): A unique identifier of the condition record 4190* [Consent](consent.html): Identifier for this record (external references) 4191* [Contract](contract.html): The identity of the contract 4192* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4193* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4194* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4195* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4196* [DeviceRequest](devicerequest.html): Business identifier for request/order 4197* [DeviceUsage](deviceusage.html): Search by identifier 4198* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4199* [DocumentReference](documentreference.html): Identifier of the attachment binary 4200* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4201* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4202* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4203* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4204* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4205* [Flag](flag.html): Business identifier 4206* [Goal](goal.html): External Ids for this goal 4207* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4208* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4209* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4210* [Immunization](immunization.html): Business identifier 4211* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4212* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4213* [Invoice](invoice.html): Business Identifier for item 4214* [List](list.html): Business identifier 4215* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4216* [Medication](medication.html): Returns medications with this external identifier 4217* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4218* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4219* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4220* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4221* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4222* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4223* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4224* [Observation](observation.html): The unique id for a particular observation 4225* [Person](person.html): A person Identifier 4226* [Procedure](procedure.html): A unique identifier for a procedure 4227* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4228* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4229* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4230* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4231* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4232* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4233* [Specimen](specimen.html): The unique identifier associated with the specimen 4234* [SupplyDelivery](supplydelivery.html): External identifier 4235* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4236* [Task](task.html): Search for a task instance by its business identifier 4237* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4238</b><br> 4239 * Type: <b>token</b><br> 4240 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4241 * </p> 4242 */ 4243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4244 4245 /** 4246 * Search parameter: <b>patient</b> 4247 * <p> 4248 * Description: <b>Multiple Resources: 4249 4250* [Account](account.html): The entity that caused the expenses 4251* [AdverseEvent](adverseevent.html): Subject impacted by event 4252* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4253* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4254* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4255* [AuditEvent](auditevent.html): Where the activity involved patient data 4256* [Basic](basic.html): Identifies the focus of this resource 4257* [BodyStructure](bodystructure.html): Who this is about 4258* [CarePlan](careplan.html): Who the care plan is for 4259* [CareTeam](careteam.html): Who care team is for 4260* [ChargeItem](chargeitem.html): Individual service was done for/to 4261* [Claim](claim.html): Patient receiving the products or services 4262* [ClaimResponse](claimresponse.html): The subject of care 4263* [ClinicalImpression](clinicalimpression.html): Patient assessed 4264* [Communication](communication.html): Focus of message 4265* [CommunicationRequest](communicationrequest.html): Focus of message 4266* [Composition](composition.html): Who and/or what the composition is about 4267* [Condition](condition.html): Who has the condition? 4268* [Consent](consent.html): Who the consent applies to 4269* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4270* [Coverage](coverage.html): Retrieve coverages for a patient 4271* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4272* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4273* [DetectedIssue](detectedissue.html): Associated patient 4274* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4275* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4276* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4277* [DocumentReference](documentreference.html): Who/what is the subject of the document 4278* [Encounter](encounter.html): The patient present at the encounter 4279* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4280* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4281* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4282* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4283* [Flag](flag.html): The identity of a subject to list flags for 4284* [Goal](goal.html): Who this goal is intended for 4285* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4286* [ImagingSelection](imagingselection.html): Who the study is about 4287* [ImagingStudy](imagingstudy.html): Who the study is about 4288* [Immunization](immunization.html): The patient for the vaccination record 4289* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4290* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4291* [Invoice](invoice.html): Recipient(s) of goods and services 4292* [List](list.html): If all resources have the same subject 4293* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4294* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4295* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4296* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4297* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4298* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4299* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4300* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4301* [Observation](observation.html): The subject that the observation is about (if patient) 4302* [Person](person.html): The Person links to this Patient 4303* [Procedure](procedure.html): Search by subject - a patient 4304* [Provenance](provenance.html): Where the activity involved patient data 4305* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4306* [RelatedPerson](relatedperson.html): The patient this related person is related to 4307* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4308* [ResearchSubject](researchsubject.html): Who or what is part of study 4309* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4310* [ServiceRequest](servicerequest.html): Search by subject - a patient 4311* [Specimen](specimen.html): The patient the specimen comes from 4312* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4313* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4314* [Task](task.html): Search by patient 4315* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4316</b><br> 4317 * Type: <b>reference</b><br> 4318 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4319 * </p> 4320 */ 4321 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4322 public static final String SP_PATIENT = "patient"; 4323 /** 4324 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4325 * <p> 4326 * Description: <b>Multiple Resources: 4327 4328* [Account](account.html): The entity that caused the expenses 4329* [AdverseEvent](adverseevent.html): Subject impacted by event 4330* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4331* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4332* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4333* [AuditEvent](auditevent.html): Where the activity involved patient data 4334* [Basic](basic.html): Identifies the focus of this resource 4335* [BodyStructure](bodystructure.html): Who this is about 4336* [CarePlan](careplan.html): Who the care plan is for 4337* [CareTeam](careteam.html): Who care team is for 4338* [ChargeItem](chargeitem.html): Individual service was done for/to 4339* [Claim](claim.html): Patient receiving the products or services 4340* [ClaimResponse](claimresponse.html): The subject of care 4341* [ClinicalImpression](clinicalimpression.html): Patient assessed 4342* [Communication](communication.html): Focus of message 4343* [CommunicationRequest](communicationrequest.html): Focus of message 4344* [Composition](composition.html): Who and/or what the composition is about 4345* [Condition](condition.html): Who has the condition? 4346* [Consent](consent.html): Who the consent applies to 4347* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4348* [Coverage](coverage.html): Retrieve coverages for a patient 4349* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4350* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4351* [DetectedIssue](detectedissue.html): Associated patient 4352* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4353* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4354* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4355* [DocumentReference](documentreference.html): Who/what is the subject of the document 4356* [Encounter](encounter.html): The patient present at the encounter 4357* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4358* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4359* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4360* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4361* [Flag](flag.html): The identity of a subject to list flags for 4362* [Goal](goal.html): Who this goal is intended for 4363* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4364* [ImagingSelection](imagingselection.html): Who the study is about 4365* [ImagingStudy](imagingstudy.html): Who the study is about 4366* [Immunization](immunization.html): The patient for the vaccination record 4367* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4368* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4369* [Invoice](invoice.html): Recipient(s) of goods and services 4370* [List](list.html): If all resources have the same subject 4371* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4372* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4373* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4374* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4375* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4376* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4377* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4378* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4379* [Observation](observation.html): The subject that the observation is about (if patient) 4380* [Person](person.html): The Person links to this Patient 4381* [Procedure](procedure.html): Search by subject - a patient 4382* [Provenance](provenance.html): Where the activity involved patient data 4383* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4384* [RelatedPerson](relatedperson.html): The patient this related person is related to 4385* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4386* [ResearchSubject](researchsubject.html): Who or what is part of study 4387* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4388* [ServiceRequest](servicerequest.html): Search by subject - a patient 4389* [Specimen](specimen.html): The patient the specimen comes from 4390* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4391* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4392* [Task](task.html): Search by patient 4393* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4394</b><br> 4395 * Type: <b>reference</b><br> 4396 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4397 * </p> 4398 */ 4399 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4400 4401/** 4402 * Constant for fluent queries to be used to add include statements. Specifies 4403 * the path value of "<b>AdverseEvent:patient</b>". 4404 */ 4405 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AdverseEvent:patient").toLocked(); 4406 4407 4408} 4409