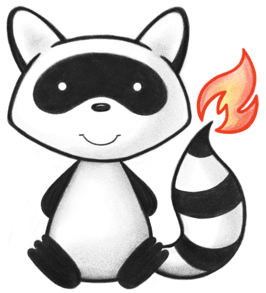
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An event (i.e. any change to current patient status) that may be related to unintended effects on a patient or research participant. The unintended effects may require additional monitoring, treatment, hospitalization, or may result in death. The AdverseEvent resource also extends to potential or avoided events that could have had such effects. There are two major domains where the AdverseEvent resource is expected to be used. One is in clinical care reported adverse events and the other is in reporting adverse events in clinical research trial management. Adverse events can be reported by healthcare providers, patients, caregivers or by medical products manufacturers. Given the differences between these two concepts, we recommend consulting the domain specific implementation guides when implementing the AdverseEvent Resource. The implementation guides include specific extensions, value sets and constraints. 052 */ 053@ResourceDef(name="AdverseEvent", profile="http://hl7.org/fhir/StructureDefinition/AdverseEvent") 054public class AdverseEvent extends DomainResource { 055 056 public enum AdverseEventActuality { 057 /** 058 * The adverse event actually happened regardless of whether anyone was affected or harmed. 059 */ 060 ACTUAL, 061 /** 062 * A potential adverse event. 063 */ 064 POTENTIAL, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static AdverseEventActuality fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("actual".equals(codeString)) 073 return ACTUAL; 074 if ("potential".equals(codeString)) 075 return POTENTIAL; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown AdverseEventActuality code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case ACTUAL: return "actual"; 084 case POTENTIAL: return "potential"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case ACTUAL: return "http://hl7.org/fhir/adverse-event-actuality"; 092 case POTENTIAL: return "http://hl7.org/fhir/adverse-event-actuality"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case ACTUAL: return "The adverse event actually happened regardless of whether anyone was affected or harmed."; 100 case POTENTIAL: return "A potential adverse event."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case ACTUAL: return "Adverse Event"; 108 case POTENTIAL: return "Potential Adverse Event"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class AdverseEventActualityEnumFactory implements EnumFactory<AdverseEventActuality> { 116 public AdverseEventActuality fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("actual".equals(codeString)) 121 return AdverseEventActuality.ACTUAL; 122 if ("potential".equals(codeString)) 123 return AdverseEventActuality.POTENTIAL; 124 throw new IllegalArgumentException("Unknown AdverseEventActuality code '"+codeString+"'"); 125 } 126 public Enumeration<AdverseEventActuality> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.NULL, code); 134 if ("actual".equals(codeString)) 135 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.ACTUAL, code); 136 if ("potential".equals(codeString)) 137 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.POTENTIAL, code); 138 throw new FHIRException("Unknown AdverseEventActuality code '"+codeString+"'"); 139 } 140 public String toCode(AdverseEventActuality code) { 141 if (code == AdverseEventActuality.NULL) 142 return null; 143 if (code == AdverseEventActuality.ACTUAL) 144 return "actual"; 145 if (code == AdverseEventActuality.POTENTIAL) 146 return "potential"; 147 return "?"; 148 } 149 public String toSystem(AdverseEventActuality code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum AdverseEventStatus { 155 /** 156 * The event is currently occurring. 157 */ 158 INPROGRESS, 159 /** 160 * The event has now concluded. 161 */ 162 COMPLETED, 163 /** 164 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".). 165 */ 166 ENTEREDINERROR, 167 /** 168 * The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 169 */ 170 UNKNOWN, 171 /** 172 * added to help the parsers with the generic types 173 */ 174 NULL; 175 public static AdverseEventStatus fromCode(String codeString) throws FHIRException { 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("in-progress".equals(codeString)) 179 return INPROGRESS; 180 if ("completed".equals(codeString)) 181 return COMPLETED; 182 if ("entered-in-error".equals(codeString)) 183 return ENTEREDINERROR; 184 if ("unknown".equals(codeString)) 185 return UNKNOWN; 186 if (Configuration.isAcceptInvalidEnums()) 187 return null; 188 else 189 throw new FHIRException("Unknown AdverseEventStatus code '"+codeString+"'"); 190 } 191 public String toCode() { 192 switch (this) { 193 case INPROGRESS: return "in-progress"; 194 case COMPLETED: return "completed"; 195 case ENTEREDINERROR: return "entered-in-error"; 196 case UNKNOWN: return "unknown"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getSystem() { 202 switch (this) { 203 case INPROGRESS: return "http://hl7.org/fhir/event-status"; 204 case COMPLETED: return "http://hl7.org/fhir/event-status"; 205 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 206 case UNKNOWN: return "http://hl7.org/fhir/event-status"; 207 case NULL: return null; 208 default: return "?"; 209 } 210 } 211 public String getDefinition() { 212 switch (this) { 213 case INPROGRESS: return "The event is currently occurring."; 214 case COMPLETED: return "The event has now concluded."; 215 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 216 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 public String getDisplay() { 222 switch (this) { 223 case INPROGRESS: return "In Progress"; 224 case COMPLETED: return "Completed"; 225 case ENTEREDINERROR: return "Entered in Error"; 226 case UNKNOWN: return "Unknown"; 227 case NULL: return null; 228 default: return "?"; 229 } 230 } 231 } 232 233 public static class AdverseEventStatusEnumFactory implements EnumFactory<AdverseEventStatus> { 234 public AdverseEventStatus fromCode(String codeString) throws IllegalArgumentException { 235 if (codeString == null || "".equals(codeString)) 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("in-progress".equals(codeString)) 239 return AdverseEventStatus.INPROGRESS; 240 if ("completed".equals(codeString)) 241 return AdverseEventStatus.COMPLETED; 242 if ("entered-in-error".equals(codeString)) 243 return AdverseEventStatus.ENTEREDINERROR; 244 if ("unknown".equals(codeString)) 245 return AdverseEventStatus.UNKNOWN; 246 throw new IllegalArgumentException("Unknown AdverseEventStatus code '"+codeString+"'"); 247 } 248 public Enumeration<AdverseEventStatus> fromType(PrimitiveType<?> code) throws FHIRException { 249 if (code == null) 250 return null; 251 if (code.isEmpty()) 252 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.NULL, code); 253 String codeString = ((PrimitiveType) code).asStringValue(); 254 if (codeString == null || "".equals(codeString)) 255 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.NULL, code); 256 if ("in-progress".equals(codeString)) 257 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.INPROGRESS, code); 258 if ("completed".equals(codeString)) 259 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.COMPLETED, code); 260 if ("entered-in-error".equals(codeString)) 261 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.ENTEREDINERROR, code); 262 if ("unknown".equals(codeString)) 263 return new Enumeration<AdverseEventStatus>(this, AdverseEventStatus.UNKNOWN, code); 264 throw new FHIRException("Unknown AdverseEventStatus code '"+codeString+"'"); 265 } 266 public String toCode(AdverseEventStatus code) { 267 if (code == AdverseEventStatus.NULL) 268 return null; 269 if (code == AdverseEventStatus.INPROGRESS) 270 return "in-progress"; 271 if (code == AdverseEventStatus.COMPLETED) 272 return "completed"; 273 if (code == AdverseEventStatus.ENTEREDINERROR) 274 return "entered-in-error"; 275 if (code == AdverseEventStatus.UNKNOWN) 276 return "unknown"; 277 return "?"; 278 } 279 public String toSystem(AdverseEventStatus code) { 280 return code.getSystem(); 281 } 282 } 283 284 @Block() 285 public static class AdverseEventParticipantComponent extends BackboneElement implements IBaseBackboneElement { 286 /** 287 * Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant. 288 */ 289 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 290 @Description(shortDefinition="Type of involvement", formalDefinition="Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant." ) 291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-participant-function") 292 protected CodeableConcept function; 293 294 /** 295 * Indicates who or what participated in the event. 296 */ 297 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class, ResearchSubject.class}, order=2, min=1, max=1, modifier=false, summary=true) 298 @Description(shortDefinition="Who was involved in the adverse event or the potential adverse event", formalDefinition="Indicates who or what participated in the event." ) 299 protected Reference actor; 300 301 private static final long serialVersionUID = -576943815L; 302 303 /** 304 * Constructor 305 */ 306 public AdverseEventParticipantComponent() { 307 super(); 308 } 309 310 /** 311 * Constructor 312 */ 313 public AdverseEventParticipantComponent(Reference actor) { 314 super(); 315 this.setActor(actor); 316 } 317 318 /** 319 * @return {@link #function} (Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.) 320 */ 321 public CodeableConcept getFunction() { 322 if (this.function == null) 323 if (Configuration.errorOnAutoCreate()) 324 throw new Error("Attempt to auto-create AdverseEventParticipantComponent.function"); 325 else if (Configuration.doAutoCreate()) 326 this.function = new CodeableConcept(); // cc 327 return this.function; 328 } 329 330 public boolean hasFunction() { 331 return this.function != null && !this.function.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #function} (Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.) 336 */ 337 public AdverseEventParticipantComponent setFunction(CodeableConcept value) { 338 this.function = value; 339 return this; 340 } 341 342 /** 343 * @return {@link #actor} (Indicates who or what participated in the event.) 344 */ 345 public Reference getActor() { 346 if (this.actor == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create AdverseEventParticipantComponent.actor"); 349 else if (Configuration.doAutoCreate()) 350 this.actor = new Reference(); // cc 351 return this.actor; 352 } 353 354 public boolean hasActor() { 355 return this.actor != null && !this.actor.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #actor} (Indicates who or what participated in the event.) 360 */ 361 public AdverseEventParticipantComponent setActor(Reference value) { 362 this.actor = value; 363 return this; 364 } 365 366 protected void listChildren(List<Property> children) { 367 super.listChildren(children); 368 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.", 0, 1, function)); 369 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson|ResearchSubject)", "Indicates who or what participated in the event.", 0, 1, actor)); 370 } 371 372 @Override 373 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 374 switch (_hash) { 375 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the adverse event, such as contributor or informant.", 0, 1, function); 376 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson|ResearchSubject)", "Indicates who or what participated in the event.", 0, 1, actor); 377 default: return super.getNamedProperty(_hash, _name, _checkValid); 378 } 379 380 } 381 382 @Override 383 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 384 switch (hash) { 385 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 386 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 387 default: return super.getProperty(hash, name, checkValid); 388 } 389 390 } 391 392 @Override 393 public Base setProperty(int hash, String name, Base value) throws FHIRException { 394 switch (hash) { 395 case 1380938712: // function 396 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 397 return value; 398 case 92645877: // actor 399 this.actor = TypeConvertor.castToReference(value); // Reference 400 return value; 401 default: return super.setProperty(hash, name, value); 402 } 403 404 } 405 406 @Override 407 public Base setProperty(String name, Base value) throws FHIRException { 408 if (name.equals("function")) { 409 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 410 } else if (name.equals("actor")) { 411 this.actor = TypeConvertor.castToReference(value); // Reference 412 } else 413 return super.setProperty(name, value); 414 return value; 415 } 416 417 @Override 418 public void removeChild(String name, Base value) throws FHIRException { 419 if (name.equals("function")) { 420 this.function = null; 421 } else if (name.equals("actor")) { 422 this.actor = null; 423 } else 424 super.removeChild(name, value); 425 426 } 427 428 @Override 429 public Base makeProperty(int hash, String name) throws FHIRException { 430 switch (hash) { 431 case 1380938712: return getFunction(); 432 case 92645877: return getActor(); 433 default: return super.makeProperty(hash, name); 434 } 435 436 } 437 438 @Override 439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 440 switch (hash) { 441 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 442 case 92645877: /*actor*/ return new String[] {"Reference"}; 443 default: return super.getTypesForProperty(hash, name); 444 } 445 446 } 447 448 @Override 449 public Base addChild(String name) throws FHIRException { 450 if (name.equals("function")) { 451 this.function = new CodeableConcept(); 452 return this.function; 453 } 454 else if (name.equals("actor")) { 455 this.actor = new Reference(); 456 return this.actor; 457 } 458 else 459 return super.addChild(name); 460 } 461 462 public AdverseEventParticipantComponent copy() { 463 AdverseEventParticipantComponent dst = new AdverseEventParticipantComponent(); 464 copyValues(dst); 465 return dst; 466 } 467 468 public void copyValues(AdverseEventParticipantComponent dst) { 469 super.copyValues(dst); 470 dst.function = function == null ? null : function.copy(); 471 dst.actor = actor == null ? null : actor.copy(); 472 } 473 474 @Override 475 public boolean equalsDeep(Base other_) { 476 if (!super.equalsDeep(other_)) 477 return false; 478 if (!(other_ instanceof AdverseEventParticipantComponent)) 479 return false; 480 AdverseEventParticipantComponent o = (AdverseEventParticipantComponent) other_; 481 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 482 } 483 484 @Override 485 public boolean equalsShallow(Base other_) { 486 if (!super.equalsShallow(other_)) 487 return false; 488 if (!(other_ instanceof AdverseEventParticipantComponent)) 489 return false; 490 AdverseEventParticipantComponent o = (AdverseEventParticipantComponent) other_; 491 return true; 492 } 493 494 public boolean isEmpty() { 495 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 496 } 497 498 public String fhirType() { 499 return "AdverseEvent.participant"; 500 501 } 502 503 } 504 505 @Block() 506 public static class AdverseEventSuspectEntityComponent extends BackboneElement implements IBaseBackboneElement { 507 /** 508 * Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device. 509 */ 510 @Child(name = "instance", type = {CodeableConcept.class, Immunization.class, Procedure.class, Substance.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, Device.class, BiologicallyDerivedProduct.class, ResearchStudy.class}, order=1, min=1, max=1, modifier=false, summary=true) 511 @Description(shortDefinition="Refers to the specific entity that caused the adverse event", formalDefinition="Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device." ) 512 protected DataType instance; 513 514 /** 515 * Information on the possible cause of the event. 516 */ 517 @Child(name = "causality", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 518 @Description(shortDefinition="Information on the possible cause of the event", formalDefinition="Information on the possible cause of the event." ) 519 protected AdverseEventSuspectEntityCausalityComponent causality; 520 521 private static final long serialVersionUID = -1455097004L; 522 523 /** 524 * Constructor 525 */ 526 public AdverseEventSuspectEntityComponent() { 527 super(); 528 } 529 530 /** 531 * Constructor 532 */ 533 public AdverseEventSuspectEntityComponent(DataType instance) { 534 super(); 535 this.setInstance(instance); 536 } 537 538 /** 539 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 540 */ 541 public DataType getInstance() { 542 return this.instance; 543 } 544 545 /** 546 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 547 */ 548 public CodeableConcept getInstanceCodeableConcept() throws FHIRException { 549 if (this.instance == null) 550 this.instance = new CodeableConcept(); 551 if (!(this.instance instanceof CodeableConcept)) 552 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.instance.getClass().getName()+" was encountered"); 553 return (CodeableConcept) this.instance; 554 } 555 556 public boolean hasInstanceCodeableConcept() { 557 return this != null && this.instance instanceof CodeableConcept; 558 } 559 560 /** 561 * @return {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 562 */ 563 public Reference getInstanceReference() throws FHIRException { 564 if (this.instance == null) 565 this.instance = new Reference(); 566 if (!(this.instance instanceof Reference)) 567 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.instance.getClass().getName()+" was encountered"); 568 return (Reference) this.instance; 569 } 570 571 public boolean hasInstanceReference() { 572 return this != null && this.instance instanceof Reference; 573 } 574 575 public boolean hasInstance() { 576 return this.instance != null && !this.instance.isEmpty(); 577 } 578 579 /** 580 * @param value {@link #instance} (Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.) 581 */ 582 public AdverseEventSuspectEntityComponent setInstance(DataType value) { 583 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 584 throw new FHIRException("Not the right type for AdverseEvent.suspectEntity.instance[x]: "+value.fhirType()); 585 this.instance = value; 586 return this; 587 } 588 589 /** 590 * @return {@link #causality} (Information on the possible cause of the event.) 591 */ 592 public AdverseEventSuspectEntityCausalityComponent getCausality() { 593 if (this.causality == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.causality"); 596 else if (Configuration.doAutoCreate()) 597 this.causality = new AdverseEventSuspectEntityCausalityComponent(); // cc 598 return this.causality; 599 } 600 601 public boolean hasCausality() { 602 return this.causality != null && !this.causality.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #causality} (Information on the possible cause of the event.) 607 */ 608 public AdverseEventSuspectEntityComponent setCausality(AdverseEventSuspectEntityCausalityComponent value) { 609 this.causality = value; 610 return this; 611 } 612 613 protected void listChildren(List<Property> children) { 614 super.listChildren(children); 615 children.add(new Property("instance[x]", "CodeableConcept|Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance)); 616 children.add(new Property("causality", "", "Information on the possible cause of the event.", 0, 1, causality)); 617 } 618 619 @Override 620 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 621 switch (_hash) { 622 case -2101998645: /*instance[x]*/ return new Property("instance[x]", "CodeableConcept|Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 623 case 555127957: /*instance*/ return new Property("instance[x]", "CodeableConcept|Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 624 case 697546316: /*instanceCodeableConcept*/ return new Property("instance[x]", "CodeableConcept", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 625 case -1675877834: /*instanceReference*/ return new Property("instance[x]", "Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device|BiologicallyDerivedProduct|ResearchStudy)", "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 0, 1, instance); 626 case -1446450521: /*causality*/ return new Property("causality", "", "Information on the possible cause of the event.", 0, 1, causality); 627 default: return super.getNamedProperty(_hash, _name, _checkValid); 628 } 629 630 } 631 632 @Override 633 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 634 switch (hash) { 635 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // DataType 636 case -1446450521: /*causality*/ return this.causality == null ? new Base[0] : new Base[] {this.causality}; // AdverseEventSuspectEntityCausalityComponent 637 default: return super.getProperty(hash, name, checkValid); 638 } 639 640 } 641 642 @Override 643 public Base setProperty(int hash, String name, Base value) throws FHIRException { 644 switch (hash) { 645 case 555127957: // instance 646 this.instance = TypeConvertor.castToType(value); // DataType 647 return value; 648 case -1446450521: // causality 649 this.causality = (AdverseEventSuspectEntityCausalityComponent) value; // AdverseEventSuspectEntityCausalityComponent 650 return value; 651 default: return super.setProperty(hash, name, value); 652 } 653 654 } 655 656 @Override 657 public Base setProperty(String name, Base value) throws FHIRException { 658 if (name.equals("instance[x]")) { 659 this.instance = TypeConvertor.castToType(value); // DataType 660 } else if (name.equals("causality")) { 661 this.causality = (AdverseEventSuspectEntityCausalityComponent) value; // AdverseEventSuspectEntityCausalityComponent 662 } else 663 return super.setProperty(name, value); 664 return value; 665 } 666 667 @Override 668 public void removeChild(String name, Base value) throws FHIRException { 669 if (name.equals("instance[x]")) { 670 this.instance = null; 671 } else if (name.equals("causality")) { 672 this.causality = (AdverseEventSuspectEntityCausalityComponent) value; // AdverseEventSuspectEntityCausalityComponent 673 } else 674 super.removeChild(name, value); 675 676 } 677 678 @Override 679 public Base makeProperty(int hash, String name) throws FHIRException { 680 switch (hash) { 681 case -2101998645: return getInstance(); 682 case 555127957: return getInstance(); 683 case -1446450521: return getCausality(); 684 default: return super.makeProperty(hash, name); 685 } 686 687 } 688 689 @Override 690 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case 555127957: /*instance*/ return new String[] {"CodeableConcept", "Reference"}; 693 case -1446450521: /*causality*/ return new String[] {}; 694 default: return super.getTypesForProperty(hash, name); 695 } 696 697 } 698 699 @Override 700 public Base addChild(String name) throws FHIRException { 701 if (name.equals("instanceCodeableConcept")) { 702 this.instance = new CodeableConcept(); 703 return this.instance; 704 } 705 else if (name.equals("instanceReference")) { 706 this.instance = new Reference(); 707 return this.instance; 708 } 709 else if (name.equals("causality")) { 710 this.causality = new AdverseEventSuspectEntityCausalityComponent(); 711 return this.causality; 712 } 713 else 714 return super.addChild(name); 715 } 716 717 public AdverseEventSuspectEntityComponent copy() { 718 AdverseEventSuspectEntityComponent dst = new AdverseEventSuspectEntityComponent(); 719 copyValues(dst); 720 return dst; 721 } 722 723 public void copyValues(AdverseEventSuspectEntityComponent dst) { 724 super.copyValues(dst); 725 dst.instance = instance == null ? null : instance.copy(); 726 dst.causality = causality == null ? null : causality.copy(); 727 } 728 729 @Override 730 public boolean equalsDeep(Base other_) { 731 if (!super.equalsDeep(other_)) 732 return false; 733 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 734 return false; 735 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 736 return compareDeep(instance, o.instance, true) && compareDeep(causality, o.causality, true); 737 } 738 739 @Override 740 public boolean equalsShallow(Base other_) { 741 if (!super.equalsShallow(other_)) 742 return false; 743 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 744 return false; 745 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 746 return true; 747 } 748 749 public boolean isEmpty() { 750 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(instance, causality); 751 } 752 753 public String fhirType() { 754 return "AdverseEvent.suspectEntity"; 755 756 } 757 758 } 759 760 @Block() 761 public static class AdverseEventSuspectEntityCausalityComponent extends BackboneElement implements IBaseBackboneElement { 762 /** 763 * The method of evaluating the relatedness of the suspected entity to the event. 764 */ 765 @Child(name = "assessmentMethod", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 766 @Description(shortDefinition="Method of evaluating the relatedness of the suspected entity to the event", formalDefinition="The method of evaluating the relatedness of the suspected entity to the event." ) 767 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-method") 768 protected CodeableConcept assessmentMethod; 769 770 /** 771 * The result of the assessment regarding the relatedness of the suspected entity to the event. 772 */ 773 @Child(name = "entityRelatedness", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 774 @Description(shortDefinition="Result of the assessment regarding the relatedness of the suspected entity to the event", formalDefinition="The result of the assessment regarding the relatedness of the suspected entity to the event." ) 775 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-causality-assess") 776 protected CodeableConcept entityRelatedness; 777 778 /** 779 * The author of the information on the possible cause of the event. 780 */ 781 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, ResearchSubject.class}, order=3, min=0, max=1, modifier=false, summary=true) 782 @Description(shortDefinition="Author of the information on the possible cause of the event", formalDefinition="The author of the information on the possible cause of the event." ) 783 protected Reference author; 784 785 private static final long serialVersionUID = 486112728L; 786 787 /** 788 * Constructor 789 */ 790 public AdverseEventSuspectEntityCausalityComponent() { 791 super(); 792 } 793 794 /** 795 * @return {@link #assessmentMethod} (The method of evaluating the relatedness of the suspected entity to the event.) 796 */ 797 public CodeableConcept getAssessmentMethod() { 798 if (this.assessmentMethod == null) 799 if (Configuration.errorOnAutoCreate()) 800 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.assessmentMethod"); 801 else if (Configuration.doAutoCreate()) 802 this.assessmentMethod = new CodeableConcept(); // cc 803 return this.assessmentMethod; 804 } 805 806 public boolean hasAssessmentMethod() { 807 return this.assessmentMethod != null && !this.assessmentMethod.isEmpty(); 808 } 809 810 /** 811 * @param value {@link #assessmentMethod} (The method of evaluating the relatedness of the suspected entity to the event.) 812 */ 813 public AdverseEventSuspectEntityCausalityComponent setAssessmentMethod(CodeableConcept value) { 814 this.assessmentMethod = value; 815 return this; 816 } 817 818 /** 819 * @return {@link #entityRelatedness} (The result of the assessment regarding the relatedness of the suspected entity to the event.) 820 */ 821 public CodeableConcept getEntityRelatedness() { 822 if (this.entityRelatedness == null) 823 if (Configuration.errorOnAutoCreate()) 824 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.entityRelatedness"); 825 else if (Configuration.doAutoCreate()) 826 this.entityRelatedness = new CodeableConcept(); // cc 827 return this.entityRelatedness; 828 } 829 830 public boolean hasEntityRelatedness() { 831 return this.entityRelatedness != null && !this.entityRelatedness.isEmpty(); 832 } 833 834 /** 835 * @param value {@link #entityRelatedness} (The result of the assessment regarding the relatedness of the suspected entity to the event.) 836 */ 837 public AdverseEventSuspectEntityCausalityComponent setEntityRelatedness(CodeableConcept value) { 838 this.entityRelatedness = value; 839 return this; 840 } 841 842 /** 843 * @return {@link #author} (The author of the information on the possible cause of the event.) 844 */ 845 public Reference getAuthor() { 846 if (this.author == null) 847 if (Configuration.errorOnAutoCreate()) 848 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.author"); 849 else if (Configuration.doAutoCreate()) 850 this.author = new Reference(); // cc 851 return this.author; 852 } 853 854 public boolean hasAuthor() { 855 return this.author != null && !this.author.isEmpty(); 856 } 857 858 /** 859 * @param value {@link #author} (The author of the information on the possible cause of the event.) 860 */ 861 public AdverseEventSuspectEntityCausalityComponent setAuthor(Reference value) { 862 this.author = value; 863 return this; 864 } 865 866 protected void listChildren(List<Property> children) { 867 super.listChildren(children); 868 children.add(new Property("assessmentMethod", "CodeableConcept", "The method of evaluating the relatedness of the suspected entity to the event.", 0, 1, assessmentMethod)); 869 children.add(new Property("entityRelatedness", "CodeableConcept", "The result of the assessment regarding the relatedness of the suspected entity to the event.", 0, 1, entityRelatedness)); 870 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|ResearchSubject)", "The author of the information on the possible cause of the event.", 0, 1, author)); 871 } 872 873 @Override 874 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 875 switch (_hash) { 876 case 1681283651: /*assessmentMethod*/ return new Property("assessmentMethod", "CodeableConcept", "The method of evaluating the relatedness of the suspected entity to the event.", 0, 1, assessmentMethod); 877 case 2000199967: /*entityRelatedness*/ return new Property("entityRelatedness", "CodeableConcept", "The result of the assessment regarding the relatedness of the suspected entity to the event.", 0, 1, entityRelatedness); 878 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|ResearchSubject)", "The author of the information on the possible cause of the event.", 0, 1, author); 879 default: return super.getNamedProperty(_hash, _name, _checkValid); 880 } 881 882 } 883 884 @Override 885 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 886 switch (hash) { 887 case 1681283651: /*assessmentMethod*/ return this.assessmentMethod == null ? new Base[0] : new Base[] {this.assessmentMethod}; // CodeableConcept 888 case 2000199967: /*entityRelatedness*/ return this.entityRelatedness == null ? new Base[0] : new Base[] {this.entityRelatedness}; // CodeableConcept 889 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 890 default: return super.getProperty(hash, name, checkValid); 891 } 892 893 } 894 895 @Override 896 public Base setProperty(int hash, String name, Base value) throws FHIRException { 897 switch (hash) { 898 case 1681283651: // assessmentMethod 899 this.assessmentMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 900 return value; 901 case 2000199967: // entityRelatedness 902 this.entityRelatedness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 903 return value; 904 case -1406328437: // author 905 this.author = TypeConvertor.castToReference(value); // Reference 906 return value; 907 default: return super.setProperty(hash, name, value); 908 } 909 910 } 911 912 @Override 913 public Base setProperty(String name, Base value) throws FHIRException { 914 if (name.equals("assessmentMethod")) { 915 this.assessmentMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 916 } else if (name.equals("entityRelatedness")) { 917 this.entityRelatedness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 918 } else if (name.equals("author")) { 919 this.author = TypeConvertor.castToReference(value); // Reference 920 } else 921 return super.setProperty(name, value); 922 return value; 923 } 924 925 @Override 926 public void removeChild(String name, Base value) throws FHIRException { 927 if (name.equals("assessmentMethod")) { 928 this.assessmentMethod = null; 929 } else if (name.equals("entityRelatedness")) { 930 this.entityRelatedness = null; 931 } else if (name.equals("author")) { 932 this.author = null; 933 } else 934 super.removeChild(name, value); 935 936 } 937 938 @Override 939 public Base makeProperty(int hash, String name) throws FHIRException { 940 switch (hash) { 941 case 1681283651: return getAssessmentMethod(); 942 case 2000199967: return getEntityRelatedness(); 943 case -1406328437: return getAuthor(); 944 default: return super.makeProperty(hash, name); 945 } 946 947 } 948 949 @Override 950 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 951 switch (hash) { 952 case 1681283651: /*assessmentMethod*/ return new String[] {"CodeableConcept"}; 953 case 2000199967: /*entityRelatedness*/ return new String[] {"CodeableConcept"}; 954 case -1406328437: /*author*/ return new String[] {"Reference"}; 955 default: return super.getTypesForProperty(hash, name); 956 } 957 958 } 959 960 @Override 961 public Base addChild(String name) throws FHIRException { 962 if (name.equals("assessmentMethod")) { 963 this.assessmentMethod = new CodeableConcept(); 964 return this.assessmentMethod; 965 } 966 else if (name.equals("entityRelatedness")) { 967 this.entityRelatedness = new CodeableConcept(); 968 return this.entityRelatedness; 969 } 970 else if (name.equals("author")) { 971 this.author = new Reference(); 972 return this.author; 973 } 974 else 975 return super.addChild(name); 976 } 977 978 public AdverseEventSuspectEntityCausalityComponent copy() { 979 AdverseEventSuspectEntityCausalityComponent dst = new AdverseEventSuspectEntityCausalityComponent(); 980 copyValues(dst); 981 return dst; 982 } 983 984 public void copyValues(AdverseEventSuspectEntityCausalityComponent dst) { 985 super.copyValues(dst); 986 dst.assessmentMethod = assessmentMethod == null ? null : assessmentMethod.copy(); 987 dst.entityRelatedness = entityRelatedness == null ? null : entityRelatedness.copy(); 988 dst.author = author == null ? null : author.copy(); 989 } 990 991 @Override 992 public boolean equalsDeep(Base other_) { 993 if (!super.equalsDeep(other_)) 994 return false; 995 if (!(other_ instanceof AdverseEventSuspectEntityCausalityComponent)) 996 return false; 997 AdverseEventSuspectEntityCausalityComponent o = (AdverseEventSuspectEntityCausalityComponent) other_; 998 return compareDeep(assessmentMethod, o.assessmentMethod, true) && compareDeep(entityRelatedness, o.entityRelatedness, true) 999 && compareDeep(author, o.author, true); 1000 } 1001 1002 @Override 1003 public boolean equalsShallow(Base other_) { 1004 if (!super.equalsShallow(other_)) 1005 return false; 1006 if (!(other_ instanceof AdverseEventSuspectEntityCausalityComponent)) 1007 return false; 1008 AdverseEventSuspectEntityCausalityComponent o = (AdverseEventSuspectEntityCausalityComponent) other_; 1009 return true; 1010 } 1011 1012 public boolean isEmpty() { 1013 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(assessmentMethod, entityRelatedness 1014 , author); 1015 } 1016 1017 public String fhirType() { 1018 return "AdverseEvent.suspectEntity.causality"; 1019 1020 } 1021 1022 } 1023 1024 @Block() 1025 public static class AdverseEventContributingFactorComponent extends BackboneElement implements IBaseBackboneElement { 1026 /** 1027 * The item that is suspected to have increased the probability or severity of the adverse event. 1028 */ 1029 @Child(name = "item", type = {Condition.class, Observation.class, AllergyIntolerance.class, FamilyMemberHistory.class, Immunization.class, Procedure.class, Device.class, DeviceUsage.class, DocumentReference.class, MedicationAdministration.class, MedicationStatement.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1030 @Description(shortDefinition="Item suspected to have increased the probability or severity of the adverse event", formalDefinition="The item that is suspected to have increased the probability or severity of the adverse event." ) 1031 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-contributing-factor") 1032 protected DataType item; 1033 1034 private static final long serialVersionUID = 1847936859L; 1035 1036 /** 1037 * Constructor 1038 */ 1039 public AdverseEventContributingFactorComponent() { 1040 super(); 1041 } 1042 1043 /** 1044 * Constructor 1045 */ 1046 public AdverseEventContributingFactorComponent(DataType item) { 1047 super(); 1048 this.setItem(item); 1049 } 1050 1051 /** 1052 * @return {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1053 */ 1054 public DataType getItem() { 1055 return this.item; 1056 } 1057 1058 /** 1059 * @return {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1060 */ 1061 public Reference getItemReference() throws FHIRException { 1062 if (this.item == null) 1063 this.item = new Reference(); 1064 if (!(this.item instanceof Reference)) 1065 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1066 return (Reference) this.item; 1067 } 1068 1069 public boolean hasItemReference() { 1070 return this != null && this.item instanceof Reference; 1071 } 1072 1073 /** 1074 * @return {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1075 */ 1076 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1077 if (this.item == null) 1078 this.item = new CodeableConcept(); 1079 if (!(this.item instanceof CodeableConcept)) 1080 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1081 return (CodeableConcept) this.item; 1082 } 1083 1084 public boolean hasItemCodeableConcept() { 1085 return this != null && this.item instanceof CodeableConcept; 1086 } 1087 1088 public boolean hasItem() { 1089 return this.item != null && !this.item.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #item} (The item that is suspected to have increased the probability or severity of the adverse event.) 1094 */ 1095 public AdverseEventContributingFactorComponent setItem(DataType value) { 1096 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1097 throw new FHIRException("Not the right type for AdverseEvent.contributingFactor.item[x]: "+value.fhirType()); 1098 this.item = value; 1099 return this; 1100 } 1101 1102 protected void listChildren(List<Property> children) { 1103 super.listChildren(children); 1104 children.add(new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)|CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item)); 1105 } 1106 1107 @Override 1108 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1109 switch (_hash) { 1110 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)|CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1111 case 3242771: /*item*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)|CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1112 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Device|DeviceUsage|DocumentReference|MedicationAdministration|MedicationStatement)", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1113 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The item that is suspected to have increased the probability or severity of the adverse event.", 0, 1, item); 1114 default: return super.getNamedProperty(_hash, _name, _checkValid); 1115 } 1116 1117 } 1118 1119 @Override 1120 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1121 switch (hash) { 1122 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1123 default: return super.getProperty(hash, name, checkValid); 1124 } 1125 1126 } 1127 1128 @Override 1129 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1130 switch (hash) { 1131 case 3242771: // item 1132 this.item = TypeConvertor.castToType(value); // DataType 1133 return value; 1134 default: return super.setProperty(hash, name, value); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base setProperty(String name, Base value) throws FHIRException { 1141 if (name.equals("item[x]")) { 1142 this.item = TypeConvertor.castToType(value); // DataType 1143 } else 1144 return super.setProperty(name, value); 1145 return value; 1146 } 1147 1148 @Override 1149 public void removeChild(String name, Base value) throws FHIRException { 1150 if (name.equals("item[x]")) { 1151 this.item = null; 1152 } else 1153 super.removeChild(name, value); 1154 1155 } 1156 1157 @Override 1158 public Base makeProperty(int hash, String name) throws FHIRException { 1159 switch (hash) { 1160 case 2116201613: return getItem(); 1161 case 3242771: return getItem(); 1162 default: return super.makeProperty(hash, name); 1163 } 1164 1165 } 1166 1167 @Override 1168 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1169 switch (hash) { 1170 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1171 default: return super.getTypesForProperty(hash, name); 1172 } 1173 1174 } 1175 1176 @Override 1177 public Base addChild(String name) throws FHIRException { 1178 if (name.equals("itemReference")) { 1179 this.item = new Reference(); 1180 return this.item; 1181 } 1182 else if (name.equals("itemCodeableConcept")) { 1183 this.item = new CodeableConcept(); 1184 return this.item; 1185 } 1186 else 1187 return super.addChild(name); 1188 } 1189 1190 public AdverseEventContributingFactorComponent copy() { 1191 AdverseEventContributingFactorComponent dst = new AdverseEventContributingFactorComponent(); 1192 copyValues(dst); 1193 return dst; 1194 } 1195 1196 public void copyValues(AdverseEventContributingFactorComponent dst) { 1197 super.copyValues(dst); 1198 dst.item = item == null ? null : item.copy(); 1199 } 1200 1201 @Override 1202 public boolean equalsDeep(Base other_) { 1203 if (!super.equalsDeep(other_)) 1204 return false; 1205 if (!(other_ instanceof AdverseEventContributingFactorComponent)) 1206 return false; 1207 AdverseEventContributingFactorComponent o = (AdverseEventContributingFactorComponent) other_; 1208 return compareDeep(item, o.item, true); 1209 } 1210 1211 @Override 1212 public boolean equalsShallow(Base other_) { 1213 if (!super.equalsShallow(other_)) 1214 return false; 1215 if (!(other_ instanceof AdverseEventContributingFactorComponent)) 1216 return false; 1217 AdverseEventContributingFactorComponent o = (AdverseEventContributingFactorComponent) other_; 1218 return true; 1219 } 1220 1221 public boolean isEmpty() { 1222 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1223 } 1224 1225 public String fhirType() { 1226 return "AdverseEvent.contributingFactor"; 1227 1228 } 1229 1230 } 1231 1232 @Block() 1233 public static class AdverseEventPreventiveActionComponent extends BackboneElement implements IBaseBackboneElement { 1234 /** 1235 * The action that contributed to avoiding the adverse event. 1236 */ 1237 @Child(name = "item", type = {Immunization.class, Procedure.class, DocumentReference.class, MedicationAdministration.class, MedicationRequest.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1238 @Description(shortDefinition="Action that contributed to avoiding the adverse event", formalDefinition="The action that contributed to avoiding the adverse event." ) 1239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-preventive-action") 1240 protected DataType item; 1241 1242 private static final long serialVersionUID = 1847936859L; 1243 1244 /** 1245 * Constructor 1246 */ 1247 public AdverseEventPreventiveActionComponent() { 1248 super(); 1249 } 1250 1251 /** 1252 * Constructor 1253 */ 1254 public AdverseEventPreventiveActionComponent(DataType item) { 1255 super(); 1256 this.setItem(item); 1257 } 1258 1259 /** 1260 * @return {@link #item} (The action that contributed to avoiding the adverse event.) 1261 */ 1262 public DataType getItem() { 1263 return this.item; 1264 } 1265 1266 /** 1267 * @return {@link #item} (The action that contributed to avoiding the adverse event.) 1268 */ 1269 public Reference getItemReference() throws FHIRException { 1270 if (this.item == null) 1271 this.item = new Reference(); 1272 if (!(this.item instanceof Reference)) 1273 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1274 return (Reference) this.item; 1275 } 1276 1277 public boolean hasItemReference() { 1278 return this != null && this.item instanceof Reference; 1279 } 1280 1281 /** 1282 * @return {@link #item} (The action that contributed to avoiding the adverse event.) 1283 */ 1284 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1285 if (this.item == null) 1286 this.item = new CodeableConcept(); 1287 if (!(this.item instanceof CodeableConcept)) 1288 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1289 return (CodeableConcept) this.item; 1290 } 1291 1292 public boolean hasItemCodeableConcept() { 1293 return this != null && this.item instanceof CodeableConcept; 1294 } 1295 1296 public boolean hasItem() { 1297 return this.item != null && !this.item.isEmpty(); 1298 } 1299 1300 /** 1301 * @param value {@link #item} (The action that contributed to avoiding the adverse event.) 1302 */ 1303 public AdverseEventPreventiveActionComponent setItem(DataType value) { 1304 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1305 throw new FHIRException("Not the right type for AdverseEvent.preventiveAction.item[x]: "+value.fhirType()); 1306 this.item = value; 1307 return this; 1308 } 1309 1310 protected void listChildren(List<Property> children) { 1311 super.listChildren(children); 1312 children.add(new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item)); 1313 } 1314 1315 @Override 1316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1317 switch (_hash) { 1318 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1319 case 3242771: /*item*/ return new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1320 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationRequest)", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1321 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The action that contributed to avoiding the adverse event.", 0, 1, item); 1322 default: return super.getNamedProperty(_hash, _name, _checkValid); 1323 } 1324 1325 } 1326 1327 @Override 1328 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1329 switch (hash) { 1330 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1331 default: return super.getProperty(hash, name, checkValid); 1332 } 1333 1334 } 1335 1336 @Override 1337 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1338 switch (hash) { 1339 case 3242771: // item 1340 this.item = TypeConvertor.castToType(value); // DataType 1341 return value; 1342 default: return super.setProperty(hash, name, value); 1343 } 1344 1345 } 1346 1347 @Override 1348 public Base setProperty(String name, Base value) throws FHIRException { 1349 if (name.equals("item[x]")) { 1350 this.item = TypeConvertor.castToType(value); // DataType 1351 } else 1352 return super.setProperty(name, value); 1353 return value; 1354 } 1355 1356 @Override 1357 public void removeChild(String name, Base value) throws FHIRException { 1358 if (name.equals("item[x]")) { 1359 this.item = null; 1360 } else 1361 super.removeChild(name, value); 1362 1363 } 1364 1365 @Override 1366 public Base makeProperty(int hash, String name) throws FHIRException { 1367 switch (hash) { 1368 case 2116201613: return getItem(); 1369 case 3242771: return getItem(); 1370 default: return super.makeProperty(hash, name); 1371 } 1372 1373 } 1374 1375 @Override 1376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1377 switch (hash) { 1378 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1379 default: return super.getTypesForProperty(hash, name); 1380 } 1381 1382 } 1383 1384 @Override 1385 public Base addChild(String name) throws FHIRException { 1386 if (name.equals("itemReference")) { 1387 this.item = new Reference(); 1388 return this.item; 1389 } 1390 else if (name.equals("itemCodeableConcept")) { 1391 this.item = new CodeableConcept(); 1392 return this.item; 1393 } 1394 else 1395 return super.addChild(name); 1396 } 1397 1398 public AdverseEventPreventiveActionComponent copy() { 1399 AdverseEventPreventiveActionComponent dst = new AdverseEventPreventiveActionComponent(); 1400 copyValues(dst); 1401 return dst; 1402 } 1403 1404 public void copyValues(AdverseEventPreventiveActionComponent dst) { 1405 super.copyValues(dst); 1406 dst.item = item == null ? null : item.copy(); 1407 } 1408 1409 @Override 1410 public boolean equalsDeep(Base other_) { 1411 if (!super.equalsDeep(other_)) 1412 return false; 1413 if (!(other_ instanceof AdverseEventPreventiveActionComponent)) 1414 return false; 1415 AdverseEventPreventiveActionComponent o = (AdverseEventPreventiveActionComponent) other_; 1416 return compareDeep(item, o.item, true); 1417 } 1418 1419 @Override 1420 public boolean equalsShallow(Base other_) { 1421 if (!super.equalsShallow(other_)) 1422 return false; 1423 if (!(other_ instanceof AdverseEventPreventiveActionComponent)) 1424 return false; 1425 AdverseEventPreventiveActionComponent o = (AdverseEventPreventiveActionComponent) other_; 1426 return true; 1427 } 1428 1429 public boolean isEmpty() { 1430 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1431 } 1432 1433 public String fhirType() { 1434 return "AdverseEvent.preventiveAction"; 1435 1436 } 1437 1438 } 1439 1440 @Block() 1441 public static class AdverseEventMitigatingActionComponent extends BackboneElement implements IBaseBackboneElement { 1442 /** 1443 * The ameliorating action taken after the adverse event occured in order to reduce the extent of harm. 1444 */ 1445 @Child(name = "item", type = {Procedure.class, DocumentReference.class, MedicationAdministration.class, MedicationRequest.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1446 @Description(shortDefinition="Ameliorating action taken after the adverse event occured in order to reduce the extent of harm", formalDefinition="The ameliorating action taken after the adverse event occured in order to reduce the extent of harm." ) 1447 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-mitigating-action") 1448 protected DataType item; 1449 1450 private static final long serialVersionUID = 1847936859L; 1451 1452 /** 1453 * Constructor 1454 */ 1455 public AdverseEventMitigatingActionComponent() { 1456 super(); 1457 } 1458 1459 /** 1460 * Constructor 1461 */ 1462 public AdverseEventMitigatingActionComponent(DataType item) { 1463 super(); 1464 this.setItem(item); 1465 } 1466 1467 /** 1468 * @return {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1469 */ 1470 public DataType getItem() { 1471 return this.item; 1472 } 1473 1474 /** 1475 * @return {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1476 */ 1477 public Reference getItemReference() throws FHIRException { 1478 if (this.item == null) 1479 this.item = new Reference(); 1480 if (!(this.item instanceof Reference)) 1481 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1482 return (Reference) this.item; 1483 } 1484 1485 public boolean hasItemReference() { 1486 return this != null && this.item instanceof Reference; 1487 } 1488 1489 /** 1490 * @return {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1491 */ 1492 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1493 if (this.item == null) 1494 this.item = new CodeableConcept(); 1495 if (!(this.item instanceof CodeableConcept)) 1496 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1497 return (CodeableConcept) this.item; 1498 } 1499 1500 public boolean hasItemCodeableConcept() { 1501 return this != null && this.item instanceof CodeableConcept; 1502 } 1503 1504 public boolean hasItem() { 1505 return this.item != null && !this.item.isEmpty(); 1506 } 1507 1508 /** 1509 * @param value {@link #item} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 1510 */ 1511 public AdverseEventMitigatingActionComponent setItem(DataType value) { 1512 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1513 throw new FHIRException("Not the right type for AdverseEvent.mitigatingAction.item[x]: "+value.fhirType()); 1514 this.item = value; 1515 return this; 1516 } 1517 1518 protected void listChildren(List<Property> children) { 1519 super.listChildren(children); 1520 children.add(new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item)); 1521 } 1522 1523 @Override 1524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1525 switch (_hash) { 1526 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1527 case 3242771: /*item*/ return new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)|CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1528 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Procedure|DocumentReference|MedicationAdministration|MedicationRequest)", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1529 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, 1, item); 1530 default: return super.getNamedProperty(_hash, _name, _checkValid); 1531 } 1532 1533 } 1534 1535 @Override 1536 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1537 switch (hash) { 1538 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1539 default: return super.getProperty(hash, name, checkValid); 1540 } 1541 1542 } 1543 1544 @Override 1545 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1546 switch (hash) { 1547 case 3242771: // item 1548 this.item = TypeConvertor.castToType(value); // DataType 1549 return value; 1550 default: return super.setProperty(hash, name, value); 1551 } 1552 1553 } 1554 1555 @Override 1556 public Base setProperty(String name, Base value) throws FHIRException { 1557 if (name.equals("item[x]")) { 1558 this.item = TypeConvertor.castToType(value); // DataType 1559 } else 1560 return super.setProperty(name, value); 1561 return value; 1562 } 1563 1564 @Override 1565 public void removeChild(String name, Base value) throws FHIRException { 1566 if (name.equals("item[x]")) { 1567 this.item = null; 1568 } else 1569 super.removeChild(name, value); 1570 1571 } 1572 1573 @Override 1574 public Base makeProperty(int hash, String name) throws FHIRException { 1575 switch (hash) { 1576 case 2116201613: return getItem(); 1577 case 3242771: return getItem(); 1578 default: return super.makeProperty(hash, name); 1579 } 1580 1581 } 1582 1583 @Override 1584 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1585 switch (hash) { 1586 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1587 default: return super.getTypesForProperty(hash, name); 1588 } 1589 1590 } 1591 1592 @Override 1593 public Base addChild(String name) throws FHIRException { 1594 if (name.equals("itemReference")) { 1595 this.item = new Reference(); 1596 return this.item; 1597 } 1598 else if (name.equals("itemCodeableConcept")) { 1599 this.item = new CodeableConcept(); 1600 return this.item; 1601 } 1602 else 1603 return super.addChild(name); 1604 } 1605 1606 public AdverseEventMitigatingActionComponent copy() { 1607 AdverseEventMitigatingActionComponent dst = new AdverseEventMitigatingActionComponent(); 1608 copyValues(dst); 1609 return dst; 1610 } 1611 1612 public void copyValues(AdverseEventMitigatingActionComponent dst) { 1613 super.copyValues(dst); 1614 dst.item = item == null ? null : item.copy(); 1615 } 1616 1617 @Override 1618 public boolean equalsDeep(Base other_) { 1619 if (!super.equalsDeep(other_)) 1620 return false; 1621 if (!(other_ instanceof AdverseEventMitigatingActionComponent)) 1622 return false; 1623 AdverseEventMitigatingActionComponent o = (AdverseEventMitigatingActionComponent) other_; 1624 return compareDeep(item, o.item, true); 1625 } 1626 1627 @Override 1628 public boolean equalsShallow(Base other_) { 1629 if (!super.equalsShallow(other_)) 1630 return false; 1631 if (!(other_ instanceof AdverseEventMitigatingActionComponent)) 1632 return false; 1633 AdverseEventMitigatingActionComponent o = (AdverseEventMitigatingActionComponent) other_; 1634 return true; 1635 } 1636 1637 public boolean isEmpty() { 1638 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1639 } 1640 1641 public String fhirType() { 1642 return "AdverseEvent.mitigatingAction"; 1643 1644 } 1645 1646 } 1647 1648 @Block() 1649 public static class AdverseEventSupportingInfoComponent extends BackboneElement implements IBaseBackboneElement { 1650 /** 1651 * Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action. 1652 */ 1653 @Child(name = "item", type = {Condition.class, Observation.class, AllergyIntolerance.class, FamilyMemberHistory.class, Immunization.class, Procedure.class, DocumentReference.class, MedicationAdministration.class, MedicationStatement.class, QuestionnaireResponse.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1654 @Description(shortDefinition="Subject medical history or document relevant to this adverse event", formalDefinition="Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action." ) 1655 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-supporting-info") 1656 protected DataType item; 1657 1658 private static final long serialVersionUID = 1847936859L; 1659 1660 /** 1661 * Constructor 1662 */ 1663 public AdverseEventSupportingInfoComponent() { 1664 super(); 1665 } 1666 1667 /** 1668 * Constructor 1669 */ 1670 public AdverseEventSupportingInfoComponent(DataType item) { 1671 super(); 1672 this.setItem(item); 1673 } 1674 1675 /** 1676 * @return {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1677 */ 1678 public DataType getItem() { 1679 return this.item; 1680 } 1681 1682 /** 1683 * @return {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1684 */ 1685 public Reference getItemReference() throws FHIRException { 1686 if (this.item == null) 1687 this.item = new Reference(); 1688 if (!(this.item instanceof Reference)) 1689 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 1690 return (Reference) this.item; 1691 } 1692 1693 public boolean hasItemReference() { 1694 return this != null && this.item instanceof Reference; 1695 } 1696 1697 /** 1698 * @return {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1699 */ 1700 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1701 if (this.item == null) 1702 this.item = new CodeableConcept(); 1703 if (!(this.item instanceof CodeableConcept)) 1704 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 1705 return (CodeableConcept) this.item; 1706 } 1707 1708 public boolean hasItemCodeableConcept() { 1709 return this != null && this.item instanceof CodeableConcept; 1710 } 1711 1712 public boolean hasItem() { 1713 return this.item != null && !this.item.isEmpty(); 1714 } 1715 1716 /** 1717 * @param value {@link #item} (Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.) 1718 */ 1719 public AdverseEventSupportingInfoComponent setItem(DataType value) { 1720 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1721 throw new FHIRException("Not the right type for AdverseEvent.supportingInfo.item[x]: "+value.fhirType()); 1722 this.item = value; 1723 return this; 1724 } 1725 1726 protected void listChildren(List<Property> children) { 1727 super.listChildren(children); 1728 children.add(new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)|CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item)); 1729 } 1730 1731 @Override 1732 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1733 switch (_hash) { 1734 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)|CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1735 case 3242771: /*item*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)|CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1736 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|DocumentReference|MedicationAdministration|MedicationStatement|QuestionnaireResponse)", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1737 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "Relevant past history for the subject. In a clinical care context, an example being a patient had an adverse event following a pencillin administration and the patient had a previously documented penicillin allergy. In a clinical trials context, an example is a bunion or rash that was present prior to the study. Additionally, the supporting item can be a document that is relevant to this instance of the adverse event that is not part of the subject's medical history. For example, a clinical note, staff list, or material safety data sheet (MSDS). Supporting information is not a contributing factor, preventive action, or mitigating action.", 0, 1, item); 1738 default: return super.getNamedProperty(_hash, _name, _checkValid); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1745 switch (hash) { 1746 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 1747 default: return super.getProperty(hash, name, checkValid); 1748 } 1749 1750 } 1751 1752 @Override 1753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1754 switch (hash) { 1755 case 3242771: // item 1756 this.item = TypeConvertor.castToType(value); // DataType 1757 return value; 1758 default: return super.setProperty(hash, name, value); 1759 } 1760 1761 } 1762 1763 @Override 1764 public Base setProperty(String name, Base value) throws FHIRException { 1765 if (name.equals("item[x]")) { 1766 this.item = TypeConvertor.castToType(value); // DataType 1767 } else 1768 return super.setProperty(name, value); 1769 return value; 1770 } 1771 1772 @Override 1773 public void removeChild(String name, Base value) throws FHIRException { 1774 if (name.equals("item[x]")) { 1775 this.item = null; 1776 } else 1777 super.removeChild(name, value); 1778 1779 } 1780 1781 @Override 1782 public Base makeProperty(int hash, String name) throws FHIRException { 1783 switch (hash) { 1784 case 2116201613: return getItem(); 1785 case 3242771: return getItem(); 1786 default: return super.makeProperty(hash, name); 1787 } 1788 1789 } 1790 1791 @Override 1792 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1793 switch (hash) { 1794 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 1795 default: return super.getTypesForProperty(hash, name); 1796 } 1797 1798 } 1799 1800 @Override 1801 public Base addChild(String name) throws FHIRException { 1802 if (name.equals("itemReference")) { 1803 this.item = new Reference(); 1804 return this.item; 1805 } 1806 else if (name.equals("itemCodeableConcept")) { 1807 this.item = new CodeableConcept(); 1808 return this.item; 1809 } 1810 else 1811 return super.addChild(name); 1812 } 1813 1814 public AdverseEventSupportingInfoComponent copy() { 1815 AdverseEventSupportingInfoComponent dst = new AdverseEventSupportingInfoComponent(); 1816 copyValues(dst); 1817 return dst; 1818 } 1819 1820 public void copyValues(AdverseEventSupportingInfoComponent dst) { 1821 super.copyValues(dst); 1822 dst.item = item == null ? null : item.copy(); 1823 } 1824 1825 @Override 1826 public boolean equalsDeep(Base other_) { 1827 if (!super.equalsDeep(other_)) 1828 return false; 1829 if (!(other_ instanceof AdverseEventSupportingInfoComponent)) 1830 return false; 1831 AdverseEventSupportingInfoComponent o = (AdverseEventSupportingInfoComponent) other_; 1832 return compareDeep(item, o.item, true); 1833 } 1834 1835 @Override 1836 public boolean equalsShallow(Base other_) { 1837 if (!super.equalsShallow(other_)) 1838 return false; 1839 if (!(other_ instanceof AdverseEventSupportingInfoComponent)) 1840 return false; 1841 AdverseEventSupportingInfoComponent o = (AdverseEventSupportingInfoComponent) other_; 1842 return true; 1843 } 1844 1845 public boolean isEmpty() { 1846 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 1847 } 1848 1849 public String fhirType() { 1850 return "AdverseEvent.supportingInfo"; 1851 1852 } 1853 1854 } 1855 1856 /** 1857 * Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 1858 */ 1859 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1860 @Description(shortDefinition="Business identifier for the event", formalDefinition="Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 1861 protected List<Identifier> identifier; 1862 1863 /** 1864 * The current state of the adverse event or potential adverse event. 1865 */ 1866 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1867 @Description(shortDefinition="in-progress | completed | entered-in-error | unknown", formalDefinition="The current state of the adverse event or potential adverse event." ) 1868 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-status") 1869 protected Enumeration<AdverseEventStatus> status; 1870 1871 /** 1872 * Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely. 1873 */ 1874 @Child(name = "actuality", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1875 @Description(shortDefinition="actual | potential", formalDefinition="Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely." ) 1876 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-actuality") 1877 protected Enumeration<AdverseEventActuality> actuality; 1878 1879 /** 1880 * The overall type of event, intended for search and filtering purposes. 1881 */ 1882 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1883 @Description(shortDefinition="wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site", formalDefinition="The overall type of event, intended for search and filtering purposes." ) 1884 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-category") 1885 protected List<CodeableConcept> category; 1886 1887 /** 1888 * Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused. 1889 */ 1890 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1891 @Description(shortDefinition="Event or incident that occurred or was averted", formalDefinition="Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused." ) 1892 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-type") 1893 protected CodeableConcept code; 1894 1895 /** 1896 * This subject or group impacted by the event. 1897 */ 1898 @Child(name = "subject", type = {Patient.class, Group.class, Practitioner.class, RelatedPerson.class, ResearchSubject.class}, order=5, min=1, max=1, modifier=false, summary=true) 1899 @Description(shortDefinition="Subject impacted by event", formalDefinition="This subject or group impacted by the event." ) 1900 protected Reference subject; 1901 1902 /** 1903 * The Encounter associated with the start of the AdverseEvent. 1904 */ 1905 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 1906 @Description(shortDefinition="The Encounter associated with the start of the AdverseEvent", formalDefinition="The Encounter associated with the start of the AdverseEvent." ) 1907 protected Reference encounter; 1908 1909 /** 1910 * The date (and perhaps time) when the adverse event occurred. 1911 */ 1912 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 1913 @Description(shortDefinition="When the event occurred", formalDefinition="The date (and perhaps time) when the adverse event occurred." ) 1914 protected DataType occurrence; 1915 1916 /** 1917 * Estimated or actual date the AdverseEvent began, in the opinion of the reporter. 1918 */ 1919 @Child(name = "detected", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1920 @Description(shortDefinition="When the event was detected", formalDefinition="Estimated or actual date the AdverseEvent began, in the opinion of the reporter." ) 1921 protected DateTimeType detected; 1922 1923 /** 1924 * The date on which the existence of the AdverseEvent was first recorded. 1925 */ 1926 @Child(name = "recordedDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1927 @Description(shortDefinition="When the event was recorded", formalDefinition="The date on which the existence of the AdverseEvent was first recorded." ) 1928 protected DateTimeType recordedDate; 1929 1930 /** 1931 * Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall. 1932 */ 1933 @Child(name = "resultingEffect", type = {Condition.class, Observation.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1934 @Description(shortDefinition="Effect on the subject due to this event", formalDefinition="Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall." ) 1935 protected List<Reference> resultingEffect; 1936 1937 /** 1938 * The information about where the adverse event occurred. 1939 */ 1940 @Child(name = "location", type = {Location.class}, order=11, min=0, max=1, modifier=false, summary=true) 1941 @Description(shortDefinition="Location where adverse event occurred", formalDefinition="The information about where the adverse event occurred." ) 1942 protected Reference location; 1943 1944 /** 1945 * Assessment whether this event, or averted event, was of clinical importance. 1946 */ 1947 @Child(name = "seriousness", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 1948 @Description(shortDefinition="Seriousness or gravity of the event", formalDefinition="Assessment whether this event, or averted event, was of clinical importance." ) 1949 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-seriousness") 1950 protected CodeableConcept seriousness; 1951 1952 /** 1953 * Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal. 1954 */ 1955 @Child(name = "outcome", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1956 @Description(shortDefinition="Type of outcome from the adverse event", formalDefinition="Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal." ) 1957 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adverse-event-outcome") 1958 protected List<CodeableConcept> outcome; 1959 1960 /** 1961 * Information on who recorded the adverse event. May be the patient or a practitioner. 1962 */ 1963 @Child(name = "recorder", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, ResearchSubject.class}, order=14, min=0, max=1, modifier=false, summary=true) 1964 @Description(shortDefinition="Who recorded the adverse event", formalDefinition="Information on who recorded the adverse event. May be the patient or a practitioner." ) 1965 protected Reference recorder; 1966 1967 /** 1968 * Indicates who or what participated in the adverse event and how they were involved. 1969 */ 1970 @Child(name = "participant", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1971 @Description(shortDefinition="Who was involved in the adverse event or the potential adverse event and what they did", formalDefinition="Indicates who or what participated in the adverse event and how they were involved." ) 1972 protected List<AdverseEventParticipantComponent> participant; 1973 1974 /** 1975 * The research study that the subject is enrolled in. 1976 */ 1977 @Child(name = "study", type = {ResearchStudy.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1978 @Description(shortDefinition="Research study that the subject is enrolled in", formalDefinition="The research study that the subject is enrolled in." ) 1979 protected List<Reference> study; 1980 1981 /** 1982 * Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely. 1983 */ 1984 @Child(name = "expectedInResearchStudy", type = {BooleanType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1985 @Description(shortDefinition="Considered likely or probable or anticipated in the research study", formalDefinition="Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely." ) 1986 protected BooleanType expectedInResearchStudy; 1987 1988 /** 1989 * Describes the entity that is suspected to have caused the adverse event. 1990 */ 1991 @Child(name = "suspectEntity", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1992 @Description(shortDefinition="The suspected agent causing the adverse event", formalDefinition="Describes the entity that is suspected to have caused the adverse event." ) 1993 protected List<AdverseEventSuspectEntityComponent> suspectEntity; 1994 1995 /** 1996 * The contributing factors suspected to have increased the probability or severity of the adverse event. 1997 */ 1998 @Child(name = "contributingFactor", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1999 @Description(shortDefinition="Contributing factors suspected to have increased the probability or severity of the adverse event", formalDefinition="The contributing factors suspected to have increased the probability or severity of the adverse event." ) 2000 protected List<AdverseEventContributingFactorComponent> contributingFactor; 2001 2002 /** 2003 * Preventive actions that contributed to avoiding the adverse event. 2004 */ 2005 @Child(name = "preventiveAction", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2006 @Description(shortDefinition="Preventive actions that contributed to avoiding the adverse event", formalDefinition="Preventive actions that contributed to avoiding the adverse event." ) 2007 protected List<AdverseEventPreventiveActionComponent> preventiveAction; 2008 2009 /** 2010 * The ameliorating action taken after the adverse event occured in order to reduce the extent of harm. 2011 */ 2012 @Child(name = "mitigatingAction", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2013 @Description(shortDefinition="Ameliorating actions taken after the adverse event occured in order to reduce the extent of harm", formalDefinition="The ameliorating action taken after the adverse event occured in order to reduce the extent of harm." ) 2014 protected List<AdverseEventMitigatingActionComponent> mitigatingAction; 2015 2016 /** 2017 * Supporting information relevant to the event. 2018 */ 2019 @Child(name = "supportingInfo", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2020 @Description(shortDefinition="Supporting information relevant to the event", formalDefinition="Supporting information relevant to the event." ) 2021 protected List<AdverseEventSupportingInfoComponent> supportingInfo; 2022 2023 /** 2024 * Comments made about the adverse event by the performer, subject or other participants. 2025 */ 2026 @Child(name = "note", type = {Annotation.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2027 @Description(shortDefinition="Comment on adverse event", formalDefinition="Comments made about the adverse event by the performer, subject or other participants." ) 2028 protected List<Annotation> note; 2029 2030 private static final long serialVersionUID = -1861590732L; 2031 2032 /** 2033 * Constructor 2034 */ 2035 public AdverseEvent() { 2036 super(); 2037 } 2038 2039 /** 2040 * Constructor 2041 */ 2042 public AdverseEvent(AdverseEventStatus status, AdverseEventActuality actuality, Reference subject) { 2043 super(); 2044 this.setStatus(status); 2045 this.setActuality(actuality); 2046 this.setSubject(subject); 2047 } 2048 2049 /** 2050 * @return {@link #identifier} (Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 2051 */ 2052 public List<Identifier> getIdentifier() { 2053 if (this.identifier == null) 2054 this.identifier = new ArrayList<Identifier>(); 2055 return this.identifier; 2056 } 2057 2058 /** 2059 * @return Returns a reference to <code>this</code> for easy method chaining 2060 */ 2061 public AdverseEvent setIdentifier(List<Identifier> theIdentifier) { 2062 this.identifier = theIdentifier; 2063 return this; 2064 } 2065 2066 public boolean hasIdentifier() { 2067 if (this.identifier == null) 2068 return false; 2069 for (Identifier item : this.identifier) 2070 if (!item.isEmpty()) 2071 return true; 2072 return false; 2073 } 2074 2075 public Identifier addIdentifier() { //3 2076 Identifier t = new Identifier(); 2077 if (this.identifier == null) 2078 this.identifier = new ArrayList<Identifier>(); 2079 this.identifier.add(t); 2080 return t; 2081 } 2082 2083 public AdverseEvent addIdentifier(Identifier t) { //3 2084 if (t == null) 2085 return this; 2086 if (this.identifier == null) 2087 this.identifier = new ArrayList<Identifier>(); 2088 this.identifier.add(t); 2089 return this; 2090 } 2091 2092 /** 2093 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2094 */ 2095 public Identifier getIdentifierFirstRep() { 2096 if (getIdentifier().isEmpty()) { 2097 addIdentifier(); 2098 } 2099 return getIdentifier().get(0); 2100 } 2101 2102 /** 2103 * @return {@link #status} (The current state of the adverse event or potential adverse event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2104 */ 2105 public Enumeration<AdverseEventStatus> getStatusElement() { 2106 if (this.status == null) 2107 if (Configuration.errorOnAutoCreate()) 2108 throw new Error("Attempt to auto-create AdverseEvent.status"); 2109 else if (Configuration.doAutoCreate()) 2110 this.status = new Enumeration<AdverseEventStatus>(new AdverseEventStatusEnumFactory()); // bb 2111 return this.status; 2112 } 2113 2114 public boolean hasStatusElement() { 2115 return this.status != null && !this.status.isEmpty(); 2116 } 2117 2118 public boolean hasStatus() { 2119 return this.status != null && !this.status.isEmpty(); 2120 } 2121 2122 /** 2123 * @param value {@link #status} (The current state of the adverse event or potential adverse event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2124 */ 2125 public AdverseEvent setStatusElement(Enumeration<AdverseEventStatus> value) { 2126 this.status = value; 2127 return this; 2128 } 2129 2130 /** 2131 * @return The current state of the adverse event or potential adverse event. 2132 */ 2133 public AdverseEventStatus getStatus() { 2134 return this.status == null ? null : this.status.getValue(); 2135 } 2136 2137 /** 2138 * @param value The current state of the adverse event or potential adverse event. 2139 */ 2140 public AdverseEvent setStatus(AdverseEventStatus value) { 2141 if (this.status == null) 2142 this.status = new Enumeration<AdverseEventStatus>(new AdverseEventStatusEnumFactory()); 2143 this.status.setValue(value); 2144 return this; 2145 } 2146 2147 /** 2148 * @return {@link #actuality} (Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.). This is the underlying object with id, value and extensions. The accessor "getActuality" gives direct access to the value 2149 */ 2150 public Enumeration<AdverseEventActuality> getActualityElement() { 2151 if (this.actuality == null) 2152 if (Configuration.errorOnAutoCreate()) 2153 throw new Error("Attempt to auto-create AdverseEvent.actuality"); 2154 else if (Configuration.doAutoCreate()) 2155 this.actuality = new Enumeration<AdverseEventActuality>(new AdverseEventActualityEnumFactory()); // bb 2156 return this.actuality; 2157 } 2158 2159 public boolean hasActualityElement() { 2160 return this.actuality != null && !this.actuality.isEmpty(); 2161 } 2162 2163 public boolean hasActuality() { 2164 return this.actuality != null && !this.actuality.isEmpty(); 2165 } 2166 2167 /** 2168 * @param value {@link #actuality} (Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.). This is the underlying object with id, value and extensions. The accessor "getActuality" gives direct access to the value 2169 */ 2170 public AdverseEvent setActualityElement(Enumeration<AdverseEventActuality> value) { 2171 this.actuality = value; 2172 return this; 2173 } 2174 2175 /** 2176 * @return Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely. 2177 */ 2178 public AdverseEventActuality getActuality() { 2179 return this.actuality == null ? null : this.actuality.getValue(); 2180 } 2181 2182 /** 2183 * @param value Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely. 2184 */ 2185 public AdverseEvent setActuality(AdverseEventActuality value) { 2186 if (this.actuality == null) 2187 this.actuality = new Enumeration<AdverseEventActuality>(new AdverseEventActualityEnumFactory()); 2188 this.actuality.setValue(value); 2189 return this; 2190 } 2191 2192 /** 2193 * @return {@link #category} (The overall type of event, intended for search and filtering purposes.) 2194 */ 2195 public List<CodeableConcept> getCategory() { 2196 if (this.category == null) 2197 this.category = new ArrayList<CodeableConcept>(); 2198 return this.category; 2199 } 2200 2201 /** 2202 * @return Returns a reference to <code>this</code> for easy method chaining 2203 */ 2204 public AdverseEvent setCategory(List<CodeableConcept> theCategory) { 2205 this.category = theCategory; 2206 return this; 2207 } 2208 2209 public boolean hasCategory() { 2210 if (this.category == null) 2211 return false; 2212 for (CodeableConcept item : this.category) 2213 if (!item.isEmpty()) 2214 return true; 2215 return false; 2216 } 2217 2218 public CodeableConcept addCategory() { //3 2219 CodeableConcept t = new CodeableConcept(); 2220 if (this.category == null) 2221 this.category = new ArrayList<CodeableConcept>(); 2222 this.category.add(t); 2223 return t; 2224 } 2225 2226 public AdverseEvent addCategory(CodeableConcept t) { //3 2227 if (t == null) 2228 return this; 2229 if (this.category == null) 2230 this.category = new ArrayList<CodeableConcept>(); 2231 this.category.add(t); 2232 return this; 2233 } 2234 2235 /** 2236 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2237 */ 2238 public CodeableConcept getCategoryFirstRep() { 2239 if (getCategory().isEmpty()) { 2240 addCategory(); 2241 } 2242 return getCategory().get(0); 2243 } 2244 2245 /** 2246 * @return {@link #code} (Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.) 2247 */ 2248 public CodeableConcept getCode() { 2249 if (this.code == null) 2250 if (Configuration.errorOnAutoCreate()) 2251 throw new Error("Attempt to auto-create AdverseEvent.code"); 2252 else if (Configuration.doAutoCreate()) 2253 this.code = new CodeableConcept(); // cc 2254 return this.code; 2255 } 2256 2257 public boolean hasCode() { 2258 return this.code != null && !this.code.isEmpty(); 2259 } 2260 2261 /** 2262 * @param value {@link #code} (Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.) 2263 */ 2264 public AdverseEvent setCode(CodeableConcept value) { 2265 this.code = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return {@link #subject} (This subject or group impacted by the event.) 2271 */ 2272 public Reference getSubject() { 2273 if (this.subject == null) 2274 if (Configuration.errorOnAutoCreate()) 2275 throw new Error("Attempt to auto-create AdverseEvent.subject"); 2276 else if (Configuration.doAutoCreate()) 2277 this.subject = new Reference(); // cc 2278 return this.subject; 2279 } 2280 2281 public boolean hasSubject() { 2282 return this.subject != null && !this.subject.isEmpty(); 2283 } 2284 2285 /** 2286 * @param value {@link #subject} (This subject or group impacted by the event.) 2287 */ 2288 public AdverseEvent setSubject(Reference value) { 2289 this.subject = value; 2290 return this; 2291 } 2292 2293 /** 2294 * @return {@link #encounter} (The Encounter associated with the start of the AdverseEvent.) 2295 */ 2296 public Reference getEncounter() { 2297 if (this.encounter == null) 2298 if (Configuration.errorOnAutoCreate()) 2299 throw new Error("Attempt to auto-create AdverseEvent.encounter"); 2300 else if (Configuration.doAutoCreate()) 2301 this.encounter = new Reference(); // cc 2302 return this.encounter; 2303 } 2304 2305 public boolean hasEncounter() { 2306 return this.encounter != null && !this.encounter.isEmpty(); 2307 } 2308 2309 /** 2310 * @param value {@link #encounter} (The Encounter associated with the start of the AdverseEvent.) 2311 */ 2312 public AdverseEvent setEncounter(Reference value) { 2313 this.encounter = value; 2314 return this; 2315 } 2316 2317 /** 2318 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2319 */ 2320 public DataType getOccurrence() { 2321 return this.occurrence; 2322 } 2323 2324 /** 2325 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2326 */ 2327 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2328 if (this.occurrence == null) 2329 this.occurrence = new DateTimeType(); 2330 if (!(this.occurrence instanceof DateTimeType)) 2331 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2332 return (DateTimeType) this.occurrence; 2333 } 2334 2335 public boolean hasOccurrenceDateTimeType() { 2336 return this != null && this.occurrence instanceof DateTimeType; 2337 } 2338 2339 /** 2340 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2341 */ 2342 public Period getOccurrencePeriod() throws FHIRException { 2343 if (this.occurrence == null) 2344 this.occurrence = new Period(); 2345 if (!(this.occurrence instanceof Period)) 2346 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2347 return (Period) this.occurrence; 2348 } 2349 2350 public boolean hasOccurrencePeriod() { 2351 return this != null && this.occurrence instanceof Period; 2352 } 2353 2354 /** 2355 * @return {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2356 */ 2357 public Timing getOccurrenceTiming() throws FHIRException { 2358 if (this.occurrence == null) 2359 this.occurrence = new Timing(); 2360 if (!(this.occurrence instanceof Timing)) 2361 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2362 return (Timing) this.occurrence; 2363 } 2364 2365 public boolean hasOccurrenceTiming() { 2366 return this != null && this.occurrence instanceof Timing; 2367 } 2368 2369 public boolean hasOccurrence() { 2370 return this.occurrence != null && !this.occurrence.isEmpty(); 2371 } 2372 2373 /** 2374 * @param value {@link #occurrence} (The date (and perhaps time) when the adverse event occurred.) 2375 */ 2376 public AdverseEvent setOccurrence(DataType value) { 2377 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 2378 throw new FHIRException("Not the right type for AdverseEvent.occurrence[x]: "+value.fhirType()); 2379 this.occurrence = value; 2380 return this; 2381 } 2382 2383 /** 2384 * @return {@link #detected} (Estimated or actual date the AdverseEvent began, in the opinion of the reporter.). This is the underlying object with id, value and extensions. The accessor "getDetected" gives direct access to the value 2385 */ 2386 public DateTimeType getDetectedElement() { 2387 if (this.detected == null) 2388 if (Configuration.errorOnAutoCreate()) 2389 throw new Error("Attempt to auto-create AdverseEvent.detected"); 2390 else if (Configuration.doAutoCreate()) 2391 this.detected = new DateTimeType(); // bb 2392 return this.detected; 2393 } 2394 2395 public boolean hasDetectedElement() { 2396 return this.detected != null && !this.detected.isEmpty(); 2397 } 2398 2399 public boolean hasDetected() { 2400 return this.detected != null && !this.detected.isEmpty(); 2401 } 2402 2403 /** 2404 * @param value {@link #detected} (Estimated or actual date the AdverseEvent began, in the opinion of the reporter.). This is the underlying object with id, value and extensions. The accessor "getDetected" gives direct access to the value 2405 */ 2406 public AdverseEvent setDetectedElement(DateTimeType value) { 2407 this.detected = value; 2408 return this; 2409 } 2410 2411 /** 2412 * @return Estimated or actual date the AdverseEvent began, in the opinion of the reporter. 2413 */ 2414 public Date getDetected() { 2415 return this.detected == null ? null : this.detected.getValue(); 2416 } 2417 2418 /** 2419 * @param value Estimated or actual date the AdverseEvent began, in the opinion of the reporter. 2420 */ 2421 public AdverseEvent setDetected(Date value) { 2422 if (value == null) 2423 this.detected = null; 2424 else { 2425 if (this.detected == null) 2426 this.detected = new DateTimeType(); 2427 this.detected.setValue(value); 2428 } 2429 return this; 2430 } 2431 2432 /** 2433 * @return {@link #recordedDate} (The date on which the existence of the AdverseEvent was first recorded.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 2434 */ 2435 public DateTimeType getRecordedDateElement() { 2436 if (this.recordedDate == null) 2437 if (Configuration.errorOnAutoCreate()) 2438 throw new Error("Attempt to auto-create AdverseEvent.recordedDate"); 2439 else if (Configuration.doAutoCreate()) 2440 this.recordedDate = new DateTimeType(); // bb 2441 return this.recordedDate; 2442 } 2443 2444 public boolean hasRecordedDateElement() { 2445 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2446 } 2447 2448 public boolean hasRecordedDate() { 2449 return this.recordedDate != null && !this.recordedDate.isEmpty(); 2450 } 2451 2452 /** 2453 * @param value {@link #recordedDate} (The date on which the existence of the AdverseEvent was first recorded.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 2454 */ 2455 public AdverseEvent setRecordedDateElement(DateTimeType value) { 2456 this.recordedDate = value; 2457 return this; 2458 } 2459 2460 /** 2461 * @return The date on which the existence of the AdverseEvent was first recorded. 2462 */ 2463 public Date getRecordedDate() { 2464 return this.recordedDate == null ? null : this.recordedDate.getValue(); 2465 } 2466 2467 /** 2468 * @param value The date on which the existence of the AdverseEvent was first recorded. 2469 */ 2470 public AdverseEvent setRecordedDate(Date value) { 2471 if (value == null) 2472 this.recordedDate = null; 2473 else { 2474 if (this.recordedDate == null) 2475 this.recordedDate = new DateTimeType(); 2476 this.recordedDate.setValue(value); 2477 } 2478 return this; 2479 } 2480 2481 /** 2482 * @return {@link #resultingEffect} (Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall.) 2483 */ 2484 public List<Reference> getResultingEffect() { 2485 if (this.resultingEffect == null) 2486 this.resultingEffect = new ArrayList<Reference>(); 2487 return this.resultingEffect; 2488 } 2489 2490 /** 2491 * @return Returns a reference to <code>this</code> for easy method chaining 2492 */ 2493 public AdverseEvent setResultingEffect(List<Reference> theResultingEffect) { 2494 this.resultingEffect = theResultingEffect; 2495 return this; 2496 } 2497 2498 public boolean hasResultingEffect() { 2499 if (this.resultingEffect == null) 2500 return false; 2501 for (Reference item : this.resultingEffect) 2502 if (!item.isEmpty()) 2503 return true; 2504 return false; 2505 } 2506 2507 public Reference addResultingEffect() { //3 2508 Reference t = new Reference(); 2509 if (this.resultingEffect == null) 2510 this.resultingEffect = new ArrayList<Reference>(); 2511 this.resultingEffect.add(t); 2512 return t; 2513 } 2514 2515 public AdverseEvent addResultingEffect(Reference t) { //3 2516 if (t == null) 2517 return this; 2518 if (this.resultingEffect == null) 2519 this.resultingEffect = new ArrayList<Reference>(); 2520 this.resultingEffect.add(t); 2521 return this; 2522 } 2523 2524 /** 2525 * @return The first repetition of repeating field {@link #resultingEffect}, creating it if it does not already exist {3} 2526 */ 2527 public Reference getResultingEffectFirstRep() { 2528 if (getResultingEffect().isEmpty()) { 2529 addResultingEffect(); 2530 } 2531 return getResultingEffect().get(0); 2532 } 2533 2534 /** 2535 * @return {@link #location} (The information about where the adverse event occurred.) 2536 */ 2537 public Reference getLocation() { 2538 if (this.location == null) 2539 if (Configuration.errorOnAutoCreate()) 2540 throw new Error("Attempt to auto-create AdverseEvent.location"); 2541 else if (Configuration.doAutoCreate()) 2542 this.location = new Reference(); // cc 2543 return this.location; 2544 } 2545 2546 public boolean hasLocation() { 2547 return this.location != null && !this.location.isEmpty(); 2548 } 2549 2550 /** 2551 * @param value {@link #location} (The information about where the adverse event occurred.) 2552 */ 2553 public AdverseEvent setLocation(Reference value) { 2554 this.location = value; 2555 return this; 2556 } 2557 2558 /** 2559 * @return {@link #seriousness} (Assessment whether this event, or averted event, was of clinical importance.) 2560 */ 2561 public CodeableConcept getSeriousness() { 2562 if (this.seriousness == null) 2563 if (Configuration.errorOnAutoCreate()) 2564 throw new Error("Attempt to auto-create AdverseEvent.seriousness"); 2565 else if (Configuration.doAutoCreate()) 2566 this.seriousness = new CodeableConcept(); // cc 2567 return this.seriousness; 2568 } 2569 2570 public boolean hasSeriousness() { 2571 return this.seriousness != null && !this.seriousness.isEmpty(); 2572 } 2573 2574 /** 2575 * @param value {@link #seriousness} (Assessment whether this event, or averted event, was of clinical importance.) 2576 */ 2577 public AdverseEvent setSeriousness(CodeableConcept value) { 2578 this.seriousness = value; 2579 return this; 2580 } 2581 2582 /** 2583 * @return {@link #outcome} (Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal.) 2584 */ 2585 public List<CodeableConcept> getOutcome() { 2586 if (this.outcome == null) 2587 this.outcome = new ArrayList<CodeableConcept>(); 2588 return this.outcome; 2589 } 2590 2591 /** 2592 * @return Returns a reference to <code>this</code> for easy method chaining 2593 */ 2594 public AdverseEvent setOutcome(List<CodeableConcept> theOutcome) { 2595 this.outcome = theOutcome; 2596 return this; 2597 } 2598 2599 public boolean hasOutcome() { 2600 if (this.outcome == null) 2601 return false; 2602 for (CodeableConcept item : this.outcome) 2603 if (!item.isEmpty()) 2604 return true; 2605 return false; 2606 } 2607 2608 public CodeableConcept addOutcome() { //3 2609 CodeableConcept t = new CodeableConcept(); 2610 if (this.outcome == null) 2611 this.outcome = new ArrayList<CodeableConcept>(); 2612 this.outcome.add(t); 2613 return t; 2614 } 2615 2616 public AdverseEvent addOutcome(CodeableConcept t) { //3 2617 if (t == null) 2618 return this; 2619 if (this.outcome == null) 2620 this.outcome = new ArrayList<CodeableConcept>(); 2621 this.outcome.add(t); 2622 return this; 2623 } 2624 2625 /** 2626 * @return The first repetition of repeating field {@link #outcome}, creating it if it does not already exist {3} 2627 */ 2628 public CodeableConcept getOutcomeFirstRep() { 2629 if (getOutcome().isEmpty()) { 2630 addOutcome(); 2631 } 2632 return getOutcome().get(0); 2633 } 2634 2635 /** 2636 * @return {@link #recorder} (Information on who recorded the adverse event. May be the patient or a practitioner.) 2637 */ 2638 public Reference getRecorder() { 2639 if (this.recorder == null) 2640 if (Configuration.errorOnAutoCreate()) 2641 throw new Error("Attempt to auto-create AdverseEvent.recorder"); 2642 else if (Configuration.doAutoCreate()) 2643 this.recorder = new Reference(); // cc 2644 return this.recorder; 2645 } 2646 2647 public boolean hasRecorder() { 2648 return this.recorder != null && !this.recorder.isEmpty(); 2649 } 2650 2651 /** 2652 * @param value {@link #recorder} (Information on who recorded the adverse event. May be the patient or a practitioner.) 2653 */ 2654 public AdverseEvent setRecorder(Reference value) { 2655 this.recorder = value; 2656 return this; 2657 } 2658 2659 /** 2660 * @return {@link #participant} (Indicates who or what participated in the adverse event and how they were involved.) 2661 */ 2662 public List<AdverseEventParticipantComponent> getParticipant() { 2663 if (this.participant == null) 2664 this.participant = new ArrayList<AdverseEventParticipantComponent>(); 2665 return this.participant; 2666 } 2667 2668 /** 2669 * @return Returns a reference to <code>this</code> for easy method chaining 2670 */ 2671 public AdverseEvent setParticipant(List<AdverseEventParticipantComponent> theParticipant) { 2672 this.participant = theParticipant; 2673 return this; 2674 } 2675 2676 public boolean hasParticipant() { 2677 if (this.participant == null) 2678 return false; 2679 for (AdverseEventParticipantComponent item : this.participant) 2680 if (!item.isEmpty()) 2681 return true; 2682 return false; 2683 } 2684 2685 public AdverseEventParticipantComponent addParticipant() { //3 2686 AdverseEventParticipantComponent t = new AdverseEventParticipantComponent(); 2687 if (this.participant == null) 2688 this.participant = new ArrayList<AdverseEventParticipantComponent>(); 2689 this.participant.add(t); 2690 return t; 2691 } 2692 2693 public AdverseEvent addParticipant(AdverseEventParticipantComponent t) { //3 2694 if (t == null) 2695 return this; 2696 if (this.participant == null) 2697 this.participant = new ArrayList<AdverseEventParticipantComponent>(); 2698 this.participant.add(t); 2699 return this; 2700 } 2701 2702 /** 2703 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 2704 */ 2705 public AdverseEventParticipantComponent getParticipantFirstRep() { 2706 if (getParticipant().isEmpty()) { 2707 addParticipant(); 2708 } 2709 return getParticipant().get(0); 2710 } 2711 2712 /** 2713 * @return {@link #study} (The research study that the subject is enrolled in.) 2714 */ 2715 public List<Reference> getStudy() { 2716 if (this.study == null) 2717 this.study = new ArrayList<Reference>(); 2718 return this.study; 2719 } 2720 2721 /** 2722 * @return Returns a reference to <code>this</code> for easy method chaining 2723 */ 2724 public AdverseEvent setStudy(List<Reference> theStudy) { 2725 this.study = theStudy; 2726 return this; 2727 } 2728 2729 public boolean hasStudy() { 2730 if (this.study == null) 2731 return false; 2732 for (Reference item : this.study) 2733 if (!item.isEmpty()) 2734 return true; 2735 return false; 2736 } 2737 2738 public Reference addStudy() { //3 2739 Reference t = new Reference(); 2740 if (this.study == null) 2741 this.study = new ArrayList<Reference>(); 2742 this.study.add(t); 2743 return t; 2744 } 2745 2746 public AdverseEvent addStudy(Reference t) { //3 2747 if (t == null) 2748 return this; 2749 if (this.study == null) 2750 this.study = new ArrayList<Reference>(); 2751 this.study.add(t); 2752 return this; 2753 } 2754 2755 /** 2756 * @return The first repetition of repeating field {@link #study}, creating it if it does not already exist {3} 2757 */ 2758 public Reference getStudyFirstRep() { 2759 if (getStudy().isEmpty()) { 2760 addStudy(); 2761 } 2762 return getStudy().get(0); 2763 } 2764 2765 /** 2766 * @return {@link #expectedInResearchStudy} (Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.). This is the underlying object with id, value and extensions. The accessor "getExpectedInResearchStudy" gives direct access to the value 2767 */ 2768 public BooleanType getExpectedInResearchStudyElement() { 2769 if (this.expectedInResearchStudy == null) 2770 if (Configuration.errorOnAutoCreate()) 2771 throw new Error("Attempt to auto-create AdverseEvent.expectedInResearchStudy"); 2772 else if (Configuration.doAutoCreate()) 2773 this.expectedInResearchStudy = new BooleanType(); // bb 2774 return this.expectedInResearchStudy; 2775 } 2776 2777 public boolean hasExpectedInResearchStudyElement() { 2778 return this.expectedInResearchStudy != null && !this.expectedInResearchStudy.isEmpty(); 2779 } 2780 2781 public boolean hasExpectedInResearchStudy() { 2782 return this.expectedInResearchStudy != null && !this.expectedInResearchStudy.isEmpty(); 2783 } 2784 2785 /** 2786 * @param value {@link #expectedInResearchStudy} (Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.). This is the underlying object with id, value and extensions. The accessor "getExpectedInResearchStudy" gives direct access to the value 2787 */ 2788 public AdverseEvent setExpectedInResearchStudyElement(BooleanType value) { 2789 this.expectedInResearchStudy = value; 2790 return this; 2791 } 2792 2793 /** 2794 * @return Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely. 2795 */ 2796 public boolean getExpectedInResearchStudy() { 2797 return this.expectedInResearchStudy == null || this.expectedInResearchStudy.isEmpty() ? false : this.expectedInResearchStudy.getValue(); 2798 } 2799 2800 /** 2801 * @param value Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely. 2802 */ 2803 public AdverseEvent setExpectedInResearchStudy(boolean value) { 2804 if (this.expectedInResearchStudy == null) 2805 this.expectedInResearchStudy = new BooleanType(); 2806 this.expectedInResearchStudy.setValue(value); 2807 return this; 2808 } 2809 2810 /** 2811 * @return {@link #suspectEntity} (Describes the entity that is suspected to have caused the adverse event.) 2812 */ 2813 public List<AdverseEventSuspectEntityComponent> getSuspectEntity() { 2814 if (this.suspectEntity == null) 2815 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2816 return this.suspectEntity; 2817 } 2818 2819 /** 2820 * @return Returns a reference to <code>this</code> for easy method chaining 2821 */ 2822 public AdverseEvent setSuspectEntity(List<AdverseEventSuspectEntityComponent> theSuspectEntity) { 2823 this.suspectEntity = theSuspectEntity; 2824 return this; 2825 } 2826 2827 public boolean hasSuspectEntity() { 2828 if (this.suspectEntity == null) 2829 return false; 2830 for (AdverseEventSuspectEntityComponent item : this.suspectEntity) 2831 if (!item.isEmpty()) 2832 return true; 2833 return false; 2834 } 2835 2836 public AdverseEventSuspectEntityComponent addSuspectEntity() { //3 2837 AdverseEventSuspectEntityComponent t = new AdverseEventSuspectEntityComponent(); 2838 if (this.suspectEntity == null) 2839 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2840 this.suspectEntity.add(t); 2841 return t; 2842 } 2843 2844 public AdverseEvent addSuspectEntity(AdverseEventSuspectEntityComponent t) { //3 2845 if (t == null) 2846 return this; 2847 if (this.suspectEntity == null) 2848 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2849 this.suspectEntity.add(t); 2850 return this; 2851 } 2852 2853 /** 2854 * @return The first repetition of repeating field {@link #suspectEntity}, creating it if it does not already exist {3} 2855 */ 2856 public AdverseEventSuspectEntityComponent getSuspectEntityFirstRep() { 2857 if (getSuspectEntity().isEmpty()) { 2858 addSuspectEntity(); 2859 } 2860 return getSuspectEntity().get(0); 2861 } 2862 2863 /** 2864 * @return {@link #contributingFactor} (The contributing factors suspected to have increased the probability or severity of the adverse event.) 2865 */ 2866 public List<AdverseEventContributingFactorComponent> getContributingFactor() { 2867 if (this.contributingFactor == null) 2868 this.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 2869 return this.contributingFactor; 2870 } 2871 2872 /** 2873 * @return Returns a reference to <code>this</code> for easy method chaining 2874 */ 2875 public AdverseEvent setContributingFactor(List<AdverseEventContributingFactorComponent> theContributingFactor) { 2876 this.contributingFactor = theContributingFactor; 2877 return this; 2878 } 2879 2880 public boolean hasContributingFactor() { 2881 if (this.contributingFactor == null) 2882 return false; 2883 for (AdverseEventContributingFactorComponent item : this.contributingFactor) 2884 if (!item.isEmpty()) 2885 return true; 2886 return false; 2887 } 2888 2889 public AdverseEventContributingFactorComponent addContributingFactor() { //3 2890 AdverseEventContributingFactorComponent t = new AdverseEventContributingFactorComponent(); 2891 if (this.contributingFactor == null) 2892 this.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 2893 this.contributingFactor.add(t); 2894 return t; 2895 } 2896 2897 public AdverseEvent addContributingFactor(AdverseEventContributingFactorComponent t) { //3 2898 if (t == null) 2899 return this; 2900 if (this.contributingFactor == null) 2901 this.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 2902 this.contributingFactor.add(t); 2903 return this; 2904 } 2905 2906 /** 2907 * @return The first repetition of repeating field {@link #contributingFactor}, creating it if it does not already exist {3} 2908 */ 2909 public AdverseEventContributingFactorComponent getContributingFactorFirstRep() { 2910 if (getContributingFactor().isEmpty()) { 2911 addContributingFactor(); 2912 } 2913 return getContributingFactor().get(0); 2914 } 2915 2916 /** 2917 * @return {@link #preventiveAction} (Preventive actions that contributed to avoiding the adverse event.) 2918 */ 2919 public List<AdverseEventPreventiveActionComponent> getPreventiveAction() { 2920 if (this.preventiveAction == null) 2921 this.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 2922 return this.preventiveAction; 2923 } 2924 2925 /** 2926 * @return Returns a reference to <code>this</code> for easy method chaining 2927 */ 2928 public AdverseEvent setPreventiveAction(List<AdverseEventPreventiveActionComponent> thePreventiveAction) { 2929 this.preventiveAction = thePreventiveAction; 2930 return this; 2931 } 2932 2933 public boolean hasPreventiveAction() { 2934 if (this.preventiveAction == null) 2935 return false; 2936 for (AdverseEventPreventiveActionComponent item : this.preventiveAction) 2937 if (!item.isEmpty()) 2938 return true; 2939 return false; 2940 } 2941 2942 public AdverseEventPreventiveActionComponent addPreventiveAction() { //3 2943 AdverseEventPreventiveActionComponent t = new AdverseEventPreventiveActionComponent(); 2944 if (this.preventiveAction == null) 2945 this.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 2946 this.preventiveAction.add(t); 2947 return t; 2948 } 2949 2950 public AdverseEvent addPreventiveAction(AdverseEventPreventiveActionComponent t) { //3 2951 if (t == null) 2952 return this; 2953 if (this.preventiveAction == null) 2954 this.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 2955 this.preventiveAction.add(t); 2956 return this; 2957 } 2958 2959 /** 2960 * @return The first repetition of repeating field {@link #preventiveAction}, creating it if it does not already exist {3} 2961 */ 2962 public AdverseEventPreventiveActionComponent getPreventiveActionFirstRep() { 2963 if (getPreventiveAction().isEmpty()) { 2964 addPreventiveAction(); 2965 } 2966 return getPreventiveAction().get(0); 2967 } 2968 2969 /** 2970 * @return {@link #mitigatingAction} (The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.) 2971 */ 2972 public List<AdverseEventMitigatingActionComponent> getMitigatingAction() { 2973 if (this.mitigatingAction == null) 2974 this.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 2975 return this.mitigatingAction; 2976 } 2977 2978 /** 2979 * @return Returns a reference to <code>this</code> for easy method chaining 2980 */ 2981 public AdverseEvent setMitigatingAction(List<AdverseEventMitigatingActionComponent> theMitigatingAction) { 2982 this.mitigatingAction = theMitigatingAction; 2983 return this; 2984 } 2985 2986 public boolean hasMitigatingAction() { 2987 if (this.mitigatingAction == null) 2988 return false; 2989 for (AdverseEventMitigatingActionComponent item : this.mitigatingAction) 2990 if (!item.isEmpty()) 2991 return true; 2992 return false; 2993 } 2994 2995 public AdverseEventMitigatingActionComponent addMitigatingAction() { //3 2996 AdverseEventMitigatingActionComponent t = new AdverseEventMitigatingActionComponent(); 2997 if (this.mitigatingAction == null) 2998 this.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 2999 this.mitigatingAction.add(t); 3000 return t; 3001 } 3002 3003 public AdverseEvent addMitigatingAction(AdverseEventMitigatingActionComponent t) { //3 3004 if (t == null) 3005 return this; 3006 if (this.mitigatingAction == null) 3007 this.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 3008 this.mitigatingAction.add(t); 3009 return this; 3010 } 3011 3012 /** 3013 * @return The first repetition of repeating field {@link #mitigatingAction}, creating it if it does not already exist {3} 3014 */ 3015 public AdverseEventMitigatingActionComponent getMitigatingActionFirstRep() { 3016 if (getMitigatingAction().isEmpty()) { 3017 addMitigatingAction(); 3018 } 3019 return getMitigatingAction().get(0); 3020 } 3021 3022 /** 3023 * @return {@link #supportingInfo} (Supporting information relevant to the event.) 3024 */ 3025 public List<AdverseEventSupportingInfoComponent> getSupportingInfo() { 3026 if (this.supportingInfo == null) 3027 this.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3028 return this.supportingInfo; 3029 } 3030 3031 /** 3032 * @return Returns a reference to <code>this</code> for easy method chaining 3033 */ 3034 public AdverseEvent setSupportingInfo(List<AdverseEventSupportingInfoComponent> theSupportingInfo) { 3035 this.supportingInfo = theSupportingInfo; 3036 return this; 3037 } 3038 3039 public boolean hasSupportingInfo() { 3040 if (this.supportingInfo == null) 3041 return false; 3042 for (AdverseEventSupportingInfoComponent item : this.supportingInfo) 3043 if (!item.isEmpty()) 3044 return true; 3045 return false; 3046 } 3047 3048 public AdverseEventSupportingInfoComponent addSupportingInfo() { //3 3049 AdverseEventSupportingInfoComponent t = new AdverseEventSupportingInfoComponent(); 3050 if (this.supportingInfo == null) 3051 this.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3052 this.supportingInfo.add(t); 3053 return t; 3054 } 3055 3056 public AdverseEvent addSupportingInfo(AdverseEventSupportingInfoComponent t) { //3 3057 if (t == null) 3058 return this; 3059 if (this.supportingInfo == null) 3060 this.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3061 this.supportingInfo.add(t); 3062 return this; 3063 } 3064 3065 /** 3066 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 3067 */ 3068 public AdverseEventSupportingInfoComponent getSupportingInfoFirstRep() { 3069 if (getSupportingInfo().isEmpty()) { 3070 addSupportingInfo(); 3071 } 3072 return getSupportingInfo().get(0); 3073 } 3074 3075 /** 3076 * @return {@link #note} (Comments made about the adverse event by the performer, subject or other participants.) 3077 */ 3078 public List<Annotation> getNote() { 3079 if (this.note == null) 3080 this.note = new ArrayList<Annotation>(); 3081 return this.note; 3082 } 3083 3084 /** 3085 * @return Returns a reference to <code>this</code> for easy method chaining 3086 */ 3087 public AdverseEvent setNote(List<Annotation> theNote) { 3088 this.note = theNote; 3089 return this; 3090 } 3091 3092 public boolean hasNote() { 3093 if (this.note == null) 3094 return false; 3095 for (Annotation item : this.note) 3096 if (!item.isEmpty()) 3097 return true; 3098 return false; 3099 } 3100 3101 public Annotation addNote() { //3 3102 Annotation t = new Annotation(); 3103 if (this.note == null) 3104 this.note = new ArrayList<Annotation>(); 3105 this.note.add(t); 3106 return t; 3107 } 3108 3109 public AdverseEvent addNote(Annotation t) { //3 3110 if (t == null) 3111 return this; 3112 if (this.note == null) 3113 this.note = new ArrayList<Annotation>(); 3114 this.note.add(t); 3115 return this; 3116 } 3117 3118 /** 3119 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3120 */ 3121 public Annotation getNoteFirstRep() { 3122 if (getNote().isEmpty()) { 3123 addNote(); 3124 } 3125 return getNote().get(0); 3126 } 3127 3128 protected void listChildren(List<Property> children) { 3129 super.listChildren(children); 3130 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3131 children.add(new Property("status", "code", "The current state of the adverse event or potential adverse event.", 0, 1, status)); 3132 children.add(new Property("actuality", "code", "Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.", 0, 1, actuality)); 3133 children.add(new Property("category", "CodeableConcept", "The overall type of event, intended for search and filtering purposes.", 0, java.lang.Integer.MAX_VALUE, category)); 3134 children.add(new Property("code", "CodeableConcept", "Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.", 0, 1, code)); 3135 children.add(new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|ResearchSubject)", "This subject or group impacted by the event.", 0, 1, subject)); 3136 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter associated with the start of the AdverseEvent.", 0, 1, encounter)); 3137 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence)); 3138 children.add(new Property("detected", "dateTime", "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.", 0, 1, detected)); 3139 children.add(new Property("recordedDate", "dateTime", "The date on which the existence of the AdverseEvent was first recorded.", 0, 1, recordedDate)); 3140 children.add(new Property("resultingEffect", "Reference(Condition|Observation)", "Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall.", 0, java.lang.Integer.MAX_VALUE, resultingEffect)); 3141 children.add(new Property("location", "Reference(Location)", "The information about where the adverse event occurred.", 0, 1, location)); 3142 children.add(new Property("seriousness", "CodeableConcept", "Assessment whether this event, or averted event, was of clinical importance.", 0, 1, seriousness)); 3143 children.add(new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal.", 0, java.lang.Integer.MAX_VALUE, outcome)); 3144 children.add(new Property("recorder", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|ResearchSubject)", "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder)); 3145 children.add(new Property("participant", "", "Indicates who or what participated in the adverse event and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant)); 3146 children.add(new Property("study", "Reference(ResearchStudy)", "The research study that the subject is enrolled in.", 0, java.lang.Integer.MAX_VALUE, study)); 3147 children.add(new Property("expectedInResearchStudy", "boolean", "Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.", 0, 1, expectedInResearchStudy)); 3148 children.add(new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, suspectEntity)); 3149 children.add(new Property("contributingFactor", "", "The contributing factors suspected to have increased the probability or severity of the adverse event.", 0, java.lang.Integer.MAX_VALUE, contributingFactor)); 3150 children.add(new Property("preventiveAction", "", "Preventive actions that contributed to avoiding the adverse event.", 0, java.lang.Integer.MAX_VALUE, preventiveAction)); 3151 children.add(new Property("mitigatingAction", "", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, java.lang.Integer.MAX_VALUE, mitigatingAction)); 3152 children.add(new Property("supportingInfo", "", "Supporting information relevant to the event.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 3153 children.add(new Property("note", "Annotation", "Comments made about the adverse event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 3154 } 3155 3156 @Override 3157 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3158 switch (_hash) { 3159 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3160 case -892481550: /*status*/ return new Property("status", "code", "The current state of the adverse event or potential adverse event.", 0, 1, status); 3161 case 528866400: /*actuality*/ return new Property("actuality", "code", "Whether the event actually happened or was a near miss. Note that this is independent of whether anyone was affected or harmed or how severely.", 0, 1, actuality); 3162 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The overall type of event, intended for search and filtering purposes.", 0, java.lang.Integer.MAX_VALUE, category); 3163 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Specific event that occurred or that was averted, such as patient fall, wrong organ removed, or wrong blood transfused.", 0, 1, code); 3164 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|ResearchSubject)", "This subject or group impacted by the event.", 0, 1, subject); 3165 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter associated with the start of the AdverseEvent.", 0, 1, encounter); 3166 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3167 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3168 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3169 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3170 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "The date (and perhaps time) when the adverse event occurred.", 0, 1, occurrence); 3171 case 1048254082: /*detected*/ return new Property("detected", "dateTime", "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.", 0, 1, detected); 3172 case -1952893826: /*recordedDate*/ return new Property("recordedDate", "dateTime", "The date on which the existence of the AdverseEvent was first recorded.", 0, 1, recordedDate); 3173 case -2113579882: /*resultingEffect*/ return new Property("resultingEffect", "Reference(Condition|Observation)", "Information about the condition that occurred as a result of the adverse event, such as hives due to the exposure to a substance (for example, a drug or a chemical) or a broken leg as a result of the fall.", 0, java.lang.Integer.MAX_VALUE, resultingEffect); 3174 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The information about where the adverse event occurred.", 0, 1, location); 3175 case -1551003909: /*seriousness*/ return new Property("seriousness", "CodeableConcept", "Assessment whether this event, or averted event, was of clinical importance.", 0, 1, seriousness); 3176 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event, such as resolved, recovering, ongoing, resolved-with-sequelae, or fatal.", 0, java.lang.Integer.MAX_VALUE, outcome); 3177 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|ResearchSubject)", "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder); 3178 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what participated in the adverse event and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant); 3179 case 109776329: /*study*/ return new Property("study", "Reference(ResearchStudy)", "The research study that the subject is enrolled in.", 0, java.lang.Integer.MAX_VALUE, study); 3180 case -1071467023: /*expectedInResearchStudy*/ return new Property("expectedInResearchStudy", "boolean", "Considered likely or probable or anticipated in the research study. Whether the reported event matches any of the outcomes for the patient that are considered by the study as known or likely.", 0, 1, expectedInResearchStudy); 3181 case -1957422662: /*suspectEntity*/ return new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, suspectEntity); 3182 case -219647527: /*contributingFactor*/ return new Property("contributingFactor", "", "The contributing factors suspected to have increased the probability or severity of the adverse event.", 0, java.lang.Integer.MAX_VALUE, contributingFactor); 3183 case 2052341334: /*preventiveAction*/ return new Property("preventiveAction", "", "Preventive actions that contributed to avoiding the adverse event.", 0, java.lang.Integer.MAX_VALUE, preventiveAction); 3184 case 1992862383: /*mitigatingAction*/ return new Property("mitigatingAction", "", "The ameliorating action taken after the adverse event occured in order to reduce the extent of harm.", 0, java.lang.Integer.MAX_VALUE, mitigatingAction); 3185 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Supporting information relevant to the event.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 3186 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the adverse event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 3187 default: return super.getNamedProperty(_hash, _name, _checkValid); 3188 } 3189 3190 } 3191 3192 @Override 3193 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3194 switch (hash) { 3195 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3196 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<AdverseEventStatus> 3197 case 528866400: /*actuality*/ return this.actuality == null ? new Base[0] : new Base[] {this.actuality}; // Enumeration<AdverseEventActuality> 3198 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3199 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3200 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3201 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3202 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 3203 case 1048254082: /*detected*/ return this.detected == null ? new Base[0] : new Base[] {this.detected}; // DateTimeType 3204 case -1952893826: /*recordedDate*/ return this.recordedDate == null ? new Base[0] : new Base[] {this.recordedDate}; // DateTimeType 3205 case -2113579882: /*resultingEffect*/ return this.resultingEffect == null ? new Base[0] : this.resultingEffect.toArray(new Base[this.resultingEffect.size()]); // Reference 3206 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 3207 case -1551003909: /*seriousness*/ return this.seriousness == null ? new Base[0] : new Base[] {this.seriousness}; // CodeableConcept 3208 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : this.outcome.toArray(new Base[this.outcome.size()]); // CodeableConcept 3209 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 3210 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // AdverseEventParticipantComponent 3211 case 109776329: /*study*/ return this.study == null ? new Base[0] : this.study.toArray(new Base[this.study.size()]); // Reference 3212 case -1071467023: /*expectedInResearchStudy*/ return this.expectedInResearchStudy == null ? new Base[0] : new Base[] {this.expectedInResearchStudy}; // BooleanType 3213 case -1957422662: /*suspectEntity*/ return this.suspectEntity == null ? new Base[0] : this.suspectEntity.toArray(new Base[this.suspectEntity.size()]); // AdverseEventSuspectEntityComponent 3214 case -219647527: /*contributingFactor*/ return this.contributingFactor == null ? new Base[0] : this.contributingFactor.toArray(new Base[this.contributingFactor.size()]); // AdverseEventContributingFactorComponent 3215 case 2052341334: /*preventiveAction*/ return this.preventiveAction == null ? new Base[0] : this.preventiveAction.toArray(new Base[this.preventiveAction.size()]); // AdverseEventPreventiveActionComponent 3216 case 1992862383: /*mitigatingAction*/ return this.mitigatingAction == null ? new Base[0] : this.mitigatingAction.toArray(new Base[this.mitigatingAction.size()]); // AdverseEventMitigatingActionComponent 3217 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // AdverseEventSupportingInfoComponent 3218 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3219 default: return super.getProperty(hash, name, checkValid); 3220 } 3221 3222 } 3223 3224 @Override 3225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3226 switch (hash) { 3227 case -1618432855: // identifier 3228 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3229 return value; 3230 case -892481550: // status 3231 value = new AdverseEventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3232 this.status = (Enumeration) value; // Enumeration<AdverseEventStatus> 3233 return value; 3234 case 528866400: // actuality 3235 value = new AdverseEventActualityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3236 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 3237 return value; 3238 case 50511102: // category 3239 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3240 return value; 3241 case 3059181: // code 3242 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3243 return value; 3244 case -1867885268: // subject 3245 this.subject = TypeConvertor.castToReference(value); // Reference 3246 return value; 3247 case 1524132147: // encounter 3248 this.encounter = TypeConvertor.castToReference(value); // Reference 3249 return value; 3250 case 1687874001: // occurrence 3251 this.occurrence = TypeConvertor.castToType(value); // DataType 3252 return value; 3253 case 1048254082: // detected 3254 this.detected = TypeConvertor.castToDateTime(value); // DateTimeType 3255 return value; 3256 case -1952893826: // recordedDate 3257 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 3258 return value; 3259 case -2113579882: // resultingEffect 3260 this.getResultingEffect().add(TypeConvertor.castToReference(value)); // Reference 3261 return value; 3262 case 1901043637: // location 3263 this.location = TypeConvertor.castToReference(value); // Reference 3264 return value; 3265 case -1551003909: // seriousness 3266 this.seriousness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3267 return value; 3268 case -1106507950: // outcome 3269 this.getOutcome().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3270 return value; 3271 case -799233858: // recorder 3272 this.recorder = TypeConvertor.castToReference(value); // Reference 3273 return value; 3274 case 767422259: // participant 3275 this.getParticipant().add((AdverseEventParticipantComponent) value); // AdverseEventParticipantComponent 3276 return value; 3277 case 109776329: // study 3278 this.getStudy().add(TypeConvertor.castToReference(value)); // Reference 3279 return value; 3280 case -1071467023: // expectedInResearchStudy 3281 this.expectedInResearchStudy = TypeConvertor.castToBoolean(value); // BooleanType 3282 return value; 3283 case -1957422662: // suspectEntity 3284 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); // AdverseEventSuspectEntityComponent 3285 return value; 3286 case -219647527: // contributingFactor 3287 this.getContributingFactor().add((AdverseEventContributingFactorComponent) value); // AdverseEventContributingFactorComponent 3288 return value; 3289 case 2052341334: // preventiveAction 3290 this.getPreventiveAction().add((AdverseEventPreventiveActionComponent) value); // AdverseEventPreventiveActionComponent 3291 return value; 3292 case 1992862383: // mitigatingAction 3293 this.getMitigatingAction().add((AdverseEventMitigatingActionComponent) value); // AdverseEventMitigatingActionComponent 3294 return value; 3295 case 1922406657: // supportingInfo 3296 this.getSupportingInfo().add((AdverseEventSupportingInfoComponent) value); // AdverseEventSupportingInfoComponent 3297 return value; 3298 case 3387378: // note 3299 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3300 return value; 3301 default: return super.setProperty(hash, name, value); 3302 } 3303 3304 } 3305 3306 @Override 3307 public Base setProperty(String name, Base value) throws FHIRException { 3308 if (name.equals("identifier")) { 3309 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3310 } else if (name.equals("status")) { 3311 value = new AdverseEventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3312 this.status = (Enumeration) value; // Enumeration<AdverseEventStatus> 3313 } else if (name.equals("actuality")) { 3314 value = new AdverseEventActualityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3315 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 3316 } else if (name.equals("category")) { 3317 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3318 } else if (name.equals("code")) { 3319 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3320 } else if (name.equals("subject")) { 3321 this.subject = TypeConvertor.castToReference(value); // Reference 3322 } else if (name.equals("encounter")) { 3323 this.encounter = TypeConvertor.castToReference(value); // Reference 3324 } else if (name.equals("occurrence[x]")) { 3325 this.occurrence = TypeConvertor.castToType(value); // DataType 3326 } else if (name.equals("detected")) { 3327 this.detected = TypeConvertor.castToDateTime(value); // DateTimeType 3328 } else if (name.equals("recordedDate")) { 3329 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 3330 } else if (name.equals("resultingEffect")) { 3331 this.getResultingEffect().add(TypeConvertor.castToReference(value)); 3332 } else if (name.equals("location")) { 3333 this.location = TypeConvertor.castToReference(value); // Reference 3334 } else if (name.equals("seriousness")) { 3335 this.seriousness = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3336 } else if (name.equals("outcome")) { 3337 this.getOutcome().add(TypeConvertor.castToCodeableConcept(value)); 3338 } else if (name.equals("recorder")) { 3339 this.recorder = TypeConvertor.castToReference(value); // Reference 3340 } else if (name.equals("participant")) { 3341 this.getParticipant().add((AdverseEventParticipantComponent) value); 3342 } else if (name.equals("study")) { 3343 this.getStudy().add(TypeConvertor.castToReference(value)); 3344 } else if (name.equals("expectedInResearchStudy")) { 3345 this.expectedInResearchStudy = TypeConvertor.castToBoolean(value); // BooleanType 3346 } else if (name.equals("suspectEntity")) { 3347 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); 3348 } else if (name.equals("contributingFactor")) { 3349 this.getContributingFactor().add((AdverseEventContributingFactorComponent) value); 3350 } else if (name.equals("preventiveAction")) { 3351 this.getPreventiveAction().add((AdverseEventPreventiveActionComponent) value); 3352 } else if (name.equals("mitigatingAction")) { 3353 this.getMitigatingAction().add((AdverseEventMitigatingActionComponent) value); 3354 } else if (name.equals("supportingInfo")) { 3355 this.getSupportingInfo().add((AdverseEventSupportingInfoComponent) value); 3356 } else if (name.equals("note")) { 3357 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3358 } else 3359 return super.setProperty(name, value); 3360 return value; 3361 } 3362 3363 @Override 3364 public void removeChild(String name, Base value) throws FHIRException { 3365 if (name.equals("identifier")) { 3366 this.getIdentifier().remove(value); 3367 } else if (name.equals("status")) { 3368 value = new AdverseEventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3369 this.status = (Enumeration) value; // Enumeration<AdverseEventStatus> 3370 } else if (name.equals("actuality")) { 3371 value = new AdverseEventActualityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3372 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 3373 } else if (name.equals("category")) { 3374 this.getCategory().remove(value); 3375 } else if (name.equals("code")) { 3376 this.code = null; 3377 } else if (name.equals("subject")) { 3378 this.subject = null; 3379 } else if (name.equals("encounter")) { 3380 this.encounter = null; 3381 } else if (name.equals("occurrence[x]")) { 3382 this.occurrence = null; 3383 } else if (name.equals("detected")) { 3384 this.detected = null; 3385 } else if (name.equals("recordedDate")) { 3386 this.recordedDate = null; 3387 } else if (name.equals("resultingEffect")) { 3388 this.getResultingEffect().remove(value); 3389 } else if (name.equals("location")) { 3390 this.location = null; 3391 } else if (name.equals("seriousness")) { 3392 this.seriousness = null; 3393 } else if (name.equals("outcome")) { 3394 this.getOutcome().remove(value); 3395 } else if (name.equals("recorder")) { 3396 this.recorder = null; 3397 } else if (name.equals("participant")) { 3398 this.getParticipant().remove((AdverseEventParticipantComponent) value); 3399 } else if (name.equals("study")) { 3400 this.getStudy().remove(value); 3401 } else if (name.equals("expectedInResearchStudy")) { 3402 this.expectedInResearchStudy = null; 3403 } else if (name.equals("suspectEntity")) { 3404 this.getSuspectEntity().remove((AdverseEventSuspectEntityComponent) value); 3405 } else if (name.equals("contributingFactor")) { 3406 this.getContributingFactor().remove((AdverseEventContributingFactorComponent) value); 3407 } else if (name.equals("preventiveAction")) { 3408 this.getPreventiveAction().remove((AdverseEventPreventiveActionComponent) value); 3409 } else if (name.equals("mitigatingAction")) { 3410 this.getMitigatingAction().remove((AdverseEventMitigatingActionComponent) value); 3411 } else if (name.equals("supportingInfo")) { 3412 this.getSupportingInfo().remove((AdverseEventSupportingInfoComponent) value); 3413 } else if (name.equals("note")) { 3414 this.getNote().remove(value); 3415 } else 3416 super.removeChild(name, value); 3417 3418 } 3419 3420 @Override 3421 public Base makeProperty(int hash, String name) throws FHIRException { 3422 switch (hash) { 3423 case -1618432855: return addIdentifier(); 3424 case -892481550: return getStatusElement(); 3425 case 528866400: return getActualityElement(); 3426 case 50511102: return addCategory(); 3427 case 3059181: return getCode(); 3428 case -1867885268: return getSubject(); 3429 case 1524132147: return getEncounter(); 3430 case -2022646513: return getOccurrence(); 3431 case 1687874001: return getOccurrence(); 3432 case 1048254082: return getDetectedElement(); 3433 case -1952893826: return getRecordedDateElement(); 3434 case -2113579882: return addResultingEffect(); 3435 case 1901043637: return getLocation(); 3436 case -1551003909: return getSeriousness(); 3437 case -1106507950: return addOutcome(); 3438 case -799233858: return getRecorder(); 3439 case 767422259: return addParticipant(); 3440 case 109776329: return addStudy(); 3441 case -1071467023: return getExpectedInResearchStudyElement(); 3442 case -1957422662: return addSuspectEntity(); 3443 case -219647527: return addContributingFactor(); 3444 case 2052341334: return addPreventiveAction(); 3445 case 1992862383: return addMitigatingAction(); 3446 case 1922406657: return addSupportingInfo(); 3447 case 3387378: return addNote(); 3448 default: return super.makeProperty(hash, name); 3449 } 3450 3451 } 3452 3453 @Override 3454 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3455 switch (hash) { 3456 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3457 case -892481550: /*status*/ return new String[] {"code"}; 3458 case 528866400: /*actuality*/ return new String[] {"code"}; 3459 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3460 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3461 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3462 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3463 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 3464 case 1048254082: /*detected*/ return new String[] {"dateTime"}; 3465 case -1952893826: /*recordedDate*/ return new String[] {"dateTime"}; 3466 case -2113579882: /*resultingEffect*/ return new String[] {"Reference"}; 3467 case 1901043637: /*location*/ return new String[] {"Reference"}; 3468 case -1551003909: /*seriousness*/ return new String[] {"CodeableConcept"}; 3469 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 3470 case -799233858: /*recorder*/ return new String[] {"Reference"}; 3471 case 767422259: /*participant*/ return new String[] {}; 3472 case 109776329: /*study*/ return new String[] {"Reference"}; 3473 case -1071467023: /*expectedInResearchStudy*/ return new String[] {"boolean"}; 3474 case -1957422662: /*suspectEntity*/ return new String[] {}; 3475 case -219647527: /*contributingFactor*/ return new String[] {}; 3476 case 2052341334: /*preventiveAction*/ return new String[] {}; 3477 case 1992862383: /*mitigatingAction*/ return new String[] {}; 3478 case 1922406657: /*supportingInfo*/ return new String[] {}; 3479 case 3387378: /*note*/ return new String[] {"Annotation"}; 3480 default: return super.getTypesForProperty(hash, name); 3481 } 3482 3483 } 3484 3485 @Override 3486 public Base addChild(String name) throws FHIRException { 3487 if (name.equals("identifier")) { 3488 return addIdentifier(); 3489 } 3490 else if (name.equals("status")) { 3491 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.status"); 3492 } 3493 else if (name.equals("actuality")) { 3494 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.actuality"); 3495 } 3496 else if (name.equals("category")) { 3497 return addCategory(); 3498 } 3499 else if (name.equals("code")) { 3500 this.code = new CodeableConcept(); 3501 return this.code; 3502 } 3503 else if (name.equals("subject")) { 3504 this.subject = new Reference(); 3505 return this.subject; 3506 } 3507 else if (name.equals("encounter")) { 3508 this.encounter = new Reference(); 3509 return this.encounter; 3510 } 3511 else if (name.equals("occurrenceDateTime")) { 3512 this.occurrence = new DateTimeType(); 3513 return this.occurrence; 3514 } 3515 else if (name.equals("occurrencePeriod")) { 3516 this.occurrence = new Period(); 3517 return this.occurrence; 3518 } 3519 else if (name.equals("occurrenceTiming")) { 3520 this.occurrence = new Timing(); 3521 return this.occurrence; 3522 } 3523 else if (name.equals("detected")) { 3524 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.detected"); 3525 } 3526 else if (name.equals("recordedDate")) { 3527 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.recordedDate"); 3528 } 3529 else if (name.equals("resultingEffect")) { 3530 return addResultingEffect(); 3531 } 3532 else if (name.equals("location")) { 3533 this.location = new Reference(); 3534 return this.location; 3535 } 3536 else if (name.equals("seriousness")) { 3537 this.seriousness = new CodeableConcept(); 3538 return this.seriousness; 3539 } 3540 else if (name.equals("outcome")) { 3541 return addOutcome(); 3542 } 3543 else if (name.equals("recorder")) { 3544 this.recorder = new Reference(); 3545 return this.recorder; 3546 } 3547 else if (name.equals("participant")) { 3548 return addParticipant(); 3549 } 3550 else if (name.equals("study")) { 3551 return addStudy(); 3552 } 3553 else if (name.equals("expectedInResearchStudy")) { 3554 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.expectedInResearchStudy"); 3555 } 3556 else if (name.equals("suspectEntity")) { 3557 return addSuspectEntity(); 3558 } 3559 else if (name.equals("contributingFactor")) { 3560 return addContributingFactor(); 3561 } 3562 else if (name.equals("preventiveAction")) { 3563 return addPreventiveAction(); 3564 } 3565 else if (name.equals("mitigatingAction")) { 3566 return addMitigatingAction(); 3567 } 3568 else if (name.equals("supportingInfo")) { 3569 return addSupportingInfo(); 3570 } 3571 else if (name.equals("note")) { 3572 return addNote(); 3573 } 3574 else 3575 return super.addChild(name); 3576 } 3577 3578 public String fhirType() { 3579 return "AdverseEvent"; 3580 3581 } 3582 3583 public AdverseEvent copy() { 3584 AdverseEvent dst = new AdverseEvent(); 3585 copyValues(dst); 3586 return dst; 3587 } 3588 3589 public void copyValues(AdverseEvent dst) { 3590 super.copyValues(dst); 3591 if (identifier != null) { 3592 dst.identifier = new ArrayList<Identifier>(); 3593 for (Identifier i : identifier) 3594 dst.identifier.add(i.copy()); 3595 }; 3596 dst.status = status == null ? null : status.copy(); 3597 dst.actuality = actuality == null ? null : actuality.copy(); 3598 if (category != null) { 3599 dst.category = new ArrayList<CodeableConcept>(); 3600 for (CodeableConcept i : category) 3601 dst.category.add(i.copy()); 3602 }; 3603 dst.code = code == null ? null : code.copy(); 3604 dst.subject = subject == null ? null : subject.copy(); 3605 dst.encounter = encounter == null ? null : encounter.copy(); 3606 dst.occurrence = occurrence == null ? null : occurrence.copy(); 3607 dst.detected = detected == null ? null : detected.copy(); 3608 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 3609 if (resultingEffect != null) { 3610 dst.resultingEffect = new ArrayList<Reference>(); 3611 for (Reference i : resultingEffect) 3612 dst.resultingEffect.add(i.copy()); 3613 }; 3614 dst.location = location == null ? null : location.copy(); 3615 dst.seriousness = seriousness == null ? null : seriousness.copy(); 3616 if (outcome != null) { 3617 dst.outcome = new ArrayList<CodeableConcept>(); 3618 for (CodeableConcept i : outcome) 3619 dst.outcome.add(i.copy()); 3620 }; 3621 dst.recorder = recorder == null ? null : recorder.copy(); 3622 if (participant != null) { 3623 dst.participant = new ArrayList<AdverseEventParticipantComponent>(); 3624 for (AdverseEventParticipantComponent i : participant) 3625 dst.participant.add(i.copy()); 3626 }; 3627 if (study != null) { 3628 dst.study = new ArrayList<Reference>(); 3629 for (Reference i : study) 3630 dst.study.add(i.copy()); 3631 }; 3632 dst.expectedInResearchStudy = expectedInResearchStudy == null ? null : expectedInResearchStudy.copy(); 3633 if (suspectEntity != null) { 3634 dst.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 3635 for (AdverseEventSuspectEntityComponent i : suspectEntity) 3636 dst.suspectEntity.add(i.copy()); 3637 }; 3638 if (contributingFactor != null) { 3639 dst.contributingFactor = new ArrayList<AdverseEventContributingFactorComponent>(); 3640 for (AdverseEventContributingFactorComponent i : contributingFactor) 3641 dst.contributingFactor.add(i.copy()); 3642 }; 3643 if (preventiveAction != null) { 3644 dst.preventiveAction = new ArrayList<AdverseEventPreventiveActionComponent>(); 3645 for (AdverseEventPreventiveActionComponent i : preventiveAction) 3646 dst.preventiveAction.add(i.copy()); 3647 }; 3648 if (mitigatingAction != null) { 3649 dst.mitigatingAction = new ArrayList<AdverseEventMitigatingActionComponent>(); 3650 for (AdverseEventMitigatingActionComponent i : mitigatingAction) 3651 dst.mitigatingAction.add(i.copy()); 3652 }; 3653 if (supportingInfo != null) { 3654 dst.supportingInfo = new ArrayList<AdverseEventSupportingInfoComponent>(); 3655 for (AdverseEventSupportingInfoComponent i : supportingInfo) 3656 dst.supportingInfo.add(i.copy()); 3657 }; 3658 if (note != null) { 3659 dst.note = new ArrayList<Annotation>(); 3660 for (Annotation i : note) 3661 dst.note.add(i.copy()); 3662 }; 3663 } 3664 3665 protected AdverseEvent typedCopy() { 3666 return copy(); 3667 } 3668 3669 @Override 3670 public boolean equalsDeep(Base other_) { 3671 if (!super.equalsDeep(other_)) 3672 return false; 3673 if (!(other_ instanceof AdverseEvent)) 3674 return false; 3675 AdverseEvent o = (AdverseEvent) other_; 3676 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(actuality, o.actuality, true) 3677 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 3678 && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) && compareDeep(detected, o.detected, true) 3679 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(resultingEffect, o.resultingEffect, true) 3680 && compareDeep(location, o.location, true) && compareDeep(seriousness, o.seriousness, true) && compareDeep(outcome, o.outcome, true) 3681 && compareDeep(recorder, o.recorder, true) && compareDeep(participant, o.participant, true) && compareDeep(study, o.study, true) 3682 && compareDeep(expectedInResearchStudy, o.expectedInResearchStudy, true) && compareDeep(suspectEntity, o.suspectEntity, true) 3683 && compareDeep(contributingFactor, o.contributingFactor, true) && compareDeep(preventiveAction, o.preventiveAction, true) 3684 && compareDeep(mitigatingAction, o.mitigatingAction, true) && compareDeep(supportingInfo, o.supportingInfo, true) 3685 && compareDeep(note, o.note, true); 3686 } 3687 3688 @Override 3689 public boolean equalsShallow(Base other_) { 3690 if (!super.equalsShallow(other_)) 3691 return false; 3692 if (!(other_ instanceof AdverseEvent)) 3693 return false; 3694 AdverseEvent o = (AdverseEvent) other_; 3695 return compareValues(status, o.status, true) && compareValues(actuality, o.actuality, true) && compareValues(detected, o.detected, true) 3696 && compareValues(recordedDate, o.recordedDate, true) && compareValues(expectedInResearchStudy, o.expectedInResearchStudy, true) 3697 ; 3698 } 3699 3700 public boolean isEmpty() { 3701 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, actuality 3702 , category, code, subject, encounter, occurrence, detected, recordedDate, resultingEffect 3703 , location, seriousness, outcome, recorder, participant, study, expectedInResearchStudy 3704 , suspectEntity, contributingFactor, preventiveAction, mitigatingAction, supportingInfo 3705 , note); 3706 } 3707 3708 @Override 3709 public ResourceType getResourceType() { 3710 return ResourceType.AdverseEvent; 3711 } 3712 3713 /** 3714 * Search parameter: <b>actuality</b> 3715 * <p> 3716 * Description: <b>actual | potential</b><br> 3717 * Type: <b>token</b><br> 3718 * Path: <b>AdverseEvent.actuality</b><br> 3719 * </p> 3720 */ 3721 @SearchParamDefinition(name="actuality", path="AdverseEvent.actuality", description="actual | potential", type="token" ) 3722 public static final String SP_ACTUALITY = "actuality"; 3723 /** 3724 * <b>Fluent Client</b> search parameter constant for <b>actuality</b> 3725 * <p> 3726 * Description: <b>actual | potential</b><br> 3727 * Type: <b>token</b><br> 3728 * Path: <b>AdverseEvent.actuality</b><br> 3729 * </p> 3730 */ 3731 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTUALITY); 3732 3733 /** 3734 * Search parameter: <b>category</b> 3735 * <p> 3736 * Description: <b>wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site</b><br> 3737 * Type: <b>token</b><br> 3738 * Path: <b>AdverseEvent.category</b><br> 3739 * </p> 3740 */ 3741 @SearchParamDefinition(name="category", path="AdverseEvent.category", description="wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site", type="token" ) 3742 public static final String SP_CATEGORY = "category"; 3743 /** 3744 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3745 * <p> 3746 * Description: <b>wrong-patient | procedure-mishap | medication-mishap | device | unsafe-physical-environment | hospital-aquired-infection | wrong-body-site</b><br> 3747 * Type: <b>token</b><br> 3748 * Path: <b>AdverseEvent.category</b><br> 3749 * </p> 3750 */ 3751 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3752 3753 /** 3754 * Search parameter: <b>location</b> 3755 * <p> 3756 * Description: <b>Location where adverse event occurred</b><br> 3757 * Type: <b>reference</b><br> 3758 * Path: <b>AdverseEvent.location</b><br> 3759 * </p> 3760 */ 3761 @SearchParamDefinition(name="location", path="AdverseEvent.location", description="Location where adverse event occurred", type="reference", target={Location.class } ) 3762 public static final String SP_LOCATION = "location"; 3763 /** 3764 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3765 * <p> 3766 * Description: <b>Location where adverse event occurred</b><br> 3767 * Type: <b>reference</b><br> 3768 * Path: <b>AdverseEvent.location</b><br> 3769 * </p> 3770 */ 3771 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 3772 3773/** 3774 * Constant for fluent queries to be used to add include statements. Specifies 3775 * the path value of "<b>AdverseEvent:location</b>". 3776 */ 3777 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("AdverseEvent:location").toLocked(); 3778 3779 /** 3780 * Search parameter: <b>recorder</b> 3781 * <p> 3782 * Description: <b>Who recorded the adverse event</b><br> 3783 * Type: <b>reference</b><br> 3784 * Path: <b>AdverseEvent.recorder</b><br> 3785 * </p> 3786 */ 3787 @SearchParamDefinition(name="recorder", path="AdverseEvent.recorder", description="Who recorded the adverse event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, ResearchSubject.class } ) 3788 public static final String SP_RECORDER = "recorder"; 3789 /** 3790 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 3791 * <p> 3792 * Description: <b>Who recorded the adverse event</b><br> 3793 * Type: <b>reference</b><br> 3794 * Path: <b>AdverseEvent.recorder</b><br> 3795 * </p> 3796 */ 3797 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECORDER); 3798 3799/** 3800 * Constant for fluent queries to be used to add include statements. Specifies 3801 * the path value of "<b>AdverseEvent:recorder</b>". 3802 */ 3803 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include("AdverseEvent:recorder").toLocked(); 3804 3805 /** 3806 * Search parameter: <b>resultingeffect</b> 3807 * <p> 3808 * Description: <b>Effect on the subject due to this event</b><br> 3809 * Type: <b>reference</b><br> 3810 * Path: <b>AdverseEvent.resultingEffect</b><br> 3811 * </p> 3812 */ 3813 @SearchParamDefinition(name="resultingeffect", path="AdverseEvent.resultingEffect", description="Effect on the subject due to this event", type="reference", target={Condition.class, Observation.class } ) 3814 public static final String SP_RESULTINGEFFECT = "resultingeffect"; 3815 /** 3816 * <b>Fluent Client</b> search parameter constant for <b>resultingeffect</b> 3817 * <p> 3818 * Description: <b>Effect on the subject due to this event</b><br> 3819 * Type: <b>reference</b><br> 3820 * Path: <b>AdverseEvent.resultingEffect</b><br> 3821 * </p> 3822 */ 3823 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULTINGEFFECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESULTINGEFFECT); 3824 3825/** 3826 * Constant for fluent queries to be used to add include statements. Specifies 3827 * the path value of "<b>AdverseEvent:resultingeffect</b>". 3828 */ 3829 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULTINGEFFECT = new ca.uhn.fhir.model.api.Include("AdverseEvent:resultingeffect").toLocked(); 3830 3831 /** 3832 * Search parameter: <b>seriousness</b> 3833 * <p> 3834 * Description: <b>Seriousness or gravity of the event</b><br> 3835 * Type: <b>token</b><br> 3836 * Path: <b>AdverseEvent.seriousness</b><br> 3837 * </p> 3838 */ 3839 @SearchParamDefinition(name="seriousness", path="AdverseEvent.seriousness", description="Seriousness or gravity of the event", type="token" ) 3840 public static final String SP_SERIOUSNESS = "seriousness"; 3841 /** 3842 * <b>Fluent Client</b> search parameter constant for <b>seriousness</b> 3843 * <p> 3844 * Description: <b>Seriousness or gravity of the event</b><br> 3845 * Type: <b>token</b><br> 3846 * Path: <b>AdverseEvent.seriousness</b><br> 3847 * </p> 3848 */ 3849 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIOUSNESS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIOUSNESS); 3850 3851 /** 3852 * Search parameter: <b>status</b> 3853 * <p> 3854 * Description: <b>in-progress | completed | entered-in-error | unknown</b><br> 3855 * Type: <b>token</b><br> 3856 * Path: <b>AdverseEvent.status</b><br> 3857 * </p> 3858 */ 3859 @SearchParamDefinition(name="status", path="AdverseEvent.status", description="in-progress | completed | entered-in-error | unknown", type="token" ) 3860 public static final String SP_STATUS = "status"; 3861 /** 3862 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3863 * <p> 3864 * Description: <b>in-progress | completed | entered-in-error | unknown</b><br> 3865 * Type: <b>token</b><br> 3866 * Path: <b>AdverseEvent.status</b><br> 3867 * </p> 3868 */ 3869 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3870 3871 /** 3872 * Search parameter: <b>study</b> 3873 * <p> 3874 * Description: <b>Research study that the subject is enrolled in</b><br> 3875 * Type: <b>reference</b><br> 3876 * Path: <b>AdverseEvent.study</b><br> 3877 * </p> 3878 */ 3879 @SearchParamDefinition(name="study", path="AdverseEvent.study", description="Research study that the subject is enrolled in", type="reference", target={ResearchStudy.class } ) 3880 public static final String SP_STUDY = "study"; 3881 /** 3882 * <b>Fluent Client</b> search parameter constant for <b>study</b> 3883 * <p> 3884 * Description: <b>Research study that the subject is enrolled in</b><br> 3885 * Type: <b>reference</b><br> 3886 * Path: <b>AdverseEvent.study</b><br> 3887 * </p> 3888 */ 3889 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_STUDY); 3890 3891/** 3892 * Constant for fluent queries to be used to add include statements. Specifies 3893 * the path value of "<b>AdverseEvent:study</b>". 3894 */ 3895 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include("AdverseEvent:study").toLocked(); 3896 3897 /** 3898 * Search parameter: <b>subject</b> 3899 * <p> 3900 * Description: <b>Subject impacted by event</b><br> 3901 * Type: <b>reference</b><br> 3902 * Path: <b>AdverseEvent.subject</b><br> 3903 * </p> 3904 */ 3905 @SearchParamDefinition(name="subject", path="AdverseEvent.subject", description="Subject impacted by event", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class, Practitioner.class, RelatedPerson.class, ResearchSubject.class } ) 3906 public static final String SP_SUBJECT = "subject"; 3907 /** 3908 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3909 * <p> 3910 * Description: <b>Subject impacted by event</b><br> 3911 * Type: <b>reference</b><br> 3912 * Path: <b>AdverseEvent.subject</b><br> 3913 * </p> 3914 */ 3915 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3916 3917/** 3918 * Constant for fluent queries to be used to add include statements. Specifies 3919 * the path value of "<b>AdverseEvent:subject</b>". 3920 */ 3921 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("AdverseEvent:subject").toLocked(); 3922 3923 /** 3924 * Search parameter: <b>substance</b> 3925 * <p> 3926 * Description: <b>Refers to the specific entity that caused the adverse event</b><br> 3927 * Type: <b>reference</b><br> 3928 * Path: <b>(AdverseEvent.suspectEntity.instance as Reference)</b><br> 3929 * </p> 3930 */ 3931 @SearchParamDefinition(name="substance", path="(AdverseEvent.suspectEntity.instance as Reference)", description="Refers to the specific entity that caused the adverse event", type="reference", target={BiologicallyDerivedProduct.class, Device.class, Immunization.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, Procedure.class, ResearchStudy.class, Substance.class } ) 3932 public static final String SP_SUBSTANCE = "substance"; 3933 /** 3934 * <b>Fluent Client</b> search parameter constant for <b>substance</b> 3935 * <p> 3936 * Description: <b>Refers to the specific entity that caused the adverse event</b><br> 3937 * Type: <b>reference</b><br> 3938 * Path: <b>(AdverseEvent.suspectEntity.instance as Reference)</b><br> 3939 * </p> 3940 */ 3941 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE); 3942 3943/** 3944 * Constant for fluent queries to be used to add include statements. Specifies 3945 * the path value of "<b>AdverseEvent:substance</b>". 3946 */ 3947 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE = new ca.uhn.fhir.model.api.Include("AdverseEvent:substance").toLocked(); 3948 3949 /** 3950 * Search parameter: <b>code</b> 3951 * <p> 3952 * Description: <b>Multiple Resources: 3953 3954* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3955* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3956* [AuditEvent](auditevent.html): More specific code for the event 3957* [Basic](basic.html): Kind of Resource 3958* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3959* [Condition](condition.html): Code for the condition 3960* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3961* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3962* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3963* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3964* [ImagingSelection](imagingselection.html): The imaging selection status 3965* [List](list.html): What the purpose of this list is 3966* [Medication](medication.html): Returns medications for a specific code 3967* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3968* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3969* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3970* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3971* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3972* [Observation](observation.html): The code of the observation type 3973* [Procedure](procedure.html): A code to identify a procedure 3974* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3975* [Task](task.html): Search by task code 3976</b><br> 3977 * Type: <b>token</b><br> 3978 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3979 * </p> 3980 */ 3981 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3982 public static final String SP_CODE = "code"; 3983 /** 3984 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3985 * <p> 3986 * Description: <b>Multiple Resources: 3987 3988* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3989* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3990* [AuditEvent](auditevent.html): More specific code for the event 3991* [Basic](basic.html): Kind of Resource 3992* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3993* [Condition](condition.html): Code for the condition 3994* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3995* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3996* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3997* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3998* [ImagingSelection](imagingselection.html): The imaging selection status 3999* [List](list.html): What the purpose of this list is 4000* [Medication](medication.html): Returns medications for a specific code 4001* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 4002* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 4003* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 4004* [MedicationStatement](medicationstatement.html): Return statements of this medication code 4005* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 4006* [Observation](observation.html): The code of the observation type 4007* [Procedure](procedure.html): A code to identify a procedure 4008* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4009* [Task](task.html): Search by task code 4010</b><br> 4011 * Type: <b>token</b><br> 4012 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4013 * </p> 4014 */ 4015 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4016 4017 /** 4018 * Search parameter: <b>date</b> 4019 * <p> 4020 * Description: <b>Multiple Resources: 4021 4022* [AdverseEvent](adverseevent.html): When the event occurred 4023* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4024* [Appointment](appointment.html): Appointment date/time. 4025* [AuditEvent](auditevent.html): Time when the event was recorded 4026* [CarePlan](careplan.html): Time period plan covers 4027* [CareTeam](careteam.html): A date within the coverage time period. 4028* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4029* [Composition](composition.html): Composition editing time 4030* [Consent](consent.html): When consent was agreed to 4031* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4032* [DocumentReference](documentreference.html): When this document reference was created 4033* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4034* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4035* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4036* [Flag](flag.html): Time period when flag is active 4037* [Immunization](immunization.html): Vaccination (non)-Administration Date 4038* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4039* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4040* [Invoice](invoice.html): Invoice date / posting date 4041* [List](list.html): When the list was prepared 4042* [MeasureReport](measurereport.html): The date of the measure report 4043* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4044* [Observation](observation.html): Clinically relevant time/time-period for observation 4045* [Procedure](procedure.html): When the procedure occurred or is occurring 4046* [ResearchSubject](researchsubject.html): Start and end of participation 4047* [RiskAssessment](riskassessment.html): When was assessment made? 4048* [SupplyRequest](supplyrequest.html): When the request was made 4049</b><br> 4050 * Type: <b>date</b><br> 4051 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4052 * </p> 4053 */ 4054 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4055 public static final String SP_DATE = "date"; 4056 /** 4057 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4058 * <p> 4059 * Description: <b>Multiple Resources: 4060 4061* [AdverseEvent](adverseevent.html): When the event occurred 4062* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4063* [Appointment](appointment.html): Appointment date/time. 4064* [AuditEvent](auditevent.html): Time when the event was recorded 4065* [CarePlan](careplan.html): Time period plan covers 4066* [CareTeam](careteam.html): A date within the coverage time period. 4067* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4068* [Composition](composition.html): Composition editing time 4069* [Consent](consent.html): When consent was agreed to 4070* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4071* [DocumentReference](documentreference.html): When this document reference was created 4072* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4073* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4074* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4075* [Flag](flag.html): Time period when flag is active 4076* [Immunization](immunization.html): Vaccination (non)-Administration Date 4077* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4078* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4079* [Invoice](invoice.html): Invoice date / posting date 4080* [List](list.html): When the list was prepared 4081* [MeasureReport](measurereport.html): The date of the measure report 4082* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4083* [Observation](observation.html): Clinically relevant time/time-period for observation 4084* [Procedure](procedure.html): When the procedure occurred or is occurring 4085* [ResearchSubject](researchsubject.html): Start and end of participation 4086* [RiskAssessment](riskassessment.html): When was assessment made? 4087* [SupplyRequest](supplyrequest.html): When the request was made 4088</b><br> 4089 * Type: <b>date</b><br> 4090 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4091 * </p> 4092 */ 4093 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4094 4095 /** 4096 * Search parameter: <b>identifier</b> 4097 * <p> 4098 * Description: <b>Multiple Resources: 4099 4100* [Account](account.html): Account number 4101* [AdverseEvent](adverseevent.html): Business identifier for the event 4102* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4103* [Appointment](appointment.html): An Identifier of the Appointment 4104* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4105* [Basic](basic.html): Business identifier 4106* [BodyStructure](bodystructure.html): Bodystructure identifier 4107* [CarePlan](careplan.html): External Ids for this plan 4108* [CareTeam](careteam.html): External Ids for this team 4109* [ChargeItem](chargeitem.html): Business Identifier for item 4110* [Claim](claim.html): The primary identifier of the financial resource 4111* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4112* [ClinicalImpression](clinicalimpression.html): Business identifier 4113* [Communication](communication.html): Unique identifier 4114* [CommunicationRequest](communicationrequest.html): Unique identifier 4115* [Composition](composition.html): Version-independent identifier for the Composition 4116* [Condition](condition.html): A unique identifier of the condition record 4117* [Consent](consent.html): Identifier for this record (external references) 4118* [Contract](contract.html): The identity of the contract 4119* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4120* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4121* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4122* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4123* [DeviceRequest](devicerequest.html): Business identifier for request/order 4124* [DeviceUsage](deviceusage.html): Search by identifier 4125* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4126* [DocumentReference](documentreference.html): Identifier of the attachment binary 4127* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4128* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4129* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4130* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4131* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4132* [Flag](flag.html): Business identifier 4133* [Goal](goal.html): External Ids for this goal 4134* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4135* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4136* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4137* [Immunization](immunization.html): Business identifier 4138* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4139* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4140* [Invoice](invoice.html): Business Identifier for item 4141* [List](list.html): Business identifier 4142* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4143* [Medication](medication.html): Returns medications with this external identifier 4144* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4145* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4146* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4147* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4148* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4149* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4150* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4151* [Observation](observation.html): The unique id for a particular observation 4152* [Person](person.html): A person Identifier 4153* [Procedure](procedure.html): A unique identifier for a procedure 4154* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4155* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4156* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4157* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4158* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4159* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4160* [Specimen](specimen.html): The unique identifier associated with the specimen 4161* [SupplyDelivery](supplydelivery.html): External identifier 4162* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4163* [Task](task.html): Search for a task instance by its business identifier 4164* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4165</b><br> 4166 * Type: <b>token</b><br> 4167 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4168 * </p> 4169 */ 4170 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4171 public static final String SP_IDENTIFIER = "identifier"; 4172 /** 4173 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4174 * <p> 4175 * Description: <b>Multiple Resources: 4176 4177* [Account](account.html): Account number 4178* [AdverseEvent](adverseevent.html): Business identifier for the event 4179* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4180* [Appointment](appointment.html): An Identifier of the Appointment 4181* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4182* [Basic](basic.html): Business identifier 4183* [BodyStructure](bodystructure.html): Bodystructure identifier 4184* [CarePlan](careplan.html): External Ids for this plan 4185* [CareTeam](careteam.html): External Ids for this team 4186* [ChargeItem](chargeitem.html): Business Identifier for item 4187* [Claim](claim.html): The primary identifier of the financial resource 4188* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4189* [ClinicalImpression](clinicalimpression.html): Business identifier 4190* [Communication](communication.html): Unique identifier 4191* [CommunicationRequest](communicationrequest.html): Unique identifier 4192* [Composition](composition.html): Version-independent identifier for the Composition 4193* [Condition](condition.html): A unique identifier of the condition record 4194* [Consent](consent.html): Identifier for this record (external references) 4195* [Contract](contract.html): The identity of the contract 4196* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4197* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4198* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4199* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4200* [DeviceRequest](devicerequest.html): Business identifier for request/order 4201* [DeviceUsage](deviceusage.html): Search by identifier 4202* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4203* [DocumentReference](documentreference.html): Identifier of the attachment binary 4204* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4205* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4206* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4207* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4208* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4209* [Flag](flag.html): Business identifier 4210* [Goal](goal.html): External Ids for this goal 4211* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4212* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4213* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4214* [Immunization](immunization.html): Business identifier 4215* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4216* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4217* [Invoice](invoice.html): Business Identifier for item 4218* [List](list.html): Business identifier 4219* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4220* [Medication](medication.html): Returns medications with this external identifier 4221* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4222* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4223* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4224* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4225* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4226* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4227* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4228* [Observation](observation.html): The unique id for a particular observation 4229* [Person](person.html): A person Identifier 4230* [Procedure](procedure.html): A unique identifier for a procedure 4231* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4232* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4233* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4234* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4235* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4236* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4237* [Specimen](specimen.html): The unique identifier associated with the specimen 4238* [SupplyDelivery](supplydelivery.html): External identifier 4239* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4240* [Task](task.html): Search for a task instance by its business identifier 4241* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4242</b><br> 4243 * Type: <b>token</b><br> 4244 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4245 * </p> 4246 */ 4247 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4248 4249 /** 4250 * Search parameter: <b>patient</b> 4251 * <p> 4252 * Description: <b>Multiple Resources: 4253 4254* [Account](account.html): The entity that caused the expenses 4255* [AdverseEvent](adverseevent.html): Subject impacted by event 4256* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4257* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4258* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4259* [AuditEvent](auditevent.html): Where the activity involved patient data 4260* [Basic](basic.html): Identifies the focus of this resource 4261* [BodyStructure](bodystructure.html): Who this is about 4262* [CarePlan](careplan.html): Who the care plan is for 4263* [CareTeam](careteam.html): Who care team is for 4264* [ChargeItem](chargeitem.html): Individual service was done for/to 4265* [Claim](claim.html): Patient receiving the products or services 4266* [ClaimResponse](claimresponse.html): The subject of care 4267* [ClinicalImpression](clinicalimpression.html): Patient assessed 4268* [Communication](communication.html): Focus of message 4269* [CommunicationRequest](communicationrequest.html): Focus of message 4270* [Composition](composition.html): Who and/or what the composition is about 4271* [Condition](condition.html): Who has the condition? 4272* [Consent](consent.html): Who the consent applies to 4273* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4274* [Coverage](coverage.html): Retrieve coverages for a patient 4275* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4276* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4277* [DetectedIssue](detectedissue.html): Associated patient 4278* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4279* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4280* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4281* [DocumentReference](documentreference.html): Who/what is the subject of the document 4282* [Encounter](encounter.html): The patient present at the encounter 4283* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4284* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4285* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4286* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4287* [Flag](flag.html): The identity of a subject to list flags for 4288* [Goal](goal.html): Who this goal is intended for 4289* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4290* [ImagingSelection](imagingselection.html): Who the study is about 4291* [ImagingStudy](imagingstudy.html): Who the study is about 4292* [Immunization](immunization.html): The patient for the vaccination record 4293* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4294* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4295* [Invoice](invoice.html): Recipient(s) of goods and services 4296* [List](list.html): If all resources have the same subject 4297* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4298* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4299* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4300* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4301* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4302* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4303* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4304* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4305* [Observation](observation.html): The subject that the observation is about (if patient) 4306* [Person](person.html): The Person links to this Patient 4307* [Procedure](procedure.html): Search by subject - a patient 4308* [Provenance](provenance.html): Where the activity involved patient data 4309* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4310* [RelatedPerson](relatedperson.html): The patient this related person is related to 4311* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4312* [ResearchSubject](researchsubject.html): Who or what is part of study 4313* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4314* [ServiceRequest](servicerequest.html): Search by subject - a patient 4315* [Specimen](specimen.html): The patient the specimen comes from 4316* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4317* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4318* [Task](task.html): Search by patient 4319* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4320</b><br> 4321 * Type: <b>reference</b><br> 4322 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4323 * </p> 4324 */ 4325 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4326 public static final String SP_PATIENT = "patient"; 4327 /** 4328 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4329 * <p> 4330 * Description: <b>Multiple Resources: 4331 4332* [Account](account.html): The entity that caused the expenses 4333* [AdverseEvent](adverseevent.html): Subject impacted by event 4334* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4335* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4336* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4337* [AuditEvent](auditevent.html): Where the activity involved patient data 4338* [Basic](basic.html): Identifies the focus of this resource 4339* [BodyStructure](bodystructure.html): Who this is about 4340* [CarePlan](careplan.html): Who the care plan is for 4341* [CareTeam](careteam.html): Who care team is for 4342* [ChargeItem](chargeitem.html): Individual service was done for/to 4343* [Claim](claim.html): Patient receiving the products or services 4344* [ClaimResponse](claimresponse.html): The subject of care 4345* [ClinicalImpression](clinicalimpression.html): Patient assessed 4346* [Communication](communication.html): Focus of message 4347* [CommunicationRequest](communicationrequest.html): Focus of message 4348* [Composition](composition.html): Who and/or what the composition is about 4349* [Condition](condition.html): Who has the condition? 4350* [Consent](consent.html): Who the consent applies to 4351* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4352* [Coverage](coverage.html): Retrieve coverages for a patient 4353* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4354* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4355* [DetectedIssue](detectedissue.html): Associated patient 4356* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4357* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4358* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4359* [DocumentReference](documentreference.html): Who/what is the subject of the document 4360* [Encounter](encounter.html): The patient present at the encounter 4361* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4362* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4363* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4364* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4365* [Flag](flag.html): The identity of a subject to list flags for 4366* [Goal](goal.html): Who this goal is intended for 4367* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4368* [ImagingSelection](imagingselection.html): Who the study is about 4369* [ImagingStudy](imagingstudy.html): Who the study is about 4370* [Immunization](immunization.html): The patient for the vaccination record 4371* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4372* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4373* [Invoice](invoice.html): Recipient(s) of goods and services 4374* [List](list.html): If all resources have the same subject 4375* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4376* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4377* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4378* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4379* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4380* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4381* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4382* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4383* [Observation](observation.html): The subject that the observation is about (if patient) 4384* [Person](person.html): The Person links to this Patient 4385* [Procedure](procedure.html): Search by subject - a patient 4386* [Provenance](provenance.html): Where the activity involved patient data 4387* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4388* [RelatedPerson](relatedperson.html): The patient this related person is related to 4389* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4390* [ResearchSubject](researchsubject.html): Who or what is part of study 4391* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4392* [ServiceRequest](servicerequest.html): Search by subject - a patient 4393* [Specimen](specimen.html): The patient the specimen comes from 4394* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4395* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4396* [Task](task.html): Search by patient 4397* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4398</b><br> 4399 * Type: <b>reference</b><br> 4400 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4401 * </p> 4402 */ 4403 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4404 4405/** 4406 * Constant for fluent queries to be used to add include statements. Specifies 4407 * the path value of "<b>AdverseEvent:patient</b>". 4408 */ 4409 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AdverseEvent:patient").toLocked(); 4410 4411 4412} 4413