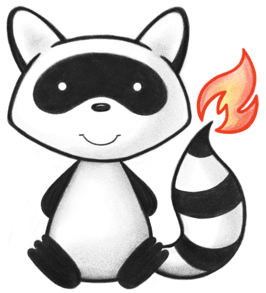
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 052 */ 053@ResourceDef(name="AllergyIntolerance", profile="http://hl7.org/fhir/StructureDefinition/AllergyIntolerance") 054public class AllergyIntolerance extends DomainResource { 055 056 public enum AllergyIntoleranceCategory { 057 /** 058 * Any substance consumed to provide nutritional support for the body. 059 */ 060 FOOD, 061 /** 062 * Substances administered to achieve a physiological effect. 063 */ 064 MEDICATION, 065 /** 066 * Any substances that are encountered in the environment, including any substance not already classified as food, medication, or biologic. 067 */ 068 ENVIRONMENT, 069 /** 070 * A preparation that is synthesized from living organisms or their products, especially a human or animal protein, such as a hormone or antitoxin, that is used as a diagnostic, preventive, or therapeutic agent. Examples of biologic medications include: vaccines; allergenic extracts, which are used for both diagnosis and treatment (for example, allergy shots); gene therapies; cellular therapies. There are other biologic products, such as tissues, which are not typically associated with allergies. 071 */ 072 BIOLOGIC, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static AllergyIntoleranceCategory fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("food".equals(codeString)) 081 return FOOD; 082 if ("medication".equals(codeString)) 083 return MEDICATION; 084 if ("environment".equals(codeString)) 085 return ENVIRONMENT; 086 if ("biologic".equals(codeString)) 087 return BIOLOGIC; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown AllergyIntoleranceCategory code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case FOOD: return "food"; 096 case MEDICATION: return "medication"; 097 case ENVIRONMENT: return "environment"; 098 case BIOLOGIC: return "biologic"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case FOOD: return "http://hl7.org/fhir/allergy-intolerance-category"; 106 case MEDICATION: return "http://hl7.org/fhir/allergy-intolerance-category"; 107 case ENVIRONMENT: return "http://hl7.org/fhir/allergy-intolerance-category"; 108 case BIOLOGIC: return "http://hl7.org/fhir/allergy-intolerance-category"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case FOOD: return "Any substance consumed to provide nutritional support for the body."; 116 case MEDICATION: return "Substances administered to achieve a physiological effect."; 117 case ENVIRONMENT: return "Any substances that are encountered in the environment, including any substance not already classified as food, medication, or biologic."; 118 case BIOLOGIC: return "A preparation that is synthesized from living organisms or their products, especially a human or animal protein, such as a hormone or antitoxin, that is used as a diagnostic, preventive, or therapeutic agent. Examples of biologic medications include: vaccines; allergenic extracts, which are used for both diagnosis and treatment (for example, allergy shots); gene therapies; cellular therapies. There are other biologic products, such as tissues, which are not typically associated with allergies."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case FOOD: return "Food"; 126 case MEDICATION: return "Medication"; 127 case ENVIRONMENT: return "Environment"; 128 case BIOLOGIC: return "Biologic"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class AllergyIntoleranceCategoryEnumFactory implements EnumFactory<AllergyIntoleranceCategory> { 136 public AllergyIntoleranceCategory fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("food".equals(codeString)) 141 return AllergyIntoleranceCategory.FOOD; 142 if ("medication".equals(codeString)) 143 return AllergyIntoleranceCategory.MEDICATION; 144 if ("environment".equals(codeString)) 145 return AllergyIntoleranceCategory.ENVIRONMENT; 146 if ("biologic".equals(codeString)) 147 return AllergyIntoleranceCategory.BIOLOGIC; 148 throw new IllegalArgumentException("Unknown AllergyIntoleranceCategory code '"+codeString+"'"); 149 } 150 public Enumeration<AllergyIntoleranceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.NULL, code); 158 if ("food".equals(codeString)) 159 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.FOOD, code); 160 if ("medication".equals(codeString)) 161 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.MEDICATION, code); 162 if ("environment".equals(codeString)) 163 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.ENVIRONMENT, code); 164 if ("biologic".equals(codeString)) 165 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.BIOLOGIC, code); 166 throw new FHIRException("Unknown AllergyIntoleranceCategory code '"+codeString+"'"); 167 } 168 public String toCode(AllergyIntoleranceCategory code) { 169 if (code == AllergyIntoleranceCategory.NULL) 170 return null; 171 if (code == AllergyIntoleranceCategory.FOOD) 172 return "food"; 173 if (code == AllergyIntoleranceCategory.MEDICATION) 174 return "medication"; 175 if (code == AllergyIntoleranceCategory.ENVIRONMENT) 176 return "environment"; 177 if (code == AllergyIntoleranceCategory.BIOLOGIC) 178 return "biologic"; 179 return "?"; 180 } 181 public String toSystem(AllergyIntoleranceCategory code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum AllergyIntoleranceCriticality { 187 /** 188 * Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure. 189 */ 190 LOW, 191 /** 192 * Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure. 193 */ 194 HIGH, 195 /** 196 * Unable to assess the worst case result of a future exposure. 197 */ 198 UNABLETOASSESS, 199 /** 200 * added to help the parsers with the generic types 201 */ 202 NULL; 203 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 204 if (codeString == null || "".equals(codeString)) 205 return null; 206 if ("low".equals(codeString)) 207 return LOW; 208 if ("high".equals(codeString)) 209 return HIGH; 210 if ("unable-to-assess".equals(codeString)) 211 return UNABLETOASSESS; 212 if (Configuration.isAcceptInvalidEnums()) 213 return null; 214 else 215 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '"+codeString+"'"); 216 } 217 public String toCode() { 218 switch (this) { 219 case LOW: return "low"; 220 case HIGH: return "high"; 221 case UNABLETOASSESS: return "unable-to-assess"; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 public String getSystem() { 227 switch (this) { 228 case LOW: return "http://hl7.org/fhir/allergy-intolerance-criticality"; 229 case HIGH: return "http://hl7.org/fhir/allergy-intolerance-criticality"; 230 case UNABLETOASSESS: return "http://hl7.org/fhir/allergy-intolerance-criticality"; 231 case NULL: return null; 232 default: return "?"; 233 } 234 } 235 public String getDefinition() { 236 switch (this) { 237 case LOW: return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 238 case HIGH: return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 239 case UNABLETOASSESS: return "Unable to assess the worst case result of a future exposure."; 240 case NULL: return null; 241 default: return "?"; 242 } 243 } 244 public String getDisplay() { 245 switch (this) { 246 case LOW: return "Low Risk"; 247 case HIGH: return "High Risk"; 248 case UNABLETOASSESS: return "Unable to Assess Risk"; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 } 254 255 public static class AllergyIntoleranceCriticalityEnumFactory implements EnumFactory<AllergyIntoleranceCriticality> { 256 public AllergyIntoleranceCriticality fromCode(String codeString) throws IllegalArgumentException { 257 if (codeString == null || "".equals(codeString)) 258 if (codeString == null || "".equals(codeString)) 259 return null; 260 if ("low".equals(codeString)) 261 return AllergyIntoleranceCriticality.LOW; 262 if ("high".equals(codeString)) 263 return AllergyIntoleranceCriticality.HIGH; 264 if ("unable-to-assess".equals(codeString)) 265 return AllergyIntoleranceCriticality.UNABLETOASSESS; 266 throw new IllegalArgumentException("Unknown AllergyIntoleranceCriticality code '"+codeString+"'"); 267 } 268 public Enumeration<AllergyIntoleranceCriticality> fromType(PrimitiveType<?> code) throws FHIRException { 269 if (code == null) 270 return null; 271 if (code.isEmpty()) 272 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.NULL, code); 273 String codeString = ((PrimitiveType) code).asStringValue(); 274 if (codeString == null || "".equals(codeString)) 275 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.NULL, code); 276 if ("low".equals(codeString)) 277 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.LOW, code); 278 if ("high".equals(codeString)) 279 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.HIGH, code); 280 if ("unable-to-assess".equals(codeString)) 281 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.UNABLETOASSESS, code); 282 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '"+codeString+"'"); 283 } 284 public String toCode(AllergyIntoleranceCriticality code) { 285 if (code == AllergyIntoleranceCriticality.NULL) 286 return null; 287 if (code == AllergyIntoleranceCriticality.LOW) 288 return "low"; 289 if (code == AllergyIntoleranceCriticality.HIGH) 290 return "high"; 291 if (code == AllergyIntoleranceCriticality.UNABLETOASSESS) 292 return "unable-to-assess"; 293 return "?"; 294 } 295 public String toSystem(AllergyIntoleranceCriticality code) { 296 return code.getSystem(); 297 } 298 } 299 300 public enum AllergyIntoleranceSeverity { 301 /** 302 * Causes mild physiological effects. 303 */ 304 MILD, 305 /** 306 * Causes moderate physiological effects. 307 */ 308 MODERATE, 309 /** 310 * Causes severe physiological effects. 311 */ 312 SEVERE, 313 /** 314 * added to help the parsers with the generic types 315 */ 316 NULL; 317 public static AllergyIntoleranceSeverity fromCode(String codeString) throws FHIRException { 318 if (codeString == null || "".equals(codeString)) 319 return null; 320 if ("mild".equals(codeString)) 321 return MILD; 322 if ("moderate".equals(codeString)) 323 return MODERATE; 324 if ("severe".equals(codeString)) 325 return SEVERE; 326 if (Configuration.isAcceptInvalidEnums()) 327 return null; 328 else 329 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '"+codeString+"'"); 330 } 331 public String toCode() { 332 switch (this) { 333 case MILD: return "mild"; 334 case MODERATE: return "moderate"; 335 case SEVERE: return "severe"; 336 case NULL: return null; 337 default: return "?"; 338 } 339 } 340 public String getSystem() { 341 switch (this) { 342 case MILD: return "http://hl7.org/fhir/reaction-event-severity"; 343 case MODERATE: return "http://hl7.org/fhir/reaction-event-severity"; 344 case SEVERE: return "http://hl7.org/fhir/reaction-event-severity"; 345 case NULL: return null; 346 default: return "?"; 347 } 348 } 349 public String getDefinition() { 350 switch (this) { 351 case MILD: return "Causes mild physiological effects."; 352 case MODERATE: return "Causes moderate physiological effects."; 353 case SEVERE: return "Causes severe physiological effects."; 354 case NULL: return null; 355 default: return "?"; 356 } 357 } 358 public String getDisplay() { 359 switch (this) { 360 case MILD: return "Mild"; 361 case MODERATE: return "Moderate"; 362 case SEVERE: return "Severe"; 363 case NULL: return null; 364 default: return "?"; 365 } 366 } 367 } 368 369 public static class AllergyIntoleranceSeverityEnumFactory implements EnumFactory<AllergyIntoleranceSeverity> { 370 public AllergyIntoleranceSeverity fromCode(String codeString) throws IllegalArgumentException { 371 if (codeString == null || "".equals(codeString)) 372 if (codeString == null || "".equals(codeString)) 373 return null; 374 if ("mild".equals(codeString)) 375 return AllergyIntoleranceSeverity.MILD; 376 if ("moderate".equals(codeString)) 377 return AllergyIntoleranceSeverity.MODERATE; 378 if ("severe".equals(codeString)) 379 return AllergyIntoleranceSeverity.SEVERE; 380 throw new IllegalArgumentException("Unknown AllergyIntoleranceSeverity code '"+codeString+"'"); 381 } 382 public Enumeration<AllergyIntoleranceSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 383 if (code == null) 384 return null; 385 if (code.isEmpty()) 386 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.NULL, code); 387 String codeString = ((PrimitiveType) code).asStringValue(); 388 if (codeString == null || "".equals(codeString)) 389 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.NULL, code); 390 if ("mild".equals(codeString)) 391 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MILD, code); 392 if ("moderate".equals(codeString)) 393 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MODERATE, code); 394 if ("severe".equals(codeString)) 395 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.SEVERE, code); 396 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '"+codeString+"'"); 397 } 398 public String toCode(AllergyIntoleranceSeverity code) { 399 if (code == AllergyIntoleranceSeverity.NULL) 400 return null; 401 if (code == AllergyIntoleranceSeverity.MILD) 402 return "mild"; 403 if (code == AllergyIntoleranceSeverity.MODERATE) 404 return "moderate"; 405 if (code == AllergyIntoleranceSeverity.SEVERE) 406 return "severe"; 407 return "?"; 408 } 409 public String toSystem(AllergyIntoleranceSeverity code) { 410 return code.getSystem(); 411 } 412 } 413 414 @Block() 415 public static class AllergyIntoleranceParticipantComponent extends BackboneElement implements IBaseBackboneElement { 416 /** 417 * Distinguishes the type of involvement of the actor in the activities related to the allergy or intolerance. 418 */ 419 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 420 @Description(shortDefinition="Type of involvement", formalDefinition="Distinguishes the type of involvement of the actor in the activities related to the allergy or intolerance." ) 421 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 422 protected CodeableConcept function; 423 424 /** 425 * Indicates who or what participated in the activities related to the allergy or intolerance. 426 */ 427 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Device.class, Organization.class, CareTeam.class}, order=2, min=1, max=1, modifier=false, summary=true) 428 @Description(shortDefinition="Who or what participated in the activities related to the allergy or intolerance", formalDefinition="Indicates who or what participated in the activities related to the allergy or intolerance." ) 429 protected Reference actor; 430 431 private static final long serialVersionUID = -576943815L; 432 433 /** 434 * Constructor 435 */ 436 public AllergyIntoleranceParticipantComponent() { 437 super(); 438 } 439 440 /** 441 * Constructor 442 */ 443 public AllergyIntoleranceParticipantComponent(Reference actor) { 444 super(); 445 this.setActor(actor); 446 } 447 448 /** 449 * @return {@link #function} (Distinguishes the type of involvement of the actor in the activities related to the allergy or intolerance.) 450 */ 451 public CodeableConcept getFunction() { 452 if (this.function == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create AllergyIntoleranceParticipantComponent.function"); 455 else if (Configuration.doAutoCreate()) 456 this.function = new CodeableConcept(); // cc 457 return this.function; 458 } 459 460 public boolean hasFunction() { 461 return this.function != null && !this.function.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #function} (Distinguishes the type of involvement of the actor in the activities related to the allergy or intolerance.) 466 */ 467 public AllergyIntoleranceParticipantComponent setFunction(CodeableConcept value) { 468 this.function = value; 469 return this; 470 } 471 472 /** 473 * @return {@link #actor} (Indicates who or what participated in the activities related to the allergy or intolerance.) 474 */ 475 public Reference getActor() { 476 if (this.actor == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create AllergyIntoleranceParticipantComponent.actor"); 479 else if (Configuration.doAutoCreate()) 480 this.actor = new Reference(); // cc 481 return this.actor; 482 } 483 484 public boolean hasActor() { 485 return this.actor != null && !this.actor.isEmpty(); 486 } 487 488 /** 489 * @param value {@link #actor} (Indicates who or what participated in the activities related to the allergy or intolerance.) 490 */ 491 public AllergyIntoleranceParticipantComponent setActor(Reference value) { 492 this.actor = value; 493 return this; 494 } 495 496 protected void listChildren(List<Property> children) { 497 super.listChildren(children); 498 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the activities related to the allergy or intolerance.", 0, 1, function)); 499 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device|Organization|CareTeam)", "Indicates who or what participated in the activities related to the allergy or intolerance.", 0, 1, actor)); 500 } 501 502 @Override 503 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 504 switch (_hash) { 505 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the activities related to the allergy or intolerance.", 0, 1, function); 506 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device|Organization|CareTeam)", "Indicates who or what participated in the activities related to the allergy or intolerance.", 0, 1, actor); 507 default: return super.getNamedProperty(_hash, _name, _checkValid); 508 } 509 510 } 511 512 @Override 513 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 514 switch (hash) { 515 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 516 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 517 default: return super.getProperty(hash, name, checkValid); 518 } 519 520 } 521 522 @Override 523 public Base setProperty(int hash, String name, Base value) throws FHIRException { 524 switch (hash) { 525 case 1380938712: // function 526 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 527 return value; 528 case 92645877: // actor 529 this.actor = TypeConvertor.castToReference(value); // Reference 530 return value; 531 default: return super.setProperty(hash, name, value); 532 } 533 534 } 535 536 @Override 537 public Base setProperty(String name, Base value) throws FHIRException { 538 if (name.equals("function")) { 539 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 540 } else if (name.equals("actor")) { 541 this.actor = TypeConvertor.castToReference(value); // Reference 542 } else 543 return super.setProperty(name, value); 544 return value; 545 } 546 547 @Override 548 public void removeChild(String name, Base value) throws FHIRException { 549 if (name.equals("function")) { 550 this.function = null; 551 } else if (name.equals("actor")) { 552 this.actor = null; 553 } else 554 super.removeChild(name, value); 555 556 } 557 558 @Override 559 public Base makeProperty(int hash, String name) throws FHIRException { 560 switch (hash) { 561 case 1380938712: return getFunction(); 562 case 92645877: return getActor(); 563 default: return super.makeProperty(hash, name); 564 } 565 566 } 567 568 @Override 569 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 570 switch (hash) { 571 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 572 case 92645877: /*actor*/ return new String[] {"Reference"}; 573 default: return super.getTypesForProperty(hash, name); 574 } 575 576 } 577 578 @Override 579 public Base addChild(String name) throws FHIRException { 580 if (name.equals("function")) { 581 this.function = new CodeableConcept(); 582 return this.function; 583 } 584 else if (name.equals("actor")) { 585 this.actor = new Reference(); 586 return this.actor; 587 } 588 else 589 return super.addChild(name); 590 } 591 592 public AllergyIntoleranceParticipantComponent copy() { 593 AllergyIntoleranceParticipantComponent dst = new AllergyIntoleranceParticipantComponent(); 594 copyValues(dst); 595 return dst; 596 } 597 598 public void copyValues(AllergyIntoleranceParticipantComponent dst) { 599 super.copyValues(dst); 600 dst.function = function == null ? null : function.copy(); 601 dst.actor = actor == null ? null : actor.copy(); 602 } 603 604 @Override 605 public boolean equalsDeep(Base other_) { 606 if (!super.equalsDeep(other_)) 607 return false; 608 if (!(other_ instanceof AllergyIntoleranceParticipantComponent)) 609 return false; 610 AllergyIntoleranceParticipantComponent o = (AllergyIntoleranceParticipantComponent) other_; 611 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 612 } 613 614 @Override 615 public boolean equalsShallow(Base other_) { 616 if (!super.equalsShallow(other_)) 617 return false; 618 if (!(other_ instanceof AllergyIntoleranceParticipantComponent)) 619 return false; 620 AllergyIntoleranceParticipantComponent o = (AllergyIntoleranceParticipantComponent) other_; 621 return true; 622 } 623 624 public boolean isEmpty() { 625 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 626 } 627 628 public String fhirType() { 629 return "AllergyIntolerance.participant"; 630 631 } 632 633 } 634 635 @Block() 636 public static class AllergyIntoleranceReactionComponent extends BackboneElement implements IBaseBackboneElement { 637 /** 638 * Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance. 639 */ 640 @Child(name = "substance", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 641 @Description(shortDefinition="Specific substance or pharmaceutical product considered to be responsible for event", formalDefinition="Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance." ) 642 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 643 protected CodeableConcept substance; 644 645 /** 646 * Clinical symptoms and/or signs that are observed or associated with the adverse reaction event. 647 */ 648 @Child(name = "manifestation", type = {CodeableReference.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 649 @Description(shortDefinition="Clinical symptoms/signs associated with the Event", formalDefinition="Clinical symptoms and/or signs that are observed or associated with the adverse reaction event." ) 650 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 651 protected List<CodeableReference> manifestation; 652 653 /** 654 * Text description about the reaction as a whole, including details of the manifestation if required. 655 */ 656 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 657 @Description(shortDefinition="Description of the event as a whole", formalDefinition="Text description about the reaction as a whole, including details of the manifestation if required." ) 658 protected StringType description; 659 660 /** 661 * Record of the date and/or time of the onset of the Reaction. 662 */ 663 @Child(name = "onset", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 664 @Description(shortDefinition="Date(/time) when manifestations showed", formalDefinition="Record of the date and/or time of the onset of the Reaction." ) 665 protected DateTimeType onset; 666 667 /** 668 * Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations. 669 */ 670 @Child(name = "severity", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 671 @Description(shortDefinition="mild | moderate | severe (of event as a whole)", formalDefinition="Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations." ) 672 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reaction-event-severity") 673 protected Enumeration<AllergyIntoleranceSeverity> severity; 674 675 /** 676 * Identification of the route by which the subject was exposed to the substance. 677 */ 678 @Child(name = "exposureRoute", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 679 @Description(shortDefinition="How the subject was exposed to the substance", formalDefinition="Identification of the route by which the subject was exposed to the substance." ) 680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 681 protected CodeableConcept exposureRoute; 682 683 /** 684 * Additional text about the adverse reaction event not captured in other fields. 685 */ 686 @Child(name = "note", type = {Annotation.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 687 @Description(shortDefinition="Text about event not captured in other fields", formalDefinition="Additional text about the adverse reaction event not captured in other fields." ) 688 protected List<Annotation> note; 689 690 private static final long serialVersionUID = 1007789961L; 691 692 /** 693 * Constructor 694 */ 695 public AllergyIntoleranceReactionComponent() { 696 super(); 697 } 698 699 /** 700 * Constructor 701 */ 702 public AllergyIntoleranceReactionComponent(CodeableReference manifestation) { 703 super(); 704 this.addManifestation(manifestation); 705 } 706 707 /** 708 * @return {@link #substance} (Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.) 709 */ 710 public CodeableConcept getSubstance() { 711 if (this.substance == null) 712 if (Configuration.errorOnAutoCreate()) 713 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.substance"); 714 else if (Configuration.doAutoCreate()) 715 this.substance = new CodeableConcept(); // cc 716 return this.substance; 717 } 718 719 public boolean hasSubstance() { 720 return this.substance != null && !this.substance.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #substance} (Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.) 725 */ 726 public AllergyIntoleranceReactionComponent setSubstance(CodeableConcept value) { 727 this.substance = value; 728 return this; 729 } 730 731 /** 732 * @return {@link #manifestation} (Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.) 733 */ 734 public List<CodeableReference> getManifestation() { 735 if (this.manifestation == null) 736 this.manifestation = new ArrayList<CodeableReference>(); 737 return this.manifestation; 738 } 739 740 /** 741 * @return Returns a reference to <code>this</code> for easy method chaining 742 */ 743 public AllergyIntoleranceReactionComponent setManifestation(List<CodeableReference> theManifestation) { 744 this.manifestation = theManifestation; 745 return this; 746 } 747 748 public boolean hasManifestation() { 749 if (this.manifestation == null) 750 return false; 751 for (CodeableReference item : this.manifestation) 752 if (!item.isEmpty()) 753 return true; 754 return false; 755 } 756 757 public CodeableReference addManifestation() { //3 758 CodeableReference t = new CodeableReference(); 759 if (this.manifestation == null) 760 this.manifestation = new ArrayList<CodeableReference>(); 761 this.manifestation.add(t); 762 return t; 763 } 764 765 public AllergyIntoleranceReactionComponent addManifestation(CodeableReference t) { //3 766 if (t == null) 767 return this; 768 if (this.manifestation == null) 769 this.manifestation = new ArrayList<CodeableReference>(); 770 this.manifestation.add(t); 771 return this; 772 } 773 774 /** 775 * @return The first repetition of repeating field {@link #manifestation}, creating it if it does not already exist {3} 776 */ 777 public CodeableReference getManifestationFirstRep() { 778 if (getManifestation().isEmpty()) { 779 addManifestation(); 780 } 781 return getManifestation().get(0); 782 } 783 784 /** 785 * @return {@link #description} (Text description about the reaction as a whole, including details of the manifestation if required.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 786 */ 787 public StringType getDescriptionElement() { 788 if (this.description == null) 789 if (Configuration.errorOnAutoCreate()) 790 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.description"); 791 else if (Configuration.doAutoCreate()) 792 this.description = new StringType(); // bb 793 return this.description; 794 } 795 796 public boolean hasDescriptionElement() { 797 return this.description != null && !this.description.isEmpty(); 798 } 799 800 public boolean hasDescription() { 801 return this.description != null && !this.description.isEmpty(); 802 } 803 804 /** 805 * @param value {@link #description} (Text description about the reaction as a whole, including details of the manifestation if required.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 806 */ 807 public AllergyIntoleranceReactionComponent setDescriptionElement(StringType value) { 808 this.description = value; 809 return this; 810 } 811 812 /** 813 * @return Text description about the reaction as a whole, including details of the manifestation if required. 814 */ 815 public String getDescription() { 816 return this.description == null ? null : this.description.getValue(); 817 } 818 819 /** 820 * @param value Text description about the reaction as a whole, including details of the manifestation if required. 821 */ 822 public AllergyIntoleranceReactionComponent setDescription(String value) { 823 if (Utilities.noString(value)) 824 this.description = null; 825 else { 826 if (this.description == null) 827 this.description = new StringType(); 828 this.description.setValue(value); 829 } 830 return this; 831 } 832 833 /** 834 * @return {@link #onset} (Record of the date and/or time of the onset of the Reaction.). This is the underlying object with id, value and extensions. The accessor "getOnset" gives direct access to the value 835 */ 836 public DateTimeType getOnsetElement() { 837 if (this.onset == null) 838 if (Configuration.errorOnAutoCreate()) 839 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.onset"); 840 else if (Configuration.doAutoCreate()) 841 this.onset = new DateTimeType(); // bb 842 return this.onset; 843 } 844 845 public boolean hasOnsetElement() { 846 return this.onset != null && !this.onset.isEmpty(); 847 } 848 849 public boolean hasOnset() { 850 return this.onset != null && !this.onset.isEmpty(); 851 } 852 853 /** 854 * @param value {@link #onset} (Record of the date and/or time of the onset of the Reaction.). This is the underlying object with id, value and extensions. The accessor "getOnset" gives direct access to the value 855 */ 856 public AllergyIntoleranceReactionComponent setOnsetElement(DateTimeType value) { 857 this.onset = value; 858 return this; 859 } 860 861 /** 862 * @return Record of the date and/or time of the onset of the Reaction. 863 */ 864 public Date getOnset() { 865 return this.onset == null ? null : this.onset.getValue(); 866 } 867 868 /** 869 * @param value Record of the date and/or time of the onset of the Reaction. 870 */ 871 public AllergyIntoleranceReactionComponent setOnset(Date value) { 872 if (value == null) 873 this.onset = null; 874 else { 875 if (this.onset == null) 876 this.onset = new DateTimeType(); 877 this.onset.setValue(value); 878 } 879 return this; 880 } 881 882 /** 883 * @return {@link #severity} (Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 884 */ 885 public Enumeration<AllergyIntoleranceSeverity> getSeverityElement() { 886 if (this.severity == null) 887 if (Configuration.errorOnAutoCreate()) 888 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.severity"); 889 else if (Configuration.doAutoCreate()) 890 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); // bb 891 return this.severity; 892 } 893 894 public boolean hasSeverityElement() { 895 return this.severity != null && !this.severity.isEmpty(); 896 } 897 898 public boolean hasSeverity() { 899 return this.severity != null && !this.severity.isEmpty(); 900 } 901 902 /** 903 * @param value {@link #severity} (Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 904 */ 905 public AllergyIntoleranceReactionComponent setSeverityElement(Enumeration<AllergyIntoleranceSeverity> value) { 906 this.severity = value; 907 return this; 908 } 909 910 /** 911 * @return Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations. 912 */ 913 public AllergyIntoleranceSeverity getSeverity() { 914 return this.severity == null ? null : this.severity.getValue(); 915 } 916 917 /** 918 * @param value Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations. 919 */ 920 public AllergyIntoleranceReactionComponent setSeverity(AllergyIntoleranceSeverity value) { 921 if (value == null) 922 this.severity = null; 923 else { 924 if (this.severity == null) 925 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); 926 this.severity.setValue(value); 927 } 928 return this; 929 } 930 931 /** 932 * @return {@link #exposureRoute} (Identification of the route by which the subject was exposed to the substance.) 933 */ 934 public CodeableConcept getExposureRoute() { 935 if (this.exposureRoute == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.exposureRoute"); 938 else if (Configuration.doAutoCreate()) 939 this.exposureRoute = new CodeableConcept(); // cc 940 return this.exposureRoute; 941 } 942 943 public boolean hasExposureRoute() { 944 return this.exposureRoute != null && !this.exposureRoute.isEmpty(); 945 } 946 947 /** 948 * @param value {@link #exposureRoute} (Identification of the route by which the subject was exposed to the substance.) 949 */ 950 public AllergyIntoleranceReactionComponent setExposureRoute(CodeableConcept value) { 951 this.exposureRoute = value; 952 return this; 953 } 954 955 /** 956 * @return {@link #note} (Additional text about the adverse reaction event not captured in other fields.) 957 */ 958 public List<Annotation> getNote() { 959 if (this.note == null) 960 this.note = new ArrayList<Annotation>(); 961 return this.note; 962 } 963 964 /** 965 * @return Returns a reference to <code>this</code> for easy method chaining 966 */ 967 public AllergyIntoleranceReactionComponent setNote(List<Annotation> theNote) { 968 this.note = theNote; 969 return this; 970 } 971 972 public boolean hasNote() { 973 if (this.note == null) 974 return false; 975 for (Annotation item : this.note) 976 if (!item.isEmpty()) 977 return true; 978 return false; 979 } 980 981 public Annotation addNote() { //3 982 Annotation t = new Annotation(); 983 if (this.note == null) 984 this.note = new ArrayList<Annotation>(); 985 this.note.add(t); 986 return t; 987 } 988 989 public AllergyIntoleranceReactionComponent addNote(Annotation t) { //3 990 if (t == null) 991 return this; 992 if (this.note == null) 993 this.note = new ArrayList<Annotation>(); 994 this.note.add(t); 995 return this; 996 } 997 998 /** 999 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1000 */ 1001 public Annotation getNoteFirstRep() { 1002 if (getNote().isEmpty()) { 1003 addNote(); 1004 } 1005 return getNote().get(0); 1006 } 1007 1008 protected void listChildren(List<Property> children) { 1009 super.listChildren(children); 1010 children.add(new Property("substance", "CodeableConcept", "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 0, 1, substance)); 1011 children.add(new Property("manifestation", "CodeableReference(Observation)", "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, java.lang.Integer.MAX_VALUE, manifestation)); 1012 children.add(new Property("description", "string", "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, description)); 1013 children.add(new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 0, 1, onset)); 1014 children.add(new Property("severity", "code", "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 0, 1, severity)); 1015 children.add(new Property("exposureRoute", "CodeableConcept", "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute)); 1016 children.add(new Property("note", "Annotation", "Additional text about the adverse reaction event not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note)); 1017 } 1018 1019 @Override 1020 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1021 switch (_hash) { 1022 case 530040176: /*substance*/ return new Property("substance", "CodeableConcept", "Identification of the specific substance (or pharmaceutical product) considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 0, 1, substance); 1023 case 1115984422: /*manifestation*/ return new Property("manifestation", "CodeableReference(Observation)", "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, java.lang.Integer.MAX_VALUE, manifestation); 1024 case -1724546052: /*description*/ return new Property("description", "string", "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1, description); 1025 case 105901603: /*onset*/ return new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 0, 1, onset); 1026 case 1478300413: /*severity*/ return new Property("severity", "code", "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 0, 1, severity); 1027 case 421286274: /*exposureRoute*/ return new Property("exposureRoute", "CodeableConcept", "Identification of the route by which the subject was exposed to the substance.", 0, 1, exposureRoute); 1028 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional text about the adverse reaction event not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note); 1029 default: return super.getNamedProperty(_hash, _name, _checkValid); 1030 } 1031 1032 } 1033 1034 @Override 1035 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1036 switch (hash) { 1037 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // CodeableConcept 1038 case 1115984422: /*manifestation*/ return this.manifestation == null ? new Base[0] : this.manifestation.toArray(new Base[this.manifestation.size()]); // CodeableReference 1039 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1040 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // DateTimeType 1041 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<AllergyIntoleranceSeverity> 1042 case 421286274: /*exposureRoute*/ return this.exposureRoute == null ? new Base[0] : new Base[] {this.exposureRoute}; // CodeableConcept 1043 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1044 default: return super.getProperty(hash, name, checkValid); 1045 } 1046 1047 } 1048 1049 @Override 1050 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1051 switch (hash) { 1052 case 530040176: // substance 1053 this.substance = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1054 return value; 1055 case 1115984422: // manifestation 1056 this.getManifestation().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1057 return value; 1058 case -1724546052: // description 1059 this.description = TypeConvertor.castToString(value); // StringType 1060 return value; 1061 case 105901603: // onset 1062 this.onset = TypeConvertor.castToDateTime(value); // DateTimeType 1063 return value; 1064 case 1478300413: // severity 1065 value = new AllergyIntoleranceSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1066 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1067 return value; 1068 case 421286274: // exposureRoute 1069 this.exposureRoute = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1070 return value; 1071 case 3387378: // note 1072 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1073 return value; 1074 default: return super.setProperty(hash, name, value); 1075 } 1076 1077 } 1078 1079 @Override 1080 public Base setProperty(String name, Base value) throws FHIRException { 1081 if (name.equals("substance")) { 1082 this.substance = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1083 } else if (name.equals("manifestation")) { 1084 this.getManifestation().add(TypeConvertor.castToCodeableReference(value)); 1085 } else if (name.equals("description")) { 1086 this.description = TypeConvertor.castToString(value); // StringType 1087 } else if (name.equals("onset")) { 1088 this.onset = TypeConvertor.castToDateTime(value); // DateTimeType 1089 } else if (name.equals("severity")) { 1090 value = new AllergyIntoleranceSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1091 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1092 } else if (name.equals("exposureRoute")) { 1093 this.exposureRoute = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1094 } else if (name.equals("note")) { 1095 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1096 } else 1097 return super.setProperty(name, value); 1098 return value; 1099 } 1100 1101 @Override 1102 public void removeChild(String name, Base value) throws FHIRException { 1103 if (name.equals("substance")) { 1104 this.substance = null; 1105 } else if (name.equals("manifestation")) { 1106 this.getManifestation().remove(value); 1107 } else if (name.equals("description")) { 1108 this.description = null; 1109 } else if (name.equals("onset")) { 1110 this.onset = null; 1111 } else if (name.equals("severity")) { 1112 value = new AllergyIntoleranceSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1113 this.severity = (Enumeration) value; // Enumeration<AllergyIntoleranceSeverity> 1114 } else if (name.equals("exposureRoute")) { 1115 this.exposureRoute = null; 1116 } else if (name.equals("note")) { 1117 this.getNote().remove(value); 1118 } else 1119 super.removeChild(name, value); 1120 1121 } 1122 1123 @Override 1124 public Base makeProperty(int hash, String name) throws FHIRException { 1125 switch (hash) { 1126 case 530040176: return getSubstance(); 1127 case 1115984422: return addManifestation(); 1128 case -1724546052: return getDescriptionElement(); 1129 case 105901603: return getOnsetElement(); 1130 case 1478300413: return getSeverityElement(); 1131 case 421286274: return getExposureRoute(); 1132 case 3387378: return addNote(); 1133 default: return super.makeProperty(hash, name); 1134 } 1135 1136 } 1137 1138 @Override 1139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1140 switch (hash) { 1141 case 530040176: /*substance*/ return new String[] {"CodeableConcept"}; 1142 case 1115984422: /*manifestation*/ return new String[] {"CodeableReference"}; 1143 case -1724546052: /*description*/ return new String[] {"string"}; 1144 case 105901603: /*onset*/ return new String[] {"dateTime"}; 1145 case 1478300413: /*severity*/ return new String[] {"code"}; 1146 case 421286274: /*exposureRoute*/ return new String[] {"CodeableConcept"}; 1147 case 3387378: /*note*/ return new String[] {"Annotation"}; 1148 default: return super.getTypesForProperty(hash, name); 1149 } 1150 1151 } 1152 1153 @Override 1154 public Base addChild(String name) throws FHIRException { 1155 if (name.equals("substance")) { 1156 this.substance = new CodeableConcept(); 1157 return this.substance; 1158 } 1159 else if (name.equals("manifestation")) { 1160 return addManifestation(); 1161 } 1162 else if (name.equals("description")) { 1163 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.reaction.description"); 1164 } 1165 else if (name.equals("onset")) { 1166 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.reaction.onset"); 1167 } 1168 else if (name.equals("severity")) { 1169 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.reaction.severity"); 1170 } 1171 else if (name.equals("exposureRoute")) { 1172 this.exposureRoute = new CodeableConcept(); 1173 return this.exposureRoute; 1174 } 1175 else if (name.equals("note")) { 1176 return addNote(); 1177 } 1178 else 1179 return super.addChild(name); 1180 } 1181 1182 public AllergyIntoleranceReactionComponent copy() { 1183 AllergyIntoleranceReactionComponent dst = new AllergyIntoleranceReactionComponent(); 1184 copyValues(dst); 1185 return dst; 1186 } 1187 1188 public void copyValues(AllergyIntoleranceReactionComponent dst) { 1189 super.copyValues(dst); 1190 dst.substance = substance == null ? null : substance.copy(); 1191 if (manifestation != null) { 1192 dst.manifestation = new ArrayList<CodeableReference>(); 1193 for (CodeableReference i : manifestation) 1194 dst.manifestation.add(i.copy()); 1195 }; 1196 dst.description = description == null ? null : description.copy(); 1197 dst.onset = onset == null ? null : onset.copy(); 1198 dst.severity = severity == null ? null : severity.copy(); 1199 dst.exposureRoute = exposureRoute == null ? null : exposureRoute.copy(); 1200 if (note != null) { 1201 dst.note = new ArrayList<Annotation>(); 1202 for (Annotation i : note) 1203 dst.note.add(i.copy()); 1204 }; 1205 } 1206 1207 @Override 1208 public boolean equalsDeep(Base other_) { 1209 if (!super.equalsDeep(other_)) 1210 return false; 1211 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1212 return false; 1213 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1214 return compareDeep(substance, o.substance, true) && compareDeep(manifestation, o.manifestation, true) 1215 && compareDeep(description, o.description, true) && compareDeep(onset, o.onset, true) && compareDeep(severity, o.severity, true) 1216 && compareDeep(exposureRoute, o.exposureRoute, true) && compareDeep(note, o.note, true); 1217 } 1218 1219 @Override 1220 public boolean equalsShallow(Base other_) { 1221 if (!super.equalsShallow(other_)) 1222 return false; 1223 if (!(other_ instanceof AllergyIntoleranceReactionComponent)) 1224 return false; 1225 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other_; 1226 return compareValues(description, o.description, true) && compareValues(onset, o.onset, true) && compareValues(severity, o.severity, true) 1227 ; 1228 } 1229 1230 public boolean isEmpty() { 1231 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, manifestation, description 1232 , onset, severity, exposureRoute, note); 1233 } 1234 1235 public String fhirType() { 1236 return "AllergyIntolerance.reaction"; 1237 1238 } 1239 1240 } 1241 1242 /** 1243 * Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 1244 */ 1245 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1246 @Description(shortDefinition="External ids for this item", formalDefinition="Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 1247 protected List<Identifier> identifier; 1248 1249 /** 1250 * The clinical status of the allergy or intolerance. 1251 */ 1252 @Child(name = "clinicalStatus", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=true, summary=true) 1253 @Description(shortDefinition="active | inactive | resolved", formalDefinition="The clinical status of the allergy or intolerance." ) 1254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergyintolerance-clinical") 1255 protected CodeableConcept clinicalStatus; 1256 1257 /** 1258 * Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). The verification status pertains to the allergy or intolerance, itself, not to any specific AllergyIntolerance attribute. 1259 */ 1260 @Child(name = "verificationStatus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=true, summary=true) 1261 @Description(shortDefinition="unconfirmed | presumed | confirmed | refuted | entered-in-error", formalDefinition="Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). The verification status pertains to the allergy or intolerance, itself, not to any specific AllergyIntolerance attribute." ) 1262 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergyintolerance-verification") 1263 protected CodeableConcept verificationStatus; 1264 1265 /** 1266 * Identification of the underlying physiological mechanism for the reaction risk. 1267 */ 1268 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1269 @Description(shortDefinition="allergy | intolerance - Underlying mechanism (if known)", formalDefinition="Identification of the underlying physiological mechanism for the reaction risk." ) 1270 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-intolerance-type") 1271 protected CodeableConcept type; 1272 1273 /** 1274 * Category of the identified substance. 1275 */ 1276 @Child(name = "category", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1277 @Description(shortDefinition="food | medication | environment | biologic", formalDefinition="Category of the identified substance." ) 1278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-intolerance-category") 1279 protected List<Enumeration<AllergyIntoleranceCategory>> category; 1280 1281 /** 1282 * Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance. 1283 */ 1284 @Child(name = "criticality", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1285 @Description(shortDefinition="low | high | unable-to-assess", formalDefinition="Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance." ) 1286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergy-intolerance-criticality") 1287 protected Enumeration<AllergyIntoleranceCriticality> criticality; 1288 1289 /** 1290 * Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., "Latex allergy"), or a negated/excluded code for a specific substance or class (e.g., "No latex allergy") or a general or categorical negated statement (e.g., "No known allergy", "No known drug allergies"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance. 1291 */ 1292 @Child(name = "code", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1293 @Description(shortDefinition="Code that identifies the allergy or intolerance", formalDefinition="Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance." ) 1294 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/allergyintolerance-code") 1295 protected CodeableConcept code; 1296 1297 /** 1298 * The patient who has the allergy or intolerance. 1299 */ 1300 @Child(name = "patient", type = {Patient.class}, order=7, min=1, max=1, modifier=false, summary=true) 1301 @Description(shortDefinition="Who the allergy or intolerance is for", formalDefinition="The patient who has the allergy or intolerance." ) 1302 protected Reference patient; 1303 1304 /** 1305 * The encounter when the allergy or intolerance was asserted. 1306 */ 1307 @Child(name = "encounter", type = {Encounter.class}, order=8, min=0, max=1, modifier=false, summary=false) 1308 @Description(shortDefinition="Encounter when the allergy or intolerance was asserted", formalDefinition="The encounter when the allergy or intolerance was asserted." ) 1309 protected Reference encounter; 1310 1311 /** 1312 * Estimated or actual date, date-time, or age when allergy or intolerance was identified. 1313 */ 1314 @Child(name = "onset", type = {DateTimeType.class, Age.class, Period.class, Range.class, StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1315 @Description(shortDefinition="When allergy or intolerance was identified", formalDefinition="Estimated or actual date, date-time, or age when allergy or intolerance was identified." ) 1316 protected DataType onset; 1317 1318 /** 1319 * The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date. 1320 */ 1321 @Child(name = "recordedDate", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 1322 @Description(shortDefinition="Date allergy or intolerance was first recorded", formalDefinition="The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date." ) 1323 protected DateTimeType recordedDate; 1324 1325 /** 1326 * Indicates who or what participated in the activities related to the allergy or intolerance and how they were involved. 1327 */ 1328 @Child(name = "participant", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1329 @Description(shortDefinition="Who or what participated in the activities related to the allergy or intolerance and how they were involved", formalDefinition="Indicates who or what participated in the activities related to the allergy or intolerance and how they were involved." ) 1330 protected List<AllergyIntoleranceParticipantComponent> participant; 1331 1332 /** 1333 * Represents the date and/or time of the last known occurrence of a reaction event. 1334 */ 1335 @Child(name = "lastOccurrence", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1336 @Description(shortDefinition="Date(/time) of last known occurrence of a reaction", formalDefinition="Represents the date and/or time of the last known occurrence of a reaction event." ) 1337 protected DateTimeType lastOccurrence; 1338 1339 /** 1340 * Additional narrative about the propensity for the Adverse Reaction, not captured in other fields. 1341 */ 1342 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1343 @Description(shortDefinition="Additional text not captured in other fields", formalDefinition="Additional narrative about the propensity for the Adverse Reaction, not captured in other fields." ) 1344 protected List<Annotation> note; 1345 1346 /** 1347 * Details about each adverse reaction event linked to exposure to the identified substance. 1348 */ 1349 @Child(name = "reaction", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1350 @Description(shortDefinition="Adverse Reaction Events linked to exposure to substance", formalDefinition="Details about each adverse reaction event linked to exposure to the identified substance." ) 1351 protected List<AllergyIntoleranceReactionComponent> reaction; 1352 1353 private static final long serialVersionUID = -1955746770L; 1354 1355 /** 1356 * Constructor 1357 */ 1358 public AllergyIntolerance() { 1359 super(); 1360 } 1361 1362 /** 1363 * Constructor 1364 */ 1365 public AllergyIntolerance(Reference patient) { 1366 super(); 1367 this.setPatient(patient); 1368 } 1369 1370 /** 1371 * @return {@link #identifier} (Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 1372 */ 1373 public List<Identifier> getIdentifier() { 1374 if (this.identifier == null) 1375 this.identifier = new ArrayList<Identifier>(); 1376 return this.identifier; 1377 } 1378 1379 /** 1380 * @return Returns a reference to <code>this</code> for easy method chaining 1381 */ 1382 public AllergyIntolerance setIdentifier(List<Identifier> theIdentifier) { 1383 this.identifier = theIdentifier; 1384 return this; 1385 } 1386 1387 public boolean hasIdentifier() { 1388 if (this.identifier == null) 1389 return false; 1390 for (Identifier item : this.identifier) 1391 if (!item.isEmpty()) 1392 return true; 1393 return false; 1394 } 1395 1396 public Identifier addIdentifier() { //3 1397 Identifier t = new Identifier(); 1398 if (this.identifier == null) 1399 this.identifier = new ArrayList<Identifier>(); 1400 this.identifier.add(t); 1401 return t; 1402 } 1403 1404 public AllergyIntolerance addIdentifier(Identifier t) { //3 1405 if (t == null) 1406 return this; 1407 if (this.identifier == null) 1408 this.identifier = new ArrayList<Identifier>(); 1409 this.identifier.add(t); 1410 return this; 1411 } 1412 1413 /** 1414 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1415 */ 1416 public Identifier getIdentifierFirstRep() { 1417 if (getIdentifier().isEmpty()) { 1418 addIdentifier(); 1419 } 1420 return getIdentifier().get(0); 1421 } 1422 1423 /** 1424 * @return {@link #clinicalStatus} (The clinical status of the allergy or intolerance.) 1425 */ 1426 public CodeableConcept getClinicalStatus() { 1427 if (this.clinicalStatus == null) 1428 if (Configuration.errorOnAutoCreate()) 1429 throw new Error("Attempt to auto-create AllergyIntolerance.clinicalStatus"); 1430 else if (Configuration.doAutoCreate()) 1431 this.clinicalStatus = new CodeableConcept(); // cc 1432 return this.clinicalStatus; 1433 } 1434 1435 public boolean hasClinicalStatus() { 1436 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 1437 } 1438 1439 /** 1440 * @param value {@link #clinicalStatus} (The clinical status of the allergy or intolerance.) 1441 */ 1442 public AllergyIntolerance setClinicalStatus(CodeableConcept value) { 1443 this.clinicalStatus = value; 1444 return this; 1445 } 1446 1447 /** 1448 * @return {@link #verificationStatus} (Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). The verification status pertains to the allergy or intolerance, itself, not to any specific AllergyIntolerance attribute.) 1449 */ 1450 public CodeableConcept getVerificationStatus() { 1451 if (this.verificationStatus == null) 1452 if (Configuration.errorOnAutoCreate()) 1453 throw new Error("Attempt to auto-create AllergyIntolerance.verificationStatus"); 1454 else if (Configuration.doAutoCreate()) 1455 this.verificationStatus = new CodeableConcept(); // cc 1456 return this.verificationStatus; 1457 } 1458 1459 public boolean hasVerificationStatus() { 1460 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1461 } 1462 1463 /** 1464 * @param value {@link #verificationStatus} (Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). The verification status pertains to the allergy or intolerance, itself, not to any specific AllergyIntolerance attribute.) 1465 */ 1466 public AllergyIntolerance setVerificationStatus(CodeableConcept value) { 1467 this.verificationStatus = value; 1468 return this; 1469 } 1470 1471 /** 1472 * @return {@link #type} (Identification of the underlying physiological mechanism for the reaction risk.) 1473 */ 1474 public CodeableConcept getType() { 1475 if (this.type == null) 1476 if (Configuration.errorOnAutoCreate()) 1477 throw new Error("Attempt to auto-create AllergyIntolerance.type"); 1478 else if (Configuration.doAutoCreate()) 1479 this.type = new CodeableConcept(); // cc 1480 return this.type; 1481 } 1482 1483 public boolean hasType() { 1484 return this.type != null && !this.type.isEmpty(); 1485 } 1486 1487 /** 1488 * @param value {@link #type} (Identification of the underlying physiological mechanism for the reaction risk.) 1489 */ 1490 public AllergyIntolerance setType(CodeableConcept value) { 1491 this.type = value; 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #category} (Category of the identified substance.) 1497 */ 1498 public List<Enumeration<AllergyIntoleranceCategory>> getCategory() { 1499 if (this.category == null) 1500 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1501 return this.category; 1502 } 1503 1504 /** 1505 * @return Returns a reference to <code>this</code> for easy method chaining 1506 */ 1507 public AllergyIntolerance setCategory(List<Enumeration<AllergyIntoleranceCategory>> theCategory) { 1508 this.category = theCategory; 1509 return this; 1510 } 1511 1512 public boolean hasCategory() { 1513 if (this.category == null) 1514 return false; 1515 for (Enumeration<AllergyIntoleranceCategory> item : this.category) 1516 if (!item.isEmpty()) 1517 return true; 1518 return false; 1519 } 1520 1521 /** 1522 * @return {@link #category} (Category of the identified substance.) 1523 */ 1524 public Enumeration<AllergyIntoleranceCategory> addCategoryElement() {//2 1525 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); 1526 if (this.category == null) 1527 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1528 this.category.add(t); 1529 return t; 1530 } 1531 1532 /** 1533 * @param value {@link #category} (Category of the identified substance.) 1534 */ 1535 public AllergyIntolerance addCategory(AllergyIntoleranceCategory value) { //1 1536 Enumeration<AllergyIntoleranceCategory> t = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); 1537 t.setValue(value); 1538 if (this.category == null) 1539 this.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 1540 this.category.add(t); 1541 return this; 1542 } 1543 1544 /** 1545 * @param value {@link #category} (Category of the identified substance.) 1546 */ 1547 public boolean hasCategory(AllergyIntoleranceCategory value) { 1548 if (this.category == null) 1549 return false; 1550 for (Enumeration<AllergyIntoleranceCategory> v : this.category) 1551 if (v.getValue().equals(value)) // code 1552 return true; 1553 return false; 1554 } 1555 1556 /** 1557 * @return {@link #criticality} (Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.). This is the underlying object with id, value and extensions. The accessor "getCriticality" gives direct access to the value 1558 */ 1559 public Enumeration<AllergyIntoleranceCriticality> getCriticalityElement() { 1560 if (this.criticality == null) 1561 if (Configuration.errorOnAutoCreate()) 1562 throw new Error("Attempt to auto-create AllergyIntolerance.criticality"); 1563 else if (Configuration.doAutoCreate()) 1564 this.criticality = new Enumeration<AllergyIntoleranceCriticality>(new AllergyIntoleranceCriticalityEnumFactory()); // bb 1565 return this.criticality; 1566 } 1567 1568 public boolean hasCriticalityElement() { 1569 return this.criticality != null && !this.criticality.isEmpty(); 1570 } 1571 1572 public boolean hasCriticality() { 1573 return this.criticality != null && !this.criticality.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #criticality} (Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.). This is the underlying object with id, value and extensions. The accessor "getCriticality" gives direct access to the value 1578 */ 1579 public AllergyIntolerance setCriticalityElement(Enumeration<AllergyIntoleranceCriticality> value) { 1580 this.criticality = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance. 1586 */ 1587 public AllergyIntoleranceCriticality getCriticality() { 1588 return this.criticality == null ? null : this.criticality.getValue(); 1589 } 1590 1591 /** 1592 * @param value Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance. 1593 */ 1594 public AllergyIntolerance setCriticality(AllergyIntoleranceCriticality value) { 1595 if (value == null) 1596 this.criticality = null; 1597 else { 1598 if (this.criticality == null) 1599 this.criticality = new Enumeration<AllergyIntoleranceCriticality>(new AllergyIntoleranceCriticalityEnumFactory()); 1600 this.criticality.setValue(value); 1601 } 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #code} (Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., "Latex allergy"), or a negated/excluded code for a specific substance or class (e.g., "No latex allergy") or a general or categorical negated statement (e.g., "No known allergy", "No known drug allergies"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.) 1607 */ 1608 public CodeableConcept getCode() { 1609 if (this.code == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create AllergyIntolerance.code"); 1612 else if (Configuration.doAutoCreate()) 1613 this.code = new CodeableConcept(); // cc 1614 return this.code; 1615 } 1616 1617 public boolean hasCode() { 1618 return this.code != null && !this.code.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #code} (Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., "Latex"), an allergy or intolerance condition (e.g., "Latex allergy"), or a negated/excluded code for a specific substance or class (e.g., "No latex allergy") or a general or categorical negated statement (e.g., "No known allergy", "No known drug allergies"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.) 1623 */ 1624 public AllergyIntolerance setCode(CodeableConcept value) { 1625 this.code = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #patient} (The patient who has the allergy or intolerance.) 1631 */ 1632 public Reference getPatient() { 1633 if (this.patient == null) 1634 if (Configuration.errorOnAutoCreate()) 1635 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1636 else if (Configuration.doAutoCreate()) 1637 this.patient = new Reference(); // cc 1638 return this.patient; 1639 } 1640 1641 public boolean hasPatient() { 1642 return this.patient != null && !this.patient.isEmpty(); 1643 } 1644 1645 /** 1646 * @param value {@link #patient} (The patient who has the allergy or intolerance.) 1647 */ 1648 public AllergyIntolerance setPatient(Reference value) { 1649 this.patient = value; 1650 return this; 1651 } 1652 1653 /** 1654 * @return {@link #encounter} (The encounter when the allergy or intolerance was asserted.) 1655 */ 1656 public Reference getEncounter() { 1657 if (this.encounter == null) 1658 if (Configuration.errorOnAutoCreate()) 1659 throw new Error("Attempt to auto-create AllergyIntolerance.encounter"); 1660 else if (Configuration.doAutoCreate()) 1661 this.encounter = new Reference(); // cc 1662 return this.encounter; 1663 } 1664 1665 public boolean hasEncounter() { 1666 return this.encounter != null && !this.encounter.isEmpty(); 1667 } 1668 1669 /** 1670 * @param value {@link #encounter} (The encounter when the allergy or intolerance was asserted.) 1671 */ 1672 public AllergyIntolerance setEncounter(Reference value) { 1673 this.encounter = value; 1674 return this; 1675 } 1676 1677 /** 1678 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1679 */ 1680 public DataType getOnset() { 1681 return this.onset; 1682 } 1683 1684 /** 1685 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1686 */ 1687 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1688 if (this.onset == null) 1689 this.onset = new DateTimeType(); 1690 if (!(this.onset instanceof DateTimeType)) 1691 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1692 return (DateTimeType) this.onset; 1693 } 1694 1695 public boolean hasOnsetDateTimeType() { 1696 return this != null && this.onset instanceof DateTimeType; 1697 } 1698 1699 /** 1700 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1701 */ 1702 public Age getOnsetAge() throws FHIRException { 1703 if (this.onset == null) 1704 this.onset = new Age(); 1705 if (!(this.onset instanceof Age)) 1706 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.onset.getClass().getName()+" was encountered"); 1707 return (Age) this.onset; 1708 } 1709 1710 public boolean hasOnsetAge() { 1711 return this != null && this.onset instanceof Age; 1712 } 1713 1714 /** 1715 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1716 */ 1717 public Period getOnsetPeriod() throws FHIRException { 1718 if (this.onset == null) 1719 this.onset = new Period(); 1720 if (!(this.onset instanceof Period)) 1721 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.onset.getClass().getName()+" was encountered"); 1722 return (Period) this.onset; 1723 } 1724 1725 public boolean hasOnsetPeriod() { 1726 return this != null && this.onset instanceof Period; 1727 } 1728 1729 /** 1730 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1731 */ 1732 public Range getOnsetRange() throws FHIRException { 1733 if (this.onset == null) 1734 this.onset = new Range(); 1735 if (!(this.onset instanceof Range)) 1736 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.onset.getClass().getName()+" was encountered"); 1737 return (Range) this.onset; 1738 } 1739 1740 public boolean hasOnsetRange() { 1741 return this != null && this.onset instanceof Range; 1742 } 1743 1744 /** 1745 * @return {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1746 */ 1747 public StringType getOnsetStringType() throws FHIRException { 1748 if (this.onset == null) 1749 this.onset = new StringType(); 1750 if (!(this.onset instanceof StringType)) 1751 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1752 return (StringType) this.onset; 1753 } 1754 1755 public boolean hasOnsetStringType() { 1756 return this != null && this.onset instanceof StringType; 1757 } 1758 1759 public boolean hasOnset() { 1760 return this.onset != null && !this.onset.isEmpty(); 1761 } 1762 1763 /** 1764 * @param value {@link #onset} (Estimated or actual date, date-time, or age when allergy or intolerance was identified.) 1765 */ 1766 public AllergyIntolerance setOnset(DataType value) { 1767 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period || value instanceof Range || value instanceof StringType)) 1768 throw new FHIRException("Not the right type for AllergyIntolerance.onset[x]: "+value.fhirType()); 1769 this.onset = value; 1770 return this; 1771 } 1772 1773 /** 1774 * @return {@link #recordedDate} (The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 1775 */ 1776 public DateTimeType getRecordedDateElement() { 1777 if (this.recordedDate == null) 1778 if (Configuration.errorOnAutoCreate()) 1779 throw new Error("Attempt to auto-create AllergyIntolerance.recordedDate"); 1780 else if (Configuration.doAutoCreate()) 1781 this.recordedDate = new DateTimeType(); // bb 1782 return this.recordedDate; 1783 } 1784 1785 public boolean hasRecordedDateElement() { 1786 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1787 } 1788 1789 public boolean hasRecordedDate() { 1790 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1791 } 1792 1793 /** 1794 * @param value {@link #recordedDate} (The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 1795 */ 1796 public AllergyIntolerance setRecordedDateElement(DateTimeType value) { 1797 this.recordedDate = value; 1798 return this; 1799 } 1800 1801 /** 1802 * @return The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date. 1803 */ 1804 public Date getRecordedDate() { 1805 return this.recordedDate == null ? null : this.recordedDate.getValue(); 1806 } 1807 1808 /** 1809 * @param value The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date. 1810 */ 1811 public AllergyIntolerance setRecordedDate(Date value) { 1812 if (value == null) 1813 this.recordedDate = null; 1814 else { 1815 if (this.recordedDate == null) 1816 this.recordedDate = new DateTimeType(); 1817 this.recordedDate.setValue(value); 1818 } 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #participant} (Indicates who or what participated in the activities related to the allergy or intolerance and how they were involved.) 1824 */ 1825 public List<AllergyIntoleranceParticipantComponent> getParticipant() { 1826 if (this.participant == null) 1827 this.participant = new ArrayList<AllergyIntoleranceParticipantComponent>(); 1828 return this.participant; 1829 } 1830 1831 /** 1832 * @return Returns a reference to <code>this</code> for easy method chaining 1833 */ 1834 public AllergyIntolerance setParticipant(List<AllergyIntoleranceParticipantComponent> theParticipant) { 1835 this.participant = theParticipant; 1836 return this; 1837 } 1838 1839 public boolean hasParticipant() { 1840 if (this.participant == null) 1841 return false; 1842 for (AllergyIntoleranceParticipantComponent item : this.participant) 1843 if (!item.isEmpty()) 1844 return true; 1845 return false; 1846 } 1847 1848 public AllergyIntoleranceParticipantComponent addParticipant() { //3 1849 AllergyIntoleranceParticipantComponent t = new AllergyIntoleranceParticipantComponent(); 1850 if (this.participant == null) 1851 this.participant = new ArrayList<AllergyIntoleranceParticipantComponent>(); 1852 this.participant.add(t); 1853 return t; 1854 } 1855 1856 public AllergyIntolerance addParticipant(AllergyIntoleranceParticipantComponent t) { //3 1857 if (t == null) 1858 return this; 1859 if (this.participant == null) 1860 this.participant = new ArrayList<AllergyIntoleranceParticipantComponent>(); 1861 this.participant.add(t); 1862 return this; 1863 } 1864 1865 /** 1866 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 1867 */ 1868 public AllergyIntoleranceParticipantComponent getParticipantFirstRep() { 1869 if (getParticipant().isEmpty()) { 1870 addParticipant(); 1871 } 1872 return getParticipant().get(0); 1873 } 1874 1875 /** 1876 * @return {@link #lastOccurrence} (Represents the date and/or time of the last known occurrence of a reaction event.). This is the underlying object with id, value and extensions. The accessor "getLastOccurrence" gives direct access to the value 1877 */ 1878 public DateTimeType getLastOccurrenceElement() { 1879 if (this.lastOccurrence == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create AllergyIntolerance.lastOccurrence"); 1882 else if (Configuration.doAutoCreate()) 1883 this.lastOccurrence = new DateTimeType(); // bb 1884 return this.lastOccurrence; 1885 } 1886 1887 public boolean hasLastOccurrenceElement() { 1888 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 1889 } 1890 1891 public boolean hasLastOccurrence() { 1892 return this.lastOccurrence != null && !this.lastOccurrence.isEmpty(); 1893 } 1894 1895 /** 1896 * @param value {@link #lastOccurrence} (Represents the date and/or time of the last known occurrence of a reaction event.). This is the underlying object with id, value and extensions. The accessor "getLastOccurrence" gives direct access to the value 1897 */ 1898 public AllergyIntolerance setLastOccurrenceElement(DateTimeType value) { 1899 this.lastOccurrence = value; 1900 return this; 1901 } 1902 1903 /** 1904 * @return Represents the date and/or time of the last known occurrence of a reaction event. 1905 */ 1906 public Date getLastOccurrence() { 1907 return this.lastOccurrence == null ? null : this.lastOccurrence.getValue(); 1908 } 1909 1910 /** 1911 * @param value Represents the date and/or time of the last known occurrence of a reaction event. 1912 */ 1913 public AllergyIntolerance setLastOccurrence(Date value) { 1914 if (value == null) 1915 this.lastOccurrence = null; 1916 else { 1917 if (this.lastOccurrence == null) 1918 this.lastOccurrence = new DateTimeType(); 1919 this.lastOccurrence.setValue(value); 1920 } 1921 return this; 1922 } 1923 1924 /** 1925 * @return {@link #note} (Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.) 1926 */ 1927 public List<Annotation> getNote() { 1928 if (this.note == null) 1929 this.note = new ArrayList<Annotation>(); 1930 return this.note; 1931 } 1932 1933 /** 1934 * @return Returns a reference to <code>this</code> for easy method chaining 1935 */ 1936 public AllergyIntolerance setNote(List<Annotation> theNote) { 1937 this.note = theNote; 1938 return this; 1939 } 1940 1941 public boolean hasNote() { 1942 if (this.note == null) 1943 return false; 1944 for (Annotation item : this.note) 1945 if (!item.isEmpty()) 1946 return true; 1947 return false; 1948 } 1949 1950 public Annotation addNote() { //3 1951 Annotation t = new Annotation(); 1952 if (this.note == null) 1953 this.note = new ArrayList<Annotation>(); 1954 this.note.add(t); 1955 return t; 1956 } 1957 1958 public AllergyIntolerance addNote(Annotation t) { //3 1959 if (t == null) 1960 return this; 1961 if (this.note == null) 1962 this.note = new ArrayList<Annotation>(); 1963 this.note.add(t); 1964 return this; 1965 } 1966 1967 /** 1968 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1969 */ 1970 public Annotation getNoteFirstRep() { 1971 if (getNote().isEmpty()) { 1972 addNote(); 1973 } 1974 return getNote().get(0); 1975 } 1976 1977 /** 1978 * @return {@link #reaction} (Details about each adverse reaction event linked to exposure to the identified substance.) 1979 */ 1980 public List<AllergyIntoleranceReactionComponent> getReaction() { 1981 if (this.reaction == null) 1982 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 1983 return this.reaction; 1984 } 1985 1986 /** 1987 * @return Returns a reference to <code>this</code> for easy method chaining 1988 */ 1989 public AllergyIntolerance setReaction(List<AllergyIntoleranceReactionComponent> theReaction) { 1990 this.reaction = theReaction; 1991 return this; 1992 } 1993 1994 public boolean hasReaction() { 1995 if (this.reaction == null) 1996 return false; 1997 for (AllergyIntoleranceReactionComponent item : this.reaction) 1998 if (!item.isEmpty()) 1999 return true; 2000 return false; 2001 } 2002 2003 public AllergyIntoleranceReactionComponent addReaction() { //3 2004 AllergyIntoleranceReactionComponent t = new AllergyIntoleranceReactionComponent(); 2005 if (this.reaction == null) 2006 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2007 this.reaction.add(t); 2008 return t; 2009 } 2010 2011 public AllergyIntolerance addReaction(AllergyIntoleranceReactionComponent t) { //3 2012 if (t == null) 2013 return this; 2014 if (this.reaction == null) 2015 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2016 this.reaction.add(t); 2017 return this; 2018 } 2019 2020 /** 2021 * @return The first repetition of repeating field {@link #reaction}, creating it if it does not already exist {3} 2022 */ 2023 public AllergyIntoleranceReactionComponent getReactionFirstRep() { 2024 if (getReaction().isEmpty()) { 2025 addReaction(); 2026 } 2027 return getReaction().get(0); 2028 } 2029 2030 protected void listChildren(List<Property> children) { 2031 super.listChildren(children); 2032 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2033 children.add(new Property("clinicalStatus", "CodeableConcept", "The clinical status of the allergy or intolerance.", 0, 1, clinicalStatus)); 2034 children.add(new Property("verificationStatus", "CodeableConcept", "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). The verification status pertains to the allergy or intolerance, itself, not to any specific AllergyIntolerance attribute.", 0, 1, verificationStatus)); 2035 children.add(new Property("type", "CodeableConcept", "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type)); 2036 children.add(new Property("category", "code", "Category of the identified substance.", 0, java.lang.Integer.MAX_VALUE, category)); 2037 children.add(new Property("criticality", "code", "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, criticality)); 2038 children.add(new Property("code", "CodeableConcept", "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 0, 1, code)); 2039 children.add(new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 1, patient)); 2040 children.add(new Property("encounter", "Reference(Encounter)", "The encounter when the allergy or intolerance was asserted.", 0, 1, encounter)); 2041 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset)); 2042 children.add(new Property("recordedDate", "dateTime", "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.", 0, 1, recordedDate)); 2043 children.add(new Property("participant", "", "Indicates who or what participated in the activities related to the allergy or intolerance and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant)); 2044 children.add(new Property("lastOccurrence", "dateTime", "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence)); 2045 children.add(new Property("note", "Annotation", "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note)); 2046 children.add(new Property("reaction", "", "Details about each adverse reaction event linked to exposure to the identified substance.", 0, java.lang.Integer.MAX_VALUE, reaction)); 2047 } 2048 2049 @Override 2050 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2051 switch (_hash) { 2052 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this AllergyIntolerance by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 2053 case -462853915: /*clinicalStatus*/ return new Property("clinicalStatus", "CodeableConcept", "The clinical status of the allergy or intolerance.", 0, 1, clinicalStatus); 2054 case -842509843: /*verificationStatus*/ return new Property("verificationStatus", "CodeableConcept", "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified substance (including pharmaceutical product). The verification status pertains to the allergy or intolerance, itself, not to any specific AllergyIntolerance attribute.", 0, 1, verificationStatus); 2055 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identification of the underlying physiological mechanism for the reaction risk.", 0, 1, type); 2056 case 50511102: /*category*/ return new Property("category", "code", "Category of the identified substance.", 0, java.lang.Integer.MAX_VALUE, category); 2057 case -1608054609: /*criticality*/ return new Property("criticality", "code", "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified substance.", 0, 1, criticality); 2058 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for an allergy or intolerance statement (either a positive or a negated/excluded statement). This may be a code for a substance or pharmaceutical product that is considered to be responsible for the adverse reaction risk (e.g., \"Latex\"), an allergy or intolerance condition (e.g., \"Latex allergy\"), or a negated/excluded code for a specific substance or class (e.g., \"No latex allergy\") or a general or categorical negated statement (e.g., \"No known allergy\", \"No known drug allergies\"). Note: the substance for a specific reaction may be different from the substance identified as the cause of the risk, but it must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite product that includes the identified substance. It must be clinically safe to only process the 'code' and ignore the 'reaction.substance'. If a receiving system is unable to confirm that AllergyIntolerance.reaction.substance falls within the semantic scope of AllergyIntolerance.code, then the receiving system should ignore AllergyIntolerance.reaction.substance.", 0, 1, code); 2059 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 1, patient); 2060 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter when the allergy or intolerance was asserted.", 0, 1, encounter); 2061 case -1886216323: /*onset[x]*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2062 case 105901603: /*onset*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2063 case -1701663010: /*onsetDateTime*/ return new Property("onset[x]", "dateTime", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2064 case -1886241828: /*onsetAge*/ return new Property("onset[x]", "Age", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2065 case -1545082428: /*onsetPeriod*/ return new Property("onset[x]", "Period", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2066 case -186664742: /*onsetRange*/ return new Property("onset[x]", "Range", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2067 case -1445342188: /*onsetString*/ return new Property("onset[x]", "string", "Estimated or actual date, date-time, or age when allergy or intolerance was identified.", 0, 1, onset); 2068 case -1952893826: /*recordedDate*/ return new Property("recordedDate", "dateTime", "The recordedDate represents when this particular AllergyIntolerance record was created in the system, which is often a system-generated date.", 0, 1, recordedDate); 2069 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what participated in the activities related to the allergy or intolerance and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant); 2070 case 1896977671: /*lastOccurrence*/ return new Property("lastOccurrence", "dateTime", "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 1, lastOccurrence); 2071 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, java.lang.Integer.MAX_VALUE, note); 2072 case -867509719: /*reaction*/ return new Property("reaction", "", "Details about each adverse reaction event linked to exposure to the identified substance.", 0, java.lang.Integer.MAX_VALUE, reaction); 2073 default: return super.getNamedProperty(_hash, _name, _checkValid); 2074 } 2075 2076 } 2077 2078 @Override 2079 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2080 switch (hash) { 2081 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2082 case -462853915: /*clinicalStatus*/ return this.clinicalStatus == null ? new Base[0] : new Base[] {this.clinicalStatus}; // CodeableConcept 2083 case -842509843: /*verificationStatus*/ return this.verificationStatus == null ? new Base[0] : new Base[] {this.verificationStatus}; // CodeableConcept 2084 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2085 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // Enumeration<AllergyIntoleranceCategory> 2086 case -1608054609: /*criticality*/ return this.criticality == null ? new Base[0] : new Base[] {this.criticality}; // Enumeration<AllergyIntoleranceCriticality> 2087 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2088 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2089 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2090 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // DataType 2091 case -1952893826: /*recordedDate*/ return this.recordedDate == null ? new Base[0] : new Base[] {this.recordedDate}; // DateTimeType 2092 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // AllergyIntoleranceParticipantComponent 2093 case 1896977671: /*lastOccurrence*/ return this.lastOccurrence == null ? new Base[0] : new Base[] {this.lastOccurrence}; // DateTimeType 2094 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2095 case -867509719: /*reaction*/ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // AllergyIntoleranceReactionComponent 2096 default: return super.getProperty(hash, name, checkValid); 2097 } 2098 2099 } 2100 2101 @Override 2102 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2103 switch (hash) { 2104 case -1618432855: // identifier 2105 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2106 return value; 2107 case -462853915: // clinicalStatus 2108 this.clinicalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2109 return value; 2110 case -842509843: // verificationStatus 2111 this.verificationStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2112 return value; 2113 case 3575610: // type 2114 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2115 return value; 2116 case 50511102: // category 2117 value = new AllergyIntoleranceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2118 this.getCategory().add((Enumeration) value); // Enumeration<AllergyIntoleranceCategory> 2119 return value; 2120 case -1608054609: // criticality 2121 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(TypeConvertor.castToCode(value)); 2122 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2123 return value; 2124 case 3059181: // code 2125 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2126 return value; 2127 case -791418107: // patient 2128 this.patient = TypeConvertor.castToReference(value); // Reference 2129 return value; 2130 case 1524132147: // encounter 2131 this.encounter = TypeConvertor.castToReference(value); // Reference 2132 return value; 2133 case 105901603: // onset 2134 this.onset = TypeConvertor.castToType(value); // DataType 2135 return value; 2136 case -1952893826: // recordedDate 2137 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 2138 return value; 2139 case 767422259: // participant 2140 this.getParticipant().add((AllergyIntoleranceParticipantComponent) value); // AllergyIntoleranceParticipantComponent 2141 return value; 2142 case 1896977671: // lastOccurrence 2143 this.lastOccurrence = TypeConvertor.castToDateTime(value); // DateTimeType 2144 return value; 2145 case 3387378: // note 2146 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2147 return value; 2148 case -867509719: // reaction 2149 this.getReaction().add((AllergyIntoleranceReactionComponent) value); // AllergyIntoleranceReactionComponent 2150 return value; 2151 default: return super.setProperty(hash, name, value); 2152 } 2153 2154 } 2155 2156 @Override 2157 public Base setProperty(String name, Base value) throws FHIRException { 2158 if (name.equals("identifier")) { 2159 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2160 } else if (name.equals("clinicalStatus")) { 2161 this.clinicalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2162 } else if (name.equals("verificationStatus")) { 2163 this.verificationStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2164 } else if (name.equals("type")) { 2165 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2166 } else if (name.equals("category")) { 2167 value = new AllergyIntoleranceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2168 this.getCategory().add((Enumeration) value); 2169 } else if (name.equals("criticality")) { 2170 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(TypeConvertor.castToCode(value)); 2171 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2172 } else if (name.equals("code")) { 2173 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2174 } else if (name.equals("patient")) { 2175 this.patient = TypeConvertor.castToReference(value); // Reference 2176 } else if (name.equals("encounter")) { 2177 this.encounter = TypeConvertor.castToReference(value); // Reference 2178 } else if (name.equals("onset[x]")) { 2179 this.onset = TypeConvertor.castToType(value); // DataType 2180 } else if (name.equals("recordedDate")) { 2181 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 2182 } else if (name.equals("participant")) { 2183 this.getParticipant().add((AllergyIntoleranceParticipantComponent) value); 2184 } else if (name.equals("lastOccurrence")) { 2185 this.lastOccurrence = TypeConvertor.castToDateTime(value); // DateTimeType 2186 } else if (name.equals("note")) { 2187 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2188 } else if (name.equals("reaction")) { 2189 this.getReaction().add((AllergyIntoleranceReactionComponent) value); 2190 } else 2191 return super.setProperty(name, value); 2192 return value; 2193 } 2194 2195 @Override 2196 public void removeChild(String name, Base value) throws FHIRException { 2197 if (name.equals("identifier")) { 2198 this.getIdentifier().remove(value); 2199 } else if (name.equals("clinicalStatus")) { 2200 this.clinicalStatus = null; 2201 } else if (name.equals("verificationStatus")) { 2202 this.verificationStatus = null; 2203 } else if (name.equals("type")) { 2204 this.type = null; 2205 } else if (name.equals("category")) { 2206 value = new AllergyIntoleranceCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 2207 this.getCategory().remove((Enumeration) value); 2208 } else if (name.equals("criticality")) { 2209 value = new AllergyIntoleranceCriticalityEnumFactory().fromType(TypeConvertor.castToCode(value)); 2210 this.criticality = (Enumeration) value; // Enumeration<AllergyIntoleranceCriticality> 2211 } else if (name.equals("code")) { 2212 this.code = null; 2213 } else if (name.equals("patient")) { 2214 this.patient = null; 2215 } else if (name.equals("encounter")) { 2216 this.encounter = null; 2217 } else if (name.equals("onset[x]")) { 2218 this.onset = null; 2219 } else if (name.equals("recordedDate")) { 2220 this.recordedDate = null; 2221 } else if (name.equals("participant")) { 2222 this.getParticipant().remove((AllergyIntoleranceParticipantComponent) value); 2223 } else if (name.equals("lastOccurrence")) { 2224 this.lastOccurrence = null; 2225 } else if (name.equals("note")) { 2226 this.getNote().remove(value); 2227 } else if (name.equals("reaction")) { 2228 this.getReaction().remove((AllergyIntoleranceReactionComponent) value); 2229 } else 2230 super.removeChild(name, value); 2231 2232 } 2233 2234 @Override 2235 public Base makeProperty(int hash, String name) throws FHIRException { 2236 switch (hash) { 2237 case -1618432855: return addIdentifier(); 2238 case -462853915: return getClinicalStatus(); 2239 case -842509843: return getVerificationStatus(); 2240 case 3575610: return getType(); 2241 case 50511102: return addCategoryElement(); 2242 case -1608054609: return getCriticalityElement(); 2243 case 3059181: return getCode(); 2244 case -791418107: return getPatient(); 2245 case 1524132147: return getEncounter(); 2246 case -1886216323: return getOnset(); 2247 case 105901603: return getOnset(); 2248 case -1952893826: return getRecordedDateElement(); 2249 case 767422259: return addParticipant(); 2250 case 1896977671: return getLastOccurrenceElement(); 2251 case 3387378: return addNote(); 2252 case -867509719: return addReaction(); 2253 default: return super.makeProperty(hash, name); 2254 } 2255 2256 } 2257 2258 @Override 2259 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2260 switch (hash) { 2261 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2262 case -462853915: /*clinicalStatus*/ return new String[] {"CodeableConcept"}; 2263 case -842509843: /*verificationStatus*/ return new String[] {"CodeableConcept"}; 2264 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2265 case 50511102: /*category*/ return new String[] {"code"}; 2266 case -1608054609: /*criticality*/ return new String[] {"code"}; 2267 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2268 case -791418107: /*patient*/ return new String[] {"Reference"}; 2269 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2270 case 105901603: /*onset*/ return new String[] {"dateTime", "Age", "Period", "Range", "string"}; 2271 case -1952893826: /*recordedDate*/ return new String[] {"dateTime"}; 2272 case 767422259: /*participant*/ return new String[] {}; 2273 case 1896977671: /*lastOccurrence*/ return new String[] {"dateTime"}; 2274 case 3387378: /*note*/ return new String[] {"Annotation"}; 2275 case -867509719: /*reaction*/ return new String[] {}; 2276 default: return super.getTypesForProperty(hash, name); 2277 } 2278 2279 } 2280 2281 @Override 2282 public Base addChild(String name) throws FHIRException { 2283 if (name.equals("identifier")) { 2284 return addIdentifier(); 2285 } 2286 else if (name.equals("clinicalStatus")) { 2287 this.clinicalStatus = new CodeableConcept(); 2288 return this.clinicalStatus; 2289 } 2290 else if (name.equals("verificationStatus")) { 2291 this.verificationStatus = new CodeableConcept(); 2292 return this.verificationStatus; 2293 } 2294 else if (name.equals("type")) { 2295 this.type = new CodeableConcept(); 2296 return this.type; 2297 } 2298 else if (name.equals("category")) { 2299 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.category"); 2300 } 2301 else if (name.equals("criticality")) { 2302 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.criticality"); 2303 } 2304 else if (name.equals("code")) { 2305 this.code = new CodeableConcept(); 2306 return this.code; 2307 } 2308 else if (name.equals("patient")) { 2309 this.patient = new Reference(); 2310 return this.patient; 2311 } 2312 else if (name.equals("encounter")) { 2313 this.encounter = new Reference(); 2314 return this.encounter; 2315 } 2316 else if (name.equals("onsetDateTime")) { 2317 this.onset = new DateTimeType(); 2318 return this.onset; 2319 } 2320 else if (name.equals("onsetAge")) { 2321 this.onset = new Age(); 2322 return this.onset; 2323 } 2324 else if (name.equals("onsetPeriod")) { 2325 this.onset = new Period(); 2326 return this.onset; 2327 } 2328 else if (name.equals("onsetRange")) { 2329 this.onset = new Range(); 2330 return this.onset; 2331 } 2332 else if (name.equals("onsetString")) { 2333 this.onset = new StringType(); 2334 return this.onset; 2335 } 2336 else if (name.equals("recordedDate")) { 2337 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.recordedDate"); 2338 } 2339 else if (name.equals("participant")) { 2340 return addParticipant(); 2341 } 2342 else if (name.equals("lastOccurrence")) { 2343 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.lastOccurrence"); 2344 } 2345 else if (name.equals("note")) { 2346 return addNote(); 2347 } 2348 else if (name.equals("reaction")) { 2349 return addReaction(); 2350 } 2351 else 2352 return super.addChild(name); 2353 } 2354 2355 public String fhirType() { 2356 return "AllergyIntolerance"; 2357 2358 } 2359 2360 public AllergyIntolerance copy() { 2361 AllergyIntolerance dst = new AllergyIntolerance(); 2362 copyValues(dst); 2363 return dst; 2364 } 2365 2366 public void copyValues(AllergyIntolerance dst) { 2367 super.copyValues(dst); 2368 if (identifier != null) { 2369 dst.identifier = new ArrayList<Identifier>(); 2370 for (Identifier i : identifier) 2371 dst.identifier.add(i.copy()); 2372 }; 2373 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 2374 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 2375 dst.type = type == null ? null : type.copy(); 2376 if (category != null) { 2377 dst.category = new ArrayList<Enumeration<AllergyIntoleranceCategory>>(); 2378 for (Enumeration<AllergyIntoleranceCategory> i : category) 2379 dst.category.add(i.copy()); 2380 }; 2381 dst.criticality = criticality == null ? null : criticality.copy(); 2382 dst.code = code == null ? null : code.copy(); 2383 dst.patient = patient == null ? null : patient.copy(); 2384 dst.encounter = encounter == null ? null : encounter.copy(); 2385 dst.onset = onset == null ? null : onset.copy(); 2386 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2387 if (participant != null) { 2388 dst.participant = new ArrayList<AllergyIntoleranceParticipantComponent>(); 2389 for (AllergyIntoleranceParticipantComponent i : participant) 2390 dst.participant.add(i.copy()); 2391 }; 2392 dst.lastOccurrence = lastOccurrence == null ? null : lastOccurrence.copy(); 2393 if (note != null) { 2394 dst.note = new ArrayList<Annotation>(); 2395 for (Annotation i : note) 2396 dst.note.add(i.copy()); 2397 }; 2398 if (reaction != null) { 2399 dst.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2400 for (AllergyIntoleranceReactionComponent i : reaction) 2401 dst.reaction.add(i.copy()); 2402 }; 2403 } 2404 2405 protected AllergyIntolerance typedCopy() { 2406 return copy(); 2407 } 2408 2409 @Override 2410 public boolean equalsDeep(Base other_) { 2411 if (!super.equalsDeep(other_)) 2412 return false; 2413 if (!(other_ instanceof AllergyIntolerance)) 2414 return false; 2415 AllergyIntolerance o = (AllergyIntolerance) other_; 2416 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 2417 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(type, o.type, true) 2418 && compareDeep(category, o.category, true) && compareDeep(criticality, o.criticality, true) && compareDeep(code, o.code, true) 2419 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) && compareDeep(onset, o.onset, true) 2420 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(participant, o.participant, true) 2421 && compareDeep(lastOccurrence, o.lastOccurrence, true) && compareDeep(note, o.note, true) && compareDeep(reaction, o.reaction, true) 2422 ; 2423 } 2424 2425 @Override 2426 public boolean equalsShallow(Base other_) { 2427 if (!super.equalsShallow(other_)) 2428 return false; 2429 if (!(other_ instanceof AllergyIntolerance)) 2430 return false; 2431 AllergyIntolerance o = (AllergyIntolerance) other_; 2432 return compareValues(category, o.category, true) && compareValues(criticality, o.criticality, true) 2433 && compareValues(recordedDate, o.recordedDate, true) && compareValues(lastOccurrence, o.lastOccurrence, true) 2434 ; 2435 } 2436 2437 public boolean isEmpty() { 2438 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus 2439 , verificationStatus, type, category, criticality, code, patient, encounter, onset 2440 , recordedDate, participant, lastOccurrence, note, reaction); 2441 } 2442 2443 @Override 2444 public ResourceType getResourceType() { 2445 return ResourceType.AllergyIntolerance; 2446 } 2447 2448 /** 2449 * Search parameter: <b>category</b> 2450 * <p> 2451 * Description: <b>food | medication | environment | biologic</b><br> 2452 * Type: <b>token</b><br> 2453 * Path: <b>AllergyIntolerance.category</b><br> 2454 * </p> 2455 */ 2456 @SearchParamDefinition(name="category", path="AllergyIntolerance.category", description="food | medication | environment | biologic", type="token" ) 2457 public static final String SP_CATEGORY = "category"; 2458 /** 2459 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2460 * <p> 2461 * Description: <b>food | medication | environment | biologic</b><br> 2462 * Type: <b>token</b><br> 2463 * Path: <b>AllergyIntolerance.category</b><br> 2464 * </p> 2465 */ 2466 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2467 2468 /** 2469 * Search parameter: <b>clinical-status</b> 2470 * <p> 2471 * Description: <b>active | inactive | resolved</b><br> 2472 * Type: <b>token</b><br> 2473 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 2474 * </p> 2475 */ 2476 @SearchParamDefinition(name="clinical-status", path="AllergyIntolerance.clinicalStatus", description="active | inactive | resolved", type="token" ) 2477 public static final String SP_CLINICAL_STATUS = "clinical-status"; 2478 /** 2479 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 2480 * <p> 2481 * Description: <b>active | inactive | resolved</b><br> 2482 * Type: <b>token</b><br> 2483 * Path: <b>AllergyIntolerance.clinicalStatus</b><br> 2484 * </p> 2485 */ 2486 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLINICAL_STATUS); 2487 2488 /** 2489 * Search parameter: <b>criticality</b> 2490 * <p> 2491 * Description: <b>low | high | unable-to-assess</b><br> 2492 * Type: <b>token</b><br> 2493 * Path: <b>AllergyIntolerance.criticality</b><br> 2494 * </p> 2495 */ 2496 @SearchParamDefinition(name="criticality", path="AllergyIntolerance.criticality", description="low | high | unable-to-assess", type="token" ) 2497 public static final String SP_CRITICALITY = "criticality"; 2498 /** 2499 * <b>Fluent Client</b> search parameter constant for <b>criticality</b> 2500 * <p> 2501 * Description: <b>low | high | unable-to-assess</b><br> 2502 * Type: <b>token</b><br> 2503 * Path: <b>AllergyIntolerance.criticality</b><br> 2504 * </p> 2505 */ 2506 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CRITICALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CRITICALITY); 2507 2508 /** 2509 * Search parameter: <b>last-date</b> 2510 * <p> 2511 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 2512 * Type: <b>date</b><br> 2513 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 2514 * </p> 2515 */ 2516 @SearchParamDefinition(name="last-date", path="AllergyIntolerance.lastOccurrence", description="Date(/time) of last known occurrence of a reaction", type="date" ) 2517 public static final String SP_LAST_DATE = "last-date"; 2518 /** 2519 * <b>Fluent Client</b> search parameter constant for <b>last-date</b> 2520 * <p> 2521 * Description: <b>Date(/time) of last known occurrence of a reaction</b><br> 2522 * Type: <b>date</b><br> 2523 * Path: <b>AllergyIntolerance.lastOccurrence</b><br> 2524 * </p> 2525 */ 2526 public static final ca.uhn.fhir.rest.gclient.DateClientParam LAST_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_LAST_DATE); 2527 2528 /** 2529 * Search parameter: <b>manifestation-code</b> 2530 * <p> 2531 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2532 * Type: <b>token</b><br> 2533 * Path: <b>AllergyIntolerance.reaction.manifestation.concept</b><br> 2534 * </p> 2535 */ 2536 @SearchParamDefinition(name="manifestation-code", path="AllergyIntolerance.reaction.manifestation.concept", description="Clinical symptoms/signs associated with the Event", type="token" ) 2537 public static final String SP_MANIFESTATION_CODE = "manifestation-code"; 2538 /** 2539 * <b>Fluent Client</b> search parameter constant for <b>manifestation-code</b> 2540 * <p> 2541 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2542 * Type: <b>token</b><br> 2543 * Path: <b>AllergyIntolerance.reaction.manifestation.concept</b><br> 2544 * </p> 2545 */ 2546 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MANIFESTATION_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MANIFESTATION_CODE); 2547 2548 /** 2549 * Search parameter: <b>manifestation-reference</b> 2550 * <p> 2551 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2552 * Type: <b>reference</b><br> 2553 * Path: <b>AllergyIntolerance.reaction.manifestation.reference</b><br> 2554 * </p> 2555 */ 2556 @SearchParamDefinition(name="manifestation-reference", path="AllergyIntolerance.reaction.manifestation.reference", description="Clinical symptoms/signs associated with the Event", type="reference", target={Observation.class } ) 2557 public static final String SP_MANIFESTATION_REFERENCE = "manifestation-reference"; 2558 /** 2559 * <b>Fluent Client</b> search parameter constant for <b>manifestation-reference</b> 2560 * <p> 2561 * Description: <b>Clinical symptoms/signs associated with the Event</b><br> 2562 * Type: <b>reference</b><br> 2563 * Path: <b>AllergyIntolerance.reaction.manifestation.reference</b><br> 2564 * </p> 2565 */ 2566 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANIFESTATION_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANIFESTATION_REFERENCE); 2567 2568/** 2569 * Constant for fluent queries to be used to add include statements. Specifies 2570 * the path value of "<b>AllergyIntolerance:manifestation-reference</b>". 2571 */ 2572 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANIFESTATION_REFERENCE = new ca.uhn.fhir.model.api.Include("AllergyIntolerance:manifestation-reference").toLocked(); 2573 2574 /** 2575 * Search parameter: <b>participant</b> 2576 * <p> 2577 * Description: <b>Who or what participated in the activities related to the allergy or intolerance</b><br> 2578 * Type: <b>reference</b><br> 2579 * Path: <b>AllergyIntolerance.participant.actor</b><br> 2580 * </p> 2581 */ 2582 @SearchParamDefinition(name="participant", path="AllergyIntolerance.participant.actor", description="Who or what participated in the activities related to the allergy or intolerance", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2583 public static final String SP_PARTICIPANT = "participant"; 2584 /** 2585 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 2586 * <p> 2587 * Description: <b>Who or what participated in the activities related to the allergy or intolerance</b><br> 2588 * Type: <b>reference</b><br> 2589 * Path: <b>AllergyIntolerance.participant.actor</b><br> 2590 * </p> 2591 */ 2592 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 2593 2594/** 2595 * Constant for fluent queries to be used to add include statements. Specifies 2596 * the path value of "<b>AllergyIntolerance:participant</b>". 2597 */ 2598 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("AllergyIntolerance:participant").toLocked(); 2599 2600 /** 2601 * Search parameter: <b>route</b> 2602 * <p> 2603 * Description: <b>How the subject was exposed to the substance</b><br> 2604 * Type: <b>token</b><br> 2605 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 2606 * </p> 2607 */ 2608 @SearchParamDefinition(name="route", path="AllergyIntolerance.reaction.exposureRoute", description="How the subject was exposed to the substance", type="token" ) 2609 public static final String SP_ROUTE = "route"; 2610 /** 2611 * <b>Fluent Client</b> search parameter constant for <b>route</b> 2612 * <p> 2613 * Description: <b>How the subject was exposed to the substance</b><br> 2614 * Type: <b>token</b><br> 2615 * Path: <b>AllergyIntolerance.reaction.exposureRoute</b><br> 2616 * </p> 2617 */ 2618 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROUTE); 2619 2620 /** 2621 * Search parameter: <b>severity</b> 2622 * <p> 2623 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2624 * Type: <b>token</b><br> 2625 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2626 * </p> 2627 */ 2628 @SearchParamDefinition(name="severity", path="AllergyIntolerance.reaction.severity", description="mild | moderate | severe (of event as a whole)", type="token" ) 2629 public static final String SP_SEVERITY = "severity"; 2630 /** 2631 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2632 * <p> 2633 * Description: <b>mild | moderate | severe (of event as a whole)</b><br> 2634 * Type: <b>token</b><br> 2635 * Path: <b>AllergyIntolerance.reaction.severity</b><br> 2636 * </p> 2637 */ 2638 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SEVERITY); 2639 2640 /** 2641 * Search parameter: <b>verification-status</b> 2642 * <p> 2643 * Description: <b>unconfirmed | presumed | confirmed | refuted | entered-in-error</b><br> 2644 * Type: <b>token</b><br> 2645 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 2646 * </p> 2647 */ 2648 @SearchParamDefinition(name="verification-status", path="AllergyIntolerance.verificationStatus", description="unconfirmed | presumed | confirmed | refuted | entered-in-error", type="token" ) 2649 public static final String SP_VERIFICATION_STATUS = "verification-status"; 2650 /** 2651 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 2652 * <p> 2653 * Description: <b>unconfirmed | presumed | confirmed | refuted | entered-in-error</b><br> 2654 * Type: <b>token</b><br> 2655 * Path: <b>AllergyIntolerance.verificationStatus</b><br> 2656 * </p> 2657 */ 2658 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERIFICATION_STATUS); 2659 2660 /** 2661 * Search parameter: <b>code</b> 2662 * <p> 2663 * Description: <b>Multiple Resources: 2664 2665* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2666* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2667* [AuditEvent](auditevent.html): More specific code for the event 2668* [Basic](basic.html): Kind of Resource 2669* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2670* [Condition](condition.html): Code for the condition 2671* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2672* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2673* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2674* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2675* [ImagingSelection](imagingselection.html): The imaging selection status 2676* [List](list.html): What the purpose of this list is 2677* [Medication](medication.html): Returns medications for a specific code 2678* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2679* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2680* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2681* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2682* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2683* [Observation](observation.html): The code of the observation type 2684* [Procedure](procedure.html): A code to identify a procedure 2685* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2686* [Task](task.html): Search by task code 2687</b><br> 2688 * Type: <b>token</b><br> 2689 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2690 * </p> 2691 */ 2692 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2693 public static final String SP_CODE = "code"; 2694 /** 2695 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2696 * <p> 2697 * Description: <b>Multiple Resources: 2698 2699* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2700* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2701* [AuditEvent](auditevent.html): More specific code for the event 2702* [Basic](basic.html): Kind of Resource 2703* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2704* [Condition](condition.html): Code for the condition 2705* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2706* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2707* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2708* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2709* [ImagingSelection](imagingselection.html): The imaging selection status 2710* [List](list.html): What the purpose of this list is 2711* [Medication](medication.html): Returns medications for a specific code 2712* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2713* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2714* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2715* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2716* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2717* [Observation](observation.html): The code of the observation type 2718* [Procedure](procedure.html): A code to identify a procedure 2719* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2720* [Task](task.html): Search by task code 2721</b><br> 2722 * Type: <b>token</b><br> 2723 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2724 * </p> 2725 */ 2726 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2727 2728 /** 2729 * Search parameter: <b>date</b> 2730 * <p> 2731 * Description: <b>Multiple Resources: 2732 2733* [AdverseEvent](adverseevent.html): When the event occurred 2734* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2735* [Appointment](appointment.html): Appointment date/time. 2736* [AuditEvent](auditevent.html): Time when the event was recorded 2737* [CarePlan](careplan.html): Time period plan covers 2738* [CareTeam](careteam.html): A date within the coverage time period. 2739* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2740* [Composition](composition.html): Composition editing time 2741* [Consent](consent.html): When consent was agreed to 2742* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2743* [DocumentReference](documentreference.html): When this document reference was created 2744* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2745* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2746* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2747* [Flag](flag.html): Time period when flag is active 2748* [Immunization](immunization.html): Vaccination (non)-Administration Date 2749* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2750* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2751* [Invoice](invoice.html): Invoice date / posting date 2752* [List](list.html): When the list was prepared 2753* [MeasureReport](measurereport.html): The date of the measure report 2754* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2755* [Observation](observation.html): Clinically relevant time/time-period for observation 2756* [Procedure](procedure.html): When the procedure occurred or is occurring 2757* [ResearchSubject](researchsubject.html): Start and end of participation 2758* [RiskAssessment](riskassessment.html): When was assessment made? 2759* [SupplyRequest](supplyrequest.html): When the request was made 2760</b><br> 2761 * Type: <b>date</b><br> 2762 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2763 * </p> 2764 */ 2765 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2766 public static final String SP_DATE = "date"; 2767 /** 2768 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2769 * <p> 2770 * Description: <b>Multiple Resources: 2771 2772* [AdverseEvent](adverseevent.html): When the event occurred 2773* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2774* [Appointment](appointment.html): Appointment date/time. 2775* [AuditEvent](auditevent.html): Time when the event was recorded 2776* [CarePlan](careplan.html): Time period plan covers 2777* [CareTeam](careteam.html): A date within the coverage time period. 2778* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2779* [Composition](composition.html): Composition editing time 2780* [Consent](consent.html): When consent was agreed to 2781* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2782* [DocumentReference](documentreference.html): When this document reference was created 2783* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2784* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2785* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2786* [Flag](flag.html): Time period when flag is active 2787* [Immunization](immunization.html): Vaccination (non)-Administration Date 2788* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2789* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2790* [Invoice](invoice.html): Invoice date / posting date 2791* [List](list.html): When the list was prepared 2792* [MeasureReport](measurereport.html): The date of the measure report 2793* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2794* [Observation](observation.html): Clinically relevant time/time-period for observation 2795* [Procedure](procedure.html): When the procedure occurred or is occurring 2796* [ResearchSubject](researchsubject.html): Start and end of participation 2797* [RiskAssessment](riskassessment.html): When was assessment made? 2798* [SupplyRequest](supplyrequest.html): When the request was made 2799</b><br> 2800 * Type: <b>date</b><br> 2801 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2802 * </p> 2803 */ 2804 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2805 2806 /** 2807 * Search parameter: <b>identifier</b> 2808 * <p> 2809 * Description: <b>Multiple Resources: 2810 2811* [Account](account.html): Account number 2812* [AdverseEvent](adverseevent.html): Business identifier for the event 2813* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2814* [Appointment](appointment.html): An Identifier of the Appointment 2815* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2816* [Basic](basic.html): Business identifier 2817* [BodyStructure](bodystructure.html): Bodystructure identifier 2818* [CarePlan](careplan.html): External Ids for this plan 2819* [CareTeam](careteam.html): External Ids for this team 2820* [ChargeItem](chargeitem.html): Business Identifier for item 2821* [Claim](claim.html): The primary identifier of the financial resource 2822* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2823* [ClinicalImpression](clinicalimpression.html): Business identifier 2824* [Communication](communication.html): Unique identifier 2825* [CommunicationRequest](communicationrequest.html): Unique identifier 2826* [Composition](composition.html): Version-independent identifier for the Composition 2827* [Condition](condition.html): A unique identifier of the condition record 2828* [Consent](consent.html): Identifier for this record (external references) 2829* [Contract](contract.html): The identity of the contract 2830* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2831* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2832* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2833* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2834* [DeviceRequest](devicerequest.html): Business identifier for request/order 2835* [DeviceUsage](deviceusage.html): Search by identifier 2836* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2837* [DocumentReference](documentreference.html): Identifier of the attachment binary 2838* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2839* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2840* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2841* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2842* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2843* [Flag](flag.html): Business identifier 2844* [Goal](goal.html): External Ids for this goal 2845* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2846* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2847* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2848* [Immunization](immunization.html): Business identifier 2849* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2850* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2851* [Invoice](invoice.html): Business Identifier for item 2852* [List](list.html): Business identifier 2853* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2854* [Medication](medication.html): Returns medications with this external identifier 2855* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2856* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2857* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2858* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2859* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2860* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2861* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2862* [Observation](observation.html): The unique id for a particular observation 2863* [Person](person.html): A person Identifier 2864* [Procedure](procedure.html): A unique identifier for a procedure 2865* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2866* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2867* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2868* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2869* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2870* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2871* [Specimen](specimen.html): The unique identifier associated with the specimen 2872* [SupplyDelivery](supplydelivery.html): External identifier 2873* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2874* [Task](task.html): Search for a task instance by its business identifier 2875* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2876</b><br> 2877 * Type: <b>token</b><br> 2878 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2879 * </p> 2880 */ 2881 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2882 public static final String SP_IDENTIFIER = "identifier"; 2883 /** 2884 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2885 * <p> 2886 * Description: <b>Multiple Resources: 2887 2888* [Account](account.html): Account number 2889* [AdverseEvent](adverseevent.html): Business identifier for the event 2890* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2891* [Appointment](appointment.html): An Identifier of the Appointment 2892* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2893* [Basic](basic.html): Business identifier 2894* [BodyStructure](bodystructure.html): Bodystructure identifier 2895* [CarePlan](careplan.html): External Ids for this plan 2896* [CareTeam](careteam.html): External Ids for this team 2897* [ChargeItem](chargeitem.html): Business Identifier for item 2898* [Claim](claim.html): The primary identifier of the financial resource 2899* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2900* [ClinicalImpression](clinicalimpression.html): Business identifier 2901* [Communication](communication.html): Unique identifier 2902* [CommunicationRequest](communicationrequest.html): Unique identifier 2903* [Composition](composition.html): Version-independent identifier for the Composition 2904* [Condition](condition.html): A unique identifier of the condition record 2905* [Consent](consent.html): Identifier for this record (external references) 2906* [Contract](contract.html): The identity of the contract 2907* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2908* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2909* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2910* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2911* [DeviceRequest](devicerequest.html): Business identifier for request/order 2912* [DeviceUsage](deviceusage.html): Search by identifier 2913* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2914* [DocumentReference](documentreference.html): Identifier of the attachment binary 2915* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2916* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2917* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2918* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2919* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2920* [Flag](flag.html): Business identifier 2921* [Goal](goal.html): External Ids for this goal 2922* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2923* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2924* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2925* [Immunization](immunization.html): Business identifier 2926* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2927* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2928* [Invoice](invoice.html): Business Identifier for item 2929* [List](list.html): Business identifier 2930* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2931* [Medication](medication.html): Returns medications with this external identifier 2932* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2933* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2934* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2935* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2936* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2937* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2938* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2939* [Observation](observation.html): The unique id for a particular observation 2940* [Person](person.html): A person Identifier 2941* [Procedure](procedure.html): A unique identifier for a procedure 2942* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2943* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2944* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2945* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2946* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2947* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2948* [Specimen](specimen.html): The unique identifier associated with the specimen 2949* [SupplyDelivery](supplydelivery.html): External identifier 2950* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2951* [Task](task.html): Search for a task instance by its business identifier 2952* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2953</b><br> 2954 * Type: <b>token</b><br> 2955 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2956 * </p> 2957 */ 2958 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2959 2960 /** 2961 * Search parameter: <b>patient</b> 2962 * <p> 2963 * Description: <b>Multiple Resources: 2964 2965* [Account](account.html): The entity that caused the expenses 2966* [AdverseEvent](adverseevent.html): Subject impacted by event 2967* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2968* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2969* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2970* [AuditEvent](auditevent.html): Where the activity involved patient data 2971* [Basic](basic.html): Identifies the focus of this resource 2972* [BodyStructure](bodystructure.html): Who this is about 2973* [CarePlan](careplan.html): Who the care plan is for 2974* [CareTeam](careteam.html): Who care team is for 2975* [ChargeItem](chargeitem.html): Individual service was done for/to 2976* [Claim](claim.html): Patient receiving the products or services 2977* [ClaimResponse](claimresponse.html): The subject of care 2978* [ClinicalImpression](clinicalimpression.html): Patient assessed 2979* [Communication](communication.html): Focus of message 2980* [CommunicationRequest](communicationrequest.html): Focus of message 2981* [Composition](composition.html): Who and/or what the composition is about 2982* [Condition](condition.html): Who has the condition? 2983* [Consent](consent.html): Who the consent applies to 2984* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2985* [Coverage](coverage.html): Retrieve coverages for a patient 2986* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2987* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2988* [DetectedIssue](detectedissue.html): Associated patient 2989* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2990* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2991* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2992* [DocumentReference](documentreference.html): Who/what is the subject of the document 2993* [Encounter](encounter.html): The patient present at the encounter 2994* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2995* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2996* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2997* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2998* [Flag](flag.html): The identity of a subject to list flags for 2999* [Goal](goal.html): Who this goal is intended for 3000* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3001* [ImagingSelection](imagingselection.html): Who the study is about 3002* [ImagingStudy](imagingstudy.html): Who the study is about 3003* [Immunization](immunization.html): The patient for the vaccination record 3004* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3005* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3006* [Invoice](invoice.html): Recipient(s) of goods and services 3007* [List](list.html): If all resources have the same subject 3008* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3009* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3010* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3011* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3012* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3013* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3014* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3015* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3016* [Observation](observation.html): The subject that the observation is about (if patient) 3017* [Person](person.html): The Person links to this Patient 3018* [Procedure](procedure.html): Search by subject - a patient 3019* [Provenance](provenance.html): Where the activity involved patient data 3020* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3021* [RelatedPerson](relatedperson.html): The patient this related person is related to 3022* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3023* [ResearchSubject](researchsubject.html): Who or what is part of study 3024* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3025* [ServiceRequest](servicerequest.html): Search by subject - a patient 3026* [Specimen](specimen.html): The patient the specimen comes from 3027* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3028* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3029* [Task](task.html): Search by patient 3030* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3031</b><br> 3032 * Type: <b>reference</b><br> 3033 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3034 * </p> 3035 */ 3036 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3037 public static final String SP_PATIENT = "patient"; 3038 /** 3039 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3040 * <p> 3041 * Description: <b>Multiple Resources: 3042 3043* [Account](account.html): The entity that caused the expenses 3044* [AdverseEvent](adverseevent.html): Subject impacted by event 3045* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3046* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3047* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3048* [AuditEvent](auditevent.html): Where the activity involved patient data 3049* [Basic](basic.html): Identifies the focus of this resource 3050* [BodyStructure](bodystructure.html): Who this is about 3051* [CarePlan](careplan.html): Who the care plan is for 3052* [CareTeam](careteam.html): Who care team is for 3053* [ChargeItem](chargeitem.html): Individual service was done for/to 3054* [Claim](claim.html): Patient receiving the products or services 3055* [ClaimResponse](claimresponse.html): The subject of care 3056* [ClinicalImpression](clinicalimpression.html): Patient assessed 3057* [Communication](communication.html): Focus of message 3058* [CommunicationRequest](communicationrequest.html): Focus of message 3059* [Composition](composition.html): Who and/or what the composition is about 3060* [Condition](condition.html): Who has the condition? 3061* [Consent](consent.html): Who the consent applies to 3062* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3063* [Coverage](coverage.html): Retrieve coverages for a patient 3064* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3065* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3066* [DetectedIssue](detectedissue.html): Associated patient 3067* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3068* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3069* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3070* [DocumentReference](documentreference.html): Who/what is the subject of the document 3071* [Encounter](encounter.html): The patient present at the encounter 3072* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3073* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3074* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3075* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3076* [Flag](flag.html): The identity of a subject to list flags for 3077* [Goal](goal.html): Who this goal is intended for 3078* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3079* [ImagingSelection](imagingselection.html): Who the study is about 3080* [ImagingStudy](imagingstudy.html): Who the study is about 3081* [Immunization](immunization.html): The patient for the vaccination record 3082* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3083* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3084* [Invoice](invoice.html): Recipient(s) of goods and services 3085* [List](list.html): If all resources have the same subject 3086* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3087* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3088* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3089* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3090* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3091* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3092* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3093* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3094* [Observation](observation.html): The subject that the observation is about (if patient) 3095* [Person](person.html): The Person links to this Patient 3096* [Procedure](procedure.html): Search by subject - a patient 3097* [Provenance](provenance.html): Where the activity involved patient data 3098* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3099* [RelatedPerson](relatedperson.html): The patient this related person is related to 3100* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3101* [ResearchSubject](researchsubject.html): Who or what is part of study 3102* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3103* [ServiceRequest](servicerequest.html): Search by subject - a patient 3104* [Specimen](specimen.html): The patient the specimen comes from 3105* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3106* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3107* [Task](task.html): Search by patient 3108* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3109</b><br> 3110 * Type: <b>reference</b><br> 3111 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3112 * </p> 3113 */ 3114 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3115 3116/** 3117 * Constant for fluent queries to be used to add include statements. Specifies 3118 * the path value of "<b>AllergyIntolerance:patient</b>". 3119 */ 3120 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AllergyIntolerance:patient").toLocked(); 3121 3122 /** 3123 * Search parameter: <b>type</b> 3124 * <p> 3125 * Description: <b>Multiple Resources: 3126 3127* [Account](account.html): E.g. patient, expense, depreciation 3128* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3129* [Composition](composition.html): Kind of composition (LOINC if possible) 3130* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3131* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3132* [Encounter](encounter.html): Specific type of encounter 3133* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3134* [Invoice](invoice.html): Type of Invoice 3135* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3136* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3137* [Specimen](specimen.html): The specimen type 3138</b><br> 3139 * Type: <b>token</b><br> 3140 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3141 * </p> 3142 */ 3143 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 3144 public static final String SP_TYPE = "type"; 3145 /** 3146 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3147 * <p> 3148 * Description: <b>Multiple Resources: 3149 3150* [Account](account.html): E.g. patient, expense, depreciation 3151* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3152* [Composition](composition.html): Kind of composition (LOINC if possible) 3153* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3154* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3155* [Encounter](encounter.html): Specific type of encounter 3156* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3157* [Invoice](invoice.html): Type of Invoice 3158* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3159* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3160* [Specimen](specimen.html): The specimen type 3161</b><br> 3162 * Type: <b>token</b><br> 3163 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3164 * </p> 3165 */ 3166 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3167 3168 3169} 3170