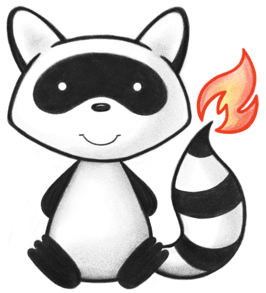
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * Annotation Type: A text note which also contains information about who made the statement and when. 049 */ 050@DatatypeDef(name="Annotation") 051public class Annotation extends DataType implements ICompositeType { 052 053 /** 054 * The individual responsible for making the annotation. 055 */ 056 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Organization.class, StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="Individual responsible for the annotation", formalDefinition="The individual responsible for making the annotation." ) 058 protected DataType author; 059 060 /** 061 * Indicates when this particular annotation was made. 062 */ 063 @Child(name = "time", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="When the annotation was made", formalDefinition="Indicates when this particular annotation was made." ) 065 protected DateTimeType time; 066 067 /** 068 * The text of the annotation in markdown format. 069 */ 070 @Child(name = "text", type = {MarkdownType.class}, order=2, min=1, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="The annotation - text content (as markdown)", formalDefinition="The text of the annotation in markdown format." ) 072 protected MarkdownType text; 073 074 private static final long serialVersionUID = 1108562171L; 075 076 /** 077 * Constructor 078 */ 079 public Annotation() { 080 super(); 081 } 082 083 /** 084 * Constructor 085 */ 086 public Annotation(String text) { 087 super(); 088 this.setText(text); 089 } 090 091 /** 092 * @return {@link #author} (The individual responsible for making the annotation.) 093 */ 094 public DataType getAuthor() { 095 return this.author; 096 } 097 098 /** 099 * @return {@link #author} (The individual responsible for making the annotation.) 100 */ 101 public Reference getAuthorReference() throws FHIRException { 102 if (this.author == null) 103 this.author = new Reference(); 104 if (!(this.author instanceof Reference)) 105 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.author.getClass().getName()+" was encountered"); 106 return (Reference) this.author; 107 } 108 109 public boolean hasAuthorReference() { 110 return this != null && this.author instanceof Reference; 111 } 112 113 /** 114 * @return {@link #author} (The individual responsible for making the annotation.) 115 */ 116 public StringType getAuthorStringType() throws FHIRException { 117 if (this.author == null) 118 this.author = new StringType(); 119 if (!(this.author instanceof StringType)) 120 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.author.getClass().getName()+" was encountered"); 121 return (StringType) this.author; 122 } 123 124 public boolean hasAuthorStringType() { 125 return this != null && this.author instanceof StringType; 126 } 127 128 public boolean hasAuthor() { 129 return this.author != null && !this.author.isEmpty(); 130 } 131 132 /** 133 * @param value {@link #author} (The individual responsible for making the annotation.) 134 */ 135 public Annotation setAuthor(DataType value) { 136 if (value != null && !(value instanceof Reference || value instanceof StringType)) 137 throw new FHIRException("Not the right type for Annotation.author[x]: "+value.fhirType()); 138 this.author = value; 139 return this; 140 } 141 142 /** 143 * @return {@link #time} (Indicates when this particular annotation was made.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 144 */ 145 public DateTimeType getTimeElement() { 146 if (this.time == null) 147 if (Configuration.errorOnAutoCreate()) 148 throw new Error("Attempt to auto-create Annotation.time"); 149 else if (Configuration.doAutoCreate()) 150 this.time = new DateTimeType(); // bb 151 return this.time; 152 } 153 154 public boolean hasTimeElement() { 155 return this.time != null && !this.time.isEmpty(); 156 } 157 158 public boolean hasTime() { 159 return this.time != null && !this.time.isEmpty(); 160 } 161 162 /** 163 * @param value {@link #time} (Indicates when this particular annotation was made.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 164 */ 165 public Annotation setTimeElement(DateTimeType value) { 166 this.time = value; 167 return this; 168 } 169 170 /** 171 * @return Indicates when this particular annotation was made. 172 */ 173 public Date getTime() { 174 return this.time == null ? null : this.time.getValue(); 175 } 176 177 /** 178 * @param value Indicates when this particular annotation was made. 179 */ 180 public Annotation setTime(Date value) { 181 if (value == null) 182 this.time = null; 183 else { 184 if (this.time == null) 185 this.time = new DateTimeType(); 186 this.time.setValue(value); 187 } 188 return this; 189 } 190 191 /** 192 * @return {@link #text} (The text of the annotation in markdown format.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 193 */ 194 public MarkdownType getTextElement() { 195 if (this.text == null) 196 if (Configuration.errorOnAutoCreate()) 197 throw new Error("Attempt to auto-create Annotation.text"); 198 else if (Configuration.doAutoCreate()) 199 this.text = new MarkdownType(); // bb 200 return this.text; 201 } 202 203 public boolean hasTextElement() { 204 return this.text != null && !this.text.isEmpty(); 205 } 206 207 public boolean hasText() { 208 return this.text != null && !this.text.isEmpty(); 209 } 210 211 /** 212 * @param value {@link #text} (The text of the annotation in markdown format.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 213 */ 214 public Annotation setTextElement(MarkdownType value) { 215 this.text = value; 216 return this; 217 } 218 219 /** 220 * @return The text of the annotation in markdown format. 221 */ 222 public String getText() { 223 return this.text == null ? null : this.text.getValue(); 224 } 225 226 /** 227 * @param value The text of the annotation in markdown format. 228 */ 229 public Annotation setText(String value) { 230 if (this.text == null) 231 this.text = new MarkdownType(); 232 this.text.setValue(value); 233 return this; 234 } 235 236 protected void listChildren(List<Property> children) { 237 super.listChildren(children); 238 children.add(new Property("author[x]", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)|string", "The individual responsible for making the annotation.", 0, 1, author)); 239 children.add(new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 1, time)); 240 children.add(new Property("text", "markdown", "The text of the annotation in markdown format.", 0, 1, text)); 241 } 242 243 @Override 244 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 245 switch (_hash) { 246 case 1475597077: /*author[x]*/ return new Property("author[x]", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)|string", "The individual responsible for making the annotation.", 0, 1, author); 247 case -1406328437: /*author*/ return new Property("author[x]", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)|string", "The individual responsible for making the annotation.", 0, 1, author); 248 case 305515008: /*authorReference*/ return new Property("author[x]", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", "The individual responsible for making the annotation.", 0, 1, author); 249 case 290249084: /*authorString*/ return new Property("author[x]", "string", "The individual responsible for making the annotation.", 0, 1, author); 250 case 3560141: /*time*/ return new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 1, time); 251 case 3556653: /*text*/ return new Property("text", "markdown", "The text of the annotation in markdown format.", 0, 1, text); 252 default: return super.getNamedProperty(_hash, _name, _checkValid); 253 } 254 255 } 256 257 @Override 258 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 259 switch (hash) { 260 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // DataType 261 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DateTimeType 262 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // MarkdownType 263 default: return super.getProperty(hash, name, checkValid); 264 } 265 266 } 267 268 @Override 269 public Base setProperty(int hash, String name, Base value) throws FHIRException { 270 switch (hash) { 271 case -1406328437: // author 272 this.author = TypeConvertor.castToType(value); // DataType 273 return value; 274 case 3560141: // time 275 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 276 return value; 277 case 3556653: // text 278 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 279 return value; 280 default: return super.setProperty(hash, name, value); 281 } 282 283 } 284 285 @Override 286 public Base setProperty(String name, Base value) throws FHIRException { 287 if (name.equals("author[x]")) { 288 this.author = TypeConvertor.castToType(value); // DataType 289 } else if (name.equals("time")) { 290 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 291 } else if (name.equals("text")) { 292 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 293 } else 294 return super.setProperty(name, value); 295 return value; 296 } 297 298 @Override 299 public void removeChild(String name, Base value) throws FHIRException { 300 if (name.equals("author[x]")) { 301 this.author = null; 302 } else if (name.equals("time")) { 303 this.time = null; 304 } else if (name.equals("text")) { 305 this.text = null; 306 } else 307 super.removeChild(name, value); 308 309 } 310 311 @Override 312 public Base makeProperty(int hash, String name) throws FHIRException { 313 switch (hash) { 314 case 1475597077: return getAuthor(); 315 case -1406328437: return getAuthor(); 316 case 3560141: return getTimeElement(); 317 case 3556653: return getTextElement(); 318 default: return super.makeProperty(hash, name); 319 } 320 321 } 322 323 @Override 324 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 325 switch (hash) { 326 case -1406328437: /*author*/ return new String[] {"Reference", "string"}; 327 case 3560141: /*time*/ return new String[] {"dateTime"}; 328 case 3556653: /*text*/ return new String[] {"markdown"}; 329 default: return super.getTypesForProperty(hash, name); 330 } 331 332 } 333 334 @Override 335 public Base addChild(String name) throws FHIRException { 336 if (name.equals("authorReference")) { 337 this.author = new Reference(); 338 return this.author; 339 } 340 else if (name.equals("authorString")) { 341 this.author = new StringType(); 342 return this.author; 343 } 344 else if (name.equals("time")) { 345 throw new FHIRException("Cannot call addChild on a singleton property Annotation.time"); 346 } 347 else if (name.equals("text")) { 348 throw new FHIRException("Cannot call addChild on a singleton property Annotation.text"); 349 } 350 else 351 return super.addChild(name); 352 } 353 354 public String fhirType() { 355 return "Annotation"; 356 357 } 358 359 public Annotation copy() { 360 Annotation dst = new Annotation(); 361 copyValues(dst); 362 return dst; 363 } 364 365 public void copyValues(Annotation dst) { 366 super.copyValues(dst); 367 dst.author = author == null ? null : author.copy(); 368 dst.time = time == null ? null : time.copy(); 369 dst.text = text == null ? null : text.copy(); 370 } 371 372 protected Annotation typedCopy() { 373 return copy(); 374 } 375 376 @Override 377 public boolean equalsDeep(Base other_) { 378 if (!super.equalsDeep(other_)) 379 return false; 380 if (!(other_ instanceof Annotation)) 381 return false; 382 Annotation o = (Annotation) other_; 383 return compareDeep(author, o.author, true) && compareDeep(time, o.time, true) && compareDeep(text, o.text, true) 384 ; 385 } 386 387 @Override 388 public boolean equalsShallow(Base other_) { 389 if (!super.equalsShallow(other_)) 390 return false; 391 if (!(other_ instanceof Annotation)) 392 return false; 393 Annotation o = (Annotation) other_; 394 return compareValues(time, o.time, true) && compareValues(text, o.text, true); 395 } 396 397 public boolean isEmpty() { 398 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(author, time, text); 399 } 400 401 402} 403