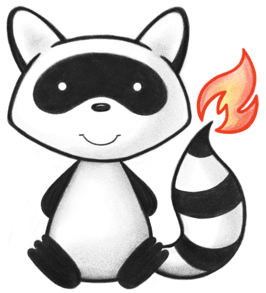
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 052 */ 053@ResourceDef(name="Appointment", profile="http://hl7.org/fhir/StructureDefinition/Appointment") 054public class Appointment extends DomainResource { 055 056 public enum AppointmentStatus { 057 /** 058 * None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time might not be set yet. 059 */ 060 PROPOSED, 061 /** 062 * Some or all of the participant(s) have not finalized their acceptance of the appointment request. 063 */ 064 PENDING, 065 /** 066 * All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified. 067 */ 068 BOOKED, 069 /** 070 * The patient/patients has/have arrived and is/are waiting to be seen. 071 */ 072 ARRIVED, 073 /** 074 * The planning stages of the appointment are now complete, the encounter resource will exist and will track further status changes. Note that an encounter may exist before the appointment status is fulfilled for many reasons. 075 */ 076 FULFILLED, 077 /** 078 * The appointment has been cancelled. 079 */ 080 CANCELLED, 081 /** 082 * Some or all of the participant(s) have not/did not appear for the appointment (usually the patient). 083 */ 084 NOSHOW, 085 /** 086 * This instance should not have been part of this patient's medical record. 087 */ 088 ENTEREDINERROR, 089 /** 090 * When checked in, all pre-encounter administrative work is complete, and the encounter may begin. (where multiple patients are involved, they are all present). 091 */ 092 CHECKEDIN, 093 /** 094 * The appointment has been placed on a waitlist, to be scheduled/confirmed in the future when a slot/service is available.\nA specific time might or might not be pre-allocated. 095 */ 096 WAITLIST, 097 /** 098 * added to help the parsers with the generic types 099 */ 100 NULL; 101 public static AppointmentStatus fromCode(String codeString) throws FHIRException { 102 if (codeString == null || "".equals(codeString)) 103 return null; 104 if ("proposed".equals(codeString)) 105 return PROPOSED; 106 if ("pending".equals(codeString)) 107 return PENDING; 108 if ("booked".equals(codeString)) 109 return BOOKED; 110 if ("arrived".equals(codeString)) 111 return ARRIVED; 112 if ("fulfilled".equals(codeString)) 113 return FULFILLED; 114 if ("cancelled".equals(codeString)) 115 return CANCELLED; 116 if ("noshow".equals(codeString)) 117 return NOSHOW; 118 if ("entered-in-error".equals(codeString)) 119 return ENTEREDINERROR; 120 if ("checked-in".equals(codeString)) 121 return CHECKEDIN; 122 if ("waitlist".equals(codeString)) 123 return WAITLIST; 124 if (Configuration.isAcceptInvalidEnums()) 125 return null; 126 else 127 throw new FHIRException("Unknown AppointmentStatus code '"+codeString+"'"); 128 } 129 public String toCode() { 130 switch (this) { 131 case PROPOSED: return "proposed"; 132 case PENDING: return "pending"; 133 case BOOKED: return "booked"; 134 case ARRIVED: return "arrived"; 135 case FULFILLED: return "fulfilled"; 136 case CANCELLED: return "cancelled"; 137 case NOSHOW: return "noshow"; 138 case ENTEREDINERROR: return "entered-in-error"; 139 case CHECKEDIN: return "checked-in"; 140 case WAITLIST: return "waitlist"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getSystem() { 146 switch (this) { 147 case PROPOSED: return "http://hl7.org/fhir/appointmentstatus"; 148 case PENDING: return "http://hl7.org/fhir/appointmentstatus"; 149 case BOOKED: return "http://hl7.org/fhir/appointmentstatus"; 150 case ARRIVED: return "http://hl7.org/fhir/appointmentstatus"; 151 case FULFILLED: return "http://hl7.org/fhir/appointmentstatus"; 152 case CANCELLED: return "http://hl7.org/fhir/appointmentstatus"; 153 case NOSHOW: return "http://hl7.org/fhir/appointmentstatus"; 154 case ENTEREDINERROR: return "http://hl7.org/fhir/appointmentstatus"; 155 case CHECKEDIN: return "http://hl7.org/fhir/appointmentstatus"; 156 case WAITLIST: return "http://hl7.org/fhir/appointmentstatus"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 public String getDefinition() { 162 switch (this) { 163 case PROPOSED: return "None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time might not be set yet."; 164 case PENDING: return "Some or all of the participant(s) have not finalized their acceptance of the appointment request."; 165 case BOOKED: return "All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified."; 166 case ARRIVED: return "The patient/patients has/have arrived and is/are waiting to be seen."; 167 case FULFILLED: return "The planning stages of the appointment are now complete, the encounter resource will exist and will track further status changes. Note that an encounter may exist before the appointment status is fulfilled for many reasons."; 168 case CANCELLED: return "The appointment has been cancelled."; 169 case NOSHOW: return "Some or all of the participant(s) have not/did not appear for the appointment (usually the patient)."; 170 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 171 case CHECKEDIN: return "When checked in, all pre-encounter administrative work is complete, and the encounter may begin. (where multiple patients are involved, they are all present)."; 172 case WAITLIST: return "The appointment has been placed on a waitlist, to be scheduled/confirmed in the future when a slot/service is available.\nA specific time might or might not be pre-allocated."; 173 case NULL: return null; 174 default: return "?"; 175 } 176 } 177 public String getDisplay() { 178 switch (this) { 179 case PROPOSED: return "Proposed"; 180 case PENDING: return "Pending"; 181 case BOOKED: return "Booked"; 182 case ARRIVED: return "Arrived"; 183 case FULFILLED: return "Fulfilled"; 184 case CANCELLED: return "Cancelled"; 185 case NOSHOW: return "No Show"; 186 case ENTEREDINERROR: return "Entered in error"; 187 case CHECKEDIN: return "Checked In"; 188 case WAITLIST: return "Waitlisted"; 189 case NULL: return null; 190 default: return "?"; 191 } 192 } 193 } 194 195 public static class AppointmentStatusEnumFactory implements EnumFactory<AppointmentStatus> { 196 public AppointmentStatus fromCode(String codeString) throws IllegalArgumentException { 197 if (codeString == null || "".equals(codeString)) 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("proposed".equals(codeString)) 201 return AppointmentStatus.PROPOSED; 202 if ("pending".equals(codeString)) 203 return AppointmentStatus.PENDING; 204 if ("booked".equals(codeString)) 205 return AppointmentStatus.BOOKED; 206 if ("arrived".equals(codeString)) 207 return AppointmentStatus.ARRIVED; 208 if ("fulfilled".equals(codeString)) 209 return AppointmentStatus.FULFILLED; 210 if ("cancelled".equals(codeString)) 211 return AppointmentStatus.CANCELLED; 212 if ("noshow".equals(codeString)) 213 return AppointmentStatus.NOSHOW; 214 if ("entered-in-error".equals(codeString)) 215 return AppointmentStatus.ENTEREDINERROR; 216 if ("checked-in".equals(codeString)) 217 return AppointmentStatus.CHECKEDIN; 218 if ("waitlist".equals(codeString)) 219 return AppointmentStatus.WAITLIST; 220 throw new IllegalArgumentException("Unknown AppointmentStatus code '"+codeString+"'"); 221 } 222 public Enumeration<AppointmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 223 if (code == null) 224 return null; 225 if (code.isEmpty()) 226 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NULL, code); 227 String codeString = ((PrimitiveType) code).asStringValue(); 228 if (codeString == null || "".equals(codeString)) 229 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NULL, code); 230 if ("proposed".equals(codeString)) 231 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PROPOSED, code); 232 if ("pending".equals(codeString)) 233 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PENDING, code); 234 if ("booked".equals(codeString)) 235 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.BOOKED, code); 236 if ("arrived".equals(codeString)) 237 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ARRIVED, code); 238 if ("fulfilled".equals(codeString)) 239 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.FULFILLED, code); 240 if ("cancelled".equals(codeString)) 241 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CANCELLED, code); 242 if ("noshow".equals(codeString)) 243 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NOSHOW, code); 244 if ("entered-in-error".equals(codeString)) 245 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ENTEREDINERROR, code); 246 if ("checked-in".equals(codeString)) 247 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CHECKEDIN, code); 248 if ("waitlist".equals(codeString)) 249 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.WAITLIST, code); 250 throw new FHIRException("Unknown AppointmentStatus code '"+codeString+"'"); 251 } 252 public String toCode(AppointmentStatus code) { 253 if (code == AppointmentStatus.NULL) 254 return null; 255 if (code == AppointmentStatus.PROPOSED) 256 return "proposed"; 257 if (code == AppointmentStatus.PENDING) 258 return "pending"; 259 if (code == AppointmentStatus.BOOKED) 260 return "booked"; 261 if (code == AppointmentStatus.ARRIVED) 262 return "arrived"; 263 if (code == AppointmentStatus.FULFILLED) 264 return "fulfilled"; 265 if (code == AppointmentStatus.CANCELLED) 266 return "cancelled"; 267 if (code == AppointmentStatus.NOSHOW) 268 return "noshow"; 269 if (code == AppointmentStatus.ENTEREDINERROR) 270 return "entered-in-error"; 271 if (code == AppointmentStatus.CHECKEDIN) 272 return "checked-in"; 273 if (code == AppointmentStatus.WAITLIST) 274 return "waitlist"; 275 return "?"; 276 } 277 public String toSystem(AppointmentStatus code) { 278 return code.getSystem(); 279 } 280 } 281 282 public enum ParticipationStatus { 283 /** 284 * The participant has accepted the appointment. 285 */ 286 ACCEPTED, 287 /** 288 * The participant has declined the appointment and will not participate in the appointment. 289 */ 290 DECLINED, 291 /** 292 * The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur. 293 */ 294 TENTATIVE, 295 /** 296 * The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses. 297 */ 298 NEEDSACTION, 299 /** 300 * added to help the parsers with the generic types 301 */ 302 NULL; 303 public static ParticipationStatus fromCode(String codeString) throws FHIRException { 304 if (codeString == null || "".equals(codeString)) 305 return null; 306 if ("accepted".equals(codeString)) 307 return ACCEPTED; 308 if ("declined".equals(codeString)) 309 return DECLINED; 310 if ("tentative".equals(codeString)) 311 return TENTATIVE; 312 if ("needs-action".equals(codeString)) 313 return NEEDSACTION; 314 if (Configuration.isAcceptInvalidEnums()) 315 return null; 316 else 317 throw new FHIRException("Unknown ParticipationStatus code '"+codeString+"'"); 318 } 319 public String toCode() { 320 switch (this) { 321 case ACCEPTED: return "accepted"; 322 case DECLINED: return "declined"; 323 case TENTATIVE: return "tentative"; 324 case NEEDSACTION: return "needs-action"; 325 case NULL: return null; 326 default: return "?"; 327 } 328 } 329 public String getSystem() { 330 switch (this) { 331 case ACCEPTED: return "http://hl7.org/fhir/participationstatus"; 332 case DECLINED: return "http://hl7.org/fhir/participationstatus"; 333 case TENTATIVE: return "http://hl7.org/fhir/participationstatus"; 334 case NEEDSACTION: return "http://hl7.org/fhir/participationstatus"; 335 case NULL: return null; 336 default: return "?"; 337 } 338 } 339 public String getDefinition() { 340 switch (this) { 341 case ACCEPTED: return "The participant has accepted the appointment."; 342 case DECLINED: return "The participant has declined the appointment and will not participate in the appointment."; 343 case TENTATIVE: return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 344 case NEEDSACTION: return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 345 case NULL: return null; 346 default: return "?"; 347 } 348 } 349 public String getDisplay() { 350 switch (this) { 351 case ACCEPTED: return "Accepted"; 352 case DECLINED: return "Declined"; 353 case TENTATIVE: return "Tentative"; 354 case NEEDSACTION: return "Needs Action"; 355 case NULL: return null; 356 default: return "?"; 357 } 358 } 359 } 360 361 public static class ParticipationStatusEnumFactory implements EnumFactory<ParticipationStatus> { 362 public ParticipationStatus fromCode(String codeString) throws IllegalArgumentException { 363 if (codeString == null || "".equals(codeString)) 364 if (codeString == null || "".equals(codeString)) 365 return null; 366 if ("accepted".equals(codeString)) 367 return ParticipationStatus.ACCEPTED; 368 if ("declined".equals(codeString)) 369 return ParticipationStatus.DECLINED; 370 if ("tentative".equals(codeString)) 371 return ParticipationStatus.TENTATIVE; 372 if ("needs-action".equals(codeString)) 373 return ParticipationStatus.NEEDSACTION; 374 throw new IllegalArgumentException("Unknown ParticipationStatus code '"+codeString+"'"); 375 } 376 public Enumeration<ParticipationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 377 if (code == null) 378 return null; 379 if (code.isEmpty()) 380 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NULL, code); 381 String codeString = ((PrimitiveType) code).asStringValue(); 382 if (codeString == null || "".equals(codeString)) 383 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NULL, code); 384 if ("accepted".equals(codeString)) 385 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.ACCEPTED, code); 386 if ("declined".equals(codeString)) 387 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.DECLINED, code); 388 if ("tentative".equals(codeString)) 389 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.TENTATIVE, code); 390 if ("needs-action".equals(codeString)) 391 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NEEDSACTION, code); 392 throw new FHIRException("Unknown ParticipationStatus code '"+codeString+"'"); 393 } 394 public String toCode(ParticipationStatus code) { 395 if (code == ParticipationStatus.NULL) 396 return null; 397 if (code == ParticipationStatus.ACCEPTED) 398 return "accepted"; 399 if (code == ParticipationStatus.DECLINED) 400 return "declined"; 401 if (code == ParticipationStatus.TENTATIVE) 402 return "tentative"; 403 if (code == ParticipationStatus.NEEDSACTION) 404 return "needs-action"; 405 return "?"; 406 } 407 public String toSystem(ParticipationStatus code) { 408 return code.getSystem(); 409 } 410 } 411 412 @Block() 413 public static class AppointmentParticipantComponent extends BackboneElement implements IBaseBackboneElement { 414 /** 415 * Role of participant in the appointment. 416 */ 417 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 418 @Description(shortDefinition="Role of participant in the appointment", formalDefinition="Role of participant in the appointment." ) 419 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-participant-type") 420 protected List<CodeableConcept> type; 421 422 /** 423 * Participation period of the actor. 424 */ 425 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 426 @Description(shortDefinition="Participation period of the actor", formalDefinition="Participation period of the actor." ) 427 protected Period period; 428 429 /** 430 * The individual, device, location, or service participating in the appointment. 431 */ 432 @Child(name = "actor", type = {Patient.class, Group.class, Practitioner.class, PractitionerRole.class, CareTeam.class, RelatedPerson.class, Device.class, HealthcareService.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=true) 433 @Description(shortDefinition="The individual, device, location, or service participating in the appointment", formalDefinition="The individual, device, location, or service participating in the appointment." ) 434 protected Reference actor; 435 436 /** 437 * Whether this participant is required to be present at the meeting. If false, the participant is optional. 438 */ 439 @Child(name = "required", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 440 @Description(shortDefinition="The participant is required to attend (optional when false)", formalDefinition="Whether this participant is required to be present at the meeting. If false, the participant is optional." ) 441 protected BooleanType required; 442 443 /** 444 * Participation status of the actor. 445 */ 446 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 447 @Description(shortDefinition="accepted | declined | tentative | needs-action", formalDefinition="Participation status of the actor." ) 448 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participationstatus") 449 protected Enumeration<ParticipationStatus> status; 450 451 private static final long serialVersionUID = 1537536134L; 452 453 /** 454 * Constructor 455 */ 456 public AppointmentParticipantComponent() { 457 super(); 458 } 459 460 /** 461 * Constructor 462 */ 463 public AppointmentParticipantComponent(ParticipationStatus status) { 464 super(); 465 this.setStatus(status); 466 } 467 468 /** 469 * @return {@link #type} (Role of participant in the appointment.) 470 */ 471 public List<CodeableConcept> getType() { 472 if (this.type == null) 473 this.type = new ArrayList<CodeableConcept>(); 474 return this.type; 475 } 476 477 /** 478 * @return Returns a reference to <code>this</code> for easy method chaining 479 */ 480 public AppointmentParticipantComponent setType(List<CodeableConcept> theType) { 481 this.type = theType; 482 return this; 483 } 484 485 public boolean hasType() { 486 if (this.type == null) 487 return false; 488 for (CodeableConcept item : this.type) 489 if (!item.isEmpty()) 490 return true; 491 return false; 492 } 493 494 public CodeableConcept addType() { //3 495 CodeableConcept t = new CodeableConcept(); 496 if (this.type == null) 497 this.type = new ArrayList<CodeableConcept>(); 498 this.type.add(t); 499 return t; 500 } 501 502 public AppointmentParticipantComponent addType(CodeableConcept t) { //3 503 if (t == null) 504 return this; 505 if (this.type == null) 506 this.type = new ArrayList<CodeableConcept>(); 507 this.type.add(t); 508 return this; 509 } 510 511 /** 512 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 513 */ 514 public CodeableConcept getTypeFirstRep() { 515 if (getType().isEmpty()) { 516 addType(); 517 } 518 return getType().get(0); 519 } 520 521 /** 522 * @return {@link #period} (Participation period of the actor.) 523 */ 524 public Period getPeriod() { 525 if (this.period == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create AppointmentParticipantComponent.period"); 528 else if (Configuration.doAutoCreate()) 529 this.period = new Period(); // cc 530 return this.period; 531 } 532 533 public boolean hasPeriod() { 534 return this.period != null && !this.period.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #period} (Participation period of the actor.) 539 */ 540 public AppointmentParticipantComponent setPeriod(Period value) { 541 this.period = value; 542 return this; 543 } 544 545 /** 546 * @return {@link #actor} (The individual, device, location, or service participating in the appointment.) 547 */ 548 public Reference getActor() { 549 if (this.actor == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create AppointmentParticipantComponent.actor"); 552 else if (Configuration.doAutoCreate()) 553 this.actor = new Reference(); // cc 554 return this.actor; 555 } 556 557 public boolean hasActor() { 558 return this.actor != null && !this.actor.isEmpty(); 559 } 560 561 /** 562 * @param value {@link #actor} (The individual, device, location, or service participating in the appointment.) 563 */ 564 public AppointmentParticipantComponent setActor(Reference value) { 565 this.actor = value; 566 return this; 567 } 568 569 /** 570 * @return {@link #required} (Whether this participant is required to be present at the meeting. If false, the participant is optional.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 571 */ 572 public BooleanType getRequiredElement() { 573 if (this.required == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create AppointmentParticipantComponent.required"); 576 else if (Configuration.doAutoCreate()) 577 this.required = new BooleanType(); // bb 578 return this.required; 579 } 580 581 public boolean hasRequiredElement() { 582 return this.required != null && !this.required.isEmpty(); 583 } 584 585 public boolean hasRequired() { 586 return this.required != null && !this.required.isEmpty(); 587 } 588 589 /** 590 * @param value {@link #required} (Whether this participant is required to be present at the meeting. If false, the participant is optional.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 591 */ 592 public AppointmentParticipantComponent setRequiredElement(BooleanType value) { 593 this.required = value; 594 return this; 595 } 596 597 /** 598 * @return Whether this participant is required to be present at the meeting. If false, the participant is optional. 599 */ 600 public boolean getRequired() { 601 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 602 } 603 604 /** 605 * @param value Whether this participant is required to be present at the meeting. If false, the participant is optional. 606 */ 607 public AppointmentParticipantComponent setRequired(boolean value) { 608 if (this.required == null) 609 this.required = new BooleanType(); 610 this.required.setValue(value); 611 return this; 612 } 613 614 /** 615 * @return {@link #status} (Participation status of the actor.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 616 */ 617 public Enumeration<ParticipationStatus> getStatusElement() { 618 if (this.status == null) 619 if (Configuration.errorOnAutoCreate()) 620 throw new Error("Attempt to auto-create AppointmentParticipantComponent.status"); 621 else if (Configuration.doAutoCreate()) 622 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); // bb 623 return this.status; 624 } 625 626 public boolean hasStatusElement() { 627 return this.status != null && !this.status.isEmpty(); 628 } 629 630 public boolean hasStatus() { 631 return this.status != null && !this.status.isEmpty(); 632 } 633 634 /** 635 * @param value {@link #status} (Participation status of the actor.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 636 */ 637 public AppointmentParticipantComponent setStatusElement(Enumeration<ParticipationStatus> value) { 638 this.status = value; 639 return this; 640 } 641 642 /** 643 * @return Participation status of the actor. 644 */ 645 public ParticipationStatus getStatus() { 646 return this.status == null ? null : this.status.getValue(); 647 } 648 649 /** 650 * @param value Participation status of the actor. 651 */ 652 public AppointmentParticipantComponent setStatus(ParticipationStatus value) { 653 if (this.status == null) 654 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); 655 this.status.setValue(value); 656 return this; 657 } 658 659 protected void listChildren(List<Property> children) { 660 super.listChildren(children); 661 children.add(new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, type)); 662 children.add(new Property("period", "Period", "Participation period of the actor.", 0, 1, period)); 663 children.add(new Property("actor", "Reference(Patient|Group|Practitioner|PractitionerRole|CareTeam|RelatedPerson|Device|HealthcareService|Location)", "The individual, device, location, or service participating in the appointment.", 0, 1, actor)); 664 children.add(new Property("required", "boolean", "Whether this participant is required to be present at the meeting. If false, the participant is optional.", 0, 1, required)); 665 children.add(new Property("status", "code", "Participation status of the actor.", 0, 1, status)); 666 } 667 668 @Override 669 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 670 switch (_hash) { 671 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, type); 672 case -991726143: /*period*/ return new Property("period", "Period", "Participation period of the actor.", 0, 1, period); 673 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Group|Practitioner|PractitionerRole|CareTeam|RelatedPerson|Device|HealthcareService|Location)", "The individual, device, location, or service participating in the appointment.", 0, 1, actor); 674 case -393139297: /*required*/ return new Property("required", "boolean", "Whether this participant is required to be present at the meeting. If false, the participant is optional.", 0, 1, required); 675 case -892481550: /*status*/ return new Property("status", "code", "Participation status of the actor.", 0, 1, status); 676 default: return super.getNamedProperty(_hash, _name, _checkValid); 677 } 678 679 } 680 681 @Override 682 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 683 switch (hash) { 684 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 685 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 686 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 687 case -393139297: /*required*/ return this.required == null ? new Base[0] : new Base[] {this.required}; // BooleanType 688 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ParticipationStatus> 689 default: return super.getProperty(hash, name, checkValid); 690 } 691 692 } 693 694 @Override 695 public Base setProperty(int hash, String name, Base value) throws FHIRException { 696 switch (hash) { 697 case 3575610: // type 698 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 699 return value; 700 case -991726143: // period 701 this.period = TypeConvertor.castToPeriod(value); // Period 702 return value; 703 case 92645877: // actor 704 this.actor = TypeConvertor.castToReference(value); // Reference 705 return value; 706 case -393139297: // required 707 this.required = TypeConvertor.castToBoolean(value); // BooleanType 708 return value; 709 case -892481550: // status 710 value = new ParticipationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 711 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 712 return value; 713 default: return super.setProperty(hash, name, value); 714 } 715 716 } 717 718 @Override 719 public Base setProperty(String name, Base value) throws FHIRException { 720 if (name.equals("type")) { 721 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 722 } else if (name.equals("period")) { 723 this.period = TypeConvertor.castToPeriod(value); // Period 724 } else if (name.equals("actor")) { 725 this.actor = TypeConvertor.castToReference(value); // Reference 726 } else if (name.equals("required")) { 727 this.required = TypeConvertor.castToBoolean(value); // BooleanType 728 } else if (name.equals("status")) { 729 value = new ParticipationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 730 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 731 } else 732 return super.setProperty(name, value); 733 return value; 734 } 735 736 @Override 737 public void removeChild(String name, Base value) throws FHIRException { 738 if (name.equals("type")) { 739 this.getType().remove(value); 740 } else if (name.equals("period")) { 741 this.period = null; 742 } else if (name.equals("actor")) { 743 this.actor = null; 744 } else if (name.equals("required")) { 745 this.required = null; 746 } else if (name.equals("status")) { 747 value = new ParticipationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 748 this.status = (Enumeration) value; // Enumeration<ParticipationStatus> 749 } else 750 super.removeChild(name, value); 751 752 } 753 754 @Override 755 public Base makeProperty(int hash, String name) throws FHIRException { 756 switch (hash) { 757 case 3575610: return addType(); 758 case -991726143: return getPeriod(); 759 case 92645877: return getActor(); 760 case -393139297: return getRequiredElement(); 761 case -892481550: return getStatusElement(); 762 default: return super.makeProperty(hash, name); 763 } 764 765 } 766 767 @Override 768 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 769 switch (hash) { 770 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 771 case -991726143: /*period*/ return new String[] {"Period"}; 772 case 92645877: /*actor*/ return new String[] {"Reference"}; 773 case -393139297: /*required*/ return new String[] {"boolean"}; 774 case -892481550: /*status*/ return new String[] {"code"}; 775 default: return super.getTypesForProperty(hash, name); 776 } 777 778 } 779 780 @Override 781 public Base addChild(String name) throws FHIRException { 782 if (name.equals("type")) { 783 return addType(); 784 } 785 else if (name.equals("period")) { 786 this.period = new Period(); 787 return this.period; 788 } 789 else if (name.equals("actor")) { 790 this.actor = new Reference(); 791 return this.actor; 792 } 793 else if (name.equals("required")) { 794 throw new FHIRException("Cannot call addChild on a singleton property Appointment.participant.required"); 795 } 796 else if (name.equals("status")) { 797 throw new FHIRException("Cannot call addChild on a singleton property Appointment.participant.status"); 798 } 799 else 800 return super.addChild(name); 801 } 802 803 public AppointmentParticipantComponent copy() { 804 AppointmentParticipantComponent dst = new AppointmentParticipantComponent(); 805 copyValues(dst); 806 return dst; 807 } 808 809 public void copyValues(AppointmentParticipantComponent dst) { 810 super.copyValues(dst); 811 if (type != null) { 812 dst.type = new ArrayList<CodeableConcept>(); 813 for (CodeableConcept i : type) 814 dst.type.add(i.copy()); 815 }; 816 dst.period = period == null ? null : period.copy(); 817 dst.actor = actor == null ? null : actor.copy(); 818 dst.required = required == null ? null : required.copy(); 819 dst.status = status == null ? null : status.copy(); 820 } 821 822 @Override 823 public boolean equalsDeep(Base other_) { 824 if (!super.equalsDeep(other_)) 825 return false; 826 if (!(other_ instanceof AppointmentParticipantComponent)) 827 return false; 828 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 829 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) 830 && compareDeep(required, o.required, true) && compareDeep(status, o.status, true); 831 } 832 833 @Override 834 public boolean equalsShallow(Base other_) { 835 if (!super.equalsShallow(other_)) 836 return false; 837 if (!(other_ instanceof AppointmentParticipantComponent)) 838 return false; 839 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other_; 840 return compareValues(required, o.required, true) && compareValues(status, o.status, true); 841 } 842 843 public boolean isEmpty() { 844 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, actor, required 845 , status); 846 } 847 848 public String fhirType() { 849 return "Appointment.participant"; 850 851 } 852 853 } 854 855 @Block() 856 public static class AppointmentRecurrenceTemplateComponent extends BackboneElement implements IBaseBackboneElement { 857 /** 858 * The timezone of the recurring appointment occurrences. 859 */ 860 @Child(name = "timezone", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 861 @Description(shortDefinition="The timezone of the occurrences", formalDefinition="The timezone of the recurring appointment occurrences." ) 862 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/timezones") 863 protected CodeableConcept timezone; 864 865 /** 866 * How often the appointment series should recur. 867 */ 868 @Child(name = "recurrenceType", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 869 @Description(shortDefinition="The frequency of the recurrence", formalDefinition="How often the appointment series should recur." ) 870 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/appointment-recurrrence-type") 871 protected CodeableConcept recurrenceType; 872 873 /** 874 * Recurring appointments will not occur after this date. 875 */ 876 @Child(name = "lastOccurrenceDate", type = {DateType.class}, order=3, min=0, max=1, modifier=false, summary=false) 877 @Description(shortDefinition="The date when the recurrence should end", formalDefinition="Recurring appointments will not occur after this date." ) 878 protected DateType lastOccurrenceDate; 879 880 /** 881 * How many appointments are planned in the recurrence. 882 */ 883 @Child(name = "occurrenceCount", type = {PositiveIntType.class}, order=4, min=0, max=1, modifier=false, summary=false) 884 @Description(shortDefinition="The number of planned occurrences", formalDefinition="How many appointments are planned in the recurrence." ) 885 protected PositiveIntType occurrenceCount; 886 887 /** 888 * The list of specific dates that will have appointments generated. 889 */ 890 @Child(name = "occurrenceDate", type = {DateType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 891 @Description(shortDefinition="Specific dates for a recurring set of appointments (no template)", formalDefinition="The list of specific dates that will have appointments generated." ) 892 protected List<DateType> occurrenceDate; 893 894 /** 895 * Information about weekly recurring appointments. 896 */ 897 @Child(name = "weeklyTemplate", type = {}, order=6, min=0, max=1, modifier=false, summary=false) 898 @Description(shortDefinition="Information about weekly recurring appointments", formalDefinition="Information about weekly recurring appointments." ) 899 protected AppointmentRecurrenceTemplateWeeklyTemplateComponent weeklyTemplate; 900 901 /** 902 * Information about monthly recurring appointments. 903 */ 904 @Child(name = "monthlyTemplate", type = {}, order=7, min=0, max=1, modifier=false, summary=false) 905 @Description(shortDefinition="Information about monthly recurring appointments", formalDefinition="Information about monthly recurring appointments." ) 906 protected AppointmentRecurrenceTemplateMonthlyTemplateComponent monthlyTemplate; 907 908 /** 909 * Information about yearly recurring appointments. 910 */ 911 @Child(name = "yearlyTemplate", type = {}, order=8, min=0, max=1, modifier=false, summary=false) 912 @Description(shortDefinition="Information about yearly recurring appointments", formalDefinition="Information about yearly recurring appointments." ) 913 protected AppointmentRecurrenceTemplateYearlyTemplateComponent yearlyTemplate; 914 915 /** 916 * Any dates, such as holidays, that should be excluded from the recurrence. 917 */ 918 @Child(name = "excludingDate", type = {DateType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 919 @Description(shortDefinition="Any dates that should be excluded from the series", formalDefinition="Any dates, such as holidays, that should be excluded from the recurrence." ) 920 protected List<DateType> excludingDate; 921 922 /** 923 * Any dates, such as holidays, that should be excluded from the recurrence. 924 */ 925 @Child(name = "excludingRecurrenceId", type = {PositiveIntType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 926 @Description(shortDefinition="Any recurrence IDs that should be excluded from the recurrence", formalDefinition="Any dates, such as holidays, that should be excluded from the recurrence." ) 927 protected List<PositiveIntType> excludingRecurrenceId; 928 929 private static final long serialVersionUID = -1582999176L; 930 931 /** 932 * Constructor 933 */ 934 public AppointmentRecurrenceTemplateComponent() { 935 super(); 936 } 937 938 /** 939 * Constructor 940 */ 941 public AppointmentRecurrenceTemplateComponent(CodeableConcept recurrenceType) { 942 super(); 943 this.setRecurrenceType(recurrenceType); 944 } 945 946 /** 947 * @return {@link #timezone} (The timezone of the recurring appointment occurrences.) 948 */ 949 public CodeableConcept getTimezone() { 950 if (this.timezone == null) 951 if (Configuration.errorOnAutoCreate()) 952 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.timezone"); 953 else if (Configuration.doAutoCreate()) 954 this.timezone = new CodeableConcept(); // cc 955 return this.timezone; 956 } 957 958 public boolean hasTimezone() { 959 return this.timezone != null && !this.timezone.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #timezone} (The timezone of the recurring appointment occurrences.) 964 */ 965 public AppointmentRecurrenceTemplateComponent setTimezone(CodeableConcept value) { 966 this.timezone = value; 967 return this; 968 } 969 970 /** 971 * @return {@link #recurrenceType} (How often the appointment series should recur.) 972 */ 973 public CodeableConcept getRecurrenceType() { 974 if (this.recurrenceType == null) 975 if (Configuration.errorOnAutoCreate()) 976 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.recurrenceType"); 977 else if (Configuration.doAutoCreate()) 978 this.recurrenceType = new CodeableConcept(); // cc 979 return this.recurrenceType; 980 } 981 982 public boolean hasRecurrenceType() { 983 return this.recurrenceType != null && !this.recurrenceType.isEmpty(); 984 } 985 986 /** 987 * @param value {@link #recurrenceType} (How often the appointment series should recur.) 988 */ 989 public AppointmentRecurrenceTemplateComponent setRecurrenceType(CodeableConcept value) { 990 this.recurrenceType = value; 991 return this; 992 } 993 994 /** 995 * @return {@link #lastOccurrenceDate} (Recurring appointments will not occur after this date.). This is the underlying object with id, value and extensions. The accessor "getLastOccurrenceDate" gives direct access to the value 996 */ 997 public DateType getLastOccurrenceDateElement() { 998 if (this.lastOccurrenceDate == null) 999 if (Configuration.errorOnAutoCreate()) 1000 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.lastOccurrenceDate"); 1001 else if (Configuration.doAutoCreate()) 1002 this.lastOccurrenceDate = new DateType(); // bb 1003 return this.lastOccurrenceDate; 1004 } 1005 1006 public boolean hasLastOccurrenceDateElement() { 1007 return this.lastOccurrenceDate != null && !this.lastOccurrenceDate.isEmpty(); 1008 } 1009 1010 public boolean hasLastOccurrenceDate() { 1011 return this.lastOccurrenceDate != null && !this.lastOccurrenceDate.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #lastOccurrenceDate} (Recurring appointments will not occur after this date.). This is the underlying object with id, value and extensions. The accessor "getLastOccurrenceDate" gives direct access to the value 1016 */ 1017 public AppointmentRecurrenceTemplateComponent setLastOccurrenceDateElement(DateType value) { 1018 this.lastOccurrenceDate = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return Recurring appointments will not occur after this date. 1024 */ 1025 public Date getLastOccurrenceDate() { 1026 return this.lastOccurrenceDate == null ? null : this.lastOccurrenceDate.getValue(); 1027 } 1028 1029 /** 1030 * @param value Recurring appointments will not occur after this date. 1031 */ 1032 public AppointmentRecurrenceTemplateComponent setLastOccurrenceDate(Date value) { 1033 if (value == null) 1034 this.lastOccurrenceDate = null; 1035 else { 1036 if (this.lastOccurrenceDate == null) 1037 this.lastOccurrenceDate = new DateType(); 1038 this.lastOccurrenceDate.setValue(value); 1039 } 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #occurrenceCount} (How many appointments are planned in the recurrence.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceCount" gives direct access to the value 1045 */ 1046 public PositiveIntType getOccurrenceCountElement() { 1047 if (this.occurrenceCount == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.occurrenceCount"); 1050 else if (Configuration.doAutoCreate()) 1051 this.occurrenceCount = new PositiveIntType(); // bb 1052 return this.occurrenceCount; 1053 } 1054 1055 public boolean hasOccurrenceCountElement() { 1056 return this.occurrenceCount != null && !this.occurrenceCount.isEmpty(); 1057 } 1058 1059 public boolean hasOccurrenceCount() { 1060 return this.occurrenceCount != null && !this.occurrenceCount.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #occurrenceCount} (How many appointments are planned in the recurrence.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceCount" gives direct access to the value 1065 */ 1066 public AppointmentRecurrenceTemplateComponent setOccurrenceCountElement(PositiveIntType value) { 1067 this.occurrenceCount = value; 1068 return this; 1069 } 1070 1071 /** 1072 * @return How many appointments are planned in the recurrence. 1073 */ 1074 public int getOccurrenceCount() { 1075 return this.occurrenceCount == null || this.occurrenceCount.isEmpty() ? 0 : this.occurrenceCount.getValue(); 1076 } 1077 1078 /** 1079 * @param value How many appointments are planned in the recurrence. 1080 */ 1081 public AppointmentRecurrenceTemplateComponent setOccurrenceCount(int value) { 1082 if (this.occurrenceCount == null) 1083 this.occurrenceCount = new PositiveIntType(); 1084 this.occurrenceCount.setValue(value); 1085 return this; 1086 } 1087 1088 /** 1089 * @return {@link #occurrenceDate} (The list of specific dates that will have appointments generated.) 1090 */ 1091 public List<DateType> getOccurrenceDate() { 1092 if (this.occurrenceDate == null) 1093 this.occurrenceDate = new ArrayList<DateType>(); 1094 return this.occurrenceDate; 1095 } 1096 1097 /** 1098 * @return Returns a reference to <code>this</code> for easy method chaining 1099 */ 1100 public AppointmentRecurrenceTemplateComponent setOccurrenceDate(List<DateType> theOccurrenceDate) { 1101 this.occurrenceDate = theOccurrenceDate; 1102 return this; 1103 } 1104 1105 public boolean hasOccurrenceDate() { 1106 if (this.occurrenceDate == null) 1107 return false; 1108 for (DateType item : this.occurrenceDate) 1109 if (!item.isEmpty()) 1110 return true; 1111 return false; 1112 } 1113 1114 /** 1115 * @return {@link #occurrenceDate} (The list of specific dates that will have appointments generated.) 1116 */ 1117 public DateType addOccurrenceDateElement() {//2 1118 DateType t = new DateType(); 1119 if (this.occurrenceDate == null) 1120 this.occurrenceDate = new ArrayList<DateType>(); 1121 this.occurrenceDate.add(t); 1122 return t; 1123 } 1124 1125 /** 1126 * @param value {@link #occurrenceDate} (The list of specific dates that will have appointments generated.) 1127 */ 1128 public AppointmentRecurrenceTemplateComponent addOccurrenceDate(Date value) { //1 1129 DateType t = new DateType(); 1130 t.setValue(value); 1131 if (this.occurrenceDate == null) 1132 this.occurrenceDate = new ArrayList<DateType>(); 1133 this.occurrenceDate.add(t); 1134 return this; 1135 } 1136 1137 /** 1138 * @param value {@link #occurrenceDate} (The list of specific dates that will have appointments generated.) 1139 */ 1140 public boolean hasOccurrenceDate(Date value) { 1141 if (this.occurrenceDate == null) 1142 return false; 1143 for (DateType v : this.occurrenceDate) 1144 if (v.getValue().equals(value)) // date 1145 return true; 1146 return false; 1147 } 1148 1149 /** 1150 * @return {@link #weeklyTemplate} (Information about weekly recurring appointments.) 1151 */ 1152 public AppointmentRecurrenceTemplateWeeklyTemplateComponent getWeeklyTemplate() { 1153 if (this.weeklyTemplate == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.weeklyTemplate"); 1156 else if (Configuration.doAutoCreate()) 1157 this.weeklyTemplate = new AppointmentRecurrenceTemplateWeeklyTemplateComponent(); // cc 1158 return this.weeklyTemplate; 1159 } 1160 1161 public boolean hasWeeklyTemplate() { 1162 return this.weeklyTemplate != null && !this.weeklyTemplate.isEmpty(); 1163 } 1164 1165 /** 1166 * @param value {@link #weeklyTemplate} (Information about weekly recurring appointments.) 1167 */ 1168 public AppointmentRecurrenceTemplateComponent setWeeklyTemplate(AppointmentRecurrenceTemplateWeeklyTemplateComponent value) { 1169 this.weeklyTemplate = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return {@link #monthlyTemplate} (Information about monthly recurring appointments.) 1175 */ 1176 public AppointmentRecurrenceTemplateMonthlyTemplateComponent getMonthlyTemplate() { 1177 if (this.monthlyTemplate == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.monthlyTemplate"); 1180 else if (Configuration.doAutoCreate()) 1181 this.monthlyTemplate = new AppointmentRecurrenceTemplateMonthlyTemplateComponent(); // cc 1182 return this.monthlyTemplate; 1183 } 1184 1185 public boolean hasMonthlyTemplate() { 1186 return this.monthlyTemplate != null && !this.monthlyTemplate.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #monthlyTemplate} (Information about monthly recurring appointments.) 1191 */ 1192 public AppointmentRecurrenceTemplateComponent setMonthlyTemplate(AppointmentRecurrenceTemplateMonthlyTemplateComponent value) { 1193 this.monthlyTemplate = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return {@link #yearlyTemplate} (Information about yearly recurring appointments.) 1199 */ 1200 public AppointmentRecurrenceTemplateYearlyTemplateComponent getYearlyTemplate() { 1201 if (this.yearlyTemplate == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateComponent.yearlyTemplate"); 1204 else if (Configuration.doAutoCreate()) 1205 this.yearlyTemplate = new AppointmentRecurrenceTemplateYearlyTemplateComponent(); // cc 1206 return this.yearlyTemplate; 1207 } 1208 1209 public boolean hasYearlyTemplate() { 1210 return this.yearlyTemplate != null && !this.yearlyTemplate.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #yearlyTemplate} (Information about yearly recurring appointments.) 1215 */ 1216 public AppointmentRecurrenceTemplateComponent setYearlyTemplate(AppointmentRecurrenceTemplateYearlyTemplateComponent value) { 1217 this.yearlyTemplate = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #excludingDate} (Any dates, such as holidays, that should be excluded from the recurrence.) 1223 */ 1224 public List<DateType> getExcludingDate() { 1225 if (this.excludingDate == null) 1226 this.excludingDate = new ArrayList<DateType>(); 1227 return this.excludingDate; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public AppointmentRecurrenceTemplateComponent setExcludingDate(List<DateType> theExcludingDate) { 1234 this.excludingDate = theExcludingDate; 1235 return this; 1236 } 1237 1238 public boolean hasExcludingDate() { 1239 if (this.excludingDate == null) 1240 return false; 1241 for (DateType item : this.excludingDate) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 /** 1248 * @return {@link #excludingDate} (Any dates, such as holidays, that should be excluded from the recurrence.) 1249 */ 1250 public DateType addExcludingDateElement() {//2 1251 DateType t = new DateType(); 1252 if (this.excludingDate == null) 1253 this.excludingDate = new ArrayList<DateType>(); 1254 this.excludingDate.add(t); 1255 return t; 1256 } 1257 1258 /** 1259 * @param value {@link #excludingDate} (Any dates, such as holidays, that should be excluded from the recurrence.) 1260 */ 1261 public AppointmentRecurrenceTemplateComponent addExcludingDate(Date value) { //1 1262 DateType t = new DateType(); 1263 t.setValue(value); 1264 if (this.excludingDate == null) 1265 this.excludingDate = new ArrayList<DateType>(); 1266 this.excludingDate.add(t); 1267 return this; 1268 } 1269 1270 /** 1271 * @param value {@link #excludingDate} (Any dates, such as holidays, that should be excluded from the recurrence.) 1272 */ 1273 public boolean hasExcludingDate(Date value) { 1274 if (this.excludingDate == null) 1275 return false; 1276 for (DateType v : this.excludingDate) 1277 if (v.getValue().equals(value)) // date 1278 return true; 1279 return false; 1280 } 1281 1282 /** 1283 * @return {@link #excludingRecurrenceId} (Any dates, such as holidays, that should be excluded from the recurrence.) 1284 */ 1285 public List<PositiveIntType> getExcludingRecurrenceId() { 1286 if (this.excludingRecurrenceId == null) 1287 this.excludingRecurrenceId = new ArrayList<PositiveIntType>(); 1288 return this.excludingRecurrenceId; 1289 } 1290 1291 /** 1292 * @return Returns a reference to <code>this</code> for easy method chaining 1293 */ 1294 public AppointmentRecurrenceTemplateComponent setExcludingRecurrenceId(List<PositiveIntType> theExcludingRecurrenceId) { 1295 this.excludingRecurrenceId = theExcludingRecurrenceId; 1296 return this; 1297 } 1298 1299 public boolean hasExcludingRecurrenceId() { 1300 if (this.excludingRecurrenceId == null) 1301 return false; 1302 for (PositiveIntType item : this.excludingRecurrenceId) 1303 if (!item.isEmpty()) 1304 return true; 1305 return false; 1306 } 1307 1308 /** 1309 * @return {@link #excludingRecurrenceId} (Any dates, such as holidays, that should be excluded from the recurrence.) 1310 */ 1311 public PositiveIntType addExcludingRecurrenceIdElement() {//2 1312 PositiveIntType t = new PositiveIntType(); 1313 if (this.excludingRecurrenceId == null) 1314 this.excludingRecurrenceId = new ArrayList<PositiveIntType>(); 1315 this.excludingRecurrenceId.add(t); 1316 return t; 1317 } 1318 1319 /** 1320 * @param value {@link #excludingRecurrenceId} (Any dates, such as holidays, that should be excluded from the recurrence.) 1321 */ 1322 public AppointmentRecurrenceTemplateComponent addExcludingRecurrenceId(int value) { //1 1323 PositiveIntType t = new PositiveIntType(); 1324 t.setValue(value); 1325 if (this.excludingRecurrenceId == null) 1326 this.excludingRecurrenceId = new ArrayList<PositiveIntType>(); 1327 this.excludingRecurrenceId.add(t); 1328 return this; 1329 } 1330 1331 /** 1332 * @param value {@link #excludingRecurrenceId} (Any dates, such as holidays, that should be excluded from the recurrence.) 1333 */ 1334 public boolean hasExcludingRecurrenceId(int value) { 1335 if (this.excludingRecurrenceId == null) 1336 return false; 1337 for (PositiveIntType v : this.excludingRecurrenceId) 1338 if (v.getValue().equals(value)) // positiveInt 1339 return true; 1340 return false; 1341 } 1342 1343 protected void listChildren(List<Property> children) { 1344 super.listChildren(children); 1345 children.add(new Property("timezone", "CodeableConcept", "The timezone of the recurring appointment occurrences.", 0, 1, timezone)); 1346 children.add(new Property("recurrenceType", "CodeableConcept", "How often the appointment series should recur.", 0, 1, recurrenceType)); 1347 children.add(new Property("lastOccurrenceDate", "date", "Recurring appointments will not occur after this date.", 0, 1, lastOccurrenceDate)); 1348 children.add(new Property("occurrenceCount", "positiveInt", "How many appointments are planned in the recurrence.", 0, 1, occurrenceCount)); 1349 children.add(new Property("occurrenceDate", "date", "The list of specific dates that will have appointments generated.", 0, java.lang.Integer.MAX_VALUE, occurrenceDate)); 1350 children.add(new Property("weeklyTemplate", "", "Information about weekly recurring appointments.", 0, 1, weeklyTemplate)); 1351 children.add(new Property("monthlyTemplate", "", "Information about monthly recurring appointments.", 0, 1, monthlyTemplate)); 1352 children.add(new Property("yearlyTemplate", "", "Information about yearly recurring appointments.", 0, 1, yearlyTemplate)); 1353 children.add(new Property("excludingDate", "date", "Any dates, such as holidays, that should be excluded from the recurrence.", 0, java.lang.Integer.MAX_VALUE, excludingDate)); 1354 children.add(new Property("excludingRecurrenceId", "positiveInt", "Any dates, such as holidays, that should be excluded from the recurrence.", 0, java.lang.Integer.MAX_VALUE, excludingRecurrenceId)); 1355 } 1356 1357 @Override 1358 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1359 switch (_hash) { 1360 case -2076227591: /*timezone*/ return new Property("timezone", "CodeableConcept", "The timezone of the recurring appointment occurrences.", 0, 1, timezone); 1361 case -381221238: /*recurrenceType*/ return new Property("recurrenceType", "CodeableConcept", "How often the appointment series should recur.", 0, 1, recurrenceType); 1362 case -1262346923: /*lastOccurrenceDate*/ return new Property("lastOccurrenceDate", "date", "Recurring appointments will not occur after this date.", 0, 1, lastOccurrenceDate); 1363 case 1834480062: /*occurrenceCount*/ return new Property("occurrenceCount", "positiveInt", "How many appointments are planned in the recurrence.", 0, 1, occurrenceCount); 1364 case 1721761055: /*occurrenceDate*/ return new Property("occurrenceDate", "date", "The list of specific dates that will have appointments generated.", 0, java.lang.Integer.MAX_VALUE, occurrenceDate); 1365 case 887136283: /*weeklyTemplate*/ return new Property("weeklyTemplate", "", "Information about weekly recurring appointments.", 0, 1, weeklyTemplate); 1366 case 2142528423: /*monthlyTemplate*/ return new Property("monthlyTemplate", "", "Information about monthly recurring appointments.", 0, 1, monthlyTemplate); 1367 case -334069468: /*yearlyTemplate*/ return new Property("yearlyTemplate", "", "Information about yearly recurring appointments.", 0, 1, yearlyTemplate); 1368 case 596601957: /*excludingDate*/ return new Property("excludingDate", "date", "Any dates, such as holidays, that should be excluded from the recurrence.", 0, java.lang.Integer.MAX_VALUE, excludingDate); 1369 case -797577694: /*excludingRecurrenceId*/ return new Property("excludingRecurrenceId", "positiveInt", "Any dates, such as holidays, that should be excluded from the recurrence.", 0, java.lang.Integer.MAX_VALUE, excludingRecurrenceId); 1370 default: return super.getNamedProperty(_hash, _name, _checkValid); 1371 } 1372 1373 } 1374 1375 @Override 1376 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1377 switch (hash) { 1378 case -2076227591: /*timezone*/ return this.timezone == null ? new Base[0] : new Base[] {this.timezone}; // CodeableConcept 1379 case -381221238: /*recurrenceType*/ return this.recurrenceType == null ? new Base[0] : new Base[] {this.recurrenceType}; // CodeableConcept 1380 case -1262346923: /*lastOccurrenceDate*/ return this.lastOccurrenceDate == null ? new Base[0] : new Base[] {this.lastOccurrenceDate}; // DateType 1381 case 1834480062: /*occurrenceCount*/ return this.occurrenceCount == null ? new Base[0] : new Base[] {this.occurrenceCount}; // PositiveIntType 1382 case 1721761055: /*occurrenceDate*/ return this.occurrenceDate == null ? new Base[0] : this.occurrenceDate.toArray(new Base[this.occurrenceDate.size()]); // DateType 1383 case 887136283: /*weeklyTemplate*/ return this.weeklyTemplate == null ? new Base[0] : new Base[] {this.weeklyTemplate}; // AppointmentRecurrenceTemplateWeeklyTemplateComponent 1384 case 2142528423: /*monthlyTemplate*/ return this.monthlyTemplate == null ? new Base[0] : new Base[] {this.monthlyTemplate}; // AppointmentRecurrenceTemplateMonthlyTemplateComponent 1385 case -334069468: /*yearlyTemplate*/ return this.yearlyTemplate == null ? new Base[0] : new Base[] {this.yearlyTemplate}; // AppointmentRecurrenceTemplateYearlyTemplateComponent 1386 case 596601957: /*excludingDate*/ return this.excludingDate == null ? new Base[0] : this.excludingDate.toArray(new Base[this.excludingDate.size()]); // DateType 1387 case -797577694: /*excludingRecurrenceId*/ return this.excludingRecurrenceId == null ? new Base[0] : this.excludingRecurrenceId.toArray(new Base[this.excludingRecurrenceId.size()]); // PositiveIntType 1388 default: return super.getProperty(hash, name, checkValid); 1389 } 1390 1391 } 1392 1393 @Override 1394 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1395 switch (hash) { 1396 case -2076227591: // timezone 1397 this.timezone = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1398 return value; 1399 case -381221238: // recurrenceType 1400 this.recurrenceType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1401 return value; 1402 case -1262346923: // lastOccurrenceDate 1403 this.lastOccurrenceDate = TypeConvertor.castToDate(value); // DateType 1404 return value; 1405 case 1834480062: // occurrenceCount 1406 this.occurrenceCount = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1407 return value; 1408 case 1721761055: // occurrenceDate 1409 this.getOccurrenceDate().add(TypeConvertor.castToDate(value)); // DateType 1410 return value; 1411 case 887136283: // weeklyTemplate 1412 this.weeklyTemplate = (AppointmentRecurrenceTemplateWeeklyTemplateComponent) value; // AppointmentRecurrenceTemplateWeeklyTemplateComponent 1413 return value; 1414 case 2142528423: // monthlyTemplate 1415 this.monthlyTemplate = (AppointmentRecurrenceTemplateMonthlyTemplateComponent) value; // AppointmentRecurrenceTemplateMonthlyTemplateComponent 1416 return value; 1417 case -334069468: // yearlyTemplate 1418 this.yearlyTemplate = (AppointmentRecurrenceTemplateYearlyTemplateComponent) value; // AppointmentRecurrenceTemplateYearlyTemplateComponent 1419 return value; 1420 case 596601957: // excludingDate 1421 this.getExcludingDate().add(TypeConvertor.castToDate(value)); // DateType 1422 return value; 1423 case -797577694: // excludingRecurrenceId 1424 this.getExcludingRecurrenceId().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 1425 return value; 1426 default: return super.setProperty(hash, name, value); 1427 } 1428 1429 } 1430 1431 @Override 1432 public Base setProperty(String name, Base value) throws FHIRException { 1433 if (name.equals("timezone")) { 1434 this.timezone = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1435 } else if (name.equals("recurrenceType")) { 1436 this.recurrenceType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1437 } else if (name.equals("lastOccurrenceDate")) { 1438 this.lastOccurrenceDate = TypeConvertor.castToDate(value); // DateType 1439 } else if (name.equals("occurrenceCount")) { 1440 this.occurrenceCount = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1441 } else if (name.equals("occurrenceDate")) { 1442 this.getOccurrenceDate().add(TypeConvertor.castToDate(value)); 1443 } else if (name.equals("weeklyTemplate")) { 1444 this.weeklyTemplate = (AppointmentRecurrenceTemplateWeeklyTemplateComponent) value; // AppointmentRecurrenceTemplateWeeklyTemplateComponent 1445 } else if (name.equals("monthlyTemplate")) { 1446 this.monthlyTemplate = (AppointmentRecurrenceTemplateMonthlyTemplateComponent) value; // AppointmentRecurrenceTemplateMonthlyTemplateComponent 1447 } else if (name.equals("yearlyTemplate")) { 1448 this.yearlyTemplate = (AppointmentRecurrenceTemplateYearlyTemplateComponent) value; // AppointmentRecurrenceTemplateYearlyTemplateComponent 1449 } else if (name.equals("excludingDate")) { 1450 this.getExcludingDate().add(TypeConvertor.castToDate(value)); 1451 } else if (name.equals("excludingRecurrenceId")) { 1452 this.getExcludingRecurrenceId().add(TypeConvertor.castToPositiveInt(value)); 1453 } else 1454 return super.setProperty(name, value); 1455 return value; 1456 } 1457 1458 @Override 1459 public void removeChild(String name, Base value) throws FHIRException { 1460 if (name.equals("timezone")) { 1461 this.timezone = null; 1462 } else if (name.equals("recurrenceType")) { 1463 this.recurrenceType = null; 1464 } else if (name.equals("lastOccurrenceDate")) { 1465 this.lastOccurrenceDate = null; 1466 } else if (name.equals("occurrenceCount")) { 1467 this.occurrenceCount = null; 1468 } else if (name.equals("occurrenceDate")) { 1469 this.getOccurrenceDate().remove(value); 1470 } else if (name.equals("weeklyTemplate")) { 1471 this.weeklyTemplate = (AppointmentRecurrenceTemplateWeeklyTemplateComponent) value; // AppointmentRecurrenceTemplateWeeklyTemplateComponent 1472 } else if (name.equals("monthlyTemplate")) { 1473 this.monthlyTemplate = (AppointmentRecurrenceTemplateMonthlyTemplateComponent) value; // AppointmentRecurrenceTemplateMonthlyTemplateComponent 1474 } else if (name.equals("yearlyTemplate")) { 1475 this.yearlyTemplate = (AppointmentRecurrenceTemplateYearlyTemplateComponent) value; // AppointmentRecurrenceTemplateYearlyTemplateComponent 1476 } else if (name.equals("excludingDate")) { 1477 this.getExcludingDate().remove(value); 1478 } else if (name.equals("excludingRecurrenceId")) { 1479 this.getExcludingRecurrenceId().remove(value); 1480 } else 1481 super.removeChild(name, value); 1482 1483 } 1484 1485 @Override 1486 public Base makeProperty(int hash, String name) throws FHIRException { 1487 switch (hash) { 1488 case -2076227591: return getTimezone(); 1489 case -381221238: return getRecurrenceType(); 1490 case -1262346923: return getLastOccurrenceDateElement(); 1491 case 1834480062: return getOccurrenceCountElement(); 1492 case 1721761055: return addOccurrenceDateElement(); 1493 case 887136283: return getWeeklyTemplate(); 1494 case 2142528423: return getMonthlyTemplate(); 1495 case -334069468: return getYearlyTemplate(); 1496 case 596601957: return addExcludingDateElement(); 1497 case -797577694: return addExcludingRecurrenceIdElement(); 1498 default: return super.makeProperty(hash, name); 1499 } 1500 1501 } 1502 1503 @Override 1504 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1505 switch (hash) { 1506 case -2076227591: /*timezone*/ return new String[] {"CodeableConcept"}; 1507 case -381221238: /*recurrenceType*/ return new String[] {"CodeableConcept"}; 1508 case -1262346923: /*lastOccurrenceDate*/ return new String[] {"date"}; 1509 case 1834480062: /*occurrenceCount*/ return new String[] {"positiveInt"}; 1510 case 1721761055: /*occurrenceDate*/ return new String[] {"date"}; 1511 case 887136283: /*weeklyTemplate*/ return new String[] {}; 1512 case 2142528423: /*monthlyTemplate*/ return new String[] {}; 1513 case -334069468: /*yearlyTemplate*/ return new String[] {}; 1514 case 596601957: /*excludingDate*/ return new String[] {"date"}; 1515 case -797577694: /*excludingRecurrenceId*/ return new String[] {"positiveInt"}; 1516 default: return super.getTypesForProperty(hash, name); 1517 } 1518 1519 } 1520 1521 @Override 1522 public Base addChild(String name) throws FHIRException { 1523 if (name.equals("timezone")) { 1524 this.timezone = new CodeableConcept(); 1525 return this.timezone; 1526 } 1527 else if (name.equals("recurrenceType")) { 1528 this.recurrenceType = new CodeableConcept(); 1529 return this.recurrenceType; 1530 } 1531 else if (name.equals("lastOccurrenceDate")) { 1532 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.lastOccurrenceDate"); 1533 } 1534 else if (name.equals("occurrenceCount")) { 1535 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.occurrenceCount"); 1536 } 1537 else if (name.equals("occurrenceDate")) { 1538 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.occurrenceDate"); 1539 } 1540 else if (name.equals("weeklyTemplate")) { 1541 this.weeklyTemplate = new AppointmentRecurrenceTemplateWeeklyTemplateComponent(); 1542 return this.weeklyTemplate; 1543 } 1544 else if (name.equals("monthlyTemplate")) { 1545 this.monthlyTemplate = new AppointmentRecurrenceTemplateMonthlyTemplateComponent(); 1546 return this.monthlyTemplate; 1547 } 1548 else if (name.equals("yearlyTemplate")) { 1549 this.yearlyTemplate = new AppointmentRecurrenceTemplateYearlyTemplateComponent(); 1550 return this.yearlyTemplate; 1551 } 1552 else if (name.equals("excludingDate")) { 1553 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.excludingDate"); 1554 } 1555 else if (name.equals("excludingRecurrenceId")) { 1556 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.excludingRecurrenceId"); 1557 } 1558 else 1559 return super.addChild(name); 1560 } 1561 1562 public AppointmentRecurrenceTemplateComponent copy() { 1563 AppointmentRecurrenceTemplateComponent dst = new AppointmentRecurrenceTemplateComponent(); 1564 copyValues(dst); 1565 return dst; 1566 } 1567 1568 public void copyValues(AppointmentRecurrenceTemplateComponent dst) { 1569 super.copyValues(dst); 1570 dst.timezone = timezone == null ? null : timezone.copy(); 1571 dst.recurrenceType = recurrenceType == null ? null : recurrenceType.copy(); 1572 dst.lastOccurrenceDate = lastOccurrenceDate == null ? null : lastOccurrenceDate.copy(); 1573 dst.occurrenceCount = occurrenceCount == null ? null : occurrenceCount.copy(); 1574 if (occurrenceDate != null) { 1575 dst.occurrenceDate = new ArrayList<DateType>(); 1576 for (DateType i : occurrenceDate) 1577 dst.occurrenceDate.add(i.copy()); 1578 }; 1579 dst.weeklyTemplate = weeklyTemplate == null ? null : weeklyTemplate.copy(); 1580 dst.monthlyTemplate = monthlyTemplate == null ? null : monthlyTemplate.copy(); 1581 dst.yearlyTemplate = yearlyTemplate == null ? null : yearlyTemplate.copy(); 1582 if (excludingDate != null) { 1583 dst.excludingDate = new ArrayList<DateType>(); 1584 for (DateType i : excludingDate) 1585 dst.excludingDate.add(i.copy()); 1586 }; 1587 if (excludingRecurrenceId != null) { 1588 dst.excludingRecurrenceId = new ArrayList<PositiveIntType>(); 1589 for (PositiveIntType i : excludingRecurrenceId) 1590 dst.excludingRecurrenceId.add(i.copy()); 1591 }; 1592 } 1593 1594 @Override 1595 public boolean equalsDeep(Base other_) { 1596 if (!super.equalsDeep(other_)) 1597 return false; 1598 if (!(other_ instanceof AppointmentRecurrenceTemplateComponent)) 1599 return false; 1600 AppointmentRecurrenceTemplateComponent o = (AppointmentRecurrenceTemplateComponent) other_; 1601 return compareDeep(timezone, o.timezone, true) && compareDeep(recurrenceType, o.recurrenceType, true) 1602 && compareDeep(lastOccurrenceDate, o.lastOccurrenceDate, true) && compareDeep(occurrenceCount, o.occurrenceCount, true) 1603 && compareDeep(occurrenceDate, o.occurrenceDate, true) && compareDeep(weeklyTemplate, o.weeklyTemplate, true) 1604 && compareDeep(monthlyTemplate, o.monthlyTemplate, true) && compareDeep(yearlyTemplate, o.yearlyTemplate, true) 1605 && compareDeep(excludingDate, o.excludingDate, true) && compareDeep(excludingRecurrenceId, o.excludingRecurrenceId, true) 1606 ; 1607 } 1608 1609 @Override 1610 public boolean equalsShallow(Base other_) { 1611 if (!super.equalsShallow(other_)) 1612 return false; 1613 if (!(other_ instanceof AppointmentRecurrenceTemplateComponent)) 1614 return false; 1615 AppointmentRecurrenceTemplateComponent o = (AppointmentRecurrenceTemplateComponent) other_; 1616 return compareValues(lastOccurrenceDate, o.lastOccurrenceDate, true) && compareValues(occurrenceCount, o.occurrenceCount, true) 1617 && compareValues(occurrenceDate, o.occurrenceDate, true) && compareValues(excludingDate, o.excludingDate, true) 1618 && compareValues(excludingRecurrenceId, o.excludingRecurrenceId, true); 1619 } 1620 1621 public boolean isEmpty() { 1622 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(timezone, recurrenceType, lastOccurrenceDate 1623 , occurrenceCount, occurrenceDate, weeklyTemplate, monthlyTemplate, yearlyTemplate 1624 , excludingDate, excludingRecurrenceId); 1625 } 1626 1627 public String fhirType() { 1628 return "Appointment.recurrenceTemplate"; 1629 1630 } 1631 1632 } 1633 1634 @Block() 1635 public static class AppointmentRecurrenceTemplateWeeklyTemplateComponent extends BackboneElement implements IBaseBackboneElement { 1636 /** 1637 * Indicates that recurring appointments should occur on Mondays. 1638 */ 1639 @Child(name = "monday", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1640 @Description(shortDefinition="Recurs on Mondays", formalDefinition="Indicates that recurring appointments should occur on Mondays." ) 1641 protected BooleanType monday; 1642 1643 /** 1644 * Indicates that recurring appointments should occur on Tuesdays. 1645 */ 1646 @Child(name = "tuesday", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1647 @Description(shortDefinition="Recurs on Tuesday", formalDefinition="Indicates that recurring appointments should occur on Tuesdays." ) 1648 protected BooleanType tuesday; 1649 1650 /** 1651 * Indicates that recurring appointments should occur on Wednesdays. 1652 */ 1653 @Child(name = "wednesday", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1654 @Description(shortDefinition="Recurs on Wednesday", formalDefinition="Indicates that recurring appointments should occur on Wednesdays." ) 1655 protected BooleanType wednesday; 1656 1657 /** 1658 * Indicates that recurring appointments should occur on Thursdays. 1659 */ 1660 @Child(name = "thursday", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1661 @Description(shortDefinition="Recurs on Thursday", formalDefinition="Indicates that recurring appointments should occur on Thursdays." ) 1662 protected BooleanType thursday; 1663 1664 /** 1665 * Indicates that recurring appointments should occur on Fridays. 1666 */ 1667 @Child(name = "friday", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1668 @Description(shortDefinition="Recurs on Friday", formalDefinition="Indicates that recurring appointments should occur on Fridays." ) 1669 protected BooleanType friday; 1670 1671 /** 1672 * Indicates that recurring appointments should occur on Saturdays. 1673 */ 1674 @Child(name = "saturday", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1675 @Description(shortDefinition="Recurs on Saturday", formalDefinition="Indicates that recurring appointments should occur on Saturdays." ) 1676 protected BooleanType saturday; 1677 1678 /** 1679 * Indicates that recurring appointments should occur on Sundays. 1680 */ 1681 @Child(name = "sunday", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=false) 1682 @Description(shortDefinition="Recurs on Sunday", formalDefinition="Indicates that recurring appointments should occur on Sundays." ) 1683 protected BooleanType sunday; 1684 1685 /** 1686 * The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more. 1687 1688e.g. For recurring every second week this interval would be 2, or every third week the interval would be 3. 1689 */ 1690 @Child(name = "weekInterval", type = {PositiveIntType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1691 @Description(shortDefinition="Recurs every nth week", formalDefinition="The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more.\r\re.g. For recurring every second week this interval would be 2, or every third week the interval would be 3." ) 1692 protected PositiveIntType weekInterval; 1693 1694 private static final long serialVersionUID = 588795188L; 1695 1696 /** 1697 * Constructor 1698 */ 1699 public AppointmentRecurrenceTemplateWeeklyTemplateComponent() { 1700 super(); 1701 } 1702 1703 /** 1704 * @return {@link #monday} (Indicates that recurring appointments should occur on Mondays.). This is the underlying object with id, value and extensions. The accessor "getMonday" gives direct access to the value 1705 */ 1706 public BooleanType getMondayElement() { 1707 if (this.monday == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.monday"); 1710 else if (Configuration.doAutoCreate()) 1711 this.monday = new BooleanType(); // bb 1712 return this.monday; 1713 } 1714 1715 public boolean hasMondayElement() { 1716 return this.monday != null && !this.monday.isEmpty(); 1717 } 1718 1719 public boolean hasMonday() { 1720 return this.monday != null && !this.monday.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #monday} (Indicates that recurring appointments should occur on Mondays.). This is the underlying object with id, value and extensions. The accessor "getMonday" gives direct access to the value 1725 */ 1726 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setMondayElement(BooleanType value) { 1727 this.monday = value; 1728 return this; 1729 } 1730 1731 /** 1732 * @return Indicates that recurring appointments should occur on Mondays. 1733 */ 1734 public boolean getMonday() { 1735 return this.monday == null || this.monday.isEmpty() ? false : this.monday.getValue(); 1736 } 1737 1738 /** 1739 * @param value Indicates that recurring appointments should occur on Mondays. 1740 */ 1741 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setMonday(boolean value) { 1742 if (this.monday == null) 1743 this.monday = new BooleanType(); 1744 this.monday.setValue(value); 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #tuesday} (Indicates that recurring appointments should occur on Tuesdays.). This is the underlying object with id, value and extensions. The accessor "getTuesday" gives direct access to the value 1750 */ 1751 public BooleanType getTuesdayElement() { 1752 if (this.tuesday == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.tuesday"); 1755 else if (Configuration.doAutoCreate()) 1756 this.tuesday = new BooleanType(); // bb 1757 return this.tuesday; 1758 } 1759 1760 public boolean hasTuesdayElement() { 1761 return this.tuesday != null && !this.tuesday.isEmpty(); 1762 } 1763 1764 public boolean hasTuesday() { 1765 return this.tuesday != null && !this.tuesday.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #tuesday} (Indicates that recurring appointments should occur on Tuesdays.). This is the underlying object with id, value and extensions. The accessor "getTuesday" gives direct access to the value 1770 */ 1771 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setTuesdayElement(BooleanType value) { 1772 this.tuesday = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return Indicates that recurring appointments should occur on Tuesdays. 1778 */ 1779 public boolean getTuesday() { 1780 return this.tuesday == null || this.tuesday.isEmpty() ? false : this.tuesday.getValue(); 1781 } 1782 1783 /** 1784 * @param value Indicates that recurring appointments should occur on Tuesdays. 1785 */ 1786 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setTuesday(boolean value) { 1787 if (this.tuesday == null) 1788 this.tuesday = new BooleanType(); 1789 this.tuesday.setValue(value); 1790 return this; 1791 } 1792 1793 /** 1794 * @return {@link #wednesday} (Indicates that recurring appointments should occur on Wednesdays.). This is the underlying object with id, value and extensions. The accessor "getWednesday" gives direct access to the value 1795 */ 1796 public BooleanType getWednesdayElement() { 1797 if (this.wednesday == null) 1798 if (Configuration.errorOnAutoCreate()) 1799 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.wednesday"); 1800 else if (Configuration.doAutoCreate()) 1801 this.wednesday = new BooleanType(); // bb 1802 return this.wednesday; 1803 } 1804 1805 public boolean hasWednesdayElement() { 1806 return this.wednesday != null && !this.wednesday.isEmpty(); 1807 } 1808 1809 public boolean hasWednesday() { 1810 return this.wednesday != null && !this.wednesday.isEmpty(); 1811 } 1812 1813 /** 1814 * @param value {@link #wednesday} (Indicates that recurring appointments should occur on Wednesdays.). This is the underlying object with id, value and extensions. The accessor "getWednesday" gives direct access to the value 1815 */ 1816 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setWednesdayElement(BooleanType value) { 1817 this.wednesday = value; 1818 return this; 1819 } 1820 1821 /** 1822 * @return Indicates that recurring appointments should occur on Wednesdays. 1823 */ 1824 public boolean getWednesday() { 1825 return this.wednesday == null || this.wednesday.isEmpty() ? false : this.wednesday.getValue(); 1826 } 1827 1828 /** 1829 * @param value Indicates that recurring appointments should occur on Wednesdays. 1830 */ 1831 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setWednesday(boolean value) { 1832 if (this.wednesday == null) 1833 this.wednesday = new BooleanType(); 1834 this.wednesday.setValue(value); 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #thursday} (Indicates that recurring appointments should occur on Thursdays.). This is the underlying object with id, value and extensions. The accessor "getThursday" gives direct access to the value 1840 */ 1841 public BooleanType getThursdayElement() { 1842 if (this.thursday == null) 1843 if (Configuration.errorOnAutoCreate()) 1844 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.thursday"); 1845 else if (Configuration.doAutoCreate()) 1846 this.thursday = new BooleanType(); // bb 1847 return this.thursday; 1848 } 1849 1850 public boolean hasThursdayElement() { 1851 return this.thursday != null && !this.thursday.isEmpty(); 1852 } 1853 1854 public boolean hasThursday() { 1855 return this.thursday != null && !this.thursday.isEmpty(); 1856 } 1857 1858 /** 1859 * @param value {@link #thursday} (Indicates that recurring appointments should occur on Thursdays.). This is the underlying object with id, value and extensions. The accessor "getThursday" gives direct access to the value 1860 */ 1861 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setThursdayElement(BooleanType value) { 1862 this.thursday = value; 1863 return this; 1864 } 1865 1866 /** 1867 * @return Indicates that recurring appointments should occur on Thursdays. 1868 */ 1869 public boolean getThursday() { 1870 return this.thursday == null || this.thursday.isEmpty() ? false : this.thursday.getValue(); 1871 } 1872 1873 /** 1874 * @param value Indicates that recurring appointments should occur on Thursdays. 1875 */ 1876 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setThursday(boolean value) { 1877 if (this.thursday == null) 1878 this.thursday = new BooleanType(); 1879 this.thursday.setValue(value); 1880 return this; 1881 } 1882 1883 /** 1884 * @return {@link #friday} (Indicates that recurring appointments should occur on Fridays.). This is the underlying object with id, value and extensions. The accessor "getFriday" gives direct access to the value 1885 */ 1886 public BooleanType getFridayElement() { 1887 if (this.friday == null) 1888 if (Configuration.errorOnAutoCreate()) 1889 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.friday"); 1890 else if (Configuration.doAutoCreate()) 1891 this.friday = new BooleanType(); // bb 1892 return this.friday; 1893 } 1894 1895 public boolean hasFridayElement() { 1896 return this.friday != null && !this.friday.isEmpty(); 1897 } 1898 1899 public boolean hasFriday() { 1900 return this.friday != null && !this.friday.isEmpty(); 1901 } 1902 1903 /** 1904 * @param value {@link #friday} (Indicates that recurring appointments should occur on Fridays.). This is the underlying object with id, value and extensions. The accessor "getFriday" gives direct access to the value 1905 */ 1906 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setFridayElement(BooleanType value) { 1907 this.friday = value; 1908 return this; 1909 } 1910 1911 /** 1912 * @return Indicates that recurring appointments should occur on Fridays. 1913 */ 1914 public boolean getFriday() { 1915 return this.friday == null || this.friday.isEmpty() ? false : this.friday.getValue(); 1916 } 1917 1918 /** 1919 * @param value Indicates that recurring appointments should occur on Fridays. 1920 */ 1921 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setFriday(boolean value) { 1922 if (this.friday == null) 1923 this.friday = new BooleanType(); 1924 this.friday.setValue(value); 1925 return this; 1926 } 1927 1928 /** 1929 * @return {@link #saturday} (Indicates that recurring appointments should occur on Saturdays.). This is the underlying object with id, value and extensions. The accessor "getSaturday" gives direct access to the value 1930 */ 1931 public BooleanType getSaturdayElement() { 1932 if (this.saturday == null) 1933 if (Configuration.errorOnAutoCreate()) 1934 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.saturday"); 1935 else if (Configuration.doAutoCreate()) 1936 this.saturday = new BooleanType(); // bb 1937 return this.saturday; 1938 } 1939 1940 public boolean hasSaturdayElement() { 1941 return this.saturday != null && !this.saturday.isEmpty(); 1942 } 1943 1944 public boolean hasSaturday() { 1945 return this.saturday != null && !this.saturday.isEmpty(); 1946 } 1947 1948 /** 1949 * @param value {@link #saturday} (Indicates that recurring appointments should occur on Saturdays.). This is the underlying object with id, value and extensions. The accessor "getSaturday" gives direct access to the value 1950 */ 1951 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setSaturdayElement(BooleanType value) { 1952 this.saturday = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return Indicates that recurring appointments should occur on Saturdays. 1958 */ 1959 public boolean getSaturday() { 1960 return this.saturday == null || this.saturday.isEmpty() ? false : this.saturday.getValue(); 1961 } 1962 1963 /** 1964 * @param value Indicates that recurring appointments should occur on Saturdays. 1965 */ 1966 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setSaturday(boolean value) { 1967 if (this.saturday == null) 1968 this.saturday = new BooleanType(); 1969 this.saturday.setValue(value); 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #sunday} (Indicates that recurring appointments should occur on Sundays.). This is the underlying object with id, value and extensions. The accessor "getSunday" gives direct access to the value 1975 */ 1976 public BooleanType getSundayElement() { 1977 if (this.sunday == null) 1978 if (Configuration.errorOnAutoCreate()) 1979 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.sunday"); 1980 else if (Configuration.doAutoCreate()) 1981 this.sunday = new BooleanType(); // bb 1982 return this.sunday; 1983 } 1984 1985 public boolean hasSundayElement() { 1986 return this.sunday != null && !this.sunday.isEmpty(); 1987 } 1988 1989 public boolean hasSunday() { 1990 return this.sunday != null && !this.sunday.isEmpty(); 1991 } 1992 1993 /** 1994 * @param value {@link #sunday} (Indicates that recurring appointments should occur on Sundays.). This is the underlying object with id, value and extensions. The accessor "getSunday" gives direct access to the value 1995 */ 1996 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setSundayElement(BooleanType value) { 1997 this.sunday = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return Indicates that recurring appointments should occur on Sundays. 2003 */ 2004 public boolean getSunday() { 2005 return this.sunday == null || this.sunday.isEmpty() ? false : this.sunday.getValue(); 2006 } 2007 2008 /** 2009 * @param value Indicates that recurring appointments should occur on Sundays. 2010 */ 2011 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setSunday(boolean value) { 2012 if (this.sunday == null) 2013 this.sunday = new BooleanType(); 2014 this.sunday.setValue(value); 2015 return this; 2016 } 2017 2018 /** 2019 * @return {@link #weekInterval} (The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more. 2020 2021e.g. For recurring every second week this interval would be 2, or every third week the interval would be 3.). This is the underlying object with id, value and extensions. The accessor "getWeekInterval" gives direct access to the value 2022 */ 2023 public PositiveIntType getWeekIntervalElement() { 2024 if (this.weekInterval == null) 2025 if (Configuration.errorOnAutoCreate()) 2026 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateWeeklyTemplateComponent.weekInterval"); 2027 else if (Configuration.doAutoCreate()) 2028 this.weekInterval = new PositiveIntType(); // bb 2029 return this.weekInterval; 2030 } 2031 2032 public boolean hasWeekIntervalElement() { 2033 return this.weekInterval != null && !this.weekInterval.isEmpty(); 2034 } 2035 2036 public boolean hasWeekInterval() { 2037 return this.weekInterval != null && !this.weekInterval.isEmpty(); 2038 } 2039 2040 /** 2041 * @param value {@link #weekInterval} (The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more. 2042 2043e.g. For recurring every second week this interval would be 2, or every third week the interval would be 3.). This is the underlying object with id, value and extensions. The accessor "getWeekInterval" gives direct access to the value 2044 */ 2045 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setWeekIntervalElement(PositiveIntType value) { 2046 this.weekInterval = value; 2047 return this; 2048 } 2049 2050 /** 2051 * @return The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more. 2052 2053e.g. For recurring every second week this interval would be 2, or every third week the interval would be 3. 2054 */ 2055 public int getWeekInterval() { 2056 return this.weekInterval == null || this.weekInterval.isEmpty() ? 0 : this.weekInterval.getValue(); 2057 } 2058 2059 /** 2060 * @param value The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more. 2061 2062e.g. For recurring every second week this interval would be 2, or every third week the interval would be 3. 2063 */ 2064 public AppointmentRecurrenceTemplateWeeklyTemplateComponent setWeekInterval(int value) { 2065 if (this.weekInterval == null) 2066 this.weekInterval = new PositiveIntType(); 2067 this.weekInterval.setValue(value); 2068 return this; 2069 } 2070 2071 protected void listChildren(List<Property> children) { 2072 super.listChildren(children); 2073 children.add(new Property("monday", "boolean", "Indicates that recurring appointments should occur on Mondays.", 0, 1, monday)); 2074 children.add(new Property("tuesday", "boolean", "Indicates that recurring appointments should occur on Tuesdays.", 0, 1, tuesday)); 2075 children.add(new Property("wednesday", "boolean", "Indicates that recurring appointments should occur on Wednesdays.", 0, 1, wednesday)); 2076 children.add(new Property("thursday", "boolean", "Indicates that recurring appointments should occur on Thursdays.", 0, 1, thursday)); 2077 children.add(new Property("friday", "boolean", "Indicates that recurring appointments should occur on Fridays.", 0, 1, friday)); 2078 children.add(new Property("saturday", "boolean", "Indicates that recurring appointments should occur on Saturdays.", 0, 1, saturday)); 2079 children.add(new Property("sunday", "boolean", "Indicates that recurring appointments should occur on Sundays.", 0, 1, sunday)); 2080 children.add(new Property("weekInterval", "positiveInt", "The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more.\r\re.g. For recurring every second week this interval would be 2, or every third week the interval would be 3.", 0, 1, weekInterval)); 2081 } 2082 2083 @Override 2084 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2085 switch (_hash) { 2086 case -1068502768: /*monday*/ return new Property("monday", "boolean", "Indicates that recurring appointments should occur on Mondays.", 0, 1, monday); 2087 case -977343923: /*tuesday*/ return new Property("tuesday", "boolean", "Indicates that recurring appointments should occur on Tuesdays.", 0, 1, tuesday); 2088 case 1393530710: /*wednesday*/ return new Property("wednesday", "boolean", "Indicates that recurring appointments should occur on Wednesdays.", 0, 1, wednesday); 2089 case 1572055514: /*thursday*/ return new Property("thursday", "boolean", "Indicates that recurring appointments should occur on Thursdays.", 0, 1, thursday); 2090 case -1266285217: /*friday*/ return new Property("friday", "boolean", "Indicates that recurring appointments should occur on Fridays.", 0, 1, friday); 2091 case -2114201671: /*saturday*/ return new Property("saturday", "boolean", "Indicates that recurring appointments should occur on Saturdays.", 0, 1, saturday); 2092 case -891186736: /*sunday*/ return new Property("sunday", "boolean", "Indicates that recurring appointments should occur on Sundays.", 0, 1, sunday); 2093 case -784550695: /*weekInterval*/ return new Property("weekInterval", "positiveInt", "The interval defines if the recurrence is every nth week. The default is every week, so it is expected that this value will be 2 or more.\r\re.g. For recurring every second week this interval would be 2, or every third week the interval would be 3.", 0, 1, weekInterval); 2094 default: return super.getNamedProperty(_hash, _name, _checkValid); 2095 } 2096 2097 } 2098 2099 @Override 2100 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2101 switch (hash) { 2102 case -1068502768: /*monday*/ return this.monday == null ? new Base[0] : new Base[] {this.monday}; // BooleanType 2103 case -977343923: /*tuesday*/ return this.tuesday == null ? new Base[0] : new Base[] {this.tuesday}; // BooleanType 2104 case 1393530710: /*wednesday*/ return this.wednesday == null ? new Base[0] : new Base[] {this.wednesday}; // BooleanType 2105 case 1572055514: /*thursday*/ return this.thursday == null ? new Base[0] : new Base[] {this.thursday}; // BooleanType 2106 case -1266285217: /*friday*/ return this.friday == null ? new Base[0] : new Base[] {this.friday}; // BooleanType 2107 case -2114201671: /*saturday*/ return this.saturday == null ? new Base[0] : new Base[] {this.saturday}; // BooleanType 2108 case -891186736: /*sunday*/ return this.sunday == null ? new Base[0] : new Base[] {this.sunday}; // BooleanType 2109 case -784550695: /*weekInterval*/ return this.weekInterval == null ? new Base[0] : new Base[] {this.weekInterval}; // PositiveIntType 2110 default: return super.getProperty(hash, name, checkValid); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2117 switch (hash) { 2118 case -1068502768: // monday 2119 this.monday = TypeConvertor.castToBoolean(value); // BooleanType 2120 return value; 2121 case -977343923: // tuesday 2122 this.tuesday = TypeConvertor.castToBoolean(value); // BooleanType 2123 return value; 2124 case 1393530710: // wednesday 2125 this.wednesday = TypeConvertor.castToBoolean(value); // BooleanType 2126 return value; 2127 case 1572055514: // thursday 2128 this.thursday = TypeConvertor.castToBoolean(value); // BooleanType 2129 return value; 2130 case -1266285217: // friday 2131 this.friday = TypeConvertor.castToBoolean(value); // BooleanType 2132 return value; 2133 case -2114201671: // saturday 2134 this.saturday = TypeConvertor.castToBoolean(value); // BooleanType 2135 return value; 2136 case -891186736: // sunday 2137 this.sunday = TypeConvertor.castToBoolean(value); // BooleanType 2138 return value; 2139 case -784550695: // weekInterval 2140 this.weekInterval = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2141 return value; 2142 default: return super.setProperty(hash, name, value); 2143 } 2144 2145 } 2146 2147 @Override 2148 public Base setProperty(String name, Base value) throws FHIRException { 2149 if (name.equals("monday")) { 2150 this.monday = TypeConvertor.castToBoolean(value); // BooleanType 2151 } else if (name.equals("tuesday")) { 2152 this.tuesday = TypeConvertor.castToBoolean(value); // BooleanType 2153 } else if (name.equals("wednesday")) { 2154 this.wednesday = TypeConvertor.castToBoolean(value); // BooleanType 2155 } else if (name.equals("thursday")) { 2156 this.thursday = TypeConvertor.castToBoolean(value); // BooleanType 2157 } else if (name.equals("friday")) { 2158 this.friday = TypeConvertor.castToBoolean(value); // BooleanType 2159 } else if (name.equals("saturday")) { 2160 this.saturday = TypeConvertor.castToBoolean(value); // BooleanType 2161 } else if (name.equals("sunday")) { 2162 this.sunday = TypeConvertor.castToBoolean(value); // BooleanType 2163 } else if (name.equals("weekInterval")) { 2164 this.weekInterval = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2165 } else 2166 return super.setProperty(name, value); 2167 return value; 2168 } 2169 2170 @Override 2171 public void removeChild(String name, Base value) throws FHIRException { 2172 if (name.equals("monday")) { 2173 this.monday = null; 2174 } else if (name.equals("tuesday")) { 2175 this.tuesday = null; 2176 } else if (name.equals("wednesday")) { 2177 this.wednesday = null; 2178 } else if (name.equals("thursday")) { 2179 this.thursday = null; 2180 } else if (name.equals("friday")) { 2181 this.friday = null; 2182 } else if (name.equals("saturday")) { 2183 this.saturday = null; 2184 } else if (name.equals("sunday")) { 2185 this.sunday = null; 2186 } else if (name.equals("weekInterval")) { 2187 this.weekInterval = null; 2188 } else 2189 super.removeChild(name, value); 2190 2191 } 2192 2193 @Override 2194 public Base makeProperty(int hash, String name) throws FHIRException { 2195 switch (hash) { 2196 case -1068502768: return getMondayElement(); 2197 case -977343923: return getTuesdayElement(); 2198 case 1393530710: return getWednesdayElement(); 2199 case 1572055514: return getThursdayElement(); 2200 case -1266285217: return getFridayElement(); 2201 case -2114201671: return getSaturdayElement(); 2202 case -891186736: return getSundayElement(); 2203 case -784550695: return getWeekIntervalElement(); 2204 default: return super.makeProperty(hash, name); 2205 } 2206 2207 } 2208 2209 @Override 2210 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2211 switch (hash) { 2212 case -1068502768: /*monday*/ return new String[] {"boolean"}; 2213 case -977343923: /*tuesday*/ return new String[] {"boolean"}; 2214 case 1393530710: /*wednesday*/ return new String[] {"boolean"}; 2215 case 1572055514: /*thursday*/ return new String[] {"boolean"}; 2216 case -1266285217: /*friday*/ return new String[] {"boolean"}; 2217 case -2114201671: /*saturday*/ return new String[] {"boolean"}; 2218 case -891186736: /*sunday*/ return new String[] {"boolean"}; 2219 case -784550695: /*weekInterval*/ return new String[] {"positiveInt"}; 2220 default: return super.getTypesForProperty(hash, name); 2221 } 2222 2223 } 2224 2225 @Override 2226 public Base addChild(String name) throws FHIRException { 2227 if (name.equals("monday")) { 2228 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.monday"); 2229 } 2230 else if (name.equals("tuesday")) { 2231 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.tuesday"); 2232 } 2233 else if (name.equals("wednesday")) { 2234 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.wednesday"); 2235 } 2236 else if (name.equals("thursday")) { 2237 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.thursday"); 2238 } 2239 else if (name.equals("friday")) { 2240 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.friday"); 2241 } 2242 else if (name.equals("saturday")) { 2243 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.saturday"); 2244 } 2245 else if (name.equals("sunday")) { 2246 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.sunday"); 2247 } 2248 else if (name.equals("weekInterval")) { 2249 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.weeklyTemplate.weekInterval"); 2250 } 2251 else 2252 return super.addChild(name); 2253 } 2254 2255 public AppointmentRecurrenceTemplateWeeklyTemplateComponent copy() { 2256 AppointmentRecurrenceTemplateWeeklyTemplateComponent dst = new AppointmentRecurrenceTemplateWeeklyTemplateComponent(); 2257 copyValues(dst); 2258 return dst; 2259 } 2260 2261 public void copyValues(AppointmentRecurrenceTemplateWeeklyTemplateComponent dst) { 2262 super.copyValues(dst); 2263 dst.monday = monday == null ? null : monday.copy(); 2264 dst.tuesday = tuesday == null ? null : tuesday.copy(); 2265 dst.wednesday = wednesday == null ? null : wednesday.copy(); 2266 dst.thursday = thursday == null ? null : thursday.copy(); 2267 dst.friday = friday == null ? null : friday.copy(); 2268 dst.saturday = saturday == null ? null : saturday.copy(); 2269 dst.sunday = sunday == null ? null : sunday.copy(); 2270 dst.weekInterval = weekInterval == null ? null : weekInterval.copy(); 2271 } 2272 2273 @Override 2274 public boolean equalsDeep(Base other_) { 2275 if (!super.equalsDeep(other_)) 2276 return false; 2277 if (!(other_ instanceof AppointmentRecurrenceTemplateWeeklyTemplateComponent)) 2278 return false; 2279 AppointmentRecurrenceTemplateWeeklyTemplateComponent o = (AppointmentRecurrenceTemplateWeeklyTemplateComponent) other_; 2280 return compareDeep(monday, o.monday, true) && compareDeep(tuesday, o.tuesday, true) && compareDeep(wednesday, o.wednesday, true) 2281 && compareDeep(thursday, o.thursday, true) && compareDeep(friday, o.friday, true) && compareDeep(saturday, o.saturday, true) 2282 && compareDeep(sunday, o.sunday, true) && compareDeep(weekInterval, o.weekInterval, true); 2283 } 2284 2285 @Override 2286 public boolean equalsShallow(Base other_) { 2287 if (!super.equalsShallow(other_)) 2288 return false; 2289 if (!(other_ instanceof AppointmentRecurrenceTemplateWeeklyTemplateComponent)) 2290 return false; 2291 AppointmentRecurrenceTemplateWeeklyTemplateComponent o = (AppointmentRecurrenceTemplateWeeklyTemplateComponent) other_; 2292 return compareValues(monday, o.monday, true) && compareValues(tuesday, o.tuesday, true) && compareValues(wednesday, o.wednesday, true) 2293 && compareValues(thursday, o.thursday, true) && compareValues(friday, o.friday, true) && compareValues(saturday, o.saturday, true) 2294 && compareValues(sunday, o.sunday, true) && compareValues(weekInterval, o.weekInterval, true); 2295 } 2296 2297 public boolean isEmpty() { 2298 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(monday, tuesday, wednesday 2299 , thursday, friday, saturday, sunday, weekInterval); 2300 } 2301 2302 public String fhirType() { 2303 return "Appointment.recurrenceTemplate.weeklyTemplate"; 2304 2305 } 2306 2307 } 2308 2309 @Block() 2310 public static class AppointmentRecurrenceTemplateMonthlyTemplateComponent extends BackboneElement implements IBaseBackboneElement { 2311 /** 2312 * Indicates that appointments in the series of recurring appointments should occur on a specific day of the month. 2313 */ 2314 @Child(name = "dayOfMonth", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2315 @Description(shortDefinition="Recurs on a specific day of the month", formalDefinition="Indicates that appointments in the series of recurring appointments should occur on a specific day of the month." ) 2316 protected PositiveIntType dayOfMonth; 2317 2318 /** 2319 * Indicates which week within a month the appointments in the series of recurring appointments should occur on. 2320 */ 2321 @Child(name = "nthWeekOfMonth", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 2322 @Description(shortDefinition="Indicates which week of the month the appointment should occur", formalDefinition="Indicates which week within a month the appointments in the series of recurring appointments should occur on." ) 2323 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/week-of-month") 2324 protected Coding nthWeekOfMonth; 2325 2326 /** 2327 * Indicates which day of the week the recurring appointments should occur each nth week. 2328 */ 2329 @Child(name = "dayOfWeek", type = {Coding.class}, order=3, min=0, max=1, modifier=false, summary=false) 2330 @Description(shortDefinition="Indicates which day of the week the appointment should occur", formalDefinition="Indicates which day of the week the recurring appointments should occur each nth week." ) 2331 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 2332 protected Coding dayOfWeek; 2333 2334 /** 2335 * Indicates that recurring appointments should occur every nth month. 2336 */ 2337 @Child(name = "monthInterval", type = {PositiveIntType.class}, order=4, min=1, max=1, modifier=false, summary=false) 2338 @Description(shortDefinition="Recurs every nth month", formalDefinition="Indicates that recurring appointments should occur every nth month." ) 2339 protected PositiveIntType monthInterval; 2340 2341 private static final long serialVersionUID = -1234046272L; 2342 2343 /** 2344 * Constructor 2345 */ 2346 public AppointmentRecurrenceTemplateMonthlyTemplateComponent() { 2347 super(); 2348 } 2349 2350 /** 2351 * Constructor 2352 */ 2353 public AppointmentRecurrenceTemplateMonthlyTemplateComponent(int monthInterval) { 2354 super(); 2355 this.setMonthInterval(monthInterval); 2356 } 2357 2358 /** 2359 * @return {@link #dayOfMonth} (Indicates that appointments in the series of recurring appointments should occur on a specific day of the month.). This is the underlying object with id, value and extensions. The accessor "getDayOfMonth" gives direct access to the value 2360 */ 2361 public PositiveIntType getDayOfMonthElement() { 2362 if (this.dayOfMonth == null) 2363 if (Configuration.errorOnAutoCreate()) 2364 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateMonthlyTemplateComponent.dayOfMonth"); 2365 else if (Configuration.doAutoCreate()) 2366 this.dayOfMonth = new PositiveIntType(); // bb 2367 return this.dayOfMonth; 2368 } 2369 2370 public boolean hasDayOfMonthElement() { 2371 return this.dayOfMonth != null && !this.dayOfMonth.isEmpty(); 2372 } 2373 2374 public boolean hasDayOfMonth() { 2375 return this.dayOfMonth != null && !this.dayOfMonth.isEmpty(); 2376 } 2377 2378 /** 2379 * @param value {@link #dayOfMonth} (Indicates that appointments in the series of recurring appointments should occur on a specific day of the month.). This is the underlying object with id, value and extensions. The accessor "getDayOfMonth" gives direct access to the value 2380 */ 2381 public AppointmentRecurrenceTemplateMonthlyTemplateComponent setDayOfMonthElement(PositiveIntType value) { 2382 this.dayOfMonth = value; 2383 return this; 2384 } 2385 2386 /** 2387 * @return Indicates that appointments in the series of recurring appointments should occur on a specific day of the month. 2388 */ 2389 public int getDayOfMonth() { 2390 return this.dayOfMonth == null || this.dayOfMonth.isEmpty() ? 0 : this.dayOfMonth.getValue(); 2391 } 2392 2393 /** 2394 * @param value Indicates that appointments in the series of recurring appointments should occur on a specific day of the month. 2395 */ 2396 public AppointmentRecurrenceTemplateMonthlyTemplateComponent setDayOfMonth(int value) { 2397 if (this.dayOfMonth == null) 2398 this.dayOfMonth = new PositiveIntType(); 2399 this.dayOfMonth.setValue(value); 2400 return this; 2401 } 2402 2403 /** 2404 * @return {@link #nthWeekOfMonth} (Indicates which week within a month the appointments in the series of recurring appointments should occur on.) 2405 */ 2406 public Coding getNthWeekOfMonth() { 2407 if (this.nthWeekOfMonth == null) 2408 if (Configuration.errorOnAutoCreate()) 2409 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateMonthlyTemplateComponent.nthWeekOfMonth"); 2410 else if (Configuration.doAutoCreate()) 2411 this.nthWeekOfMonth = new Coding(); // cc 2412 return this.nthWeekOfMonth; 2413 } 2414 2415 public boolean hasNthWeekOfMonth() { 2416 return this.nthWeekOfMonth != null && !this.nthWeekOfMonth.isEmpty(); 2417 } 2418 2419 /** 2420 * @param value {@link #nthWeekOfMonth} (Indicates which week within a month the appointments in the series of recurring appointments should occur on.) 2421 */ 2422 public AppointmentRecurrenceTemplateMonthlyTemplateComponent setNthWeekOfMonth(Coding value) { 2423 this.nthWeekOfMonth = value; 2424 return this; 2425 } 2426 2427 /** 2428 * @return {@link #dayOfWeek} (Indicates which day of the week the recurring appointments should occur each nth week.) 2429 */ 2430 public Coding getDayOfWeek() { 2431 if (this.dayOfWeek == null) 2432 if (Configuration.errorOnAutoCreate()) 2433 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateMonthlyTemplateComponent.dayOfWeek"); 2434 else if (Configuration.doAutoCreate()) 2435 this.dayOfWeek = new Coding(); // cc 2436 return this.dayOfWeek; 2437 } 2438 2439 public boolean hasDayOfWeek() { 2440 return this.dayOfWeek != null && !this.dayOfWeek.isEmpty(); 2441 } 2442 2443 /** 2444 * @param value {@link #dayOfWeek} (Indicates which day of the week the recurring appointments should occur each nth week.) 2445 */ 2446 public AppointmentRecurrenceTemplateMonthlyTemplateComponent setDayOfWeek(Coding value) { 2447 this.dayOfWeek = value; 2448 return this; 2449 } 2450 2451 /** 2452 * @return {@link #monthInterval} (Indicates that recurring appointments should occur every nth month.). This is the underlying object with id, value and extensions. The accessor "getMonthInterval" gives direct access to the value 2453 */ 2454 public PositiveIntType getMonthIntervalElement() { 2455 if (this.monthInterval == null) 2456 if (Configuration.errorOnAutoCreate()) 2457 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateMonthlyTemplateComponent.monthInterval"); 2458 else if (Configuration.doAutoCreate()) 2459 this.monthInterval = new PositiveIntType(); // bb 2460 return this.monthInterval; 2461 } 2462 2463 public boolean hasMonthIntervalElement() { 2464 return this.monthInterval != null && !this.monthInterval.isEmpty(); 2465 } 2466 2467 public boolean hasMonthInterval() { 2468 return this.monthInterval != null && !this.monthInterval.isEmpty(); 2469 } 2470 2471 /** 2472 * @param value {@link #monthInterval} (Indicates that recurring appointments should occur every nth month.). This is the underlying object with id, value and extensions. The accessor "getMonthInterval" gives direct access to the value 2473 */ 2474 public AppointmentRecurrenceTemplateMonthlyTemplateComponent setMonthIntervalElement(PositiveIntType value) { 2475 this.monthInterval = value; 2476 return this; 2477 } 2478 2479 /** 2480 * @return Indicates that recurring appointments should occur every nth month. 2481 */ 2482 public int getMonthInterval() { 2483 return this.monthInterval == null || this.monthInterval.isEmpty() ? 0 : this.monthInterval.getValue(); 2484 } 2485 2486 /** 2487 * @param value Indicates that recurring appointments should occur every nth month. 2488 */ 2489 public AppointmentRecurrenceTemplateMonthlyTemplateComponent setMonthInterval(int value) { 2490 if (this.monthInterval == null) 2491 this.monthInterval = new PositiveIntType(); 2492 this.monthInterval.setValue(value); 2493 return this; 2494 } 2495 2496 protected void listChildren(List<Property> children) { 2497 super.listChildren(children); 2498 children.add(new Property("dayOfMonth", "positiveInt", "Indicates that appointments in the series of recurring appointments should occur on a specific day of the month.", 0, 1, dayOfMonth)); 2499 children.add(new Property("nthWeekOfMonth", "Coding", "Indicates which week within a month the appointments in the series of recurring appointments should occur on.", 0, 1, nthWeekOfMonth)); 2500 children.add(new Property("dayOfWeek", "Coding", "Indicates which day of the week the recurring appointments should occur each nth week.", 0, 1, dayOfWeek)); 2501 children.add(new Property("monthInterval", "positiveInt", "Indicates that recurring appointments should occur every nth month.", 0, 1, monthInterval)); 2502 } 2503 2504 @Override 2505 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2506 switch (_hash) { 2507 case -1181204563: /*dayOfMonth*/ return new Property("dayOfMonth", "positiveInt", "Indicates that appointments in the series of recurring appointments should occur on a specific day of the month.", 0, 1, dayOfMonth); 2508 case 724728723: /*nthWeekOfMonth*/ return new Property("nthWeekOfMonth", "Coding", "Indicates which week within a month the appointments in the series of recurring appointments should occur on.", 0, 1, nthWeekOfMonth); 2509 case -730552025: /*dayOfWeek*/ return new Property("dayOfWeek", "Coding", "Indicates which day of the week the recurring appointments should occur each nth week.", 0, 1, dayOfWeek); 2510 case -251401371: /*monthInterval*/ return new Property("monthInterval", "positiveInt", "Indicates that recurring appointments should occur every nth month.", 0, 1, monthInterval); 2511 default: return super.getNamedProperty(_hash, _name, _checkValid); 2512 } 2513 2514 } 2515 2516 @Override 2517 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2518 switch (hash) { 2519 case -1181204563: /*dayOfMonth*/ return this.dayOfMonth == null ? new Base[0] : new Base[] {this.dayOfMonth}; // PositiveIntType 2520 case 724728723: /*nthWeekOfMonth*/ return this.nthWeekOfMonth == null ? new Base[0] : new Base[] {this.nthWeekOfMonth}; // Coding 2521 case -730552025: /*dayOfWeek*/ return this.dayOfWeek == null ? new Base[0] : new Base[] {this.dayOfWeek}; // Coding 2522 case -251401371: /*monthInterval*/ return this.monthInterval == null ? new Base[0] : new Base[] {this.monthInterval}; // PositiveIntType 2523 default: return super.getProperty(hash, name, checkValid); 2524 } 2525 2526 } 2527 2528 @Override 2529 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2530 switch (hash) { 2531 case -1181204563: // dayOfMonth 2532 this.dayOfMonth = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2533 return value; 2534 case 724728723: // nthWeekOfMonth 2535 this.nthWeekOfMonth = TypeConvertor.castToCoding(value); // Coding 2536 return value; 2537 case -730552025: // dayOfWeek 2538 this.dayOfWeek = TypeConvertor.castToCoding(value); // Coding 2539 return value; 2540 case -251401371: // monthInterval 2541 this.monthInterval = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2542 return value; 2543 default: return super.setProperty(hash, name, value); 2544 } 2545 2546 } 2547 2548 @Override 2549 public Base setProperty(String name, Base value) throws FHIRException { 2550 if (name.equals("dayOfMonth")) { 2551 this.dayOfMonth = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2552 } else if (name.equals("nthWeekOfMonth")) { 2553 this.nthWeekOfMonth = TypeConvertor.castToCoding(value); // Coding 2554 } else if (name.equals("dayOfWeek")) { 2555 this.dayOfWeek = TypeConvertor.castToCoding(value); // Coding 2556 } else if (name.equals("monthInterval")) { 2557 this.monthInterval = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2558 } else 2559 return super.setProperty(name, value); 2560 return value; 2561 } 2562 2563 @Override 2564 public void removeChild(String name, Base value) throws FHIRException { 2565 if (name.equals("dayOfMonth")) { 2566 this.dayOfMonth = null; 2567 } else if (name.equals("nthWeekOfMonth")) { 2568 this.nthWeekOfMonth = null; 2569 } else if (name.equals("dayOfWeek")) { 2570 this.dayOfWeek = null; 2571 } else if (name.equals("monthInterval")) { 2572 this.monthInterval = null; 2573 } else 2574 super.removeChild(name, value); 2575 2576 } 2577 2578 @Override 2579 public Base makeProperty(int hash, String name) throws FHIRException { 2580 switch (hash) { 2581 case -1181204563: return getDayOfMonthElement(); 2582 case 724728723: return getNthWeekOfMonth(); 2583 case -730552025: return getDayOfWeek(); 2584 case -251401371: return getMonthIntervalElement(); 2585 default: return super.makeProperty(hash, name); 2586 } 2587 2588 } 2589 2590 @Override 2591 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2592 switch (hash) { 2593 case -1181204563: /*dayOfMonth*/ return new String[] {"positiveInt"}; 2594 case 724728723: /*nthWeekOfMonth*/ return new String[] {"Coding"}; 2595 case -730552025: /*dayOfWeek*/ return new String[] {"Coding"}; 2596 case -251401371: /*monthInterval*/ return new String[] {"positiveInt"}; 2597 default: return super.getTypesForProperty(hash, name); 2598 } 2599 2600 } 2601 2602 @Override 2603 public Base addChild(String name) throws FHIRException { 2604 if (name.equals("dayOfMonth")) { 2605 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.monthlyTemplate.dayOfMonth"); 2606 } 2607 else if (name.equals("nthWeekOfMonth")) { 2608 this.nthWeekOfMonth = new Coding(); 2609 return this.nthWeekOfMonth; 2610 } 2611 else if (name.equals("dayOfWeek")) { 2612 this.dayOfWeek = new Coding(); 2613 return this.dayOfWeek; 2614 } 2615 else if (name.equals("monthInterval")) { 2616 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.monthlyTemplate.monthInterval"); 2617 } 2618 else 2619 return super.addChild(name); 2620 } 2621 2622 public AppointmentRecurrenceTemplateMonthlyTemplateComponent copy() { 2623 AppointmentRecurrenceTemplateMonthlyTemplateComponent dst = new AppointmentRecurrenceTemplateMonthlyTemplateComponent(); 2624 copyValues(dst); 2625 return dst; 2626 } 2627 2628 public void copyValues(AppointmentRecurrenceTemplateMonthlyTemplateComponent dst) { 2629 super.copyValues(dst); 2630 dst.dayOfMonth = dayOfMonth == null ? null : dayOfMonth.copy(); 2631 dst.nthWeekOfMonth = nthWeekOfMonth == null ? null : nthWeekOfMonth.copy(); 2632 dst.dayOfWeek = dayOfWeek == null ? null : dayOfWeek.copy(); 2633 dst.monthInterval = monthInterval == null ? null : monthInterval.copy(); 2634 } 2635 2636 @Override 2637 public boolean equalsDeep(Base other_) { 2638 if (!super.equalsDeep(other_)) 2639 return false; 2640 if (!(other_ instanceof AppointmentRecurrenceTemplateMonthlyTemplateComponent)) 2641 return false; 2642 AppointmentRecurrenceTemplateMonthlyTemplateComponent o = (AppointmentRecurrenceTemplateMonthlyTemplateComponent) other_; 2643 return compareDeep(dayOfMonth, o.dayOfMonth, true) && compareDeep(nthWeekOfMonth, o.nthWeekOfMonth, true) 2644 && compareDeep(dayOfWeek, o.dayOfWeek, true) && compareDeep(monthInterval, o.monthInterval, true) 2645 ; 2646 } 2647 2648 @Override 2649 public boolean equalsShallow(Base other_) { 2650 if (!super.equalsShallow(other_)) 2651 return false; 2652 if (!(other_ instanceof AppointmentRecurrenceTemplateMonthlyTemplateComponent)) 2653 return false; 2654 AppointmentRecurrenceTemplateMonthlyTemplateComponent o = (AppointmentRecurrenceTemplateMonthlyTemplateComponent) other_; 2655 return compareValues(dayOfMonth, o.dayOfMonth, true) && compareValues(monthInterval, o.monthInterval, true) 2656 ; 2657 } 2658 2659 public boolean isEmpty() { 2660 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(dayOfMonth, nthWeekOfMonth 2661 , dayOfWeek, monthInterval); 2662 } 2663 2664 public String fhirType() { 2665 return "Appointment.recurrenceTemplate.monthlyTemplate"; 2666 2667 } 2668 2669 } 2670 2671 @Block() 2672 public static class AppointmentRecurrenceTemplateYearlyTemplateComponent extends BackboneElement implements IBaseBackboneElement { 2673 /** 2674 * Appointment recurs every nth year. 2675 */ 2676 @Child(name = "yearInterval", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2677 @Description(shortDefinition="Recurs every nth year", formalDefinition="Appointment recurs every nth year." ) 2678 protected PositiveIntType yearInterval; 2679 2680 private static final long serialVersionUID = -120794476L; 2681 2682 /** 2683 * Constructor 2684 */ 2685 public AppointmentRecurrenceTemplateYearlyTemplateComponent() { 2686 super(); 2687 } 2688 2689 /** 2690 * Constructor 2691 */ 2692 public AppointmentRecurrenceTemplateYearlyTemplateComponent(int yearInterval) { 2693 super(); 2694 this.setYearInterval(yearInterval); 2695 } 2696 2697 /** 2698 * @return {@link #yearInterval} (Appointment recurs every nth year.). This is the underlying object with id, value and extensions. The accessor "getYearInterval" gives direct access to the value 2699 */ 2700 public PositiveIntType getYearIntervalElement() { 2701 if (this.yearInterval == null) 2702 if (Configuration.errorOnAutoCreate()) 2703 throw new Error("Attempt to auto-create AppointmentRecurrenceTemplateYearlyTemplateComponent.yearInterval"); 2704 else if (Configuration.doAutoCreate()) 2705 this.yearInterval = new PositiveIntType(); // bb 2706 return this.yearInterval; 2707 } 2708 2709 public boolean hasYearIntervalElement() { 2710 return this.yearInterval != null && !this.yearInterval.isEmpty(); 2711 } 2712 2713 public boolean hasYearInterval() { 2714 return this.yearInterval != null && !this.yearInterval.isEmpty(); 2715 } 2716 2717 /** 2718 * @param value {@link #yearInterval} (Appointment recurs every nth year.). This is the underlying object with id, value and extensions. The accessor "getYearInterval" gives direct access to the value 2719 */ 2720 public AppointmentRecurrenceTemplateYearlyTemplateComponent setYearIntervalElement(PositiveIntType value) { 2721 this.yearInterval = value; 2722 return this; 2723 } 2724 2725 /** 2726 * @return Appointment recurs every nth year. 2727 */ 2728 public int getYearInterval() { 2729 return this.yearInterval == null || this.yearInterval.isEmpty() ? 0 : this.yearInterval.getValue(); 2730 } 2731 2732 /** 2733 * @param value Appointment recurs every nth year. 2734 */ 2735 public AppointmentRecurrenceTemplateYearlyTemplateComponent setYearInterval(int value) { 2736 if (this.yearInterval == null) 2737 this.yearInterval = new PositiveIntType(); 2738 this.yearInterval.setValue(value); 2739 return this; 2740 } 2741 2742 protected void listChildren(List<Property> children) { 2743 super.listChildren(children); 2744 children.add(new Property("yearInterval", "positiveInt", "Appointment recurs every nth year.", 0, 1, yearInterval)); 2745 } 2746 2747 @Override 2748 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2749 switch (_hash) { 2750 case 492389410: /*yearInterval*/ return new Property("yearInterval", "positiveInt", "Appointment recurs every nth year.", 0, 1, yearInterval); 2751 default: return super.getNamedProperty(_hash, _name, _checkValid); 2752 } 2753 2754 } 2755 2756 @Override 2757 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2758 switch (hash) { 2759 case 492389410: /*yearInterval*/ return this.yearInterval == null ? new Base[0] : new Base[] {this.yearInterval}; // PositiveIntType 2760 default: return super.getProperty(hash, name, checkValid); 2761 } 2762 2763 } 2764 2765 @Override 2766 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2767 switch (hash) { 2768 case 492389410: // yearInterval 2769 this.yearInterval = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2770 return value; 2771 default: return super.setProperty(hash, name, value); 2772 } 2773 2774 } 2775 2776 @Override 2777 public Base setProperty(String name, Base value) throws FHIRException { 2778 if (name.equals("yearInterval")) { 2779 this.yearInterval = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2780 } else 2781 return super.setProperty(name, value); 2782 return value; 2783 } 2784 2785 @Override 2786 public void removeChild(String name, Base value) throws FHIRException { 2787 if (name.equals("yearInterval")) { 2788 this.yearInterval = null; 2789 } else 2790 super.removeChild(name, value); 2791 2792 } 2793 2794 @Override 2795 public Base makeProperty(int hash, String name) throws FHIRException { 2796 switch (hash) { 2797 case 492389410: return getYearIntervalElement(); 2798 default: return super.makeProperty(hash, name); 2799 } 2800 2801 } 2802 2803 @Override 2804 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2805 switch (hash) { 2806 case 492389410: /*yearInterval*/ return new String[] {"positiveInt"}; 2807 default: return super.getTypesForProperty(hash, name); 2808 } 2809 2810 } 2811 2812 @Override 2813 public Base addChild(String name) throws FHIRException { 2814 if (name.equals("yearInterval")) { 2815 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceTemplate.yearlyTemplate.yearInterval"); 2816 } 2817 else 2818 return super.addChild(name); 2819 } 2820 2821 public AppointmentRecurrenceTemplateYearlyTemplateComponent copy() { 2822 AppointmentRecurrenceTemplateYearlyTemplateComponent dst = new AppointmentRecurrenceTemplateYearlyTemplateComponent(); 2823 copyValues(dst); 2824 return dst; 2825 } 2826 2827 public void copyValues(AppointmentRecurrenceTemplateYearlyTemplateComponent dst) { 2828 super.copyValues(dst); 2829 dst.yearInterval = yearInterval == null ? null : yearInterval.copy(); 2830 } 2831 2832 @Override 2833 public boolean equalsDeep(Base other_) { 2834 if (!super.equalsDeep(other_)) 2835 return false; 2836 if (!(other_ instanceof AppointmentRecurrenceTemplateYearlyTemplateComponent)) 2837 return false; 2838 AppointmentRecurrenceTemplateYearlyTemplateComponent o = (AppointmentRecurrenceTemplateYearlyTemplateComponent) other_; 2839 return compareDeep(yearInterval, o.yearInterval, true); 2840 } 2841 2842 @Override 2843 public boolean equalsShallow(Base other_) { 2844 if (!super.equalsShallow(other_)) 2845 return false; 2846 if (!(other_ instanceof AppointmentRecurrenceTemplateYearlyTemplateComponent)) 2847 return false; 2848 AppointmentRecurrenceTemplateYearlyTemplateComponent o = (AppointmentRecurrenceTemplateYearlyTemplateComponent) other_; 2849 return compareValues(yearInterval, o.yearInterval, true); 2850 } 2851 2852 public boolean isEmpty() { 2853 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(yearInterval); 2854 } 2855 2856 public String fhirType() { 2857 return "Appointment.recurrenceTemplate.yearlyTemplate"; 2858 2859 } 2860 2861 } 2862 2863 /** 2864 * This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 2865 */ 2866 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2867 @Description(shortDefinition="External Ids for this item", formalDefinition="This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 2868 protected List<Identifier> identifier; 2869 2870 /** 2871 * The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status. 2872 */ 2873 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2874 @Description(shortDefinition="proposed | pending | booked | arrived | fulfilled | cancelled | noshow | entered-in-error | checked-in | waitlist", formalDefinition="The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status." ) 2875 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/appointmentstatus") 2876 protected Enumeration<AppointmentStatus> status; 2877 2878 /** 2879 * The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply. 2880 */ 2881 @Child(name = "cancellationReason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2882 @Description(shortDefinition="The coded reason for the appointment being cancelled", formalDefinition="The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply." ) 2883 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/appointment-cancellation-reason") 2884 protected CodeableConcept cancellationReason; 2885 2886 /** 2887 * Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations. 2888 */ 2889 @Child(name = "class", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2890 @Description(shortDefinition="Classification when becoming an encounter", formalDefinition="Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations." ) 2891 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/EncounterClass") 2892 protected List<CodeableConcept> class_; 2893 2894 /** 2895 * A broad categorization of the service that is to be performed during this appointment. 2896 */ 2897 @Child(name = "serviceCategory", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2898 @Description(shortDefinition="A broad categorization of the service that is to be performed during this appointment", formalDefinition="A broad categorization of the service that is to be performed during this appointment." ) 2899 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 2900 protected List<CodeableConcept> serviceCategory; 2901 2902 /** 2903 * The specific service that is to be performed during this appointment. 2904 */ 2905 @Child(name = "serviceType", type = {CodeableReference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2906 @Description(shortDefinition="The specific service that is to be performed during this appointment", formalDefinition="The specific service that is to be performed during this appointment." ) 2907 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 2908 protected List<CodeableReference> serviceType; 2909 2910 /** 2911 * The specialty of a practitioner that would be required to perform the service requested in this appointment. 2912 */ 2913 @Child(name = "specialty", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2914 @Description(shortDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment." ) 2915 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 2916 protected List<CodeableConcept> specialty; 2917 2918 /** 2919 * The style of appointment or patient that has been booked in the slot (not service type). 2920 */ 2921 @Child(name = "appointmentType", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 2922 @Description(shortDefinition="The style of appointment or patient that has been booked in the slot (not service type)", formalDefinition="The style of appointment or patient that has been booked in the slot (not service type)." ) 2923 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0276") 2924 protected CodeableConcept appointmentType; 2925 2926 /** 2927 * The reason that this appointment is being scheduled. This is more clinical than administrative. This can be coded, or as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure. 2928 */ 2929 @Child(name = "reason", type = {CodeableReference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2930 @Description(shortDefinition="Reason this appointment is scheduled", formalDefinition="The reason that this appointment is being scheduled. This is more clinical than administrative. This can be coded, or as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure." ) 2931 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason") 2932 protected List<CodeableReference> reason; 2933 2934 /** 2935 * The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority). 2936 */ 2937 @Child(name = "priority", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 2938 @Description(shortDefinition="Used to make informed decisions if needing to re-prioritize", formalDefinition="The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority)." ) 2939 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActPriority") 2940 protected CodeableConcept priority; 2941 2942 /** 2943 * The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field. 2944 */ 2945 @Child(name = "description", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 2946 @Description(shortDefinition="Shown on a subject line in a meeting request, or appointment list", formalDefinition="The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field." ) 2947 protected StringType description; 2948 2949 /** 2950 * Appointment replaced by this Appointment in cases where there is a cancellation, the details of the cancellation can be found in the cancellationReason property (on the referenced resource). 2951 */ 2952 @Child(name = "replaces", type = {Appointment.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2953 @Description(shortDefinition="Appointment replaced by this Appointment", formalDefinition="Appointment replaced by this Appointment in cases where there is a cancellation, the details of the cancellation can be found in the cancellationReason property (on the referenced resource)." ) 2954 protected List<Reference> replaces; 2955 2956 /** 2957 * Connection details of a virtual service (e.g. conference call). 2958 */ 2959 @Child(name = "virtualService", type = {VirtualServiceDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2960 @Description(shortDefinition="Connection details of a virtual service (e.g. conference call)", formalDefinition="Connection details of a virtual service (e.g. conference call)." ) 2961 protected List<VirtualServiceDetail> virtualService; 2962 2963 /** 2964 * Additional information to support the appointment provided when making the appointment. 2965 */ 2966 @Child(name = "supportingInformation", type = {Reference.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2967 @Description(shortDefinition="Additional information to support the appointment", formalDefinition="Additional information to support the appointment provided when making the appointment." ) 2968 protected List<Reference> supportingInformation; 2969 2970 /** 2971 * The previous appointment in a series of related appointments. 2972 */ 2973 @Child(name = "previousAppointment", type = {Appointment.class}, order=14, min=0, max=1, modifier=false, summary=false) 2974 @Description(shortDefinition="The previous appointment in a series", formalDefinition="The previous appointment in a series of related appointments." ) 2975 protected Reference previousAppointment; 2976 2977 /** 2978 * The originating appointment in a recurring set of related appointments. 2979 */ 2980 @Child(name = "originatingAppointment", type = {Appointment.class}, order=15, min=0, max=1, modifier=false, summary=false) 2981 @Description(shortDefinition="The originating appointment in a recurring set of appointments", formalDefinition="The originating appointment in a recurring set of related appointments." ) 2982 protected Reference originatingAppointment; 2983 2984 /** 2985 * Date/Time that the appointment is to take place. 2986 */ 2987 @Child(name = "start", type = {InstantType.class}, order=16, min=0, max=1, modifier=false, summary=true) 2988 @Description(shortDefinition="When appointment is to take place", formalDefinition="Date/Time that the appointment is to take place." ) 2989 protected InstantType start; 2990 2991 /** 2992 * Date/Time that the appointment is to conclude. 2993 */ 2994 @Child(name = "end", type = {InstantType.class}, order=17, min=0, max=1, modifier=false, summary=true) 2995 @Description(shortDefinition="When appointment is to conclude", formalDefinition="Date/Time that the appointment is to conclude." ) 2996 protected InstantType end; 2997 2998 /** 2999 * Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end. 3000 */ 3001 @Child(name = "minutesDuration", type = {PositiveIntType.class}, order=18, min=0, max=1, modifier=false, summary=false) 3002 @Description(shortDefinition="Can be less than start/end (e.g. estimate)", formalDefinition="Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end." ) 3003 protected PositiveIntType minutesDuration; 3004 3005 /** 3006 * A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. 3007 3008The duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system. 3009 */ 3010 @Child(name = "requestedPeriod", type = {Period.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3011 @Description(shortDefinition="Potential date/time interval(s) requested to allocate the appointment within", formalDefinition="A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system." ) 3012 protected List<Period> requestedPeriod; 3013 3014 /** 3015 * The slots from the participants' schedules that will be filled by the appointment. 3016 */ 3017 @Child(name = "slot", type = {Slot.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3018 @Description(shortDefinition="The slots that this appointment is filling", formalDefinition="The slots from the participants' schedules that will be filled by the appointment." ) 3019 protected List<Reference> slot; 3020 3021 /** 3022 * The set of accounts that is expected to be used for billing the activities that result from this Appointment. 3023 */ 3024 @Child(name = "account", type = {Account.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3025 @Description(shortDefinition="The set of accounts that may be used for billing for this Appointment", formalDefinition="The set of accounts that is expected to be used for billing the activities that result from this Appointment." ) 3026 protected List<Reference> account; 3027 3028 /** 3029 * The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment. 3030 */ 3031 @Child(name = "created", type = {DateTimeType.class}, order=22, min=0, max=1, modifier=false, summary=false) 3032 @Description(shortDefinition="The date that this appointment was initially created", formalDefinition="The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment." ) 3033 protected DateTimeType created; 3034 3035 /** 3036 * The date/time describing when the appointment was cancelled. 3037 */ 3038 @Child(name = "cancellationDate", type = {DateTimeType.class}, order=23, min=0, max=1, modifier=false, summary=false) 3039 @Description(shortDefinition="When the appointment was cancelled", formalDefinition="The date/time describing when the appointment was cancelled." ) 3040 protected DateTimeType cancellationDate; 3041 3042 /** 3043 * Additional notes/comments about the appointment. 3044 */ 3045 @Child(name = "note", type = {Annotation.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3046 @Description(shortDefinition="Additional comments", formalDefinition="Additional notes/comments about the appointment." ) 3047 protected List<Annotation> note; 3048 3049 /** 3050 * While Appointment.note contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before). 3051 */ 3052 @Child(name = "patientInstruction", type = {CodeableReference.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3053 @Description(shortDefinition="Detailed information and instructions for the patient", formalDefinition="While Appointment.note contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before)." ) 3054 protected List<CodeableReference> patientInstruction; 3055 3056 /** 3057 * The request this appointment is allocated to assess (e.g. incoming referral or procedure request). 3058 */ 3059 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, MedicationRequest.class, ServiceRequest.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3060 @Description(shortDefinition="The request this appointment is allocated to assess", formalDefinition="The request this appointment is allocated to assess (e.g. incoming referral or procedure request)." ) 3061 protected List<Reference> basedOn; 3062 3063 /** 3064 * The patient or group associated with the appointment, if they are to be present (usually) then they should also be included in the participant backbone element. 3065 */ 3066 @Child(name = "subject", type = {Patient.class, Group.class}, order=27, min=0, max=1, modifier=false, summary=true) 3067 @Description(shortDefinition="The patient or group associated with the appointment", formalDefinition="The patient or group associated with the appointment, if they are to be present (usually) then they should also be included in the participant backbone element." ) 3068 protected Reference subject; 3069 3070 /** 3071 * List of participants involved in the appointment. 3072 */ 3073 @Child(name = "participant", type = {}, order=28, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3074 @Description(shortDefinition="Participants involved in appointment", formalDefinition="List of participants involved in the appointment." ) 3075 protected List<AppointmentParticipantComponent> participant; 3076 3077 /** 3078 * The sequence number that identifies a specific appointment in a recurring pattern. 3079 */ 3080 @Child(name = "recurrenceId", type = {PositiveIntType.class}, order=29, min=0, max=1, modifier=false, summary=false) 3081 @Description(shortDefinition="The sequence number in the recurrence", formalDefinition="The sequence number that identifies a specific appointment in a recurring pattern." ) 3082 protected PositiveIntType recurrenceId; 3083 3084 /** 3085 * This appointment varies from the recurring pattern. 3086 */ 3087 @Child(name = "occurrenceChanged", type = {BooleanType.class}, order=30, min=0, max=1, modifier=false, summary=false) 3088 @Description(shortDefinition="Indicates that this appointment varies from a recurrence pattern", formalDefinition="This appointment varies from the recurring pattern." ) 3089 protected BooleanType occurrenceChanged; 3090 3091 /** 3092 * The details of the recurrence pattern or template that is used to generate recurring appointments. 3093 */ 3094 @Child(name = "recurrenceTemplate", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3095 @Description(shortDefinition="Details of the recurrence pattern/template used to generate occurrences", formalDefinition="The details of the recurrence pattern or template that is used to generate recurring appointments." ) 3096 protected List<AppointmentRecurrenceTemplateComponent> recurrenceTemplate; 3097 3098 private static final long serialVersionUID = 883088259L; 3099 3100 /** 3101 * Constructor 3102 */ 3103 public Appointment() { 3104 super(); 3105 } 3106 3107 /** 3108 * Constructor 3109 */ 3110 public Appointment(AppointmentStatus status, AppointmentParticipantComponent participant) { 3111 super(); 3112 this.setStatus(status); 3113 this.addParticipant(participant); 3114 } 3115 3116 /** 3117 * @return {@link #identifier} (This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 3118 */ 3119 public List<Identifier> getIdentifier() { 3120 if (this.identifier == null) 3121 this.identifier = new ArrayList<Identifier>(); 3122 return this.identifier; 3123 } 3124 3125 /** 3126 * @return Returns a reference to <code>this</code> for easy method chaining 3127 */ 3128 public Appointment setIdentifier(List<Identifier> theIdentifier) { 3129 this.identifier = theIdentifier; 3130 return this; 3131 } 3132 3133 public boolean hasIdentifier() { 3134 if (this.identifier == null) 3135 return false; 3136 for (Identifier item : this.identifier) 3137 if (!item.isEmpty()) 3138 return true; 3139 return false; 3140 } 3141 3142 public Identifier addIdentifier() { //3 3143 Identifier t = new Identifier(); 3144 if (this.identifier == null) 3145 this.identifier = new ArrayList<Identifier>(); 3146 this.identifier.add(t); 3147 return t; 3148 } 3149 3150 public Appointment addIdentifier(Identifier t) { //3 3151 if (t == null) 3152 return this; 3153 if (this.identifier == null) 3154 this.identifier = new ArrayList<Identifier>(); 3155 this.identifier.add(t); 3156 return this; 3157 } 3158 3159 /** 3160 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3161 */ 3162 public Identifier getIdentifierFirstRep() { 3163 if (getIdentifier().isEmpty()) { 3164 addIdentifier(); 3165 } 3166 return getIdentifier().get(0); 3167 } 3168 3169 /** 3170 * @return {@link #status} (The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3171 */ 3172 public Enumeration<AppointmentStatus> getStatusElement() { 3173 if (this.status == null) 3174 if (Configuration.errorOnAutoCreate()) 3175 throw new Error("Attempt to auto-create Appointment.status"); 3176 else if (Configuration.doAutoCreate()) 3177 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); // bb 3178 return this.status; 3179 } 3180 3181 public boolean hasStatusElement() { 3182 return this.status != null && !this.status.isEmpty(); 3183 } 3184 3185 public boolean hasStatus() { 3186 return this.status != null && !this.status.isEmpty(); 3187 } 3188 3189 /** 3190 * @param value {@link #status} (The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3191 */ 3192 public Appointment setStatusElement(Enumeration<AppointmentStatus> value) { 3193 this.status = value; 3194 return this; 3195 } 3196 3197 /** 3198 * @return The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status. 3199 */ 3200 public AppointmentStatus getStatus() { 3201 return this.status == null ? null : this.status.getValue(); 3202 } 3203 3204 /** 3205 * @param value The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status. 3206 */ 3207 public Appointment setStatus(AppointmentStatus value) { 3208 if (this.status == null) 3209 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); 3210 this.status.setValue(value); 3211 return this; 3212 } 3213 3214 /** 3215 * @return {@link #cancellationReason} (The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.) 3216 */ 3217 public CodeableConcept getCancellationReason() { 3218 if (this.cancellationReason == null) 3219 if (Configuration.errorOnAutoCreate()) 3220 throw new Error("Attempt to auto-create Appointment.cancellationReason"); 3221 else if (Configuration.doAutoCreate()) 3222 this.cancellationReason = new CodeableConcept(); // cc 3223 return this.cancellationReason; 3224 } 3225 3226 public boolean hasCancellationReason() { 3227 return this.cancellationReason != null && !this.cancellationReason.isEmpty(); 3228 } 3229 3230 /** 3231 * @param value {@link #cancellationReason} (The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.) 3232 */ 3233 public Appointment setCancellationReason(CodeableConcept value) { 3234 this.cancellationReason = value; 3235 return this; 3236 } 3237 3238 /** 3239 * @return {@link #class_} (Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.) 3240 */ 3241 public List<CodeableConcept> getClass_() { 3242 if (this.class_ == null) 3243 this.class_ = new ArrayList<CodeableConcept>(); 3244 return this.class_; 3245 } 3246 3247 /** 3248 * @return Returns a reference to <code>this</code> for easy method chaining 3249 */ 3250 public Appointment setClass_(List<CodeableConcept> theClass_) { 3251 this.class_ = theClass_; 3252 return this; 3253 } 3254 3255 public boolean hasClass_() { 3256 if (this.class_ == null) 3257 return false; 3258 for (CodeableConcept item : this.class_) 3259 if (!item.isEmpty()) 3260 return true; 3261 return false; 3262 } 3263 3264 public CodeableConcept addClass_() { //3 3265 CodeableConcept t = new CodeableConcept(); 3266 if (this.class_ == null) 3267 this.class_ = new ArrayList<CodeableConcept>(); 3268 this.class_.add(t); 3269 return t; 3270 } 3271 3272 public Appointment addClass_(CodeableConcept t) { //3 3273 if (t == null) 3274 return this; 3275 if (this.class_ == null) 3276 this.class_ = new ArrayList<CodeableConcept>(); 3277 this.class_.add(t); 3278 return this; 3279 } 3280 3281 /** 3282 * @return The first repetition of repeating field {@link #class_}, creating it if it does not already exist {3} 3283 */ 3284 public CodeableConcept getClass_FirstRep() { 3285 if (getClass_().isEmpty()) { 3286 addClass_(); 3287 } 3288 return getClass_().get(0); 3289 } 3290 3291 /** 3292 * @return {@link #serviceCategory} (A broad categorization of the service that is to be performed during this appointment.) 3293 */ 3294 public List<CodeableConcept> getServiceCategory() { 3295 if (this.serviceCategory == null) 3296 this.serviceCategory = new ArrayList<CodeableConcept>(); 3297 return this.serviceCategory; 3298 } 3299 3300 /** 3301 * @return Returns a reference to <code>this</code> for easy method chaining 3302 */ 3303 public Appointment setServiceCategory(List<CodeableConcept> theServiceCategory) { 3304 this.serviceCategory = theServiceCategory; 3305 return this; 3306 } 3307 3308 public boolean hasServiceCategory() { 3309 if (this.serviceCategory == null) 3310 return false; 3311 for (CodeableConcept item : this.serviceCategory) 3312 if (!item.isEmpty()) 3313 return true; 3314 return false; 3315 } 3316 3317 public CodeableConcept addServiceCategory() { //3 3318 CodeableConcept t = new CodeableConcept(); 3319 if (this.serviceCategory == null) 3320 this.serviceCategory = new ArrayList<CodeableConcept>(); 3321 this.serviceCategory.add(t); 3322 return t; 3323 } 3324 3325 public Appointment addServiceCategory(CodeableConcept t) { //3 3326 if (t == null) 3327 return this; 3328 if (this.serviceCategory == null) 3329 this.serviceCategory = new ArrayList<CodeableConcept>(); 3330 this.serviceCategory.add(t); 3331 return this; 3332 } 3333 3334 /** 3335 * @return The first repetition of repeating field {@link #serviceCategory}, creating it if it does not already exist {3} 3336 */ 3337 public CodeableConcept getServiceCategoryFirstRep() { 3338 if (getServiceCategory().isEmpty()) { 3339 addServiceCategory(); 3340 } 3341 return getServiceCategory().get(0); 3342 } 3343 3344 /** 3345 * @return {@link #serviceType} (The specific service that is to be performed during this appointment.) 3346 */ 3347 public List<CodeableReference> getServiceType() { 3348 if (this.serviceType == null) 3349 this.serviceType = new ArrayList<CodeableReference>(); 3350 return this.serviceType; 3351 } 3352 3353 /** 3354 * @return Returns a reference to <code>this</code> for easy method chaining 3355 */ 3356 public Appointment setServiceType(List<CodeableReference> theServiceType) { 3357 this.serviceType = theServiceType; 3358 return this; 3359 } 3360 3361 public boolean hasServiceType() { 3362 if (this.serviceType == null) 3363 return false; 3364 for (CodeableReference item : this.serviceType) 3365 if (!item.isEmpty()) 3366 return true; 3367 return false; 3368 } 3369 3370 public CodeableReference addServiceType() { //3 3371 CodeableReference t = new CodeableReference(); 3372 if (this.serviceType == null) 3373 this.serviceType = new ArrayList<CodeableReference>(); 3374 this.serviceType.add(t); 3375 return t; 3376 } 3377 3378 public Appointment addServiceType(CodeableReference t) { //3 3379 if (t == null) 3380 return this; 3381 if (this.serviceType == null) 3382 this.serviceType = new ArrayList<CodeableReference>(); 3383 this.serviceType.add(t); 3384 return this; 3385 } 3386 3387 /** 3388 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist {3} 3389 */ 3390 public CodeableReference getServiceTypeFirstRep() { 3391 if (getServiceType().isEmpty()) { 3392 addServiceType(); 3393 } 3394 return getServiceType().get(0); 3395 } 3396 3397 /** 3398 * @return {@link #specialty} (The specialty of a practitioner that would be required to perform the service requested in this appointment.) 3399 */ 3400 public List<CodeableConcept> getSpecialty() { 3401 if (this.specialty == null) 3402 this.specialty = new ArrayList<CodeableConcept>(); 3403 return this.specialty; 3404 } 3405 3406 /** 3407 * @return Returns a reference to <code>this</code> for easy method chaining 3408 */ 3409 public Appointment setSpecialty(List<CodeableConcept> theSpecialty) { 3410 this.specialty = theSpecialty; 3411 return this; 3412 } 3413 3414 public boolean hasSpecialty() { 3415 if (this.specialty == null) 3416 return false; 3417 for (CodeableConcept item : this.specialty) 3418 if (!item.isEmpty()) 3419 return true; 3420 return false; 3421 } 3422 3423 public CodeableConcept addSpecialty() { //3 3424 CodeableConcept t = new CodeableConcept(); 3425 if (this.specialty == null) 3426 this.specialty = new ArrayList<CodeableConcept>(); 3427 this.specialty.add(t); 3428 return t; 3429 } 3430 3431 public Appointment addSpecialty(CodeableConcept t) { //3 3432 if (t == null) 3433 return this; 3434 if (this.specialty == null) 3435 this.specialty = new ArrayList<CodeableConcept>(); 3436 this.specialty.add(t); 3437 return this; 3438 } 3439 3440 /** 3441 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist {3} 3442 */ 3443 public CodeableConcept getSpecialtyFirstRep() { 3444 if (getSpecialty().isEmpty()) { 3445 addSpecialty(); 3446 } 3447 return getSpecialty().get(0); 3448 } 3449 3450 /** 3451 * @return {@link #appointmentType} (The style of appointment or patient that has been booked in the slot (not service type).) 3452 */ 3453 public CodeableConcept getAppointmentType() { 3454 if (this.appointmentType == null) 3455 if (Configuration.errorOnAutoCreate()) 3456 throw new Error("Attempt to auto-create Appointment.appointmentType"); 3457 else if (Configuration.doAutoCreate()) 3458 this.appointmentType = new CodeableConcept(); // cc 3459 return this.appointmentType; 3460 } 3461 3462 public boolean hasAppointmentType() { 3463 return this.appointmentType != null && !this.appointmentType.isEmpty(); 3464 } 3465 3466 /** 3467 * @param value {@link #appointmentType} (The style of appointment or patient that has been booked in the slot (not service type).) 3468 */ 3469 public Appointment setAppointmentType(CodeableConcept value) { 3470 this.appointmentType = value; 3471 return this; 3472 } 3473 3474 /** 3475 * @return {@link #reason} (The reason that this appointment is being scheduled. This is more clinical than administrative. This can be coded, or as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 3476 */ 3477 public List<CodeableReference> getReason() { 3478 if (this.reason == null) 3479 this.reason = new ArrayList<CodeableReference>(); 3480 return this.reason; 3481 } 3482 3483 /** 3484 * @return Returns a reference to <code>this</code> for easy method chaining 3485 */ 3486 public Appointment setReason(List<CodeableReference> theReason) { 3487 this.reason = theReason; 3488 return this; 3489 } 3490 3491 public boolean hasReason() { 3492 if (this.reason == null) 3493 return false; 3494 for (CodeableReference item : this.reason) 3495 if (!item.isEmpty()) 3496 return true; 3497 return false; 3498 } 3499 3500 public CodeableReference addReason() { //3 3501 CodeableReference t = new CodeableReference(); 3502 if (this.reason == null) 3503 this.reason = new ArrayList<CodeableReference>(); 3504 this.reason.add(t); 3505 return t; 3506 } 3507 3508 public Appointment addReason(CodeableReference t) { //3 3509 if (t == null) 3510 return this; 3511 if (this.reason == null) 3512 this.reason = new ArrayList<CodeableReference>(); 3513 this.reason.add(t); 3514 return this; 3515 } 3516 3517 /** 3518 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 3519 */ 3520 public CodeableReference getReasonFirstRep() { 3521 if (getReason().isEmpty()) { 3522 addReason(); 3523 } 3524 return getReason().get(0); 3525 } 3526 3527 /** 3528 * @return {@link #priority} (The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).) 3529 */ 3530 public CodeableConcept getPriority() { 3531 if (this.priority == null) 3532 if (Configuration.errorOnAutoCreate()) 3533 throw new Error("Attempt to auto-create Appointment.priority"); 3534 else if (Configuration.doAutoCreate()) 3535 this.priority = new CodeableConcept(); // cc 3536 return this.priority; 3537 } 3538 3539 public boolean hasPriority() { 3540 return this.priority != null && !this.priority.isEmpty(); 3541 } 3542 3543 /** 3544 * @param value {@link #priority} (The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).) 3545 */ 3546 public Appointment setPriority(CodeableConcept value) { 3547 this.priority = value; 3548 return this; 3549 } 3550 3551 /** 3552 * @return {@link #description} (The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3553 */ 3554 public StringType getDescriptionElement() { 3555 if (this.description == null) 3556 if (Configuration.errorOnAutoCreate()) 3557 throw new Error("Attempt to auto-create Appointment.description"); 3558 else if (Configuration.doAutoCreate()) 3559 this.description = new StringType(); // bb 3560 return this.description; 3561 } 3562 3563 public boolean hasDescriptionElement() { 3564 return this.description != null && !this.description.isEmpty(); 3565 } 3566 3567 public boolean hasDescription() { 3568 return this.description != null && !this.description.isEmpty(); 3569 } 3570 3571 /** 3572 * @param value {@link #description} (The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3573 */ 3574 public Appointment setDescriptionElement(StringType value) { 3575 this.description = value; 3576 return this; 3577 } 3578 3579 /** 3580 * @return The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field. 3581 */ 3582 public String getDescription() { 3583 return this.description == null ? null : this.description.getValue(); 3584 } 3585 3586 /** 3587 * @param value The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field. 3588 */ 3589 public Appointment setDescription(String value) { 3590 if (Utilities.noString(value)) 3591 this.description = null; 3592 else { 3593 if (this.description == null) 3594 this.description = new StringType(); 3595 this.description.setValue(value); 3596 } 3597 return this; 3598 } 3599 3600 /** 3601 * @return {@link #replaces} (Appointment replaced by this Appointment in cases where there is a cancellation, the details of the cancellation can be found in the cancellationReason property (on the referenced resource).) 3602 */ 3603 public List<Reference> getReplaces() { 3604 if (this.replaces == null) 3605 this.replaces = new ArrayList<Reference>(); 3606 return this.replaces; 3607 } 3608 3609 /** 3610 * @return Returns a reference to <code>this</code> for easy method chaining 3611 */ 3612 public Appointment setReplaces(List<Reference> theReplaces) { 3613 this.replaces = theReplaces; 3614 return this; 3615 } 3616 3617 public boolean hasReplaces() { 3618 if (this.replaces == null) 3619 return false; 3620 for (Reference item : this.replaces) 3621 if (!item.isEmpty()) 3622 return true; 3623 return false; 3624 } 3625 3626 public Reference addReplaces() { //3 3627 Reference t = new Reference(); 3628 if (this.replaces == null) 3629 this.replaces = new ArrayList<Reference>(); 3630 this.replaces.add(t); 3631 return t; 3632 } 3633 3634 public Appointment addReplaces(Reference t) { //3 3635 if (t == null) 3636 return this; 3637 if (this.replaces == null) 3638 this.replaces = new ArrayList<Reference>(); 3639 this.replaces.add(t); 3640 return this; 3641 } 3642 3643 /** 3644 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist {3} 3645 */ 3646 public Reference getReplacesFirstRep() { 3647 if (getReplaces().isEmpty()) { 3648 addReplaces(); 3649 } 3650 return getReplaces().get(0); 3651 } 3652 3653 /** 3654 * @return {@link #virtualService} (Connection details of a virtual service (e.g. conference call).) 3655 */ 3656 public List<VirtualServiceDetail> getVirtualService() { 3657 if (this.virtualService == null) 3658 this.virtualService = new ArrayList<VirtualServiceDetail>(); 3659 return this.virtualService; 3660 } 3661 3662 /** 3663 * @return Returns a reference to <code>this</code> for easy method chaining 3664 */ 3665 public Appointment setVirtualService(List<VirtualServiceDetail> theVirtualService) { 3666 this.virtualService = theVirtualService; 3667 return this; 3668 } 3669 3670 public boolean hasVirtualService() { 3671 if (this.virtualService == null) 3672 return false; 3673 for (VirtualServiceDetail item : this.virtualService) 3674 if (!item.isEmpty()) 3675 return true; 3676 return false; 3677 } 3678 3679 public VirtualServiceDetail addVirtualService() { //3 3680 VirtualServiceDetail t = new VirtualServiceDetail(); 3681 if (this.virtualService == null) 3682 this.virtualService = new ArrayList<VirtualServiceDetail>(); 3683 this.virtualService.add(t); 3684 return t; 3685 } 3686 3687 public Appointment addVirtualService(VirtualServiceDetail t) { //3 3688 if (t == null) 3689 return this; 3690 if (this.virtualService == null) 3691 this.virtualService = new ArrayList<VirtualServiceDetail>(); 3692 this.virtualService.add(t); 3693 return this; 3694 } 3695 3696 /** 3697 * @return The first repetition of repeating field {@link #virtualService}, creating it if it does not already exist {3} 3698 */ 3699 public VirtualServiceDetail getVirtualServiceFirstRep() { 3700 if (getVirtualService().isEmpty()) { 3701 addVirtualService(); 3702 } 3703 return getVirtualService().get(0); 3704 } 3705 3706 /** 3707 * @return {@link #supportingInformation} (Additional information to support the appointment provided when making the appointment.) 3708 */ 3709 public List<Reference> getSupportingInformation() { 3710 if (this.supportingInformation == null) 3711 this.supportingInformation = new ArrayList<Reference>(); 3712 return this.supportingInformation; 3713 } 3714 3715 /** 3716 * @return Returns a reference to <code>this</code> for easy method chaining 3717 */ 3718 public Appointment setSupportingInformation(List<Reference> theSupportingInformation) { 3719 this.supportingInformation = theSupportingInformation; 3720 return this; 3721 } 3722 3723 public boolean hasSupportingInformation() { 3724 if (this.supportingInformation == null) 3725 return false; 3726 for (Reference item : this.supportingInformation) 3727 if (!item.isEmpty()) 3728 return true; 3729 return false; 3730 } 3731 3732 public Reference addSupportingInformation() { //3 3733 Reference t = new Reference(); 3734 if (this.supportingInformation == null) 3735 this.supportingInformation = new ArrayList<Reference>(); 3736 this.supportingInformation.add(t); 3737 return t; 3738 } 3739 3740 public Appointment addSupportingInformation(Reference t) { //3 3741 if (t == null) 3742 return this; 3743 if (this.supportingInformation == null) 3744 this.supportingInformation = new ArrayList<Reference>(); 3745 this.supportingInformation.add(t); 3746 return this; 3747 } 3748 3749 /** 3750 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 3751 */ 3752 public Reference getSupportingInformationFirstRep() { 3753 if (getSupportingInformation().isEmpty()) { 3754 addSupportingInformation(); 3755 } 3756 return getSupportingInformation().get(0); 3757 } 3758 3759 /** 3760 * @return {@link #previousAppointment} (The previous appointment in a series of related appointments.) 3761 */ 3762 public Reference getPreviousAppointment() { 3763 if (this.previousAppointment == null) 3764 if (Configuration.errorOnAutoCreate()) 3765 throw new Error("Attempt to auto-create Appointment.previousAppointment"); 3766 else if (Configuration.doAutoCreate()) 3767 this.previousAppointment = new Reference(); // cc 3768 return this.previousAppointment; 3769 } 3770 3771 public boolean hasPreviousAppointment() { 3772 return this.previousAppointment != null && !this.previousAppointment.isEmpty(); 3773 } 3774 3775 /** 3776 * @param value {@link #previousAppointment} (The previous appointment in a series of related appointments.) 3777 */ 3778 public Appointment setPreviousAppointment(Reference value) { 3779 this.previousAppointment = value; 3780 return this; 3781 } 3782 3783 /** 3784 * @return {@link #originatingAppointment} (The originating appointment in a recurring set of related appointments.) 3785 */ 3786 public Reference getOriginatingAppointment() { 3787 if (this.originatingAppointment == null) 3788 if (Configuration.errorOnAutoCreate()) 3789 throw new Error("Attempt to auto-create Appointment.originatingAppointment"); 3790 else if (Configuration.doAutoCreate()) 3791 this.originatingAppointment = new Reference(); // cc 3792 return this.originatingAppointment; 3793 } 3794 3795 public boolean hasOriginatingAppointment() { 3796 return this.originatingAppointment != null && !this.originatingAppointment.isEmpty(); 3797 } 3798 3799 /** 3800 * @param value {@link #originatingAppointment} (The originating appointment in a recurring set of related appointments.) 3801 */ 3802 public Appointment setOriginatingAppointment(Reference value) { 3803 this.originatingAppointment = value; 3804 return this; 3805 } 3806 3807 /** 3808 * @return {@link #start} (Date/Time that the appointment is to take place.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 3809 */ 3810 public InstantType getStartElement() { 3811 if (this.start == null) 3812 if (Configuration.errorOnAutoCreate()) 3813 throw new Error("Attempt to auto-create Appointment.start"); 3814 else if (Configuration.doAutoCreate()) 3815 this.start = new InstantType(); // bb 3816 return this.start; 3817 } 3818 3819 public boolean hasStartElement() { 3820 return this.start != null && !this.start.isEmpty(); 3821 } 3822 3823 public boolean hasStart() { 3824 return this.start != null && !this.start.isEmpty(); 3825 } 3826 3827 /** 3828 * @param value {@link #start} (Date/Time that the appointment is to take place.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 3829 */ 3830 public Appointment setStartElement(InstantType value) { 3831 this.start = value; 3832 return this; 3833 } 3834 3835 /** 3836 * @return Date/Time that the appointment is to take place. 3837 */ 3838 public Date getStart() { 3839 return this.start == null ? null : this.start.getValue(); 3840 } 3841 3842 /** 3843 * @param value Date/Time that the appointment is to take place. 3844 */ 3845 public Appointment setStart(Date value) { 3846 if (value == null) 3847 this.start = null; 3848 else { 3849 if (this.start == null) 3850 this.start = new InstantType(); 3851 this.start.setValue(value); 3852 } 3853 return this; 3854 } 3855 3856 /** 3857 * @return {@link #end} (Date/Time that the appointment is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 3858 */ 3859 public InstantType getEndElement() { 3860 if (this.end == null) 3861 if (Configuration.errorOnAutoCreate()) 3862 throw new Error("Attempt to auto-create Appointment.end"); 3863 else if (Configuration.doAutoCreate()) 3864 this.end = new InstantType(); // bb 3865 return this.end; 3866 } 3867 3868 public boolean hasEndElement() { 3869 return this.end != null && !this.end.isEmpty(); 3870 } 3871 3872 public boolean hasEnd() { 3873 return this.end != null && !this.end.isEmpty(); 3874 } 3875 3876 /** 3877 * @param value {@link #end} (Date/Time that the appointment is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 3878 */ 3879 public Appointment setEndElement(InstantType value) { 3880 this.end = value; 3881 return this; 3882 } 3883 3884 /** 3885 * @return Date/Time that the appointment is to conclude. 3886 */ 3887 public Date getEnd() { 3888 return this.end == null ? null : this.end.getValue(); 3889 } 3890 3891 /** 3892 * @param value Date/Time that the appointment is to conclude. 3893 */ 3894 public Appointment setEnd(Date value) { 3895 if (value == null) 3896 this.end = null; 3897 else { 3898 if (this.end == null) 3899 this.end = new InstantType(); 3900 this.end.setValue(value); 3901 } 3902 return this; 3903 } 3904 3905 /** 3906 * @return {@link #minutesDuration} (Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.). This is the underlying object with id, value and extensions. The accessor "getMinutesDuration" gives direct access to the value 3907 */ 3908 public PositiveIntType getMinutesDurationElement() { 3909 if (this.minutesDuration == null) 3910 if (Configuration.errorOnAutoCreate()) 3911 throw new Error("Attempt to auto-create Appointment.minutesDuration"); 3912 else if (Configuration.doAutoCreate()) 3913 this.minutesDuration = new PositiveIntType(); // bb 3914 return this.minutesDuration; 3915 } 3916 3917 public boolean hasMinutesDurationElement() { 3918 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 3919 } 3920 3921 public boolean hasMinutesDuration() { 3922 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 3923 } 3924 3925 /** 3926 * @param value {@link #minutesDuration} (Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.). This is the underlying object with id, value and extensions. The accessor "getMinutesDuration" gives direct access to the value 3927 */ 3928 public Appointment setMinutesDurationElement(PositiveIntType value) { 3929 this.minutesDuration = value; 3930 return this; 3931 } 3932 3933 /** 3934 * @return Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end. 3935 */ 3936 public int getMinutesDuration() { 3937 return this.minutesDuration == null || this.minutesDuration.isEmpty() ? 0 : this.minutesDuration.getValue(); 3938 } 3939 3940 /** 3941 * @param value Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end. 3942 */ 3943 public Appointment setMinutesDuration(int value) { 3944 if (this.minutesDuration == null) 3945 this.minutesDuration = new PositiveIntType(); 3946 this.minutesDuration.setValue(value); 3947 return this; 3948 } 3949 3950 /** 3951 * @return {@link #requestedPeriod} (A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within. 3952 3953The duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.) 3954 */ 3955 public List<Period> getRequestedPeriod() { 3956 if (this.requestedPeriod == null) 3957 this.requestedPeriod = new ArrayList<Period>(); 3958 return this.requestedPeriod; 3959 } 3960 3961 /** 3962 * @return Returns a reference to <code>this</code> for easy method chaining 3963 */ 3964 public Appointment setRequestedPeriod(List<Period> theRequestedPeriod) { 3965 this.requestedPeriod = theRequestedPeriod; 3966 return this; 3967 } 3968 3969 public boolean hasRequestedPeriod() { 3970 if (this.requestedPeriod == null) 3971 return false; 3972 for (Period item : this.requestedPeriod) 3973 if (!item.isEmpty()) 3974 return true; 3975 return false; 3976 } 3977 3978 public Period addRequestedPeriod() { //3 3979 Period t = new Period(); 3980 if (this.requestedPeriod == null) 3981 this.requestedPeriod = new ArrayList<Period>(); 3982 this.requestedPeriod.add(t); 3983 return t; 3984 } 3985 3986 public Appointment addRequestedPeriod(Period t) { //3 3987 if (t == null) 3988 return this; 3989 if (this.requestedPeriod == null) 3990 this.requestedPeriod = new ArrayList<Period>(); 3991 this.requestedPeriod.add(t); 3992 return this; 3993 } 3994 3995 /** 3996 * @return The first repetition of repeating field {@link #requestedPeriod}, creating it if it does not already exist {3} 3997 */ 3998 public Period getRequestedPeriodFirstRep() { 3999 if (getRequestedPeriod().isEmpty()) { 4000 addRequestedPeriod(); 4001 } 4002 return getRequestedPeriod().get(0); 4003 } 4004 4005 /** 4006 * @return {@link #slot} (The slots from the participants' schedules that will be filled by the appointment.) 4007 */ 4008 public List<Reference> getSlot() { 4009 if (this.slot == null) 4010 this.slot = new ArrayList<Reference>(); 4011 return this.slot; 4012 } 4013 4014 /** 4015 * @return Returns a reference to <code>this</code> for easy method chaining 4016 */ 4017 public Appointment setSlot(List<Reference> theSlot) { 4018 this.slot = theSlot; 4019 return this; 4020 } 4021 4022 public boolean hasSlot() { 4023 if (this.slot == null) 4024 return false; 4025 for (Reference item : this.slot) 4026 if (!item.isEmpty()) 4027 return true; 4028 return false; 4029 } 4030 4031 public Reference addSlot() { //3 4032 Reference t = new Reference(); 4033 if (this.slot == null) 4034 this.slot = new ArrayList<Reference>(); 4035 this.slot.add(t); 4036 return t; 4037 } 4038 4039 public Appointment addSlot(Reference t) { //3 4040 if (t == null) 4041 return this; 4042 if (this.slot == null) 4043 this.slot = new ArrayList<Reference>(); 4044 this.slot.add(t); 4045 return this; 4046 } 4047 4048 /** 4049 * @return The first repetition of repeating field {@link #slot}, creating it if it does not already exist {3} 4050 */ 4051 public Reference getSlotFirstRep() { 4052 if (getSlot().isEmpty()) { 4053 addSlot(); 4054 } 4055 return getSlot().get(0); 4056 } 4057 4058 /** 4059 * @return {@link #account} (The set of accounts that is expected to be used for billing the activities that result from this Appointment.) 4060 */ 4061 public List<Reference> getAccount() { 4062 if (this.account == null) 4063 this.account = new ArrayList<Reference>(); 4064 return this.account; 4065 } 4066 4067 /** 4068 * @return Returns a reference to <code>this</code> for easy method chaining 4069 */ 4070 public Appointment setAccount(List<Reference> theAccount) { 4071 this.account = theAccount; 4072 return this; 4073 } 4074 4075 public boolean hasAccount() { 4076 if (this.account == null) 4077 return false; 4078 for (Reference item : this.account) 4079 if (!item.isEmpty()) 4080 return true; 4081 return false; 4082 } 4083 4084 public Reference addAccount() { //3 4085 Reference t = new Reference(); 4086 if (this.account == null) 4087 this.account = new ArrayList<Reference>(); 4088 this.account.add(t); 4089 return t; 4090 } 4091 4092 public Appointment addAccount(Reference t) { //3 4093 if (t == null) 4094 return this; 4095 if (this.account == null) 4096 this.account = new ArrayList<Reference>(); 4097 this.account.add(t); 4098 return this; 4099 } 4100 4101 /** 4102 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist {3} 4103 */ 4104 public Reference getAccountFirstRep() { 4105 if (getAccount().isEmpty()) { 4106 addAccount(); 4107 } 4108 return getAccount().get(0); 4109 } 4110 4111 /** 4112 * @return {@link #created} (The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 4113 */ 4114 public DateTimeType getCreatedElement() { 4115 if (this.created == null) 4116 if (Configuration.errorOnAutoCreate()) 4117 throw new Error("Attempt to auto-create Appointment.created"); 4118 else if (Configuration.doAutoCreate()) 4119 this.created = new DateTimeType(); // bb 4120 return this.created; 4121 } 4122 4123 public boolean hasCreatedElement() { 4124 return this.created != null && !this.created.isEmpty(); 4125 } 4126 4127 public boolean hasCreated() { 4128 return this.created != null && !this.created.isEmpty(); 4129 } 4130 4131 /** 4132 * @param value {@link #created} (The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 4133 */ 4134 public Appointment setCreatedElement(DateTimeType value) { 4135 this.created = value; 4136 return this; 4137 } 4138 4139 /** 4140 * @return The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment. 4141 */ 4142 public Date getCreated() { 4143 return this.created == null ? null : this.created.getValue(); 4144 } 4145 4146 /** 4147 * @param value The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment. 4148 */ 4149 public Appointment setCreated(Date value) { 4150 if (value == null) 4151 this.created = null; 4152 else { 4153 if (this.created == null) 4154 this.created = new DateTimeType(); 4155 this.created.setValue(value); 4156 } 4157 return this; 4158 } 4159 4160 /** 4161 * @return {@link #cancellationDate} (The date/time describing when the appointment was cancelled.). This is the underlying object with id, value and extensions. The accessor "getCancellationDate" gives direct access to the value 4162 */ 4163 public DateTimeType getCancellationDateElement() { 4164 if (this.cancellationDate == null) 4165 if (Configuration.errorOnAutoCreate()) 4166 throw new Error("Attempt to auto-create Appointment.cancellationDate"); 4167 else if (Configuration.doAutoCreate()) 4168 this.cancellationDate = new DateTimeType(); // bb 4169 return this.cancellationDate; 4170 } 4171 4172 public boolean hasCancellationDateElement() { 4173 return this.cancellationDate != null && !this.cancellationDate.isEmpty(); 4174 } 4175 4176 public boolean hasCancellationDate() { 4177 return this.cancellationDate != null && !this.cancellationDate.isEmpty(); 4178 } 4179 4180 /** 4181 * @param value {@link #cancellationDate} (The date/time describing when the appointment was cancelled.). This is the underlying object with id, value and extensions. The accessor "getCancellationDate" gives direct access to the value 4182 */ 4183 public Appointment setCancellationDateElement(DateTimeType value) { 4184 this.cancellationDate = value; 4185 return this; 4186 } 4187 4188 /** 4189 * @return The date/time describing when the appointment was cancelled. 4190 */ 4191 public Date getCancellationDate() { 4192 return this.cancellationDate == null ? null : this.cancellationDate.getValue(); 4193 } 4194 4195 /** 4196 * @param value The date/time describing when the appointment was cancelled. 4197 */ 4198 public Appointment setCancellationDate(Date value) { 4199 if (value == null) 4200 this.cancellationDate = null; 4201 else { 4202 if (this.cancellationDate == null) 4203 this.cancellationDate = new DateTimeType(); 4204 this.cancellationDate.setValue(value); 4205 } 4206 return this; 4207 } 4208 4209 /** 4210 * @return {@link #note} (Additional notes/comments about the appointment.) 4211 */ 4212 public List<Annotation> getNote() { 4213 if (this.note == null) 4214 this.note = new ArrayList<Annotation>(); 4215 return this.note; 4216 } 4217 4218 /** 4219 * @return Returns a reference to <code>this</code> for easy method chaining 4220 */ 4221 public Appointment setNote(List<Annotation> theNote) { 4222 this.note = theNote; 4223 return this; 4224 } 4225 4226 public boolean hasNote() { 4227 if (this.note == null) 4228 return false; 4229 for (Annotation item : this.note) 4230 if (!item.isEmpty()) 4231 return true; 4232 return false; 4233 } 4234 4235 public Annotation addNote() { //3 4236 Annotation t = new Annotation(); 4237 if (this.note == null) 4238 this.note = new ArrayList<Annotation>(); 4239 this.note.add(t); 4240 return t; 4241 } 4242 4243 public Appointment addNote(Annotation t) { //3 4244 if (t == null) 4245 return this; 4246 if (this.note == null) 4247 this.note = new ArrayList<Annotation>(); 4248 this.note.add(t); 4249 return this; 4250 } 4251 4252 /** 4253 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 4254 */ 4255 public Annotation getNoteFirstRep() { 4256 if (getNote().isEmpty()) { 4257 addNote(); 4258 } 4259 return getNote().get(0); 4260 } 4261 4262 /** 4263 * @return {@link #patientInstruction} (While Appointment.note contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).) 4264 */ 4265 public List<CodeableReference> getPatientInstruction() { 4266 if (this.patientInstruction == null) 4267 this.patientInstruction = new ArrayList<CodeableReference>(); 4268 return this.patientInstruction; 4269 } 4270 4271 /** 4272 * @return Returns a reference to <code>this</code> for easy method chaining 4273 */ 4274 public Appointment setPatientInstruction(List<CodeableReference> thePatientInstruction) { 4275 this.patientInstruction = thePatientInstruction; 4276 return this; 4277 } 4278 4279 public boolean hasPatientInstruction() { 4280 if (this.patientInstruction == null) 4281 return false; 4282 for (CodeableReference item : this.patientInstruction) 4283 if (!item.isEmpty()) 4284 return true; 4285 return false; 4286 } 4287 4288 public CodeableReference addPatientInstruction() { //3 4289 CodeableReference t = new CodeableReference(); 4290 if (this.patientInstruction == null) 4291 this.patientInstruction = new ArrayList<CodeableReference>(); 4292 this.patientInstruction.add(t); 4293 return t; 4294 } 4295 4296 public Appointment addPatientInstruction(CodeableReference t) { //3 4297 if (t == null) 4298 return this; 4299 if (this.patientInstruction == null) 4300 this.patientInstruction = new ArrayList<CodeableReference>(); 4301 this.patientInstruction.add(t); 4302 return this; 4303 } 4304 4305 /** 4306 * @return The first repetition of repeating field {@link #patientInstruction}, creating it if it does not already exist {3} 4307 */ 4308 public CodeableReference getPatientInstructionFirstRep() { 4309 if (getPatientInstruction().isEmpty()) { 4310 addPatientInstruction(); 4311 } 4312 return getPatientInstruction().get(0); 4313 } 4314 4315 /** 4316 * @return {@link #basedOn} (The request this appointment is allocated to assess (e.g. incoming referral or procedure request).) 4317 */ 4318 public List<Reference> getBasedOn() { 4319 if (this.basedOn == null) 4320 this.basedOn = new ArrayList<Reference>(); 4321 return this.basedOn; 4322 } 4323 4324 /** 4325 * @return Returns a reference to <code>this</code> for easy method chaining 4326 */ 4327 public Appointment setBasedOn(List<Reference> theBasedOn) { 4328 this.basedOn = theBasedOn; 4329 return this; 4330 } 4331 4332 public boolean hasBasedOn() { 4333 if (this.basedOn == null) 4334 return false; 4335 for (Reference item : this.basedOn) 4336 if (!item.isEmpty()) 4337 return true; 4338 return false; 4339 } 4340 4341 public Reference addBasedOn() { //3 4342 Reference t = new Reference(); 4343 if (this.basedOn == null) 4344 this.basedOn = new ArrayList<Reference>(); 4345 this.basedOn.add(t); 4346 return t; 4347 } 4348 4349 public Appointment addBasedOn(Reference t) { //3 4350 if (t == null) 4351 return this; 4352 if (this.basedOn == null) 4353 this.basedOn = new ArrayList<Reference>(); 4354 this.basedOn.add(t); 4355 return this; 4356 } 4357 4358 /** 4359 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 4360 */ 4361 public Reference getBasedOnFirstRep() { 4362 if (getBasedOn().isEmpty()) { 4363 addBasedOn(); 4364 } 4365 return getBasedOn().get(0); 4366 } 4367 4368 /** 4369 * @return {@link #subject} (The patient or group associated with the appointment, if they are to be present (usually) then they should also be included in the participant backbone element.) 4370 */ 4371 public Reference getSubject() { 4372 if (this.subject == null) 4373 if (Configuration.errorOnAutoCreate()) 4374 throw new Error("Attempt to auto-create Appointment.subject"); 4375 else if (Configuration.doAutoCreate()) 4376 this.subject = new Reference(); // cc 4377 return this.subject; 4378 } 4379 4380 public boolean hasSubject() { 4381 return this.subject != null && !this.subject.isEmpty(); 4382 } 4383 4384 /** 4385 * @param value {@link #subject} (The patient or group associated with the appointment, if they are to be present (usually) then they should also be included in the participant backbone element.) 4386 */ 4387 public Appointment setSubject(Reference value) { 4388 this.subject = value; 4389 return this; 4390 } 4391 4392 /** 4393 * @return {@link #participant} (List of participants involved in the appointment.) 4394 */ 4395 public List<AppointmentParticipantComponent> getParticipant() { 4396 if (this.participant == null) 4397 this.participant = new ArrayList<AppointmentParticipantComponent>(); 4398 return this.participant; 4399 } 4400 4401 /** 4402 * @return Returns a reference to <code>this</code> for easy method chaining 4403 */ 4404 public Appointment setParticipant(List<AppointmentParticipantComponent> theParticipant) { 4405 this.participant = theParticipant; 4406 return this; 4407 } 4408 4409 public boolean hasParticipant() { 4410 if (this.participant == null) 4411 return false; 4412 for (AppointmentParticipantComponent item : this.participant) 4413 if (!item.isEmpty()) 4414 return true; 4415 return false; 4416 } 4417 4418 public AppointmentParticipantComponent addParticipant() { //3 4419 AppointmentParticipantComponent t = new AppointmentParticipantComponent(); 4420 if (this.participant == null) 4421 this.participant = new ArrayList<AppointmentParticipantComponent>(); 4422 this.participant.add(t); 4423 return t; 4424 } 4425 4426 public Appointment addParticipant(AppointmentParticipantComponent t) { //3 4427 if (t == null) 4428 return this; 4429 if (this.participant == null) 4430 this.participant = new ArrayList<AppointmentParticipantComponent>(); 4431 this.participant.add(t); 4432 return this; 4433 } 4434 4435 /** 4436 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 4437 */ 4438 public AppointmentParticipantComponent getParticipantFirstRep() { 4439 if (getParticipant().isEmpty()) { 4440 addParticipant(); 4441 } 4442 return getParticipant().get(0); 4443 } 4444 4445 /** 4446 * @return {@link #recurrenceId} (The sequence number that identifies a specific appointment in a recurring pattern.). This is the underlying object with id, value and extensions. The accessor "getRecurrenceId" gives direct access to the value 4447 */ 4448 public PositiveIntType getRecurrenceIdElement() { 4449 if (this.recurrenceId == null) 4450 if (Configuration.errorOnAutoCreate()) 4451 throw new Error("Attempt to auto-create Appointment.recurrenceId"); 4452 else if (Configuration.doAutoCreate()) 4453 this.recurrenceId = new PositiveIntType(); // bb 4454 return this.recurrenceId; 4455 } 4456 4457 public boolean hasRecurrenceIdElement() { 4458 return this.recurrenceId != null && !this.recurrenceId.isEmpty(); 4459 } 4460 4461 public boolean hasRecurrenceId() { 4462 return this.recurrenceId != null && !this.recurrenceId.isEmpty(); 4463 } 4464 4465 /** 4466 * @param value {@link #recurrenceId} (The sequence number that identifies a specific appointment in a recurring pattern.). This is the underlying object with id, value and extensions. The accessor "getRecurrenceId" gives direct access to the value 4467 */ 4468 public Appointment setRecurrenceIdElement(PositiveIntType value) { 4469 this.recurrenceId = value; 4470 return this; 4471 } 4472 4473 /** 4474 * @return The sequence number that identifies a specific appointment in a recurring pattern. 4475 */ 4476 public int getRecurrenceId() { 4477 return this.recurrenceId == null || this.recurrenceId.isEmpty() ? 0 : this.recurrenceId.getValue(); 4478 } 4479 4480 /** 4481 * @param value The sequence number that identifies a specific appointment in a recurring pattern. 4482 */ 4483 public Appointment setRecurrenceId(int value) { 4484 if (this.recurrenceId == null) 4485 this.recurrenceId = new PositiveIntType(); 4486 this.recurrenceId.setValue(value); 4487 return this; 4488 } 4489 4490 /** 4491 * @return {@link #occurrenceChanged} (This appointment varies from the recurring pattern.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceChanged" gives direct access to the value 4492 */ 4493 public BooleanType getOccurrenceChangedElement() { 4494 if (this.occurrenceChanged == null) 4495 if (Configuration.errorOnAutoCreate()) 4496 throw new Error("Attempt to auto-create Appointment.occurrenceChanged"); 4497 else if (Configuration.doAutoCreate()) 4498 this.occurrenceChanged = new BooleanType(); // bb 4499 return this.occurrenceChanged; 4500 } 4501 4502 public boolean hasOccurrenceChangedElement() { 4503 return this.occurrenceChanged != null && !this.occurrenceChanged.isEmpty(); 4504 } 4505 4506 public boolean hasOccurrenceChanged() { 4507 return this.occurrenceChanged != null && !this.occurrenceChanged.isEmpty(); 4508 } 4509 4510 /** 4511 * @param value {@link #occurrenceChanged} (This appointment varies from the recurring pattern.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceChanged" gives direct access to the value 4512 */ 4513 public Appointment setOccurrenceChangedElement(BooleanType value) { 4514 this.occurrenceChanged = value; 4515 return this; 4516 } 4517 4518 /** 4519 * @return This appointment varies from the recurring pattern. 4520 */ 4521 public boolean getOccurrenceChanged() { 4522 return this.occurrenceChanged == null || this.occurrenceChanged.isEmpty() ? false : this.occurrenceChanged.getValue(); 4523 } 4524 4525 /** 4526 * @param value This appointment varies from the recurring pattern. 4527 */ 4528 public Appointment setOccurrenceChanged(boolean value) { 4529 if (this.occurrenceChanged == null) 4530 this.occurrenceChanged = new BooleanType(); 4531 this.occurrenceChanged.setValue(value); 4532 return this; 4533 } 4534 4535 /** 4536 * @return {@link #recurrenceTemplate} (The details of the recurrence pattern or template that is used to generate recurring appointments.) 4537 */ 4538 public List<AppointmentRecurrenceTemplateComponent> getRecurrenceTemplate() { 4539 if (this.recurrenceTemplate == null) 4540 this.recurrenceTemplate = new ArrayList<AppointmentRecurrenceTemplateComponent>(); 4541 return this.recurrenceTemplate; 4542 } 4543 4544 /** 4545 * @return Returns a reference to <code>this</code> for easy method chaining 4546 */ 4547 public Appointment setRecurrenceTemplate(List<AppointmentRecurrenceTemplateComponent> theRecurrenceTemplate) { 4548 this.recurrenceTemplate = theRecurrenceTemplate; 4549 return this; 4550 } 4551 4552 public boolean hasRecurrenceTemplate() { 4553 if (this.recurrenceTemplate == null) 4554 return false; 4555 for (AppointmentRecurrenceTemplateComponent item : this.recurrenceTemplate) 4556 if (!item.isEmpty()) 4557 return true; 4558 return false; 4559 } 4560 4561 public AppointmentRecurrenceTemplateComponent addRecurrenceTemplate() { //3 4562 AppointmentRecurrenceTemplateComponent t = new AppointmentRecurrenceTemplateComponent(); 4563 if (this.recurrenceTemplate == null) 4564 this.recurrenceTemplate = new ArrayList<AppointmentRecurrenceTemplateComponent>(); 4565 this.recurrenceTemplate.add(t); 4566 return t; 4567 } 4568 4569 public Appointment addRecurrenceTemplate(AppointmentRecurrenceTemplateComponent t) { //3 4570 if (t == null) 4571 return this; 4572 if (this.recurrenceTemplate == null) 4573 this.recurrenceTemplate = new ArrayList<AppointmentRecurrenceTemplateComponent>(); 4574 this.recurrenceTemplate.add(t); 4575 return this; 4576 } 4577 4578 /** 4579 * @return The first repetition of repeating field {@link #recurrenceTemplate}, creating it if it does not already exist {3} 4580 */ 4581 public AppointmentRecurrenceTemplateComponent getRecurrenceTemplateFirstRep() { 4582 if (getRecurrenceTemplate().isEmpty()) { 4583 addRecurrenceTemplate(); 4584 } 4585 return getRecurrenceTemplate().get(0); 4586 } 4587 4588 protected void listChildren(List<Property> children) { 4589 super.listChildren(children); 4590 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 4591 children.add(new Property("status", "code", "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 0, 1, status)); 4592 children.add(new Property("cancellationReason", "CodeableConcept", "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.", 0, 1, cancellationReason)); 4593 children.add(new Property("class", "CodeableConcept", "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 0, java.lang.Integer.MAX_VALUE, class_)); 4594 children.add(new Property("serviceCategory", "CodeableConcept", "A broad categorization of the service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceCategory)); 4595 children.add(new Property("serviceType", "CodeableReference(HealthcareService)", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType)); 4596 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty)); 4597 children.add(new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, appointmentType)); 4598 children.add(new Property("reason", "CodeableReference(Condition|Procedure|Observation|ImmunizationRecommendation)", "The reason that this appointment is being scheduled. This is more clinical than administrative. This can be coded, or as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 0, java.lang.Integer.MAX_VALUE, reason)); 4599 children.add(new Property("priority", "CodeableConcept", "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 0, 1, priority)); 4600 children.add(new Property("description", "string", "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field.", 0, 1, description)); 4601 children.add(new Property("replaces", "Reference(Appointment)", "Appointment replaced by this Appointment in cases where there is a cancellation, the details of the cancellation can be found in the cancellationReason property (on the referenced resource).", 0, java.lang.Integer.MAX_VALUE, replaces)); 4602 children.add(new Property("virtualService", "VirtualServiceDetail", "Connection details of a virtual service (e.g. conference call).", 0, java.lang.Integer.MAX_VALUE, virtualService)); 4603 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information to support the appointment provided when making the appointment.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 4604 children.add(new Property("previousAppointment", "Reference(Appointment)", "The previous appointment in a series of related appointments.", 0, 1, previousAppointment)); 4605 children.add(new Property("originatingAppointment", "Reference(Appointment)", "The originating appointment in a recurring set of related appointments.", 0, 1, originatingAppointment)); 4606 children.add(new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, start)); 4607 children.add(new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end)); 4608 children.add(new Property("minutesDuration", "positiveInt", "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.", 0, 1, minutesDuration)); 4609 children.add(new Property("requestedPeriod", "Period", "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.", 0, java.lang.Integer.MAX_VALUE, requestedPeriod)); 4610 children.add(new Property("slot", "Reference(Slot)", "The slots from the participants' schedules that will be filled by the appointment.", 0, java.lang.Integer.MAX_VALUE, slot)); 4611 children.add(new Property("account", "Reference(Account)", "The set of accounts that is expected to be used for billing the activities that result from this Appointment.", 0, java.lang.Integer.MAX_VALUE, account)); 4612 children.add(new Property("created", "dateTime", "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 0, 1, created)); 4613 children.add(new Property("cancellationDate", "dateTime", "The date/time describing when the appointment was cancelled.", 0, 1, cancellationDate)); 4614 children.add(new Property("note", "Annotation", "Additional notes/comments about the appointment.", 0, java.lang.Integer.MAX_VALUE, note)); 4615 children.add(new Property("patientInstruction", "CodeableReference(DocumentReference|Binary|Communication)", "While Appointment.note contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).", 0, java.lang.Integer.MAX_VALUE, patientInstruction)); 4616 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|MedicationRequest|ServiceRequest)", "The request this appointment is allocated to assess (e.g. incoming referral or procedure request).", 0, java.lang.Integer.MAX_VALUE, basedOn)); 4617 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group associated with the appointment, if they are to be present (usually) then they should also be included in the participant backbone element.", 0, 1, subject)); 4618 children.add(new Property("participant", "", "List of participants involved in the appointment.", 0, java.lang.Integer.MAX_VALUE, participant)); 4619 children.add(new Property("recurrenceId", "positiveInt", "The sequence number that identifies a specific appointment in a recurring pattern.", 0, 1, recurrenceId)); 4620 children.add(new Property("occurrenceChanged", "boolean", "This appointment varies from the recurring pattern.", 0, 1, occurrenceChanged)); 4621 children.add(new Property("recurrenceTemplate", "", "The details of the recurrence pattern or template that is used to generate recurring appointments.", 0, java.lang.Integer.MAX_VALUE, recurrenceTemplate)); 4622 } 4623 4624 @Override 4625 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4626 switch (_hash) { 4627 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 4628 case -892481550: /*status*/ return new Property("status", "code", "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 0, 1, status); 4629 case 2135095591: /*cancellationReason*/ return new Property("cancellationReason", "CodeableConcept", "The coded reason for the appointment being cancelled. This is often used in reporting/billing/futher processing to determine if further actions are required, or specific fees apply.", 0, 1, cancellationReason); 4630 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 0, java.lang.Integer.MAX_VALUE, class_); 4631 case 1281188563: /*serviceCategory*/ return new Property("serviceCategory", "CodeableConcept", "A broad categorization of the service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceCategory); 4632 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableReference(HealthcareService)", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType); 4633 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty); 4634 case -1596426375: /*appointmentType*/ return new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that has been booked in the slot (not service type).", 0, 1, appointmentType); 4635 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Procedure|Observation|ImmunizationRecommendation)", "The reason that this appointment is being scheduled. This is more clinical than administrative. This can be coded, or as specified using information from another resource. When the patient arrives and the encounter begins it may be used as the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 0, java.lang.Integer.MAX_VALUE, reason); 4636 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 0, 1, priority); 4637 case -1724546052: /*description*/ return new Property("description", "string", "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the note field.", 0, 1, description); 4638 case -430332865: /*replaces*/ return new Property("replaces", "Reference(Appointment)", "Appointment replaced by this Appointment in cases where there is a cancellation, the details of the cancellation can be found in the cancellationReason property (on the referenced resource).", 0, java.lang.Integer.MAX_VALUE, replaces); 4639 case 1420774698: /*virtualService*/ return new Property("virtualService", "VirtualServiceDetail", "Connection details of a virtual service (e.g. conference call).", 0, java.lang.Integer.MAX_VALUE, virtualService); 4640 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information to support the appointment provided when making the appointment.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 4641 case -1676044248: /*previousAppointment*/ return new Property("previousAppointment", "Reference(Appointment)", "The previous appointment in a series of related appointments.", 0, 1, previousAppointment); 4642 case 1841882230: /*originatingAppointment*/ return new Property("originatingAppointment", "Reference(Appointment)", "The originating appointment in a recurring set of related appointments.", 0, 1, originatingAppointment); 4643 case 109757538: /*start*/ return new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1, start); 4644 case 100571: /*end*/ return new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1, end); 4645 case -413630573: /*minutesDuration*/ return new Property("minutesDuration", "positiveInt", "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times. For example, where the actual time of appointment is only an estimate or if a 30 minute appointment is being requested, but any time would work. Also, if there is, for example, a planned 15 minute break in the middle of a long appointment, the duration may be 15 minutes less than the difference between the start and end.", 0, 1, minutesDuration); 4646 case -897241393: /*requestedPeriod*/ return new Property("requestedPeriod", "Period", "A set of date ranges (potentially including times) that the appointment is preferred to be scheduled within.\n\nThe duration (usually in minutes) could also be provided to indicate the length of the appointment to fill and populate the start/end times for the actual allocated time. However, in other situations the duration may be calculated by the scheduling system.", 0, java.lang.Integer.MAX_VALUE, requestedPeriod); 4647 case 3533310: /*slot*/ return new Property("slot", "Reference(Slot)", "The slots from the participants' schedules that will be filled by the appointment.", 0, java.lang.Integer.MAX_VALUE, slot); 4648 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "The set of accounts that is expected to be used for billing the activities that result from this Appointment.", 0, java.lang.Integer.MAX_VALUE, account); 4649 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date that this appointment was initially created. This could be different to the meta.lastModified value on the initial entry, as this could have been before the resource was created on the FHIR server, and should remain unchanged over the lifespan of the appointment.", 0, 1, created); 4650 case 806269777: /*cancellationDate*/ return new Property("cancellationDate", "dateTime", "The date/time describing when the appointment was cancelled.", 0, 1, cancellationDate); 4651 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes/comments about the appointment.", 0, java.lang.Integer.MAX_VALUE, note); 4652 case 737543241: /*patientInstruction*/ return new Property("patientInstruction", "CodeableReference(DocumentReference|Binary|Communication)", "While Appointment.note contains information for internal use, Appointment.patientInstructions is used to capture patient facing information about the Appointment (e.g. please bring your referral or fast from 8pm night before).", 0, java.lang.Integer.MAX_VALUE, patientInstruction); 4653 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|MedicationRequest|ServiceRequest)", "The request this appointment is allocated to assess (e.g. incoming referral or procedure request).", 0, java.lang.Integer.MAX_VALUE, basedOn); 4654 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group associated with the appointment, if they are to be present (usually) then they should also be included in the participant backbone element.", 0, 1, subject); 4655 case 767422259: /*participant*/ return new Property("participant", "", "List of participants involved in the appointment.", 0, java.lang.Integer.MAX_VALUE, participant); 4656 case -362407829: /*recurrenceId*/ return new Property("recurrenceId", "positiveInt", "The sequence number that identifies a specific appointment in a recurring pattern.", 0, 1, recurrenceId); 4657 case 1779864483: /*occurrenceChanged*/ return new Property("occurrenceChanged", "boolean", "This appointment varies from the recurring pattern.", 0, 1, occurrenceChanged); 4658 case 597629898: /*recurrenceTemplate*/ return new Property("recurrenceTemplate", "", "The details of the recurrence pattern or template that is used to generate recurring appointments.", 0, java.lang.Integer.MAX_VALUE, recurrenceTemplate); 4659 default: return super.getNamedProperty(_hash, _name, _checkValid); 4660 } 4661 4662 } 4663 4664 @Override 4665 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4666 switch (hash) { 4667 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4668 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<AppointmentStatus> 4669 case 2135095591: /*cancellationReason*/ return this.cancellationReason == null ? new Base[0] : new Base[] {this.cancellationReason}; // CodeableConcept 4670 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // CodeableConcept 4671 case 1281188563: /*serviceCategory*/ return this.serviceCategory == null ? new Base[0] : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 4672 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableReference 4673 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 4674 case -1596426375: /*appointmentType*/ return this.appointmentType == null ? new Base[0] : new Base[] {this.appointmentType}; // CodeableConcept 4675 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 4676 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 4677 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 4678 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 4679 case 1420774698: /*virtualService*/ return this.virtualService == null ? new Base[0] : this.virtualService.toArray(new Base[this.virtualService.size()]); // VirtualServiceDetail 4680 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 4681 case -1676044248: /*previousAppointment*/ return this.previousAppointment == null ? new Base[0] : new Base[] {this.previousAppointment}; // Reference 4682 case 1841882230: /*originatingAppointment*/ return this.originatingAppointment == null ? new Base[0] : new Base[] {this.originatingAppointment}; // Reference 4683 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // InstantType 4684 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 4685 case -413630573: /*minutesDuration*/ return this.minutesDuration == null ? new Base[0] : new Base[] {this.minutesDuration}; // PositiveIntType 4686 case -897241393: /*requestedPeriod*/ return this.requestedPeriod == null ? new Base[0] : this.requestedPeriod.toArray(new Base[this.requestedPeriod.size()]); // Period 4687 case 3533310: /*slot*/ return this.slot == null ? new Base[0] : this.slot.toArray(new Base[this.slot.size()]); // Reference 4688 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 4689 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 4690 case 806269777: /*cancellationDate*/ return this.cancellationDate == null ? new Base[0] : new Base[] {this.cancellationDate}; // DateTimeType 4691 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4692 case 737543241: /*patientInstruction*/ return this.patientInstruction == null ? new Base[0] : this.patientInstruction.toArray(new Base[this.patientInstruction.size()]); // CodeableReference 4693 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4694 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 4695 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // AppointmentParticipantComponent 4696 case -362407829: /*recurrenceId*/ return this.recurrenceId == null ? new Base[0] : new Base[] {this.recurrenceId}; // PositiveIntType 4697 case 1779864483: /*occurrenceChanged*/ return this.occurrenceChanged == null ? new Base[0] : new Base[] {this.occurrenceChanged}; // BooleanType 4698 case 597629898: /*recurrenceTemplate*/ return this.recurrenceTemplate == null ? new Base[0] : this.recurrenceTemplate.toArray(new Base[this.recurrenceTemplate.size()]); // AppointmentRecurrenceTemplateComponent 4699 default: return super.getProperty(hash, name, checkValid); 4700 } 4701 4702 } 4703 4704 @Override 4705 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4706 switch (hash) { 4707 case -1618432855: // identifier 4708 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4709 return value; 4710 case -892481550: // status 4711 value = new AppointmentStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4712 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 4713 return value; 4714 case 2135095591: // cancellationReason 4715 this.cancellationReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4716 return value; 4717 case 94742904: // class 4718 this.getClass_().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4719 return value; 4720 case 1281188563: // serviceCategory 4721 this.getServiceCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4722 return value; 4723 case -1928370289: // serviceType 4724 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4725 return value; 4726 case -1694759682: // specialty 4727 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4728 return value; 4729 case -1596426375: // appointmentType 4730 this.appointmentType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4731 return value; 4732 case -934964668: // reason 4733 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4734 return value; 4735 case -1165461084: // priority 4736 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4737 return value; 4738 case -1724546052: // description 4739 this.description = TypeConvertor.castToString(value); // StringType 4740 return value; 4741 case -430332865: // replaces 4742 this.getReplaces().add(TypeConvertor.castToReference(value)); // Reference 4743 return value; 4744 case 1420774698: // virtualService 4745 this.getVirtualService().add(TypeConvertor.castToVirtualServiceDetail(value)); // VirtualServiceDetail 4746 return value; 4747 case -1248768647: // supportingInformation 4748 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 4749 return value; 4750 case -1676044248: // previousAppointment 4751 this.previousAppointment = TypeConvertor.castToReference(value); // Reference 4752 return value; 4753 case 1841882230: // originatingAppointment 4754 this.originatingAppointment = TypeConvertor.castToReference(value); // Reference 4755 return value; 4756 case 109757538: // start 4757 this.start = TypeConvertor.castToInstant(value); // InstantType 4758 return value; 4759 case 100571: // end 4760 this.end = TypeConvertor.castToInstant(value); // InstantType 4761 return value; 4762 case -413630573: // minutesDuration 4763 this.minutesDuration = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4764 return value; 4765 case -897241393: // requestedPeriod 4766 this.getRequestedPeriod().add(TypeConvertor.castToPeriod(value)); // Period 4767 return value; 4768 case 3533310: // slot 4769 this.getSlot().add(TypeConvertor.castToReference(value)); // Reference 4770 return value; 4771 case -1177318867: // account 4772 this.getAccount().add(TypeConvertor.castToReference(value)); // Reference 4773 return value; 4774 case 1028554472: // created 4775 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 4776 return value; 4777 case 806269777: // cancellationDate 4778 this.cancellationDate = TypeConvertor.castToDateTime(value); // DateTimeType 4779 return value; 4780 case 3387378: // note 4781 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4782 return value; 4783 case 737543241: // patientInstruction 4784 this.getPatientInstruction().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4785 return value; 4786 case -332612366: // basedOn 4787 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 4788 return value; 4789 case -1867885268: // subject 4790 this.subject = TypeConvertor.castToReference(value); // Reference 4791 return value; 4792 case 767422259: // participant 4793 this.getParticipant().add((AppointmentParticipantComponent) value); // AppointmentParticipantComponent 4794 return value; 4795 case -362407829: // recurrenceId 4796 this.recurrenceId = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4797 return value; 4798 case 1779864483: // occurrenceChanged 4799 this.occurrenceChanged = TypeConvertor.castToBoolean(value); // BooleanType 4800 return value; 4801 case 597629898: // recurrenceTemplate 4802 this.getRecurrenceTemplate().add((AppointmentRecurrenceTemplateComponent) value); // AppointmentRecurrenceTemplateComponent 4803 return value; 4804 default: return super.setProperty(hash, name, value); 4805 } 4806 4807 } 4808 4809 @Override 4810 public Base setProperty(String name, Base value) throws FHIRException { 4811 if (name.equals("identifier")) { 4812 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4813 } else if (name.equals("status")) { 4814 value = new AppointmentStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4815 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 4816 } else if (name.equals("cancellationReason")) { 4817 this.cancellationReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4818 } else if (name.equals("class")) { 4819 this.getClass_().add(TypeConvertor.castToCodeableConcept(value)); 4820 } else if (name.equals("serviceCategory")) { 4821 this.getServiceCategory().add(TypeConvertor.castToCodeableConcept(value)); 4822 } else if (name.equals("serviceType")) { 4823 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); 4824 } else if (name.equals("specialty")) { 4825 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); 4826 } else if (name.equals("appointmentType")) { 4827 this.appointmentType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4828 } else if (name.equals("reason")) { 4829 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 4830 } else if (name.equals("priority")) { 4831 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4832 } else if (name.equals("description")) { 4833 this.description = TypeConvertor.castToString(value); // StringType 4834 } else if (name.equals("replaces")) { 4835 this.getReplaces().add(TypeConvertor.castToReference(value)); 4836 } else if (name.equals("virtualService")) { 4837 this.getVirtualService().add(TypeConvertor.castToVirtualServiceDetail(value)); 4838 } else if (name.equals("supportingInformation")) { 4839 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 4840 } else if (name.equals("previousAppointment")) { 4841 this.previousAppointment = TypeConvertor.castToReference(value); // Reference 4842 } else if (name.equals("originatingAppointment")) { 4843 this.originatingAppointment = TypeConvertor.castToReference(value); // Reference 4844 } else if (name.equals("start")) { 4845 this.start = TypeConvertor.castToInstant(value); // InstantType 4846 } else if (name.equals("end")) { 4847 this.end = TypeConvertor.castToInstant(value); // InstantType 4848 } else if (name.equals("minutesDuration")) { 4849 this.minutesDuration = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4850 } else if (name.equals("requestedPeriod")) { 4851 this.getRequestedPeriod().add(TypeConvertor.castToPeriod(value)); 4852 } else if (name.equals("slot")) { 4853 this.getSlot().add(TypeConvertor.castToReference(value)); 4854 } else if (name.equals("account")) { 4855 this.getAccount().add(TypeConvertor.castToReference(value)); 4856 } else if (name.equals("created")) { 4857 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 4858 } else if (name.equals("cancellationDate")) { 4859 this.cancellationDate = TypeConvertor.castToDateTime(value); // DateTimeType 4860 } else if (name.equals("note")) { 4861 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4862 } else if (name.equals("patientInstruction")) { 4863 this.getPatientInstruction().add(TypeConvertor.castToCodeableReference(value)); 4864 } else if (name.equals("basedOn")) { 4865 this.getBasedOn().add(TypeConvertor.castToReference(value)); 4866 } else if (name.equals("subject")) { 4867 this.subject = TypeConvertor.castToReference(value); // Reference 4868 } else if (name.equals("participant")) { 4869 this.getParticipant().add((AppointmentParticipantComponent) value); 4870 } else if (name.equals("recurrenceId")) { 4871 this.recurrenceId = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4872 } else if (name.equals("occurrenceChanged")) { 4873 this.occurrenceChanged = TypeConvertor.castToBoolean(value); // BooleanType 4874 } else if (name.equals("recurrenceTemplate")) { 4875 this.getRecurrenceTemplate().add((AppointmentRecurrenceTemplateComponent) value); 4876 } else 4877 return super.setProperty(name, value); 4878 return value; 4879 } 4880 4881 @Override 4882 public void removeChild(String name, Base value) throws FHIRException { 4883 if (name.equals("identifier")) { 4884 this.getIdentifier().remove(value); 4885 } else if (name.equals("status")) { 4886 value = new AppointmentStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4887 this.status = (Enumeration) value; // Enumeration<AppointmentStatus> 4888 } else if (name.equals("cancellationReason")) { 4889 this.cancellationReason = null; 4890 } else if (name.equals("class")) { 4891 this.getClass_().remove(value); 4892 } else if (name.equals("serviceCategory")) { 4893 this.getServiceCategory().remove(value); 4894 } else if (name.equals("serviceType")) { 4895 this.getServiceType().remove(value); 4896 } else if (name.equals("specialty")) { 4897 this.getSpecialty().remove(value); 4898 } else if (name.equals("appointmentType")) { 4899 this.appointmentType = null; 4900 } else if (name.equals("reason")) { 4901 this.getReason().remove(value); 4902 } else if (name.equals("priority")) { 4903 this.priority = null; 4904 } else if (name.equals("description")) { 4905 this.description = null; 4906 } else if (name.equals("replaces")) { 4907 this.getReplaces().remove(value); 4908 } else if (name.equals("virtualService")) { 4909 this.getVirtualService().remove(value); 4910 } else if (name.equals("supportingInformation")) { 4911 this.getSupportingInformation().remove(value); 4912 } else if (name.equals("previousAppointment")) { 4913 this.previousAppointment = null; 4914 } else if (name.equals("originatingAppointment")) { 4915 this.originatingAppointment = null; 4916 } else if (name.equals("start")) { 4917 this.start = null; 4918 } else if (name.equals("end")) { 4919 this.end = null; 4920 } else if (name.equals("minutesDuration")) { 4921 this.minutesDuration = null; 4922 } else if (name.equals("requestedPeriod")) { 4923 this.getRequestedPeriod().remove(value); 4924 } else if (name.equals("slot")) { 4925 this.getSlot().remove(value); 4926 } else if (name.equals("account")) { 4927 this.getAccount().remove(value); 4928 } else if (name.equals("created")) { 4929 this.created = null; 4930 } else if (name.equals("cancellationDate")) { 4931 this.cancellationDate = null; 4932 } else if (name.equals("note")) { 4933 this.getNote().remove(value); 4934 } else if (name.equals("patientInstruction")) { 4935 this.getPatientInstruction().remove(value); 4936 } else if (name.equals("basedOn")) { 4937 this.getBasedOn().remove(value); 4938 } else if (name.equals("subject")) { 4939 this.subject = null; 4940 } else if (name.equals("participant")) { 4941 this.getParticipant().remove((AppointmentParticipantComponent) value); 4942 } else if (name.equals("recurrenceId")) { 4943 this.recurrenceId = null; 4944 } else if (name.equals("occurrenceChanged")) { 4945 this.occurrenceChanged = null; 4946 } else if (name.equals("recurrenceTemplate")) { 4947 this.getRecurrenceTemplate().remove((AppointmentRecurrenceTemplateComponent) value); 4948 } else 4949 super.removeChild(name, value); 4950 4951 } 4952 4953 @Override 4954 public Base makeProperty(int hash, String name) throws FHIRException { 4955 switch (hash) { 4956 case -1618432855: return addIdentifier(); 4957 case -892481550: return getStatusElement(); 4958 case 2135095591: return getCancellationReason(); 4959 case 94742904: return addClass_(); 4960 case 1281188563: return addServiceCategory(); 4961 case -1928370289: return addServiceType(); 4962 case -1694759682: return addSpecialty(); 4963 case -1596426375: return getAppointmentType(); 4964 case -934964668: return addReason(); 4965 case -1165461084: return getPriority(); 4966 case -1724546052: return getDescriptionElement(); 4967 case -430332865: return addReplaces(); 4968 case 1420774698: return addVirtualService(); 4969 case -1248768647: return addSupportingInformation(); 4970 case -1676044248: return getPreviousAppointment(); 4971 case 1841882230: return getOriginatingAppointment(); 4972 case 109757538: return getStartElement(); 4973 case 100571: return getEndElement(); 4974 case -413630573: return getMinutesDurationElement(); 4975 case -897241393: return addRequestedPeriod(); 4976 case 3533310: return addSlot(); 4977 case -1177318867: return addAccount(); 4978 case 1028554472: return getCreatedElement(); 4979 case 806269777: return getCancellationDateElement(); 4980 case 3387378: return addNote(); 4981 case 737543241: return addPatientInstruction(); 4982 case -332612366: return addBasedOn(); 4983 case -1867885268: return getSubject(); 4984 case 767422259: return addParticipant(); 4985 case -362407829: return getRecurrenceIdElement(); 4986 case 1779864483: return getOccurrenceChangedElement(); 4987 case 597629898: return addRecurrenceTemplate(); 4988 default: return super.makeProperty(hash, name); 4989 } 4990 4991 } 4992 4993 @Override 4994 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4995 switch (hash) { 4996 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4997 case -892481550: /*status*/ return new String[] {"code"}; 4998 case 2135095591: /*cancellationReason*/ return new String[] {"CodeableConcept"}; 4999 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 5000 case 1281188563: /*serviceCategory*/ return new String[] {"CodeableConcept"}; 5001 case -1928370289: /*serviceType*/ return new String[] {"CodeableReference"}; 5002 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 5003 case -1596426375: /*appointmentType*/ return new String[] {"CodeableConcept"}; 5004 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 5005 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 5006 case -1724546052: /*description*/ return new String[] {"string"}; 5007 case -430332865: /*replaces*/ return new String[] {"Reference"}; 5008 case 1420774698: /*virtualService*/ return new String[] {"VirtualServiceDetail"}; 5009 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 5010 case -1676044248: /*previousAppointment*/ return new String[] {"Reference"}; 5011 case 1841882230: /*originatingAppointment*/ return new String[] {"Reference"}; 5012 case 109757538: /*start*/ return new String[] {"instant"}; 5013 case 100571: /*end*/ return new String[] {"instant"}; 5014 case -413630573: /*minutesDuration*/ return new String[] {"positiveInt"}; 5015 case -897241393: /*requestedPeriod*/ return new String[] {"Period"}; 5016 case 3533310: /*slot*/ return new String[] {"Reference"}; 5017 case -1177318867: /*account*/ return new String[] {"Reference"}; 5018 case 1028554472: /*created*/ return new String[] {"dateTime"}; 5019 case 806269777: /*cancellationDate*/ return new String[] {"dateTime"}; 5020 case 3387378: /*note*/ return new String[] {"Annotation"}; 5021 case 737543241: /*patientInstruction*/ return new String[] {"CodeableReference"}; 5022 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 5023 case -1867885268: /*subject*/ return new String[] {"Reference"}; 5024 case 767422259: /*participant*/ return new String[] {}; 5025 case -362407829: /*recurrenceId*/ return new String[] {"positiveInt"}; 5026 case 1779864483: /*occurrenceChanged*/ return new String[] {"boolean"}; 5027 case 597629898: /*recurrenceTemplate*/ return new String[] {}; 5028 default: return super.getTypesForProperty(hash, name); 5029 } 5030 5031 } 5032 5033 @Override 5034 public Base addChild(String name) throws FHIRException { 5035 if (name.equals("identifier")) { 5036 return addIdentifier(); 5037 } 5038 else if (name.equals("status")) { 5039 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 5040 } 5041 else if (name.equals("cancellationReason")) { 5042 this.cancellationReason = new CodeableConcept(); 5043 return this.cancellationReason; 5044 } 5045 else if (name.equals("class")) { 5046 return addClass_(); 5047 } 5048 else if (name.equals("serviceCategory")) { 5049 return addServiceCategory(); 5050 } 5051 else if (name.equals("serviceType")) { 5052 return addServiceType(); 5053 } 5054 else if (name.equals("specialty")) { 5055 return addSpecialty(); 5056 } 5057 else if (name.equals("appointmentType")) { 5058 this.appointmentType = new CodeableConcept(); 5059 return this.appointmentType; 5060 } 5061 else if (name.equals("reason")) { 5062 return addReason(); 5063 } 5064 else if (name.equals("priority")) { 5065 this.priority = new CodeableConcept(); 5066 return this.priority; 5067 } 5068 else if (name.equals("description")) { 5069 throw new FHIRException("Cannot call addChild on a singleton property Appointment.description"); 5070 } 5071 else if (name.equals("replaces")) { 5072 return addReplaces(); 5073 } 5074 else if (name.equals("virtualService")) { 5075 return addVirtualService(); 5076 } 5077 else if (name.equals("supportingInformation")) { 5078 return addSupportingInformation(); 5079 } 5080 else if (name.equals("previousAppointment")) { 5081 this.previousAppointment = new Reference(); 5082 return this.previousAppointment; 5083 } 5084 else if (name.equals("originatingAppointment")) { 5085 this.originatingAppointment = new Reference(); 5086 return this.originatingAppointment; 5087 } 5088 else if (name.equals("start")) { 5089 throw new FHIRException("Cannot call addChild on a singleton property Appointment.start"); 5090 } 5091 else if (name.equals("end")) { 5092 throw new FHIRException("Cannot call addChild on a singleton property Appointment.end"); 5093 } 5094 else if (name.equals("minutesDuration")) { 5095 throw new FHIRException("Cannot call addChild on a singleton property Appointment.minutesDuration"); 5096 } 5097 else if (name.equals("requestedPeriod")) { 5098 return addRequestedPeriod(); 5099 } 5100 else if (name.equals("slot")) { 5101 return addSlot(); 5102 } 5103 else if (name.equals("account")) { 5104 return addAccount(); 5105 } 5106 else if (name.equals("created")) { 5107 throw new FHIRException("Cannot call addChild on a singleton property Appointment.created"); 5108 } 5109 else if (name.equals("cancellationDate")) { 5110 throw new FHIRException("Cannot call addChild on a singleton property Appointment.cancellationDate"); 5111 } 5112 else if (name.equals("note")) { 5113 return addNote(); 5114 } 5115 else if (name.equals("patientInstruction")) { 5116 return addPatientInstruction(); 5117 } 5118 else if (name.equals("basedOn")) { 5119 return addBasedOn(); 5120 } 5121 else if (name.equals("subject")) { 5122 this.subject = new Reference(); 5123 return this.subject; 5124 } 5125 else if (name.equals("participant")) { 5126 return addParticipant(); 5127 } 5128 else if (name.equals("recurrenceId")) { 5129 throw new FHIRException("Cannot call addChild on a singleton property Appointment.recurrenceId"); 5130 } 5131 else if (name.equals("occurrenceChanged")) { 5132 throw new FHIRException("Cannot call addChild on a singleton property Appointment.occurrenceChanged"); 5133 } 5134 else if (name.equals("recurrenceTemplate")) { 5135 return addRecurrenceTemplate(); 5136 } 5137 else 5138 return super.addChild(name); 5139 } 5140 5141 public String fhirType() { 5142 return "Appointment"; 5143 5144 } 5145 5146 public Appointment copy() { 5147 Appointment dst = new Appointment(); 5148 copyValues(dst); 5149 return dst; 5150 } 5151 5152 public void copyValues(Appointment dst) { 5153 super.copyValues(dst); 5154 if (identifier != null) { 5155 dst.identifier = new ArrayList<Identifier>(); 5156 for (Identifier i : identifier) 5157 dst.identifier.add(i.copy()); 5158 }; 5159 dst.status = status == null ? null : status.copy(); 5160 dst.cancellationReason = cancellationReason == null ? null : cancellationReason.copy(); 5161 if (class_ != null) { 5162 dst.class_ = new ArrayList<CodeableConcept>(); 5163 for (CodeableConcept i : class_) 5164 dst.class_.add(i.copy()); 5165 }; 5166 if (serviceCategory != null) { 5167 dst.serviceCategory = new ArrayList<CodeableConcept>(); 5168 for (CodeableConcept i : serviceCategory) 5169 dst.serviceCategory.add(i.copy()); 5170 }; 5171 if (serviceType != null) { 5172 dst.serviceType = new ArrayList<CodeableReference>(); 5173 for (CodeableReference i : serviceType) 5174 dst.serviceType.add(i.copy()); 5175 }; 5176 if (specialty != null) { 5177 dst.specialty = new ArrayList<CodeableConcept>(); 5178 for (CodeableConcept i : specialty) 5179 dst.specialty.add(i.copy()); 5180 }; 5181 dst.appointmentType = appointmentType == null ? null : appointmentType.copy(); 5182 if (reason != null) { 5183 dst.reason = new ArrayList<CodeableReference>(); 5184 for (CodeableReference i : reason) 5185 dst.reason.add(i.copy()); 5186 }; 5187 dst.priority = priority == null ? null : priority.copy(); 5188 dst.description = description == null ? null : description.copy(); 5189 if (replaces != null) { 5190 dst.replaces = new ArrayList<Reference>(); 5191 for (Reference i : replaces) 5192 dst.replaces.add(i.copy()); 5193 }; 5194 if (virtualService != null) { 5195 dst.virtualService = new ArrayList<VirtualServiceDetail>(); 5196 for (VirtualServiceDetail i : virtualService) 5197 dst.virtualService.add(i.copy()); 5198 }; 5199 if (supportingInformation != null) { 5200 dst.supportingInformation = new ArrayList<Reference>(); 5201 for (Reference i : supportingInformation) 5202 dst.supportingInformation.add(i.copy()); 5203 }; 5204 dst.previousAppointment = previousAppointment == null ? null : previousAppointment.copy(); 5205 dst.originatingAppointment = originatingAppointment == null ? null : originatingAppointment.copy(); 5206 dst.start = start == null ? null : start.copy(); 5207 dst.end = end == null ? null : end.copy(); 5208 dst.minutesDuration = minutesDuration == null ? null : minutesDuration.copy(); 5209 if (requestedPeriod != null) { 5210 dst.requestedPeriod = new ArrayList<Period>(); 5211 for (Period i : requestedPeriod) 5212 dst.requestedPeriod.add(i.copy()); 5213 }; 5214 if (slot != null) { 5215 dst.slot = new ArrayList<Reference>(); 5216 for (Reference i : slot) 5217 dst.slot.add(i.copy()); 5218 }; 5219 if (account != null) { 5220 dst.account = new ArrayList<Reference>(); 5221 for (Reference i : account) 5222 dst.account.add(i.copy()); 5223 }; 5224 dst.created = created == null ? null : created.copy(); 5225 dst.cancellationDate = cancellationDate == null ? null : cancellationDate.copy(); 5226 if (note != null) { 5227 dst.note = new ArrayList<Annotation>(); 5228 for (Annotation i : note) 5229 dst.note.add(i.copy()); 5230 }; 5231 if (patientInstruction != null) { 5232 dst.patientInstruction = new ArrayList<CodeableReference>(); 5233 for (CodeableReference i : patientInstruction) 5234 dst.patientInstruction.add(i.copy()); 5235 }; 5236 if (basedOn != null) { 5237 dst.basedOn = new ArrayList<Reference>(); 5238 for (Reference i : basedOn) 5239 dst.basedOn.add(i.copy()); 5240 }; 5241 dst.subject = subject == null ? null : subject.copy(); 5242 if (participant != null) { 5243 dst.participant = new ArrayList<AppointmentParticipantComponent>(); 5244 for (AppointmentParticipantComponent i : participant) 5245 dst.participant.add(i.copy()); 5246 }; 5247 dst.recurrenceId = recurrenceId == null ? null : recurrenceId.copy(); 5248 dst.occurrenceChanged = occurrenceChanged == null ? null : occurrenceChanged.copy(); 5249 if (recurrenceTemplate != null) { 5250 dst.recurrenceTemplate = new ArrayList<AppointmentRecurrenceTemplateComponent>(); 5251 for (AppointmentRecurrenceTemplateComponent i : recurrenceTemplate) 5252 dst.recurrenceTemplate.add(i.copy()); 5253 }; 5254 } 5255 5256 protected Appointment typedCopy() { 5257 return copy(); 5258 } 5259 5260 @Override 5261 public boolean equalsDeep(Base other_) { 5262 if (!super.equalsDeep(other_)) 5263 return false; 5264 if (!(other_ instanceof Appointment)) 5265 return false; 5266 Appointment o = (Appointment) other_; 5267 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(cancellationReason, o.cancellationReason, true) 5268 && compareDeep(class_, o.class_, true) && compareDeep(serviceCategory, o.serviceCategory, true) 5269 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) && compareDeep(appointmentType, o.appointmentType, true) 5270 && compareDeep(reason, o.reason, true) && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) 5271 && compareDeep(replaces, o.replaces, true) && compareDeep(virtualService, o.virtualService, true) 5272 && compareDeep(supportingInformation, o.supportingInformation, true) && compareDeep(previousAppointment, o.previousAppointment, true) 5273 && compareDeep(originatingAppointment, o.originatingAppointment, true) && compareDeep(start, o.start, true) 5274 && compareDeep(end, o.end, true) && compareDeep(minutesDuration, o.minutesDuration, true) && compareDeep(requestedPeriod, o.requestedPeriod, true) 5275 && compareDeep(slot, o.slot, true) && compareDeep(account, o.account, true) && compareDeep(created, o.created, true) 5276 && compareDeep(cancellationDate, o.cancellationDate, true) && compareDeep(note, o.note, true) && compareDeep(patientInstruction, o.patientInstruction, true) 5277 && compareDeep(basedOn, o.basedOn, true) && compareDeep(subject, o.subject, true) && compareDeep(participant, o.participant, true) 5278 && compareDeep(recurrenceId, o.recurrenceId, true) && compareDeep(occurrenceChanged, o.occurrenceChanged, true) 5279 && compareDeep(recurrenceTemplate, o.recurrenceTemplate, true); 5280 } 5281 5282 @Override 5283 public boolean equalsShallow(Base other_) { 5284 if (!super.equalsShallow(other_)) 5285 return false; 5286 if (!(other_ instanceof Appointment)) 5287 return false; 5288 Appointment o = (Appointment) other_; 5289 return compareValues(status, o.status, true) && compareValues(description, o.description, true) && compareValues(start, o.start, true) 5290 && compareValues(end, o.end, true) && compareValues(minutesDuration, o.minutesDuration, true) && compareValues(created, o.created, true) 5291 && compareValues(cancellationDate, o.cancellationDate, true) && compareValues(recurrenceId, o.recurrenceId, true) 5292 && compareValues(occurrenceChanged, o.occurrenceChanged, true); 5293 } 5294 5295 public boolean isEmpty() { 5296 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, cancellationReason 5297 , class_, serviceCategory, serviceType, specialty, appointmentType, reason, priority 5298 , description, replaces, virtualService, supportingInformation, previousAppointment 5299 , originatingAppointment, start, end, minutesDuration, requestedPeriod, slot, account 5300 , created, cancellationDate, note, patientInstruction, basedOn, subject, participant 5301 , recurrenceId, occurrenceChanged, recurrenceTemplate); 5302 } 5303 5304 @Override 5305 public ResourceType getResourceType() { 5306 return ResourceType.Appointment; 5307 } 5308 5309 /** 5310 * Search parameter: <b>actor</b> 5311 * <p> 5312 * Description: <b>Any one of the individuals participating in the appointment</b><br> 5313 * Type: <b>reference</b><br> 5314 * Path: <b>Appointment.participant.actor</b><br> 5315 * </p> 5316 */ 5317 @SearchParamDefinition(name="actor", path="Appointment.participant.actor", description="Any one of the individuals participating in the appointment", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 5318 public static final String SP_ACTOR = "actor"; 5319 /** 5320 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 5321 * <p> 5322 * Description: <b>Any one of the individuals participating in the appointment</b><br> 5323 * Type: <b>reference</b><br> 5324 * Path: <b>Appointment.participant.actor</b><br> 5325 * </p> 5326 */ 5327 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 5328 5329/** 5330 * Constant for fluent queries to be used to add include statements. Specifies 5331 * the path value of "<b>Appointment:actor</b>". 5332 */ 5333 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Appointment:actor").toLocked(); 5334 5335 /** 5336 * Search parameter: <b>appointment-type</b> 5337 * <p> 5338 * Description: <b>The style of appointment or patient that has been booked in the slot (not service type)</b><br> 5339 * Type: <b>token</b><br> 5340 * Path: <b>Appointment.appointmentType</b><br> 5341 * </p> 5342 */ 5343 @SearchParamDefinition(name="appointment-type", path="Appointment.appointmentType", description="The style of appointment or patient that has been booked in the slot (not service type)", type="token" ) 5344 public static final String SP_APPOINTMENT_TYPE = "appointment-type"; 5345 /** 5346 * <b>Fluent Client</b> search parameter constant for <b>appointment-type</b> 5347 * <p> 5348 * Description: <b>The style of appointment or patient that has been booked in the slot (not service type)</b><br> 5349 * Type: <b>token</b><br> 5350 * Path: <b>Appointment.appointmentType</b><br> 5351 * </p> 5352 */ 5353 public static final ca.uhn.fhir.rest.gclient.TokenClientParam APPOINTMENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_APPOINTMENT_TYPE); 5354 5355 /** 5356 * Search parameter: <b>based-on</b> 5357 * <p> 5358 * Description: <b>The service request this appointment is allocated to assess</b><br> 5359 * Type: <b>reference</b><br> 5360 * Path: <b>Appointment.basedOn</b><br> 5361 * </p> 5362 */ 5363 @SearchParamDefinition(name="based-on", path="Appointment.basedOn", description="The service request this appointment is allocated to assess", type="reference", target={CarePlan.class, DeviceRequest.class, MedicationRequest.class, ServiceRequest.class } ) 5364 public static final String SP_BASED_ON = "based-on"; 5365 /** 5366 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 5367 * <p> 5368 * Description: <b>The service request this appointment is allocated to assess</b><br> 5369 * Type: <b>reference</b><br> 5370 * Path: <b>Appointment.basedOn</b><br> 5371 * </p> 5372 */ 5373 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 5374 5375/** 5376 * Constant for fluent queries to be used to add include statements. Specifies 5377 * the path value of "<b>Appointment:based-on</b>". 5378 */ 5379 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Appointment:based-on").toLocked(); 5380 5381 /** 5382 * Search parameter: <b>group</b> 5383 * <p> 5384 * Description: <b>One of the individuals of the appointment is this patient</b><br> 5385 * Type: <b>reference</b><br> 5386 * Path: <b>Appointment.participant.actor.where(resolve() is Group) | Appointment.subject.where(resolve() is Group)</b><br> 5387 * </p> 5388 */ 5389 @SearchParamDefinition(name="group", path="Appointment.participant.actor.where(resolve() is Group) | Appointment.subject.where(resolve() is Group)", description="One of the individuals of the appointment is this patient", type="reference", target={Group.class } ) 5390 public static final String SP_GROUP = "group"; 5391 /** 5392 * <b>Fluent Client</b> search parameter constant for <b>group</b> 5393 * <p> 5394 * Description: <b>One of the individuals of the appointment is this patient</b><br> 5395 * Type: <b>reference</b><br> 5396 * Path: <b>Appointment.participant.actor.where(resolve() is Group) | Appointment.subject.where(resolve() is Group)</b><br> 5397 * </p> 5398 */ 5399 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GROUP = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GROUP); 5400 5401/** 5402 * Constant for fluent queries to be used to add include statements. Specifies 5403 * the path value of "<b>Appointment:group</b>". 5404 */ 5405 public static final ca.uhn.fhir.model.api.Include INCLUDE_GROUP = new ca.uhn.fhir.model.api.Include("Appointment:group").toLocked(); 5406 5407 /** 5408 * Search parameter: <b>location</b> 5409 * <p> 5410 * Description: <b>This location is listed in the participants of the appointment</b><br> 5411 * Type: <b>reference</b><br> 5412 * Path: <b>Appointment.participant.actor.where(resolve() is Location)</b><br> 5413 * </p> 5414 */ 5415 @SearchParamDefinition(name="location", path="Appointment.participant.actor.where(resolve() is Location)", description="This location is listed in the participants of the appointment", type="reference", target={Location.class } ) 5416 public static final String SP_LOCATION = "location"; 5417 /** 5418 * <b>Fluent Client</b> search parameter constant for <b>location</b> 5419 * <p> 5420 * Description: <b>This location is listed in the participants of the appointment</b><br> 5421 * Type: <b>reference</b><br> 5422 * Path: <b>Appointment.participant.actor.where(resolve() is Location)</b><br> 5423 * </p> 5424 */ 5425 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 5426 5427/** 5428 * Constant for fluent queries to be used to add include statements. Specifies 5429 * the path value of "<b>Appointment:location</b>". 5430 */ 5431 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Appointment:location").toLocked(); 5432 5433 /** 5434 * Search parameter: <b>part-status</b> 5435 * <p> 5436 * Description: <b>The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.</b><br> 5437 * Type: <b>token</b><br> 5438 * Path: <b>Appointment.participant.status</b><br> 5439 * </p> 5440 */ 5441 @SearchParamDefinition(name="part-status", path="Appointment.participant.status", description="The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.", type="token" ) 5442 public static final String SP_PART_STATUS = "part-status"; 5443 /** 5444 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 5445 * <p> 5446 * Description: <b>The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.</b><br> 5447 * Type: <b>token</b><br> 5448 * Path: <b>Appointment.participant.status</b><br> 5449 * </p> 5450 */ 5451 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PART_STATUS); 5452 5453 /** 5454 * Search parameter: <b>practitioner</b> 5455 * <p> 5456 * Description: <b>One of the individuals of the appointment is this practitioner</b><br> 5457 * Type: <b>reference</b><br> 5458 * Path: <b>Appointment.participant.actor.where(resolve() is Practitioner)</b><br> 5459 * </p> 5460 */ 5461 @SearchParamDefinition(name="practitioner", path="Appointment.participant.actor.where(resolve() is Practitioner)", description="One of the individuals of the appointment is this practitioner", type="reference", target={Practitioner.class } ) 5462 public static final String SP_PRACTITIONER = "practitioner"; 5463 /** 5464 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 5465 * <p> 5466 * Description: <b>One of the individuals of the appointment is this practitioner</b><br> 5467 * Type: <b>reference</b><br> 5468 * Path: <b>Appointment.participant.actor.where(resolve() is Practitioner)</b><br> 5469 * </p> 5470 */ 5471 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 5472 5473/** 5474 * Constant for fluent queries to be used to add include statements. Specifies 5475 * the path value of "<b>Appointment:practitioner</b>". 5476 */ 5477 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Appointment:practitioner").toLocked(); 5478 5479 /** 5480 * Search parameter: <b>reason-code</b> 5481 * <p> 5482 * Description: <b>Reference to a concept (by class)</b><br> 5483 * Type: <b>token</b><br> 5484 * Path: <b>Appointment.reason.concept</b><br> 5485 * </p> 5486 */ 5487 @SearchParamDefinition(name="reason-code", path="Appointment.reason.concept", description="Reference to a concept (by class)", type="token" ) 5488 public static final String SP_REASON_CODE = "reason-code"; 5489 /** 5490 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 5491 * <p> 5492 * Description: <b>Reference to a concept (by class)</b><br> 5493 * Type: <b>token</b><br> 5494 * Path: <b>Appointment.reason.concept</b><br> 5495 * </p> 5496 */ 5497 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_CODE); 5498 5499 /** 5500 * Search parameter: <b>reason-reference</b> 5501 * <p> 5502 * Description: <b>Reference to a resource (by instance)</b><br> 5503 * Type: <b>reference</b><br> 5504 * Path: <b>Appointment.reason.reference</b><br> 5505 * </p> 5506 */ 5507 @SearchParamDefinition(name="reason-reference", path="Appointment.reason.reference", description="Reference to a resource (by instance)", type="reference", target={Condition.class, ImmunizationRecommendation.class, Observation.class, Procedure.class } ) 5508 public static final String SP_REASON_REFERENCE = "reason-reference"; 5509 /** 5510 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 5511 * <p> 5512 * Description: <b>Reference to a resource (by instance)</b><br> 5513 * Type: <b>reference</b><br> 5514 * Path: <b>Appointment.reason.reference</b><br> 5515 * </p> 5516 */ 5517 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REASON_REFERENCE); 5518 5519/** 5520 * Constant for fluent queries to be used to add include statements. Specifies 5521 * the path value of "<b>Appointment:reason-reference</b>". 5522 */ 5523 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include("Appointment:reason-reference").toLocked(); 5524 5525 /** 5526 * Search parameter: <b>requested-period</b> 5527 * <p> 5528 * Description: <b>During what period was the Appointment requested to take place</b><br> 5529 * Type: <b>date</b><br> 5530 * Path: <b>requestedPeriod</b><br> 5531 * </p> 5532 */ 5533 @SearchParamDefinition(name="requested-period", path="requestedPeriod", description="During what period was the Appointment requested to take place", type="date" ) 5534 public static final String SP_REQUESTED_PERIOD = "requested-period"; 5535 /** 5536 * <b>Fluent Client</b> search parameter constant for <b>requested-period</b> 5537 * <p> 5538 * Description: <b>During what period was the Appointment requested to take place</b><br> 5539 * Type: <b>date</b><br> 5540 * Path: <b>requestedPeriod</b><br> 5541 * </p> 5542 */ 5543 public static final ca.uhn.fhir.rest.gclient.DateClientParam REQUESTED_PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_REQUESTED_PERIOD); 5544 5545 /** 5546 * Search parameter: <b>service-category</b> 5547 * <p> 5548 * Description: <b>A broad categorization of the service that is to be performed during this appointment</b><br> 5549 * Type: <b>token</b><br> 5550 * Path: <b>Appointment.serviceCategory</b><br> 5551 * </p> 5552 */ 5553 @SearchParamDefinition(name="service-category", path="Appointment.serviceCategory", description="A broad categorization of the service that is to be performed during this appointment", type="token" ) 5554 public static final String SP_SERVICE_CATEGORY = "service-category"; 5555 /** 5556 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 5557 * <p> 5558 * Description: <b>A broad categorization of the service that is to be performed during this appointment</b><br> 5559 * Type: <b>token</b><br> 5560 * Path: <b>Appointment.serviceCategory</b><br> 5561 * </p> 5562 */ 5563 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_CATEGORY); 5564 5565 /** 5566 * Search parameter: <b>service-type-reference</b> 5567 * <p> 5568 * Description: <b>The specific service (by HealthcareService) that is to be performed during this appointment</b><br> 5569 * Type: <b>reference</b><br> 5570 * Path: <b>Appointment.serviceType.reference</b><br> 5571 * </p> 5572 */ 5573 @SearchParamDefinition(name="service-type-reference", path="Appointment.serviceType.reference", description="The specific service (by HealthcareService) that is to be performed during this appointment", type="reference", target={HealthcareService.class } ) 5574 public static final String SP_SERVICE_TYPE_REFERENCE = "service-type-reference"; 5575 /** 5576 * <b>Fluent Client</b> search parameter constant for <b>service-type-reference</b> 5577 * <p> 5578 * Description: <b>The specific service (by HealthcareService) that is to be performed during this appointment</b><br> 5579 * Type: <b>reference</b><br> 5580 * Path: <b>Appointment.serviceType.reference</b><br> 5581 * </p> 5582 */ 5583 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_TYPE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE_TYPE_REFERENCE); 5584 5585/** 5586 * Constant for fluent queries to be used to add include statements. Specifies 5587 * the path value of "<b>Appointment:service-type-reference</b>". 5588 */ 5589 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_TYPE_REFERENCE = new ca.uhn.fhir.model.api.Include("Appointment:service-type-reference").toLocked(); 5590 5591 /** 5592 * Search parameter: <b>service-type</b> 5593 * <p> 5594 * Description: <b>The specific service (by coding) that is to be performed during this appointment</b><br> 5595 * Type: <b>token</b><br> 5596 * Path: <b>Appointment.serviceType.concept</b><br> 5597 * </p> 5598 */ 5599 @SearchParamDefinition(name="service-type", path="Appointment.serviceType.concept", description="The specific service (by coding) that is to be performed during this appointment", type="token" ) 5600 public static final String SP_SERVICE_TYPE = "service-type"; 5601 /** 5602 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 5603 * <p> 5604 * Description: <b>The specific service (by coding) that is to be performed during this appointment</b><br> 5605 * Type: <b>token</b><br> 5606 * Path: <b>Appointment.serviceType.concept</b><br> 5607 * </p> 5608 */ 5609 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_TYPE); 5610 5611 /** 5612 * Search parameter: <b>slot</b> 5613 * <p> 5614 * Description: <b>The slots that this appointment is filling</b><br> 5615 * Type: <b>reference</b><br> 5616 * Path: <b>Appointment.slot</b><br> 5617 * </p> 5618 */ 5619 @SearchParamDefinition(name="slot", path="Appointment.slot", description="The slots that this appointment is filling", type="reference", target={Slot.class } ) 5620 public static final String SP_SLOT = "slot"; 5621 /** 5622 * <b>Fluent Client</b> search parameter constant for <b>slot</b> 5623 * <p> 5624 * Description: <b>The slots that this appointment is filling</b><br> 5625 * Type: <b>reference</b><br> 5626 * Path: <b>Appointment.slot</b><br> 5627 * </p> 5628 */ 5629 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SLOT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SLOT); 5630 5631/** 5632 * Constant for fluent queries to be used to add include statements. Specifies 5633 * the path value of "<b>Appointment:slot</b>". 5634 */ 5635 public static final ca.uhn.fhir.model.api.Include INCLUDE_SLOT = new ca.uhn.fhir.model.api.Include("Appointment:slot").toLocked(); 5636 5637 /** 5638 * Search parameter: <b>specialty</b> 5639 * <p> 5640 * Description: <b>The specialty of a practitioner that would be required to perform the service requested in this appointment</b><br> 5641 * Type: <b>token</b><br> 5642 * Path: <b>Appointment.specialty</b><br> 5643 * </p> 5644 */ 5645 @SearchParamDefinition(name="specialty", path="Appointment.specialty", description="The specialty of a practitioner that would be required to perform the service requested in this appointment", type="token" ) 5646 public static final String SP_SPECIALTY = "specialty"; 5647 /** 5648 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 5649 * <p> 5650 * Description: <b>The specialty of a practitioner that would be required to perform the service requested in this appointment</b><br> 5651 * Type: <b>token</b><br> 5652 * Path: <b>Appointment.specialty</b><br> 5653 * </p> 5654 */ 5655 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 5656 5657 /** 5658 * Search parameter: <b>status</b> 5659 * <p> 5660 * Description: <b>The overall status of the appointment</b><br> 5661 * Type: <b>token</b><br> 5662 * Path: <b>Appointment.status</b><br> 5663 * </p> 5664 */ 5665 @SearchParamDefinition(name="status", path="Appointment.status", description="The overall status of the appointment", type="token" ) 5666 public static final String SP_STATUS = "status"; 5667 /** 5668 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5669 * <p> 5670 * Description: <b>The overall status of the appointment</b><br> 5671 * Type: <b>token</b><br> 5672 * Path: <b>Appointment.status</b><br> 5673 * </p> 5674 */ 5675 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5676 5677 /** 5678 * Search parameter: <b>subject</b> 5679 * <p> 5680 * Description: <b>One of the individuals of the appointment is this patient</b><br> 5681 * Type: <b>reference</b><br> 5682 * Path: <b>Appointment.subject</b><br> 5683 * </p> 5684 */ 5685 @SearchParamDefinition(name="subject", path="Appointment.subject", description="One of the individuals of the appointment is this patient", type="reference", target={Group.class, Patient.class } ) 5686 public static final String SP_SUBJECT = "subject"; 5687 /** 5688 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5689 * <p> 5690 * Description: <b>One of the individuals of the appointment is this patient</b><br> 5691 * Type: <b>reference</b><br> 5692 * Path: <b>Appointment.subject</b><br> 5693 * </p> 5694 */ 5695 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 5696 5697/** 5698 * Constant for fluent queries to be used to add include statements. Specifies 5699 * the path value of "<b>Appointment:subject</b>". 5700 */ 5701 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Appointment:subject").toLocked(); 5702 5703 /** 5704 * Search parameter: <b>supporting-info</b> 5705 * <p> 5706 * Description: <b>Additional information to support the appointment</b><br> 5707 * Type: <b>reference</b><br> 5708 * Path: <b>Appointment.supportingInformation</b><br> 5709 * </p> 5710 */ 5711 @SearchParamDefinition(name="supporting-info", path="Appointment.supportingInformation", description="Additional information to support the appointment", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 5712 public static final String SP_SUPPORTING_INFO = "supporting-info"; 5713 /** 5714 * <b>Fluent Client</b> search parameter constant for <b>supporting-info</b> 5715 * <p> 5716 * Description: <b>Additional information to support the appointment</b><br> 5717 * Type: <b>reference</b><br> 5718 * Path: <b>Appointment.supportingInformation</b><br> 5719 * </p> 5720 */ 5721 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTING_INFO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPORTING_INFO); 5722 5723/** 5724 * Constant for fluent queries to be used to add include statements. Specifies 5725 * the path value of "<b>Appointment:supporting-info</b>". 5726 */ 5727 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTING_INFO = new ca.uhn.fhir.model.api.Include("Appointment:supporting-info").toLocked(); 5728 5729 /** 5730 * Search parameter: <b>date</b> 5731 * <p> 5732 * Description: <b>Multiple Resources: 5733 5734* [AdverseEvent](adverseevent.html): When the event occurred 5735* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 5736* [Appointment](appointment.html): Appointment date/time. 5737* [AuditEvent](auditevent.html): Time when the event was recorded 5738* [CarePlan](careplan.html): Time period plan covers 5739* [CareTeam](careteam.html): A date within the coverage time period. 5740* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 5741* [Composition](composition.html): Composition editing time 5742* [Consent](consent.html): When consent was agreed to 5743* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 5744* [DocumentReference](documentreference.html): When this document reference was created 5745* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 5746* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 5747* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 5748* [Flag](flag.html): Time period when flag is active 5749* [Immunization](immunization.html): Vaccination (non)-Administration Date 5750* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 5751* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 5752* [Invoice](invoice.html): Invoice date / posting date 5753* [List](list.html): When the list was prepared 5754* [MeasureReport](measurereport.html): The date of the measure report 5755* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 5756* [Observation](observation.html): Clinically relevant time/time-period for observation 5757* [Procedure](procedure.html): When the procedure occurred or is occurring 5758* [ResearchSubject](researchsubject.html): Start and end of participation 5759* [RiskAssessment](riskassessment.html): When was assessment made? 5760* [SupplyRequest](supplyrequest.html): When the request was made 5761</b><br> 5762 * Type: <b>date</b><br> 5763 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 5764 * </p> 5765 */ 5766 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 5767 public static final String SP_DATE = "date"; 5768 /** 5769 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5770 * <p> 5771 * Description: <b>Multiple Resources: 5772 5773* [AdverseEvent](adverseevent.html): When the event occurred 5774* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 5775* [Appointment](appointment.html): Appointment date/time. 5776* [AuditEvent](auditevent.html): Time when the event was recorded 5777* [CarePlan](careplan.html): Time period plan covers 5778* [CareTeam](careteam.html): A date within the coverage time period. 5779* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 5780* [Composition](composition.html): Composition editing time 5781* [Consent](consent.html): When consent was agreed to 5782* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 5783* [DocumentReference](documentreference.html): When this document reference was created 5784* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 5785* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 5786* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 5787* [Flag](flag.html): Time period when flag is active 5788* [Immunization](immunization.html): Vaccination (non)-Administration Date 5789* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 5790* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 5791* [Invoice](invoice.html): Invoice date / posting date 5792* [List](list.html): When the list was prepared 5793* [MeasureReport](measurereport.html): The date of the measure report 5794* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 5795* [Observation](observation.html): Clinically relevant time/time-period for observation 5796* [Procedure](procedure.html): When the procedure occurred or is occurring 5797* [ResearchSubject](researchsubject.html): Start and end of participation 5798* [RiskAssessment](riskassessment.html): When was assessment made? 5799* [SupplyRequest](supplyrequest.html): When the request was made 5800</b><br> 5801 * Type: <b>date</b><br> 5802 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 5803 * </p> 5804 */ 5805 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5806 5807 /** 5808 * Search parameter: <b>identifier</b> 5809 * <p> 5810 * Description: <b>Multiple Resources: 5811 5812* [Account](account.html): Account number 5813* [AdverseEvent](adverseevent.html): Business identifier for the event 5814* [AllergyIntolerance](allergyintolerance.html): External ids for this item 5815* [Appointment](appointment.html): An Identifier of the Appointment 5816* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 5817* [Basic](basic.html): Business identifier 5818* [BodyStructure](bodystructure.html): Bodystructure identifier 5819* [CarePlan](careplan.html): External Ids for this plan 5820* [CareTeam](careteam.html): External Ids for this team 5821* [ChargeItem](chargeitem.html): Business Identifier for item 5822* [Claim](claim.html): The primary identifier of the financial resource 5823* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 5824* [ClinicalImpression](clinicalimpression.html): Business identifier 5825* [Communication](communication.html): Unique identifier 5826* [CommunicationRequest](communicationrequest.html): Unique identifier 5827* [Composition](composition.html): Version-independent identifier for the Composition 5828* [Condition](condition.html): A unique identifier of the condition record 5829* [Consent](consent.html): Identifier for this record (external references) 5830* [Contract](contract.html): The identity of the contract 5831* [Coverage](coverage.html): The primary identifier of the insured and the coverage 5832* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 5833* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 5834* [DetectedIssue](detectedissue.html): Unique id for the detected issue 5835* [DeviceRequest](devicerequest.html): Business identifier for request/order 5836* [DeviceUsage](deviceusage.html): Search by identifier 5837* [DiagnosticReport](diagnosticreport.html): An identifier for the report 5838* [DocumentReference](documentreference.html): Identifier of the attachment binary 5839* [Encounter](encounter.html): Identifier(s) by which this encounter is known 5840* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 5841* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 5842* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 5843* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 5844* [Flag](flag.html): Business identifier 5845* [Goal](goal.html): External Ids for this goal 5846* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 5847* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 5848* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 5849* [Immunization](immunization.html): Business identifier 5850* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 5851* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 5852* [Invoice](invoice.html): Business Identifier for item 5853* [List](list.html): Business identifier 5854* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5855* [Medication](medication.html): Returns medications with this external identifier 5856* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5857* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5858* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5859* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5860* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5861* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5862* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5863* [Observation](observation.html): The unique id for a particular observation 5864* [Person](person.html): A person Identifier 5865* [Procedure](procedure.html): A unique identifier for a procedure 5866* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5867* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5868* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5869* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5870* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5871* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5872* [Specimen](specimen.html): The unique identifier associated with the specimen 5873* [SupplyDelivery](supplydelivery.html): External identifier 5874* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5875* [Task](task.html): Search for a task instance by its business identifier 5876* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5877</b><br> 5878 * Type: <b>token</b><br> 5879 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5880 * </p> 5881 */ 5882 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 5883 public static final String SP_IDENTIFIER = "identifier"; 5884 /** 5885 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5886 * <p> 5887 * Description: <b>Multiple Resources: 5888 5889* [Account](account.html): Account number 5890* [AdverseEvent](adverseevent.html): Business identifier for the event 5891* [AllergyIntolerance](allergyintolerance.html): External ids for this item 5892* [Appointment](appointment.html): An Identifier of the Appointment 5893* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 5894* [Basic](basic.html): Business identifier 5895* [BodyStructure](bodystructure.html): Bodystructure identifier 5896* [CarePlan](careplan.html): External Ids for this plan 5897* [CareTeam](careteam.html): External Ids for this team 5898* [ChargeItem](chargeitem.html): Business Identifier for item 5899* [Claim](claim.html): The primary identifier of the financial resource 5900* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 5901* [ClinicalImpression](clinicalimpression.html): Business identifier 5902* [Communication](communication.html): Unique identifier 5903* [CommunicationRequest](communicationrequest.html): Unique identifier 5904* [Composition](composition.html): Version-independent identifier for the Composition 5905* [Condition](condition.html): A unique identifier of the condition record 5906* [Consent](consent.html): Identifier for this record (external references) 5907* [Contract](contract.html): The identity of the contract 5908* [Coverage](coverage.html): The primary identifier of the insured and the coverage 5909* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 5910* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 5911* [DetectedIssue](detectedissue.html): Unique id for the detected issue 5912* [DeviceRequest](devicerequest.html): Business identifier for request/order 5913* [DeviceUsage](deviceusage.html): Search by identifier 5914* [DiagnosticReport](diagnosticreport.html): An identifier for the report 5915* [DocumentReference](documentreference.html): Identifier of the attachment binary 5916* [Encounter](encounter.html): Identifier(s) by which this encounter is known 5917* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 5918* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 5919* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 5920* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 5921* [Flag](flag.html): Business identifier 5922* [Goal](goal.html): External Ids for this goal 5923* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 5924* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 5925* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 5926* [Immunization](immunization.html): Business identifier 5927* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 5928* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 5929* [Invoice](invoice.html): Business Identifier for item 5930* [List](list.html): Business identifier 5931* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 5932* [Medication](medication.html): Returns medications with this external identifier 5933* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 5934* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 5935* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 5936* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 5937* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 5938* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 5939* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 5940* [Observation](observation.html): The unique id for a particular observation 5941* [Person](person.html): A person Identifier 5942* [Procedure](procedure.html): A unique identifier for a procedure 5943* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 5944* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 5945* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 5946* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 5947* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 5948* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 5949* [Specimen](specimen.html): The unique identifier associated with the specimen 5950* [SupplyDelivery](supplydelivery.html): External identifier 5951* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 5952* [Task](task.html): Search for a task instance by its business identifier 5953* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 5954</b><br> 5955 * Type: <b>token</b><br> 5956 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 5957 * </p> 5958 */ 5959 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5960 5961 /** 5962 * Search parameter: <b>patient</b> 5963 * <p> 5964 * Description: <b>Multiple Resources: 5965 5966* [Account](account.html): The entity that caused the expenses 5967* [AdverseEvent](adverseevent.html): Subject impacted by event 5968* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 5969* [Appointment](appointment.html): One of the individuals of the appointment is this patient 5970* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 5971* [AuditEvent](auditevent.html): Where the activity involved patient data 5972* [Basic](basic.html): Identifies the focus of this resource 5973* [BodyStructure](bodystructure.html): Who this is about 5974* [CarePlan](careplan.html): Who the care plan is for 5975* [CareTeam](careteam.html): Who care team is for 5976* [ChargeItem](chargeitem.html): Individual service was done for/to 5977* [Claim](claim.html): Patient receiving the products or services 5978* [ClaimResponse](claimresponse.html): The subject of care 5979* [ClinicalImpression](clinicalimpression.html): Patient assessed 5980* [Communication](communication.html): Focus of message 5981* [CommunicationRequest](communicationrequest.html): Focus of message 5982* [Composition](composition.html): Who and/or what the composition is about 5983* [Condition](condition.html): Who has the condition? 5984* [Consent](consent.html): Who the consent applies to 5985* [Contract](contract.html): The identity of the subject of the contract (if a patient) 5986* [Coverage](coverage.html): Retrieve coverages for a patient 5987* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 5988* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 5989* [DetectedIssue](detectedissue.html): Associated patient 5990* [DeviceRequest](devicerequest.html): Individual the service is ordered for 5991* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 5992* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 5993* [DocumentReference](documentreference.html): Who/what is the subject of the document 5994* [Encounter](encounter.html): The patient present at the encounter 5995* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 5996* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 5997* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5998* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5999* [Flag](flag.html): The identity of a subject to list flags for 6000* [Goal](goal.html): Who this goal is intended for 6001* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6002* [ImagingSelection](imagingselection.html): Who the study is about 6003* [ImagingStudy](imagingstudy.html): Who the study is about 6004* [Immunization](immunization.html): The patient for the vaccination record 6005* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6006* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6007* [Invoice](invoice.html): Recipient(s) of goods and services 6008* [List](list.html): If all resources have the same subject 6009* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6010* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6011* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6012* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6013* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6014* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6015* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6016* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6017* [Observation](observation.html): The subject that the observation is about (if patient) 6018* [Person](person.html): The Person links to this Patient 6019* [Procedure](procedure.html): Search by subject - a patient 6020* [Provenance](provenance.html): Where the activity involved patient data 6021* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6022* [RelatedPerson](relatedperson.html): The patient this related person is related to 6023* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6024* [ResearchSubject](researchsubject.html): Who or what is part of study 6025* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6026* [ServiceRequest](servicerequest.html): Search by subject - a patient 6027* [Specimen](specimen.html): The patient the specimen comes from 6028* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6029* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6030* [Task](task.html): Search by patient 6031* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6032</b><br> 6033 * Type: <b>reference</b><br> 6034 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6035 * </p> 6036 */ 6037 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 6038 public static final String SP_PATIENT = "patient"; 6039 /** 6040 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6041 * <p> 6042 * Description: <b>Multiple Resources: 6043 6044* [Account](account.html): The entity that caused the expenses 6045* [AdverseEvent](adverseevent.html): Subject impacted by event 6046* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 6047* [Appointment](appointment.html): One of the individuals of the appointment is this patient 6048* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 6049* [AuditEvent](auditevent.html): Where the activity involved patient data 6050* [Basic](basic.html): Identifies the focus of this resource 6051* [BodyStructure](bodystructure.html): Who this is about 6052* [CarePlan](careplan.html): Who the care plan is for 6053* [CareTeam](careteam.html): Who care team is for 6054* [ChargeItem](chargeitem.html): Individual service was done for/to 6055* [Claim](claim.html): Patient receiving the products or services 6056* [ClaimResponse](claimresponse.html): The subject of care 6057* [ClinicalImpression](clinicalimpression.html): Patient assessed 6058* [Communication](communication.html): Focus of message 6059* [CommunicationRequest](communicationrequest.html): Focus of message 6060* [Composition](composition.html): Who and/or what the composition is about 6061* [Condition](condition.html): Who has the condition? 6062* [Consent](consent.html): Who the consent applies to 6063* [Contract](contract.html): The identity of the subject of the contract (if a patient) 6064* [Coverage](coverage.html): Retrieve coverages for a patient 6065* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 6066* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 6067* [DetectedIssue](detectedissue.html): Associated patient 6068* [DeviceRequest](devicerequest.html): Individual the service is ordered for 6069* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 6070* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 6071* [DocumentReference](documentreference.html): Who/what is the subject of the document 6072* [Encounter](encounter.html): The patient present at the encounter 6073* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 6074* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 6075* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 6076* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 6077* [Flag](flag.html): The identity of a subject to list flags for 6078* [Goal](goal.html): Who this goal is intended for 6079* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6080* [ImagingSelection](imagingselection.html): Who the study is about 6081* [ImagingStudy](imagingstudy.html): Who the study is about 6082* [Immunization](immunization.html): The patient for the vaccination record 6083* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6084* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6085* [Invoice](invoice.html): Recipient(s) of goods and services 6086* [List](list.html): If all resources have the same subject 6087* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6088* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6089* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6090* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6091* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6092* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6093* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6094* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6095* [Observation](observation.html): The subject that the observation is about (if patient) 6096* [Person](person.html): The Person links to this Patient 6097* [Procedure](procedure.html): Search by subject - a patient 6098* [Provenance](provenance.html): Where the activity involved patient data 6099* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6100* [RelatedPerson](relatedperson.html): The patient this related person is related to 6101* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6102* [ResearchSubject](researchsubject.html): Who or what is part of study 6103* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6104* [ServiceRequest](servicerequest.html): Search by subject - a patient 6105* [Specimen](specimen.html): The patient the specimen comes from 6106* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6107* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6108* [Task](task.html): Search by patient 6109* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6110</b><br> 6111 * Type: <b>reference</b><br> 6112 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6113 * </p> 6114 */ 6115 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 6116 6117/** 6118 * Constant for fluent queries to be used to add include statements. Specifies 6119 * the path value of "<b>Appointment:patient</b>". 6120 */ 6121 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Appointment:patient").toLocked(); 6122 6123 6124} 6125