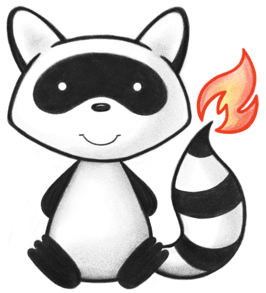
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 052 */ 053@ResourceDef(name="AppointmentResponse", profile="http://hl7.org/fhir/StructureDefinition/AppointmentResponse") 054public class AppointmentResponse extends DomainResource { 055 056 public enum AppointmentResponseStatus { 057 /** 058 * The participant has accepted the appointment. 059 */ 060 ACCEPTED, 061 /** 062 * The participant has declined the appointment and will not participate in the appointment. 063 */ 064 DECLINED, 065 /** 066 * The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur. 067 */ 068 TENTATIVE, 069 /** 070 * The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses. 071 */ 072 NEEDSACTION, 073 /** 074 * This instance should not have been part of this patient's medical record. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static AppointmentResponseStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("accepted".equals(codeString)) 085 return ACCEPTED; 086 if ("declined".equals(codeString)) 087 return DECLINED; 088 if ("tentative".equals(codeString)) 089 return TENTATIVE; 090 if ("needs-action".equals(codeString)) 091 return NEEDSACTION; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown AppointmentResponseStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case ACCEPTED: return "accepted"; 102 case DECLINED: return "declined"; 103 case TENTATIVE: return "tentative"; 104 case NEEDSACTION: return "needs-action"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case ACCEPTED: return "http://hl7.org/fhir/participationstatus"; 113 case DECLINED: return "http://hl7.org/fhir/participationstatus"; 114 case TENTATIVE: return "http://hl7.org/fhir/participationstatus"; 115 case NEEDSACTION: return "http://hl7.org/fhir/participationstatus"; 116 case ENTEREDINERROR: return "http://hl7.org/fhir/appointmentstatus"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case ACCEPTED: return "The participant has accepted the appointment."; 124 case DECLINED: return "The participant has declined the appointment and will not participate in the appointment."; 125 case TENTATIVE: return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 126 case NEEDSACTION: return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 127 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case ACCEPTED: return "Accepted"; 135 case DECLINED: return "Declined"; 136 case TENTATIVE: return "Tentative"; 137 case NEEDSACTION: return "Needs Action"; 138 case ENTEREDINERROR: return "Entered in error"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class AppointmentResponseStatusEnumFactory implements EnumFactory<AppointmentResponseStatus> { 146 public AppointmentResponseStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("accepted".equals(codeString)) 151 return AppointmentResponseStatus.ACCEPTED; 152 if ("declined".equals(codeString)) 153 return AppointmentResponseStatus.DECLINED; 154 if ("tentative".equals(codeString)) 155 return AppointmentResponseStatus.TENTATIVE; 156 if ("needs-action".equals(codeString)) 157 return AppointmentResponseStatus.NEEDSACTION; 158 if ("entered-in-error".equals(codeString)) 159 return AppointmentResponseStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown AppointmentResponseStatus code '"+codeString+"'"); 161 } 162 public Enumeration<AppointmentResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.NULL, code); 170 if ("accepted".equals(codeString)) 171 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.ACCEPTED, code); 172 if ("declined".equals(codeString)) 173 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.DECLINED, code); 174 if ("tentative".equals(codeString)) 175 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.TENTATIVE, code); 176 if ("needs-action".equals(codeString)) 177 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.NEEDSACTION, code); 178 if ("entered-in-error".equals(codeString)) 179 return new Enumeration<AppointmentResponseStatus>(this, AppointmentResponseStatus.ENTEREDINERROR, code); 180 throw new FHIRException("Unknown AppointmentResponseStatus code '"+codeString+"'"); 181 } 182 public String toCode(AppointmentResponseStatus code) { 183 if (code == AppointmentResponseStatus.NULL) 184 return null; 185 if (code == AppointmentResponseStatus.ACCEPTED) 186 return "accepted"; 187 if (code == AppointmentResponseStatus.DECLINED) 188 return "declined"; 189 if (code == AppointmentResponseStatus.TENTATIVE) 190 return "tentative"; 191 if (code == AppointmentResponseStatus.NEEDSACTION) 192 return "needs-action"; 193 if (code == AppointmentResponseStatus.ENTEREDINERROR) 194 return "entered-in-error"; 195 return "?"; 196 } 197 public String toSystem(AppointmentResponseStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 /** 203 * This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate. 204 */ 205 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 206 @Description(shortDefinition="External Ids for this item", formalDefinition="This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate." ) 207 protected List<Identifier> identifier; 208 209 /** 210 * Appointment that this response is replying to. 211 */ 212 @Child(name = "appointment", type = {Appointment.class}, order=1, min=1, max=1, modifier=false, summary=true) 213 @Description(shortDefinition="Appointment this response relates to", formalDefinition="Appointment that this response is replying to." ) 214 protected Reference appointment; 215 216 /** 217 * Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties. 218 */ 219 @Child(name = "proposedNewTime", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 220 @Description(shortDefinition="Indicator for a counter proposal", formalDefinition="Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties." ) 221 protected BooleanType proposedNewTime; 222 223 /** 224 * Date/Time that the appointment is to take place, or requested new start time. 225 */ 226 @Child(name = "start", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=false) 227 @Description(shortDefinition="Time from appointment, or requested new start time", formalDefinition="Date/Time that the appointment is to take place, or requested new start time." ) 228 protected InstantType start; 229 230 /** 231 * This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time. 232 */ 233 @Child(name = "end", type = {InstantType.class}, order=4, min=0, max=1, modifier=false, summary=false) 234 @Description(shortDefinition="Time from appointment, or requested new end time", formalDefinition="This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time." ) 235 protected InstantType end; 236 237 /** 238 * Role of participant in the appointment. 239 */ 240 @Child(name = "participantType", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 241 @Description(shortDefinition="Role of participant in the appointment", formalDefinition="Role of participant in the appointment." ) 242 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-participant-type") 243 protected List<CodeableConcept> participantType; 244 245 /** 246 * A Person, Location, HealthcareService, or Device that is participating in the appointment. 247 */ 248 @Child(name = "actor", type = {Patient.class, Group.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Device.class, HealthcareService.class, Location.class}, order=6, min=0, max=1, modifier=false, summary=true) 249 @Description(shortDefinition="Person(s), Location, HealthcareService, or Device", formalDefinition="A Person, Location, HealthcareService, or Device that is participating in the appointment." ) 250 protected Reference actor; 251 252 /** 253 * Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty. 254 */ 255 @Child(name = "participantStatus", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 256 @Description(shortDefinition="accepted | declined | tentative | needs-action | entered-in-error", formalDefinition="Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty." ) 257 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/appointmentresponse-status") 258 protected Enumeration<AppointmentResponseStatus> participantStatus; 259 260 /** 261 * Additional comments about the appointment. 262 */ 263 @Child(name = "comment", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 264 @Description(shortDefinition="Additional comments", formalDefinition="Additional comments about the appointment." ) 265 protected MarkdownType comment; 266 267 /** 268 * Indicates that this AppointmentResponse applies to all occurrences in a recurring request. 269 */ 270 @Child(name = "recurring", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 271 @Description(shortDefinition="This response is for all occurrences in a recurring request", formalDefinition="Indicates that this AppointmentResponse applies to all occurrences in a recurring request." ) 272 protected BooleanType recurring; 273 274 /** 275 * The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`). 276 */ 277 @Child(name = "occurrenceDate", type = {DateType.class}, order=10, min=0, max=1, modifier=false, summary=false) 278 @Description(shortDefinition="Original date within a recurring request", formalDefinition="The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`)." ) 279 protected DateType occurrenceDate; 280 281 /** 282 * The recurrence ID (sequence number) of the specific appointment when responding to a recurring request. 283 */ 284 @Child(name = "recurrenceId", type = {PositiveIntType.class}, order=11, min=0, max=1, modifier=false, summary=false) 285 @Description(shortDefinition="The recurrence ID of the specific recurring request", formalDefinition="The recurrence ID (sequence number) of the specific appointment when responding to a recurring request." ) 286 protected PositiveIntType recurrenceId; 287 288 private static final long serialVersionUID = -780212279L; 289 290 /** 291 * Constructor 292 */ 293 public AppointmentResponse() { 294 super(); 295 } 296 297 /** 298 * Constructor 299 */ 300 public AppointmentResponse(Reference appointment, AppointmentResponseStatus participantStatus) { 301 super(); 302 this.setAppointment(appointment); 303 this.setParticipantStatus(participantStatus); 304 } 305 306 /** 307 * @return {@link #identifier} (This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.) 308 */ 309 public List<Identifier> getIdentifier() { 310 if (this.identifier == null) 311 this.identifier = new ArrayList<Identifier>(); 312 return this.identifier; 313 } 314 315 /** 316 * @return Returns a reference to <code>this</code> for easy method chaining 317 */ 318 public AppointmentResponse setIdentifier(List<Identifier> theIdentifier) { 319 this.identifier = theIdentifier; 320 return this; 321 } 322 323 public boolean hasIdentifier() { 324 if (this.identifier == null) 325 return false; 326 for (Identifier item : this.identifier) 327 if (!item.isEmpty()) 328 return true; 329 return false; 330 } 331 332 public Identifier addIdentifier() { //3 333 Identifier t = new Identifier(); 334 if (this.identifier == null) 335 this.identifier = new ArrayList<Identifier>(); 336 this.identifier.add(t); 337 return t; 338 } 339 340 public AppointmentResponse addIdentifier(Identifier t) { //3 341 if (t == null) 342 return this; 343 if (this.identifier == null) 344 this.identifier = new ArrayList<Identifier>(); 345 this.identifier.add(t); 346 return this; 347 } 348 349 /** 350 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 351 */ 352 public Identifier getIdentifierFirstRep() { 353 if (getIdentifier().isEmpty()) { 354 addIdentifier(); 355 } 356 return getIdentifier().get(0); 357 } 358 359 /** 360 * @return {@link #appointment} (Appointment that this response is replying to.) 361 */ 362 public Reference getAppointment() { 363 if (this.appointment == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 366 else if (Configuration.doAutoCreate()) 367 this.appointment = new Reference(); // cc 368 return this.appointment; 369 } 370 371 public boolean hasAppointment() { 372 return this.appointment != null && !this.appointment.isEmpty(); 373 } 374 375 /** 376 * @param value {@link #appointment} (Appointment that this response is replying to.) 377 */ 378 public AppointmentResponse setAppointment(Reference value) { 379 this.appointment = value; 380 return this; 381 } 382 383 /** 384 * @return {@link #proposedNewTime} (Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties.). This is the underlying object with id, value and extensions. The accessor "getProposedNewTime" gives direct access to the value 385 */ 386 public BooleanType getProposedNewTimeElement() { 387 if (this.proposedNewTime == null) 388 if (Configuration.errorOnAutoCreate()) 389 throw new Error("Attempt to auto-create AppointmentResponse.proposedNewTime"); 390 else if (Configuration.doAutoCreate()) 391 this.proposedNewTime = new BooleanType(); // bb 392 return this.proposedNewTime; 393 } 394 395 public boolean hasProposedNewTimeElement() { 396 return this.proposedNewTime != null && !this.proposedNewTime.isEmpty(); 397 } 398 399 public boolean hasProposedNewTime() { 400 return this.proposedNewTime != null && !this.proposedNewTime.isEmpty(); 401 } 402 403 /** 404 * @param value {@link #proposedNewTime} (Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties.). This is the underlying object with id, value and extensions. The accessor "getProposedNewTime" gives direct access to the value 405 */ 406 public AppointmentResponse setProposedNewTimeElement(BooleanType value) { 407 this.proposedNewTime = value; 408 return this; 409 } 410 411 /** 412 * @return Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties. 413 */ 414 public boolean getProposedNewTime() { 415 return this.proposedNewTime == null || this.proposedNewTime.isEmpty() ? false : this.proposedNewTime.getValue(); 416 } 417 418 /** 419 * @param value Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties. 420 */ 421 public AppointmentResponse setProposedNewTime(boolean value) { 422 if (this.proposedNewTime == null) 423 this.proposedNewTime = new BooleanType(); 424 this.proposedNewTime.setValue(value); 425 return this; 426 } 427 428 /** 429 * @return {@link #start} (Date/Time that the appointment is to take place, or requested new start time.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 430 */ 431 public InstantType getStartElement() { 432 if (this.start == null) 433 if (Configuration.errorOnAutoCreate()) 434 throw new Error("Attempt to auto-create AppointmentResponse.start"); 435 else if (Configuration.doAutoCreate()) 436 this.start = new InstantType(); // bb 437 return this.start; 438 } 439 440 public boolean hasStartElement() { 441 return this.start != null && !this.start.isEmpty(); 442 } 443 444 public boolean hasStart() { 445 return this.start != null && !this.start.isEmpty(); 446 } 447 448 /** 449 * @param value {@link #start} (Date/Time that the appointment is to take place, or requested new start time.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 450 */ 451 public AppointmentResponse setStartElement(InstantType value) { 452 this.start = value; 453 return this; 454 } 455 456 /** 457 * @return Date/Time that the appointment is to take place, or requested new start time. 458 */ 459 public Date getStart() { 460 return this.start == null ? null : this.start.getValue(); 461 } 462 463 /** 464 * @param value Date/Time that the appointment is to take place, or requested new start time. 465 */ 466 public AppointmentResponse setStart(Date value) { 467 if (value == null) 468 this.start = null; 469 else { 470 if (this.start == null) 471 this.start = new InstantType(); 472 this.start.setValue(value); 473 } 474 return this; 475 } 476 477 /** 478 * @return {@link #end} (This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 479 */ 480 public InstantType getEndElement() { 481 if (this.end == null) 482 if (Configuration.errorOnAutoCreate()) 483 throw new Error("Attempt to auto-create AppointmentResponse.end"); 484 else if (Configuration.doAutoCreate()) 485 this.end = new InstantType(); // bb 486 return this.end; 487 } 488 489 public boolean hasEndElement() { 490 return this.end != null && !this.end.isEmpty(); 491 } 492 493 public boolean hasEnd() { 494 return this.end != null && !this.end.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #end} (This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 499 */ 500 public AppointmentResponse setEndElement(InstantType value) { 501 this.end = value; 502 return this; 503 } 504 505 /** 506 * @return This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time. 507 */ 508 public Date getEnd() { 509 return this.end == null ? null : this.end.getValue(); 510 } 511 512 /** 513 * @param value This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time. 514 */ 515 public AppointmentResponse setEnd(Date value) { 516 if (value == null) 517 this.end = null; 518 else { 519 if (this.end == null) 520 this.end = new InstantType(); 521 this.end.setValue(value); 522 } 523 return this; 524 } 525 526 /** 527 * @return {@link #participantType} (Role of participant in the appointment.) 528 */ 529 public List<CodeableConcept> getParticipantType() { 530 if (this.participantType == null) 531 this.participantType = new ArrayList<CodeableConcept>(); 532 return this.participantType; 533 } 534 535 /** 536 * @return Returns a reference to <code>this</code> for easy method chaining 537 */ 538 public AppointmentResponse setParticipantType(List<CodeableConcept> theParticipantType) { 539 this.participantType = theParticipantType; 540 return this; 541 } 542 543 public boolean hasParticipantType() { 544 if (this.participantType == null) 545 return false; 546 for (CodeableConcept item : this.participantType) 547 if (!item.isEmpty()) 548 return true; 549 return false; 550 } 551 552 public CodeableConcept addParticipantType() { //3 553 CodeableConcept t = new CodeableConcept(); 554 if (this.participantType == null) 555 this.participantType = new ArrayList<CodeableConcept>(); 556 this.participantType.add(t); 557 return t; 558 } 559 560 public AppointmentResponse addParticipantType(CodeableConcept t) { //3 561 if (t == null) 562 return this; 563 if (this.participantType == null) 564 this.participantType = new ArrayList<CodeableConcept>(); 565 this.participantType.add(t); 566 return this; 567 } 568 569 /** 570 * @return The first repetition of repeating field {@link #participantType}, creating it if it does not already exist {3} 571 */ 572 public CodeableConcept getParticipantTypeFirstRep() { 573 if (getParticipantType().isEmpty()) { 574 addParticipantType(); 575 } 576 return getParticipantType().get(0); 577 } 578 579 /** 580 * @return {@link #actor} (A Person, Location, HealthcareService, or Device that is participating in the appointment.) 581 */ 582 public Reference getActor() { 583 if (this.actor == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create AppointmentResponse.actor"); 586 else if (Configuration.doAutoCreate()) 587 this.actor = new Reference(); // cc 588 return this.actor; 589 } 590 591 public boolean hasActor() { 592 return this.actor != null && !this.actor.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #actor} (A Person, Location, HealthcareService, or Device that is participating in the appointment.) 597 */ 598 public AppointmentResponse setActor(Reference value) { 599 this.actor = value; 600 return this; 601 } 602 603 /** 604 * @return {@link #participantStatus} (Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.). This is the underlying object with id, value and extensions. The accessor "getParticipantStatus" gives direct access to the value 605 */ 606 public Enumeration<AppointmentResponseStatus> getParticipantStatusElement() { 607 if (this.participantStatus == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create AppointmentResponse.participantStatus"); 610 else if (Configuration.doAutoCreate()) 611 this.participantStatus = new Enumeration<AppointmentResponseStatus>(new AppointmentResponseStatusEnumFactory()); // bb 612 return this.participantStatus; 613 } 614 615 public boolean hasParticipantStatusElement() { 616 return this.participantStatus != null && !this.participantStatus.isEmpty(); 617 } 618 619 public boolean hasParticipantStatus() { 620 return this.participantStatus != null && !this.participantStatus.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #participantStatus} (Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.). This is the underlying object with id, value and extensions. The accessor "getParticipantStatus" gives direct access to the value 625 */ 626 public AppointmentResponse setParticipantStatusElement(Enumeration<AppointmentResponseStatus> value) { 627 this.participantStatus = value; 628 return this; 629 } 630 631 /** 632 * @return Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty. 633 */ 634 public AppointmentResponseStatus getParticipantStatus() { 635 return this.participantStatus == null ? null : this.participantStatus.getValue(); 636 } 637 638 /** 639 * @param value Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty. 640 */ 641 public AppointmentResponse setParticipantStatus(AppointmentResponseStatus value) { 642 if (this.participantStatus == null) 643 this.participantStatus = new Enumeration<AppointmentResponseStatus>(new AppointmentResponseStatusEnumFactory()); 644 this.participantStatus.setValue(value); 645 return this; 646 } 647 648 /** 649 * @return {@link #comment} (Additional comments about the appointment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 650 */ 651 public MarkdownType getCommentElement() { 652 if (this.comment == null) 653 if (Configuration.errorOnAutoCreate()) 654 throw new Error("Attempt to auto-create AppointmentResponse.comment"); 655 else if (Configuration.doAutoCreate()) 656 this.comment = new MarkdownType(); // bb 657 return this.comment; 658 } 659 660 public boolean hasCommentElement() { 661 return this.comment != null && !this.comment.isEmpty(); 662 } 663 664 public boolean hasComment() { 665 return this.comment != null && !this.comment.isEmpty(); 666 } 667 668 /** 669 * @param value {@link #comment} (Additional comments about the appointment.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 670 */ 671 public AppointmentResponse setCommentElement(MarkdownType value) { 672 this.comment = value; 673 return this; 674 } 675 676 /** 677 * @return Additional comments about the appointment. 678 */ 679 public String getComment() { 680 return this.comment == null ? null : this.comment.getValue(); 681 } 682 683 /** 684 * @param value Additional comments about the appointment. 685 */ 686 public AppointmentResponse setComment(String value) { 687 if (Utilities.noString(value)) 688 this.comment = null; 689 else { 690 if (this.comment == null) 691 this.comment = new MarkdownType(); 692 this.comment.setValue(value); 693 } 694 return this; 695 } 696 697 /** 698 * @return {@link #recurring} (Indicates that this AppointmentResponse applies to all occurrences in a recurring request.). This is the underlying object with id, value and extensions. The accessor "getRecurring" gives direct access to the value 699 */ 700 public BooleanType getRecurringElement() { 701 if (this.recurring == null) 702 if (Configuration.errorOnAutoCreate()) 703 throw new Error("Attempt to auto-create AppointmentResponse.recurring"); 704 else if (Configuration.doAutoCreate()) 705 this.recurring = new BooleanType(); // bb 706 return this.recurring; 707 } 708 709 public boolean hasRecurringElement() { 710 return this.recurring != null && !this.recurring.isEmpty(); 711 } 712 713 public boolean hasRecurring() { 714 return this.recurring != null && !this.recurring.isEmpty(); 715 } 716 717 /** 718 * @param value {@link #recurring} (Indicates that this AppointmentResponse applies to all occurrences in a recurring request.). This is the underlying object with id, value and extensions. The accessor "getRecurring" gives direct access to the value 719 */ 720 public AppointmentResponse setRecurringElement(BooleanType value) { 721 this.recurring = value; 722 return this; 723 } 724 725 /** 726 * @return Indicates that this AppointmentResponse applies to all occurrences in a recurring request. 727 */ 728 public boolean getRecurring() { 729 return this.recurring == null || this.recurring.isEmpty() ? false : this.recurring.getValue(); 730 } 731 732 /** 733 * @param value Indicates that this AppointmentResponse applies to all occurrences in a recurring request. 734 */ 735 public AppointmentResponse setRecurring(boolean value) { 736 if (this.recurring == null) 737 this.recurring = new BooleanType(); 738 this.recurring.setValue(value); 739 return this; 740 } 741 742 /** 743 * @return {@link #occurrenceDate} (The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`).). This is the underlying object with id, value and extensions. The accessor "getOccurrenceDate" gives direct access to the value 744 */ 745 public DateType getOccurrenceDateElement() { 746 if (this.occurrenceDate == null) 747 if (Configuration.errorOnAutoCreate()) 748 throw new Error("Attempt to auto-create AppointmentResponse.occurrenceDate"); 749 else if (Configuration.doAutoCreate()) 750 this.occurrenceDate = new DateType(); // bb 751 return this.occurrenceDate; 752 } 753 754 public boolean hasOccurrenceDateElement() { 755 return this.occurrenceDate != null && !this.occurrenceDate.isEmpty(); 756 } 757 758 public boolean hasOccurrenceDate() { 759 return this.occurrenceDate != null && !this.occurrenceDate.isEmpty(); 760 } 761 762 /** 763 * @param value {@link #occurrenceDate} (The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`).). This is the underlying object with id, value and extensions. The accessor "getOccurrenceDate" gives direct access to the value 764 */ 765 public AppointmentResponse setOccurrenceDateElement(DateType value) { 766 this.occurrenceDate = value; 767 return this; 768 } 769 770 /** 771 * @return The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`). 772 */ 773 public Date getOccurrenceDate() { 774 return this.occurrenceDate == null ? null : this.occurrenceDate.getValue(); 775 } 776 777 /** 778 * @param value The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`). 779 */ 780 public AppointmentResponse setOccurrenceDate(Date value) { 781 if (value == null) 782 this.occurrenceDate = null; 783 else { 784 if (this.occurrenceDate == null) 785 this.occurrenceDate = new DateType(); 786 this.occurrenceDate.setValue(value); 787 } 788 return this; 789 } 790 791 /** 792 * @return {@link #recurrenceId} (The recurrence ID (sequence number) of the specific appointment when responding to a recurring request.). This is the underlying object with id, value and extensions. The accessor "getRecurrenceId" gives direct access to the value 793 */ 794 public PositiveIntType getRecurrenceIdElement() { 795 if (this.recurrenceId == null) 796 if (Configuration.errorOnAutoCreate()) 797 throw new Error("Attempt to auto-create AppointmentResponse.recurrenceId"); 798 else if (Configuration.doAutoCreate()) 799 this.recurrenceId = new PositiveIntType(); // bb 800 return this.recurrenceId; 801 } 802 803 public boolean hasRecurrenceIdElement() { 804 return this.recurrenceId != null && !this.recurrenceId.isEmpty(); 805 } 806 807 public boolean hasRecurrenceId() { 808 return this.recurrenceId != null && !this.recurrenceId.isEmpty(); 809 } 810 811 /** 812 * @param value {@link #recurrenceId} (The recurrence ID (sequence number) of the specific appointment when responding to a recurring request.). This is the underlying object with id, value and extensions. The accessor "getRecurrenceId" gives direct access to the value 813 */ 814 public AppointmentResponse setRecurrenceIdElement(PositiveIntType value) { 815 this.recurrenceId = value; 816 return this; 817 } 818 819 /** 820 * @return The recurrence ID (sequence number) of the specific appointment when responding to a recurring request. 821 */ 822 public int getRecurrenceId() { 823 return this.recurrenceId == null || this.recurrenceId.isEmpty() ? 0 : this.recurrenceId.getValue(); 824 } 825 826 /** 827 * @param value The recurrence ID (sequence number) of the specific appointment when responding to a recurring request. 828 */ 829 public AppointmentResponse setRecurrenceId(int value) { 830 if (this.recurrenceId == null) 831 this.recurrenceId = new PositiveIntType(); 832 this.recurrenceId.setValue(value); 833 return this; 834 } 835 836 protected void listChildren(List<Property> children) { 837 super.listChildren(children); 838 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier)); 839 children.add(new Property("appointment", "Reference(Appointment)", "Appointment that this response is replying to.", 0, 1, appointment)); 840 children.add(new Property("proposedNewTime", "boolean", "Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties.", 0, 1, proposedNewTime)); 841 children.add(new Property("start", "instant", "Date/Time that the appointment is to take place, or requested new start time.", 0, 1, start)); 842 children.add(new Property("end", "instant", "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 0, 1, end)); 843 children.add(new Property("participantType", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, participantType)); 844 children.add(new Property("actor", "Reference(Patient|Group|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", "A Person, Location, HealthcareService, or Device that is participating in the appointment.", 0, 1, actor)); 845 children.add(new Property("participantStatus", "code", "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 0, 1, participantStatus)); 846 children.add(new Property("comment", "markdown", "Additional comments about the appointment.", 0, 1, comment)); 847 children.add(new Property("recurring", "boolean", "Indicates that this AppointmentResponse applies to all occurrences in a recurring request.", 0, 1, recurring)); 848 children.add(new Property("occurrenceDate", "date", "The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`).", 0, 1, occurrenceDate)); 849 children.add(new Property("recurrenceId", "positiveInt", "The recurrence ID (sequence number) of the specific appointment when responding to a recurring request.", 0, 1, recurrenceId)); 850 } 851 852 @Override 853 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 854 switch (_hash) { 855 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, java.lang.Integer.MAX_VALUE, identifier); 856 case -1474995297: /*appointment*/ return new Property("appointment", "Reference(Appointment)", "Appointment that this response is replying to.", 0, 1, appointment); 857 case -577024441: /*proposedNewTime*/ return new Property("proposedNewTime", "boolean", "Indicates that the response is proposing a different time that was initially requested. The new proposed time will be indicated in the start and end properties.", 0, 1, proposedNewTime); 858 case 109757538: /*start*/ return new Property("start", "instant", "Date/Time that the appointment is to take place, or requested new start time.", 0, 1, start); 859 case 100571: /*end*/ return new Property("end", "instant", "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 0, 1, end); 860 case 841294093: /*participantType*/ return new Property("participantType", "CodeableConcept", "Role of participant in the appointment.", 0, java.lang.Integer.MAX_VALUE, participantType); 861 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Group|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", "A Person, Location, HealthcareService, or Device that is participating in the appointment.", 0, 1, actor); 862 case 996096261: /*participantStatus*/ return new Property("participantStatus", "code", "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 0, 1, participantStatus); 863 case 950398559: /*comment*/ return new Property("comment", "markdown", "Additional comments about the appointment.", 0, 1, comment); 864 case 1165749981: /*recurring*/ return new Property("recurring", "boolean", "Indicates that this AppointmentResponse applies to all occurrences in a recurring request.", 0, 1, recurring); 865 case 1721761055: /*occurrenceDate*/ return new Property("occurrenceDate", "date", "The original date within a recurring request. This could be used in place of the recurrenceId to be more direct (or where the template is provided through the simple list of dates in `Appointment.occurrenceDate`).", 0, 1, occurrenceDate); 866 case -362407829: /*recurrenceId*/ return new Property("recurrenceId", "positiveInt", "The recurrence ID (sequence number) of the specific appointment when responding to a recurring request.", 0, 1, recurrenceId); 867 default: return super.getNamedProperty(_hash, _name, _checkValid); 868 } 869 870 } 871 872 @Override 873 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 874 switch (hash) { 875 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 876 case -1474995297: /*appointment*/ return this.appointment == null ? new Base[0] : new Base[] {this.appointment}; // Reference 877 case -577024441: /*proposedNewTime*/ return this.proposedNewTime == null ? new Base[0] : new Base[] {this.proposedNewTime}; // BooleanType 878 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // InstantType 879 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 880 case 841294093: /*participantType*/ return this.participantType == null ? new Base[0] : this.participantType.toArray(new Base[this.participantType.size()]); // CodeableConcept 881 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 882 case 996096261: /*participantStatus*/ return this.participantStatus == null ? new Base[0] : new Base[] {this.participantStatus}; // Enumeration<AppointmentResponseStatus> 883 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 884 case 1165749981: /*recurring*/ return this.recurring == null ? new Base[0] : new Base[] {this.recurring}; // BooleanType 885 case 1721761055: /*occurrenceDate*/ return this.occurrenceDate == null ? new Base[0] : new Base[] {this.occurrenceDate}; // DateType 886 case -362407829: /*recurrenceId*/ return this.recurrenceId == null ? new Base[0] : new Base[] {this.recurrenceId}; // PositiveIntType 887 default: return super.getProperty(hash, name, checkValid); 888 } 889 890 } 891 892 @Override 893 public Base setProperty(int hash, String name, Base value) throws FHIRException { 894 switch (hash) { 895 case -1618432855: // identifier 896 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 897 return value; 898 case -1474995297: // appointment 899 this.appointment = TypeConvertor.castToReference(value); // Reference 900 return value; 901 case -577024441: // proposedNewTime 902 this.proposedNewTime = TypeConvertor.castToBoolean(value); // BooleanType 903 return value; 904 case 109757538: // start 905 this.start = TypeConvertor.castToInstant(value); // InstantType 906 return value; 907 case 100571: // end 908 this.end = TypeConvertor.castToInstant(value); // InstantType 909 return value; 910 case 841294093: // participantType 911 this.getParticipantType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 912 return value; 913 case 92645877: // actor 914 this.actor = TypeConvertor.castToReference(value); // Reference 915 return value; 916 case 996096261: // participantStatus 917 value = new AppointmentResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 918 this.participantStatus = (Enumeration) value; // Enumeration<AppointmentResponseStatus> 919 return value; 920 case 950398559: // comment 921 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 922 return value; 923 case 1165749981: // recurring 924 this.recurring = TypeConvertor.castToBoolean(value); // BooleanType 925 return value; 926 case 1721761055: // occurrenceDate 927 this.occurrenceDate = TypeConvertor.castToDate(value); // DateType 928 return value; 929 case -362407829: // recurrenceId 930 this.recurrenceId = TypeConvertor.castToPositiveInt(value); // PositiveIntType 931 return value; 932 default: return super.setProperty(hash, name, value); 933 } 934 935 } 936 937 @Override 938 public Base setProperty(String name, Base value) throws FHIRException { 939 if (name.equals("identifier")) { 940 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 941 } else if (name.equals("appointment")) { 942 this.appointment = TypeConvertor.castToReference(value); // Reference 943 } else if (name.equals("proposedNewTime")) { 944 this.proposedNewTime = TypeConvertor.castToBoolean(value); // BooleanType 945 } else if (name.equals("start")) { 946 this.start = TypeConvertor.castToInstant(value); // InstantType 947 } else if (name.equals("end")) { 948 this.end = TypeConvertor.castToInstant(value); // InstantType 949 } else if (name.equals("participantType")) { 950 this.getParticipantType().add(TypeConvertor.castToCodeableConcept(value)); 951 } else if (name.equals("actor")) { 952 this.actor = TypeConvertor.castToReference(value); // Reference 953 } else if (name.equals("participantStatus")) { 954 value = new AppointmentResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 955 this.participantStatus = (Enumeration) value; // Enumeration<AppointmentResponseStatus> 956 } else if (name.equals("comment")) { 957 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 958 } else if (name.equals("recurring")) { 959 this.recurring = TypeConvertor.castToBoolean(value); // BooleanType 960 } else if (name.equals("occurrenceDate")) { 961 this.occurrenceDate = TypeConvertor.castToDate(value); // DateType 962 } else if (name.equals("recurrenceId")) { 963 this.recurrenceId = TypeConvertor.castToPositiveInt(value); // PositiveIntType 964 } else 965 return super.setProperty(name, value); 966 return value; 967 } 968 969 @Override 970 public void removeChild(String name, Base value) throws FHIRException { 971 if (name.equals("identifier")) { 972 this.getIdentifier().remove(value); 973 } else if (name.equals("appointment")) { 974 this.appointment = null; 975 } else if (name.equals("proposedNewTime")) { 976 this.proposedNewTime = null; 977 } else if (name.equals("start")) { 978 this.start = null; 979 } else if (name.equals("end")) { 980 this.end = null; 981 } else if (name.equals("participantType")) { 982 this.getParticipantType().remove(value); 983 } else if (name.equals("actor")) { 984 this.actor = null; 985 } else if (name.equals("participantStatus")) { 986 value = new AppointmentResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 987 this.participantStatus = (Enumeration) value; // Enumeration<AppointmentResponseStatus> 988 } else if (name.equals("comment")) { 989 this.comment = null; 990 } else if (name.equals("recurring")) { 991 this.recurring = null; 992 } else if (name.equals("occurrenceDate")) { 993 this.occurrenceDate = null; 994 } else if (name.equals("recurrenceId")) { 995 this.recurrenceId = null; 996 } else 997 super.removeChild(name, value); 998 999 } 1000 1001 @Override 1002 public Base makeProperty(int hash, String name) throws FHIRException { 1003 switch (hash) { 1004 case -1618432855: return addIdentifier(); 1005 case -1474995297: return getAppointment(); 1006 case -577024441: return getProposedNewTimeElement(); 1007 case 109757538: return getStartElement(); 1008 case 100571: return getEndElement(); 1009 case 841294093: return addParticipantType(); 1010 case 92645877: return getActor(); 1011 case 996096261: return getParticipantStatusElement(); 1012 case 950398559: return getCommentElement(); 1013 case 1165749981: return getRecurringElement(); 1014 case 1721761055: return getOccurrenceDateElement(); 1015 case -362407829: return getRecurrenceIdElement(); 1016 default: return super.makeProperty(hash, name); 1017 } 1018 1019 } 1020 1021 @Override 1022 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1023 switch (hash) { 1024 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1025 case -1474995297: /*appointment*/ return new String[] {"Reference"}; 1026 case -577024441: /*proposedNewTime*/ return new String[] {"boolean"}; 1027 case 109757538: /*start*/ return new String[] {"instant"}; 1028 case 100571: /*end*/ return new String[] {"instant"}; 1029 case 841294093: /*participantType*/ return new String[] {"CodeableConcept"}; 1030 case 92645877: /*actor*/ return new String[] {"Reference"}; 1031 case 996096261: /*participantStatus*/ return new String[] {"code"}; 1032 case 950398559: /*comment*/ return new String[] {"markdown"}; 1033 case 1165749981: /*recurring*/ return new String[] {"boolean"}; 1034 case 1721761055: /*occurrenceDate*/ return new String[] {"date"}; 1035 case -362407829: /*recurrenceId*/ return new String[] {"positiveInt"}; 1036 default: return super.getTypesForProperty(hash, name); 1037 } 1038 1039 } 1040 1041 @Override 1042 public Base addChild(String name) throws FHIRException { 1043 if (name.equals("identifier")) { 1044 return addIdentifier(); 1045 } 1046 else if (name.equals("appointment")) { 1047 this.appointment = new Reference(); 1048 return this.appointment; 1049 } 1050 else if (name.equals("proposedNewTime")) { 1051 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.proposedNewTime"); 1052 } 1053 else if (name.equals("start")) { 1054 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.start"); 1055 } 1056 else if (name.equals("end")) { 1057 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.end"); 1058 } 1059 else if (name.equals("participantType")) { 1060 return addParticipantType(); 1061 } 1062 else if (name.equals("actor")) { 1063 this.actor = new Reference(); 1064 return this.actor; 1065 } 1066 else if (name.equals("participantStatus")) { 1067 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.participantStatus"); 1068 } 1069 else if (name.equals("comment")) { 1070 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.comment"); 1071 } 1072 else if (name.equals("recurring")) { 1073 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.recurring"); 1074 } 1075 else if (name.equals("occurrenceDate")) { 1076 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.occurrenceDate"); 1077 } 1078 else if (name.equals("recurrenceId")) { 1079 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.recurrenceId"); 1080 } 1081 else 1082 return super.addChild(name); 1083 } 1084 1085 public String fhirType() { 1086 return "AppointmentResponse"; 1087 1088 } 1089 1090 public AppointmentResponse copy() { 1091 AppointmentResponse dst = new AppointmentResponse(); 1092 copyValues(dst); 1093 return dst; 1094 } 1095 1096 public void copyValues(AppointmentResponse dst) { 1097 super.copyValues(dst); 1098 if (identifier != null) { 1099 dst.identifier = new ArrayList<Identifier>(); 1100 for (Identifier i : identifier) 1101 dst.identifier.add(i.copy()); 1102 }; 1103 dst.appointment = appointment == null ? null : appointment.copy(); 1104 dst.proposedNewTime = proposedNewTime == null ? null : proposedNewTime.copy(); 1105 dst.start = start == null ? null : start.copy(); 1106 dst.end = end == null ? null : end.copy(); 1107 if (participantType != null) { 1108 dst.participantType = new ArrayList<CodeableConcept>(); 1109 for (CodeableConcept i : participantType) 1110 dst.participantType.add(i.copy()); 1111 }; 1112 dst.actor = actor == null ? null : actor.copy(); 1113 dst.participantStatus = participantStatus == null ? null : participantStatus.copy(); 1114 dst.comment = comment == null ? null : comment.copy(); 1115 dst.recurring = recurring == null ? null : recurring.copy(); 1116 dst.occurrenceDate = occurrenceDate == null ? null : occurrenceDate.copy(); 1117 dst.recurrenceId = recurrenceId == null ? null : recurrenceId.copy(); 1118 } 1119 1120 protected AppointmentResponse typedCopy() { 1121 return copy(); 1122 } 1123 1124 @Override 1125 public boolean equalsDeep(Base other_) { 1126 if (!super.equalsDeep(other_)) 1127 return false; 1128 if (!(other_ instanceof AppointmentResponse)) 1129 return false; 1130 AppointmentResponse o = (AppointmentResponse) other_; 1131 return compareDeep(identifier, o.identifier, true) && compareDeep(appointment, o.appointment, true) 1132 && compareDeep(proposedNewTime, o.proposedNewTime, true) && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 1133 && compareDeep(participantType, o.participantType, true) && compareDeep(actor, o.actor, true) && compareDeep(participantStatus, o.participantStatus, true) 1134 && compareDeep(comment, o.comment, true) && compareDeep(recurring, o.recurring, true) && compareDeep(occurrenceDate, o.occurrenceDate, true) 1135 && compareDeep(recurrenceId, o.recurrenceId, true); 1136 } 1137 1138 @Override 1139 public boolean equalsShallow(Base other_) { 1140 if (!super.equalsShallow(other_)) 1141 return false; 1142 if (!(other_ instanceof AppointmentResponse)) 1143 return false; 1144 AppointmentResponse o = (AppointmentResponse) other_; 1145 return compareValues(proposedNewTime, o.proposedNewTime, true) && compareValues(start, o.start, true) 1146 && compareValues(end, o.end, true) && compareValues(participantStatus, o.participantStatus, true) && compareValues(comment, o.comment, true) 1147 && compareValues(recurring, o.recurring, true) && compareValues(occurrenceDate, o.occurrenceDate, true) 1148 && compareValues(recurrenceId, o.recurrenceId, true); 1149 } 1150 1151 public boolean isEmpty() { 1152 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, appointment, proposedNewTime 1153 , start, end, participantType, actor, participantStatus, comment, recurring, occurrenceDate 1154 , recurrenceId); 1155 } 1156 1157 @Override 1158 public ResourceType getResourceType() { 1159 return ResourceType.AppointmentResponse; 1160 } 1161 1162 /** 1163 * Search parameter: <b>actor</b> 1164 * <p> 1165 * Description: <b>The Person, Location/HealthcareService or Device that this appointment response replies for</b><br> 1166 * Type: <b>reference</b><br> 1167 * Path: <b>AppointmentResponse.actor</b><br> 1168 * </p> 1169 */ 1170 @SearchParamDefinition(name="actor", path="AppointmentResponse.actor", description="The Person, Location/HealthcareService or Device that this appointment response replies for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Group.class, HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1171 public static final String SP_ACTOR = "actor"; 1172 /** 1173 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 1174 * <p> 1175 * Description: <b>The Person, Location/HealthcareService or Device that this appointment response replies for</b><br> 1176 * Type: <b>reference</b><br> 1177 * Path: <b>AppointmentResponse.actor</b><br> 1178 * </p> 1179 */ 1180 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 1181 1182/** 1183 * Constant for fluent queries to be used to add include statements. Specifies 1184 * the path value of "<b>AppointmentResponse:actor</b>". 1185 */ 1186 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("AppointmentResponse:actor").toLocked(); 1187 1188 /** 1189 * Search parameter: <b>appointment</b> 1190 * <p> 1191 * Description: <b>The appointment that the response is attached to</b><br> 1192 * Type: <b>reference</b><br> 1193 * Path: <b>AppointmentResponse.appointment</b><br> 1194 * </p> 1195 */ 1196 @SearchParamDefinition(name="appointment", path="AppointmentResponse.appointment", description="The appointment that the response is attached to", type="reference", target={Appointment.class } ) 1197 public static final String SP_APPOINTMENT = "appointment"; 1198 /** 1199 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 1200 * <p> 1201 * Description: <b>The appointment that the response is attached to</b><br> 1202 * Type: <b>reference</b><br> 1203 * Path: <b>AppointmentResponse.appointment</b><br> 1204 * </p> 1205 */ 1206 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_APPOINTMENT); 1207 1208/** 1209 * Constant for fluent queries to be used to add include statements. Specifies 1210 * the path value of "<b>AppointmentResponse:appointment</b>". 1211 */ 1212 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include("AppointmentResponse:appointment").toLocked(); 1213 1214 /** 1215 * Search parameter: <b>group</b> 1216 * <p> 1217 * Description: <b>This Response is for this Group</b><br> 1218 * Type: <b>reference</b><br> 1219 * Path: <b>AppointmentResponse.actor.where(resolve() is Group)</b><br> 1220 * </p> 1221 */ 1222 @SearchParamDefinition(name="group", path="AppointmentResponse.actor.where(resolve() is Group)", description="This Response is for this Group", type="reference", target={Group.class } ) 1223 public static final String SP_GROUP = "group"; 1224 /** 1225 * <b>Fluent Client</b> search parameter constant for <b>group</b> 1226 * <p> 1227 * Description: <b>This Response is for this Group</b><br> 1228 * Type: <b>reference</b><br> 1229 * Path: <b>AppointmentResponse.actor.where(resolve() is Group)</b><br> 1230 * </p> 1231 */ 1232 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GROUP = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GROUP); 1233 1234/** 1235 * Constant for fluent queries to be used to add include statements. Specifies 1236 * the path value of "<b>AppointmentResponse:group</b>". 1237 */ 1238 public static final ca.uhn.fhir.model.api.Include INCLUDE_GROUP = new ca.uhn.fhir.model.api.Include("AppointmentResponse:group").toLocked(); 1239 1240 /** 1241 * Search parameter: <b>location</b> 1242 * <p> 1243 * Description: <b>This Response is for this Location</b><br> 1244 * Type: <b>reference</b><br> 1245 * Path: <b>AppointmentResponse.actor.where(resolve() is Location)</b><br> 1246 * </p> 1247 */ 1248 @SearchParamDefinition(name="location", path="AppointmentResponse.actor.where(resolve() is Location)", description="This Response is for this Location", type="reference", target={Location.class } ) 1249 public static final String SP_LOCATION = "location"; 1250 /** 1251 * <b>Fluent Client</b> search parameter constant for <b>location</b> 1252 * <p> 1253 * Description: <b>This Response is for this Location</b><br> 1254 * Type: <b>reference</b><br> 1255 * Path: <b>AppointmentResponse.actor.where(resolve() is Location)</b><br> 1256 * </p> 1257 */ 1258 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 1259 1260/** 1261 * Constant for fluent queries to be used to add include statements. Specifies 1262 * the path value of "<b>AppointmentResponse:location</b>". 1263 */ 1264 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("AppointmentResponse:location").toLocked(); 1265 1266 /** 1267 * Search parameter: <b>part-status</b> 1268 * <p> 1269 * Description: <b>The participants acceptance status for this appointment</b><br> 1270 * Type: <b>token</b><br> 1271 * Path: <b>AppointmentResponse.participantStatus</b><br> 1272 * </p> 1273 */ 1274 @SearchParamDefinition(name="part-status", path="AppointmentResponse.participantStatus", description="The participants acceptance status for this appointment", type="token" ) 1275 public static final String SP_PART_STATUS = "part-status"; 1276 /** 1277 * <b>Fluent Client</b> search parameter constant for <b>part-status</b> 1278 * <p> 1279 * Description: <b>The participants acceptance status for this appointment</b><br> 1280 * Type: <b>token</b><br> 1281 * Path: <b>AppointmentResponse.participantStatus</b><br> 1282 * </p> 1283 */ 1284 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PART_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PART_STATUS); 1285 1286 /** 1287 * Search parameter: <b>practitioner</b> 1288 * <p> 1289 * Description: <b>This Response is for this Practitioner</b><br> 1290 * Type: <b>reference</b><br> 1291 * Path: <b>AppointmentResponse.actor.where(resolve() is Practitioner)</b><br> 1292 * </p> 1293 */ 1294 @SearchParamDefinition(name="practitioner", path="AppointmentResponse.actor.where(resolve() is Practitioner)", description="This Response is for this Practitioner", type="reference", target={Practitioner.class } ) 1295 public static final String SP_PRACTITIONER = "practitioner"; 1296 /** 1297 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 1298 * <p> 1299 * Description: <b>This Response is for this Practitioner</b><br> 1300 * Type: <b>reference</b><br> 1301 * Path: <b>AppointmentResponse.actor.where(resolve() is Practitioner)</b><br> 1302 * </p> 1303 */ 1304 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 1305 1306/** 1307 * Constant for fluent queries to be used to add include statements. Specifies 1308 * the path value of "<b>AppointmentResponse:practitioner</b>". 1309 */ 1310 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("AppointmentResponse:practitioner").toLocked(); 1311 1312 /** 1313 * Search parameter: <b>identifier</b> 1314 * <p> 1315 * Description: <b>Multiple Resources: 1316 1317* [Account](account.html): Account number 1318* [AdverseEvent](adverseevent.html): Business identifier for the event 1319* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1320* [Appointment](appointment.html): An Identifier of the Appointment 1321* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1322* [Basic](basic.html): Business identifier 1323* [BodyStructure](bodystructure.html): Bodystructure identifier 1324* [CarePlan](careplan.html): External Ids for this plan 1325* [CareTeam](careteam.html): External Ids for this team 1326* [ChargeItem](chargeitem.html): Business Identifier for item 1327* [Claim](claim.html): The primary identifier of the financial resource 1328* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1329* [ClinicalImpression](clinicalimpression.html): Business identifier 1330* [Communication](communication.html): Unique identifier 1331* [CommunicationRequest](communicationrequest.html): Unique identifier 1332* [Composition](composition.html): Version-independent identifier for the Composition 1333* [Condition](condition.html): A unique identifier of the condition record 1334* [Consent](consent.html): Identifier for this record (external references) 1335* [Contract](contract.html): The identity of the contract 1336* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1337* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1338* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1339* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1340* [DeviceRequest](devicerequest.html): Business identifier for request/order 1341* [DeviceUsage](deviceusage.html): Search by identifier 1342* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1343* [DocumentReference](documentreference.html): Identifier of the attachment binary 1344* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1345* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1346* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1347* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1348* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1349* [Flag](flag.html): Business identifier 1350* [Goal](goal.html): External Ids for this goal 1351* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1352* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1353* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1354* [Immunization](immunization.html): Business identifier 1355* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1356* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1357* [Invoice](invoice.html): Business Identifier for item 1358* [List](list.html): Business identifier 1359* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1360* [Medication](medication.html): Returns medications with this external identifier 1361* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1362* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1363* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1364* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1365* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1366* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1367* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1368* [Observation](observation.html): The unique id for a particular observation 1369* [Person](person.html): A person Identifier 1370* [Procedure](procedure.html): A unique identifier for a procedure 1371* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1372* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1373* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1374* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1375* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1376* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1377* [Specimen](specimen.html): The unique identifier associated with the specimen 1378* [SupplyDelivery](supplydelivery.html): External identifier 1379* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1380* [Task](task.html): Search for a task instance by its business identifier 1381* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1382</b><br> 1383 * Type: <b>token</b><br> 1384 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1385 * </p> 1386 */ 1387 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1388 public static final String SP_IDENTIFIER = "identifier"; 1389 /** 1390 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1391 * <p> 1392 * Description: <b>Multiple Resources: 1393 1394* [Account](account.html): Account number 1395* [AdverseEvent](adverseevent.html): Business identifier for the event 1396* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1397* [Appointment](appointment.html): An Identifier of the Appointment 1398* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1399* [Basic](basic.html): Business identifier 1400* [BodyStructure](bodystructure.html): Bodystructure identifier 1401* [CarePlan](careplan.html): External Ids for this plan 1402* [CareTeam](careteam.html): External Ids for this team 1403* [ChargeItem](chargeitem.html): Business Identifier for item 1404* [Claim](claim.html): The primary identifier of the financial resource 1405* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1406* [ClinicalImpression](clinicalimpression.html): Business identifier 1407* [Communication](communication.html): Unique identifier 1408* [CommunicationRequest](communicationrequest.html): Unique identifier 1409* [Composition](composition.html): Version-independent identifier for the Composition 1410* [Condition](condition.html): A unique identifier of the condition record 1411* [Consent](consent.html): Identifier for this record (external references) 1412* [Contract](contract.html): The identity of the contract 1413* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1414* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1415* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1416* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1417* [DeviceRequest](devicerequest.html): Business identifier for request/order 1418* [DeviceUsage](deviceusage.html): Search by identifier 1419* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1420* [DocumentReference](documentreference.html): Identifier of the attachment binary 1421* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1422* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1423* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1424* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1425* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1426* [Flag](flag.html): Business identifier 1427* [Goal](goal.html): External Ids for this goal 1428* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1429* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1430* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1431* [Immunization](immunization.html): Business identifier 1432* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1433* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1434* [Invoice](invoice.html): Business Identifier for item 1435* [List](list.html): Business identifier 1436* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1437* [Medication](medication.html): Returns medications with this external identifier 1438* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1439* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1440* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1441* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1442* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1443* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1444* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1445* [Observation](observation.html): The unique id for a particular observation 1446* [Person](person.html): A person Identifier 1447* [Procedure](procedure.html): A unique identifier for a procedure 1448* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1449* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1450* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1451* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1452* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1453* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1454* [Specimen](specimen.html): The unique identifier associated with the specimen 1455* [SupplyDelivery](supplydelivery.html): External identifier 1456* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1457* [Task](task.html): Search for a task instance by its business identifier 1458* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1459</b><br> 1460 * Type: <b>token</b><br> 1461 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1462 * </p> 1463 */ 1464 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1465 1466 /** 1467 * Search parameter: <b>patient</b> 1468 * <p> 1469 * Description: <b>Multiple Resources: 1470 1471* [Account](account.html): The entity that caused the expenses 1472* [AdverseEvent](adverseevent.html): Subject impacted by event 1473* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1474* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1475* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1476* [AuditEvent](auditevent.html): Where the activity involved patient data 1477* [Basic](basic.html): Identifies the focus of this resource 1478* [BodyStructure](bodystructure.html): Who this is about 1479* [CarePlan](careplan.html): Who the care plan is for 1480* [CareTeam](careteam.html): Who care team is for 1481* [ChargeItem](chargeitem.html): Individual service was done for/to 1482* [Claim](claim.html): Patient receiving the products or services 1483* [ClaimResponse](claimresponse.html): The subject of care 1484* [ClinicalImpression](clinicalimpression.html): Patient assessed 1485* [Communication](communication.html): Focus of message 1486* [CommunicationRequest](communicationrequest.html): Focus of message 1487* [Composition](composition.html): Who and/or what the composition is about 1488* [Condition](condition.html): Who has the condition? 1489* [Consent](consent.html): Who the consent applies to 1490* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1491* [Coverage](coverage.html): Retrieve coverages for a patient 1492* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1493* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1494* [DetectedIssue](detectedissue.html): Associated patient 1495* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1496* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1497* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1498* [DocumentReference](documentreference.html): Who/what is the subject of the document 1499* [Encounter](encounter.html): The patient present at the encounter 1500* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1501* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1502* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1503* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1504* [Flag](flag.html): The identity of a subject to list flags for 1505* [Goal](goal.html): Who this goal is intended for 1506* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1507* [ImagingSelection](imagingselection.html): Who the study is about 1508* [ImagingStudy](imagingstudy.html): Who the study is about 1509* [Immunization](immunization.html): The patient for the vaccination record 1510* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1511* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1512* [Invoice](invoice.html): Recipient(s) of goods and services 1513* [List](list.html): If all resources have the same subject 1514* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1515* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1516* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1517* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1518* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1519* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1520* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1521* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1522* [Observation](observation.html): The subject that the observation is about (if patient) 1523* [Person](person.html): The Person links to this Patient 1524* [Procedure](procedure.html): Search by subject - a patient 1525* [Provenance](provenance.html): Where the activity involved patient data 1526* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1527* [RelatedPerson](relatedperson.html): The patient this related person is related to 1528* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1529* [ResearchSubject](researchsubject.html): Who or what is part of study 1530* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1531* [ServiceRequest](servicerequest.html): Search by subject - a patient 1532* [Specimen](specimen.html): The patient the specimen comes from 1533* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1534* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1535* [Task](task.html): Search by patient 1536* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1537</b><br> 1538 * Type: <b>reference</b><br> 1539 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1540 * </p> 1541 */ 1542 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 1543 public static final String SP_PATIENT = "patient"; 1544 /** 1545 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1546 * <p> 1547 * Description: <b>Multiple Resources: 1548 1549* [Account](account.html): The entity that caused the expenses 1550* [AdverseEvent](adverseevent.html): Subject impacted by event 1551* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1552* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1553* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1554* [AuditEvent](auditevent.html): Where the activity involved patient data 1555* [Basic](basic.html): Identifies the focus of this resource 1556* [BodyStructure](bodystructure.html): Who this is about 1557* [CarePlan](careplan.html): Who the care plan is for 1558* [CareTeam](careteam.html): Who care team is for 1559* [ChargeItem](chargeitem.html): Individual service was done for/to 1560* [Claim](claim.html): Patient receiving the products or services 1561* [ClaimResponse](claimresponse.html): The subject of care 1562* [ClinicalImpression](clinicalimpression.html): Patient assessed 1563* [Communication](communication.html): Focus of message 1564* [CommunicationRequest](communicationrequest.html): Focus of message 1565* [Composition](composition.html): Who and/or what the composition is about 1566* [Condition](condition.html): Who has the condition? 1567* [Consent](consent.html): Who the consent applies to 1568* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1569* [Coverage](coverage.html): Retrieve coverages for a patient 1570* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1571* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1572* [DetectedIssue](detectedissue.html): Associated patient 1573* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1574* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1575* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1576* [DocumentReference](documentreference.html): Who/what is the subject of the document 1577* [Encounter](encounter.html): The patient present at the encounter 1578* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1579* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1580* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1581* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1582* [Flag](flag.html): The identity of a subject to list flags for 1583* [Goal](goal.html): Who this goal is intended for 1584* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1585* [ImagingSelection](imagingselection.html): Who the study is about 1586* [ImagingStudy](imagingstudy.html): Who the study is about 1587* [Immunization](immunization.html): The patient for the vaccination record 1588* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1589* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1590* [Invoice](invoice.html): Recipient(s) of goods and services 1591* [List](list.html): If all resources have the same subject 1592* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1593* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1594* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1595* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1596* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1597* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1598* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1599* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1600* [Observation](observation.html): The subject that the observation is about (if patient) 1601* [Person](person.html): The Person links to this Patient 1602* [Procedure](procedure.html): Search by subject - a patient 1603* [Provenance](provenance.html): Where the activity involved patient data 1604* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1605* [RelatedPerson](relatedperson.html): The patient this related person is related to 1606* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1607* [ResearchSubject](researchsubject.html): Who or what is part of study 1608* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1609* [ServiceRequest](servicerequest.html): Search by subject - a patient 1610* [Specimen](specimen.html): The patient the specimen comes from 1611* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1612* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1613* [Task](task.html): Search by patient 1614* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1615</b><br> 1616 * Type: <b>reference</b><br> 1617 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1618 * </p> 1619 */ 1620 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1621 1622/** 1623 * Constant for fluent queries to be used to add include statements. Specifies 1624 * the path value of "<b>AppointmentResponse:patient</b>". 1625 */ 1626 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AppointmentResponse:patient").toLocked(); 1627 1628 1629} 1630