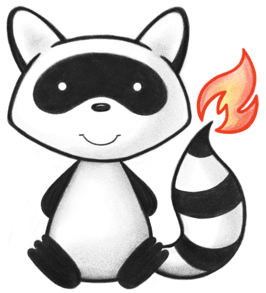
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This Resource provides one or more comments, classifiers or ratings about a Resource and supports attribution and rights management metadata for the added content. 052 */ 053@ResourceDef(name="ArtifactAssessment", profile="http://hl7.org/fhir/StructureDefinition/ArtifactAssessment") 054public class ArtifactAssessment extends DomainResource { 055 056 public enum ArtifactAssessmentDisposition { 057 /** 058 * The comment is unresolved 059 */ 060 UNRESOLVED, 061 /** 062 * The comment is not persuasive (rejected in full) 063 */ 064 NOTPERSUASIVE, 065 /** 066 * The comment is persuasive (accepted in full) 067 */ 068 PERSUASIVE, 069 /** 070 * The comment is persuasive with modification (partially accepted) 071 */ 072 PERSUASIVEWITHMODIFICATION, 073 /** 074 * The comment is not persuasive with modification (partially rejected) 075 */ 076 NOTPERSUASIVEWITHMODIFICATION, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static ArtifactAssessmentDisposition fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("unresolved".equals(codeString)) 085 return UNRESOLVED; 086 if ("not-persuasive".equals(codeString)) 087 return NOTPERSUASIVE; 088 if ("persuasive".equals(codeString)) 089 return PERSUASIVE; 090 if ("persuasive-with-modification".equals(codeString)) 091 return PERSUASIVEWITHMODIFICATION; 092 if ("not-persuasive-with-modification".equals(codeString)) 093 return NOTPERSUASIVEWITHMODIFICATION; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown ArtifactAssessmentDisposition code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case UNRESOLVED: return "unresolved"; 102 case NOTPERSUASIVE: return "not-persuasive"; 103 case PERSUASIVE: return "persuasive"; 104 case PERSUASIVEWITHMODIFICATION: return "persuasive-with-modification"; 105 case NOTPERSUASIVEWITHMODIFICATION: return "not-persuasive-with-modification"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case UNRESOLVED: return "http://hl7.org/fhir/artifactassessment-disposition"; 113 case NOTPERSUASIVE: return "http://hl7.org/fhir/artifactassessment-disposition"; 114 case PERSUASIVE: return "http://hl7.org/fhir/artifactassessment-disposition"; 115 case PERSUASIVEWITHMODIFICATION: return "http://hl7.org/fhir/artifactassessment-disposition"; 116 case NOTPERSUASIVEWITHMODIFICATION: return "http://hl7.org/fhir/artifactassessment-disposition"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case UNRESOLVED: return "The comment is unresolved"; 124 case NOTPERSUASIVE: return "The comment is not persuasive (rejected in full)"; 125 case PERSUASIVE: return "The comment is persuasive (accepted in full)"; 126 case PERSUASIVEWITHMODIFICATION: return "The comment is persuasive with modification (partially accepted)"; 127 case NOTPERSUASIVEWITHMODIFICATION: return "The comment is not persuasive with modification (partially rejected)"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case UNRESOLVED: return "Unresolved"; 135 case NOTPERSUASIVE: return "Not Persuasive"; 136 case PERSUASIVE: return "Persuasive"; 137 case PERSUASIVEWITHMODIFICATION: return "Persuasive with Modification"; 138 case NOTPERSUASIVEWITHMODIFICATION: return "Not Persuasive with Modification"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class ArtifactAssessmentDispositionEnumFactory implements EnumFactory<ArtifactAssessmentDisposition> { 146 public ArtifactAssessmentDisposition fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("unresolved".equals(codeString)) 151 return ArtifactAssessmentDisposition.UNRESOLVED; 152 if ("not-persuasive".equals(codeString)) 153 return ArtifactAssessmentDisposition.NOTPERSUASIVE; 154 if ("persuasive".equals(codeString)) 155 return ArtifactAssessmentDisposition.PERSUASIVE; 156 if ("persuasive-with-modification".equals(codeString)) 157 return ArtifactAssessmentDisposition.PERSUASIVEWITHMODIFICATION; 158 if ("not-persuasive-with-modification".equals(codeString)) 159 return ArtifactAssessmentDisposition.NOTPERSUASIVEWITHMODIFICATION; 160 throw new IllegalArgumentException("Unknown ArtifactAssessmentDisposition code '"+codeString+"'"); 161 } 162 public Enumeration<ArtifactAssessmentDisposition> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.NULL, code); 170 if ("unresolved".equals(codeString)) 171 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.UNRESOLVED, code); 172 if ("not-persuasive".equals(codeString)) 173 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.NOTPERSUASIVE, code); 174 if ("persuasive".equals(codeString)) 175 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.PERSUASIVE, code); 176 if ("persuasive-with-modification".equals(codeString)) 177 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.PERSUASIVEWITHMODIFICATION, code); 178 if ("not-persuasive-with-modification".equals(codeString)) 179 return new Enumeration<ArtifactAssessmentDisposition>(this, ArtifactAssessmentDisposition.NOTPERSUASIVEWITHMODIFICATION, code); 180 throw new FHIRException("Unknown ArtifactAssessmentDisposition code '"+codeString+"'"); 181 } 182 public String toCode(ArtifactAssessmentDisposition code) { 183 if (code == ArtifactAssessmentDisposition.NULL) 184 return null; 185 if (code == ArtifactAssessmentDisposition.UNRESOLVED) 186 return "unresolved"; 187 if (code == ArtifactAssessmentDisposition.NOTPERSUASIVE) 188 return "not-persuasive"; 189 if (code == ArtifactAssessmentDisposition.PERSUASIVE) 190 return "persuasive"; 191 if (code == ArtifactAssessmentDisposition.PERSUASIVEWITHMODIFICATION) 192 return "persuasive-with-modification"; 193 if (code == ArtifactAssessmentDisposition.NOTPERSUASIVEWITHMODIFICATION) 194 return "not-persuasive-with-modification"; 195 return "?"; 196 } 197 public String toSystem(ArtifactAssessmentDisposition code) { 198 return code.getSystem(); 199 } 200 } 201 202 public enum ArtifactAssessmentInformationType { 203 /** 204 * A comment on the artifact 205 */ 206 COMMENT, 207 /** 208 * A classifier of the artifact 209 */ 210 CLASSIFIER, 211 /** 212 * A rating of the artifact 213 */ 214 RATING, 215 /** 216 * A container for multiple components 217 */ 218 CONTAINER, 219 /** 220 * A response to a comment 221 */ 222 RESPONSE, 223 /** 224 * A change request for the artifact 225 */ 226 CHANGEREQUEST, 227 /** 228 * added to help the parsers with the generic types 229 */ 230 NULL; 231 public static ArtifactAssessmentInformationType fromCode(String codeString) throws FHIRException { 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("comment".equals(codeString)) 235 return COMMENT; 236 if ("classifier".equals(codeString)) 237 return CLASSIFIER; 238 if ("rating".equals(codeString)) 239 return RATING; 240 if ("container".equals(codeString)) 241 return CONTAINER; 242 if ("response".equals(codeString)) 243 return RESPONSE; 244 if ("change-request".equals(codeString)) 245 return CHANGEREQUEST; 246 if (Configuration.isAcceptInvalidEnums()) 247 return null; 248 else 249 throw new FHIRException("Unknown ArtifactAssessmentInformationType code '"+codeString+"'"); 250 } 251 public String toCode() { 252 switch (this) { 253 case COMMENT: return "comment"; 254 case CLASSIFIER: return "classifier"; 255 case RATING: return "rating"; 256 case CONTAINER: return "container"; 257 case RESPONSE: return "response"; 258 case CHANGEREQUEST: return "change-request"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 public String getSystem() { 264 switch (this) { 265 case COMMENT: return "http://hl7.org/fhir/artifactassessment-information-type"; 266 case CLASSIFIER: return "http://hl7.org/fhir/artifactassessment-information-type"; 267 case RATING: return "http://hl7.org/fhir/artifactassessment-information-type"; 268 case CONTAINER: return "http://hl7.org/fhir/artifactassessment-information-type"; 269 case RESPONSE: return "http://hl7.org/fhir/artifactassessment-information-type"; 270 case CHANGEREQUEST: return "http://hl7.org/fhir/artifactassessment-information-type"; 271 case NULL: return null; 272 default: return "?"; 273 } 274 } 275 public String getDefinition() { 276 switch (this) { 277 case COMMENT: return "A comment on the artifact"; 278 case CLASSIFIER: return "A classifier of the artifact"; 279 case RATING: return "A rating of the artifact"; 280 case CONTAINER: return "A container for multiple components"; 281 case RESPONSE: return "A response to a comment"; 282 case CHANGEREQUEST: return "A change request for the artifact"; 283 case NULL: return null; 284 default: return "?"; 285 } 286 } 287 public String getDisplay() { 288 switch (this) { 289 case COMMENT: return "Comment"; 290 case CLASSIFIER: return "Classifier"; 291 case RATING: return "Rating"; 292 case CONTAINER: return "Container"; 293 case RESPONSE: return "Response"; 294 case CHANGEREQUEST: return "Change Request"; 295 case NULL: return null; 296 default: return "?"; 297 } 298 } 299 } 300 301 public static class ArtifactAssessmentInformationTypeEnumFactory implements EnumFactory<ArtifactAssessmentInformationType> { 302 public ArtifactAssessmentInformationType fromCode(String codeString) throws IllegalArgumentException { 303 if (codeString == null || "".equals(codeString)) 304 if (codeString == null || "".equals(codeString)) 305 return null; 306 if ("comment".equals(codeString)) 307 return ArtifactAssessmentInformationType.COMMENT; 308 if ("classifier".equals(codeString)) 309 return ArtifactAssessmentInformationType.CLASSIFIER; 310 if ("rating".equals(codeString)) 311 return ArtifactAssessmentInformationType.RATING; 312 if ("container".equals(codeString)) 313 return ArtifactAssessmentInformationType.CONTAINER; 314 if ("response".equals(codeString)) 315 return ArtifactAssessmentInformationType.RESPONSE; 316 if ("change-request".equals(codeString)) 317 return ArtifactAssessmentInformationType.CHANGEREQUEST; 318 throw new IllegalArgumentException("Unknown ArtifactAssessmentInformationType code '"+codeString+"'"); 319 } 320 public Enumeration<ArtifactAssessmentInformationType> fromType(PrimitiveType<?> code) throws FHIRException { 321 if (code == null) 322 return null; 323 if (code.isEmpty()) 324 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.NULL, code); 325 String codeString = ((PrimitiveType) code).asStringValue(); 326 if (codeString == null || "".equals(codeString)) 327 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.NULL, code); 328 if ("comment".equals(codeString)) 329 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.COMMENT, code); 330 if ("classifier".equals(codeString)) 331 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.CLASSIFIER, code); 332 if ("rating".equals(codeString)) 333 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.RATING, code); 334 if ("container".equals(codeString)) 335 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.CONTAINER, code); 336 if ("response".equals(codeString)) 337 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.RESPONSE, code); 338 if ("change-request".equals(codeString)) 339 return new Enumeration<ArtifactAssessmentInformationType>(this, ArtifactAssessmentInformationType.CHANGEREQUEST, code); 340 throw new FHIRException("Unknown ArtifactAssessmentInformationType code '"+codeString+"'"); 341 } 342 public String toCode(ArtifactAssessmentInformationType code) { 343 if (code == ArtifactAssessmentInformationType.NULL) 344 return null; 345 if (code == ArtifactAssessmentInformationType.COMMENT) 346 return "comment"; 347 if (code == ArtifactAssessmentInformationType.CLASSIFIER) 348 return "classifier"; 349 if (code == ArtifactAssessmentInformationType.RATING) 350 return "rating"; 351 if (code == ArtifactAssessmentInformationType.CONTAINER) 352 return "container"; 353 if (code == ArtifactAssessmentInformationType.RESPONSE) 354 return "response"; 355 if (code == ArtifactAssessmentInformationType.CHANGEREQUEST) 356 return "change-request"; 357 return "?"; 358 } 359 public String toSystem(ArtifactAssessmentInformationType code) { 360 return code.getSystem(); 361 } 362 } 363 364 public enum ArtifactAssessmentWorkflowStatus { 365 /** 366 * The comment has been submitted, but the responsible party has not yet been determined, or the responsible party has not yet determined the next steps to be taken. 367 */ 368 SUBMITTED, 369 /** 370 * The comment has been triaged, meaning the responsible party has been determined and next steps have been identified to address the comment. 371 */ 372 TRIAGED, 373 /** 374 * The comment is waiting for input from a specific party before next steps can be taken. 375 */ 376 WAITINGFORINPUT, 377 /** 378 * The comment has been resolved and no changes resulted from the resolution 379 */ 380 RESOLVEDNOCHANGE, 381 /** 382 * The comment has been resolved and changes are required to address the comment 383 */ 384 RESOLVEDCHANGEREQUIRED, 385 /** 386 * The comment is acceptable, but resolution of the comment and application of any associated changes have been deferred 387 */ 388 DEFERRED, 389 /** 390 * The comment is a duplicate of another comment already received 391 */ 392 DUPLICATE, 393 /** 394 * The comment is resolved and any necessary changes have been applied 395 */ 396 APPLIED, 397 /** 398 * The necessary changes to the artifact have been published in a new version of the artifact 399 */ 400 PUBLISHED, 401 /** 402 * The assessment was entered in error 403 */ 404 ENTEREDINERROR, 405 /** 406 * added to help the parsers with the generic types 407 */ 408 NULL; 409 public static ArtifactAssessmentWorkflowStatus fromCode(String codeString) throws FHIRException { 410 if (codeString == null || "".equals(codeString)) 411 return null; 412 if ("submitted".equals(codeString)) 413 return SUBMITTED; 414 if ("triaged".equals(codeString)) 415 return TRIAGED; 416 if ("waiting-for-input".equals(codeString)) 417 return WAITINGFORINPUT; 418 if ("resolved-no-change".equals(codeString)) 419 return RESOLVEDNOCHANGE; 420 if ("resolved-change-required".equals(codeString)) 421 return RESOLVEDCHANGEREQUIRED; 422 if ("deferred".equals(codeString)) 423 return DEFERRED; 424 if ("duplicate".equals(codeString)) 425 return DUPLICATE; 426 if ("applied".equals(codeString)) 427 return APPLIED; 428 if ("published".equals(codeString)) 429 return PUBLISHED; 430 if ("entered-in-error".equals(codeString)) 431 return ENTEREDINERROR; 432 if (Configuration.isAcceptInvalidEnums()) 433 return null; 434 else 435 throw new FHIRException("Unknown ArtifactAssessmentWorkflowStatus code '"+codeString+"'"); 436 } 437 public String toCode() { 438 switch (this) { 439 case SUBMITTED: return "submitted"; 440 case TRIAGED: return "triaged"; 441 case WAITINGFORINPUT: return "waiting-for-input"; 442 case RESOLVEDNOCHANGE: return "resolved-no-change"; 443 case RESOLVEDCHANGEREQUIRED: return "resolved-change-required"; 444 case DEFERRED: return "deferred"; 445 case DUPLICATE: return "duplicate"; 446 case APPLIED: return "applied"; 447 case PUBLISHED: return "published"; 448 case ENTEREDINERROR: return "entered-in-error"; 449 case NULL: return null; 450 default: return "?"; 451 } 452 } 453 public String getSystem() { 454 switch (this) { 455 case SUBMITTED: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 456 case TRIAGED: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 457 case WAITINGFORINPUT: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 458 case RESOLVEDNOCHANGE: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 459 case RESOLVEDCHANGEREQUIRED: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 460 case DEFERRED: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 461 case DUPLICATE: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 462 case APPLIED: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 463 case PUBLISHED: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 464 case ENTEREDINERROR: return "http://hl7.org/fhir/artifactassessment-workflow-status"; 465 case NULL: return null; 466 default: return "?"; 467 } 468 } 469 public String getDefinition() { 470 switch (this) { 471 case SUBMITTED: return "The comment has been submitted, but the responsible party has not yet been determined, or the responsible party has not yet determined the next steps to be taken."; 472 case TRIAGED: return "The comment has been triaged, meaning the responsible party has been determined and next steps have been identified to address the comment."; 473 case WAITINGFORINPUT: return "The comment is waiting for input from a specific party before next steps can be taken."; 474 case RESOLVEDNOCHANGE: return "The comment has been resolved and no changes resulted from the resolution"; 475 case RESOLVEDCHANGEREQUIRED: return "The comment has been resolved and changes are required to address the comment"; 476 case DEFERRED: return "The comment is acceptable, but resolution of the comment and application of any associated changes have been deferred"; 477 case DUPLICATE: return "The comment is a duplicate of another comment already received"; 478 case APPLIED: return "The comment is resolved and any necessary changes have been applied"; 479 case PUBLISHED: return "The necessary changes to the artifact have been published in a new version of the artifact"; 480 case ENTEREDINERROR: return "The assessment was entered in error"; 481 case NULL: return null; 482 default: return "?"; 483 } 484 } 485 public String getDisplay() { 486 switch (this) { 487 case SUBMITTED: return "Submitted"; 488 case TRIAGED: return "Triaged"; 489 case WAITINGFORINPUT: return "Waiting for Input"; 490 case RESOLVEDNOCHANGE: return "Resolved - No Change"; 491 case RESOLVEDCHANGEREQUIRED: return "Resolved - Change Required"; 492 case DEFERRED: return "Deferred"; 493 case DUPLICATE: return "Duplicate"; 494 case APPLIED: return "Applied"; 495 case PUBLISHED: return "Published"; 496 case ENTEREDINERROR: return "Entered in Error"; 497 case NULL: return null; 498 default: return "?"; 499 } 500 } 501 } 502 503 public static class ArtifactAssessmentWorkflowStatusEnumFactory implements EnumFactory<ArtifactAssessmentWorkflowStatus> { 504 public ArtifactAssessmentWorkflowStatus fromCode(String codeString) throws IllegalArgumentException { 505 if (codeString == null || "".equals(codeString)) 506 if (codeString == null || "".equals(codeString)) 507 return null; 508 if ("submitted".equals(codeString)) 509 return ArtifactAssessmentWorkflowStatus.SUBMITTED; 510 if ("triaged".equals(codeString)) 511 return ArtifactAssessmentWorkflowStatus.TRIAGED; 512 if ("waiting-for-input".equals(codeString)) 513 return ArtifactAssessmentWorkflowStatus.WAITINGFORINPUT; 514 if ("resolved-no-change".equals(codeString)) 515 return ArtifactAssessmentWorkflowStatus.RESOLVEDNOCHANGE; 516 if ("resolved-change-required".equals(codeString)) 517 return ArtifactAssessmentWorkflowStatus.RESOLVEDCHANGEREQUIRED; 518 if ("deferred".equals(codeString)) 519 return ArtifactAssessmentWorkflowStatus.DEFERRED; 520 if ("duplicate".equals(codeString)) 521 return ArtifactAssessmentWorkflowStatus.DUPLICATE; 522 if ("applied".equals(codeString)) 523 return ArtifactAssessmentWorkflowStatus.APPLIED; 524 if ("published".equals(codeString)) 525 return ArtifactAssessmentWorkflowStatus.PUBLISHED; 526 if ("entered-in-error".equals(codeString)) 527 return ArtifactAssessmentWorkflowStatus.ENTEREDINERROR; 528 throw new IllegalArgumentException("Unknown ArtifactAssessmentWorkflowStatus code '"+codeString+"'"); 529 } 530 public Enumeration<ArtifactAssessmentWorkflowStatus> fromType(PrimitiveType<?> code) throws FHIRException { 531 if (code == null) 532 return null; 533 if (code.isEmpty()) 534 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.NULL, code); 535 String codeString = ((PrimitiveType) code).asStringValue(); 536 if (codeString == null || "".equals(codeString)) 537 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.NULL, code); 538 if ("submitted".equals(codeString)) 539 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.SUBMITTED, code); 540 if ("triaged".equals(codeString)) 541 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.TRIAGED, code); 542 if ("waiting-for-input".equals(codeString)) 543 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.WAITINGFORINPUT, code); 544 if ("resolved-no-change".equals(codeString)) 545 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.RESOLVEDNOCHANGE, code); 546 if ("resolved-change-required".equals(codeString)) 547 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.RESOLVEDCHANGEREQUIRED, code); 548 if ("deferred".equals(codeString)) 549 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.DEFERRED, code); 550 if ("duplicate".equals(codeString)) 551 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.DUPLICATE, code); 552 if ("applied".equals(codeString)) 553 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.APPLIED, code); 554 if ("published".equals(codeString)) 555 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.PUBLISHED, code); 556 if ("entered-in-error".equals(codeString)) 557 return new Enumeration<ArtifactAssessmentWorkflowStatus>(this, ArtifactAssessmentWorkflowStatus.ENTEREDINERROR, code); 558 throw new FHIRException("Unknown ArtifactAssessmentWorkflowStatus code '"+codeString+"'"); 559 } 560 public String toCode(ArtifactAssessmentWorkflowStatus code) { 561 if (code == ArtifactAssessmentWorkflowStatus.NULL) 562 return null; 563 if (code == ArtifactAssessmentWorkflowStatus.SUBMITTED) 564 return "submitted"; 565 if (code == ArtifactAssessmentWorkflowStatus.TRIAGED) 566 return "triaged"; 567 if (code == ArtifactAssessmentWorkflowStatus.WAITINGFORINPUT) 568 return "waiting-for-input"; 569 if (code == ArtifactAssessmentWorkflowStatus.RESOLVEDNOCHANGE) 570 return "resolved-no-change"; 571 if (code == ArtifactAssessmentWorkflowStatus.RESOLVEDCHANGEREQUIRED) 572 return "resolved-change-required"; 573 if (code == ArtifactAssessmentWorkflowStatus.DEFERRED) 574 return "deferred"; 575 if (code == ArtifactAssessmentWorkflowStatus.DUPLICATE) 576 return "duplicate"; 577 if (code == ArtifactAssessmentWorkflowStatus.APPLIED) 578 return "applied"; 579 if (code == ArtifactAssessmentWorkflowStatus.PUBLISHED) 580 return "published"; 581 if (code == ArtifactAssessmentWorkflowStatus.ENTEREDINERROR) 582 return "entered-in-error"; 583 return "?"; 584 } 585 public String toSystem(ArtifactAssessmentWorkflowStatus code) { 586 return code.getSystem(); 587 } 588 } 589 590 @Block() 591 public static class ArtifactAssessmentContentComponent extends BackboneElement implements IBaseBackboneElement { 592 /** 593 * The type of information this component of the content represents. 594 */ 595 @Child(name = "informationType", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 596 @Description(shortDefinition="comment | classifier | rating | container | response | change-request", formalDefinition="The type of information this component of the content represents." ) 597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/artifactassessment-information-type") 598 protected Enumeration<ArtifactAssessmentInformationType> informationType; 599 600 /** 601 * A brief summary of the content of this component. 602 */ 603 @Child(name = "summary", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 604 @Description(shortDefinition="Brief summary of the content", formalDefinition="A brief summary of the content of this component." ) 605 protected MarkdownType summary; 606 607 /** 608 * Indicates what type of content this component represents. 609 */ 610 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 611 @Description(shortDefinition="What type of content", formalDefinition="Indicates what type of content this component represents." ) 612 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/certainty-type") 613 protected CodeableConcept type; 614 615 /** 616 * Represents a rating, classifier, or assessment of the artifact. 617 */ 618 @Child(name = "classifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 619 @Description(shortDefinition="Rating, classifier, or assessment", formalDefinition="Represents a rating, classifier, or assessment of the artifact." ) 620 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/certainty-rating") 621 protected List<CodeableConcept> classifier; 622 623 /** 624 * A quantitative rating of the artifact. 625 */ 626 @Child(name = "quantity", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 627 @Description(shortDefinition="Quantitative rating", formalDefinition="A quantitative rating of the artifact." ) 628 protected Quantity quantity; 629 630 /** 631 * Indicates who or what authored the content. 632 */ 633 @Child(name = "author", type = {Patient.class, Practitioner.class, PractitionerRole.class, Organization.class, Device.class}, order=6, min=0, max=1, modifier=false, summary=false) 634 @Description(shortDefinition="Who authored the content", formalDefinition="Indicates who or what authored the content." ) 635 protected Reference author; 636 637 /** 638 * A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource. 639 */ 640 @Child(name = "path", type = {UriType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 641 @Description(shortDefinition="What the comment is directed to", formalDefinition="A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource." ) 642 protected List<UriType> path; 643 644 /** 645 * Additional related artifacts that provide supporting documentation, additional evidence, or further information related to the content. 646 */ 647 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 648 @Description(shortDefinition="Additional information", formalDefinition="Additional related artifacts that provide supporting documentation, additional evidence, or further information related to the content." ) 649 protected List<RelatedArtifact> relatedArtifact; 650 651 /** 652 * Acceptable to publicly share the comment, classifier or rating. 653 */ 654 @Child(name = "freeToShare", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 655 @Description(shortDefinition="Acceptable to publicly share the resource content", formalDefinition="Acceptable to publicly share the comment, classifier or rating." ) 656 protected BooleanType freeToShare; 657 658 /** 659 * If the informationType is container, the components of the content. 660 */ 661 @Child(name = "component", type = {ArtifactAssessmentContentComponent.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 662 @Description(shortDefinition="Contained content", formalDefinition="If the informationType is container, the components of the content." ) 663 protected List<ArtifactAssessmentContentComponent> component; 664 665 private static final long serialVersionUID = -111630435L; 666 667 /** 668 * Constructor 669 */ 670 public ArtifactAssessmentContentComponent() { 671 super(); 672 } 673 674 /** 675 * @return {@link #informationType} (The type of information this component of the content represents.). This is the underlying object with id, value and extensions. The accessor "getInformationType" gives direct access to the value 676 */ 677 public Enumeration<ArtifactAssessmentInformationType> getInformationTypeElement() { 678 if (this.informationType == null) 679 if (Configuration.errorOnAutoCreate()) 680 throw new Error("Attempt to auto-create ArtifactAssessmentContentComponent.informationType"); 681 else if (Configuration.doAutoCreate()) 682 this.informationType = new Enumeration<ArtifactAssessmentInformationType>(new ArtifactAssessmentInformationTypeEnumFactory()); // bb 683 return this.informationType; 684 } 685 686 public boolean hasInformationTypeElement() { 687 return this.informationType != null && !this.informationType.isEmpty(); 688 } 689 690 public boolean hasInformationType() { 691 return this.informationType != null && !this.informationType.isEmpty(); 692 } 693 694 /** 695 * @param value {@link #informationType} (The type of information this component of the content represents.). This is the underlying object with id, value and extensions. The accessor "getInformationType" gives direct access to the value 696 */ 697 public ArtifactAssessmentContentComponent setInformationTypeElement(Enumeration<ArtifactAssessmentInformationType> value) { 698 this.informationType = value; 699 return this; 700 } 701 702 /** 703 * @return The type of information this component of the content represents. 704 */ 705 public ArtifactAssessmentInformationType getInformationType() { 706 return this.informationType == null ? null : this.informationType.getValue(); 707 } 708 709 /** 710 * @param value The type of information this component of the content represents. 711 */ 712 public ArtifactAssessmentContentComponent setInformationType(ArtifactAssessmentInformationType value) { 713 if (value == null) 714 this.informationType = null; 715 else { 716 if (this.informationType == null) 717 this.informationType = new Enumeration<ArtifactAssessmentInformationType>(new ArtifactAssessmentInformationTypeEnumFactory()); 718 this.informationType.setValue(value); 719 } 720 return this; 721 } 722 723 /** 724 * @return {@link #summary} (A brief summary of the content of this component.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 725 */ 726 public MarkdownType getSummaryElement() { 727 if (this.summary == null) 728 if (Configuration.errorOnAutoCreate()) 729 throw new Error("Attempt to auto-create ArtifactAssessmentContentComponent.summary"); 730 else if (Configuration.doAutoCreate()) 731 this.summary = new MarkdownType(); // bb 732 return this.summary; 733 } 734 735 public boolean hasSummaryElement() { 736 return this.summary != null && !this.summary.isEmpty(); 737 } 738 739 public boolean hasSummary() { 740 return this.summary != null && !this.summary.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #summary} (A brief summary of the content of this component.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 745 */ 746 public ArtifactAssessmentContentComponent setSummaryElement(MarkdownType value) { 747 this.summary = value; 748 return this; 749 } 750 751 /** 752 * @return A brief summary of the content of this component. 753 */ 754 public String getSummary() { 755 return this.summary == null ? null : this.summary.getValue(); 756 } 757 758 /** 759 * @param value A brief summary of the content of this component. 760 */ 761 public ArtifactAssessmentContentComponent setSummary(String value) { 762 if (Utilities.noString(value)) 763 this.summary = null; 764 else { 765 if (this.summary == null) 766 this.summary = new MarkdownType(); 767 this.summary.setValue(value); 768 } 769 return this; 770 } 771 772 /** 773 * @return {@link #type} (Indicates what type of content this component represents.) 774 */ 775 public CodeableConcept getType() { 776 if (this.type == null) 777 if (Configuration.errorOnAutoCreate()) 778 throw new Error("Attempt to auto-create ArtifactAssessmentContentComponent.type"); 779 else if (Configuration.doAutoCreate()) 780 this.type = new CodeableConcept(); // cc 781 return this.type; 782 } 783 784 public boolean hasType() { 785 return this.type != null && !this.type.isEmpty(); 786 } 787 788 /** 789 * @param value {@link #type} (Indicates what type of content this component represents.) 790 */ 791 public ArtifactAssessmentContentComponent setType(CodeableConcept value) { 792 this.type = value; 793 return this; 794 } 795 796 /** 797 * @return {@link #classifier} (Represents a rating, classifier, or assessment of the artifact.) 798 */ 799 public List<CodeableConcept> getClassifier() { 800 if (this.classifier == null) 801 this.classifier = new ArrayList<CodeableConcept>(); 802 return this.classifier; 803 } 804 805 /** 806 * @return Returns a reference to <code>this</code> for easy method chaining 807 */ 808 public ArtifactAssessmentContentComponent setClassifier(List<CodeableConcept> theClassifier) { 809 this.classifier = theClassifier; 810 return this; 811 } 812 813 public boolean hasClassifier() { 814 if (this.classifier == null) 815 return false; 816 for (CodeableConcept item : this.classifier) 817 if (!item.isEmpty()) 818 return true; 819 return false; 820 } 821 822 public CodeableConcept addClassifier() { //3 823 CodeableConcept t = new CodeableConcept(); 824 if (this.classifier == null) 825 this.classifier = new ArrayList<CodeableConcept>(); 826 this.classifier.add(t); 827 return t; 828 } 829 830 public ArtifactAssessmentContentComponent addClassifier(CodeableConcept t) { //3 831 if (t == null) 832 return this; 833 if (this.classifier == null) 834 this.classifier = new ArrayList<CodeableConcept>(); 835 this.classifier.add(t); 836 return this; 837 } 838 839 /** 840 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 841 */ 842 public CodeableConcept getClassifierFirstRep() { 843 if (getClassifier().isEmpty()) { 844 addClassifier(); 845 } 846 return getClassifier().get(0); 847 } 848 849 /** 850 * @return {@link #quantity} (A quantitative rating of the artifact.) 851 */ 852 public Quantity getQuantity() { 853 if (this.quantity == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create ArtifactAssessmentContentComponent.quantity"); 856 else if (Configuration.doAutoCreate()) 857 this.quantity = new Quantity(); // cc 858 return this.quantity; 859 } 860 861 public boolean hasQuantity() { 862 return this.quantity != null && !this.quantity.isEmpty(); 863 } 864 865 /** 866 * @param value {@link #quantity} (A quantitative rating of the artifact.) 867 */ 868 public ArtifactAssessmentContentComponent setQuantity(Quantity value) { 869 this.quantity = value; 870 return this; 871 } 872 873 /** 874 * @return {@link #author} (Indicates who or what authored the content.) 875 */ 876 public Reference getAuthor() { 877 if (this.author == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create ArtifactAssessmentContentComponent.author"); 880 else if (Configuration.doAutoCreate()) 881 this.author = new Reference(); // cc 882 return this.author; 883 } 884 885 public boolean hasAuthor() { 886 return this.author != null && !this.author.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #author} (Indicates who or what authored the content.) 891 */ 892 public ArtifactAssessmentContentComponent setAuthor(Reference value) { 893 this.author = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #path} (A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource.) 899 */ 900 public List<UriType> getPath() { 901 if (this.path == null) 902 this.path = new ArrayList<UriType>(); 903 return this.path; 904 } 905 906 /** 907 * @return Returns a reference to <code>this</code> for easy method chaining 908 */ 909 public ArtifactAssessmentContentComponent setPath(List<UriType> thePath) { 910 this.path = thePath; 911 return this; 912 } 913 914 public boolean hasPath() { 915 if (this.path == null) 916 return false; 917 for (UriType item : this.path) 918 if (!item.isEmpty()) 919 return true; 920 return false; 921 } 922 923 /** 924 * @return {@link #path} (A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource.) 925 */ 926 public UriType addPathElement() {//2 927 UriType t = new UriType(); 928 if (this.path == null) 929 this.path = new ArrayList<UriType>(); 930 this.path.add(t); 931 return t; 932 } 933 934 /** 935 * @param value {@link #path} (A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource.) 936 */ 937 public ArtifactAssessmentContentComponent addPath(String value) { //1 938 UriType t = new UriType(); 939 t.setValue(value); 940 if (this.path == null) 941 this.path = new ArrayList<UriType>(); 942 this.path.add(t); 943 return this; 944 } 945 946 /** 947 * @param value {@link #path} (A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource.) 948 */ 949 public boolean hasPath(String value) { 950 if (this.path == null) 951 return false; 952 for (UriType v : this.path) 953 if (v.getValue().equals(value)) // uri 954 return true; 955 return false; 956 } 957 958 /** 959 * @return {@link #relatedArtifact} (Additional related artifacts that provide supporting documentation, additional evidence, or further information related to the content.) 960 */ 961 public List<RelatedArtifact> getRelatedArtifact() { 962 if (this.relatedArtifact == null) 963 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 964 return this.relatedArtifact; 965 } 966 967 /** 968 * @return Returns a reference to <code>this</code> for easy method chaining 969 */ 970 public ArtifactAssessmentContentComponent setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 971 this.relatedArtifact = theRelatedArtifact; 972 return this; 973 } 974 975 public boolean hasRelatedArtifact() { 976 if (this.relatedArtifact == null) 977 return false; 978 for (RelatedArtifact item : this.relatedArtifact) 979 if (!item.isEmpty()) 980 return true; 981 return false; 982 } 983 984 public RelatedArtifact addRelatedArtifact() { //3 985 RelatedArtifact t = new RelatedArtifact(); 986 if (this.relatedArtifact == null) 987 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 988 this.relatedArtifact.add(t); 989 return t; 990 } 991 992 public ArtifactAssessmentContentComponent addRelatedArtifact(RelatedArtifact t) { //3 993 if (t == null) 994 return this; 995 if (this.relatedArtifact == null) 996 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 997 this.relatedArtifact.add(t); 998 return this; 999 } 1000 1001 /** 1002 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 1003 */ 1004 public RelatedArtifact getRelatedArtifactFirstRep() { 1005 if (getRelatedArtifact().isEmpty()) { 1006 addRelatedArtifact(); 1007 } 1008 return getRelatedArtifact().get(0); 1009 } 1010 1011 /** 1012 * @return {@link #freeToShare} (Acceptable to publicly share the comment, classifier or rating.). This is the underlying object with id, value and extensions. The accessor "getFreeToShare" gives direct access to the value 1013 */ 1014 public BooleanType getFreeToShareElement() { 1015 if (this.freeToShare == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create ArtifactAssessmentContentComponent.freeToShare"); 1018 else if (Configuration.doAutoCreate()) 1019 this.freeToShare = new BooleanType(); // bb 1020 return this.freeToShare; 1021 } 1022 1023 public boolean hasFreeToShareElement() { 1024 return this.freeToShare != null && !this.freeToShare.isEmpty(); 1025 } 1026 1027 public boolean hasFreeToShare() { 1028 return this.freeToShare != null && !this.freeToShare.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #freeToShare} (Acceptable to publicly share the comment, classifier or rating.). This is the underlying object with id, value and extensions. The accessor "getFreeToShare" gives direct access to the value 1033 */ 1034 public ArtifactAssessmentContentComponent setFreeToShareElement(BooleanType value) { 1035 this.freeToShare = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return Acceptable to publicly share the comment, classifier or rating. 1041 */ 1042 public boolean getFreeToShare() { 1043 return this.freeToShare == null || this.freeToShare.isEmpty() ? false : this.freeToShare.getValue(); 1044 } 1045 1046 /** 1047 * @param value Acceptable to publicly share the comment, classifier or rating. 1048 */ 1049 public ArtifactAssessmentContentComponent setFreeToShare(boolean value) { 1050 if (this.freeToShare == null) 1051 this.freeToShare = new BooleanType(); 1052 this.freeToShare.setValue(value); 1053 return this; 1054 } 1055 1056 /** 1057 * @return {@link #component} (If the informationType is container, the components of the content.) 1058 */ 1059 public List<ArtifactAssessmentContentComponent> getComponent() { 1060 if (this.component == null) 1061 this.component = new ArrayList<ArtifactAssessmentContentComponent>(); 1062 return this.component; 1063 } 1064 1065 /** 1066 * @return Returns a reference to <code>this</code> for easy method chaining 1067 */ 1068 public ArtifactAssessmentContentComponent setComponent(List<ArtifactAssessmentContentComponent> theComponent) { 1069 this.component = theComponent; 1070 return this; 1071 } 1072 1073 public boolean hasComponent() { 1074 if (this.component == null) 1075 return false; 1076 for (ArtifactAssessmentContentComponent item : this.component) 1077 if (!item.isEmpty()) 1078 return true; 1079 return false; 1080 } 1081 1082 public ArtifactAssessmentContentComponent addComponent() { //3 1083 ArtifactAssessmentContentComponent t = new ArtifactAssessmentContentComponent(); 1084 if (this.component == null) 1085 this.component = new ArrayList<ArtifactAssessmentContentComponent>(); 1086 this.component.add(t); 1087 return t; 1088 } 1089 1090 public ArtifactAssessmentContentComponent addComponent(ArtifactAssessmentContentComponent t) { //3 1091 if (t == null) 1092 return this; 1093 if (this.component == null) 1094 this.component = new ArrayList<ArtifactAssessmentContentComponent>(); 1095 this.component.add(t); 1096 return this; 1097 } 1098 1099 /** 1100 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 1101 */ 1102 public ArtifactAssessmentContentComponent getComponentFirstRep() { 1103 if (getComponent().isEmpty()) { 1104 addComponent(); 1105 } 1106 return getComponent().get(0); 1107 } 1108 1109 protected void listChildren(List<Property> children) { 1110 super.listChildren(children); 1111 children.add(new Property("informationType", "code", "The type of information this component of the content represents.", 0, 1, informationType)); 1112 children.add(new Property("summary", "markdown", "A brief summary of the content of this component.", 0, 1, summary)); 1113 children.add(new Property("type", "CodeableConcept", "Indicates what type of content this component represents.", 0, 1, type)); 1114 children.add(new Property("classifier", "CodeableConcept", "Represents a rating, classifier, or assessment of the artifact.", 0, java.lang.Integer.MAX_VALUE, classifier)); 1115 children.add(new Property("quantity", "Quantity", "A quantitative rating of the artifact.", 0, 1, quantity)); 1116 children.add(new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization|Device)", "Indicates who or what authored the content.", 0, 1, author)); 1117 children.add(new Property("path", "uri", "A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource.", 0, java.lang.Integer.MAX_VALUE, path)); 1118 children.add(new Property("relatedArtifact", "RelatedArtifact", "Additional related artifacts that provide supporting documentation, additional evidence, or further information related to the content.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 1119 children.add(new Property("freeToShare", "boolean", "Acceptable to publicly share the comment, classifier or rating.", 0, 1, freeToShare)); 1120 children.add(new Property("component", "@ArtifactAssessment.content", "If the informationType is container, the components of the content.", 0, java.lang.Integer.MAX_VALUE, component)); 1121 } 1122 1123 @Override 1124 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1125 switch (_hash) { 1126 case 1302856326: /*informationType*/ return new Property("informationType", "code", "The type of information this component of the content represents.", 0, 1, informationType); 1127 case -1857640538: /*summary*/ return new Property("summary", "markdown", "A brief summary of the content of this component.", 0, 1, summary); 1128 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates what type of content this component represents.", 0, 1, type); 1129 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "Represents a rating, classifier, or assessment of the artifact.", 0, java.lang.Integer.MAX_VALUE, classifier); 1130 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "A quantitative rating of the artifact.", 0, 1, quantity); 1131 case -1406328437: /*author*/ return new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization|Device)", "Indicates who or what authored the content.", 0, 1, author); 1132 case 3433509: /*path*/ return new Property("path", "uri", "A URI that points to what the comment is about, such as a line of text in the CQL, or a specific element in a resource.", 0, java.lang.Integer.MAX_VALUE, path); 1133 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Additional related artifacts that provide supporting documentation, additional evidence, or further information related to the content.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 1134 case -1268656616: /*freeToShare*/ return new Property("freeToShare", "boolean", "Acceptable to publicly share the comment, classifier or rating.", 0, 1, freeToShare); 1135 case -1399907075: /*component*/ return new Property("component", "@ArtifactAssessment.content", "If the informationType is container, the components of the content.", 0, java.lang.Integer.MAX_VALUE, component); 1136 default: return super.getNamedProperty(_hash, _name, _checkValid); 1137 } 1138 1139 } 1140 1141 @Override 1142 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1143 switch (hash) { 1144 case 1302856326: /*informationType*/ return this.informationType == null ? new Base[0] : new Base[] {this.informationType}; // Enumeration<ArtifactAssessmentInformationType> 1145 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : new Base[] {this.summary}; // MarkdownType 1146 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1147 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 1148 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1149 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1150 case 3433509: /*path*/ return this.path == null ? new Base[0] : this.path.toArray(new Base[this.path.size()]); // UriType 1151 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 1152 case -1268656616: /*freeToShare*/ return this.freeToShare == null ? new Base[0] : new Base[] {this.freeToShare}; // BooleanType 1153 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ArtifactAssessmentContentComponent 1154 default: return super.getProperty(hash, name, checkValid); 1155 } 1156 1157 } 1158 1159 @Override 1160 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1161 switch (hash) { 1162 case 1302856326: // informationType 1163 value = new ArtifactAssessmentInformationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1164 this.informationType = (Enumeration) value; // Enumeration<ArtifactAssessmentInformationType> 1165 return value; 1166 case -1857640538: // summary 1167 this.summary = TypeConvertor.castToMarkdown(value); // MarkdownType 1168 return value; 1169 case 3575610: // type 1170 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1171 return value; 1172 case -281470431: // classifier 1173 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1174 return value; 1175 case -1285004149: // quantity 1176 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1177 return value; 1178 case -1406328437: // author 1179 this.author = TypeConvertor.castToReference(value); // Reference 1180 return value; 1181 case 3433509: // path 1182 this.getPath().add(TypeConvertor.castToUri(value)); // UriType 1183 return value; 1184 case 666807069: // relatedArtifact 1185 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 1186 return value; 1187 case -1268656616: // freeToShare 1188 this.freeToShare = TypeConvertor.castToBoolean(value); // BooleanType 1189 return value; 1190 case -1399907075: // component 1191 this.getComponent().add((ArtifactAssessmentContentComponent) value); // ArtifactAssessmentContentComponent 1192 return value; 1193 default: return super.setProperty(hash, name, value); 1194 } 1195 1196 } 1197 1198 @Override 1199 public Base setProperty(String name, Base value) throws FHIRException { 1200 if (name.equals("informationType")) { 1201 value = new ArtifactAssessmentInformationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1202 this.informationType = (Enumeration) value; // Enumeration<ArtifactAssessmentInformationType> 1203 } else if (name.equals("summary")) { 1204 this.summary = TypeConvertor.castToMarkdown(value); // MarkdownType 1205 } else if (name.equals("type")) { 1206 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1207 } else if (name.equals("classifier")) { 1208 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 1209 } else if (name.equals("quantity")) { 1210 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1211 } else if (name.equals("author")) { 1212 this.author = TypeConvertor.castToReference(value); // Reference 1213 } else if (name.equals("path")) { 1214 this.getPath().add(TypeConvertor.castToUri(value)); 1215 } else if (name.equals("relatedArtifact")) { 1216 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 1217 } else if (name.equals("freeToShare")) { 1218 this.freeToShare = TypeConvertor.castToBoolean(value); // BooleanType 1219 } else if (name.equals("component")) { 1220 this.getComponent().add((ArtifactAssessmentContentComponent) value); 1221 } else 1222 return super.setProperty(name, value); 1223 return value; 1224 } 1225 1226 @Override 1227 public void removeChild(String name, Base value) throws FHIRException { 1228 if (name.equals("informationType")) { 1229 value = new ArtifactAssessmentInformationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1230 this.informationType = (Enumeration) value; // Enumeration<ArtifactAssessmentInformationType> 1231 } else if (name.equals("summary")) { 1232 this.summary = null; 1233 } else if (name.equals("type")) { 1234 this.type = null; 1235 } else if (name.equals("classifier")) { 1236 this.getClassifier().remove(value); 1237 } else if (name.equals("quantity")) { 1238 this.quantity = null; 1239 } else if (name.equals("author")) { 1240 this.author = null; 1241 } else if (name.equals("path")) { 1242 this.getPath().remove(value); 1243 } else if (name.equals("relatedArtifact")) { 1244 this.getRelatedArtifact().remove(value); 1245 } else if (name.equals("freeToShare")) { 1246 this.freeToShare = null; 1247 } else if (name.equals("component")) { 1248 this.getComponent().remove((ArtifactAssessmentContentComponent) value); 1249 } else 1250 super.removeChild(name, value); 1251 1252 } 1253 1254 @Override 1255 public Base makeProperty(int hash, String name) throws FHIRException { 1256 switch (hash) { 1257 case 1302856326: return getInformationTypeElement(); 1258 case -1857640538: return getSummaryElement(); 1259 case 3575610: return getType(); 1260 case -281470431: return addClassifier(); 1261 case -1285004149: return getQuantity(); 1262 case -1406328437: return getAuthor(); 1263 case 3433509: return addPathElement(); 1264 case 666807069: return addRelatedArtifact(); 1265 case -1268656616: return getFreeToShareElement(); 1266 case -1399907075: return addComponent(); 1267 default: return super.makeProperty(hash, name); 1268 } 1269 1270 } 1271 1272 @Override 1273 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1274 switch (hash) { 1275 case 1302856326: /*informationType*/ return new String[] {"code"}; 1276 case -1857640538: /*summary*/ return new String[] {"markdown"}; 1277 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1278 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 1279 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1280 case -1406328437: /*author*/ return new String[] {"Reference"}; 1281 case 3433509: /*path*/ return new String[] {"uri"}; 1282 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 1283 case -1268656616: /*freeToShare*/ return new String[] {"boolean"}; 1284 case -1399907075: /*component*/ return new String[] {"@ArtifactAssessment.content"}; 1285 default: return super.getTypesForProperty(hash, name); 1286 } 1287 1288 } 1289 1290 @Override 1291 public Base addChild(String name) throws FHIRException { 1292 if (name.equals("informationType")) { 1293 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.content.informationType"); 1294 } 1295 else if (name.equals("summary")) { 1296 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.content.summary"); 1297 } 1298 else if (name.equals("type")) { 1299 this.type = new CodeableConcept(); 1300 return this.type; 1301 } 1302 else if (name.equals("classifier")) { 1303 return addClassifier(); 1304 } 1305 else if (name.equals("quantity")) { 1306 this.quantity = new Quantity(); 1307 return this.quantity; 1308 } 1309 else if (name.equals("author")) { 1310 this.author = new Reference(); 1311 return this.author; 1312 } 1313 else if (name.equals("path")) { 1314 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.content.path"); 1315 } 1316 else if (name.equals("relatedArtifact")) { 1317 return addRelatedArtifact(); 1318 } 1319 else if (name.equals("freeToShare")) { 1320 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.content.freeToShare"); 1321 } 1322 else if (name.equals("component")) { 1323 return addComponent(); 1324 } 1325 else 1326 return super.addChild(name); 1327 } 1328 1329 public ArtifactAssessmentContentComponent copy() { 1330 ArtifactAssessmentContentComponent dst = new ArtifactAssessmentContentComponent(); 1331 copyValues(dst); 1332 return dst; 1333 } 1334 1335 public void copyValues(ArtifactAssessmentContentComponent dst) { 1336 super.copyValues(dst); 1337 dst.informationType = informationType == null ? null : informationType.copy(); 1338 dst.summary = summary == null ? null : summary.copy(); 1339 dst.type = type == null ? null : type.copy(); 1340 if (classifier != null) { 1341 dst.classifier = new ArrayList<CodeableConcept>(); 1342 for (CodeableConcept i : classifier) 1343 dst.classifier.add(i.copy()); 1344 }; 1345 dst.quantity = quantity == null ? null : quantity.copy(); 1346 dst.author = author == null ? null : author.copy(); 1347 if (path != null) { 1348 dst.path = new ArrayList<UriType>(); 1349 for (UriType i : path) 1350 dst.path.add(i.copy()); 1351 }; 1352 if (relatedArtifact != null) { 1353 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 1354 for (RelatedArtifact i : relatedArtifact) 1355 dst.relatedArtifact.add(i.copy()); 1356 }; 1357 dst.freeToShare = freeToShare == null ? null : freeToShare.copy(); 1358 if (component != null) { 1359 dst.component = new ArrayList<ArtifactAssessmentContentComponent>(); 1360 for (ArtifactAssessmentContentComponent i : component) 1361 dst.component.add(i.copy()); 1362 }; 1363 } 1364 1365 @Override 1366 public boolean equalsDeep(Base other_) { 1367 if (!super.equalsDeep(other_)) 1368 return false; 1369 if (!(other_ instanceof ArtifactAssessmentContentComponent)) 1370 return false; 1371 ArtifactAssessmentContentComponent o = (ArtifactAssessmentContentComponent) other_; 1372 return compareDeep(informationType, o.informationType, true) && compareDeep(summary, o.summary, true) 1373 && compareDeep(type, o.type, true) && compareDeep(classifier, o.classifier, true) && compareDeep(quantity, o.quantity, true) 1374 && compareDeep(author, o.author, true) && compareDeep(path, o.path, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 1375 && compareDeep(freeToShare, o.freeToShare, true) && compareDeep(component, o.component, true); 1376 } 1377 1378 @Override 1379 public boolean equalsShallow(Base other_) { 1380 if (!super.equalsShallow(other_)) 1381 return false; 1382 if (!(other_ instanceof ArtifactAssessmentContentComponent)) 1383 return false; 1384 ArtifactAssessmentContentComponent o = (ArtifactAssessmentContentComponent) other_; 1385 return compareValues(informationType, o.informationType, true) && compareValues(summary, o.summary, true) 1386 && compareValues(path, o.path, true) && compareValues(freeToShare, o.freeToShare, true); 1387 } 1388 1389 public boolean isEmpty() { 1390 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(informationType, summary, type 1391 , classifier, quantity, author, path, relatedArtifact, freeToShare, component 1392 ); 1393 } 1394 1395 public String fhirType() { 1396 return "ArtifactAssessment.content"; 1397 1398 } 1399 1400 } 1401 1402 /** 1403 * A formal identifier that is used to identify this artifact assessment when it is represented in other formats, or referenced in a specification, model, design or an instance. 1404 */ 1405 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1406 @Description(shortDefinition="Additional identifier for the artifact assessment", formalDefinition="A formal identifier that is used to identify this artifact assessment when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1407 protected List<Identifier> identifier; 1408 1409 /** 1410 * A short title for the assessment for use in displaying and selecting. 1411 */ 1412 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1413 @Description(shortDefinition="A short title for the assessment for use in displaying and selecting", formalDefinition="A short title for the assessment for use in displaying and selecting." ) 1414 protected StringType title; 1415 1416 /** 1417 * Display of or reference to the bibliographic citation of the comment, classifier, or rating. 1418 */ 1419 @Child(name = "citeAs", type = {Citation.class, MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1420 @Description(shortDefinition="How to cite the comment or rating", formalDefinition="Display of or reference to the bibliographic citation of the comment, classifier, or rating." ) 1421 protected DataType citeAs; 1422 1423 /** 1424 * The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes. 1425 */ 1426 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1427 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes." ) 1428 protected DateTimeType date; 1429 1430 /** 1431 * A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment. 1432 */ 1433 @Child(name = "copyright", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1434 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment." ) 1435 protected MarkdownType copyright; 1436 1437 /** 1438 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1439 */ 1440 @Child(name = "approvalDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1441 @Description(shortDefinition="When the artifact assessment was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1442 protected DateType approvalDate; 1443 1444 /** 1445 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1446 */ 1447 @Child(name = "lastReviewDate", type = {DateType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1448 @Description(shortDefinition="When the artifact assessment was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 1449 protected DateType lastReviewDate; 1450 1451 /** 1452 * A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about. 1453 */ 1454 @Child(name = "artifact", type = {Reference.class, CanonicalType.class, UriType.class}, order=7, min=1, max=1, modifier=false, summary=true) 1455 @Description(shortDefinition="The artifact assessed, commented upon or rated", formalDefinition="A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about." ) 1456 protected DataType artifact; 1457 1458 /** 1459 * A component comment, classifier, or rating of the artifact. 1460 */ 1461 @Child(name = "content", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1462 @Description(shortDefinition="Comment, classifier, or rating content", formalDefinition="A component comment, classifier, or rating of the artifact." ) 1463 protected List<ArtifactAssessmentContentComponent> content; 1464 1465 /** 1466 * Indicates the workflow status of the comment or change request. 1467 */ 1468 @Child(name = "workflowStatus", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1469 @Description(shortDefinition="submitted | triaged | waiting-for-input | resolved-no-change | resolved-change-required | deferred | duplicate | applied | published | entered-in-error", formalDefinition="Indicates the workflow status of the comment or change request." ) 1470 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/artifactassessment-workflow-status") 1471 protected Enumeration<ArtifactAssessmentWorkflowStatus> workflowStatus; 1472 1473 /** 1474 * Indicates the disposition of the responsible party to the comment or change request. 1475 */ 1476 @Child(name = "disposition", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1477 @Description(shortDefinition="unresolved | not-persuasive | persuasive | persuasive-with-modification | not-persuasive-with-modification", formalDefinition="Indicates the disposition of the responsible party to the comment or change request." ) 1478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/artifactassessment-disposition") 1479 protected Enumeration<ArtifactAssessmentDisposition> disposition; 1480 1481 private static final long serialVersionUID = 525457507L; 1482 1483 /** 1484 * Constructor 1485 */ 1486 public ArtifactAssessment() { 1487 super(); 1488 } 1489 1490 /** 1491 * Constructor 1492 */ 1493 public ArtifactAssessment(DataType artifact) { 1494 super(); 1495 this.setArtifact(artifact); 1496 } 1497 1498 /** 1499 * @return {@link #identifier} (A formal identifier that is used to identify this artifact assessment when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1500 */ 1501 public List<Identifier> getIdentifier() { 1502 if (this.identifier == null) 1503 this.identifier = new ArrayList<Identifier>(); 1504 return this.identifier; 1505 } 1506 1507 /** 1508 * @return Returns a reference to <code>this</code> for easy method chaining 1509 */ 1510 public ArtifactAssessment setIdentifier(List<Identifier> theIdentifier) { 1511 this.identifier = theIdentifier; 1512 return this; 1513 } 1514 1515 public boolean hasIdentifier() { 1516 if (this.identifier == null) 1517 return false; 1518 for (Identifier item : this.identifier) 1519 if (!item.isEmpty()) 1520 return true; 1521 return false; 1522 } 1523 1524 public Identifier addIdentifier() { //3 1525 Identifier t = new Identifier(); 1526 if (this.identifier == null) 1527 this.identifier = new ArrayList<Identifier>(); 1528 this.identifier.add(t); 1529 return t; 1530 } 1531 1532 public ArtifactAssessment addIdentifier(Identifier t) { //3 1533 if (t == null) 1534 return this; 1535 if (this.identifier == null) 1536 this.identifier = new ArrayList<Identifier>(); 1537 this.identifier.add(t); 1538 return this; 1539 } 1540 1541 /** 1542 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1543 */ 1544 public Identifier getIdentifierFirstRep() { 1545 if (getIdentifier().isEmpty()) { 1546 addIdentifier(); 1547 } 1548 return getIdentifier().get(0); 1549 } 1550 1551 /** 1552 * @return {@link #title} (A short title for the assessment for use in displaying and selecting.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1553 */ 1554 public StringType getTitleElement() { 1555 if (this.title == null) 1556 if (Configuration.errorOnAutoCreate()) 1557 throw new Error("Attempt to auto-create ArtifactAssessment.title"); 1558 else if (Configuration.doAutoCreate()) 1559 this.title = new StringType(); // bb 1560 return this.title; 1561 } 1562 1563 public boolean hasTitleElement() { 1564 return this.title != null && !this.title.isEmpty(); 1565 } 1566 1567 public boolean hasTitle() { 1568 return this.title != null && !this.title.isEmpty(); 1569 } 1570 1571 /** 1572 * @param value {@link #title} (A short title for the assessment for use in displaying and selecting.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1573 */ 1574 public ArtifactAssessment setTitleElement(StringType value) { 1575 this.title = value; 1576 return this; 1577 } 1578 1579 /** 1580 * @return A short title for the assessment for use in displaying and selecting. 1581 */ 1582 public String getTitle() { 1583 return this.title == null ? null : this.title.getValue(); 1584 } 1585 1586 /** 1587 * @param value A short title for the assessment for use in displaying and selecting. 1588 */ 1589 public ArtifactAssessment setTitle(String value) { 1590 if (Utilities.noString(value)) 1591 this.title = null; 1592 else { 1593 if (this.title == null) 1594 this.title = new StringType(); 1595 this.title.setValue(value); 1596 } 1597 return this; 1598 } 1599 1600 /** 1601 * @return {@link #citeAs} (Display of or reference to the bibliographic citation of the comment, classifier, or rating.) 1602 */ 1603 public DataType getCiteAs() { 1604 return this.citeAs; 1605 } 1606 1607 /** 1608 * @return {@link #citeAs} (Display of or reference to the bibliographic citation of the comment, classifier, or rating.) 1609 */ 1610 public Reference getCiteAsReference() throws FHIRException { 1611 if (this.citeAs == null) 1612 this.citeAs = new Reference(); 1613 if (!(this.citeAs instanceof Reference)) 1614 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 1615 return (Reference) this.citeAs; 1616 } 1617 1618 public boolean hasCiteAsReference() { 1619 return this != null && this.citeAs instanceof Reference; 1620 } 1621 1622 /** 1623 * @return {@link #citeAs} (Display of or reference to the bibliographic citation of the comment, classifier, or rating.) 1624 */ 1625 public MarkdownType getCiteAsMarkdownType() throws FHIRException { 1626 if (this.citeAs == null) 1627 this.citeAs = new MarkdownType(); 1628 if (!(this.citeAs instanceof MarkdownType)) 1629 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 1630 return (MarkdownType) this.citeAs; 1631 } 1632 1633 public boolean hasCiteAsMarkdownType() { 1634 return this != null && this.citeAs instanceof MarkdownType; 1635 } 1636 1637 public boolean hasCiteAs() { 1638 return this.citeAs != null && !this.citeAs.isEmpty(); 1639 } 1640 1641 /** 1642 * @param value {@link #citeAs} (Display of or reference to the bibliographic citation of the comment, classifier, or rating.) 1643 */ 1644 public ArtifactAssessment setCiteAs(DataType value) { 1645 if (value != null && !(value instanceof Reference || value instanceof MarkdownType)) 1646 throw new FHIRException("Not the right type for ArtifactAssessment.citeAs[x]: "+value.fhirType()); 1647 this.citeAs = value; 1648 return this; 1649 } 1650 1651 /** 1652 * @return {@link #date} (The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1653 */ 1654 public DateTimeType getDateElement() { 1655 if (this.date == null) 1656 if (Configuration.errorOnAutoCreate()) 1657 throw new Error("Attempt to auto-create ArtifactAssessment.date"); 1658 else if (Configuration.doAutoCreate()) 1659 this.date = new DateTimeType(); // bb 1660 return this.date; 1661 } 1662 1663 public boolean hasDateElement() { 1664 return this.date != null && !this.date.isEmpty(); 1665 } 1666 1667 public boolean hasDate() { 1668 return this.date != null && !this.date.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #date} (The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1673 */ 1674 public ArtifactAssessment setDateElement(DateTimeType value) { 1675 this.date = value; 1676 return this; 1677 } 1678 1679 /** 1680 * @return The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes. 1681 */ 1682 public Date getDate() { 1683 return this.date == null ? null : this.date.getValue(); 1684 } 1685 1686 /** 1687 * @param value The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes. 1688 */ 1689 public ArtifactAssessment setDate(Date value) { 1690 if (value == null) 1691 this.date = null; 1692 else { 1693 if (this.date == null) 1694 this.date = new DateTimeType(); 1695 this.date.setValue(value); 1696 } 1697 return this; 1698 } 1699 1700 /** 1701 * @return {@link #copyright} (A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1702 */ 1703 public MarkdownType getCopyrightElement() { 1704 if (this.copyright == null) 1705 if (Configuration.errorOnAutoCreate()) 1706 throw new Error("Attempt to auto-create ArtifactAssessment.copyright"); 1707 else if (Configuration.doAutoCreate()) 1708 this.copyright = new MarkdownType(); // bb 1709 return this.copyright; 1710 } 1711 1712 public boolean hasCopyrightElement() { 1713 return this.copyright != null && !this.copyright.isEmpty(); 1714 } 1715 1716 public boolean hasCopyright() { 1717 return this.copyright != null && !this.copyright.isEmpty(); 1718 } 1719 1720 /** 1721 * @param value {@link #copyright} (A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1722 */ 1723 public ArtifactAssessment setCopyrightElement(MarkdownType value) { 1724 this.copyright = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment. 1730 */ 1731 public String getCopyright() { 1732 return this.copyright == null ? null : this.copyright.getValue(); 1733 } 1734 1735 /** 1736 * @param value A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment. 1737 */ 1738 public ArtifactAssessment setCopyright(String value) { 1739 if (Utilities.noString(value)) 1740 this.copyright = null; 1741 else { 1742 if (this.copyright == null) 1743 this.copyright = new MarkdownType(); 1744 this.copyright.setValue(value); 1745 } 1746 return this; 1747 } 1748 1749 /** 1750 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1751 */ 1752 public DateType getApprovalDateElement() { 1753 if (this.approvalDate == null) 1754 if (Configuration.errorOnAutoCreate()) 1755 throw new Error("Attempt to auto-create ArtifactAssessment.approvalDate"); 1756 else if (Configuration.doAutoCreate()) 1757 this.approvalDate = new DateType(); // bb 1758 return this.approvalDate; 1759 } 1760 1761 public boolean hasApprovalDateElement() { 1762 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1763 } 1764 1765 public boolean hasApprovalDate() { 1766 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1767 } 1768 1769 /** 1770 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1771 */ 1772 public ArtifactAssessment setApprovalDateElement(DateType value) { 1773 this.approvalDate = value; 1774 return this; 1775 } 1776 1777 /** 1778 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1779 */ 1780 public Date getApprovalDate() { 1781 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1782 } 1783 1784 /** 1785 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1786 */ 1787 public ArtifactAssessment setApprovalDate(Date value) { 1788 if (value == null) 1789 this.approvalDate = null; 1790 else { 1791 if (this.approvalDate == null) 1792 this.approvalDate = new DateType(); 1793 this.approvalDate.setValue(value); 1794 } 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1800 */ 1801 public DateType getLastReviewDateElement() { 1802 if (this.lastReviewDate == null) 1803 if (Configuration.errorOnAutoCreate()) 1804 throw new Error("Attempt to auto-create ArtifactAssessment.lastReviewDate"); 1805 else if (Configuration.doAutoCreate()) 1806 this.lastReviewDate = new DateType(); // bb 1807 return this.lastReviewDate; 1808 } 1809 1810 public boolean hasLastReviewDateElement() { 1811 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1812 } 1813 1814 public boolean hasLastReviewDate() { 1815 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1816 } 1817 1818 /** 1819 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1820 */ 1821 public ArtifactAssessment setLastReviewDateElement(DateType value) { 1822 this.lastReviewDate = value; 1823 return this; 1824 } 1825 1826 /** 1827 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1828 */ 1829 public Date getLastReviewDate() { 1830 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1831 } 1832 1833 /** 1834 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1835 */ 1836 public ArtifactAssessment setLastReviewDate(Date value) { 1837 if (value == null) 1838 this.lastReviewDate = null; 1839 else { 1840 if (this.lastReviewDate == null) 1841 this.lastReviewDate = new DateType(); 1842 this.lastReviewDate.setValue(value); 1843 } 1844 return this; 1845 } 1846 1847 /** 1848 * @return {@link #artifact} (A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.) 1849 */ 1850 public DataType getArtifact() { 1851 return this.artifact; 1852 } 1853 1854 /** 1855 * @return {@link #artifact} (A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.) 1856 */ 1857 public Reference getArtifactReference() throws FHIRException { 1858 if (this.artifact == null) 1859 this.artifact = new Reference(); 1860 if (!(this.artifact instanceof Reference)) 1861 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.artifact.getClass().getName()+" was encountered"); 1862 return (Reference) this.artifact; 1863 } 1864 1865 public boolean hasArtifactReference() { 1866 return this != null && this.artifact instanceof Reference; 1867 } 1868 1869 /** 1870 * @return {@link #artifact} (A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.) 1871 */ 1872 public CanonicalType getArtifactCanonicalType() throws FHIRException { 1873 if (this.artifact == null) 1874 this.artifact = new CanonicalType(); 1875 if (!(this.artifact instanceof CanonicalType)) 1876 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.artifact.getClass().getName()+" was encountered"); 1877 return (CanonicalType) this.artifact; 1878 } 1879 1880 public boolean hasArtifactCanonicalType() { 1881 return this != null && this.artifact instanceof CanonicalType; 1882 } 1883 1884 /** 1885 * @return {@link #artifact} (A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.) 1886 */ 1887 public UriType getArtifactUriType() throws FHIRException { 1888 if (this.artifact == null) 1889 this.artifact = new UriType(); 1890 if (!(this.artifact instanceof UriType)) 1891 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.artifact.getClass().getName()+" was encountered"); 1892 return (UriType) this.artifact; 1893 } 1894 1895 public boolean hasArtifactUriType() { 1896 return this != null && this.artifact instanceof UriType; 1897 } 1898 1899 public boolean hasArtifact() { 1900 return this.artifact != null && !this.artifact.isEmpty(); 1901 } 1902 1903 /** 1904 * @param value {@link #artifact} (A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.) 1905 */ 1906 public ArtifactAssessment setArtifact(DataType value) { 1907 if (value != null && !(value instanceof Reference || value instanceof CanonicalType || value instanceof UriType)) 1908 throw new FHIRException("Not the right type for ArtifactAssessment.artifact[x]: "+value.fhirType()); 1909 this.artifact = value; 1910 return this; 1911 } 1912 1913 /** 1914 * @return {@link #content} (A component comment, classifier, or rating of the artifact.) 1915 */ 1916 public List<ArtifactAssessmentContentComponent> getContent() { 1917 if (this.content == null) 1918 this.content = new ArrayList<ArtifactAssessmentContentComponent>(); 1919 return this.content; 1920 } 1921 1922 /** 1923 * @return Returns a reference to <code>this</code> for easy method chaining 1924 */ 1925 public ArtifactAssessment setContent(List<ArtifactAssessmentContentComponent> theContent) { 1926 this.content = theContent; 1927 return this; 1928 } 1929 1930 public boolean hasContent() { 1931 if (this.content == null) 1932 return false; 1933 for (ArtifactAssessmentContentComponent item : this.content) 1934 if (!item.isEmpty()) 1935 return true; 1936 return false; 1937 } 1938 1939 public ArtifactAssessmentContentComponent addContent() { //3 1940 ArtifactAssessmentContentComponent t = new ArtifactAssessmentContentComponent(); 1941 if (this.content == null) 1942 this.content = new ArrayList<ArtifactAssessmentContentComponent>(); 1943 this.content.add(t); 1944 return t; 1945 } 1946 1947 public ArtifactAssessment addContent(ArtifactAssessmentContentComponent t) { //3 1948 if (t == null) 1949 return this; 1950 if (this.content == null) 1951 this.content = new ArrayList<ArtifactAssessmentContentComponent>(); 1952 this.content.add(t); 1953 return this; 1954 } 1955 1956 /** 1957 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist {3} 1958 */ 1959 public ArtifactAssessmentContentComponent getContentFirstRep() { 1960 if (getContent().isEmpty()) { 1961 addContent(); 1962 } 1963 return getContent().get(0); 1964 } 1965 1966 /** 1967 * @return {@link #workflowStatus} (Indicates the workflow status of the comment or change request.). This is the underlying object with id, value and extensions. The accessor "getWorkflowStatus" gives direct access to the value 1968 */ 1969 public Enumeration<ArtifactAssessmentWorkflowStatus> getWorkflowStatusElement() { 1970 if (this.workflowStatus == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create ArtifactAssessment.workflowStatus"); 1973 else if (Configuration.doAutoCreate()) 1974 this.workflowStatus = new Enumeration<ArtifactAssessmentWorkflowStatus>(new ArtifactAssessmentWorkflowStatusEnumFactory()); // bb 1975 return this.workflowStatus; 1976 } 1977 1978 public boolean hasWorkflowStatusElement() { 1979 return this.workflowStatus != null && !this.workflowStatus.isEmpty(); 1980 } 1981 1982 public boolean hasWorkflowStatus() { 1983 return this.workflowStatus != null && !this.workflowStatus.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #workflowStatus} (Indicates the workflow status of the comment or change request.). This is the underlying object with id, value and extensions. The accessor "getWorkflowStatus" gives direct access to the value 1988 */ 1989 public ArtifactAssessment setWorkflowStatusElement(Enumeration<ArtifactAssessmentWorkflowStatus> value) { 1990 this.workflowStatus = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return Indicates the workflow status of the comment or change request. 1996 */ 1997 public ArtifactAssessmentWorkflowStatus getWorkflowStatus() { 1998 return this.workflowStatus == null ? null : this.workflowStatus.getValue(); 1999 } 2000 2001 /** 2002 * @param value Indicates the workflow status of the comment or change request. 2003 */ 2004 public ArtifactAssessment setWorkflowStatus(ArtifactAssessmentWorkflowStatus value) { 2005 if (value == null) 2006 this.workflowStatus = null; 2007 else { 2008 if (this.workflowStatus == null) 2009 this.workflowStatus = new Enumeration<ArtifactAssessmentWorkflowStatus>(new ArtifactAssessmentWorkflowStatusEnumFactory()); 2010 this.workflowStatus.setValue(value); 2011 } 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #disposition} (Indicates the disposition of the responsible party to the comment or change request.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 2017 */ 2018 public Enumeration<ArtifactAssessmentDisposition> getDispositionElement() { 2019 if (this.disposition == null) 2020 if (Configuration.errorOnAutoCreate()) 2021 throw new Error("Attempt to auto-create ArtifactAssessment.disposition"); 2022 else if (Configuration.doAutoCreate()) 2023 this.disposition = new Enumeration<ArtifactAssessmentDisposition>(new ArtifactAssessmentDispositionEnumFactory()); // bb 2024 return this.disposition; 2025 } 2026 2027 public boolean hasDispositionElement() { 2028 return this.disposition != null && !this.disposition.isEmpty(); 2029 } 2030 2031 public boolean hasDisposition() { 2032 return this.disposition != null && !this.disposition.isEmpty(); 2033 } 2034 2035 /** 2036 * @param value {@link #disposition} (Indicates the disposition of the responsible party to the comment or change request.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 2037 */ 2038 public ArtifactAssessment setDispositionElement(Enumeration<ArtifactAssessmentDisposition> value) { 2039 this.disposition = value; 2040 return this; 2041 } 2042 2043 /** 2044 * @return Indicates the disposition of the responsible party to the comment or change request. 2045 */ 2046 public ArtifactAssessmentDisposition getDisposition() { 2047 return this.disposition == null ? null : this.disposition.getValue(); 2048 } 2049 2050 /** 2051 * @param value Indicates the disposition of the responsible party to the comment or change request. 2052 */ 2053 public ArtifactAssessment setDisposition(ArtifactAssessmentDisposition value) { 2054 if (value == null) 2055 this.disposition = null; 2056 else { 2057 if (this.disposition == null) 2058 this.disposition = new Enumeration<ArtifactAssessmentDisposition>(new ArtifactAssessmentDispositionEnumFactory()); 2059 this.disposition.setValue(value); 2060 } 2061 return this; 2062 } 2063 2064 protected void listChildren(List<Property> children) { 2065 super.listChildren(children); 2066 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this artifact assessment when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2067 children.add(new Property("title", "string", "A short title for the assessment for use in displaying and selecting.", 0, 1, title)); 2068 children.add(new Property("citeAs[x]", "Reference(Citation)|markdown", "Display of or reference to the bibliographic citation of the comment, classifier, or rating.", 0, 1, citeAs)); 2069 children.add(new Property("date", "dateTime", "The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes.", 0, 1, date)); 2070 children.add(new Property("copyright", "markdown", "A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment.", 0, 1, copyright)); 2071 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 2072 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 2073 children.add(new Property("artifact[x]", "Reference(Any)|canonical|uri", "A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.", 0, 1, artifact)); 2074 children.add(new Property("content", "", "A component comment, classifier, or rating of the artifact.", 0, java.lang.Integer.MAX_VALUE, content)); 2075 children.add(new Property("workflowStatus", "code", "Indicates the workflow status of the comment or change request.", 0, 1, workflowStatus)); 2076 children.add(new Property("disposition", "code", "Indicates the disposition of the responsible party to the comment or change request.", 0, 1, disposition)); 2077 } 2078 2079 @Override 2080 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2081 switch (_hash) { 2082 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this artifact assessment when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2083 case 110371416: /*title*/ return new Property("title", "string", "A short title for the assessment for use in displaying and selecting.", 0, 1, title); 2084 case -1706539017: /*citeAs[x]*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Display of or reference to the bibliographic citation of the comment, classifier, or rating.", 0, 1, citeAs); 2085 case -1360156695: /*citeAs*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Display of or reference to the bibliographic citation of the comment, classifier, or rating.", 0, 1, citeAs); 2086 case 1269009762: /*citeAsReference*/ return new Property("citeAs[x]", "Reference(Citation)", "Display of or reference to the bibliographic citation of the comment, classifier, or rating.", 0, 1, citeAs); 2087 case 456265720: /*citeAsMarkdown*/ return new Property("citeAs[x]", "markdown", "Display of or reference to the bibliographic citation of the comment, classifier, or rating.", 0, 1, citeAs); 2088 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the artifact assessment was published. The date must change when the disposition changes and it must change if the workflow status code changes. In addition, it should change when the substantive content of the artifact assessment changes.", 0, 1, date); 2089 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the artifact assessment and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the artifact assessment.", 0, 1, copyright); 2090 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 2091 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 2092 case -1130056338: /*artifact[x]*/ return new Property("artifact[x]", "Reference(Any)|canonical|uri", "A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.", 0, 1, artifact); 2093 case -1228798510: /*artifact*/ return new Property("artifact[x]", "Reference(Any)|canonical|uri", "A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.", 0, 1, artifact); 2094 case -683686503: /*artifactReference*/ return new Property("artifact[x]", "Reference(Any)", "A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.", 0, 1, artifact); 2095 case 1069820738: /*artifactCanonical*/ return new Property("artifact[x]", "canonical", "A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.", 0, 1, artifact); 2096 case -1130062278: /*artifactUri*/ return new Property("artifact[x]", "uri", "A reference to a resource, canonical resource, or non-FHIR resource which the comment or assessment is about.", 0, 1, artifact); 2097 case 951530617: /*content*/ return new Property("content", "", "A component comment, classifier, or rating of the artifact.", 0, java.lang.Integer.MAX_VALUE, content); 2098 case 697796753: /*workflowStatus*/ return new Property("workflowStatus", "code", "Indicates the workflow status of the comment or change request.", 0, 1, workflowStatus); 2099 case 583380919: /*disposition*/ return new Property("disposition", "code", "Indicates the disposition of the responsible party to the comment or change request.", 0, 1, disposition); 2100 default: return super.getNamedProperty(_hash, _name, _checkValid); 2101 } 2102 2103 } 2104 2105 @Override 2106 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2107 switch (hash) { 2108 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2109 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2110 case -1360156695: /*citeAs*/ return this.citeAs == null ? new Base[0] : new Base[] {this.citeAs}; // DataType 2111 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2112 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2113 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 2114 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 2115 case -1228798510: /*artifact*/ return this.artifact == null ? new Base[0] : new Base[] {this.artifact}; // DataType 2116 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // ArtifactAssessmentContentComponent 2117 case 697796753: /*workflowStatus*/ return this.workflowStatus == null ? new Base[0] : new Base[] {this.workflowStatus}; // Enumeration<ArtifactAssessmentWorkflowStatus> 2118 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // Enumeration<ArtifactAssessmentDisposition> 2119 default: return super.getProperty(hash, name, checkValid); 2120 } 2121 2122 } 2123 2124 @Override 2125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2126 switch (hash) { 2127 case -1618432855: // identifier 2128 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2129 return value; 2130 case 110371416: // title 2131 this.title = TypeConvertor.castToString(value); // StringType 2132 return value; 2133 case -1360156695: // citeAs 2134 this.citeAs = TypeConvertor.castToType(value); // DataType 2135 return value; 2136 case 3076014: // date 2137 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2138 return value; 2139 case 1522889671: // copyright 2140 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2141 return value; 2142 case 223539345: // approvalDate 2143 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2144 return value; 2145 case -1687512484: // lastReviewDate 2146 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2147 return value; 2148 case -1228798510: // artifact 2149 this.artifact = TypeConvertor.castToType(value); // DataType 2150 return value; 2151 case 951530617: // content 2152 this.getContent().add((ArtifactAssessmentContentComponent) value); // ArtifactAssessmentContentComponent 2153 return value; 2154 case 697796753: // workflowStatus 2155 value = new ArtifactAssessmentWorkflowStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2156 this.workflowStatus = (Enumeration) value; // Enumeration<ArtifactAssessmentWorkflowStatus> 2157 return value; 2158 case 583380919: // disposition 2159 value = new ArtifactAssessmentDispositionEnumFactory().fromType(TypeConvertor.castToCode(value)); 2160 this.disposition = (Enumeration) value; // Enumeration<ArtifactAssessmentDisposition> 2161 return value; 2162 default: return super.setProperty(hash, name, value); 2163 } 2164 2165 } 2166 2167 @Override 2168 public Base setProperty(String name, Base value) throws FHIRException { 2169 if (name.equals("identifier")) { 2170 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2171 } else if (name.equals("title")) { 2172 this.title = TypeConvertor.castToString(value); // StringType 2173 } else if (name.equals("citeAs[x]")) { 2174 this.citeAs = TypeConvertor.castToType(value); // DataType 2175 } else if (name.equals("date")) { 2176 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2177 } else if (name.equals("copyright")) { 2178 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2179 } else if (name.equals("approvalDate")) { 2180 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2181 } else if (name.equals("lastReviewDate")) { 2182 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2183 } else if (name.equals("artifact[x]")) { 2184 this.artifact = TypeConvertor.castToType(value); // DataType 2185 } else if (name.equals("content")) { 2186 this.getContent().add((ArtifactAssessmentContentComponent) value); 2187 } else if (name.equals("workflowStatus")) { 2188 value = new ArtifactAssessmentWorkflowStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2189 this.workflowStatus = (Enumeration) value; // Enumeration<ArtifactAssessmentWorkflowStatus> 2190 } else if (name.equals("disposition")) { 2191 value = new ArtifactAssessmentDispositionEnumFactory().fromType(TypeConvertor.castToCode(value)); 2192 this.disposition = (Enumeration) value; // Enumeration<ArtifactAssessmentDisposition> 2193 } else 2194 return super.setProperty(name, value); 2195 return value; 2196 } 2197 2198 @Override 2199 public void removeChild(String name, Base value) throws FHIRException { 2200 if (name.equals("identifier")) { 2201 this.getIdentifier().remove(value); 2202 } else if (name.equals("title")) { 2203 this.title = null; 2204 } else if (name.equals("citeAs[x]")) { 2205 this.citeAs = null; 2206 } else if (name.equals("date")) { 2207 this.date = null; 2208 } else if (name.equals("copyright")) { 2209 this.copyright = null; 2210 } else if (name.equals("approvalDate")) { 2211 this.approvalDate = null; 2212 } else if (name.equals("lastReviewDate")) { 2213 this.lastReviewDate = null; 2214 } else if (name.equals("artifact[x]")) { 2215 this.artifact = null; 2216 } else if (name.equals("content")) { 2217 this.getContent().remove((ArtifactAssessmentContentComponent) value); 2218 } else if (name.equals("workflowStatus")) { 2219 value = new ArtifactAssessmentWorkflowStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2220 this.workflowStatus = (Enumeration) value; // Enumeration<ArtifactAssessmentWorkflowStatus> 2221 } else if (name.equals("disposition")) { 2222 value = new ArtifactAssessmentDispositionEnumFactory().fromType(TypeConvertor.castToCode(value)); 2223 this.disposition = (Enumeration) value; // Enumeration<ArtifactAssessmentDisposition> 2224 } else 2225 super.removeChild(name, value); 2226 2227 } 2228 2229 @Override 2230 public Base makeProperty(int hash, String name) throws FHIRException { 2231 switch (hash) { 2232 case -1618432855: return addIdentifier(); 2233 case 110371416: return getTitleElement(); 2234 case -1706539017: return getCiteAs(); 2235 case -1360156695: return getCiteAs(); 2236 case 3076014: return getDateElement(); 2237 case 1522889671: return getCopyrightElement(); 2238 case 223539345: return getApprovalDateElement(); 2239 case -1687512484: return getLastReviewDateElement(); 2240 case -1130056338: return getArtifact(); 2241 case -1228798510: return getArtifact(); 2242 case 951530617: return addContent(); 2243 case 697796753: return getWorkflowStatusElement(); 2244 case 583380919: return getDispositionElement(); 2245 default: return super.makeProperty(hash, name); 2246 } 2247 2248 } 2249 2250 @Override 2251 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2252 switch (hash) { 2253 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2254 case 110371416: /*title*/ return new String[] {"string"}; 2255 case -1360156695: /*citeAs*/ return new String[] {"Reference", "markdown"}; 2256 case 3076014: /*date*/ return new String[] {"dateTime"}; 2257 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2258 case 223539345: /*approvalDate*/ return new String[] {"date"}; 2259 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 2260 case -1228798510: /*artifact*/ return new String[] {"Reference", "canonical", "uri"}; 2261 case 951530617: /*content*/ return new String[] {}; 2262 case 697796753: /*workflowStatus*/ return new String[] {"code"}; 2263 case 583380919: /*disposition*/ return new String[] {"code"}; 2264 default: return super.getTypesForProperty(hash, name); 2265 } 2266 2267 } 2268 2269 @Override 2270 public Base addChild(String name) throws FHIRException { 2271 if (name.equals("identifier")) { 2272 return addIdentifier(); 2273 } 2274 else if (name.equals("title")) { 2275 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.title"); 2276 } 2277 else if (name.equals("citeAsReference")) { 2278 this.citeAs = new Reference(); 2279 return this.citeAs; 2280 } 2281 else if (name.equals("citeAsMarkdown")) { 2282 this.citeAs = new MarkdownType(); 2283 return this.citeAs; 2284 } 2285 else if (name.equals("date")) { 2286 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.date"); 2287 } 2288 else if (name.equals("copyright")) { 2289 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.copyright"); 2290 } 2291 else if (name.equals("approvalDate")) { 2292 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.approvalDate"); 2293 } 2294 else if (name.equals("lastReviewDate")) { 2295 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.lastReviewDate"); 2296 } 2297 else if (name.equals("artifactReference")) { 2298 this.artifact = new Reference(); 2299 return this.artifact; 2300 } 2301 else if (name.equals("artifactCanonical")) { 2302 this.artifact = new CanonicalType(); 2303 return this.artifact; 2304 } 2305 else if (name.equals("artifactUri")) { 2306 this.artifact = new UriType(); 2307 return this.artifact; 2308 } 2309 else if (name.equals("content")) { 2310 return addContent(); 2311 } 2312 else if (name.equals("workflowStatus")) { 2313 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.workflowStatus"); 2314 } 2315 else if (name.equals("disposition")) { 2316 throw new FHIRException("Cannot call addChild on a singleton property ArtifactAssessment.disposition"); 2317 } 2318 else 2319 return super.addChild(name); 2320 } 2321 2322 public String fhirType() { 2323 return "ArtifactAssessment"; 2324 2325 } 2326 2327 public ArtifactAssessment copy() { 2328 ArtifactAssessment dst = new ArtifactAssessment(); 2329 copyValues(dst); 2330 return dst; 2331 } 2332 2333 public void copyValues(ArtifactAssessment dst) { 2334 super.copyValues(dst); 2335 if (identifier != null) { 2336 dst.identifier = new ArrayList<Identifier>(); 2337 for (Identifier i : identifier) 2338 dst.identifier.add(i.copy()); 2339 }; 2340 dst.title = title == null ? null : title.copy(); 2341 dst.citeAs = citeAs == null ? null : citeAs.copy(); 2342 dst.date = date == null ? null : date.copy(); 2343 dst.copyright = copyright == null ? null : copyright.copy(); 2344 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2345 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2346 dst.artifact = artifact == null ? null : artifact.copy(); 2347 if (content != null) { 2348 dst.content = new ArrayList<ArtifactAssessmentContentComponent>(); 2349 for (ArtifactAssessmentContentComponent i : content) 2350 dst.content.add(i.copy()); 2351 }; 2352 dst.workflowStatus = workflowStatus == null ? null : workflowStatus.copy(); 2353 dst.disposition = disposition == null ? null : disposition.copy(); 2354 } 2355 2356 protected ArtifactAssessment typedCopy() { 2357 return copy(); 2358 } 2359 2360 @Override 2361 public boolean equalsDeep(Base other_) { 2362 if (!super.equalsDeep(other_)) 2363 return false; 2364 if (!(other_ instanceof ArtifactAssessment)) 2365 return false; 2366 ArtifactAssessment o = (ArtifactAssessment) other_; 2367 return compareDeep(identifier, o.identifier, true) && compareDeep(title, o.title, true) && compareDeep(citeAs, o.citeAs, true) 2368 && compareDeep(date, o.date, true) && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 2369 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(artifact, o.artifact, true) 2370 && compareDeep(content, o.content, true) && compareDeep(workflowStatus, o.workflowStatus, true) 2371 && compareDeep(disposition, o.disposition, true); 2372 } 2373 2374 @Override 2375 public boolean equalsShallow(Base other_) { 2376 if (!super.equalsShallow(other_)) 2377 return false; 2378 if (!(other_ instanceof ArtifactAssessment)) 2379 return false; 2380 ArtifactAssessment o = (ArtifactAssessment) other_; 2381 return compareValues(title, o.title, true) && compareValues(date, o.date, true) && compareValues(copyright, o.copyright, true) 2382 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 2383 && compareValues(workflowStatus, o.workflowStatus, true) && compareValues(disposition, o.disposition, true) 2384 ; 2385 } 2386 2387 public boolean isEmpty() { 2388 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, title, citeAs 2389 , date, copyright, approvalDate, lastReviewDate, artifact, content, workflowStatus 2390 , disposition); 2391 } 2392 2393 @Override 2394 public ResourceType getResourceType() { 2395 return ResourceType.ArtifactAssessment; 2396 } 2397 2398 /** 2399 * Search parameter: <b>date</b> 2400 * <p> 2401 * Description: <b>The artifact assessment publication date</b><br> 2402 * Type: <b>date</b><br> 2403 * Path: <b>ArtifactAssessment.date</b><br> 2404 * </p> 2405 */ 2406 @SearchParamDefinition(name="date", path="ArtifactAssessment.date", description="The artifact assessment publication date", type="date" ) 2407 public static final String SP_DATE = "date"; 2408 /** 2409 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2410 * <p> 2411 * Description: <b>The artifact assessment publication date</b><br> 2412 * Type: <b>date</b><br> 2413 * Path: <b>ArtifactAssessment.date</b><br> 2414 * </p> 2415 */ 2416 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2417 2418 /** 2419 * Search parameter: <b>identifier</b> 2420 * <p> 2421 * Description: <b>The artifact assessment identifier</b><br> 2422 * Type: <b>token</b><br> 2423 * Path: <b>ArtifactAssessment.identifier</b><br> 2424 * </p> 2425 */ 2426 @SearchParamDefinition(name="identifier", path="ArtifactAssessment.identifier", description="The artifact assessment identifier", type="token" ) 2427 public static final String SP_IDENTIFIER = "identifier"; 2428 /** 2429 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2430 * <p> 2431 * Description: <b>The artifact assessment identifier</b><br> 2432 * Type: <b>token</b><br> 2433 * Path: <b>ArtifactAssessment.identifier</b><br> 2434 * </p> 2435 */ 2436 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2437 2438 2439} 2440