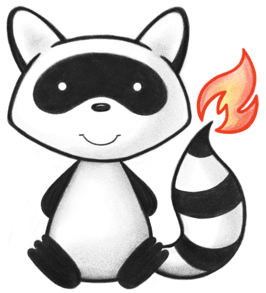
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Attachment Type: For referring to data content defined in other formats. 051 */ 052@DatatypeDef(name="Attachment") 053public class Attachment extends DataType implements ICompositeType { 054 055 /** 056 * Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate. 057 */ 058 @Child(name = "contentType", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Mime type of the content, with charset etc.", formalDefinition="Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate." ) 060 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 061 protected CodeType contentType; 062 063 /** 064 * The human language of the content. The value can be any valid value according to BCP 47. 065 */ 066 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 067 @Description(shortDefinition="Human language of the content (BCP-47)", formalDefinition="The human language of the content. The value can be any valid value according to BCP 47." ) 068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 069 protected CodeType language; 070 071 /** 072 * The actual data of the attachment - a sequence of bytes, base64 encoded. 073 */ 074 @Child(name = "data", type = {Base64BinaryType.class}, order=2, min=0, max=1, modifier=false, summary=false) 075 @Description(shortDefinition="Data inline, base64ed", formalDefinition="The actual data of the attachment - a sequence of bytes, base64 encoded." ) 076 protected Base64BinaryType data; 077 078 /** 079 * A location where the data can be accessed. 080 */ 081 @Child(name = "url", type = {UrlType.class}, order=3, min=0, max=1, modifier=false, summary=true) 082 @Description(shortDefinition="Uri where the data can be found", formalDefinition="A location where the data can be accessed." ) 083 protected UrlType url; 084 085 /** 086 * The number of bytes of data that make up this attachment (before base64 encoding, if that is done). 087 */ 088 @Child(name = "size", type = {Integer64Type.class}, order=4, min=0, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Number of bytes of content (if url provided)", formalDefinition="The number of bytes of data that make up this attachment (before base64 encoding, if that is done)." ) 090 protected Integer64Type size; 091 092 /** 093 * The calculated hash of the data using SHA-1. Represented using base64. 094 */ 095 @Child(name = "hash", type = {Base64BinaryType.class}, order=5, min=0, max=1, modifier=false, summary=true) 096 @Description(shortDefinition="Hash of the data (sha-1, base64ed)", formalDefinition="The calculated hash of the data using SHA-1. Represented using base64." ) 097 protected Base64BinaryType hash; 098 099 /** 100 * A label or set of text to display in place of the data. 101 */ 102 @Child(name = "title", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 103 @Description(shortDefinition="Label to display in place of the data", formalDefinition="A label or set of text to display in place of the data." ) 104 protected StringType title; 105 106 /** 107 * The date that the attachment was first created. 108 */ 109 @Child(name = "creation", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 110 @Description(shortDefinition="Date attachment was first created", formalDefinition="The date that the attachment was first created." ) 111 protected DateTimeType creation; 112 113 /** 114 * Height of the image in pixels (photo/video). 115 */ 116 @Child(name = "height", type = {PositiveIntType.class}, order=8, min=0, max=1, modifier=false, summary=false) 117 @Description(shortDefinition="Height of the image in pixels (photo/video)", formalDefinition="Height of the image in pixels (photo/video)." ) 118 protected PositiveIntType height; 119 120 /** 121 * Width of the image in pixels (photo/video). 122 */ 123 @Child(name = "width", type = {PositiveIntType.class}, order=9, min=0, max=1, modifier=false, summary=false) 124 @Description(shortDefinition="Width of the image in pixels (photo/video)", formalDefinition="Width of the image in pixels (photo/video)." ) 125 protected PositiveIntType width; 126 127 /** 128 * The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required. 129 */ 130 @Child(name = "frames", type = {PositiveIntType.class}, order=10, min=0, max=1, modifier=false, summary=false) 131 @Description(shortDefinition="Number of frames if > 1 (photo)", formalDefinition="The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required." ) 132 protected PositiveIntType frames; 133 134 /** 135 * The duration of the recording in seconds - for audio and video. 136 */ 137 @Child(name = "duration", type = {DecimalType.class}, order=11, min=0, max=1, modifier=false, summary=false) 138 @Description(shortDefinition="Length in seconds (audio / video)", formalDefinition="The duration of the recording in seconds - for audio and video." ) 139 protected DecimalType duration; 140 141 /** 142 * The number of pages when printed. 143 */ 144 @Child(name = "pages", type = {PositiveIntType.class}, order=12, min=0, max=1, modifier=false, summary=false) 145 @Description(shortDefinition="Number of printed pages", formalDefinition="The number of pages when printed." ) 146 protected PositiveIntType pages; 147 148 private static final long serialVersionUID = -1904332061L; 149 150 /** 151 * Constructor 152 */ 153 public Attachment() { 154 super(); 155 } 156 157 /** 158 * @return {@link #contentType} (Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 159 */ 160 public CodeType getContentTypeElement() { 161 if (this.contentType == null) 162 if (Configuration.errorOnAutoCreate()) 163 throw new Error("Attempt to auto-create Attachment.contentType"); 164 else if (Configuration.doAutoCreate()) 165 this.contentType = new CodeType(); // bb 166 return this.contentType; 167 } 168 169 public boolean hasContentTypeElement() { 170 return this.contentType != null && !this.contentType.isEmpty(); 171 } 172 173 public boolean hasContentType() { 174 return this.contentType != null && !this.contentType.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #contentType} (Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 179 */ 180 public Attachment setContentTypeElement(CodeType value) { 181 this.contentType = value; 182 return this; 183 } 184 185 /** 186 * @return Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate. 187 */ 188 public String getContentType() { 189 return this.contentType == null ? null : this.contentType.getValue(); 190 } 191 192 /** 193 * @param value Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate. 194 */ 195 public Attachment setContentType(String value) { 196 if (Utilities.noString(value)) 197 this.contentType = null; 198 else { 199 if (this.contentType == null) 200 this.contentType = new CodeType(); 201 this.contentType.setValue(value); 202 } 203 return this; 204 } 205 206 /** 207 * @return {@link #language} (The human language of the content. The value can be any valid value according to BCP 47.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 208 */ 209 public CodeType getLanguageElement() { 210 if (this.language == null) 211 if (Configuration.errorOnAutoCreate()) 212 throw new Error("Attempt to auto-create Attachment.language"); 213 else if (Configuration.doAutoCreate()) 214 this.language = new CodeType(); // bb 215 return this.language; 216 } 217 218 public boolean hasLanguageElement() { 219 return this.language != null && !this.language.isEmpty(); 220 } 221 222 public boolean hasLanguage() { 223 return this.language != null && !this.language.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #language} (The human language of the content. The value can be any valid value according to BCP 47.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 228 */ 229 public Attachment setLanguageElement(CodeType value) { 230 this.language = value; 231 return this; 232 } 233 234 /** 235 * @return The human language of the content. The value can be any valid value according to BCP 47. 236 */ 237 public String getLanguage() { 238 return this.language == null ? null : this.language.getValue(); 239 } 240 241 /** 242 * @param value The human language of the content. The value can be any valid value according to BCP 47. 243 */ 244 public Attachment setLanguage(String value) { 245 if (Utilities.noString(value)) 246 this.language = null; 247 else { 248 if (this.language == null) 249 this.language = new CodeType(); 250 this.language.setValue(value); 251 } 252 return this; 253 } 254 255 /** 256 * @return {@link #data} (The actual data of the attachment - a sequence of bytes, base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 257 */ 258 public Base64BinaryType getDataElement() { 259 if (this.data == null) 260 if (Configuration.errorOnAutoCreate()) 261 throw new Error("Attempt to auto-create Attachment.data"); 262 else if (Configuration.doAutoCreate()) 263 this.data = new Base64BinaryType(); // bb 264 return this.data; 265 } 266 267 public boolean hasDataElement() { 268 return this.data != null && !this.data.isEmpty(); 269 } 270 271 public boolean hasData() { 272 return this.data != null && !this.data.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #data} (The actual data of the attachment - a sequence of bytes, base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 277 */ 278 public Attachment setDataElement(Base64BinaryType value) { 279 this.data = value; 280 return this; 281 } 282 283 /** 284 * @return The actual data of the attachment - a sequence of bytes, base64 encoded. 285 */ 286 public byte[] getData() { 287 return this.data == null ? null : this.data.getValue(); 288 } 289 290 /** 291 * @param value The actual data of the attachment - a sequence of bytes, base64 encoded. 292 */ 293 public Attachment setData(byte[] value) { 294 if (value == null) 295 this.data = null; 296 else { 297 if (this.data == null) 298 this.data = new Base64BinaryType(); 299 this.data.setValue(value); 300 } 301 return this; 302 } 303 304 /** 305 * @return {@link #url} (A location where the data can be accessed.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 306 */ 307 public UrlType getUrlElement() { 308 if (this.url == null) 309 if (Configuration.errorOnAutoCreate()) 310 throw new Error("Attempt to auto-create Attachment.url"); 311 else if (Configuration.doAutoCreate()) 312 this.url = new UrlType(); // bb 313 return this.url; 314 } 315 316 public boolean hasUrlElement() { 317 return this.url != null && !this.url.isEmpty(); 318 } 319 320 public boolean hasUrl() { 321 return this.url != null && !this.url.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #url} (A location where the data can be accessed.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 326 */ 327 public Attachment setUrlElement(UrlType value) { 328 this.url = value; 329 return this; 330 } 331 332 /** 333 * @return A location where the data can be accessed. 334 */ 335 public String getUrl() { 336 return this.url == null ? null : this.url.getValue(); 337 } 338 339 /** 340 * @param value A location where the data can be accessed. 341 */ 342 public Attachment setUrl(String value) { 343 if (Utilities.noString(value)) 344 this.url = null; 345 else { 346 if (this.url == null) 347 this.url = new UrlType(); 348 this.url.setValue(value); 349 } 350 return this; 351 } 352 353 /** 354 * @return {@link #size} (The number of bytes of data that make up this attachment (before base64 encoding, if that is done).). This is the underlying object with id, value and extensions. The accessor "getSize" gives direct access to the value 355 */ 356 public Integer64Type getSizeElement() { 357 if (this.size == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create Attachment.size"); 360 else if (Configuration.doAutoCreate()) 361 this.size = new Integer64Type(); // bb 362 return this.size; 363 } 364 365 public boolean hasSizeElement() { 366 return this.size != null && !this.size.isEmpty(); 367 } 368 369 public boolean hasSize() { 370 return this.size != null && !this.size.isEmpty(); 371 } 372 373 /** 374 * @param value {@link #size} (The number of bytes of data that make up this attachment (before base64 encoding, if that is done).). This is the underlying object with id, value and extensions. The accessor "getSize" gives direct access to the value 375 */ 376 public Attachment setSizeElement(Integer64Type value) { 377 this.size = value; 378 return this; 379 } 380 381 /** 382 * @return The number of bytes of data that make up this attachment (before base64 encoding, if that is done). 383 */ 384 public long getSize() { 385 return this.size == null || this.size.isEmpty() ? 0 : this.size.getValue(); 386 } 387 388 /** 389 * @param value The number of bytes of data that make up this attachment (before base64 encoding, if that is done). 390 */ 391 public Attachment setSize(long value) { 392 this.size = new Integer64Type(); 393 this.size.setValue(value); 394 return this; 395 } 396 397 /** 398 * @return {@link #hash} (The calculated hash of the data using SHA-1. Represented using base64.). This is the underlying object with id, value and extensions. The accessor "getHash" gives direct access to the value 399 */ 400 public Base64BinaryType getHashElement() { 401 if (this.hash == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create Attachment.hash"); 404 else if (Configuration.doAutoCreate()) 405 this.hash = new Base64BinaryType(); // bb 406 return this.hash; 407 } 408 409 public boolean hasHashElement() { 410 return this.hash != null && !this.hash.isEmpty(); 411 } 412 413 public boolean hasHash() { 414 return this.hash != null && !this.hash.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #hash} (The calculated hash of the data using SHA-1. Represented using base64.). This is the underlying object with id, value and extensions. The accessor "getHash" gives direct access to the value 419 */ 420 public Attachment setHashElement(Base64BinaryType value) { 421 this.hash = value; 422 return this; 423 } 424 425 /** 426 * @return The calculated hash of the data using SHA-1. Represented using base64. 427 */ 428 public byte[] getHash() { 429 return this.hash == null ? null : this.hash.getValue(); 430 } 431 432 /** 433 * @param value The calculated hash of the data using SHA-1. Represented using base64. 434 */ 435 public Attachment setHash(byte[] value) { 436 if (value == null) 437 this.hash = null; 438 else { 439 if (this.hash == null) 440 this.hash = new Base64BinaryType(); 441 this.hash.setValue(value); 442 } 443 return this; 444 } 445 446 /** 447 * @return {@link #title} (A label or set of text to display in place of the data.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 448 */ 449 public StringType getTitleElement() { 450 if (this.title == null) 451 if (Configuration.errorOnAutoCreate()) 452 throw new Error("Attempt to auto-create Attachment.title"); 453 else if (Configuration.doAutoCreate()) 454 this.title = new StringType(); // bb 455 return this.title; 456 } 457 458 public boolean hasTitleElement() { 459 return this.title != null && !this.title.isEmpty(); 460 } 461 462 public boolean hasTitle() { 463 return this.title != null && !this.title.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #title} (A label or set of text to display in place of the data.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 468 */ 469 public Attachment setTitleElement(StringType value) { 470 this.title = value; 471 return this; 472 } 473 474 /** 475 * @return A label or set of text to display in place of the data. 476 */ 477 public String getTitle() { 478 return this.title == null ? null : this.title.getValue(); 479 } 480 481 /** 482 * @param value A label or set of text to display in place of the data. 483 */ 484 public Attachment setTitle(String value) { 485 if (Utilities.noString(value)) 486 this.title = null; 487 else { 488 if (this.title == null) 489 this.title = new StringType(); 490 this.title.setValue(value); 491 } 492 return this; 493 } 494 495 /** 496 * @return {@link #creation} (The date that the attachment was first created.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 497 */ 498 public DateTimeType getCreationElement() { 499 if (this.creation == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create Attachment.creation"); 502 else if (Configuration.doAutoCreate()) 503 this.creation = new DateTimeType(); // bb 504 return this.creation; 505 } 506 507 public boolean hasCreationElement() { 508 return this.creation != null && !this.creation.isEmpty(); 509 } 510 511 public boolean hasCreation() { 512 return this.creation != null && !this.creation.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #creation} (The date that the attachment was first created.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 517 */ 518 public Attachment setCreationElement(DateTimeType value) { 519 this.creation = value; 520 return this; 521 } 522 523 /** 524 * @return The date that the attachment was first created. 525 */ 526 public Date getCreation() { 527 return this.creation == null ? null : this.creation.getValue(); 528 } 529 530 /** 531 * @param value The date that the attachment was first created. 532 */ 533 public Attachment setCreation(Date value) { 534 if (value == null) 535 this.creation = null; 536 else { 537 if (this.creation == null) 538 this.creation = new DateTimeType(); 539 this.creation.setValue(value); 540 } 541 return this; 542 } 543 544 /** 545 * @return {@link #height} (Height of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getHeight" gives direct access to the value 546 */ 547 public PositiveIntType getHeightElement() { 548 if (this.height == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create Attachment.height"); 551 else if (Configuration.doAutoCreate()) 552 this.height = new PositiveIntType(); // bb 553 return this.height; 554 } 555 556 public boolean hasHeightElement() { 557 return this.height != null && !this.height.isEmpty(); 558 } 559 560 public boolean hasHeight() { 561 return this.height != null && !this.height.isEmpty(); 562 } 563 564 /** 565 * @param value {@link #height} (Height of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getHeight" gives direct access to the value 566 */ 567 public Attachment setHeightElement(PositiveIntType value) { 568 this.height = value; 569 return this; 570 } 571 572 /** 573 * @return Height of the image in pixels (photo/video). 574 */ 575 public int getHeight() { 576 return this.height == null || this.height.isEmpty() ? 0 : this.height.getValue(); 577 } 578 579 /** 580 * @param value Height of the image in pixels (photo/video). 581 */ 582 public Attachment setHeight(int value) { 583 if (this.height == null) 584 this.height = new PositiveIntType(); 585 this.height.setValue(value); 586 return this; 587 } 588 589 /** 590 * @return {@link #width} (Width of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getWidth" gives direct access to the value 591 */ 592 public PositiveIntType getWidthElement() { 593 if (this.width == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create Attachment.width"); 596 else if (Configuration.doAutoCreate()) 597 this.width = new PositiveIntType(); // bb 598 return this.width; 599 } 600 601 public boolean hasWidthElement() { 602 return this.width != null && !this.width.isEmpty(); 603 } 604 605 public boolean hasWidth() { 606 return this.width != null && !this.width.isEmpty(); 607 } 608 609 /** 610 * @param value {@link #width} (Width of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getWidth" gives direct access to the value 611 */ 612 public Attachment setWidthElement(PositiveIntType value) { 613 this.width = value; 614 return this; 615 } 616 617 /** 618 * @return Width of the image in pixels (photo/video). 619 */ 620 public int getWidth() { 621 return this.width == null || this.width.isEmpty() ? 0 : this.width.getValue(); 622 } 623 624 /** 625 * @param value Width of the image in pixels (photo/video). 626 */ 627 public Attachment setWidth(int value) { 628 if (this.width == null) 629 this.width = new PositiveIntType(); 630 this.width.setValue(value); 631 return this; 632 } 633 634 /** 635 * @return {@link #frames} (The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.). This is the underlying object with id, value and extensions. The accessor "getFrames" gives direct access to the value 636 */ 637 public PositiveIntType getFramesElement() { 638 if (this.frames == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create Attachment.frames"); 641 else if (Configuration.doAutoCreate()) 642 this.frames = new PositiveIntType(); // bb 643 return this.frames; 644 } 645 646 public boolean hasFramesElement() { 647 return this.frames != null && !this.frames.isEmpty(); 648 } 649 650 public boolean hasFrames() { 651 return this.frames != null && !this.frames.isEmpty(); 652 } 653 654 /** 655 * @param value {@link #frames} (The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.). This is the underlying object with id, value and extensions. The accessor "getFrames" gives direct access to the value 656 */ 657 public Attachment setFramesElement(PositiveIntType value) { 658 this.frames = value; 659 return this; 660 } 661 662 /** 663 * @return The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required. 664 */ 665 public int getFrames() { 666 return this.frames == null || this.frames.isEmpty() ? 0 : this.frames.getValue(); 667 } 668 669 /** 670 * @param value The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required. 671 */ 672 public Attachment setFrames(int value) { 673 if (this.frames == null) 674 this.frames = new PositiveIntType(); 675 this.frames.setValue(value); 676 return this; 677 } 678 679 /** 680 * @return {@link #duration} (The duration of the recording in seconds - for audio and video.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 681 */ 682 public DecimalType getDurationElement() { 683 if (this.duration == null) 684 if (Configuration.errorOnAutoCreate()) 685 throw new Error("Attempt to auto-create Attachment.duration"); 686 else if (Configuration.doAutoCreate()) 687 this.duration = new DecimalType(); // bb 688 return this.duration; 689 } 690 691 public boolean hasDurationElement() { 692 return this.duration != null && !this.duration.isEmpty(); 693 } 694 695 public boolean hasDuration() { 696 return this.duration != null && !this.duration.isEmpty(); 697 } 698 699 /** 700 * @param value {@link #duration} (The duration of the recording in seconds - for audio and video.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 701 */ 702 public Attachment setDurationElement(DecimalType value) { 703 this.duration = value; 704 return this; 705 } 706 707 /** 708 * @return The duration of the recording in seconds - for audio and video. 709 */ 710 public BigDecimal getDuration() { 711 return this.duration == null ? null : this.duration.getValue(); 712 } 713 714 /** 715 * @param value The duration of the recording in seconds - for audio and video. 716 */ 717 public Attachment setDuration(BigDecimal value) { 718 if (value == null) 719 this.duration = null; 720 else { 721 if (this.duration == null) 722 this.duration = new DecimalType(); 723 this.duration.setValue(value); 724 } 725 return this; 726 } 727 728 /** 729 * @param value The duration of the recording in seconds - for audio and video. 730 */ 731 public Attachment setDuration(long value) { 732 this.duration = new DecimalType(); 733 this.duration.setValue(value); 734 return this; 735 } 736 737 /** 738 * @param value The duration of the recording in seconds - for audio and video. 739 */ 740 public Attachment setDuration(double value) { 741 this.duration = new DecimalType(); 742 this.duration.setValue(value); 743 return this; 744 } 745 746 /** 747 * @return {@link #pages} (The number of pages when printed.). This is the underlying object with id, value and extensions. The accessor "getPages" gives direct access to the value 748 */ 749 public PositiveIntType getPagesElement() { 750 if (this.pages == null) 751 if (Configuration.errorOnAutoCreate()) 752 throw new Error("Attempt to auto-create Attachment.pages"); 753 else if (Configuration.doAutoCreate()) 754 this.pages = new PositiveIntType(); // bb 755 return this.pages; 756 } 757 758 public boolean hasPagesElement() { 759 return this.pages != null && !this.pages.isEmpty(); 760 } 761 762 public boolean hasPages() { 763 return this.pages != null && !this.pages.isEmpty(); 764 } 765 766 /** 767 * @param value {@link #pages} (The number of pages when printed.). This is the underlying object with id, value and extensions. The accessor "getPages" gives direct access to the value 768 */ 769 public Attachment setPagesElement(PositiveIntType value) { 770 this.pages = value; 771 return this; 772 } 773 774 /** 775 * @return The number of pages when printed. 776 */ 777 public int getPages() { 778 return this.pages == null || this.pages.isEmpty() ? 0 : this.pages.getValue(); 779 } 780 781 /** 782 * @param value The number of pages when printed. 783 */ 784 public Attachment setPages(int value) { 785 if (this.pages == null) 786 this.pages = new PositiveIntType(); 787 this.pages.setValue(value); 788 return this; 789 } 790 791 protected void listChildren(List<Property> children) { 792 super.listChildren(children); 793 children.add(new Property("contentType", "code", "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 0, 1, contentType)); 794 children.add(new Property("language", "code", "The human language of the content. The value can be any valid value according to BCP 47.", 0, 1, language)); 795 children.add(new Property("data", "base64Binary", "The actual data of the attachment - a sequence of bytes, base64 encoded.", 0, 1, data)); 796 children.add(new Property("url", "url", "A location where the data can be accessed.", 0, 1, url)); 797 children.add(new Property("size", "integer64", "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).", 0, 1, size)); 798 children.add(new Property("hash", "base64Binary", "The calculated hash of the data using SHA-1. Represented using base64.", 0, 1, hash)); 799 children.add(new Property("title", "string", "A label or set of text to display in place of the data.", 0, 1, title)); 800 children.add(new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 1, creation)); 801 children.add(new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, height)); 802 children.add(new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, width)); 803 children.add(new Property("frames", "positiveInt", "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 0, 1, frames)); 804 children.add(new Property("duration", "decimal", "The duration of the recording in seconds - for audio and video.", 0, 1, duration)); 805 children.add(new Property("pages", "positiveInt", "The number of pages when printed.", 0, 1, pages)); 806 } 807 808 @Override 809 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 810 switch (_hash) { 811 case -389131437: /*contentType*/ return new Property("contentType", "code", "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 0, 1, contentType); 812 case -1613589672: /*language*/ return new Property("language", "code", "The human language of the content. The value can be any valid value according to BCP 47.", 0, 1, language); 813 case 3076010: /*data*/ return new Property("data", "base64Binary", "The actual data of the attachment - a sequence of bytes, base64 encoded.", 0, 1, data); 814 case 116079: /*url*/ return new Property("url", "url", "A location where the data can be accessed.", 0, 1, url); 815 case 3530753: /*size*/ return new Property("size", "integer64", "The number of bytes of data that make up this attachment (before base64 encoding, if that is done).", 0, 1, size); 816 case 3195150: /*hash*/ return new Property("hash", "base64Binary", "The calculated hash of the data using SHA-1. Represented using base64.", 0, 1, hash); 817 case 110371416: /*title*/ return new Property("title", "string", "A label or set of text to display in place of the data.", 0, 1, title); 818 case 1820421855: /*creation*/ return new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 1, creation); 819 case -1221029593: /*height*/ return new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, height); 820 case 113126854: /*width*/ return new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, width); 821 case -1266514778: /*frames*/ return new Property("frames", "positiveInt", "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 0, 1, frames); 822 case -1992012396: /*duration*/ return new Property("duration", "decimal", "The duration of the recording in seconds - for audio and video.", 0, 1, duration); 823 case 106426308: /*pages*/ return new Property("pages", "positiveInt", "The number of pages when printed.", 0, 1, pages); 824 default: return super.getNamedProperty(_hash, _name, _checkValid); 825 } 826 827 } 828 829 @Override 830 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 831 switch (hash) { 832 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 833 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 834 case 3076010: /*data*/ return this.data == null ? new Base[0] : new Base[] {this.data}; // Base64BinaryType 835 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 836 case 3530753: /*size*/ return this.size == null ? new Base[0] : new Base[] {this.size}; // Integer64Type 837 case 3195150: /*hash*/ return this.hash == null ? new Base[0] : new Base[] {this.hash}; // Base64BinaryType 838 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 839 case 1820421855: /*creation*/ return this.creation == null ? new Base[0] : new Base[] {this.creation}; // DateTimeType 840 case -1221029593: /*height*/ return this.height == null ? new Base[0] : new Base[] {this.height}; // PositiveIntType 841 case 113126854: /*width*/ return this.width == null ? new Base[0] : new Base[] {this.width}; // PositiveIntType 842 case -1266514778: /*frames*/ return this.frames == null ? new Base[0] : new Base[] {this.frames}; // PositiveIntType 843 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // DecimalType 844 case 106426308: /*pages*/ return this.pages == null ? new Base[0] : new Base[] {this.pages}; // PositiveIntType 845 default: return super.getProperty(hash, name, checkValid); 846 } 847 848 } 849 850 @Override 851 public Base setProperty(int hash, String name, Base value) throws FHIRException { 852 switch (hash) { 853 case -389131437: // contentType 854 this.contentType = TypeConvertor.castToCode(value); // CodeType 855 return value; 856 case -1613589672: // language 857 this.language = TypeConvertor.castToCode(value); // CodeType 858 return value; 859 case 3076010: // data 860 this.data = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 861 return value; 862 case 116079: // url 863 this.url = TypeConvertor.castToUrl(value); // UrlType 864 return value; 865 case 3530753: // size 866 this.size = TypeConvertor.castToInteger64(value); // Integer64Type 867 return value; 868 case 3195150: // hash 869 this.hash = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 870 return value; 871 case 110371416: // title 872 this.title = TypeConvertor.castToString(value); // StringType 873 return value; 874 case 1820421855: // creation 875 this.creation = TypeConvertor.castToDateTime(value); // DateTimeType 876 return value; 877 case -1221029593: // height 878 this.height = TypeConvertor.castToPositiveInt(value); // PositiveIntType 879 return value; 880 case 113126854: // width 881 this.width = TypeConvertor.castToPositiveInt(value); // PositiveIntType 882 return value; 883 case -1266514778: // frames 884 this.frames = TypeConvertor.castToPositiveInt(value); // PositiveIntType 885 return value; 886 case -1992012396: // duration 887 this.duration = TypeConvertor.castToDecimal(value); // DecimalType 888 return value; 889 case 106426308: // pages 890 this.pages = TypeConvertor.castToPositiveInt(value); // PositiveIntType 891 return value; 892 default: return super.setProperty(hash, name, value); 893 } 894 895 } 896 897 @Override 898 public Base setProperty(String name, Base value) throws FHIRException { 899 if (name.equals("contentType")) { 900 this.contentType = TypeConvertor.castToCode(value); // CodeType 901 } else if (name.equals("language")) { 902 this.language = TypeConvertor.castToCode(value); // CodeType 903 } else if (name.equals("data")) { 904 this.data = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 905 } else if (name.equals("url")) { 906 this.url = TypeConvertor.castToUrl(value); // UrlType 907 } else if (name.equals("size")) { 908 this.size = TypeConvertor.castToInteger64(value); // Integer64Type 909 } else if (name.equals("hash")) { 910 this.hash = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 911 } else if (name.equals("title")) { 912 this.title = TypeConvertor.castToString(value); // StringType 913 } else if (name.equals("creation")) { 914 this.creation = TypeConvertor.castToDateTime(value); // DateTimeType 915 } else if (name.equals("height")) { 916 this.height = TypeConvertor.castToPositiveInt(value); // PositiveIntType 917 } else if (name.equals("width")) { 918 this.width = TypeConvertor.castToPositiveInt(value); // PositiveIntType 919 } else if (name.equals("frames")) { 920 this.frames = TypeConvertor.castToPositiveInt(value); // PositiveIntType 921 } else if (name.equals("duration")) { 922 this.duration = TypeConvertor.castToDecimal(value); // DecimalType 923 } else if (name.equals("pages")) { 924 this.pages = TypeConvertor.castToPositiveInt(value); // PositiveIntType 925 } else 926 return super.setProperty(name, value); 927 return value; 928 } 929 930 @Override 931 public void removeChild(String name, Base value) throws FHIRException { 932 if (name.equals("contentType")) { 933 this.contentType = null; 934 } else if (name.equals("language")) { 935 this.language = null; 936 } else if (name.equals("data")) { 937 this.data = null; 938 } else if (name.equals("url")) { 939 this.url = null; 940 } else if (name.equals("size")) { 941 this.size = null; 942 } else if (name.equals("hash")) { 943 this.hash = null; 944 } else if (name.equals("title")) { 945 this.title = null; 946 } else if (name.equals("creation")) { 947 this.creation = null; 948 } else if (name.equals("height")) { 949 this.height = null; 950 } else if (name.equals("width")) { 951 this.width = null; 952 } else if (name.equals("frames")) { 953 this.frames = null; 954 } else if (name.equals("duration")) { 955 this.duration = null; 956 } else if (name.equals("pages")) { 957 this.pages = null; 958 } else 959 super.removeChild(name, value); 960 961 } 962 963 @Override 964 public Base makeProperty(int hash, String name) throws FHIRException { 965 switch (hash) { 966 case -389131437: return getContentTypeElement(); 967 case -1613589672: return getLanguageElement(); 968 case 3076010: return getDataElement(); 969 case 116079: return getUrlElement(); 970 case 3530753: return getSizeElement(); 971 case 3195150: return getHashElement(); 972 case 110371416: return getTitleElement(); 973 case 1820421855: return getCreationElement(); 974 case -1221029593: return getHeightElement(); 975 case 113126854: return getWidthElement(); 976 case -1266514778: return getFramesElement(); 977 case -1992012396: return getDurationElement(); 978 case 106426308: return getPagesElement(); 979 default: return super.makeProperty(hash, name); 980 } 981 982 } 983 984 @Override 985 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 986 switch (hash) { 987 case -389131437: /*contentType*/ return new String[] {"code"}; 988 case -1613589672: /*language*/ return new String[] {"code"}; 989 case 3076010: /*data*/ return new String[] {"base64Binary"}; 990 case 116079: /*url*/ return new String[] {"url"}; 991 case 3530753: /*size*/ return new String[] {"integer64"}; 992 case 3195150: /*hash*/ return new String[] {"base64Binary"}; 993 case 110371416: /*title*/ return new String[] {"string"}; 994 case 1820421855: /*creation*/ return new String[] {"dateTime"}; 995 case -1221029593: /*height*/ return new String[] {"positiveInt"}; 996 case 113126854: /*width*/ return new String[] {"positiveInt"}; 997 case -1266514778: /*frames*/ return new String[] {"positiveInt"}; 998 case -1992012396: /*duration*/ return new String[] {"decimal"}; 999 case 106426308: /*pages*/ return new String[] {"positiveInt"}; 1000 default: return super.getTypesForProperty(hash, name); 1001 } 1002 1003 } 1004 1005 @Override 1006 public Base addChild(String name) throws FHIRException { 1007 if (name.equals("contentType")) { 1008 throw new FHIRException("Cannot call addChild on a singleton property Attachment.contentType"); 1009 } 1010 else if (name.equals("language")) { 1011 throw new FHIRException("Cannot call addChild on a singleton property Attachment.language"); 1012 } 1013 else if (name.equals("data")) { 1014 throw new FHIRException("Cannot call addChild on a singleton property Attachment.data"); 1015 } 1016 else if (name.equals("url")) { 1017 throw new FHIRException("Cannot call addChild on a singleton property Attachment.url"); 1018 } 1019 else if (name.equals("size")) { 1020 throw new FHIRException("Cannot call addChild on a singleton property Attachment.size"); 1021 } 1022 else if (name.equals("hash")) { 1023 throw new FHIRException("Cannot call addChild on a singleton property Attachment.hash"); 1024 } 1025 else if (name.equals("title")) { 1026 throw new FHIRException("Cannot call addChild on a singleton property Attachment.title"); 1027 } 1028 else if (name.equals("creation")) { 1029 throw new FHIRException("Cannot call addChild on a singleton property Attachment.creation"); 1030 } 1031 else if (name.equals("height")) { 1032 throw new FHIRException("Cannot call addChild on a singleton property Attachment.height"); 1033 } 1034 else if (name.equals("width")) { 1035 throw new FHIRException("Cannot call addChild on a singleton property Attachment.width"); 1036 } 1037 else if (name.equals("frames")) { 1038 throw new FHIRException("Cannot call addChild on a singleton property Attachment.frames"); 1039 } 1040 else if (name.equals("duration")) { 1041 throw new FHIRException("Cannot call addChild on a singleton property Attachment.duration"); 1042 } 1043 else if (name.equals("pages")) { 1044 throw new FHIRException("Cannot call addChild on a singleton property Attachment.pages"); 1045 } 1046 else 1047 return super.addChild(name); 1048 } 1049 1050 public String fhirType() { 1051 return "Attachment"; 1052 1053 } 1054 1055 public Attachment copy() { 1056 Attachment dst = new Attachment(); 1057 copyValues(dst); 1058 return dst; 1059 } 1060 1061 public void copyValues(Attachment dst) { 1062 super.copyValues(dst); 1063 dst.contentType = contentType == null ? null : contentType.copy(); 1064 dst.language = language == null ? null : language.copy(); 1065 dst.data = data == null ? null : data.copy(); 1066 dst.url = url == null ? null : url.copy(); 1067 dst.size = size == null ? null : size.copy(); 1068 dst.hash = hash == null ? null : hash.copy(); 1069 dst.title = title == null ? null : title.copy(); 1070 dst.creation = creation == null ? null : creation.copy(); 1071 dst.height = height == null ? null : height.copy(); 1072 dst.width = width == null ? null : width.copy(); 1073 dst.frames = frames == null ? null : frames.copy(); 1074 dst.duration = duration == null ? null : duration.copy(); 1075 dst.pages = pages == null ? null : pages.copy(); 1076 } 1077 1078 protected Attachment typedCopy() { 1079 return copy(); 1080 } 1081 1082 @Override 1083 public boolean equalsDeep(Base other_) { 1084 if (!super.equalsDeep(other_)) 1085 return false; 1086 if (!(other_ instanceof Attachment)) 1087 return false; 1088 Attachment o = (Attachment) other_; 1089 return compareDeep(contentType, o.contentType, true) && compareDeep(language, o.language, true) 1090 && compareDeep(data, o.data, true) && compareDeep(url, o.url, true) && compareDeep(size, o.size, true) 1091 && compareDeep(hash, o.hash, true) && compareDeep(title, o.title, true) && compareDeep(creation, o.creation, true) 1092 && compareDeep(height, o.height, true) && compareDeep(width, o.width, true) && compareDeep(frames, o.frames, true) 1093 && compareDeep(duration, o.duration, true) && compareDeep(pages, o.pages, true); 1094 } 1095 1096 @Override 1097 public boolean equalsShallow(Base other_) { 1098 if (!super.equalsShallow(other_)) 1099 return false; 1100 if (!(other_ instanceof Attachment)) 1101 return false; 1102 Attachment o = (Attachment) other_; 1103 return compareValues(contentType, o.contentType, true) && compareValues(language, o.language, true) 1104 && compareValues(data, o.data, true) && compareValues(url, o.url, true) && compareValues(size, o.size, true) 1105 && compareValues(hash, o.hash, true) && compareValues(title, o.title, true) && compareValues(creation, o.creation, true) 1106 && compareValues(height, o.height, true) && compareValues(width, o.width, true) && compareValues(frames, o.frames, true) 1107 && compareValues(duration, o.duration, true) && compareValues(pages, o.pages, true); 1108 } 1109 1110 public boolean isEmpty() { 1111 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(contentType, language, data 1112 , url, size, hash, title, creation, height, width, frames, duration, pages 1113 ); 1114 } 1115 1116 1117} 1118