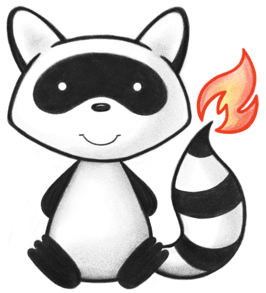
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of an event relevant for purposes such as operations, privacy, security, maintenance, and performance analysis. 052 */ 053@ResourceDef(name="AuditEvent", profile="http://hl7.org/fhir/StructureDefinition/AuditEvent") 054public class AuditEvent extends DomainResource { 055 056 public enum AuditEventAction { 057 /** 058 * Create a new database object, such as placing an order. 059 */ 060 C, 061 /** 062 * Read data, such as to print or display to a doctor. 063 */ 064 R, 065 /** 066 * Update data, such as revise patient information. 067 */ 068 U, 069 /** 070 * Delete items, such as a doctor master file record. 071 */ 072 D, 073 /** 074 * Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation. 075 */ 076 E, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static AuditEventAction fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("C".equals(codeString)) 085 return C; 086 if ("R".equals(codeString)) 087 return R; 088 if ("U".equals(codeString)) 089 return U; 090 if ("D".equals(codeString)) 091 return D; 092 if ("E".equals(codeString)) 093 return E; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown AuditEventAction code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case C: return "C"; 102 case R: return "R"; 103 case U: return "U"; 104 case D: return "D"; 105 case E: return "E"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case C: return "http://hl7.org/fhir/audit-event-action"; 113 case R: return "http://hl7.org/fhir/audit-event-action"; 114 case U: return "http://hl7.org/fhir/audit-event-action"; 115 case D: return "http://hl7.org/fhir/audit-event-action"; 116 case E: return "http://hl7.org/fhir/audit-event-action"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case C: return "Create a new database object, such as placing an order."; 124 case R: return "Read data, such as to print or display to a doctor."; 125 case U: return "Update data, such as revise patient information."; 126 case D: return "Delete items, such as a doctor master file record."; 127 case E: return "Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case C: return "Create"; 135 case R: return "Read"; 136 case U: return "Update"; 137 case D: return "Delete"; 138 case E: return "Execute"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class AuditEventActionEnumFactory implements EnumFactory<AuditEventAction> { 146 public AuditEventAction fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("C".equals(codeString)) 151 return AuditEventAction.C; 152 if ("R".equals(codeString)) 153 return AuditEventAction.R; 154 if ("U".equals(codeString)) 155 return AuditEventAction.U; 156 if ("D".equals(codeString)) 157 return AuditEventAction.D; 158 if ("E".equals(codeString)) 159 return AuditEventAction.E; 160 throw new IllegalArgumentException("Unknown AuditEventAction code '"+codeString+"'"); 161 } 162 public Enumeration<AuditEventAction> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<AuditEventAction>(this, AuditEventAction.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<AuditEventAction>(this, AuditEventAction.NULL, code); 170 if ("C".equals(codeString)) 171 return new Enumeration<AuditEventAction>(this, AuditEventAction.C, code); 172 if ("R".equals(codeString)) 173 return new Enumeration<AuditEventAction>(this, AuditEventAction.R, code); 174 if ("U".equals(codeString)) 175 return new Enumeration<AuditEventAction>(this, AuditEventAction.U, code); 176 if ("D".equals(codeString)) 177 return new Enumeration<AuditEventAction>(this, AuditEventAction.D, code); 178 if ("E".equals(codeString)) 179 return new Enumeration<AuditEventAction>(this, AuditEventAction.E, code); 180 throw new FHIRException("Unknown AuditEventAction code '"+codeString+"'"); 181 } 182 public String toCode(AuditEventAction code) { 183 if (code == AuditEventAction.NULL) 184 return null; 185 if (code == AuditEventAction.C) 186 return "C"; 187 if (code == AuditEventAction.R) 188 return "R"; 189 if (code == AuditEventAction.U) 190 return "U"; 191 if (code == AuditEventAction.D) 192 return "D"; 193 if (code == AuditEventAction.E) 194 return "E"; 195 return "?"; 196 } 197 public String toSystem(AuditEventAction code) { 198 return code.getSystem(); 199 } 200 } 201 202 public enum AuditEventSeverity { 203 /** 204 * System is unusable. e.g., This level should only be reported by infrastructure and should not be used by applications. 205 */ 206 EMERGENCY, 207 /** 208 * Notification should be sent to trigger action be taken. e.g., Loss of the primary network connection needing attention. 209 */ 210 ALERT, 211 /** 212 * Critical conditions. e.g., A failure in the system's primary application that will reset automatically. 213 */ 214 CRITICAL, 215 /** 216 * Error conditions. e.g., An application has exceeded its file storage limit and attempts to write are failing. 217 */ 218 ERROR, 219 /** 220 * Warning conditions. May indicate that an error will occur if action is not taken. e.g., A non-root file system has only 2GB remaining. 221 */ 222 WARNING, 223 /** 224 * Notice messages. Normal but significant condition. Events that are unusual, but not error conditions. 225 */ 226 NOTICE, 227 /** 228 * Normal operational messages that require no action. e.g., An application has started, paused, or ended successfully. 229 */ 230 INFORMATIONAL, 231 /** 232 * Debug-level messages. Information useful to developers for debugging the application. 233 */ 234 DEBUG, 235 /** 236 * added to help the parsers with the generic types 237 */ 238 NULL; 239 public static AuditEventSeverity fromCode(String codeString) throws FHIRException { 240 if (codeString == null || "".equals(codeString)) 241 return null; 242 if ("emergency".equals(codeString)) 243 return EMERGENCY; 244 if ("alert".equals(codeString)) 245 return ALERT; 246 if ("critical".equals(codeString)) 247 return CRITICAL; 248 if ("error".equals(codeString)) 249 return ERROR; 250 if ("warning".equals(codeString)) 251 return WARNING; 252 if ("notice".equals(codeString)) 253 return NOTICE; 254 if ("informational".equals(codeString)) 255 return INFORMATIONAL; 256 if ("debug".equals(codeString)) 257 return DEBUG; 258 if (Configuration.isAcceptInvalidEnums()) 259 return null; 260 else 261 throw new FHIRException("Unknown AuditEventSeverity code '"+codeString+"'"); 262 } 263 public String toCode() { 264 switch (this) { 265 case EMERGENCY: return "emergency"; 266 case ALERT: return "alert"; 267 case CRITICAL: return "critical"; 268 case ERROR: return "error"; 269 case WARNING: return "warning"; 270 case NOTICE: return "notice"; 271 case INFORMATIONAL: return "informational"; 272 case DEBUG: return "debug"; 273 case NULL: return null; 274 default: return "?"; 275 } 276 } 277 public String getSystem() { 278 switch (this) { 279 case EMERGENCY: return "http://hl7.org/fhir/audit-event-severity"; 280 case ALERT: return "http://hl7.org/fhir/audit-event-severity"; 281 case CRITICAL: return "http://hl7.org/fhir/audit-event-severity"; 282 case ERROR: return "http://hl7.org/fhir/audit-event-severity"; 283 case WARNING: return "http://hl7.org/fhir/audit-event-severity"; 284 case NOTICE: return "http://hl7.org/fhir/audit-event-severity"; 285 case INFORMATIONAL: return "http://hl7.org/fhir/audit-event-severity"; 286 case DEBUG: return "http://hl7.org/fhir/audit-event-severity"; 287 case NULL: return null; 288 default: return "?"; 289 } 290 } 291 public String getDefinition() { 292 switch (this) { 293 case EMERGENCY: return "System is unusable. e.g., This level should only be reported by infrastructure and should not be used by applications."; 294 case ALERT: return "Notification should be sent to trigger action be taken. e.g., Loss of the primary network connection needing attention."; 295 case CRITICAL: return "Critical conditions. e.g., A failure in the system's primary application that will reset automatically."; 296 case ERROR: return "Error conditions. e.g., An application has exceeded its file storage limit and attempts to write are failing. "; 297 case WARNING: return "Warning conditions. May indicate that an error will occur if action is not taken. e.g., A non-root file system has only 2GB remaining."; 298 case NOTICE: return "Notice messages. Normal but significant condition. Events that are unusual, but not error conditions."; 299 case INFORMATIONAL: return "Normal operational messages that require no action. e.g., An application has started, paused, or ended successfully."; 300 case DEBUG: return "Debug-level messages. Information useful to developers for debugging the application."; 301 case NULL: return null; 302 default: return "?"; 303 } 304 } 305 public String getDisplay() { 306 switch (this) { 307 case EMERGENCY: return "Emergency"; 308 case ALERT: return "Alert"; 309 case CRITICAL: return "Critical"; 310 case ERROR: return "Error"; 311 case WARNING: return "Warning"; 312 case NOTICE: return "Notice"; 313 case INFORMATIONAL: return "Informational"; 314 case DEBUG: return "Debug"; 315 case NULL: return null; 316 default: return "?"; 317 } 318 } 319 } 320 321 public static class AuditEventSeverityEnumFactory implements EnumFactory<AuditEventSeverity> { 322 public AuditEventSeverity fromCode(String codeString) throws IllegalArgumentException { 323 if (codeString == null || "".equals(codeString)) 324 if (codeString == null || "".equals(codeString)) 325 return null; 326 if ("emergency".equals(codeString)) 327 return AuditEventSeverity.EMERGENCY; 328 if ("alert".equals(codeString)) 329 return AuditEventSeverity.ALERT; 330 if ("critical".equals(codeString)) 331 return AuditEventSeverity.CRITICAL; 332 if ("error".equals(codeString)) 333 return AuditEventSeverity.ERROR; 334 if ("warning".equals(codeString)) 335 return AuditEventSeverity.WARNING; 336 if ("notice".equals(codeString)) 337 return AuditEventSeverity.NOTICE; 338 if ("informational".equals(codeString)) 339 return AuditEventSeverity.INFORMATIONAL; 340 if ("debug".equals(codeString)) 341 return AuditEventSeverity.DEBUG; 342 throw new IllegalArgumentException("Unknown AuditEventSeverity code '"+codeString+"'"); 343 } 344 public Enumeration<AuditEventSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 345 if (code == null) 346 return null; 347 if (code.isEmpty()) 348 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.NULL, code); 349 String codeString = ((PrimitiveType) code).asStringValue(); 350 if (codeString == null || "".equals(codeString)) 351 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.NULL, code); 352 if ("emergency".equals(codeString)) 353 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.EMERGENCY, code); 354 if ("alert".equals(codeString)) 355 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.ALERT, code); 356 if ("critical".equals(codeString)) 357 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.CRITICAL, code); 358 if ("error".equals(codeString)) 359 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.ERROR, code); 360 if ("warning".equals(codeString)) 361 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.WARNING, code); 362 if ("notice".equals(codeString)) 363 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.NOTICE, code); 364 if ("informational".equals(codeString)) 365 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.INFORMATIONAL, code); 366 if ("debug".equals(codeString)) 367 return new Enumeration<AuditEventSeverity>(this, AuditEventSeverity.DEBUG, code); 368 throw new FHIRException("Unknown AuditEventSeverity code '"+codeString+"'"); 369 } 370 public String toCode(AuditEventSeverity code) { 371 if (code == AuditEventSeverity.NULL) 372 return null; 373 if (code == AuditEventSeverity.EMERGENCY) 374 return "emergency"; 375 if (code == AuditEventSeverity.ALERT) 376 return "alert"; 377 if (code == AuditEventSeverity.CRITICAL) 378 return "critical"; 379 if (code == AuditEventSeverity.ERROR) 380 return "error"; 381 if (code == AuditEventSeverity.WARNING) 382 return "warning"; 383 if (code == AuditEventSeverity.NOTICE) 384 return "notice"; 385 if (code == AuditEventSeverity.INFORMATIONAL) 386 return "informational"; 387 if (code == AuditEventSeverity.DEBUG) 388 return "debug"; 389 return "?"; 390 } 391 public String toSystem(AuditEventSeverity code) { 392 return code.getSystem(); 393 } 394 } 395 396 @Block() 397 public static class AuditEventOutcomeComponent extends BackboneElement implements IBaseBackboneElement { 398 /** 399 * Indicates whether the event succeeded or failed. 400 */ 401 @Child(name = "code", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=true) 402 @Description(shortDefinition="Whether the event succeeded or failed", formalDefinition="Indicates whether the event succeeded or failed." ) 403 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-outcome") 404 protected Coding code; 405 406 /** 407 * Additional details about the error. This may be a text description of the error or a system code that identifies the error. 408 */ 409 @Child(name = "detail", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 410 @Description(shortDefinition="Additional outcome detail", formalDefinition="Additional details about the error. This may be a text description of the error or a system code that identifies the error." ) 411 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-outcome-detail") 412 protected List<CodeableConcept> detail; 413 414 private static final long serialVersionUID = -1108329559L; 415 416 /** 417 * Constructor 418 */ 419 public AuditEventOutcomeComponent() { 420 super(); 421 } 422 423 /** 424 * Constructor 425 */ 426 public AuditEventOutcomeComponent(Coding code) { 427 super(); 428 this.setCode(code); 429 } 430 431 /** 432 * @return {@link #code} (Indicates whether the event succeeded or failed.) 433 */ 434 public Coding getCode() { 435 if (this.code == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create AuditEventOutcomeComponent.code"); 438 else if (Configuration.doAutoCreate()) 439 this.code = new Coding(); // cc 440 return this.code; 441 } 442 443 public boolean hasCode() { 444 return this.code != null && !this.code.isEmpty(); 445 } 446 447 /** 448 * @param value {@link #code} (Indicates whether the event succeeded or failed.) 449 */ 450 public AuditEventOutcomeComponent setCode(Coding value) { 451 this.code = value; 452 return this; 453 } 454 455 /** 456 * @return {@link #detail} (Additional details about the error. This may be a text description of the error or a system code that identifies the error.) 457 */ 458 public List<CodeableConcept> getDetail() { 459 if (this.detail == null) 460 this.detail = new ArrayList<CodeableConcept>(); 461 return this.detail; 462 } 463 464 /** 465 * @return Returns a reference to <code>this</code> for easy method chaining 466 */ 467 public AuditEventOutcomeComponent setDetail(List<CodeableConcept> theDetail) { 468 this.detail = theDetail; 469 return this; 470 } 471 472 public boolean hasDetail() { 473 if (this.detail == null) 474 return false; 475 for (CodeableConcept item : this.detail) 476 if (!item.isEmpty()) 477 return true; 478 return false; 479 } 480 481 public CodeableConcept addDetail() { //3 482 CodeableConcept t = new CodeableConcept(); 483 if (this.detail == null) 484 this.detail = new ArrayList<CodeableConcept>(); 485 this.detail.add(t); 486 return t; 487 } 488 489 public AuditEventOutcomeComponent addDetail(CodeableConcept t) { //3 490 if (t == null) 491 return this; 492 if (this.detail == null) 493 this.detail = new ArrayList<CodeableConcept>(); 494 this.detail.add(t); 495 return this; 496 } 497 498 /** 499 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 500 */ 501 public CodeableConcept getDetailFirstRep() { 502 if (getDetail().isEmpty()) { 503 addDetail(); 504 } 505 return getDetail().get(0); 506 } 507 508 protected void listChildren(List<Property> children) { 509 super.listChildren(children); 510 children.add(new Property("code", "Coding", "Indicates whether the event succeeded or failed.", 0, 1, code)); 511 children.add(new Property("detail", "CodeableConcept", "Additional details about the error. This may be a text description of the error or a system code that identifies the error.", 0, java.lang.Integer.MAX_VALUE, detail)); 512 } 513 514 @Override 515 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 516 switch (_hash) { 517 case 3059181: /*code*/ return new Property("code", "Coding", "Indicates whether the event succeeded or failed.", 0, 1, code); 518 case -1335224239: /*detail*/ return new Property("detail", "CodeableConcept", "Additional details about the error. This may be a text description of the error or a system code that identifies the error.", 0, java.lang.Integer.MAX_VALUE, detail); 519 default: return super.getNamedProperty(_hash, _name, _checkValid); 520 } 521 522 } 523 524 @Override 525 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 526 switch (hash) { 527 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Coding 528 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // CodeableConcept 529 default: return super.getProperty(hash, name, checkValid); 530 } 531 532 } 533 534 @Override 535 public Base setProperty(int hash, String name, Base value) throws FHIRException { 536 switch (hash) { 537 case 3059181: // code 538 this.code = TypeConvertor.castToCoding(value); // Coding 539 return value; 540 case -1335224239: // detail 541 this.getDetail().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 542 return value; 543 default: return super.setProperty(hash, name, value); 544 } 545 546 } 547 548 @Override 549 public Base setProperty(String name, Base value) throws FHIRException { 550 if (name.equals("code")) { 551 this.code = TypeConvertor.castToCoding(value); // Coding 552 } else if (name.equals("detail")) { 553 this.getDetail().add(TypeConvertor.castToCodeableConcept(value)); 554 } else 555 return super.setProperty(name, value); 556 return value; 557 } 558 559 @Override 560 public void removeChild(String name, Base value) throws FHIRException { 561 if (name.equals("code")) { 562 this.code = null; 563 } else if (name.equals("detail")) { 564 this.getDetail().remove(value); 565 } else 566 super.removeChild(name, value); 567 568 } 569 570 @Override 571 public Base makeProperty(int hash, String name) throws FHIRException { 572 switch (hash) { 573 case 3059181: return getCode(); 574 case -1335224239: return addDetail(); 575 default: return super.makeProperty(hash, name); 576 } 577 578 } 579 580 @Override 581 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 582 switch (hash) { 583 case 3059181: /*code*/ return new String[] {"Coding"}; 584 case -1335224239: /*detail*/ return new String[] {"CodeableConcept"}; 585 default: return super.getTypesForProperty(hash, name); 586 } 587 588 } 589 590 @Override 591 public Base addChild(String name) throws FHIRException { 592 if (name.equals("code")) { 593 this.code = new Coding(); 594 return this.code; 595 } 596 else if (name.equals("detail")) { 597 return addDetail(); 598 } 599 else 600 return super.addChild(name); 601 } 602 603 public AuditEventOutcomeComponent copy() { 604 AuditEventOutcomeComponent dst = new AuditEventOutcomeComponent(); 605 copyValues(dst); 606 return dst; 607 } 608 609 public void copyValues(AuditEventOutcomeComponent dst) { 610 super.copyValues(dst); 611 dst.code = code == null ? null : code.copy(); 612 if (detail != null) { 613 dst.detail = new ArrayList<CodeableConcept>(); 614 for (CodeableConcept i : detail) 615 dst.detail.add(i.copy()); 616 }; 617 } 618 619 @Override 620 public boolean equalsDeep(Base other_) { 621 if (!super.equalsDeep(other_)) 622 return false; 623 if (!(other_ instanceof AuditEventOutcomeComponent)) 624 return false; 625 AuditEventOutcomeComponent o = (AuditEventOutcomeComponent) other_; 626 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 627 } 628 629 @Override 630 public boolean equalsShallow(Base other_) { 631 if (!super.equalsShallow(other_)) 632 return false; 633 if (!(other_ instanceof AuditEventOutcomeComponent)) 634 return false; 635 AuditEventOutcomeComponent o = (AuditEventOutcomeComponent) other_; 636 return true; 637 } 638 639 public boolean isEmpty() { 640 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 641 } 642 643 public String fhirType() { 644 return "AuditEvent.outcome"; 645 646 } 647 648 } 649 650 @Block() 651 public static class AuditEventAgentComponent extends BackboneElement implements IBaseBackboneElement { 652 /** 653 * The Functional Role of the user when performing the event. 654 */ 655 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 656 @Description(shortDefinition="How agent participated", formalDefinition="The Functional Role of the user when performing the event." ) 657 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 658 protected CodeableConcept type; 659 660 /** 661 * The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity. 662 */ 663 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 664 @Description(shortDefinition="Agent role in the event", formalDefinition="The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity." ) 665 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 666 protected List<CodeableConcept> role; 667 668 /** 669 * Reference to who this agent is that was involved in the event. 670 */ 671 @Child(name = "who", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class}, order=3, min=1, max=1, modifier=false, summary=true) 672 @Description(shortDefinition="Identifier of who", formalDefinition="Reference to who this agent is that was involved in the event." ) 673 protected Reference who; 674 675 /** 676 * Indicator that the user is or is not the requestor, or initiator, for the event being audited. 677 */ 678 @Child(name = "requestor", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 679 @Description(shortDefinition="Whether user is initiator", formalDefinition="Indicator that the user is or is not the requestor, or initiator, for the event being audited." ) 680 protected BooleanType requestor; 681 682 /** 683 * Where the agent location is known, the agent location when the event occurred. 684 */ 685 @Child(name = "location", type = {Location.class}, order=5, min=0, max=1, modifier=false, summary=false) 686 @Description(shortDefinition="The agent location when the event occurred", formalDefinition="Where the agent location is known, the agent location when the event occurred." ) 687 protected Reference location; 688 689 /** 690 * Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used. 691 */ 692 @Child(name = "policy", type = {UriType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 693 @Description(shortDefinition="Policy that authorized the agent participation in the event", formalDefinition="Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used." ) 694 protected List<UriType> policy; 695 696 /** 697 * When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details. 698 */ 699 @Child(name = "network", type = {Endpoint.class, UriType.class, StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 700 @Description(shortDefinition="This agent network location for the activity", formalDefinition="When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details." ) 701 protected DataType network; 702 703 /** 704 * The authorization (e.g., PurposeOfUse) that was used during the event being recorded. 705 */ 706 @Child(name = "authorization", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 707 @Description(shortDefinition="Allowable authorization for this agent", formalDefinition="The authorization (e.g., PurposeOfUse) that was used during the event being recorded." ) 708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 709 protected List<CodeableConcept> authorization; 710 711 private static final long serialVersionUID = 509129255L; 712 713 /** 714 * Constructor 715 */ 716 public AuditEventAgentComponent() { 717 super(); 718 } 719 720 /** 721 * Constructor 722 */ 723 public AuditEventAgentComponent(Reference who) { 724 super(); 725 this.setWho(who); 726 } 727 728 /** 729 * @return {@link #type} (The Functional Role of the user when performing the event.) 730 */ 731 public CodeableConcept getType() { 732 if (this.type == null) 733 if (Configuration.errorOnAutoCreate()) 734 throw new Error("Attempt to auto-create AuditEventAgentComponent.type"); 735 else if (Configuration.doAutoCreate()) 736 this.type = new CodeableConcept(); // cc 737 return this.type; 738 } 739 740 public boolean hasType() { 741 return this.type != null && !this.type.isEmpty(); 742 } 743 744 /** 745 * @param value {@link #type} (The Functional Role of the user when performing the event.) 746 */ 747 public AuditEventAgentComponent setType(CodeableConcept value) { 748 this.type = value; 749 return this; 750 } 751 752 /** 753 * @return {@link #role} (The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity.) 754 */ 755 public List<CodeableConcept> getRole() { 756 if (this.role == null) 757 this.role = new ArrayList<CodeableConcept>(); 758 return this.role; 759 } 760 761 /** 762 * @return Returns a reference to <code>this</code> for easy method chaining 763 */ 764 public AuditEventAgentComponent setRole(List<CodeableConcept> theRole) { 765 this.role = theRole; 766 return this; 767 } 768 769 public boolean hasRole() { 770 if (this.role == null) 771 return false; 772 for (CodeableConcept item : this.role) 773 if (!item.isEmpty()) 774 return true; 775 return false; 776 } 777 778 public CodeableConcept addRole() { //3 779 CodeableConcept t = new CodeableConcept(); 780 if (this.role == null) 781 this.role = new ArrayList<CodeableConcept>(); 782 this.role.add(t); 783 return t; 784 } 785 786 public AuditEventAgentComponent addRole(CodeableConcept t) { //3 787 if (t == null) 788 return this; 789 if (this.role == null) 790 this.role = new ArrayList<CodeableConcept>(); 791 this.role.add(t); 792 return this; 793 } 794 795 /** 796 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist {3} 797 */ 798 public CodeableConcept getRoleFirstRep() { 799 if (getRole().isEmpty()) { 800 addRole(); 801 } 802 return getRole().get(0); 803 } 804 805 /** 806 * @return {@link #who} (Reference to who this agent is that was involved in the event.) 807 */ 808 public Reference getWho() { 809 if (this.who == null) 810 if (Configuration.errorOnAutoCreate()) 811 throw new Error("Attempt to auto-create AuditEventAgentComponent.who"); 812 else if (Configuration.doAutoCreate()) 813 this.who = new Reference(); // cc 814 return this.who; 815 } 816 817 public boolean hasWho() { 818 return this.who != null && !this.who.isEmpty(); 819 } 820 821 /** 822 * @param value {@link #who} (Reference to who this agent is that was involved in the event.) 823 */ 824 public AuditEventAgentComponent setWho(Reference value) { 825 this.who = value; 826 return this; 827 } 828 829 /** 830 * @return {@link #requestor} (Indicator that the user is or is not the requestor, or initiator, for the event being audited.). This is the underlying object with id, value and extensions. The accessor "getRequestor" gives direct access to the value 831 */ 832 public BooleanType getRequestorElement() { 833 if (this.requestor == null) 834 if (Configuration.errorOnAutoCreate()) 835 throw new Error("Attempt to auto-create AuditEventAgentComponent.requestor"); 836 else if (Configuration.doAutoCreate()) 837 this.requestor = new BooleanType(); // bb 838 return this.requestor; 839 } 840 841 public boolean hasRequestorElement() { 842 return this.requestor != null && !this.requestor.isEmpty(); 843 } 844 845 public boolean hasRequestor() { 846 return this.requestor != null && !this.requestor.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #requestor} (Indicator that the user is or is not the requestor, or initiator, for the event being audited.). This is the underlying object with id, value and extensions. The accessor "getRequestor" gives direct access to the value 851 */ 852 public AuditEventAgentComponent setRequestorElement(BooleanType value) { 853 this.requestor = value; 854 return this; 855 } 856 857 /** 858 * @return Indicator that the user is or is not the requestor, or initiator, for the event being audited. 859 */ 860 public boolean getRequestor() { 861 return this.requestor == null || this.requestor.isEmpty() ? false : this.requestor.getValue(); 862 } 863 864 /** 865 * @param value Indicator that the user is or is not the requestor, or initiator, for the event being audited. 866 */ 867 public AuditEventAgentComponent setRequestor(boolean value) { 868 if (this.requestor == null) 869 this.requestor = new BooleanType(); 870 this.requestor.setValue(value); 871 return this; 872 } 873 874 /** 875 * @return {@link #location} (Where the agent location is known, the agent location when the event occurred.) 876 */ 877 public Reference getLocation() { 878 if (this.location == null) 879 if (Configuration.errorOnAutoCreate()) 880 throw new Error("Attempt to auto-create AuditEventAgentComponent.location"); 881 else if (Configuration.doAutoCreate()) 882 this.location = new Reference(); // cc 883 return this.location; 884 } 885 886 public boolean hasLocation() { 887 return this.location != null && !this.location.isEmpty(); 888 } 889 890 /** 891 * @param value {@link #location} (Where the agent location is known, the agent location when the event occurred.) 892 */ 893 public AuditEventAgentComponent setLocation(Reference value) { 894 this.location = value; 895 return this; 896 } 897 898 /** 899 * @return {@link #policy} (Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 900 */ 901 public List<UriType> getPolicy() { 902 if (this.policy == null) 903 this.policy = new ArrayList<UriType>(); 904 return this.policy; 905 } 906 907 /** 908 * @return Returns a reference to <code>this</code> for easy method chaining 909 */ 910 public AuditEventAgentComponent setPolicy(List<UriType> thePolicy) { 911 this.policy = thePolicy; 912 return this; 913 } 914 915 public boolean hasPolicy() { 916 if (this.policy == null) 917 return false; 918 for (UriType item : this.policy) 919 if (!item.isEmpty()) 920 return true; 921 return false; 922 } 923 924 /** 925 * @return {@link #policy} (Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 926 */ 927 public UriType addPolicyElement() {//2 928 UriType t = new UriType(); 929 if (this.policy == null) 930 this.policy = new ArrayList<UriType>(); 931 this.policy.add(t); 932 return t; 933 } 934 935 /** 936 * @param value {@link #policy} (Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 937 */ 938 public AuditEventAgentComponent addPolicy(String value) { //1 939 UriType t = new UriType(); 940 t.setValue(value); 941 if (this.policy == null) 942 this.policy = new ArrayList<UriType>(); 943 this.policy.add(t); 944 return this; 945 } 946 947 /** 948 * @param value {@link #policy} (Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.) 949 */ 950 public boolean hasPolicy(String value) { 951 if (this.policy == null) 952 return false; 953 for (UriType v : this.policy) 954 if (v.getValue().equals(value)) // uri 955 return true; 956 return false; 957 } 958 959 /** 960 * @return {@link #network} (When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.) 961 */ 962 public DataType getNetwork() { 963 return this.network; 964 } 965 966 /** 967 * @return {@link #network} (When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.) 968 */ 969 public Reference getNetworkReference() throws FHIRException { 970 if (this.network == null) 971 this.network = new Reference(); 972 if (!(this.network instanceof Reference)) 973 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.network.getClass().getName()+" was encountered"); 974 return (Reference) this.network; 975 } 976 977 public boolean hasNetworkReference() { 978 return this.network instanceof Reference; 979 } 980 981 /** 982 * @return {@link #network} (When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.) 983 */ 984 public UriType getNetworkUriType() throws FHIRException { 985 if (this.network == null) 986 this.network = new UriType(); 987 if (!(this.network instanceof UriType)) 988 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.network.getClass().getName()+" was encountered"); 989 return (UriType) this.network; 990 } 991 992 public boolean hasNetworkUriType() { 993 return this.network instanceof UriType; 994 } 995 996 /** 997 * @return {@link #network} (When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.) 998 */ 999 public StringType getNetworkStringType() throws FHIRException { 1000 if (this.network == null) 1001 this.network = new StringType(); 1002 if (!(this.network instanceof StringType)) 1003 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.network.getClass().getName()+" was encountered"); 1004 return (StringType) this.network; 1005 } 1006 1007 public boolean hasNetworkStringType() { 1008 return this.network instanceof StringType; 1009 } 1010 1011 public boolean hasNetwork() { 1012 return this.network != null && !this.network.isEmpty(); 1013 } 1014 1015 /** 1016 * @param value {@link #network} (When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.) 1017 */ 1018 public AuditEventAgentComponent setNetwork(DataType value) { 1019 if (value != null && !(value instanceof Reference || value instanceof UriType || value instanceof StringType)) 1020 throw new FHIRException("Not the right type for AuditEvent.agent.network[x]: "+value.fhirType()); 1021 this.network = value; 1022 return this; 1023 } 1024 1025 /** 1026 * @return {@link #authorization} (The authorization (e.g., PurposeOfUse) that was used during the event being recorded.) 1027 */ 1028 public List<CodeableConcept> getAuthorization() { 1029 if (this.authorization == null) 1030 this.authorization = new ArrayList<CodeableConcept>(); 1031 return this.authorization; 1032 } 1033 1034 /** 1035 * @return Returns a reference to <code>this</code> for easy method chaining 1036 */ 1037 public AuditEventAgentComponent setAuthorization(List<CodeableConcept> theAuthorization) { 1038 this.authorization = theAuthorization; 1039 return this; 1040 } 1041 1042 public boolean hasAuthorization() { 1043 if (this.authorization == null) 1044 return false; 1045 for (CodeableConcept item : this.authorization) 1046 if (!item.isEmpty()) 1047 return true; 1048 return false; 1049 } 1050 1051 public CodeableConcept addAuthorization() { //3 1052 CodeableConcept t = new CodeableConcept(); 1053 if (this.authorization == null) 1054 this.authorization = new ArrayList<CodeableConcept>(); 1055 this.authorization.add(t); 1056 return t; 1057 } 1058 1059 public AuditEventAgentComponent addAuthorization(CodeableConcept t) { //3 1060 if (t == null) 1061 return this; 1062 if (this.authorization == null) 1063 this.authorization = new ArrayList<CodeableConcept>(); 1064 this.authorization.add(t); 1065 return this; 1066 } 1067 1068 /** 1069 * @return The first repetition of repeating field {@link #authorization}, creating it if it does not already exist {3} 1070 */ 1071 public CodeableConcept getAuthorizationFirstRep() { 1072 if (getAuthorization().isEmpty()) { 1073 addAuthorization(); 1074 } 1075 return getAuthorization().get(0); 1076 } 1077 1078 protected void listChildren(List<Property> children) { 1079 super.listChildren(children); 1080 children.add(new Property("type", "CodeableConcept", "The Functional Role of the user when performing the event.", 0, 1, type)); 1081 children.add(new Property("role", "CodeableConcept", "The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity.", 0, java.lang.Integer.MAX_VALUE, role)); 1082 children.add(new Property("who", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Reference to who this agent is that was involved in the event.", 0, 1, who)); 1083 children.add(new Property("requestor", "boolean", "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, requestor)); 1084 children.add(new Property("location", "Reference(Location)", "Where the agent location is known, the agent location when the event occurred.", 0, 1, location)); 1085 children.add(new Property("policy", "uri", "Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 0, java.lang.Integer.MAX_VALUE, policy)); 1086 children.add(new Property("network[x]", "Reference(Endpoint)|uri|string", "When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.", 0, 1, network)); 1087 children.add(new Property("authorization", "CodeableConcept", "The authorization (e.g., PurposeOfUse) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, authorization)); 1088 } 1089 1090 @Override 1091 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1092 switch (_hash) { 1093 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The Functional Role of the user when performing the event.", 0, 1, type); 1094 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The structural roles of the agent indicating the agent's competency. The security role enabling the agent with respect to the activity.", 0, java.lang.Integer.MAX_VALUE, role); 1095 case 117694: /*who*/ return new Property("who", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Reference to who this agent is that was involved in the event.", 0, 1, who); 1096 case 693934258: /*requestor*/ return new Property("requestor", "boolean", "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1, requestor); 1097 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Where the agent location is known, the agent location when the event occurred.", 0, 1, location); 1098 case -982670030: /*policy*/ return new Property("policy", "uri", "Where the policy(ies) are known that authorized the agent participation in the event. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 0, java.lang.Integer.MAX_VALUE, policy); 1099 case -478235758: /*network[x]*/ return new Property("network[x]", "Reference(Endpoint)|uri|string", "When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.", 0, 1, network); 1100 case 1843485230: /*network*/ return new Property("network[x]", "Reference(Endpoint)|uri|string", "When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.", 0, 1, network); 1101 case -1769760579: /*networkReference*/ return new Property("network[x]", "Reference(Endpoint)", "When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.", 0, 1, network); 1102 case -478241698: /*networkUri*/ return new Property("network[x]", "uri", "When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.", 0, 1, network); 1103 case -946943009: /*networkString*/ return new Property("network[x]", "string", "When the event utilizes a network there should be an agent describing the local system, and an agent describing remote system, with the network interface details.", 0, 1, network); 1104 case -1385570183: /*authorization*/ return new Property("authorization", "CodeableConcept", "The authorization (e.g., PurposeOfUse) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, authorization); 1105 default: return super.getNamedProperty(_hash, _name, _checkValid); 1106 } 1107 1108 } 1109 1110 @Override 1111 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1112 switch (hash) { 1113 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1114 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 1115 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Reference 1116 case 693934258: /*requestor*/ return this.requestor == null ? new Base[0] : new Base[] {this.requestor}; // BooleanType 1117 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1118 case -982670030: /*policy*/ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // UriType 1119 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // DataType 1120 case -1385570183: /*authorization*/ return this.authorization == null ? new Base[0] : this.authorization.toArray(new Base[this.authorization.size()]); // CodeableConcept 1121 default: return super.getProperty(hash, name, checkValid); 1122 } 1123 1124 } 1125 1126 @Override 1127 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1128 switch (hash) { 1129 case 3575610: // type 1130 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1131 return value; 1132 case 3506294: // role 1133 this.getRole().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1134 return value; 1135 case 117694: // who 1136 this.who = TypeConvertor.castToReference(value); // Reference 1137 return value; 1138 case 693934258: // requestor 1139 this.requestor = TypeConvertor.castToBoolean(value); // BooleanType 1140 return value; 1141 case 1901043637: // location 1142 this.location = TypeConvertor.castToReference(value); // Reference 1143 return value; 1144 case -982670030: // policy 1145 this.getPolicy().add(TypeConvertor.castToUri(value)); // UriType 1146 return value; 1147 case 1843485230: // network 1148 this.network = TypeConvertor.castToType(value); // DataType 1149 return value; 1150 case -1385570183: // authorization 1151 this.getAuthorization().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1152 return value; 1153 default: return super.setProperty(hash, name, value); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base setProperty(String name, Base value) throws FHIRException { 1160 if (name.equals("type")) { 1161 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1162 } else if (name.equals("role")) { 1163 this.getRole().add(TypeConvertor.castToCodeableConcept(value)); 1164 } else if (name.equals("who")) { 1165 this.who = TypeConvertor.castToReference(value); // Reference 1166 } else if (name.equals("requestor")) { 1167 this.requestor = TypeConvertor.castToBoolean(value); // BooleanType 1168 } else if (name.equals("location")) { 1169 this.location = TypeConvertor.castToReference(value); // Reference 1170 } else if (name.equals("policy")) { 1171 this.getPolicy().add(TypeConvertor.castToUri(value)); 1172 } else if (name.equals("network[x]")) { 1173 this.network = TypeConvertor.castToType(value); // DataType 1174 } else if (name.equals("authorization")) { 1175 this.getAuthorization().add(TypeConvertor.castToCodeableConcept(value)); 1176 } else 1177 return super.setProperty(name, value); 1178 return value; 1179 } 1180 1181 @Override 1182 public void removeChild(String name, Base value) throws FHIRException { 1183 if (name.equals("type")) { 1184 this.type = null; 1185 } else if (name.equals("role")) { 1186 this.getRole().remove(value); 1187 } else if (name.equals("who")) { 1188 this.who = null; 1189 } else if (name.equals("requestor")) { 1190 this.requestor = null; 1191 } else if (name.equals("location")) { 1192 this.location = null; 1193 } else if (name.equals("policy")) { 1194 this.getPolicy().remove(value); 1195 } else if (name.equals("network[x]")) { 1196 this.network = null; 1197 } else if (name.equals("authorization")) { 1198 this.getAuthorization().remove(value); 1199 } else 1200 super.removeChild(name, value); 1201 1202 } 1203 1204 @Override 1205 public Base makeProperty(int hash, String name) throws FHIRException { 1206 switch (hash) { 1207 case 3575610: return getType(); 1208 case 3506294: return addRole(); 1209 case 117694: return getWho(); 1210 case 693934258: return getRequestorElement(); 1211 case 1901043637: return getLocation(); 1212 case -982670030: return addPolicyElement(); 1213 case -478235758: return getNetwork(); 1214 case 1843485230: return getNetwork(); 1215 case -1385570183: return addAuthorization(); 1216 default: return super.makeProperty(hash, name); 1217 } 1218 1219 } 1220 1221 @Override 1222 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1223 switch (hash) { 1224 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1225 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1226 case 117694: /*who*/ return new String[] {"Reference"}; 1227 case 693934258: /*requestor*/ return new String[] {"boolean"}; 1228 case 1901043637: /*location*/ return new String[] {"Reference"}; 1229 case -982670030: /*policy*/ return new String[] {"uri"}; 1230 case 1843485230: /*network*/ return new String[] {"Reference", "uri", "string"}; 1231 case -1385570183: /*authorization*/ return new String[] {"CodeableConcept"}; 1232 default: return super.getTypesForProperty(hash, name); 1233 } 1234 1235 } 1236 1237 @Override 1238 public Base addChild(String name) throws FHIRException { 1239 if (name.equals("type")) { 1240 this.type = new CodeableConcept(); 1241 return this.type; 1242 } 1243 else if (name.equals("role")) { 1244 return addRole(); 1245 } 1246 else if (name.equals("who")) { 1247 this.who = new Reference(); 1248 return this.who; 1249 } 1250 else if (name.equals("requestor")) { 1251 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.agent.requestor"); 1252 } 1253 else if (name.equals("location")) { 1254 this.location = new Reference(); 1255 return this.location; 1256 } 1257 else if (name.equals("policy")) { 1258 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.agent.policy"); 1259 } 1260 else if (name.equals("networkReference")) { 1261 this.network = new Reference(); 1262 return this.network; 1263 } 1264 else if (name.equals("networkUri")) { 1265 this.network = new UriType(); 1266 return this.network; 1267 } 1268 else if (name.equals("networkString")) { 1269 this.network = new StringType(); 1270 return this.network; 1271 } 1272 else if (name.equals("authorization")) { 1273 return addAuthorization(); 1274 } 1275 else 1276 return super.addChild(name); 1277 } 1278 1279 public AuditEventAgentComponent copy() { 1280 AuditEventAgentComponent dst = new AuditEventAgentComponent(); 1281 copyValues(dst); 1282 return dst; 1283 } 1284 1285 public void copyValues(AuditEventAgentComponent dst) { 1286 super.copyValues(dst); 1287 dst.type = type == null ? null : type.copy(); 1288 if (role != null) { 1289 dst.role = new ArrayList<CodeableConcept>(); 1290 for (CodeableConcept i : role) 1291 dst.role.add(i.copy()); 1292 }; 1293 dst.who = who == null ? null : who.copy(); 1294 dst.requestor = requestor == null ? null : requestor.copy(); 1295 dst.location = location == null ? null : location.copy(); 1296 if (policy != null) { 1297 dst.policy = new ArrayList<UriType>(); 1298 for (UriType i : policy) 1299 dst.policy.add(i.copy()); 1300 }; 1301 dst.network = network == null ? null : network.copy(); 1302 if (authorization != null) { 1303 dst.authorization = new ArrayList<CodeableConcept>(); 1304 for (CodeableConcept i : authorization) 1305 dst.authorization.add(i.copy()); 1306 }; 1307 } 1308 1309 @Override 1310 public boolean equalsDeep(Base other_) { 1311 if (!super.equalsDeep(other_)) 1312 return false; 1313 if (!(other_ instanceof AuditEventAgentComponent)) 1314 return false; 1315 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1316 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true) && compareDeep(who, o.who, true) 1317 && compareDeep(requestor, o.requestor, true) && compareDeep(location, o.location, true) && compareDeep(policy, o.policy, true) 1318 && compareDeep(network, o.network, true) && compareDeep(authorization, o.authorization, true); 1319 } 1320 1321 @Override 1322 public boolean equalsShallow(Base other_) { 1323 if (!super.equalsShallow(other_)) 1324 return false; 1325 if (!(other_ instanceof AuditEventAgentComponent)) 1326 return false; 1327 AuditEventAgentComponent o = (AuditEventAgentComponent) other_; 1328 return compareValues(requestor, o.requestor, true) && compareValues(policy, o.policy, true); 1329 } 1330 1331 public boolean isEmpty() { 1332 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role, who, requestor 1333 , location, policy, network, authorization); 1334 } 1335 1336 public String fhirType() { 1337 return "AuditEvent.agent"; 1338 1339 } 1340 1341 } 1342 1343 @Block() 1344 public static class AuditEventSourceComponent extends BackboneElement implements IBaseBackboneElement { 1345 /** 1346 * Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group. 1347 */ 1348 @Child(name = "site", type = {Location.class}, order=1, min=0, max=1, modifier=false, summary=false) 1349 @Description(shortDefinition="Logical source location within the enterprise", formalDefinition="Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group." ) 1350 protected Reference site; 1351 1352 /** 1353 * Identifier of the source where the event was detected. 1354 */ 1355 @Child(name = "observer", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=true) 1356 @Description(shortDefinition="The identity of source detecting the event", formalDefinition="Identifier of the source where the event was detected." ) 1357 protected Reference observer; 1358 1359 /** 1360 * Code specifying the type of source where event originated. 1361 */ 1362 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1363 @Description(shortDefinition="The type of source where event originated", formalDefinition="Code specifying the type of source where event originated." ) 1364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-source-type") 1365 protected List<CodeableConcept> type; 1366 1367 private static final long serialVersionUID = -140005578L; 1368 1369 /** 1370 * Constructor 1371 */ 1372 public AuditEventSourceComponent() { 1373 super(); 1374 } 1375 1376 /** 1377 * Constructor 1378 */ 1379 public AuditEventSourceComponent(Reference observer) { 1380 super(); 1381 this.setObserver(observer); 1382 } 1383 1384 /** 1385 * @return {@link #site} (Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.) 1386 */ 1387 public Reference getSite() { 1388 if (this.site == null) 1389 if (Configuration.errorOnAutoCreate()) 1390 throw new Error("Attempt to auto-create AuditEventSourceComponent.site"); 1391 else if (Configuration.doAutoCreate()) 1392 this.site = new Reference(); // cc 1393 return this.site; 1394 } 1395 1396 public boolean hasSite() { 1397 return this.site != null && !this.site.isEmpty(); 1398 } 1399 1400 /** 1401 * @param value {@link #site} (Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.) 1402 */ 1403 public AuditEventSourceComponent setSite(Reference value) { 1404 this.site = value; 1405 return this; 1406 } 1407 1408 /** 1409 * @return {@link #observer} (Identifier of the source where the event was detected.) 1410 */ 1411 public Reference getObserver() { 1412 if (this.observer == null) 1413 if (Configuration.errorOnAutoCreate()) 1414 throw new Error("Attempt to auto-create AuditEventSourceComponent.observer"); 1415 else if (Configuration.doAutoCreate()) 1416 this.observer = new Reference(); // cc 1417 return this.observer; 1418 } 1419 1420 public boolean hasObserver() { 1421 return this.observer != null && !this.observer.isEmpty(); 1422 } 1423 1424 /** 1425 * @param value {@link #observer} (Identifier of the source where the event was detected.) 1426 */ 1427 public AuditEventSourceComponent setObserver(Reference value) { 1428 this.observer = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return {@link #type} (Code specifying the type of source where event originated.) 1434 */ 1435 public List<CodeableConcept> getType() { 1436 if (this.type == null) 1437 this.type = new ArrayList<CodeableConcept>(); 1438 return this.type; 1439 } 1440 1441 /** 1442 * @return Returns a reference to <code>this</code> for easy method chaining 1443 */ 1444 public AuditEventSourceComponent setType(List<CodeableConcept> theType) { 1445 this.type = theType; 1446 return this; 1447 } 1448 1449 public boolean hasType() { 1450 if (this.type == null) 1451 return false; 1452 for (CodeableConcept item : this.type) 1453 if (!item.isEmpty()) 1454 return true; 1455 return false; 1456 } 1457 1458 public CodeableConcept addType() { //3 1459 CodeableConcept t = new CodeableConcept(); 1460 if (this.type == null) 1461 this.type = new ArrayList<CodeableConcept>(); 1462 this.type.add(t); 1463 return t; 1464 } 1465 1466 public AuditEventSourceComponent addType(CodeableConcept t) { //3 1467 if (t == null) 1468 return this; 1469 if (this.type == null) 1470 this.type = new ArrayList<CodeableConcept>(); 1471 this.type.add(t); 1472 return this; 1473 } 1474 1475 /** 1476 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1477 */ 1478 public CodeableConcept getTypeFirstRep() { 1479 if (getType().isEmpty()) { 1480 addType(); 1481 } 1482 return getType().get(0); 1483 } 1484 1485 protected void listChildren(List<Property> children) { 1486 super.listChildren(children); 1487 children.add(new Property("site", "Reference(Location)", "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 0, 1, site)); 1488 children.add(new Property("observer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Identifier of the source where the event was detected.", 0, 1, observer)); 1489 children.add(new Property("type", "CodeableConcept", "Code specifying the type of source where event originated.", 0, java.lang.Integer.MAX_VALUE, type)); 1490 } 1491 1492 @Override 1493 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1494 switch (_hash) { 1495 case 3530567: /*site*/ return new Property("site", "Reference(Location)", "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 0, 1, site); 1496 case 348607190: /*observer*/ return new Property("observer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", "Identifier of the source where the event was detected.", 0, 1, observer); 1497 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code specifying the type of source where event originated.", 0, java.lang.Integer.MAX_VALUE, type); 1498 default: return super.getNamedProperty(_hash, _name, _checkValid); 1499 } 1500 1501 } 1502 1503 @Override 1504 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1505 switch (hash) { 1506 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // Reference 1507 case 348607190: /*observer*/ return this.observer == null ? new Base[0] : new Base[] {this.observer}; // Reference 1508 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1509 default: return super.getProperty(hash, name, checkValid); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1516 switch (hash) { 1517 case 3530567: // site 1518 this.site = TypeConvertor.castToReference(value); // Reference 1519 return value; 1520 case 348607190: // observer 1521 this.observer = TypeConvertor.castToReference(value); // Reference 1522 return value; 1523 case 3575610: // type 1524 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1525 return value; 1526 default: return super.setProperty(hash, name, value); 1527 } 1528 1529 } 1530 1531 @Override 1532 public Base setProperty(String name, Base value) throws FHIRException { 1533 if (name.equals("site")) { 1534 this.site = TypeConvertor.castToReference(value); // Reference 1535 } else if (name.equals("observer")) { 1536 this.observer = TypeConvertor.castToReference(value); // Reference 1537 } else if (name.equals("type")) { 1538 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1539 } else 1540 return super.setProperty(name, value); 1541 return value; 1542 } 1543 1544 @Override 1545 public void removeChild(String name, Base value) throws FHIRException { 1546 if (name.equals("site")) { 1547 this.site = null; 1548 } else if (name.equals("observer")) { 1549 this.observer = null; 1550 } else if (name.equals("type")) { 1551 this.getType().remove(value); 1552 } else 1553 super.removeChild(name, value); 1554 1555 } 1556 1557 @Override 1558 public Base makeProperty(int hash, String name) throws FHIRException { 1559 switch (hash) { 1560 case 3530567: return getSite(); 1561 case 348607190: return getObserver(); 1562 case 3575610: return addType(); 1563 default: return super.makeProperty(hash, name); 1564 } 1565 1566 } 1567 1568 @Override 1569 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1570 switch (hash) { 1571 case 3530567: /*site*/ return new String[] {"Reference"}; 1572 case 348607190: /*observer*/ return new String[] {"Reference"}; 1573 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1574 default: return super.getTypesForProperty(hash, name); 1575 } 1576 1577 } 1578 1579 @Override 1580 public Base addChild(String name) throws FHIRException { 1581 if (name.equals("site")) { 1582 this.site = new Reference(); 1583 return this.site; 1584 } 1585 else if (name.equals("observer")) { 1586 this.observer = new Reference(); 1587 return this.observer; 1588 } 1589 else if (name.equals("type")) { 1590 return addType(); 1591 } 1592 else 1593 return super.addChild(name); 1594 } 1595 1596 public AuditEventSourceComponent copy() { 1597 AuditEventSourceComponent dst = new AuditEventSourceComponent(); 1598 copyValues(dst); 1599 return dst; 1600 } 1601 1602 public void copyValues(AuditEventSourceComponent dst) { 1603 super.copyValues(dst); 1604 dst.site = site == null ? null : site.copy(); 1605 dst.observer = observer == null ? null : observer.copy(); 1606 if (type != null) { 1607 dst.type = new ArrayList<CodeableConcept>(); 1608 for (CodeableConcept i : type) 1609 dst.type.add(i.copy()); 1610 }; 1611 } 1612 1613 @Override 1614 public boolean equalsDeep(Base other_) { 1615 if (!super.equalsDeep(other_)) 1616 return false; 1617 if (!(other_ instanceof AuditEventSourceComponent)) 1618 return false; 1619 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 1620 return compareDeep(site, o.site, true) && compareDeep(observer, o.observer, true) && compareDeep(type, o.type, true) 1621 ; 1622 } 1623 1624 @Override 1625 public boolean equalsShallow(Base other_) { 1626 if (!super.equalsShallow(other_)) 1627 return false; 1628 if (!(other_ instanceof AuditEventSourceComponent)) 1629 return false; 1630 AuditEventSourceComponent o = (AuditEventSourceComponent) other_; 1631 return true; 1632 } 1633 1634 public boolean isEmpty() { 1635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, observer, type); 1636 } 1637 1638 public String fhirType() { 1639 return "AuditEvent.source"; 1640 1641 } 1642 1643 } 1644 1645 @Block() 1646 public static class AuditEventEntityComponent extends BackboneElement implements IBaseBackboneElement { 1647 /** 1648 * Identifies a specific instance of the entity. The reference should be version specific. This is allowed to be a Parameters resource. 1649 */ 1650 @Child(name = "what", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=true) 1651 @Description(shortDefinition="Specific instance of resource", formalDefinition="Identifies a specific instance of the entity. The reference should be version specific. This is allowed to be a Parameters resource." ) 1652 protected Reference what; 1653 1654 /** 1655 * Code representing the role the entity played in the event being audited. 1656 */ 1657 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1658 @Description(shortDefinition="What role the entity played", formalDefinition="Code representing the role the entity played in the event being audited." ) 1659 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/object-role") 1660 protected CodeableConcept role; 1661 1662 /** 1663 * Security labels for the identified entity. 1664 */ 1665 @Child(name = "securityLabel", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1666 @Description(shortDefinition="Security labels on the entity", formalDefinition="Security labels for the identified entity." ) 1667 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-label-examples") 1668 protected List<CodeableConcept> securityLabel; 1669 1670 /** 1671 * The query parameters for a query-type entities. 1672 */ 1673 @Child(name = "query", type = {Base64BinaryType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1674 @Description(shortDefinition="Query parameters", formalDefinition="The query parameters for a query-type entities." ) 1675 protected Base64BinaryType query; 1676 1677 /** 1678 * Tagged value pairs for conveying additional information about the entity. 1679 */ 1680 @Child(name = "detail", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1681 @Description(shortDefinition="Additional Information about the entity", formalDefinition="Tagged value pairs for conveying additional information about the entity." ) 1682 protected List<AuditEventEntityDetailComponent> detail; 1683 1684 /** 1685 * The entity is attributed to an agent to express the agent's responsibility for that entity in the activity. This is most used to indicate when persistence media (the entity) are used by an agent. For example when importing data from a device, the device would be described in an entity, and the user importing data from that media would be indicated as the entity.agent. 1686 */ 1687 @Child(name = "agent", type = {AuditEventAgentComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1688 @Description(shortDefinition="Entity is attributed to this agent", formalDefinition="The entity is attributed to an agent to express the agent's responsibility for that entity in the activity. This is most used to indicate when persistence media (the entity) are used by an agent. For example when importing data from a device, the device would be described in an entity, and the user importing data from that media would be indicated as the entity.agent." ) 1689 protected List<AuditEventAgentComponent> agent; 1690 1691 private static final long serialVersionUID = -711898650L; 1692 1693 /** 1694 * Constructor 1695 */ 1696 public AuditEventEntityComponent() { 1697 super(); 1698 } 1699 1700 /** 1701 * @return {@link #what} (Identifies a specific instance of the entity. The reference should be version specific. This is allowed to be a Parameters resource.) 1702 */ 1703 public Reference getWhat() { 1704 if (this.what == null) 1705 if (Configuration.errorOnAutoCreate()) 1706 throw new Error("Attempt to auto-create AuditEventEntityComponent.what"); 1707 else if (Configuration.doAutoCreate()) 1708 this.what = new Reference(); // cc 1709 return this.what; 1710 } 1711 1712 public boolean hasWhat() { 1713 return this.what != null && !this.what.isEmpty(); 1714 } 1715 1716 /** 1717 * @param value {@link #what} (Identifies a specific instance of the entity. The reference should be version specific. This is allowed to be a Parameters resource.) 1718 */ 1719 public AuditEventEntityComponent setWhat(Reference value) { 1720 this.what = value; 1721 return this; 1722 } 1723 1724 /** 1725 * @return {@link #role} (Code representing the role the entity played in the event being audited.) 1726 */ 1727 public CodeableConcept getRole() { 1728 if (this.role == null) 1729 if (Configuration.errorOnAutoCreate()) 1730 throw new Error("Attempt to auto-create AuditEventEntityComponent.role"); 1731 else if (Configuration.doAutoCreate()) 1732 this.role = new CodeableConcept(); // cc 1733 return this.role; 1734 } 1735 1736 public boolean hasRole() { 1737 return this.role != null && !this.role.isEmpty(); 1738 } 1739 1740 /** 1741 * @param value {@link #role} (Code representing the role the entity played in the event being audited.) 1742 */ 1743 public AuditEventEntityComponent setRole(CodeableConcept value) { 1744 this.role = value; 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #securityLabel} (Security labels for the identified entity.) 1750 */ 1751 public List<CodeableConcept> getSecurityLabel() { 1752 if (this.securityLabel == null) 1753 this.securityLabel = new ArrayList<CodeableConcept>(); 1754 return this.securityLabel; 1755 } 1756 1757 /** 1758 * @return Returns a reference to <code>this</code> for easy method chaining 1759 */ 1760 public AuditEventEntityComponent setSecurityLabel(List<CodeableConcept> theSecurityLabel) { 1761 this.securityLabel = theSecurityLabel; 1762 return this; 1763 } 1764 1765 public boolean hasSecurityLabel() { 1766 if (this.securityLabel == null) 1767 return false; 1768 for (CodeableConcept item : this.securityLabel) 1769 if (!item.isEmpty()) 1770 return true; 1771 return false; 1772 } 1773 1774 public CodeableConcept addSecurityLabel() { //3 1775 CodeableConcept t = new CodeableConcept(); 1776 if (this.securityLabel == null) 1777 this.securityLabel = new ArrayList<CodeableConcept>(); 1778 this.securityLabel.add(t); 1779 return t; 1780 } 1781 1782 public AuditEventEntityComponent addSecurityLabel(CodeableConcept t) { //3 1783 if (t == null) 1784 return this; 1785 if (this.securityLabel == null) 1786 this.securityLabel = new ArrayList<CodeableConcept>(); 1787 this.securityLabel.add(t); 1788 return this; 1789 } 1790 1791 /** 1792 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist {3} 1793 */ 1794 public CodeableConcept getSecurityLabelFirstRep() { 1795 if (getSecurityLabel().isEmpty()) { 1796 addSecurityLabel(); 1797 } 1798 return getSecurityLabel().get(0); 1799 } 1800 1801 /** 1802 * @return {@link #query} (The query parameters for a query-type entities.). This is the underlying object with id, value and extensions. The accessor "getQuery" gives direct access to the value 1803 */ 1804 public Base64BinaryType getQueryElement() { 1805 if (this.query == null) 1806 if (Configuration.errorOnAutoCreate()) 1807 throw new Error("Attempt to auto-create AuditEventEntityComponent.query"); 1808 else if (Configuration.doAutoCreate()) 1809 this.query = new Base64BinaryType(); // bb 1810 return this.query; 1811 } 1812 1813 public boolean hasQueryElement() { 1814 return this.query != null && !this.query.isEmpty(); 1815 } 1816 1817 public boolean hasQuery() { 1818 return this.query != null && !this.query.isEmpty(); 1819 } 1820 1821 /** 1822 * @param value {@link #query} (The query parameters for a query-type entities.). This is the underlying object with id, value and extensions. The accessor "getQuery" gives direct access to the value 1823 */ 1824 public AuditEventEntityComponent setQueryElement(Base64BinaryType value) { 1825 this.query = value; 1826 return this; 1827 } 1828 1829 /** 1830 * @return The query parameters for a query-type entities. 1831 */ 1832 public byte[] getQuery() { 1833 return this.query == null ? null : this.query.getValue(); 1834 } 1835 1836 /** 1837 * @param value The query parameters for a query-type entities. 1838 */ 1839 public AuditEventEntityComponent setQuery(byte[] value) { 1840 if (value == null) 1841 this.query = null; 1842 else { 1843 if (this.query == null) 1844 this.query = new Base64BinaryType(); 1845 this.query.setValue(value); 1846 } 1847 return this; 1848 } 1849 1850 /** 1851 * @return {@link #detail} (Tagged value pairs for conveying additional information about the entity.) 1852 */ 1853 public List<AuditEventEntityDetailComponent> getDetail() { 1854 if (this.detail == null) 1855 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 1856 return this.detail; 1857 } 1858 1859 /** 1860 * @return Returns a reference to <code>this</code> for easy method chaining 1861 */ 1862 public AuditEventEntityComponent setDetail(List<AuditEventEntityDetailComponent> theDetail) { 1863 this.detail = theDetail; 1864 return this; 1865 } 1866 1867 public boolean hasDetail() { 1868 if (this.detail == null) 1869 return false; 1870 for (AuditEventEntityDetailComponent item : this.detail) 1871 if (!item.isEmpty()) 1872 return true; 1873 return false; 1874 } 1875 1876 public AuditEventEntityDetailComponent addDetail() { //3 1877 AuditEventEntityDetailComponent t = new AuditEventEntityDetailComponent(); 1878 if (this.detail == null) 1879 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 1880 this.detail.add(t); 1881 return t; 1882 } 1883 1884 public AuditEventEntityComponent addDetail(AuditEventEntityDetailComponent t) { //3 1885 if (t == null) 1886 return this; 1887 if (this.detail == null) 1888 this.detail = new ArrayList<AuditEventEntityDetailComponent>(); 1889 this.detail.add(t); 1890 return this; 1891 } 1892 1893 /** 1894 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 1895 */ 1896 public AuditEventEntityDetailComponent getDetailFirstRep() { 1897 if (getDetail().isEmpty()) { 1898 addDetail(); 1899 } 1900 return getDetail().get(0); 1901 } 1902 1903 /** 1904 * @return {@link #agent} (The entity is attributed to an agent to express the agent's responsibility for that entity in the activity. This is most used to indicate when persistence media (the entity) are used by an agent. For example when importing data from a device, the device would be described in an entity, and the user importing data from that media would be indicated as the entity.agent.) 1905 */ 1906 public List<AuditEventAgentComponent> getAgent() { 1907 if (this.agent == null) 1908 this.agent = new ArrayList<AuditEventAgentComponent>(); 1909 return this.agent; 1910 } 1911 1912 /** 1913 * @return Returns a reference to <code>this</code> for easy method chaining 1914 */ 1915 public AuditEventEntityComponent setAgent(List<AuditEventAgentComponent> theAgent) { 1916 this.agent = theAgent; 1917 return this; 1918 } 1919 1920 public boolean hasAgent() { 1921 if (this.agent == null) 1922 return false; 1923 for (AuditEventAgentComponent item : this.agent) 1924 if (!item.isEmpty()) 1925 return true; 1926 return false; 1927 } 1928 1929 public AuditEventAgentComponent addAgent() { //3 1930 AuditEventAgentComponent t = new AuditEventAgentComponent(); 1931 if (this.agent == null) 1932 this.agent = new ArrayList<AuditEventAgentComponent>(); 1933 this.agent.add(t); 1934 return t; 1935 } 1936 1937 public AuditEventEntityComponent addAgent(AuditEventAgentComponent t) { //3 1938 if (t == null) 1939 return this; 1940 if (this.agent == null) 1941 this.agent = new ArrayList<AuditEventAgentComponent>(); 1942 this.agent.add(t); 1943 return this; 1944 } 1945 1946 /** 1947 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist {3} 1948 */ 1949 public AuditEventAgentComponent getAgentFirstRep() { 1950 if (getAgent().isEmpty()) { 1951 addAgent(); 1952 } 1953 return getAgent().get(0); 1954 } 1955 1956 protected void listChildren(List<Property> children) { 1957 super.listChildren(children); 1958 children.add(new Property("what", "Reference(Any)", "Identifies a specific instance of the entity. The reference should be version specific. This is allowed to be a Parameters resource.", 0, 1, what)); 1959 children.add(new Property("role", "CodeableConcept", "Code representing the role the entity played in the event being audited.", 0, 1, role)); 1960 children.add(new Property("securityLabel", "CodeableConcept", "Security labels for the identified entity.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1961 children.add(new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 1, query)); 1962 children.add(new Property("detail", "", "Tagged value pairs for conveying additional information about the entity.", 0, java.lang.Integer.MAX_VALUE, detail)); 1963 children.add(new Property("agent", "@AuditEvent.agent", "The entity is attributed to an agent to express the agent's responsibility for that entity in the activity. This is most used to indicate when persistence media (the entity) are used by an agent. For example when importing data from a device, the device would be described in an entity, and the user importing data from that media would be indicated as the entity.agent.", 0, java.lang.Integer.MAX_VALUE, agent)); 1964 } 1965 1966 @Override 1967 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1968 switch (_hash) { 1969 case 3648196: /*what*/ return new Property("what", "Reference(Any)", "Identifies a specific instance of the entity. The reference should be version specific. This is allowed to be a Parameters resource.", 0, 1, what); 1970 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Code representing the role the entity played in the event being audited.", 0, 1, role); 1971 case -722296940: /*securityLabel*/ return new Property("securityLabel", "CodeableConcept", "Security labels for the identified entity.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 1972 case 107944136: /*query*/ return new Property("query", "base64Binary", "The query parameters for a query-type entities.", 0, 1, query); 1973 case -1335224239: /*detail*/ return new Property("detail", "", "Tagged value pairs for conveying additional information about the entity.", 0, java.lang.Integer.MAX_VALUE, detail); 1974 case 92750597: /*agent*/ return new Property("agent", "@AuditEvent.agent", "The entity is attributed to an agent to express the agent's responsibility for that entity in the activity. This is most used to indicate when persistence media (the entity) are used by an agent. For example when importing data from a device, the device would be described in an entity, and the user importing data from that media would be indicated as the entity.agent.", 0, java.lang.Integer.MAX_VALUE, agent); 1975 default: return super.getNamedProperty(_hash, _name, _checkValid); 1976 } 1977 1978 } 1979 1980 @Override 1981 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1982 switch (hash) { 1983 case 3648196: /*what*/ return this.what == null ? new Base[0] : new Base[] {this.what}; // Reference 1984 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1985 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // CodeableConcept 1986 case 107944136: /*query*/ return this.query == null ? new Base[0] : new Base[] {this.query}; // Base64BinaryType 1987 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AuditEventEntityDetailComponent 1988 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // AuditEventAgentComponent 1989 default: return super.getProperty(hash, name, checkValid); 1990 } 1991 1992 } 1993 1994 @Override 1995 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1996 switch (hash) { 1997 case 3648196: // what 1998 this.what = TypeConvertor.castToReference(value); // Reference 1999 return value; 2000 case 3506294: // role 2001 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2002 return value; 2003 case -722296940: // securityLabel 2004 this.getSecurityLabel().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2005 return value; 2006 case 107944136: // query 2007 this.query = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 2008 return value; 2009 case -1335224239: // detail 2010 this.getDetail().add((AuditEventEntityDetailComponent) value); // AuditEventEntityDetailComponent 2011 return value; 2012 case 92750597: // agent 2013 this.getAgent().add((AuditEventAgentComponent) value); // AuditEventAgentComponent 2014 return value; 2015 default: return super.setProperty(hash, name, value); 2016 } 2017 2018 } 2019 2020 @Override 2021 public Base setProperty(String name, Base value) throws FHIRException { 2022 if (name.equals("what")) { 2023 this.what = TypeConvertor.castToReference(value); // Reference 2024 } else if (name.equals("role")) { 2025 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2026 } else if (name.equals("securityLabel")) { 2027 this.getSecurityLabel().add(TypeConvertor.castToCodeableConcept(value)); 2028 } else if (name.equals("query")) { 2029 this.query = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 2030 } else if (name.equals("detail")) { 2031 this.getDetail().add((AuditEventEntityDetailComponent) value); 2032 } else if (name.equals("agent")) { 2033 this.getAgent().add((AuditEventAgentComponent) value); 2034 } else 2035 return super.setProperty(name, value); 2036 return value; 2037 } 2038 2039 @Override 2040 public void removeChild(String name, Base value) throws FHIRException { 2041 if (name.equals("what")) { 2042 this.what = null; 2043 } else if (name.equals("role")) { 2044 this.role = null; 2045 } else if (name.equals("securityLabel")) { 2046 this.getSecurityLabel().remove(value); 2047 } else if (name.equals("query")) { 2048 this.query = null; 2049 } else if (name.equals("detail")) { 2050 this.getDetail().remove((AuditEventEntityDetailComponent) value); 2051 } else if (name.equals("agent")) { 2052 this.getAgent().remove((AuditEventAgentComponent) value); 2053 } else 2054 super.removeChild(name, value); 2055 2056 } 2057 2058 @Override 2059 public Base makeProperty(int hash, String name) throws FHIRException { 2060 switch (hash) { 2061 case 3648196: return getWhat(); 2062 case 3506294: return getRole(); 2063 case -722296940: return addSecurityLabel(); 2064 case 107944136: return getQueryElement(); 2065 case -1335224239: return addDetail(); 2066 case 92750597: return addAgent(); 2067 default: return super.makeProperty(hash, name); 2068 } 2069 2070 } 2071 2072 @Override 2073 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2074 switch (hash) { 2075 case 3648196: /*what*/ return new String[] {"Reference"}; 2076 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2077 case -722296940: /*securityLabel*/ return new String[] {"CodeableConcept"}; 2078 case 107944136: /*query*/ return new String[] {"base64Binary"}; 2079 case -1335224239: /*detail*/ return new String[] {}; 2080 case 92750597: /*agent*/ return new String[] {"@AuditEvent.agent"}; 2081 default: return super.getTypesForProperty(hash, name); 2082 } 2083 2084 } 2085 2086 @Override 2087 public Base addChild(String name) throws FHIRException { 2088 if (name.equals("what")) { 2089 this.what = new Reference(); 2090 return this.what; 2091 } 2092 else if (name.equals("role")) { 2093 this.role = new CodeableConcept(); 2094 return this.role; 2095 } 2096 else if (name.equals("securityLabel")) { 2097 return addSecurityLabel(); 2098 } 2099 else if (name.equals("query")) { 2100 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.entity.query"); 2101 } 2102 else if (name.equals("detail")) { 2103 return addDetail(); 2104 } 2105 else if (name.equals("agent")) { 2106 return addAgent(); 2107 } 2108 else 2109 return super.addChild(name); 2110 } 2111 2112 public AuditEventEntityComponent copy() { 2113 AuditEventEntityComponent dst = new AuditEventEntityComponent(); 2114 copyValues(dst); 2115 return dst; 2116 } 2117 2118 public void copyValues(AuditEventEntityComponent dst) { 2119 super.copyValues(dst); 2120 dst.what = what == null ? null : what.copy(); 2121 dst.role = role == null ? null : role.copy(); 2122 if (securityLabel != null) { 2123 dst.securityLabel = new ArrayList<CodeableConcept>(); 2124 for (CodeableConcept i : securityLabel) 2125 dst.securityLabel.add(i.copy()); 2126 }; 2127 dst.query = query == null ? null : query.copy(); 2128 if (detail != null) { 2129 dst.detail = new ArrayList<AuditEventEntityDetailComponent>(); 2130 for (AuditEventEntityDetailComponent i : detail) 2131 dst.detail.add(i.copy()); 2132 }; 2133 if (agent != null) { 2134 dst.agent = new ArrayList<AuditEventAgentComponent>(); 2135 for (AuditEventAgentComponent i : agent) 2136 dst.agent.add(i.copy()); 2137 }; 2138 } 2139 2140 @Override 2141 public boolean equalsDeep(Base other_) { 2142 if (!super.equalsDeep(other_)) 2143 return false; 2144 if (!(other_ instanceof AuditEventEntityComponent)) 2145 return false; 2146 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 2147 return compareDeep(what, o.what, true) && compareDeep(role, o.role, true) && compareDeep(securityLabel, o.securityLabel, true) 2148 && compareDeep(query, o.query, true) && compareDeep(detail, o.detail, true) && compareDeep(agent, o.agent, true) 2149 ; 2150 } 2151 2152 @Override 2153 public boolean equalsShallow(Base other_) { 2154 if (!super.equalsShallow(other_)) 2155 return false; 2156 if (!(other_ instanceof AuditEventEntityComponent)) 2157 return false; 2158 AuditEventEntityComponent o = (AuditEventEntityComponent) other_; 2159 return compareValues(query, o.query, true); 2160 } 2161 2162 public boolean isEmpty() { 2163 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(what, role, securityLabel 2164 , query, detail, agent); 2165 } 2166 2167 public String fhirType() { 2168 return "AuditEvent.entity"; 2169 2170 } 2171 2172 } 2173 2174 @Block() 2175 public static class AuditEventEntityDetailComponent extends BackboneElement implements IBaseBackboneElement { 2176 /** 2177 * The type of extra detail provided in the value. 2178 */ 2179 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2180 @Description(shortDefinition="Name of the property", formalDefinition="The type of extra detail provided in the value." ) 2181 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-type") 2182 protected CodeableConcept type; 2183 2184 /** 2185 * The value of the extra detail. 2186 */ 2187 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Ratio.class, TimeType.class, DateTimeType.class, Period.class, Base64BinaryType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2188 @Description(shortDefinition="Property value", formalDefinition="The value of the extra detail." ) 2189 protected DataType value; 2190 2191 private static final long serialVersionUID = -1659186716L; 2192 2193 /** 2194 * Constructor 2195 */ 2196 public AuditEventEntityDetailComponent() { 2197 super(); 2198 } 2199 2200 /** 2201 * Constructor 2202 */ 2203 public AuditEventEntityDetailComponent(CodeableConcept type, DataType value) { 2204 super(); 2205 this.setType(type); 2206 this.setValue(value); 2207 } 2208 2209 /** 2210 * @return {@link #type} (The type of extra detail provided in the value.) 2211 */ 2212 public CodeableConcept getType() { 2213 if (this.type == null) 2214 if (Configuration.errorOnAutoCreate()) 2215 throw new Error("Attempt to auto-create AuditEventEntityDetailComponent.type"); 2216 else if (Configuration.doAutoCreate()) 2217 this.type = new CodeableConcept(); // cc 2218 return this.type; 2219 } 2220 2221 public boolean hasType() { 2222 return this.type != null && !this.type.isEmpty(); 2223 } 2224 2225 /** 2226 * @param value {@link #type} (The type of extra detail provided in the value.) 2227 */ 2228 public AuditEventEntityDetailComponent setType(CodeableConcept value) { 2229 this.type = value; 2230 return this; 2231 } 2232 2233 /** 2234 * @return {@link #value} (The value of the extra detail.) 2235 */ 2236 public DataType getValue() { 2237 return this.value; 2238 } 2239 2240 /** 2241 * @return {@link #value} (The value of the extra detail.) 2242 */ 2243 public Quantity getValueQuantity() throws FHIRException { 2244 if (this.value == null) 2245 this.value = new Quantity(); 2246 if (!(this.value instanceof Quantity)) 2247 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2248 return (Quantity) this.value; 2249 } 2250 2251 public boolean hasValueQuantity() { 2252 return this.value instanceof Quantity; 2253 } 2254 2255 /** 2256 * @return {@link #value} (The value of the extra detail.) 2257 */ 2258 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2259 if (this.value == null) 2260 this.value = new CodeableConcept(); 2261 if (!(this.value instanceof CodeableConcept)) 2262 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2263 return (CodeableConcept) this.value; 2264 } 2265 2266 public boolean hasValueCodeableConcept() { 2267 return this.value instanceof CodeableConcept; 2268 } 2269 2270 /** 2271 * @return {@link #value} (The value of the extra detail.) 2272 */ 2273 public StringType getValueStringType() throws FHIRException { 2274 if (this.value == null) 2275 this.value = new StringType(); 2276 if (!(this.value instanceof StringType)) 2277 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2278 return (StringType) this.value; 2279 } 2280 2281 public boolean hasValueStringType() { 2282 return this.value instanceof StringType; 2283 } 2284 2285 /** 2286 * @return {@link #value} (The value of the extra detail.) 2287 */ 2288 public BooleanType getValueBooleanType() throws FHIRException { 2289 if (this.value == null) 2290 this.value = new BooleanType(); 2291 if (!(this.value instanceof BooleanType)) 2292 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2293 return (BooleanType) this.value; 2294 } 2295 2296 public boolean hasValueBooleanType() { 2297 return this.value instanceof BooleanType; 2298 } 2299 2300 /** 2301 * @return {@link #value} (The value of the extra detail.) 2302 */ 2303 public IntegerType getValueIntegerType() throws FHIRException { 2304 if (this.value == null) 2305 this.value = new IntegerType(); 2306 if (!(this.value instanceof IntegerType)) 2307 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2308 return (IntegerType) this.value; 2309 } 2310 2311 public boolean hasValueIntegerType() { 2312 return this.value instanceof IntegerType; 2313 } 2314 2315 /** 2316 * @return {@link #value} (The value of the extra detail.) 2317 */ 2318 public Range getValueRange() throws FHIRException { 2319 if (this.value == null) 2320 this.value = new Range(); 2321 if (!(this.value instanceof Range)) 2322 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2323 return (Range) this.value; 2324 } 2325 2326 public boolean hasValueRange() { 2327 return this.value instanceof Range; 2328 } 2329 2330 /** 2331 * @return {@link #value} (The value of the extra detail.) 2332 */ 2333 public Ratio getValueRatio() throws FHIRException { 2334 if (this.value == null) 2335 this.value = new Ratio(); 2336 if (!(this.value instanceof Ratio)) 2337 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 2338 return (Ratio) this.value; 2339 } 2340 2341 public boolean hasValueRatio() { 2342 return this.value instanceof Ratio; 2343 } 2344 2345 /** 2346 * @return {@link #value} (The value of the extra detail.) 2347 */ 2348 public TimeType getValueTimeType() throws FHIRException { 2349 if (this.value == null) 2350 this.value = new TimeType(); 2351 if (!(this.value instanceof TimeType)) 2352 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2353 return (TimeType) this.value; 2354 } 2355 2356 public boolean hasValueTimeType() { 2357 return this.value instanceof TimeType; 2358 } 2359 2360 /** 2361 * @return {@link #value} (The value of the extra detail.) 2362 */ 2363 public DateTimeType getValueDateTimeType() throws FHIRException { 2364 if (this.value == null) 2365 this.value = new DateTimeType(); 2366 if (!(this.value instanceof DateTimeType)) 2367 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2368 return (DateTimeType) this.value; 2369 } 2370 2371 public boolean hasValueDateTimeType() { 2372 return this.value instanceof DateTimeType; 2373 } 2374 2375 /** 2376 * @return {@link #value} (The value of the extra detail.) 2377 */ 2378 public Period getValuePeriod() throws FHIRException { 2379 if (this.value == null) 2380 this.value = new Period(); 2381 if (!(this.value instanceof Period)) 2382 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 2383 return (Period) this.value; 2384 } 2385 2386 public boolean hasValuePeriod() { 2387 return this.value instanceof Period; 2388 } 2389 2390 /** 2391 * @return {@link #value} (The value of the extra detail.) 2392 */ 2393 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 2394 if (this.value == null) 2395 this.value = new Base64BinaryType(); 2396 if (!(this.value instanceof Base64BinaryType)) 2397 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 2398 return (Base64BinaryType) this.value; 2399 } 2400 2401 public boolean hasValueBase64BinaryType() { 2402 return this.value instanceof Base64BinaryType; 2403 } 2404 2405 public boolean hasValue() { 2406 return this.value != null && !this.value.isEmpty(); 2407 } 2408 2409 /** 2410 * @param value {@link #value} (The value of the extra detail.) 2411 */ 2412 public AuditEventEntityDetailComponent setValue(DataType value) { 2413 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Ratio || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period || value instanceof Base64BinaryType)) 2414 throw new FHIRException("Not the right type for AuditEvent.entity.detail.value[x]: "+value.fhirType()); 2415 this.value = value; 2416 return this; 2417 } 2418 2419 protected void listChildren(List<Property> children) { 2420 super.listChildren(children); 2421 children.add(new Property("type", "CodeableConcept", "The type of extra detail provided in the value.", 0, 1, type)); 2422 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|time|dateTime|Period|base64Binary", "The value of the extra detail.", 0, 1, value)); 2423 } 2424 2425 @Override 2426 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2427 switch (_hash) { 2428 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of extra detail provided in the value.", 0, 1, type); 2429 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|time|dateTime|Period|base64Binary", "The value of the extra detail.", 0, 1, value); 2430 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|time|dateTime|Period|base64Binary", "The value of the extra detail.", 0, 1, value); 2431 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the extra detail.", 0, 1, value); 2432 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the extra detail.", 0, 1, value); 2433 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the extra detail.", 0, 1, value); 2434 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the extra detail.", 0, 1, value); 2435 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the extra detail.", 0, 1, value); 2436 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the extra detail.", 0, 1, value); 2437 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the extra detail.", 0, 1, value); 2438 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The value of the extra detail.", 0, 1, value); 2439 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the extra detail.", 0, 1, value); 2440 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the extra detail.", 0, 1, value); 2441 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The value of the extra detail.", 0, 1, value); 2442 default: return super.getNamedProperty(_hash, _name, _checkValid); 2443 } 2444 2445 } 2446 2447 @Override 2448 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2449 switch (hash) { 2450 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2451 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2452 default: return super.getProperty(hash, name, checkValid); 2453 } 2454 2455 } 2456 2457 @Override 2458 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2459 switch (hash) { 2460 case 3575610: // type 2461 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2462 return value; 2463 case 111972721: // value 2464 this.value = TypeConvertor.castToType(value); // DataType 2465 return value; 2466 default: return super.setProperty(hash, name, value); 2467 } 2468 2469 } 2470 2471 @Override 2472 public Base setProperty(String name, Base value) throws FHIRException { 2473 if (name.equals("type")) { 2474 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2475 } else if (name.equals("value[x]")) { 2476 this.value = TypeConvertor.castToType(value); // DataType 2477 } else 2478 return super.setProperty(name, value); 2479 return value; 2480 } 2481 2482 @Override 2483 public void removeChild(String name, Base value) throws FHIRException { 2484 if (name.equals("type")) { 2485 this.type = null; 2486 } else if (name.equals("value[x]")) { 2487 this.value = null; 2488 } else 2489 super.removeChild(name, value); 2490 2491 } 2492 2493 @Override 2494 public Base makeProperty(int hash, String name) throws FHIRException { 2495 switch (hash) { 2496 case 3575610: return getType(); 2497 case -1410166417: return getValue(); 2498 case 111972721: return getValue(); 2499 default: return super.makeProperty(hash, name); 2500 } 2501 2502 } 2503 2504 @Override 2505 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2506 switch (hash) { 2507 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2508 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", "time", "dateTime", "Period", "base64Binary"}; 2509 default: return super.getTypesForProperty(hash, name); 2510 } 2511 2512 } 2513 2514 @Override 2515 public Base addChild(String name) throws FHIRException { 2516 if (name.equals("type")) { 2517 this.type = new CodeableConcept(); 2518 return this.type; 2519 } 2520 else if (name.equals("valueQuantity")) { 2521 this.value = new Quantity(); 2522 return this.value; 2523 } 2524 else if (name.equals("valueCodeableConcept")) { 2525 this.value = new CodeableConcept(); 2526 return this.value; 2527 } 2528 else if (name.equals("valueString")) { 2529 this.value = new StringType(); 2530 return this.value; 2531 } 2532 else if (name.equals("valueBoolean")) { 2533 this.value = new BooleanType(); 2534 return this.value; 2535 } 2536 else if (name.equals("valueInteger")) { 2537 this.value = new IntegerType(); 2538 return this.value; 2539 } 2540 else if (name.equals("valueRange")) { 2541 this.value = new Range(); 2542 return this.value; 2543 } 2544 else if (name.equals("valueRatio")) { 2545 this.value = new Ratio(); 2546 return this.value; 2547 } 2548 else if (name.equals("valueTime")) { 2549 this.value = new TimeType(); 2550 return this.value; 2551 } 2552 else if (name.equals("valueDateTime")) { 2553 this.value = new DateTimeType(); 2554 return this.value; 2555 } 2556 else if (name.equals("valuePeriod")) { 2557 this.value = new Period(); 2558 return this.value; 2559 } 2560 else if (name.equals("valueBase64Binary")) { 2561 this.value = new Base64BinaryType(); 2562 return this.value; 2563 } 2564 else 2565 return super.addChild(name); 2566 } 2567 2568 public AuditEventEntityDetailComponent copy() { 2569 AuditEventEntityDetailComponent dst = new AuditEventEntityDetailComponent(); 2570 copyValues(dst); 2571 return dst; 2572 } 2573 2574 public void copyValues(AuditEventEntityDetailComponent dst) { 2575 super.copyValues(dst); 2576 dst.type = type == null ? null : type.copy(); 2577 dst.value = value == null ? null : value.copy(); 2578 } 2579 2580 @Override 2581 public boolean equalsDeep(Base other_) { 2582 if (!super.equalsDeep(other_)) 2583 return false; 2584 if (!(other_ instanceof AuditEventEntityDetailComponent)) 2585 return false; 2586 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 2587 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2588 } 2589 2590 @Override 2591 public boolean equalsShallow(Base other_) { 2592 if (!super.equalsShallow(other_)) 2593 return false; 2594 if (!(other_ instanceof AuditEventEntityDetailComponent)) 2595 return false; 2596 AuditEventEntityDetailComponent o = (AuditEventEntityDetailComponent) other_; 2597 return true; 2598 } 2599 2600 public boolean isEmpty() { 2601 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2602 } 2603 2604 public String fhirType() { 2605 return "AuditEvent.entity.detail"; 2606 2607 } 2608 2609 } 2610 2611 /** 2612 * Classification of the type of event. 2613 */ 2614 @Child(name = "category", type = {CodeableConcept.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2615 @Description(shortDefinition="Type/identifier of event", formalDefinition="Classification of the type of event." ) 2616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-type") 2617 protected List<CodeableConcept> category; 2618 2619 /** 2620 * Describes what happened. The most specific code for the event. 2621 */ 2622 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 2623 @Description(shortDefinition="Specific type of event", formalDefinition="Describes what happened. The most specific code for the event." ) 2624 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-sub-type") 2625 protected CodeableConcept code; 2626 2627 /** 2628 * Indicator for type of action performed during the event that generated the audit. 2629 */ 2630 @Child(name = "action", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2631 @Description(shortDefinition="Type of action performed during the event", formalDefinition="Indicator for type of action performed during the event that generated the audit." ) 2632 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-action") 2633 protected Enumeration<AuditEventAction> action; 2634 2635 /** 2636 * Indicates and enables segmentation of various severity including debugging from critical. 2637 */ 2638 @Child(name = "severity", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2639 @Description(shortDefinition="emergency | alert | critical | error | warning | notice | informational | debug", formalDefinition="Indicates and enables segmentation of various severity including debugging from critical." ) 2640 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/audit-event-severity") 2641 protected Enumeration<AuditEventSeverity> severity; 2642 2643 /** 2644 * The time or period during which the activity occurred. 2645 */ 2646 @Child(name = "occurred", type = {Period.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2647 @Description(shortDefinition="When the activity occurred", formalDefinition="The time or period during which the activity occurred." ) 2648 protected DataType occurred; 2649 2650 /** 2651 * The time when the event was recorded. 2652 */ 2653 @Child(name = "recorded", type = {InstantType.class}, order=5, min=1, max=1, modifier=false, summary=true) 2654 @Description(shortDefinition="Time when the event was recorded", formalDefinition="The time when the event was recorded." ) 2655 protected InstantType recorded; 2656 2657 /** 2658 * Indicates whether the event succeeded or failed. A free text descripiton can be given in outcome.text. 2659 */ 2660 @Child(name = "outcome", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 2661 @Description(shortDefinition="Whether the event succeeded or failed", formalDefinition="Indicates whether the event succeeded or failed. A free text descripiton can be given in outcome.text." ) 2662 protected AuditEventOutcomeComponent outcome; 2663 2664 /** 2665 * The authorization (e.g., PurposeOfUse) that was used during the event being recorded. 2666 */ 2667 @Child(name = "authorization", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2668 @Description(shortDefinition="Authorization related to the event", formalDefinition="The authorization (e.g., PurposeOfUse) that was used during the event being recorded." ) 2669 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 2670 protected List<CodeableConcept> authorization; 2671 2672 /** 2673 * Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon. 2674 */ 2675 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, Task.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2676 @Description(shortDefinition="Workflow authorization within which this event occurred", formalDefinition="Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon." ) 2677 protected List<Reference> basedOn; 2678 2679 /** 2680 * The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity. 2681 */ 2682 @Child(name = "patient", type = {Patient.class}, order=9, min=0, max=1, modifier=false, summary=false) 2683 @Description(shortDefinition="The patient is the subject of the data used/created/updated/deleted during the activity", formalDefinition="The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity." ) 2684 protected Reference patient; 2685 2686 /** 2687 * This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests). 2688 */ 2689 @Child(name = "encounter", type = {Encounter.class}, order=10, min=0, max=1, modifier=false, summary=false) 2690 @Description(shortDefinition="Encounter within which this event occurred or which the event is tightly associated", formalDefinition="This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests)." ) 2691 protected Reference encounter; 2692 2693 /** 2694 * An actor taking an active role in the event or activity that is logged. 2695 */ 2696 @Child(name = "agent", type = {}, order=11, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2697 @Description(shortDefinition="Actor involved in the event", formalDefinition="An actor taking an active role in the event or activity that is logged." ) 2698 protected List<AuditEventAgentComponent> agent; 2699 2700 /** 2701 * The actor that is reporting the event. 2702 */ 2703 @Child(name = "source", type = {}, order=12, min=1, max=1, modifier=false, summary=true) 2704 @Description(shortDefinition="Audit Event Reporter", formalDefinition="The actor that is reporting the event." ) 2705 protected AuditEventSourceComponent source; 2706 2707 /** 2708 * Specific instances of data or objects that have been accessed. 2709 */ 2710 @Child(name = "entity", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2711 @Description(shortDefinition="Data or objects used", formalDefinition="Specific instances of data or objects that have been accessed." ) 2712 protected List<AuditEventEntityComponent> entity; 2713 2714 private static final long serialVersionUID = -1335832480L; 2715 2716 /** 2717 * Constructor 2718 */ 2719 public AuditEvent() { 2720 super(); 2721 } 2722 2723 /** 2724 * Constructor 2725 */ 2726 public AuditEvent(CodeableConcept code, Date recorded, AuditEventAgentComponent agent, AuditEventSourceComponent source) { 2727 super(); 2728 this.setCode(code); 2729 this.setRecorded(recorded); 2730 this.addAgent(agent); 2731 this.setSource(source); 2732 } 2733 2734 /** 2735 * @return {@link #category} (Classification of the type of event.) 2736 */ 2737 public List<CodeableConcept> getCategory() { 2738 if (this.category == null) 2739 this.category = new ArrayList<CodeableConcept>(); 2740 return this.category; 2741 } 2742 2743 /** 2744 * @return Returns a reference to <code>this</code> for easy method chaining 2745 */ 2746 public AuditEvent setCategory(List<CodeableConcept> theCategory) { 2747 this.category = theCategory; 2748 return this; 2749 } 2750 2751 public boolean hasCategory() { 2752 if (this.category == null) 2753 return false; 2754 for (CodeableConcept item : this.category) 2755 if (!item.isEmpty()) 2756 return true; 2757 return false; 2758 } 2759 2760 public CodeableConcept addCategory() { //3 2761 CodeableConcept t = new CodeableConcept(); 2762 if (this.category == null) 2763 this.category = new ArrayList<CodeableConcept>(); 2764 this.category.add(t); 2765 return t; 2766 } 2767 2768 public AuditEvent addCategory(CodeableConcept t) { //3 2769 if (t == null) 2770 return this; 2771 if (this.category == null) 2772 this.category = new ArrayList<CodeableConcept>(); 2773 this.category.add(t); 2774 return this; 2775 } 2776 2777 /** 2778 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2779 */ 2780 public CodeableConcept getCategoryFirstRep() { 2781 if (getCategory().isEmpty()) { 2782 addCategory(); 2783 } 2784 return getCategory().get(0); 2785 } 2786 2787 /** 2788 * @return {@link #code} (Describes what happened. The most specific code for the event.) 2789 */ 2790 public CodeableConcept getCode() { 2791 if (this.code == null) 2792 if (Configuration.errorOnAutoCreate()) 2793 throw new Error("Attempt to auto-create AuditEvent.code"); 2794 else if (Configuration.doAutoCreate()) 2795 this.code = new CodeableConcept(); // cc 2796 return this.code; 2797 } 2798 2799 public boolean hasCode() { 2800 return this.code != null && !this.code.isEmpty(); 2801 } 2802 2803 /** 2804 * @param value {@link #code} (Describes what happened. The most specific code for the event.) 2805 */ 2806 public AuditEvent setCode(CodeableConcept value) { 2807 this.code = value; 2808 return this; 2809 } 2810 2811 /** 2812 * @return {@link #action} (Indicator for type of action performed during the event that generated the audit.). This is the underlying object with id, value and extensions. The accessor "getAction" gives direct access to the value 2813 */ 2814 public Enumeration<AuditEventAction> getActionElement() { 2815 if (this.action == null) 2816 if (Configuration.errorOnAutoCreate()) 2817 throw new Error("Attempt to auto-create AuditEvent.action"); 2818 else if (Configuration.doAutoCreate()) 2819 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); // bb 2820 return this.action; 2821 } 2822 2823 public boolean hasActionElement() { 2824 return this.action != null && !this.action.isEmpty(); 2825 } 2826 2827 public boolean hasAction() { 2828 return this.action != null && !this.action.isEmpty(); 2829 } 2830 2831 /** 2832 * @param value {@link #action} (Indicator for type of action performed during the event that generated the audit.). This is the underlying object with id, value and extensions. The accessor "getAction" gives direct access to the value 2833 */ 2834 public AuditEvent setActionElement(Enumeration<AuditEventAction> value) { 2835 this.action = value; 2836 return this; 2837 } 2838 2839 /** 2840 * @return Indicator for type of action performed during the event that generated the audit. 2841 */ 2842 public AuditEventAction getAction() { 2843 return this.action == null ? null : this.action.getValue(); 2844 } 2845 2846 /** 2847 * @param value Indicator for type of action performed during the event that generated the audit. 2848 */ 2849 public AuditEvent setAction(AuditEventAction value) { 2850 if (value == null) 2851 this.action = null; 2852 else { 2853 if (this.action == null) 2854 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); 2855 this.action.setValue(value); 2856 } 2857 return this; 2858 } 2859 2860 /** 2861 * @return {@link #severity} (Indicates and enables segmentation of various severity including debugging from critical.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 2862 */ 2863 public Enumeration<AuditEventSeverity> getSeverityElement() { 2864 if (this.severity == null) 2865 if (Configuration.errorOnAutoCreate()) 2866 throw new Error("Attempt to auto-create AuditEvent.severity"); 2867 else if (Configuration.doAutoCreate()) 2868 this.severity = new Enumeration<AuditEventSeverity>(new AuditEventSeverityEnumFactory()); // bb 2869 return this.severity; 2870 } 2871 2872 public boolean hasSeverityElement() { 2873 return this.severity != null && !this.severity.isEmpty(); 2874 } 2875 2876 public boolean hasSeverity() { 2877 return this.severity != null && !this.severity.isEmpty(); 2878 } 2879 2880 /** 2881 * @param value {@link #severity} (Indicates and enables segmentation of various severity including debugging from critical.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 2882 */ 2883 public AuditEvent setSeverityElement(Enumeration<AuditEventSeverity> value) { 2884 this.severity = value; 2885 return this; 2886 } 2887 2888 /** 2889 * @return Indicates and enables segmentation of various severity including debugging from critical. 2890 */ 2891 public AuditEventSeverity getSeverity() { 2892 return this.severity == null ? null : this.severity.getValue(); 2893 } 2894 2895 /** 2896 * @param value Indicates and enables segmentation of various severity including debugging from critical. 2897 */ 2898 public AuditEvent setSeverity(AuditEventSeverity value) { 2899 if (value == null) 2900 this.severity = null; 2901 else { 2902 if (this.severity == null) 2903 this.severity = new Enumeration<AuditEventSeverity>(new AuditEventSeverityEnumFactory()); 2904 this.severity.setValue(value); 2905 } 2906 return this; 2907 } 2908 2909 /** 2910 * @return {@link #occurred} (The time or period during which the activity occurred.) 2911 */ 2912 public DataType getOccurred() { 2913 return this.occurred; 2914 } 2915 2916 /** 2917 * @return {@link #occurred} (The time or period during which the activity occurred.) 2918 */ 2919 public Period getOccurredPeriod() throws FHIRException { 2920 if (this.occurred == null) 2921 this.occurred = new Period(); 2922 if (!(this.occurred instanceof Period)) 2923 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurred.getClass().getName()+" was encountered"); 2924 return (Period) this.occurred; 2925 } 2926 2927 public boolean hasOccurredPeriod() { 2928 return this.occurred instanceof Period; 2929 } 2930 2931 /** 2932 * @return {@link #occurred} (The time or period during which the activity occurred.) 2933 */ 2934 public DateTimeType getOccurredDateTimeType() throws FHIRException { 2935 if (this.occurred == null) 2936 this.occurred = new DateTimeType(); 2937 if (!(this.occurred instanceof DateTimeType)) 2938 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurred.getClass().getName()+" was encountered"); 2939 return (DateTimeType) this.occurred; 2940 } 2941 2942 public boolean hasOccurredDateTimeType() { 2943 return this.occurred instanceof DateTimeType; 2944 } 2945 2946 public boolean hasOccurred() { 2947 return this.occurred != null && !this.occurred.isEmpty(); 2948 } 2949 2950 /** 2951 * @param value {@link #occurred} (The time or period during which the activity occurred.) 2952 */ 2953 public AuditEvent setOccurred(DataType value) { 2954 if (value != null && !(value instanceof Period || value instanceof DateTimeType)) 2955 throw new FHIRException("Not the right type for AuditEvent.occurred[x]: "+value.fhirType()); 2956 this.occurred = value; 2957 return this; 2958 } 2959 2960 /** 2961 * @return {@link #recorded} (The time when the event was recorded.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 2962 */ 2963 public InstantType getRecordedElement() { 2964 if (this.recorded == null) 2965 if (Configuration.errorOnAutoCreate()) 2966 throw new Error("Attempt to auto-create AuditEvent.recorded"); 2967 else if (Configuration.doAutoCreate()) 2968 this.recorded = new InstantType(); // bb 2969 return this.recorded; 2970 } 2971 2972 public boolean hasRecordedElement() { 2973 return this.recorded != null && !this.recorded.isEmpty(); 2974 } 2975 2976 public boolean hasRecorded() { 2977 return this.recorded != null && !this.recorded.isEmpty(); 2978 } 2979 2980 /** 2981 * @param value {@link #recorded} (The time when the event was recorded.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 2982 */ 2983 public AuditEvent setRecordedElement(InstantType value) { 2984 this.recorded = value; 2985 return this; 2986 } 2987 2988 /** 2989 * @return The time when the event was recorded. 2990 */ 2991 public Date getRecorded() { 2992 return this.recorded == null ? null : this.recorded.getValue(); 2993 } 2994 2995 /** 2996 * @param value The time when the event was recorded. 2997 */ 2998 public AuditEvent setRecorded(Date value) { 2999 if (this.recorded == null) 3000 this.recorded = new InstantType(); 3001 this.recorded.setValue(value); 3002 return this; 3003 } 3004 3005 /** 3006 * @return {@link #outcome} (Indicates whether the event succeeded or failed. A free text descripiton can be given in outcome.text.) 3007 */ 3008 public AuditEventOutcomeComponent getOutcome() { 3009 if (this.outcome == null) 3010 if (Configuration.errorOnAutoCreate()) 3011 throw new Error("Attempt to auto-create AuditEvent.outcome"); 3012 else if (Configuration.doAutoCreate()) 3013 this.outcome = new AuditEventOutcomeComponent(); // cc 3014 return this.outcome; 3015 } 3016 3017 public boolean hasOutcome() { 3018 return this.outcome != null && !this.outcome.isEmpty(); 3019 } 3020 3021 /** 3022 * @param value {@link #outcome} (Indicates whether the event succeeded or failed. A free text descripiton can be given in outcome.text.) 3023 */ 3024 public AuditEvent setOutcome(AuditEventOutcomeComponent value) { 3025 this.outcome = value; 3026 return this; 3027 } 3028 3029 /** 3030 * @return {@link #authorization} (The authorization (e.g., PurposeOfUse) that was used during the event being recorded.) 3031 */ 3032 public List<CodeableConcept> getAuthorization() { 3033 if (this.authorization == null) 3034 this.authorization = new ArrayList<CodeableConcept>(); 3035 return this.authorization; 3036 } 3037 3038 /** 3039 * @return Returns a reference to <code>this</code> for easy method chaining 3040 */ 3041 public AuditEvent setAuthorization(List<CodeableConcept> theAuthorization) { 3042 this.authorization = theAuthorization; 3043 return this; 3044 } 3045 3046 public boolean hasAuthorization() { 3047 if (this.authorization == null) 3048 return false; 3049 for (CodeableConcept item : this.authorization) 3050 if (!item.isEmpty()) 3051 return true; 3052 return false; 3053 } 3054 3055 public CodeableConcept addAuthorization() { //3 3056 CodeableConcept t = new CodeableConcept(); 3057 if (this.authorization == null) 3058 this.authorization = new ArrayList<CodeableConcept>(); 3059 this.authorization.add(t); 3060 return t; 3061 } 3062 3063 public AuditEvent addAuthorization(CodeableConcept t) { //3 3064 if (t == null) 3065 return this; 3066 if (this.authorization == null) 3067 this.authorization = new ArrayList<CodeableConcept>(); 3068 this.authorization.add(t); 3069 return this; 3070 } 3071 3072 /** 3073 * @return The first repetition of repeating field {@link #authorization}, creating it if it does not already exist {3} 3074 */ 3075 public CodeableConcept getAuthorizationFirstRep() { 3076 if (getAuthorization().isEmpty()) { 3077 addAuthorization(); 3078 } 3079 return getAuthorization().get(0); 3080 } 3081 3082 /** 3083 * @return {@link #basedOn} (Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon.) 3084 */ 3085 public List<Reference> getBasedOn() { 3086 if (this.basedOn == null) 3087 this.basedOn = new ArrayList<Reference>(); 3088 return this.basedOn; 3089 } 3090 3091 /** 3092 * @return Returns a reference to <code>this</code> for easy method chaining 3093 */ 3094 public AuditEvent setBasedOn(List<Reference> theBasedOn) { 3095 this.basedOn = theBasedOn; 3096 return this; 3097 } 3098 3099 public boolean hasBasedOn() { 3100 if (this.basedOn == null) 3101 return false; 3102 for (Reference item : this.basedOn) 3103 if (!item.isEmpty()) 3104 return true; 3105 return false; 3106 } 3107 3108 public Reference addBasedOn() { //3 3109 Reference t = new Reference(); 3110 if (this.basedOn == null) 3111 this.basedOn = new ArrayList<Reference>(); 3112 this.basedOn.add(t); 3113 return t; 3114 } 3115 3116 public AuditEvent addBasedOn(Reference t) { //3 3117 if (t == null) 3118 return this; 3119 if (this.basedOn == null) 3120 this.basedOn = new ArrayList<Reference>(); 3121 this.basedOn.add(t); 3122 return this; 3123 } 3124 3125 /** 3126 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 3127 */ 3128 public Reference getBasedOnFirstRep() { 3129 if (getBasedOn().isEmpty()) { 3130 addBasedOn(); 3131 } 3132 return getBasedOn().get(0); 3133 } 3134 3135 /** 3136 * @return {@link #patient} (The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.) 3137 */ 3138 public Reference getPatient() { 3139 if (this.patient == null) 3140 if (Configuration.errorOnAutoCreate()) 3141 throw new Error("Attempt to auto-create AuditEvent.patient"); 3142 else if (Configuration.doAutoCreate()) 3143 this.patient = new Reference(); // cc 3144 return this.patient; 3145 } 3146 3147 public boolean hasPatient() { 3148 return this.patient != null && !this.patient.isEmpty(); 3149 } 3150 3151 /** 3152 * @param value {@link #patient} (The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.) 3153 */ 3154 public AuditEvent setPatient(Reference value) { 3155 this.patient = value; 3156 return this; 3157 } 3158 3159 /** 3160 * @return {@link #encounter} (This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).) 3161 */ 3162 public Reference getEncounter() { 3163 if (this.encounter == null) 3164 if (Configuration.errorOnAutoCreate()) 3165 throw new Error("Attempt to auto-create AuditEvent.encounter"); 3166 else if (Configuration.doAutoCreate()) 3167 this.encounter = new Reference(); // cc 3168 return this.encounter; 3169 } 3170 3171 public boolean hasEncounter() { 3172 return this.encounter != null && !this.encounter.isEmpty(); 3173 } 3174 3175 /** 3176 * @param value {@link #encounter} (This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).) 3177 */ 3178 public AuditEvent setEncounter(Reference value) { 3179 this.encounter = value; 3180 return this; 3181 } 3182 3183 /** 3184 * @return {@link #agent} (An actor taking an active role in the event or activity that is logged.) 3185 */ 3186 public List<AuditEventAgentComponent> getAgent() { 3187 if (this.agent == null) 3188 this.agent = new ArrayList<AuditEventAgentComponent>(); 3189 return this.agent; 3190 } 3191 3192 /** 3193 * @return Returns a reference to <code>this</code> for easy method chaining 3194 */ 3195 public AuditEvent setAgent(List<AuditEventAgentComponent> theAgent) { 3196 this.agent = theAgent; 3197 return this; 3198 } 3199 3200 public boolean hasAgent() { 3201 if (this.agent == null) 3202 return false; 3203 for (AuditEventAgentComponent item : this.agent) 3204 if (!item.isEmpty()) 3205 return true; 3206 return false; 3207 } 3208 3209 public AuditEventAgentComponent addAgent() { //3 3210 AuditEventAgentComponent t = new AuditEventAgentComponent(); 3211 if (this.agent == null) 3212 this.agent = new ArrayList<AuditEventAgentComponent>(); 3213 this.agent.add(t); 3214 return t; 3215 } 3216 3217 public AuditEvent addAgent(AuditEventAgentComponent t) { //3 3218 if (t == null) 3219 return this; 3220 if (this.agent == null) 3221 this.agent = new ArrayList<AuditEventAgentComponent>(); 3222 this.agent.add(t); 3223 return this; 3224 } 3225 3226 /** 3227 * @return The first repetition of repeating field {@link #agent}, creating it if it does not already exist {3} 3228 */ 3229 public AuditEventAgentComponent getAgentFirstRep() { 3230 if (getAgent().isEmpty()) { 3231 addAgent(); 3232 } 3233 return getAgent().get(0); 3234 } 3235 3236 /** 3237 * @return {@link #source} (The actor that is reporting the event.) 3238 */ 3239 public AuditEventSourceComponent getSource() { 3240 if (this.source == null) 3241 if (Configuration.errorOnAutoCreate()) 3242 throw new Error("Attempt to auto-create AuditEvent.source"); 3243 else if (Configuration.doAutoCreate()) 3244 this.source = new AuditEventSourceComponent(); // cc 3245 return this.source; 3246 } 3247 3248 public boolean hasSource() { 3249 return this.source != null && !this.source.isEmpty(); 3250 } 3251 3252 /** 3253 * @param value {@link #source} (The actor that is reporting the event.) 3254 */ 3255 public AuditEvent setSource(AuditEventSourceComponent value) { 3256 this.source = value; 3257 return this; 3258 } 3259 3260 /** 3261 * @return {@link #entity} (Specific instances of data or objects that have been accessed.) 3262 */ 3263 public List<AuditEventEntityComponent> getEntity() { 3264 if (this.entity == null) 3265 this.entity = new ArrayList<AuditEventEntityComponent>(); 3266 return this.entity; 3267 } 3268 3269 /** 3270 * @return Returns a reference to <code>this</code> for easy method chaining 3271 */ 3272 public AuditEvent setEntity(List<AuditEventEntityComponent> theEntity) { 3273 this.entity = theEntity; 3274 return this; 3275 } 3276 3277 public boolean hasEntity() { 3278 if (this.entity == null) 3279 return false; 3280 for (AuditEventEntityComponent item : this.entity) 3281 if (!item.isEmpty()) 3282 return true; 3283 return false; 3284 } 3285 3286 public AuditEventEntityComponent addEntity() { //3 3287 AuditEventEntityComponent t = new AuditEventEntityComponent(); 3288 if (this.entity == null) 3289 this.entity = new ArrayList<AuditEventEntityComponent>(); 3290 this.entity.add(t); 3291 return t; 3292 } 3293 3294 public AuditEvent addEntity(AuditEventEntityComponent t) { //3 3295 if (t == null) 3296 return this; 3297 if (this.entity == null) 3298 this.entity = new ArrayList<AuditEventEntityComponent>(); 3299 this.entity.add(t); 3300 return this; 3301 } 3302 3303 /** 3304 * @return The first repetition of repeating field {@link #entity}, creating it if it does not already exist {3} 3305 */ 3306 public AuditEventEntityComponent getEntityFirstRep() { 3307 if (getEntity().isEmpty()) { 3308 addEntity(); 3309 } 3310 return getEntity().get(0); 3311 } 3312 3313 protected void listChildren(List<Property> children) { 3314 super.listChildren(children); 3315 children.add(new Property("category", "CodeableConcept", "Classification of the type of event.", 0, java.lang.Integer.MAX_VALUE, category)); 3316 children.add(new Property("code", "CodeableConcept", "Describes what happened. The most specific code for the event.", 0, 1, code)); 3317 children.add(new Property("action", "code", "Indicator for type of action performed during the event that generated the audit.", 0, 1, action)); 3318 children.add(new Property("severity", "code", "Indicates and enables segmentation of various severity including debugging from critical.", 0, 1, severity)); 3319 children.add(new Property("occurred[x]", "Period|dateTime", "The time or period during which the activity occurred.", 0, 1, occurred)); 3320 children.add(new Property("recorded", "instant", "The time when the event was recorded.", 0, 1, recorded)); 3321 children.add(new Property("outcome", "", "Indicates whether the event succeeded or failed. A free text descripiton can be given in outcome.text.", 0, 1, outcome)); 3322 children.add(new Property("authorization", "CodeableConcept", "The authorization (e.g., PurposeOfUse) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, authorization)); 3323 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest|Task)", "Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3324 children.add(new Property("patient", "Reference(Patient)", "The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.", 0, 1, patient)); 3325 children.add(new Property("encounter", "Reference(Encounter)", "This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).", 0, 1, encounter)); 3326 children.add(new Property("agent", "", "An actor taking an active role in the event or activity that is logged.", 0, java.lang.Integer.MAX_VALUE, agent)); 3327 children.add(new Property("source", "", "The actor that is reporting the event.", 0, 1, source)); 3328 children.add(new Property("entity", "", "Specific instances of data or objects that have been accessed.", 0, java.lang.Integer.MAX_VALUE, entity)); 3329 } 3330 3331 @Override 3332 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3333 switch (_hash) { 3334 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Classification of the type of event.", 0, java.lang.Integer.MAX_VALUE, category); 3335 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Describes what happened. The most specific code for the event.", 0, 1, code); 3336 case -1422950858: /*action*/ return new Property("action", "code", "Indicator for type of action performed during the event that generated the audit.", 0, 1, action); 3337 case 1478300413: /*severity*/ return new Property("severity", "code", "Indicates and enables segmentation of various severity including debugging from critical.", 0, 1, severity); 3338 case 784181563: /*occurred[x]*/ return new Property("occurred[x]", "Period|dateTime", "The time or period during which the activity occurred.", 0, 1, occurred); 3339 case 792816933: /*occurred*/ return new Property("occurred[x]", "Period|dateTime", "The time or period during which the activity occurred.", 0, 1, occurred); 3340 case 894082886: /*occurredPeriod*/ return new Property("occurred[x]", "Period", "The time or period during which the activity occurred.", 0, 1, occurred); 3341 case 1579027424: /*occurredDateTime*/ return new Property("occurred[x]", "dateTime", "The time or period during which the activity occurred.", 0, 1, occurred); 3342 case -799233872: /*recorded*/ return new Property("recorded", "instant", "The time when the event was recorded.", 0, 1, recorded); 3343 case -1106507950: /*outcome*/ return new Property("outcome", "", "Indicates whether the event succeeded or failed. A free text descripiton can be given in outcome.text.", 0, 1, outcome); 3344 case -1385570183: /*authorization*/ return new Property("authorization", "CodeableConcept", "The authorization (e.g., PurposeOfUse) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, authorization); 3345 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest|Task)", "Allows tracing of authorizatino for the events and tracking whether proposals/recommendations were acted upon.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3346 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient element is available to enable deterministic tracking of activities that involve the patient as the subject of the data used in an activity.", 0, 1, patient); 3347 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "This will typically be the encounter the event occurred, but some events may be initiated prior to or after the official completion of an encounter but still be tied to the context of the encounter (e.g. pre-admission lab tests).", 0, 1, encounter); 3348 case 92750597: /*agent*/ return new Property("agent", "", "An actor taking an active role in the event or activity that is logged.", 0, java.lang.Integer.MAX_VALUE, agent); 3349 case -896505829: /*source*/ return new Property("source", "", "The actor that is reporting the event.", 0, 1, source); 3350 case -1298275357: /*entity*/ return new Property("entity", "", "Specific instances of data or objects that have been accessed.", 0, java.lang.Integer.MAX_VALUE, entity); 3351 default: return super.getNamedProperty(_hash, _name, _checkValid); 3352 } 3353 3354 } 3355 3356 @Override 3357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3358 switch (hash) { 3359 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3360 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3361 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // Enumeration<AuditEventAction> 3362 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<AuditEventSeverity> 3363 case 792816933: /*occurred*/ return this.occurred == null ? new Base[0] : new Base[] {this.occurred}; // DataType 3364 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // InstantType 3365 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // AuditEventOutcomeComponent 3366 case -1385570183: /*authorization*/ return this.authorization == null ? new Base[0] : this.authorization.toArray(new Base[this.authorization.size()]); // CodeableConcept 3367 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3368 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 3369 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3370 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : this.agent.toArray(new Base[this.agent.size()]); // AuditEventAgentComponent 3371 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // AuditEventSourceComponent 3372 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : this.entity.toArray(new Base[this.entity.size()]); // AuditEventEntityComponent 3373 default: return super.getProperty(hash, name, checkValid); 3374 } 3375 3376 } 3377 3378 @Override 3379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3380 switch (hash) { 3381 case 50511102: // category 3382 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3383 return value; 3384 case 3059181: // code 3385 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3386 return value; 3387 case -1422950858: // action 3388 value = new AuditEventActionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3389 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 3390 return value; 3391 case 1478300413: // severity 3392 value = new AuditEventSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3393 this.severity = (Enumeration) value; // Enumeration<AuditEventSeverity> 3394 return value; 3395 case 792816933: // occurred 3396 this.occurred = TypeConvertor.castToType(value); // DataType 3397 return value; 3398 case -799233872: // recorded 3399 this.recorded = TypeConvertor.castToInstant(value); // InstantType 3400 return value; 3401 case -1106507950: // outcome 3402 this.outcome = (AuditEventOutcomeComponent) value; // AuditEventOutcomeComponent 3403 return value; 3404 case -1385570183: // authorization 3405 this.getAuthorization().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3406 return value; 3407 case -332612366: // basedOn 3408 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3409 return value; 3410 case -791418107: // patient 3411 this.patient = TypeConvertor.castToReference(value); // Reference 3412 return value; 3413 case 1524132147: // encounter 3414 this.encounter = TypeConvertor.castToReference(value); // Reference 3415 return value; 3416 case 92750597: // agent 3417 this.getAgent().add((AuditEventAgentComponent) value); // AuditEventAgentComponent 3418 return value; 3419 case -896505829: // source 3420 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 3421 return value; 3422 case -1298275357: // entity 3423 this.getEntity().add((AuditEventEntityComponent) value); // AuditEventEntityComponent 3424 return value; 3425 default: return super.setProperty(hash, name, value); 3426 } 3427 3428 } 3429 3430 @Override 3431 public Base setProperty(String name, Base value) throws FHIRException { 3432 if (name.equals("category")) { 3433 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3434 } else if (name.equals("code")) { 3435 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3436 } else if (name.equals("action")) { 3437 value = new AuditEventActionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3438 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 3439 } else if (name.equals("severity")) { 3440 value = new AuditEventSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3441 this.severity = (Enumeration) value; // Enumeration<AuditEventSeverity> 3442 } else if (name.equals("occurred[x]")) { 3443 this.occurred = TypeConvertor.castToType(value); // DataType 3444 } else if (name.equals("recorded")) { 3445 this.recorded = TypeConvertor.castToInstant(value); // InstantType 3446 } else if (name.equals("outcome")) { 3447 this.outcome = (AuditEventOutcomeComponent) value; // AuditEventOutcomeComponent 3448 } else if (name.equals("authorization")) { 3449 this.getAuthorization().add(TypeConvertor.castToCodeableConcept(value)); 3450 } else if (name.equals("basedOn")) { 3451 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3452 } else if (name.equals("patient")) { 3453 this.patient = TypeConvertor.castToReference(value); // Reference 3454 } else if (name.equals("encounter")) { 3455 this.encounter = TypeConvertor.castToReference(value); // Reference 3456 } else if (name.equals("agent")) { 3457 this.getAgent().add((AuditEventAgentComponent) value); 3458 } else if (name.equals("source")) { 3459 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 3460 } else if (name.equals("entity")) { 3461 this.getEntity().add((AuditEventEntityComponent) value); 3462 } else 3463 return super.setProperty(name, value); 3464 return value; 3465 } 3466 3467 @Override 3468 public void removeChild(String name, Base value) throws FHIRException { 3469 if (name.equals("category")) { 3470 this.getCategory().remove(value); 3471 } else if (name.equals("code")) { 3472 this.code = null; 3473 } else if (name.equals("action")) { 3474 value = new AuditEventActionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3475 this.action = (Enumeration) value; // Enumeration<AuditEventAction> 3476 } else if (name.equals("severity")) { 3477 value = new AuditEventSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3478 this.severity = (Enumeration) value; // Enumeration<AuditEventSeverity> 3479 } else if (name.equals("occurred[x]")) { 3480 this.occurred = null; 3481 } else if (name.equals("recorded")) { 3482 this.recorded = null; 3483 } else if (name.equals("outcome")) { 3484 this.outcome = (AuditEventOutcomeComponent) value; // AuditEventOutcomeComponent 3485 } else if (name.equals("authorization")) { 3486 this.getAuthorization().remove(value); 3487 } else if (name.equals("basedOn")) { 3488 this.getBasedOn().remove(value); 3489 } else if (name.equals("patient")) { 3490 this.patient = null; 3491 } else if (name.equals("encounter")) { 3492 this.encounter = null; 3493 } else if (name.equals("agent")) { 3494 this.getAgent().remove((AuditEventAgentComponent) value); 3495 } else if (name.equals("source")) { 3496 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 3497 } else if (name.equals("entity")) { 3498 this.getEntity().remove((AuditEventEntityComponent) value); 3499 } else 3500 super.removeChild(name, value); 3501 3502 } 3503 3504 @Override 3505 public Base makeProperty(int hash, String name) throws FHIRException { 3506 switch (hash) { 3507 case 50511102: return addCategory(); 3508 case 3059181: return getCode(); 3509 case -1422950858: return getActionElement(); 3510 case 1478300413: return getSeverityElement(); 3511 case 784181563: return getOccurred(); 3512 case 792816933: return getOccurred(); 3513 case -799233872: return getRecordedElement(); 3514 case -1106507950: return getOutcome(); 3515 case -1385570183: return addAuthorization(); 3516 case -332612366: return addBasedOn(); 3517 case -791418107: return getPatient(); 3518 case 1524132147: return getEncounter(); 3519 case 92750597: return addAgent(); 3520 case -896505829: return getSource(); 3521 case -1298275357: return addEntity(); 3522 default: return super.makeProperty(hash, name); 3523 } 3524 3525 } 3526 3527 @Override 3528 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3529 switch (hash) { 3530 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3531 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3532 case -1422950858: /*action*/ return new String[] {"code"}; 3533 case 1478300413: /*severity*/ return new String[] {"code"}; 3534 case 792816933: /*occurred*/ return new String[] {"Period", "dateTime"}; 3535 case -799233872: /*recorded*/ return new String[] {"instant"}; 3536 case -1106507950: /*outcome*/ return new String[] {}; 3537 case -1385570183: /*authorization*/ return new String[] {"CodeableConcept"}; 3538 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3539 case -791418107: /*patient*/ return new String[] {"Reference"}; 3540 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3541 case 92750597: /*agent*/ return new String[] {}; 3542 case -896505829: /*source*/ return new String[] {}; 3543 case -1298275357: /*entity*/ return new String[] {}; 3544 default: return super.getTypesForProperty(hash, name); 3545 } 3546 3547 } 3548 3549 @Override 3550 public Base addChild(String name) throws FHIRException { 3551 if (name.equals("category")) { 3552 return addCategory(); 3553 } 3554 else if (name.equals("code")) { 3555 this.code = new CodeableConcept(); 3556 return this.code; 3557 } 3558 else if (name.equals("action")) { 3559 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.action"); 3560 } 3561 else if (name.equals("severity")) { 3562 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.severity"); 3563 } 3564 else if (name.equals("occurredPeriod")) { 3565 this.occurred = new Period(); 3566 return this.occurred; 3567 } 3568 else if (name.equals("occurredDateTime")) { 3569 this.occurred = new DateTimeType(); 3570 return this.occurred; 3571 } 3572 else if (name.equals("recorded")) { 3573 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.recorded"); 3574 } 3575 else if (name.equals("outcome")) { 3576 this.outcome = new AuditEventOutcomeComponent(); 3577 return this.outcome; 3578 } 3579 else if (name.equals("authorization")) { 3580 return addAuthorization(); 3581 } 3582 else if (name.equals("basedOn")) { 3583 return addBasedOn(); 3584 } 3585 else if (name.equals("patient")) { 3586 this.patient = new Reference(); 3587 return this.patient; 3588 } 3589 else if (name.equals("encounter")) { 3590 this.encounter = new Reference(); 3591 return this.encounter; 3592 } 3593 else if (name.equals("agent")) { 3594 return addAgent(); 3595 } 3596 else if (name.equals("source")) { 3597 this.source = new AuditEventSourceComponent(); 3598 return this.source; 3599 } 3600 else if (name.equals("entity")) { 3601 return addEntity(); 3602 } 3603 else 3604 return super.addChild(name); 3605 } 3606 3607 public String fhirType() { 3608 return "AuditEvent"; 3609 3610 } 3611 3612 public AuditEvent copy() { 3613 AuditEvent dst = new AuditEvent(); 3614 copyValues(dst); 3615 return dst; 3616 } 3617 3618 public void copyValues(AuditEvent dst) { 3619 super.copyValues(dst); 3620 if (category != null) { 3621 dst.category = new ArrayList<CodeableConcept>(); 3622 for (CodeableConcept i : category) 3623 dst.category.add(i.copy()); 3624 }; 3625 dst.code = code == null ? null : code.copy(); 3626 dst.action = action == null ? null : action.copy(); 3627 dst.severity = severity == null ? null : severity.copy(); 3628 dst.occurred = occurred == null ? null : occurred.copy(); 3629 dst.recorded = recorded == null ? null : recorded.copy(); 3630 dst.outcome = outcome == null ? null : outcome.copy(); 3631 if (authorization != null) { 3632 dst.authorization = new ArrayList<CodeableConcept>(); 3633 for (CodeableConcept i : authorization) 3634 dst.authorization.add(i.copy()); 3635 }; 3636 if (basedOn != null) { 3637 dst.basedOn = new ArrayList<Reference>(); 3638 for (Reference i : basedOn) 3639 dst.basedOn.add(i.copy()); 3640 }; 3641 dst.patient = patient == null ? null : patient.copy(); 3642 dst.encounter = encounter == null ? null : encounter.copy(); 3643 if (agent != null) { 3644 dst.agent = new ArrayList<AuditEventAgentComponent>(); 3645 for (AuditEventAgentComponent i : agent) 3646 dst.agent.add(i.copy()); 3647 }; 3648 dst.source = source == null ? null : source.copy(); 3649 if (entity != null) { 3650 dst.entity = new ArrayList<AuditEventEntityComponent>(); 3651 for (AuditEventEntityComponent i : entity) 3652 dst.entity.add(i.copy()); 3653 }; 3654 } 3655 3656 protected AuditEvent typedCopy() { 3657 return copy(); 3658 } 3659 3660 @Override 3661 public boolean equalsDeep(Base other_) { 3662 if (!super.equalsDeep(other_)) 3663 return false; 3664 if (!(other_ instanceof AuditEvent)) 3665 return false; 3666 AuditEvent o = (AuditEvent) other_; 3667 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(action, o.action, true) 3668 && compareDeep(severity, o.severity, true) && compareDeep(occurred, o.occurred, true) && compareDeep(recorded, o.recorded, true) 3669 && compareDeep(outcome, o.outcome, true) && compareDeep(authorization, o.authorization, true) && compareDeep(basedOn, o.basedOn, true) 3670 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) && compareDeep(agent, o.agent, true) 3671 && compareDeep(source, o.source, true) && compareDeep(entity, o.entity, true); 3672 } 3673 3674 @Override 3675 public boolean equalsShallow(Base other_) { 3676 if (!super.equalsShallow(other_)) 3677 return false; 3678 if (!(other_ instanceof AuditEvent)) 3679 return false; 3680 AuditEvent o = (AuditEvent) other_; 3681 return compareValues(action, o.action, true) && compareValues(severity, o.severity, true) && compareValues(recorded, o.recorded, true) 3682 ; 3683 } 3684 3685 public boolean isEmpty() { 3686 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code, action, severity 3687 , occurred, recorded, outcome, authorization, basedOn, patient, encounter, agent 3688 , source, entity); 3689 } 3690 3691 @Override 3692 public ResourceType getResourceType() { 3693 return ResourceType.AuditEvent; 3694 } 3695 3696 /** 3697 * Search parameter: <b>action</b> 3698 * <p> 3699 * Description: <b>Type of action performed during the event</b><br> 3700 * Type: <b>token</b><br> 3701 * Path: <b>AuditEvent.action</b><br> 3702 * </p> 3703 */ 3704 @SearchParamDefinition(name="action", path="AuditEvent.action", description="Type of action performed during the event", type="token" ) 3705 public static final String SP_ACTION = "action"; 3706 /** 3707 * <b>Fluent Client</b> search parameter constant for <b>action</b> 3708 * <p> 3709 * Description: <b>Type of action performed during the event</b><br> 3710 * Type: <b>token</b><br> 3711 * Path: <b>AuditEvent.action</b><br> 3712 * </p> 3713 */ 3714 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 3715 3716 /** 3717 * Search parameter: <b>agent-role</b> 3718 * <p> 3719 * Description: <b>Agent role in the event</b><br> 3720 * Type: <b>token</b><br> 3721 * Path: <b>AuditEvent.agent.role</b><br> 3722 * </p> 3723 */ 3724 @SearchParamDefinition(name="agent-role", path="AuditEvent.agent.role", description="Agent role in the event", type="token" ) 3725 public static final String SP_AGENT_ROLE = "agent-role"; 3726 /** 3727 * <b>Fluent Client</b> search parameter constant for <b>agent-role</b> 3728 * <p> 3729 * Description: <b>Agent role in the event</b><br> 3730 * Type: <b>token</b><br> 3731 * Path: <b>AuditEvent.agent.role</b><br> 3732 * </p> 3733 */ 3734 public static final ca.uhn.fhir.rest.gclient.TokenClientParam AGENT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_AGENT_ROLE); 3735 3736 /** 3737 * Search parameter: <b>agent</b> 3738 * <p> 3739 * Description: <b>Identifier of who</b><br> 3740 * Type: <b>reference</b><br> 3741 * Path: <b>AuditEvent.agent.who</b><br> 3742 * </p> 3743 */ 3744 @SearchParamDefinition(name="agent", path="AuditEvent.agent.who", description="Identifier of who", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3745 public static final String SP_AGENT = "agent"; 3746 /** 3747 * <b>Fluent Client</b> search parameter constant for <b>agent</b> 3748 * <p> 3749 * Description: <b>Identifier of who</b><br> 3750 * Type: <b>reference</b><br> 3751 * Path: <b>AuditEvent.agent.who</b><br> 3752 * </p> 3753 */ 3754 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AGENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AGENT); 3755 3756/** 3757 * Constant for fluent queries to be used to add include statements. Specifies 3758 * the path value of "<b>AuditEvent:agent</b>". 3759 */ 3760 public static final ca.uhn.fhir.model.api.Include INCLUDE_AGENT = new ca.uhn.fhir.model.api.Include("AuditEvent:agent").toLocked(); 3761 3762 /** 3763 * Search parameter: <b>based-on</b> 3764 * <p> 3765 * Description: <b>Reference to the service request.</b><br> 3766 * Type: <b>reference</b><br> 3767 * Path: <b>AuditEvent.basedOn</b><br> 3768 * </p> 3769 */ 3770 @SearchParamDefinition(name="based-on", path="AuditEvent.basedOn", description="Reference to the service request.", type="reference", target={CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, Task.class } ) 3771 public static final String SP_BASED_ON = "based-on"; 3772 /** 3773 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3774 * <p> 3775 * Description: <b>Reference to the service request.</b><br> 3776 * Type: <b>reference</b><br> 3777 * Path: <b>AuditEvent.basedOn</b><br> 3778 * </p> 3779 */ 3780 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3781 3782/** 3783 * Constant for fluent queries to be used to add include statements. Specifies 3784 * the path value of "<b>AuditEvent:based-on</b>". 3785 */ 3786 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("AuditEvent:based-on").toLocked(); 3787 3788 /** 3789 * Search parameter: <b>category</b> 3790 * <p> 3791 * Description: <b>Category of event</b><br> 3792 * Type: <b>token</b><br> 3793 * Path: <b>AuditEvent.category</b><br> 3794 * </p> 3795 */ 3796 @SearchParamDefinition(name="category", path="AuditEvent.category", description="Category of event", type="token" ) 3797 public static final String SP_CATEGORY = "category"; 3798 /** 3799 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3800 * <p> 3801 * Description: <b>Category of event</b><br> 3802 * Type: <b>token</b><br> 3803 * Path: <b>AuditEvent.category</b><br> 3804 * </p> 3805 */ 3806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3807 3808 /** 3809 * Search parameter: <b>entity-role</b> 3810 * <p> 3811 * Description: <b>What role the entity played</b><br> 3812 * Type: <b>token</b><br> 3813 * Path: <b>AuditEvent.entity.role</b><br> 3814 * </p> 3815 */ 3816 @SearchParamDefinition(name="entity-role", path="AuditEvent.entity.role", description="What role the entity played", type="token" ) 3817 public static final String SP_ENTITY_ROLE = "entity-role"; 3818 /** 3819 * <b>Fluent Client</b> search parameter constant for <b>entity-role</b> 3820 * <p> 3821 * Description: <b>What role the entity played</b><br> 3822 * Type: <b>token</b><br> 3823 * Path: <b>AuditEvent.entity.role</b><br> 3824 * </p> 3825 */ 3826 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ENTITY_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ENTITY_ROLE); 3827 3828 /** 3829 * Search parameter: <b>entity</b> 3830 * <p> 3831 * Description: <b>Specific instance of resource</b><br> 3832 * Type: <b>reference</b><br> 3833 * Path: <b>AuditEvent.entity.what</b><br> 3834 * </p> 3835 */ 3836 @SearchParamDefinition(name="entity", path="AuditEvent.entity.what", description="Specific instance of resource", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3837 public static final String SP_ENTITY = "entity"; 3838 /** 3839 * <b>Fluent Client</b> search parameter constant for <b>entity</b> 3840 * <p> 3841 * Description: <b>Specific instance of resource</b><br> 3842 * Type: <b>reference</b><br> 3843 * Path: <b>AuditEvent.entity.what</b><br> 3844 * </p> 3845 */ 3846 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTITY); 3847 3848/** 3849 * Constant for fluent queries to be used to add include statements. Specifies 3850 * the path value of "<b>AuditEvent:entity</b>". 3851 */ 3852 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTITY = new ca.uhn.fhir.model.api.Include("AuditEvent:entity").toLocked(); 3853 3854 /** 3855 * Search parameter: <b>outcome</b> 3856 * <p> 3857 * Description: <b>Whether the event succeeded or failed</b><br> 3858 * Type: <b>token</b><br> 3859 * Path: <b>AuditEvent.outcome.code</b><br> 3860 * </p> 3861 */ 3862 @SearchParamDefinition(name="outcome", path="AuditEvent.outcome.code", description="Whether the event succeeded or failed", type="token" ) 3863 public static final String SP_OUTCOME = "outcome"; 3864 /** 3865 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 3866 * <p> 3867 * Description: <b>Whether the event succeeded or failed</b><br> 3868 * Type: <b>token</b><br> 3869 * Path: <b>AuditEvent.outcome.code</b><br> 3870 * </p> 3871 */ 3872 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 3873 3874 /** 3875 * Search parameter: <b>policy</b> 3876 * <p> 3877 * Description: <b>Policy that authorized event</b><br> 3878 * Type: <b>uri</b><br> 3879 * Path: <b>AuditEvent.agent.policy</b><br> 3880 * </p> 3881 */ 3882 @SearchParamDefinition(name="policy", path="AuditEvent.agent.policy", description="Policy that authorized event", type="uri" ) 3883 public static final String SP_POLICY = "policy"; 3884 /** 3885 * <b>Fluent Client</b> search parameter constant for <b>policy</b> 3886 * <p> 3887 * Description: <b>Policy that authorized event</b><br> 3888 * Type: <b>uri</b><br> 3889 * Path: <b>AuditEvent.agent.policy</b><br> 3890 * </p> 3891 */ 3892 public static final ca.uhn.fhir.rest.gclient.UriClientParam POLICY = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_POLICY); 3893 3894 /** 3895 * Search parameter: <b>purpose</b> 3896 * <p> 3897 * Description: <b>The authorization (purposeOfUse) of the event</b><br> 3898 * Type: <b>token</b><br> 3899 * Path: <b>AuditEvent.authorization | AuditEvent.agent.authorization</b><br> 3900 * </p> 3901 */ 3902 @SearchParamDefinition(name="purpose", path="AuditEvent.authorization | AuditEvent.agent.authorization", description="The authorization (purposeOfUse) of the event", type="token" ) 3903 public static final String SP_PURPOSE = "purpose"; 3904 /** 3905 * <b>Fluent Client</b> search parameter constant for <b>purpose</b> 3906 * <p> 3907 * Description: <b>The authorization (purposeOfUse) of the event</b><br> 3908 * Type: <b>token</b><br> 3909 * Path: <b>AuditEvent.authorization | AuditEvent.agent.authorization</b><br> 3910 * </p> 3911 */ 3912 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PURPOSE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PURPOSE); 3913 3914 /** 3915 * Search parameter: <b>source</b> 3916 * <p> 3917 * Description: <b>The identity of source detecting the event</b><br> 3918 * Type: <b>reference</b><br> 3919 * Path: <b>AuditEvent.source.observer</b><br> 3920 * </p> 3921 */ 3922 @SearchParamDefinition(name="source", path="AuditEvent.source.observer", description="The identity of source detecting the event", type="reference", target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3923 public static final String SP_SOURCE = "source"; 3924 /** 3925 * <b>Fluent Client</b> search parameter constant for <b>source</b> 3926 * <p> 3927 * Description: <b>The identity of source detecting the event</b><br> 3928 * Type: <b>reference</b><br> 3929 * Path: <b>AuditEvent.source.observer</b><br> 3930 * </p> 3931 */ 3932 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 3933 3934/** 3935 * Constant for fluent queries to be used to add include statements. Specifies 3936 * the path value of "<b>AuditEvent:source</b>". 3937 */ 3938 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("AuditEvent:source").toLocked(); 3939 3940 /** 3941 * Search parameter: <b>code</b> 3942 * <p> 3943 * Description: <b>Multiple Resources: 3944 3945* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3946* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3947* [AuditEvent](auditevent.html): More specific code for the event 3948* [Basic](basic.html): Kind of Resource 3949* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3950* [Condition](condition.html): Code for the condition 3951* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3952* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3953* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3954* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3955* [ImagingSelection](imagingselection.html): The imaging selection status 3956* [List](list.html): What the purpose of this list is 3957* [Medication](medication.html): Returns medications for a specific code 3958* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3959* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3960* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3961* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3962* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3963* [Observation](observation.html): The code of the observation type 3964* [Procedure](procedure.html): A code to identify a procedure 3965* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3966* [Task](task.html): Search by task code 3967</b><br> 3968 * Type: <b>token</b><br> 3969 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3970 * </p> 3971 */ 3972 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3973 public static final String SP_CODE = "code"; 3974 /** 3975 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3976 * <p> 3977 * Description: <b>Multiple Resources: 3978 3979* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3980* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3981* [AuditEvent](auditevent.html): More specific code for the event 3982* [Basic](basic.html): Kind of Resource 3983* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3984* [Condition](condition.html): Code for the condition 3985* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3986* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3987* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3988* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3989* [ImagingSelection](imagingselection.html): The imaging selection status 3990* [List](list.html): What the purpose of this list is 3991* [Medication](medication.html): Returns medications for a specific code 3992* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3993* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3994* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3995* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3996* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3997* [Observation](observation.html): The code of the observation type 3998* [Procedure](procedure.html): A code to identify a procedure 3999* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 4000* [Task](task.html): Search by task code 4001</b><br> 4002 * Type: <b>token</b><br> 4003 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 4004 * </p> 4005 */ 4006 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4007 4008 /** 4009 * Search parameter: <b>date</b> 4010 * <p> 4011 * Description: <b>Multiple Resources: 4012 4013* [AdverseEvent](adverseevent.html): When the event occurred 4014* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4015* [Appointment](appointment.html): Appointment date/time. 4016* [AuditEvent](auditevent.html): Time when the event was recorded 4017* [CarePlan](careplan.html): Time period plan covers 4018* [CareTeam](careteam.html): A date within the coverage time period. 4019* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4020* [Composition](composition.html): Composition editing time 4021* [Consent](consent.html): When consent was agreed to 4022* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4023* [DocumentReference](documentreference.html): When this document reference was created 4024* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4025* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4026* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4027* [Flag](flag.html): Time period when flag is active 4028* [Immunization](immunization.html): Vaccination (non)-Administration Date 4029* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4030* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4031* [Invoice](invoice.html): Invoice date / posting date 4032* [List](list.html): When the list was prepared 4033* [MeasureReport](measurereport.html): The date of the measure report 4034* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4035* [Observation](observation.html): Clinically relevant time/time-period for observation 4036* [Procedure](procedure.html): When the procedure occurred or is occurring 4037* [ResearchSubject](researchsubject.html): Start and end of participation 4038* [RiskAssessment](riskassessment.html): When was assessment made? 4039* [SupplyRequest](supplyrequest.html): When the request was made 4040</b><br> 4041 * Type: <b>date</b><br> 4042 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4043 * </p> 4044 */ 4045 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4046 public static final String SP_DATE = "date"; 4047 /** 4048 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4049 * <p> 4050 * Description: <b>Multiple Resources: 4051 4052* [AdverseEvent](adverseevent.html): When the event occurred 4053* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4054* [Appointment](appointment.html): Appointment date/time. 4055* [AuditEvent](auditevent.html): Time when the event was recorded 4056* [CarePlan](careplan.html): Time period plan covers 4057* [CareTeam](careteam.html): A date within the coverage time period. 4058* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4059* [Composition](composition.html): Composition editing time 4060* [Consent](consent.html): When consent was agreed to 4061* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4062* [DocumentReference](documentreference.html): When this document reference was created 4063* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4064* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4065* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4066* [Flag](flag.html): Time period when flag is active 4067* [Immunization](immunization.html): Vaccination (non)-Administration Date 4068* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4069* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4070* [Invoice](invoice.html): Invoice date / posting date 4071* [List](list.html): When the list was prepared 4072* [MeasureReport](measurereport.html): The date of the measure report 4073* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4074* [Observation](observation.html): Clinically relevant time/time-period for observation 4075* [Procedure](procedure.html): When the procedure occurred or is occurring 4076* [ResearchSubject](researchsubject.html): Start and end of participation 4077* [RiskAssessment](riskassessment.html): When was assessment made? 4078* [SupplyRequest](supplyrequest.html): When the request was made 4079</b><br> 4080 * Type: <b>date</b><br> 4081 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4082 * </p> 4083 */ 4084 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4085 4086 /** 4087 * Search parameter: <b>encounter</b> 4088 * <p> 4089 * Description: <b>Multiple Resources: 4090 4091* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 4092* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 4093* [ChargeItem](chargeitem.html): Encounter associated with event 4094* [Claim](claim.html): Encounters associated with a billed line item 4095* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 4096* [Communication](communication.html): The Encounter during which this Communication was created 4097* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 4098* [Composition](composition.html): Context of the Composition 4099* [Condition](condition.html): The Encounter during which this Condition was created 4100* [DeviceRequest](devicerequest.html): Encounter during which request was created 4101* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 4102* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 4103* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 4104* [Flag](flag.html): Alert relevant during encounter 4105* [ImagingStudy](imagingstudy.html): The context of the study 4106* [List](list.html): Context in which list created 4107* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 4108* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 4109* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 4110* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 4111* [Observation](observation.html): Encounter related to the observation 4112* [Procedure](procedure.html): The Encounter during which this Procedure was created 4113* [Provenance](provenance.html): Encounter related to the Provenance 4114* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 4115* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 4116* [RiskAssessment](riskassessment.html): Where was assessment performed? 4117* [ServiceRequest](servicerequest.html): An encounter in which this request is made 4118* [Task](task.html): Search by encounter 4119* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 4120</b><br> 4121 * Type: <b>reference</b><br> 4122 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 4123 * </p> 4124 */ 4125 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 4126 public static final String SP_ENCOUNTER = "encounter"; 4127 /** 4128 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4129 * <p> 4130 * Description: <b>Multiple Resources: 4131 4132* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 4133* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 4134* [ChargeItem](chargeitem.html): Encounter associated with event 4135* [Claim](claim.html): Encounters associated with a billed line item 4136* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 4137* [Communication](communication.html): The Encounter during which this Communication was created 4138* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 4139* [Composition](composition.html): Context of the Composition 4140* [Condition](condition.html): The Encounter during which this Condition was created 4141* [DeviceRequest](devicerequest.html): Encounter during which request was created 4142* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 4143* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 4144* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 4145* [Flag](flag.html): Alert relevant during encounter 4146* [ImagingStudy](imagingstudy.html): The context of the study 4147* [List](list.html): Context in which list created 4148* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 4149* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 4150* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 4151* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 4152* [Observation](observation.html): Encounter related to the observation 4153* [Procedure](procedure.html): The Encounter during which this Procedure was created 4154* [Provenance](provenance.html): Encounter related to the Provenance 4155* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 4156* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 4157* [RiskAssessment](riskassessment.html): Where was assessment performed? 4158* [ServiceRequest](servicerequest.html): An encounter in which this request is made 4159* [Task](task.html): Search by encounter 4160* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 4161</b><br> 4162 * Type: <b>reference</b><br> 4163 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 4164 * </p> 4165 */ 4166 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4167 4168/** 4169 * Constant for fluent queries to be used to add include statements. Specifies 4170 * the path value of "<b>AuditEvent:encounter</b>". 4171 */ 4172 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("AuditEvent:encounter").toLocked(); 4173 4174 /** 4175 * Search parameter: <b>patient</b> 4176 * <p> 4177 * Description: <b>Multiple Resources: 4178 4179* [Account](account.html): The entity that caused the expenses 4180* [AdverseEvent](adverseevent.html): Subject impacted by event 4181* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4182* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4183* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4184* [AuditEvent](auditevent.html): Where the activity involved patient data 4185* [Basic](basic.html): Identifies the focus of this resource 4186* [BodyStructure](bodystructure.html): Who this is about 4187* [CarePlan](careplan.html): Who the care plan is for 4188* [CareTeam](careteam.html): Who care team is for 4189* [ChargeItem](chargeitem.html): Individual service was done for/to 4190* [Claim](claim.html): Patient receiving the products or services 4191* [ClaimResponse](claimresponse.html): The subject of care 4192* [ClinicalImpression](clinicalimpression.html): Patient assessed 4193* [Communication](communication.html): Focus of message 4194* [CommunicationRequest](communicationrequest.html): Focus of message 4195* [Composition](composition.html): Who and/or what the composition is about 4196* [Condition](condition.html): Who has the condition? 4197* [Consent](consent.html): Who the consent applies to 4198* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4199* [Coverage](coverage.html): Retrieve coverages for a patient 4200* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4201* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4202* [DetectedIssue](detectedissue.html): Associated patient 4203* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4204* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4205* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4206* [DocumentReference](documentreference.html): Who/what is the subject of the document 4207* [Encounter](encounter.html): The patient present at the encounter 4208* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4209* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4210* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4211* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4212* [Flag](flag.html): The identity of a subject to list flags for 4213* [Goal](goal.html): Who this goal is intended for 4214* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4215* [ImagingSelection](imagingselection.html): Who the study is about 4216* [ImagingStudy](imagingstudy.html): Who the study is about 4217* [Immunization](immunization.html): The patient for the vaccination record 4218* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4219* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4220* [Invoice](invoice.html): Recipient(s) of goods and services 4221* [List](list.html): If all resources have the same subject 4222* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4223* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4224* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4225* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4226* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4227* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4228* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4229* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4230* [Observation](observation.html): The subject that the observation is about (if patient) 4231* [Person](person.html): The Person links to this Patient 4232* [Procedure](procedure.html): Search by subject - a patient 4233* [Provenance](provenance.html): Where the activity involved patient data 4234* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4235* [RelatedPerson](relatedperson.html): The patient this related person is related to 4236* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4237* [ResearchSubject](researchsubject.html): Who or what is part of study 4238* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4239* [ServiceRequest](servicerequest.html): Search by subject - a patient 4240* [Specimen](specimen.html): The patient the specimen comes from 4241* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4242* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4243* [Task](task.html): Search by patient 4244* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4245</b><br> 4246 * Type: <b>reference</b><br> 4247 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4248 * </p> 4249 */ 4250 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 4251 public static final String SP_PATIENT = "patient"; 4252 /** 4253 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4254 * <p> 4255 * Description: <b>Multiple Resources: 4256 4257* [Account](account.html): The entity that caused the expenses 4258* [AdverseEvent](adverseevent.html): Subject impacted by event 4259* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4260* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4261* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4262* [AuditEvent](auditevent.html): Where the activity involved patient data 4263* [Basic](basic.html): Identifies the focus of this resource 4264* [BodyStructure](bodystructure.html): Who this is about 4265* [CarePlan](careplan.html): Who the care plan is for 4266* [CareTeam](careteam.html): Who care team is for 4267* [ChargeItem](chargeitem.html): Individual service was done for/to 4268* [Claim](claim.html): Patient receiving the products or services 4269* [ClaimResponse](claimresponse.html): The subject of care 4270* [ClinicalImpression](clinicalimpression.html): Patient assessed 4271* [Communication](communication.html): Focus of message 4272* [CommunicationRequest](communicationrequest.html): Focus of message 4273* [Composition](composition.html): Who and/or what the composition is about 4274* [Condition](condition.html): Who has the condition? 4275* [Consent](consent.html): Who the consent applies to 4276* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4277* [Coverage](coverage.html): Retrieve coverages for a patient 4278* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4279* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4280* [DetectedIssue](detectedissue.html): Associated patient 4281* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4282* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4283* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4284* [DocumentReference](documentreference.html): Who/what is the subject of the document 4285* [Encounter](encounter.html): The patient present at the encounter 4286* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4287* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4288* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4289* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4290* [Flag](flag.html): The identity of a subject to list flags for 4291* [Goal](goal.html): Who this goal is intended for 4292* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4293* [ImagingSelection](imagingselection.html): Who the study is about 4294* [ImagingStudy](imagingstudy.html): Who the study is about 4295* [Immunization](immunization.html): The patient for the vaccination record 4296* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4297* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4298* [Invoice](invoice.html): Recipient(s) of goods and services 4299* [List](list.html): If all resources have the same subject 4300* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4301* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4302* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4303* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4304* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4305* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4306* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4307* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4308* [Observation](observation.html): The subject that the observation is about (if patient) 4309* [Person](person.html): The Person links to this Patient 4310* [Procedure](procedure.html): Search by subject - a patient 4311* [Provenance](provenance.html): Where the activity involved patient data 4312* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4313* [RelatedPerson](relatedperson.html): The patient this related person is related to 4314* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4315* [ResearchSubject](researchsubject.html): Who or what is part of study 4316* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4317* [ServiceRequest](servicerequest.html): Search by subject - a patient 4318* [Specimen](specimen.html): The patient the specimen comes from 4319* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4320* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4321* [Task](task.html): Search by patient 4322* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4323</b><br> 4324 * Type: <b>reference</b><br> 4325 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4326 * </p> 4327 */ 4328 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4329 4330/** 4331 * Constant for fluent queries to be used to add include statements. Specifies 4332 * the path value of "<b>AuditEvent:patient</b>". 4333 */ 4334 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("AuditEvent:patient").toLocked(); 4335 4336 4337} 4338