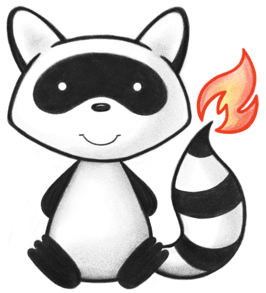
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Availability Type: Availability data for an {item}. 050 */ 051@DatatypeDef(name="Availability") 052public class Availability extends DataType implements ICompositeType { 053 054 @Block() 055 public static class AvailabilityAvailableTimeComponent extends Element implements IBaseDatatypeElement { 056 /** 057 * mon | tue | wed | thu | fri | sat | sun. 058 */ 059 @Child(name = "daysOfWeek", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 060 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="mon | tue | wed | thu | fri | sat | sun." ) 061 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 062 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 063 064 /** 065 * Always available? i.e. 24 hour service. 066 */ 067 @Child(name = "allDay", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 068 @Description(shortDefinition="Always available? i.e. 24 hour service", formalDefinition="Always available? i.e. 24 hour service." ) 069 protected BooleanType allDay; 070 071 /** 072 * Opening time of day (ignored if allDay = true). 073 */ 074 @Child(name = "availableStartTime", type = {TimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 075 @Description(shortDefinition="Opening time of day (ignored if allDay = true)", formalDefinition="Opening time of day (ignored if allDay = true)." ) 076 protected TimeType availableStartTime; 077 078 /** 079 * Closing time of day (ignored if allDay = true). 080 */ 081 @Child(name = "availableEndTime", type = {TimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 082 @Description(shortDefinition="Closing time of day (ignored if allDay = true)", formalDefinition="Closing time of day (ignored if allDay = true)." ) 083 protected TimeType availableEndTime; 084 085 private static final long serialVersionUID = -2139510127L; 086 087 /** 088 * Constructor 089 */ 090 public AvailabilityAvailableTimeComponent() { 091 super(); 092 } 093 094 /** 095 * @return {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 096 */ 097 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 098 if (this.daysOfWeek == null) 099 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 100 return this.daysOfWeek; 101 } 102 103 /** 104 * @return Returns a reference to <code>this</code> for easy method chaining 105 */ 106 public AvailabilityAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 107 this.daysOfWeek = theDaysOfWeek; 108 return this; 109 } 110 111 public boolean hasDaysOfWeek() { 112 if (this.daysOfWeek == null) 113 return false; 114 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 115 if (!item.isEmpty()) 116 return true; 117 return false; 118 } 119 120 /** 121 * @return {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 122 */ 123 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {//2 124 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 125 if (this.daysOfWeek == null) 126 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 127 this.daysOfWeek.add(t); 128 return t; 129 } 130 131 /** 132 * @param value {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 133 */ 134 public AvailabilityAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { //1 135 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 136 t.setValue(value); 137 if (this.daysOfWeek == null) 138 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 139 this.daysOfWeek.add(t); 140 return this; 141 } 142 143 /** 144 * @param value {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 145 */ 146 public boolean hasDaysOfWeek(DaysOfWeek value) { 147 if (this.daysOfWeek == null) 148 return false; 149 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 150 if (v.getValue().equals(value)) // code 151 return true; 152 return false; 153 } 154 155 /** 156 * @return {@link #allDay} (Always available? i.e. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 157 */ 158 public BooleanType getAllDayElement() { 159 if (this.allDay == null) 160 if (Configuration.errorOnAutoCreate()) 161 throw new Error("Attempt to auto-create AvailabilityAvailableTimeComponent.allDay"); 162 else if (Configuration.doAutoCreate()) 163 this.allDay = new BooleanType(); // bb 164 return this.allDay; 165 } 166 167 public boolean hasAllDayElement() { 168 return this.allDay != null && !this.allDay.isEmpty(); 169 } 170 171 public boolean hasAllDay() { 172 return this.allDay != null && !this.allDay.isEmpty(); 173 } 174 175 /** 176 * @param value {@link #allDay} (Always available? i.e. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 177 */ 178 public AvailabilityAvailableTimeComponent setAllDayElement(BooleanType value) { 179 this.allDay = value; 180 return this; 181 } 182 183 /** 184 * @return Always available? i.e. 24 hour service. 185 */ 186 public boolean getAllDay() { 187 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 188 } 189 190 /** 191 * @param value Always available? i.e. 24 hour service. 192 */ 193 public AvailabilityAvailableTimeComponent setAllDay(boolean value) { 194 if (this.allDay == null) 195 this.allDay = new BooleanType(); 196 this.allDay.setValue(value); 197 return this; 198 } 199 200 /** 201 * @return {@link #availableStartTime} (Opening time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 202 */ 203 public TimeType getAvailableStartTimeElement() { 204 if (this.availableStartTime == null) 205 if (Configuration.errorOnAutoCreate()) 206 throw new Error("Attempt to auto-create AvailabilityAvailableTimeComponent.availableStartTime"); 207 else if (Configuration.doAutoCreate()) 208 this.availableStartTime = new TimeType(); // bb 209 return this.availableStartTime; 210 } 211 212 public boolean hasAvailableStartTimeElement() { 213 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 214 } 215 216 public boolean hasAvailableStartTime() { 217 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #availableStartTime} (Opening time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 222 */ 223 public AvailabilityAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 224 this.availableStartTime = value; 225 return this; 226 } 227 228 /** 229 * @return Opening time of day (ignored if allDay = true). 230 */ 231 public String getAvailableStartTime() { 232 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 233 } 234 235 /** 236 * @param value Opening time of day (ignored if allDay = true). 237 */ 238 public AvailabilityAvailableTimeComponent setAvailableStartTime(String value) { 239 if (value == null) 240 this.availableStartTime = null; 241 else { 242 if (this.availableStartTime == null) 243 this.availableStartTime = new TimeType(); 244 this.availableStartTime.setValue(value); 245 } 246 return this; 247 } 248 249 /** 250 * @return {@link #availableEndTime} (Closing time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 251 */ 252 public TimeType getAvailableEndTimeElement() { 253 if (this.availableEndTime == null) 254 if (Configuration.errorOnAutoCreate()) 255 throw new Error("Attempt to auto-create AvailabilityAvailableTimeComponent.availableEndTime"); 256 else if (Configuration.doAutoCreate()) 257 this.availableEndTime = new TimeType(); // bb 258 return this.availableEndTime; 259 } 260 261 public boolean hasAvailableEndTimeElement() { 262 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 263 } 264 265 public boolean hasAvailableEndTime() { 266 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 267 } 268 269 /** 270 * @param value {@link #availableEndTime} (Closing time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 271 */ 272 public AvailabilityAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 273 this.availableEndTime = value; 274 return this; 275 } 276 277 /** 278 * @return Closing time of day (ignored if allDay = true). 279 */ 280 public String getAvailableEndTime() { 281 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 282 } 283 284 /** 285 * @param value Closing time of day (ignored if allDay = true). 286 */ 287 public AvailabilityAvailableTimeComponent setAvailableEndTime(String value) { 288 if (value == null) 289 this.availableEndTime = null; 290 else { 291 if (this.availableEndTime == null) 292 this.availableEndTime = new TimeType(); 293 this.availableEndTime.setValue(value); 294 } 295 return this; 296 } 297 298 protected void listChildren(List<Property> children) { 299 super.listChildren(children); 300 children.add(new Property("daysOfWeek", "code", "mon | tue | wed | thu | fri | sat | sun.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek)); 301 children.add(new Property("allDay", "boolean", "Always available? i.e. 24 hour service.", 0, 1, allDay)); 302 children.add(new Property("availableStartTime", "time", "Opening time of day (ignored if allDay = true).", 0, 1, availableStartTime)); 303 children.add(new Property("availableEndTime", "time", "Closing time of day (ignored if allDay = true).", 0, 1, availableEndTime)); 304 } 305 306 @Override 307 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 308 switch (_hash) { 309 case 68050338: /*daysOfWeek*/ return new Property("daysOfWeek", "code", "mon | tue | wed | thu | fri | sat | sun.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek); 310 case -1414913477: /*allDay*/ return new Property("allDay", "boolean", "Always available? i.e. 24 hour service.", 0, 1, allDay); 311 case -1039453818: /*availableStartTime*/ return new Property("availableStartTime", "time", "Opening time of day (ignored if allDay = true).", 0, 1, availableStartTime); 312 case 101151551: /*availableEndTime*/ return new Property("availableEndTime", "time", "Closing time of day (ignored if allDay = true).", 0, 1, availableEndTime); 313 default: return super.getNamedProperty(_hash, _name, _checkValid); 314 } 315 316 } 317 318 @Override 319 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 320 switch (hash) { 321 case 68050338: /*daysOfWeek*/ return this.daysOfWeek == null ? new Base[0] : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 322 case -1414913477: /*allDay*/ return this.allDay == null ? new Base[0] : new Base[] {this.allDay}; // BooleanType 323 case -1039453818: /*availableStartTime*/ return this.availableStartTime == null ? new Base[0] : new Base[] {this.availableStartTime}; // TimeType 324 case 101151551: /*availableEndTime*/ return this.availableEndTime == null ? new Base[0] : new Base[] {this.availableEndTime}; // TimeType 325 default: return super.getProperty(hash, name, checkValid); 326 } 327 328 } 329 330 @Override 331 public Base setProperty(int hash, String name, Base value) throws FHIRException { 332 switch (hash) { 333 case 68050338: // daysOfWeek 334 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 335 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 336 return value; 337 case -1414913477: // allDay 338 this.allDay = TypeConvertor.castToBoolean(value); // BooleanType 339 return value; 340 case -1039453818: // availableStartTime 341 this.availableStartTime = TypeConvertor.castToTime(value); // TimeType 342 return value; 343 case 101151551: // availableEndTime 344 this.availableEndTime = TypeConvertor.castToTime(value); // TimeType 345 return value; 346 default: return super.setProperty(hash, name, value); 347 } 348 349 } 350 351 @Override 352 public Base setProperty(String name, Base value) throws FHIRException { 353 if (name.equals("daysOfWeek")) { 354 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 355 this.getDaysOfWeek().add((Enumeration) value); 356 } else if (name.equals("allDay")) { 357 this.allDay = TypeConvertor.castToBoolean(value); // BooleanType 358 } else if (name.equals("availableStartTime")) { 359 this.availableStartTime = TypeConvertor.castToTime(value); // TimeType 360 } else if (name.equals("availableEndTime")) { 361 this.availableEndTime = TypeConvertor.castToTime(value); // TimeType 362 } else 363 return super.setProperty(name, value); 364 return value; 365 } 366 367 @Override 368 public Base makeProperty(int hash, String name) throws FHIRException { 369 switch (hash) { 370 case 68050338: return addDaysOfWeekElement(); 371 case -1414913477: return getAllDayElement(); 372 case -1039453818: return getAvailableStartTimeElement(); 373 case 101151551: return getAvailableEndTimeElement(); 374 default: return super.makeProperty(hash, name); 375 } 376 377 } 378 379 @Override 380 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 381 switch (hash) { 382 case 68050338: /*daysOfWeek*/ return new String[] {"code"}; 383 case -1414913477: /*allDay*/ return new String[] {"boolean"}; 384 case -1039453818: /*availableStartTime*/ return new String[] {"time"}; 385 case 101151551: /*availableEndTime*/ return new String[] {"time"}; 386 default: return super.getTypesForProperty(hash, name); 387 } 388 389 } 390 391 @Override 392 public Base addChild(String name) throws FHIRException { 393 if (name.equals("daysOfWeek")) { 394 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.daysOfWeek"); 395 } 396 else if (name.equals("allDay")) { 397 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.allDay"); 398 } 399 else if (name.equals("availableStartTime")) { 400 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.availableStartTime"); 401 } 402 else if (name.equals("availableEndTime")) { 403 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.availableEndTime"); 404 } 405 else 406 return super.addChild(name); 407 } 408 409 public AvailabilityAvailableTimeComponent copy() { 410 AvailabilityAvailableTimeComponent dst = new AvailabilityAvailableTimeComponent(); 411 copyValues(dst); 412 return dst; 413 } 414 415 public void copyValues(AvailabilityAvailableTimeComponent dst) { 416 super.copyValues(dst); 417 if (daysOfWeek != null) { 418 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 419 for (Enumeration<DaysOfWeek> i : daysOfWeek) 420 dst.daysOfWeek.add(i.copy()); 421 }; 422 dst.allDay = allDay == null ? null : allDay.copy(); 423 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 424 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 425 } 426 427 @Override 428 public boolean equalsDeep(Base other_) { 429 if (!super.equalsDeep(other_)) 430 return false; 431 if (!(other_ instanceof AvailabilityAvailableTimeComponent)) 432 return false; 433 AvailabilityAvailableTimeComponent o = (AvailabilityAvailableTimeComponent) other_; 434 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) && compareDeep(availableStartTime, o.availableStartTime, true) 435 && compareDeep(availableEndTime, o.availableEndTime, true); 436 } 437 438 @Override 439 public boolean equalsShallow(Base other_) { 440 if (!super.equalsShallow(other_)) 441 return false; 442 if (!(other_ instanceof AvailabilityAvailableTimeComponent)) 443 return false; 444 AvailabilityAvailableTimeComponent o = (AvailabilityAvailableTimeComponent) other_; 445 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) && compareValues(availableStartTime, o.availableStartTime, true) 446 && compareValues(availableEndTime, o.availableEndTime, true); 447 } 448 449 public boolean isEmpty() { 450 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime 451 , availableEndTime); 452 } 453 454 public String fhirType() { 455 return "Availability.availableTime"; 456 457 } 458 459 } 460 461 @Block() 462 public static class AvailabilityNotAvailableTimeComponent extends Element implements IBaseDatatypeElement { 463 /** 464 * Reason presented to the user explaining why time not available. 465 */ 466 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 467 @Description(shortDefinition="Reason presented to the user explaining why time not available", formalDefinition="Reason presented to the user explaining why time not available." ) 468 protected StringType description; 469 470 /** 471 * Service not available during this period. 472 */ 473 @Child(name = "during", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 474 @Description(shortDefinition="Service not available during this period", formalDefinition="Service not available during this period." ) 475 protected Period during; 476 477 private static final long serialVersionUID = 310849929L; 478 479 /** 480 * Constructor 481 */ 482 public AvailabilityNotAvailableTimeComponent() { 483 super(); 484 } 485 486 /** 487 * @return {@link #description} (Reason presented to the user explaining why time not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 488 */ 489 public StringType getDescriptionElement() { 490 if (this.description == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create AvailabilityNotAvailableTimeComponent.description"); 493 else if (Configuration.doAutoCreate()) 494 this.description = new StringType(); // bb 495 return this.description; 496 } 497 498 public boolean hasDescriptionElement() { 499 return this.description != null && !this.description.isEmpty(); 500 } 501 502 public boolean hasDescription() { 503 return this.description != null && !this.description.isEmpty(); 504 } 505 506 /** 507 * @param value {@link #description} (Reason presented to the user explaining why time not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 508 */ 509 public AvailabilityNotAvailableTimeComponent setDescriptionElement(StringType value) { 510 this.description = value; 511 return this; 512 } 513 514 /** 515 * @return Reason presented to the user explaining why time not available. 516 */ 517 public String getDescription() { 518 return this.description == null ? null : this.description.getValue(); 519 } 520 521 /** 522 * @param value Reason presented to the user explaining why time not available. 523 */ 524 public AvailabilityNotAvailableTimeComponent setDescription(String value) { 525 if (Utilities.noString(value)) 526 this.description = null; 527 else { 528 if (this.description == null) 529 this.description = new StringType(); 530 this.description.setValue(value); 531 } 532 return this; 533 } 534 535 /** 536 * @return {@link #during} (Service not available during this period.) 537 */ 538 public Period getDuring() { 539 if (this.during == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create AvailabilityNotAvailableTimeComponent.during"); 542 else if (Configuration.doAutoCreate()) 543 this.during = new Period(); // cc 544 return this.during; 545 } 546 547 public boolean hasDuring() { 548 return this.during != null && !this.during.isEmpty(); 549 } 550 551 /** 552 * @param value {@link #during} (Service not available during this period.) 553 */ 554 public AvailabilityNotAvailableTimeComponent setDuring(Period value) { 555 this.during = value; 556 return this; 557 } 558 559 protected void listChildren(List<Property> children) { 560 super.listChildren(children); 561 children.add(new Property("description", "string", "Reason presented to the user explaining why time not available.", 0, 1, description)); 562 children.add(new Property("during", "Period", "Service not available during this period.", 0, 1, during)); 563 } 564 565 @Override 566 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 567 switch (_hash) { 568 case -1724546052: /*description*/ return new Property("description", "string", "Reason presented to the user explaining why time not available.", 0, 1, description); 569 case -1320499647: /*during*/ return new Property("during", "Period", "Service not available during this period.", 0, 1, during); 570 default: return super.getNamedProperty(_hash, _name, _checkValid); 571 } 572 573 } 574 575 @Override 576 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 577 switch (hash) { 578 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 579 case -1320499647: /*during*/ return this.during == null ? new Base[0] : new Base[] {this.during}; // Period 580 default: return super.getProperty(hash, name, checkValid); 581 } 582 583 } 584 585 @Override 586 public Base setProperty(int hash, String name, Base value) throws FHIRException { 587 switch (hash) { 588 case -1724546052: // description 589 this.description = TypeConvertor.castToString(value); // StringType 590 return value; 591 case -1320499647: // during 592 this.during = TypeConvertor.castToPeriod(value); // Period 593 return value; 594 default: return super.setProperty(hash, name, value); 595 } 596 597 } 598 599 @Override 600 public Base setProperty(String name, Base value) throws FHIRException { 601 if (name.equals("description")) { 602 this.description = TypeConvertor.castToString(value); // StringType 603 } else if (name.equals("during")) { 604 this.during = TypeConvertor.castToPeriod(value); // Period 605 } else 606 return super.setProperty(name, value); 607 return value; 608 } 609 610 @Override 611 public Base makeProperty(int hash, String name) throws FHIRException { 612 switch (hash) { 613 case -1724546052: return getDescriptionElement(); 614 case -1320499647: return getDuring(); 615 default: return super.makeProperty(hash, name); 616 } 617 618 } 619 620 @Override 621 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 622 switch (hash) { 623 case -1724546052: /*description*/ return new String[] {"string"}; 624 case -1320499647: /*during*/ return new String[] {"Period"}; 625 default: return super.getTypesForProperty(hash, name); 626 } 627 628 } 629 630 @Override 631 public Base addChild(String name) throws FHIRException { 632 if (name.equals("description")) { 633 throw new FHIRException("Cannot call addChild on a singleton property Availability.notAvailableTime.description"); 634 } 635 else if (name.equals("during")) { 636 this.during = new Period(); 637 return this.during; 638 } 639 else 640 return super.addChild(name); 641 } 642 643 public AvailabilityNotAvailableTimeComponent copy() { 644 AvailabilityNotAvailableTimeComponent dst = new AvailabilityNotAvailableTimeComponent(); 645 copyValues(dst); 646 return dst; 647 } 648 649 public void copyValues(AvailabilityNotAvailableTimeComponent dst) { 650 super.copyValues(dst); 651 dst.description = description == null ? null : description.copy(); 652 dst.during = during == null ? null : during.copy(); 653 } 654 655 @Override 656 public boolean equalsDeep(Base other_) { 657 if (!super.equalsDeep(other_)) 658 return false; 659 if (!(other_ instanceof AvailabilityNotAvailableTimeComponent)) 660 return false; 661 AvailabilityNotAvailableTimeComponent o = (AvailabilityNotAvailableTimeComponent) other_; 662 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 663 } 664 665 @Override 666 public boolean equalsShallow(Base other_) { 667 if (!super.equalsShallow(other_)) 668 return false; 669 if (!(other_ instanceof AvailabilityNotAvailableTimeComponent)) 670 return false; 671 AvailabilityNotAvailableTimeComponent o = (AvailabilityNotAvailableTimeComponent) other_; 672 return compareValues(description, o.description, true); 673 } 674 675 public boolean isEmpty() { 676 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 677 } 678 679 public String fhirType() { 680 return "Availability.notAvailableTime"; 681 682 } 683 684 } 685 686 /** 687 * Times the {item} is available. 688 */ 689 @Child(name = "availableTime", type = {}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 690 @Description(shortDefinition="Times the {item} is available", formalDefinition="Times the {item} is available." ) 691 protected List<AvailabilityAvailableTimeComponent> availableTime; 692 693 /** 694 * Not available during this time due to provided reason. 695 */ 696 @Child(name = "notAvailableTime", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 697 @Description(shortDefinition="Not available during this time due to provided reason", formalDefinition="Not available during this time due to provided reason." ) 698 protected List<AvailabilityNotAvailableTimeComponent> notAvailableTime; 699 700 private static final long serialVersionUID = -444820754L; 701 702 /** 703 * Constructor 704 */ 705 public Availability() { 706 super(); 707 } 708 709 /** 710 * @return {@link #availableTime} (Times the {item} is available.) 711 */ 712 public List<AvailabilityAvailableTimeComponent> getAvailableTime() { 713 if (this.availableTime == null) 714 this.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 715 return this.availableTime; 716 } 717 718 /** 719 * @return Returns a reference to <code>this</code> for easy method chaining 720 */ 721 public Availability setAvailableTime(List<AvailabilityAvailableTimeComponent> theAvailableTime) { 722 this.availableTime = theAvailableTime; 723 return this; 724 } 725 726 public boolean hasAvailableTime() { 727 if (this.availableTime == null) 728 return false; 729 for (AvailabilityAvailableTimeComponent item : this.availableTime) 730 if (!item.isEmpty()) 731 return true; 732 return false; 733 } 734 735 public AvailabilityAvailableTimeComponent addAvailableTime() { //3 736 AvailabilityAvailableTimeComponent t = new AvailabilityAvailableTimeComponent(); 737 if (this.availableTime == null) 738 this.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 739 this.availableTime.add(t); 740 return t; 741 } 742 743 public Availability addAvailableTime(AvailabilityAvailableTimeComponent t) { //3 744 if (t == null) 745 return this; 746 if (this.availableTime == null) 747 this.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 748 this.availableTime.add(t); 749 return this; 750 } 751 752 /** 753 * @return The first repetition of repeating field {@link #availableTime}, creating it if it does not already exist {3} 754 */ 755 public AvailabilityAvailableTimeComponent getAvailableTimeFirstRep() { 756 if (getAvailableTime().isEmpty()) { 757 addAvailableTime(); 758 } 759 return getAvailableTime().get(0); 760 } 761 762 /** 763 * @return {@link #notAvailableTime} (Not available during this time due to provided reason.) 764 */ 765 public List<AvailabilityNotAvailableTimeComponent> getNotAvailableTime() { 766 if (this.notAvailableTime == null) 767 this.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 768 return this.notAvailableTime; 769 } 770 771 /** 772 * @return Returns a reference to <code>this</code> for easy method chaining 773 */ 774 public Availability setNotAvailableTime(List<AvailabilityNotAvailableTimeComponent> theNotAvailableTime) { 775 this.notAvailableTime = theNotAvailableTime; 776 return this; 777 } 778 779 public boolean hasNotAvailableTime() { 780 if (this.notAvailableTime == null) 781 return false; 782 for (AvailabilityNotAvailableTimeComponent item : this.notAvailableTime) 783 if (!item.isEmpty()) 784 return true; 785 return false; 786 } 787 788 public AvailabilityNotAvailableTimeComponent addNotAvailableTime() { //3 789 AvailabilityNotAvailableTimeComponent t = new AvailabilityNotAvailableTimeComponent(); 790 if (this.notAvailableTime == null) 791 this.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 792 this.notAvailableTime.add(t); 793 return t; 794 } 795 796 public Availability addNotAvailableTime(AvailabilityNotAvailableTimeComponent t) { //3 797 if (t == null) 798 return this; 799 if (this.notAvailableTime == null) 800 this.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 801 this.notAvailableTime.add(t); 802 return this; 803 } 804 805 /** 806 * @return The first repetition of repeating field {@link #notAvailableTime}, creating it if it does not already exist {3} 807 */ 808 public AvailabilityNotAvailableTimeComponent getNotAvailableTimeFirstRep() { 809 if (getNotAvailableTime().isEmpty()) { 810 addNotAvailableTime(); 811 } 812 return getNotAvailableTime().get(0); 813 } 814 815 protected void listChildren(List<Property> children) { 816 super.listChildren(children); 817 children.add(new Property("availableTime", "", "Times the {item} is available.", 0, java.lang.Integer.MAX_VALUE, availableTime)); 818 children.add(new Property("notAvailableTime", "", "Not available during this time due to provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailableTime)); 819 } 820 821 @Override 822 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 823 switch (_hash) { 824 case 1873069366: /*availableTime*/ return new Property("availableTime", "", "Times the {item} is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 825 case -627853021: /*notAvailableTime*/ return new Property("notAvailableTime", "", "Not available during this time due to provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailableTime); 826 default: return super.getNamedProperty(_hash, _name, _checkValid); 827 } 828 829 } 830 831 @Override 832 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 833 switch (hash) { 834 case 1873069366: /*availableTime*/ return this.availableTime == null ? new Base[0] : this.availableTime.toArray(new Base[this.availableTime.size()]); // AvailabilityAvailableTimeComponent 835 case -627853021: /*notAvailableTime*/ return this.notAvailableTime == null ? new Base[0] : this.notAvailableTime.toArray(new Base[this.notAvailableTime.size()]); // AvailabilityNotAvailableTimeComponent 836 default: return super.getProperty(hash, name, checkValid); 837 } 838 839 } 840 841 @Override 842 public Base setProperty(int hash, String name, Base value) throws FHIRException { 843 switch (hash) { 844 case 1873069366: // availableTime 845 this.getAvailableTime().add((AvailabilityAvailableTimeComponent) value); // AvailabilityAvailableTimeComponent 846 return value; 847 case -627853021: // notAvailableTime 848 this.getNotAvailableTime().add((AvailabilityNotAvailableTimeComponent) value); // AvailabilityNotAvailableTimeComponent 849 return value; 850 default: return super.setProperty(hash, name, value); 851 } 852 853 } 854 855 @Override 856 public Base setProperty(String name, Base value) throws FHIRException { 857 if (name.equals("availableTime")) { 858 this.getAvailableTime().add((AvailabilityAvailableTimeComponent) value); 859 } else if (name.equals("notAvailableTime")) { 860 this.getNotAvailableTime().add((AvailabilityNotAvailableTimeComponent) value); 861 } else 862 return super.setProperty(name, value); 863 return value; 864 } 865 866 @Override 867 public Base makeProperty(int hash, String name) throws FHIRException { 868 switch (hash) { 869 case 1873069366: return addAvailableTime(); 870 case -627853021: return addNotAvailableTime(); 871 default: return super.makeProperty(hash, name); 872 } 873 874 } 875 876 @Override 877 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 878 switch (hash) { 879 case 1873069366: /*availableTime*/ return new String[] {}; 880 case -627853021: /*notAvailableTime*/ return new String[] {}; 881 default: return super.getTypesForProperty(hash, name); 882 } 883 884 } 885 886 @Override 887 public Base addChild(String name) throws FHIRException { 888 if (name.equals("availableTime")) { 889 return addAvailableTime(); 890 } 891 else if (name.equals("notAvailableTime")) { 892 return addNotAvailableTime(); 893 } 894 else 895 return super.addChild(name); 896 } 897 898 public String fhirType() { 899 return "Availability"; 900 901 } 902 903 public Availability copy() { 904 Availability dst = new Availability(); 905 copyValues(dst); 906 return dst; 907 } 908 909 public void copyValues(Availability dst) { 910 super.copyValues(dst); 911 if (availableTime != null) { 912 dst.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 913 for (AvailabilityAvailableTimeComponent i : availableTime) 914 dst.availableTime.add(i.copy()); 915 }; 916 if (notAvailableTime != null) { 917 dst.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 918 for (AvailabilityNotAvailableTimeComponent i : notAvailableTime) 919 dst.notAvailableTime.add(i.copy()); 920 }; 921 } 922 923 protected Availability typedCopy() { 924 return copy(); 925 } 926 927 @Override 928 public boolean equalsDeep(Base other_) { 929 if (!super.equalsDeep(other_)) 930 return false; 931 if (!(other_ instanceof Availability)) 932 return false; 933 Availability o = (Availability) other_; 934 return compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailableTime, o.notAvailableTime, true) 935 ; 936 } 937 938 @Override 939 public boolean equalsShallow(Base other_) { 940 if (!super.equalsShallow(other_)) 941 return false; 942 if (!(other_ instanceof Availability)) 943 return false; 944 Availability o = (Availability) other_; 945 return true; 946 } 947 948 public boolean isEmpty() { 949 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(availableTime, notAvailableTime 950 ); 951 } 952 953 954} 955