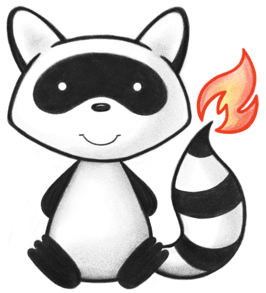
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Availability Type: Availability data for an {item}. 050 */ 051@DatatypeDef(name="Availability") 052public class Availability extends DataType implements ICompositeType { 053 054 @Block() 055 public static class AvailabilityAvailableTimeComponent extends Element implements IBaseDatatypeElement { 056 /** 057 * mon | tue | wed | thu | fri | sat | sun. 058 */ 059 @Child(name = "daysOfWeek", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 060 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="mon | tue | wed | thu | fri | sat | sun." ) 061 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 062 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 063 064 /** 065 * Always available? i.e. 24 hour service. 066 */ 067 @Child(name = "allDay", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 068 @Description(shortDefinition="Always available? i.e. 24 hour service", formalDefinition="Always available? i.e. 24 hour service." ) 069 protected BooleanType allDay; 070 071 /** 072 * Opening time of day (ignored if allDay = true). 073 */ 074 @Child(name = "availableStartTime", type = {TimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 075 @Description(shortDefinition="Opening time of day (ignored if allDay = true)", formalDefinition="Opening time of day (ignored if allDay = true)." ) 076 protected TimeType availableStartTime; 077 078 /** 079 * Closing time of day (ignored if allDay = true). 080 */ 081 @Child(name = "availableEndTime", type = {TimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 082 @Description(shortDefinition="Closing time of day (ignored if allDay = true)", formalDefinition="Closing time of day (ignored if allDay = true)." ) 083 protected TimeType availableEndTime; 084 085 private static final long serialVersionUID = -2139510127L; 086 087 /** 088 * Constructor 089 */ 090 public AvailabilityAvailableTimeComponent() { 091 super(); 092 } 093 094 /** 095 * @return {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 096 */ 097 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 098 if (this.daysOfWeek == null) 099 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 100 return this.daysOfWeek; 101 } 102 103 /** 104 * @return Returns a reference to <code>this</code> for easy method chaining 105 */ 106 public AvailabilityAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 107 this.daysOfWeek = theDaysOfWeek; 108 return this; 109 } 110 111 public boolean hasDaysOfWeek() { 112 if (this.daysOfWeek == null) 113 return false; 114 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 115 if (!item.isEmpty()) 116 return true; 117 return false; 118 } 119 120 /** 121 * @return {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 122 */ 123 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {//2 124 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 125 if (this.daysOfWeek == null) 126 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 127 this.daysOfWeek.add(t); 128 return t; 129 } 130 131 /** 132 * @param value {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 133 */ 134 public AvailabilityAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { //1 135 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 136 t.setValue(value); 137 if (this.daysOfWeek == null) 138 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 139 this.daysOfWeek.add(t); 140 return this; 141 } 142 143 /** 144 * @param value {@link #daysOfWeek} (mon | tue | wed | thu | fri | sat | sun.) 145 */ 146 public boolean hasDaysOfWeek(DaysOfWeek value) { 147 if (this.daysOfWeek == null) 148 return false; 149 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 150 if (v.getValue().equals(value)) // code 151 return true; 152 return false; 153 } 154 155 /** 156 * @return {@link #allDay} (Always available? i.e. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 157 */ 158 public BooleanType getAllDayElement() { 159 if (this.allDay == null) 160 if (Configuration.errorOnAutoCreate()) 161 throw new Error("Attempt to auto-create AvailabilityAvailableTimeComponent.allDay"); 162 else if (Configuration.doAutoCreate()) 163 this.allDay = new BooleanType(); // bb 164 return this.allDay; 165 } 166 167 public boolean hasAllDayElement() { 168 return this.allDay != null && !this.allDay.isEmpty(); 169 } 170 171 public boolean hasAllDay() { 172 return this.allDay != null && !this.allDay.isEmpty(); 173 } 174 175 /** 176 * @param value {@link #allDay} (Always available? i.e. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 177 */ 178 public AvailabilityAvailableTimeComponent setAllDayElement(BooleanType value) { 179 this.allDay = value; 180 return this; 181 } 182 183 /** 184 * @return Always available? i.e. 24 hour service. 185 */ 186 public boolean getAllDay() { 187 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 188 } 189 190 /** 191 * @param value Always available? i.e. 24 hour service. 192 */ 193 public AvailabilityAvailableTimeComponent setAllDay(boolean value) { 194 if (this.allDay == null) 195 this.allDay = new BooleanType(); 196 this.allDay.setValue(value); 197 return this; 198 } 199 200 /** 201 * @return {@link #availableStartTime} (Opening time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 202 */ 203 public TimeType getAvailableStartTimeElement() { 204 if (this.availableStartTime == null) 205 if (Configuration.errorOnAutoCreate()) 206 throw new Error("Attempt to auto-create AvailabilityAvailableTimeComponent.availableStartTime"); 207 else if (Configuration.doAutoCreate()) 208 this.availableStartTime = new TimeType(); // bb 209 return this.availableStartTime; 210 } 211 212 public boolean hasAvailableStartTimeElement() { 213 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 214 } 215 216 public boolean hasAvailableStartTime() { 217 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #availableStartTime} (Opening time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 222 */ 223 public AvailabilityAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 224 this.availableStartTime = value; 225 return this; 226 } 227 228 /** 229 * @return Opening time of day (ignored if allDay = true). 230 */ 231 public String getAvailableStartTime() { 232 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 233 } 234 235 /** 236 * @param value Opening time of day (ignored if allDay = true). 237 */ 238 public AvailabilityAvailableTimeComponent setAvailableStartTime(String value) { 239 if (value == null) 240 this.availableStartTime = null; 241 else { 242 if (this.availableStartTime == null) 243 this.availableStartTime = new TimeType(); 244 this.availableStartTime.setValue(value); 245 } 246 return this; 247 } 248 249 /** 250 * @return {@link #availableEndTime} (Closing time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 251 */ 252 public TimeType getAvailableEndTimeElement() { 253 if (this.availableEndTime == null) 254 if (Configuration.errorOnAutoCreate()) 255 throw new Error("Attempt to auto-create AvailabilityAvailableTimeComponent.availableEndTime"); 256 else if (Configuration.doAutoCreate()) 257 this.availableEndTime = new TimeType(); // bb 258 return this.availableEndTime; 259 } 260 261 public boolean hasAvailableEndTimeElement() { 262 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 263 } 264 265 public boolean hasAvailableEndTime() { 266 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 267 } 268 269 /** 270 * @param value {@link #availableEndTime} (Closing time of day (ignored if allDay = true).). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 271 */ 272 public AvailabilityAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 273 this.availableEndTime = value; 274 return this; 275 } 276 277 /** 278 * @return Closing time of day (ignored if allDay = true). 279 */ 280 public String getAvailableEndTime() { 281 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 282 } 283 284 /** 285 * @param value Closing time of day (ignored if allDay = true). 286 */ 287 public AvailabilityAvailableTimeComponent setAvailableEndTime(String value) { 288 if (value == null) 289 this.availableEndTime = null; 290 else { 291 if (this.availableEndTime == null) 292 this.availableEndTime = new TimeType(); 293 this.availableEndTime.setValue(value); 294 } 295 return this; 296 } 297 298 protected void listChildren(List<Property> children) { 299 super.listChildren(children); 300 children.add(new Property("daysOfWeek", "code", "mon | tue | wed | thu | fri | sat | sun.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek)); 301 children.add(new Property("allDay", "boolean", "Always available? i.e. 24 hour service.", 0, 1, allDay)); 302 children.add(new Property("availableStartTime", "time", "Opening time of day (ignored if allDay = true).", 0, 1, availableStartTime)); 303 children.add(new Property("availableEndTime", "time", "Closing time of day (ignored if allDay = true).", 0, 1, availableEndTime)); 304 } 305 306 @Override 307 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 308 switch (_hash) { 309 case 68050338: /*daysOfWeek*/ return new Property("daysOfWeek", "code", "mon | tue | wed | thu | fri | sat | sun.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek); 310 case -1414913477: /*allDay*/ return new Property("allDay", "boolean", "Always available? i.e. 24 hour service.", 0, 1, allDay); 311 case -1039453818: /*availableStartTime*/ return new Property("availableStartTime", "time", "Opening time of day (ignored if allDay = true).", 0, 1, availableStartTime); 312 case 101151551: /*availableEndTime*/ return new Property("availableEndTime", "time", "Closing time of day (ignored if allDay = true).", 0, 1, availableEndTime); 313 default: return super.getNamedProperty(_hash, _name, _checkValid); 314 } 315 316 } 317 318 @Override 319 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 320 switch (hash) { 321 case 68050338: /*daysOfWeek*/ return this.daysOfWeek == null ? new Base[0] : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 322 case -1414913477: /*allDay*/ return this.allDay == null ? new Base[0] : new Base[] {this.allDay}; // BooleanType 323 case -1039453818: /*availableStartTime*/ return this.availableStartTime == null ? new Base[0] : new Base[] {this.availableStartTime}; // TimeType 324 case 101151551: /*availableEndTime*/ return this.availableEndTime == null ? new Base[0] : new Base[] {this.availableEndTime}; // TimeType 325 default: return super.getProperty(hash, name, checkValid); 326 } 327 328 } 329 330 @Override 331 public Base setProperty(int hash, String name, Base value) throws FHIRException { 332 switch (hash) { 333 case 68050338: // daysOfWeek 334 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 335 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 336 return value; 337 case -1414913477: // allDay 338 this.allDay = TypeConvertor.castToBoolean(value); // BooleanType 339 return value; 340 case -1039453818: // availableStartTime 341 this.availableStartTime = TypeConvertor.castToTime(value); // TimeType 342 return value; 343 case 101151551: // availableEndTime 344 this.availableEndTime = TypeConvertor.castToTime(value); // TimeType 345 return value; 346 default: return super.setProperty(hash, name, value); 347 } 348 349 } 350 351 @Override 352 public Base setProperty(String name, Base value) throws FHIRException { 353 if (name.equals("daysOfWeek")) { 354 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 355 this.getDaysOfWeek().add((Enumeration) value); 356 } else if (name.equals("allDay")) { 357 this.allDay = TypeConvertor.castToBoolean(value); // BooleanType 358 } else if (name.equals("availableStartTime")) { 359 this.availableStartTime = TypeConvertor.castToTime(value); // TimeType 360 } else if (name.equals("availableEndTime")) { 361 this.availableEndTime = TypeConvertor.castToTime(value); // TimeType 362 } else 363 return super.setProperty(name, value); 364 return value; 365 } 366 367 @Override 368 public void removeChild(String name, Base value) throws FHIRException { 369 if (name.equals("daysOfWeek")) { 370 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 371 this.getDaysOfWeek().remove((Enumeration) value); 372 } else if (name.equals("allDay")) { 373 this.allDay = null; 374 } else if (name.equals("availableStartTime")) { 375 this.availableStartTime = null; 376 } else if (name.equals("availableEndTime")) { 377 this.availableEndTime = null; 378 } else 379 super.removeChild(name, value); 380 381 } 382 383 @Override 384 public Base makeProperty(int hash, String name) throws FHIRException { 385 switch (hash) { 386 case 68050338: return addDaysOfWeekElement(); 387 case -1414913477: return getAllDayElement(); 388 case -1039453818: return getAvailableStartTimeElement(); 389 case 101151551: return getAvailableEndTimeElement(); 390 default: return super.makeProperty(hash, name); 391 } 392 393 } 394 395 @Override 396 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 397 switch (hash) { 398 case 68050338: /*daysOfWeek*/ return new String[] {"code"}; 399 case -1414913477: /*allDay*/ return new String[] {"boolean"}; 400 case -1039453818: /*availableStartTime*/ return new String[] {"time"}; 401 case 101151551: /*availableEndTime*/ return new String[] {"time"}; 402 default: return super.getTypesForProperty(hash, name); 403 } 404 405 } 406 407 @Override 408 public Base addChild(String name) throws FHIRException { 409 if (name.equals("daysOfWeek")) { 410 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.daysOfWeek"); 411 } 412 else if (name.equals("allDay")) { 413 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.allDay"); 414 } 415 else if (name.equals("availableStartTime")) { 416 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.availableStartTime"); 417 } 418 else if (name.equals("availableEndTime")) { 419 throw new FHIRException("Cannot call addChild on a singleton property Availability.availableTime.availableEndTime"); 420 } 421 else 422 return super.addChild(name); 423 } 424 425 public AvailabilityAvailableTimeComponent copy() { 426 AvailabilityAvailableTimeComponent dst = new AvailabilityAvailableTimeComponent(); 427 copyValues(dst); 428 return dst; 429 } 430 431 public void copyValues(AvailabilityAvailableTimeComponent dst) { 432 super.copyValues(dst); 433 if (daysOfWeek != null) { 434 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 435 for (Enumeration<DaysOfWeek> i : daysOfWeek) 436 dst.daysOfWeek.add(i.copy()); 437 }; 438 dst.allDay = allDay == null ? null : allDay.copy(); 439 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 440 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 441 } 442 443 @Override 444 public boolean equalsDeep(Base other_) { 445 if (!super.equalsDeep(other_)) 446 return false; 447 if (!(other_ instanceof AvailabilityAvailableTimeComponent)) 448 return false; 449 AvailabilityAvailableTimeComponent o = (AvailabilityAvailableTimeComponent) other_; 450 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) && compareDeep(availableStartTime, o.availableStartTime, true) 451 && compareDeep(availableEndTime, o.availableEndTime, true); 452 } 453 454 @Override 455 public boolean equalsShallow(Base other_) { 456 if (!super.equalsShallow(other_)) 457 return false; 458 if (!(other_ instanceof AvailabilityAvailableTimeComponent)) 459 return false; 460 AvailabilityAvailableTimeComponent o = (AvailabilityAvailableTimeComponent) other_; 461 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) && compareValues(availableStartTime, o.availableStartTime, true) 462 && compareValues(availableEndTime, o.availableEndTime, true); 463 } 464 465 public boolean isEmpty() { 466 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime 467 , availableEndTime); 468 } 469 470 public String fhirType() { 471 return "Availability.availableTime"; 472 473 } 474 475 } 476 477 @Block() 478 public static class AvailabilityNotAvailableTimeComponent extends Element implements IBaseDatatypeElement { 479 /** 480 * Reason presented to the user explaining why time not available. 481 */ 482 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 483 @Description(shortDefinition="Reason presented to the user explaining why time not available", formalDefinition="Reason presented to the user explaining why time not available." ) 484 protected StringType description; 485 486 /** 487 * Service not available during this period. 488 */ 489 @Child(name = "during", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 490 @Description(shortDefinition="Service not available during this period", formalDefinition="Service not available during this period." ) 491 protected Period during; 492 493 private static final long serialVersionUID = 310849929L; 494 495 /** 496 * Constructor 497 */ 498 public AvailabilityNotAvailableTimeComponent() { 499 super(); 500 } 501 502 /** 503 * @return {@link #description} (Reason presented to the user explaining why time not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 504 */ 505 public StringType getDescriptionElement() { 506 if (this.description == null) 507 if (Configuration.errorOnAutoCreate()) 508 throw new Error("Attempt to auto-create AvailabilityNotAvailableTimeComponent.description"); 509 else if (Configuration.doAutoCreate()) 510 this.description = new StringType(); // bb 511 return this.description; 512 } 513 514 public boolean hasDescriptionElement() { 515 return this.description != null && !this.description.isEmpty(); 516 } 517 518 public boolean hasDescription() { 519 return this.description != null && !this.description.isEmpty(); 520 } 521 522 /** 523 * @param value {@link #description} (Reason presented to the user explaining why time not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 524 */ 525 public AvailabilityNotAvailableTimeComponent setDescriptionElement(StringType value) { 526 this.description = value; 527 return this; 528 } 529 530 /** 531 * @return Reason presented to the user explaining why time not available. 532 */ 533 public String getDescription() { 534 return this.description == null ? null : this.description.getValue(); 535 } 536 537 /** 538 * @param value Reason presented to the user explaining why time not available. 539 */ 540 public AvailabilityNotAvailableTimeComponent setDescription(String value) { 541 if (Utilities.noString(value)) 542 this.description = null; 543 else { 544 if (this.description == null) 545 this.description = new StringType(); 546 this.description.setValue(value); 547 } 548 return this; 549 } 550 551 /** 552 * @return {@link #during} (Service not available during this period.) 553 */ 554 public Period getDuring() { 555 if (this.during == null) 556 if (Configuration.errorOnAutoCreate()) 557 throw new Error("Attempt to auto-create AvailabilityNotAvailableTimeComponent.during"); 558 else if (Configuration.doAutoCreate()) 559 this.during = new Period(); // cc 560 return this.during; 561 } 562 563 public boolean hasDuring() { 564 return this.during != null && !this.during.isEmpty(); 565 } 566 567 /** 568 * @param value {@link #during} (Service not available during this period.) 569 */ 570 public AvailabilityNotAvailableTimeComponent setDuring(Period value) { 571 this.during = value; 572 return this; 573 } 574 575 protected void listChildren(List<Property> children) { 576 super.listChildren(children); 577 children.add(new Property("description", "string", "Reason presented to the user explaining why time not available.", 0, 1, description)); 578 children.add(new Property("during", "Period", "Service not available during this period.", 0, 1, during)); 579 } 580 581 @Override 582 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 583 switch (_hash) { 584 case -1724546052: /*description*/ return new Property("description", "string", "Reason presented to the user explaining why time not available.", 0, 1, description); 585 case -1320499647: /*during*/ return new Property("during", "Period", "Service not available during this period.", 0, 1, during); 586 default: return super.getNamedProperty(_hash, _name, _checkValid); 587 } 588 589 } 590 591 @Override 592 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 593 switch (hash) { 594 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 595 case -1320499647: /*during*/ return this.during == null ? new Base[0] : new Base[] {this.during}; // Period 596 default: return super.getProperty(hash, name, checkValid); 597 } 598 599 } 600 601 @Override 602 public Base setProperty(int hash, String name, Base value) throws FHIRException { 603 switch (hash) { 604 case -1724546052: // description 605 this.description = TypeConvertor.castToString(value); // StringType 606 return value; 607 case -1320499647: // during 608 this.during = TypeConvertor.castToPeriod(value); // Period 609 return value; 610 default: return super.setProperty(hash, name, value); 611 } 612 613 } 614 615 @Override 616 public Base setProperty(String name, Base value) throws FHIRException { 617 if (name.equals("description")) { 618 this.description = TypeConvertor.castToString(value); // StringType 619 } else if (name.equals("during")) { 620 this.during = TypeConvertor.castToPeriod(value); // Period 621 } else 622 return super.setProperty(name, value); 623 return value; 624 } 625 626 @Override 627 public void removeChild(String name, Base value) throws FHIRException { 628 if (name.equals("description")) { 629 this.description = null; 630 } else if (name.equals("during")) { 631 this.during = null; 632 } else 633 super.removeChild(name, value); 634 635 } 636 637 @Override 638 public Base makeProperty(int hash, String name) throws FHIRException { 639 switch (hash) { 640 case -1724546052: return getDescriptionElement(); 641 case -1320499647: return getDuring(); 642 default: return super.makeProperty(hash, name); 643 } 644 645 } 646 647 @Override 648 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 649 switch (hash) { 650 case -1724546052: /*description*/ return new String[] {"string"}; 651 case -1320499647: /*during*/ return new String[] {"Period"}; 652 default: return super.getTypesForProperty(hash, name); 653 } 654 655 } 656 657 @Override 658 public Base addChild(String name) throws FHIRException { 659 if (name.equals("description")) { 660 throw new FHIRException("Cannot call addChild on a singleton property Availability.notAvailableTime.description"); 661 } 662 else if (name.equals("during")) { 663 this.during = new Period(); 664 return this.during; 665 } 666 else 667 return super.addChild(name); 668 } 669 670 public AvailabilityNotAvailableTimeComponent copy() { 671 AvailabilityNotAvailableTimeComponent dst = new AvailabilityNotAvailableTimeComponent(); 672 copyValues(dst); 673 return dst; 674 } 675 676 public void copyValues(AvailabilityNotAvailableTimeComponent dst) { 677 super.copyValues(dst); 678 dst.description = description == null ? null : description.copy(); 679 dst.during = during == null ? null : during.copy(); 680 } 681 682 @Override 683 public boolean equalsDeep(Base other_) { 684 if (!super.equalsDeep(other_)) 685 return false; 686 if (!(other_ instanceof AvailabilityNotAvailableTimeComponent)) 687 return false; 688 AvailabilityNotAvailableTimeComponent o = (AvailabilityNotAvailableTimeComponent) other_; 689 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 690 } 691 692 @Override 693 public boolean equalsShallow(Base other_) { 694 if (!super.equalsShallow(other_)) 695 return false; 696 if (!(other_ instanceof AvailabilityNotAvailableTimeComponent)) 697 return false; 698 AvailabilityNotAvailableTimeComponent o = (AvailabilityNotAvailableTimeComponent) other_; 699 return compareValues(description, o.description, true); 700 } 701 702 public boolean isEmpty() { 703 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 704 } 705 706 public String fhirType() { 707 return "Availability.notAvailableTime"; 708 709 } 710 711 } 712 713 /** 714 * Times the {item} is available. 715 */ 716 @Child(name = "availableTime", type = {}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 717 @Description(shortDefinition="Times the {item} is available", formalDefinition="Times the {item} is available." ) 718 protected List<AvailabilityAvailableTimeComponent> availableTime; 719 720 /** 721 * Not available during this time due to provided reason. 722 */ 723 @Child(name = "notAvailableTime", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 724 @Description(shortDefinition="Not available during this time due to provided reason", formalDefinition="Not available during this time due to provided reason." ) 725 protected List<AvailabilityNotAvailableTimeComponent> notAvailableTime; 726 727 private static final long serialVersionUID = -444820754L; 728 729 /** 730 * Constructor 731 */ 732 public Availability() { 733 super(); 734 } 735 736 /** 737 * @return {@link #availableTime} (Times the {item} is available.) 738 */ 739 public List<AvailabilityAvailableTimeComponent> getAvailableTime() { 740 if (this.availableTime == null) 741 this.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 742 return this.availableTime; 743 } 744 745 /** 746 * @return Returns a reference to <code>this</code> for easy method chaining 747 */ 748 public Availability setAvailableTime(List<AvailabilityAvailableTimeComponent> theAvailableTime) { 749 this.availableTime = theAvailableTime; 750 return this; 751 } 752 753 public boolean hasAvailableTime() { 754 if (this.availableTime == null) 755 return false; 756 for (AvailabilityAvailableTimeComponent item : this.availableTime) 757 if (!item.isEmpty()) 758 return true; 759 return false; 760 } 761 762 public AvailabilityAvailableTimeComponent addAvailableTime() { //3 763 AvailabilityAvailableTimeComponent t = new AvailabilityAvailableTimeComponent(); 764 if (this.availableTime == null) 765 this.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 766 this.availableTime.add(t); 767 return t; 768 } 769 770 public Availability addAvailableTime(AvailabilityAvailableTimeComponent t) { //3 771 if (t == null) 772 return this; 773 if (this.availableTime == null) 774 this.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 775 this.availableTime.add(t); 776 return this; 777 } 778 779 /** 780 * @return The first repetition of repeating field {@link #availableTime}, creating it if it does not already exist {3} 781 */ 782 public AvailabilityAvailableTimeComponent getAvailableTimeFirstRep() { 783 if (getAvailableTime().isEmpty()) { 784 addAvailableTime(); 785 } 786 return getAvailableTime().get(0); 787 } 788 789 /** 790 * @return {@link #notAvailableTime} (Not available during this time due to provided reason.) 791 */ 792 public List<AvailabilityNotAvailableTimeComponent> getNotAvailableTime() { 793 if (this.notAvailableTime == null) 794 this.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 795 return this.notAvailableTime; 796 } 797 798 /** 799 * @return Returns a reference to <code>this</code> for easy method chaining 800 */ 801 public Availability setNotAvailableTime(List<AvailabilityNotAvailableTimeComponent> theNotAvailableTime) { 802 this.notAvailableTime = theNotAvailableTime; 803 return this; 804 } 805 806 public boolean hasNotAvailableTime() { 807 if (this.notAvailableTime == null) 808 return false; 809 for (AvailabilityNotAvailableTimeComponent item : this.notAvailableTime) 810 if (!item.isEmpty()) 811 return true; 812 return false; 813 } 814 815 public AvailabilityNotAvailableTimeComponent addNotAvailableTime() { //3 816 AvailabilityNotAvailableTimeComponent t = new AvailabilityNotAvailableTimeComponent(); 817 if (this.notAvailableTime == null) 818 this.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 819 this.notAvailableTime.add(t); 820 return t; 821 } 822 823 public Availability addNotAvailableTime(AvailabilityNotAvailableTimeComponent t) { //3 824 if (t == null) 825 return this; 826 if (this.notAvailableTime == null) 827 this.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 828 this.notAvailableTime.add(t); 829 return this; 830 } 831 832 /** 833 * @return The first repetition of repeating field {@link #notAvailableTime}, creating it if it does not already exist {3} 834 */ 835 public AvailabilityNotAvailableTimeComponent getNotAvailableTimeFirstRep() { 836 if (getNotAvailableTime().isEmpty()) { 837 addNotAvailableTime(); 838 } 839 return getNotAvailableTime().get(0); 840 } 841 842 protected void listChildren(List<Property> children) { 843 super.listChildren(children); 844 children.add(new Property("availableTime", "", "Times the {item} is available.", 0, java.lang.Integer.MAX_VALUE, availableTime)); 845 children.add(new Property("notAvailableTime", "", "Not available during this time due to provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailableTime)); 846 } 847 848 @Override 849 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 850 switch (_hash) { 851 case 1873069366: /*availableTime*/ return new Property("availableTime", "", "Times the {item} is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 852 case -627853021: /*notAvailableTime*/ return new Property("notAvailableTime", "", "Not available during this time due to provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailableTime); 853 default: return super.getNamedProperty(_hash, _name, _checkValid); 854 } 855 856 } 857 858 @Override 859 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 860 switch (hash) { 861 case 1873069366: /*availableTime*/ return this.availableTime == null ? new Base[0] : this.availableTime.toArray(new Base[this.availableTime.size()]); // AvailabilityAvailableTimeComponent 862 case -627853021: /*notAvailableTime*/ return this.notAvailableTime == null ? new Base[0] : this.notAvailableTime.toArray(new Base[this.notAvailableTime.size()]); // AvailabilityNotAvailableTimeComponent 863 default: return super.getProperty(hash, name, checkValid); 864 } 865 866 } 867 868 @Override 869 public Base setProperty(int hash, String name, Base value) throws FHIRException { 870 switch (hash) { 871 case 1873069366: // availableTime 872 this.getAvailableTime().add((AvailabilityAvailableTimeComponent) value); // AvailabilityAvailableTimeComponent 873 return value; 874 case -627853021: // notAvailableTime 875 this.getNotAvailableTime().add((AvailabilityNotAvailableTimeComponent) value); // AvailabilityNotAvailableTimeComponent 876 return value; 877 default: return super.setProperty(hash, name, value); 878 } 879 880 } 881 882 @Override 883 public Base setProperty(String name, Base value) throws FHIRException { 884 if (name.equals("availableTime")) { 885 this.getAvailableTime().add((AvailabilityAvailableTimeComponent) value); 886 } else if (name.equals("notAvailableTime")) { 887 this.getNotAvailableTime().add((AvailabilityNotAvailableTimeComponent) value); 888 } else 889 return super.setProperty(name, value); 890 return value; 891 } 892 893 @Override 894 public void removeChild(String name, Base value) throws FHIRException { 895 if (name.equals("availableTime")) { 896 this.getAvailableTime().remove((AvailabilityAvailableTimeComponent) value); 897 } else if (name.equals("notAvailableTime")) { 898 this.getNotAvailableTime().remove((AvailabilityNotAvailableTimeComponent) value); 899 } else 900 super.removeChild(name, value); 901 902 } 903 904 @Override 905 public Base makeProperty(int hash, String name) throws FHIRException { 906 switch (hash) { 907 case 1873069366: return addAvailableTime(); 908 case -627853021: return addNotAvailableTime(); 909 default: return super.makeProperty(hash, name); 910 } 911 912 } 913 914 @Override 915 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 916 switch (hash) { 917 case 1873069366: /*availableTime*/ return new String[] {}; 918 case -627853021: /*notAvailableTime*/ return new String[] {}; 919 default: return super.getTypesForProperty(hash, name); 920 } 921 922 } 923 924 @Override 925 public Base addChild(String name) throws FHIRException { 926 if (name.equals("availableTime")) { 927 return addAvailableTime(); 928 } 929 else if (name.equals("notAvailableTime")) { 930 return addNotAvailableTime(); 931 } 932 else 933 return super.addChild(name); 934 } 935 936 public String fhirType() { 937 return "Availability"; 938 939 } 940 941 public Availability copy() { 942 Availability dst = new Availability(); 943 copyValues(dst); 944 return dst; 945 } 946 947 public void copyValues(Availability dst) { 948 super.copyValues(dst); 949 if (availableTime != null) { 950 dst.availableTime = new ArrayList<AvailabilityAvailableTimeComponent>(); 951 for (AvailabilityAvailableTimeComponent i : availableTime) 952 dst.availableTime.add(i.copy()); 953 }; 954 if (notAvailableTime != null) { 955 dst.notAvailableTime = new ArrayList<AvailabilityNotAvailableTimeComponent>(); 956 for (AvailabilityNotAvailableTimeComponent i : notAvailableTime) 957 dst.notAvailableTime.add(i.copy()); 958 }; 959 } 960 961 protected Availability typedCopy() { 962 return copy(); 963 } 964 965 @Override 966 public boolean equalsDeep(Base other_) { 967 if (!super.equalsDeep(other_)) 968 return false; 969 if (!(other_ instanceof Availability)) 970 return false; 971 Availability o = (Availability) other_; 972 return compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailableTime, o.notAvailableTime, true) 973 ; 974 } 975 976 @Override 977 public boolean equalsShallow(Base other_) { 978 if (!super.equalsShallow(other_)) 979 return false; 980 if (!(other_ instanceof Availability)) 981 return false; 982 Availability o = (Availability) other_; 983 return true; 984 } 985 986 public boolean isEmpty() { 987 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(availableTime, notAvailableTime 988 ); 989 } 990 991 992} 993