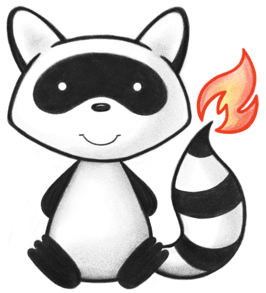
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * BackboneElement Type: Base definition for all elements that are defined inside a resource - but not those in a data type. 051 */ 052@DatatypeDef(name="BackboneElement") 053public abstract class BackboneElement extends Element implements IBaseBackboneElement { 054 055 /** 056 * May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 057 058Modifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself). 059 */ 060 @Child(name = "modifierExtension", type = {Extension.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 061 @Description(shortDefinition="Extensions that cannot be ignored even if unrecognized", formalDefinition="May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself)." ) 062 protected List<Extension> modifierExtension; 063 064 private static final long serialVersionUID = -1431673179L; 065 066 /** 067 * Constructor 068 */ 069 public BackboneElement() { 070 super(); 071 } 072 073 /** 074 * @return {@link #modifierExtension} (May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 075 076Modifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).) 077 */ 078 public List<Extension> getModifierExtension() { 079 if (this.modifierExtension == null) 080 this.modifierExtension = new ArrayList<Extension>(); 081 return this.modifierExtension; 082 } 083 084 /** 085 * @return Returns a reference to <code>this</code> for easy method chaining 086 */ 087 public BackboneElement setModifierExtension(List<Extension> theModifierExtension) { 088 this.modifierExtension = theModifierExtension; 089 return this; 090 } 091 092 public boolean hasModifierExtension() { 093 if (this.modifierExtension == null) 094 return false; 095 for (Extension item : this.modifierExtension) 096 if (!item.isEmpty()) 097 return true; 098 return false; 099 } 100 101 public Extension addModifierExtension() { //3 102 Extension t = new Extension(); 103 if (this.modifierExtension == null) 104 this.modifierExtension = new ArrayList<Extension>(); 105 this.modifierExtension.add(t); 106 return t; 107 } 108 109 public BackboneElement addModifierExtension(Extension t) { //3 110 if (t == null) 111 return this; 112 if (this.modifierExtension == null) 113 this.modifierExtension = new ArrayList<Extension>(); 114 this.modifierExtension.add(t); 115 return this; 116 } 117 118 /** 119 * @return The first repetition of repeating field {@link #modifierExtension}, creating it if it does not already exist {3} 120 */ 121 public Extension getModifierExtensionFirstRep() { 122 if (getModifierExtension().isEmpty()) { 123 addModifierExtension(); 124 } 125 return getModifierExtension().get(0); 126 } 127 128 protected void listChildren(List<Property> children) { 129 super.listChildren(children); 130 children.add(new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 131 } 132 133 @Override 134 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 135 switch (_hash) { 136 case -298878168: /*modifierExtension*/ return new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 0, java.lang.Integer.MAX_VALUE, modifierExtension); 137 default: return super.getNamedProperty(_hash, _name, _checkValid); 138 } 139 140 } 141 142 @Override 143 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 144 switch (hash) { 145 case -298878168: /*modifierExtension*/ return this.modifierExtension == null ? new Base[0] : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 146 default: return super.getProperty(hash, name, checkValid); 147 } 148 149 } 150 151 @Override 152 public Base setProperty(int hash, String name, Base value) throws FHIRException { 153 switch (hash) { 154 case -298878168: // modifierExtension 155 this.getModifierExtension().add(TypeConvertor.castToExtension(value)); // Extension 156 return value; 157 default: return super.setProperty(hash, name, value); 158 } 159 160 } 161 162 @Override 163 public Base setProperty(String name, Base value) throws FHIRException { 164 if (name.equals("modifierExtension")) { 165 this.getModifierExtension().add(TypeConvertor.castToExtension(value)); 166 } else 167 return super.setProperty(name, value); 168 return value; 169 } 170 171 @Override 172 public void removeChild(String name, Base value) throws FHIRException { 173 if (name.equals("modifierExtension")) { 174 this.getModifierExtension().remove(value); 175 } else 176 super.removeChild(name, value); 177 178 } 179 180 @Override 181 public Base makeProperty(int hash, String name) throws FHIRException { 182 switch (hash) { 183 case -298878168: return addModifierExtension(); 184 default: return super.makeProperty(hash, name); 185 } 186 187 } 188 189 @Override 190 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 191 switch (hash) { 192 case -298878168: /*modifierExtension*/ return new String[] {"Extension"}; 193 default: return super.getTypesForProperty(hash, name); 194 } 195 196 } 197 198 @Override 199 public Base addChild(String name) throws FHIRException { 200 if (name.equals("modifierExtension")) { 201 return addModifierExtension(); 202 } 203 else 204 return super.addChild(name); 205 } 206 207 public String fhirType() { 208 return "BackboneElement"; 209 210 } 211 212 public abstract BackboneElement copy(); 213 214 public void copyValues(BackboneElement dst) { 215 super.copyValues(dst); 216 if (modifierExtension != null) { 217 dst.modifierExtension = new ArrayList<Extension>(); 218 for (Extension i : modifierExtension) 219 dst.modifierExtension.add(i.copy()); 220 }; 221 } 222 223 @Override 224 public boolean equalsDeep(Base other_) { 225 if (!super.equalsDeep(other_)) 226 return false; 227 if (!(other_ instanceof BackboneElement)) 228 return false; 229 BackboneElement o = (BackboneElement) other_; 230 return compareDeep(modifierExtension, o.modifierExtension, true); 231 } 232 233 @Override 234 public boolean equalsShallow(Base other_) { 235 if (!super.equalsShallow(other_)) 236 return false; 237 if (!(other_ instanceof BackboneElement)) 238 return false; 239 BackboneElement o = (BackboneElement) other_; 240 return true; 241 } 242 243 public boolean isEmpty() { 244 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifierExtension); 245 } 246 247// Manual code (from Configuration.txt): 248 public void checkNoModifiers(String noun, String verb) throws FHIRException { 249 if (hasModifierExtension()) { 250 throw new FHIRException("Found unknown Modifier Exceptions on "+noun+" doing "+verb); 251 } 252 253 } 254 255 public void addModifierExtension(String url, DataType value) { 256 if (isDisallowExtensions()) 257 throw new Error("Extensions are not allowed in this context"); 258 Extension ex = new Extension(); 259 ex.setUrl(url); 260 ex.setValue(value); 261 getModifierExtension().add(ex); 262 } 263 264 265 @Override 266 public Extension getExtensionByUrl(String theUrl) { 267 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 268 ArrayList<Extension> retVal = new ArrayList<Extension>(); 269 Extension res = super.getExtensionByUrl(theUrl); 270 if (res != null) { 271 retVal.add(res); 272 } 273 for (Extension next : getModifierExtension()) { 274 if (theUrl.equals(next.getUrl())) { 275 retVal.add(next); 276 } 277 } 278 if (retVal.size() == 0) 279 return null; 280 else { 281 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url "+theUrl+" must have only one match"); 282 return retVal.get(0); 283 } 284 } 285 286 @Override 287 public void removeExtension(String theUrl) { 288 for (int i = getModifierExtension().size()-1; i >= 0; i--) { 289 if (theUrl.equals(getExtension().get(i).getUrl())) 290 getExtension().remove(i); 291 } 292 super.removeExtension(theUrl); 293 } 294 295 296 /** 297 * Returns an unmodifiable list containing all extensions on this element which 298 * match the given URL. 299 * 300 * @param theUrl The URL. Must not be blank or null. 301 * @return an unmodifiable list containing all extensions on this element which 302 * match the given URL 303 */ 304 @Override 305 public List<Extension> getExtensionsByUrl(String theUrl) { 306 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 307 ArrayList<Extension> retVal = new ArrayList<Extension>(); 308 retVal.addAll(super.getExtensionsByUrl(theUrl)); 309 for (Extension next : getModifierExtension()) { 310 if (theUrl.equals(next.getUrl())) { 311 retVal.add(next); 312 } 313 } 314 return java.util.Collections.unmodifiableList(retVal); 315 } 316 317 public void copyExtensions(org.hl7.fhir.r5.model.BackboneElement src, String... urls) { 318 super.copyExtensions(src,urls); 319 for (Extension e : src.getModifierExtension()) { 320 if (Utilities.existsInList(e.getUrl(), urls)) { 321 addModifierExtension(e.copy()); 322 } 323 } 324 } 325 326 public List<Extension> getExtensionsByUrl(String... theUrls) { 327 328 ArrayList<Extension> retVal = new ArrayList<>(); 329 for (Extension next : getModifierExtension()) { 330 if (Utilities.existsInList(next.getUrl(), theUrls)) { 331 retVal.add(next); 332 } 333 } 334 retVal.addAll(super.getExtensionsByUrl(theUrls)); 335 return java.util.Collections.unmodifiableList(retVal); 336 } 337 338 339 public boolean hasExtension(String... theUrls) { 340 for (Extension next : getModifierExtension()) { 341 if (Utilities.existsInList(next.getUrl(), theUrls)) { 342 return true; 343 } 344 } 345 return super.hasExtension(theUrls); 346 } 347 348 349 public boolean hasExtension(String theUrl) { 350 for (Extension ext : getModifierExtension()) { 351 if (theUrl.equals(ext.getUrl())) { 352 return true; 353 } 354 } 355 356 return super.hasExtension(theUrl); 357 } 358 359 360 public void copyNewExtensions(org.hl7.fhir.r5.model.BackboneElement src, String... urls) { 361 for (Extension e : src.getModifierExtension()) { 362 if (Utilities.existsInList(e.getUrl(), urls) && !!hasExtension(e.getUrl())) { 363 addExtension(e.copy()); 364 } 365 } 366 super.copyNewExtensions(src, urls); 367 } 368 369 370 public Base getExtensionValue(String... theUrls) { 371 for (Extension next : getModifierExtension()) { 372 if (Utilities.existsInList(next.getUrl(), theUrls)) { 373 return next.getValue(); 374 } 375 } 376 return super.getExtensionValue(theUrls); 377 } 378 379// end addition 380 381} 382