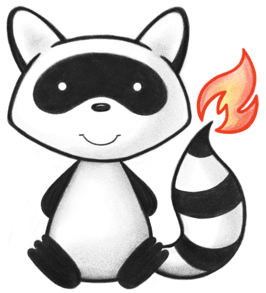
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import ca.uhn.fhir.model.api.IElement; 035import ca.uhn.fhir.model.api.annotation.DatatypeDef; 036import ca.uhn.fhir.parser.DataFormatException; 037import org.apache.commons.codec.binary.Base64; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039import org.hl7.fhir.instance.model.api.IPrimitiveType; 040 041import java.io.Externalizable; 042import java.io.IOException; 043import java.io.ObjectInput; 044import java.io.ObjectOutput; 045 046/** 047 * Primitive type "base64Binary" in FHIR: a sequence of bytes represented in base64 048 */ 049@DatatypeDef(name = "base64Binary") 050public class Base64BinaryType extends PrimitiveType<byte[]> implements IPrimitiveType<byte[]>, IBaseHasExtensions, IElement, Externalizable { 051 052 private static final long serialVersionUID = 3L; 053 private byte[] myValue; 054 055 /** 056 * Constructor 057 */ 058 public Base64BinaryType() { 059 super(); 060 } 061 062 public Base64BinaryType(byte[] theBytes) { 063 super(); 064 setValue(theBytes); 065 } 066 067 public Base64BinaryType(String theValue) { 068 super(); 069 // Null values still result in non-null instance being created 070 setValueAsString(theValue); 071 } 072 073 protected byte[] parse(String theValue) { 074 if (theValue != null) { 075 return Base64.decodeBase64(theValue.getBytes(ca.uhn.fhir.rest.api.Constants.CHARSET_UTF8)); 076 } else { 077 return null; 078 } 079 } 080 081 protected String encode(byte[] theValue) { 082 if (theValue == null) { 083 return null; 084 } 085 return new String(Base64.encodeBase64(theValue), ca.uhn.fhir.rest.api.Constants.CHARSET_UTF8); 086 } 087 088 @Override 089 public Base64BinaryType copy() { 090 return new Base64BinaryType(getValue()); 091 } 092 093 @Override 094 protected DataType typedCopy() { 095 return copy(); 096 } 097 098 public String fhirType() { 099 return "base64Binary"; 100 } 101 102 @Override 103 public void writeExternal(ObjectOutput out) throws IOException { 104 out.writeObject(getValue()); 105 } 106 107 @Override 108 public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException { 109 setValue((byte[]) in.readObject()); 110 } 111 112 @Override 113 public String getValueAsString() { 114 return encode(myValue); 115 } 116 117 @Override 118 public void setValueAsString(String theValue) throws IllegalArgumentException { 119 if (theValue != null) checkValidBase64(theValue); 120 fromStringValue(theValue); 121 setValue(parse(theValue)); 122 } 123 124 @Override 125 public byte[] getValue() { 126 return myValue; 127 } 128 129 @Override 130 public Base64BinaryType setValue(byte[] theValue) throws IllegalArgumentException { 131 myValue = theValue; 132 return (Base64BinaryType) super.setValue(theValue); 133 } 134 135 @Override 136 public boolean hasValue() { 137 return myValue != null && myValue.length > 0; 138 } 139 140 @Override 141 public boolean isEmpty() { 142 // Custom isEmpty() in order to avoid generating the text representation unneccessarily 143 return ca.uhn.fhir.util.ElementUtil.isEmpty(id, extension) && !hasValue(); 144 } 145 146 @Override 147 public String primitiveValue() { 148 return encode(myValue); 149 } 150 151 /** 152 * Checks if the passed in String is a valid {@link Base64} encoded String. Will throw a {@link DataFormatException} if not 153 * formatted correctly. 154 * 155 * @param toCheck {@link String} to check if valid {@link Base64} 156 * @throws DataFormatException 157 */ 158 public void checkValidBase64(String toCheck) throws DataFormatException { 159 if (!org.hl7.fhir.utilities.Base64.isBase64(toCheck.getBytes())) { 160 throw new DataFormatException(""); 161 } 162 } 163}