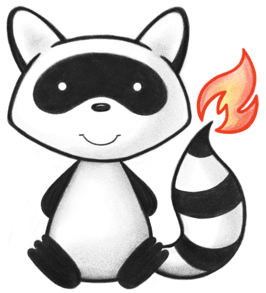
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 051 */ 052@ResourceDef(name="Basic", profile="http://hl7.org/fhir/StructureDefinition/Basic") 053public class Basic extends DomainResource { 054 055 /** 056 * Identifier assigned to the resource for business purposes, outside the context of FHIR. 057 */ 058 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 059 @Description(shortDefinition="Business identifier", formalDefinition="Identifier assigned to the resource for business purposes, outside the context of FHIR." ) 060 protected List<Identifier> identifier; 061 062 /** 063 * Identifies the 'type' of resource - equivalent to the resource name for other resources. 064 */ 065 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=true, summary=true) 066 @Description(shortDefinition="Kind of Resource", formalDefinition="Identifies the 'type' of resource - equivalent to the resource name for other resources." ) 067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/basic-resource-type") 068 protected CodeableConcept code; 069 070 /** 071 * Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource. 072 */ 073 @Child(name = "subject", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Identifies the focus of this resource", formalDefinition="Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource." ) 075 protected Reference subject; 076 077 /** 078 * Identifies when the resource was first created. 079 */ 080 @Child(name = "created", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="When created", formalDefinition="Identifies when the resource was first created." ) 082 protected DateTimeType created; 083 084 /** 085 * Indicates who was responsible for creating the resource instance. 086 */ 087 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Organization.class, Device.class, CareTeam.class}, order=4, min=0, max=1, modifier=false, summary=true) 088 @Description(shortDefinition="Who created", formalDefinition="Indicates who was responsible for creating the resource instance." ) 089 protected Reference author; 090 091 private static final long serialVersionUID = -1635508686L; 092 093 /** 094 * Constructor 095 */ 096 public Basic() { 097 super(); 098 } 099 100 /** 101 * Constructor 102 */ 103 public Basic(CodeableConcept code) { 104 super(); 105 this.setCode(code); 106 } 107 108 /** 109 * @return {@link #identifier} (Identifier assigned to the resource for business purposes, outside the context of FHIR.) 110 */ 111 public List<Identifier> getIdentifier() { 112 if (this.identifier == null) 113 this.identifier = new ArrayList<Identifier>(); 114 return this.identifier; 115 } 116 117 /** 118 * @return Returns a reference to <code>this</code> for easy method chaining 119 */ 120 public Basic setIdentifier(List<Identifier> theIdentifier) { 121 this.identifier = theIdentifier; 122 return this; 123 } 124 125 public boolean hasIdentifier() { 126 if (this.identifier == null) 127 return false; 128 for (Identifier item : this.identifier) 129 if (!item.isEmpty()) 130 return true; 131 return false; 132 } 133 134 public Identifier addIdentifier() { //3 135 Identifier t = new Identifier(); 136 if (this.identifier == null) 137 this.identifier = new ArrayList<Identifier>(); 138 this.identifier.add(t); 139 return t; 140 } 141 142 public Basic addIdentifier(Identifier t) { //3 143 if (t == null) 144 return this; 145 if (this.identifier == null) 146 this.identifier = new ArrayList<Identifier>(); 147 this.identifier.add(t); 148 return this; 149 } 150 151 /** 152 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 153 */ 154 public Identifier getIdentifierFirstRep() { 155 if (getIdentifier().isEmpty()) { 156 addIdentifier(); 157 } 158 return getIdentifier().get(0); 159 } 160 161 /** 162 * @return {@link #code} (Identifies the 'type' of resource - equivalent to the resource name for other resources.) 163 */ 164 public CodeableConcept getCode() { 165 if (this.code == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create Basic.code"); 168 else if (Configuration.doAutoCreate()) 169 this.code = new CodeableConcept(); // cc 170 return this.code; 171 } 172 173 public boolean hasCode() { 174 return this.code != null && !this.code.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #code} (Identifies the 'type' of resource - equivalent to the resource name for other resources.) 179 */ 180 public Basic setCode(CodeableConcept value) { 181 this.code = value; 182 return this; 183 } 184 185 /** 186 * @return {@link #subject} (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 187 */ 188 public Reference getSubject() { 189 if (this.subject == null) 190 if (Configuration.errorOnAutoCreate()) 191 throw new Error("Attempt to auto-create Basic.subject"); 192 else if (Configuration.doAutoCreate()) 193 this.subject = new Reference(); // cc 194 return this.subject; 195 } 196 197 public boolean hasSubject() { 198 return this.subject != null && !this.subject.isEmpty(); 199 } 200 201 /** 202 * @param value {@link #subject} (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 203 */ 204 public Basic setSubject(Reference value) { 205 this.subject = value; 206 return this; 207 } 208 209 /** 210 * @return {@link #created} (Identifies when the resource was first created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 211 */ 212 public DateTimeType getCreatedElement() { 213 if (this.created == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create Basic.created"); 216 else if (Configuration.doAutoCreate()) 217 this.created = new DateTimeType(); // bb 218 return this.created; 219 } 220 221 public boolean hasCreatedElement() { 222 return this.created != null && !this.created.isEmpty(); 223 } 224 225 public boolean hasCreated() { 226 return this.created != null && !this.created.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #created} (Identifies when the resource was first created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 231 */ 232 public Basic setCreatedElement(DateTimeType value) { 233 this.created = value; 234 return this; 235 } 236 237 /** 238 * @return Identifies when the resource was first created. 239 */ 240 public Date getCreated() { 241 return this.created == null ? null : this.created.getValue(); 242 } 243 244 /** 245 * @param value Identifies when the resource was first created. 246 */ 247 public Basic setCreated(Date value) { 248 if (value == null) 249 this.created = null; 250 else { 251 if (this.created == null) 252 this.created = new DateTimeType(); 253 this.created.setValue(value); 254 } 255 return this; 256 } 257 258 /** 259 * @return {@link #author} (Indicates who was responsible for creating the resource instance.) 260 */ 261 public Reference getAuthor() { 262 if (this.author == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create Basic.author"); 265 else if (Configuration.doAutoCreate()) 266 this.author = new Reference(); // cc 267 return this.author; 268 } 269 270 public boolean hasAuthor() { 271 return this.author != null && !this.author.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #author} (Indicates who was responsible for creating the resource instance.) 276 */ 277 public Basic setAuthor(Reference value) { 278 this.author = value; 279 return this; 280 } 281 282 protected void listChildren(List<Property> children) { 283 super.listChildren(children); 284 children.add(new Property("identifier", "Identifier", "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier)); 285 children.add(new Property("code", "CodeableConcept", "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code)); 286 children.add(new Property("subject", "Reference(Any)", "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 1, subject)); 287 children.add(new Property("created", "dateTime", "Identifies when the resource was first created.", 0, 1, created)); 288 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization|Device|CareTeam)", "Indicates who was responsible for creating the resource instance.", 0, 1, author)); 289 } 290 291 @Override 292 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 293 switch (_hash) { 294 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier); 295 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code); 296 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 1, subject); 297 case 1028554472: /*created*/ return new Property("created", "dateTime", "Identifies when the resource was first created.", 0, 1, created); 298 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization|Device|CareTeam)", "Indicates who was responsible for creating the resource instance.", 0, 1, author); 299 default: return super.getNamedProperty(_hash, _name, _checkValid); 300 } 301 302 } 303 304 @Override 305 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 306 switch (hash) { 307 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 308 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 309 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 310 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 311 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 312 default: return super.getProperty(hash, name, checkValid); 313 } 314 315 } 316 317 @Override 318 public Base setProperty(int hash, String name, Base value) throws FHIRException { 319 switch (hash) { 320 case -1618432855: // identifier 321 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 322 return value; 323 case 3059181: // code 324 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 325 return value; 326 case -1867885268: // subject 327 this.subject = TypeConvertor.castToReference(value); // Reference 328 return value; 329 case 1028554472: // created 330 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 331 return value; 332 case -1406328437: // author 333 this.author = TypeConvertor.castToReference(value); // Reference 334 return value; 335 default: return super.setProperty(hash, name, value); 336 } 337 338 } 339 340 @Override 341 public Base setProperty(String name, Base value) throws FHIRException { 342 if (name.equals("identifier")) { 343 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 344 } else if (name.equals("code")) { 345 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 346 } else if (name.equals("subject")) { 347 this.subject = TypeConvertor.castToReference(value); // Reference 348 } else if (name.equals("created")) { 349 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 350 } else if (name.equals("author")) { 351 this.author = TypeConvertor.castToReference(value); // Reference 352 } else 353 return super.setProperty(name, value); 354 return value; 355 } 356 357 @Override 358 public void removeChild(String name, Base value) throws FHIRException { 359 if (name.equals("identifier")) { 360 this.getIdentifier().remove(value); 361 } else if (name.equals("code")) { 362 this.code = null; 363 } else if (name.equals("subject")) { 364 this.subject = null; 365 } else if (name.equals("created")) { 366 this.created = null; 367 } else if (name.equals("author")) { 368 this.author = null; 369 } else 370 super.removeChild(name, value); 371 372 } 373 374 @Override 375 public Base makeProperty(int hash, String name) throws FHIRException { 376 switch (hash) { 377 case -1618432855: return addIdentifier(); 378 case 3059181: return getCode(); 379 case -1867885268: return getSubject(); 380 case 1028554472: return getCreatedElement(); 381 case -1406328437: return getAuthor(); 382 default: return super.makeProperty(hash, name); 383 } 384 385 } 386 387 @Override 388 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 389 switch (hash) { 390 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 391 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 392 case -1867885268: /*subject*/ return new String[] {"Reference"}; 393 case 1028554472: /*created*/ return new String[] {"dateTime"}; 394 case -1406328437: /*author*/ return new String[] {"Reference"}; 395 default: return super.getTypesForProperty(hash, name); 396 } 397 398 } 399 400 @Override 401 public Base addChild(String name) throws FHIRException { 402 if (name.equals("identifier")) { 403 return addIdentifier(); 404 } 405 else if (name.equals("code")) { 406 this.code = new CodeableConcept(); 407 return this.code; 408 } 409 else if (name.equals("subject")) { 410 this.subject = new Reference(); 411 return this.subject; 412 } 413 else if (name.equals("created")) { 414 throw new FHIRException("Cannot call addChild on a singleton property Basic.created"); 415 } 416 else if (name.equals("author")) { 417 this.author = new Reference(); 418 return this.author; 419 } 420 else 421 return super.addChild(name); 422 } 423 424 public String fhirType() { 425 return "Basic"; 426 427 } 428 429 public Basic copy() { 430 Basic dst = new Basic(); 431 copyValues(dst); 432 return dst; 433 } 434 435 public void copyValues(Basic dst) { 436 super.copyValues(dst); 437 if (identifier != null) { 438 dst.identifier = new ArrayList<Identifier>(); 439 for (Identifier i : identifier) 440 dst.identifier.add(i.copy()); 441 }; 442 dst.code = code == null ? null : code.copy(); 443 dst.subject = subject == null ? null : subject.copy(); 444 dst.created = created == null ? null : created.copy(); 445 dst.author = author == null ? null : author.copy(); 446 } 447 448 protected Basic typedCopy() { 449 return copy(); 450 } 451 452 @Override 453 public boolean equalsDeep(Base other_) { 454 if (!super.equalsDeep(other_)) 455 return false; 456 if (!(other_ instanceof Basic)) 457 return false; 458 Basic o = (Basic) other_; 459 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 460 && compareDeep(created, o.created, true) && compareDeep(author, o.author, true); 461 } 462 463 @Override 464 public boolean equalsShallow(Base other_) { 465 if (!super.equalsShallow(other_)) 466 return false; 467 if (!(other_ instanceof Basic)) 468 return false; 469 Basic o = (Basic) other_; 470 return compareValues(created, o.created, true); 471 } 472 473 public boolean isEmpty() { 474 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, subject 475 , created, author); 476 } 477 478 @Override 479 public ResourceType getResourceType() { 480 return ResourceType.Basic; 481 } 482 483 /** 484 * Search parameter: <b>author</b> 485 * <p> 486 * Description: <b>Who created</b><br> 487 * Type: <b>reference</b><br> 488 * Path: <b>Basic.author</b><br> 489 * </p> 490 */ 491 @SearchParamDefinition(name="author", path="Basic.author", description="Who created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 492 public static final String SP_AUTHOR = "author"; 493 /** 494 * <b>Fluent Client</b> search parameter constant for <b>author</b> 495 * <p> 496 * Description: <b>Who created</b><br> 497 * Type: <b>reference</b><br> 498 * Path: <b>Basic.author</b><br> 499 * </p> 500 */ 501 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 502 503/** 504 * Constant for fluent queries to be used to add include statements. Specifies 505 * the path value of "<b>Basic:author</b>". 506 */ 507 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Basic:author").toLocked(); 508 509 /** 510 * Search parameter: <b>created</b> 511 * <p> 512 * Description: <b>When created</b><br> 513 * Type: <b>date</b><br> 514 * Path: <b>Basic.created</b><br> 515 * </p> 516 */ 517 @SearchParamDefinition(name="created", path="Basic.created", description="When created", type="date" ) 518 public static final String SP_CREATED = "created"; 519 /** 520 * <b>Fluent Client</b> search parameter constant for <b>created</b> 521 * <p> 522 * Description: <b>When created</b><br> 523 * Type: <b>date</b><br> 524 * Path: <b>Basic.created</b><br> 525 * </p> 526 */ 527 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 528 529 /** 530 * Search parameter: <b>subject</b> 531 * <p> 532 * Description: <b>Identifies the focus of this resource</b><br> 533 * Type: <b>reference</b><br> 534 * Path: <b>Basic.subject</b><br> 535 * </p> 536 */ 537 @SearchParamDefinition(name="subject", path="Basic.subject", description="Identifies the focus of this resource", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 538 public static final String SP_SUBJECT = "subject"; 539 /** 540 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 541 * <p> 542 * Description: <b>Identifies the focus of this resource</b><br> 543 * Type: <b>reference</b><br> 544 * Path: <b>Basic.subject</b><br> 545 * </p> 546 */ 547 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 548 549/** 550 * Constant for fluent queries to be used to add include statements. Specifies 551 * the path value of "<b>Basic:subject</b>". 552 */ 553 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Basic:subject").toLocked(); 554 555 /** 556 * Search parameter: <b>code</b> 557 * <p> 558 * Description: <b>Multiple Resources: 559 560* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 561* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 562* [AuditEvent](auditevent.html): More specific code for the event 563* [Basic](basic.html): Kind of Resource 564* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 565* [Condition](condition.html): Code for the condition 566* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 567* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 568* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 569* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 570* [ImagingSelection](imagingselection.html): The imaging selection status 571* [List](list.html): What the purpose of this list is 572* [Medication](medication.html): Returns medications for a specific code 573* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 574* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 575* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 576* [MedicationStatement](medicationstatement.html): Return statements of this medication code 577* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 578* [Observation](observation.html): The code of the observation type 579* [Procedure](procedure.html): A code to identify a procedure 580* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 581* [Task](task.html): Search by task code 582</b><br> 583 * Type: <b>token</b><br> 584 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 585 * </p> 586 */ 587 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 588 public static final String SP_CODE = "code"; 589 /** 590 * <b>Fluent Client</b> search parameter constant for <b>code</b> 591 * <p> 592 * Description: <b>Multiple Resources: 593 594* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 595* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 596* [AuditEvent](auditevent.html): More specific code for the event 597* [Basic](basic.html): Kind of Resource 598* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 599* [Condition](condition.html): Code for the condition 600* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 601* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 602* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 603* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 604* [ImagingSelection](imagingselection.html): The imaging selection status 605* [List](list.html): What the purpose of this list is 606* [Medication](medication.html): Returns medications for a specific code 607* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 608* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 609* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 610* [MedicationStatement](medicationstatement.html): Return statements of this medication code 611* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 612* [Observation](observation.html): The code of the observation type 613* [Procedure](procedure.html): A code to identify a procedure 614* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 615* [Task](task.html): Search by task code 616</b><br> 617 * Type: <b>token</b><br> 618 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 619 * </p> 620 */ 621 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 622 623 /** 624 * Search parameter: <b>identifier</b> 625 * <p> 626 * Description: <b>Multiple Resources: 627 628* [Account](account.html): Account number 629* [AdverseEvent](adverseevent.html): Business identifier for the event 630* [AllergyIntolerance](allergyintolerance.html): External ids for this item 631* [Appointment](appointment.html): An Identifier of the Appointment 632* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 633* [Basic](basic.html): Business identifier 634* [BodyStructure](bodystructure.html): Bodystructure identifier 635* [CarePlan](careplan.html): External Ids for this plan 636* [CareTeam](careteam.html): External Ids for this team 637* [ChargeItem](chargeitem.html): Business Identifier for item 638* [Claim](claim.html): The primary identifier of the financial resource 639* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 640* [ClinicalImpression](clinicalimpression.html): Business identifier 641* [Communication](communication.html): Unique identifier 642* [CommunicationRequest](communicationrequest.html): Unique identifier 643* [Composition](composition.html): Version-independent identifier for the Composition 644* [Condition](condition.html): A unique identifier of the condition record 645* [Consent](consent.html): Identifier for this record (external references) 646* [Contract](contract.html): The identity of the contract 647* [Coverage](coverage.html): The primary identifier of the insured and the coverage 648* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 649* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 650* [DetectedIssue](detectedissue.html): Unique id for the detected issue 651* [DeviceRequest](devicerequest.html): Business identifier for request/order 652* [DeviceUsage](deviceusage.html): Search by identifier 653* [DiagnosticReport](diagnosticreport.html): An identifier for the report 654* [DocumentReference](documentreference.html): Identifier of the attachment binary 655* [Encounter](encounter.html): Identifier(s) by which this encounter is known 656* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 657* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 658* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 659* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 660* [Flag](flag.html): Business identifier 661* [Goal](goal.html): External Ids for this goal 662* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 663* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 664* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 665* [Immunization](immunization.html): Business identifier 666* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 667* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 668* [Invoice](invoice.html): Business Identifier for item 669* [List](list.html): Business identifier 670* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 671* [Medication](medication.html): Returns medications with this external identifier 672* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 673* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 674* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 675* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 676* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 677* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 678* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 679* [Observation](observation.html): The unique id for a particular observation 680* [Person](person.html): A person Identifier 681* [Procedure](procedure.html): A unique identifier for a procedure 682* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 683* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 684* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 685* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 686* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 687* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 688* [Specimen](specimen.html): The unique identifier associated with the specimen 689* [SupplyDelivery](supplydelivery.html): External identifier 690* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 691* [Task](task.html): Search for a task instance by its business identifier 692* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 693</b><br> 694 * Type: <b>token</b><br> 695 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 696 * </p> 697 */ 698 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 699 public static final String SP_IDENTIFIER = "identifier"; 700 /** 701 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 702 * <p> 703 * Description: <b>Multiple Resources: 704 705* [Account](account.html): Account number 706* [AdverseEvent](adverseevent.html): Business identifier for the event 707* [AllergyIntolerance](allergyintolerance.html): External ids for this item 708* [Appointment](appointment.html): An Identifier of the Appointment 709* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 710* [Basic](basic.html): Business identifier 711* [BodyStructure](bodystructure.html): Bodystructure identifier 712* [CarePlan](careplan.html): External Ids for this plan 713* [CareTeam](careteam.html): External Ids for this team 714* [ChargeItem](chargeitem.html): Business Identifier for item 715* [Claim](claim.html): The primary identifier of the financial resource 716* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 717* [ClinicalImpression](clinicalimpression.html): Business identifier 718* [Communication](communication.html): Unique identifier 719* [CommunicationRequest](communicationrequest.html): Unique identifier 720* [Composition](composition.html): Version-independent identifier for the Composition 721* [Condition](condition.html): A unique identifier of the condition record 722* [Consent](consent.html): Identifier for this record (external references) 723* [Contract](contract.html): The identity of the contract 724* [Coverage](coverage.html): The primary identifier of the insured and the coverage 725* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 726* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 727* [DetectedIssue](detectedissue.html): Unique id for the detected issue 728* [DeviceRequest](devicerequest.html): Business identifier for request/order 729* [DeviceUsage](deviceusage.html): Search by identifier 730* [DiagnosticReport](diagnosticreport.html): An identifier for the report 731* [DocumentReference](documentreference.html): Identifier of the attachment binary 732* [Encounter](encounter.html): Identifier(s) by which this encounter is known 733* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 734* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 735* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 736* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 737* [Flag](flag.html): Business identifier 738* [Goal](goal.html): External Ids for this goal 739* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 740* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 741* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 742* [Immunization](immunization.html): Business identifier 743* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 744* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 745* [Invoice](invoice.html): Business Identifier for item 746* [List](list.html): Business identifier 747* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 748* [Medication](medication.html): Returns medications with this external identifier 749* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 750* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 751* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 752* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 753* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 754* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 755* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 756* [Observation](observation.html): The unique id for a particular observation 757* [Person](person.html): A person Identifier 758* [Procedure](procedure.html): A unique identifier for a procedure 759* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 760* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 761* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 762* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 763* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 764* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 765* [Specimen](specimen.html): The unique identifier associated with the specimen 766* [SupplyDelivery](supplydelivery.html): External identifier 767* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 768* [Task](task.html): Search for a task instance by its business identifier 769* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 770</b><br> 771 * Type: <b>token</b><br> 772 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 773 * </p> 774 */ 775 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 776 777 /** 778 * Search parameter: <b>patient</b> 779 * <p> 780 * Description: <b>Multiple Resources: 781 782* [Account](account.html): The entity that caused the expenses 783* [AdverseEvent](adverseevent.html): Subject impacted by event 784* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 785* [Appointment](appointment.html): One of the individuals of the appointment is this patient 786* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 787* [AuditEvent](auditevent.html): Where the activity involved patient data 788* [Basic](basic.html): Identifies the focus of this resource 789* [BodyStructure](bodystructure.html): Who this is about 790* [CarePlan](careplan.html): Who the care plan is for 791* [CareTeam](careteam.html): Who care team is for 792* [ChargeItem](chargeitem.html): Individual service was done for/to 793* [Claim](claim.html): Patient receiving the products or services 794* [ClaimResponse](claimresponse.html): The subject of care 795* [ClinicalImpression](clinicalimpression.html): Patient assessed 796* [Communication](communication.html): Focus of message 797* [CommunicationRequest](communicationrequest.html): Focus of message 798* [Composition](composition.html): Who and/or what the composition is about 799* [Condition](condition.html): Who has the condition? 800* [Consent](consent.html): Who the consent applies to 801* [Contract](contract.html): The identity of the subject of the contract (if a patient) 802* [Coverage](coverage.html): Retrieve coverages for a patient 803* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 804* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 805* [DetectedIssue](detectedissue.html): Associated patient 806* [DeviceRequest](devicerequest.html): Individual the service is ordered for 807* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 808* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 809* [DocumentReference](documentreference.html): Who/what is the subject of the document 810* [Encounter](encounter.html): The patient present at the encounter 811* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 812* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 813* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 814* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 815* [Flag](flag.html): The identity of a subject to list flags for 816* [Goal](goal.html): Who this goal is intended for 817* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 818* [ImagingSelection](imagingselection.html): Who the study is about 819* [ImagingStudy](imagingstudy.html): Who the study is about 820* [Immunization](immunization.html): The patient for the vaccination record 821* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 822* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 823* [Invoice](invoice.html): Recipient(s) of goods and services 824* [List](list.html): If all resources have the same subject 825* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 826* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 827* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 828* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 829* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 830* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 831* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 832* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 833* [Observation](observation.html): The subject that the observation is about (if patient) 834* [Person](person.html): The Person links to this Patient 835* [Procedure](procedure.html): Search by subject - a patient 836* [Provenance](provenance.html): Where the activity involved patient data 837* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 838* [RelatedPerson](relatedperson.html): The patient this related person is related to 839* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 840* [ResearchSubject](researchsubject.html): Who or what is part of study 841* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 842* [ServiceRequest](servicerequest.html): Search by subject - a patient 843* [Specimen](specimen.html): The patient the specimen comes from 844* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 845* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 846* [Task](task.html): Search by patient 847* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 848</b><br> 849 * Type: <b>reference</b><br> 850 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 851 * </p> 852 */ 853 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 854 public static final String SP_PATIENT = "patient"; 855 /** 856 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 857 * <p> 858 * Description: <b>Multiple Resources: 859 860* [Account](account.html): The entity that caused the expenses 861* [AdverseEvent](adverseevent.html): Subject impacted by event 862* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 863* [Appointment](appointment.html): One of the individuals of the appointment is this patient 864* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 865* [AuditEvent](auditevent.html): Where the activity involved patient data 866* [Basic](basic.html): Identifies the focus of this resource 867* [BodyStructure](bodystructure.html): Who this is about 868* [CarePlan](careplan.html): Who the care plan is for 869* [CareTeam](careteam.html): Who care team is for 870* [ChargeItem](chargeitem.html): Individual service was done for/to 871* [Claim](claim.html): Patient receiving the products or services 872* [ClaimResponse](claimresponse.html): The subject of care 873* [ClinicalImpression](clinicalimpression.html): Patient assessed 874* [Communication](communication.html): Focus of message 875* [CommunicationRequest](communicationrequest.html): Focus of message 876* [Composition](composition.html): Who and/or what the composition is about 877* [Condition](condition.html): Who has the condition? 878* [Consent](consent.html): Who the consent applies to 879* [Contract](contract.html): The identity of the subject of the contract (if a patient) 880* [Coverage](coverage.html): Retrieve coverages for a patient 881* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 882* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 883* [DetectedIssue](detectedissue.html): Associated patient 884* [DeviceRequest](devicerequest.html): Individual the service is ordered for 885* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 886* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 887* [DocumentReference](documentreference.html): Who/what is the subject of the document 888* [Encounter](encounter.html): The patient present at the encounter 889* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 890* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 891* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 892* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 893* [Flag](flag.html): The identity of a subject to list flags for 894* [Goal](goal.html): Who this goal is intended for 895* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 896* [ImagingSelection](imagingselection.html): Who the study is about 897* [ImagingStudy](imagingstudy.html): Who the study is about 898* [Immunization](immunization.html): The patient for the vaccination record 899* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 900* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 901* [Invoice](invoice.html): Recipient(s) of goods and services 902* [List](list.html): If all resources have the same subject 903* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 904* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 905* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 906* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 907* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 908* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 909* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 910* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 911* [Observation](observation.html): The subject that the observation is about (if patient) 912* [Person](person.html): The Person links to this Patient 913* [Procedure](procedure.html): Search by subject - a patient 914* [Provenance](provenance.html): Where the activity involved patient data 915* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 916* [RelatedPerson](relatedperson.html): The patient this related person is related to 917* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 918* [ResearchSubject](researchsubject.html): Who or what is part of study 919* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 920* [ServiceRequest](servicerequest.html): Search by subject - a patient 921* [Specimen](specimen.html): The patient the specimen comes from 922* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 923* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 924* [Task](task.html): Search by patient 925* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 926</b><br> 927 * Type: <b>reference</b><br> 928 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 929 * </p> 930 */ 931 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 932 933/** 934 * Constant for fluent queries to be used to add include statements. Specifies 935 * the path value of "<b>Basic:patient</b>". 936 */ 937 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Basic:patient").toLocked(); 938 939 940} 941