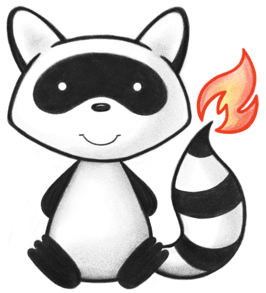
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050import org.hl7.fhir.instance.model.api.IBaseBinary; 051/** 052 * A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc. 053 */ 054@ResourceDef(name="Binary", profile="http://hl7.org/fhir/StructureDefinition/Binary") 055public class Binary extends BaseBinary implements IBaseBinary { 056 057 /** 058 * MimeType of the binary content represented as a standard MimeType (BCP 13). 059 */ 060 @Child(name = "contentType", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="MimeType of the binary content", formalDefinition="MimeType of the binary content represented as a standard MimeType (BCP 13)." ) 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 063 protected CodeType contentType; 064 065 /** 066 * This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient. 067 */ 068 @Child(name = "securityContext", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Identifies another resource to use as proxy when enforcing access control", formalDefinition="This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient." ) 070 protected Reference securityContext; 071 072 /** 073 * The actual content, base64 encoded. 074 */ 075 @Child(name = "data", type = {Base64BinaryType.class}, order=2, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="The actual content", formalDefinition="The actual content, base64 encoded." ) 077 protected Base64BinaryType data; 078 079 private static final long serialVersionUID = 65831526L; 080 081 /** 082 * Constructor 083 */ 084 public Binary() { 085 super(); 086 } 087 088 /** 089 * Constructor 090 */ 091 public Binary(String contentType) { 092 super(); 093 this.setContentType(contentType); 094 } 095 096 /** 097 * @return {@link #contentType} (MimeType of the binary content represented as a standard MimeType (BCP 13).). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 098 */ 099 public CodeType getContentTypeElement() { 100 if (this.contentType == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create Binary.contentType"); 103 else if (Configuration.doAutoCreate()) 104 this.contentType = new CodeType(); // bb 105 return this.contentType; 106 } 107 108 public boolean hasContentTypeElement() { 109 return this.contentType != null && !this.contentType.isEmpty(); 110 } 111 112 public boolean hasContentType() { 113 return this.contentType != null && !this.contentType.isEmpty(); 114 } 115 116 /** 117 * @param value {@link #contentType} (MimeType of the binary content represented as a standard MimeType (BCP 13).). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 118 */ 119 public Binary setContentTypeElement(CodeType value) { 120 this.contentType = value; 121 return this; 122 } 123 124 /** 125 * @return MimeType of the binary content represented as a standard MimeType (BCP 13). 126 */ 127 public String getContentType() { 128 return this.contentType == null ? null : this.contentType.getValue(); 129 } 130 131 /** 132 * @param value MimeType of the binary content represented as a standard MimeType (BCP 13). 133 */ 134 public Binary setContentType(String value) { 135 if (this.contentType == null) 136 this.contentType = new CodeType(); 137 this.contentType.setValue(value); 138 return this; 139 } 140 141 /** 142 * @return {@link #securityContext} (This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.) 143 */ 144 public Reference getSecurityContext() { 145 if (this.securityContext == null) 146 if (Configuration.errorOnAutoCreate()) 147 throw new Error("Attempt to auto-create Binary.securityContext"); 148 else if (Configuration.doAutoCreate()) 149 this.securityContext = new Reference(); // cc 150 return this.securityContext; 151 } 152 153 public boolean hasSecurityContext() { 154 return this.securityContext != null && !this.securityContext.isEmpty(); 155 } 156 157 /** 158 * @param value {@link #securityContext} (This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.) 159 */ 160 public Binary setSecurityContext(Reference value) { 161 this.securityContext = value; 162 return this; 163 } 164 165 /** 166 * @return {@link #data} (The actual content, base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 167 */ 168 public Base64BinaryType getDataElement() { 169 if (this.data == null) 170 if (Configuration.errorOnAutoCreate()) 171 throw new Error("Attempt to auto-create Binary.data"); 172 else if (Configuration.doAutoCreate()) 173 this.data = new Base64BinaryType(); // bb 174 return this.data; 175 } 176 177 public boolean hasDataElement() { 178 return this.data != null && !this.data.isEmpty(); 179 } 180 181 public boolean hasData() { 182 return this.data != null && !this.data.isEmpty(); 183 } 184 185 /** 186 * @param value {@link #data} (The actual content, base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 187 */ 188 public Binary setDataElement(Base64BinaryType value) { 189 this.data = value; 190 return this; 191 } 192 193 /** 194 * @return The actual content, base64 encoded. 195 */ 196 public byte[] getData() { 197 return this.data == null ? null : this.data.getValue(); 198 } 199 200 /** 201 * @param value The actual content, base64 encoded. 202 */ 203 public Binary setData(byte[] value) { 204 if (value == null) 205 this.data = null; 206 else { 207 if (this.data == null) 208 this.data = new Base64BinaryType(); 209 this.data.setValue(value); 210 } 211 return this; 212 } 213 214 protected void listChildren(List<Property> children) { 215 super.listChildren(children); 216 children.add(new Property("contentType", "code", "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, 1, contentType)); 217 children.add(new Property("securityContext", "Reference(Any)", "This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.", 0, 1, securityContext)); 218 children.add(new Property("data", "base64Binary", "The actual content, base64 encoded.", 0, 1, data)); 219 } 220 221 @Override 222 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 223 switch (_hash) { 224 case -389131437: /*contentType*/ return new Property("contentType", "code", "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, 1, contentType); 225 case -1622888881: /*securityContext*/ return new Property("securityContext", "Reference(Any)", "This element identifies another resource that can be used as a proxy of the security sensitivity to use when deciding and enforcing access control rules for the Binary resource. Given that the Binary resource contains very few elements that can be used to determine the sensitivity of the data and relationships to individuals, the referenced resource stands in as a proxy equivalent for this purpose. This referenced resource may be related to the Binary (e.g. DocumentReference), or may be some non-related Resource purely as a security proxy. E.g. to identify that the binary resource relates to a patient, and access should only be granted to applications that have access to the patient.", 0, 1, securityContext); 226 case 3076010: /*data*/ return new Property("data", "base64Binary", "The actual content, base64 encoded.", 0, 1, data); 227 default: return super.getNamedProperty(_hash, _name, _checkValid); 228 } 229 230 } 231 232 @Override 233 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 234 switch (hash) { 235 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 236 case -1622888881: /*securityContext*/ return this.securityContext == null ? new Base[0] : new Base[] {this.securityContext}; // Reference 237 case 3076010: /*data*/ return this.data == null ? new Base[0] : new Base[] {this.data}; // Base64BinaryType 238 default: return super.getProperty(hash, name, checkValid); 239 } 240 241 } 242 243 @Override 244 public Base setProperty(int hash, String name, Base value) throws FHIRException { 245 switch (hash) { 246 case -389131437: // contentType 247 this.contentType = TypeConvertor.castToCode(value); // CodeType 248 return value; 249 case -1622888881: // securityContext 250 this.securityContext = TypeConvertor.castToReference(value); // Reference 251 return value; 252 case 3076010: // data 253 this.data = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 254 return value; 255 default: return super.setProperty(hash, name, value); 256 } 257 258 } 259 260 @Override 261 public Base setProperty(String name, Base value) throws FHIRException { 262 if (name.equals("contentType")) { 263 this.contentType = TypeConvertor.castToCode(value); // CodeType 264 } else if (name.equals("securityContext")) { 265 this.securityContext = TypeConvertor.castToReference(value); // Reference 266 } else if (name.equals("data")) { 267 this.data = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 268 } else 269 return super.setProperty(name, value); 270 return value; 271 } 272 273 @Override 274 public void removeChild(String name, Base value) throws FHIRException { 275 if (name.equals("contentType")) { 276 this.contentType = null; 277 } else if (name.equals("securityContext")) { 278 this.securityContext = null; 279 } else if (name.equals("data")) { 280 this.data = null; 281 } else 282 super.removeChild(name, value); 283 284 } 285 286 @Override 287 public Base makeProperty(int hash, String name) throws FHIRException { 288 switch (hash) { 289 case -389131437: return getContentTypeElement(); 290 case -1622888881: return getSecurityContext(); 291 case 3076010: return getDataElement(); 292 default: return super.makeProperty(hash, name); 293 } 294 295 } 296 297 @Override 298 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 299 switch (hash) { 300 case -389131437: /*contentType*/ return new String[] {"code"}; 301 case -1622888881: /*securityContext*/ return new String[] {"Reference"}; 302 case 3076010: /*data*/ return new String[] {"base64Binary"}; 303 default: return super.getTypesForProperty(hash, name); 304 } 305 306 } 307 308 @Override 309 public Base addChild(String name) throws FHIRException { 310 if (name.equals("contentType")) { 311 throw new FHIRException("Cannot call addChild on a singleton property Binary.contentType"); 312 } 313 else if (name.equals("securityContext")) { 314 this.securityContext = new Reference(); 315 return this.securityContext; 316 } 317 else if (name.equals("data")) { 318 throw new FHIRException("Cannot call addChild on a singleton property Binary.data"); 319 } 320 else 321 return super.addChild(name); 322 } 323 324 public String fhirType() { 325 return "Binary"; 326 327 } 328 329 public Binary copy() { 330 Binary dst = new Binary(); 331 copyValues(dst); 332 return dst; 333 } 334 335 public void copyValues(Binary dst) { 336 super.copyValues(dst); 337 dst.contentType = contentType == null ? null : contentType.copy(); 338 dst.securityContext = securityContext == null ? null : securityContext.copy(); 339 dst.data = data == null ? null : data.copy(); 340 } 341 342 protected Binary typedCopy() { 343 return copy(); 344 } 345 346 @Override 347 public boolean equalsDeep(Base other_) { 348 if (!super.equalsDeep(other_)) 349 return false; 350 if (!(other_ instanceof Binary)) 351 return false; 352 Binary o = (Binary) other_; 353 return compareDeep(contentType, o.contentType, true) && compareDeep(securityContext, o.securityContext, true) 354 && compareDeep(data, o.data, true); 355 } 356 357 @Override 358 public boolean equalsShallow(Base other_) { 359 if (!super.equalsShallow(other_)) 360 return false; 361 if (!(other_ instanceof Binary)) 362 return false; 363 Binary o = (Binary) other_; 364 return compareValues(contentType, o.contentType, true) && compareValues(data, o.data, true); 365 } 366 367 public boolean isEmpty() { 368 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(contentType, securityContext 369 , data); 370 } 371 372 @Override 373 public ResourceType getResourceType() { 374 return ResourceType.Binary; 375 } 376 377// Manual code (from Configuration.txt): 378@Override 379 public byte[] getContent() { 380 return getData(); 381 } 382 383 @Override 384 public IBaseBinary setContent(byte[] arg0) { 385 return setData(arg0); 386 } 387 388 @Override 389 public Base64BinaryType getContentElement() { 390 return getDataElement(); 391 } 392// end addition 393 394} 395