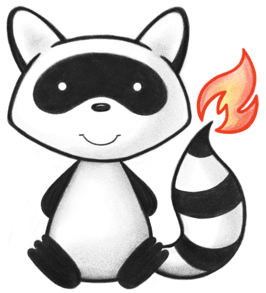
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A biological material originating from a biological entity intended to be transplanted or infused into another (possibly the same) biological entity. 052 */ 053@ResourceDef(name="BiologicallyDerivedProduct", profile="http://hl7.org/fhir/StructureDefinition/BiologicallyDerivedProduct") 054public class BiologicallyDerivedProduct extends DomainResource { 055 056 @Block() 057 public static class BiologicallyDerivedProductCollectionComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Healthcare professional who is performing the collection. 060 */ 061 @Child(name = "collector", type = {Practitioner.class, PractitionerRole.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Individual performing collection", formalDefinition="Healthcare professional who is performing the collection." ) 063 protected Reference collector; 064 065 /** 066 * The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product. 067 */ 068 @Child(name = "source", type = {Patient.class, Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="The patient who underwent the medical procedure to collect the product or the organization that facilitated the collection", formalDefinition="The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product." ) 070 protected Reference source; 071 072 /** 073 * Time of product collection. 074 */ 075 @Child(name = "collected", type = {DateTimeType.class, Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="Time of product collection", formalDefinition="Time of product collection." ) 077 protected DataType collected; 078 079 private static final long serialVersionUID = 626956533L; 080 081 /** 082 * Constructor 083 */ 084 public BiologicallyDerivedProductCollectionComponent() { 085 super(); 086 } 087 088 /** 089 * @return {@link #collector} (Healthcare professional who is performing the collection.) 090 */ 091 public Reference getCollector() { 092 if (this.collector == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create BiologicallyDerivedProductCollectionComponent.collector"); 095 else if (Configuration.doAutoCreate()) 096 this.collector = new Reference(); // cc 097 return this.collector; 098 } 099 100 public boolean hasCollector() { 101 return this.collector != null && !this.collector.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #collector} (Healthcare professional who is performing the collection.) 106 */ 107 public BiologicallyDerivedProductCollectionComponent setCollector(Reference value) { 108 this.collector = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #source} (The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.) 114 */ 115 public Reference getSource() { 116 if (this.source == null) 117 if (Configuration.errorOnAutoCreate()) 118 throw new Error("Attempt to auto-create BiologicallyDerivedProductCollectionComponent.source"); 119 else if (Configuration.doAutoCreate()) 120 this.source = new Reference(); // cc 121 return this.source; 122 } 123 124 public boolean hasSource() { 125 return this.source != null && !this.source.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #source} (The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.) 130 */ 131 public BiologicallyDerivedProductCollectionComponent setSource(Reference value) { 132 this.source = value; 133 return this; 134 } 135 136 /** 137 * @return {@link #collected} (Time of product collection.) 138 */ 139 public DataType getCollected() { 140 return this.collected; 141 } 142 143 /** 144 * @return {@link #collected} (Time of product collection.) 145 */ 146 public DateTimeType getCollectedDateTimeType() throws FHIRException { 147 if (this.collected == null) 148 this.collected = new DateTimeType(); 149 if (!(this.collected instanceof DateTimeType)) 150 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.collected.getClass().getName()+" was encountered"); 151 return (DateTimeType) this.collected; 152 } 153 154 public boolean hasCollectedDateTimeType() { 155 return this.collected instanceof DateTimeType; 156 } 157 158 /** 159 * @return {@link #collected} (Time of product collection.) 160 */ 161 public Period getCollectedPeriod() throws FHIRException { 162 if (this.collected == null) 163 this.collected = new Period(); 164 if (!(this.collected instanceof Period)) 165 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.collected.getClass().getName()+" was encountered"); 166 return (Period) this.collected; 167 } 168 169 public boolean hasCollectedPeriod() { 170 return this.collected instanceof Period; 171 } 172 173 public boolean hasCollected() { 174 return this.collected != null && !this.collected.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #collected} (Time of product collection.) 179 */ 180 public BiologicallyDerivedProductCollectionComponent setCollected(DataType value) { 181 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 182 throw new FHIRException("Not the right type for BiologicallyDerivedProduct.collection.collected[x]: "+value.fhirType()); 183 this.collected = value; 184 return this; 185 } 186 187 protected void listChildren(List<Property> children) { 188 super.listChildren(children); 189 children.add(new Property("collector", "Reference(Practitioner|PractitionerRole)", "Healthcare professional who is performing the collection.", 0, 1, collector)); 190 children.add(new Property("source", "Reference(Patient|Organization)", "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.", 0, 1, source)); 191 children.add(new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, collected)); 192 } 193 194 @Override 195 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 196 switch (_hash) { 197 case 1883491469: /*collector*/ return new Property("collector", "Reference(Practitioner|PractitionerRole)", "Healthcare professional who is performing the collection.", 0, 1, collector); 198 case -896505829: /*source*/ return new Property("source", "Reference(Patient|Organization)", "The patient or entity, such as a hospital or vendor in the case of a processed/manipulated/manufactured product, providing the product.", 0, 1, source); 199 case 1632037015: /*collected[x]*/ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, collected); 200 case 1883491145: /*collected*/ return new Property("collected[x]", "dateTime|Period", "Time of product collection.", 0, 1, collected); 201 case 2005009924: /*collectedDateTime*/ return new Property("collected[x]", "dateTime", "Time of product collection.", 0, 1, collected); 202 case 653185642: /*collectedPeriod*/ return new Property("collected[x]", "Period", "Time of product collection.", 0, 1, collected); 203 default: return super.getNamedProperty(_hash, _name, _checkValid); 204 } 205 206 } 207 208 @Override 209 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 210 switch (hash) { 211 case 1883491469: /*collector*/ return this.collector == null ? new Base[0] : new Base[] {this.collector}; // Reference 212 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 213 case 1883491145: /*collected*/ return this.collected == null ? new Base[0] : new Base[] {this.collected}; // DataType 214 default: return super.getProperty(hash, name, checkValid); 215 } 216 217 } 218 219 @Override 220 public Base setProperty(int hash, String name, Base value) throws FHIRException { 221 switch (hash) { 222 case 1883491469: // collector 223 this.collector = TypeConvertor.castToReference(value); // Reference 224 return value; 225 case -896505829: // source 226 this.source = TypeConvertor.castToReference(value); // Reference 227 return value; 228 case 1883491145: // collected 229 this.collected = TypeConvertor.castToType(value); // DataType 230 return value; 231 default: return super.setProperty(hash, name, value); 232 } 233 234 } 235 236 @Override 237 public Base setProperty(String name, Base value) throws FHIRException { 238 if (name.equals("collector")) { 239 this.collector = TypeConvertor.castToReference(value); // Reference 240 } else if (name.equals("source")) { 241 this.source = TypeConvertor.castToReference(value); // Reference 242 } else if (name.equals("collected[x]")) { 243 this.collected = TypeConvertor.castToType(value); // DataType 244 } else 245 return super.setProperty(name, value); 246 return value; 247 } 248 249 @Override 250 public void removeChild(String name, Base value) throws FHIRException { 251 if (name.equals("collector")) { 252 this.collector = null; 253 } else if (name.equals("source")) { 254 this.source = null; 255 } else if (name.equals("collected[x]")) { 256 this.collected = null; 257 } else 258 super.removeChild(name, value); 259 260 } 261 262 @Override 263 public Base makeProperty(int hash, String name) throws FHIRException { 264 switch (hash) { 265 case 1883491469: return getCollector(); 266 case -896505829: return getSource(); 267 case 1632037015: return getCollected(); 268 case 1883491145: return getCollected(); 269 default: return super.makeProperty(hash, name); 270 } 271 272 } 273 274 @Override 275 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 276 switch (hash) { 277 case 1883491469: /*collector*/ return new String[] {"Reference"}; 278 case -896505829: /*source*/ return new String[] {"Reference"}; 279 case 1883491145: /*collected*/ return new String[] {"dateTime", "Period"}; 280 default: return super.getTypesForProperty(hash, name); 281 } 282 283 } 284 285 @Override 286 public Base addChild(String name) throws FHIRException { 287 if (name.equals("collector")) { 288 this.collector = new Reference(); 289 return this.collector; 290 } 291 else if (name.equals("source")) { 292 this.source = new Reference(); 293 return this.source; 294 } 295 else if (name.equals("collectedDateTime")) { 296 this.collected = new DateTimeType(); 297 return this.collected; 298 } 299 else if (name.equals("collectedPeriod")) { 300 this.collected = new Period(); 301 return this.collected; 302 } 303 else 304 return super.addChild(name); 305 } 306 307 public BiologicallyDerivedProductCollectionComponent copy() { 308 BiologicallyDerivedProductCollectionComponent dst = new BiologicallyDerivedProductCollectionComponent(); 309 copyValues(dst); 310 return dst; 311 } 312 313 public void copyValues(BiologicallyDerivedProductCollectionComponent dst) { 314 super.copyValues(dst); 315 dst.collector = collector == null ? null : collector.copy(); 316 dst.source = source == null ? null : source.copy(); 317 dst.collected = collected == null ? null : collected.copy(); 318 } 319 320 @Override 321 public boolean equalsDeep(Base other_) { 322 if (!super.equalsDeep(other_)) 323 return false; 324 if (!(other_ instanceof BiologicallyDerivedProductCollectionComponent)) 325 return false; 326 BiologicallyDerivedProductCollectionComponent o = (BiologicallyDerivedProductCollectionComponent) other_; 327 return compareDeep(collector, o.collector, true) && compareDeep(source, o.source, true) && compareDeep(collected, o.collected, true) 328 ; 329 } 330 331 @Override 332 public boolean equalsShallow(Base other_) { 333 if (!super.equalsShallow(other_)) 334 return false; 335 if (!(other_ instanceof BiologicallyDerivedProductCollectionComponent)) 336 return false; 337 BiologicallyDerivedProductCollectionComponent o = (BiologicallyDerivedProductCollectionComponent) other_; 338 return true; 339 } 340 341 public boolean isEmpty() { 342 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, source, collected 343 ); 344 } 345 346 public String fhirType() { 347 return "BiologicallyDerivedProduct.collection"; 348 349 } 350 351 } 352 353 @Block() 354 public static class BiologicallyDerivedProductPropertyComponent extends BackboneElement implements IBaseBackboneElement { 355 /** 356 * Code that specifies the property. It should reference an established coding system. 357 */ 358 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 359 @Description(shortDefinition="Code that specifies the property", formalDefinition="Code that specifies the property. It should reference an established coding system." ) 360 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderived-product-property-type-codes") 361 protected CodeableConcept type; 362 363 /** 364 * Property values. 365 */ 366 @Child(name = "value", type = {BooleanType.class, IntegerType.class, CodeableConcept.class, Period.class, Quantity.class, Range.class, Ratio.class, StringType.class, Attachment.class}, order=2, min=1, max=1, modifier=false, summary=false) 367 @Description(shortDefinition="Property values", formalDefinition="Property values." ) 368 protected DataType value; 369 370 private static final long serialVersionUID = -1659186716L; 371 372 /** 373 * Constructor 374 */ 375 public BiologicallyDerivedProductPropertyComponent() { 376 super(); 377 } 378 379 /** 380 * Constructor 381 */ 382 public BiologicallyDerivedProductPropertyComponent(CodeableConcept type, DataType value) { 383 super(); 384 this.setType(type); 385 this.setValue(value); 386 } 387 388 /** 389 * @return {@link #type} (Code that specifies the property. It should reference an established coding system.) 390 */ 391 public CodeableConcept getType() { 392 if (this.type == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create BiologicallyDerivedProductPropertyComponent.type"); 395 else if (Configuration.doAutoCreate()) 396 this.type = new CodeableConcept(); // cc 397 return this.type; 398 } 399 400 public boolean hasType() { 401 return this.type != null && !this.type.isEmpty(); 402 } 403 404 /** 405 * @param value {@link #type} (Code that specifies the property. It should reference an established coding system.) 406 */ 407 public BiologicallyDerivedProductPropertyComponent setType(CodeableConcept value) { 408 this.type = value; 409 return this; 410 } 411 412 /** 413 * @return {@link #value} (Property values.) 414 */ 415 public DataType getValue() { 416 return this.value; 417 } 418 419 /** 420 * @return {@link #value} (Property values.) 421 */ 422 public BooleanType getValueBooleanType() throws FHIRException { 423 if (this.value == null) 424 this.value = new BooleanType(); 425 if (!(this.value instanceof BooleanType)) 426 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 427 return (BooleanType) this.value; 428 } 429 430 public boolean hasValueBooleanType() { 431 return this.value instanceof BooleanType; 432 } 433 434 /** 435 * @return {@link #value} (Property values.) 436 */ 437 public IntegerType getValueIntegerType() throws FHIRException { 438 if (this.value == null) 439 this.value = new IntegerType(); 440 if (!(this.value instanceof IntegerType)) 441 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 442 return (IntegerType) this.value; 443 } 444 445 public boolean hasValueIntegerType() { 446 return this.value instanceof IntegerType; 447 } 448 449 /** 450 * @return {@link #value} (Property values.) 451 */ 452 public CodeableConcept getValueCodeableConcept() throws FHIRException { 453 if (this.value == null) 454 this.value = new CodeableConcept(); 455 if (!(this.value instanceof CodeableConcept)) 456 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 457 return (CodeableConcept) this.value; 458 } 459 460 public boolean hasValueCodeableConcept() { 461 return this.value instanceof CodeableConcept; 462 } 463 464 /** 465 * @return {@link #value} (Property values.) 466 */ 467 public Period getValuePeriod() throws FHIRException { 468 if (this.value == null) 469 this.value = new Period(); 470 if (!(this.value instanceof Period)) 471 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 472 return (Period) this.value; 473 } 474 475 public boolean hasValuePeriod() { 476 return this.value instanceof Period; 477 } 478 479 /** 480 * @return {@link #value} (Property values.) 481 */ 482 public Quantity getValueQuantity() throws FHIRException { 483 if (this.value == null) 484 this.value = new Quantity(); 485 if (!(this.value instanceof Quantity)) 486 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 487 return (Quantity) this.value; 488 } 489 490 public boolean hasValueQuantity() { 491 return this.value instanceof Quantity; 492 } 493 494 /** 495 * @return {@link #value} (Property values.) 496 */ 497 public Range getValueRange() throws FHIRException { 498 if (this.value == null) 499 this.value = new Range(); 500 if (!(this.value instanceof Range)) 501 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 502 return (Range) this.value; 503 } 504 505 public boolean hasValueRange() { 506 return this.value instanceof Range; 507 } 508 509 /** 510 * @return {@link #value} (Property values.) 511 */ 512 public Ratio getValueRatio() throws FHIRException { 513 if (this.value == null) 514 this.value = new Ratio(); 515 if (!(this.value instanceof Ratio)) 516 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 517 return (Ratio) this.value; 518 } 519 520 public boolean hasValueRatio() { 521 return this.value instanceof Ratio; 522 } 523 524 /** 525 * @return {@link #value} (Property values.) 526 */ 527 public StringType getValueStringType() throws FHIRException { 528 if (this.value == null) 529 this.value = new StringType(); 530 if (!(this.value instanceof StringType)) 531 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 532 return (StringType) this.value; 533 } 534 535 public boolean hasValueStringType() { 536 return this.value instanceof StringType; 537 } 538 539 /** 540 * @return {@link #value} (Property values.) 541 */ 542 public Attachment getValueAttachment() throws FHIRException { 543 if (this.value == null) 544 this.value = new Attachment(); 545 if (!(this.value instanceof Attachment)) 546 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 547 return (Attachment) this.value; 548 } 549 550 public boolean hasValueAttachment() { 551 return this.value instanceof Attachment; 552 } 553 554 public boolean hasValue() { 555 return this.value != null && !this.value.isEmpty(); 556 } 557 558 /** 559 * @param value {@link #value} (Property values.) 560 */ 561 public BiologicallyDerivedProductPropertyComponent setValue(DataType value) { 562 if (value != null && !(value instanceof BooleanType || value instanceof IntegerType || value instanceof CodeableConcept || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof StringType || value instanceof Attachment)) 563 throw new FHIRException("Not the right type for BiologicallyDerivedProduct.property.value[x]: "+value.fhirType()); 564 this.value = value; 565 return this; 566 } 567 568 protected void listChildren(List<Property> children) { 569 super.listChildren(children); 570 children.add(new Property("type", "CodeableConcept", "Code that specifies the property. It should reference an established coding system.", 0, 1, type)); 571 children.add(new Property("value[x]", "boolean|integer|CodeableConcept|Period|Quantity|Range|Ratio|string|Attachment", "Property values.", 0, 1, value)); 572 } 573 574 @Override 575 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 576 switch (_hash) { 577 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code that specifies the property. It should reference an established coding system.", 0, 1, type); 578 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|integer|CodeableConcept|Period|Quantity|Range|Ratio|string|Attachment", "Property values.", 0, 1, value); 579 case 111972721: /*value*/ return new Property("value[x]", "boolean|integer|CodeableConcept|Period|Quantity|Range|Ratio|string|Attachment", "Property values.", 0, 1, value); 580 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Property values.", 0, 1, value); 581 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "Property values.", 0, 1, value); 582 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Property values.", 0, 1, value); 583 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "Property values.", 0, 1, value); 584 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Property values.", 0, 1, value); 585 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Property values.", 0, 1, value); 586 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "Property values.", 0, 1, value); 587 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Property values.", 0, 1, value); 588 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Property values.", 0, 1, value); 589 default: return super.getNamedProperty(_hash, _name, _checkValid); 590 } 591 592 } 593 594 @Override 595 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 596 switch (hash) { 597 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 598 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 599 default: return super.getProperty(hash, name, checkValid); 600 } 601 602 } 603 604 @Override 605 public Base setProperty(int hash, String name, Base value) throws FHIRException { 606 switch (hash) { 607 case 3575610: // type 608 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 609 return value; 610 case 111972721: // value 611 this.value = TypeConvertor.castToType(value); // DataType 612 return value; 613 default: return super.setProperty(hash, name, value); 614 } 615 616 } 617 618 @Override 619 public Base setProperty(String name, Base value) throws FHIRException { 620 if (name.equals("type")) { 621 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 622 } else if (name.equals("value[x]")) { 623 this.value = TypeConvertor.castToType(value); // DataType 624 } else 625 return super.setProperty(name, value); 626 return value; 627 } 628 629 @Override 630 public void removeChild(String name, Base value) throws FHIRException { 631 if (name.equals("type")) { 632 this.type = null; 633 } else if (name.equals("value[x]")) { 634 this.value = null; 635 } else 636 super.removeChild(name, value); 637 638 } 639 640 @Override 641 public Base makeProperty(int hash, String name) throws FHIRException { 642 switch (hash) { 643 case 3575610: return getType(); 644 case -1410166417: return getValue(); 645 case 111972721: return getValue(); 646 default: return super.makeProperty(hash, name); 647 } 648 649 } 650 651 @Override 652 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 653 switch (hash) { 654 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 655 case 111972721: /*value*/ return new String[] {"boolean", "integer", "CodeableConcept", "Period", "Quantity", "Range", "Ratio", "string", "Attachment"}; 656 default: return super.getTypesForProperty(hash, name); 657 } 658 659 } 660 661 @Override 662 public Base addChild(String name) throws FHIRException { 663 if (name.equals("type")) { 664 this.type = new CodeableConcept(); 665 return this.type; 666 } 667 else if (name.equals("valueBoolean")) { 668 this.value = new BooleanType(); 669 return this.value; 670 } 671 else if (name.equals("valueInteger")) { 672 this.value = new IntegerType(); 673 return this.value; 674 } 675 else if (name.equals("valueCodeableConcept")) { 676 this.value = new CodeableConcept(); 677 return this.value; 678 } 679 else if (name.equals("valuePeriod")) { 680 this.value = new Period(); 681 return this.value; 682 } 683 else if (name.equals("valueQuantity")) { 684 this.value = new Quantity(); 685 return this.value; 686 } 687 else if (name.equals("valueRange")) { 688 this.value = new Range(); 689 return this.value; 690 } 691 else if (name.equals("valueRatio")) { 692 this.value = new Ratio(); 693 return this.value; 694 } 695 else if (name.equals("valueString")) { 696 this.value = new StringType(); 697 return this.value; 698 } 699 else if (name.equals("valueAttachment")) { 700 this.value = new Attachment(); 701 return this.value; 702 } 703 else 704 return super.addChild(name); 705 } 706 707 public BiologicallyDerivedProductPropertyComponent copy() { 708 BiologicallyDerivedProductPropertyComponent dst = new BiologicallyDerivedProductPropertyComponent(); 709 copyValues(dst); 710 return dst; 711 } 712 713 public void copyValues(BiologicallyDerivedProductPropertyComponent dst) { 714 super.copyValues(dst); 715 dst.type = type == null ? null : type.copy(); 716 dst.value = value == null ? null : value.copy(); 717 } 718 719 @Override 720 public boolean equalsDeep(Base other_) { 721 if (!super.equalsDeep(other_)) 722 return false; 723 if (!(other_ instanceof BiologicallyDerivedProductPropertyComponent)) 724 return false; 725 BiologicallyDerivedProductPropertyComponent o = (BiologicallyDerivedProductPropertyComponent) other_; 726 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 727 } 728 729 @Override 730 public boolean equalsShallow(Base other_) { 731 if (!super.equalsShallow(other_)) 732 return false; 733 if (!(other_ instanceof BiologicallyDerivedProductPropertyComponent)) 734 return false; 735 BiologicallyDerivedProductPropertyComponent o = (BiologicallyDerivedProductPropertyComponent) other_; 736 return true; 737 } 738 739 public boolean isEmpty() { 740 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 741 } 742 743 public String fhirType() { 744 return "BiologicallyDerivedProduct.property"; 745 746 } 747 748 } 749 750 /** 751 * Broad category of this product. 752 */ 753 @Child(name = "productCategory", type = {Coding.class}, order=0, min=0, max=1, modifier=false, summary=false) 754 @Description(shortDefinition="organ | tissue | fluid | cells | biologicalAgent", formalDefinition="Broad category of this product." ) 755 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-category") 756 protected Coding productCategory; 757 758 /** 759 * A codified value that systematically supports characterization and classification of medical products of human origin inclusive of processing conditions such as additives, volumes and handling conditions. 760 */ 761 @Child(name = "productCode", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 762 @Description(shortDefinition="A code that identifies the kind of this biologically derived product", formalDefinition="A codified value that systematically supports characterization and classification of medical products of human origin inclusive of processing conditions such as additives, volumes and handling conditions." ) 763 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderived-productcodes") 764 protected CodeableConcept productCode; 765 766 /** 767 * Parent product (if any) for this biologically-derived product. 768 */ 769 @Child(name = "parent", type = {BiologicallyDerivedProduct.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 770 @Description(shortDefinition="The parent biologically-derived product", formalDefinition="Parent product (if any) for this biologically-derived product." ) 771 protected List<Reference> parent; 772 773 /** 774 * Request to obtain and/or infuse this biologically derived product. 775 */ 776 @Child(name = "request", type = {ServiceRequest.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 777 @Description(shortDefinition="Request to obtain and/or infuse this product", formalDefinition="Request to obtain and/or infuse this biologically derived product." ) 778 protected List<Reference> request; 779 780 /** 781 * Unique instance identifiers assigned to a biologically derived product. Note: This is a business identifier, not a resource identifier. 782 */ 783 @Child(name = "identifier", type = {Identifier.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 784 @Description(shortDefinition="Instance identifier", formalDefinition="Unique instance identifiers assigned to a biologically derived product. Note: This is a business identifier, not a resource identifier." ) 785 protected List<Identifier> identifier; 786 787 /** 788 * An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled. 789 */ 790 @Child(name = "biologicalSourceEvent", type = {Identifier.class}, order=5, min=0, max=1, modifier=false, summary=true) 791 @Description(shortDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled", formalDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled." ) 792 protected Identifier biologicalSourceEvent; 793 794 /** 795 * Processing facilities responsible for the labeling and distribution of this biologically derived product. 796 */ 797 @Child(name = "processingFacility", type = {Organization.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 798 @Description(shortDefinition="Processing facilities responsible for the labeling and distribution of this biologically derived product", formalDefinition="Processing facilities responsible for the labeling and distribution of this biologically derived product." ) 799 protected List<Reference> processingFacility; 800 801 /** 802 * A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers. 803 */ 804 @Child(name = "division", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 805 @Description(shortDefinition="A unique identifier for an aliquot of a product", formalDefinition="A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers." ) 806 protected StringType division; 807 808 /** 809 * Whether the product is currently available. 810 */ 811 @Child(name = "productStatus", type = {Coding.class}, order=8, min=0, max=1, modifier=false, summary=false) 812 @Description(shortDefinition="available | unavailable", formalDefinition="Whether the product is currently available." ) 813 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderived-product-status") 814 protected Coding productStatus; 815 816 /** 817 * Date, and where relevant time, of expiration. 818 */ 819 @Child(name = "expirationDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 820 @Description(shortDefinition="Date, and where relevant time, of expiration", formalDefinition="Date, and where relevant time, of expiration." ) 821 protected DateTimeType expirationDate; 822 823 /** 824 * How this product was collected. 825 */ 826 @Child(name = "collection", type = {}, order=10, min=0, max=1, modifier=false, summary=false) 827 @Description(shortDefinition="How this product was collected", formalDefinition="How this product was collected." ) 828 protected BiologicallyDerivedProductCollectionComponent collection; 829 830 /** 831 * The temperature requirements for storage of the biologically-derived product. 832 */ 833 @Child(name = "storageTempRequirements", type = {Range.class}, order=11, min=0, max=1, modifier=false, summary=false) 834 @Description(shortDefinition="Product storage temperature requirements", formalDefinition="The temperature requirements for storage of the biologically-derived product." ) 835 protected Range storageTempRequirements; 836 837 /** 838 * A property that is specific to this BiologicallyDerviedProduct instance. 839 */ 840 @Child(name = "property", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 841 @Description(shortDefinition="A property that is specific to this BiologicallyDerviedProduct instance", formalDefinition="A property that is specific to this BiologicallyDerviedProduct instance." ) 842 protected List<BiologicallyDerivedProductPropertyComponent> property; 843 844 private static final long serialVersionUID = 452844848L; 845 846 /** 847 * Constructor 848 */ 849 public BiologicallyDerivedProduct() { 850 super(); 851 } 852 853 /** 854 * @return {@link #productCategory} (Broad category of this product.) 855 */ 856 public Coding getProductCategory() { 857 if (this.productCategory == null) 858 if (Configuration.errorOnAutoCreate()) 859 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productCategory"); 860 else if (Configuration.doAutoCreate()) 861 this.productCategory = new Coding(); // cc 862 return this.productCategory; 863 } 864 865 public boolean hasProductCategory() { 866 return this.productCategory != null && !this.productCategory.isEmpty(); 867 } 868 869 /** 870 * @param value {@link #productCategory} (Broad category of this product.) 871 */ 872 public BiologicallyDerivedProduct setProductCategory(Coding value) { 873 this.productCategory = value; 874 return this; 875 } 876 877 /** 878 * @return {@link #productCode} (A codified value that systematically supports characterization and classification of medical products of human origin inclusive of processing conditions such as additives, volumes and handling conditions.) 879 */ 880 public CodeableConcept getProductCode() { 881 if (this.productCode == null) 882 if (Configuration.errorOnAutoCreate()) 883 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productCode"); 884 else if (Configuration.doAutoCreate()) 885 this.productCode = new CodeableConcept(); // cc 886 return this.productCode; 887 } 888 889 public boolean hasProductCode() { 890 return this.productCode != null && !this.productCode.isEmpty(); 891 } 892 893 /** 894 * @param value {@link #productCode} (A codified value that systematically supports characterization and classification of medical products of human origin inclusive of processing conditions such as additives, volumes and handling conditions.) 895 */ 896 public BiologicallyDerivedProduct setProductCode(CodeableConcept value) { 897 this.productCode = value; 898 return this; 899 } 900 901 /** 902 * @return {@link #parent} (Parent product (if any) for this biologically-derived product.) 903 */ 904 public List<Reference> getParent() { 905 if (this.parent == null) 906 this.parent = new ArrayList<Reference>(); 907 return this.parent; 908 } 909 910 /** 911 * @return Returns a reference to <code>this</code> for easy method chaining 912 */ 913 public BiologicallyDerivedProduct setParent(List<Reference> theParent) { 914 this.parent = theParent; 915 return this; 916 } 917 918 public boolean hasParent() { 919 if (this.parent == null) 920 return false; 921 for (Reference item : this.parent) 922 if (!item.isEmpty()) 923 return true; 924 return false; 925 } 926 927 public Reference addParent() { //3 928 Reference t = new Reference(); 929 if (this.parent == null) 930 this.parent = new ArrayList<Reference>(); 931 this.parent.add(t); 932 return t; 933 } 934 935 public BiologicallyDerivedProduct addParent(Reference t) { //3 936 if (t == null) 937 return this; 938 if (this.parent == null) 939 this.parent = new ArrayList<Reference>(); 940 this.parent.add(t); 941 return this; 942 } 943 944 /** 945 * @return The first repetition of repeating field {@link #parent}, creating it if it does not already exist {3} 946 */ 947 public Reference getParentFirstRep() { 948 if (getParent().isEmpty()) { 949 addParent(); 950 } 951 return getParent().get(0); 952 } 953 954 /** 955 * @return {@link #request} (Request to obtain and/or infuse this biologically derived product.) 956 */ 957 public List<Reference> getRequest() { 958 if (this.request == null) 959 this.request = new ArrayList<Reference>(); 960 return this.request; 961 } 962 963 /** 964 * @return Returns a reference to <code>this</code> for easy method chaining 965 */ 966 public BiologicallyDerivedProduct setRequest(List<Reference> theRequest) { 967 this.request = theRequest; 968 return this; 969 } 970 971 public boolean hasRequest() { 972 if (this.request == null) 973 return false; 974 for (Reference item : this.request) 975 if (!item.isEmpty()) 976 return true; 977 return false; 978 } 979 980 public Reference addRequest() { //3 981 Reference t = new Reference(); 982 if (this.request == null) 983 this.request = new ArrayList<Reference>(); 984 this.request.add(t); 985 return t; 986 } 987 988 public BiologicallyDerivedProduct addRequest(Reference t) { //3 989 if (t == null) 990 return this; 991 if (this.request == null) 992 this.request = new ArrayList<Reference>(); 993 this.request.add(t); 994 return this; 995 } 996 997 /** 998 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 999 */ 1000 public Reference getRequestFirstRep() { 1001 if (getRequest().isEmpty()) { 1002 addRequest(); 1003 } 1004 return getRequest().get(0); 1005 } 1006 1007 /** 1008 * @return {@link #identifier} (Unique instance identifiers assigned to a biologically derived product. Note: This is a business identifier, not a resource identifier.) 1009 */ 1010 public List<Identifier> getIdentifier() { 1011 if (this.identifier == null) 1012 this.identifier = new ArrayList<Identifier>(); 1013 return this.identifier; 1014 } 1015 1016 /** 1017 * @return Returns a reference to <code>this</code> for easy method chaining 1018 */ 1019 public BiologicallyDerivedProduct setIdentifier(List<Identifier> theIdentifier) { 1020 this.identifier = theIdentifier; 1021 return this; 1022 } 1023 1024 public boolean hasIdentifier() { 1025 if (this.identifier == null) 1026 return false; 1027 for (Identifier item : this.identifier) 1028 if (!item.isEmpty()) 1029 return true; 1030 return false; 1031 } 1032 1033 public Identifier addIdentifier() { //3 1034 Identifier t = new Identifier(); 1035 if (this.identifier == null) 1036 this.identifier = new ArrayList<Identifier>(); 1037 this.identifier.add(t); 1038 return t; 1039 } 1040 1041 public BiologicallyDerivedProduct addIdentifier(Identifier t) { //3 1042 if (t == null) 1043 return this; 1044 if (this.identifier == null) 1045 this.identifier = new ArrayList<Identifier>(); 1046 this.identifier.add(t); 1047 return this; 1048 } 1049 1050 /** 1051 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1052 */ 1053 public Identifier getIdentifierFirstRep() { 1054 if (getIdentifier().isEmpty()) { 1055 addIdentifier(); 1056 } 1057 return getIdentifier().get(0); 1058 } 1059 1060 /** 1061 * @return {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 1062 */ 1063 public Identifier getBiologicalSourceEvent() { 1064 if (this.biologicalSourceEvent == null) 1065 if (Configuration.errorOnAutoCreate()) 1066 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.biologicalSourceEvent"); 1067 else if (Configuration.doAutoCreate()) 1068 this.biologicalSourceEvent = new Identifier(); // cc 1069 return this.biologicalSourceEvent; 1070 } 1071 1072 public boolean hasBiologicalSourceEvent() { 1073 return this.biologicalSourceEvent != null && !this.biologicalSourceEvent.isEmpty(); 1074 } 1075 1076 /** 1077 * @param value {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 1078 */ 1079 public BiologicallyDerivedProduct setBiologicalSourceEvent(Identifier value) { 1080 this.biologicalSourceEvent = value; 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #processingFacility} (Processing facilities responsible for the labeling and distribution of this biologically derived product.) 1086 */ 1087 public List<Reference> getProcessingFacility() { 1088 if (this.processingFacility == null) 1089 this.processingFacility = new ArrayList<Reference>(); 1090 return this.processingFacility; 1091 } 1092 1093 /** 1094 * @return Returns a reference to <code>this</code> for easy method chaining 1095 */ 1096 public BiologicallyDerivedProduct setProcessingFacility(List<Reference> theProcessingFacility) { 1097 this.processingFacility = theProcessingFacility; 1098 return this; 1099 } 1100 1101 public boolean hasProcessingFacility() { 1102 if (this.processingFacility == null) 1103 return false; 1104 for (Reference item : this.processingFacility) 1105 if (!item.isEmpty()) 1106 return true; 1107 return false; 1108 } 1109 1110 public Reference addProcessingFacility() { //3 1111 Reference t = new Reference(); 1112 if (this.processingFacility == null) 1113 this.processingFacility = new ArrayList<Reference>(); 1114 this.processingFacility.add(t); 1115 return t; 1116 } 1117 1118 public BiologicallyDerivedProduct addProcessingFacility(Reference t) { //3 1119 if (t == null) 1120 return this; 1121 if (this.processingFacility == null) 1122 this.processingFacility = new ArrayList<Reference>(); 1123 this.processingFacility.add(t); 1124 return this; 1125 } 1126 1127 /** 1128 * @return The first repetition of repeating field {@link #processingFacility}, creating it if it does not already exist {3} 1129 */ 1130 public Reference getProcessingFacilityFirstRep() { 1131 if (getProcessingFacility().isEmpty()) { 1132 addProcessingFacility(); 1133 } 1134 return getProcessingFacility().get(0); 1135 } 1136 1137 /** 1138 * @return {@link #division} (A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers.). This is the underlying object with id, value and extensions. The accessor "getDivision" gives direct access to the value 1139 */ 1140 public StringType getDivisionElement() { 1141 if (this.division == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.division"); 1144 else if (Configuration.doAutoCreate()) 1145 this.division = new StringType(); // bb 1146 return this.division; 1147 } 1148 1149 public boolean hasDivisionElement() { 1150 return this.division != null && !this.division.isEmpty(); 1151 } 1152 1153 public boolean hasDivision() { 1154 return this.division != null && !this.division.isEmpty(); 1155 } 1156 1157 /** 1158 * @param value {@link #division} (A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers.). This is the underlying object with id, value and extensions. The accessor "getDivision" gives direct access to the value 1159 */ 1160 public BiologicallyDerivedProduct setDivisionElement(StringType value) { 1161 this.division = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers. 1167 */ 1168 public String getDivision() { 1169 return this.division == null ? null : this.division.getValue(); 1170 } 1171 1172 /** 1173 * @param value A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers. 1174 */ 1175 public BiologicallyDerivedProduct setDivision(String value) { 1176 if (Utilities.noString(value)) 1177 this.division = null; 1178 else { 1179 if (this.division == null) 1180 this.division = new StringType(); 1181 this.division.setValue(value); 1182 } 1183 return this; 1184 } 1185 1186 /** 1187 * @return {@link #productStatus} (Whether the product is currently available.) 1188 */ 1189 public Coding getProductStatus() { 1190 if (this.productStatus == null) 1191 if (Configuration.errorOnAutoCreate()) 1192 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.productStatus"); 1193 else if (Configuration.doAutoCreate()) 1194 this.productStatus = new Coding(); // cc 1195 return this.productStatus; 1196 } 1197 1198 public boolean hasProductStatus() { 1199 return this.productStatus != null && !this.productStatus.isEmpty(); 1200 } 1201 1202 /** 1203 * @param value {@link #productStatus} (Whether the product is currently available.) 1204 */ 1205 public BiologicallyDerivedProduct setProductStatus(Coding value) { 1206 this.productStatus = value; 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #expirationDate} (Date, and where relevant time, of expiration.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1212 */ 1213 public DateTimeType getExpirationDateElement() { 1214 if (this.expirationDate == null) 1215 if (Configuration.errorOnAutoCreate()) 1216 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.expirationDate"); 1217 else if (Configuration.doAutoCreate()) 1218 this.expirationDate = new DateTimeType(); // bb 1219 return this.expirationDate; 1220 } 1221 1222 public boolean hasExpirationDateElement() { 1223 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1224 } 1225 1226 public boolean hasExpirationDate() { 1227 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1228 } 1229 1230 /** 1231 * @param value {@link #expirationDate} (Date, and where relevant time, of expiration.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1232 */ 1233 public BiologicallyDerivedProduct setExpirationDateElement(DateTimeType value) { 1234 this.expirationDate = value; 1235 return this; 1236 } 1237 1238 /** 1239 * @return Date, and where relevant time, of expiration. 1240 */ 1241 public Date getExpirationDate() { 1242 return this.expirationDate == null ? null : this.expirationDate.getValue(); 1243 } 1244 1245 /** 1246 * @param value Date, and where relevant time, of expiration. 1247 */ 1248 public BiologicallyDerivedProduct setExpirationDate(Date value) { 1249 if (value == null) 1250 this.expirationDate = null; 1251 else { 1252 if (this.expirationDate == null) 1253 this.expirationDate = new DateTimeType(); 1254 this.expirationDate.setValue(value); 1255 } 1256 return this; 1257 } 1258 1259 /** 1260 * @return {@link #collection} (How this product was collected.) 1261 */ 1262 public BiologicallyDerivedProductCollectionComponent getCollection() { 1263 if (this.collection == null) 1264 if (Configuration.errorOnAutoCreate()) 1265 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.collection"); 1266 else if (Configuration.doAutoCreate()) 1267 this.collection = new BiologicallyDerivedProductCollectionComponent(); // cc 1268 return this.collection; 1269 } 1270 1271 public boolean hasCollection() { 1272 return this.collection != null && !this.collection.isEmpty(); 1273 } 1274 1275 /** 1276 * @param value {@link #collection} (How this product was collected.) 1277 */ 1278 public BiologicallyDerivedProduct setCollection(BiologicallyDerivedProductCollectionComponent value) { 1279 this.collection = value; 1280 return this; 1281 } 1282 1283 /** 1284 * @return {@link #storageTempRequirements} (The temperature requirements for storage of the biologically-derived product.) 1285 */ 1286 public Range getStorageTempRequirements() { 1287 if (this.storageTempRequirements == null) 1288 if (Configuration.errorOnAutoCreate()) 1289 throw new Error("Attempt to auto-create BiologicallyDerivedProduct.storageTempRequirements"); 1290 else if (Configuration.doAutoCreate()) 1291 this.storageTempRequirements = new Range(); // cc 1292 return this.storageTempRequirements; 1293 } 1294 1295 public boolean hasStorageTempRequirements() { 1296 return this.storageTempRequirements != null && !this.storageTempRequirements.isEmpty(); 1297 } 1298 1299 /** 1300 * @param value {@link #storageTempRequirements} (The temperature requirements for storage of the biologically-derived product.) 1301 */ 1302 public BiologicallyDerivedProduct setStorageTempRequirements(Range value) { 1303 this.storageTempRequirements = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return {@link #property} (A property that is specific to this BiologicallyDerviedProduct instance.) 1309 */ 1310 public List<BiologicallyDerivedProductPropertyComponent> getProperty() { 1311 if (this.property == null) 1312 this.property = new ArrayList<BiologicallyDerivedProductPropertyComponent>(); 1313 return this.property; 1314 } 1315 1316 /** 1317 * @return Returns a reference to <code>this</code> for easy method chaining 1318 */ 1319 public BiologicallyDerivedProduct setProperty(List<BiologicallyDerivedProductPropertyComponent> theProperty) { 1320 this.property = theProperty; 1321 return this; 1322 } 1323 1324 public boolean hasProperty() { 1325 if (this.property == null) 1326 return false; 1327 for (BiologicallyDerivedProductPropertyComponent item : this.property) 1328 if (!item.isEmpty()) 1329 return true; 1330 return false; 1331 } 1332 1333 public BiologicallyDerivedProductPropertyComponent addProperty() { //3 1334 BiologicallyDerivedProductPropertyComponent t = new BiologicallyDerivedProductPropertyComponent(); 1335 if (this.property == null) 1336 this.property = new ArrayList<BiologicallyDerivedProductPropertyComponent>(); 1337 this.property.add(t); 1338 return t; 1339 } 1340 1341 public BiologicallyDerivedProduct addProperty(BiologicallyDerivedProductPropertyComponent t) { //3 1342 if (t == null) 1343 return this; 1344 if (this.property == null) 1345 this.property = new ArrayList<BiologicallyDerivedProductPropertyComponent>(); 1346 this.property.add(t); 1347 return this; 1348 } 1349 1350 /** 1351 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 1352 */ 1353 public BiologicallyDerivedProductPropertyComponent getPropertyFirstRep() { 1354 if (getProperty().isEmpty()) { 1355 addProperty(); 1356 } 1357 return getProperty().get(0); 1358 } 1359 1360 protected void listChildren(List<Property> children) { 1361 super.listChildren(children); 1362 children.add(new Property("productCategory", "Coding", "Broad category of this product.", 0, 1, productCategory)); 1363 children.add(new Property("productCode", "CodeableConcept", "A codified value that systematically supports characterization and classification of medical products of human origin inclusive of processing conditions such as additives, volumes and handling conditions.", 0, 1, productCode)); 1364 children.add(new Property("parent", "Reference(BiologicallyDerivedProduct)", "Parent product (if any) for this biologically-derived product.", 0, java.lang.Integer.MAX_VALUE, parent)); 1365 children.add(new Property("request", "Reference(ServiceRequest)", "Request to obtain and/or infuse this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, request)); 1366 children.add(new Property("identifier", "Identifier", "Unique instance identifiers assigned to a biologically derived product. Note: This is a business identifier, not a resource identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1367 children.add(new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent)); 1368 children.add(new Property("processingFacility", "Reference(Organization)", "Processing facilities responsible for the labeling and distribution of this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, processingFacility)); 1369 children.add(new Property("division", "string", "A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers.", 0, 1, division)); 1370 children.add(new Property("productStatus", "Coding", "Whether the product is currently available.", 0, 1, productStatus)); 1371 children.add(new Property("expirationDate", "dateTime", "Date, and where relevant time, of expiration.", 0, 1, expirationDate)); 1372 children.add(new Property("collection", "", "How this product was collected.", 0, 1, collection)); 1373 children.add(new Property("storageTempRequirements", "Range", "The temperature requirements for storage of the biologically-derived product.", 0, 1, storageTempRequirements)); 1374 children.add(new Property("property", "", "A property that is specific to this BiologicallyDerviedProduct instance.", 0, java.lang.Integer.MAX_VALUE, property)); 1375 } 1376 1377 @Override 1378 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1379 switch (_hash) { 1380 case 197299981: /*productCategory*/ return new Property("productCategory", "Coding", "Broad category of this product.", 0, 1, productCategory); 1381 case -1492131972: /*productCode*/ return new Property("productCode", "CodeableConcept", "A codified value that systematically supports characterization and classification of medical products of human origin inclusive of processing conditions such as additives, volumes and handling conditions.", 0, 1, productCode); 1382 case -995424086: /*parent*/ return new Property("parent", "Reference(BiologicallyDerivedProduct)", "Parent product (if any) for this biologically-derived product.", 0, java.lang.Integer.MAX_VALUE, parent); 1383 case 1095692943: /*request*/ return new Property("request", "Reference(ServiceRequest)", "Request to obtain and/or infuse this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, request); 1384 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique instance identifiers assigned to a biologically derived product. Note: This is a business identifier, not a resource identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 1385 case -654468482: /*biologicalSourceEvent*/ return new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent); 1386 case 39337686: /*processingFacility*/ return new Property("processingFacility", "Reference(Organization)", "Processing facilities responsible for the labeling and distribution of this biologically derived product.", 0, java.lang.Integer.MAX_VALUE, processingFacility); 1387 case 364720301: /*division*/ return new Property("division", "string", "A unique identifier for an aliquot of a product. Used to distinguish individual aliquots of a product carrying the same biologicalSource and productCode identifiers.", 0, 1, division); 1388 case 1042864577: /*productStatus*/ return new Property("productStatus", "Coding", "Whether the product is currently available.", 0, 1, productStatus); 1389 case -668811523: /*expirationDate*/ return new Property("expirationDate", "dateTime", "Date, and where relevant time, of expiration.", 0, 1, expirationDate); 1390 case -1741312354: /*collection*/ return new Property("collection", "", "How this product was collected.", 0, 1, collection); 1391 case 1643599647: /*storageTempRequirements*/ return new Property("storageTempRequirements", "Range", "The temperature requirements for storage of the biologically-derived product.", 0, 1, storageTempRequirements); 1392 case -993141291: /*property*/ return new Property("property", "", "A property that is specific to this BiologicallyDerviedProduct instance.", 0, java.lang.Integer.MAX_VALUE, property); 1393 default: return super.getNamedProperty(_hash, _name, _checkValid); 1394 } 1395 1396 } 1397 1398 @Override 1399 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1400 switch (hash) { 1401 case 197299981: /*productCategory*/ return this.productCategory == null ? new Base[0] : new Base[] {this.productCategory}; // Coding 1402 case -1492131972: /*productCode*/ return this.productCode == null ? new Base[0] : new Base[] {this.productCode}; // CodeableConcept 1403 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 1404 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 1405 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1406 case -654468482: /*biologicalSourceEvent*/ return this.biologicalSourceEvent == null ? new Base[0] : new Base[] {this.biologicalSourceEvent}; // Identifier 1407 case 39337686: /*processingFacility*/ return this.processingFacility == null ? new Base[0] : this.processingFacility.toArray(new Base[this.processingFacility.size()]); // Reference 1408 case 364720301: /*division*/ return this.division == null ? new Base[0] : new Base[] {this.division}; // StringType 1409 case 1042864577: /*productStatus*/ return this.productStatus == null ? new Base[0] : new Base[] {this.productStatus}; // Coding 1410 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateTimeType 1411 case -1741312354: /*collection*/ return this.collection == null ? new Base[0] : new Base[] {this.collection}; // BiologicallyDerivedProductCollectionComponent 1412 case 1643599647: /*storageTempRequirements*/ return this.storageTempRequirements == null ? new Base[0] : new Base[] {this.storageTempRequirements}; // Range 1413 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // BiologicallyDerivedProductPropertyComponent 1414 default: return super.getProperty(hash, name, checkValid); 1415 } 1416 1417 } 1418 1419 @Override 1420 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1421 switch (hash) { 1422 case 197299981: // productCategory 1423 this.productCategory = TypeConvertor.castToCoding(value); // Coding 1424 return value; 1425 case -1492131972: // productCode 1426 this.productCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1427 return value; 1428 case -995424086: // parent 1429 this.getParent().add(TypeConvertor.castToReference(value)); // Reference 1430 return value; 1431 case 1095692943: // request 1432 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 1433 return value; 1434 case -1618432855: // identifier 1435 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1436 return value; 1437 case -654468482: // biologicalSourceEvent 1438 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 1439 return value; 1440 case 39337686: // processingFacility 1441 this.getProcessingFacility().add(TypeConvertor.castToReference(value)); // Reference 1442 return value; 1443 case 364720301: // division 1444 this.division = TypeConvertor.castToString(value); // StringType 1445 return value; 1446 case 1042864577: // productStatus 1447 this.productStatus = TypeConvertor.castToCoding(value); // Coding 1448 return value; 1449 case -668811523: // expirationDate 1450 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 1451 return value; 1452 case -1741312354: // collection 1453 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 1454 return value; 1455 case 1643599647: // storageTempRequirements 1456 this.storageTempRequirements = TypeConvertor.castToRange(value); // Range 1457 return value; 1458 case -993141291: // property 1459 this.getProperty().add((BiologicallyDerivedProductPropertyComponent) value); // BiologicallyDerivedProductPropertyComponent 1460 return value; 1461 default: return super.setProperty(hash, name, value); 1462 } 1463 1464 } 1465 1466 @Override 1467 public Base setProperty(String name, Base value) throws FHIRException { 1468 if (name.equals("productCategory")) { 1469 this.productCategory = TypeConvertor.castToCoding(value); // Coding 1470 } else if (name.equals("productCode")) { 1471 this.productCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1472 } else if (name.equals("parent")) { 1473 this.getParent().add(TypeConvertor.castToReference(value)); 1474 } else if (name.equals("request")) { 1475 this.getRequest().add(TypeConvertor.castToReference(value)); 1476 } else if (name.equals("identifier")) { 1477 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1478 } else if (name.equals("biologicalSourceEvent")) { 1479 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 1480 } else if (name.equals("processingFacility")) { 1481 this.getProcessingFacility().add(TypeConvertor.castToReference(value)); 1482 } else if (name.equals("division")) { 1483 this.division = TypeConvertor.castToString(value); // StringType 1484 } else if (name.equals("productStatus")) { 1485 this.productStatus = TypeConvertor.castToCoding(value); // Coding 1486 } else if (name.equals("expirationDate")) { 1487 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 1488 } else if (name.equals("collection")) { 1489 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 1490 } else if (name.equals("storageTempRequirements")) { 1491 this.storageTempRequirements = TypeConvertor.castToRange(value); // Range 1492 } else if (name.equals("property")) { 1493 this.getProperty().add((BiologicallyDerivedProductPropertyComponent) value); 1494 } else 1495 return super.setProperty(name, value); 1496 return value; 1497 } 1498 1499 @Override 1500 public void removeChild(String name, Base value) throws FHIRException { 1501 if (name.equals("productCategory")) { 1502 this.productCategory = null; 1503 } else if (name.equals("productCode")) { 1504 this.productCode = null; 1505 } else if (name.equals("parent")) { 1506 this.getParent().remove(value); 1507 } else if (name.equals("request")) { 1508 this.getRequest().remove(value); 1509 } else if (name.equals("identifier")) { 1510 this.getIdentifier().remove(value); 1511 } else if (name.equals("biologicalSourceEvent")) { 1512 this.biologicalSourceEvent = null; 1513 } else if (name.equals("processingFacility")) { 1514 this.getProcessingFacility().remove(value); 1515 } else if (name.equals("division")) { 1516 this.division = null; 1517 } else if (name.equals("productStatus")) { 1518 this.productStatus = null; 1519 } else if (name.equals("expirationDate")) { 1520 this.expirationDate = null; 1521 } else if (name.equals("collection")) { 1522 this.collection = (BiologicallyDerivedProductCollectionComponent) value; // BiologicallyDerivedProductCollectionComponent 1523 } else if (name.equals("storageTempRequirements")) { 1524 this.storageTempRequirements = null; 1525 } else if (name.equals("property")) { 1526 this.getProperty().remove((BiologicallyDerivedProductPropertyComponent) value); 1527 } else 1528 super.removeChild(name, value); 1529 1530 } 1531 1532 @Override 1533 public Base makeProperty(int hash, String name) throws FHIRException { 1534 switch (hash) { 1535 case 197299981: return getProductCategory(); 1536 case -1492131972: return getProductCode(); 1537 case -995424086: return addParent(); 1538 case 1095692943: return addRequest(); 1539 case -1618432855: return addIdentifier(); 1540 case -654468482: return getBiologicalSourceEvent(); 1541 case 39337686: return addProcessingFacility(); 1542 case 364720301: return getDivisionElement(); 1543 case 1042864577: return getProductStatus(); 1544 case -668811523: return getExpirationDateElement(); 1545 case -1741312354: return getCollection(); 1546 case 1643599647: return getStorageTempRequirements(); 1547 case -993141291: return addProperty(); 1548 default: return super.makeProperty(hash, name); 1549 } 1550 1551 } 1552 1553 @Override 1554 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1555 switch (hash) { 1556 case 197299981: /*productCategory*/ return new String[] {"Coding"}; 1557 case -1492131972: /*productCode*/ return new String[] {"CodeableConcept"}; 1558 case -995424086: /*parent*/ return new String[] {"Reference"}; 1559 case 1095692943: /*request*/ return new String[] {"Reference"}; 1560 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1561 case -654468482: /*biologicalSourceEvent*/ return new String[] {"Identifier"}; 1562 case 39337686: /*processingFacility*/ return new String[] {"Reference"}; 1563 case 364720301: /*division*/ return new String[] {"string"}; 1564 case 1042864577: /*productStatus*/ return new String[] {"Coding"}; 1565 case -668811523: /*expirationDate*/ return new String[] {"dateTime"}; 1566 case -1741312354: /*collection*/ return new String[] {}; 1567 case 1643599647: /*storageTempRequirements*/ return new String[] {"Range"}; 1568 case -993141291: /*property*/ return new String[] {}; 1569 default: return super.getTypesForProperty(hash, name); 1570 } 1571 1572 } 1573 1574 @Override 1575 public Base addChild(String name) throws FHIRException { 1576 if (name.equals("productCategory")) { 1577 this.productCategory = new Coding(); 1578 return this.productCategory; 1579 } 1580 else if (name.equals("productCode")) { 1581 this.productCode = new CodeableConcept(); 1582 return this.productCode; 1583 } 1584 else if (name.equals("parent")) { 1585 return addParent(); 1586 } 1587 else if (name.equals("request")) { 1588 return addRequest(); 1589 } 1590 else if (name.equals("identifier")) { 1591 return addIdentifier(); 1592 } 1593 else if (name.equals("biologicalSourceEvent")) { 1594 this.biologicalSourceEvent = new Identifier(); 1595 return this.biologicalSourceEvent; 1596 } 1597 else if (name.equals("processingFacility")) { 1598 return addProcessingFacility(); 1599 } 1600 else if (name.equals("division")) { 1601 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.division"); 1602 } 1603 else if (name.equals("productStatus")) { 1604 this.productStatus = new Coding(); 1605 return this.productStatus; 1606 } 1607 else if (name.equals("expirationDate")) { 1608 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProduct.expirationDate"); 1609 } 1610 else if (name.equals("collection")) { 1611 this.collection = new BiologicallyDerivedProductCollectionComponent(); 1612 return this.collection; 1613 } 1614 else if (name.equals("storageTempRequirements")) { 1615 this.storageTempRequirements = new Range(); 1616 return this.storageTempRequirements; 1617 } 1618 else if (name.equals("property")) { 1619 return addProperty(); 1620 } 1621 else 1622 return super.addChild(name); 1623 } 1624 1625 public String fhirType() { 1626 return "BiologicallyDerivedProduct"; 1627 1628 } 1629 1630 public BiologicallyDerivedProduct copy() { 1631 BiologicallyDerivedProduct dst = new BiologicallyDerivedProduct(); 1632 copyValues(dst); 1633 return dst; 1634 } 1635 1636 public void copyValues(BiologicallyDerivedProduct dst) { 1637 super.copyValues(dst); 1638 dst.productCategory = productCategory == null ? null : productCategory.copy(); 1639 dst.productCode = productCode == null ? null : productCode.copy(); 1640 if (parent != null) { 1641 dst.parent = new ArrayList<Reference>(); 1642 for (Reference i : parent) 1643 dst.parent.add(i.copy()); 1644 }; 1645 if (request != null) { 1646 dst.request = new ArrayList<Reference>(); 1647 for (Reference i : request) 1648 dst.request.add(i.copy()); 1649 }; 1650 if (identifier != null) { 1651 dst.identifier = new ArrayList<Identifier>(); 1652 for (Identifier i : identifier) 1653 dst.identifier.add(i.copy()); 1654 }; 1655 dst.biologicalSourceEvent = biologicalSourceEvent == null ? null : biologicalSourceEvent.copy(); 1656 if (processingFacility != null) { 1657 dst.processingFacility = new ArrayList<Reference>(); 1658 for (Reference i : processingFacility) 1659 dst.processingFacility.add(i.copy()); 1660 }; 1661 dst.division = division == null ? null : division.copy(); 1662 dst.productStatus = productStatus == null ? null : productStatus.copy(); 1663 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 1664 dst.collection = collection == null ? null : collection.copy(); 1665 dst.storageTempRequirements = storageTempRequirements == null ? null : storageTempRequirements.copy(); 1666 if (property != null) { 1667 dst.property = new ArrayList<BiologicallyDerivedProductPropertyComponent>(); 1668 for (BiologicallyDerivedProductPropertyComponent i : property) 1669 dst.property.add(i.copy()); 1670 }; 1671 } 1672 1673 protected BiologicallyDerivedProduct typedCopy() { 1674 return copy(); 1675 } 1676 1677 @Override 1678 public boolean equalsDeep(Base other_) { 1679 if (!super.equalsDeep(other_)) 1680 return false; 1681 if (!(other_ instanceof BiologicallyDerivedProduct)) 1682 return false; 1683 BiologicallyDerivedProduct o = (BiologicallyDerivedProduct) other_; 1684 return compareDeep(productCategory, o.productCategory, true) && compareDeep(productCode, o.productCode, true) 1685 && compareDeep(parent, o.parent, true) && compareDeep(request, o.request, true) && compareDeep(identifier, o.identifier, true) 1686 && compareDeep(biologicalSourceEvent, o.biologicalSourceEvent, true) && compareDeep(processingFacility, o.processingFacility, true) 1687 && compareDeep(division, o.division, true) && compareDeep(productStatus, o.productStatus, true) 1688 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(collection, o.collection, true) 1689 && compareDeep(storageTempRequirements, o.storageTempRequirements, true) && compareDeep(property, o.property, true) 1690 ; 1691 } 1692 1693 @Override 1694 public boolean equalsShallow(Base other_) { 1695 if (!super.equalsShallow(other_)) 1696 return false; 1697 if (!(other_ instanceof BiologicallyDerivedProduct)) 1698 return false; 1699 BiologicallyDerivedProduct o = (BiologicallyDerivedProduct) other_; 1700 return compareValues(division, o.division, true) && compareValues(expirationDate, o.expirationDate, true) 1701 ; 1702 } 1703 1704 public boolean isEmpty() { 1705 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productCategory, productCode 1706 , parent, request, identifier, biologicalSourceEvent, processingFacility, division 1707 , productStatus, expirationDate, collection, storageTempRequirements, property); 1708 } 1709 1710 @Override 1711 public ResourceType getResourceType() { 1712 return ResourceType.BiologicallyDerivedProduct; 1713 } 1714 1715 /** 1716 * Search parameter: <b>biological-source-event</b> 1717 * <p> 1718 * Description: <b>The biological source for the biologically derived product</b><br> 1719 * Type: <b>token</b><br> 1720 * Path: <b>BiologicallyDerivedProduct.biologicalSourceEvent</b><br> 1721 * </p> 1722 */ 1723 @SearchParamDefinition(name="biological-source-event", path="BiologicallyDerivedProduct.biologicalSourceEvent", description="The biological source for the biologically derived product", type="token" ) 1724 public static final String SP_BIOLOGICAL_SOURCE_EVENT = "biological-source-event"; 1725 /** 1726 * <b>Fluent Client</b> search parameter constant for <b>biological-source-event</b> 1727 * <p> 1728 * Description: <b>The biological source for the biologically derived product</b><br> 1729 * Type: <b>token</b><br> 1730 * Path: <b>BiologicallyDerivedProduct.biologicalSourceEvent</b><br> 1731 * </p> 1732 */ 1733 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BIOLOGICAL_SOURCE_EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BIOLOGICAL_SOURCE_EVENT); 1734 1735 /** 1736 * Search parameter: <b>code</b> 1737 * <p> 1738 * Description: <b>A code that identifies the kind of this biologically derived product (SNOMED CT code).</b><br> 1739 * Type: <b>token</b><br> 1740 * Path: <b>BiologicallyDerivedProduct.productCode</b><br> 1741 * </p> 1742 */ 1743 @SearchParamDefinition(name="code", path="BiologicallyDerivedProduct.productCode", description="A code that identifies the kind of this biologically derived product (SNOMED CT code).", type="token" ) 1744 public static final String SP_CODE = "code"; 1745 /** 1746 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1747 * <p> 1748 * Description: <b>A code that identifies the kind of this biologically derived product (SNOMED CT code).</b><br> 1749 * Type: <b>token</b><br> 1750 * Path: <b>BiologicallyDerivedProduct.productCode</b><br> 1751 * </p> 1752 */ 1753 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1754 1755 /** 1756 * Search parameter: <b>collector</b> 1757 * <p> 1758 * Description: <b>Procedure request to obtain this biologically derived product.</b><br> 1759 * Type: <b>reference</b><br> 1760 * Path: <b>BiologicallyDerivedProduct.collection.collector</b><br> 1761 * </p> 1762 */ 1763 @SearchParamDefinition(name="collector", path="BiologicallyDerivedProduct.collection.collector", description="Procedure request to obtain this biologically derived product.", type="reference", target={Practitioner.class, PractitionerRole.class } ) 1764 public static final String SP_COLLECTOR = "collector"; 1765 /** 1766 * <b>Fluent Client</b> search parameter constant for <b>collector</b> 1767 * <p> 1768 * Description: <b>Procedure request to obtain this biologically derived product.</b><br> 1769 * Type: <b>reference</b><br> 1770 * Path: <b>BiologicallyDerivedProduct.collection.collector</b><br> 1771 * </p> 1772 */ 1773 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COLLECTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COLLECTOR); 1774 1775/** 1776 * Constant for fluent queries to be used to add include statements. Specifies 1777 * the path value of "<b>BiologicallyDerivedProduct:collector</b>". 1778 */ 1779 public static final ca.uhn.fhir.model.api.Include INCLUDE_COLLECTOR = new ca.uhn.fhir.model.api.Include("BiologicallyDerivedProduct:collector").toLocked(); 1780 1781 /** 1782 * Search parameter: <b>identifier</b> 1783 * <p> 1784 * Description: <b>Identifier</b><br> 1785 * Type: <b>token</b><br> 1786 * Path: <b>BiologicallyDerivedProduct.identifier</b><br> 1787 * </p> 1788 */ 1789 @SearchParamDefinition(name="identifier", path="BiologicallyDerivedProduct.identifier", description="Identifier", type="token" ) 1790 public static final String SP_IDENTIFIER = "identifier"; 1791 /** 1792 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1793 * <p> 1794 * Description: <b>Identifier</b><br> 1795 * Type: <b>token</b><br> 1796 * Path: <b>BiologicallyDerivedProduct.identifier</b><br> 1797 * </p> 1798 */ 1799 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1800 1801 /** 1802 * Search parameter: <b>product-category</b> 1803 * <p> 1804 * Description: <b>Broad category of this product.</b><br> 1805 * Type: <b>token</b><br> 1806 * Path: <b>BiologicallyDerivedProduct.productCategory</b><br> 1807 * </p> 1808 */ 1809 @SearchParamDefinition(name="product-category", path="BiologicallyDerivedProduct.productCategory", description="Broad category of this product.", type="token" ) 1810 public static final String SP_PRODUCT_CATEGORY = "product-category"; 1811 /** 1812 * <b>Fluent Client</b> search parameter constant for <b>product-category</b> 1813 * <p> 1814 * Description: <b>Broad category of this product.</b><br> 1815 * Type: <b>token</b><br> 1816 * Path: <b>BiologicallyDerivedProduct.productCategory</b><br> 1817 * </p> 1818 */ 1819 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRODUCT_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRODUCT_CATEGORY); 1820 1821 /** 1822 * Search parameter: <b>product-status</b> 1823 * <p> 1824 * Description: <b>Whether the product is currently available.</b><br> 1825 * Type: <b>token</b><br> 1826 * Path: <b>BiologicallyDerivedProduct.productStatus</b><br> 1827 * </p> 1828 */ 1829 @SearchParamDefinition(name="product-status", path="BiologicallyDerivedProduct.productStatus", description="Whether the product is currently available.", type="token" ) 1830 public static final String SP_PRODUCT_STATUS = "product-status"; 1831 /** 1832 * <b>Fluent Client</b> search parameter constant for <b>product-status</b> 1833 * <p> 1834 * Description: <b>Whether the product is currently available.</b><br> 1835 * Type: <b>token</b><br> 1836 * Path: <b>BiologicallyDerivedProduct.productStatus</b><br> 1837 * </p> 1838 */ 1839 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRODUCT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRODUCT_STATUS); 1840 1841 /** 1842 * Search parameter: <b>request</b> 1843 * <p> 1844 * Description: <b>Procedure request to obtain this biologically derived product.</b><br> 1845 * Type: <b>reference</b><br> 1846 * Path: <b>BiologicallyDerivedProduct.request</b><br> 1847 * </p> 1848 */ 1849 @SearchParamDefinition(name="request", path="BiologicallyDerivedProduct.request", description="Procedure request to obtain this biologically derived product.", type="reference", target={ServiceRequest.class } ) 1850 public static final String SP_REQUEST = "request"; 1851 /** 1852 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1853 * <p> 1854 * Description: <b>Procedure request to obtain this biologically derived product.</b><br> 1855 * Type: <b>reference</b><br> 1856 * Path: <b>BiologicallyDerivedProduct.request</b><br> 1857 * </p> 1858 */ 1859 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 1860 1861/** 1862 * Constant for fluent queries to be used to add include statements. Specifies 1863 * the path value of "<b>BiologicallyDerivedProduct:request</b>". 1864 */ 1865 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("BiologicallyDerivedProduct:request").toLocked(); 1866 1867 /** 1868 * Search parameter: <b>serial-number</b> 1869 * <p> 1870 * Description: <b>Identifier</b><br> 1871 * Type: <b>token</b><br> 1872 * Path: <b>BiologicallyDerivedProduct.identifier</b><br> 1873 * </p> 1874 */ 1875 @SearchParamDefinition(name="serial-number", path="BiologicallyDerivedProduct.identifier", description="Identifier", type="token" ) 1876 public static final String SP_SERIAL_NUMBER = "serial-number"; 1877 /** 1878 * <b>Fluent Client</b> search parameter constant for <b>serial-number</b> 1879 * <p> 1880 * Description: <b>Identifier</b><br> 1881 * Type: <b>token</b><br> 1882 * Path: <b>BiologicallyDerivedProduct.identifier</b><br> 1883 * </p> 1884 */ 1885 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIAL_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIAL_NUMBER); 1886 1887 1888} 1889