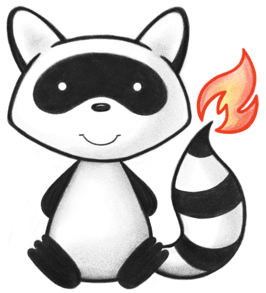
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of dispensation of a biologically derived product. 052 */ 053@ResourceDef(name="BiologicallyDerivedProductDispense", profile="http://hl7.org/fhir/StructureDefinition/BiologicallyDerivedProductDispense") 054public class BiologicallyDerivedProductDispense extends DomainResource { 055 056 public enum BiologicallyDerivedProductDispenseCodes { 057 /** 058 * The dispense process has started but not yet completed. 059 */ 060 PREPARATION, 061 /** 062 * The dispense process is in progress. 063 */ 064 INPROGRESS, 065 /** 066 * The requested product has been allocated and is ready for transport. 067 */ 068 ALLOCATED, 069 /** 070 * The dispensed product has been picked up. 071 */ 072 ISSUED, 073 /** 074 * The dispense could not be completed. 075 */ 076 UNFULFILLED, 077 /** 078 * The dispensed product was returned. 079 */ 080 RETURNED, 081 /** 082 * The dispense was entered in error and therefore nullified. 083 */ 084 ENTEREDINERROR, 085 /** 086 * The authoring system does not know which of the status values applies for this dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just not known which one. 087 */ 088 UNKNOWN, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static BiologicallyDerivedProductDispenseCodes fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("preparation".equals(codeString)) 097 return PREPARATION; 098 if ("in-progress".equals(codeString)) 099 return INPROGRESS; 100 if ("allocated".equals(codeString)) 101 return ALLOCATED; 102 if ("issued".equals(codeString)) 103 return ISSUED; 104 if ("unfulfilled".equals(codeString)) 105 return UNFULFILLED; 106 if ("returned".equals(codeString)) 107 return RETURNED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if ("unknown".equals(codeString)) 111 return UNKNOWN; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown BiologicallyDerivedProductDispenseCodes code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case PREPARATION: return "preparation"; 120 case INPROGRESS: return "in-progress"; 121 case ALLOCATED: return "allocated"; 122 case ISSUED: return "issued"; 123 case UNFULFILLED: return "unfulfilled"; 124 case RETURNED: return "returned"; 125 case ENTEREDINERROR: return "entered-in-error"; 126 case UNKNOWN: return "unknown"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getSystem() { 132 switch (this) { 133 case PREPARATION: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 134 case INPROGRESS: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 135 case ALLOCATED: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 136 case ISSUED: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 137 case UNFULFILLED: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 138 case RETURNED: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 139 case ENTEREDINERROR: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 140 case UNKNOWN: return "http://hl7.org/fhir/biologicallyderivedproductdispense-status"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getDefinition() { 146 switch (this) { 147 case PREPARATION: return "The dispense process has started but not yet completed."; 148 case INPROGRESS: return "The dispense process is in progress."; 149 case ALLOCATED: return "The requested product has been allocated and is ready for transport."; 150 case ISSUED: return "The dispensed product has been picked up."; 151 case UNFULFILLED: return "The dispense could not be completed."; 152 case RETURNED: return "The dispensed product was returned."; 153 case ENTEREDINERROR: return "The dispense was entered in error and therefore nullified."; 154 case UNKNOWN: return "The authoring system does not know which of the status values applies for this dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just not known which one."; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 public String getDisplay() { 160 switch (this) { 161 case PREPARATION: return "Preparation"; 162 case INPROGRESS: return "In Progress"; 163 case ALLOCATED: return "Allocated"; 164 case ISSUED: return "Issued"; 165 case UNFULFILLED: return "Unfulfilled"; 166 case RETURNED: return "Returned"; 167 case ENTEREDINERROR: return "Entered in Error"; 168 case UNKNOWN: return "Unknown"; 169 case NULL: return null; 170 default: return "?"; 171 } 172 } 173 } 174 175 public static class BiologicallyDerivedProductDispenseCodesEnumFactory implements EnumFactory<BiologicallyDerivedProductDispenseCodes> { 176 public BiologicallyDerivedProductDispenseCodes fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("preparation".equals(codeString)) 181 return BiologicallyDerivedProductDispenseCodes.PREPARATION; 182 if ("in-progress".equals(codeString)) 183 return BiologicallyDerivedProductDispenseCodes.INPROGRESS; 184 if ("allocated".equals(codeString)) 185 return BiologicallyDerivedProductDispenseCodes.ALLOCATED; 186 if ("issued".equals(codeString)) 187 return BiologicallyDerivedProductDispenseCodes.ISSUED; 188 if ("unfulfilled".equals(codeString)) 189 return BiologicallyDerivedProductDispenseCodes.UNFULFILLED; 190 if ("returned".equals(codeString)) 191 return BiologicallyDerivedProductDispenseCodes.RETURNED; 192 if ("entered-in-error".equals(codeString)) 193 return BiologicallyDerivedProductDispenseCodes.ENTEREDINERROR; 194 if ("unknown".equals(codeString)) 195 return BiologicallyDerivedProductDispenseCodes.UNKNOWN; 196 throw new IllegalArgumentException("Unknown BiologicallyDerivedProductDispenseCodes code '"+codeString+"'"); 197 } 198 public Enumeration<BiologicallyDerivedProductDispenseCodes> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.NULL, code); 203 String codeString = ((PrimitiveType) code).asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.NULL, code); 206 if ("preparation".equals(codeString)) 207 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.PREPARATION, code); 208 if ("in-progress".equals(codeString)) 209 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.INPROGRESS, code); 210 if ("allocated".equals(codeString)) 211 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.ALLOCATED, code); 212 if ("issued".equals(codeString)) 213 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.ISSUED, code); 214 if ("unfulfilled".equals(codeString)) 215 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.UNFULFILLED, code); 216 if ("returned".equals(codeString)) 217 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.RETURNED, code); 218 if ("entered-in-error".equals(codeString)) 219 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.ENTEREDINERROR, code); 220 if ("unknown".equals(codeString)) 221 return new Enumeration<BiologicallyDerivedProductDispenseCodes>(this, BiologicallyDerivedProductDispenseCodes.UNKNOWN, code); 222 throw new FHIRException("Unknown BiologicallyDerivedProductDispenseCodes code '"+codeString+"'"); 223 } 224 public String toCode(BiologicallyDerivedProductDispenseCodes code) { 225 if (code == BiologicallyDerivedProductDispenseCodes.NULL) 226 return null; 227 if (code == BiologicallyDerivedProductDispenseCodes.PREPARATION) 228 return "preparation"; 229 if (code == BiologicallyDerivedProductDispenseCodes.INPROGRESS) 230 return "in-progress"; 231 if (code == BiologicallyDerivedProductDispenseCodes.ALLOCATED) 232 return "allocated"; 233 if (code == BiologicallyDerivedProductDispenseCodes.ISSUED) 234 return "issued"; 235 if (code == BiologicallyDerivedProductDispenseCodes.UNFULFILLED) 236 return "unfulfilled"; 237 if (code == BiologicallyDerivedProductDispenseCodes.RETURNED) 238 return "returned"; 239 if (code == BiologicallyDerivedProductDispenseCodes.ENTEREDINERROR) 240 return "entered-in-error"; 241 if (code == BiologicallyDerivedProductDispenseCodes.UNKNOWN) 242 return "unknown"; 243 return "?"; 244 } 245 public String toSystem(BiologicallyDerivedProductDispenseCodes code) { 246 return code.getSystem(); 247 } 248 } 249 250 @Block() 251 public static class BiologicallyDerivedProductDispensePerformerComponent extends BackboneElement implements IBaseBackboneElement { 252 /** 253 * Identifies the function of the performer during the dispense. 254 */ 255 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 256 @Description(shortDefinition="Identifies the function of the performer during the dispense", formalDefinition="Identifies the function of the performer during the dispense." ) 257 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderivedproductdispense-performer-function") 258 protected CodeableConcept function; 259 260 /** 261 * Identifies the person responsible for the action. 262 */ 263 @Child(name = "actor", type = {Practitioner.class}, order=2, min=1, max=1, modifier=false, summary=true) 264 @Description(shortDefinition="Who performed the action", formalDefinition="Identifies the person responsible for the action." ) 265 protected Reference actor; 266 267 private static final long serialVersionUID = -576943815L; 268 269 /** 270 * Constructor 271 */ 272 public BiologicallyDerivedProductDispensePerformerComponent() { 273 super(); 274 } 275 276 /** 277 * Constructor 278 */ 279 public BiologicallyDerivedProductDispensePerformerComponent(Reference actor) { 280 super(); 281 this.setActor(actor); 282 } 283 284 /** 285 * @return {@link #function} (Identifies the function of the performer during the dispense.) 286 */ 287 public CodeableConcept getFunction() { 288 if (this.function == null) 289 if (Configuration.errorOnAutoCreate()) 290 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispensePerformerComponent.function"); 291 else if (Configuration.doAutoCreate()) 292 this.function = new CodeableConcept(); // cc 293 return this.function; 294 } 295 296 public boolean hasFunction() { 297 return this.function != null && !this.function.isEmpty(); 298 } 299 300 /** 301 * @param value {@link #function} (Identifies the function of the performer during the dispense.) 302 */ 303 public BiologicallyDerivedProductDispensePerformerComponent setFunction(CodeableConcept value) { 304 this.function = value; 305 return this; 306 } 307 308 /** 309 * @return {@link #actor} (Identifies the person responsible for the action.) 310 */ 311 public Reference getActor() { 312 if (this.actor == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispensePerformerComponent.actor"); 315 else if (Configuration.doAutoCreate()) 316 this.actor = new Reference(); // cc 317 return this.actor; 318 } 319 320 public boolean hasActor() { 321 return this.actor != null && !this.actor.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #actor} (Identifies the person responsible for the action.) 326 */ 327 public BiologicallyDerivedProductDispensePerformerComponent setActor(Reference value) { 328 this.actor = value; 329 return this; 330 } 331 332 protected void listChildren(List<Property> children) { 333 super.listChildren(children); 334 children.add(new Property("function", "CodeableConcept", "Identifies the function of the performer during the dispense.", 0, 1, function)); 335 children.add(new Property("actor", "Reference(Practitioner)", "Identifies the person responsible for the action.", 0, 1, actor)); 336 } 337 338 @Override 339 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 340 switch (_hash) { 341 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Identifies the function of the performer during the dispense.", 0, 1, function); 342 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner)", "Identifies the person responsible for the action.", 0, 1, actor); 343 default: return super.getNamedProperty(_hash, _name, _checkValid); 344 } 345 346 } 347 348 @Override 349 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 350 switch (hash) { 351 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 352 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 353 default: return super.getProperty(hash, name, checkValid); 354 } 355 356 } 357 358 @Override 359 public Base setProperty(int hash, String name, Base value) throws FHIRException { 360 switch (hash) { 361 case 1380938712: // function 362 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 363 return value; 364 case 92645877: // actor 365 this.actor = TypeConvertor.castToReference(value); // Reference 366 return value; 367 default: return super.setProperty(hash, name, value); 368 } 369 370 } 371 372 @Override 373 public Base setProperty(String name, Base value) throws FHIRException { 374 if (name.equals("function")) { 375 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 376 } else if (name.equals("actor")) { 377 this.actor = TypeConvertor.castToReference(value); // Reference 378 } else 379 return super.setProperty(name, value); 380 return value; 381 } 382 383 @Override 384 public void removeChild(String name, Base value) throws FHIRException { 385 if (name.equals("function")) { 386 this.function = null; 387 } else if (name.equals("actor")) { 388 this.actor = null; 389 } else 390 super.removeChild(name, value); 391 392 } 393 394 @Override 395 public Base makeProperty(int hash, String name) throws FHIRException { 396 switch (hash) { 397 case 1380938712: return getFunction(); 398 case 92645877: return getActor(); 399 default: return super.makeProperty(hash, name); 400 } 401 402 } 403 404 @Override 405 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 406 switch (hash) { 407 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 408 case 92645877: /*actor*/ return new String[] {"Reference"}; 409 default: return super.getTypesForProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public Base addChild(String name) throws FHIRException { 416 if (name.equals("function")) { 417 this.function = new CodeableConcept(); 418 return this.function; 419 } 420 else if (name.equals("actor")) { 421 this.actor = new Reference(); 422 return this.actor; 423 } 424 else 425 return super.addChild(name); 426 } 427 428 public BiologicallyDerivedProductDispensePerformerComponent copy() { 429 BiologicallyDerivedProductDispensePerformerComponent dst = new BiologicallyDerivedProductDispensePerformerComponent(); 430 copyValues(dst); 431 return dst; 432 } 433 434 public void copyValues(BiologicallyDerivedProductDispensePerformerComponent dst) { 435 super.copyValues(dst); 436 dst.function = function == null ? null : function.copy(); 437 dst.actor = actor == null ? null : actor.copy(); 438 } 439 440 @Override 441 public boolean equalsDeep(Base other_) { 442 if (!super.equalsDeep(other_)) 443 return false; 444 if (!(other_ instanceof BiologicallyDerivedProductDispensePerformerComponent)) 445 return false; 446 BiologicallyDerivedProductDispensePerformerComponent o = (BiologicallyDerivedProductDispensePerformerComponent) other_; 447 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 448 } 449 450 @Override 451 public boolean equalsShallow(Base other_) { 452 if (!super.equalsShallow(other_)) 453 return false; 454 if (!(other_ instanceof BiologicallyDerivedProductDispensePerformerComponent)) 455 return false; 456 BiologicallyDerivedProductDispensePerformerComponent o = (BiologicallyDerivedProductDispensePerformerComponent) other_; 457 return true; 458 } 459 460 public boolean isEmpty() { 461 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 462 } 463 464 public String fhirType() { 465 return "BiologicallyDerivedProductDispense.performer"; 466 467 } 468 469 } 470 471 /** 472 * Unique instance identifiers assigned to a biologically derived product dispense. Note: This is a business identifier, not a resource identifier. 473 */ 474 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 475 @Description(shortDefinition="Business identifier for this dispense", formalDefinition="Unique instance identifiers assigned to a biologically derived product dispense. Note: This is a business identifier, not a resource identifier." ) 476 protected List<Identifier> identifier; 477 478 /** 479 * The order or request that the dispense is fulfilling. This is a reference to a ServiceRequest resource. 480 */ 481 @Child(name = "basedOn", type = {ServiceRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 482 @Description(shortDefinition="The order or request that this dispense is fulfilling", formalDefinition="The order or request that the dispense is fulfilling. This is a reference to a ServiceRequest resource." ) 483 protected List<Reference> basedOn; 484 485 /** 486 * A larger event of which this particular event is a component. 487 */ 488 @Child(name = "partOf", type = {BiologicallyDerivedProductDispense.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 489 @Description(shortDefinition="Short description", formalDefinition="A larger event of which this particular event is a component." ) 490 protected List<Reference> partOf; 491 492 /** 493 * A code specifying the state of the dispense event. 494 */ 495 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 496 @Description(shortDefinition="preparation | in-progress | allocated | issued | unfulfilled | returned | entered-in-error | unknown", formalDefinition="A code specifying the state of the dispense event." ) 497 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderivedproductdispense-status") 498 protected Enumeration<BiologicallyDerivedProductDispenseCodes> status; 499 500 /** 501 * Indicates the relationship between the donor of the biologically derived product and the intended recipient. 502 */ 503 @Child(name = "originRelationshipType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 504 @Description(shortDefinition="Relationship between the donor and intended recipient", formalDefinition="Indicates the relationship between the donor of the biologically derived product and the intended recipient." ) 505 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderivedproductdispense-origin-relationship") 506 protected CodeableConcept originRelationshipType; 507 508 /** 509 * A link to a resource identifying the biologically derived product that is being dispensed. 510 */ 511 @Child(name = "product", type = {BiologicallyDerivedProduct.class}, order=5, min=1, max=1, modifier=false, summary=true) 512 @Description(shortDefinition="The BiologicallyDerivedProduct that is dispensed", formalDefinition="A link to a resource identifying the biologically derived product that is being dispensed." ) 513 protected Reference product; 514 515 /** 516 * A link to a resource representing the patient that the product is dispensed for. 517 */ 518 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 519 @Description(shortDefinition="The intended recipient of the dispensed product", formalDefinition="A link to a resource representing the patient that the product is dispensed for." ) 520 protected Reference patient; 521 522 /** 523 * Indicates the type of matching associated with the dispense. 524 */ 525 @Child(name = "matchStatus", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 526 @Description(shortDefinition="Indicates the type of matching associated with the dispense", formalDefinition="Indicates the type of matching associated with the dispense." ) 527 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/biologicallyderivedproductdispense-match-status") 528 protected CodeableConcept matchStatus; 529 530 /** 531 * Indicates who or what performed an action. 532 */ 533 @Child(name = "performer", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 534 @Description(shortDefinition="Indicates who or what performed an action", formalDefinition="Indicates who or what performed an action." ) 535 protected List<BiologicallyDerivedProductDispensePerformerComponent> performer; 536 537 /** 538 * The physical location where the dispense was performed. 539 */ 540 @Child(name = "location", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=true) 541 @Description(shortDefinition="Where the dispense occurred", formalDefinition="The physical location where the dispense was performed." ) 542 protected Reference location; 543 544 /** 545 * The amount of product in the dispense. Quantity will depend on the product being dispensed. Examples are: volume; cell count; concentration. 546 */ 547 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=true) 548 @Description(shortDefinition="Amount dispensed", formalDefinition="The amount of product in the dispense. Quantity will depend on the product being dispensed. Examples are: volume; cell count; concentration." ) 549 protected Quantity quantity; 550 551 /** 552 * When the product was selected/ matched. 553 */ 554 @Child(name = "preparedDate", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 555 @Description(shortDefinition="When product was selected/matched", formalDefinition="When the product was selected/ matched." ) 556 protected DateTimeType preparedDate; 557 558 /** 559 * When the product was dispatched for clinical use. 560 */ 561 @Child(name = "whenHandedOver", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=true) 562 @Description(shortDefinition="When the product was dispatched", formalDefinition="When the product was dispatched for clinical use." ) 563 protected DateTimeType whenHandedOver; 564 565 /** 566 * Link to a resource identifying the physical location that the product was dispatched to. 567 */ 568 @Child(name = "destination", type = {Location.class}, order=13, min=0, max=1, modifier=false, summary=true) 569 @Description(shortDefinition="Where the product was dispatched to", formalDefinition="Link to a resource identifying the physical location that the product was dispatched to." ) 570 protected Reference destination; 571 572 /** 573 * Additional notes. 574 */ 575 @Child(name = "note", type = {Annotation.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 576 @Description(shortDefinition="Additional notes", formalDefinition="Additional notes." ) 577 protected List<Annotation> note; 578 579 /** 580 * Specific instructions for use. 581 */ 582 @Child(name = "usageInstruction", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=true) 583 @Description(shortDefinition="Specific instructions for use", formalDefinition="Specific instructions for use." ) 584 protected StringType usageInstruction; 585 586 private static final long serialVersionUID = -109945994L; 587 588 /** 589 * Constructor 590 */ 591 public BiologicallyDerivedProductDispense() { 592 super(); 593 } 594 595 /** 596 * Constructor 597 */ 598 public BiologicallyDerivedProductDispense(BiologicallyDerivedProductDispenseCodes status, Reference product, Reference patient) { 599 super(); 600 this.setStatus(status); 601 this.setProduct(product); 602 this.setPatient(patient); 603 } 604 605 /** 606 * @return {@link #identifier} (Unique instance identifiers assigned to a biologically derived product dispense. Note: This is a business identifier, not a resource identifier.) 607 */ 608 public List<Identifier> getIdentifier() { 609 if (this.identifier == null) 610 this.identifier = new ArrayList<Identifier>(); 611 return this.identifier; 612 } 613 614 /** 615 * @return Returns a reference to <code>this</code> for easy method chaining 616 */ 617 public BiologicallyDerivedProductDispense setIdentifier(List<Identifier> theIdentifier) { 618 this.identifier = theIdentifier; 619 return this; 620 } 621 622 public boolean hasIdentifier() { 623 if (this.identifier == null) 624 return false; 625 for (Identifier item : this.identifier) 626 if (!item.isEmpty()) 627 return true; 628 return false; 629 } 630 631 public Identifier addIdentifier() { //3 632 Identifier t = new Identifier(); 633 if (this.identifier == null) 634 this.identifier = new ArrayList<Identifier>(); 635 this.identifier.add(t); 636 return t; 637 } 638 639 public BiologicallyDerivedProductDispense addIdentifier(Identifier t) { //3 640 if (t == null) 641 return this; 642 if (this.identifier == null) 643 this.identifier = new ArrayList<Identifier>(); 644 this.identifier.add(t); 645 return this; 646 } 647 648 /** 649 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 650 */ 651 public Identifier getIdentifierFirstRep() { 652 if (getIdentifier().isEmpty()) { 653 addIdentifier(); 654 } 655 return getIdentifier().get(0); 656 } 657 658 /** 659 * @return {@link #basedOn} (The order or request that the dispense is fulfilling. This is a reference to a ServiceRequest resource.) 660 */ 661 public List<Reference> getBasedOn() { 662 if (this.basedOn == null) 663 this.basedOn = new ArrayList<Reference>(); 664 return this.basedOn; 665 } 666 667 /** 668 * @return Returns a reference to <code>this</code> for easy method chaining 669 */ 670 public BiologicallyDerivedProductDispense setBasedOn(List<Reference> theBasedOn) { 671 this.basedOn = theBasedOn; 672 return this; 673 } 674 675 public boolean hasBasedOn() { 676 if (this.basedOn == null) 677 return false; 678 for (Reference item : this.basedOn) 679 if (!item.isEmpty()) 680 return true; 681 return false; 682 } 683 684 public Reference addBasedOn() { //3 685 Reference t = new Reference(); 686 if (this.basedOn == null) 687 this.basedOn = new ArrayList<Reference>(); 688 this.basedOn.add(t); 689 return t; 690 } 691 692 public BiologicallyDerivedProductDispense addBasedOn(Reference t) { //3 693 if (t == null) 694 return this; 695 if (this.basedOn == null) 696 this.basedOn = new ArrayList<Reference>(); 697 this.basedOn.add(t); 698 return this; 699 } 700 701 /** 702 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 703 */ 704 public Reference getBasedOnFirstRep() { 705 if (getBasedOn().isEmpty()) { 706 addBasedOn(); 707 } 708 return getBasedOn().get(0); 709 } 710 711 /** 712 * @return {@link #partOf} (A larger event of which this particular event is a component.) 713 */ 714 public List<Reference> getPartOf() { 715 if (this.partOf == null) 716 this.partOf = new ArrayList<Reference>(); 717 return this.partOf; 718 } 719 720 /** 721 * @return Returns a reference to <code>this</code> for easy method chaining 722 */ 723 public BiologicallyDerivedProductDispense setPartOf(List<Reference> thePartOf) { 724 this.partOf = thePartOf; 725 return this; 726 } 727 728 public boolean hasPartOf() { 729 if (this.partOf == null) 730 return false; 731 for (Reference item : this.partOf) 732 if (!item.isEmpty()) 733 return true; 734 return false; 735 } 736 737 public Reference addPartOf() { //3 738 Reference t = new Reference(); 739 if (this.partOf == null) 740 this.partOf = new ArrayList<Reference>(); 741 this.partOf.add(t); 742 return t; 743 } 744 745 public BiologicallyDerivedProductDispense addPartOf(Reference t) { //3 746 if (t == null) 747 return this; 748 if (this.partOf == null) 749 this.partOf = new ArrayList<Reference>(); 750 this.partOf.add(t); 751 return this; 752 } 753 754 /** 755 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 756 */ 757 public Reference getPartOfFirstRep() { 758 if (getPartOf().isEmpty()) { 759 addPartOf(); 760 } 761 return getPartOf().get(0); 762 } 763 764 /** 765 * @return {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 766 */ 767 public Enumeration<BiologicallyDerivedProductDispenseCodes> getStatusElement() { 768 if (this.status == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.status"); 771 else if (Configuration.doAutoCreate()) 772 this.status = new Enumeration<BiologicallyDerivedProductDispenseCodes>(new BiologicallyDerivedProductDispenseCodesEnumFactory()); // bb 773 return this.status; 774 } 775 776 public boolean hasStatusElement() { 777 return this.status != null && !this.status.isEmpty(); 778 } 779 780 public boolean hasStatus() { 781 return this.status != null && !this.status.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 786 */ 787 public BiologicallyDerivedProductDispense setStatusElement(Enumeration<BiologicallyDerivedProductDispenseCodes> value) { 788 this.status = value; 789 return this; 790 } 791 792 /** 793 * @return A code specifying the state of the dispense event. 794 */ 795 public BiologicallyDerivedProductDispenseCodes getStatus() { 796 return this.status == null ? null : this.status.getValue(); 797 } 798 799 /** 800 * @param value A code specifying the state of the dispense event. 801 */ 802 public BiologicallyDerivedProductDispense setStatus(BiologicallyDerivedProductDispenseCodes value) { 803 if (this.status == null) 804 this.status = new Enumeration<BiologicallyDerivedProductDispenseCodes>(new BiologicallyDerivedProductDispenseCodesEnumFactory()); 805 this.status.setValue(value); 806 return this; 807 } 808 809 /** 810 * @return {@link #originRelationshipType} (Indicates the relationship between the donor of the biologically derived product and the intended recipient.) 811 */ 812 public CodeableConcept getOriginRelationshipType() { 813 if (this.originRelationshipType == null) 814 if (Configuration.errorOnAutoCreate()) 815 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.originRelationshipType"); 816 else if (Configuration.doAutoCreate()) 817 this.originRelationshipType = new CodeableConcept(); // cc 818 return this.originRelationshipType; 819 } 820 821 public boolean hasOriginRelationshipType() { 822 return this.originRelationshipType != null && !this.originRelationshipType.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #originRelationshipType} (Indicates the relationship between the donor of the biologically derived product and the intended recipient.) 827 */ 828 public BiologicallyDerivedProductDispense setOriginRelationshipType(CodeableConcept value) { 829 this.originRelationshipType = value; 830 return this; 831 } 832 833 /** 834 * @return {@link #product} (A link to a resource identifying the biologically derived product that is being dispensed.) 835 */ 836 public Reference getProduct() { 837 if (this.product == null) 838 if (Configuration.errorOnAutoCreate()) 839 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.product"); 840 else if (Configuration.doAutoCreate()) 841 this.product = new Reference(); // cc 842 return this.product; 843 } 844 845 public boolean hasProduct() { 846 return this.product != null && !this.product.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #product} (A link to a resource identifying the biologically derived product that is being dispensed.) 851 */ 852 public BiologicallyDerivedProductDispense setProduct(Reference value) { 853 this.product = value; 854 return this; 855 } 856 857 /** 858 * @return {@link #patient} (A link to a resource representing the patient that the product is dispensed for.) 859 */ 860 public Reference getPatient() { 861 if (this.patient == null) 862 if (Configuration.errorOnAutoCreate()) 863 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.patient"); 864 else if (Configuration.doAutoCreate()) 865 this.patient = new Reference(); // cc 866 return this.patient; 867 } 868 869 public boolean hasPatient() { 870 return this.patient != null && !this.patient.isEmpty(); 871 } 872 873 /** 874 * @param value {@link #patient} (A link to a resource representing the patient that the product is dispensed for.) 875 */ 876 public BiologicallyDerivedProductDispense setPatient(Reference value) { 877 this.patient = value; 878 return this; 879 } 880 881 /** 882 * @return {@link #matchStatus} (Indicates the type of matching associated with the dispense.) 883 */ 884 public CodeableConcept getMatchStatus() { 885 if (this.matchStatus == null) 886 if (Configuration.errorOnAutoCreate()) 887 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.matchStatus"); 888 else if (Configuration.doAutoCreate()) 889 this.matchStatus = new CodeableConcept(); // cc 890 return this.matchStatus; 891 } 892 893 public boolean hasMatchStatus() { 894 return this.matchStatus != null && !this.matchStatus.isEmpty(); 895 } 896 897 /** 898 * @param value {@link #matchStatus} (Indicates the type of matching associated with the dispense.) 899 */ 900 public BiologicallyDerivedProductDispense setMatchStatus(CodeableConcept value) { 901 this.matchStatus = value; 902 return this; 903 } 904 905 /** 906 * @return {@link #performer} (Indicates who or what performed an action.) 907 */ 908 public List<BiologicallyDerivedProductDispensePerformerComponent> getPerformer() { 909 if (this.performer == null) 910 this.performer = new ArrayList<BiologicallyDerivedProductDispensePerformerComponent>(); 911 return this.performer; 912 } 913 914 /** 915 * @return Returns a reference to <code>this</code> for easy method chaining 916 */ 917 public BiologicallyDerivedProductDispense setPerformer(List<BiologicallyDerivedProductDispensePerformerComponent> thePerformer) { 918 this.performer = thePerformer; 919 return this; 920 } 921 922 public boolean hasPerformer() { 923 if (this.performer == null) 924 return false; 925 for (BiologicallyDerivedProductDispensePerformerComponent item : this.performer) 926 if (!item.isEmpty()) 927 return true; 928 return false; 929 } 930 931 public BiologicallyDerivedProductDispensePerformerComponent addPerformer() { //3 932 BiologicallyDerivedProductDispensePerformerComponent t = new BiologicallyDerivedProductDispensePerformerComponent(); 933 if (this.performer == null) 934 this.performer = new ArrayList<BiologicallyDerivedProductDispensePerformerComponent>(); 935 this.performer.add(t); 936 return t; 937 } 938 939 public BiologicallyDerivedProductDispense addPerformer(BiologicallyDerivedProductDispensePerformerComponent t) { //3 940 if (t == null) 941 return this; 942 if (this.performer == null) 943 this.performer = new ArrayList<BiologicallyDerivedProductDispensePerformerComponent>(); 944 this.performer.add(t); 945 return this; 946 } 947 948 /** 949 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 950 */ 951 public BiologicallyDerivedProductDispensePerformerComponent getPerformerFirstRep() { 952 if (getPerformer().isEmpty()) { 953 addPerformer(); 954 } 955 return getPerformer().get(0); 956 } 957 958 /** 959 * @return {@link #location} (The physical location where the dispense was performed.) 960 */ 961 public Reference getLocation() { 962 if (this.location == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.location"); 965 else if (Configuration.doAutoCreate()) 966 this.location = new Reference(); // cc 967 return this.location; 968 } 969 970 public boolean hasLocation() { 971 return this.location != null && !this.location.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #location} (The physical location where the dispense was performed.) 976 */ 977 public BiologicallyDerivedProductDispense setLocation(Reference value) { 978 this.location = value; 979 return this; 980 } 981 982 /** 983 * @return {@link #quantity} (The amount of product in the dispense. Quantity will depend on the product being dispensed. Examples are: volume; cell count; concentration.) 984 */ 985 public Quantity getQuantity() { 986 if (this.quantity == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.quantity"); 989 else if (Configuration.doAutoCreate()) 990 this.quantity = new Quantity(); // cc 991 return this.quantity; 992 } 993 994 public boolean hasQuantity() { 995 return this.quantity != null && !this.quantity.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #quantity} (The amount of product in the dispense. Quantity will depend on the product being dispensed. Examples are: volume; cell count; concentration.) 1000 */ 1001 public BiologicallyDerivedProductDispense setQuantity(Quantity value) { 1002 this.quantity = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #preparedDate} (When the product was selected/ matched.). This is the underlying object with id, value and extensions. The accessor "getPreparedDate" gives direct access to the value 1008 */ 1009 public DateTimeType getPreparedDateElement() { 1010 if (this.preparedDate == null) 1011 if (Configuration.errorOnAutoCreate()) 1012 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.preparedDate"); 1013 else if (Configuration.doAutoCreate()) 1014 this.preparedDate = new DateTimeType(); // bb 1015 return this.preparedDate; 1016 } 1017 1018 public boolean hasPreparedDateElement() { 1019 return this.preparedDate != null && !this.preparedDate.isEmpty(); 1020 } 1021 1022 public boolean hasPreparedDate() { 1023 return this.preparedDate != null && !this.preparedDate.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #preparedDate} (When the product was selected/ matched.). This is the underlying object with id, value and extensions. The accessor "getPreparedDate" gives direct access to the value 1028 */ 1029 public BiologicallyDerivedProductDispense setPreparedDateElement(DateTimeType value) { 1030 this.preparedDate = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return When the product was selected/ matched. 1036 */ 1037 public Date getPreparedDate() { 1038 return this.preparedDate == null ? null : this.preparedDate.getValue(); 1039 } 1040 1041 /** 1042 * @param value When the product was selected/ matched. 1043 */ 1044 public BiologicallyDerivedProductDispense setPreparedDate(Date value) { 1045 if (value == null) 1046 this.preparedDate = null; 1047 else { 1048 if (this.preparedDate == null) 1049 this.preparedDate = new DateTimeType(); 1050 this.preparedDate.setValue(value); 1051 } 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #whenHandedOver} (When the product was dispatched for clinical use.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1057 */ 1058 public DateTimeType getWhenHandedOverElement() { 1059 if (this.whenHandedOver == null) 1060 if (Configuration.errorOnAutoCreate()) 1061 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.whenHandedOver"); 1062 else if (Configuration.doAutoCreate()) 1063 this.whenHandedOver = new DateTimeType(); // bb 1064 return this.whenHandedOver; 1065 } 1066 1067 public boolean hasWhenHandedOverElement() { 1068 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1069 } 1070 1071 public boolean hasWhenHandedOver() { 1072 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1073 } 1074 1075 /** 1076 * @param value {@link #whenHandedOver} (When the product was dispatched for clinical use.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1077 */ 1078 public BiologicallyDerivedProductDispense setWhenHandedOverElement(DateTimeType value) { 1079 this.whenHandedOver = value; 1080 return this; 1081 } 1082 1083 /** 1084 * @return When the product was dispatched for clinical use. 1085 */ 1086 public Date getWhenHandedOver() { 1087 return this.whenHandedOver == null ? null : this.whenHandedOver.getValue(); 1088 } 1089 1090 /** 1091 * @param value When the product was dispatched for clinical use. 1092 */ 1093 public BiologicallyDerivedProductDispense setWhenHandedOver(Date value) { 1094 if (value == null) 1095 this.whenHandedOver = null; 1096 else { 1097 if (this.whenHandedOver == null) 1098 this.whenHandedOver = new DateTimeType(); 1099 this.whenHandedOver.setValue(value); 1100 } 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #destination} (Link to a resource identifying the physical location that the product was dispatched to.) 1106 */ 1107 public Reference getDestination() { 1108 if (this.destination == null) 1109 if (Configuration.errorOnAutoCreate()) 1110 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.destination"); 1111 else if (Configuration.doAutoCreate()) 1112 this.destination = new Reference(); // cc 1113 return this.destination; 1114 } 1115 1116 public boolean hasDestination() { 1117 return this.destination != null && !this.destination.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #destination} (Link to a resource identifying the physical location that the product was dispatched to.) 1122 */ 1123 public BiologicallyDerivedProductDispense setDestination(Reference value) { 1124 this.destination = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #note} (Additional notes.) 1130 */ 1131 public List<Annotation> getNote() { 1132 if (this.note == null) 1133 this.note = new ArrayList<Annotation>(); 1134 return this.note; 1135 } 1136 1137 /** 1138 * @return Returns a reference to <code>this</code> for easy method chaining 1139 */ 1140 public BiologicallyDerivedProductDispense setNote(List<Annotation> theNote) { 1141 this.note = theNote; 1142 return this; 1143 } 1144 1145 public boolean hasNote() { 1146 if (this.note == null) 1147 return false; 1148 for (Annotation item : this.note) 1149 if (!item.isEmpty()) 1150 return true; 1151 return false; 1152 } 1153 1154 public Annotation addNote() { //3 1155 Annotation t = new Annotation(); 1156 if (this.note == null) 1157 this.note = new ArrayList<Annotation>(); 1158 this.note.add(t); 1159 return t; 1160 } 1161 1162 public BiologicallyDerivedProductDispense addNote(Annotation t) { //3 1163 if (t == null) 1164 return this; 1165 if (this.note == null) 1166 this.note = new ArrayList<Annotation>(); 1167 this.note.add(t); 1168 return this; 1169 } 1170 1171 /** 1172 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1173 */ 1174 public Annotation getNoteFirstRep() { 1175 if (getNote().isEmpty()) { 1176 addNote(); 1177 } 1178 return getNote().get(0); 1179 } 1180 1181 /** 1182 * @return {@link #usageInstruction} (Specific instructions for use.). This is the underlying object with id, value and extensions. The accessor "getUsageInstruction" gives direct access to the value 1183 */ 1184 public StringType getUsageInstructionElement() { 1185 if (this.usageInstruction == null) 1186 if (Configuration.errorOnAutoCreate()) 1187 throw new Error("Attempt to auto-create BiologicallyDerivedProductDispense.usageInstruction"); 1188 else if (Configuration.doAutoCreate()) 1189 this.usageInstruction = new StringType(); // bb 1190 return this.usageInstruction; 1191 } 1192 1193 public boolean hasUsageInstructionElement() { 1194 return this.usageInstruction != null && !this.usageInstruction.isEmpty(); 1195 } 1196 1197 public boolean hasUsageInstruction() { 1198 return this.usageInstruction != null && !this.usageInstruction.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #usageInstruction} (Specific instructions for use.). This is the underlying object with id, value and extensions. The accessor "getUsageInstruction" gives direct access to the value 1203 */ 1204 public BiologicallyDerivedProductDispense setUsageInstructionElement(StringType value) { 1205 this.usageInstruction = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return Specific instructions for use. 1211 */ 1212 public String getUsageInstruction() { 1213 return this.usageInstruction == null ? null : this.usageInstruction.getValue(); 1214 } 1215 1216 /** 1217 * @param value Specific instructions for use. 1218 */ 1219 public BiologicallyDerivedProductDispense setUsageInstruction(String value) { 1220 if (Utilities.noString(value)) 1221 this.usageInstruction = null; 1222 else { 1223 if (this.usageInstruction == null) 1224 this.usageInstruction = new StringType(); 1225 this.usageInstruction.setValue(value); 1226 } 1227 return this; 1228 } 1229 1230 protected void listChildren(List<Property> children) { 1231 super.listChildren(children); 1232 children.add(new Property("identifier", "Identifier", "Unique instance identifiers assigned to a biologically derived product dispense. Note: This is a business identifier, not a resource identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1233 children.add(new Property("basedOn", "Reference(ServiceRequest)", "The order or request that the dispense is fulfilling. This is a reference to a ServiceRequest resource.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1234 children.add(new Property("partOf", "Reference(BiologicallyDerivedProductDispense)", "A larger event of which this particular event is a component.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1235 children.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status)); 1236 children.add(new Property("originRelationshipType", "CodeableConcept", "Indicates the relationship between the donor of the biologically derived product and the intended recipient.", 0, 1, originRelationshipType)); 1237 children.add(new Property("product", "Reference(BiologicallyDerivedProduct)", "A link to a resource identifying the biologically derived product that is being dispensed.", 0, 1, product)); 1238 children.add(new Property("patient", "Reference(Patient)", "A link to a resource representing the patient that the product is dispensed for.", 0, 1, patient)); 1239 children.add(new Property("matchStatus", "CodeableConcept", "Indicates the type of matching associated with the dispense.", 0, 1, matchStatus)); 1240 children.add(new Property("performer", "", "Indicates who or what performed an action.", 0, java.lang.Integer.MAX_VALUE, performer)); 1241 children.add(new Property("location", "Reference(Location)", "The physical location where the dispense was performed.", 0, 1, location)); 1242 children.add(new Property("quantity", "Quantity", "The amount of product in the dispense. Quantity will depend on the product being dispensed. Examples are: volume; cell count; concentration.", 0, 1, quantity)); 1243 children.add(new Property("preparedDate", "dateTime", "When the product was selected/ matched.", 0, 1, preparedDate)); 1244 children.add(new Property("whenHandedOver", "dateTime", "When the product was dispatched for clinical use.", 0, 1, whenHandedOver)); 1245 children.add(new Property("destination", "Reference(Location)", "Link to a resource identifying the physical location that the product was dispatched to.", 0, 1, destination)); 1246 children.add(new Property("note", "Annotation", "Additional notes.", 0, java.lang.Integer.MAX_VALUE, note)); 1247 children.add(new Property("usageInstruction", "string", "Specific instructions for use.", 0, 1, usageInstruction)); 1248 } 1249 1250 @Override 1251 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1252 switch (_hash) { 1253 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique instance identifiers assigned to a biologically derived product dispense. Note: This is a business identifier, not a resource identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 1254 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ServiceRequest)", "The order or request that the dispense is fulfilling. This is a reference to a ServiceRequest resource.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1255 case -995410646: /*partOf*/ return new Property("partOf", "Reference(BiologicallyDerivedProductDispense)", "A larger event of which this particular event is a component.", 0, java.lang.Integer.MAX_VALUE, partOf); 1256 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status); 1257 case 1746240728: /*originRelationshipType*/ return new Property("originRelationshipType", "CodeableConcept", "Indicates the relationship between the donor of the biologically derived product and the intended recipient.", 0, 1, originRelationshipType); 1258 case -309474065: /*product*/ return new Property("product", "Reference(BiologicallyDerivedProduct)", "A link to a resource identifying the biologically derived product that is being dispensed.", 0, 1, product); 1259 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A link to a resource representing the patient that the product is dispensed for.", 0, 1, patient); 1260 case 1644523031: /*matchStatus*/ return new Property("matchStatus", "CodeableConcept", "Indicates the type of matching associated with the dispense.", 0, 1, matchStatus); 1261 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed an action.", 0, java.lang.Integer.MAX_VALUE, performer); 1262 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The physical location where the dispense was performed.", 0, 1, location); 1263 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of product in the dispense. Quantity will depend on the product being dispensed. Examples are: volume; cell count; concentration.", 0, 1, quantity); 1264 case -2024959605: /*preparedDate*/ return new Property("preparedDate", "dateTime", "When the product was selected/ matched.", 0, 1, preparedDate); 1265 case -940241380: /*whenHandedOver*/ return new Property("whenHandedOver", "dateTime", "When the product was dispatched for clinical use.", 0, 1, whenHandedOver); 1266 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Link to a resource identifying the physical location that the product was dispatched to.", 0, 1, destination); 1267 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes.", 0, java.lang.Integer.MAX_VALUE, note); 1268 case 2138372141: /*usageInstruction*/ return new Property("usageInstruction", "string", "Specific instructions for use.", 0, 1, usageInstruction); 1269 default: return super.getNamedProperty(_hash, _name, _checkValid); 1270 } 1271 1272 } 1273 1274 @Override 1275 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1276 switch (hash) { 1277 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1278 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1279 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1280 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<BiologicallyDerivedProductDispenseCodes> 1281 case 1746240728: /*originRelationshipType*/ return this.originRelationshipType == null ? new Base[0] : new Base[] {this.originRelationshipType}; // CodeableConcept 1282 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // Reference 1283 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1284 case 1644523031: /*matchStatus*/ return this.matchStatus == null ? new Base[0] : new Base[] {this.matchStatus}; // CodeableConcept 1285 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // BiologicallyDerivedProductDispensePerformerComponent 1286 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1287 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1288 case -2024959605: /*preparedDate*/ return this.preparedDate == null ? new Base[0] : new Base[] {this.preparedDate}; // DateTimeType 1289 case -940241380: /*whenHandedOver*/ return this.whenHandedOver == null ? new Base[0] : new Base[] {this.whenHandedOver}; // DateTimeType 1290 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1291 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1292 case 2138372141: /*usageInstruction*/ return this.usageInstruction == null ? new Base[0] : new Base[] {this.usageInstruction}; // StringType 1293 default: return super.getProperty(hash, name, checkValid); 1294 } 1295 1296 } 1297 1298 @Override 1299 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1300 switch (hash) { 1301 case -1618432855: // identifier 1302 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1303 return value; 1304 case -332612366: // basedOn 1305 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1306 return value; 1307 case -995410646: // partOf 1308 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1309 return value; 1310 case -892481550: // status 1311 value = new BiologicallyDerivedProductDispenseCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1312 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductDispenseCodes> 1313 return value; 1314 case 1746240728: // originRelationshipType 1315 this.originRelationshipType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1316 return value; 1317 case -309474065: // product 1318 this.product = TypeConvertor.castToReference(value); // Reference 1319 return value; 1320 case -791418107: // patient 1321 this.patient = TypeConvertor.castToReference(value); // Reference 1322 return value; 1323 case 1644523031: // matchStatus 1324 this.matchStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1325 return value; 1326 case 481140686: // performer 1327 this.getPerformer().add((BiologicallyDerivedProductDispensePerformerComponent) value); // BiologicallyDerivedProductDispensePerformerComponent 1328 return value; 1329 case 1901043637: // location 1330 this.location = TypeConvertor.castToReference(value); // Reference 1331 return value; 1332 case -1285004149: // quantity 1333 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1334 return value; 1335 case -2024959605: // preparedDate 1336 this.preparedDate = TypeConvertor.castToDateTime(value); // DateTimeType 1337 return value; 1338 case -940241380: // whenHandedOver 1339 this.whenHandedOver = TypeConvertor.castToDateTime(value); // DateTimeType 1340 return value; 1341 case -1429847026: // destination 1342 this.destination = TypeConvertor.castToReference(value); // Reference 1343 return value; 1344 case 3387378: // note 1345 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1346 return value; 1347 case 2138372141: // usageInstruction 1348 this.usageInstruction = TypeConvertor.castToString(value); // StringType 1349 return value; 1350 default: return super.setProperty(hash, name, value); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base setProperty(String name, Base value) throws FHIRException { 1357 if (name.equals("identifier")) { 1358 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1359 } else if (name.equals("basedOn")) { 1360 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1361 } else if (name.equals("partOf")) { 1362 this.getPartOf().add(TypeConvertor.castToReference(value)); 1363 } else if (name.equals("status")) { 1364 value = new BiologicallyDerivedProductDispenseCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1365 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductDispenseCodes> 1366 } else if (name.equals("originRelationshipType")) { 1367 this.originRelationshipType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1368 } else if (name.equals("product")) { 1369 this.product = TypeConvertor.castToReference(value); // Reference 1370 } else if (name.equals("patient")) { 1371 this.patient = TypeConvertor.castToReference(value); // Reference 1372 } else if (name.equals("matchStatus")) { 1373 this.matchStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1374 } else if (name.equals("performer")) { 1375 this.getPerformer().add((BiologicallyDerivedProductDispensePerformerComponent) value); 1376 } else if (name.equals("location")) { 1377 this.location = TypeConvertor.castToReference(value); // Reference 1378 } else if (name.equals("quantity")) { 1379 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1380 } else if (name.equals("preparedDate")) { 1381 this.preparedDate = TypeConvertor.castToDateTime(value); // DateTimeType 1382 } else if (name.equals("whenHandedOver")) { 1383 this.whenHandedOver = TypeConvertor.castToDateTime(value); // DateTimeType 1384 } else if (name.equals("destination")) { 1385 this.destination = TypeConvertor.castToReference(value); // Reference 1386 } else if (name.equals("note")) { 1387 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1388 } else if (name.equals("usageInstruction")) { 1389 this.usageInstruction = TypeConvertor.castToString(value); // StringType 1390 } else 1391 return super.setProperty(name, value); 1392 return value; 1393 } 1394 1395 @Override 1396 public void removeChild(String name, Base value) throws FHIRException { 1397 if (name.equals("identifier")) { 1398 this.getIdentifier().remove(value); 1399 } else if (name.equals("basedOn")) { 1400 this.getBasedOn().remove(value); 1401 } else if (name.equals("partOf")) { 1402 this.getPartOf().remove(value); 1403 } else if (name.equals("status")) { 1404 value = new BiologicallyDerivedProductDispenseCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1405 this.status = (Enumeration) value; // Enumeration<BiologicallyDerivedProductDispenseCodes> 1406 } else if (name.equals("originRelationshipType")) { 1407 this.originRelationshipType = null; 1408 } else if (name.equals("product")) { 1409 this.product = null; 1410 } else if (name.equals("patient")) { 1411 this.patient = null; 1412 } else if (name.equals("matchStatus")) { 1413 this.matchStatus = null; 1414 } else if (name.equals("performer")) { 1415 this.getPerformer().remove((BiologicallyDerivedProductDispensePerformerComponent) value); 1416 } else if (name.equals("location")) { 1417 this.location = null; 1418 } else if (name.equals("quantity")) { 1419 this.quantity = null; 1420 } else if (name.equals("preparedDate")) { 1421 this.preparedDate = null; 1422 } else if (name.equals("whenHandedOver")) { 1423 this.whenHandedOver = null; 1424 } else if (name.equals("destination")) { 1425 this.destination = null; 1426 } else if (name.equals("note")) { 1427 this.getNote().remove(value); 1428 } else if (name.equals("usageInstruction")) { 1429 this.usageInstruction = null; 1430 } else 1431 super.removeChild(name, value); 1432 1433 } 1434 1435 @Override 1436 public Base makeProperty(int hash, String name) throws FHIRException { 1437 switch (hash) { 1438 case -1618432855: return addIdentifier(); 1439 case -332612366: return addBasedOn(); 1440 case -995410646: return addPartOf(); 1441 case -892481550: return getStatusElement(); 1442 case 1746240728: return getOriginRelationshipType(); 1443 case -309474065: return getProduct(); 1444 case -791418107: return getPatient(); 1445 case 1644523031: return getMatchStatus(); 1446 case 481140686: return addPerformer(); 1447 case 1901043637: return getLocation(); 1448 case -1285004149: return getQuantity(); 1449 case -2024959605: return getPreparedDateElement(); 1450 case -940241380: return getWhenHandedOverElement(); 1451 case -1429847026: return getDestination(); 1452 case 3387378: return addNote(); 1453 case 2138372141: return getUsageInstructionElement(); 1454 default: return super.makeProperty(hash, name); 1455 } 1456 1457 } 1458 1459 @Override 1460 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1461 switch (hash) { 1462 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1463 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1464 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1465 case -892481550: /*status*/ return new String[] {"code"}; 1466 case 1746240728: /*originRelationshipType*/ return new String[] {"CodeableConcept"}; 1467 case -309474065: /*product*/ return new String[] {"Reference"}; 1468 case -791418107: /*patient*/ return new String[] {"Reference"}; 1469 case 1644523031: /*matchStatus*/ return new String[] {"CodeableConcept"}; 1470 case 481140686: /*performer*/ return new String[] {}; 1471 case 1901043637: /*location*/ return new String[] {"Reference"}; 1472 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1473 case -2024959605: /*preparedDate*/ return new String[] {"dateTime"}; 1474 case -940241380: /*whenHandedOver*/ return new String[] {"dateTime"}; 1475 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1476 case 3387378: /*note*/ return new String[] {"Annotation"}; 1477 case 2138372141: /*usageInstruction*/ return new String[] {"string"}; 1478 default: return super.getTypesForProperty(hash, name); 1479 } 1480 1481 } 1482 1483 @Override 1484 public Base addChild(String name) throws FHIRException { 1485 if (name.equals("identifier")) { 1486 return addIdentifier(); 1487 } 1488 else if (name.equals("basedOn")) { 1489 return addBasedOn(); 1490 } 1491 else if (name.equals("partOf")) { 1492 return addPartOf(); 1493 } 1494 else if (name.equals("status")) { 1495 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProductDispense.status"); 1496 } 1497 else if (name.equals("originRelationshipType")) { 1498 this.originRelationshipType = new CodeableConcept(); 1499 return this.originRelationshipType; 1500 } 1501 else if (name.equals("product")) { 1502 this.product = new Reference(); 1503 return this.product; 1504 } 1505 else if (name.equals("patient")) { 1506 this.patient = new Reference(); 1507 return this.patient; 1508 } 1509 else if (name.equals("matchStatus")) { 1510 this.matchStatus = new CodeableConcept(); 1511 return this.matchStatus; 1512 } 1513 else if (name.equals("performer")) { 1514 return addPerformer(); 1515 } 1516 else if (name.equals("location")) { 1517 this.location = new Reference(); 1518 return this.location; 1519 } 1520 else if (name.equals("quantity")) { 1521 this.quantity = new Quantity(); 1522 return this.quantity; 1523 } 1524 else if (name.equals("preparedDate")) { 1525 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProductDispense.preparedDate"); 1526 } 1527 else if (name.equals("whenHandedOver")) { 1528 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProductDispense.whenHandedOver"); 1529 } 1530 else if (name.equals("destination")) { 1531 this.destination = new Reference(); 1532 return this.destination; 1533 } 1534 else if (name.equals("note")) { 1535 return addNote(); 1536 } 1537 else if (name.equals("usageInstruction")) { 1538 throw new FHIRException("Cannot call addChild on a singleton property BiologicallyDerivedProductDispense.usageInstruction"); 1539 } 1540 else 1541 return super.addChild(name); 1542 } 1543 1544 public String fhirType() { 1545 return "BiologicallyDerivedProductDispense"; 1546 1547 } 1548 1549 public BiologicallyDerivedProductDispense copy() { 1550 BiologicallyDerivedProductDispense dst = new BiologicallyDerivedProductDispense(); 1551 copyValues(dst); 1552 return dst; 1553 } 1554 1555 public void copyValues(BiologicallyDerivedProductDispense dst) { 1556 super.copyValues(dst); 1557 if (identifier != null) { 1558 dst.identifier = new ArrayList<Identifier>(); 1559 for (Identifier i : identifier) 1560 dst.identifier.add(i.copy()); 1561 }; 1562 if (basedOn != null) { 1563 dst.basedOn = new ArrayList<Reference>(); 1564 for (Reference i : basedOn) 1565 dst.basedOn.add(i.copy()); 1566 }; 1567 if (partOf != null) { 1568 dst.partOf = new ArrayList<Reference>(); 1569 for (Reference i : partOf) 1570 dst.partOf.add(i.copy()); 1571 }; 1572 dst.status = status == null ? null : status.copy(); 1573 dst.originRelationshipType = originRelationshipType == null ? null : originRelationshipType.copy(); 1574 dst.product = product == null ? null : product.copy(); 1575 dst.patient = patient == null ? null : patient.copy(); 1576 dst.matchStatus = matchStatus == null ? null : matchStatus.copy(); 1577 if (performer != null) { 1578 dst.performer = new ArrayList<BiologicallyDerivedProductDispensePerformerComponent>(); 1579 for (BiologicallyDerivedProductDispensePerformerComponent i : performer) 1580 dst.performer.add(i.copy()); 1581 }; 1582 dst.location = location == null ? null : location.copy(); 1583 dst.quantity = quantity == null ? null : quantity.copy(); 1584 dst.preparedDate = preparedDate == null ? null : preparedDate.copy(); 1585 dst.whenHandedOver = whenHandedOver == null ? null : whenHandedOver.copy(); 1586 dst.destination = destination == null ? null : destination.copy(); 1587 if (note != null) { 1588 dst.note = new ArrayList<Annotation>(); 1589 for (Annotation i : note) 1590 dst.note.add(i.copy()); 1591 }; 1592 dst.usageInstruction = usageInstruction == null ? null : usageInstruction.copy(); 1593 } 1594 1595 protected BiologicallyDerivedProductDispense typedCopy() { 1596 return copy(); 1597 } 1598 1599 @Override 1600 public boolean equalsDeep(Base other_) { 1601 if (!super.equalsDeep(other_)) 1602 return false; 1603 if (!(other_ instanceof BiologicallyDerivedProductDispense)) 1604 return false; 1605 BiologicallyDerivedProductDispense o = (BiologicallyDerivedProductDispense) other_; 1606 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1607 && compareDeep(status, o.status, true) && compareDeep(originRelationshipType, o.originRelationshipType, true) 1608 && compareDeep(product, o.product, true) && compareDeep(patient, o.patient, true) && compareDeep(matchStatus, o.matchStatus, true) 1609 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) && compareDeep(quantity, o.quantity, true) 1610 && compareDeep(preparedDate, o.preparedDate, true) && compareDeep(whenHandedOver, o.whenHandedOver, true) 1611 && compareDeep(destination, o.destination, true) && compareDeep(note, o.note, true) && compareDeep(usageInstruction, o.usageInstruction, true) 1612 ; 1613 } 1614 1615 @Override 1616 public boolean equalsShallow(Base other_) { 1617 if (!super.equalsShallow(other_)) 1618 return false; 1619 if (!(other_ instanceof BiologicallyDerivedProductDispense)) 1620 return false; 1621 BiologicallyDerivedProductDispense o = (BiologicallyDerivedProductDispense) other_; 1622 return compareValues(status, o.status, true) && compareValues(preparedDate, o.preparedDate, true) && compareValues(whenHandedOver, o.whenHandedOver, true) 1623 && compareValues(usageInstruction, o.usageInstruction, true); 1624 } 1625 1626 public boolean isEmpty() { 1627 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1628 , status, originRelationshipType, product, patient, matchStatus, performer, location 1629 , quantity, preparedDate, whenHandedOver, destination, note, usageInstruction); 1630 } 1631 1632 @Override 1633 public ResourceType getResourceType() { 1634 return ResourceType.BiologicallyDerivedProductDispense; 1635 } 1636 1637 /** 1638 * Search parameter: <b>identifier</b> 1639 * <p> 1640 * Description: <b>The identifier of the dispense</b><br> 1641 * Type: <b>token</b><br> 1642 * Path: <b>BiologicallyDerivedProductDispense.identifier</b><br> 1643 * </p> 1644 */ 1645 @SearchParamDefinition(name="identifier", path="BiologicallyDerivedProductDispense.identifier", description="The identifier of the dispense", type="token" ) 1646 public static final String SP_IDENTIFIER = "identifier"; 1647 /** 1648 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1649 * <p> 1650 * Description: <b>The identifier of the dispense</b><br> 1651 * Type: <b>token</b><br> 1652 * Path: <b>BiologicallyDerivedProductDispense.identifier</b><br> 1653 * </p> 1654 */ 1655 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1656 1657 /** 1658 * Search parameter: <b>patient</b> 1659 * <p> 1660 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 1661 * Type: <b>reference</b><br> 1662 * Path: <b>BiologicallyDerivedProductDispense.patient</b><br> 1663 * </p> 1664 */ 1665 @SearchParamDefinition(name="patient", path="BiologicallyDerivedProductDispense.patient", description="The identity of a patient for whom to list dispenses", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1666 public static final String SP_PATIENT = "patient"; 1667 /** 1668 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1669 * <p> 1670 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 1671 * Type: <b>reference</b><br> 1672 * Path: <b>BiologicallyDerivedProductDispense.patient</b><br> 1673 * </p> 1674 */ 1675 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1676 1677/** 1678 * Constant for fluent queries to be used to add include statements. Specifies 1679 * the path value of "<b>BiologicallyDerivedProductDispense:patient</b>". 1680 */ 1681 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("BiologicallyDerivedProductDispense:patient").toLocked(); 1682 1683 /** 1684 * Search parameter: <b>performer</b> 1685 * <p> 1686 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 1687 * Type: <b>reference</b><br> 1688 * Path: <b>BiologicallyDerivedProductDispense.performer.actor</b><br> 1689 * </p> 1690 */ 1691 @SearchParamDefinition(name="performer", path="BiologicallyDerivedProductDispense.performer.actor", description="The identity of a patient for whom to list dispenses", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class } ) 1692 public static final String SP_PERFORMER = "performer"; 1693 /** 1694 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 1695 * <p> 1696 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 1697 * Type: <b>reference</b><br> 1698 * Path: <b>BiologicallyDerivedProductDispense.performer.actor</b><br> 1699 * </p> 1700 */ 1701 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 1702 1703/** 1704 * Constant for fluent queries to be used to add include statements. Specifies 1705 * the path value of "<b>BiologicallyDerivedProductDispense:performer</b>". 1706 */ 1707 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("BiologicallyDerivedProductDispense:performer").toLocked(); 1708 1709 /** 1710 * Search parameter: <b>product</b> 1711 * <p> 1712 * Description: <b>Search for products that match this code</b><br> 1713 * Type: <b>reference</b><br> 1714 * Path: <b>BiologicallyDerivedProductDispense.product</b><br> 1715 * </p> 1716 */ 1717 @SearchParamDefinition(name="product", path="BiologicallyDerivedProductDispense.product", description="Search for products that match this code", type="reference", target={BiologicallyDerivedProduct.class } ) 1718 public static final String SP_PRODUCT = "product"; 1719 /** 1720 * <b>Fluent Client</b> search parameter constant for <b>product</b> 1721 * <p> 1722 * Description: <b>Search for products that match this code</b><br> 1723 * Type: <b>reference</b><br> 1724 * Path: <b>BiologicallyDerivedProductDispense.product</b><br> 1725 * </p> 1726 */ 1727 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRODUCT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRODUCT); 1728 1729/** 1730 * Constant for fluent queries to be used to add include statements. Specifies 1731 * the path value of "<b>BiologicallyDerivedProductDispense:product</b>". 1732 */ 1733 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRODUCT = new ca.uhn.fhir.model.api.Include("BiologicallyDerivedProductDispense:product").toLocked(); 1734 1735 /** 1736 * Search parameter: <b>status</b> 1737 * <p> 1738 * Description: <b>The status of the dispense</b><br> 1739 * Type: <b>token</b><br> 1740 * Path: <b>BiologicallyDerivedProductDispense.status</b><br> 1741 * </p> 1742 */ 1743 @SearchParamDefinition(name="status", path="BiologicallyDerivedProductDispense.status", description="The status of the dispense", type="token" ) 1744 public static final String SP_STATUS = "status"; 1745 /** 1746 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1747 * <p> 1748 * Description: <b>The status of the dispense</b><br> 1749 * Type: <b>token</b><br> 1750 * Path: <b>BiologicallyDerivedProductDispense.status</b><br> 1751 * </p> 1752 */ 1753 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1754 1755 1756} 1757