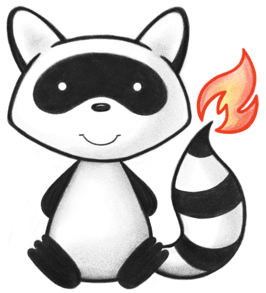
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049import org.hl7.fhir.utilities.Utilities; 050/** 051 * Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 052 */ 053@ResourceDef(name="BodyStructure", profile="http://hl7.org/fhir/StructureDefinition/BodyStructure") 054public class BodyStructure extends DomainResource { 055 056 @Block() 057 public static class BodyStructureIncludedStructureComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Code that represents the included structure. 060 */ 061 @Child(name = "structure", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Code that represents the included structure", formalDefinition="Code that represents the included structure." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 064 protected CodeableConcept structure; 065 066 /** 067 * Code that represents the included structure laterality. 068 */ 069 @Child(name = "laterality", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Code that represents the included structure laterality", formalDefinition="Code that represents the included structure laterality." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodystructure-relative-location") 072 protected CodeableConcept laterality; 073 074 /** 075 * Body locations in relation to a specific body landmark (tatoo, scar, other body structure). 076 */ 077 @Child(name = "bodyLandmarkOrientation", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 078 @Description(shortDefinition="Landmark relative location", formalDefinition="Body locations in relation to a specific body landmark (tatoo, scar, other body structure)." ) 079 protected List<BodyStructureIncludedStructureBodyLandmarkOrientationComponent> bodyLandmarkOrientation; 080 081 /** 082 * XY or XYZ-coordinate orientation for structure. 083 */ 084 @Child(name = "spatialReference", type = {ImagingSelection.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 085 @Description(shortDefinition="Cartesian reference for structure", formalDefinition="XY or XYZ-coordinate orientation for structure." ) 086 protected List<Reference> spatialReference; 087 088 /** 089 * Code that represents the included structure qualifier. 090 */ 091 @Child(name = "qualifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 092 @Description(shortDefinition="Code that represents the included structure qualifier", formalDefinition="Code that represents the included structure qualifier." ) 093 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodystructure-relative-location") 094 protected List<CodeableConcept> qualifier; 095 096 private static final long serialVersionUID = -1341852782L; 097 098 /** 099 * Constructor 100 */ 101 public BodyStructureIncludedStructureComponent() { 102 super(); 103 } 104 105 /** 106 * Constructor 107 */ 108 public BodyStructureIncludedStructureComponent(CodeableConcept structure) { 109 super(); 110 this.setStructure(structure); 111 } 112 113 /** 114 * @return {@link #structure} (Code that represents the included structure.) 115 */ 116 public CodeableConcept getStructure() { 117 if (this.structure == null) 118 if (Configuration.errorOnAutoCreate()) 119 throw new Error("Attempt to auto-create BodyStructureIncludedStructureComponent.structure"); 120 else if (Configuration.doAutoCreate()) 121 this.structure = new CodeableConcept(); // cc 122 return this.structure; 123 } 124 125 public boolean hasStructure() { 126 return this.structure != null && !this.structure.isEmpty(); 127 } 128 129 /** 130 * @param value {@link #structure} (Code that represents the included structure.) 131 */ 132 public BodyStructureIncludedStructureComponent setStructure(CodeableConcept value) { 133 this.structure = value; 134 return this; 135 } 136 137 /** 138 * @return {@link #laterality} (Code that represents the included structure laterality.) 139 */ 140 public CodeableConcept getLaterality() { 141 if (this.laterality == null) 142 if (Configuration.errorOnAutoCreate()) 143 throw new Error("Attempt to auto-create BodyStructureIncludedStructureComponent.laterality"); 144 else if (Configuration.doAutoCreate()) 145 this.laterality = new CodeableConcept(); // cc 146 return this.laterality; 147 } 148 149 public boolean hasLaterality() { 150 return this.laterality != null && !this.laterality.isEmpty(); 151 } 152 153 /** 154 * @param value {@link #laterality} (Code that represents the included structure laterality.) 155 */ 156 public BodyStructureIncludedStructureComponent setLaterality(CodeableConcept value) { 157 this.laterality = value; 158 return this; 159 } 160 161 /** 162 * @return {@link #bodyLandmarkOrientation} (Body locations in relation to a specific body landmark (tatoo, scar, other body structure).) 163 */ 164 public List<BodyStructureIncludedStructureBodyLandmarkOrientationComponent> getBodyLandmarkOrientation() { 165 if (this.bodyLandmarkOrientation == null) 166 this.bodyLandmarkOrientation = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationComponent>(); 167 return this.bodyLandmarkOrientation; 168 } 169 170 /** 171 * @return Returns a reference to <code>this</code> for easy method chaining 172 */ 173 public BodyStructureIncludedStructureComponent setBodyLandmarkOrientation(List<BodyStructureIncludedStructureBodyLandmarkOrientationComponent> theBodyLandmarkOrientation) { 174 this.bodyLandmarkOrientation = theBodyLandmarkOrientation; 175 return this; 176 } 177 178 public boolean hasBodyLandmarkOrientation() { 179 if (this.bodyLandmarkOrientation == null) 180 return false; 181 for (BodyStructureIncludedStructureBodyLandmarkOrientationComponent item : this.bodyLandmarkOrientation) 182 if (!item.isEmpty()) 183 return true; 184 return false; 185 } 186 187 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent addBodyLandmarkOrientation() { //3 188 BodyStructureIncludedStructureBodyLandmarkOrientationComponent t = new BodyStructureIncludedStructureBodyLandmarkOrientationComponent(); 189 if (this.bodyLandmarkOrientation == null) 190 this.bodyLandmarkOrientation = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationComponent>(); 191 this.bodyLandmarkOrientation.add(t); 192 return t; 193 } 194 195 public BodyStructureIncludedStructureComponent addBodyLandmarkOrientation(BodyStructureIncludedStructureBodyLandmarkOrientationComponent t) { //3 196 if (t == null) 197 return this; 198 if (this.bodyLandmarkOrientation == null) 199 this.bodyLandmarkOrientation = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationComponent>(); 200 this.bodyLandmarkOrientation.add(t); 201 return this; 202 } 203 204 /** 205 * @return The first repetition of repeating field {@link #bodyLandmarkOrientation}, creating it if it does not already exist {3} 206 */ 207 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent getBodyLandmarkOrientationFirstRep() { 208 if (getBodyLandmarkOrientation().isEmpty()) { 209 addBodyLandmarkOrientation(); 210 } 211 return getBodyLandmarkOrientation().get(0); 212 } 213 214 /** 215 * @return {@link #spatialReference} (XY or XYZ-coordinate orientation for structure.) 216 */ 217 public List<Reference> getSpatialReference() { 218 if (this.spatialReference == null) 219 this.spatialReference = new ArrayList<Reference>(); 220 return this.spatialReference; 221 } 222 223 /** 224 * @return Returns a reference to <code>this</code> for easy method chaining 225 */ 226 public BodyStructureIncludedStructureComponent setSpatialReference(List<Reference> theSpatialReference) { 227 this.spatialReference = theSpatialReference; 228 return this; 229 } 230 231 public boolean hasSpatialReference() { 232 if (this.spatialReference == null) 233 return false; 234 for (Reference item : this.spatialReference) 235 if (!item.isEmpty()) 236 return true; 237 return false; 238 } 239 240 public Reference addSpatialReference() { //3 241 Reference t = new Reference(); 242 if (this.spatialReference == null) 243 this.spatialReference = new ArrayList<Reference>(); 244 this.spatialReference.add(t); 245 return t; 246 } 247 248 public BodyStructureIncludedStructureComponent addSpatialReference(Reference t) { //3 249 if (t == null) 250 return this; 251 if (this.spatialReference == null) 252 this.spatialReference = new ArrayList<Reference>(); 253 this.spatialReference.add(t); 254 return this; 255 } 256 257 /** 258 * @return The first repetition of repeating field {@link #spatialReference}, creating it if it does not already exist {3} 259 */ 260 public Reference getSpatialReferenceFirstRep() { 261 if (getSpatialReference().isEmpty()) { 262 addSpatialReference(); 263 } 264 return getSpatialReference().get(0); 265 } 266 267 /** 268 * @return {@link #qualifier} (Code that represents the included structure qualifier.) 269 */ 270 public List<CodeableConcept> getQualifier() { 271 if (this.qualifier == null) 272 this.qualifier = new ArrayList<CodeableConcept>(); 273 return this.qualifier; 274 } 275 276 /** 277 * @return Returns a reference to <code>this</code> for easy method chaining 278 */ 279 public BodyStructureIncludedStructureComponent setQualifier(List<CodeableConcept> theQualifier) { 280 this.qualifier = theQualifier; 281 return this; 282 } 283 284 public boolean hasQualifier() { 285 if (this.qualifier == null) 286 return false; 287 for (CodeableConcept item : this.qualifier) 288 if (!item.isEmpty()) 289 return true; 290 return false; 291 } 292 293 public CodeableConcept addQualifier() { //3 294 CodeableConcept t = new CodeableConcept(); 295 if (this.qualifier == null) 296 this.qualifier = new ArrayList<CodeableConcept>(); 297 this.qualifier.add(t); 298 return t; 299 } 300 301 public BodyStructureIncludedStructureComponent addQualifier(CodeableConcept t) { //3 302 if (t == null) 303 return this; 304 if (this.qualifier == null) 305 this.qualifier = new ArrayList<CodeableConcept>(); 306 this.qualifier.add(t); 307 return this; 308 } 309 310 /** 311 * @return The first repetition of repeating field {@link #qualifier}, creating it if it does not already exist {3} 312 */ 313 public CodeableConcept getQualifierFirstRep() { 314 if (getQualifier().isEmpty()) { 315 addQualifier(); 316 } 317 return getQualifier().get(0); 318 } 319 320 protected void listChildren(List<Property> children) { 321 super.listChildren(children); 322 children.add(new Property("structure", "CodeableConcept", "Code that represents the included structure.", 0, 1, structure)); 323 children.add(new Property("laterality", "CodeableConcept", "Code that represents the included structure laterality.", 0, 1, laterality)); 324 children.add(new Property("bodyLandmarkOrientation", "", "Body locations in relation to a specific body landmark (tatoo, scar, other body structure).", 0, java.lang.Integer.MAX_VALUE, bodyLandmarkOrientation)); 325 children.add(new Property("spatialReference", "Reference(ImagingSelection)", "XY or XYZ-coordinate orientation for structure.", 0, java.lang.Integer.MAX_VALUE, spatialReference)); 326 children.add(new Property("qualifier", "CodeableConcept", "Code that represents the included structure qualifier.", 0, java.lang.Integer.MAX_VALUE, qualifier)); 327 } 328 329 @Override 330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 331 switch (_hash) { 332 case 144518515: /*structure*/ return new Property("structure", "CodeableConcept", "Code that represents the included structure.", 0, 1, structure); 333 case -170291817: /*laterality*/ return new Property("laterality", "CodeableConcept", "Code that represents the included structure laterality.", 0, 1, laterality); 334 case -994716042: /*bodyLandmarkOrientation*/ return new Property("bodyLandmarkOrientation", "", "Body locations in relation to a specific body landmark (tatoo, scar, other body structure).", 0, java.lang.Integer.MAX_VALUE, bodyLandmarkOrientation); 335 case 784017063: /*spatialReference*/ return new Property("spatialReference", "Reference(ImagingSelection)", "XY or XYZ-coordinate orientation for structure.", 0, java.lang.Integer.MAX_VALUE, spatialReference); 336 case -1247940438: /*qualifier*/ return new Property("qualifier", "CodeableConcept", "Code that represents the included structure qualifier.", 0, java.lang.Integer.MAX_VALUE, qualifier); 337 default: return super.getNamedProperty(_hash, _name, _checkValid); 338 } 339 340 } 341 342 @Override 343 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 344 switch (hash) { 345 case 144518515: /*structure*/ return this.structure == null ? new Base[0] : new Base[] {this.structure}; // CodeableConcept 346 case -170291817: /*laterality*/ return this.laterality == null ? new Base[0] : new Base[] {this.laterality}; // CodeableConcept 347 case -994716042: /*bodyLandmarkOrientation*/ return this.bodyLandmarkOrientation == null ? new Base[0] : this.bodyLandmarkOrientation.toArray(new Base[this.bodyLandmarkOrientation.size()]); // BodyStructureIncludedStructureBodyLandmarkOrientationComponent 348 case 784017063: /*spatialReference*/ return this.spatialReference == null ? new Base[0] : this.spatialReference.toArray(new Base[this.spatialReference.size()]); // Reference 349 case -1247940438: /*qualifier*/ return this.qualifier == null ? new Base[0] : this.qualifier.toArray(new Base[this.qualifier.size()]); // CodeableConcept 350 default: return super.getProperty(hash, name, checkValid); 351 } 352 353 } 354 355 @Override 356 public Base setProperty(int hash, String name, Base value) throws FHIRException { 357 switch (hash) { 358 case 144518515: // structure 359 this.structure = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 360 return value; 361 case -170291817: // laterality 362 this.laterality = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 363 return value; 364 case -994716042: // bodyLandmarkOrientation 365 this.getBodyLandmarkOrientation().add((BodyStructureIncludedStructureBodyLandmarkOrientationComponent) value); // BodyStructureIncludedStructureBodyLandmarkOrientationComponent 366 return value; 367 case 784017063: // spatialReference 368 this.getSpatialReference().add(TypeConvertor.castToReference(value)); // Reference 369 return value; 370 case -1247940438: // qualifier 371 this.getQualifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 372 return value; 373 default: return super.setProperty(hash, name, value); 374 } 375 376 } 377 378 @Override 379 public Base setProperty(String name, Base value) throws FHIRException { 380 if (name.equals("structure")) { 381 this.structure = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 382 } else if (name.equals("laterality")) { 383 this.laterality = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 384 } else if (name.equals("bodyLandmarkOrientation")) { 385 this.getBodyLandmarkOrientation().add((BodyStructureIncludedStructureBodyLandmarkOrientationComponent) value); 386 } else if (name.equals("spatialReference")) { 387 this.getSpatialReference().add(TypeConvertor.castToReference(value)); 388 } else if (name.equals("qualifier")) { 389 this.getQualifier().add(TypeConvertor.castToCodeableConcept(value)); 390 } else 391 return super.setProperty(name, value); 392 return value; 393 } 394 395 @Override 396 public void removeChild(String name, Base value) throws FHIRException { 397 if (name.equals("structure")) { 398 this.structure = null; 399 } else if (name.equals("laterality")) { 400 this.laterality = null; 401 } else if (name.equals("bodyLandmarkOrientation")) { 402 this.getBodyLandmarkOrientation().remove((BodyStructureIncludedStructureBodyLandmarkOrientationComponent) value); 403 } else if (name.equals("spatialReference")) { 404 this.getSpatialReference().remove(value); 405 } else if (name.equals("qualifier")) { 406 this.getQualifier().remove(value); 407 } else 408 super.removeChild(name, value); 409 410 } 411 412 @Override 413 public Base makeProperty(int hash, String name) throws FHIRException { 414 switch (hash) { 415 case 144518515: return getStructure(); 416 case -170291817: return getLaterality(); 417 case -994716042: return addBodyLandmarkOrientation(); 418 case 784017063: return addSpatialReference(); 419 case -1247940438: return addQualifier(); 420 default: return super.makeProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 427 switch (hash) { 428 case 144518515: /*structure*/ return new String[] {"CodeableConcept"}; 429 case -170291817: /*laterality*/ return new String[] {"CodeableConcept"}; 430 case -994716042: /*bodyLandmarkOrientation*/ return new String[] {}; 431 case 784017063: /*spatialReference*/ return new String[] {"Reference"}; 432 case -1247940438: /*qualifier*/ return new String[] {"CodeableConcept"}; 433 default: return super.getTypesForProperty(hash, name); 434 } 435 436 } 437 438 @Override 439 public Base addChild(String name) throws FHIRException { 440 if (name.equals("structure")) { 441 this.structure = new CodeableConcept(); 442 return this.structure; 443 } 444 else if (name.equals("laterality")) { 445 this.laterality = new CodeableConcept(); 446 return this.laterality; 447 } 448 else if (name.equals("bodyLandmarkOrientation")) { 449 return addBodyLandmarkOrientation(); 450 } 451 else if (name.equals("spatialReference")) { 452 return addSpatialReference(); 453 } 454 else if (name.equals("qualifier")) { 455 return addQualifier(); 456 } 457 else 458 return super.addChild(name); 459 } 460 461 public BodyStructureIncludedStructureComponent copy() { 462 BodyStructureIncludedStructureComponent dst = new BodyStructureIncludedStructureComponent(); 463 copyValues(dst); 464 return dst; 465 } 466 467 public void copyValues(BodyStructureIncludedStructureComponent dst) { 468 super.copyValues(dst); 469 dst.structure = structure == null ? null : structure.copy(); 470 dst.laterality = laterality == null ? null : laterality.copy(); 471 if (bodyLandmarkOrientation != null) { 472 dst.bodyLandmarkOrientation = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationComponent>(); 473 for (BodyStructureIncludedStructureBodyLandmarkOrientationComponent i : bodyLandmarkOrientation) 474 dst.bodyLandmarkOrientation.add(i.copy()); 475 }; 476 if (spatialReference != null) { 477 dst.spatialReference = new ArrayList<Reference>(); 478 for (Reference i : spatialReference) 479 dst.spatialReference.add(i.copy()); 480 }; 481 if (qualifier != null) { 482 dst.qualifier = new ArrayList<CodeableConcept>(); 483 for (CodeableConcept i : qualifier) 484 dst.qualifier.add(i.copy()); 485 }; 486 } 487 488 @Override 489 public boolean equalsDeep(Base other_) { 490 if (!super.equalsDeep(other_)) 491 return false; 492 if (!(other_ instanceof BodyStructureIncludedStructureComponent)) 493 return false; 494 BodyStructureIncludedStructureComponent o = (BodyStructureIncludedStructureComponent) other_; 495 return compareDeep(structure, o.structure, true) && compareDeep(laterality, o.laterality, true) 496 && compareDeep(bodyLandmarkOrientation, o.bodyLandmarkOrientation, true) && compareDeep(spatialReference, o.spatialReference, true) 497 && compareDeep(qualifier, o.qualifier, true); 498 } 499 500 @Override 501 public boolean equalsShallow(Base other_) { 502 if (!super.equalsShallow(other_)) 503 return false; 504 if (!(other_ instanceof BodyStructureIncludedStructureComponent)) 505 return false; 506 BodyStructureIncludedStructureComponent o = (BodyStructureIncludedStructureComponent) other_; 507 return true; 508 } 509 510 public boolean isEmpty() { 511 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(structure, laterality, bodyLandmarkOrientation 512 , spatialReference, qualifier); 513 } 514 515 public String fhirType() { 516 return "BodyStructure.includedStructure"; 517 518 } 519 520 } 521 522 @Block() 523 public static class BodyStructureIncludedStructureBodyLandmarkOrientationComponent extends BackboneElement implements IBaseBackboneElement { 524 /** 525 * A description of a landmark on the body used as a reference to locate something else. 526 */ 527 @Child(name = "landmarkDescription", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 528 @Description(shortDefinition="Body ]andmark description", formalDefinition="A description of a landmark on the body used as a reference to locate something else." ) 529 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 530 protected List<CodeableConcept> landmarkDescription; 531 532 /** 533 * An description of the direction away from a landmark something is located based on a radial clock dial. 534 */ 535 @Child(name = "clockFacePosition", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 536 @Description(shortDefinition="Clockface orientation", formalDefinition="An description of the direction away from a landmark something is located based on a radial clock dial." ) 537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodystructure-bodylandmarkorientation-clockface-position") 538 protected List<CodeableConcept> clockFacePosition; 539 540 /** 541 * The distance in centimeters a certain observation is made from a body landmark. 542 */ 543 @Child(name = "distanceFromLandmark", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 544 @Description(shortDefinition="Landmark relative location", formalDefinition="The distance in centimeters a certain observation is made from a body landmark." ) 545 protected List<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent> distanceFromLandmark; 546 547 /** 548 * The surface area a body location is in relation to a landmark. 549 */ 550 @Child(name = "surfaceOrientation", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 551 @Description(shortDefinition="Relative landmark surface orientation", formalDefinition="The surface area a body location is in relation to a landmark." ) 552 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodystructure-relative-location") 553 protected List<CodeableConcept> surfaceOrientation; 554 555 private static final long serialVersionUID = -365770277L; 556 557 /** 558 * Constructor 559 */ 560 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent() { 561 super(); 562 } 563 564 /** 565 * @return {@link #landmarkDescription} (A description of a landmark on the body used as a reference to locate something else.) 566 */ 567 public List<CodeableConcept> getLandmarkDescription() { 568 if (this.landmarkDescription == null) 569 this.landmarkDescription = new ArrayList<CodeableConcept>(); 570 return this.landmarkDescription; 571 } 572 573 /** 574 * @return Returns a reference to <code>this</code> for easy method chaining 575 */ 576 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent setLandmarkDescription(List<CodeableConcept> theLandmarkDescription) { 577 this.landmarkDescription = theLandmarkDescription; 578 return this; 579 } 580 581 public boolean hasLandmarkDescription() { 582 if (this.landmarkDescription == null) 583 return false; 584 for (CodeableConcept item : this.landmarkDescription) 585 if (!item.isEmpty()) 586 return true; 587 return false; 588 } 589 590 public CodeableConcept addLandmarkDescription() { //3 591 CodeableConcept t = new CodeableConcept(); 592 if (this.landmarkDescription == null) 593 this.landmarkDescription = new ArrayList<CodeableConcept>(); 594 this.landmarkDescription.add(t); 595 return t; 596 } 597 598 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent addLandmarkDescription(CodeableConcept t) { //3 599 if (t == null) 600 return this; 601 if (this.landmarkDescription == null) 602 this.landmarkDescription = new ArrayList<CodeableConcept>(); 603 this.landmarkDescription.add(t); 604 return this; 605 } 606 607 /** 608 * @return The first repetition of repeating field {@link #landmarkDescription}, creating it if it does not already exist {3} 609 */ 610 public CodeableConcept getLandmarkDescriptionFirstRep() { 611 if (getLandmarkDescription().isEmpty()) { 612 addLandmarkDescription(); 613 } 614 return getLandmarkDescription().get(0); 615 } 616 617 /** 618 * @return {@link #clockFacePosition} (An description of the direction away from a landmark something is located based on a radial clock dial.) 619 */ 620 public List<CodeableConcept> getClockFacePosition() { 621 if (this.clockFacePosition == null) 622 this.clockFacePosition = new ArrayList<CodeableConcept>(); 623 return this.clockFacePosition; 624 } 625 626 /** 627 * @return Returns a reference to <code>this</code> for easy method chaining 628 */ 629 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent setClockFacePosition(List<CodeableConcept> theClockFacePosition) { 630 this.clockFacePosition = theClockFacePosition; 631 return this; 632 } 633 634 public boolean hasClockFacePosition() { 635 if (this.clockFacePosition == null) 636 return false; 637 for (CodeableConcept item : this.clockFacePosition) 638 if (!item.isEmpty()) 639 return true; 640 return false; 641 } 642 643 public CodeableConcept addClockFacePosition() { //3 644 CodeableConcept t = new CodeableConcept(); 645 if (this.clockFacePosition == null) 646 this.clockFacePosition = new ArrayList<CodeableConcept>(); 647 this.clockFacePosition.add(t); 648 return t; 649 } 650 651 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent addClockFacePosition(CodeableConcept t) { //3 652 if (t == null) 653 return this; 654 if (this.clockFacePosition == null) 655 this.clockFacePosition = new ArrayList<CodeableConcept>(); 656 this.clockFacePosition.add(t); 657 return this; 658 } 659 660 /** 661 * @return The first repetition of repeating field {@link #clockFacePosition}, creating it if it does not already exist {3} 662 */ 663 public CodeableConcept getClockFacePositionFirstRep() { 664 if (getClockFacePosition().isEmpty()) { 665 addClockFacePosition(); 666 } 667 return getClockFacePosition().get(0); 668 } 669 670 /** 671 * @return {@link #distanceFromLandmark} (The distance in centimeters a certain observation is made from a body landmark.) 672 */ 673 public List<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent> getDistanceFromLandmark() { 674 if (this.distanceFromLandmark == null) 675 this.distanceFromLandmark = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent>(); 676 return this.distanceFromLandmark; 677 } 678 679 /** 680 * @return Returns a reference to <code>this</code> for easy method chaining 681 */ 682 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent setDistanceFromLandmark(List<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent> theDistanceFromLandmark) { 683 this.distanceFromLandmark = theDistanceFromLandmark; 684 return this; 685 } 686 687 public boolean hasDistanceFromLandmark() { 688 if (this.distanceFromLandmark == null) 689 return false; 690 for (BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent item : this.distanceFromLandmark) 691 if (!item.isEmpty()) 692 return true; 693 return false; 694 } 695 696 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent addDistanceFromLandmark() { //3 697 BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent t = new BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent(); 698 if (this.distanceFromLandmark == null) 699 this.distanceFromLandmark = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent>(); 700 this.distanceFromLandmark.add(t); 701 return t; 702 } 703 704 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent addDistanceFromLandmark(BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent t) { //3 705 if (t == null) 706 return this; 707 if (this.distanceFromLandmark == null) 708 this.distanceFromLandmark = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent>(); 709 this.distanceFromLandmark.add(t); 710 return this; 711 } 712 713 /** 714 * @return The first repetition of repeating field {@link #distanceFromLandmark}, creating it if it does not already exist {3} 715 */ 716 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent getDistanceFromLandmarkFirstRep() { 717 if (getDistanceFromLandmark().isEmpty()) { 718 addDistanceFromLandmark(); 719 } 720 return getDistanceFromLandmark().get(0); 721 } 722 723 /** 724 * @return {@link #surfaceOrientation} (The surface area a body location is in relation to a landmark.) 725 */ 726 public List<CodeableConcept> getSurfaceOrientation() { 727 if (this.surfaceOrientation == null) 728 this.surfaceOrientation = new ArrayList<CodeableConcept>(); 729 return this.surfaceOrientation; 730 } 731 732 /** 733 * @return Returns a reference to <code>this</code> for easy method chaining 734 */ 735 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent setSurfaceOrientation(List<CodeableConcept> theSurfaceOrientation) { 736 this.surfaceOrientation = theSurfaceOrientation; 737 return this; 738 } 739 740 public boolean hasSurfaceOrientation() { 741 if (this.surfaceOrientation == null) 742 return false; 743 for (CodeableConcept item : this.surfaceOrientation) 744 if (!item.isEmpty()) 745 return true; 746 return false; 747 } 748 749 public CodeableConcept addSurfaceOrientation() { //3 750 CodeableConcept t = new CodeableConcept(); 751 if (this.surfaceOrientation == null) 752 this.surfaceOrientation = new ArrayList<CodeableConcept>(); 753 this.surfaceOrientation.add(t); 754 return t; 755 } 756 757 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent addSurfaceOrientation(CodeableConcept t) { //3 758 if (t == null) 759 return this; 760 if (this.surfaceOrientation == null) 761 this.surfaceOrientation = new ArrayList<CodeableConcept>(); 762 this.surfaceOrientation.add(t); 763 return this; 764 } 765 766 /** 767 * @return The first repetition of repeating field {@link #surfaceOrientation}, creating it if it does not already exist {3} 768 */ 769 public CodeableConcept getSurfaceOrientationFirstRep() { 770 if (getSurfaceOrientation().isEmpty()) { 771 addSurfaceOrientation(); 772 } 773 return getSurfaceOrientation().get(0); 774 } 775 776 protected void listChildren(List<Property> children) { 777 super.listChildren(children); 778 children.add(new Property("landmarkDescription", "CodeableConcept", "A description of a landmark on the body used as a reference to locate something else.", 0, java.lang.Integer.MAX_VALUE, landmarkDescription)); 779 children.add(new Property("clockFacePosition", "CodeableConcept", "An description of the direction away from a landmark something is located based on a radial clock dial.", 0, java.lang.Integer.MAX_VALUE, clockFacePosition)); 780 children.add(new Property("distanceFromLandmark", "", "The distance in centimeters a certain observation is made from a body landmark.", 0, java.lang.Integer.MAX_VALUE, distanceFromLandmark)); 781 children.add(new Property("surfaceOrientation", "CodeableConcept", "The surface area a body location is in relation to a landmark.", 0, java.lang.Integer.MAX_VALUE, surfaceOrientation)); 782 } 783 784 @Override 785 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 786 switch (_hash) { 787 case 1187892644: /*landmarkDescription*/ return new Property("landmarkDescription", "CodeableConcept", "A description of a landmark on the body used as a reference to locate something else.", 0, java.lang.Integer.MAX_VALUE, landmarkDescription); 788 case 133476820: /*clockFacePosition*/ return new Property("clockFacePosition", "CodeableConcept", "An description of the direction away from a landmark something is located based on a radial clock dial.", 0, java.lang.Integer.MAX_VALUE, clockFacePosition); 789 case 1350792599: /*distanceFromLandmark*/ return new Property("distanceFromLandmark", "", "The distance in centimeters a certain observation is made from a body landmark.", 0, java.lang.Integer.MAX_VALUE, distanceFromLandmark); 790 case 1408496355: /*surfaceOrientation*/ return new Property("surfaceOrientation", "CodeableConcept", "The surface area a body location is in relation to a landmark.", 0, java.lang.Integer.MAX_VALUE, surfaceOrientation); 791 default: return super.getNamedProperty(_hash, _name, _checkValid); 792 } 793 794 } 795 796 @Override 797 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 798 switch (hash) { 799 case 1187892644: /*landmarkDescription*/ return this.landmarkDescription == null ? new Base[0] : this.landmarkDescription.toArray(new Base[this.landmarkDescription.size()]); // CodeableConcept 800 case 133476820: /*clockFacePosition*/ return this.clockFacePosition == null ? new Base[0] : this.clockFacePosition.toArray(new Base[this.clockFacePosition.size()]); // CodeableConcept 801 case 1350792599: /*distanceFromLandmark*/ return this.distanceFromLandmark == null ? new Base[0] : this.distanceFromLandmark.toArray(new Base[this.distanceFromLandmark.size()]); // BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent 802 case 1408496355: /*surfaceOrientation*/ return this.surfaceOrientation == null ? new Base[0] : this.surfaceOrientation.toArray(new Base[this.surfaceOrientation.size()]); // CodeableConcept 803 default: return super.getProperty(hash, name, checkValid); 804 } 805 806 } 807 808 @Override 809 public Base setProperty(int hash, String name, Base value) throws FHIRException { 810 switch (hash) { 811 case 1187892644: // landmarkDescription 812 this.getLandmarkDescription().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 813 return value; 814 case 133476820: // clockFacePosition 815 this.getClockFacePosition().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 816 return value; 817 case 1350792599: // distanceFromLandmark 818 this.getDistanceFromLandmark().add((BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent) value); // BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent 819 return value; 820 case 1408496355: // surfaceOrientation 821 this.getSurfaceOrientation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 822 return value; 823 default: return super.setProperty(hash, name, value); 824 } 825 826 } 827 828 @Override 829 public Base setProperty(String name, Base value) throws FHIRException { 830 if (name.equals("landmarkDescription")) { 831 this.getLandmarkDescription().add(TypeConvertor.castToCodeableConcept(value)); 832 } else if (name.equals("clockFacePosition")) { 833 this.getClockFacePosition().add(TypeConvertor.castToCodeableConcept(value)); 834 } else if (name.equals("distanceFromLandmark")) { 835 this.getDistanceFromLandmark().add((BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent) value); 836 } else if (name.equals("surfaceOrientation")) { 837 this.getSurfaceOrientation().add(TypeConvertor.castToCodeableConcept(value)); 838 } else 839 return super.setProperty(name, value); 840 return value; 841 } 842 843 @Override 844 public void removeChild(String name, Base value) throws FHIRException { 845 if (name.equals("landmarkDescription")) { 846 this.getLandmarkDescription().remove(value); 847 } else if (name.equals("clockFacePosition")) { 848 this.getClockFacePosition().remove(value); 849 } else if (name.equals("distanceFromLandmark")) { 850 this.getDistanceFromLandmark().remove((BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent) value); 851 } else if (name.equals("surfaceOrientation")) { 852 this.getSurfaceOrientation().remove(value); 853 } else 854 super.removeChild(name, value); 855 856 } 857 858 @Override 859 public Base makeProperty(int hash, String name) throws FHIRException { 860 switch (hash) { 861 case 1187892644: return addLandmarkDescription(); 862 case 133476820: return addClockFacePosition(); 863 case 1350792599: return addDistanceFromLandmark(); 864 case 1408496355: return addSurfaceOrientation(); 865 default: return super.makeProperty(hash, name); 866 } 867 868 } 869 870 @Override 871 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 872 switch (hash) { 873 case 1187892644: /*landmarkDescription*/ return new String[] {"CodeableConcept"}; 874 case 133476820: /*clockFacePosition*/ return new String[] {"CodeableConcept"}; 875 case 1350792599: /*distanceFromLandmark*/ return new String[] {}; 876 case 1408496355: /*surfaceOrientation*/ return new String[] {"CodeableConcept"}; 877 default: return super.getTypesForProperty(hash, name); 878 } 879 880 } 881 882 @Override 883 public Base addChild(String name) throws FHIRException { 884 if (name.equals("landmarkDescription")) { 885 return addLandmarkDescription(); 886 } 887 else if (name.equals("clockFacePosition")) { 888 return addClockFacePosition(); 889 } 890 else if (name.equals("distanceFromLandmark")) { 891 return addDistanceFromLandmark(); 892 } 893 else if (name.equals("surfaceOrientation")) { 894 return addSurfaceOrientation(); 895 } 896 else 897 return super.addChild(name); 898 } 899 900 public BodyStructureIncludedStructureBodyLandmarkOrientationComponent copy() { 901 BodyStructureIncludedStructureBodyLandmarkOrientationComponent dst = new BodyStructureIncludedStructureBodyLandmarkOrientationComponent(); 902 copyValues(dst); 903 return dst; 904 } 905 906 public void copyValues(BodyStructureIncludedStructureBodyLandmarkOrientationComponent dst) { 907 super.copyValues(dst); 908 if (landmarkDescription != null) { 909 dst.landmarkDescription = new ArrayList<CodeableConcept>(); 910 for (CodeableConcept i : landmarkDescription) 911 dst.landmarkDescription.add(i.copy()); 912 }; 913 if (clockFacePosition != null) { 914 dst.clockFacePosition = new ArrayList<CodeableConcept>(); 915 for (CodeableConcept i : clockFacePosition) 916 dst.clockFacePosition.add(i.copy()); 917 }; 918 if (distanceFromLandmark != null) { 919 dst.distanceFromLandmark = new ArrayList<BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent>(); 920 for (BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent i : distanceFromLandmark) 921 dst.distanceFromLandmark.add(i.copy()); 922 }; 923 if (surfaceOrientation != null) { 924 dst.surfaceOrientation = new ArrayList<CodeableConcept>(); 925 for (CodeableConcept i : surfaceOrientation) 926 dst.surfaceOrientation.add(i.copy()); 927 }; 928 } 929 930 @Override 931 public boolean equalsDeep(Base other_) { 932 if (!super.equalsDeep(other_)) 933 return false; 934 if (!(other_ instanceof BodyStructureIncludedStructureBodyLandmarkOrientationComponent)) 935 return false; 936 BodyStructureIncludedStructureBodyLandmarkOrientationComponent o = (BodyStructureIncludedStructureBodyLandmarkOrientationComponent) other_; 937 return compareDeep(landmarkDescription, o.landmarkDescription, true) && compareDeep(clockFacePosition, o.clockFacePosition, true) 938 && compareDeep(distanceFromLandmark, o.distanceFromLandmark, true) && compareDeep(surfaceOrientation, o.surfaceOrientation, true) 939 ; 940 } 941 942 @Override 943 public boolean equalsShallow(Base other_) { 944 if (!super.equalsShallow(other_)) 945 return false; 946 if (!(other_ instanceof BodyStructureIncludedStructureBodyLandmarkOrientationComponent)) 947 return false; 948 BodyStructureIncludedStructureBodyLandmarkOrientationComponent o = (BodyStructureIncludedStructureBodyLandmarkOrientationComponent) other_; 949 return true; 950 } 951 952 public boolean isEmpty() { 953 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(landmarkDescription, clockFacePosition 954 , distanceFromLandmark, surfaceOrientation); 955 } 956 957 public String fhirType() { 958 return "BodyStructure.includedStructure.bodyLandmarkOrientation"; 959 960 } 961 962 } 963 964 @Block() 965 public static class BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent extends BackboneElement implements IBaseBackboneElement { 966 /** 967 * An instrument, tool, analyzer, etc. used in the measurement. 968 */ 969 @Child(name = "device", type = {CodeableReference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 970 @Description(shortDefinition="Measurement device", formalDefinition="An instrument, tool, analyzer, etc. used in the measurement." ) 971 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 972 protected List<CodeableReference> device; 973 974 /** 975 * The measured distance (e.g., in cm) from a body landmark. 976 */ 977 @Child(name = "value", type = {Quantity.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 978 @Description(shortDefinition="Measured distance from body landmark", formalDefinition="The measured distance (e.g., in cm) from a body landmark." ) 979 protected List<Quantity> value; 980 981 private static final long serialVersionUID = 1883586968L; 982 983 /** 984 * Constructor 985 */ 986 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent() { 987 super(); 988 } 989 990 /** 991 * @return {@link #device} (An instrument, tool, analyzer, etc. used in the measurement.) 992 */ 993 public List<CodeableReference> getDevice() { 994 if (this.device == null) 995 this.device = new ArrayList<CodeableReference>(); 996 return this.device; 997 } 998 999 /** 1000 * @return Returns a reference to <code>this</code> for easy method chaining 1001 */ 1002 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent setDevice(List<CodeableReference> theDevice) { 1003 this.device = theDevice; 1004 return this; 1005 } 1006 1007 public boolean hasDevice() { 1008 if (this.device == null) 1009 return false; 1010 for (CodeableReference item : this.device) 1011 if (!item.isEmpty()) 1012 return true; 1013 return false; 1014 } 1015 1016 public CodeableReference addDevice() { //3 1017 CodeableReference t = new CodeableReference(); 1018 if (this.device == null) 1019 this.device = new ArrayList<CodeableReference>(); 1020 this.device.add(t); 1021 return t; 1022 } 1023 1024 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent addDevice(CodeableReference t) { //3 1025 if (t == null) 1026 return this; 1027 if (this.device == null) 1028 this.device = new ArrayList<CodeableReference>(); 1029 this.device.add(t); 1030 return this; 1031 } 1032 1033 /** 1034 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 1035 */ 1036 public CodeableReference getDeviceFirstRep() { 1037 if (getDevice().isEmpty()) { 1038 addDevice(); 1039 } 1040 return getDevice().get(0); 1041 } 1042 1043 /** 1044 * @return {@link #value} (The measured distance (e.g., in cm) from a body landmark.) 1045 */ 1046 public List<Quantity> getValue() { 1047 if (this.value == null) 1048 this.value = new ArrayList<Quantity>(); 1049 return this.value; 1050 } 1051 1052 /** 1053 * @return Returns a reference to <code>this</code> for easy method chaining 1054 */ 1055 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent setValue(List<Quantity> theValue) { 1056 this.value = theValue; 1057 return this; 1058 } 1059 1060 public boolean hasValue() { 1061 if (this.value == null) 1062 return false; 1063 for (Quantity item : this.value) 1064 if (!item.isEmpty()) 1065 return true; 1066 return false; 1067 } 1068 1069 public Quantity addValue() { //3 1070 Quantity t = new Quantity(); 1071 if (this.value == null) 1072 this.value = new ArrayList<Quantity>(); 1073 this.value.add(t); 1074 return t; 1075 } 1076 1077 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent addValue(Quantity t) { //3 1078 if (t == null) 1079 return this; 1080 if (this.value == null) 1081 this.value = new ArrayList<Quantity>(); 1082 this.value.add(t); 1083 return this; 1084 } 1085 1086 /** 1087 * @return The first repetition of repeating field {@link #value}, creating it if it does not already exist {3} 1088 */ 1089 public Quantity getValueFirstRep() { 1090 if (getValue().isEmpty()) { 1091 addValue(); 1092 } 1093 return getValue().get(0); 1094 } 1095 1096 protected void listChildren(List<Property> children) { 1097 super.listChildren(children); 1098 children.add(new Property("device", "CodeableReference(Device)", "An instrument, tool, analyzer, etc. used in the measurement.", 0, java.lang.Integer.MAX_VALUE, device)); 1099 children.add(new Property("value", "Quantity", "The measured distance (e.g., in cm) from a body landmark.", 0, java.lang.Integer.MAX_VALUE, value)); 1100 } 1101 1102 @Override 1103 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1104 switch (_hash) { 1105 case -1335157162: /*device*/ return new Property("device", "CodeableReference(Device)", "An instrument, tool, analyzer, etc. used in the measurement.", 0, java.lang.Integer.MAX_VALUE, device); 1106 case 111972721: /*value*/ return new Property("value", "Quantity", "The measured distance (e.g., in cm) from a body landmark.", 0, java.lang.Integer.MAX_VALUE, value); 1107 default: return super.getNamedProperty(_hash, _name, _checkValid); 1108 } 1109 1110 } 1111 1112 @Override 1113 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1114 switch (hash) { 1115 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // CodeableReference 1116 case 111972721: /*value*/ return this.value == null ? new Base[0] : this.value.toArray(new Base[this.value.size()]); // Quantity 1117 default: return super.getProperty(hash, name, checkValid); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1124 switch (hash) { 1125 case -1335157162: // device 1126 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1127 return value; 1128 case 111972721: // value 1129 this.getValue().add(TypeConvertor.castToQuantity(value)); // Quantity 1130 return value; 1131 default: return super.setProperty(hash, name, value); 1132 } 1133 1134 } 1135 1136 @Override 1137 public Base setProperty(String name, Base value) throws FHIRException { 1138 if (name.equals("device")) { 1139 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); 1140 } else if (name.equals("value")) { 1141 this.getValue().add(TypeConvertor.castToQuantity(value)); 1142 } else 1143 return super.setProperty(name, value); 1144 return value; 1145 } 1146 1147 @Override 1148 public void removeChild(String name, Base value) throws FHIRException { 1149 if (name.equals("device")) { 1150 this.getDevice().remove(value); 1151 } else if (name.equals("value")) { 1152 this.getValue().remove(value); 1153 } else 1154 super.removeChild(name, value); 1155 1156 } 1157 1158 @Override 1159 public Base makeProperty(int hash, String name) throws FHIRException { 1160 switch (hash) { 1161 case -1335157162: return addDevice(); 1162 case 111972721: return addValue(); 1163 default: return super.makeProperty(hash, name); 1164 } 1165 1166 } 1167 1168 @Override 1169 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1170 switch (hash) { 1171 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 1172 case 111972721: /*value*/ return new String[] {"Quantity"}; 1173 default: return super.getTypesForProperty(hash, name); 1174 } 1175 1176 } 1177 1178 @Override 1179 public Base addChild(String name) throws FHIRException { 1180 if (name.equals("device")) { 1181 return addDevice(); 1182 } 1183 else if (name.equals("value")) { 1184 return addValue(); 1185 } 1186 else 1187 return super.addChild(name); 1188 } 1189 1190 public BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent copy() { 1191 BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent dst = new BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent(); 1192 copyValues(dst); 1193 return dst; 1194 } 1195 1196 public void copyValues(BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent dst) { 1197 super.copyValues(dst); 1198 if (device != null) { 1199 dst.device = new ArrayList<CodeableReference>(); 1200 for (CodeableReference i : device) 1201 dst.device.add(i.copy()); 1202 }; 1203 if (value != null) { 1204 dst.value = new ArrayList<Quantity>(); 1205 for (Quantity i : value) 1206 dst.value.add(i.copy()); 1207 }; 1208 } 1209 1210 @Override 1211 public boolean equalsDeep(Base other_) { 1212 if (!super.equalsDeep(other_)) 1213 return false; 1214 if (!(other_ instanceof BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent)) 1215 return false; 1216 BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent o = (BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent) other_; 1217 return compareDeep(device, o.device, true) && compareDeep(value, o.value, true); 1218 } 1219 1220 @Override 1221 public boolean equalsShallow(Base other_) { 1222 if (!super.equalsShallow(other_)) 1223 return false; 1224 if (!(other_ instanceof BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent)) 1225 return false; 1226 BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent o = (BodyStructureIncludedStructureBodyLandmarkOrientationDistanceFromLandmarkComponent) other_; 1227 return true; 1228 } 1229 1230 public boolean isEmpty() { 1231 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(device, value); 1232 } 1233 1234 public String fhirType() { 1235 return "BodyStructure.includedStructure.bodyLandmarkOrientation.distanceFromLandmark"; 1236 1237 } 1238 1239 } 1240 1241 /** 1242 * Identifier for this instance of the anatomical structure. 1243 */ 1244 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1245 @Description(shortDefinition="Bodystructure identifier", formalDefinition="Identifier for this instance of the anatomical structure." ) 1246 protected List<Identifier> identifier; 1247 1248 /** 1249 * Whether this body site is in active use. 1250 */ 1251 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1252 @Description(shortDefinition="Whether this record is in active use", formalDefinition="Whether this body site is in active use." ) 1253 protected BooleanType active; 1254 1255 /** 1256 * The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies. 1257 */ 1258 @Child(name = "morphology", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1259 @Description(shortDefinition="Kind of Structure", formalDefinition="The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies." ) 1260 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodystructure-code") 1261 protected CodeableConcept morphology; 1262 1263 /** 1264 * The anatomical location(s) or region(s) of the specimen, lesion, or body structure. 1265 */ 1266 @Child(name = "includedStructure", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1267 @Description(shortDefinition="Included anatomic location(s)", formalDefinition="The anatomical location(s) or region(s) of the specimen, lesion, or body structure." ) 1268 protected List<BodyStructureIncludedStructureComponent> includedStructure; 1269 1270 /** 1271 * The anatomical location(s) or region(s) not occupied or represented by the specimen, lesion, or body structure. 1272 */ 1273 @Child(name = "excludedStructure", type = {BodyStructureIncludedStructureComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1274 @Description(shortDefinition="Excluded anatomic locations(s)", formalDefinition="The anatomical location(s) or region(s) not occupied or represented by the specimen, lesion, or body structure." ) 1275 protected List<BodyStructureIncludedStructureComponent> excludedStructure; 1276 1277 /** 1278 * A summary, characterization or explanation of the body structure. 1279 */ 1280 @Child(name = "description", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1281 @Description(shortDefinition="Text description", formalDefinition="A summary, characterization or explanation of the body structure." ) 1282 protected MarkdownType description; 1283 1284 /** 1285 * Image or images used to identify a location. 1286 */ 1287 @Child(name = "image", type = {Attachment.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1288 @Description(shortDefinition="Attached images", formalDefinition="Image or images used to identify a location." ) 1289 protected List<Attachment> image; 1290 1291 /** 1292 * The person to which the body site belongs. 1293 */ 1294 @Child(name = "patient", type = {Patient.class}, order=7, min=1, max=1, modifier=false, summary=true) 1295 @Description(shortDefinition="Who this is about", formalDefinition="The person to which the body site belongs." ) 1296 protected Reference patient; 1297 1298 private static final long serialVersionUID = -1014863022L; 1299 1300 /** 1301 * Constructor 1302 */ 1303 public BodyStructure() { 1304 super(); 1305 } 1306 1307 /** 1308 * Constructor 1309 */ 1310 public BodyStructure(BodyStructureIncludedStructureComponent includedStructure, Reference patient) { 1311 super(); 1312 this.addIncludedStructure(includedStructure); 1313 this.setPatient(patient); 1314 } 1315 1316 /** 1317 * @return {@link #identifier} (Identifier for this instance of the anatomical structure.) 1318 */ 1319 public List<Identifier> getIdentifier() { 1320 if (this.identifier == null) 1321 this.identifier = new ArrayList<Identifier>(); 1322 return this.identifier; 1323 } 1324 1325 /** 1326 * @return Returns a reference to <code>this</code> for easy method chaining 1327 */ 1328 public BodyStructure setIdentifier(List<Identifier> theIdentifier) { 1329 this.identifier = theIdentifier; 1330 return this; 1331 } 1332 1333 public boolean hasIdentifier() { 1334 if (this.identifier == null) 1335 return false; 1336 for (Identifier item : this.identifier) 1337 if (!item.isEmpty()) 1338 return true; 1339 return false; 1340 } 1341 1342 public Identifier addIdentifier() { //3 1343 Identifier t = new Identifier(); 1344 if (this.identifier == null) 1345 this.identifier = new ArrayList<Identifier>(); 1346 this.identifier.add(t); 1347 return t; 1348 } 1349 1350 public BodyStructure addIdentifier(Identifier t) { //3 1351 if (t == null) 1352 return this; 1353 if (this.identifier == null) 1354 this.identifier = new ArrayList<Identifier>(); 1355 this.identifier.add(t); 1356 return this; 1357 } 1358 1359 /** 1360 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1361 */ 1362 public Identifier getIdentifierFirstRep() { 1363 if (getIdentifier().isEmpty()) { 1364 addIdentifier(); 1365 } 1366 return getIdentifier().get(0); 1367 } 1368 1369 /** 1370 * @return {@link #active} (Whether this body site is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1371 */ 1372 public BooleanType getActiveElement() { 1373 if (this.active == null) 1374 if (Configuration.errorOnAutoCreate()) 1375 throw new Error("Attempt to auto-create BodyStructure.active"); 1376 else if (Configuration.doAutoCreate()) 1377 this.active = new BooleanType(); // bb 1378 return this.active; 1379 } 1380 1381 public boolean hasActiveElement() { 1382 return this.active != null && !this.active.isEmpty(); 1383 } 1384 1385 public boolean hasActive() { 1386 return this.active != null && !this.active.isEmpty(); 1387 } 1388 1389 /** 1390 * @param value {@link #active} (Whether this body site is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1391 */ 1392 public BodyStructure setActiveElement(BooleanType value) { 1393 this.active = value; 1394 return this; 1395 } 1396 1397 /** 1398 * @return Whether this body site is in active use. 1399 */ 1400 public boolean getActive() { 1401 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1402 } 1403 1404 /** 1405 * @param value Whether this body site is in active use. 1406 */ 1407 public BodyStructure setActive(boolean value) { 1408 if (this.active == null) 1409 this.active = new BooleanType(); 1410 this.active.setValue(value); 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #morphology} (The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.) 1416 */ 1417 public CodeableConcept getMorphology() { 1418 if (this.morphology == null) 1419 if (Configuration.errorOnAutoCreate()) 1420 throw new Error("Attempt to auto-create BodyStructure.morphology"); 1421 else if (Configuration.doAutoCreate()) 1422 this.morphology = new CodeableConcept(); // cc 1423 return this.morphology; 1424 } 1425 1426 public boolean hasMorphology() { 1427 return this.morphology != null && !this.morphology.isEmpty(); 1428 } 1429 1430 /** 1431 * @param value {@link #morphology} (The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.) 1432 */ 1433 public BodyStructure setMorphology(CodeableConcept value) { 1434 this.morphology = value; 1435 return this; 1436 } 1437 1438 /** 1439 * @return {@link #includedStructure} (The anatomical location(s) or region(s) of the specimen, lesion, or body structure.) 1440 */ 1441 public List<BodyStructureIncludedStructureComponent> getIncludedStructure() { 1442 if (this.includedStructure == null) 1443 this.includedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1444 return this.includedStructure; 1445 } 1446 1447 /** 1448 * @return Returns a reference to <code>this</code> for easy method chaining 1449 */ 1450 public BodyStructure setIncludedStructure(List<BodyStructureIncludedStructureComponent> theIncludedStructure) { 1451 this.includedStructure = theIncludedStructure; 1452 return this; 1453 } 1454 1455 public boolean hasIncludedStructure() { 1456 if (this.includedStructure == null) 1457 return false; 1458 for (BodyStructureIncludedStructureComponent item : this.includedStructure) 1459 if (!item.isEmpty()) 1460 return true; 1461 return false; 1462 } 1463 1464 public BodyStructureIncludedStructureComponent addIncludedStructure() { //3 1465 BodyStructureIncludedStructureComponent t = new BodyStructureIncludedStructureComponent(); 1466 if (this.includedStructure == null) 1467 this.includedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1468 this.includedStructure.add(t); 1469 return t; 1470 } 1471 1472 public BodyStructure addIncludedStructure(BodyStructureIncludedStructureComponent t) { //3 1473 if (t == null) 1474 return this; 1475 if (this.includedStructure == null) 1476 this.includedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1477 this.includedStructure.add(t); 1478 return this; 1479 } 1480 1481 /** 1482 * @return The first repetition of repeating field {@link #includedStructure}, creating it if it does not already exist {3} 1483 */ 1484 public BodyStructureIncludedStructureComponent getIncludedStructureFirstRep() { 1485 if (getIncludedStructure().isEmpty()) { 1486 addIncludedStructure(); 1487 } 1488 return getIncludedStructure().get(0); 1489 } 1490 1491 /** 1492 * @return {@link #excludedStructure} (The anatomical location(s) or region(s) not occupied or represented by the specimen, lesion, or body structure.) 1493 */ 1494 public List<BodyStructureIncludedStructureComponent> getExcludedStructure() { 1495 if (this.excludedStructure == null) 1496 this.excludedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1497 return this.excludedStructure; 1498 } 1499 1500 /** 1501 * @return Returns a reference to <code>this</code> for easy method chaining 1502 */ 1503 public BodyStructure setExcludedStructure(List<BodyStructureIncludedStructureComponent> theExcludedStructure) { 1504 this.excludedStructure = theExcludedStructure; 1505 return this; 1506 } 1507 1508 public boolean hasExcludedStructure() { 1509 if (this.excludedStructure == null) 1510 return false; 1511 for (BodyStructureIncludedStructureComponent item : this.excludedStructure) 1512 if (!item.isEmpty()) 1513 return true; 1514 return false; 1515 } 1516 1517 public BodyStructureIncludedStructureComponent addExcludedStructure() { //3 1518 BodyStructureIncludedStructureComponent t = new BodyStructureIncludedStructureComponent(); 1519 if (this.excludedStructure == null) 1520 this.excludedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1521 this.excludedStructure.add(t); 1522 return t; 1523 } 1524 1525 public BodyStructure addExcludedStructure(BodyStructureIncludedStructureComponent t) { //3 1526 if (t == null) 1527 return this; 1528 if (this.excludedStructure == null) 1529 this.excludedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1530 this.excludedStructure.add(t); 1531 return this; 1532 } 1533 1534 /** 1535 * @return The first repetition of repeating field {@link #excludedStructure}, creating it if it does not already exist {3} 1536 */ 1537 public BodyStructureIncludedStructureComponent getExcludedStructureFirstRep() { 1538 if (getExcludedStructure().isEmpty()) { 1539 addExcludedStructure(); 1540 } 1541 return getExcludedStructure().get(0); 1542 } 1543 1544 /** 1545 * @return {@link #description} (A summary, characterization or explanation of the body structure.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1546 */ 1547 public MarkdownType getDescriptionElement() { 1548 if (this.description == null) 1549 if (Configuration.errorOnAutoCreate()) 1550 throw new Error("Attempt to auto-create BodyStructure.description"); 1551 else if (Configuration.doAutoCreate()) 1552 this.description = new MarkdownType(); // bb 1553 return this.description; 1554 } 1555 1556 public boolean hasDescriptionElement() { 1557 return this.description != null && !this.description.isEmpty(); 1558 } 1559 1560 public boolean hasDescription() { 1561 return this.description != null && !this.description.isEmpty(); 1562 } 1563 1564 /** 1565 * @param value {@link #description} (A summary, characterization or explanation of the body structure.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1566 */ 1567 public BodyStructure setDescriptionElement(MarkdownType value) { 1568 this.description = value; 1569 return this; 1570 } 1571 1572 /** 1573 * @return A summary, characterization or explanation of the body structure. 1574 */ 1575 public String getDescription() { 1576 return this.description == null ? null : this.description.getValue(); 1577 } 1578 1579 /** 1580 * @param value A summary, characterization or explanation of the body structure. 1581 */ 1582 public BodyStructure setDescription(String value) { 1583 if (Utilities.noString(value)) 1584 this.description = null; 1585 else { 1586 if (this.description == null) 1587 this.description = new MarkdownType(); 1588 this.description.setValue(value); 1589 } 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #image} (Image or images used to identify a location.) 1595 */ 1596 public List<Attachment> getImage() { 1597 if (this.image == null) 1598 this.image = new ArrayList<Attachment>(); 1599 return this.image; 1600 } 1601 1602 /** 1603 * @return Returns a reference to <code>this</code> for easy method chaining 1604 */ 1605 public BodyStructure setImage(List<Attachment> theImage) { 1606 this.image = theImage; 1607 return this; 1608 } 1609 1610 public boolean hasImage() { 1611 if (this.image == null) 1612 return false; 1613 for (Attachment item : this.image) 1614 if (!item.isEmpty()) 1615 return true; 1616 return false; 1617 } 1618 1619 public Attachment addImage() { //3 1620 Attachment t = new Attachment(); 1621 if (this.image == null) 1622 this.image = new ArrayList<Attachment>(); 1623 this.image.add(t); 1624 return t; 1625 } 1626 1627 public BodyStructure addImage(Attachment t) { //3 1628 if (t == null) 1629 return this; 1630 if (this.image == null) 1631 this.image = new ArrayList<Attachment>(); 1632 this.image.add(t); 1633 return this; 1634 } 1635 1636 /** 1637 * @return The first repetition of repeating field {@link #image}, creating it if it does not already exist {3} 1638 */ 1639 public Attachment getImageFirstRep() { 1640 if (getImage().isEmpty()) { 1641 addImage(); 1642 } 1643 return getImage().get(0); 1644 } 1645 1646 /** 1647 * @return {@link #patient} (The person to which the body site belongs.) 1648 */ 1649 public Reference getPatient() { 1650 if (this.patient == null) 1651 if (Configuration.errorOnAutoCreate()) 1652 throw new Error("Attempt to auto-create BodyStructure.patient"); 1653 else if (Configuration.doAutoCreate()) 1654 this.patient = new Reference(); // cc 1655 return this.patient; 1656 } 1657 1658 public boolean hasPatient() { 1659 return this.patient != null && !this.patient.isEmpty(); 1660 } 1661 1662 /** 1663 * @param value {@link #patient} (The person to which the body site belongs.) 1664 */ 1665 public BodyStructure setPatient(Reference value) { 1666 this.patient = value; 1667 return this; 1668 } 1669 1670 protected void listChildren(List<Property> children) { 1671 super.listChildren(children); 1672 children.add(new Property("identifier", "Identifier", "Identifier for this instance of the anatomical structure.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1673 children.add(new Property("active", "boolean", "Whether this body site is in active use.", 0, 1, active)); 1674 children.add(new Property("morphology", "CodeableConcept", "The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.", 0, 1, morphology)); 1675 children.add(new Property("includedStructure", "", "The anatomical location(s) or region(s) of the specimen, lesion, or body structure.", 0, java.lang.Integer.MAX_VALUE, includedStructure)); 1676 children.add(new Property("excludedStructure", "@BodyStructure.includedStructure", "The anatomical location(s) or region(s) not occupied or represented by the specimen, lesion, or body structure.", 0, java.lang.Integer.MAX_VALUE, excludedStructure)); 1677 children.add(new Property("description", "markdown", "A summary, characterization or explanation of the body structure.", 0, 1, description)); 1678 children.add(new Property("image", "Attachment", "Image or images used to identify a location.", 0, java.lang.Integer.MAX_VALUE, image)); 1679 children.add(new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 0, 1, patient)); 1680 } 1681 1682 @Override 1683 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1684 switch (_hash) { 1685 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for this instance of the anatomical structure.", 0, java.lang.Integer.MAX_VALUE, identifier); 1686 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this body site is in active use.", 0, 1, active); 1687 case 1807231644: /*morphology*/ return new Property("morphology", "CodeableConcept", "The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.", 0, 1, morphology); 1688 case -1174069225: /*includedStructure*/ return new Property("includedStructure", "", "The anatomical location(s) or region(s) of the specimen, lesion, or body structure.", 0, java.lang.Integer.MAX_VALUE, includedStructure); 1689 case 1192252105: /*excludedStructure*/ return new Property("excludedStructure", "@BodyStructure.includedStructure", "The anatomical location(s) or region(s) not occupied or represented by the specimen, lesion, or body structure.", 0, java.lang.Integer.MAX_VALUE, excludedStructure); 1690 case -1724546052: /*description*/ return new Property("description", "markdown", "A summary, characterization or explanation of the body structure.", 0, 1, description); 1691 case 100313435: /*image*/ return new Property("image", "Attachment", "Image or images used to identify a location.", 0, java.lang.Integer.MAX_VALUE, image); 1692 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 0, 1, patient); 1693 default: return super.getNamedProperty(_hash, _name, _checkValid); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1700 switch (hash) { 1701 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1702 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1703 case 1807231644: /*morphology*/ return this.morphology == null ? new Base[0] : new Base[] {this.morphology}; // CodeableConcept 1704 case -1174069225: /*includedStructure*/ return this.includedStructure == null ? new Base[0] : this.includedStructure.toArray(new Base[this.includedStructure.size()]); // BodyStructureIncludedStructureComponent 1705 case 1192252105: /*excludedStructure*/ return this.excludedStructure == null ? new Base[0] : this.excludedStructure.toArray(new Base[this.excludedStructure.size()]); // BodyStructureIncludedStructureComponent 1706 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1707 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // Attachment 1708 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1709 default: return super.getProperty(hash, name, checkValid); 1710 } 1711 1712 } 1713 1714 @Override 1715 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1716 switch (hash) { 1717 case -1618432855: // identifier 1718 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1719 return value; 1720 case -1422950650: // active 1721 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1722 return value; 1723 case 1807231644: // morphology 1724 this.morphology = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1725 return value; 1726 case -1174069225: // includedStructure 1727 this.getIncludedStructure().add((BodyStructureIncludedStructureComponent) value); // BodyStructureIncludedStructureComponent 1728 return value; 1729 case 1192252105: // excludedStructure 1730 this.getExcludedStructure().add((BodyStructureIncludedStructureComponent) value); // BodyStructureIncludedStructureComponent 1731 return value; 1732 case -1724546052: // description 1733 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1734 return value; 1735 case 100313435: // image 1736 this.getImage().add(TypeConvertor.castToAttachment(value)); // Attachment 1737 return value; 1738 case -791418107: // patient 1739 this.patient = TypeConvertor.castToReference(value); // Reference 1740 return value; 1741 default: return super.setProperty(hash, name, value); 1742 } 1743 1744 } 1745 1746 @Override 1747 public Base setProperty(String name, Base value) throws FHIRException { 1748 if (name.equals("identifier")) { 1749 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1750 } else if (name.equals("active")) { 1751 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1752 } else if (name.equals("morphology")) { 1753 this.morphology = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1754 } else if (name.equals("includedStructure")) { 1755 this.getIncludedStructure().add((BodyStructureIncludedStructureComponent) value); 1756 } else if (name.equals("excludedStructure")) { 1757 this.getExcludedStructure().add((BodyStructureIncludedStructureComponent) value); 1758 } else if (name.equals("description")) { 1759 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1760 } else if (name.equals("image")) { 1761 this.getImage().add(TypeConvertor.castToAttachment(value)); 1762 } else if (name.equals("patient")) { 1763 this.patient = TypeConvertor.castToReference(value); // Reference 1764 } else 1765 return super.setProperty(name, value); 1766 return value; 1767 } 1768 1769 @Override 1770 public void removeChild(String name, Base value) throws FHIRException { 1771 if (name.equals("identifier")) { 1772 this.getIdentifier().remove(value); 1773 } else if (name.equals("active")) { 1774 this.active = null; 1775 } else if (name.equals("morphology")) { 1776 this.morphology = null; 1777 } else if (name.equals("includedStructure")) { 1778 this.getIncludedStructure().remove((BodyStructureIncludedStructureComponent) value); 1779 } else if (name.equals("excludedStructure")) { 1780 this.getExcludedStructure().remove((BodyStructureIncludedStructureComponent) value); 1781 } else if (name.equals("description")) { 1782 this.description = null; 1783 } else if (name.equals("image")) { 1784 this.getImage().remove(value); 1785 } else if (name.equals("patient")) { 1786 this.patient = null; 1787 } else 1788 super.removeChild(name, value); 1789 1790 } 1791 1792 @Override 1793 public Base makeProperty(int hash, String name) throws FHIRException { 1794 switch (hash) { 1795 case -1618432855: return addIdentifier(); 1796 case -1422950650: return getActiveElement(); 1797 case 1807231644: return getMorphology(); 1798 case -1174069225: return addIncludedStructure(); 1799 case 1192252105: return addExcludedStructure(); 1800 case -1724546052: return getDescriptionElement(); 1801 case 100313435: return addImage(); 1802 case -791418107: return getPatient(); 1803 default: return super.makeProperty(hash, name); 1804 } 1805 1806 } 1807 1808 @Override 1809 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1810 switch (hash) { 1811 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1812 case -1422950650: /*active*/ return new String[] {"boolean"}; 1813 case 1807231644: /*morphology*/ return new String[] {"CodeableConcept"}; 1814 case -1174069225: /*includedStructure*/ return new String[] {}; 1815 case 1192252105: /*excludedStructure*/ return new String[] {"@BodyStructure.includedStructure"}; 1816 case -1724546052: /*description*/ return new String[] {"markdown"}; 1817 case 100313435: /*image*/ return new String[] {"Attachment"}; 1818 case -791418107: /*patient*/ return new String[] {"Reference"}; 1819 default: return super.getTypesForProperty(hash, name); 1820 } 1821 1822 } 1823 1824 @Override 1825 public Base addChild(String name) throws FHIRException { 1826 if (name.equals("identifier")) { 1827 return addIdentifier(); 1828 } 1829 else if (name.equals("active")) { 1830 throw new FHIRException("Cannot call addChild on a singleton property BodyStructure.active"); 1831 } 1832 else if (name.equals("morphology")) { 1833 this.morphology = new CodeableConcept(); 1834 return this.morphology; 1835 } 1836 else if (name.equals("includedStructure")) { 1837 return addIncludedStructure(); 1838 } 1839 else if (name.equals("excludedStructure")) { 1840 return addExcludedStructure(); 1841 } 1842 else if (name.equals("description")) { 1843 throw new FHIRException("Cannot call addChild on a singleton property BodyStructure.description"); 1844 } 1845 else if (name.equals("image")) { 1846 return addImage(); 1847 } 1848 else if (name.equals("patient")) { 1849 this.patient = new Reference(); 1850 return this.patient; 1851 } 1852 else 1853 return super.addChild(name); 1854 } 1855 1856 public String fhirType() { 1857 return "BodyStructure"; 1858 1859 } 1860 1861 public BodyStructure copy() { 1862 BodyStructure dst = new BodyStructure(); 1863 copyValues(dst); 1864 return dst; 1865 } 1866 1867 public void copyValues(BodyStructure dst) { 1868 super.copyValues(dst); 1869 if (identifier != null) { 1870 dst.identifier = new ArrayList<Identifier>(); 1871 for (Identifier i : identifier) 1872 dst.identifier.add(i.copy()); 1873 }; 1874 dst.active = active == null ? null : active.copy(); 1875 dst.morphology = morphology == null ? null : morphology.copy(); 1876 if (includedStructure != null) { 1877 dst.includedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1878 for (BodyStructureIncludedStructureComponent i : includedStructure) 1879 dst.includedStructure.add(i.copy()); 1880 }; 1881 if (excludedStructure != null) { 1882 dst.excludedStructure = new ArrayList<BodyStructureIncludedStructureComponent>(); 1883 for (BodyStructureIncludedStructureComponent i : excludedStructure) 1884 dst.excludedStructure.add(i.copy()); 1885 }; 1886 dst.description = description == null ? null : description.copy(); 1887 if (image != null) { 1888 dst.image = new ArrayList<Attachment>(); 1889 for (Attachment i : image) 1890 dst.image.add(i.copy()); 1891 }; 1892 dst.patient = patient == null ? null : patient.copy(); 1893 } 1894 1895 protected BodyStructure typedCopy() { 1896 return copy(); 1897 } 1898 1899 @Override 1900 public boolean equalsDeep(Base other_) { 1901 if (!super.equalsDeep(other_)) 1902 return false; 1903 if (!(other_ instanceof BodyStructure)) 1904 return false; 1905 BodyStructure o = (BodyStructure) other_; 1906 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(morphology, o.morphology, true) 1907 && compareDeep(includedStructure, o.includedStructure, true) && compareDeep(excludedStructure, o.excludedStructure, true) 1908 && compareDeep(description, o.description, true) && compareDeep(image, o.image, true) && compareDeep(patient, o.patient, true) 1909 ; 1910 } 1911 1912 @Override 1913 public boolean equalsShallow(Base other_) { 1914 if (!super.equalsShallow(other_)) 1915 return false; 1916 if (!(other_ instanceof BodyStructure)) 1917 return false; 1918 BodyStructure o = (BodyStructure) other_; 1919 return compareValues(active, o.active, true) && compareValues(description, o.description, true); 1920 } 1921 1922 public boolean isEmpty() { 1923 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, morphology 1924 , includedStructure, excludedStructure, description, image, patient); 1925 } 1926 1927 @Override 1928 public ResourceType getResourceType() { 1929 return ResourceType.BodyStructure; 1930 } 1931 1932 /** 1933 * Search parameter: <b>excluded_structure</b> 1934 * <p> 1935 * Description: <b>Body site excludedStructure structure</b><br> 1936 * Type: <b>token</b><br> 1937 * Path: <b>BodyStructure.excludedStructure.structure</b><br> 1938 * </p> 1939 */ 1940 @SearchParamDefinition(name="excluded_structure", path="BodyStructure.excludedStructure.structure", description="Body site excludedStructure structure", type="token" ) 1941 public static final String SP_EXCLUDEDSTRUCTURE = "excluded_structure"; 1942 /** 1943 * <b>Fluent Client</b> search parameter constant for <b>excluded_structure</b> 1944 * <p> 1945 * Description: <b>Body site excludedStructure structure</b><br> 1946 * Type: <b>token</b><br> 1947 * Path: <b>BodyStructure.excludedStructure.structure</b><br> 1948 * </p> 1949 */ 1950 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXCLUDEDSTRUCTURE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXCLUDEDSTRUCTURE); 1951 1952 /** 1953 * Search parameter: <b>included_structure</b> 1954 * <p> 1955 * Description: <b>Body site includedStructure structure</b><br> 1956 * Type: <b>token</b><br> 1957 * Path: <b>BodyStructure.includedStructure.structure</b><br> 1958 * </p> 1959 */ 1960 @SearchParamDefinition(name="included_structure", path="BodyStructure.includedStructure.structure", description="Body site includedStructure structure", type="token" ) 1961 public static final String SP_INCLUDEDSTRUCTURE = "included_structure"; 1962 /** 1963 * <b>Fluent Client</b> search parameter constant for <b>included_structure</b> 1964 * <p> 1965 * Description: <b>Body site includedStructure structure</b><br> 1966 * Type: <b>token</b><br> 1967 * Path: <b>BodyStructure.includedStructure.structure</b><br> 1968 * </p> 1969 */ 1970 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INCLUDEDSTRUCTURE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INCLUDEDSTRUCTURE); 1971 1972 /** 1973 * Search parameter: <b>morphology</b> 1974 * <p> 1975 * Description: <b>Kind of Structure</b><br> 1976 * Type: <b>token</b><br> 1977 * Path: <b>BodyStructure.morphology</b><br> 1978 * </p> 1979 */ 1980 @SearchParamDefinition(name="morphology", path="BodyStructure.morphology", description="Kind of Structure", type="token" ) 1981 public static final String SP_MORPHOLOGY = "morphology"; 1982 /** 1983 * <b>Fluent Client</b> search parameter constant for <b>morphology</b> 1984 * <p> 1985 * Description: <b>Kind of Structure</b><br> 1986 * Type: <b>token</b><br> 1987 * Path: <b>BodyStructure.morphology</b><br> 1988 * </p> 1989 */ 1990 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MORPHOLOGY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MORPHOLOGY); 1991 1992 /** 1993 * Search parameter: <b>identifier</b> 1994 * <p> 1995 * Description: <b>Multiple Resources: 1996 1997* [Account](account.html): Account number 1998* [AdverseEvent](adverseevent.html): Business identifier for the event 1999* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2000* [Appointment](appointment.html): An Identifier of the Appointment 2001* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2002* [Basic](basic.html): Business identifier 2003* [BodyStructure](bodystructure.html): Bodystructure identifier 2004* [CarePlan](careplan.html): External Ids for this plan 2005* [CareTeam](careteam.html): External Ids for this team 2006* [ChargeItem](chargeitem.html): Business Identifier for item 2007* [Claim](claim.html): The primary identifier of the financial resource 2008* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2009* [ClinicalImpression](clinicalimpression.html): Business identifier 2010* [Communication](communication.html): Unique identifier 2011* [CommunicationRequest](communicationrequest.html): Unique identifier 2012* [Composition](composition.html): Version-independent identifier for the Composition 2013* [Condition](condition.html): A unique identifier of the condition record 2014* [Consent](consent.html): Identifier for this record (external references) 2015* [Contract](contract.html): The identity of the contract 2016* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2017* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2018* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2019* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2020* [DeviceRequest](devicerequest.html): Business identifier for request/order 2021* [DeviceUsage](deviceusage.html): Search by identifier 2022* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2023* [DocumentReference](documentreference.html): Identifier of the attachment binary 2024* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2025* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2026* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2027* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2028* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2029* [Flag](flag.html): Business identifier 2030* [Goal](goal.html): External Ids for this goal 2031* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2032* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2033* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2034* [Immunization](immunization.html): Business identifier 2035* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2036* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2037* [Invoice](invoice.html): Business Identifier for item 2038* [List](list.html): Business identifier 2039* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2040* [Medication](medication.html): Returns medications with this external identifier 2041* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2042* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2043* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2044* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2045* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2046* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2047* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2048* [Observation](observation.html): The unique id for a particular observation 2049* [Person](person.html): A person Identifier 2050* [Procedure](procedure.html): A unique identifier for a procedure 2051* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2052* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2053* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2054* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2055* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2056* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2057* [Specimen](specimen.html): The unique identifier associated with the specimen 2058* [SupplyDelivery](supplydelivery.html): External identifier 2059* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2060* [Task](task.html): Search for a task instance by its business identifier 2061* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2062</b><br> 2063 * Type: <b>token</b><br> 2064 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2065 * </p> 2066 */ 2067 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2068 public static final String SP_IDENTIFIER = "identifier"; 2069 /** 2070 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2071 * <p> 2072 * Description: <b>Multiple Resources: 2073 2074* [Account](account.html): Account number 2075* [AdverseEvent](adverseevent.html): Business identifier for the event 2076* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2077* [Appointment](appointment.html): An Identifier of the Appointment 2078* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2079* [Basic](basic.html): Business identifier 2080* [BodyStructure](bodystructure.html): Bodystructure identifier 2081* [CarePlan](careplan.html): External Ids for this plan 2082* [CareTeam](careteam.html): External Ids for this team 2083* [ChargeItem](chargeitem.html): Business Identifier for item 2084* [Claim](claim.html): The primary identifier of the financial resource 2085* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2086* [ClinicalImpression](clinicalimpression.html): Business identifier 2087* [Communication](communication.html): Unique identifier 2088* [CommunicationRequest](communicationrequest.html): Unique identifier 2089* [Composition](composition.html): Version-independent identifier for the Composition 2090* [Condition](condition.html): A unique identifier of the condition record 2091* [Consent](consent.html): Identifier for this record (external references) 2092* [Contract](contract.html): The identity of the contract 2093* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2094* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2095* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2096* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2097* [DeviceRequest](devicerequest.html): Business identifier for request/order 2098* [DeviceUsage](deviceusage.html): Search by identifier 2099* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2100* [DocumentReference](documentreference.html): Identifier of the attachment binary 2101* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2102* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2103* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2104* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2105* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2106* [Flag](flag.html): Business identifier 2107* [Goal](goal.html): External Ids for this goal 2108* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2109* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2110* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2111* [Immunization](immunization.html): Business identifier 2112* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2113* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2114* [Invoice](invoice.html): Business Identifier for item 2115* [List](list.html): Business identifier 2116* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2117* [Medication](medication.html): Returns medications with this external identifier 2118* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2119* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2120* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2121* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2122* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2123* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2124* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2125* [Observation](observation.html): The unique id for a particular observation 2126* [Person](person.html): A person Identifier 2127* [Procedure](procedure.html): A unique identifier for a procedure 2128* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2129* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2130* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2131* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2132* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2133* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2134* [Specimen](specimen.html): The unique identifier associated with the specimen 2135* [SupplyDelivery](supplydelivery.html): External identifier 2136* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2137* [Task](task.html): Search for a task instance by its business identifier 2138* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2139</b><br> 2140 * Type: <b>token</b><br> 2141 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2142 * </p> 2143 */ 2144 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2145 2146 /** 2147 * Search parameter: <b>patient</b> 2148 * <p> 2149 * Description: <b>Multiple Resources: 2150 2151* [Account](account.html): The entity that caused the expenses 2152* [AdverseEvent](adverseevent.html): Subject impacted by event 2153* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2154* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2155* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2156* [AuditEvent](auditevent.html): Where the activity involved patient data 2157* [Basic](basic.html): Identifies the focus of this resource 2158* [BodyStructure](bodystructure.html): Who this is about 2159* [CarePlan](careplan.html): Who the care plan is for 2160* [CareTeam](careteam.html): Who care team is for 2161* [ChargeItem](chargeitem.html): Individual service was done for/to 2162* [Claim](claim.html): Patient receiving the products or services 2163* [ClaimResponse](claimresponse.html): The subject of care 2164* [ClinicalImpression](clinicalimpression.html): Patient assessed 2165* [Communication](communication.html): Focus of message 2166* [CommunicationRequest](communicationrequest.html): Focus of message 2167* [Composition](composition.html): Who and/or what the composition is about 2168* [Condition](condition.html): Who has the condition? 2169* [Consent](consent.html): Who the consent applies to 2170* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2171* [Coverage](coverage.html): Retrieve coverages for a patient 2172* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2173* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2174* [DetectedIssue](detectedissue.html): Associated patient 2175* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2176* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2177* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2178* [DocumentReference](documentreference.html): Who/what is the subject of the document 2179* [Encounter](encounter.html): The patient present at the encounter 2180* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2181* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2182* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2183* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2184* [Flag](flag.html): The identity of a subject to list flags for 2185* [Goal](goal.html): Who this goal is intended for 2186* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2187* [ImagingSelection](imagingselection.html): Who the study is about 2188* [ImagingStudy](imagingstudy.html): Who the study is about 2189* [Immunization](immunization.html): The patient for the vaccination record 2190* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2191* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2192* [Invoice](invoice.html): Recipient(s) of goods and services 2193* [List](list.html): If all resources have the same subject 2194* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2195* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2196* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2197* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2198* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2199* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2200* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2201* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2202* [Observation](observation.html): The subject that the observation is about (if patient) 2203* [Person](person.html): The Person links to this Patient 2204* [Procedure](procedure.html): Search by subject - a patient 2205* [Provenance](provenance.html): Where the activity involved patient data 2206* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2207* [RelatedPerson](relatedperson.html): The patient this related person is related to 2208* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2209* [ResearchSubject](researchsubject.html): Who or what is part of study 2210* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2211* [ServiceRequest](servicerequest.html): Search by subject - a patient 2212* [Specimen](specimen.html): The patient the specimen comes from 2213* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2214* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2215* [Task](task.html): Search by patient 2216* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2217</b><br> 2218 * Type: <b>reference</b><br> 2219 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2220 * </p> 2221 */ 2222 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2223 public static final String SP_PATIENT = "patient"; 2224 /** 2225 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2226 * <p> 2227 * Description: <b>Multiple Resources: 2228 2229* [Account](account.html): The entity that caused the expenses 2230* [AdverseEvent](adverseevent.html): Subject impacted by event 2231* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2232* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2233* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2234* [AuditEvent](auditevent.html): Where the activity involved patient data 2235* [Basic](basic.html): Identifies the focus of this resource 2236* [BodyStructure](bodystructure.html): Who this is about 2237* [CarePlan](careplan.html): Who the care plan is for 2238* [CareTeam](careteam.html): Who care team is for 2239* [ChargeItem](chargeitem.html): Individual service was done for/to 2240* [Claim](claim.html): Patient receiving the products or services 2241* [ClaimResponse](claimresponse.html): The subject of care 2242* [ClinicalImpression](clinicalimpression.html): Patient assessed 2243* [Communication](communication.html): Focus of message 2244* [CommunicationRequest](communicationrequest.html): Focus of message 2245* [Composition](composition.html): Who and/or what the composition is about 2246* [Condition](condition.html): Who has the condition? 2247* [Consent](consent.html): Who the consent applies to 2248* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2249* [Coverage](coverage.html): Retrieve coverages for a patient 2250* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2251* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2252* [DetectedIssue](detectedissue.html): Associated patient 2253* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2254* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2255* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2256* [DocumentReference](documentreference.html): Who/what is the subject of the document 2257* [Encounter](encounter.html): The patient present at the encounter 2258* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2259* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2260* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2261* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2262* [Flag](flag.html): The identity of a subject to list flags for 2263* [Goal](goal.html): Who this goal is intended for 2264* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2265* [ImagingSelection](imagingselection.html): Who the study is about 2266* [ImagingStudy](imagingstudy.html): Who the study is about 2267* [Immunization](immunization.html): The patient for the vaccination record 2268* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2269* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2270* [Invoice](invoice.html): Recipient(s) of goods and services 2271* [List](list.html): If all resources have the same subject 2272* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2273* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2274* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2275* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2276* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2277* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2278* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2279* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2280* [Observation](observation.html): The subject that the observation is about (if patient) 2281* [Person](person.html): The Person links to this Patient 2282* [Procedure](procedure.html): Search by subject - a patient 2283* [Provenance](provenance.html): Where the activity involved patient data 2284* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2285* [RelatedPerson](relatedperson.html): The patient this related person is related to 2286* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2287* [ResearchSubject](researchsubject.html): Who or what is part of study 2288* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2289* [ServiceRequest](servicerequest.html): Search by subject - a patient 2290* [Specimen](specimen.html): The patient the specimen comes from 2291* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2292* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2293* [Task](task.html): Search by patient 2294* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2295</b><br> 2296 * Type: <b>reference</b><br> 2297 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2298 * </p> 2299 */ 2300 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2301 2302/** 2303 * Constant for fluent queries to be used to add include statements. Specifies 2304 * the path value of "<b>BodyStructure:patient</b>". 2305 */ 2306 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("BodyStructure:patient").toLocked(); 2307 2308 2309} 2310