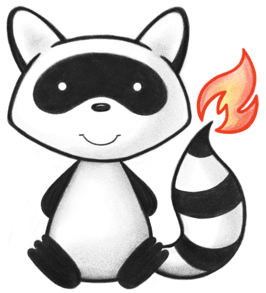
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051import org.hl7.fhir.instance.model.api.IBaseBundle; 052/** 053 * A container for a collection of resources. 054 */ 055@ResourceDef(name="Bundle", profile="http://hl7.org/fhir/StructureDefinition/Bundle") 056public class Bundle extends Resource implements IBaseBundle { 057 058 public enum BundleType { 059 /** 060 * The bundle is a document. The first resource is a Composition. 061 */ 062 DOCUMENT, 063 /** 064 * The bundle is a message. The first resource is a MessageHeader. 065 */ 066 MESSAGE, 067 /** 068 * The bundle is a transaction - intended to be processed by a server as an atomic commit. 069 */ 070 TRANSACTION, 071 /** 072 * The bundle is a transaction response. Because the response is a transaction response, the transaction has succeeded, and all responses are error free. 073 */ 074 TRANSACTIONRESPONSE, 075 /** 076 * The bundle is a set of actions - intended to be processed by a server as a group of independent actions. 077 */ 078 BATCH, 079 /** 080 * The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success. 081 */ 082 BATCHRESPONSE, 083 /** 084 * The bundle is a list of resources from a history interaction on a server. 085 */ 086 HISTORY, 087 /** 088 * The bundle is a list of resources returned as a result of a search/query interaction, operation, or message. 089 */ 090 SEARCHSET, 091 /** 092 * The bundle is a set of resources collected into a single package for ease of distribution that imposes no processing obligations or behavioral rules beyond persistence. 093 */ 094 COLLECTION, 095 /** 096 * The bundle has been generated by a Subscription to communicate information to a client. 097 */ 098 SUBSCRIPTIONNOTIFICATION, 099 /** 100 * added to help the parsers with the generic types 101 */ 102 NULL; 103 public static BundleType fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("document".equals(codeString)) 107 return DOCUMENT; 108 if ("message".equals(codeString)) 109 return MESSAGE; 110 if ("transaction".equals(codeString)) 111 return TRANSACTION; 112 if ("transaction-response".equals(codeString)) 113 return TRANSACTIONRESPONSE; 114 if ("batch".equals(codeString)) 115 return BATCH; 116 if ("batch-response".equals(codeString)) 117 return BATCHRESPONSE; 118 if ("history".equals(codeString)) 119 return HISTORY; 120 if ("searchset".equals(codeString)) 121 return SEARCHSET; 122 if ("collection".equals(codeString)) 123 return COLLECTION; 124 if ("subscription-notification".equals(codeString)) 125 return SUBSCRIPTIONNOTIFICATION; 126 if (Configuration.isAcceptInvalidEnums()) 127 return null; 128 else 129 throw new FHIRException("Unknown BundleType code '"+codeString+"'"); 130 } 131 public String toCode() { 132 switch (this) { 133 case DOCUMENT: return "document"; 134 case MESSAGE: return "message"; 135 case TRANSACTION: return "transaction"; 136 case TRANSACTIONRESPONSE: return "transaction-response"; 137 case BATCH: return "batch"; 138 case BATCHRESPONSE: return "batch-response"; 139 case HISTORY: return "history"; 140 case SEARCHSET: return "searchset"; 141 case COLLECTION: return "collection"; 142 case SUBSCRIPTIONNOTIFICATION: return "subscription-notification"; 143 case NULL: return null; 144 default: return "?"; 145 } 146 } 147 public String getSystem() { 148 switch (this) { 149 case DOCUMENT: return "http://hl7.org/fhir/bundle-type"; 150 case MESSAGE: return "http://hl7.org/fhir/bundle-type"; 151 case TRANSACTION: return "http://hl7.org/fhir/bundle-type"; 152 case TRANSACTIONRESPONSE: return "http://hl7.org/fhir/bundle-type"; 153 case BATCH: return "http://hl7.org/fhir/bundle-type"; 154 case BATCHRESPONSE: return "http://hl7.org/fhir/bundle-type"; 155 case HISTORY: return "http://hl7.org/fhir/bundle-type"; 156 case SEARCHSET: return "http://hl7.org/fhir/bundle-type"; 157 case COLLECTION: return "http://hl7.org/fhir/bundle-type"; 158 case SUBSCRIPTIONNOTIFICATION: return "http://hl7.org/fhir/bundle-type"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 public String getDefinition() { 164 switch (this) { 165 case DOCUMENT: return "The bundle is a document. The first resource is a Composition."; 166 case MESSAGE: return "The bundle is a message. The first resource is a MessageHeader."; 167 case TRANSACTION: return "The bundle is a transaction - intended to be processed by a server as an atomic commit."; 168 case TRANSACTIONRESPONSE: return "The bundle is a transaction response. Because the response is a transaction response, the transaction has succeeded, and all responses are error free."; 169 case BATCH: return "The bundle is a set of actions - intended to be processed by a server as a group of independent actions."; 170 case BATCHRESPONSE: return "The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success."; 171 case HISTORY: return "The bundle is a list of resources from a history interaction on a server."; 172 case SEARCHSET: return "The bundle is a list of resources returned as a result of a search/query interaction, operation, or message."; 173 case COLLECTION: return "The bundle is a set of resources collected into a single package for ease of distribution that imposes no processing obligations or behavioral rules beyond persistence."; 174 case SUBSCRIPTIONNOTIFICATION: return "The bundle has been generated by a Subscription to communicate information to a client."; 175 case NULL: return null; 176 default: return "?"; 177 } 178 } 179 public String getDisplay() { 180 switch (this) { 181 case DOCUMENT: return "Document"; 182 case MESSAGE: return "Message"; 183 case TRANSACTION: return "Transaction"; 184 case TRANSACTIONRESPONSE: return "Transaction Response"; 185 case BATCH: return "Batch"; 186 case BATCHRESPONSE: return "Batch Response"; 187 case HISTORY: return "History List"; 188 case SEARCHSET: return "Search Results"; 189 case COLLECTION: return "Collection"; 190 case SUBSCRIPTIONNOTIFICATION: return "Subscription Notification"; 191 case NULL: return null; 192 default: return "?"; 193 } 194 } 195 } 196 197 public static class BundleTypeEnumFactory implements EnumFactory<BundleType> { 198 public BundleType fromCode(String codeString) throws IllegalArgumentException { 199 if (codeString == null || "".equals(codeString)) 200 if (codeString == null || "".equals(codeString)) 201 return null; 202 if ("document".equals(codeString)) 203 return BundleType.DOCUMENT; 204 if ("message".equals(codeString)) 205 return BundleType.MESSAGE; 206 if ("transaction".equals(codeString)) 207 return BundleType.TRANSACTION; 208 if ("transaction-response".equals(codeString)) 209 return BundleType.TRANSACTIONRESPONSE; 210 if ("batch".equals(codeString)) 211 return BundleType.BATCH; 212 if ("batch-response".equals(codeString)) 213 return BundleType.BATCHRESPONSE; 214 if ("history".equals(codeString)) 215 return BundleType.HISTORY; 216 if ("searchset".equals(codeString)) 217 return BundleType.SEARCHSET; 218 if ("collection".equals(codeString)) 219 return BundleType.COLLECTION; 220 if ("subscription-notification".equals(codeString)) 221 return BundleType.SUBSCRIPTIONNOTIFICATION; 222 throw new IllegalArgumentException("Unknown BundleType code '"+codeString+"'"); 223 } 224 public Enumeration<BundleType> fromType(PrimitiveType<?> code) throws FHIRException { 225 if (code == null) 226 return null; 227 if (code.isEmpty()) 228 return new Enumeration<BundleType>(this, BundleType.NULL, code); 229 String codeString = ((PrimitiveType) code).asStringValue(); 230 if (codeString == null || "".equals(codeString)) 231 return new Enumeration<BundleType>(this, BundleType.NULL, code); 232 if ("document".equals(codeString)) 233 return new Enumeration<BundleType>(this, BundleType.DOCUMENT, code); 234 if ("message".equals(codeString)) 235 return new Enumeration<BundleType>(this, BundleType.MESSAGE, code); 236 if ("transaction".equals(codeString)) 237 return new Enumeration<BundleType>(this, BundleType.TRANSACTION, code); 238 if ("transaction-response".equals(codeString)) 239 return new Enumeration<BundleType>(this, BundleType.TRANSACTIONRESPONSE, code); 240 if ("batch".equals(codeString)) 241 return new Enumeration<BundleType>(this, BundleType.BATCH, code); 242 if ("batch-response".equals(codeString)) 243 return new Enumeration<BundleType>(this, BundleType.BATCHRESPONSE, code); 244 if ("history".equals(codeString)) 245 return new Enumeration<BundleType>(this, BundleType.HISTORY, code); 246 if ("searchset".equals(codeString)) 247 return new Enumeration<BundleType>(this, BundleType.SEARCHSET, code); 248 if ("collection".equals(codeString)) 249 return new Enumeration<BundleType>(this, BundleType.COLLECTION, code); 250 if ("subscription-notification".equals(codeString)) 251 return new Enumeration<BundleType>(this, BundleType.SUBSCRIPTIONNOTIFICATION, code); 252 throw new FHIRException("Unknown BundleType code '"+codeString+"'"); 253 } 254 public String toCode(BundleType code) { 255 if (code == BundleType.NULL) 256 return null; 257 if (code == BundleType.DOCUMENT) 258 return "document"; 259 if (code == BundleType.MESSAGE) 260 return "message"; 261 if (code == BundleType.TRANSACTION) 262 return "transaction"; 263 if (code == BundleType.TRANSACTIONRESPONSE) 264 return "transaction-response"; 265 if (code == BundleType.BATCH) 266 return "batch"; 267 if (code == BundleType.BATCHRESPONSE) 268 return "batch-response"; 269 if (code == BundleType.HISTORY) 270 return "history"; 271 if (code == BundleType.SEARCHSET) 272 return "searchset"; 273 if (code == BundleType.COLLECTION) 274 return "collection"; 275 if (code == BundleType.SUBSCRIPTIONNOTIFICATION) 276 return "subscription-notification"; 277 return "?"; 278 } 279 public String toSystem(BundleType code) { 280 return code.getSystem(); 281 } 282 } 283 284 public enum HTTPVerb { 285 /** 286 * HTTP GET Command. 287 */ 288 GET, 289 /** 290 * HTTP HEAD Command. 291 */ 292 HEAD, 293 /** 294 * HTTP POST Command. 295 */ 296 POST, 297 /** 298 * HTTP PUT Command. 299 */ 300 PUT, 301 /** 302 * HTTP DELETE Command. 303 */ 304 DELETE, 305 /** 306 * HTTP PATCH Command. 307 */ 308 PATCH, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 public static HTTPVerb fromCode(String codeString) throws FHIRException { 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("GET".equals(codeString)) 317 return GET; 318 if ("HEAD".equals(codeString)) 319 return HEAD; 320 if ("POST".equals(codeString)) 321 return POST; 322 if ("PUT".equals(codeString)) 323 return PUT; 324 if ("DELETE".equals(codeString)) 325 return DELETE; 326 if ("PATCH".equals(codeString)) 327 return PATCH; 328 if (Configuration.isAcceptInvalidEnums()) 329 return null; 330 else 331 throw new FHIRException("Unknown HTTPVerb code '"+codeString+"'"); 332 } 333 public String toCode() { 334 switch (this) { 335 case GET: return "GET"; 336 case HEAD: return "HEAD"; 337 case POST: return "POST"; 338 case PUT: return "PUT"; 339 case DELETE: return "DELETE"; 340 case PATCH: return "PATCH"; 341 case NULL: return null; 342 default: return "?"; 343 } 344 } 345 public String getSystem() { 346 switch (this) { 347 case GET: return "http://hl7.org/fhir/http-verb"; 348 case HEAD: return "http://hl7.org/fhir/http-verb"; 349 case POST: return "http://hl7.org/fhir/http-verb"; 350 case PUT: return "http://hl7.org/fhir/http-verb"; 351 case DELETE: return "http://hl7.org/fhir/http-verb"; 352 case PATCH: return "http://hl7.org/fhir/http-verb"; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 public String getDefinition() { 358 switch (this) { 359 case GET: return "HTTP GET Command."; 360 case HEAD: return "HTTP HEAD Command."; 361 case POST: return "HTTP POST Command."; 362 case PUT: return "HTTP PUT Command."; 363 case DELETE: return "HTTP DELETE Command."; 364 case PATCH: return "HTTP PATCH Command."; 365 case NULL: return null; 366 default: return "?"; 367 } 368 } 369 public String getDisplay() { 370 switch (this) { 371 case GET: return "GET"; 372 case HEAD: return "HEAD"; 373 case POST: return "POST"; 374 case PUT: return "PUT"; 375 case DELETE: return "DELETE"; 376 case PATCH: return "PATCH"; 377 case NULL: return null; 378 default: return "?"; 379 } 380 } 381 } 382 383 public static class HTTPVerbEnumFactory implements EnumFactory<HTTPVerb> { 384 public HTTPVerb fromCode(String codeString) throws IllegalArgumentException { 385 if (codeString == null || "".equals(codeString)) 386 if (codeString == null || "".equals(codeString)) 387 return null; 388 if ("GET".equals(codeString)) 389 return HTTPVerb.GET; 390 if ("HEAD".equals(codeString)) 391 return HTTPVerb.HEAD; 392 if ("POST".equals(codeString)) 393 return HTTPVerb.POST; 394 if ("PUT".equals(codeString)) 395 return HTTPVerb.PUT; 396 if ("DELETE".equals(codeString)) 397 return HTTPVerb.DELETE; 398 if ("PATCH".equals(codeString)) 399 return HTTPVerb.PATCH; 400 throw new IllegalArgumentException("Unknown HTTPVerb code '"+codeString+"'"); 401 } 402 public Enumeration<HTTPVerb> fromType(PrimitiveType<?> code) throws FHIRException { 403 if (code == null) 404 return null; 405 if (code.isEmpty()) 406 return new Enumeration<HTTPVerb>(this, HTTPVerb.NULL, code); 407 String codeString = ((PrimitiveType) code).asStringValue(); 408 if (codeString == null || "".equals(codeString)) 409 return new Enumeration<HTTPVerb>(this, HTTPVerb.NULL, code); 410 if ("GET".equals(codeString)) 411 return new Enumeration<HTTPVerb>(this, HTTPVerb.GET, code); 412 if ("HEAD".equals(codeString)) 413 return new Enumeration<HTTPVerb>(this, HTTPVerb.HEAD, code); 414 if ("POST".equals(codeString)) 415 return new Enumeration<HTTPVerb>(this, HTTPVerb.POST, code); 416 if ("PUT".equals(codeString)) 417 return new Enumeration<HTTPVerb>(this, HTTPVerb.PUT, code); 418 if ("DELETE".equals(codeString)) 419 return new Enumeration<HTTPVerb>(this, HTTPVerb.DELETE, code); 420 if ("PATCH".equals(codeString)) 421 return new Enumeration<HTTPVerb>(this, HTTPVerb.PATCH, code); 422 throw new FHIRException("Unknown HTTPVerb code '"+codeString+"'"); 423 } 424 public String toCode(HTTPVerb code) { 425 if (code == HTTPVerb.NULL) 426 return null; 427 if (code == HTTPVerb.GET) 428 return "GET"; 429 if (code == HTTPVerb.HEAD) 430 return "HEAD"; 431 if (code == HTTPVerb.POST) 432 return "POST"; 433 if (code == HTTPVerb.PUT) 434 return "PUT"; 435 if (code == HTTPVerb.DELETE) 436 return "DELETE"; 437 if (code == HTTPVerb.PATCH) 438 return "PATCH"; 439 return "?"; 440 } 441 public String toSystem(HTTPVerb code) { 442 return code.getSystem(); 443 } 444 } 445 446 public enum LinkRelationTypes { 447 /** 448 * Refers to a resource that is the subject of the link's context. 449 */ 450 ABOUT, 451 /** 452 * Asserts that the link target provides an access control description for the link context. 453 */ 454 ACL, 455 /** 456 * Refers to a substitute for this context 457 */ 458 ALTERNATE, 459 /** 460 * Used to reference alternative content that uses the AMP profile of the HTML format. 461 */ 462 AMPHTML, 463 /** 464 * Refers to an appendix. 465 */ 466 APPENDIX, 467 /** 468 * Refers to an icon for the context. Synonym for icon. 469 */ 470 APPLETOUCHICON, 471 /** 472 * Refers to a launch screen for the context. 473 */ 474 APPLETOUCHSTARTUPIMAGE, 475 /** 476 * Refers to a collection of records, documents, or other\n materials of historical interest. 477 */ 478 ARCHIVES, 479 /** 480 * Refers to the context's author. 481 */ 482 AUTHOR, 483 /** 484 * Identifies the entity that blocks access to a resource\n following receipt of a legal demand. 485 */ 486 BLOCKEDBY, 487 /** 488 * Gives a permanent link to use for bookmarking purposes. 489 */ 490 BOOKMARK, 491 /** 492 * Designates the preferred version of a resource (the IRI and its contents). 493 */ 494 CANONICAL, 495 /** 496 * Refers to a chapter in a collection of resources. 497 */ 498 CHAPTER, 499 /** 500 * Indicates that the link target is preferred over the link context for the purpose of permanent citation. 501 */ 502 CITEAS, 503 /** 504 * The target IRI points to a resource which represents the collection resource for the context IRI. 505 */ 506 COLLECTION, 507 /** 508 * Refers to a table of contents. 509 */ 510 CONTENTS, 511 /** 512 * The document linked to was later converted to the\n document that contains this link relation. For example, an RFC can\n have a link to the Internet-Draft that became the RFC; in that case,\n the link relation would be \"convertedFrom\". 513 */ 514 CONVERTEDFROM, 515 /** 516 * Refers to a copyright statement that applies to the\n link's context. 517 */ 518 COPYRIGHT, 519 /** 520 * The target IRI points to a resource where a submission form can be obtained. 521 */ 522 CREATEFORM, 523 /** 524 * Refers to a resource containing the most recent\n item(s) in a collection of resources. 525 */ 526 CURRENT, 527 /** 528 * Refers to a resource providing information about the\n link's context. 529 */ 530 DESCRIBEDBY, 531 /** 532 * The relationship A 'describes' B asserts that\n resource A provides a description of resource B. There are no\n constraints on the format or representation of either A or B,\n neither are there any further constraints on either resource. 533 */ 534 DESCRIBES, 535 /** 536 * Refers to a list of patent disclosures made with respect to \n material for which 'disclosure' relation is specified. 537 */ 538 DISCLOSURE, 539 /** 540 * Used to indicate an origin that will be used to fetch required \n resources for the link context, and that the user agent ought to resolve \n as early as possible. 541 */ 542 DNSPREFETCH, 543 /** 544 * Refers to a resource whose available representations\n are byte-for-byte identical with the corresponding representations of\n the context IRI. 545 */ 546 DUPLICATE, 547 /** 548 * Refers to a resource that can be used to edit the\n link's context. 549 */ 550 EDIT, 551 /** 552 * The target IRI points to a resource where a submission form for\n editing associated resource can be obtained. 553 */ 554 EDITFORM, 555 /** 556 * Refers to a resource that can be used to edit media\n associated with the link's context. 557 */ 558 EDITMEDIA, 559 /** 560 * Identifies a related resource that is potentially\n large and might require special handling. 561 */ 562 ENCLOSURE, 563 /** 564 * Refers to a resource that is not part of the same site as the current context. 565 */ 566 EXTERNAL, 567 /** 568 * An IRI that refers to the furthest preceding resource\n in a series of resources. 569 */ 570 FIRST, 571 /** 572 * Refers to a glossary of terms. 573 */ 574 GLOSSARY, 575 /** 576 * Refers to context-sensitive help. 577 */ 578 HELP, 579 /** 580 * Refers to a resource hosted by the server indicated by\n the link context. 581 */ 582 HOSTS, 583 /** 584 * Refers to a hub that enables registration for\n notification of updates to the context. 585 */ 586 HUB, 587 /** 588 * Refers to an icon representing the link's context. 589 */ 590 ICON, 591 /** 592 * Refers to an index. 593 */ 594 INDEX, 595 /** 596 * refers to a resource associated with a time interval that ends before the beginning of the time interval associated with the context resource 597 */ 598 INTERVALAFTER, 599 /** 600 * refers to a resource associated with a time interval that begins after the end of the time interval associated with the context resource 601 */ 602 INTERVALBEFORE, 603 /** 604 * refers to a resource associated with a time interval that begins after the beginning of the time interval associated with the context resource, and ends before the end of the time interval associated with the context resource 605 */ 606 INTERVALCONTAINS, 607 /** 608 * refers to a resource associated with a time interval that begins after the end of the time interval associated with the context resource, or ends before the beginning of the time interval associated with the context resource 609 */ 610 INTERVALDISJOINT, 611 /** 612 * refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource 613 */ 614 INTERVALDURING, 615 /** 616 * refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource 617 */ 618 INTERVALEQUALS, 619 /** 620 * refers to a resource associated with a time interval that begins after the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource 621 */ 622 INTERVALFINISHEDBY, 623 /** 624 * refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource 625 */ 626 INTERVALFINISHES, 627 /** 628 * refers to a resource associated with a time interval that begins before or is coincident with the beginning of the time interval associated with the context resource, and ends after or is coincident with the end of the time interval associated with the context resource 629 */ 630 INTERVALIN, 631 /** 632 * refers to a resource associated with a time interval whose beginning coincides with the end of the time interval associated with the context resource 633 */ 634 INTERVALMEETS, 635 /** 636 * refers to a resource associated with a time interval whose end coincides with the beginning of the time interval associated with the context resource 637 */ 638 INTERVALMETBY, 639 /** 640 * refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and ends after the beginning of the time interval associated with the context resource 641 */ 642 INTERVALOVERLAPPEDBY, 643 /** 644 * refers to a resource associated with a time interval that begins before the end of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource 645 */ 646 INTERVALOVERLAPS, 647 /** 648 * refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and ends before the end of the time interval associated with the context resource 649 */ 650 INTERVALSTARTEDBY, 651 /** 652 * refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource 653 */ 654 INTERVALSTARTS, 655 /** 656 * The target IRI points to a resource that is a member of the collection represented by the context IRI. 657 */ 658 ITEM, 659 /** 660 * An IRI that refers to the furthest following resource\n in a series of resources. 661 */ 662 LAST, 663 /** 664 * Points to a resource containing the latest (e.g.,\n current) version of the context. 665 */ 666 LATESTVERSION, 667 /** 668 * Refers to a license associated with this context. 669 */ 670 LICENSE, 671 /** 672 * The link target of a link with the \"linkset\" relation\n type provides a set of links, including links in which the link\n context of the link participates.\n 673 */ 674 LINKSET, 675 /** 676 * Refers to further information about the link's context,\n expressed as a LRDD (\"Link-based Resource Descriptor Document\")\n resource. See for information about\n processing this relation type in host-meta documents. When used\n elsewhere, it refers to additional links and other metadata.\n Multiple instances indicate additional LRDD resources. LRDD\n resources MUST have an \"application/xrd+xml\" representation, and\n MAY have others. 677 */ 678 LRDD, 679 /** 680 * Links to a manifest file for the context. 681 */ 682 MANIFEST, 683 /** 684 * Refers to a mask that can be applied to the icon for the context. 685 */ 686 MASKICON, 687 /** 688 * Refers to a feed of personalised media recommendations relevant to the link context. 689 */ 690 MEDIAFEED, 691 /** 692 * The Target IRI points to a Memento, a fixed resource that will not change state anymore. 693 */ 694 MEMENTO, 695 /** 696 * Links to the context's Micropub endpoint. 697 */ 698 MICROPUB, 699 /** 700 * Refers to a module that the user agent is to preemptively fetch and store for use in the current context. 701 */ 702 MODULEPRELOAD, 703 /** 704 * Refers to a resource that can be used to monitor changes in an HTTP resource.\n 705 */ 706 MONITOR, 707 /** 708 * Refers to a resource that can be used to monitor changes in a specified group of HTTP resources.\n 709 */ 710 MONITORGROUP, 711 /** 712 * Indicates that the link's context is a part of a series, and\n that the next in the series is the link target.\n 713 */ 714 NEXT, 715 /** 716 * Refers to the immediately following archive resource. 717 */ 718 NEXTARCHIVE, 719 /** 720 * Indicates that the context?s original author or publisher does not endorse the link target. 721 */ 722 NOFOLLOW, 723 /** 724 * Indicates that any newly created top-level browsing context which results from following the link will not be an auxiliary browsing context. 725 */ 726 NOOPENER, 727 /** 728 * Indicates that no referrer information is to be leaked when following the link. 729 */ 730 NOREFERRER, 731 /** 732 * Indicates that any newly created top-level browsing context which results from following the link will be an auxiliary browsing context. 733 */ 734 OPENER, 735 /** 736 * Refers to an OpenID Authentication server on which the context relies for an assertion that the end user controls an Identifier. 737 */ 738 OPENID2_LOCALID, 739 /** 740 * Refers to a resource which accepts OpenID Authentication protocol messages for the context. 741 */ 742 OPENID2_PROVIDER, 743 /** 744 * The Target IRI points to an Original Resource. 745 */ 746 ORIGINAL, 747 /** 748 * Refers to a P3P privacy policy for the context. 749 */ 750 P3PV1, 751 /** 752 * Indicates a resource where payment is accepted. 753 */ 754 PAYMENT, 755 /** 756 * Gives the address of the pingback resource for the link context. 757 */ 758 PINGBACK, 759 /** 760 * Used to indicate an origin that will be used to fetch required \n resources for the link context. Initiating an early connection, which \n includes the DNS lookup, TCP handshake, and optional TLS negotiation, \n allows the user agent to mask the high latency costs of establishing a \n connection. 761 */ 762 PRECONNECT, 763 /** 764 * Points to a resource containing the predecessor\n version in the version history.\n 765 */ 766 PREDECESSORVERSION, 767 /** 768 * The prefetch link relation type is used to identify a resource \n that might be required by the next navigation from the link context, and \n that the user agent ought to fetch, such that the user agent can deliver a \n faster response once the resource is requested in the future. 769 */ 770 PREFETCH, 771 /** 772 * Refers to a resource that should be loaded early in the \n processing of the link's context, without blocking rendering. 773 */ 774 PRELOAD, 775 /** 776 * Used to identify a resource that might be required by the next \n navigation from the link context, and that the user agent ought to fetch \n and execute, such that the user agent can deliver a faster response once \n the resource is requested in the future. 777 */ 778 PRERENDER, 779 /** 780 * Indicates that the link's context is a part of a series, and\n that the previous in the series is the link target.\n 781 */ 782 PREV, 783 /** 784 * Refers to a resource that provides a preview of the link's context. 785 */ 786 PREVIEW, 787 /** 788 * Refers to the previous resource in an ordered series\n of resources. Synonym for \"prev\". 789 */ 790 PREVIOUS, 791 /** 792 * Refers to the immediately preceding archive resource. 793 */ 794 PREVARCHIVE, 795 /** 796 * Refers to a privacy policy associated with the link's context. 797 */ 798 PRIVACYPOLICY, 799 /** 800 * Identifying that a resource representation conforms\nto a certain profile, without affecting the non-profile semantics\nof the resource representation. 801 */ 802 PROFILE, 803 /** 804 * Links to a publication manifest. A manifest represents \n structured information about a publication, such as informative metadata, \n a list of resources, and a default reading order. 805 */ 806 PUBLICATION, 807 /** 808 * Identifies a related resource. 809 */ 810 RELATED, 811 /** 812 * Identifies the root of RESTCONF API as configured on this HTTP server.\n The \"restconf\" relation defines the root of the API defined in RFC8040.\n Subsequent revisions of RESTCONF will use alternate relation values to support \n protocol versioning. 813 */ 814 RESTCONF, 815 /** 816 * Identifies a resource that is a reply to the context\n of the link.\n 817 */ 818 REPLIES, 819 /** 820 * The resource identified by the link target provides an input value to an \n instance of a rule, where the resource which represents the rule instance is \n identified by the link context.\n 821 */ 822 RULEINPUT, 823 /** 824 * Refers to a resource that can be used to search through\n the link's context and related resources. 825 */ 826 SEARCH, 827 /** 828 * Refers to a section in a collection of resources. 829 */ 830 SECTION, 831 /** 832 * Conveys an identifier for the link's context.\n 833 */ 834 SELF, 835 /** 836 * Indicates a URI that can be used to retrieve a\n service document. 837 */ 838 SERVICE, 839 /** 840 * Identifies service description for the context that\n is primarily intended for consumption by machines. 841 */ 842 SERVICEDESC, 843 /** 844 * Identifies service documentation for the context that\n is primarily intended for human consumption. 845 */ 846 SERVICEDOC, 847 /** 848 * Identifies general metadata for the context that is\n primarily intended for consumption by machines. 849 */ 850 SERVICEMETA, 851 /** 852 * Refers to a resource that is within a context that is \n sponsored (such as advertising or another compensation agreement). 853 */ 854 SPONSORED, 855 /** 856 * Refers to the first resource in a collection of\n resources. 857 */ 858 START, 859 /** 860 * Identifies a resource that represents the context's\n status. 861 */ 862 STATUS, 863 /** 864 * Refers to a stylesheet. 865 */ 866 STYLESHEET, 867 /** 868 * Refers to a resource serving as a subsection in a\n collection of resources. 869 */ 870 SUBSECTION, 871 /** 872 * Points to a resource containing the successor version\n in the version history.\n 873 */ 874 SUCCESSORVERSION, 875 /** 876 * Identifies a resource that provides information about\n the context's retirement policy.\n 877 */ 878 SUNSET, 879 /** 880 * Gives a tag (identified by the given address) that applies to\n the current document.\n 881 */ 882 TAG, 883 /** 884 * Refers to the terms of service associated with the link's context. 885 */ 886 TERMSOFSERVICE, 887 /** 888 * The Target IRI points to a TimeGate for an Original Resource. 889 */ 890 TIMEGATE, 891 /** 892 * The Target IRI points to a TimeMap for an Original Resource. 893 */ 894 TIMEMAP, 895 /** 896 * Refers to a resource identifying the abstract semantic type of which the link's context is considered to be an instance. 897 */ 898 TYPE, 899 /** 900 * Refers to a resource that is within a context that is User Generated Content.\n 901 */ 902 UGC, 903 /** 904 * Refers to a parent document in a hierarchy of\n documents.\n 905 */ 906 UP, 907 /** 908 * Points to a resource containing the version history\n for the context.\n 909 */ 910 VERSIONHISTORY, 911 /** 912 * Identifies a resource that is the source of the\n information in the link's context.\n 913 */ 914 VIA, 915 /** 916 * Identifies a target URI that supports the Webmention protocol.\n This allows clients that mention a resource in some form of publishing process\n to contact that endpoint and inform it that this resource has been mentioned. 917 */ 918 WEBMENTION, 919 /** 920 * Points to a working copy for this resource. 921 */ 922 WORKINGCOPY, 923 /** 924 * Points to the versioned resource from which this\n working copy was obtained.\n 925 */ 926 WORKINGCOPYOF, 927 /** 928 * added to help the parsers with the generic types 929 */ 930 NULL; 931 public static LinkRelationTypes fromCode(String codeString) throws FHIRException { 932 if (codeString == null || "".equals(codeString)) 933 return null; 934 if ("about".equals(codeString)) 935 return ABOUT; 936 if ("acl".equals(codeString)) 937 return ACL; 938 if ("alternate".equals(codeString)) 939 return ALTERNATE; 940 if ("amphtml".equals(codeString)) 941 return AMPHTML; 942 if ("appendix".equals(codeString)) 943 return APPENDIX; 944 if ("apple-touch-icon".equals(codeString)) 945 return APPLETOUCHICON; 946 if ("apple-touch-startup-image".equals(codeString)) 947 return APPLETOUCHSTARTUPIMAGE; 948 if ("archives".equals(codeString)) 949 return ARCHIVES; 950 if ("author".equals(codeString)) 951 return AUTHOR; 952 if ("blocked-by".equals(codeString)) 953 return BLOCKEDBY; 954 if ("bookmark".equals(codeString)) 955 return BOOKMARK; 956 if ("canonical".equals(codeString)) 957 return CANONICAL; 958 if ("chapter".equals(codeString)) 959 return CHAPTER; 960 if ("cite-as".equals(codeString)) 961 return CITEAS; 962 if ("collection".equals(codeString)) 963 return COLLECTION; 964 if ("contents".equals(codeString)) 965 return CONTENTS; 966 if ("convertedFrom".equals(codeString)) 967 return CONVERTEDFROM; 968 if ("copyright".equals(codeString)) 969 return COPYRIGHT; 970 if ("create-form".equals(codeString)) 971 return CREATEFORM; 972 if ("current".equals(codeString)) 973 return CURRENT; 974 if ("describedby".equals(codeString)) 975 return DESCRIBEDBY; 976 if ("describes".equals(codeString)) 977 return DESCRIBES; 978 if ("disclosure".equals(codeString)) 979 return DISCLOSURE; 980 if ("dns-prefetch".equals(codeString)) 981 return DNSPREFETCH; 982 if ("duplicate".equals(codeString)) 983 return DUPLICATE; 984 if ("edit".equals(codeString)) 985 return EDIT; 986 if ("edit-form".equals(codeString)) 987 return EDITFORM; 988 if ("edit-media".equals(codeString)) 989 return EDITMEDIA; 990 if ("enclosure".equals(codeString)) 991 return ENCLOSURE; 992 if ("external".equals(codeString)) 993 return EXTERNAL; 994 if ("first".equals(codeString)) 995 return FIRST; 996 if ("glossary".equals(codeString)) 997 return GLOSSARY; 998 if ("help".equals(codeString)) 999 return HELP; 1000 if ("hosts".equals(codeString)) 1001 return HOSTS; 1002 if ("hub".equals(codeString)) 1003 return HUB; 1004 if ("icon".equals(codeString)) 1005 return ICON; 1006 if ("index".equals(codeString)) 1007 return INDEX; 1008 if ("intervalAfter".equals(codeString)) 1009 return INTERVALAFTER; 1010 if ("intervalBefore".equals(codeString)) 1011 return INTERVALBEFORE; 1012 if ("intervalContains".equals(codeString)) 1013 return INTERVALCONTAINS; 1014 if ("intervalDisjoint".equals(codeString)) 1015 return INTERVALDISJOINT; 1016 if ("intervalDuring".equals(codeString)) 1017 return INTERVALDURING; 1018 if ("intervalEquals".equals(codeString)) 1019 return INTERVALEQUALS; 1020 if ("intervalFinishedBy".equals(codeString)) 1021 return INTERVALFINISHEDBY; 1022 if ("intervalFinishes".equals(codeString)) 1023 return INTERVALFINISHES; 1024 if ("intervalIn".equals(codeString)) 1025 return INTERVALIN; 1026 if ("intervalMeets".equals(codeString)) 1027 return INTERVALMEETS; 1028 if ("intervalMetBy".equals(codeString)) 1029 return INTERVALMETBY; 1030 if ("intervalOverlappedBy".equals(codeString)) 1031 return INTERVALOVERLAPPEDBY; 1032 if ("intervalOverlaps".equals(codeString)) 1033 return INTERVALOVERLAPS; 1034 if ("intervalStartedBy".equals(codeString)) 1035 return INTERVALSTARTEDBY; 1036 if ("intervalStarts".equals(codeString)) 1037 return INTERVALSTARTS; 1038 if ("item".equals(codeString)) 1039 return ITEM; 1040 if ("last".equals(codeString)) 1041 return LAST; 1042 if ("latest-version".equals(codeString)) 1043 return LATESTVERSION; 1044 if ("license".equals(codeString)) 1045 return LICENSE; 1046 if ("linkset".equals(codeString)) 1047 return LINKSET; 1048 if ("lrdd".equals(codeString)) 1049 return LRDD; 1050 if ("manifest".equals(codeString)) 1051 return MANIFEST; 1052 if ("mask-icon".equals(codeString)) 1053 return MASKICON; 1054 if ("media-feed".equals(codeString)) 1055 return MEDIAFEED; 1056 if ("memento".equals(codeString)) 1057 return MEMENTO; 1058 if ("micropub".equals(codeString)) 1059 return MICROPUB; 1060 if ("modulepreload".equals(codeString)) 1061 return MODULEPRELOAD; 1062 if ("monitor".equals(codeString)) 1063 return MONITOR; 1064 if ("monitor-group".equals(codeString)) 1065 return MONITORGROUP; 1066 if ("next".equals(codeString)) 1067 return NEXT; 1068 if ("next-archive".equals(codeString)) 1069 return NEXTARCHIVE; 1070 if ("nofollow".equals(codeString)) 1071 return NOFOLLOW; 1072 if ("noopener".equals(codeString)) 1073 return NOOPENER; 1074 if ("noreferrer".equals(codeString)) 1075 return NOREFERRER; 1076 if ("opener".equals(codeString)) 1077 return OPENER; 1078 if ("openid2.local_id".equals(codeString)) 1079 return OPENID2_LOCALID; 1080 if ("openid2.provider".equals(codeString)) 1081 return OPENID2_PROVIDER; 1082 if ("original".equals(codeString)) 1083 return ORIGINAL; 1084 if ("P3Pv1".equals(codeString)) 1085 return P3PV1; 1086 if ("payment".equals(codeString)) 1087 return PAYMENT; 1088 if ("pingback".equals(codeString)) 1089 return PINGBACK; 1090 if ("preconnect".equals(codeString)) 1091 return PRECONNECT; 1092 if ("predecessor-version".equals(codeString)) 1093 return PREDECESSORVERSION; 1094 if ("prefetch".equals(codeString)) 1095 return PREFETCH; 1096 if ("preload".equals(codeString)) 1097 return PRELOAD; 1098 if ("prerender".equals(codeString)) 1099 return PRERENDER; 1100 if ("prev".equals(codeString)) 1101 return PREV; 1102 if ("preview".equals(codeString)) 1103 return PREVIEW; 1104 if ("previous".equals(codeString)) 1105 return PREVIOUS; 1106 if ("prev-archive".equals(codeString)) 1107 return PREVARCHIVE; 1108 if ("privacy-policy".equals(codeString)) 1109 return PRIVACYPOLICY; 1110 if ("profile".equals(codeString)) 1111 return PROFILE; 1112 if ("publication".equals(codeString)) 1113 return PUBLICATION; 1114 if ("related".equals(codeString)) 1115 return RELATED; 1116 if ("restconf".equals(codeString)) 1117 return RESTCONF; 1118 if ("replies".equals(codeString)) 1119 return REPLIES; 1120 if ("ruleinput".equals(codeString)) 1121 return RULEINPUT; 1122 if ("search".equals(codeString)) 1123 return SEARCH; 1124 if ("section".equals(codeString)) 1125 return SECTION; 1126 if ("self".equals(codeString)) 1127 return SELF; 1128 if ("service".equals(codeString)) 1129 return SERVICE; 1130 if ("service-desc".equals(codeString)) 1131 return SERVICEDESC; 1132 if ("service-doc".equals(codeString)) 1133 return SERVICEDOC; 1134 if ("service-meta".equals(codeString)) 1135 return SERVICEMETA; 1136 if ("sponsored".equals(codeString)) 1137 return SPONSORED; 1138 if ("start".equals(codeString)) 1139 return START; 1140 if ("status".equals(codeString)) 1141 return STATUS; 1142 if ("stylesheet".equals(codeString)) 1143 return STYLESHEET; 1144 if ("subsection".equals(codeString)) 1145 return SUBSECTION; 1146 if ("successor-version".equals(codeString)) 1147 return SUCCESSORVERSION; 1148 if ("sunset".equals(codeString)) 1149 return SUNSET; 1150 if ("tag".equals(codeString)) 1151 return TAG; 1152 if ("terms-of-service".equals(codeString)) 1153 return TERMSOFSERVICE; 1154 if ("timegate".equals(codeString)) 1155 return TIMEGATE; 1156 if ("timemap".equals(codeString)) 1157 return TIMEMAP; 1158 if ("type".equals(codeString)) 1159 return TYPE; 1160 if ("ugc".equals(codeString)) 1161 return UGC; 1162 if ("up".equals(codeString)) 1163 return UP; 1164 if ("version-history".equals(codeString)) 1165 return VERSIONHISTORY; 1166 if ("via".equals(codeString)) 1167 return VIA; 1168 if ("webmention".equals(codeString)) 1169 return WEBMENTION; 1170 if ("working-copy".equals(codeString)) 1171 return WORKINGCOPY; 1172 if ("working-copy-of".equals(codeString)) 1173 return WORKINGCOPYOF; 1174 if (Configuration.isAcceptInvalidEnums()) 1175 return null; 1176 else 1177 throw new FHIRException("Unknown LinkRelationTypes code '"+codeString+"'"); 1178 } 1179 public String toCode() { 1180 switch (this) { 1181 case ABOUT: return "about"; 1182 case ACL: return "acl"; 1183 case ALTERNATE: return "alternate"; 1184 case AMPHTML: return "amphtml"; 1185 case APPENDIX: return "appendix"; 1186 case APPLETOUCHICON: return "apple-touch-icon"; 1187 case APPLETOUCHSTARTUPIMAGE: return "apple-touch-startup-image"; 1188 case ARCHIVES: return "archives"; 1189 case AUTHOR: return "author"; 1190 case BLOCKEDBY: return "blocked-by"; 1191 case BOOKMARK: return "bookmark"; 1192 case CANONICAL: return "canonical"; 1193 case CHAPTER: return "chapter"; 1194 case CITEAS: return "cite-as"; 1195 case COLLECTION: return "collection"; 1196 case CONTENTS: return "contents"; 1197 case CONVERTEDFROM: return "convertedFrom"; 1198 case COPYRIGHT: return "copyright"; 1199 case CREATEFORM: return "create-form"; 1200 case CURRENT: return "current"; 1201 case DESCRIBEDBY: return "describedby"; 1202 case DESCRIBES: return "describes"; 1203 case DISCLOSURE: return "disclosure"; 1204 case DNSPREFETCH: return "dns-prefetch"; 1205 case DUPLICATE: return "duplicate"; 1206 case EDIT: return "edit"; 1207 case EDITFORM: return "edit-form"; 1208 case EDITMEDIA: return "edit-media"; 1209 case ENCLOSURE: return "enclosure"; 1210 case EXTERNAL: return "external"; 1211 case FIRST: return "first"; 1212 case GLOSSARY: return "glossary"; 1213 case HELP: return "help"; 1214 case HOSTS: return "hosts"; 1215 case HUB: return "hub"; 1216 case ICON: return "icon"; 1217 case INDEX: return "index"; 1218 case INTERVALAFTER: return "intervalAfter"; 1219 case INTERVALBEFORE: return "intervalBefore"; 1220 case INTERVALCONTAINS: return "intervalContains"; 1221 case INTERVALDISJOINT: return "intervalDisjoint"; 1222 case INTERVALDURING: return "intervalDuring"; 1223 case INTERVALEQUALS: return "intervalEquals"; 1224 case INTERVALFINISHEDBY: return "intervalFinishedBy"; 1225 case INTERVALFINISHES: return "intervalFinishes"; 1226 case INTERVALIN: return "intervalIn"; 1227 case INTERVALMEETS: return "intervalMeets"; 1228 case INTERVALMETBY: return "intervalMetBy"; 1229 case INTERVALOVERLAPPEDBY: return "intervalOverlappedBy"; 1230 case INTERVALOVERLAPS: return "intervalOverlaps"; 1231 case INTERVALSTARTEDBY: return "intervalStartedBy"; 1232 case INTERVALSTARTS: return "intervalStarts"; 1233 case ITEM: return "item"; 1234 case LAST: return "last"; 1235 case LATESTVERSION: return "latest-version"; 1236 case LICENSE: return "license"; 1237 case LINKSET: return "linkset"; 1238 case LRDD: return "lrdd"; 1239 case MANIFEST: return "manifest"; 1240 case MASKICON: return "mask-icon"; 1241 case MEDIAFEED: return "media-feed"; 1242 case MEMENTO: return "memento"; 1243 case MICROPUB: return "micropub"; 1244 case MODULEPRELOAD: return "modulepreload"; 1245 case MONITOR: return "monitor"; 1246 case MONITORGROUP: return "monitor-group"; 1247 case NEXT: return "next"; 1248 case NEXTARCHIVE: return "next-archive"; 1249 case NOFOLLOW: return "nofollow"; 1250 case NOOPENER: return "noopener"; 1251 case NOREFERRER: return "noreferrer"; 1252 case OPENER: return "opener"; 1253 case OPENID2_LOCALID: return "openid2.local_id"; 1254 case OPENID2_PROVIDER: return "openid2.provider"; 1255 case ORIGINAL: return "original"; 1256 case P3PV1: return "P3Pv1"; 1257 case PAYMENT: return "payment"; 1258 case PINGBACK: return "pingback"; 1259 case PRECONNECT: return "preconnect"; 1260 case PREDECESSORVERSION: return "predecessor-version"; 1261 case PREFETCH: return "prefetch"; 1262 case PRELOAD: return "preload"; 1263 case PRERENDER: return "prerender"; 1264 case PREV: return "prev"; 1265 case PREVIEW: return "preview"; 1266 case PREVIOUS: return "previous"; 1267 case PREVARCHIVE: return "prev-archive"; 1268 case PRIVACYPOLICY: return "privacy-policy"; 1269 case PROFILE: return "profile"; 1270 case PUBLICATION: return "publication"; 1271 case RELATED: return "related"; 1272 case RESTCONF: return "restconf"; 1273 case REPLIES: return "replies"; 1274 case RULEINPUT: return "ruleinput"; 1275 case SEARCH: return "search"; 1276 case SECTION: return "section"; 1277 case SELF: return "self"; 1278 case SERVICE: return "service"; 1279 case SERVICEDESC: return "service-desc"; 1280 case SERVICEDOC: return "service-doc"; 1281 case SERVICEMETA: return "service-meta"; 1282 case SPONSORED: return "sponsored"; 1283 case START: return "start"; 1284 case STATUS: return "status"; 1285 case STYLESHEET: return "stylesheet"; 1286 case SUBSECTION: return "subsection"; 1287 case SUCCESSORVERSION: return "successor-version"; 1288 case SUNSET: return "sunset"; 1289 case TAG: return "tag"; 1290 case TERMSOFSERVICE: return "terms-of-service"; 1291 case TIMEGATE: return "timegate"; 1292 case TIMEMAP: return "timemap"; 1293 case TYPE: return "type"; 1294 case UGC: return "ugc"; 1295 case UP: return "up"; 1296 case VERSIONHISTORY: return "version-history"; 1297 case VIA: return "via"; 1298 case WEBMENTION: return "webmention"; 1299 case WORKINGCOPY: return "working-copy"; 1300 case WORKINGCOPYOF: return "working-copy-of"; 1301 case NULL: return null; 1302 default: return "?"; 1303 } 1304 } 1305 public String getSystem() { 1306 switch (this) { 1307 case ABOUT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1308 case ACL: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1309 case ALTERNATE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1310 case AMPHTML: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1311 case APPENDIX: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1312 case APPLETOUCHICON: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1313 case APPLETOUCHSTARTUPIMAGE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1314 case ARCHIVES: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1315 case AUTHOR: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1316 case BLOCKEDBY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1317 case BOOKMARK: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1318 case CANONICAL: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1319 case CHAPTER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1320 case CITEAS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1321 case COLLECTION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1322 case CONTENTS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1323 case CONVERTEDFROM: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1324 case COPYRIGHT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1325 case CREATEFORM: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1326 case CURRENT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1327 case DESCRIBEDBY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1328 case DESCRIBES: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1329 case DISCLOSURE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1330 case DNSPREFETCH: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1331 case DUPLICATE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1332 case EDIT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1333 case EDITFORM: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1334 case EDITMEDIA: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1335 case ENCLOSURE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1336 case EXTERNAL: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1337 case FIRST: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1338 case GLOSSARY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1339 case HELP: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1340 case HOSTS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1341 case HUB: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1342 case ICON: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1343 case INDEX: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1344 case INTERVALAFTER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1345 case INTERVALBEFORE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1346 case INTERVALCONTAINS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1347 case INTERVALDISJOINT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1348 case INTERVALDURING: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1349 case INTERVALEQUALS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1350 case INTERVALFINISHEDBY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1351 case INTERVALFINISHES: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1352 case INTERVALIN: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1353 case INTERVALMEETS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1354 case INTERVALMETBY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1355 case INTERVALOVERLAPPEDBY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1356 case INTERVALOVERLAPS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1357 case INTERVALSTARTEDBY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1358 case INTERVALSTARTS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1359 case ITEM: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1360 case LAST: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1361 case LATESTVERSION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1362 case LICENSE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1363 case LINKSET: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1364 case LRDD: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1365 case MANIFEST: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1366 case MASKICON: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1367 case MEDIAFEED: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1368 case MEMENTO: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1369 case MICROPUB: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1370 case MODULEPRELOAD: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1371 case MONITOR: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1372 case MONITORGROUP: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1373 case NEXT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1374 case NEXTARCHIVE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1375 case NOFOLLOW: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1376 case NOOPENER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1377 case NOREFERRER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1378 case OPENER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1379 case OPENID2_LOCALID: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1380 case OPENID2_PROVIDER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1381 case ORIGINAL: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1382 case P3PV1: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1383 case PAYMENT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1384 case PINGBACK: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1385 case PRECONNECT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1386 case PREDECESSORVERSION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1387 case PREFETCH: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1388 case PRELOAD: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1389 case PRERENDER: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1390 case PREV: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1391 case PREVIEW: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1392 case PREVIOUS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1393 case PREVARCHIVE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1394 case PRIVACYPOLICY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1395 case PROFILE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1396 case PUBLICATION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1397 case RELATED: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1398 case RESTCONF: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1399 case REPLIES: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1400 case RULEINPUT: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1401 case SEARCH: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1402 case SECTION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1403 case SELF: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1404 case SERVICE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1405 case SERVICEDESC: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1406 case SERVICEDOC: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1407 case SERVICEMETA: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1408 case SPONSORED: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1409 case START: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1410 case STATUS: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1411 case STYLESHEET: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1412 case SUBSECTION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1413 case SUCCESSORVERSION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1414 case SUNSET: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1415 case TAG: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1416 case TERMSOFSERVICE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1417 case TIMEGATE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1418 case TIMEMAP: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1419 case TYPE: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1420 case UGC: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1421 case UP: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1422 case VERSIONHISTORY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1423 case VIA: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1424 case WEBMENTION: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1425 case WORKINGCOPY: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1426 case WORKINGCOPYOF: return "http://hl7.org/fhir/CodeSystem/iana-link-relations"; 1427 case NULL: return null; 1428 default: return "?"; 1429 } 1430 } 1431 public String getDefinition() { 1432 switch (this) { 1433 case ABOUT: return "Refers to a resource that is the subject of the link's context."; 1434 case ACL: return "Asserts that the link target provides an access control description for the link context."; 1435 case ALTERNATE: return "Refers to a substitute for this context"; 1436 case AMPHTML: return "Used to reference alternative content that uses the AMP profile of the HTML format."; 1437 case APPENDIX: return "Refers to an appendix."; 1438 case APPLETOUCHICON: return "Refers to an icon for the context. Synonym for icon."; 1439 case APPLETOUCHSTARTUPIMAGE: return "Refers to a launch screen for the context."; 1440 case ARCHIVES: return "Refers to a collection of records, documents, or other\n materials of historical interest."; 1441 case AUTHOR: return "Refers to the context's author."; 1442 case BLOCKEDBY: return "Identifies the entity that blocks access to a resource\n following receipt of a legal demand."; 1443 case BOOKMARK: return "Gives a permanent link to use for bookmarking purposes."; 1444 case CANONICAL: return "Designates the preferred version of a resource (the IRI and its contents)."; 1445 case CHAPTER: return "Refers to a chapter in a collection of resources."; 1446 case CITEAS: return "Indicates that the link target is preferred over the link context for the purpose of permanent citation."; 1447 case COLLECTION: return "The target IRI points to a resource which represents the collection resource for the context IRI."; 1448 case CONTENTS: return "Refers to a table of contents."; 1449 case CONVERTEDFROM: return "The document linked to was later converted to the\n document that contains this link relation. For example, an RFC can\n have a link to the Internet-Draft that became the RFC; in that case,\n the link relation would be \"convertedFrom\"."; 1450 case COPYRIGHT: return "Refers to a copyright statement that applies to the\n link's context."; 1451 case CREATEFORM: return "The target IRI points to a resource where a submission form can be obtained."; 1452 case CURRENT: return "Refers to a resource containing the most recent\n item(s) in a collection of resources."; 1453 case DESCRIBEDBY: return "Refers to a resource providing information about the\n link's context."; 1454 case DESCRIBES: return "The relationship A 'describes' B asserts that\n resource A provides a description of resource B. There are no\n constraints on the format or representation of either A or B,\n neither are there any further constraints on either resource."; 1455 case DISCLOSURE: return "Refers to a list of patent disclosures made with respect to \n material for which 'disclosure' relation is specified."; 1456 case DNSPREFETCH: return "Used to indicate an origin that will be used to fetch required \n resources for the link context, and that the user agent ought to resolve \n as early as possible."; 1457 case DUPLICATE: return "Refers to a resource whose available representations\n are byte-for-byte identical with the corresponding representations of\n the context IRI."; 1458 case EDIT: return "Refers to a resource that can be used to edit the\n link's context."; 1459 case EDITFORM: return "The target IRI points to a resource where a submission form for\n editing associated resource can be obtained."; 1460 case EDITMEDIA: return "Refers to a resource that can be used to edit media\n associated with the link's context."; 1461 case ENCLOSURE: return "Identifies a related resource that is potentially\n large and might require special handling."; 1462 case EXTERNAL: return "Refers to a resource that is not part of the same site as the current context."; 1463 case FIRST: return "An IRI that refers to the furthest preceding resource\n in a series of resources."; 1464 case GLOSSARY: return "Refers to a glossary of terms."; 1465 case HELP: return "Refers to context-sensitive help."; 1466 case HOSTS: return "Refers to a resource hosted by the server indicated by\n the link context."; 1467 case HUB: return "Refers to a hub that enables registration for\n notification of updates to the context."; 1468 case ICON: return "Refers to an icon representing the link's context."; 1469 case INDEX: return "Refers to an index."; 1470 case INTERVALAFTER: return "refers to a resource associated with a time interval that ends before the beginning of the time interval associated with the context resource"; 1471 case INTERVALBEFORE: return "refers to a resource associated with a time interval that begins after the end of the time interval associated with the context resource"; 1472 case INTERVALCONTAINS: return "refers to a resource associated with a time interval that begins after the beginning of the time interval associated with the context resource, and ends before the end of the time interval associated with the context resource"; 1473 case INTERVALDISJOINT: return "refers to a resource associated with a time interval that begins after the end of the time interval associated with the context resource, or ends before the beginning of the time interval associated with the context resource"; 1474 case INTERVALDURING: return "refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource"; 1475 case INTERVALEQUALS: return "refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource"; 1476 case INTERVALFINISHEDBY: return "refers to a resource associated with a time interval that begins after the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource"; 1477 case INTERVALFINISHES: return "refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource"; 1478 case INTERVALIN: return "refers to a resource associated with a time interval that begins before or is coincident with the beginning of the time interval associated with the context resource, and ends after or is coincident with the end of the time interval associated with the context resource"; 1479 case INTERVALMEETS: return "refers to a resource associated with a time interval whose beginning coincides with the end of the time interval associated with the context resource"; 1480 case INTERVALMETBY: return "refers to a resource associated with a time interval whose end coincides with the beginning of the time interval associated with the context resource"; 1481 case INTERVALOVERLAPPEDBY: return "refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and ends after the beginning of the time interval associated with the context resource"; 1482 case INTERVALOVERLAPS: return "refers to a resource associated with a time interval that begins before the end of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource"; 1483 case INTERVALSTARTEDBY: return "refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and ends before the end of the time interval associated with the context resource"; 1484 case INTERVALSTARTS: return "refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource"; 1485 case ITEM: return "The target IRI points to a resource that is a member of the collection represented by the context IRI."; 1486 case LAST: return "An IRI that refers to the furthest following resource\n in a series of resources."; 1487 case LATESTVERSION: return "Points to a resource containing the latest (e.g.,\n current) version of the context."; 1488 case LICENSE: return "Refers to a license associated with this context."; 1489 case LINKSET: return "The link target of a link with the \"linkset\" relation\n type provides a set of links, including links in which the link\n context of the link participates.\n "; 1490 case LRDD: return "Refers to further information about the link's context,\n expressed as a LRDD (\"Link-based Resource Descriptor Document\")\n resource. See for information about\n processing this relation type in host-meta documents. When used\n elsewhere, it refers to additional links and other metadata.\n Multiple instances indicate additional LRDD resources. LRDD\n resources MUST have an \"application/xrd+xml\" representation, and\n MAY have others."; 1491 case MANIFEST: return "Links to a manifest file for the context."; 1492 case MASKICON: return "Refers to a mask that can be applied to the icon for the context."; 1493 case MEDIAFEED: return "Refers to a feed of personalised media recommendations relevant to the link context."; 1494 case MEMENTO: return "The Target IRI points to a Memento, a fixed resource that will not change state anymore."; 1495 case MICROPUB: return "Links to the context's Micropub endpoint."; 1496 case MODULEPRELOAD: return "Refers to a module that the user agent is to preemptively fetch and store for use in the current context."; 1497 case MONITOR: return "Refers to a resource that can be used to monitor changes in an HTTP resource.\n "; 1498 case MONITORGROUP: return "Refers to a resource that can be used to monitor changes in a specified group of HTTP resources.\n "; 1499 case NEXT: return "Indicates that the link's context is a part of a series, and\n that the next in the series is the link target.\n "; 1500 case NEXTARCHIVE: return "Refers to the immediately following archive resource."; 1501 case NOFOLLOW: return "Indicates that the context?s original author or publisher does not endorse the link target."; 1502 case NOOPENER: return "Indicates that any newly created top-level browsing context which results from following the link will not be an auxiliary browsing context."; 1503 case NOREFERRER: return "Indicates that no referrer information is to be leaked when following the link."; 1504 case OPENER: return "Indicates that any newly created top-level browsing context which results from following the link will be an auxiliary browsing context."; 1505 case OPENID2_LOCALID: return "Refers to an OpenID Authentication server on which the context relies for an assertion that the end user controls an Identifier."; 1506 case OPENID2_PROVIDER: return "Refers to a resource which accepts OpenID Authentication protocol messages for the context."; 1507 case ORIGINAL: return "The Target IRI points to an Original Resource."; 1508 case P3PV1: return "Refers to a P3P privacy policy for the context."; 1509 case PAYMENT: return "Indicates a resource where payment is accepted."; 1510 case PINGBACK: return "Gives the address of the pingback resource for the link context."; 1511 case PRECONNECT: return "Used to indicate an origin that will be used to fetch required \n resources for the link context. Initiating an early connection, which \n includes the DNS lookup, TCP handshake, and optional TLS negotiation, \n allows the user agent to mask the high latency costs of establishing a \n connection."; 1512 case PREDECESSORVERSION: return "Points to a resource containing the predecessor\n version in the version history.\n "; 1513 case PREFETCH: return "The prefetch link relation type is used to identify a resource \n that might be required by the next navigation from the link context, and \n that the user agent ought to fetch, such that the user agent can deliver a \n faster response once the resource is requested in the future."; 1514 case PRELOAD: return "Refers to a resource that should be loaded early in the \n processing of the link's context, without blocking rendering."; 1515 case PRERENDER: return "Used to identify a resource that might be required by the next \n navigation from the link context, and that the user agent ought to fetch \n and execute, such that the user agent can deliver a faster response once \n the resource is requested in the future."; 1516 case PREV: return "Indicates that the link's context is a part of a series, and\n that the previous in the series is the link target.\n "; 1517 case PREVIEW: return "Refers to a resource that provides a preview of the link's context."; 1518 case PREVIOUS: return "Refers to the previous resource in an ordered series\n of resources. Synonym for \"prev\"."; 1519 case PREVARCHIVE: return "Refers to the immediately preceding archive resource."; 1520 case PRIVACYPOLICY: return "Refers to a privacy policy associated with the link's context."; 1521 case PROFILE: return "Identifying that a resource representation conforms\nto a certain profile, without affecting the non-profile semantics\nof the resource representation."; 1522 case PUBLICATION: return "Links to a publication manifest. A manifest represents \n structured information about a publication, such as informative metadata, \n a list of resources, and a default reading order."; 1523 case RELATED: return "Identifies a related resource."; 1524 case RESTCONF: return "Identifies the root of RESTCONF API as configured on this HTTP server.\n The \"restconf\" relation defines the root of the API defined in RFC8040.\n Subsequent revisions of RESTCONF will use alternate relation values to support \n protocol versioning."; 1525 case REPLIES: return "Identifies a resource that is a reply to the context\n of the link.\n "; 1526 case RULEINPUT: return "The resource identified by the link target provides an input value to an \n instance of a rule, where the resource which represents the rule instance is \n identified by the link context.\n "; 1527 case SEARCH: return "Refers to a resource that can be used to search through\n the link's context and related resources."; 1528 case SECTION: return "Refers to a section in a collection of resources."; 1529 case SELF: return "Conveys an identifier for the link's context.\n "; 1530 case SERVICE: return "Indicates a URI that can be used to retrieve a\n service document."; 1531 case SERVICEDESC: return "Identifies service description for the context that\n is primarily intended for consumption by machines."; 1532 case SERVICEDOC: return "Identifies service documentation for the context that\n is primarily intended for human consumption."; 1533 case SERVICEMETA: return "Identifies general metadata for the context that is\n primarily intended for consumption by machines."; 1534 case SPONSORED: return "Refers to a resource that is within a context that is \n sponsored (such as advertising or another compensation agreement)."; 1535 case START: return "Refers to the first resource in a collection of\n resources."; 1536 case STATUS: return "Identifies a resource that represents the context's\n status."; 1537 case STYLESHEET: return "Refers to a stylesheet."; 1538 case SUBSECTION: return "Refers to a resource serving as a subsection in a\n collection of resources."; 1539 case SUCCESSORVERSION: return "Points to a resource containing the successor version\n in the version history.\n "; 1540 case SUNSET: return "Identifies a resource that provides information about\n the context's retirement policy.\n "; 1541 case TAG: return "Gives a tag (identified by the given address) that applies to\n the current document.\n "; 1542 case TERMSOFSERVICE: return "Refers to the terms of service associated with the link's context."; 1543 case TIMEGATE: return "The Target IRI points to a TimeGate for an Original Resource."; 1544 case TIMEMAP: return "The Target IRI points to a TimeMap for an Original Resource."; 1545 case TYPE: return "Refers to a resource identifying the abstract semantic type of which the link's context is considered to be an instance."; 1546 case UGC: return "Refers to a resource that is within a context that is User Generated Content.\n "; 1547 case UP: return "Refers to a parent document in a hierarchy of\n documents.\n "; 1548 case VERSIONHISTORY: return "Points to a resource containing the version history\n for the context.\n "; 1549 case VIA: return "Identifies a resource that is the source of the\n information in the link's context.\n "; 1550 case WEBMENTION: return "Identifies a target URI that supports the Webmention protocol.\n This allows clients that mention a resource in some form of publishing process\n to contact that endpoint and inform it that this resource has been mentioned."; 1551 case WORKINGCOPY: return "Points to a working copy for this resource."; 1552 case WORKINGCOPYOF: return "Points to the versioned resource from which this\n working copy was obtained.\n "; 1553 case NULL: return null; 1554 default: return "?"; 1555 } 1556 } 1557 public String getDisplay() { 1558 switch (this) { 1559 case ABOUT: return "Refers to a resource that is the subject of the link's context."; 1560 case ACL: return "Asserts that the link target provides an access control description for the link context."; 1561 case ALTERNATE: return "Refers to a substitute for this context"; 1562 case AMPHTML: return "Used to reference alternative content that uses the AMP profile of the HTML format."; 1563 case APPENDIX: return "Refers to an appendix."; 1564 case APPLETOUCHICON: return "Refers to an icon for the context. Synonym for icon."; 1565 case APPLETOUCHSTARTUPIMAGE: return "Refers to a launch screen for the context."; 1566 case ARCHIVES: return "Refers to a collection of records, documents, or other\n materials of historical interest."; 1567 case AUTHOR: return "Refers to the context's author."; 1568 case BLOCKEDBY: return "Identifies the entity that blocks access to a resource\n following receipt of a legal demand."; 1569 case BOOKMARK: return "Gives a permanent link to use for bookmarking purposes."; 1570 case CANONICAL: return "Designates the preferred version of a resource (the IRI and its contents)."; 1571 case CHAPTER: return "Refers to a chapter in a collection of resources."; 1572 case CITEAS: return "Indicates that the link target is preferred over the link context for the purpose of permanent citation."; 1573 case COLLECTION: return "The target IRI points to a resource which represents the collection resource for the context IRI."; 1574 case CONTENTS: return "Refers to a table of contents."; 1575 case CONVERTEDFROM: return "The document linked to was later converted to the\n document that contains this link relation. For example, an RFC can\n have a link to the Internet-Draft that became the RFC; in that case,\n the link relation would be \"convertedFrom\"."; 1576 case COPYRIGHT: return "Refers to a copyright statement that applies to the\n link's context."; 1577 case CREATEFORM: return "The target IRI points to a resource where a submission form can be obtained."; 1578 case CURRENT: return "Refers to a resource containing the most recent\n item(s) in a collection of resources."; 1579 case DESCRIBEDBY: return "Refers to a resource providing information about the\n link's context."; 1580 case DESCRIBES: return "The relationship A 'describes' B asserts that\n resource A provides a description of resource B. There are no\n constraints on the format or representation of either A or B,\n neither are there any further constraints on either resource."; 1581 case DISCLOSURE: return "Refers to a list of patent disclosures made with respect to \n material for which 'disclosure' relation is specified."; 1582 case DNSPREFETCH: return "Used to indicate an origin that will be used to fetch required \n resources for the link context, and that the user agent ought to resolve \n as early as possible."; 1583 case DUPLICATE: return "Refers to a resource whose available representations\n are byte-for-byte identical with the corresponding representations of\n the context IRI."; 1584 case EDIT: return "Refers to a resource that can be used to edit the\n link's context."; 1585 case EDITFORM: return "The target IRI points to a resource where a submission form for\n editing associated resource can be obtained."; 1586 case EDITMEDIA: return "Refers to a resource that can be used to edit media\n associated with the link's context."; 1587 case ENCLOSURE: return "Identifies a related resource that is potentially\n large and might require special handling."; 1588 case EXTERNAL: return "Refers to a resource that is not part of the same site as the current context."; 1589 case FIRST: return "An IRI that refers to the furthest preceding resource\n in a series of resources."; 1590 case GLOSSARY: return "Refers to a glossary of terms."; 1591 case HELP: return "Refers to context-sensitive help."; 1592 case HOSTS: return "Refers to a resource hosted by the server indicated by\n the link context."; 1593 case HUB: return "Refers to a hub that enables registration for\n notification of updates to the context."; 1594 case ICON: return "Refers to an icon representing the link's context."; 1595 case INDEX: return "Refers to an index."; 1596 case INTERVALAFTER: return "refers to a resource associated with a time interval that ends before the beginning of the time interval associated with the context resource"; 1597 case INTERVALBEFORE: return "refers to a resource associated with a time interval that begins after the end of the time interval associated with the context resource"; 1598 case INTERVALCONTAINS: return "refers to a resource associated with a time interval that begins after the beginning of the time interval associated with the context resource, and ends before the end of the time interval associated with the context resource"; 1599 case INTERVALDISJOINT: return "refers to a resource associated with a time interval that begins after the end of the time interval associated with the context resource, or ends before the beginning of the time interval associated with the context resource"; 1600 case INTERVALDURING: return "refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource"; 1601 case INTERVALEQUALS: return "refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource"; 1602 case INTERVALFINISHEDBY: return "refers to a resource associated with a time interval that begins after the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource"; 1603 case INTERVALFINISHES: return "refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and whose end coincides with the end of the time interval associated with the context resource"; 1604 case INTERVALIN: return "refers to a resource associated with a time interval that begins before or is coincident with the beginning of the time interval associated with the context resource, and ends after or is coincident with the end of the time interval associated with the context resource"; 1605 case INTERVALMEETS: return "refers to a resource associated with a time interval whose beginning coincides with the end of the time interval associated with the context resource"; 1606 case INTERVALMETBY: return "refers to a resource associated with a time interval whose end coincides with the beginning of the time interval associated with the context resource"; 1607 case INTERVALOVERLAPPEDBY: return "refers to a resource associated with a time interval that begins before the beginning of the time interval associated with the context resource, and ends after the beginning of the time interval associated with the context resource"; 1608 case INTERVALOVERLAPS: return "refers to a resource associated with a time interval that begins before the end of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource"; 1609 case INTERVALSTARTEDBY: return "refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and ends before the end of the time interval associated with the context resource"; 1610 case INTERVALSTARTS: return "refers to a resource associated with a time interval whose beginning coincides with the beginning of the time interval associated with the context resource, and ends after the end of the time interval associated with the context resource"; 1611 case ITEM: return "The target IRI points to a resource that is a member of the collection represented by the context IRI."; 1612 case LAST: return "An IRI that refers to the furthest following resource\n in a series of resources."; 1613 case LATESTVERSION: return "Points to a resource containing the latest (e.g.,\n current) version of the context."; 1614 case LICENSE: return "Refers to a license associated with this context."; 1615 case LINKSET: return "The link target of a link with the \"linkset\" relation\n type provides a set of links, including links in which the link\n context of the link participates.\n "; 1616 case LRDD: return "Refers to further information about the link's context,\n expressed as a LRDD (\"Link-based Resource Descriptor Document\")\n resource. See for information about\n processing this relation type in host-meta documents. When used\n elsewhere, it refers to additional links and other metadata.\n Multiple instances indicate additional LRDD resources. LRDD\n resources MUST have an \"application/xrd+xml\" representation, and\n MAY have others."; 1617 case MANIFEST: return "Links to a manifest file for the context."; 1618 case MASKICON: return "Refers to a mask that can be applied to the icon for the context."; 1619 case MEDIAFEED: return "Refers to a feed of personalised media recommendations relevant to the link context."; 1620 case MEMENTO: return "The Target IRI points to a Memento, a fixed resource that will not change state anymore."; 1621 case MICROPUB: return "Links to the context's Micropub endpoint."; 1622 case MODULEPRELOAD: return "Refers to a module that the user agent is to preemptively fetch and store for use in the current context."; 1623 case MONITOR: return "Refers to a resource that can be used to monitor changes in an HTTP resource.\n "; 1624 case MONITORGROUP: return "Refers to a resource that can be used to monitor changes in a specified group of HTTP resources.\n "; 1625 case NEXT: return "Indicates that the link's context is a part of a series, and\n that the next in the series is the link target.\n "; 1626 case NEXTARCHIVE: return "Refers to the immediately following archive resource."; 1627 case NOFOLLOW: return "Indicates that the context?s original author or publisher does not endorse the link target."; 1628 case NOOPENER: return "Indicates that any newly created top-level browsing context which results from following the link will not be an auxiliary browsing context."; 1629 case NOREFERRER: return "Indicates that no referrer information is to be leaked when following the link."; 1630 case OPENER: return "Indicates that any newly created top-level browsing context which results from following the link will be an auxiliary browsing context."; 1631 case OPENID2_LOCALID: return "Refers to an OpenID Authentication server on which the context relies for an assertion that the end user controls an Identifier."; 1632 case OPENID2_PROVIDER: return "Refers to a resource which accepts OpenID Authentication protocol messages for the context."; 1633 case ORIGINAL: return "The Target IRI points to an Original Resource."; 1634 case P3PV1: return "Refers to a P3P privacy policy for the context."; 1635 case PAYMENT: return "Indicates a resource where payment is accepted."; 1636 case PINGBACK: return "Gives the address of the pingback resource for the link context."; 1637 case PRECONNECT: return "Used to indicate an origin that will be used to fetch required \n resources for the link context. Initiating an early connection, which \n includes the DNS lookup, TCP handshake, and optional TLS negotiation, \n allows the user agent to mask the high latency costs of establishing a \n connection."; 1638 case PREDECESSORVERSION: return "Points to a resource containing the predecessor\n version in the version history.\n "; 1639 case PREFETCH: return "The prefetch link relation type is used to identify a resource \n that might be required by the next navigation from the link context, and \n that the user agent ought to fetch, such that the user agent can deliver a \n faster response once the resource is requested in the future."; 1640 case PRELOAD: return "Refers to a resource that should be loaded early in the \n processing of the link's context, without blocking rendering."; 1641 case PRERENDER: return "Used to identify a resource that might be required by the next \n navigation from the link context, and that the user agent ought to fetch \n and execute, such that the user agent can deliver a faster response once \n the resource is requested in the future."; 1642 case PREV: return "Indicates that the link's context is a part of a series, and\n that the previous in the series is the link target.\n "; 1643 case PREVIEW: return "Refers to a resource that provides a preview of the link's context."; 1644 case PREVIOUS: return "Refers to the previous resource in an ordered series\n of resources. Synonym for \"prev\"."; 1645 case PREVARCHIVE: return "Refers to the immediately preceding archive resource."; 1646 case PRIVACYPOLICY: return "Refers to a privacy policy associated with the link's context."; 1647 case PROFILE: return "Identifying that a resource representation conforms\nto a certain profile, without affecting the non-profile semantics\nof the resource representation."; 1648 case PUBLICATION: return "Links to a publication manifest. A manifest represents \n structured information about a publication, such as informative metadata, \n a list of resources, and a default reading order."; 1649 case RELATED: return "Identifies a related resource."; 1650 case RESTCONF: return "Identifies the root of RESTCONF API as configured on this HTTP server.\n The \"restconf\" relation defines the root of the API defined in RFC8040.\n Subsequent revisions of RESTCONF will use alternate relation values to support \n protocol versioning."; 1651 case REPLIES: return "Identifies a resource that is a reply to the context\n of the link.\n "; 1652 case RULEINPUT: return "The resource identified by the link target provides an input value to an \n instance of a rule, where the resource which represents the rule instance is \n identified by the link context.\n "; 1653 case SEARCH: return "Refers to a resource that can be used to search through\n the link's context and related resources."; 1654 case SECTION: return "Refers to a section in a collection of resources."; 1655 case SELF: return "Conveys an identifier for the link's context.\n "; 1656 case SERVICE: return "Indicates a URI that can be used to retrieve a\n service document."; 1657 case SERVICEDESC: return "Identifies service description for the context that\n is primarily intended for consumption by machines."; 1658 case SERVICEDOC: return "Identifies service documentation for the context that\n is primarily intended for human consumption."; 1659 case SERVICEMETA: return "Identifies general metadata for the context that is\n primarily intended for consumption by machines."; 1660 case SPONSORED: return "Refers to a resource that is within a context that is \n sponsored (such as advertising or another compensation agreement)."; 1661 case START: return "Refers to the first resource in a collection of\n resources."; 1662 case STATUS: return "Identifies a resource that represents the context's\n status."; 1663 case STYLESHEET: return "Refers to a stylesheet."; 1664 case SUBSECTION: return "Refers to a resource serving as a subsection in a\n collection of resources."; 1665 case SUCCESSORVERSION: return "Points to a resource containing the successor version\n in the version history.\n "; 1666 case SUNSET: return "Identifies a resource that provides information about\n the context's retirement policy.\n "; 1667 case TAG: return "Gives a tag (identified by the given address) that applies to\n the current document.\n "; 1668 case TERMSOFSERVICE: return "Refers to the terms of service associated with the link's context."; 1669 case TIMEGATE: return "The Target IRI points to a TimeGate for an Original Resource."; 1670 case TIMEMAP: return "The Target IRI points to a TimeMap for an Original Resource."; 1671 case TYPE: return "Refers to a resource identifying the abstract semantic type of which the link's context is considered to be an instance."; 1672 case UGC: return "Refers to a resource that is within a context that is User Generated Content.\n "; 1673 case UP: return "Refers to a parent document in a hierarchy of\n documents.\n "; 1674 case VERSIONHISTORY: return "Points to a resource containing the version history\n for the context.\n "; 1675 case VIA: return "Identifies a resource that is the source of the\n information in the link's context.\n "; 1676 case WEBMENTION: return "Identifies a target URI that supports the Webmention protocol.\n This allows clients that mention a resource in some form of publishing process\n to contact that endpoint and inform it that this resource has been mentioned."; 1677 case WORKINGCOPY: return "Points to a working copy for this resource."; 1678 case WORKINGCOPYOF: return "Points to the versioned resource from which this\n working copy was obtained.\n "; 1679 case NULL: return null; 1680 default: return "?"; 1681 } 1682 } 1683 } 1684 1685 public static class LinkRelationTypesEnumFactory implements EnumFactory<LinkRelationTypes> { 1686 public LinkRelationTypes fromCode(String codeString) throws IllegalArgumentException { 1687 if (codeString == null || "".equals(codeString)) 1688 if (codeString == null || "".equals(codeString)) 1689 return null; 1690 if ("about".equals(codeString)) 1691 return LinkRelationTypes.ABOUT; 1692 if ("acl".equals(codeString)) 1693 return LinkRelationTypes.ACL; 1694 if ("alternate".equals(codeString)) 1695 return LinkRelationTypes.ALTERNATE; 1696 if ("amphtml".equals(codeString)) 1697 return LinkRelationTypes.AMPHTML; 1698 if ("appendix".equals(codeString)) 1699 return LinkRelationTypes.APPENDIX; 1700 if ("apple-touch-icon".equals(codeString)) 1701 return LinkRelationTypes.APPLETOUCHICON; 1702 if ("apple-touch-startup-image".equals(codeString)) 1703 return LinkRelationTypes.APPLETOUCHSTARTUPIMAGE; 1704 if ("archives".equals(codeString)) 1705 return LinkRelationTypes.ARCHIVES; 1706 if ("author".equals(codeString)) 1707 return LinkRelationTypes.AUTHOR; 1708 if ("blocked-by".equals(codeString)) 1709 return LinkRelationTypes.BLOCKEDBY; 1710 if ("bookmark".equals(codeString)) 1711 return LinkRelationTypes.BOOKMARK; 1712 if ("canonical".equals(codeString)) 1713 return LinkRelationTypes.CANONICAL; 1714 if ("chapter".equals(codeString)) 1715 return LinkRelationTypes.CHAPTER; 1716 if ("cite-as".equals(codeString)) 1717 return LinkRelationTypes.CITEAS; 1718 if ("collection".equals(codeString)) 1719 return LinkRelationTypes.COLLECTION; 1720 if ("contents".equals(codeString)) 1721 return LinkRelationTypes.CONTENTS; 1722 if ("convertedFrom".equals(codeString)) 1723 return LinkRelationTypes.CONVERTEDFROM; 1724 if ("copyright".equals(codeString)) 1725 return LinkRelationTypes.COPYRIGHT; 1726 if ("create-form".equals(codeString)) 1727 return LinkRelationTypes.CREATEFORM; 1728 if ("current".equals(codeString)) 1729 return LinkRelationTypes.CURRENT; 1730 if ("describedby".equals(codeString)) 1731 return LinkRelationTypes.DESCRIBEDBY; 1732 if ("describes".equals(codeString)) 1733 return LinkRelationTypes.DESCRIBES; 1734 if ("disclosure".equals(codeString)) 1735 return LinkRelationTypes.DISCLOSURE; 1736 if ("dns-prefetch".equals(codeString)) 1737 return LinkRelationTypes.DNSPREFETCH; 1738 if ("duplicate".equals(codeString)) 1739 return LinkRelationTypes.DUPLICATE; 1740 if ("edit".equals(codeString)) 1741 return LinkRelationTypes.EDIT; 1742 if ("edit-form".equals(codeString)) 1743 return LinkRelationTypes.EDITFORM; 1744 if ("edit-media".equals(codeString)) 1745 return LinkRelationTypes.EDITMEDIA; 1746 if ("enclosure".equals(codeString)) 1747 return LinkRelationTypes.ENCLOSURE; 1748 if ("external".equals(codeString)) 1749 return LinkRelationTypes.EXTERNAL; 1750 if ("first".equals(codeString)) 1751 return LinkRelationTypes.FIRST; 1752 if ("glossary".equals(codeString)) 1753 return LinkRelationTypes.GLOSSARY; 1754 if ("help".equals(codeString)) 1755 return LinkRelationTypes.HELP; 1756 if ("hosts".equals(codeString)) 1757 return LinkRelationTypes.HOSTS; 1758 if ("hub".equals(codeString)) 1759 return LinkRelationTypes.HUB; 1760 if ("icon".equals(codeString)) 1761 return LinkRelationTypes.ICON; 1762 if ("index".equals(codeString)) 1763 return LinkRelationTypes.INDEX; 1764 if ("intervalAfter".equals(codeString)) 1765 return LinkRelationTypes.INTERVALAFTER; 1766 if ("intervalBefore".equals(codeString)) 1767 return LinkRelationTypes.INTERVALBEFORE; 1768 if ("intervalContains".equals(codeString)) 1769 return LinkRelationTypes.INTERVALCONTAINS; 1770 if ("intervalDisjoint".equals(codeString)) 1771 return LinkRelationTypes.INTERVALDISJOINT; 1772 if ("intervalDuring".equals(codeString)) 1773 return LinkRelationTypes.INTERVALDURING; 1774 if ("intervalEquals".equals(codeString)) 1775 return LinkRelationTypes.INTERVALEQUALS; 1776 if ("intervalFinishedBy".equals(codeString)) 1777 return LinkRelationTypes.INTERVALFINISHEDBY; 1778 if ("intervalFinishes".equals(codeString)) 1779 return LinkRelationTypes.INTERVALFINISHES; 1780 if ("intervalIn".equals(codeString)) 1781 return LinkRelationTypes.INTERVALIN; 1782 if ("intervalMeets".equals(codeString)) 1783 return LinkRelationTypes.INTERVALMEETS; 1784 if ("intervalMetBy".equals(codeString)) 1785 return LinkRelationTypes.INTERVALMETBY; 1786 if ("intervalOverlappedBy".equals(codeString)) 1787 return LinkRelationTypes.INTERVALOVERLAPPEDBY; 1788 if ("intervalOverlaps".equals(codeString)) 1789 return LinkRelationTypes.INTERVALOVERLAPS; 1790 if ("intervalStartedBy".equals(codeString)) 1791 return LinkRelationTypes.INTERVALSTARTEDBY; 1792 if ("intervalStarts".equals(codeString)) 1793 return LinkRelationTypes.INTERVALSTARTS; 1794 if ("item".equals(codeString)) 1795 return LinkRelationTypes.ITEM; 1796 if ("last".equals(codeString)) 1797 return LinkRelationTypes.LAST; 1798 if ("latest-version".equals(codeString)) 1799 return LinkRelationTypes.LATESTVERSION; 1800 if ("license".equals(codeString)) 1801 return LinkRelationTypes.LICENSE; 1802 if ("linkset".equals(codeString)) 1803 return LinkRelationTypes.LINKSET; 1804 if ("lrdd".equals(codeString)) 1805 return LinkRelationTypes.LRDD; 1806 if ("manifest".equals(codeString)) 1807 return LinkRelationTypes.MANIFEST; 1808 if ("mask-icon".equals(codeString)) 1809 return LinkRelationTypes.MASKICON; 1810 if ("media-feed".equals(codeString)) 1811 return LinkRelationTypes.MEDIAFEED; 1812 if ("memento".equals(codeString)) 1813 return LinkRelationTypes.MEMENTO; 1814 if ("micropub".equals(codeString)) 1815 return LinkRelationTypes.MICROPUB; 1816 if ("modulepreload".equals(codeString)) 1817 return LinkRelationTypes.MODULEPRELOAD; 1818 if ("monitor".equals(codeString)) 1819 return LinkRelationTypes.MONITOR; 1820 if ("monitor-group".equals(codeString)) 1821 return LinkRelationTypes.MONITORGROUP; 1822 if ("next".equals(codeString)) 1823 return LinkRelationTypes.NEXT; 1824 if ("next-archive".equals(codeString)) 1825 return LinkRelationTypes.NEXTARCHIVE; 1826 if ("nofollow".equals(codeString)) 1827 return LinkRelationTypes.NOFOLLOW; 1828 if ("noopener".equals(codeString)) 1829 return LinkRelationTypes.NOOPENER; 1830 if ("noreferrer".equals(codeString)) 1831 return LinkRelationTypes.NOREFERRER; 1832 if ("opener".equals(codeString)) 1833 return LinkRelationTypes.OPENER; 1834 if ("openid2.local_id".equals(codeString)) 1835 return LinkRelationTypes.OPENID2_LOCALID; 1836 if ("openid2.provider".equals(codeString)) 1837 return LinkRelationTypes.OPENID2_PROVIDER; 1838 if ("original".equals(codeString)) 1839 return LinkRelationTypes.ORIGINAL; 1840 if ("P3Pv1".equals(codeString)) 1841 return LinkRelationTypes.P3PV1; 1842 if ("payment".equals(codeString)) 1843 return LinkRelationTypes.PAYMENT; 1844 if ("pingback".equals(codeString)) 1845 return LinkRelationTypes.PINGBACK; 1846 if ("preconnect".equals(codeString)) 1847 return LinkRelationTypes.PRECONNECT; 1848 if ("predecessor-version".equals(codeString)) 1849 return LinkRelationTypes.PREDECESSORVERSION; 1850 if ("prefetch".equals(codeString)) 1851 return LinkRelationTypes.PREFETCH; 1852 if ("preload".equals(codeString)) 1853 return LinkRelationTypes.PRELOAD; 1854 if ("prerender".equals(codeString)) 1855 return LinkRelationTypes.PRERENDER; 1856 if ("prev".equals(codeString)) 1857 return LinkRelationTypes.PREV; 1858 if ("preview".equals(codeString)) 1859 return LinkRelationTypes.PREVIEW; 1860 if ("previous".equals(codeString)) 1861 return LinkRelationTypes.PREVIOUS; 1862 if ("prev-archive".equals(codeString)) 1863 return LinkRelationTypes.PREVARCHIVE; 1864 if ("privacy-policy".equals(codeString)) 1865 return LinkRelationTypes.PRIVACYPOLICY; 1866 if ("profile".equals(codeString)) 1867 return LinkRelationTypes.PROFILE; 1868 if ("publication".equals(codeString)) 1869 return LinkRelationTypes.PUBLICATION; 1870 if ("related".equals(codeString)) 1871 return LinkRelationTypes.RELATED; 1872 if ("restconf".equals(codeString)) 1873 return LinkRelationTypes.RESTCONF; 1874 if ("replies".equals(codeString)) 1875 return LinkRelationTypes.REPLIES; 1876 if ("ruleinput".equals(codeString)) 1877 return LinkRelationTypes.RULEINPUT; 1878 if ("search".equals(codeString)) 1879 return LinkRelationTypes.SEARCH; 1880 if ("section".equals(codeString)) 1881 return LinkRelationTypes.SECTION; 1882 if ("self".equals(codeString)) 1883 return LinkRelationTypes.SELF; 1884 if ("service".equals(codeString)) 1885 return LinkRelationTypes.SERVICE; 1886 if ("service-desc".equals(codeString)) 1887 return LinkRelationTypes.SERVICEDESC; 1888 if ("service-doc".equals(codeString)) 1889 return LinkRelationTypes.SERVICEDOC; 1890 if ("service-meta".equals(codeString)) 1891 return LinkRelationTypes.SERVICEMETA; 1892 if ("sponsored".equals(codeString)) 1893 return LinkRelationTypes.SPONSORED; 1894 if ("start".equals(codeString)) 1895 return LinkRelationTypes.START; 1896 if ("status".equals(codeString)) 1897 return LinkRelationTypes.STATUS; 1898 if ("stylesheet".equals(codeString)) 1899 return LinkRelationTypes.STYLESHEET; 1900 if ("subsection".equals(codeString)) 1901 return LinkRelationTypes.SUBSECTION; 1902 if ("successor-version".equals(codeString)) 1903 return LinkRelationTypes.SUCCESSORVERSION; 1904 if ("sunset".equals(codeString)) 1905 return LinkRelationTypes.SUNSET; 1906 if ("tag".equals(codeString)) 1907 return LinkRelationTypes.TAG; 1908 if ("terms-of-service".equals(codeString)) 1909 return LinkRelationTypes.TERMSOFSERVICE; 1910 if ("timegate".equals(codeString)) 1911 return LinkRelationTypes.TIMEGATE; 1912 if ("timemap".equals(codeString)) 1913 return LinkRelationTypes.TIMEMAP; 1914 if ("type".equals(codeString)) 1915 return LinkRelationTypes.TYPE; 1916 if ("ugc".equals(codeString)) 1917 return LinkRelationTypes.UGC; 1918 if ("up".equals(codeString)) 1919 return LinkRelationTypes.UP; 1920 if ("version-history".equals(codeString)) 1921 return LinkRelationTypes.VERSIONHISTORY; 1922 if ("via".equals(codeString)) 1923 return LinkRelationTypes.VIA; 1924 if ("webmention".equals(codeString)) 1925 return LinkRelationTypes.WEBMENTION; 1926 if ("working-copy".equals(codeString)) 1927 return LinkRelationTypes.WORKINGCOPY; 1928 if ("working-copy-of".equals(codeString)) 1929 return LinkRelationTypes.WORKINGCOPYOF; 1930 throw new IllegalArgumentException("Unknown LinkRelationTypes code '"+codeString+"'"); 1931 } 1932 public Enumeration<LinkRelationTypes> fromType(PrimitiveType<?> code) throws FHIRException { 1933 if (code == null) 1934 return null; 1935 if (code.isEmpty()) 1936 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NULL, code); 1937 String codeString = ((PrimitiveType) code).asStringValue(); 1938 if (codeString == null || "".equals(codeString)) 1939 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NULL, code); 1940 if ("about".equals(codeString)) 1941 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ABOUT, code); 1942 if ("acl".equals(codeString)) 1943 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ACL, code); 1944 if ("alternate".equals(codeString)) 1945 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ALTERNATE, code); 1946 if ("amphtml".equals(codeString)) 1947 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.AMPHTML, code); 1948 if ("appendix".equals(codeString)) 1949 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.APPENDIX, code); 1950 if ("apple-touch-icon".equals(codeString)) 1951 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.APPLETOUCHICON, code); 1952 if ("apple-touch-startup-image".equals(codeString)) 1953 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.APPLETOUCHSTARTUPIMAGE, code); 1954 if ("archives".equals(codeString)) 1955 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ARCHIVES, code); 1956 if ("author".equals(codeString)) 1957 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.AUTHOR, code); 1958 if ("blocked-by".equals(codeString)) 1959 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.BLOCKEDBY, code); 1960 if ("bookmark".equals(codeString)) 1961 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.BOOKMARK, code); 1962 if ("canonical".equals(codeString)) 1963 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CANONICAL, code); 1964 if ("chapter".equals(codeString)) 1965 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CHAPTER, code); 1966 if ("cite-as".equals(codeString)) 1967 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CITEAS, code); 1968 if ("collection".equals(codeString)) 1969 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.COLLECTION, code); 1970 if ("contents".equals(codeString)) 1971 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CONTENTS, code); 1972 if ("convertedFrom".equals(codeString)) 1973 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CONVERTEDFROM, code); 1974 if ("copyright".equals(codeString)) 1975 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.COPYRIGHT, code); 1976 if ("create-form".equals(codeString)) 1977 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CREATEFORM, code); 1978 if ("current".equals(codeString)) 1979 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.CURRENT, code); 1980 if ("describedby".equals(codeString)) 1981 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.DESCRIBEDBY, code); 1982 if ("describes".equals(codeString)) 1983 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.DESCRIBES, code); 1984 if ("disclosure".equals(codeString)) 1985 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.DISCLOSURE, code); 1986 if ("dns-prefetch".equals(codeString)) 1987 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.DNSPREFETCH, code); 1988 if ("duplicate".equals(codeString)) 1989 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.DUPLICATE, code); 1990 if ("edit".equals(codeString)) 1991 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.EDIT, code); 1992 if ("edit-form".equals(codeString)) 1993 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.EDITFORM, code); 1994 if ("edit-media".equals(codeString)) 1995 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.EDITMEDIA, code); 1996 if ("enclosure".equals(codeString)) 1997 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ENCLOSURE, code); 1998 if ("external".equals(codeString)) 1999 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.EXTERNAL, code); 2000 if ("first".equals(codeString)) 2001 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.FIRST, code); 2002 if ("glossary".equals(codeString)) 2003 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.GLOSSARY, code); 2004 if ("help".equals(codeString)) 2005 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.HELP, code); 2006 if ("hosts".equals(codeString)) 2007 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.HOSTS, code); 2008 if ("hub".equals(codeString)) 2009 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.HUB, code); 2010 if ("icon".equals(codeString)) 2011 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ICON, code); 2012 if ("index".equals(codeString)) 2013 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INDEX, code); 2014 if ("intervalAfter".equals(codeString)) 2015 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALAFTER, code); 2016 if ("intervalBefore".equals(codeString)) 2017 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALBEFORE, code); 2018 if ("intervalContains".equals(codeString)) 2019 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALCONTAINS, code); 2020 if ("intervalDisjoint".equals(codeString)) 2021 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALDISJOINT, code); 2022 if ("intervalDuring".equals(codeString)) 2023 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALDURING, code); 2024 if ("intervalEquals".equals(codeString)) 2025 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALEQUALS, code); 2026 if ("intervalFinishedBy".equals(codeString)) 2027 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALFINISHEDBY, code); 2028 if ("intervalFinishes".equals(codeString)) 2029 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALFINISHES, code); 2030 if ("intervalIn".equals(codeString)) 2031 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALIN, code); 2032 if ("intervalMeets".equals(codeString)) 2033 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALMEETS, code); 2034 if ("intervalMetBy".equals(codeString)) 2035 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALMETBY, code); 2036 if ("intervalOverlappedBy".equals(codeString)) 2037 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALOVERLAPPEDBY, code); 2038 if ("intervalOverlaps".equals(codeString)) 2039 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALOVERLAPS, code); 2040 if ("intervalStartedBy".equals(codeString)) 2041 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALSTARTEDBY, code); 2042 if ("intervalStarts".equals(codeString)) 2043 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.INTERVALSTARTS, code); 2044 if ("item".equals(codeString)) 2045 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ITEM, code); 2046 if ("last".equals(codeString)) 2047 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.LAST, code); 2048 if ("latest-version".equals(codeString)) 2049 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.LATESTVERSION, code); 2050 if ("license".equals(codeString)) 2051 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.LICENSE, code); 2052 if ("linkset".equals(codeString)) 2053 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.LINKSET, code); 2054 if ("lrdd".equals(codeString)) 2055 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.LRDD, code); 2056 if ("manifest".equals(codeString)) 2057 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MANIFEST, code); 2058 if ("mask-icon".equals(codeString)) 2059 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MASKICON, code); 2060 if ("media-feed".equals(codeString)) 2061 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MEDIAFEED, code); 2062 if ("memento".equals(codeString)) 2063 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MEMENTO, code); 2064 if ("micropub".equals(codeString)) 2065 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MICROPUB, code); 2066 if ("modulepreload".equals(codeString)) 2067 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MODULEPRELOAD, code); 2068 if ("monitor".equals(codeString)) 2069 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MONITOR, code); 2070 if ("monitor-group".equals(codeString)) 2071 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.MONITORGROUP, code); 2072 if ("next".equals(codeString)) 2073 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NEXT, code); 2074 if ("next-archive".equals(codeString)) 2075 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NEXTARCHIVE, code); 2076 if ("nofollow".equals(codeString)) 2077 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NOFOLLOW, code); 2078 if ("noopener".equals(codeString)) 2079 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NOOPENER, code); 2080 if ("noreferrer".equals(codeString)) 2081 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.NOREFERRER, code); 2082 if ("opener".equals(codeString)) 2083 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.OPENER, code); 2084 if ("openid2.local_id".equals(codeString)) 2085 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.OPENID2_LOCALID, code); 2086 if ("openid2.provider".equals(codeString)) 2087 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.OPENID2_PROVIDER, code); 2088 if ("original".equals(codeString)) 2089 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.ORIGINAL, code); 2090 if ("P3Pv1".equals(codeString)) 2091 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.P3PV1, code); 2092 if ("payment".equals(codeString)) 2093 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PAYMENT, code); 2094 if ("pingback".equals(codeString)) 2095 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PINGBACK, code); 2096 if ("preconnect".equals(codeString)) 2097 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PRECONNECT, code); 2098 if ("predecessor-version".equals(codeString)) 2099 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PREDECESSORVERSION, code); 2100 if ("prefetch".equals(codeString)) 2101 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PREFETCH, code); 2102 if ("preload".equals(codeString)) 2103 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PRELOAD, code); 2104 if ("prerender".equals(codeString)) 2105 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PRERENDER, code); 2106 if ("prev".equals(codeString)) 2107 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PREV, code); 2108 if ("preview".equals(codeString)) 2109 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PREVIEW, code); 2110 if ("previous".equals(codeString)) 2111 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PREVIOUS, code); 2112 if ("prev-archive".equals(codeString)) 2113 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PREVARCHIVE, code); 2114 if ("privacy-policy".equals(codeString)) 2115 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PRIVACYPOLICY, code); 2116 if ("profile".equals(codeString)) 2117 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PROFILE, code); 2118 if ("publication".equals(codeString)) 2119 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.PUBLICATION, code); 2120 if ("related".equals(codeString)) 2121 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.RELATED, code); 2122 if ("restconf".equals(codeString)) 2123 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.RESTCONF, code); 2124 if ("replies".equals(codeString)) 2125 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.REPLIES, code); 2126 if ("ruleinput".equals(codeString)) 2127 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.RULEINPUT, code); 2128 if ("search".equals(codeString)) 2129 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SEARCH, code); 2130 if ("section".equals(codeString)) 2131 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SECTION, code); 2132 if ("self".equals(codeString)) 2133 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SELF, code); 2134 if ("service".equals(codeString)) 2135 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SERVICE, code); 2136 if ("service-desc".equals(codeString)) 2137 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SERVICEDESC, code); 2138 if ("service-doc".equals(codeString)) 2139 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SERVICEDOC, code); 2140 if ("service-meta".equals(codeString)) 2141 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SERVICEMETA, code); 2142 if ("sponsored".equals(codeString)) 2143 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SPONSORED, code); 2144 if ("start".equals(codeString)) 2145 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.START, code); 2146 if ("status".equals(codeString)) 2147 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.STATUS, code); 2148 if ("stylesheet".equals(codeString)) 2149 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.STYLESHEET, code); 2150 if ("subsection".equals(codeString)) 2151 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SUBSECTION, code); 2152 if ("successor-version".equals(codeString)) 2153 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SUCCESSORVERSION, code); 2154 if ("sunset".equals(codeString)) 2155 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.SUNSET, code); 2156 if ("tag".equals(codeString)) 2157 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.TAG, code); 2158 if ("terms-of-service".equals(codeString)) 2159 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.TERMSOFSERVICE, code); 2160 if ("timegate".equals(codeString)) 2161 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.TIMEGATE, code); 2162 if ("timemap".equals(codeString)) 2163 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.TIMEMAP, code); 2164 if ("type".equals(codeString)) 2165 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.TYPE, code); 2166 if ("ugc".equals(codeString)) 2167 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.UGC, code); 2168 if ("up".equals(codeString)) 2169 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.UP, code); 2170 if ("version-history".equals(codeString)) 2171 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.VERSIONHISTORY, code); 2172 if ("via".equals(codeString)) 2173 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.VIA, code); 2174 if ("webmention".equals(codeString)) 2175 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.WEBMENTION, code); 2176 if ("working-copy".equals(codeString)) 2177 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.WORKINGCOPY, code); 2178 if ("working-copy-of".equals(codeString)) 2179 return new Enumeration<LinkRelationTypes>(this, LinkRelationTypes.WORKINGCOPYOF, code); 2180 throw new FHIRException("Unknown LinkRelationTypes code '"+codeString+"'"); 2181 } 2182 public String toCode(LinkRelationTypes code) { 2183 if (code == LinkRelationTypes.NULL) 2184 return null; 2185 if (code == LinkRelationTypes.ABOUT) 2186 return "about"; 2187 if (code == LinkRelationTypes.ACL) 2188 return "acl"; 2189 if (code == LinkRelationTypes.ALTERNATE) 2190 return "alternate"; 2191 if (code == LinkRelationTypes.AMPHTML) 2192 return "amphtml"; 2193 if (code == LinkRelationTypes.APPENDIX) 2194 return "appendix"; 2195 if (code == LinkRelationTypes.APPLETOUCHICON) 2196 return "apple-touch-icon"; 2197 if (code == LinkRelationTypes.APPLETOUCHSTARTUPIMAGE) 2198 return "apple-touch-startup-image"; 2199 if (code == LinkRelationTypes.ARCHIVES) 2200 return "archives"; 2201 if (code == LinkRelationTypes.AUTHOR) 2202 return "author"; 2203 if (code == LinkRelationTypes.BLOCKEDBY) 2204 return "blocked-by"; 2205 if (code == LinkRelationTypes.BOOKMARK) 2206 return "bookmark"; 2207 if (code == LinkRelationTypes.CANONICAL) 2208 return "canonical"; 2209 if (code == LinkRelationTypes.CHAPTER) 2210 return "chapter"; 2211 if (code == LinkRelationTypes.CITEAS) 2212 return "cite-as"; 2213 if (code == LinkRelationTypes.COLLECTION) 2214 return "collection"; 2215 if (code == LinkRelationTypes.CONTENTS) 2216 return "contents"; 2217 if (code == LinkRelationTypes.CONVERTEDFROM) 2218 return "convertedFrom"; 2219 if (code == LinkRelationTypes.COPYRIGHT) 2220 return "copyright"; 2221 if (code == LinkRelationTypes.CREATEFORM) 2222 return "create-form"; 2223 if (code == LinkRelationTypes.CURRENT) 2224 return "current"; 2225 if (code == LinkRelationTypes.DESCRIBEDBY) 2226 return "describedby"; 2227 if (code == LinkRelationTypes.DESCRIBES) 2228 return "describes"; 2229 if (code == LinkRelationTypes.DISCLOSURE) 2230 return "disclosure"; 2231 if (code == LinkRelationTypes.DNSPREFETCH) 2232 return "dns-prefetch"; 2233 if (code == LinkRelationTypes.DUPLICATE) 2234 return "duplicate"; 2235 if (code == LinkRelationTypes.EDIT) 2236 return "edit"; 2237 if (code == LinkRelationTypes.EDITFORM) 2238 return "edit-form"; 2239 if (code == LinkRelationTypes.EDITMEDIA) 2240 return "edit-media"; 2241 if (code == LinkRelationTypes.ENCLOSURE) 2242 return "enclosure"; 2243 if (code == LinkRelationTypes.EXTERNAL) 2244 return "external"; 2245 if (code == LinkRelationTypes.FIRST) 2246 return "first"; 2247 if (code == LinkRelationTypes.GLOSSARY) 2248 return "glossary"; 2249 if (code == LinkRelationTypes.HELP) 2250 return "help"; 2251 if (code == LinkRelationTypes.HOSTS) 2252 return "hosts"; 2253 if (code == LinkRelationTypes.HUB) 2254 return "hub"; 2255 if (code == LinkRelationTypes.ICON) 2256 return "icon"; 2257 if (code == LinkRelationTypes.INDEX) 2258 return "index"; 2259 if (code == LinkRelationTypes.INTERVALAFTER) 2260 return "intervalAfter"; 2261 if (code == LinkRelationTypes.INTERVALBEFORE) 2262 return "intervalBefore"; 2263 if (code == LinkRelationTypes.INTERVALCONTAINS) 2264 return "intervalContains"; 2265 if (code == LinkRelationTypes.INTERVALDISJOINT) 2266 return "intervalDisjoint"; 2267 if (code == LinkRelationTypes.INTERVALDURING) 2268 return "intervalDuring"; 2269 if (code == LinkRelationTypes.INTERVALEQUALS) 2270 return "intervalEquals"; 2271 if (code == LinkRelationTypes.INTERVALFINISHEDBY) 2272 return "intervalFinishedBy"; 2273 if (code == LinkRelationTypes.INTERVALFINISHES) 2274 return "intervalFinishes"; 2275 if (code == LinkRelationTypes.INTERVALIN) 2276 return "intervalIn"; 2277 if (code == LinkRelationTypes.INTERVALMEETS) 2278 return "intervalMeets"; 2279 if (code == LinkRelationTypes.INTERVALMETBY) 2280 return "intervalMetBy"; 2281 if (code == LinkRelationTypes.INTERVALOVERLAPPEDBY) 2282 return "intervalOverlappedBy"; 2283 if (code == LinkRelationTypes.INTERVALOVERLAPS) 2284 return "intervalOverlaps"; 2285 if (code == LinkRelationTypes.INTERVALSTARTEDBY) 2286 return "intervalStartedBy"; 2287 if (code == LinkRelationTypes.INTERVALSTARTS) 2288 return "intervalStarts"; 2289 if (code == LinkRelationTypes.ITEM) 2290 return "item"; 2291 if (code == LinkRelationTypes.LAST) 2292 return "last"; 2293 if (code == LinkRelationTypes.LATESTVERSION) 2294 return "latest-version"; 2295 if (code == LinkRelationTypes.LICENSE) 2296 return "license"; 2297 if (code == LinkRelationTypes.LINKSET) 2298 return "linkset"; 2299 if (code == LinkRelationTypes.LRDD) 2300 return "lrdd"; 2301 if (code == LinkRelationTypes.MANIFEST) 2302 return "manifest"; 2303 if (code == LinkRelationTypes.MASKICON) 2304 return "mask-icon"; 2305 if (code == LinkRelationTypes.MEDIAFEED) 2306 return "media-feed"; 2307 if (code == LinkRelationTypes.MEMENTO) 2308 return "memento"; 2309 if (code == LinkRelationTypes.MICROPUB) 2310 return "micropub"; 2311 if (code == LinkRelationTypes.MODULEPRELOAD) 2312 return "modulepreload"; 2313 if (code == LinkRelationTypes.MONITOR) 2314 return "monitor"; 2315 if (code == LinkRelationTypes.MONITORGROUP) 2316 return "monitor-group"; 2317 if (code == LinkRelationTypes.NEXT) 2318 return "next"; 2319 if (code == LinkRelationTypes.NEXTARCHIVE) 2320 return "next-archive"; 2321 if (code == LinkRelationTypes.NOFOLLOW) 2322 return "nofollow"; 2323 if (code == LinkRelationTypes.NOOPENER) 2324 return "noopener"; 2325 if (code == LinkRelationTypes.NOREFERRER) 2326 return "noreferrer"; 2327 if (code == LinkRelationTypes.OPENER) 2328 return "opener"; 2329 if (code == LinkRelationTypes.OPENID2_LOCALID) 2330 return "openid2.local_id"; 2331 if (code == LinkRelationTypes.OPENID2_PROVIDER) 2332 return "openid2.provider"; 2333 if (code == LinkRelationTypes.ORIGINAL) 2334 return "original"; 2335 if (code == LinkRelationTypes.P3PV1) 2336 return "P3Pv1"; 2337 if (code == LinkRelationTypes.PAYMENT) 2338 return "payment"; 2339 if (code == LinkRelationTypes.PINGBACK) 2340 return "pingback"; 2341 if (code == LinkRelationTypes.PRECONNECT) 2342 return "preconnect"; 2343 if (code == LinkRelationTypes.PREDECESSORVERSION) 2344 return "predecessor-version"; 2345 if (code == LinkRelationTypes.PREFETCH) 2346 return "prefetch"; 2347 if (code == LinkRelationTypes.PRELOAD) 2348 return "preload"; 2349 if (code == LinkRelationTypes.PRERENDER) 2350 return "prerender"; 2351 if (code == LinkRelationTypes.PREV) 2352 return "prev"; 2353 if (code == LinkRelationTypes.PREVIEW) 2354 return "preview"; 2355 if (code == LinkRelationTypes.PREVIOUS) 2356 return "previous"; 2357 if (code == LinkRelationTypes.PREVARCHIVE) 2358 return "prev-archive"; 2359 if (code == LinkRelationTypes.PRIVACYPOLICY) 2360 return "privacy-policy"; 2361 if (code == LinkRelationTypes.PROFILE) 2362 return "profile"; 2363 if (code == LinkRelationTypes.PUBLICATION) 2364 return "publication"; 2365 if (code == LinkRelationTypes.RELATED) 2366 return "related"; 2367 if (code == LinkRelationTypes.RESTCONF) 2368 return "restconf"; 2369 if (code == LinkRelationTypes.REPLIES) 2370 return "replies"; 2371 if (code == LinkRelationTypes.RULEINPUT) 2372 return "ruleinput"; 2373 if (code == LinkRelationTypes.SEARCH) 2374 return "search"; 2375 if (code == LinkRelationTypes.SECTION) 2376 return "section"; 2377 if (code == LinkRelationTypes.SELF) 2378 return "self"; 2379 if (code == LinkRelationTypes.SERVICE) 2380 return "service"; 2381 if (code == LinkRelationTypes.SERVICEDESC) 2382 return "service-desc"; 2383 if (code == LinkRelationTypes.SERVICEDOC) 2384 return "service-doc"; 2385 if (code == LinkRelationTypes.SERVICEMETA) 2386 return "service-meta"; 2387 if (code == LinkRelationTypes.SPONSORED) 2388 return "sponsored"; 2389 if (code == LinkRelationTypes.START) 2390 return "start"; 2391 if (code == LinkRelationTypes.STATUS) 2392 return "status"; 2393 if (code == LinkRelationTypes.STYLESHEET) 2394 return "stylesheet"; 2395 if (code == LinkRelationTypes.SUBSECTION) 2396 return "subsection"; 2397 if (code == LinkRelationTypes.SUCCESSORVERSION) 2398 return "successor-version"; 2399 if (code == LinkRelationTypes.SUNSET) 2400 return "sunset"; 2401 if (code == LinkRelationTypes.TAG) 2402 return "tag"; 2403 if (code == LinkRelationTypes.TERMSOFSERVICE) 2404 return "terms-of-service"; 2405 if (code == LinkRelationTypes.TIMEGATE) 2406 return "timegate"; 2407 if (code == LinkRelationTypes.TIMEMAP) 2408 return "timemap"; 2409 if (code == LinkRelationTypes.TYPE) 2410 return "type"; 2411 if (code == LinkRelationTypes.UGC) 2412 return "ugc"; 2413 if (code == LinkRelationTypes.UP) 2414 return "up"; 2415 if (code == LinkRelationTypes.VERSIONHISTORY) 2416 return "version-history"; 2417 if (code == LinkRelationTypes.VIA) 2418 return "via"; 2419 if (code == LinkRelationTypes.WEBMENTION) 2420 return "webmention"; 2421 if (code == LinkRelationTypes.WORKINGCOPY) 2422 return "working-copy"; 2423 if (code == LinkRelationTypes.WORKINGCOPYOF) 2424 return "working-copy-of"; 2425 return "?"; 2426 } 2427 public String toSystem(LinkRelationTypes code) { 2428 return code.getSystem(); 2429 } 2430 } 2431 2432 public enum SearchEntryMode { 2433 /** 2434 * This resource matched the search specification. 2435 */ 2436 MATCH, 2437 /** 2438 * This resource is returned because it is referred to from another resource in the search set. 2439 */ 2440 INCLUDE, 2441 /** 2442 * An OperationOutcome that provides additional information about the processing of a search. 2443 */ 2444 OUTCOME, 2445 /** 2446 * added to help the parsers with the generic types 2447 */ 2448 NULL; 2449 public static SearchEntryMode fromCode(String codeString) throws FHIRException { 2450 if (codeString == null || "".equals(codeString)) 2451 return null; 2452 if ("match".equals(codeString)) 2453 return MATCH; 2454 if ("include".equals(codeString)) 2455 return INCLUDE; 2456 if ("outcome".equals(codeString)) 2457 return OUTCOME; 2458 if (Configuration.isAcceptInvalidEnums()) 2459 return null; 2460 else 2461 throw new FHIRException("Unknown SearchEntryMode code '"+codeString+"'"); 2462 } 2463 public String toCode() { 2464 switch (this) { 2465 case MATCH: return "match"; 2466 case INCLUDE: return "include"; 2467 case OUTCOME: return "outcome"; 2468 case NULL: return null; 2469 default: return "?"; 2470 } 2471 } 2472 public String getSystem() { 2473 switch (this) { 2474 case MATCH: return "http://hl7.org/fhir/search-entry-mode"; 2475 case INCLUDE: return "http://hl7.org/fhir/search-entry-mode"; 2476 case OUTCOME: return "http://hl7.org/fhir/search-entry-mode"; 2477 case NULL: return null; 2478 default: return "?"; 2479 } 2480 } 2481 public String getDefinition() { 2482 switch (this) { 2483 case MATCH: return "This resource matched the search specification."; 2484 case INCLUDE: return "This resource is returned because it is referred to from another resource in the search set."; 2485 case OUTCOME: return "An OperationOutcome that provides additional information about the processing of a search."; 2486 case NULL: return null; 2487 default: return "?"; 2488 } 2489 } 2490 public String getDisplay() { 2491 switch (this) { 2492 case MATCH: return "Match"; 2493 case INCLUDE: return "Include"; 2494 case OUTCOME: return "Outcome"; 2495 case NULL: return null; 2496 default: return "?"; 2497 } 2498 } 2499 } 2500 2501 public static class SearchEntryModeEnumFactory implements EnumFactory<SearchEntryMode> { 2502 public SearchEntryMode fromCode(String codeString) throws IllegalArgumentException { 2503 if (codeString == null || "".equals(codeString)) 2504 if (codeString == null || "".equals(codeString)) 2505 return null; 2506 if ("match".equals(codeString)) 2507 return SearchEntryMode.MATCH; 2508 if ("include".equals(codeString)) 2509 return SearchEntryMode.INCLUDE; 2510 if ("outcome".equals(codeString)) 2511 return SearchEntryMode.OUTCOME; 2512 throw new IllegalArgumentException("Unknown SearchEntryMode code '"+codeString+"'"); 2513 } 2514 public Enumeration<SearchEntryMode> fromType(PrimitiveType<?> code) throws FHIRException { 2515 if (code == null) 2516 return null; 2517 if (code.isEmpty()) 2518 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.NULL, code); 2519 String codeString = ((PrimitiveType) code).asStringValue(); 2520 if (codeString == null || "".equals(codeString)) 2521 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.NULL, code); 2522 if ("match".equals(codeString)) 2523 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.MATCH, code); 2524 if ("include".equals(codeString)) 2525 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.INCLUDE, code); 2526 if ("outcome".equals(codeString)) 2527 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.OUTCOME, code); 2528 throw new FHIRException("Unknown SearchEntryMode code '"+codeString+"'"); 2529 } 2530 public String toCode(SearchEntryMode code) { 2531 if (code == SearchEntryMode.NULL) 2532 return null; 2533 if (code == SearchEntryMode.MATCH) 2534 return "match"; 2535 if (code == SearchEntryMode.INCLUDE) 2536 return "include"; 2537 if (code == SearchEntryMode.OUTCOME) 2538 return "outcome"; 2539 return "?"; 2540 } 2541 public String toSystem(SearchEntryMode code) { 2542 return code.getSystem(); 2543 } 2544 } 2545 2546 @Block() 2547 public static class BundleLinkComponent extends BackboneElement implements IBaseBackboneElement { 2548 /** 2549 * A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 2550 */ 2551 @Child(name = "relation", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2552 @Description(shortDefinition="See http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1", formalDefinition="A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1)." ) 2553 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/iana-link-relations") 2554 protected Enumeration<LinkRelationTypes> relation; 2555 2556 /** 2557 * The reference details for the link. 2558 */ 2559 @Child(name = "url", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 2560 @Description(shortDefinition="Reference details for the link", formalDefinition="The reference details for the link." ) 2561 protected UriType url; 2562 2563 private static final long serialVersionUID = -878418349L; 2564 2565 /** 2566 * Constructor 2567 */ 2568 public BundleLinkComponent() { 2569 super(); 2570 } 2571 2572 /** 2573 * Constructor 2574 */ 2575 public BundleLinkComponent(LinkRelationTypes relation, String url) { 2576 super(); 2577 this.setRelation(relation); 2578 this.setUrl(url); 2579 } 2580 2581 /** 2582 * @return {@link #relation} (A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).). This is the underlying object with id, value and extensions. The accessor "getRelation" gives direct access to the value 2583 */ 2584 public Enumeration<LinkRelationTypes> getRelationElement() { 2585 if (this.relation == null) 2586 if (Configuration.errorOnAutoCreate()) 2587 throw new Error("Attempt to auto-create BundleLinkComponent.relation"); 2588 else if (Configuration.doAutoCreate()) 2589 this.relation = new Enumeration<LinkRelationTypes>(new LinkRelationTypesEnumFactory()); // bb 2590 return this.relation; 2591 } 2592 2593 public boolean hasRelationElement() { 2594 return this.relation != null && !this.relation.isEmpty(); 2595 } 2596 2597 public boolean hasRelation() { 2598 return this.relation != null && !this.relation.isEmpty(); 2599 } 2600 2601 /** 2602 * @param value {@link #relation} (A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).). This is the underlying object with id, value and extensions. The accessor "getRelation" gives direct access to the value 2603 */ 2604 public BundleLinkComponent setRelationElement(Enumeration<LinkRelationTypes> value) { 2605 this.relation = value; 2606 return this; 2607 } 2608 2609 /** 2610 * @return A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 2611 */ 2612 public LinkRelationTypes getRelation() { 2613 return this.relation == null ? null : this.relation.getValue(); 2614 } 2615 2616 /** 2617 * @param value A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1). 2618 */ 2619 public BundleLinkComponent setRelation(LinkRelationTypes value) { 2620 if (this.relation == null) 2621 this.relation = new Enumeration<LinkRelationTypes>(new LinkRelationTypesEnumFactory()); 2622 this.relation.setValue(value); 2623 return this; 2624 } 2625 2626 /** 2627 * @return {@link #url} (The reference details for the link.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2628 */ 2629 public UriType getUrlElement() { 2630 if (this.url == null) 2631 if (Configuration.errorOnAutoCreate()) 2632 throw new Error("Attempt to auto-create BundleLinkComponent.url"); 2633 else if (Configuration.doAutoCreate()) 2634 this.url = new UriType(); // bb 2635 return this.url; 2636 } 2637 2638 public boolean hasUrlElement() { 2639 return this.url != null && !this.url.isEmpty(); 2640 } 2641 2642 public boolean hasUrl() { 2643 return this.url != null && !this.url.isEmpty(); 2644 } 2645 2646 /** 2647 * @param value {@link #url} (The reference details for the link.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2648 */ 2649 public BundleLinkComponent setUrlElement(UriType value) { 2650 this.url = value; 2651 return this; 2652 } 2653 2654 /** 2655 * @return The reference details for the link. 2656 */ 2657 public String getUrl() { 2658 return this.url == null ? null : this.url.getValue(); 2659 } 2660 2661 /** 2662 * @param value The reference details for the link. 2663 */ 2664 public BundleLinkComponent setUrl(String value) { 2665 if (this.url == null) 2666 this.url = new UriType(); 2667 this.url.setValue(value); 2668 return this; 2669 } 2670 2671 protected void listChildren(List<Property> children) { 2672 super.listChildren(children); 2673 children.add(new Property("relation", "code", "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).", 0, 1, relation)); 2674 children.add(new Property("url", "uri", "The reference details for the link.", 0, 1, url)); 2675 } 2676 2677 @Override 2678 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2679 switch (_hash) { 2680 case -554436100: /*relation*/ return new Property("relation", "code", "A name which details the functional use for this link - see [http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1](http://www.iana.org/assignments/link-relations/link-relations.xhtml#link-relations-1).", 0, 1, relation); 2681 case 116079: /*url*/ return new Property("url", "uri", "The reference details for the link.", 0, 1, url); 2682 default: return super.getNamedProperty(_hash, _name, _checkValid); 2683 } 2684 2685 } 2686 2687 @Override 2688 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2689 switch (hash) { 2690 case -554436100: /*relation*/ return this.relation == null ? new Base[0] : new Base[] {this.relation}; // Enumeration<LinkRelationTypes> 2691 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2692 default: return super.getProperty(hash, name, checkValid); 2693 } 2694 2695 } 2696 2697 @Override 2698 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2699 switch (hash) { 2700 case -554436100: // relation 2701 value = new LinkRelationTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2702 this.relation = (Enumeration) value; // Enumeration<LinkRelationTypes> 2703 return value; 2704 case 116079: // url 2705 this.url = TypeConvertor.castToUri(value); // UriType 2706 return value; 2707 default: return super.setProperty(hash, name, value); 2708 } 2709 2710 } 2711 2712 @Override 2713 public Base setProperty(String name, Base value) throws FHIRException { 2714 if (name.equals("relation")) { 2715 value = new LinkRelationTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2716 this.relation = (Enumeration) value; // Enumeration<LinkRelationTypes> 2717 } else if (name.equals("url")) { 2718 this.url = TypeConvertor.castToUri(value); // UriType 2719 } else 2720 return super.setProperty(name, value); 2721 return value; 2722 } 2723 2724 @Override 2725 public void removeChild(String name, Base value) throws FHIRException { 2726 if (name.equals("relation")) { 2727 value = new LinkRelationTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2728 this.relation = (Enumeration) value; // Enumeration<LinkRelationTypes> 2729 } else if (name.equals("url")) { 2730 this.url = null; 2731 } else 2732 super.removeChild(name, value); 2733 2734 } 2735 2736 @Override 2737 public Base makeProperty(int hash, String name) throws FHIRException { 2738 switch (hash) { 2739 case -554436100: return getRelationElement(); 2740 case 116079: return getUrlElement(); 2741 default: return super.makeProperty(hash, name); 2742 } 2743 2744 } 2745 2746 @Override 2747 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2748 switch (hash) { 2749 case -554436100: /*relation*/ return new String[] {"code"}; 2750 case 116079: /*url*/ return new String[] {"uri"}; 2751 default: return super.getTypesForProperty(hash, name); 2752 } 2753 2754 } 2755 2756 @Override 2757 public Base addChild(String name) throws FHIRException { 2758 if (name.equals("relation")) { 2759 throw new FHIRException("Cannot call addChild on a singleton property Bundle.link.relation"); 2760 } 2761 else if (name.equals("url")) { 2762 throw new FHIRException("Cannot call addChild on a singleton property Bundle.link.url"); 2763 } 2764 else 2765 return super.addChild(name); 2766 } 2767 2768 public BundleLinkComponent copy() { 2769 BundleLinkComponent dst = new BundleLinkComponent(); 2770 copyValues(dst); 2771 return dst; 2772 } 2773 2774 public void copyValues(BundleLinkComponent dst) { 2775 super.copyValues(dst); 2776 dst.relation = relation == null ? null : relation.copy(); 2777 dst.url = url == null ? null : url.copy(); 2778 } 2779 2780 @Override 2781 public boolean equalsDeep(Base other_) { 2782 if (!super.equalsDeep(other_)) 2783 return false; 2784 if (!(other_ instanceof BundleLinkComponent)) 2785 return false; 2786 BundleLinkComponent o = (BundleLinkComponent) other_; 2787 return compareDeep(relation, o.relation, true) && compareDeep(url, o.url, true); 2788 } 2789 2790 @Override 2791 public boolean equalsShallow(Base other_) { 2792 if (!super.equalsShallow(other_)) 2793 return false; 2794 if (!(other_ instanceof BundleLinkComponent)) 2795 return false; 2796 BundleLinkComponent o = (BundleLinkComponent) other_; 2797 return compareValues(relation, o.relation, true) && compareValues(url, o.url, true); 2798 } 2799 2800 public boolean isEmpty() { 2801 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relation, url); 2802 } 2803 2804 public String fhirType() { 2805 return "Bundle.link"; 2806 2807 } 2808 2809 } 2810 2811 @Block() 2812 public static class BundleEntryComponent extends BackboneElement implements IBaseBackboneElement { 2813 /** 2814 * A series of links that provide context to this entry. 2815 */ 2816 @Child(name = "link", type = {BundleLinkComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2817 @Description(shortDefinition="Links related to this entry", formalDefinition="A series of links that provide context to this entry." ) 2818 protected List<BundleLinkComponent> link; 2819 2820 /** 2821 * The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: 2822* invoking a create 2823* invoking or responding to an operation where the body is not a single identified resource 2824* invoking or returning the results of a search or history operation. 2825 */ 2826 @Child(name = "fullUrl", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2827 @Description(shortDefinition="URI for resource (e.g. the absolute URL server address, URI for UUID/OID, etc.)", formalDefinition="The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: \n* invoking a create\n* invoking or responding to an operation where the body is not a single identified resource\n* invoking or returning the results of a search or history operation." ) 2828 protected UriType fullUrl; 2829 2830 /** 2831 * The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type. This is allowed to be a Parameters resource if and only if it is referenced by something else within the Bundle that provides context/meaning. 2832 */ 2833 @Child(name = "resource", type = {Resource.class}, order=3, min=0, max=1, modifier=false, summary=true) 2834 @Description(shortDefinition="A resource in the bundle", formalDefinition="The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type. This is allowed to be a Parameters resource if and only if it is referenced by something else within the Bundle that provides context/meaning." ) 2835 protected Resource resource; 2836 2837 /** 2838 * Information about the search process that lead to the creation of this entry. 2839 */ 2840 @Child(name = "search", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 2841 @Description(shortDefinition="Search related information", formalDefinition="Information about the search process that lead to the creation of this entry." ) 2842 protected BundleEntrySearchComponent search; 2843 2844 /** 2845 * Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry. 2846 */ 2847 @Child(name = "request", type = {}, order=5, min=0, max=1, modifier=false, summary=true) 2848 @Description(shortDefinition="Additional execution information (transaction/batch/history)", formalDefinition="Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry." ) 2849 protected BundleEntryRequestComponent request; 2850 2851 /** 2852 * Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history. 2853 */ 2854 @Child(name = "response", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 2855 @Description(shortDefinition="Results of execution (transaction/batch/history)", formalDefinition="Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history." ) 2856 protected BundleEntryResponseComponent response; 2857 2858 private static final long serialVersionUID = 517783054L; 2859 2860 /** 2861 * Constructor 2862 */ 2863 public BundleEntryComponent() { 2864 super(); 2865 } 2866 2867 /** 2868 * @return {@link #link} (A series of links that provide context to this entry.) 2869 */ 2870 public List<BundleLinkComponent> getLink() { 2871 if (this.link == null) 2872 this.link = new ArrayList<BundleLinkComponent>(); 2873 return this.link; 2874 } 2875 2876 /** 2877 * @return Returns a reference to <code>this</code> for easy method chaining 2878 */ 2879 public BundleEntryComponent setLink(List<BundleLinkComponent> theLink) { 2880 this.link = theLink; 2881 return this; 2882 } 2883 2884 public boolean hasLink() { 2885 if (this.link == null) 2886 return false; 2887 for (BundleLinkComponent item : this.link) 2888 if (!item.isEmpty()) 2889 return true; 2890 return false; 2891 } 2892 2893 public BundleLinkComponent addLink() { //3 2894 BundleLinkComponent t = new BundleLinkComponent(); 2895 if (this.link == null) 2896 this.link = new ArrayList<BundleLinkComponent>(); 2897 this.link.add(t); 2898 return t; 2899 } 2900 2901 public BundleEntryComponent addLink(BundleLinkComponent t) { //3 2902 if (t == null) 2903 return this; 2904 if (this.link == null) 2905 this.link = new ArrayList<BundleLinkComponent>(); 2906 this.link.add(t); 2907 return this; 2908 } 2909 2910 /** 2911 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 2912 */ 2913 public BundleLinkComponent getLinkFirstRep() { 2914 if (getLink().isEmpty()) { 2915 addLink(); 2916 } 2917 return getLink().get(0); 2918 } 2919 2920 /** 2921 * @return {@link #fullUrl} (The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: 2922* invoking a create 2923* invoking or responding to an operation where the body is not a single identified resource 2924* invoking or returning the results of a search or history operation.). This is the underlying object with id, value and extensions. The accessor "getFullUrl" gives direct access to the value 2925 */ 2926 public UriType getFullUrlElement() { 2927 if (this.fullUrl == null) 2928 if (Configuration.errorOnAutoCreate()) 2929 throw new Error("Attempt to auto-create BundleEntryComponent.fullUrl"); 2930 else if (Configuration.doAutoCreate()) 2931 this.fullUrl = new UriType(); // bb 2932 return this.fullUrl; 2933 } 2934 2935 public boolean hasFullUrlElement() { 2936 return this.fullUrl != null && !this.fullUrl.isEmpty(); 2937 } 2938 2939 public boolean hasFullUrl() { 2940 return this.fullUrl != null && !this.fullUrl.isEmpty(); 2941 } 2942 2943 /** 2944 * @param value {@link #fullUrl} (The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: 2945* invoking a create 2946* invoking or responding to an operation where the body is not a single identified resource 2947* invoking or returning the results of a search or history operation.). This is the underlying object with id, value and extensions. The accessor "getFullUrl" gives direct access to the value 2948 */ 2949 public BundleEntryComponent setFullUrlElement(UriType value) { 2950 this.fullUrl = value; 2951 return this; 2952 } 2953 2954 /** 2955 * @return The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: 2956* invoking a create 2957* invoking or responding to an operation where the body is not a single identified resource 2958* invoking or returning the results of a search or history operation. 2959 */ 2960 public String getFullUrl() { 2961 return this.fullUrl == null ? null : this.fullUrl.getValue(); 2962 } 2963 2964 /** 2965 * @param value The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: 2966* invoking a create 2967* invoking or responding to an operation where the body is not a single identified resource 2968* invoking or returning the results of a search or history operation. 2969 */ 2970 public BundleEntryComponent setFullUrl(String value) { 2971 if (Utilities.noString(value)) 2972 this.fullUrl = null; 2973 else { 2974 if (this.fullUrl == null) 2975 this.fullUrl = new UriType(); 2976 this.fullUrl.setValue(value); 2977 } 2978 return this; 2979 } 2980 2981 /** 2982 * @return {@link #resource} (The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type. This is allowed to be a Parameters resource if and only if it is referenced by something else within the Bundle that provides context/meaning.) 2983 */ 2984 public Resource getResource() { 2985 return this.resource; 2986 } 2987 2988 public boolean hasResource() { 2989 return this.resource != null && !this.resource.isEmpty(); 2990 } 2991 2992 /** 2993 * @param value {@link #resource} (The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type. This is allowed to be a Parameters resource if and only if it is referenced by something else within the Bundle that provides context/meaning.) 2994 */ 2995 public BundleEntryComponent setResource(Resource value) { 2996 this.resource = value; 2997 return this; 2998 } 2999 3000 /** 3001 * @return {@link #search} (Information about the search process that lead to the creation of this entry.) 3002 */ 3003 public BundleEntrySearchComponent getSearch() { 3004 if (this.search == null) 3005 if (Configuration.errorOnAutoCreate()) 3006 throw new Error("Attempt to auto-create BundleEntryComponent.search"); 3007 else if (Configuration.doAutoCreate()) 3008 this.search = new BundleEntrySearchComponent(); // cc 3009 return this.search; 3010 } 3011 3012 public boolean hasSearch() { 3013 return this.search != null && !this.search.isEmpty(); 3014 } 3015 3016 /** 3017 * @param value {@link #search} (Information about the search process that lead to the creation of this entry.) 3018 */ 3019 public BundleEntryComponent setSearch(BundleEntrySearchComponent value) { 3020 this.search = value; 3021 return this; 3022 } 3023 3024 /** 3025 * @return {@link #request} (Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.) 3026 */ 3027 public BundleEntryRequestComponent getRequest() { 3028 if (this.request == null) 3029 if (Configuration.errorOnAutoCreate()) 3030 throw new Error("Attempt to auto-create BundleEntryComponent.request"); 3031 else if (Configuration.doAutoCreate()) 3032 this.request = new BundleEntryRequestComponent(); // cc 3033 return this.request; 3034 } 3035 3036 public boolean hasRequest() { 3037 return this.request != null && !this.request.isEmpty(); 3038 } 3039 3040 /** 3041 * @param value {@link #request} (Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.) 3042 */ 3043 public BundleEntryComponent setRequest(BundleEntryRequestComponent value) { 3044 this.request = value; 3045 return this; 3046 } 3047 3048 /** 3049 * @return {@link #response} (Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.) 3050 */ 3051 public BundleEntryResponseComponent getResponse() { 3052 if (this.response == null) 3053 if (Configuration.errorOnAutoCreate()) 3054 throw new Error("Attempt to auto-create BundleEntryComponent.response"); 3055 else if (Configuration.doAutoCreate()) 3056 this.response = new BundleEntryResponseComponent(); // cc 3057 return this.response; 3058 } 3059 3060 public boolean hasResponse() { 3061 return this.response != null && !this.response.isEmpty(); 3062 } 3063 3064 /** 3065 * @param value {@link #response} (Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.) 3066 */ 3067 public BundleEntryComponent setResponse(BundleEntryResponseComponent value) { 3068 this.response = value; 3069 return this; 3070 } 3071 3072 protected void listChildren(List<Property> children) { 3073 super.listChildren(children); 3074 children.add(new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 0, java.lang.Integer.MAX_VALUE, link)); 3075 children.add(new Property("fullUrl", "uri", "The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: \n* invoking a create\n* invoking or responding to an operation where the body is not a single identified resource\n* invoking or returning the results of a search or history operation.", 0, 1, fullUrl)); 3076 children.add(new Property("resource", "Resource", "The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type. This is allowed to be a Parameters resource if and only if it is referenced by something else within the Bundle that provides context/meaning.", 0, 1, resource)); 3077 children.add(new Property("search", "", "Information about the search process that lead to the creation of this entry.", 0, 1, search)); 3078 children.add(new Property("request", "", "Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.", 0, 1, request)); 3079 children.add(new Property("response", "", "Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.", 0, 1, response)); 3080 } 3081 3082 @Override 3083 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3084 switch (_hash) { 3085 case 3321850: /*link*/ return new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 0, java.lang.Integer.MAX_VALUE, link); 3086 case -511251360: /*fullUrl*/ return new Property("fullUrl", "uri", "The Absolute URL for the resource. Except for transactions and batches, each entry in a Bundle must have a fullUrl. The fullUrl SHALL NOT disagree with the id in the resource - i.e. if the fullUrl is not a urn:uuid, the URL shall be version-independent URL consistent with the Resource.id. The fullUrl is a version independent reference to the resource. Even when not required, fullUrl MAY be set to a urn:uuid to allow referencing entries in a transaction. The fullUrl can be an arbitrary URI and is not limited to urn:uuid, urn:oid, http, and https. The fullUrl element SHALL have a value except when: \n* invoking a create\n* invoking or responding to an operation where the body is not a single identified resource\n* invoking or returning the results of a search or history operation.", 0, 1, fullUrl); 3087 case -341064690: /*resource*/ return new Property("resource", "Resource", "The Resource for the entry. The purpose/meaning of the resource is determined by the Bundle.type. This is allowed to be a Parameters resource if and only if it is referenced by something else within the Bundle that provides context/meaning.", 0, 1, resource); 3088 case -906336856: /*search*/ return new Property("search", "", "Information about the search process that lead to the creation of this entry.", 0, 1, search); 3089 case 1095692943: /*request*/ return new Property("request", "", "Additional information about how this entry should be processed as part of a transaction or batch. For history, it shows how the entry was processed to create the version contained in the entry.", 0, 1, request); 3090 case -340323263: /*response*/ return new Property("response", "", "Indicates the results of processing the corresponding 'request' entry in the batch or transaction being responded to or what the results of an operation where when returning history.", 0, 1, response); 3091 default: return super.getNamedProperty(_hash, _name, _checkValid); 3092 } 3093 3094 } 3095 3096 @Override 3097 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3098 switch (hash) { 3099 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // BundleLinkComponent 3100 case -511251360: /*fullUrl*/ return this.fullUrl == null ? new Base[0] : new Base[] {this.fullUrl}; // UriType 3101 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Resource 3102 case -906336856: /*search*/ return this.search == null ? new Base[0] : new Base[] {this.search}; // BundleEntrySearchComponent 3103 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // BundleEntryRequestComponent 3104 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // BundleEntryResponseComponent 3105 default: return super.getProperty(hash, name, checkValid); 3106 } 3107 3108 } 3109 3110 @Override 3111 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3112 switch (hash) { 3113 case 3321850: // link 3114 this.getLink().add((BundleLinkComponent) value); // BundleLinkComponent 3115 return value; 3116 case -511251360: // fullUrl 3117 this.fullUrl = TypeConvertor.castToUri(value); // UriType 3118 return value; 3119 case -341064690: // resource 3120 this.resource = TypeConvertor.castToResource(value); // Resource 3121 return value; 3122 case -906336856: // search 3123 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 3124 return value; 3125 case 1095692943: // request 3126 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 3127 return value; 3128 case -340323263: // response 3129 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 3130 return value; 3131 default: return super.setProperty(hash, name, value); 3132 } 3133 3134 } 3135 3136 @Override 3137 public Base setProperty(String name, Base value) throws FHIRException { 3138 if (name.equals("link")) { 3139 this.getLink().add((BundleLinkComponent) value); 3140 } else if (name.equals("fullUrl")) { 3141 this.fullUrl = TypeConvertor.castToUri(value); // UriType 3142 } else if (name.equals("resource")) { 3143 this.resource = TypeConvertor.castToResource(value); // Resource 3144 } else if (name.equals("search")) { 3145 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 3146 } else if (name.equals("request")) { 3147 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 3148 } else if (name.equals("response")) { 3149 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 3150 } else 3151 return super.setProperty(name, value); 3152 return value; 3153 } 3154 3155 @Override 3156 public void removeChild(String name, Base value) throws FHIRException { 3157 if (name.equals("link")) { 3158 this.getLink().remove((BundleLinkComponent) value); 3159 } else if (name.equals("fullUrl")) { 3160 this.fullUrl = null; 3161 } else if (name.equals("resource")) { 3162 this.resource = null; 3163 } else if (name.equals("search")) { 3164 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 3165 } else if (name.equals("request")) { 3166 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 3167 } else if (name.equals("response")) { 3168 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 3169 } else 3170 super.removeChild(name, value); 3171 3172 } 3173 3174 @Override 3175 public Base makeProperty(int hash, String name) throws FHIRException { 3176 switch (hash) { 3177 case 3321850: return addLink(); 3178 case -511251360: return getFullUrlElement(); 3179 case -341064690: throw new FHIRException("Cannot make property resource as it is not a complex type"); // Resource 3180 case -906336856: return getSearch(); 3181 case 1095692943: return getRequest(); 3182 case -340323263: return getResponse(); 3183 default: return super.makeProperty(hash, name); 3184 } 3185 3186 } 3187 3188 @Override 3189 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3190 switch (hash) { 3191 case 3321850: /*link*/ return new String[] {"@Bundle.link"}; 3192 case -511251360: /*fullUrl*/ return new String[] {"uri"}; 3193 case -341064690: /*resource*/ return new String[] {"Resource"}; 3194 case -906336856: /*search*/ return new String[] {}; 3195 case 1095692943: /*request*/ return new String[] {}; 3196 case -340323263: /*response*/ return new String[] {}; 3197 default: return super.getTypesForProperty(hash, name); 3198 } 3199 3200 } 3201 3202 @Override 3203 public Base addChild(String name) throws FHIRException { 3204 if (name.equals("link")) { 3205 return addLink(); 3206 } 3207 else if (name.equals("fullUrl")) { 3208 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.fullUrl"); 3209 } 3210 else if (name.equals("resource")) { 3211 throw new FHIRException("Cannot call addChild on an abstract type Bundle.entry.resource"); 3212 } 3213 else if (name.equals("search")) { 3214 this.search = new BundleEntrySearchComponent(); 3215 return this.search; 3216 } 3217 else if (name.equals("request")) { 3218 this.request = new BundleEntryRequestComponent(); 3219 return this.request; 3220 } 3221 else if (name.equals("response")) { 3222 this.response = new BundleEntryResponseComponent(); 3223 return this.response; 3224 } 3225 else 3226 return super.addChild(name); 3227 } 3228 3229 public BundleEntryComponent copy() { 3230 BundleEntryComponent dst = new BundleEntryComponent(); 3231 copyValues(dst); 3232 return dst; 3233 } 3234 3235 public void copyValues(BundleEntryComponent dst) { 3236 super.copyValues(dst); 3237 if (link != null) { 3238 dst.link = new ArrayList<BundleLinkComponent>(); 3239 for (BundleLinkComponent i : link) 3240 dst.link.add(i.copy()); 3241 }; 3242 dst.fullUrl = fullUrl == null ? null : fullUrl.copy(); 3243 dst.resource = resource == null ? null : resource.copy(); 3244 dst.search = search == null ? null : search.copy(); 3245 dst.request = request == null ? null : request.copy(); 3246 dst.response = response == null ? null : response.copy(); 3247 } 3248 3249 @Override 3250 public boolean equalsDeep(Base other_) { 3251 if (!super.equalsDeep(other_)) 3252 return false; 3253 if (!(other_ instanceof BundleEntryComponent)) 3254 return false; 3255 BundleEntryComponent o = (BundleEntryComponent) other_; 3256 return compareDeep(link, o.link, true) && compareDeep(fullUrl, o.fullUrl, true) && compareDeep(resource, o.resource, true) 3257 && compareDeep(search, o.search, true) && compareDeep(request, o.request, true) && compareDeep(response, o.response, true) 3258 ; 3259 } 3260 3261 @Override 3262 public boolean equalsShallow(Base other_) { 3263 if (!super.equalsShallow(other_)) 3264 return false; 3265 if (!(other_ instanceof BundleEntryComponent)) 3266 return false; 3267 BundleEntryComponent o = (BundleEntryComponent) other_; 3268 return compareValues(fullUrl, o.fullUrl, true); 3269 } 3270 3271 public boolean isEmpty() { 3272 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link, fullUrl, resource 3273 , search, request, response); 3274 } 3275 3276 public String fhirType() { 3277 return "Bundle.entry"; 3278 3279 } 3280 3281// added from java-adornments.txt: 3282/** 3283 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 3284 * If no link is found which matches the given relation, returns <code>null</code>. If more than one 3285 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 3286 * 3287 * @param theRelation 3288 * The relation, such as \"next\", or \"self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 3289 * @return Returns a matching BundleLinkComponent, or <code>null</code> 3290 * @see IBaseBundle#LINK_NEXT 3291 * @see IBaseBundle#LINK_PREV 3292 * @see IBaseBundle#LINK_SELF 3293 */ 3294 public BundleLinkComponent getLink(String theRelation) { 3295 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 3296 for (BundleLinkComponent next : getLink()) { 3297 if (theRelation.equals(next.getRelation().toCode())) { 3298 return next; 3299 } 3300 } 3301 return null; 3302 } 3303 3304 /** 3305 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 3306 * If no link is found which matches the given relation, creates a new BundleLinkComponent with the 3307 * given relation and adds it to this Bundle. If more than one 3308 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 3309 * 3310 * @param theRelation 3311 * The relation, such as \"next\", or \"self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 3312 * @return Returns a matching BundleLinkComponent, or <code>null</code> 3313 * @see IBaseBundle#LINK_NEXT 3314 * @see IBaseBundle#LINK_PREV 3315 * @see IBaseBundle#LINK_SELF 3316 */ 3317 public BundleLinkComponent getLinkOrCreate(String theRelation) { 3318 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 3319 for (BundleLinkComponent next : getLink()) { 3320 if (theRelation.equals(next.getRelation().toCode())) { 3321 return next; 3322 } 3323 } 3324 BundleLinkComponent retVal = new BundleLinkComponent(); 3325 retVal.setRelation(LinkRelationTypes.fromCode(theRelation)); 3326 getLink().add(retVal); 3327 return retVal; 3328 } 3329// end addition 3330 } 3331 3332 @Block() 3333 public static class BundleEntrySearchComponent extends BackboneElement implements IBaseBackboneElement { 3334 /** 3335 * Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process. 3336 */ 3337 @Child(name = "mode", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3338 @Description(shortDefinition="match | include - why this is in the result set", formalDefinition="Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process." ) 3339 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-entry-mode") 3340 protected Enumeration<SearchEntryMode> mode; 3341 3342 /** 3343 * When searching, the server's search ranking score for the entry. 3344 */ 3345 @Child(name = "score", type = {DecimalType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3346 @Description(shortDefinition="Search ranking (between 0 and 1)", formalDefinition="When searching, the server's search ranking score for the entry." ) 3347 protected DecimalType score; 3348 3349 private static final long serialVersionUID = 837739866L; 3350 3351 /** 3352 * Constructor 3353 */ 3354 public BundleEntrySearchComponent() { 3355 super(); 3356 } 3357 3358 /** 3359 * @return {@link #mode} (Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 3360 */ 3361 public Enumeration<SearchEntryMode> getModeElement() { 3362 if (this.mode == null) 3363 if (Configuration.errorOnAutoCreate()) 3364 throw new Error("Attempt to auto-create BundleEntrySearchComponent.mode"); 3365 else if (Configuration.doAutoCreate()) 3366 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); // bb 3367 return this.mode; 3368 } 3369 3370 public boolean hasModeElement() { 3371 return this.mode != null && !this.mode.isEmpty(); 3372 } 3373 3374 public boolean hasMode() { 3375 return this.mode != null && !this.mode.isEmpty(); 3376 } 3377 3378 /** 3379 * @param value {@link #mode} (Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 3380 */ 3381 public BundleEntrySearchComponent setModeElement(Enumeration<SearchEntryMode> value) { 3382 this.mode = value; 3383 return this; 3384 } 3385 3386 /** 3387 * @return Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process. 3388 */ 3389 public SearchEntryMode getMode() { 3390 return this.mode == null ? null : this.mode.getValue(); 3391 } 3392 3393 /** 3394 * @param value Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process. 3395 */ 3396 public BundleEntrySearchComponent setMode(SearchEntryMode value) { 3397 if (value == null) 3398 this.mode = null; 3399 else { 3400 if (this.mode == null) 3401 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); 3402 this.mode.setValue(value); 3403 } 3404 return this; 3405 } 3406 3407 /** 3408 * @return {@link #score} (When searching, the server's search ranking score for the entry.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3409 */ 3410 public DecimalType getScoreElement() { 3411 if (this.score == null) 3412 if (Configuration.errorOnAutoCreate()) 3413 throw new Error("Attempt to auto-create BundleEntrySearchComponent.score"); 3414 else if (Configuration.doAutoCreate()) 3415 this.score = new DecimalType(); // bb 3416 return this.score; 3417 } 3418 3419 public boolean hasScoreElement() { 3420 return this.score != null && !this.score.isEmpty(); 3421 } 3422 3423 public boolean hasScore() { 3424 return this.score != null && !this.score.isEmpty(); 3425 } 3426 3427 /** 3428 * @param value {@link #score} (When searching, the server's search ranking score for the entry.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3429 */ 3430 public BundleEntrySearchComponent setScoreElement(DecimalType value) { 3431 this.score = value; 3432 return this; 3433 } 3434 3435 /** 3436 * @return When searching, the server's search ranking score for the entry. 3437 */ 3438 public BigDecimal getScore() { 3439 return this.score == null ? null : this.score.getValue(); 3440 } 3441 3442 /** 3443 * @param value When searching, the server's search ranking score for the entry. 3444 */ 3445 public BundleEntrySearchComponent setScore(BigDecimal value) { 3446 if (value == null) 3447 this.score = null; 3448 else { 3449 if (this.score == null) 3450 this.score = new DecimalType(); 3451 this.score.setValue(value); 3452 } 3453 return this; 3454 } 3455 3456 /** 3457 * @param value When searching, the server's search ranking score for the entry. 3458 */ 3459 public BundleEntrySearchComponent setScore(long value) { 3460 this.score = new DecimalType(); 3461 this.score.setValue(value); 3462 return this; 3463 } 3464 3465 /** 3466 * @param value When searching, the server's search ranking score for the entry. 3467 */ 3468 public BundleEntrySearchComponent setScore(double value) { 3469 this.score = new DecimalType(); 3470 this.score.setValue(value); 3471 return this; 3472 } 3473 3474 protected void listChildren(List<Property> children) { 3475 super.listChildren(children); 3476 children.add(new Property("mode", "code", "Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.", 0, 1, mode)); 3477 children.add(new Property("score", "decimal", "When searching, the server's search ranking score for the entry.", 0, 1, score)); 3478 } 3479 3480 @Override 3481 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3482 switch (_hash) { 3483 case 3357091: /*mode*/ return new Property("mode", "code", "Why this entry is in the result set - whether it's included as a match or because of an _include requirement, or to convey information or warning information about the search process.", 0, 1, mode); 3484 case 109264530: /*score*/ return new Property("score", "decimal", "When searching, the server's search ranking score for the entry.", 0, 1, score); 3485 default: return super.getNamedProperty(_hash, _name, _checkValid); 3486 } 3487 3488 } 3489 3490 @Override 3491 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3492 switch (hash) { 3493 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<SearchEntryMode> 3494 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // DecimalType 3495 default: return super.getProperty(hash, name, checkValid); 3496 } 3497 3498 } 3499 3500 @Override 3501 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3502 switch (hash) { 3503 case 3357091: // mode 3504 value = new SearchEntryModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3505 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 3506 return value; 3507 case 109264530: // score 3508 this.score = TypeConvertor.castToDecimal(value); // DecimalType 3509 return value; 3510 default: return super.setProperty(hash, name, value); 3511 } 3512 3513 } 3514 3515 @Override 3516 public Base setProperty(String name, Base value) throws FHIRException { 3517 if (name.equals("mode")) { 3518 value = new SearchEntryModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3519 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 3520 } else if (name.equals("score")) { 3521 this.score = TypeConvertor.castToDecimal(value); // DecimalType 3522 } else 3523 return super.setProperty(name, value); 3524 return value; 3525 } 3526 3527 @Override 3528 public void removeChild(String name, Base value) throws FHIRException { 3529 if (name.equals("mode")) { 3530 value = new SearchEntryModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3531 this.mode = (Enumeration) value; // Enumeration<SearchEntryMode> 3532 } else if (name.equals("score")) { 3533 this.score = null; 3534 } else 3535 super.removeChild(name, value); 3536 3537 } 3538 3539 @Override 3540 public Base makeProperty(int hash, String name) throws FHIRException { 3541 switch (hash) { 3542 case 3357091: return getModeElement(); 3543 case 109264530: return getScoreElement(); 3544 default: return super.makeProperty(hash, name); 3545 } 3546 3547 } 3548 3549 @Override 3550 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3551 switch (hash) { 3552 case 3357091: /*mode*/ return new String[] {"code"}; 3553 case 109264530: /*score*/ return new String[] {"decimal"}; 3554 default: return super.getTypesForProperty(hash, name); 3555 } 3556 3557 } 3558 3559 @Override 3560 public Base addChild(String name) throws FHIRException { 3561 if (name.equals("mode")) { 3562 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.search.mode"); 3563 } 3564 else if (name.equals("score")) { 3565 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.search.score"); 3566 } 3567 else 3568 return super.addChild(name); 3569 } 3570 3571 public BundleEntrySearchComponent copy() { 3572 BundleEntrySearchComponent dst = new BundleEntrySearchComponent(); 3573 copyValues(dst); 3574 return dst; 3575 } 3576 3577 public void copyValues(BundleEntrySearchComponent dst) { 3578 super.copyValues(dst); 3579 dst.mode = mode == null ? null : mode.copy(); 3580 dst.score = score == null ? null : score.copy(); 3581 } 3582 3583 @Override 3584 public boolean equalsDeep(Base other_) { 3585 if (!super.equalsDeep(other_)) 3586 return false; 3587 if (!(other_ instanceof BundleEntrySearchComponent)) 3588 return false; 3589 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other_; 3590 return compareDeep(mode, o.mode, true) && compareDeep(score, o.score, true); 3591 } 3592 3593 @Override 3594 public boolean equalsShallow(Base other_) { 3595 if (!super.equalsShallow(other_)) 3596 return false; 3597 if (!(other_ instanceof BundleEntrySearchComponent)) 3598 return false; 3599 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other_; 3600 return compareValues(mode, o.mode, true) && compareValues(score, o.score, true); 3601 } 3602 3603 public boolean isEmpty() { 3604 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, score); 3605 } 3606 3607 public String fhirType() { 3608 return "Bundle.entry.search"; 3609 3610 } 3611 3612 } 3613 3614 @Block() 3615 public static class BundleEntryRequestComponent extends BackboneElement implements IBaseBackboneElement { 3616 /** 3617 * In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred. 3618 */ 3619 @Child(name = "method", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3620 @Description(shortDefinition="GET | HEAD | POST | PUT | DELETE | PATCH", formalDefinition="In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred." ) 3621 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/http-verb") 3622 protected Enumeration<HTTPVerb> method; 3623 3624 /** 3625 * The URL for this entry, relative to the root (the address to which the request is posted). 3626 */ 3627 @Child(name = "url", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 3628 @Description(shortDefinition="URL for HTTP equivalent of this entry", formalDefinition="The URL for this entry, relative to the root (the address to which the request is posted)." ) 3629 protected UriType url; 3630 3631 /** 3632 * If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread). 3633 */ 3634 @Child(name = "ifNoneMatch", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 3635 @Description(shortDefinition="For managing cache validation", formalDefinition="If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread)." ) 3636 protected StringType ifNoneMatch; 3637 3638 /** 3639 * Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread). 3640 */ 3641 @Child(name = "ifModifiedSince", type = {InstantType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3642 @Description(shortDefinition="For managing cache currency", formalDefinition="Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread)." ) 3643 protected InstantType ifModifiedSince; 3644 3645 /** 3646 * Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency). 3647 */ 3648 @Child(name = "ifMatch", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3649 @Description(shortDefinition="For managing update contention", formalDefinition="Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency)." ) 3650 protected StringType ifMatch; 3651 3652 /** 3653 * Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?"). 3654 */ 3655 @Child(name = "ifNoneExist", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3656 @Description(shortDefinition="For conditional creates", formalDefinition="Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\")." ) 3657 protected StringType ifNoneExist; 3658 3659 private static final long serialVersionUID = -1349769744L; 3660 3661 /** 3662 * Constructor 3663 */ 3664 public BundleEntryRequestComponent() { 3665 super(); 3666 } 3667 3668 /** 3669 * Constructor 3670 */ 3671 public BundleEntryRequestComponent(HTTPVerb method, String url) { 3672 super(); 3673 this.setMethod(method); 3674 this.setUrl(url); 3675 } 3676 3677 /** 3678 * @return {@link #method} (In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.). This is the underlying object with id, value and extensions. The accessor "getMethod" gives direct access to the value 3679 */ 3680 public Enumeration<HTTPVerb> getMethodElement() { 3681 if (this.method == null) 3682 if (Configuration.errorOnAutoCreate()) 3683 throw new Error("Attempt to auto-create BundleEntryRequestComponent.method"); 3684 else if (Configuration.doAutoCreate()) 3685 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); // bb 3686 return this.method; 3687 } 3688 3689 public boolean hasMethodElement() { 3690 return this.method != null && !this.method.isEmpty(); 3691 } 3692 3693 public boolean hasMethod() { 3694 return this.method != null && !this.method.isEmpty(); 3695 } 3696 3697 /** 3698 * @param value {@link #method} (In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.). This is the underlying object with id, value and extensions. The accessor "getMethod" gives direct access to the value 3699 */ 3700 public BundleEntryRequestComponent setMethodElement(Enumeration<HTTPVerb> value) { 3701 this.method = value; 3702 return this; 3703 } 3704 3705 /** 3706 * @return In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred. 3707 */ 3708 public HTTPVerb getMethod() { 3709 return this.method == null ? null : this.method.getValue(); 3710 } 3711 3712 /** 3713 * @param value In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred. 3714 */ 3715 public BundleEntryRequestComponent setMethod(HTTPVerb value) { 3716 if (this.method == null) 3717 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); 3718 this.method.setValue(value); 3719 return this; 3720 } 3721 3722 /** 3723 * @return {@link #url} (The URL for this entry, relative to the root (the address to which the request is posted).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3724 */ 3725 public UriType getUrlElement() { 3726 if (this.url == null) 3727 if (Configuration.errorOnAutoCreate()) 3728 throw new Error("Attempt to auto-create BundleEntryRequestComponent.url"); 3729 else if (Configuration.doAutoCreate()) 3730 this.url = new UriType(); // bb 3731 return this.url; 3732 } 3733 3734 public boolean hasUrlElement() { 3735 return this.url != null && !this.url.isEmpty(); 3736 } 3737 3738 public boolean hasUrl() { 3739 return this.url != null && !this.url.isEmpty(); 3740 } 3741 3742 /** 3743 * @param value {@link #url} (The URL for this entry, relative to the root (the address to which the request is posted).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3744 */ 3745 public BundleEntryRequestComponent setUrlElement(UriType value) { 3746 this.url = value; 3747 return this; 3748 } 3749 3750 /** 3751 * @return The URL for this entry, relative to the root (the address to which the request is posted). 3752 */ 3753 public String getUrl() { 3754 return this.url == null ? null : this.url.getValue(); 3755 } 3756 3757 /** 3758 * @param value The URL for this entry, relative to the root (the address to which the request is posted). 3759 */ 3760 public BundleEntryRequestComponent setUrl(String value) { 3761 if (this.url == null) 3762 this.url = new UriType(); 3763 this.url.setValue(value); 3764 return this; 3765 } 3766 3767 /** 3768 * @return {@link #ifNoneMatch} (If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfNoneMatch" gives direct access to the value 3769 */ 3770 public StringType getIfNoneMatchElement() { 3771 if (this.ifNoneMatch == null) 3772 if (Configuration.errorOnAutoCreate()) 3773 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneMatch"); 3774 else if (Configuration.doAutoCreate()) 3775 this.ifNoneMatch = new StringType(); // bb 3776 return this.ifNoneMatch; 3777 } 3778 3779 public boolean hasIfNoneMatchElement() { 3780 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 3781 } 3782 3783 public boolean hasIfNoneMatch() { 3784 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 3785 } 3786 3787 /** 3788 * @param value {@link #ifNoneMatch} (If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfNoneMatch" gives direct access to the value 3789 */ 3790 public BundleEntryRequestComponent setIfNoneMatchElement(StringType value) { 3791 this.ifNoneMatch = value; 3792 return this; 3793 } 3794 3795 /** 3796 * @return If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread). 3797 */ 3798 public String getIfNoneMatch() { 3799 return this.ifNoneMatch == null ? null : this.ifNoneMatch.getValue(); 3800 } 3801 3802 /** 3803 * @param value If the ETag values match, return a 304 Not Modified status. See the API documentation for ["Conditional Read"](http.html#cread). 3804 */ 3805 public BundleEntryRequestComponent setIfNoneMatch(String value) { 3806 if (Utilities.noString(value)) 3807 this.ifNoneMatch = null; 3808 else { 3809 if (this.ifNoneMatch == null) 3810 this.ifNoneMatch = new StringType(); 3811 this.ifNoneMatch.setValue(value); 3812 } 3813 return this; 3814 } 3815 3816 /** 3817 * @return {@link #ifModifiedSince} (Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfModifiedSince" gives direct access to the value 3818 */ 3819 public InstantType getIfModifiedSinceElement() { 3820 if (this.ifModifiedSince == null) 3821 if (Configuration.errorOnAutoCreate()) 3822 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifModifiedSince"); 3823 else if (Configuration.doAutoCreate()) 3824 this.ifModifiedSince = new InstantType(); // bb 3825 return this.ifModifiedSince; 3826 } 3827 3828 public boolean hasIfModifiedSinceElement() { 3829 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 3830 } 3831 3832 public boolean hasIfModifiedSince() { 3833 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 3834 } 3835 3836 /** 3837 * @param value {@link #ifModifiedSince} (Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread).). This is the underlying object with id, value and extensions. The accessor "getIfModifiedSince" gives direct access to the value 3838 */ 3839 public BundleEntryRequestComponent setIfModifiedSinceElement(InstantType value) { 3840 this.ifModifiedSince = value; 3841 return this; 3842 } 3843 3844 /** 3845 * @return Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread). 3846 */ 3847 public Date getIfModifiedSince() { 3848 return this.ifModifiedSince == null ? null : this.ifModifiedSince.getValue(); 3849 } 3850 3851 /** 3852 * @param value Only perform the operation if the last updated date matches. See the API documentation for ["Conditional Read"](http.html#cread). 3853 */ 3854 public BundleEntryRequestComponent setIfModifiedSince(Date value) { 3855 if (value == null) 3856 this.ifModifiedSince = null; 3857 else { 3858 if (this.ifModifiedSince == null) 3859 this.ifModifiedSince = new InstantType(); 3860 this.ifModifiedSince.setValue(value); 3861 } 3862 return this; 3863 } 3864 3865 /** 3866 * @return {@link #ifMatch} (Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency).). This is the underlying object with id, value and extensions. The accessor "getIfMatch" gives direct access to the value 3867 */ 3868 public StringType getIfMatchElement() { 3869 if (this.ifMatch == null) 3870 if (Configuration.errorOnAutoCreate()) 3871 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifMatch"); 3872 else if (Configuration.doAutoCreate()) 3873 this.ifMatch = new StringType(); // bb 3874 return this.ifMatch; 3875 } 3876 3877 public boolean hasIfMatchElement() { 3878 return this.ifMatch != null && !this.ifMatch.isEmpty(); 3879 } 3880 3881 public boolean hasIfMatch() { 3882 return this.ifMatch != null && !this.ifMatch.isEmpty(); 3883 } 3884 3885 /** 3886 * @param value {@link #ifMatch} (Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency).). This is the underlying object with id, value and extensions. The accessor "getIfMatch" gives direct access to the value 3887 */ 3888 public BundleEntryRequestComponent setIfMatchElement(StringType value) { 3889 this.ifMatch = value; 3890 return this; 3891 } 3892 3893 /** 3894 * @return Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency). 3895 */ 3896 public String getIfMatch() { 3897 return this.ifMatch == null ? null : this.ifMatch.getValue(); 3898 } 3899 3900 /** 3901 * @param value Only perform the operation if the Etag value matches. For more information, see the API section ["Managing Resource Contention"](http.html#concurrency). 3902 */ 3903 public BundleEntryRequestComponent setIfMatch(String value) { 3904 if (Utilities.noString(value)) 3905 this.ifMatch = null; 3906 else { 3907 if (this.ifMatch == null) 3908 this.ifMatch = new StringType(); 3909 this.ifMatch.setValue(value); 3910 } 3911 return this; 3912 } 3913 3914 /** 3915 * @return {@link #ifNoneExist} (Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?").). This is the underlying object with id, value and extensions. The accessor "getIfNoneExist" gives direct access to the value 3916 */ 3917 public StringType getIfNoneExistElement() { 3918 if (this.ifNoneExist == null) 3919 if (Configuration.errorOnAutoCreate()) 3920 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneExist"); 3921 else if (Configuration.doAutoCreate()) 3922 this.ifNoneExist = new StringType(); // bb 3923 return this.ifNoneExist; 3924 } 3925 3926 public boolean hasIfNoneExistElement() { 3927 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 3928 } 3929 3930 public boolean hasIfNoneExist() { 3931 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 3932 } 3933 3934 /** 3935 * @param value {@link #ifNoneExist} (Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?").). This is the underlying object with id, value and extensions. The accessor "getIfNoneExist" gives direct access to the value 3936 */ 3937 public BundleEntryRequestComponent setIfNoneExistElement(StringType value) { 3938 this.ifNoneExist = value; 3939 return this; 3940 } 3941 3942 /** 3943 * @return Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?"). 3944 */ 3945 public String getIfNoneExist() { 3946 return this.ifNoneExist == null ? null : this.ifNoneExist.getValue(); 3947 } 3948 3949 /** 3950 * @param value Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for ["Conditional Create"](http.html#ccreate). This is just the query portion of the URL - what follows the "?" (not including the "?"). 3951 */ 3952 public BundleEntryRequestComponent setIfNoneExist(String value) { 3953 if (Utilities.noString(value)) 3954 this.ifNoneExist = null; 3955 else { 3956 if (this.ifNoneExist == null) 3957 this.ifNoneExist = new StringType(); 3958 this.ifNoneExist.setValue(value); 3959 } 3960 return this; 3961 } 3962 3963 protected void listChildren(List<Property> children) { 3964 super.listChildren(children); 3965 children.add(new Property("method", "code", "In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.", 0, 1, method)); 3966 children.add(new Property("url", "uri", "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1, url)); 3967 children.add(new Property("ifNoneMatch", "string", "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifNoneMatch)); 3968 children.add(new Property("ifModifiedSince", "instant", "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifModifiedSince)); 3969 children.add(new Property("ifMatch", "string", "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 0, 1, ifMatch)); 3970 children.add(new Property("ifNoneExist", "string", "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 0, 1, ifNoneExist)); 3971 } 3972 3973 @Override 3974 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3975 switch (_hash) { 3976 case -1077554975: /*method*/ return new Property("method", "code", "In a transaction or batch, this is the HTTP action to be executed for this entry. In a history bundle, this indicates the HTTP action that occurred.", 0, 1, method); 3977 case 116079: /*url*/ return new Property("url", "uri", "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1, url); 3978 case 171868368: /*ifNoneMatch*/ return new Property("ifNoneMatch", "string", "If the ETag values match, return a 304 Not Modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifNoneMatch); 3979 case -2061602860: /*ifModifiedSince*/ return new Property("ifModifiedSince", "instant", "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 0, 1, ifModifiedSince); 3980 case 1692894888: /*ifMatch*/ return new Property("ifMatch", "string", "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 0, 1, ifMatch); 3981 case 165155330: /*ifNoneExist*/ return new Property("ifNoneExist", "string", "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 0, 1, ifNoneExist); 3982 default: return super.getNamedProperty(_hash, _name, _checkValid); 3983 } 3984 3985 } 3986 3987 @Override 3988 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3989 switch (hash) { 3990 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // Enumeration<HTTPVerb> 3991 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3992 case 171868368: /*ifNoneMatch*/ return this.ifNoneMatch == null ? new Base[0] : new Base[] {this.ifNoneMatch}; // StringType 3993 case -2061602860: /*ifModifiedSince*/ return this.ifModifiedSince == null ? new Base[0] : new Base[] {this.ifModifiedSince}; // InstantType 3994 case 1692894888: /*ifMatch*/ return this.ifMatch == null ? new Base[0] : new Base[] {this.ifMatch}; // StringType 3995 case 165155330: /*ifNoneExist*/ return this.ifNoneExist == null ? new Base[0] : new Base[] {this.ifNoneExist}; // StringType 3996 default: return super.getProperty(hash, name, checkValid); 3997 } 3998 3999 } 4000 4001 @Override 4002 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4003 switch (hash) { 4004 case -1077554975: // method 4005 value = new HTTPVerbEnumFactory().fromType(TypeConvertor.castToCode(value)); 4006 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 4007 return value; 4008 case 116079: // url 4009 this.url = TypeConvertor.castToUri(value); // UriType 4010 return value; 4011 case 171868368: // ifNoneMatch 4012 this.ifNoneMatch = TypeConvertor.castToString(value); // StringType 4013 return value; 4014 case -2061602860: // ifModifiedSince 4015 this.ifModifiedSince = TypeConvertor.castToInstant(value); // InstantType 4016 return value; 4017 case 1692894888: // ifMatch 4018 this.ifMatch = TypeConvertor.castToString(value); // StringType 4019 return value; 4020 case 165155330: // ifNoneExist 4021 this.ifNoneExist = TypeConvertor.castToString(value); // StringType 4022 return value; 4023 default: return super.setProperty(hash, name, value); 4024 } 4025 4026 } 4027 4028 @Override 4029 public Base setProperty(String name, Base value) throws FHIRException { 4030 if (name.equals("method")) { 4031 value = new HTTPVerbEnumFactory().fromType(TypeConvertor.castToCode(value)); 4032 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 4033 } else if (name.equals("url")) { 4034 this.url = TypeConvertor.castToUri(value); // UriType 4035 } else if (name.equals("ifNoneMatch")) { 4036 this.ifNoneMatch = TypeConvertor.castToString(value); // StringType 4037 } else if (name.equals("ifModifiedSince")) { 4038 this.ifModifiedSince = TypeConvertor.castToInstant(value); // InstantType 4039 } else if (name.equals("ifMatch")) { 4040 this.ifMatch = TypeConvertor.castToString(value); // StringType 4041 } else if (name.equals("ifNoneExist")) { 4042 this.ifNoneExist = TypeConvertor.castToString(value); // StringType 4043 } else 4044 return super.setProperty(name, value); 4045 return value; 4046 } 4047 4048 @Override 4049 public void removeChild(String name, Base value) throws FHIRException { 4050 if (name.equals("method")) { 4051 value = new HTTPVerbEnumFactory().fromType(TypeConvertor.castToCode(value)); 4052 this.method = (Enumeration) value; // Enumeration<HTTPVerb> 4053 } else if (name.equals("url")) { 4054 this.url = null; 4055 } else if (name.equals("ifNoneMatch")) { 4056 this.ifNoneMatch = null; 4057 } else if (name.equals("ifModifiedSince")) { 4058 this.ifModifiedSince = null; 4059 } else if (name.equals("ifMatch")) { 4060 this.ifMatch = null; 4061 } else if (name.equals("ifNoneExist")) { 4062 this.ifNoneExist = null; 4063 } else 4064 super.removeChild(name, value); 4065 4066 } 4067 4068 @Override 4069 public Base makeProperty(int hash, String name) throws FHIRException { 4070 switch (hash) { 4071 case -1077554975: return getMethodElement(); 4072 case 116079: return getUrlElement(); 4073 case 171868368: return getIfNoneMatchElement(); 4074 case -2061602860: return getIfModifiedSinceElement(); 4075 case 1692894888: return getIfMatchElement(); 4076 case 165155330: return getIfNoneExistElement(); 4077 default: return super.makeProperty(hash, name); 4078 } 4079 4080 } 4081 4082 @Override 4083 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4084 switch (hash) { 4085 case -1077554975: /*method*/ return new String[] {"code"}; 4086 case 116079: /*url*/ return new String[] {"uri"}; 4087 case 171868368: /*ifNoneMatch*/ return new String[] {"string"}; 4088 case -2061602860: /*ifModifiedSince*/ return new String[] {"instant"}; 4089 case 1692894888: /*ifMatch*/ return new String[] {"string"}; 4090 case 165155330: /*ifNoneExist*/ return new String[] {"string"}; 4091 default: return super.getTypesForProperty(hash, name); 4092 } 4093 4094 } 4095 4096 @Override 4097 public Base addChild(String name) throws FHIRException { 4098 if (name.equals("method")) { 4099 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.request.method"); 4100 } 4101 else if (name.equals("url")) { 4102 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.request.url"); 4103 } 4104 else if (name.equals("ifNoneMatch")) { 4105 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.request.ifNoneMatch"); 4106 } 4107 else if (name.equals("ifModifiedSince")) { 4108 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.request.ifModifiedSince"); 4109 } 4110 else if (name.equals("ifMatch")) { 4111 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.request.ifMatch"); 4112 } 4113 else if (name.equals("ifNoneExist")) { 4114 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.request.ifNoneExist"); 4115 } 4116 else 4117 return super.addChild(name); 4118 } 4119 4120 public BundleEntryRequestComponent copy() { 4121 BundleEntryRequestComponent dst = new BundleEntryRequestComponent(); 4122 copyValues(dst); 4123 return dst; 4124 } 4125 4126 public void copyValues(BundleEntryRequestComponent dst) { 4127 super.copyValues(dst); 4128 dst.method = method == null ? null : method.copy(); 4129 dst.url = url == null ? null : url.copy(); 4130 dst.ifNoneMatch = ifNoneMatch == null ? null : ifNoneMatch.copy(); 4131 dst.ifModifiedSince = ifModifiedSince == null ? null : ifModifiedSince.copy(); 4132 dst.ifMatch = ifMatch == null ? null : ifMatch.copy(); 4133 dst.ifNoneExist = ifNoneExist == null ? null : ifNoneExist.copy(); 4134 } 4135 4136 @Override 4137 public boolean equalsDeep(Base other_) { 4138 if (!super.equalsDeep(other_)) 4139 return false; 4140 if (!(other_ instanceof BundleEntryRequestComponent)) 4141 return false; 4142 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other_; 4143 return compareDeep(method, o.method, true) && compareDeep(url, o.url, true) && compareDeep(ifNoneMatch, o.ifNoneMatch, true) 4144 && compareDeep(ifModifiedSince, o.ifModifiedSince, true) && compareDeep(ifMatch, o.ifMatch, true) 4145 && compareDeep(ifNoneExist, o.ifNoneExist, true); 4146 } 4147 4148 @Override 4149 public boolean equalsShallow(Base other_) { 4150 if (!super.equalsShallow(other_)) 4151 return false; 4152 if (!(other_ instanceof BundleEntryRequestComponent)) 4153 return false; 4154 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other_; 4155 return compareValues(method, o.method, true) && compareValues(url, o.url, true) && compareValues(ifNoneMatch, o.ifNoneMatch, true) 4156 && compareValues(ifModifiedSince, o.ifModifiedSince, true) && compareValues(ifMatch, o.ifMatch, true) 4157 && compareValues(ifNoneExist, o.ifNoneExist, true); 4158 } 4159 4160 public boolean isEmpty() { 4161 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(method, url, ifNoneMatch 4162 , ifModifiedSince, ifMatch, ifNoneExist); 4163 } 4164 4165 public String fhirType() { 4166 return "Bundle.entry.request"; 4167 4168 } 4169 4170 } 4171 4172 @Block() 4173 public static class BundleEntryResponseComponent extends BackboneElement implements IBaseBackboneElement { 4174 /** 4175 * The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code. 4176 */ 4177 @Child(name = "status", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4178 @Description(shortDefinition="Status response code (text optional)", formalDefinition="The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code." ) 4179 protected StringType status; 4180 4181 /** 4182 * The location header created by processing this operation, populated if the operation returns a location. 4183 */ 4184 @Child(name = "location", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4185 @Description(shortDefinition="The location (if the operation returns a location)", formalDefinition="The location header created by processing this operation, populated if the operation returns a location." ) 4186 protected UriType location; 4187 4188 /** 4189 * The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)). 4190 */ 4191 @Child(name = "etag", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4192 @Description(shortDefinition="The Etag for the resource (if relevant)", formalDefinition="The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency))." ) 4193 protected StringType etag; 4194 4195 /** 4196 * The date/time that the resource was modified on the server. 4197 */ 4198 @Child(name = "lastModified", type = {InstantType.class}, order=4, min=0, max=1, modifier=false, summary=true) 4199 @Description(shortDefinition="Server's date time modified", formalDefinition="The date/time that the resource was modified on the server." ) 4200 protected InstantType lastModified; 4201 4202 /** 4203 * An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction. 4204 */ 4205 @Child(name = "outcome", type = {Resource.class}, order=5, min=0, max=1, modifier=false, summary=true) 4206 @Description(shortDefinition="OperationOutcome with hints and warnings (for batch/transaction)", formalDefinition="An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction." ) 4207 protected Resource outcome; 4208 4209 private static final long serialVersionUID = 923278008L; 4210 4211 /** 4212 * Constructor 4213 */ 4214 public BundleEntryResponseComponent() { 4215 super(); 4216 } 4217 4218 /** 4219 * Constructor 4220 */ 4221 public BundleEntryResponseComponent(String status) { 4222 super(); 4223 this.setStatus(status); 4224 } 4225 4226 /** 4227 * @return {@link #status} (The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4228 */ 4229 public StringType getStatusElement() { 4230 if (this.status == null) 4231 if (Configuration.errorOnAutoCreate()) 4232 throw new Error("Attempt to auto-create BundleEntryResponseComponent.status"); 4233 else if (Configuration.doAutoCreate()) 4234 this.status = new StringType(); // bb 4235 return this.status; 4236 } 4237 4238 public boolean hasStatusElement() { 4239 return this.status != null && !this.status.isEmpty(); 4240 } 4241 4242 public boolean hasStatus() { 4243 return this.status != null && !this.status.isEmpty(); 4244 } 4245 4246 /** 4247 * @param value {@link #status} (The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4248 */ 4249 public BundleEntryResponseComponent setStatusElement(StringType value) { 4250 this.status = value; 4251 return this; 4252 } 4253 4254 /** 4255 * @return The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code. 4256 */ 4257 public String getStatus() { 4258 return this.status == null ? null : this.status.getValue(); 4259 } 4260 4261 /** 4262 * @param value The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code. 4263 */ 4264 public BundleEntryResponseComponent setStatus(String value) { 4265 if (this.status == null) 4266 this.status = new StringType(); 4267 this.status.setValue(value); 4268 return this; 4269 } 4270 4271 /** 4272 * @return {@link #location} (The location header created by processing this operation, populated if the operation returns a location.). This is the underlying object with id, value and extensions. The accessor "getLocation" gives direct access to the value 4273 */ 4274 public UriType getLocationElement() { 4275 if (this.location == null) 4276 if (Configuration.errorOnAutoCreate()) 4277 throw new Error("Attempt to auto-create BundleEntryResponseComponent.location"); 4278 else if (Configuration.doAutoCreate()) 4279 this.location = new UriType(); // bb 4280 return this.location; 4281 } 4282 4283 public boolean hasLocationElement() { 4284 return this.location != null && !this.location.isEmpty(); 4285 } 4286 4287 public boolean hasLocation() { 4288 return this.location != null && !this.location.isEmpty(); 4289 } 4290 4291 /** 4292 * @param value {@link #location} (The location header created by processing this operation, populated if the operation returns a location.). This is the underlying object with id, value and extensions. The accessor "getLocation" gives direct access to the value 4293 */ 4294 public BundleEntryResponseComponent setLocationElement(UriType value) { 4295 this.location = value; 4296 return this; 4297 } 4298 4299 /** 4300 * @return The location header created by processing this operation, populated if the operation returns a location. 4301 */ 4302 public String getLocation() { 4303 return this.location == null ? null : this.location.getValue(); 4304 } 4305 4306 /** 4307 * @param value The location header created by processing this operation, populated if the operation returns a location. 4308 */ 4309 public BundleEntryResponseComponent setLocation(String value) { 4310 if (Utilities.noString(value)) 4311 this.location = null; 4312 else { 4313 if (this.location == null) 4314 this.location = new UriType(); 4315 this.location.setValue(value); 4316 } 4317 return this; 4318 } 4319 4320 /** 4321 * @return {@link #etag} (The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).). This is the underlying object with id, value and extensions. The accessor "getEtag" gives direct access to the value 4322 */ 4323 public StringType getEtagElement() { 4324 if (this.etag == null) 4325 if (Configuration.errorOnAutoCreate()) 4326 throw new Error("Attempt to auto-create BundleEntryResponseComponent.etag"); 4327 else if (Configuration.doAutoCreate()) 4328 this.etag = new StringType(); // bb 4329 return this.etag; 4330 } 4331 4332 public boolean hasEtagElement() { 4333 return this.etag != null && !this.etag.isEmpty(); 4334 } 4335 4336 public boolean hasEtag() { 4337 return this.etag != null && !this.etag.isEmpty(); 4338 } 4339 4340 /** 4341 * @param value {@link #etag} (The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).). This is the underlying object with id, value and extensions. The accessor "getEtag" gives direct access to the value 4342 */ 4343 public BundleEntryResponseComponent setEtagElement(StringType value) { 4344 this.etag = value; 4345 return this; 4346 } 4347 4348 /** 4349 * @return The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)). 4350 */ 4351 public String getEtag() { 4352 return this.etag == null ? null : this.etag.getValue(); 4353 } 4354 4355 /** 4356 * @param value The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)). 4357 */ 4358 public BundleEntryResponseComponent setEtag(String value) { 4359 if (Utilities.noString(value)) 4360 this.etag = null; 4361 else { 4362 if (this.etag == null) 4363 this.etag = new StringType(); 4364 this.etag.setValue(value); 4365 } 4366 return this; 4367 } 4368 4369 /** 4370 * @return {@link #lastModified} (The date/time that the resource was modified on the server.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 4371 */ 4372 public InstantType getLastModifiedElement() { 4373 if (this.lastModified == null) 4374 if (Configuration.errorOnAutoCreate()) 4375 throw new Error("Attempt to auto-create BundleEntryResponseComponent.lastModified"); 4376 else if (Configuration.doAutoCreate()) 4377 this.lastModified = new InstantType(); // bb 4378 return this.lastModified; 4379 } 4380 4381 public boolean hasLastModifiedElement() { 4382 return this.lastModified != null && !this.lastModified.isEmpty(); 4383 } 4384 4385 public boolean hasLastModified() { 4386 return this.lastModified != null && !this.lastModified.isEmpty(); 4387 } 4388 4389 /** 4390 * @param value {@link #lastModified} (The date/time that the resource was modified on the server.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 4391 */ 4392 public BundleEntryResponseComponent setLastModifiedElement(InstantType value) { 4393 this.lastModified = value; 4394 return this; 4395 } 4396 4397 /** 4398 * @return The date/time that the resource was modified on the server. 4399 */ 4400 public Date getLastModified() { 4401 return this.lastModified == null ? null : this.lastModified.getValue(); 4402 } 4403 4404 /** 4405 * @param value The date/time that the resource was modified on the server. 4406 */ 4407 public BundleEntryResponseComponent setLastModified(Date value) { 4408 if (value == null) 4409 this.lastModified = null; 4410 else { 4411 if (this.lastModified == null) 4412 this.lastModified = new InstantType(); 4413 this.lastModified.setValue(value); 4414 } 4415 return this; 4416 } 4417 4418 /** 4419 * @return {@link #outcome} (An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.) 4420 */ 4421 public Resource getOutcome() { 4422 return this.outcome; 4423 } 4424 4425 public boolean hasOutcome() { 4426 return this.outcome != null && !this.outcome.isEmpty(); 4427 } 4428 4429 /** 4430 * @param value {@link #outcome} (An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.) 4431 */ 4432 public BundleEntryResponseComponent setOutcome(Resource value) { 4433 this.outcome = value; 4434 return this; 4435 } 4436 4437 protected void listChildren(List<Property> children) { 4438 super.listChildren(children); 4439 children.add(new Property("status", "string", "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.", 0, 1, status)); 4440 children.add(new Property("location", "uri", "The location header created by processing this operation, populated if the operation returns a location.", 0, 1, location)); 4441 children.add(new Property("etag", "string", "The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).", 0, 1, etag)); 4442 children.add(new Property("lastModified", "instant", "The date/time that the resource was modified on the server.", 0, 1, lastModified)); 4443 children.add(new Property("outcome", "Resource", "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.", 0, 1, outcome)); 4444 } 4445 4446 @Override 4447 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4448 switch (_hash) { 4449 case -892481550: /*status*/ return new Property("status", "string", "The status code returned by processing this entry. The status SHALL start with a 3 digit HTTP code (e.g. 404) and may contain the standard HTTP description associated with the status code.", 0, 1, status); 4450 case 1901043637: /*location*/ return new Property("location", "uri", "The location header created by processing this operation, populated if the operation returns a location.", 0, 1, location); 4451 case 3123477: /*etag*/ return new Property("etag", "string", "The Etag for the resource, if the operation for the entry produced a versioned resource (see [Resource Metadata and Versioning](http.html#versioning) and [Managing Resource Contention](http.html#concurrency)).", 0, 1, etag); 4452 case 1959003007: /*lastModified*/ return new Property("lastModified", "instant", "The date/time that the resource was modified on the server.", 0, 1, lastModified); 4453 case -1106507950: /*outcome*/ return new Property("outcome", "Resource", "An OperationOutcome containing hints and warnings produced as part of processing this entry in a batch or transaction.", 0, 1, outcome); 4454 default: return super.getNamedProperty(_hash, _name, _checkValid); 4455 } 4456 4457 } 4458 4459 @Override 4460 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4461 switch (hash) { 4462 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // StringType 4463 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // UriType 4464 case 3123477: /*etag*/ return this.etag == null ? new Base[0] : new Base[] {this.etag}; // StringType 4465 case 1959003007: /*lastModified*/ return this.lastModified == null ? new Base[0] : new Base[] {this.lastModified}; // InstantType 4466 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Resource 4467 default: return super.getProperty(hash, name, checkValid); 4468 } 4469 4470 } 4471 4472 @Override 4473 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4474 switch (hash) { 4475 case -892481550: // status 4476 this.status = TypeConvertor.castToString(value); // StringType 4477 return value; 4478 case 1901043637: // location 4479 this.location = TypeConvertor.castToUri(value); // UriType 4480 return value; 4481 case 3123477: // etag 4482 this.etag = TypeConvertor.castToString(value); // StringType 4483 return value; 4484 case 1959003007: // lastModified 4485 this.lastModified = TypeConvertor.castToInstant(value); // InstantType 4486 return value; 4487 case -1106507950: // outcome 4488 this.outcome = TypeConvertor.castToResource(value); // Resource 4489 return value; 4490 default: return super.setProperty(hash, name, value); 4491 } 4492 4493 } 4494 4495 @Override 4496 public Base setProperty(String name, Base value) throws FHIRException { 4497 if (name.equals("status")) { 4498 this.status = TypeConvertor.castToString(value); // StringType 4499 } else if (name.equals("location")) { 4500 this.location = TypeConvertor.castToUri(value); // UriType 4501 } else if (name.equals("etag")) { 4502 this.etag = TypeConvertor.castToString(value); // StringType 4503 } else if (name.equals("lastModified")) { 4504 this.lastModified = TypeConvertor.castToInstant(value); // InstantType 4505 } else if (name.equals("outcome")) { 4506 this.outcome = TypeConvertor.castToResource(value); // Resource 4507 } else 4508 return super.setProperty(name, value); 4509 return value; 4510 } 4511 4512 @Override 4513 public void removeChild(String name, Base value) throws FHIRException { 4514 if (name.equals("status")) { 4515 this.status = null; 4516 } else if (name.equals("location")) { 4517 this.location = null; 4518 } else if (name.equals("etag")) { 4519 this.etag = null; 4520 } else if (name.equals("lastModified")) { 4521 this.lastModified = null; 4522 } else if (name.equals("outcome")) { 4523 this.outcome = null; 4524 } else 4525 super.removeChild(name, value); 4526 4527 } 4528 4529 @Override 4530 public Base makeProperty(int hash, String name) throws FHIRException { 4531 switch (hash) { 4532 case -892481550: return getStatusElement(); 4533 case 1901043637: return getLocationElement(); 4534 case 3123477: return getEtagElement(); 4535 case 1959003007: return getLastModifiedElement(); 4536 case -1106507950: throw new FHIRException("Cannot make property outcome as it is not a complex type"); // Resource 4537 default: return super.makeProperty(hash, name); 4538 } 4539 4540 } 4541 4542 @Override 4543 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4544 switch (hash) { 4545 case -892481550: /*status*/ return new String[] {"string"}; 4546 case 1901043637: /*location*/ return new String[] {"uri"}; 4547 case 3123477: /*etag*/ return new String[] {"string"}; 4548 case 1959003007: /*lastModified*/ return new String[] {"instant"}; 4549 case -1106507950: /*outcome*/ return new String[] {"Resource"}; 4550 default: return super.getTypesForProperty(hash, name); 4551 } 4552 4553 } 4554 4555 @Override 4556 public Base addChild(String name) throws FHIRException { 4557 if (name.equals("status")) { 4558 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.response.status"); 4559 } 4560 else if (name.equals("location")) { 4561 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.response.location"); 4562 } 4563 else if (name.equals("etag")) { 4564 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.response.etag"); 4565 } 4566 else if (name.equals("lastModified")) { 4567 throw new FHIRException("Cannot call addChild on a singleton property Bundle.entry.response.lastModified"); 4568 } 4569 else if (name.equals("outcome")) { 4570 throw new FHIRException("Cannot call addChild on an abstract type Bundle.entry.response.outcome"); 4571 } 4572 else 4573 return super.addChild(name); 4574 } 4575 4576 public BundleEntryResponseComponent copy() { 4577 BundleEntryResponseComponent dst = new BundleEntryResponseComponent(); 4578 copyValues(dst); 4579 return dst; 4580 } 4581 4582 public void copyValues(BundleEntryResponseComponent dst) { 4583 super.copyValues(dst); 4584 dst.status = status == null ? null : status.copy(); 4585 dst.location = location == null ? null : location.copy(); 4586 dst.etag = etag == null ? null : etag.copy(); 4587 dst.lastModified = lastModified == null ? null : lastModified.copy(); 4588 dst.outcome = outcome == null ? null : outcome.copy(); 4589 } 4590 4591 @Override 4592 public boolean equalsDeep(Base other_) { 4593 if (!super.equalsDeep(other_)) 4594 return false; 4595 if (!(other_ instanceof BundleEntryResponseComponent)) 4596 return false; 4597 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other_; 4598 return compareDeep(status, o.status, true) && compareDeep(location, o.location, true) && compareDeep(etag, o.etag, true) 4599 && compareDeep(lastModified, o.lastModified, true) && compareDeep(outcome, o.outcome, true); 4600 } 4601 4602 @Override 4603 public boolean equalsShallow(Base other_) { 4604 if (!super.equalsShallow(other_)) 4605 return false; 4606 if (!(other_ instanceof BundleEntryResponseComponent)) 4607 return false; 4608 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other_; 4609 return compareValues(status, o.status, true) && compareValues(location, o.location, true) && compareValues(etag, o.etag, true) 4610 && compareValues(lastModified, o.lastModified, true); 4611 } 4612 4613 public boolean isEmpty() { 4614 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, location, etag, lastModified 4615 , outcome); 4616 } 4617 4618 public String fhirType() { 4619 return "Bundle.entry.response"; 4620 4621 } 4622 4623 } 4624 4625 /** 4626 * A persistent identifier for the bundle that won't change as a bundle is copied from server to server. 4627 */ 4628 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 4629 @Description(shortDefinition="Persistent identifier for the bundle", formalDefinition="A persistent identifier for the bundle that won't change as a bundle is copied from server to server." ) 4630 protected Identifier identifier; 4631 4632 /** 4633 * Indicates the purpose of this bundle - how it is intended to be used. 4634 */ 4635 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4636 @Description(shortDefinition="document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection | subscription-notification", formalDefinition="Indicates the purpose of this bundle - how it is intended to be used." ) 4637 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bundle-type") 4638 protected Enumeration<BundleType> type; 4639 4640 /** 4641 * The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle. 4642 */ 4643 @Child(name = "timestamp", type = {InstantType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4644 @Description(shortDefinition="When the bundle was assembled", formalDefinition="The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle." ) 4645 protected InstantType timestamp; 4646 4647 /** 4648 * If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle. 4649 */ 4650 @Child(name = "total", type = {UnsignedIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4651 @Description(shortDefinition="If search, the total number of matches", formalDefinition="If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle." ) 4652 protected UnsignedIntType total; 4653 4654 /** 4655 * A series of links that provide context to this bundle. 4656 */ 4657 @Child(name = "link", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4658 @Description(shortDefinition="Links related to this Bundle", formalDefinition="A series of links that provide context to this bundle." ) 4659 protected List<BundleLinkComponent> link; 4660 4661 /** 4662 * An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only). 4663 */ 4664 @Child(name = "entry", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4665 @Description(shortDefinition="Entry in the bundle - will have a resource or information", formalDefinition="An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only)." ) 4666 protected List<BundleEntryComponent> entry; 4667 4668 /** 4669 * Digital Signature - base64 encoded. XML-DSig or a JWS. 4670 */ 4671 @Child(name = "signature", type = {Signature.class}, order=6, min=0, max=1, modifier=false, summary=true) 4672 @Description(shortDefinition="Digital Signature", formalDefinition="Digital Signature - base64 encoded. XML-DSig or a JWS." ) 4673 protected Signature signature; 4674 4675 /** 4676 * Captures issues and warnings that relate to the construction of the Bundle and the content within it. 4677 */ 4678 @Child(name = "issues", type = {Resource.class}, order=7, min=0, max=1, modifier=false, summary=true) 4679 @Description(shortDefinition="Issues with the Bundle", formalDefinition="Captures issues and warnings that relate to the construction of the Bundle and the content within it." ) 4680 protected Resource issues; 4681 4682 private static final long serialVersionUID = -843739668L; 4683 4684 /** 4685 * Constructor 4686 */ 4687 public Bundle() { 4688 super(); 4689 } 4690 4691 /** 4692 * Constructor 4693 */ 4694 public Bundle(BundleType type) { 4695 super(); 4696 this.setType(type); 4697 } 4698 4699 /** 4700 * @return {@link #identifier} (A persistent identifier for the bundle that won't change as a bundle is copied from server to server.) 4701 */ 4702 public Identifier getIdentifier() { 4703 if (this.identifier == null) 4704 if (Configuration.errorOnAutoCreate()) 4705 throw new Error("Attempt to auto-create Bundle.identifier"); 4706 else if (Configuration.doAutoCreate()) 4707 this.identifier = new Identifier(); // cc 4708 return this.identifier; 4709 } 4710 4711 public boolean hasIdentifier() { 4712 return this.identifier != null && !this.identifier.isEmpty(); 4713 } 4714 4715 /** 4716 * @param value {@link #identifier} (A persistent identifier for the bundle that won't change as a bundle is copied from server to server.) 4717 */ 4718 public Bundle setIdentifier(Identifier value) { 4719 this.identifier = value; 4720 return this; 4721 } 4722 4723 /** 4724 * @return {@link #type} (Indicates the purpose of this bundle - how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4725 */ 4726 public Enumeration<BundleType> getTypeElement() { 4727 if (this.type == null) 4728 if (Configuration.errorOnAutoCreate()) 4729 throw new Error("Attempt to auto-create Bundle.type"); 4730 else if (Configuration.doAutoCreate()) 4731 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); // bb 4732 return this.type; 4733 } 4734 4735 public boolean hasTypeElement() { 4736 return this.type != null && !this.type.isEmpty(); 4737 } 4738 4739 public boolean hasType() { 4740 return this.type != null && !this.type.isEmpty(); 4741 } 4742 4743 /** 4744 * @param value {@link #type} (Indicates the purpose of this bundle - how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4745 */ 4746 public Bundle setTypeElement(Enumeration<BundleType> value) { 4747 this.type = value; 4748 return this; 4749 } 4750 4751 /** 4752 * @return Indicates the purpose of this bundle - how it is intended to be used. 4753 */ 4754 public BundleType getType() { 4755 return this.type == null ? null : this.type.getValue(); 4756 } 4757 4758 /** 4759 * @param value Indicates the purpose of this bundle - how it is intended to be used. 4760 */ 4761 public Bundle setType(BundleType value) { 4762 if (this.type == null) 4763 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); 4764 this.type.setValue(value); 4765 return this; 4766 } 4767 4768 /** 4769 * @return {@link #timestamp} (The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 4770 */ 4771 public InstantType getTimestampElement() { 4772 if (this.timestamp == null) 4773 if (Configuration.errorOnAutoCreate()) 4774 throw new Error("Attempt to auto-create Bundle.timestamp"); 4775 else if (Configuration.doAutoCreate()) 4776 this.timestamp = new InstantType(); // bb 4777 return this.timestamp; 4778 } 4779 4780 public boolean hasTimestampElement() { 4781 return this.timestamp != null && !this.timestamp.isEmpty(); 4782 } 4783 4784 public boolean hasTimestamp() { 4785 return this.timestamp != null && !this.timestamp.isEmpty(); 4786 } 4787 4788 /** 4789 * @param value {@link #timestamp} (The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 4790 */ 4791 public Bundle setTimestampElement(InstantType value) { 4792 this.timestamp = value; 4793 return this; 4794 } 4795 4796 /** 4797 * @return The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle. 4798 */ 4799 public Date getTimestamp() { 4800 return this.timestamp == null ? null : this.timestamp.getValue(); 4801 } 4802 4803 /** 4804 * @param value The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle. 4805 */ 4806 public Bundle setTimestamp(Date value) { 4807 if (value == null) 4808 this.timestamp = null; 4809 else { 4810 if (this.timestamp == null) 4811 this.timestamp = new InstantType(); 4812 this.timestamp.setValue(value); 4813 } 4814 return this; 4815 } 4816 4817 /** 4818 * @return {@link #total} (If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 4819 */ 4820 public UnsignedIntType getTotalElement() { 4821 if (this.total == null) 4822 if (Configuration.errorOnAutoCreate()) 4823 throw new Error("Attempt to auto-create Bundle.total"); 4824 else if (Configuration.doAutoCreate()) 4825 this.total = new UnsignedIntType(); // bb 4826 return this.total; 4827 } 4828 4829 public boolean hasTotalElement() { 4830 return this.total != null && !this.total.isEmpty(); 4831 } 4832 4833 public boolean hasTotal() { 4834 return this.total != null && !this.total.isEmpty(); 4835 } 4836 4837 /** 4838 * @param value {@link #total} (If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 4839 */ 4840 public Bundle setTotalElement(UnsignedIntType value) { 4841 this.total = value; 4842 return this; 4843 } 4844 4845 /** 4846 * @return If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle. 4847 */ 4848 public int getTotal() { 4849 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 4850 } 4851 4852 /** 4853 * @param value If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle. 4854 */ 4855 public Bundle setTotal(int value) { 4856 if (this.total == null) 4857 this.total = new UnsignedIntType(); 4858 this.total.setValue(value); 4859 return this; 4860 } 4861 4862 /** 4863 * @return {@link #link} (A series of links that provide context to this bundle.) 4864 */ 4865 public List<BundleLinkComponent> getLink() { 4866 if (this.link == null) 4867 this.link = new ArrayList<BundleLinkComponent>(); 4868 return this.link; 4869 } 4870 4871 /** 4872 * @return Returns a reference to <code>this</code> for easy method chaining 4873 */ 4874 public Bundle setLink(List<BundleLinkComponent> theLink) { 4875 this.link = theLink; 4876 return this; 4877 } 4878 4879 public boolean hasLink() { 4880 if (this.link == null) 4881 return false; 4882 for (BundleLinkComponent item : this.link) 4883 if (!item.isEmpty()) 4884 return true; 4885 return false; 4886 } 4887 4888 public BundleLinkComponent addLink() { //3 4889 BundleLinkComponent t = new BundleLinkComponent(); 4890 if (this.link == null) 4891 this.link = new ArrayList<BundleLinkComponent>(); 4892 this.link.add(t); 4893 return t; 4894 } 4895 4896 public Bundle addLink(BundleLinkComponent t) { //3 4897 if (t == null) 4898 return this; 4899 if (this.link == null) 4900 this.link = new ArrayList<BundleLinkComponent>(); 4901 this.link.add(t); 4902 return this; 4903 } 4904 4905 /** 4906 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 4907 */ 4908 public BundleLinkComponent getLinkFirstRep() { 4909 if (getLink().isEmpty()) { 4910 addLink(); 4911 } 4912 return getLink().get(0); 4913 } 4914 4915 /** 4916 * @return {@link #entry} (An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).) 4917 */ 4918 public List<BundleEntryComponent> getEntry() { 4919 if (this.entry == null) 4920 this.entry = new ArrayList<BundleEntryComponent>(); 4921 return this.entry; 4922 } 4923 4924 /** 4925 * @return Returns a reference to <code>this</code> for easy method chaining 4926 */ 4927 public Bundle setEntry(List<BundleEntryComponent> theEntry) { 4928 this.entry = theEntry; 4929 return this; 4930 } 4931 4932 public boolean hasEntry() { 4933 if (this.entry == null) 4934 return false; 4935 for (BundleEntryComponent item : this.entry) 4936 if (!item.isEmpty()) 4937 return true; 4938 return false; 4939 } 4940 4941 public BundleEntryComponent addEntry() { //3 4942 BundleEntryComponent t = new BundleEntryComponent(); 4943 if (this.entry == null) 4944 this.entry = new ArrayList<BundleEntryComponent>(); 4945 this.entry.add(t); 4946 return t; 4947 } 4948 4949 public Bundle addEntry(BundleEntryComponent t) { //3 4950 if (t == null) 4951 return this; 4952 if (this.entry == null) 4953 this.entry = new ArrayList<BundleEntryComponent>(); 4954 this.entry.add(t); 4955 return this; 4956 } 4957 4958 /** 4959 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist {3} 4960 */ 4961 public BundleEntryComponent getEntryFirstRep() { 4962 if (getEntry().isEmpty()) { 4963 addEntry(); 4964 } 4965 return getEntry().get(0); 4966 } 4967 4968 /** 4969 * @return {@link #signature} (Digital Signature - base64 encoded. XML-DSig or a JWS.) 4970 */ 4971 public Signature getSignature() { 4972 if (this.signature == null) 4973 if (Configuration.errorOnAutoCreate()) 4974 throw new Error("Attempt to auto-create Bundle.signature"); 4975 else if (Configuration.doAutoCreate()) 4976 this.signature = new Signature(); // cc 4977 return this.signature; 4978 } 4979 4980 public boolean hasSignature() { 4981 return this.signature != null && !this.signature.isEmpty(); 4982 } 4983 4984 /** 4985 * @param value {@link #signature} (Digital Signature - base64 encoded. XML-DSig or a JWS.) 4986 */ 4987 public Bundle setSignature(Signature value) { 4988 this.signature = value; 4989 return this; 4990 } 4991 4992 /** 4993 * @return {@link #issues} (Captures issues and warnings that relate to the construction of the Bundle and the content within it.) 4994 */ 4995 public Resource getIssues() { 4996 return this.issues; 4997 } 4998 4999 public boolean hasIssues() { 5000 return this.issues != null && !this.issues.isEmpty(); 5001 } 5002 5003 /** 5004 * @param value {@link #issues} (Captures issues and warnings that relate to the construction of the Bundle and the content within it.) 5005 */ 5006 public Bundle setIssues(Resource value) { 5007 this.issues = value; 5008 return this; 5009 } 5010 5011 protected void listChildren(List<Property> children) { 5012 super.listChildren(children); 5013 children.add(new Property("identifier", "Identifier", "A persistent identifier for the bundle that won't change as a bundle is copied from server to server.", 0, 1, identifier)); 5014 children.add(new Property("type", "code", "Indicates the purpose of this bundle - how it is intended to be used.", 0, 1, type)); 5015 children.add(new Property("timestamp", "instant", "The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.", 0, 1, timestamp)); 5016 children.add(new Property("total", "unsignedInt", "If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.", 0, 1, total)); 5017 children.add(new Property("link", "", "A series of links that provide context to this bundle.", 0, java.lang.Integer.MAX_VALUE, link)); 5018 children.add(new Property("entry", "", "An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).", 0, java.lang.Integer.MAX_VALUE, entry)); 5019 children.add(new Property("signature", "Signature", "Digital Signature - base64 encoded. XML-DSig or a JWS.", 0, 1, signature)); 5020 children.add(new Property("issues", "Resource", "Captures issues and warnings that relate to the construction of the Bundle and the content within it.", 0, 1, issues)); 5021 } 5022 5023 @Override 5024 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5025 switch (_hash) { 5026 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A persistent identifier for the bundle that won't change as a bundle is copied from server to server.", 0, 1, identifier); 5027 case 3575610: /*type*/ return new Property("type", "code", "Indicates the purpose of this bundle - how it is intended to be used.", 0, 1, type); 5028 case 55126294: /*timestamp*/ return new Property("timestamp", "instant", "The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.", 0, 1, timestamp); 5029 case 110549828: /*total*/ return new Property("total", "unsignedInt", "If a set of search matches, this is the (potentially estimated) total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.", 0, 1, total); 5030 case 3321850: /*link*/ return new Property("link", "", "A series of links that provide context to this bundle.", 0, java.lang.Integer.MAX_VALUE, link); 5031 case 96667762: /*entry*/ return new Property("entry", "", "An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).", 0, java.lang.Integer.MAX_VALUE, entry); 5032 case 1073584312: /*signature*/ return new Property("signature", "Signature", "Digital Signature - base64 encoded. XML-DSig or a JWS.", 0, 1, signature); 5033 case -1179159878: /*issues*/ return new Property("issues", "Resource", "Captures issues and warnings that relate to the construction of the Bundle and the content within it.", 0, 1, issues); 5034 default: return super.getNamedProperty(_hash, _name, _checkValid); 5035 } 5036 5037 } 5038 5039 @Override 5040 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5041 switch (hash) { 5042 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 5043 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<BundleType> 5044 case 55126294: /*timestamp*/ return this.timestamp == null ? new Base[0] : new Base[] {this.timestamp}; // InstantType 5045 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // UnsignedIntType 5046 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // BundleLinkComponent 5047 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // BundleEntryComponent 5048 case 1073584312: /*signature*/ return this.signature == null ? new Base[0] : new Base[] {this.signature}; // Signature 5049 case -1179159878: /*issues*/ return this.issues == null ? new Base[0] : new Base[] {this.issues}; // Resource 5050 default: return super.getProperty(hash, name, checkValid); 5051 } 5052 5053 } 5054 5055 @Override 5056 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5057 switch (hash) { 5058 case -1618432855: // identifier 5059 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 5060 return value; 5061 case 3575610: // type 5062 value = new BundleTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5063 this.type = (Enumeration) value; // Enumeration<BundleType> 5064 return value; 5065 case 55126294: // timestamp 5066 this.timestamp = TypeConvertor.castToInstant(value); // InstantType 5067 return value; 5068 case 110549828: // total 5069 this.total = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 5070 return value; 5071 case 3321850: // link 5072 this.getLink().add((BundleLinkComponent) value); // BundleLinkComponent 5073 return value; 5074 case 96667762: // entry 5075 this.getEntry().add((BundleEntryComponent) value); // BundleEntryComponent 5076 return value; 5077 case 1073584312: // signature 5078 this.signature = TypeConvertor.castToSignature(value); // Signature 5079 return value; 5080 case -1179159878: // issues 5081 this.issues = TypeConvertor.castToResource(value); // Resource 5082 return value; 5083 default: return super.setProperty(hash, name, value); 5084 } 5085 5086 } 5087 5088 @Override 5089 public Base setProperty(String name, Base value) throws FHIRException { 5090 if (name.equals("identifier")) { 5091 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 5092 } else if (name.equals("type")) { 5093 value = new BundleTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5094 this.type = (Enumeration) value; // Enumeration<BundleType> 5095 } else if (name.equals("timestamp")) { 5096 this.timestamp = TypeConvertor.castToInstant(value); // InstantType 5097 } else if (name.equals("total")) { 5098 this.total = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 5099 } else if (name.equals("link")) { 5100 this.getLink().add((BundleLinkComponent) value); 5101 } else if (name.equals("entry")) { 5102 this.getEntry().add((BundleEntryComponent) value); 5103 } else if (name.equals("signature")) { 5104 this.signature = TypeConvertor.castToSignature(value); // Signature 5105 } else if (name.equals("issues")) { 5106 this.issues = TypeConvertor.castToResource(value); // Resource 5107 } else 5108 return super.setProperty(name, value); 5109 return value; 5110 } 5111 5112 @Override 5113 public void removeChild(String name, Base value) throws FHIRException { 5114 if (name.equals("identifier")) { 5115 this.identifier = null; 5116 } else if (name.equals("type")) { 5117 value = new BundleTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5118 this.type = (Enumeration) value; // Enumeration<BundleType> 5119 } else if (name.equals("timestamp")) { 5120 this.timestamp = null; 5121 } else if (name.equals("total")) { 5122 this.total = null; 5123 } else if (name.equals("link")) { 5124 this.getLink().remove((BundleLinkComponent) value); 5125 } else if (name.equals("entry")) { 5126 this.getEntry().remove((BundleEntryComponent) value); 5127 } else if (name.equals("signature")) { 5128 this.signature = null; 5129 } else if (name.equals("issues")) { 5130 this.issues = null; 5131 } else 5132 super.removeChild(name, value); 5133 5134 } 5135 5136 @Override 5137 public Base makeProperty(int hash, String name) throws FHIRException { 5138 switch (hash) { 5139 case -1618432855: return getIdentifier(); 5140 case 3575610: return getTypeElement(); 5141 case 55126294: return getTimestampElement(); 5142 case 110549828: return getTotalElement(); 5143 case 3321850: return addLink(); 5144 case 96667762: return addEntry(); 5145 case 1073584312: return getSignature(); 5146 case -1179159878: throw new FHIRException("Cannot make property issues as it is not a complex type"); // Resource 5147 default: return super.makeProperty(hash, name); 5148 } 5149 5150 } 5151 5152 @Override 5153 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5154 switch (hash) { 5155 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5156 case 3575610: /*type*/ return new String[] {"code"}; 5157 case 55126294: /*timestamp*/ return new String[] {"instant"}; 5158 case 110549828: /*total*/ return new String[] {"unsignedInt"}; 5159 case 3321850: /*link*/ return new String[] {}; 5160 case 96667762: /*entry*/ return new String[] {}; 5161 case 1073584312: /*signature*/ return new String[] {"Signature"}; 5162 case -1179159878: /*issues*/ return new String[] {"Resource"}; 5163 default: return super.getTypesForProperty(hash, name); 5164 } 5165 5166 } 5167 5168 @Override 5169 public Base addChild(String name) throws FHIRException { 5170 if (name.equals("identifier")) { 5171 this.identifier = new Identifier(); 5172 return this.identifier; 5173 } 5174 else if (name.equals("type")) { 5175 throw new FHIRException("Cannot call addChild on a singleton property Bundle.type"); 5176 } 5177 else if (name.equals("timestamp")) { 5178 throw new FHIRException("Cannot call addChild on a singleton property Bundle.timestamp"); 5179 } 5180 else if (name.equals("total")) { 5181 throw new FHIRException("Cannot call addChild on a singleton property Bundle.total"); 5182 } 5183 else if (name.equals("link")) { 5184 return addLink(); 5185 } 5186 else if (name.equals("entry")) { 5187 return addEntry(); 5188 } 5189 else if (name.equals("signature")) { 5190 this.signature = new Signature(); 5191 return this.signature; 5192 } 5193 else if (name.equals("issues")) { 5194 throw new FHIRException("Cannot call addChild on an abstract type Bundle.issues"); 5195 } 5196 else 5197 return super.addChild(name); 5198 } 5199 5200 public String fhirType() { 5201 return "Bundle"; 5202 5203 } 5204 5205 public Bundle copy() { 5206 Bundle dst = new Bundle(); 5207 copyValues(dst); 5208 return dst; 5209 } 5210 5211 public void copyValues(Bundle dst) { 5212 super.copyValues(dst); 5213 dst.identifier = identifier == null ? null : identifier.copy(); 5214 dst.type = type == null ? null : type.copy(); 5215 dst.timestamp = timestamp == null ? null : timestamp.copy(); 5216 dst.total = total == null ? null : total.copy(); 5217 if (link != null) { 5218 dst.link = new ArrayList<BundleLinkComponent>(); 5219 for (BundleLinkComponent i : link) 5220 dst.link.add(i.copy()); 5221 }; 5222 if (entry != null) { 5223 dst.entry = new ArrayList<BundleEntryComponent>(); 5224 for (BundleEntryComponent i : entry) 5225 dst.entry.add(i.copy()); 5226 }; 5227 dst.signature = signature == null ? null : signature.copy(); 5228 dst.issues = issues == null ? null : issues.copy(); 5229 } 5230 5231 protected Bundle typedCopy() { 5232 return copy(); 5233 } 5234 5235 @Override 5236 public boolean equalsDeep(Base other_) { 5237 if (!super.equalsDeep(other_)) 5238 return false; 5239 if (!(other_ instanceof Bundle)) 5240 return false; 5241 Bundle o = (Bundle) other_; 5242 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(timestamp, o.timestamp, true) 5243 && compareDeep(total, o.total, true) && compareDeep(link, o.link, true) && compareDeep(entry, o.entry, true) 5244 && compareDeep(signature, o.signature, true) && compareDeep(issues, o.issues, true); 5245 } 5246 5247 @Override 5248 public boolean equalsShallow(Base other_) { 5249 if (!super.equalsShallow(other_)) 5250 return false; 5251 if (!(other_ instanceof Bundle)) 5252 return false; 5253 Bundle o = (Bundle) other_; 5254 return compareValues(type, o.type, true) && compareValues(timestamp, o.timestamp, true) && compareValues(total, o.total, true) 5255 ; 5256 } 5257 5258 public boolean isEmpty() { 5259 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, timestamp 5260 , total, link, entry, signature, issues); 5261 } 5262 5263 @Override 5264 public ResourceType getResourceType() { 5265 return ResourceType.Bundle; 5266 } 5267 5268 /** 5269 * Search parameter: <b>composition</b> 5270 * <p> 5271 * Description: <b>The first resource in the bundle, if the bundle type is "document" - this is a composition, and this parameter provides access to search its contents</b><br> 5272 * Type: <b>reference</b><br> 5273 * Path: <b>Bundle.entry[0].resource as Composition</b><br> 5274 * </p> 5275 */ 5276 @SearchParamDefinition(name="composition", path="Bundle.entry[0].resource as Composition", description="The first resource in the bundle, if the bundle type is \"document\" - this is a composition, and this parameter provides access to search its contents", type="reference", target={Composition.class } ) 5277 public static final String SP_COMPOSITION = "composition"; 5278 /** 5279 * <b>Fluent Client</b> search parameter constant for <b>composition</b> 5280 * <p> 5281 * Description: <b>The first resource in the bundle, if the bundle type is "document" - this is a composition, and this parameter provides access to search its contents</b><br> 5282 * Type: <b>reference</b><br> 5283 * Path: <b>Bundle.entry[0].resource as Composition</b><br> 5284 * </p> 5285 */ 5286 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSITION); 5287 5288/** 5289 * Constant for fluent queries to be used to add include statements. Specifies 5290 * the path value of "<b>Bundle:composition</b>". 5291 */ 5292 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSITION = new ca.uhn.fhir.model.api.Include("Bundle:composition").toLocked(); 5293 5294 /** 5295 * Search parameter: <b>identifier</b> 5296 * <p> 5297 * Description: <b>Persistent identifier for the bundle</b><br> 5298 * Type: <b>token</b><br> 5299 * Path: <b>Bundle.identifier</b><br> 5300 * </p> 5301 */ 5302 @SearchParamDefinition(name="identifier", path="Bundle.identifier", description="Persistent identifier for the bundle", type="token" ) 5303 public static final String SP_IDENTIFIER = "identifier"; 5304 /** 5305 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5306 * <p> 5307 * Description: <b>Persistent identifier for the bundle</b><br> 5308 * Type: <b>token</b><br> 5309 * Path: <b>Bundle.identifier</b><br> 5310 * </p> 5311 */ 5312 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5313 5314 /** 5315 * Search parameter: <b>message</b> 5316 * <p> 5317 * Description: <b>The first resource in the bundle, if the bundle type is "message" - this is a message header, and this parameter provides access to search its contents</b><br> 5318 * Type: <b>reference</b><br> 5319 * Path: <b>Bundle.entry[0].resource as MessageHeader</b><br> 5320 * </p> 5321 */ 5322 @SearchParamDefinition(name="message", path="Bundle.entry[0].resource as MessageHeader", description="The first resource in the bundle, if the bundle type is \"message\" - this is a message header, and this parameter provides access to search its contents", type="reference", target={MessageHeader.class } ) 5323 public static final String SP_MESSAGE = "message"; 5324 /** 5325 * <b>Fluent Client</b> search parameter constant for <b>message</b> 5326 * <p> 5327 * Description: <b>The first resource in the bundle, if the bundle type is "message" - this is a message header, and this parameter provides access to search its contents</b><br> 5328 * Type: <b>reference</b><br> 5329 * Path: <b>Bundle.entry[0].resource as MessageHeader</b><br> 5330 * </p> 5331 */ 5332 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MESSAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MESSAGE); 5333 5334/** 5335 * Constant for fluent queries to be used to add include statements. Specifies 5336 * the path value of "<b>Bundle:message</b>". 5337 */ 5338 public static final ca.uhn.fhir.model.api.Include INCLUDE_MESSAGE = new ca.uhn.fhir.model.api.Include("Bundle:message").toLocked(); 5339 5340 /** 5341 * Search parameter: <b>timestamp</b> 5342 * <p> 5343 * Description: <b>When the bundle was assembled</b><br> 5344 * Type: <b>date</b><br> 5345 * Path: <b>Bundle.timestamp</b><br> 5346 * </p> 5347 */ 5348 @SearchParamDefinition(name="timestamp", path="Bundle.timestamp", description="When the bundle was assembled", type="date" ) 5349 public static final String SP_TIMESTAMP = "timestamp"; 5350 /** 5351 * <b>Fluent Client</b> search parameter constant for <b>timestamp</b> 5352 * <p> 5353 * Description: <b>When the bundle was assembled</b><br> 5354 * Type: <b>date</b><br> 5355 * Path: <b>Bundle.timestamp</b><br> 5356 * </p> 5357 */ 5358 public static final ca.uhn.fhir.rest.gclient.DateClientParam TIMESTAMP = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_TIMESTAMP); 5359 5360 /** 5361 * Search parameter: <b>type</b> 5362 * <p> 5363 * Description: <b>document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection | subscription-notification</b><br> 5364 * Type: <b>token</b><br> 5365 * Path: <b>Bundle.type</b><br> 5366 * </p> 5367 */ 5368 @SearchParamDefinition(name="type", path="Bundle.type", description="document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection | subscription-notification", type="token" ) 5369 public static final String SP_TYPE = "type"; 5370 /** 5371 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5372 * <p> 5373 * Description: <b>document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection | subscription-notification</b><br> 5374 * Type: <b>token</b><br> 5375 * Path: <b>Bundle.type</b><br> 5376 * </p> 5377 */ 5378 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 5379 5380 /** 5381 * Search parameter: <b>example-constraint</b> 5382 * <p> 5383 * Description: <b>Search Composition Bundle</b><br> 5384 * Type: <b>reference</b><br> 5385 * Path: <b>Bundle.entry[0].resource</b><br> 5386 * </p> 5387 */ 5388 @SearchParamDefinition(name="example-constraint", path="Bundle.entry[0].resource", description="Search Composition Bundle", type="reference", target={Composition.class } ) 5389 public static final String SP_EXAMPLE_CONSTRAINT = "example-constraint"; 5390 /** 5391 * <b>Fluent Client</b> search parameter constant for <b>example-constraint</b> 5392 * <p> 5393 * Description: <b>Search Composition Bundle</b><br> 5394 * Type: <b>reference</b><br> 5395 * Path: <b>Bundle.entry[0].resource</b><br> 5396 * </p> 5397 */ 5398 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EXAMPLE_CONSTRAINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EXAMPLE_CONSTRAINT); 5399 5400/** 5401 * Constant for fluent queries to be used to add include statements. Specifies 5402 * the path value of "<b>Bundle:example-constraint</b>". 5403 */ 5404 public static final ca.uhn.fhir.model.api.Include INCLUDE_EXAMPLE_CONSTRAINT = new ca.uhn.fhir.model.api.Include("Bundle:example-constraint").toLocked(); 5405 5406// Manual code (from Configuration.txt): 5407/** 5408 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 5409 * If no link is found which matches the given relation, returns <code>null</code>. If more than one 5410 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 5411 * 5412 * @param theRelation 5413 * The relation, such as \"next\", or \"self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 5414 * @return Returns a matching BundleLinkComponent, or <code>null</code> 5415 * @see IBaseBundle#LINK_NEXT 5416 * @see IBaseBundle#LINK_PREV 5417 * @see IBaseBundle#LINK_SELF 5418 */ 5419 public BundleLinkComponent getLink(String theRelation) { 5420 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 5421 for (BundleLinkComponent next : getLink()) { 5422 if (theRelation.equals(next.getRelation().toCode())) { 5423 return next; 5424 } 5425 } 5426 return null; 5427 } 5428 5429 /** 5430 * Returns the {@link #getLink() link} which matches a given {@link BundleLinkComponent#getRelation() relation}. 5431 * If no link is found which matches the given relation, creates a new BundleLinkComponent with the 5432 * given relation and adds it to this Bundle. If more than one 5433 * link is found which matches the given relation, returns the first matching BundleLinkComponent. 5434 * 5435 * @param theRelation 5436 * The relation, such as \"next\", or \"self. See the constants such as {@link IBaseBundle#LINK_SELF} and {@link IBaseBundle#LINK_NEXT}. 5437 * @return Returns a matching BundleLinkComponent, or <code>null</code> 5438 * @see IBaseBundle#LINK_NEXT 5439 * @see IBaseBundle#LINK_PREV 5440 * @see IBaseBundle#LINK_SELF 5441 */ 5442 public BundleLinkComponent getLinkOrCreate(String theRelation) { 5443 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 5444 for (BundleLinkComponent next : getLink()) { 5445 if (theRelation.equals(next.getRelation().toCode())) { 5446 return next; 5447 } 5448 } 5449 BundleLinkComponent retVal = new BundleLinkComponent(); 5450 retVal.setRelation(LinkRelationTypes.fromCode(theRelation)); 5451 getLink().add(retVal); 5452 return retVal; 5453 } 5454// end addition 5455 5456} 5457