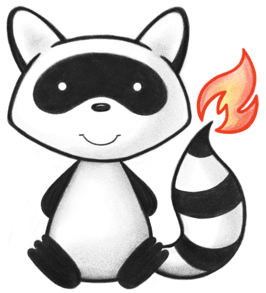
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.r5.utils.UserDataNames; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * Common Interface declaration for conformance and knowledge artifact resources. 053 */ 054public abstract class CanonicalResource extends DomainResource { 055 056 private static final long serialVersionUID = 0L; 057 058 /** 059 * Constructor 060 */ 061 public CanonicalResource() { 062 super(); 063 } 064 065 /** 066 * Constructor 067 */ 068 public CanonicalResource(PublicationStatus status) { 069 super(); 070 this.setStatus(status); 071 } 072 073 /** 074 * How many allowed for this property by the implementation 075 */ 076 public int getUrlMax() { 077 return 1; 078 } 079 /** 080 * @return {@link #url} (An absolute URI that is used to identify this canonical resource when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this canonical resource is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the canonical resource is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 081 */ 082 public abstract UriType getUrlElement(); 083 084 public abstract boolean hasUrlElement(); 085 public abstract boolean hasUrl(); 086 087 /** 088 * @param value {@link #url} (An absolute URI that is used to identify this canonical resource when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this canonical resource is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the canonical resource is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 089 */ 090 public abstract CanonicalResource setUrlElement(UriType value); 091 /** 092 * @return An absolute URI that is used to identify this canonical resource when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this canonical resource is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the canonical resource is stored on different servers. 093 */ 094 public abstract String getUrl(); 095 /** 096 * @param value An absolute URI that is used to identify this canonical resource when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this canonical resource is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the canonical resource is stored on different servers. 097 */ 098 public abstract CanonicalResource setUrl(String value); 099 /** 100 * How many allowed for this property by the implementation 101 */ 102 public int getIdentifierMax() { 103 return Integer.MAX_VALUE; 104 } 105 /** 106 * @return {@link #identifier} (A formal identifier that is used to identify this canonical resource when it is represented in other formats, or referenced in a specification, model, design or an instance.) 107 */ 108 public abstract List<Identifier> getIdentifier(); 109 /** 110 * @return Returns a reference to <code>this</code> for easy method chaining 111 */ 112 public abstract CanonicalResource setIdentifier(List<Identifier> theIdentifier); 113 public abstract boolean hasIdentifier(); 114 115 public abstract Identifier addIdentifier(); //3 116 public abstract CanonicalResource addIdentifier(Identifier t); //3 117 /** 118 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {1} 119 */ 120 public abstract Identifier getIdentifierFirstRep(); 121 /** 122 * How many allowed for this property by the implementation 123 */ 124 public int getVersionMax() { 125 return 1; 126 } 127 /** 128 * @return {@link #version} (The identifier that is used to identify this version of the canonical resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the canonical resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.)). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 129 */ 130 public abstract StringType getVersionElement(); 131 132 public abstract boolean hasVersionElement(); 133 public abstract boolean hasVersion(); 134 135 /** 136 * @param value {@link #version} (The identifier that is used to identify this version of the canonical resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the canonical resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.)). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 137 */ 138 public abstract CanonicalResource setVersionElement(StringType value); 139 /** 140 * @return The identifier that is used to identify this version of the canonical resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the canonical resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.) 141 */ 142 public abstract String getVersion(); 143 /** 144 * @param value The identifier that is used to identify this version of the canonical resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the canonical resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.) 145 */ 146 public abstract CanonicalResource setVersion(String value); 147 /** 148 * How many allowed for this property by the implementation 149 */ 150 public int getVersionAlgorithmMax() { 151 return 1; 152 } 153 /** 154 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 155 */ 156 public abstract DataType getVersionAlgorithm(); 157 /** 158 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 159 */ 160 public abstract StringType getVersionAlgorithmStringType() throws FHIRException; 161 public abstract boolean hasVersionAlgorithmStringType(); 162 /** 163 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 164 */ 165 public abstract Coding getVersionAlgorithmCoding() throws FHIRException; 166 public abstract boolean hasVersionAlgorithmCoding(); 167 public abstract boolean hasVersionAlgorithm(); 168 /** 169 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 170 */ 171 public abstract CanonicalResource setVersionAlgorithm(DataType value); 172 173 /** 174 * How many allowed for this property by the implementation 175 */ 176 public int getNameMax() { 177 return 1; 178 } 179 /** 180 * @return {@link #name} (A natural language name identifying the canonical resource. This name should be usable as an identifier for the resource by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 181 */ 182 public abstract StringType getNameElement(); 183 184 public abstract boolean hasNameElement(); 185 public abstract boolean hasName(); 186 187 /** 188 * @param value {@link #name} (A natural language name identifying the canonical resource. This name should be usable as an identifier for the resource by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 189 */ 190 public abstract CanonicalResource setNameElement(StringType value); 191 /** 192 * @return A natural language name identifying the canonical resource. This name should be usable as an identifier for the resource by machine processing applications such as code generation. 193 */ 194 public abstract String getName(); 195 /** 196 * @param value A natural language name identifying the canonical resource. This name should be usable as an identifier for the resource by machine processing applications such as code generation. 197 */ 198 public abstract CanonicalResource setName(String value); 199 /** 200 * How many allowed for this property by the implementation 201 */ 202 public int getTitleMax() { 203 return 1; 204 } 205 /** 206 * @return {@link #title} (A short, descriptive, user-friendly title for the canonical resource.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 207 */ 208 public abstract StringType getTitleElement(); 209 210 public abstract boolean hasTitleElement(); 211 public abstract boolean hasTitle(); 212 213 /** 214 * @param value {@link #title} (A short, descriptive, user-friendly title for the canonical resource.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 215 */ 216 public abstract CanonicalResource setTitleElement(StringType value); 217 /** 218 * @return A short, descriptive, user-friendly title for the canonical resource. 219 */ 220 public abstract String getTitle(); 221 /** 222 * @param value A short, descriptive, user-friendly title for the canonical resource. 223 */ 224 public abstract CanonicalResource setTitle(String value); 225 /** 226 * How many allowed for this property by the implementation 227 */ 228 public int getStatusMax() { 229 return 1; 230 } 231 /** 232 * @return {@link #status} (The current state of this canonical resource. ). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 233 */ 234 public abstract Enumeration<PublicationStatus> getStatusElement(); 235 236 public abstract boolean hasStatusElement(); 237 public abstract boolean hasStatus(); 238 239 /** 240 * @param value {@link #status} (The current state of this canonical resource. ). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 241 */ 242 public abstract CanonicalResource setStatusElement(Enumeration<PublicationStatus> value); 243 /** 244 * @return The current state of this canonical resource. 245 */ 246 public abstract PublicationStatus getStatus(); 247 /** 248 * @param value The current state of this canonical resource. 249 */ 250 public abstract CanonicalResource setStatus(PublicationStatus value); 251 /** 252 * How many allowed for this property by the implementation 253 */ 254 public int getExperimentalMax() { 255 return 1; 256 } 257 /** 258 * @return {@link #experimental} (A Boolean value to indicate that this canonical resource is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 259 */ 260 public abstract BooleanType getExperimentalElement(); 261 262 public abstract boolean hasExperimentalElement(); 263 public abstract boolean hasExperimental(); 264 265 /** 266 * @param value {@link #experimental} (A Boolean value to indicate that this canonical resource is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 267 */ 268 public abstract CanonicalResource setExperimentalElement(BooleanType value); 269 /** 270 * @return A Boolean value to indicate that this canonical resource is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 271 */ 272 public abstract boolean getExperimental(); 273 /** 274 * @param value A Boolean value to indicate that this canonical resource is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 275 */ 276 public abstract CanonicalResource setExperimental(boolean value); 277 /** 278 * How many allowed for this property by the implementation 279 */ 280 public int getDateMax() { 281 return 1; 282 } 283 /** 284 * @return {@link #date} (The date (and optionally time) when the canonical resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the canonical resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 285 */ 286 public abstract DateTimeType getDateElement(); 287 288 public abstract boolean hasDateElement(); 289 public abstract boolean hasDate(); 290 291 /** 292 * @param value {@link #date} (The date (and optionally time) when the canonical resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the canonical resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 293 */ 294 public abstract CanonicalResource setDateElement(DateTimeType value); 295 /** 296 * @return The date (and optionally time) when the canonical resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the canonical resource changes. 297 */ 298 public abstract Date getDate(); 299 /** 300 * @param value The date (and optionally time) when the canonical resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the canonical resource changes. 301 */ 302 public abstract CanonicalResource setDate(Date value); 303 /** 304 * How many allowed for this property by the implementation 305 */ 306 public int getPublisherMax() { 307 return 1; 308 } 309 /** 310 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the canonical resource.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 311 */ 312 public abstract StringType getPublisherElement(); 313 314 public abstract boolean hasPublisherElement(); 315 public abstract boolean hasPublisher(); 316 317 /** 318 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the canonical resource.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 319 */ 320 public abstract CanonicalResource setPublisherElement(StringType value); 321 /** 322 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the canonical resource. 323 */ 324 public abstract String getPublisher(); 325 /** 326 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the canonical resource. 327 */ 328 public abstract CanonicalResource setPublisher(String value); 329 /** 330 * How many allowed for this property by the implementation 331 */ 332 public int getContactMax() { 333 return Integer.MAX_VALUE; 334 } 335 /** 336 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 337 */ 338 public abstract List<ContactDetail> getContact(); 339 /** 340 * @return Returns a reference to <code>this</code> for easy method chaining 341 */ 342 public abstract CanonicalResource setContact(List<ContactDetail> theContact); 343 public abstract boolean hasContact(); 344 345 public abstract ContactDetail addContact(); //3 346 public abstract CanonicalResource addContact(ContactDetail t); //3 347 /** 348 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {1} 349 */ 350 public abstract ContactDetail getContactFirstRep(); 351 /** 352 * How many allowed for this property by the implementation 353 */ 354 public int getDescriptionMax() { 355 return 1; 356 } 357 /** 358 * @return {@link #description} (A free text natural language description of the canonical resource from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 359 */ 360 public abstract MarkdownType getDescriptionElement(); 361 362 public abstract boolean hasDescriptionElement(); 363 public abstract boolean hasDescription(); 364 365 /** 366 * @param value {@link #description} (A free text natural language description of the canonical resource from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 367 */ 368 public abstract CanonicalResource setDescriptionElement(MarkdownType value); 369 /** 370 * @return A free text natural language description of the canonical resource from a consumer's perspective. 371 */ 372 public abstract String getDescription(); 373 /** 374 * @param value A free text natural language description of the canonical resource from a consumer's perspective. 375 */ 376 public abstract CanonicalResource setDescription(String value); 377 /** 378 * How many allowed for this property by the implementation 379 */ 380 public int getUseContextMax() { 381 return Integer.MAX_VALUE; 382 } 383 /** 384 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate canonical resources.) 385 */ 386 public abstract List<UsageContext> getUseContext(); 387 /** 388 * @return Returns a reference to <code>this</code> for easy method chaining 389 */ 390 public abstract CanonicalResource setUseContext(List<UsageContext> theUseContext); 391 public abstract boolean hasUseContext(); 392 393 public abstract UsageContext addUseContext(); //3 394 public abstract CanonicalResource addUseContext(UsageContext t); //3 395 /** 396 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {1} 397 */ 398 public abstract UsageContext getUseContextFirstRep(); 399 /** 400 * How many allowed for this property by the implementation 401 */ 402 public int getJurisdictionMax() { 403 return Integer.MAX_VALUE; 404 } 405 /** 406 * @return {@link #jurisdiction} (A legal or geographic region in which the canonical resource is intended to be used.) 407 */ 408 public abstract List<CodeableConcept> getJurisdiction(); 409 /** 410 * @return Returns a reference to <code>this</code> for easy method chaining 411 */ 412 public abstract CanonicalResource setJurisdiction(List<CodeableConcept> theJurisdiction); 413 public abstract boolean hasJurisdiction(); 414 415 public abstract CodeableConcept addJurisdiction(); //3 416 public abstract CanonicalResource addJurisdiction(CodeableConcept t); //3 417 /** 418 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {1} 419 */ 420 public abstract CodeableConcept getJurisdictionFirstRep(); 421 /** 422 * How many allowed for this property by the implementation 423 */ 424 public int getPurposeMax() { 425 return 1; 426 } 427 /** 428 * @return {@link #purpose} (Explanation of why this canonical resource is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 429 */ 430 public abstract MarkdownType getPurposeElement(); 431 432 public abstract boolean hasPurposeElement(); 433 public abstract boolean hasPurpose(); 434 435 /** 436 * @param value {@link #purpose} (Explanation of why this canonical resource is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 437 */ 438 public abstract CanonicalResource setPurposeElement(MarkdownType value); 439 /** 440 * @return Explanation of why this canonical resource is needed and why it has been designed as it has. 441 */ 442 public abstract String getPurpose(); 443 /** 444 * @param value Explanation of why this canonical resource is needed and why it has been designed as it has. 445 */ 446 public abstract CanonicalResource setPurpose(String value); 447 /** 448 * How many allowed for this property by the implementation 449 */ 450 public int getCopyrightMax() { 451 return 1; 452 } 453 /** 454 * @return {@link #copyright} (A copyright statement relating to the canonical resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the canonical resource.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 455 */ 456 public abstract MarkdownType getCopyrightElement(); 457 458 public abstract boolean hasCopyrightElement(); 459 public abstract boolean hasCopyright(); 460 461 /** 462 * @param value {@link #copyright} (A copyright statement relating to the canonical resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the canonical resource.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 463 */ 464 public abstract CanonicalResource setCopyrightElement(MarkdownType value); 465 /** 466 * @return A copyright statement relating to the canonical resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the canonical resource. 467 */ 468 public abstract String getCopyright(); 469 /** 470 * @param value A copyright statement relating to the canonical resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the canonical resource. 471 */ 472 public abstract CanonicalResource setCopyright(String value); 473 /** 474 * How many allowed for this property by the implementation 475 */ 476 public int getCopyrightLabelMax() { 477 return 1; 478 } 479 /** 480 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 481 */ 482 public abstract StringType getCopyrightLabelElement(); 483 484 public abstract boolean hasCopyrightLabelElement(); 485 public abstract boolean hasCopyrightLabel(); 486 487 /** 488 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 489 */ 490 public abstract CanonicalResource setCopyrightLabelElement(StringType value); 491 /** 492 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 493 */ 494 public abstract String getCopyrightLabel(); 495 /** 496 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 497 */ 498 public abstract CanonicalResource setCopyrightLabel(String value); 499 protected void listChildren(List<Property> children) { 500 super.listChildren(children); 501 } 502 503 @Override 504 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 505 switch (_hash) { 506 default: return super.getNamedProperty(_hash, _name, _checkValid); 507 } 508 509 } 510 511 @Override 512 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 513 switch (hash) { 514 default: return super.getProperty(hash, name, checkValid); 515 } 516 517 } 518 519 @Override 520 public Base setProperty(int hash, String name, Base value) throws FHIRException { 521 switch (hash) { 522 default: return super.setProperty(hash, name, value); 523 } 524 525 } 526 527 @Override 528 public Base setProperty(String name, Base value) throws FHIRException { 529 return super.setProperty(name, value); 530 } 531 532 @Override 533 public void removeChild(String name, Base value) throws FHIRException { 534 super.removeChild(name, value); 535 } 536 537 @Override 538 public Base makeProperty(int hash, String name) throws FHIRException { 539 switch (hash) { 540 default: return super.makeProperty(hash, name); 541 } 542 543 } 544 545 @Override 546 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 547 switch (hash) { 548 default: return super.getTypesForProperty(hash, name); 549 } 550 551 } 552 553 @Override 554 public Base addChild(String name) throws FHIRException { 555 return super.addChild(name); 556 } 557 558 public String fhirType() { 559 return "CanonicalResource"; 560 561 } 562 563 public abstract CanonicalResource copy(); 564 565 public void copyValues(CanonicalResource dst) { 566 super.copyValues(dst); 567 } 568 569 @Override 570 public boolean equalsDeep(Base other_) { 571 if (!super.equalsDeep(other_)) 572 return false; 573 if (!(other_ instanceof CanonicalResource)) 574 return false; 575 CanonicalResource o = (CanonicalResource) other_; 576 return true; 577 } 578 579 @Override 580 public boolean equalsShallow(Base other_) { 581 if (!super.equalsShallow(other_)) 582 return false; 583 if (!(other_ instanceof CanonicalResource)) 584 return false; 585 CanonicalResource o = (CanonicalResource) other_; 586 return true; 587 } 588 589 public boolean isEmpty() { 590 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(); 591 } 592 593// Manual code (from Configuration.txt): 594 @Override 595 public String toString() { 596 return fhirType()+"["+getUrl()+(hasVersion() ? "|"+getVersion(): "")+"]"; 597 } 598 599 public String present() { 600 if (hasUserData(UserDataNames.render_presentation)) { 601 return getUserString(UserDataNames.render_presentation); 602 } 603 if (hasTitle()) 604 return getTitle(); 605 if (hasName()) 606 return getName(); 607 return toString(); 608 } 609 610 public String getVUrl() { 611 return getUrl() + (hasVersion() ? "|"+getVersion() : ""); 612 } 613 614 public boolean supportsCopyright() { 615 return true; 616 } 617 618 public String getVersionedUrl() { 619 return hasVersion() ? getUrl()+"|"+getVersion() : getUrl(); 620 } 621 622 623 public String oid() { 624 for (Identifier id : getIdentifier()) { 625 if (id.getValue().startsWith("urn:oid:")) { 626 return id.getValue().substring(8); 627 } 628 } 629 return null; 630 } 631 632 public String getOid() { 633 for (Identifier id : getIdentifier()) { 634 if ("urn:ietf:rfc:3986".equals(id.getSystem()) && id.hasValue() && id.getValue().startsWith("urn:oid:")) { 635 return id.getValue().substring(8); 636 } 637 } 638 return null; 639 } 640 641// end addition 642 643} 644