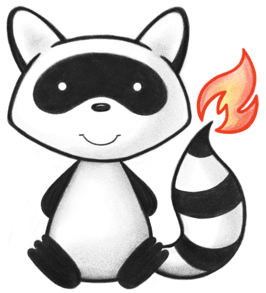
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050import org.hl7.fhir.instance.model.api.IBaseConformance; 051/** 052 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server or Client for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 053 */ 054@ResourceDef(name="CapabilityStatement", profile="http://hl7.org/fhir/StructureDefinition/CapabilityStatement") 055public class CapabilityStatement extends CanonicalResource implements IBaseConformance { 056 057 public enum ConditionalDeleteStatus { 058 /** 059 * No support for conditional deletes. 060 */ 061 NOTSUPPORTED, 062 /** 063 * Conditional deletes are supported, but only single resources at a time. 064 */ 065 SINGLE, 066 /** 067 * Conditional deletes are supported, and multiple resources can be deleted in a single interaction. 068 */ 069 MULTIPLE, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static ConditionalDeleteStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("not-supported".equals(codeString)) 078 return NOTSUPPORTED; 079 if ("single".equals(codeString)) 080 return SINGLE; 081 if ("multiple".equals(codeString)) 082 return MULTIPLE; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown ConditionalDeleteStatus code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case NOTSUPPORTED: return "not-supported"; 091 case SINGLE: return "single"; 092 case MULTIPLE: return "multiple"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getSystem() { 098 switch (this) { 099 case NOTSUPPORTED: return "http://hl7.org/fhir/conditional-delete-status"; 100 case SINGLE: return "http://hl7.org/fhir/conditional-delete-status"; 101 case MULTIPLE: return "http://hl7.org/fhir/conditional-delete-status"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getDefinition() { 107 switch (this) { 108 case NOTSUPPORTED: return "No support for conditional deletes."; 109 case SINGLE: return "Conditional deletes are supported, but only single resources at a time."; 110 case MULTIPLE: return "Conditional deletes are supported, and multiple resources can be deleted in a single interaction."; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getDisplay() { 116 switch (this) { 117 case NOTSUPPORTED: return "Not Supported"; 118 case SINGLE: return "Single Deletes Supported"; 119 case MULTIPLE: return "Multiple Deletes Supported"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 } 125 126 public static class ConditionalDeleteStatusEnumFactory implements EnumFactory<ConditionalDeleteStatus> { 127 public ConditionalDeleteStatus fromCode(String codeString) throws IllegalArgumentException { 128 if (codeString == null || "".equals(codeString)) 129 if (codeString == null || "".equals(codeString)) 130 return null; 131 if ("not-supported".equals(codeString)) 132 return ConditionalDeleteStatus.NOTSUPPORTED; 133 if ("single".equals(codeString)) 134 return ConditionalDeleteStatus.SINGLE; 135 if ("multiple".equals(codeString)) 136 return ConditionalDeleteStatus.MULTIPLE; 137 throw new IllegalArgumentException("Unknown ConditionalDeleteStatus code '"+codeString+"'"); 138 } 139 public Enumeration<ConditionalDeleteStatus> fromType(PrimitiveType<?> code) throws FHIRException { 140 if (code == null) 141 return null; 142 if (code.isEmpty()) 143 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NULL, code); 144 String codeString = ((PrimitiveType) code).asStringValue(); 145 if (codeString == null || "".equals(codeString)) 146 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NULL, code); 147 if ("not-supported".equals(codeString)) 148 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.NOTSUPPORTED, code); 149 if ("single".equals(codeString)) 150 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.SINGLE, code); 151 if ("multiple".equals(codeString)) 152 return new Enumeration<ConditionalDeleteStatus>(this, ConditionalDeleteStatus.MULTIPLE, code); 153 throw new FHIRException("Unknown ConditionalDeleteStatus code '"+codeString+"'"); 154 } 155 public String toCode(ConditionalDeleteStatus code) { 156 if (code == ConditionalDeleteStatus.NULL) 157 return null; 158 if (code == ConditionalDeleteStatus.NOTSUPPORTED) 159 return "not-supported"; 160 if (code == ConditionalDeleteStatus.SINGLE) 161 return "single"; 162 if (code == ConditionalDeleteStatus.MULTIPLE) 163 return "multiple"; 164 return "?"; 165 } 166 public String toSystem(ConditionalDeleteStatus code) { 167 return code.getSystem(); 168 } 169 } 170 171 public enum ConditionalReadStatus { 172 /** 173 * No support for conditional reads. 174 */ 175 NOTSUPPORTED, 176 /** 177 * Conditional reads are supported, but only with the If-Modified-Since HTTP Header. 178 */ 179 MODIFIEDSINCE, 180 /** 181 * Conditional reads are supported, but only with the If-None-Match HTTP Header. 182 */ 183 NOTMATCH, 184 /** 185 * Conditional reads are supported, with both If-Modified-Since and If-None-Match HTTP Headers. 186 */ 187 FULLSUPPORT, 188 /** 189 * added to help the parsers with the generic types 190 */ 191 NULL; 192 public static ConditionalReadStatus fromCode(String codeString) throws FHIRException { 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("not-supported".equals(codeString)) 196 return NOTSUPPORTED; 197 if ("modified-since".equals(codeString)) 198 return MODIFIEDSINCE; 199 if ("not-match".equals(codeString)) 200 return NOTMATCH; 201 if ("full-support".equals(codeString)) 202 return FULLSUPPORT; 203 if (Configuration.isAcceptInvalidEnums()) 204 return null; 205 else 206 throw new FHIRException("Unknown ConditionalReadStatus code '"+codeString+"'"); 207 } 208 public String toCode() { 209 switch (this) { 210 case NOTSUPPORTED: return "not-supported"; 211 case MODIFIEDSINCE: return "modified-since"; 212 case NOTMATCH: return "not-match"; 213 case FULLSUPPORT: return "full-support"; 214 case NULL: return null; 215 default: return "?"; 216 } 217 } 218 public String getSystem() { 219 switch (this) { 220 case NOTSUPPORTED: return "http://hl7.org/fhir/conditional-read-status"; 221 case MODIFIEDSINCE: return "http://hl7.org/fhir/conditional-read-status"; 222 case NOTMATCH: return "http://hl7.org/fhir/conditional-read-status"; 223 case FULLSUPPORT: return "http://hl7.org/fhir/conditional-read-status"; 224 case NULL: return null; 225 default: return "?"; 226 } 227 } 228 public String getDefinition() { 229 switch (this) { 230 case NOTSUPPORTED: return "No support for conditional reads."; 231 case MODIFIEDSINCE: return "Conditional reads are supported, but only with the If-Modified-Since HTTP Header."; 232 case NOTMATCH: return "Conditional reads are supported, but only with the If-None-Match HTTP Header."; 233 case FULLSUPPORT: return "Conditional reads are supported, with both If-Modified-Since and If-None-Match HTTP Headers."; 234 case NULL: return null; 235 default: return "?"; 236 } 237 } 238 public String getDisplay() { 239 switch (this) { 240 case NOTSUPPORTED: return "Not Supported"; 241 case MODIFIEDSINCE: return "If-Modified-Since"; 242 case NOTMATCH: return "If-None-Match"; 243 case FULLSUPPORT: return "Full Support"; 244 case NULL: return null; 245 default: return "?"; 246 } 247 } 248 } 249 250 public static class ConditionalReadStatusEnumFactory implements EnumFactory<ConditionalReadStatus> { 251 public ConditionalReadStatus fromCode(String codeString) throws IllegalArgumentException { 252 if (codeString == null || "".equals(codeString)) 253 if (codeString == null || "".equals(codeString)) 254 return null; 255 if ("not-supported".equals(codeString)) 256 return ConditionalReadStatus.NOTSUPPORTED; 257 if ("modified-since".equals(codeString)) 258 return ConditionalReadStatus.MODIFIEDSINCE; 259 if ("not-match".equals(codeString)) 260 return ConditionalReadStatus.NOTMATCH; 261 if ("full-support".equals(codeString)) 262 return ConditionalReadStatus.FULLSUPPORT; 263 throw new IllegalArgumentException("Unknown ConditionalReadStatus code '"+codeString+"'"); 264 } 265 public Enumeration<ConditionalReadStatus> fromType(PrimitiveType<?> code) throws FHIRException { 266 if (code == null) 267 return null; 268 if (code.isEmpty()) 269 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NULL, code); 270 String codeString = ((PrimitiveType) code).asStringValue(); 271 if (codeString == null || "".equals(codeString)) 272 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NULL, code); 273 if ("not-supported".equals(codeString)) 274 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTSUPPORTED, code); 275 if ("modified-since".equals(codeString)) 276 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.MODIFIEDSINCE, code); 277 if ("not-match".equals(codeString)) 278 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.NOTMATCH, code); 279 if ("full-support".equals(codeString)) 280 return new Enumeration<ConditionalReadStatus>(this, ConditionalReadStatus.FULLSUPPORT, code); 281 throw new FHIRException("Unknown ConditionalReadStatus code '"+codeString+"'"); 282 } 283 public String toCode(ConditionalReadStatus code) { 284 if (code == ConditionalReadStatus.NULL) 285 return null; 286 if (code == ConditionalReadStatus.NOTSUPPORTED) 287 return "not-supported"; 288 if (code == ConditionalReadStatus.MODIFIEDSINCE) 289 return "modified-since"; 290 if (code == ConditionalReadStatus.NOTMATCH) 291 return "not-match"; 292 if (code == ConditionalReadStatus.FULLSUPPORT) 293 return "full-support"; 294 return "?"; 295 } 296 public String toSystem(ConditionalReadStatus code) { 297 return code.getSystem(); 298 } 299 } 300 301 public enum DocumentMode { 302 /** 303 * The application produces documents of the specified type. 304 */ 305 PRODUCER, 306 /** 307 * The application consumes documents of the specified type. 308 */ 309 CONSUMER, 310 /** 311 * added to help the parsers with the generic types 312 */ 313 NULL; 314 public static DocumentMode fromCode(String codeString) throws FHIRException { 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("producer".equals(codeString)) 318 return PRODUCER; 319 if ("consumer".equals(codeString)) 320 return CONSUMER; 321 if (Configuration.isAcceptInvalidEnums()) 322 return null; 323 else 324 throw new FHIRException("Unknown DocumentMode code '"+codeString+"'"); 325 } 326 public String toCode() { 327 switch (this) { 328 case PRODUCER: return "producer"; 329 case CONSUMER: return "consumer"; 330 case NULL: return null; 331 default: return "?"; 332 } 333 } 334 public String getSystem() { 335 switch (this) { 336 case PRODUCER: return "http://hl7.org/fhir/document-mode"; 337 case CONSUMER: return "http://hl7.org/fhir/document-mode"; 338 case NULL: return null; 339 default: return "?"; 340 } 341 } 342 public String getDefinition() { 343 switch (this) { 344 case PRODUCER: return "The application produces documents of the specified type."; 345 case CONSUMER: return "The application consumes documents of the specified type."; 346 case NULL: return null; 347 default: return "?"; 348 } 349 } 350 public String getDisplay() { 351 switch (this) { 352 case PRODUCER: return "Producer"; 353 case CONSUMER: return "Consumer"; 354 case NULL: return null; 355 default: return "?"; 356 } 357 } 358 } 359 360 public static class DocumentModeEnumFactory implements EnumFactory<DocumentMode> { 361 public DocumentMode fromCode(String codeString) throws IllegalArgumentException { 362 if (codeString == null || "".equals(codeString)) 363 if (codeString == null || "".equals(codeString)) 364 return null; 365 if ("producer".equals(codeString)) 366 return DocumentMode.PRODUCER; 367 if ("consumer".equals(codeString)) 368 return DocumentMode.CONSUMER; 369 throw new IllegalArgumentException("Unknown DocumentMode code '"+codeString+"'"); 370 } 371 public Enumeration<DocumentMode> fromType(PrimitiveType<?> code) throws FHIRException { 372 if (code == null) 373 return null; 374 if (code.isEmpty()) 375 return new Enumeration<DocumentMode>(this, DocumentMode.NULL, code); 376 String codeString = ((PrimitiveType) code).asStringValue(); 377 if (codeString == null || "".equals(codeString)) 378 return new Enumeration<DocumentMode>(this, DocumentMode.NULL, code); 379 if ("producer".equals(codeString)) 380 return new Enumeration<DocumentMode>(this, DocumentMode.PRODUCER, code); 381 if ("consumer".equals(codeString)) 382 return new Enumeration<DocumentMode>(this, DocumentMode.CONSUMER, code); 383 throw new FHIRException("Unknown DocumentMode code '"+codeString+"'"); 384 } 385 public String toCode(DocumentMode code) { 386 if (code == DocumentMode.NULL) 387 return null; 388 if (code == DocumentMode.PRODUCER) 389 return "producer"; 390 if (code == DocumentMode.CONSUMER) 391 return "consumer"; 392 return "?"; 393 } 394 public String toSystem(DocumentMode code) { 395 return code.getSystem(); 396 } 397 } 398 399 public enum EventCapabilityMode { 400 /** 401 * The application sends requests and receives responses. 402 */ 403 SENDER, 404 /** 405 * The application receives requests and sends responses. 406 */ 407 RECEIVER, 408 /** 409 * added to help the parsers with the generic types 410 */ 411 NULL; 412 public static EventCapabilityMode fromCode(String codeString) throws FHIRException { 413 if (codeString == null || "".equals(codeString)) 414 return null; 415 if ("sender".equals(codeString)) 416 return SENDER; 417 if ("receiver".equals(codeString)) 418 return RECEIVER; 419 if (Configuration.isAcceptInvalidEnums()) 420 return null; 421 else 422 throw new FHIRException("Unknown EventCapabilityMode code '"+codeString+"'"); 423 } 424 public String toCode() { 425 switch (this) { 426 case SENDER: return "sender"; 427 case RECEIVER: return "receiver"; 428 case NULL: return null; 429 default: return "?"; 430 } 431 } 432 public String getSystem() { 433 switch (this) { 434 case SENDER: return "http://hl7.org/fhir/event-capability-mode"; 435 case RECEIVER: return "http://hl7.org/fhir/event-capability-mode"; 436 case NULL: return null; 437 default: return "?"; 438 } 439 } 440 public String getDefinition() { 441 switch (this) { 442 case SENDER: return "The application sends requests and receives responses."; 443 case RECEIVER: return "The application receives requests and sends responses."; 444 case NULL: return null; 445 default: return "?"; 446 } 447 } 448 public String getDisplay() { 449 switch (this) { 450 case SENDER: return "Sender"; 451 case RECEIVER: return "Receiver"; 452 case NULL: return null; 453 default: return "?"; 454 } 455 } 456 } 457 458 public static class EventCapabilityModeEnumFactory implements EnumFactory<EventCapabilityMode> { 459 public EventCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 460 if (codeString == null || "".equals(codeString)) 461 if (codeString == null || "".equals(codeString)) 462 return null; 463 if ("sender".equals(codeString)) 464 return EventCapabilityMode.SENDER; 465 if ("receiver".equals(codeString)) 466 return EventCapabilityMode.RECEIVER; 467 throw new IllegalArgumentException("Unknown EventCapabilityMode code '"+codeString+"'"); 468 } 469 public Enumeration<EventCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 470 if (code == null) 471 return null; 472 if (code.isEmpty()) 473 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.NULL, code); 474 String codeString = ((PrimitiveType) code).asStringValue(); 475 if (codeString == null || "".equals(codeString)) 476 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.NULL, code); 477 if ("sender".equals(codeString)) 478 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.SENDER, code); 479 if ("receiver".equals(codeString)) 480 return new Enumeration<EventCapabilityMode>(this, EventCapabilityMode.RECEIVER, code); 481 throw new FHIRException("Unknown EventCapabilityMode code '"+codeString+"'"); 482 } 483 public String toCode(EventCapabilityMode code) { 484 if (code == EventCapabilityMode.NULL) 485 return null; 486 if (code == EventCapabilityMode.SENDER) 487 return "sender"; 488 if (code == EventCapabilityMode.RECEIVER) 489 return "receiver"; 490 return "?"; 491 } 492 public String toSystem(EventCapabilityMode code) { 493 return code.getSystem(); 494 } 495 } 496 497 public enum ReferenceHandlingPolicy { 498 /** 499 * The server supports and populates Literal references (i.e. using Reference.reference) where they are known (this code does not guarantee that all references are literal; see 'enforced'). 500 */ 501 LITERAL, 502 /** 503 * The server allows logical references (i.e. using Reference.identifier). 504 */ 505 LOGICAL, 506 /** 507 * The server will attempt to resolve logical references to literal references - i.e. converting Reference.identifier to Reference.reference (if resolution fails, the server may still accept resources; see logical). 508 */ 509 RESOLVES, 510 /** 511 * The server enforces that references have integrity - e.g. it ensures that references can always be resolved. This is typically the case for clinical record systems, but often not the case for middleware/proxy systems. 512 */ 513 ENFORCED, 514 /** 515 * The server does not support references that point to other servers. 516 */ 517 LOCAL, 518 /** 519 * added to help the parsers with the generic types 520 */ 521 NULL; 522 public static ReferenceHandlingPolicy fromCode(String codeString) throws FHIRException { 523 if (codeString == null || "".equals(codeString)) 524 return null; 525 if ("literal".equals(codeString)) 526 return LITERAL; 527 if ("logical".equals(codeString)) 528 return LOGICAL; 529 if ("resolves".equals(codeString)) 530 return RESOLVES; 531 if ("enforced".equals(codeString)) 532 return ENFORCED; 533 if ("local".equals(codeString)) 534 return LOCAL; 535 if (Configuration.isAcceptInvalidEnums()) 536 return null; 537 else 538 throw new FHIRException("Unknown ReferenceHandlingPolicy code '"+codeString+"'"); 539 } 540 public String toCode() { 541 switch (this) { 542 case LITERAL: return "literal"; 543 case LOGICAL: return "logical"; 544 case RESOLVES: return "resolves"; 545 case ENFORCED: return "enforced"; 546 case LOCAL: return "local"; 547 case NULL: return null; 548 default: return "?"; 549 } 550 } 551 public String getSystem() { 552 switch (this) { 553 case LITERAL: return "http://hl7.org/fhir/reference-handling-policy"; 554 case LOGICAL: return "http://hl7.org/fhir/reference-handling-policy"; 555 case RESOLVES: return "http://hl7.org/fhir/reference-handling-policy"; 556 case ENFORCED: return "http://hl7.org/fhir/reference-handling-policy"; 557 case LOCAL: return "http://hl7.org/fhir/reference-handling-policy"; 558 case NULL: return null; 559 default: return "?"; 560 } 561 } 562 public String getDefinition() { 563 switch (this) { 564 case LITERAL: return "The server supports and populates Literal references (i.e. using Reference.reference) where they are known (this code does not guarantee that all references are literal; see 'enforced')."; 565 case LOGICAL: return "The server allows logical references (i.e. using Reference.identifier)."; 566 case RESOLVES: return "The server will attempt to resolve logical references to literal references - i.e. converting Reference.identifier to Reference.reference (if resolution fails, the server may still accept resources; see logical)."; 567 case ENFORCED: return "The server enforces that references have integrity - e.g. it ensures that references can always be resolved. This is typically the case for clinical record systems, but often not the case for middleware/proxy systems."; 568 case LOCAL: return "The server does not support references that point to other servers."; 569 case NULL: return null; 570 default: return "?"; 571 } 572 } 573 public String getDisplay() { 574 switch (this) { 575 case LITERAL: return "Literal References"; 576 case LOGICAL: return "Logical References"; 577 case RESOLVES: return "Resolves References"; 578 case ENFORCED: return "Reference Integrity Enforced"; 579 case LOCAL: return "Local References Only"; 580 case NULL: return null; 581 default: return "?"; 582 } 583 } 584 } 585 586 public static class ReferenceHandlingPolicyEnumFactory implements EnumFactory<ReferenceHandlingPolicy> { 587 public ReferenceHandlingPolicy fromCode(String codeString) throws IllegalArgumentException { 588 if (codeString == null || "".equals(codeString)) 589 if (codeString == null || "".equals(codeString)) 590 return null; 591 if ("literal".equals(codeString)) 592 return ReferenceHandlingPolicy.LITERAL; 593 if ("logical".equals(codeString)) 594 return ReferenceHandlingPolicy.LOGICAL; 595 if ("resolves".equals(codeString)) 596 return ReferenceHandlingPolicy.RESOLVES; 597 if ("enforced".equals(codeString)) 598 return ReferenceHandlingPolicy.ENFORCED; 599 if ("local".equals(codeString)) 600 return ReferenceHandlingPolicy.LOCAL; 601 throw new IllegalArgumentException("Unknown ReferenceHandlingPolicy code '"+codeString+"'"); 602 } 603 public Enumeration<ReferenceHandlingPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 604 if (code == null) 605 return null; 606 if (code.isEmpty()) 607 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.NULL, code); 608 String codeString = ((PrimitiveType) code).asStringValue(); 609 if (codeString == null || "".equals(codeString)) 610 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.NULL, code); 611 if ("literal".equals(codeString)) 612 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LITERAL, code); 613 if ("logical".equals(codeString)) 614 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOGICAL, code); 615 if ("resolves".equals(codeString)) 616 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.RESOLVES, code); 617 if ("enforced".equals(codeString)) 618 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.ENFORCED, code); 619 if ("local".equals(codeString)) 620 return new Enumeration<ReferenceHandlingPolicy>(this, ReferenceHandlingPolicy.LOCAL, code); 621 throw new FHIRException("Unknown ReferenceHandlingPolicy code '"+codeString+"'"); 622 } 623 public String toCode(ReferenceHandlingPolicy code) { 624 if (code == ReferenceHandlingPolicy.NULL) 625 return null; 626 if (code == ReferenceHandlingPolicy.LITERAL) 627 return "literal"; 628 if (code == ReferenceHandlingPolicy.LOGICAL) 629 return "logical"; 630 if (code == ReferenceHandlingPolicy.RESOLVES) 631 return "resolves"; 632 if (code == ReferenceHandlingPolicy.ENFORCED) 633 return "enforced"; 634 if (code == ReferenceHandlingPolicy.LOCAL) 635 return "local"; 636 return "?"; 637 } 638 public String toSystem(ReferenceHandlingPolicy code) { 639 return code.getSystem(); 640 } 641 } 642 643 public enum ResourceVersionPolicy { 644 /** 645 * VersionId meta-property is not supported (server) or used (client). 646 */ 647 NOVERSION, 648 /** 649 * VersionId meta-property is supported (server) or used (client). 650 */ 651 VERSIONED, 652 /** 653 * Supports version-aware updates (server) or will be specified (If-match header) for updates (client). 654 */ 655 VERSIONEDUPDATE, 656 /** 657 * added to help the parsers with the generic types 658 */ 659 NULL; 660 public static ResourceVersionPolicy fromCode(String codeString) throws FHIRException { 661 if (codeString == null || "".equals(codeString)) 662 return null; 663 if ("no-version".equals(codeString)) 664 return NOVERSION; 665 if ("versioned".equals(codeString)) 666 return VERSIONED; 667 if ("versioned-update".equals(codeString)) 668 return VERSIONEDUPDATE; 669 if (Configuration.isAcceptInvalidEnums()) 670 return null; 671 else 672 throw new FHIRException("Unknown ResourceVersionPolicy code '"+codeString+"'"); 673 } 674 public String toCode() { 675 switch (this) { 676 case NOVERSION: return "no-version"; 677 case VERSIONED: return "versioned"; 678 case VERSIONEDUPDATE: return "versioned-update"; 679 case NULL: return null; 680 default: return "?"; 681 } 682 } 683 public String getSystem() { 684 switch (this) { 685 case NOVERSION: return "http://hl7.org/fhir/versioning-policy"; 686 case VERSIONED: return "http://hl7.org/fhir/versioning-policy"; 687 case VERSIONEDUPDATE: return "http://hl7.org/fhir/versioning-policy"; 688 case NULL: return null; 689 default: return "?"; 690 } 691 } 692 public String getDefinition() { 693 switch (this) { 694 case NOVERSION: return "VersionId meta-property is not supported (server) or used (client)."; 695 case VERSIONED: return "VersionId meta-property is supported (server) or used (client)."; 696 case VERSIONEDUPDATE: return "Supports version-aware updates (server) or will be specified (If-match header) for updates (client)."; 697 case NULL: return null; 698 default: return "?"; 699 } 700 } 701 public String getDisplay() { 702 switch (this) { 703 case NOVERSION: return "No VersionId Support"; 704 case VERSIONED: return "Versioned"; 705 case VERSIONEDUPDATE: return "VersionId tracked fully"; 706 case NULL: return null; 707 default: return "?"; 708 } 709 } 710 } 711 712 public static class ResourceVersionPolicyEnumFactory implements EnumFactory<ResourceVersionPolicy> { 713 public ResourceVersionPolicy fromCode(String codeString) throws IllegalArgumentException { 714 if (codeString == null || "".equals(codeString)) 715 if (codeString == null || "".equals(codeString)) 716 return null; 717 if ("no-version".equals(codeString)) 718 return ResourceVersionPolicy.NOVERSION; 719 if ("versioned".equals(codeString)) 720 return ResourceVersionPolicy.VERSIONED; 721 if ("versioned-update".equals(codeString)) 722 return ResourceVersionPolicy.VERSIONEDUPDATE; 723 throw new IllegalArgumentException("Unknown ResourceVersionPolicy code '"+codeString+"'"); 724 } 725 public Enumeration<ResourceVersionPolicy> fromType(PrimitiveType<?> code) throws FHIRException { 726 if (code == null) 727 return null; 728 if (code.isEmpty()) 729 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NULL, code); 730 String codeString = ((PrimitiveType) code).asStringValue(); 731 if (codeString == null || "".equals(codeString)) 732 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NULL, code); 733 if ("no-version".equals(codeString)) 734 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.NOVERSION, code); 735 if ("versioned".equals(codeString)) 736 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONED, code); 737 if ("versioned-update".equals(codeString)) 738 return new Enumeration<ResourceVersionPolicy>(this, ResourceVersionPolicy.VERSIONEDUPDATE, code); 739 throw new FHIRException("Unknown ResourceVersionPolicy code '"+codeString+"'"); 740 } 741 public String toCode(ResourceVersionPolicy code) { 742 if (code == ResourceVersionPolicy.NULL) 743 return null; 744 if (code == ResourceVersionPolicy.NOVERSION) 745 return "no-version"; 746 if (code == ResourceVersionPolicy.VERSIONED) 747 return "versioned"; 748 if (code == ResourceVersionPolicy.VERSIONEDUPDATE) 749 return "versioned-update"; 750 return "?"; 751 } 752 public String toSystem(ResourceVersionPolicy code) { 753 return code.getSystem(); 754 } 755 } 756 757 public enum RestfulCapabilityMode { 758 /** 759 * The application acts as a client for this resource. 760 */ 761 CLIENT, 762 /** 763 * The application acts as a server for this resource. 764 */ 765 SERVER, 766 /** 767 * added to help the parsers with the generic types 768 */ 769 NULL; 770 public static RestfulCapabilityMode fromCode(String codeString) throws FHIRException { 771 if (codeString == null || "".equals(codeString)) 772 return null; 773 if ("client".equals(codeString)) 774 return CLIENT; 775 if ("server".equals(codeString)) 776 return SERVER; 777 if (Configuration.isAcceptInvalidEnums()) 778 return null; 779 else 780 throw new FHIRException("Unknown RestfulCapabilityMode code '"+codeString+"'"); 781 } 782 public String toCode() { 783 switch (this) { 784 case CLIENT: return "client"; 785 case SERVER: return "server"; 786 case NULL: return null; 787 default: return "?"; 788 } 789 } 790 public String getSystem() { 791 switch (this) { 792 case CLIENT: return "http://hl7.org/fhir/restful-capability-mode"; 793 case SERVER: return "http://hl7.org/fhir/restful-capability-mode"; 794 case NULL: return null; 795 default: return "?"; 796 } 797 } 798 public String getDefinition() { 799 switch (this) { 800 case CLIENT: return "The application acts as a client for this resource."; 801 case SERVER: return "The application acts as a server for this resource."; 802 case NULL: return null; 803 default: return "?"; 804 } 805 } 806 public String getDisplay() { 807 switch (this) { 808 case CLIENT: return "Client"; 809 case SERVER: return "Server"; 810 case NULL: return null; 811 default: return "?"; 812 } 813 } 814 } 815 816 public static class RestfulCapabilityModeEnumFactory implements EnumFactory<RestfulCapabilityMode> { 817 public RestfulCapabilityMode fromCode(String codeString) throws IllegalArgumentException { 818 if (codeString == null || "".equals(codeString)) 819 if (codeString == null || "".equals(codeString)) 820 return null; 821 if ("client".equals(codeString)) 822 return RestfulCapabilityMode.CLIENT; 823 if ("server".equals(codeString)) 824 return RestfulCapabilityMode.SERVER; 825 throw new IllegalArgumentException("Unknown RestfulCapabilityMode code '"+codeString+"'"); 826 } 827 public Enumeration<RestfulCapabilityMode> fromType(PrimitiveType<?> code) throws FHIRException { 828 if (code == null) 829 return null; 830 if (code.isEmpty()) 831 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.NULL, code); 832 String codeString = ((PrimitiveType) code).asStringValue(); 833 if (codeString == null || "".equals(codeString)) 834 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.NULL, code); 835 if ("client".equals(codeString)) 836 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.CLIENT, code); 837 if ("server".equals(codeString)) 838 return new Enumeration<RestfulCapabilityMode>(this, RestfulCapabilityMode.SERVER, code); 839 throw new FHIRException("Unknown RestfulCapabilityMode code '"+codeString+"'"); 840 } 841 public String toCode(RestfulCapabilityMode code) { 842 if (code == RestfulCapabilityMode.NULL) 843 return null; 844 if (code == RestfulCapabilityMode.CLIENT) 845 return "client"; 846 if (code == RestfulCapabilityMode.SERVER) 847 return "server"; 848 return "?"; 849 } 850 public String toSystem(RestfulCapabilityMode code) { 851 return code.getSystem(); 852 } 853 } 854 855 public enum SystemRestfulInteraction { 856 /** 857 * Update, create or delete a set of resources as a single transaction. 858 */ 859 TRANSACTION, 860 /** 861 * perform a set of a separate interactions in a single http operation 862 */ 863 BATCH, 864 /** 865 * Search all resources based on some filter criteria. 866 */ 867 SEARCHSYSTEM, 868 /** 869 * Retrieve the change history for all resources on a system. 870 */ 871 HISTORYSYSTEM, 872 /** 873 * added to help the parsers with the generic types 874 */ 875 NULL; 876 public static SystemRestfulInteraction fromCode(String codeString) throws FHIRException { 877 if (codeString == null || "".equals(codeString)) 878 return null; 879 if ("transaction".equals(codeString)) 880 return TRANSACTION; 881 if ("batch".equals(codeString)) 882 return BATCH; 883 if ("search-system".equals(codeString)) 884 return SEARCHSYSTEM; 885 if ("history-system".equals(codeString)) 886 return HISTORYSYSTEM; 887 if (Configuration.isAcceptInvalidEnums()) 888 return null; 889 else 890 throw new FHIRException("Unknown SystemRestfulInteraction code '"+codeString+"'"); 891 } 892 public String toCode() { 893 switch (this) { 894 case TRANSACTION: return "transaction"; 895 case BATCH: return "batch"; 896 case SEARCHSYSTEM: return "search-system"; 897 case HISTORYSYSTEM: return "history-system"; 898 case NULL: return null; 899 default: return "?"; 900 } 901 } 902 public String getSystem() { 903 switch (this) { 904 case TRANSACTION: return "http://hl7.org/fhir/restful-interaction"; 905 case BATCH: return "http://hl7.org/fhir/restful-interaction"; 906 case SEARCHSYSTEM: return "http://hl7.org/fhir/restful-interaction"; 907 case HISTORYSYSTEM: return "http://hl7.org/fhir/restful-interaction"; 908 case NULL: return null; 909 default: return "?"; 910 } 911 } 912 public String getDefinition() { 913 switch (this) { 914 case TRANSACTION: return "Update, create or delete a set of resources as a single transaction."; 915 case BATCH: return "perform a set of a separate interactions in a single http operation"; 916 case SEARCHSYSTEM: return "Search all resources based on some filter criteria."; 917 case HISTORYSYSTEM: return "Retrieve the change history for all resources on a system."; 918 case NULL: return null; 919 default: return "?"; 920 } 921 } 922 public String getDisplay() { 923 switch (this) { 924 case TRANSACTION: return "transaction"; 925 case BATCH: return "batch"; 926 case SEARCHSYSTEM: return "search-system"; 927 case HISTORYSYSTEM: return "history-system"; 928 case NULL: return null; 929 default: return "?"; 930 } 931 } 932 } 933 934 public static class SystemRestfulInteractionEnumFactory implements EnumFactory<SystemRestfulInteraction> { 935 public SystemRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 936 if (codeString == null || "".equals(codeString)) 937 if (codeString == null || "".equals(codeString)) 938 return null; 939 if ("transaction".equals(codeString)) 940 return SystemRestfulInteraction.TRANSACTION; 941 if ("batch".equals(codeString)) 942 return SystemRestfulInteraction.BATCH; 943 if ("search-system".equals(codeString)) 944 return SystemRestfulInteraction.SEARCHSYSTEM; 945 if ("history-system".equals(codeString)) 946 return SystemRestfulInteraction.HISTORYSYSTEM; 947 throw new IllegalArgumentException("Unknown SystemRestfulInteraction code '"+codeString+"'"); 948 } 949 public Enumeration<SystemRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 950 if (code == null) 951 return null; 952 if (code.isEmpty()) 953 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.NULL, code); 954 String codeString = ((PrimitiveType) code).asStringValue(); 955 if (codeString == null || "".equals(codeString)) 956 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.NULL, code); 957 if ("transaction".equals(codeString)) 958 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.TRANSACTION, code); 959 if ("batch".equals(codeString)) 960 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.BATCH, code); 961 if ("search-system".equals(codeString)) 962 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.SEARCHSYSTEM, code); 963 if ("history-system".equals(codeString)) 964 return new Enumeration<SystemRestfulInteraction>(this, SystemRestfulInteraction.HISTORYSYSTEM, code); 965 throw new FHIRException("Unknown SystemRestfulInteraction code '"+codeString+"'"); 966 } 967 public String toCode(SystemRestfulInteraction code) { 968 if (code == SystemRestfulInteraction.NULL) 969 return null; 970 if (code == SystemRestfulInteraction.TRANSACTION) 971 return "transaction"; 972 if (code == SystemRestfulInteraction.BATCH) 973 return "batch"; 974 if (code == SystemRestfulInteraction.SEARCHSYSTEM) 975 return "search-system"; 976 if (code == SystemRestfulInteraction.HISTORYSYSTEM) 977 return "history-system"; 978 return "?"; 979 } 980 public String toSystem(SystemRestfulInteraction code) { 981 return code.getSystem(); 982 } 983 } 984 985 public enum TypeRestfulInteraction { 986 /** 987 * Read the current state of the resource. 988 */ 989 READ, 990 /** 991 * Read the state of a specific version of the resource. 992 */ 993 VREAD, 994 /** 995 * Update an existing resource by its id (or create it if it is new). 996 */ 997 UPDATE, 998 /** 999 * Update an existing resource by posting a set of changes to it. 1000 */ 1001 PATCH, 1002 /** 1003 * Delete a resource. 1004 */ 1005 DELETE, 1006 /** 1007 * Retrieve the change history for a particular resource. 1008 */ 1009 HISTORYINSTANCE, 1010 /** 1011 * Retrieve the change history for all resources of a particular type. 1012 */ 1013 HISTORYTYPE, 1014 /** 1015 * Create a new resource with a server assigned id. 1016 */ 1017 CREATE, 1018 /** 1019 * Search all resources of the specified type based on some filter criteria. 1020 */ 1021 SEARCHTYPE, 1022 /** 1023 * added to help the parsers with the generic types 1024 */ 1025 NULL; 1026 public static TypeRestfulInteraction fromCode(String codeString) throws FHIRException { 1027 if (codeString == null || "".equals(codeString)) 1028 return null; 1029 if ("read".equals(codeString)) 1030 return READ; 1031 if ("vread".equals(codeString)) 1032 return VREAD; 1033 if ("update".equals(codeString)) 1034 return UPDATE; 1035 if ("patch".equals(codeString)) 1036 return PATCH; 1037 if ("delete".equals(codeString)) 1038 return DELETE; 1039 if ("history-instance".equals(codeString)) 1040 return HISTORYINSTANCE; 1041 if ("history-type".equals(codeString)) 1042 return HISTORYTYPE; 1043 if ("create".equals(codeString)) 1044 return CREATE; 1045 if ("search-type".equals(codeString)) 1046 return SEARCHTYPE; 1047 if (Configuration.isAcceptInvalidEnums()) 1048 return null; 1049 else 1050 throw new FHIRException("Unknown TypeRestfulInteraction code '"+codeString+"'"); 1051 } 1052 public String toCode() { 1053 switch (this) { 1054 case READ: return "read"; 1055 case VREAD: return "vread"; 1056 case UPDATE: return "update"; 1057 case PATCH: return "patch"; 1058 case DELETE: return "delete"; 1059 case HISTORYINSTANCE: return "history-instance"; 1060 case HISTORYTYPE: return "history-type"; 1061 case CREATE: return "create"; 1062 case SEARCHTYPE: return "search-type"; 1063 case NULL: return null; 1064 default: return "?"; 1065 } 1066 } 1067 public String getSystem() { 1068 switch (this) { 1069 case READ: return "http://hl7.org/fhir/restful-interaction"; 1070 case VREAD: return "http://hl7.org/fhir/restful-interaction"; 1071 case UPDATE: return "http://hl7.org/fhir/restful-interaction"; 1072 case PATCH: return "http://hl7.org/fhir/restful-interaction"; 1073 case DELETE: return "http://hl7.org/fhir/restful-interaction"; 1074 case HISTORYINSTANCE: return "http://hl7.org/fhir/restful-interaction"; 1075 case HISTORYTYPE: return "http://hl7.org/fhir/restful-interaction"; 1076 case CREATE: return "http://hl7.org/fhir/restful-interaction"; 1077 case SEARCHTYPE: return "http://hl7.org/fhir/restful-interaction"; 1078 case NULL: return null; 1079 default: return "?"; 1080 } 1081 } 1082 public String getDefinition() { 1083 switch (this) { 1084 case READ: return "Read the current state of the resource."; 1085 case VREAD: return "Read the state of a specific version of the resource."; 1086 case UPDATE: return "Update an existing resource by its id (or create it if it is new)."; 1087 case PATCH: return "Update an existing resource by posting a set of changes to it."; 1088 case DELETE: return "Delete a resource."; 1089 case HISTORYINSTANCE: return "Retrieve the change history for a particular resource."; 1090 case HISTORYTYPE: return "Retrieve the change history for all resources of a particular type."; 1091 case CREATE: return "Create a new resource with a server assigned id."; 1092 case SEARCHTYPE: return "Search all resources of the specified type based on some filter criteria."; 1093 case NULL: return null; 1094 default: return "?"; 1095 } 1096 } 1097 public String getDisplay() { 1098 switch (this) { 1099 case READ: return "read"; 1100 case VREAD: return "vread"; 1101 case UPDATE: return "update"; 1102 case PATCH: return "patch"; 1103 case DELETE: return "delete"; 1104 case HISTORYINSTANCE: return "history-instance"; 1105 case HISTORYTYPE: return "history-type"; 1106 case CREATE: return "create"; 1107 case SEARCHTYPE: return "search-type"; 1108 case NULL: return null; 1109 default: return "?"; 1110 } 1111 } 1112 } 1113 1114 public static class TypeRestfulInteractionEnumFactory implements EnumFactory<TypeRestfulInteraction> { 1115 public TypeRestfulInteraction fromCode(String codeString) throws IllegalArgumentException { 1116 if (codeString == null || "".equals(codeString)) 1117 if (codeString == null || "".equals(codeString)) 1118 return null; 1119 if ("read".equals(codeString)) 1120 return TypeRestfulInteraction.READ; 1121 if ("vread".equals(codeString)) 1122 return TypeRestfulInteraction.VREAD; 1123 if ("update".equals(codeString)) 1124 return TypeRestfulInteraction.UPDATE; 1125 if ("patch".equals(codeString)) 1126 return TypeRestfulInteraction.PATCH; 1127 if ("delete".equals(codeString)) 1128 return TypeRestfulInteraction.DELETE; 1129 if ("history-instance".equals(codeString)) 1130 return TypeRestfulInteraction.HISTORYINSTANCE; 1131 if ("history-type".equals(codeString)) 1132 return TypeRestfulInteraction.HISTORYTYPE; 1133 if ("create".equals(codeString)) 1134 return TypeRestfulInteraction.CREATE; 1135 if ("search-type".equals(codeString)) 1136 return TypeRestfulInteraction.SEARCHTYPE; 1137 throw new IllegalArgumentException("Unknown TypeRestfulInteraction code '"+codeString+"'"); 1138 } 1139 public Enumeration<TypeRestfulInteraction> fromType(PrimitiveType<?> code) throws FHIRException { 1140 if (code == null) 1141 return null; 1142 if (code.isEmpty()) 1143 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.NULL, code); 1144 String codeString = ((PrimitiveType) code).asStringValue(); 1145 if (codeString == null || "".equals(codeString)) 1146 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.NULL, code); 1147 if ("read".equals(codeString)) 1148 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.READ, code); 1149 if ("vread".equals(codeString)) 1150 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.VREAD, code); 1151 if ("update".equals(codeString)) 1152 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.UPDATE, code); 1153 if ("patch".equals(codeString)) 1154 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.PATCH, code); 1155 if ("delete".equals(codeString)) 1156 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.DELETE, code); 1157 if ("history-instance".equals(codeString)) 1158 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYINSTANCE, code); 1159 if ("history-type".equals(codeString)) 1160 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.HISTORYTYPE, code); 1161 if ("create".equals(codeString)) 1162 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.CREATE, code); 1163 if ("search-type".equals(codeString)) 1164 return new Enumeration<TypeRestfulInteraction>(this, TypeRestfulInteraction.SEARCHTYPE, code); 1165 throw new FHIRException("Unknown TypeRestfulInteraction code '"+codeString+"'"); 1166 } 1167 public String toCode(TypeRestfulInteraction code) { 1168 if (code == TypeRestfulInteraction.NULL) 1169 return null; 1170 if (code == TypeRestfulInteraction.READ) 1171 return "read"; 1172 if (code == TypeRestfulInteraction.VREAD) 1173 return "vread"; 1174 if (code == TypeRestfulInteraction.UPDATE) 1175 return "update"; 1176 if (code == TypeRestfulInteraction.PATCH) 1177 return "patch"; 1178 if (code == TypeRestfulInteraction.DELETE) 1179 return "delete"; 1180 if (code == TypeRestfulInteraction.HISTORYINSTANCE) 1181 return "history-instance"; 1182 if (code == TypeRestfulInteraction.HISTORYTYPE) 1183 return "history-type"; 1184 if (code == TypeRestfulInteraction.CREATE) 1185 return "create"; 1186 if (code == TypeRestfulInteraction.SEARCHTYPE) 1187 return "search-type"; 1188 return "?"; 1189 } 1190 public String toSystem(TypeRestfulInteraction code) { 1191 return code.getSystem(); 1192 } 1193 } 1194 1195 @Block() 1196 public static class CapabilityStatementSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 1197 /** 1198 * Name the software is known by. 1199 */ 1200 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1201 @Description(shortDefinition="A name the software is known by", formalDefinition="Name the software is known by." ) 1202 protected StringType name; 1203 1204 /** 1205 * The version identifier for the software covered by this statement. 1206 */ 1207 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1208 @Description(shortDefinition="Version covered by this statement", formalDefinition="The version identifier for the software covered by this statement." ) 1209 protected StringType version; 1210 1211 /** 1212 * Date this version of the software was released. 1213 */ 1214 @Child(name = "releaseDate", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1215 @Description(shortDefinition="Date this version was released", formalDefinition="Date this version of the software was released." ) 1216 protected DateTimeType releaseDate; 1217 1218 private static final long serialVersionUID = 1819769027L; 1219 1220 /** 1221 * Constructor 1222 */ 1223 public CapabilityStatementSoftwareComponent() { 1224 super(); 1225 } 1226 1227 /** 1228 * Constructor 1229 */ 1230 public CapabilityStatementSoftwareComponent(String name) { 1231 super(); 1232 this.setName(name); 1233 } 1234 1235 /** 1236 * @return {@link #name} (Name the software is known by.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1237 */ 1238 public StringType getNameElement() { 1239 if (this.name == null) 1240 if (Configuration.errorOnAutoCreate()) 1241 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.name"); 1242 else if (Configuration.doAutoCreate()) 1243 this.name = new StringType(); // bb 1244 return this.name; 1245 } 1246 1247 public boolean hasNameElement() { 1248 return this.name != null && !this.name.isEmpty(); 1249 } 1250 1251 public boolean hasName() { 1252 return this.name != null && !this.name.isEmpty(); 1253 } 1254 1255 /** 1256 * @param value {@link #name} (Name the software is known by.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1257 */ 1258 public CapabilityStatementSoftwareComponent setNameElement(StringType value) { 1259 this.name = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return Name the software is known by. 1265 */ 1266 public String getName() { 1267 return this.name == null ? null : this.name.getValue(); 1268 } 1269 1270 /** 1271 * @param value Name the software is known by. 1272 */ 1273 public CapabilityStatementSoftwareComponent setName(String value) { 1274 if (this.name == null) 1275 this.name = new StringType(); 1276 this.name.setValue(value); 1277 return this; 1278 } 1279 1280 /** 1281 * @return {@link #version} (The version identifier for the software covered by this statement.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1282 */ 1283 public StringType getVersionElement() { 1284 if (this.version == null) 1285 if (Configuration.errorOnAutoCreate()) 1286 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.version"); 1287 else if (Configuration.doAutoCreate()) 1288 this.version = new StringType(); // bb 1289 return this.version; 1290 } 1291 1292 public boolean hasVersionElement() { 1293 return this.version != null && !this.version.isEmpty(); 1294 } 1295 1296 public boolean hasVersion() { 1297 return this.version != null && !this.version.isEmpty(); 1298 } 1299 1300 /** 1301 * @param value {@link #version} (The version identifier for the software covered by this statement.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1302 */ 1303 public CapabilityStatementSoftwareComponent setVersionElement(StringType value) { 1304 this.version = value; 1305 return this; 1306 } 1307 1308 /** 1309 * @return The version identifier for the software covered by this statement. 1310 */ 1311 public String getVersion() { 1312 return this.version == null ? null : this.version.getValue(); 1313 } 1314 1315 /** 1316 * @param value The version identifier for the software covered by this statement. 1317 */ 1318 public CapabilityStatementSoftwareComponent setVersion(String value) { 1319 if (Utilities.noString(value)) 1320 this.version = null; 1321 else { 1322 if (this.version == null) 1323 this.version = new StringType(); 1324 this.version.setValue(value); 1325 } 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #releaseDate} (Date this version of the software was released.). This is the underlying object with id, value and extensions. The accessor "getReleaseDate" gives direct access to the value 1331 */ 1332 public DateTimeType getReleaseDateElement() { 1333 if (this.releaseDate == null) 1334 if (Configuration.errorOnAutoCreate()) 1335 throw new Error("Attempt to auto-create CapabilityStatementSoftwareComponent.releaseDate"); 1336 else if (Configuration.doAutoCreate()) 1337 this.releaseDate = new DateTimeType(); // bb 1338 return this.releaseDate; 1339 } 1340 1341 public boolean hasReleaseDateElement() { 1342 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1343 } 1344 1345 public boolean hasReleaseDate() { 1346 return this.releaseDate != null && !this.releaseDate.isEmpty(); 1347 } 1348 1349 /** 1350 * @param value {@link #releaseDate} (Date this version of the software was released.). This is the underlying object with id, value and extensions. The accessor "getReleaseDate" gives direct access to the value 1351 */ 1352 public CapabilityStatementSoftwareComponent setReleaseDateElement(DateTimeType value) { 1353 this.releaseDate = value; 1354 return this; 1355 } 1356 1357 /** 1358 * @return Date this version of the software was released. 1359 */ 1360 public Date getReleaseDate() { 1361 return this.releaseDate == null ? null : this.releaseDate.getValue(); 1362 } 1363 1364 /** 1365 * @param value Date this version of the software was released. 1366 */ 1367 public CapabilityStatementSoftwareComponent setReleaseDate(Date value) { 1368 if (value == null) 1369 this.releaseDate = null; 1370 else { 1371 if (this.releaseDate == null) 1372 this.releaseDate = new DateTimeType(); 1373 this.releaseDate.setValue(value); 1374 } 1375 return this; 1376 } 1377 1378 protected void listChildren(List<Property> children) { 1379 super.listChildren(children); 1380 children.add(new Property("name", "string", "Name the software is known by.", 0, 1, name)); 1381 children.add(new Property("version", "string", "The version identifier for the software covered by this statement.", 0, 1, version)); 1382 children.add(new Property("releaseDate", "dateTime", "Date this version of the software was released.", 0, 1, releaseDate)); 1383 } 1384 1385 @Override 1386 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1387 switch (_hash) { 1388 case 3373707: /*name*/ return new Property("name", "string", "Name the software is known by.", 0, 1, name); 1389 case 351608024: /*version*/ return new Property("version", "string", "The version identifier for the software covered by this statement.", 0, 1, version); 1390 case 212873301: /*releaseDate*/ return new Property("releaseDate", "dateTime", "Date this version of the software was released.", 0, 1, releaseDate); 1391 default: return super.getNamedProperty(_hash, _name, _checkValid); 1392 } 1393 1394 } 1395 1396 @Override 1397 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1398 switch (hash) { 1399 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1400 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1401 case 212873301: /*releaseDate*/ return this.releaseDate == null ? new Base[0] : new Base[] {this.releaseDate}; // DateTimeType 1402 default: return super.getProperty(hash, name, checkValid); 1403 } 1404 1405 } 1406 1407 @Override 1408 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1409 switch (hash) { 1410 case 3373707: // name 1411 this.name = TypeConvertor.castToString(value); // StringType 1412 return value; 1413 case 351608024: // version 1414 this.version = TypeConvertor.castToString(value); // StringType 1415 return value; 1416 case 212873301: // releaseDate 1417 this.releaseDate = TypeConvertor.castToDateTime(value); // DateTimeType 1418 return value; 1419 default: return super.setProperty(hash, name, value); 1420 } 1421 1422 } 1423 1424 @Override 1425 public Base setProperty(String name, Base value) throws FHIRException { 1426 if (name.equals("name")) { 1427 this.name = TypeConvertor.castToString(value); // StringType 1428 } else if (name.equals("version")) { 1429 this.version = TypeConvertor.castToString(value); // StringType 1430 } else if (name.equals("releaseDate")) { 1431 this.releaseDate = TypeConvertor.castToDateTime(value); // DateTimeType 1432 } else 1433 return super.setProperty(name, value); 1434 return value; 1435 } 1436 1437 @Override 1438 public void removeChild(String name, Base value) throws FHIRException { 1439 if (name.equals("name")) { 1440 this.name = null; 1441 } else if (name.equals("version")) { 1442 this.version = null; 1443 } else if (name.equals("releaseDate")) { 1444 this.releaseDate = null; 1445 } else 1446 super.removeChild(name, value); 1447 1448 } 1449 1450 @Override 1451 public Base makeProperty(int hash, String name) throws FHIRException { 1452 switch (hash) { 1453 case 3373707: return getNameElement(); 1454 case 351608024: return getVersionElement(); 1455 case 212873301: return getReleaseDateElement(); 1456 default: return super.makeProperty(hash, name); 1457 } 1458 1459 } 1460 1461 @Override 1462 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1463 switch (hash) { 1464 case 3373707: /*name*/ return new String[] {"string"}; 1465 case 351608024: /*version*/ return new String[] {"string"}; 1466 case 212873301: /*releaseDate*/ return new String[] {"dateTime"}; 1467 default: return super.getTypesForProperty(hash, name); 1468 } 1469 1470 } 1471 1472 @Override 1473 public Base addChild(String name) throws FHIRException { 1474 if (name.equals("name")) { 1475 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.software.name"); 1476 } 1477 else if (name.equals("version")) { 1478 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.software.version"); 1479 } 1480 else if (name.equals("releaseDate")) { 1481 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.software.releaseDate"); 1482 } 1483 else 1484 return super.addChild(name); 1485 } 1486 1487 public CapabilityStatementSoftwareComponent copy() { 1488 CapabilityStatementSoftwareComponent dst = new CapabilityStatementSoftwareComponent(); 1489 copyValues(dst); 1490 return dst; 1491 } 1492 1493 public void copyValues(CapabilityStatementSoftwareComponent dst) { 1494 super.copyValues(dst); 1495 dst.name = name == null ? null : name.copy(); 1496 dst.version = version == null ? null : version.copy(); 1497 dst.releaseDate = releaseDate == null ? null : releaseDate.copy(); 1498 } 1499 1500 @Override 1501 public boolean equalsDeep(Base other_) { 1502 if (!super.equalsDeep(other_)) 1503 return false; 1504 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1505 return false; 1506 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1507 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true) && compareDeep(releaseDate, o.releaseDate, true) 1508 ; 1509 } 1510 1511 @Override 1512 public boolean equalsShallow(Base other_) { 1513 if (!super.equalsShallow(other_)) 1514 return false; 1515 if (!(other_ instanceof CapabilityStatementSoftwareComponent)) 1516 return false; 1517 CapabilityStatementSoftwareComponent o = (CapabilityStatementSoftwareComponent) other_; 1518 return compareValues(name, o.name, true) && compareValues(version, o.version, true) && compareValues(releaseDate, o.releaseDate, true) 1519 ; 1520 } 1521 1522 public boolean isEmpty() { 1523 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, version, releaseDate 1524 ); 1525 } 1526 1527 public String fhirType() { 1528 return "CapabilityStatement.software"; 1529 1530 } 1531 1532 } 1533 1534 @Block() 1535 public static class CapabilityStatementImplementationComponent extends BackboneElement implements IBaseBackboneElement { 1536 /** 1537 * Information about the specific installation that this capability statement relates to. 1538 */ 1539 @Child(name = "description", type = {MarkdownType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1540 @Description(shortDefinition="Describes this specific instance", formalDefinition="Information about the specific installation that this capability statement relates to." ) 1541 protected MarkdownType description; 1542 1543 /** 1544 * An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces. 1545 */ 1546 @Child(name = "url", type = {UrlType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1547 @Description(shortDefinition="Base URL for the installation", formalDefinition="An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces." ) 1548 protected UrlType url; 1549 1550 /** 1551 * The organization responsible for the management of the instance and oversight of the data on the server at the specified URL. 1552 */ 1553 @Child(name = "custodian", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 1554 @Description(shortDefinition="Organization that manages the data", formalDefinition="The organization responsible for the management of the instance and oversight of the data on the server at the specified URL." ) 1555 protected Reference custodian; 1556 1557 private static final long serialVersionUID = -1848514556L; 1558 1559 /** 1560 * Constructor 1561 */ 1562 public CapabilityStatementImplementationComponent() { 1563 super(); 1564 } 1565 1566 /** 1567 * Constructor 1568 */ 1569 public CapabilityStatementImplementationComponent(String description) { 1570 super(); 1571 this.setDescription(description); 1572 } 1573 1574 /** 1575 * @return {@link #description} (Information about the specific installation that this capability statement relates to.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1576 */ 1577 public MarkdownType getDescriptionElement() { 1578 if (this.description == null) 1579 if (Configuration.errorOnAutoCreate()) 1580 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.description"); 1581 else if (Configuration.doAutoCreate()) 1582 this.description = new MarkdownType(); // bb 1583 return this.description; 1584 } 1585 1586 public boolean hasDescriptionElement() { 1587 return this.description != null && !this.description.isEmpty(); 1588 } 1589 1590 public boolean hasDescription() { 1591 return this.description != null && !this.description.isEmpty(); 1592 } 1593 1594 /** 1595 * @param value {@link #description} (Information about the specific installation that this capability statement relates to.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1596 */ 1597 public CapabilityStatementImplementationComponent setDescriptionElement(MarkdownType value) { 1598 this.description = value; 1599 return this; 1600 } 1601 1602 /** 1603 * @return Information about the specific installation that this capability statement relates to. 1604 */ 1605 public String getDescription() { 1606 return this.description == null ? null : this.description.getValue(); 1607 } 1608 1609 /** 1610 * @param value Information about the specific installation that this capability statement relates to. 1611 */ 1612 public CapabilityStatementImplementationComponent setDescription(String value) { 1613 if (this.description == null) 1614 this.description = new MarkdownType(); 1615 this.description.setValue(value); 1616 return this; 1617 } 1618 1619 /** 1620 * @return {@link #url} (An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1621 */ 1622 public UrlType getUrlElement() { 1623 if (this.url == null) 1624 if (Configuration.errorOnAutoCreate()) 1625 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.url"); 1626 else if (Configuration.doAutoCreate()) 1627 this.url = new UrlType(); // bb 1628 return this.url; 1629 } 1630 1631 public boolean hasUrlElement() { 1632 return this.url != null && !this.url.isEmpty(); 1633 } 1634 1635 public boolean hasUrl() { 1636 return this.url != null && !this.url.isEmpty(); 1637 } 1638 1639 /** 1640 * @param value {@link #url} (An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1641 */ 1642 public CapabilityStatementImplementationComponent setUrlElement(UrlType value) { 1643 this.url = value; 1644 return this; 1645 } 1646 1647 /** 1648 * @return An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces. 1649 */ 1650 public String getUrl() { 1651 return this.url == null ? null : this.url.getValue(); 1652 } 1653 1654 /** 1655 * @param value An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces. 1656 */ 1657 public CapabilityStatementImplementationComponent setUrl(String value) { 1658 if (Utilities.noString(value)) 1659 this.url = null; 1660 else { 1661 if (this.url == null) 1662 this.url = new UrlType(); 1663 this.url.setValue(value); 1664 } 1665 return this; 1666 } 1667 1668 /** 1669 * @return {@link #custodian} (The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.) 1670 */ 1671 public Reference getCustodian() { 1672 if (this.custodian == null) 1673 if (Configuration.errorOnAutoCreate()) 1674 throw new Error("Attempt to auto-create CapabilityStatementImplementationComponent.custodian"); 1675 else if (Configuration.doAutoCreate()) 1676 this.custodian = new Reference(); // cc 1677 return this.custodian; 1678 } 1679 1680 public boolean hasCustodian() { 1681 return this.custodian != null && !this.custodian.isEmpty(); 1682 } 1683 1684 /** 1685 * @param value {@link #custodian} (The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.) 1686 */ 1687 public CapabilityStatementImplementationComponent setCustodian(Reference value) { 1688 this.custodian = value; 1689 return this; 1690 } 1691 1692 protected void listChildren(List<Property> children) { 1693 super.listChildren(children); 1694 children.add(new Property("description", "markdown", "Information about the specific installation that this capability statement relates to.", 0, 1, description)); 1695 children.add(new Property("url", "url", "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 0, 1, url)); 1696 children.add(new Property("custodian", "Reference(Organization)", "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.", 0, 1, custodian)); 1697 } 1698 1699 @Override 1700 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1701 switch (_hash) { 1702 case -1724546052: /*description*/ return new Property("description", "markdown", "Information about the specific installation that this capability statement relates to.", 0, 1, description); 1703 case 116079: /*url*/ return new Property("url", "url", "An absolute base URL for the implementation. This forms the base for REST interfaces as well as the mailbox and document interfaces.", 0, 1, url); 1704 case 1611297262: /*custodian*/ return new Property("custodian", "Reference(Organization)", "The organization responsible for the management of the instance and oversight of the data on the server at the specified URL.", 0, 1, custodian); 1705 default: return super.getNamedProperty(_hash, _name, _checkValid); 1706 } 1707 1708 } 1709 1710 @Override 1711 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1712 switch (hash) { 1713 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1714 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 1715 case 1611297262: /*custodian*/ return this.custodian == null ? new Base[0] : new Base[] {this.custodian}; // Reference 1716 default: return super.getProperty(hash, name, checkValid); 1717 } 1718 1719 } 1720 1721 @Override 1722 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1723 switch (hash) { 1724 case -1724546052: // description 1725 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1726 return value; 1727 case 116079: // url 1728 this.url = TypeConvertor.castToUrl(value); // UrlType 1729 return value; 1730 case 1611297262: // custodian 1731 this.custodian = TypeConvertor.castToReference(value); // Reference 1732 return value; 1733 default: return super.setProperty(hash, name, value); 1734 } 1735 1736 } 1737 1738 @Override 1739 public Base setProperty(String name, Base value) throws FHIRException { 1740 if (name.equals("description")) { 1741 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1742 } else if (name.equals("url")) { 1743 this.url = TypeConvertor.castToUrl(value); // UrlType 1744 } else if (name.equals("custodian")) { 1745 this.custodian = TypeConvertor.castToReference(value); // Reference 1746 } else 1747 return super.setProperty(name, value); 1748 return value; 1749 } 1750 1751 @Override 1752 public void removeChild(String name, Base value) throws FHIRException { 1753 if (name.equals("description")) { 1754 this.description = null; 1755 } else if (name.equals("url")) { 1756 this.url = null; 1757 } else if (name.equals("custodian")) { 1758 this.custodian = null; 1759 } else 1760 super.removeChild(name, value); 1761 1762 } 1763 1764 @Override 1765 public Base makeProperty(int hash, String name) throws FHIRException { 1766 switch (hash) { 1767 case -1724546052: return getDescriptionElement(); 1768 case 116079: return getUrlElement(); 1769 case 1611297262: return getCustodian(); 1770 default: return super.makeProperty(hash, name); 1771 } 1772 1773 } 1774 1775 @Override 1776 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1777 switch (hash) { 1778 case -1724546052: /*description*/ return new String[] {"markdown"}; 1779 case 116079: /*url*/ return new String[] {"url"}; 1780 case 1611297262: /*custodian*/ return new String[] {"Reference"}; 1781 default: return super.getTypesForProperty(hash, name); 1782 } 1783 1784 } 1785 1786 @Override 1787 public Base addChild(String name) throws FHIRException { 1788 if (name.equals("description")) { 1789 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.implementation.description"); 1790 } 1791 else if (name.equals("url")) { 1792 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.implementation.url"); 1793 } 1794 else if (name.equals("custodian")) { 1795 this.custodian = new Reference(); 1796 return this.custodian; 1797 } 1798 else 1799 return super.addChild(name); 1800 } 1801 1802 public CapabilityStatementImplementationComponent copy() { 1803 CapabilityStatementImplementationComponent dst = new CapabilityStatementImplementationComponent(); 1804 copyValues(dst); 1805 return dst; 1806 } 1807 1808 public void copyValues(CapabilityStatementImplementationComponent dst) { 1809 super.copyValues(dst); 1810 dst.description = description == null ? null : description.copy(); 1811 dst.url = url == null ? null : url.copy(); 1812 dst.custodian = custodian == null ? null : custodian.copy(); 1813 } 1814 1815 @Override 1816 public boolean equalsDeep(Base other_) { 1817 if (!super.equalsDeep(other_)) 1818 return false; 1819 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 1820 return false; 1821 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 1822 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true) && compareDeep(custodian, o.custodian, true) 1823 ; 1824 } 1825 1826 @Override 1827 public boolean equalsShallow(Base other_) { 1828 if (!super.equalsShallow(other_)) 1829 return false; 1830 if (!(other_ instanceof CapabilityStatementImplementationComponent)) 1831 return false; 1832 CapabilityStatementImplementationComponent o = (CapabilityStatementImplementationComponent) other_; 1833 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 1834 } 1835 1836 public boolean isEmpty() { 1837 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, url, custodian 1838 ); 1839 } 1840 1841 public String fhirType() { 1842 return "CapabilityStatement.implementation"; 1843 1844 } 1845 1846 } 1847 1848 @Block() 1849 public static class CapabilityStatementRestComponent extends BackboneElement implements IBaseBackboneElement { 1850 /** 1851 * Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations. 1852 */ 1853 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1854 @Description(shortDefinition="client | server", formalDefinition="Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations." ) 1855 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/restful-capability-mode") 1856 protected Enumeration<RestfulCapabilityMode> mode; 1857 1858 /** 1859 * Information about the system's restful capabilities that apply across all applications, such as security. 1860 */ 1861 @Child(name = "documentation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1862 @Description(shortDefinition="General description of implementation", formalDefinition="Information about the system's restful capabilities that apply across all applications, such as security." ) 1863 protected MarkdownType documentation; 1864 1865 /** 1866 * Information about security implementation from an interface perspective - what a client needs to know. 1867 */ 1868 @Child(name = "security", type = {}, order=3, min=0, max=1, modifier=false, summary=true) 1869 @Description(shortDefinition="Information about security of implementation", formalDefinition="Information about security implementation from an interface perspective - what a client needs to know." ) 1870 protected CapabilityStatementRestSecurityComponent security; 1871 1872 /** 1873 * A specification of the restful capabilities of the solution for a specific resource type. 1874 */ 1875 @Child(name = "resource", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1876 @Description(shortDefinition="Resource served on the REST interface", formalDefinition="A specification of the restful capabilities of the solution for a specific resource type." ) 1877 protected List<CapabilityStatementRestResourceComponent> resource; 1878 1879 /** 1880 * A specification of restful operations supported by the system. 1881 */ 1882 @Child(name = "interaction", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1883 @Description(shortDefinition="What operations are supported?", formalDefinition="A specification of restful operations supported by the system." ) 1884 protected List<SystemInteractionComponent> interaction; 1885 1886 /** 1887 * Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. This is only for searches executed against the system-level endpoint. 1888 */ 1889 @Child(name = "searchParam", type = {CapabilityStatementRestResourceSearchParamComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1890 @Description(shortDefinition="Search parameters for searching all resources", formalDefinition="Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. This is only for searches executed against the system-level endpoint." ) 1891 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 1892 1893 /** 1894 * Definition of an operation or a named query together with its parameters and their meaning and type. 1895 */ 1896 @Child(name = "operation", type = {CapabilityStatementRestResourceOperationComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1897 @Description(shortDefinition="Definition of a system level operation", formalDefinition="Definition of an operation or a named query together with its parameters and their meaning and type." ) 1898 protected List<CapabilityStatementRestResourceOperationComponent> operation; 1899 1900 /** 1901 * An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL . 1902 */ 1903 @Child(name = "compartment", type = {CanonicalType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1904 @Description(shortDefinition="Compartments served/used by system", formalDefinition="An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL ." ) 1905 protected List<CanonicalType> compartment; 1906 1907 private static final long serialVersionUID = -1442029817L; 1908 1909 /** 1910 * Constructor 1911 */ 1912 public CapabilityStatementRestComponent() { 1913 super(); 1914 } 1915 1916 /** 1917 * Constructor 1918 */ 1919 public CapabilityStatementRestComponent(RestfulCapabilityMode mode) { 1920 super(); 1921 this.setMode(mode); 1922 } 1923 1924 /** 1925 * @return {@link #mode} (Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1926 */ 1927 public Enumeration<RestfulCapabilityMode> getModeElement() { 1928 if (this.mode == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.mode"); 1931 else if (Configuration.doAutoCreate()) 1932 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); // bb 1933 return this.mode; 1934 } 1935 1936 public boolean hasModeElement() { 1937 return this.mode != null && !this.mode.isEmpty(); 1938 } 1939 1940 public boolean hasMode() { 1941 return this.mode != null && !this.mode.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #mode} (Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1946 */ 1947 public CapabilityStatementRestComponent setModeElement(Enumeration<RestfulCapabilityMode> value) { 1948 this.mode = value; 1949 return this; 1950 } 1951 1952 /** 1953 * @return Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations. 1954 */ 1955 public RestfulCapabilityMode getMode() { 1956 return this.mode == null ? null : this.mode.getValue(); 1957 } 1958 1959 /** 1960 * @param value Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations. 1961 */ 1962 public CapabilityStatementRestComponent setMode(RestfulCapabilityMode value) { 1963 if (this.mode == null) 1964 this.mode = new Enumeration<RestfulCapabilityMode>(new RestfulCapabilityModeEnumFactory()); 1965 this.mode.setValue(value); 1966 return this; 1967 } 1968 1969 /** 1970 * @return {@link #documentation} (Information about the system's restful capabilities that apply across all applications, such as security.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1971 */ 1972 public MarkdownType getDocumentationElement() { 1973 if (this.documentation == null) 1974 if (Configuration.errorOnAutoCreate()) 1975 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.documentation"); 1976 else if (Configuration.doAutoCreate()) 1977 this.documentation = new MarkdownType(); // bb 1978 return this.documentation; 1979 } 1980 1981 public boolean hasDocumentationElement() { 1982 return this.documentation != null && !this.documentation.isEmpty(); 1983 } 1984 1985 public boolean hasDocumentation() { 1986 return this.documentation != null && !this.documentation.isEmpty(); 1987 } 1988 1989 /** 1990 * @param value {@link #documentation} (Information about the system's restful capabilities that apply across all applications, such as security.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1991 */ 1992 public CapabilityStatementRestComponent setDocumentationElement(MarkdownType value) { 1993 this.documentation = value; 1994 return this; 1995 } 1996 1997 /** 1998 * @return Information about the system's restful capabilities that apply across all applications, such as security. 1999 */ 2000 public String getDocumentation() { 2001 return this.documentation == null ? null : this.documentation.getValue(); 2002 } 2003 2004 /** 2005 * @param value Information about the system's restful capabilities that apply across all applications, such as security. 2006 */ 2007 public CapabilityStatementRestComponent setDocumentation(String value) { 2008 if (Utilities.noString(value)) 2009 this.documentation = null; 2010 else { 2011 if (this.documentation == null) 2012 this.documentation = new MarkdownType(); 2013 this.documentation.setValue(value); 2014 } 2015 return this; 2016 } 2017 2018 /** 2019 * @return {@link #security} (Information about security implementation from an interface perspective - what a client needs to know.) 2020 */ 2021 public CapabilityStatementRestSecurityComponent getSecurity() { 2022 if (this.security == null) 2023 if (Configuration.errorOnAutoCreate()) 2024 throw new Error("Attempt to auto-create CapabilityStatementRestComponent.security"); 2025 else if (Configuration.doAutoCreate()) 2026 this.security = new CapabilityStatementRestSecurityComponent(); // cc 2027 return this.security; 2028 } 2029 2030 public boolean hasSecurity() { 2031 return this.security != null && !this.security.isEmpty(); 2032 } 2033 2034 /** 2035 * @param value {@link #security} (Information about security implementation from an interface perspective - what a client needs to know.) 2036 */ 2037 public CapabilityStatementRestComponent setSecurity(CapabilityStatementRestSecurityComponent value) { 2038 this.security = value; 2039 return this; 2040 } 2041 2042 /** 2043 * @return {@link #resource} (A specification of the restful capabilities of the solution for a specific resource type.) 2044 */ 2045 public List<CapabilityStatementRestResourceComponent> getResource() { 2046 if (this.resource == null) 2047 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2048 return this.resource; 2049 } 2050 2051 /** 2052 * @return Returns a reference to <code>this</code> for easy method chaining 2053 */ 2054 public CapabilityStatementRestComponent setResource(List<CapabilityStatementRestResourceComponent> theResource) { 2055 this.resource = theResource; 2056 return this; 2057 } 2058 2059 public boolean hasResource() { 2060 if (this.resource == null) 2061 return false; 2062 for (CapabilityStatementRestResourceComponent item : this.resource) 2063 if (!item.isEmpty()) 2064 return true; 2065 return false; 2066 } 2067 2068 public CapabilityStatementRestResourceComponent addResource() { //3 2069 CapabilityStatementRestResourceComponent t = new CapabilityStatementRestResourceComponent(); 2070 if (this.resource == null) 2071 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2072 this.resource.add(t); 2073 return t; 2074 } 2075 2076 public CapabilityStatementRestComponent addResource(CapabilityStatementRestResourceComponent t) { //3 2077 if (t == null) 2078 return this; 2079 if (this.resource == null) 2080 this.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2081 this.resource.add(t); 2082 return this; 2083 } 2084 2085 /** 2086 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist {3} 2087 */ 2088 public CapabilityStatementRestResourceComponent getResourceFirstRep() { 2089 if (getResource().isEmpty()) { 2090 addResource(); 2091 } 2092 return getResource().get(0); 2093 } 2094 2095 /** 2096 * @return {@link #interaction} (A specification of restful operations supported by the system.) 2097 */ 2098 public List<SystemInteractionComponent> getInteraction() { 2099 if (this.interaction == null) 2100 this.interaction = new ArrayList<SystemInteractionComponent>(); 2101 return this.interaction; 2102 } 2103 2104 /** 2105 * @return Returns a reference to <code>this</code> for easy method chaining 2106 */ 2107 public CapabilityStatementRestComponent setInteraction(List<SystemInteractionComponent> theInteraction) { 2108 this.interaction = theInteraction; 2109 return this; 2110 } 2111 2112 public boolean hasInteraction() { 2113 if (this.interaction == null) 2114 return false; 2115 for (SystemInteractionComponent item : this.interaction) 2116 if (!item.isEmpty()) 2117 return true; 2118 return false; 2119 } 2120 2121 public SystemInteractionComponent addInteraction() { //3 2122 SystemInteractionComponent t = new SystemInteractionComponent(); 2123 if (this.interaction == null) 2124 this.interaction = new ArrayList<SystemInteractionComponent>(); 2125 this.interaction.add(t); 2126 return t; 2127 } 2128 2129 public CapabilityStatementRestComponent addInteraction(SystemInteractionComponent t) { //3 2130 if (t == null) 2131 return this; 2132 if (this.interaction == null) 2133 this.interaction = new ArrayList<SystemInteractionComponent>(); 2134 this.interaction.add(t); 2135 return this; 2136 } 2137 2138 /** 2139 * @return The first repetition of repeating field {@link #interaction}, creating it if it does not already exist {3} 2140 */ 2141 public SystemInteractionComponent getInteractionFirstRep() { 2142 if (getInteraction().isEmpty()) { 2143 addInteraction(); 2144 } 2145 return getInteraction().get(0); 2146 } 2147 2148 /** 2149 * @return {@link #searchParam} (Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. This is only for searches executed against the system-level endpoint.) 2150 */ 2151 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 2152 if (this.searchParam == null) 2153 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2154 return this.searchParam; 2155 } 2156 2157 /** 2158 * @return Returns a reference to <code>this</code> for easy method chaining 2159 */ 2160 public CapabilityStatementRestComponent setSearchParam(List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 2161 this.searchParam = theSearchParam; 2162 return this; 2163 } 2164 2165 public boolean hasSearchParam() { 2166 if (this.searchParam == null) 2167 return false; 2168 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 2169 if (!item.isEmpty()) 2170 return true; 2171 return false; 2172 } 2173 2174 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { //3 2175 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 2176 if (this.searchParam == null) 2177 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2178 this.searchParam.add(t); 2179 return t; 2180 } 2181 2182 public CapabilityStatementRestComponent addSearchParam(CapabilityStatementRestResourceSearchParamComponent t) { //3 2183 if (t == null) 2184 return this; 2185 if (this.searchParam == null) 2186 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2187 this.searchParam.add(t); 2188 return this; 2189 } 2190 2191 /** 2192 * @return The first repetition of repeating field {@link #searchParam}, creating it if it does not already exist {3} 2193 */ 2194 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 2195 if (getSearchParam().isEmpty()) { 2196 addSearchParam(); 2197 } 2198 return getSearchParam().get(0); 2199 } 2200 2201 /** 2202 * @return {@link #operation} (Definition of an operation or a named query together with its parameters and their meaning and type.) 2203 */ 2204 public List<CapabilityStatementRestResourceOperationComponent> getOperation() { 2205 if (this.operation == null) 2206 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2207 return this.operation; 2208 } 2209 2210 /** 2211 * @return Returns a reference to <code>this</code> for easy method chaining 2212 */ 2213 public CapabilityStatementRestComponent setOperation(List<CapabilityStatementRestResourceOperationComponent> theOperation) { 2214 this.operation = theOperation; 2215 return this; 2216 } 2217 2218 public boolean hasOperation() { 2219 if (this.operation == null) 2220 return false; 2221 for (CapabilityStatementRestResourceOperationComponent item : this.operation) 2222 if (!item.isEmpty()) 2223 return true; 2224 return false; 2225 } 2226 2227 public CapabilityStatementRestResourceOperationComponent addOperation() { //3 2228 CapabilityStatementRestResourceOperationComponent t = new CapabilityStatementRestResourceOperationComponent(); 2229 if (this.operation == null) 2230 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2231 this.operation.add(t); 2232 return t; 2233 } 2234 2235 public CapabilityStatementRestComponent addOperation(CapabilityStatementRestResourceOperationComponent t) { //3 2236 if (t == null) 2237 return this; 2238 if (this.operation == null) 2239 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2240 this.operation.add(t); 2241 return this; 2242 } 2243 2244 /** 2245 * @return The first repetition of repeating field {@link #operation}, creating it if it does not already exist {3} 2246 */ 2247 public CapabilityStatementRestResourceOperationComponent getOperationFirstRep() { 2248 if (getOperation().isEmpty()) { 2249 addOperation(); 2250 } 2251 return getOperation().get(0); 2252 } 2253 2254 /** 2255 * @return {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2256 */ 2257 public List<CanonicalType> getCompartment() { 2258 if (this.compartment == null) 2259 this.compartment = new ArrayList<CanonicalType>(); 2260 return this.compartment; 2261 } 2262 2263 /** 2264 * @return Returns a reference to <code>this</code> for easy method chaining 2265 */ 2266 public CapabilityStatementRestComponent setCompartment(List<CanonicalType> theCompartment) { 2267 this.compartment = theCompartment; 2268 return this; 2269 } 2270 2271 public boolean hasCompartment() { 2272 if (this.compartment == null) 2273 return false; 2274 for (CanonicalType item : this.compartment) 2275 if (!item.isEmpty()) 2276 return true; 2277 return false; 2278 } 2279 2280 /** 2281 * @return {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2282 */ 2283 public CanonicalType addCompartmentElement() {//2 2284 CanonicalType t = new CanonicalType(); 2285 if (this.compartment == null) 2286 this.compartment = new ArrayList<CanonicalType>(); 2287 this.compartment.add(t); 2288 return t; 2289 } 2290 2291 /** 2292 * @param value {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2293 */ 2294 public CapabilityStatementRestComponent addCompartment(String value) { //1 2295 CanonicalType t = new CanonicalType(); 2296 t.setValue(value); 2297 if (this.compartment == null) 2298 this.compartment = new ArrayList<CanonicalType>(); 2299 this.compartment.add(t); 2300 return this; 2301 } 2302 2303 /** 2304 * @param value {@link #compartment} (An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .) 2305 */ 2306 public boolean hasCompartment(String value) { 2307 if (this.compartment == null) 2308 return false; 2309 for (CanonicalType v : this.compartment) 2310 if (v.getValue().equals(value)) // canonical 2311 return true; 2312 return false; 2313 } 2314 2315 protected void listChildren(List<Property> children) { 2316 super.listChildren(children); 2317 children.add(new Property("mode", "code", "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 0, 1, mode)); 2318 children.add(new Property("documentation", "markdown", "Information about the system's restful capabilities that apply across all applications, such as security.", 0, 1, documentation)); 2319 children.add(new Property("security", "", "Information about security implementation from an interface perspective - what a client needs to know.", 0, 1, security)); 2320 children.add(new Property("resource", "", "A specification of the restful capabilities of the solution for a specific resource type.", 0, java.lang.Integer.MAX_VALUE, resource)); 2321 children.add(new Property("interaction", "", "A specification of restful operations supported by the system.", 0, java.lang.Integer.MAX_VALUE, interaction)); 2322 children.add(new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. This is only for searches executed against the system-level endpoint.", 0, java.lang.Integer.MAX_VALUE, searchParam)); 2323 children.add(new Property("operation", "@CapabilityStatement.rest.resource.operation", "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, java.lang.Integer.MAX_VALUE, operation)); 2324 children.add(new Property("compartment", "canonical(CompartmentDefinition)", "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 0, java.lang.Integer.MAX_VALUE, compartment)); 2325 } 2326 2327 @Override 2328 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2329 switch (_hash) { 2330 case 3357091: /*mode*/ return new Property("mode", "code", "Identifies whether this portion of the statement is describing the ability to initiate or receive restful operations.", 0, 1, mode); 2331 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Information about the system's restful capabilities that apply across all applications, such as security.", 0, 1, documentation); 2332 case 949122880: /*security*/ return new Property("security", "", "Information about security implementation from an interface perspective - what a client needs to know.", 0, 1, security); 2333 case -341064690: /*resource*/ return new Property("resource", "", "A specification of the restful capabilities of the solution for a specific resource type.", 0, java.lang.Integer.MAX_VALUE, resource); 2334 case 1844104722: /*interaction*/ return new Property("interaction", "", "A specification of restful operations supported by the system.", 0, java.lang.Integer.MAX_VALUE, interaction); 2335 case -553645115: /*searchParam*/ return new Property("searchParam", "@CapabilityStatement.rest.resource.searchParam", "Search parameters that are supported for searching all resources for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. This is only for searches executed against the system-level endpoint.", 0, java.lang.Integer.MAX_VALUE, searchParam); 2336 case 1662702951: /*operation*/ return new Property("operation", "@CapabilityStatement.rest.resource.operation", "Definition of an operation or a named query together with its parameters and their meaning and type.", 0, java.lang.Integer.MAX_VALUE, operation); 2337 case -397756334: /*compartment*/ return new Property("compartment", "canonical(CompartmentDefinition)", "An absolute URI which is a reference to the definition of a compartment that the system supports. The reference is to a CompartmentDefinition resource by its canonical URL .", 0, java.lang.Integer.MAX_VALUE, compartment); 2338 default: return super.getNamedProperty(_hash, _name, _checkValid); 2339 } 2340 2341 } 2342 2343 @Override 2344 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2345 switch (hash) { 2346 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<RestfulCapabilityMode> 2347 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 2348 case 949122880: /*security*/ return this.security == null ? new Base[0] : new Base[] {this.security}; // CapabilityStatementRestSecurityComponent 2349 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CapabilityStatementRestResourceComponent 2350 case 1844104722: /*interaction*/ return this.interaction == null ? new Base[0] : this.interaction.toArray(new Base[this.interaction.size()]); // SystemInteractionComponent 2351 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 2352 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestResourceOperationComponent 2353 case -397756334: /*compartment*/ return this.compartment == null ? new Base[0] : this.compartment.toArray(new Base[this.compartment.size()]); // CanonicalType 2354 default: return super.getProperty(hash, name, checkValid); 2355 } 2356 2357 } 2358 2359 @Override 2360 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2361 switch (hash) { 2362 case 3357091: // mode 2363 value = new RestfulCapabilityModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2364 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 2365 return value; 2366 case 1587405498: // documentation 2367 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 2368 return value; 2369 case 949122880: // security 2370 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 2371 return value; 2372 case -341064690: // resource 2373 this.getResource().add((CapabilityStatementRestResourceComponent) value); // CapabilityStatementRestResourceComponent 2374 return value; 2375 case 1844104722: // interaction 2376 this.getInteraction().add((SystemInteractionComponent) value); // SystemInteractionComponent 2377 return value; 2378 case -553645115: // searchParam 2379 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 2380 return value; 2381 case 1662702951: // operation 2382 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); // CapabilityStatementRestResourceOperationComponent 2383 return value; 2384 case -397756334: // compartment 2385 this.getCompartment().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2386 return value; 2387 default: return super.setProperty(hash, name, value); 2388 } 2389 2390 } 2391 2392 @Override 2393 public Base setProperty(String name, Base value) throws FHIRException { 2394 if (name.equals("mode")) { 2395 value = new RestfulCapabilityModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2396 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 2397 } else if (name.equals("documentation")) { 2398 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 2399 } else if (name.equals("security")) { 2400 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 2401 } else if (name.equals("resource")) { 2402 this.getResource().add((CapabilityStatementRestResourceComponent) value); 2403 } else if (name.equals("interaction")) { 2404 this.getInteraction().add((SystemInteractionComponent) value); 2405 } else if (name.equals("searchParam")) { 2406 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 2407 } else if (name.equals("operation")) { 2408 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); 2409 } else if (name.equals("compartment")) { 2410 this.getCompartment().add(TypeConvertor.castToCanonical(value)); 2411 } else 2412 return super.setProperty(name, value); 2413 return value; 2414 } 2415 2416 @Override 2417 public void removeChild(String name, Base value) throws FHIRException { 2418 if (name.equals("mode")) { 2419 value = new RestfulCapabilityModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2420 this.mode = (Enumeration) value; // Enumeration<RestfulCapabilityMode> 2421 } else if (name.equals("documentation")) { 2422 this.documentation = null; 2423 } else if (name.equals("security")) { 2424 this.security = (CapabilityStatementRestSecurityComponent) value; // CapabilityStatementRestSecurityComponent 2425 } else if (name.equals("resource")) { 2426 this.getResource().remove((CapabilityStatementRestResourceComponent) value); 2427 } else if (name.equals("interaction")) { 2428 this.getInteraction().remove((SystemInteractionComponent) value); 2429 } else if (name.equals("searchParam")) { 2430 this.getSearchParam().remove((CapabilityStatementRestResourceSearchParamComponent) value); 2431 } else if (name.equals("operation")) { 2432 this.getOperation().remove((CapabilityStatementRestResourceOperationComponent) value); 2433 } else if (name.equals("compartment")) { 2434 this.getCompartment().remove(value); 2435 } else 2436 super.removeChild(name, value); 2437 2438 } 2439 2440 @Override 2441 public Base makeProperty(int hash, String name) throws FHIRException { 2442 switch (hash) { 2443 case 3357091: return getModeElement(); 2444 case 1587405498: return getDocumentationElement(); 2445 case 949122880: return getSecurity(); 2446 case -341064690: return addResource(); 2447 case 1844104722: return addInteraction(); 2448 case -553645115: return addSearchParam(); 2449 case 1662702951: return addOperation(); 2450 case -397756334: return addCompartmentElement(); 2451 default: return super.makeProperty(hash, name); 2452 } 2453 2454 } 2455 2456 @Override 2457 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2458 switch (hash) { 2459 case 3357091: /*mode*/ return new String[] {"code"}; 2460 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 2461 case 949122880: /*security*/ return new String[] {}; 2462 case -341064690: /*resource*/ return new String[] {}; 2463 case 1844104722: /*interaction*/ return new String[] {}; 2464 case -553645115: /*searchParam*/ return new String[] {"@CapabilityStatement.rest.resource.searchParam"}; 2465 case 1662702951: /*operation*/ return new String[] {"@CapabilityStatement.rest.resource.operation"}; 2466 case -397756334: /*compartment*/ return new String[] {"canonical"}; 2467 default: return super.getTypesForProperty(hash, name); 2468 } 2469 2470 } 2471 2472 @Override 2473 public Base addChild(String name) throws FHIRException { 2474 if (name.equals("mode")) { 2475 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.mode"); 2476 } 2477 else if (name.equals("documentation")) { 2478 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.documentation"); 2479 } 2480 else if (name.equals("security")) { 2481 this.security = new CapabilityStatementRestSecurityComponent(); 2482 return this.security; 2483 } 2484 else if (name.equals("resource")) { 2485 return addResource(); 2486 } 2487 else if (name.equals("interaction")) { 2488 return addInteraction(); 2489 } 2490 else if (name.equals("searchParam")) { 2491 return addSearchParam(); 2492 } 2493 else if (name.equals("operation")) { 2494 return addOperation(); 2495 } 2496 else if (name.equals("compartment")) { 2497 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.compartment"); 2498 } 2499 else 2500 return super.addChild(name); 2501 } 2502 2503 public CapabilityStatementRestComponent copy() { 2504 CapabilityStatementRestComponent dst = new CapabilityStatementRestComponent(); 2505 copyValues(dst); 2506 return dst; 2507 } 2508 2509 public void copyValues(CapabilityStatementRestComponent dst) { 2510 super.copyValues(dst); 2511 dst.mode = mode == null ? null : mode.copy(); 2512 dst.documentation = documentation == null ? null : documentation.copy(); 2513 dst.security = security == null ? null : security.copy(); 2514 if (resource != null) { 2515 dst.resource = new ArrayList<CapabilityStatementRestResourceComponent>(); 2516 for (CapabilityStatementRestResourceComponent i : resource) 2517 dst.resource.add(i.copy()); 2518 }; 2519 if (interaction != null) { 2520 dst.interaction = new ArrayList<SystemInteractionComponent>(); 2521 for (SystemInteractionComponent i : interaction) 2522 dst.interaction.add(i.copy()); 2523 }; 2524 if (searchParam != null) { 2525 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 2526 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 2527 dst.searchParam.add(i.copy()); 2528 }; 2529 if (operation != null) { 2530 dst.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 2531 for (CapabilityStatementRestResourceOperationComponent i : operation) 2532 dst.operation.add(i.copy()); 2533 }; 2534 if (compartment != null) { 2535 dst.compartment = new ArrayList<CanonicalType>(); 2536 for (CanonicalType i : compartment) 2537 dst.compartment.add(i.copy()); 2538 }; 2539 } 2540 2541 @Override 2542 public boolean equalsDeep(Base other_) { 2543 if (!super.equalsDeep(other_)) 2544 return false; 2545 if (!(other_ instanceof CapabilityStatementRestComponent)) 2546 return false; 2547 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 2548 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) && compareDeep(security, o.security, true) 2549 && compareDeep(resource, o.resource, true) && compareDeep(interaction, o.interaction, true) && compareDeep(searchParam, o.searchParam, true) 2550 && compareDeep(operation, o.operation, true) && compareDeep(compartment, o.compartment, true); 2551 } 2552 2553 @Override 2554 public boolean equalsShallow(Base other_) { 2555 if (!super.equalsShallow(other_)) 2556 return false; 2557 if (!(other_ instanceof CapabilityStatementRestComponent)) 2558 return false; 2559 CapabilityStatementRestComponent o = (CapabilityStatementRestComponent) other_; 2560 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true) && compareValues(compartment, o.compartment, true) 2561 ; 2562 } 2563 2564 public boolean isEmpty() { 2565 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, security 2566 , resource, interaction, searchParam, operation, compartment); 2567 } 2568 2569 public String fhirType() { 2570 return "CapabilityStatement.rest"; 2571 2572 } 2573 2574 } 2575 2576 @Block() 2577 public static class CapabilityStatementRestSecurityComponent extends BackboneElement implements IBaseBackboneElement { 2578 /** 2579 * Server adds CORS headers when responding to requests - this enables Javascript applications to use the server. 2580 */ 2581 @Child(name = "cors", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2582 @Description(shortDefinition="Adds CORS Headers (http://enable-cors.org/)", formalDefinition="Server adds CORS headers when responding to requests - this enables Javascript applications to use the server." ) 2583 protected BooleanType cors; 2584 2585 /** 2586 * Types of security services that are supported/required by the system. 2587 */ 2588 @Child(name = "service", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2589 @Description(shortDefinition="OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", formalDefinition="Types of security services that are supported/required by the system." ) 2590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/restful-security-service") 2591 protected List<CodeableConcept> service; 2592 2593 /** 2594 * General description of how security works. 2595 */ 2596 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2597 @Description(shortDefinition="General description of how security works", formalDefinition="General description of how security works." ) 2598 protected MarkdownType description; 2599 2600 private static final long serialVersionUID = -1348900500L; 2601 2602 /** 2603 * Constructor 2604 */ 2605 public CapabilityStatementRestSecurityComponent() { 2606 super(); 2607 } 2608 2609 /** 2610 * @return {@link #cors} (Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.). This is the underlying object with id, value and extensions. The accessor "getCors" gives direct access to the value 2611 */ 2612 public BooleanType getCorsElement() { 2613 if (this.cors == null) 2614 if (Configuration.errorOnAutoCreate()) 2615 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.cors"); 2616 else if (Configuration.doAutoCreate()) 2617 this.cors = new BooleanType(); // bb 2618 return this.cors; 2619 } 2620 2621 public boolean hasCorsElement() { 2622 return this.cors != null && !this.cors.isEmpty(); 2623 } 2624 2625 public boolean hasCors() { 2626 return this.cors != null && !this.cors.isEmpty(); 2627 } 2628 2629 /** 2630 * @param value {@link #cors} (Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.). This is the underlying object with id, value and extensions. The accessor "getCors" gives direct access to the value 2631 */ 2632 public CapabilityStatementRestSecurityComponent setCorsElement(BooleanType value) { 2633 this.cors = value; 2634 return this; 2635 } 2636 2637 /** 2638 * @return Server adds CORS headers when responding to requests - this enables Javascript applications to use the server. 2639 */ 2640 public boolean getCors() { 2641 return this.cors == null || this.cors.isEmpty() ? false : this.cors.getValue(); 2642 } 2643 2644 /** 2645 * @param value Server adds CORS headers when responding to requests - this enables Javascript applications to use the server. 2646 */ 2647 public CapabilityStatementRestSecurityComponent setCors(boolean value) { 2648 if (this.cors == null) 2649 this.cors = new BooleanType(); 2650 this.cors.setValue(value); 2651 return this; 2652 } 2653 2654 /** 2655 * @return {@link #service} (Types of security services that are supported/required by the system.) 2656 */ 2657 public List<CodeableConcept> getService() { 2658 if (this.service == null) 2659 this.service = new ArrayList<CodeableConcept>(); 2660 return this.service; 2661 } 2662 2663 /** 2664 * @return Returns a reference to <code>this</code> for easy method chaining 2665 */ 2666 public CapabilityStatementRestSecurityComponent setService(List<CodeableConcept> theService) { 2667 this.service = theService; 2668 return this; 2669 } 2670 2671 public boolean hasService() { 2672 if (this.service == null) 2673 return false; 2674 for (CodeableConcept item : this.service) 2675 if (!item.isEmpty()) 2676 return true; 2677 return false; 2678 } 2679 2680 public CodeableConcept addService() { //3 2681 CodeableConcept t = new CodeableConcept(); 2682 if (this.service == null) 2683 this.service = new ArrayList<CodeableConcept>(); 2684 this.service.add(t); 2685 return t; 2686 } 2687 2688 public CapabilityStatementRestSecurityComponent addService(CodeableConcept t) { //3 2689 if (t == null) 2690 return this; 2691 if (this.service == null) 2692 this.service = new ArrayList<CodeableConcept>(); 2693 this.service.add(t); 2694 return this; 2695 } 2696 2697 /** 2698 * @return The first repetition of repeating field {@link #service}, creating it if it does not already exist {3} 2699 */ 2700 public CodeableConcept getServiceFirstRep() { 2701 if (getService().isEmpty()) { 2702 addService(); 2703 } 2704 return getService().get(0); 2705 } 2706 2707 /** 2708 * @return {@link #description} (General description of how security works.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2709 */ 2710 public MarkdownType getDescriptionElement() { 2711 if (this.description == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create CapabilityStatementRestSecurityComponent.description"); 2714 else if (Configuration.doAutoCreate()) 2715 this.description = new MarkdownType(); // bb 2716 return this.description; 2717 } 2718 2719 public boolean hasDescriptionElement() { 2720 return this.description != null && !this.description.isEmpty(); 2721 } 2722 2723 public boolean hasDescription() { 2724 return this.description != null && !this.description.isEmpty(); 2725 } 2726 2727 /** 2728 * @param value {@link #description} (General description of how security works.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2729 */ 2730 public CapabilityStatementRestSecurityComponent setDescriptionElement(MarkdownType value) { 2731 this.description = value; 2732 return this; 2733 } 2734 2735 /** 2736 * @return General description of how security works. 2737 */ 2738 public String getDescription() { 2739 return this.description == null ? null : this.description.getValue(); 2740 } 2741 2742 /** 2743 * @param value General description of how security works. 2744 */ 2745 public CapabilityStatementRestSecurityComponent setDescription(String value) { 2746 if (Utilities.noString(value)) 2747 this.description = null; 2748 else { 2749 if (this.description == null) 2750 this.description = new MarkdownType(); 2751 this.description.setValue(value); 2752 } 2753 return this; 2754 } 2755 2756 protected void listChildren(List<Property> children) { 2757 super.listChildren(children); 2758 children.add(new Property("cors", "boolean", "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.", 0, 1, cors)); 2759 children.add(new Property("service", "CodeableConcept", "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, service)); 2760 children.add(new Property("description", "markdown", "General description of how security works.", 0, 1, description)); 2761 } 2762 2763 @Override 2764 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2765 switch (_hash) { 2766 case 3059629: /*cors*/ return new Property("cors", "boolean", "Server adds CORS headers when responding to requests - this enables Javascript applications to use the server.", 0, 1, cors); 2767 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "Types of security services that are supported/required by the system.", 0, java.lang.Integer.MAX_VALUE, service); 2768 case -1724546052: /*description*/ return new Property("description", "markdown", "General description of how security works.", 0, 1, description); 2769 default: return super.getNamedProperty(_hash, _name, _checkValid); 2770 } 2771 2772 } 2773 2774 @Override 2775 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2776 switch (hash) { 2777 case 3059629: /*cors*/ return this.cors == null ? new Base[0] : new Base[] {this.cors}; // BooleanType 2778 case 1984153269: /*service*/ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // CodeableConcept 2779 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2780 default: return super.getProperty(hash, name, checkValid); 2781 } 2782 2783 } 2784 2785 @Override 2786 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2787 switch (hash) { 2788 case 3059629: // cors 2789 this.cors = TypeConvertor.castToBoolean(value); // BooleanType 2790 return value; 2791 case 1984153269: // service 2792 this.getService().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2793 return value; 2794 case -1724546052: // description 2795 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2796 return value; 2797 default: return super.setProperty(hash, name, value); 2798 } 2799 2800 } 2801 2802 @Override 2803 public Base setProperty(String name, Base value) throws FHIRException { 2804 if (name.equals("cors")) { 2805 this.cors = TypeConvertor.castToBoolean(value); // BooleanType 2806 } else if (name.equals("service")) { 2807 this.getService().add(TypeConvertor.castToCodeableConcept(value)); 2808 } else if (name.equals("description")) { 2809 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2810 } else 2811 return super.setProperty(name, value); 2812 return value; 2813 } 2814 2815 @Override 2816 public void removeChild(String name, Base value) throws FHIRException { 2817 if (name.equals("cors")) { 2818 this.cors = null; 2819 } else if (name.equals("service")) { 2820 this.getService().remove(value); 2821 } else if (name.equals("description")) { 2822 this.description = null; 2823 } else 2824 super.removeChild(name, value); 2825 2826 } 2827 2828 @Override 2829 public Base makeProperty(int hash, String name) throws FHIRException { 2830 switch (hash) { 2831 case 3059629: return getCorsElement(); 2832 case 1984153269: return addService(); 2833 case -1724546052: return getDescriptionElement(); 2834 default: return super.makeProperty(hash, name); 2835 } 2836 2837 } 2838 2839 @Override 2840 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2841 switch (hash) { 2842 case 3059629: /*cors*/ return new String[] {"boolean"}; 2843 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 2844 case -1724546052: /*description*/ return new String[] {"markdown"}; 2845 default: return super.getTypesForProperty(hash, name); 2846 } 2847 2848 } 2849 2850 @Override 2851 public Base addChild(String name) throws FHIRException { 2852 if (name.equals("cors")) { 2853 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.security.cors"); 2854 } 2855 else if (name.equals("service")) { 2856 return addService(); 2857 } 2858 else if (name.equals("description")) { 2859 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.security.description"); 2860 } 2861 else 2862 return super.addChild(name); 2863 } 2864 2865 public CapabilityStatementRestSecurityComponent copy() { 2866 CapabilityStatementRestSecurityComponent dst = new CapabilityStatementRestSecurityComponent(); 2867 copyValues(dst); 2868 return dst; 2869 } 2870 2871 public void copyValues(CapabilityStatementRestSecurityComponent dst) { 2872 super.copyValues(dst); 2873 dst.cors = cors == null ? null : cors.copy(); 2874 if (service != null) { 2875 dst.service = new ArrayList<CodeableConcept>(); 2876 for (CodeableConcept i : service) 2877 dst.service.add(i.copy()); 2878 }; 2879 dst.description = description == null ? null : description.copy(); 2880 } 2881 2882 @Override 2883 public boolean equalsDeep(Base other_) { 2884 if (!super.equalsDeep(other_)) 2885 return false; 2886 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 2887 return false; 2888 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 2889 return compareDeep(cors, o.cors, true) && compareDeep(service, o.service, true) && compareDeep(description, o.description, true) 2890 ; 2891 } 2892 2893 @Override 2894 public boolean equalsShallow(Base other_) { 2895 if (!super.equalsShallow(other_)) 2896 return false; 2897 if (!(other_ instanceof CapabilityStatementRestSecurityComponent)) 2898 return false; 2899 CapabilityStatementRestSecurityComponent o = (CapabilityStatementRestSecurityComponent) other_; 2900 return compareValues(cors, o.cors, true) && compareValues(description, o.description, true); 2901 } 2902 2903 public boolean isEmpty() { 2904 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cors, service, description 2905 ); 2906 } 2907 2908 public String fhirType() { 2909 return "CapabilityStatement.rest.security"; 2910 2911 } 2912 2913 } 2914 2915 @Block() 2916 public static class CapabilityStatementRestResourceComponent extends BackboneElement implements IBaseBackboneElement { 2917 /** 2918 * A type of resource exposed via the restful interface. 2919 */ 2920 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2921 @Description(shortDefinition="A resource type that is supported", formalDefinition="A type of resource exposed via the restful interface." ) 2922 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 2923 protected CodeType type; 2924 2925 /** 2926 * A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the "superset" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses). 2927 */ 2928 @Child(name = "profile", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2929 @Description(shortDefinition="System-wide profile", formalDefinition="A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the \"superset\" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses)." ) 2930 protected CanonicalType profile; 2931 2932 /** 2933 * A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses). 2934 */ 2935 @Child(name = "supportedProfile", type = {CanonicalType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2936 @Description(shortDefinition="Use-case specific profiles", formalDefinition="A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses)." ) 2937 protected List<CanonicalType> supportedProfile; 2938 2939 /** 2940 * Additional information about the resource type used by the system. 2941 */ 2942 @Child(name = "documentation", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2943 @Description(shortDefinition="Additional information about the use of the resource type", formalDefinition="Additional information about the resource type used by the system." ) 2944 protected MarkdownType documentation; 2945 2946 /** 2947 * Identifies a restful operation supported by the solution. 2948 */ 2949 @Child(name = "interaction", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2950 @Description(shortDefinition="What operations are supported?", formalDefinition="Identifies a restful operation supported by the solution." ) 2951 protected List<ResourceInteractionComponent> interaction; 2952 2953 /** 2954 * This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API. 2955 */ 2956 @Child(name = "versioning", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2957 @Description(shortDefinition="no-version | versioned | versioned-update", formalDefinition="This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API." ) 2958 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/versioning-policy") 2959 protected Enumeration<ResourceVersionPolicy> versioning; 2960 2961 /** 2962 * A flag for whether the server is able to return past versions as part of the vRead operation. 2963 */ 2964 @Child(name = "readHistory", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=false) 2965 @Description(shortDefinition="Whether vRead can return past versions", formalDefinition="A flag for whether the server is able to return past versions as part of the vRead operation." ) 2966 protected BooleanType readHistory; 2967 2968 /** 2969 * A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server. 2970 */ 2971 @Child(name = "updateCreate", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2972 @Description(shortDefinition="If update can commit to a new identity", formalDefinition="A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server." ) 2973 protected BooleanType updateCreate; 2974 2975 /** 2976 * A flag that indicates that the server supports conditional create. 2977 */ 2978 @Child(name = "conditionalCreate", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 2979 @Description(shortDefinition="If allows/uses conditional create", formalDefinition="A flag that indicates that the server supports conditional create." ) 2980 protected BooleanType conditionalCreate; 2981 2982 /** 2983 * A code that indicates how the server supports conditional read. 2984 */ 2985 @Child(name = "conditionalRead", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 2986 @Description(shortDefinition="not-supported | modified-since | not-match | full-support", formalDefinition="A code that indicates how the server supports conditional read." ) 2987 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conditional-read-status") 2988 protected Enumeration<ConditionalReadStatus> conditionalRead; 2989 2990 /** 2991 * A flag that indicates that the server supports conditional update. 2992 */ 2993 @Child(name = "conditionalUpdate", type = {BooleanType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2994 @Description(shortDefinition="If allows/uses conditional update", formalDefinition="A flag that indicates that the server supports conditional update." ) 2995 protected BooleanType conditionalUpdate; 2996 2997 /** 2998 * A flag that indicates that the server supports conditional patch. 2999 */ 3000 @Child(name = "conditionalPatch", type = {BooleanType.class}, order=12, min=0, max=1, modifier=false, summary=false) 3001 @Description(shortDefinition="If allows/uses conditional patch", formalDefinition="A flag that indicates that the server supports conditional patch." ) 3002 protected BooleanType conditionalPatch; 3003 3004 /** 3005 * A code that indicates how the server supports conditional delete. 3006 */ 3007 @Child(name = "conditionalDelete", type = {CodeType.class}, order=13, min=0, max=1, modifier=false, summary=false) 3008 @Description(shortDefinition="not-supported | single | multiple - how conditional delete is supported", formalDefinition="A code that indicates how the server supports conditional delete." ) 3009 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conditional-delete-status") 3010 protected Enumeration<ConditionalDeleteStatus> conditionalDelete; 3011 3012 /** 3013 * A set of flags that defines how references are supported. 3014 */ 3015 @Child(name = "referencePolicy", type = {CodeType.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3016 @Description(shortDefinition="literal | logical | resolves | enforced | local", formalDefinition="A set of flags that defines how references are supported." ) 3017 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reference-handling-policy") 3018 protected List<Enumeration<ReferenceHandlingPolicy>> referencePolicy; 3019 3020 /** 3021 * A list of _include values supported by the server. 3022 */ 3023 @Child(name = "searchInclude", type = {StringType.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3024 @Description(shortDefinition="_include values supported by the server", formalDefinition="A list of _include values supported by the server." ) 3025 protected List<StringType> searchInclude; 3026 3027 /** 3028 * A list of _revinclude (reverse include) values supported by the server. 3029 */ 3030 @Child(name = "searchRevInclude", type = {StringType.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3031 @Description(shortDefinition="_revinclude values supported by the server", formalDefinition="A list of _revinclude (reverse include) values supported by the server." ) 3032 protected List<StringType> searchRevInclude; 3033 3034 /** 3035 * Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation. 3036 */ 3037 @Child(name = "searchParam", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3038 @Description(shortDefinition="Search parameters supported by implementation", formalDefinition="Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation." ) 3039 protected List<CapabilityStatementRestResourceSearchParamComponent> searchParam; 3040 3041 /** 3042 * Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters. 3043 */ 3044 @Child(name = "operation", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3045 @Description(shortDefinition="Definition of a resource operation", formalDefinition="Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters." ) 3046 protected List<CapabilityStatementRestResourceOperationComponent> operation; 3047 3048 private static final long serialVersionUID = -1565226425L; 3049 3050 /** 3051 * Constructor 3052 */ 3053 public CapabilityStatementRestResourceComponent() { 3054 super(); 3055 } 3056 3057 /** 3058 * Constructor 3059 */ 3060 public CapabilityStatementRestResourceComponent(String type) { 3061 super(); 3062 this.setType(type); 3063 } 3064 3065 /** 3066 * @return {@link #type} (A type of resource exposed via the restful interface.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3067 */ 3068 public CodeType getTypeElement() { 3069 if (this.type == null) 3070 if (Configuration.errorOnAutoCreate()) 3071 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.type"); 3072 else if (Configuration.doAutoCreate()) 3073 this.type = new CodeType(); // bb 3074 return this.type; 3075 } 3076 3077 public boolean hasTypeElement() { 3078 return this.type != null && !this.type.isEmpty(); 3079 } 3080 3081 public boolean hasType() { 3082 return this.type != null && !this.type.isEmpty(); 3083 } 3084 3085 /** 3086 * @param value {@link #type} (A type of resource exposed via the restful interface.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3087 */ 3088 public CapabilityStatementRestResourceComponent setTypeElement(CodeType value) { 3089 this.type = value; 3090 return this; 3091 } 3092 3093 /** 3094 * @return A type of resource exposed via the restful interface. 3095 */ 3096 public String getType() { 3097 return this.type == null ? null : this.type.getValue(); 3098 } 3099 3100 /** 3101 * @param value A type of resource exposed via the restful interface. 3102 */ 3103 public CapabilityStatementRestResourceComponent setType(String value) { 3104 if (this.type == null) 3105 this.type = new CodeType(); 3106 this.type.setValue(value); 3107 return this; 3108 } 3109 3110 /** 3111 * @return {@link #profile} (A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the "superset" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses).). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 3112 */ 3113 public CanonicalType getProfileElement() { 3114 if (this.profile == null) 3115 if (Configuration.errorOnAutoCreate()) 3116 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.profile"); 3117 else if (Configuration.doAutoCreate()) 3118 this.profile = new CanonicalType(); // bb 3119 return this.profile; 3120 } 3121 3122 public boolean hasProfileElement() { 3123 return this.profile != null && !this.profile.isEmpty(); 3124 } 3125 3126 public boolean hasProfile() { 3127 return this.profile != null && !this.profile.isEmpty(); 3128 } 3129 3130 /** 3131 * @param value {@link #profile} (A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the "superset" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses).). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 3132 */ 3133 public CapabilityStatementRestResourceComponent setProfileElement(CanonicalType value) { 3134 this.profile = value; 3135 return this; 3136 } 3137 3138 /** 3139 * @return A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the "superset" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses). 3140 */ 3141 public String getProfile() { 3142 return this.profile == null ? null : this.profile.getValue(); 3143 } 3144 3145 /** 3146 * @param value A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the "superset" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses). 3147 */ 3148 public CapabilityStatementRestResourceComponent setProfile(String value) { 3149 if (Utilities.noString(value)) 3150 this.profile = null; 3151 else { 3152 if (this.profile == null) 3153 this.profile = new CanonicalType(); 3154 this.profile.setValue(value); 3155 } 3156 return this; 3157 } 3158 3159 /** 3160 * @return {@link #supportedProfile} (A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3161 */ 3162 public List<CanonicalType> getSupportedProfile() { 3163 if (this.supportedProfile == null) 3164 this.supportedProfile = new ArrayList<CanonicalType>(); 3165 return this.supportedProfile; 3166 } 3167 3168 /** 3169 * @return Returns a reference to <code>this</code> for easy method chaining 3170 */ 3171 public CapabilityStatementRestResourceComponent setSupportedProfile(List<CanonicalType> theSupportedProfile) { 3172 this.supportedProfile = theSupportedProfile; 3173 return this; 3174 } 3175 3176 public boolean hasSupportedProfile() { 3177 if (this.supportedProfile == null) 3178 return false; 3179 for (CanonicalType item : this.supportedProfile) 3180 if (!item.isEmpty()) 3181 return true; 3182 return false; 3183 } 3184 3185 /** 3186 * @return {@link #supportedProfile} (A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3187 */ 3188 public CanonicalType addSupportedProfileElement() {//2 3189 CanonicalType t = new CanonicalType(); 3190 if (this.supportedProfile == null) 3191 this.supportedProfile = new ArrayList<CanonicalType>(); 3192 this.supportedProfile.add(t); 3193 return t; 3194 } 3195 3196 /** 3197 * @param value {@link #supportedProfile} (A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3198 */ 3199 public CapabilityStatementRestResourceComponent addSupportedProfile(String value) { //1 3200 CanonicalType t = new CanonicalType(); 3201 t.setValue(value); 3202 if (this.supportedProfile == null) 3203 this.supportedProfile = new ArrayList<CanonicalType>(); 3204 this.supportedProfile.add(t); 3205 return this; 3206 } 3207 3208 /** 3209 * @param value {@link #supportedProfile} (A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses).) 3210 */ 3211 public boolean hasSupportedProfile(String value) { 3212 if (this.supportedProfile == null) 3213 return false; 3214 for (CanonicalType v : this.supportedProfile) 3215 if (v.getValue().equals(value)) // canonical 3216 return true; 3217 return false; 3218 } 3219 3220 /** 3221 * @return {@link #documentation} (Additional information about the resource type used by the system.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 3222 */ 3223 public MarkdownType getDocumentationElement() { 3224 if (this.documentation == null) 3225 if (Configuration.errorOnAutoCreate()) 3226 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.documentation"); 3227 else if (Configuration.doAutoCreate()) 3228 this.documentation = new MarkdownType(); // bb 3229 return this.documentation; 3230 } 3231 3232 public boolean hasDocumentationElement() { 3233 return this.documentation != null && !this.documentation.isEmpty(); 3234 } 3235 3236 public boolean hasDocumentation() { 3237 return this.documentation != null && !this.documentation.isEmpty(); 3238 } 3239 3240 /** 3241 * @param value {@link #documentation} (Additional information about the resource type used by the system.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 3242 */ 3243 public CapabilityStatementRestResourceComponent setDocumentationElement(MarkdownType value) { 3244 this.documentation = value; 3245 return this; 3246 } 3247 3248 /** 3249 * @return Additional information about the resource type used by the system. 3250 */ 3251 public String getDocumentation() { 3252 return this.documentation == null ? null : this.documentation.getValue(); 3253 } 3254 3255 /** 3256 * @param value Additional information about the resource type used by the system. 3257 */ 3258 public CapabilityStatementRestResourceComponent setDocumentation(String value) { 3259 if (Utilities.noString(value)) 3260 this.documentation = null; 3261 else { 3262 if (this.documentation == null) 3263 this.documentation = new MarkdownType(); 3264 this.documentation.setValue(value); 3265 } 3266 return this; 3267 } 3268 3269 /** 3270 * @return {@link #interaction} (Identifies a restful operation supported by the solution.) 3271 */ 3272 public List<ResourceInteractionComponent> getInteraction() { 3273 if (this.interaction == null) 3274 this.interaction = new ArrayList<ResourceInteractionComponent>(); 3275 return this.interaction; 3276 } 3277 3278 /** 3279 * @return Returns a reference to <code>this</code> for easy method chaining 3280 */ 3281 public CapabilityStatementRestResourceComponent setInteraction(List<ResourceInteractionComponent> theInteraction) { 3282 this.interaction = theInteraction; 3283 return this; 3284 } 3285 3286 public boolean hasInteraction() { 3287 if (this.interaction == null) 3288 return false; 3289 for (ResourceInteractionComponent item : this.interaction) 3290 if (!item.isEmpty()) 3291 return true; 3292 return false; 3293 } 3294 3295 public ResourceInteractionComponent addInteraction() { //3 3296 ResourceInteractionComponent t = new ResourceInteractionComponent(); 3297 if (this.interaction == null) 3298 this.interaction = new ArrayList<ResourceInteractionComponent>(); 3299 this.interaction.add(t); 3300 return t; 3301 } 3302 3303 public CapabilityStatementRestResourceComponent addInteraction(ResourceInteractionComponent t) { //3 3304 if (t == null) 3305 return this; 3306 if (this.interaction == null) 3307 this.interaction = new ArrayList<ResourceInteractionComponent>(); 3308 this.interaction.add(t); 3309 return this; 3310 } 3311 3312 /** 3313 * @return The first repetition of repeating field {@link #interaction}, creating it if it does not already exist {3} 3314 */ 3315 public ResourceInteractionComponent getInteractionFirstRep() { 3316 if (getInteraction().isEmpty()) { 3317 addInteraction(); 3318 } 3319 return getInteraction().get(0); 3320 } 3321 3322 /** 3323 * @return {@link #versioning} (This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 3324 */ 3325 public Enumeration<ResourceVersionPolicy> getVersioningElement() { 3326 if (this.versioning == null) 3327 if (Configuration.errorOnAutoCreate()) 3328 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.versioning"); 3329 else if (Configuration.doAutoCreate()) 3330 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); // bb 3331 return this.versioning; 3332 } 3333 3334 public boolean hasVersioningElement() { 3335 return this.versioning != null && !this.versioning.isEmpty(); 3336 } 3337 3338 public boolean hasVersioning() { 3339 return this.versioning != null && !this.versioning.isEmpty(); 3340 } 3341 3342 /** 3343 * @param value {@link #versioning} (This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 3344 */ 3345 public CapabilityStatementRestResourceComponent setVersioningElement(Enumeration<ResourceVersionPolicy> value) { 3346 this.versioning = value; 3347 return this; 3348 } 3349 3350 /** 3351 * @return This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API. 3352 */ 3353 public ResourceVersionPolicy getVersioning() { 3354 return this.versioning == null ? null : this.versioning.getValue(); 3355 } 3356 3357 /** 3358 * @param value This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API. 3359 */ 3360 public CapabilityStatementRestResourceComponent setVersioning(ResourceVersionPolicy value) { 3361 if (value == null) 3362 this.versioning = null; 3363 else { 3364 if (this.versioning == null) 3365 this.versioning = new Enumeration<ResourceVersionPolicy>(new ResourceVersionPolicyEnumFactory()); 3366 this.versioning.setValue(value); 3367 } 3368 return this; 3369 } 3370 3371 /** 3372 * @return {@link #readHistory} (A flag for whether the server is able to return past versions as part of the vRead operation.). This is the underlying object with id, value and extensions. The accessor "getReadHistory" gives direct access to the value 3373 */ 3374 public BooleanType getReadHistoryElement() { 3375 if (this.readHistory == null) 3376 if (Configuration.errorOnAutoCreate()) 3377 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.readHistory"); 3378 else if (Configuration.doAutoCreate()) 3379 this.readHistory = new BooleanType(); // bb 3380 return this.readHistory; 3381 } 3382 3383 public boolean hasReadHistoryElement() { 3384 return this.readHistory != null && !this.readHistory.isEmpty(); 3385 } 3386 3387 public boolean hasReadHistory() { 3388 return this.readHistory != null && !this.readHistory.isEmpty(); 3389 } 3390 3391 /** 3392 * @param value {@link #readHistory} (A flag for whether the server is able to return past versions as part of the vRead operation.). This is the underlying object with id, value and extensions. The accessor "getReadHistory" gives direct access to the value 3393 */ 3394 public CapabilityStatementRestResourceComponent setReadHistoryElement(BooleanType value) { 3395 this.readHistory = value; 3396 return this; 3397 } 3398 3399 /** 3400 * @return A flag for whether the server is able to return past versions as part of the vRead operation. 3401 */ 3402 public boolean getReadHistory() { 3403 return this.readHistory == null || this.readHistory.isEmpty() ? false : this.readHistory.getValue(); 3404 } 3405 3406 /** 3407 * @param value A flag for whether the server is able to return past versions as part of the vRead operation. 3408 */ 3409 public CapabilityStatementRestResourceComponent setReadHistory(boolean value) { 3410 if (this.readHistory == null) 3411 this.readHistory = new BooleanType(); 3412 this.readHistory.setValue(value); 3413 return this; 3414 } 3415 3416 /** 3417 * @return {@link #updateCreate} (A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.). This is the underlying object with id, value and extensions. The accessor "getUpdateCreate" gives direct access to the value 3418 */ 3419 public BooleanType getUpdateCreateElement() { 3420 if (this.updateCreate == null) 3421 if (Configuration.errorOnAutoCreate()) 3422 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.updateCreate"); 3423 else if (Configuration.doAutoCreate()) 3424 this.updateCreate = new BooleanType(); // bb 3425 return this.updateCreate; 3426 } 3427 3428 public boolean hasUpdateCreateElement() { 3429 return this.updateCreate != null && !this.updateCreate.isEmpty(); 3430 } 3431 3432 public boolean hasUpdateCreate() { 3433 return this.updateCreate != null && !this.updateCreate.isEmpty(); 3434 } 3435 3436 /** 3437 * @param value {@link #updateCreate} (A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.). This is the underlying object with id, value and extensions. The accessor "getUpdateCreate" gives direct access to the value 3438 */ 3439 public CapabilityStatementRestResourceComponent setUpdateCreateElement(BooleanType value) { 3440 this.updateCreate = value; 3441 return this; 3442 } 3443 3444 /** 3445 * @return A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server. 3446 */ 3447 public boolean getUpdateCreate() { 3448 return this.updateCreate == null || this.updateCreate.isEmpty() ? false : this.updateCreate.getValue(); 3449 } 3450 3451 /** 3452 * @param value A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server. 3453 */ 3454 public CapabilityStatementRestResourceComponent setUpdateCreate(boolean value) { 3455 if (this.updateCreate == null) 3456 this.updateCreate = new BooleanType(); 3457 this.updateCreate.setValue(value); 3458 return this; 3459 } 3460 3461 /** 3462 * @return {@link #conditionalCreate} (A flag that indicates that the server supports conditional create.). This is the underlying object with id, value and extensions. The accessor "getConditionalCreate" gives direct access to the value 3463 */ 3464 public BooleanType getConditionalCreateElement() { 3465 if (this.conditionalCreate == null) 3466 if (Configuration.errorOnAutoCreate()) 3467 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalCreate"); 3468 else if (Configuration.doAutoCreate()) 3469 this.conditionalCreate = new BooleanType(); // bb 3470 return this.conditionalCreate; 3471 } 3472 3473 public boolean hasConditionalCreateElement() { 3474 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 3475 } 3476 3477 public boolean hasConditionalCreate() { 3478 return this.conditionalCreate != null && !this.conditionalCreate.isEmpty(); 3479 } 3480 3481 /** 3482 * @param value {@link #conditionalCreate} (A flag that indicates that the server supports conditional create.). This is the underlying object with id, value and extensions. The accessor "getConditionalCreate" gives direct access to the value 3483 */ 3484 public CapabilityStatementRestResourceComponent setConditionalCreateElement(BooleanType value) { 3485 this.conditionalCreate = value; 3486 return this; 3487 } 3488 3489 /** 3490 * @return A flag that indicates that the server supports conditional create. 3491 */ 3492 public boolean getConditionalCreate() { 3493 return this.conditionalCreate == null || this.conditionalCreate.isEmpty() ? false : this.conditionalCreate.getValue(); 3494 } 3495 3496 /** 3497 * @param value A flag that indicates that the server supports conditional create. 3498 */ 3499 public CapabilityStatementRestResourceComponent setConditionalCreate(boolean value) { 3500 if (this.conditionalCreate == null) 3501 this.conditionalCreate = new BooleanType(); 3502 this.conditionalCreate.setValue(value); 3503 return this; 3504 } 3505 3506 /** 3507 * @return {@link #conditionalRead} (A code that indicates how the server supports conditional read.). This is the underlying object with id, value and extensions. The accessor "getConditionalRead" gives direct access to the value 3508 */ 3509 public Enumeration<ConditionalReadStatus> getConditionalReadElement() { 3510 if (this.conditionalRead == null) 3511 if (Configuration.errorOnAutoCreate()) 3512 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalRead"); 3513 else if (Configuration.doAutoCreate()) 3514 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); // bb 3515 return this.conditionalRead; 3516 } 3517 3518 public boolean hasConditionalReadElement() { 3519 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 3520 } 3521 3522 public boolean hasConditionalRead() { 3523 return this.conditionalRead != null && !this.conditionalRead.isEmpty(); 3524 } 3525 3526 /** 3527 * @param value {@link #conditionalRead} (A code that indicates how the server supports conditional read.). This is the underlying object with id, value and extensions. The accessor "getConditionalRead" gives direct access to the value 3528 */ 3529 public CapabilityStatementRestResourceComponent setConditionalReadElement(Enumeration<ConditionalReadStatus> value) { 3530 this.conditionalRead = value; 3531 return this; 3532 } 3533 3534 /** 3535 * @return A code that indicates how the server supports conditional read. 3536 */ 3537 public ConditionalReadStatus getConditionalRead() { 3538 return this.conditionalRead == null ? null : this.conditionalRead.getValue(); 3539 } 3540 3541 /** 3542 * @param value A code that indicates how the server supports conditional read. 3543 */ 3544 public CapabilityStatementRestResourceComponent setConditionalRead(ConditionalReadStatus value) { 3545 if (value == null) 3546 this.conditionalRead = null; 3547 else { 3548 if (this.conditionalRead == null) 3549 this.conditionalRead = new Enumeration<ConditionalReadStatus>(new ConditionalReadStatusEnumFactory()); 3550 this.conditionalRead.setValue(value); 3551 } 3552 return this; 3553 } 3554 3555 /** 3556 * @return {@link #conditionalUpdate} (A flag that indicates that the server supports conditional update.). This is the underlying object with id, value and extensions. The accessor "getConditionalUpdate" gives direct access to the value 3557 */ 3558 public BooleanType getConditionalUpdateElement() { 3559 if (this.conditionalUpdate == null) 3560 if (Configuration.errorOnAutoCreate()) 3561 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalUpdate"); 3562 else if (Configuration.doAutoCreate()) 3563 this.conditionalUpdate = new BooleanType(); // bb 3564 return this.conditionalUpdate; 3565 } 3566 3567 public boolean hasConditionalUpdateElement() { 3568 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 3569 } 3570 3571 public boolean hasConditionalUpdate() { 3572 return this.conditionalUpdate != null && !this.conditionalUpdate.isEmpty(); 3573 } 3574 3575 /** 3576 * @param value {@link #conditionalUpdate} (A flag that indicates that the server supports conditional update.). This is the underlying object with id, value and extensions. The accessor "getConditionalUpdate" gives direct access to the value 3577 */ 3578 public CapabilityStatementRestResourceComponent setConditionalUpdateElement(BooleanType value) { 3579 this.conditionalUpdate = value; 3580 return this; 3581 } 3582 3583 /** 3584 * @return A flag that indicates that the server supports conditional update. 3585 */ 3586 public boolean getConditionalUpdate() { 3587 return this.conditionalUpdate == null || this.conditionalUpdate.isEmpty() ? false : this.conditionalUpdate.getValue(); 3588 } 3589 3590 /** 3591 * @param value A flag that indicates that the server supports conditional update. 3592 */ 3593 public CapabilityStatementRestResourceComponent setConditionalUpdate(boolean value) { 3594 if (this.conditionalUpdate == null) 3595 this.conditionalUpdate = new BooleanType(); 3596 this.conditionalUpdate.setValue(value); 3597 return this; 3598 } 3599 3600 /** 3601 * @return {@link #conditionalPatch} (A flag that indicates that the server supports conditional patch.). This is the underlying object with id, value and extensions. The accessor "getConditionalPatch" gives direct access to the value 3602 */ 3603 public BooleanType getConditionalPatchElement() { 3604 if (this.conditionalPatch == null) 3605 if (Configuration.errorOnAutoCreate()) 3606 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalPatch"); 3607 else if (Configuration.doAutoCreate()) 3608 this.conditionalPatch = new BooleanType(); // bb 3609 return this.conditionalPatch; 3610 } 3611 3612 public boolean hasConditionalPatchElement() { 3613 return this.conditionalPatch != null && !this.conditionalPatch.isEmpty(); 3614 } 3615 3616 public boolean hasConditionalPatch() { 3617 return this.conditionalPatch != null && !this.conditionalPatch.isEmpty(); 3618 } 3619 3620 /** 3621 * @param value {@link #conditionalPatch} (A flag that indicates that the server supports conditional patch.). This is the underlying object with id, value and extensions. The accessor "getConditionalPatch" gives direct access to the value 3622 */ 3623 public CapabilityStatementRestResourceComponent setConditionalPatchElement(BooleanType value) { 3624 this.conditionalPatch = value; 3625 return this; 3626 } 3627 3628 /** 3629 * @return A flag that indicates that the server supports conditional patch. 3630 */ 3631 public boolean getConditionalPatch() { 3632 return this.conditionalPatch == null || this.conditionalPatch.isEmpty() ? false : this.conditionalPatch.getValue(); 3633 } 3634 3635 /** 3636 * @param value A flag that indicates that the server supports conditional patch. 3637 */ 3638 public CapabilityStatementRestResourceComponent setConditionalPatch(boolean value) { 3639 if (this.conditionalPatch == null) 3640 this.conditionalPatch = new BooleanType(); 3641 this.conditionalPatch.setValue(value); 3642 return this; 3643 } 3644 3645 /** 3646 * @return {@link #conditionalDelete} (A code that indicates how the server supports conditional delete.). This is the underlying object with id, value and extensions. The accessor "getConditionalDelete" gives direct access to the value 3647 */ 3648 public Enumeration<ConditionalDeleteStatus> getConditionalDeleteElement() { 3649 if (this.conditionalDelete == null) 3650 if (Configuration.errorOnAutoCreate()) 3651 throw new Error("Attempt to auto-create CapabilityStatementRestResourceComponent.conditionalDelete"); 3652 else if (Configuration.doAutoCreate()) 3653 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); // bb 3654 return this.conditionalDelete; 3655 } 3656 3657 public boolean hasConditionalDeleteElement() { 3658 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 3659 } 3660 3661 public boolean hasConditionalDelete() { 3662 return this.conditionalDelete != null && !this.conditionalDelete.isEmpty(); 3663 } 3664 3665 /** 3666 * @param value {@link #conditionalDelete} (A code that indicates how the server supports conditional delete.). This is the underlying object with id, value and extensions. The accessor "getConditionalDelete" gives direct access to the value 3667 */ 3668 public CapabilityStatementRestResourceComponent setConditionalDeleteElement(Enumeration<ConditionalDeleteStatus> value) { 3669 this.conditionalDelete = value; 3670 return this; 3671 } 3672 3673 /** 3674 * @return A code that indicates how the server supports conditional delete. 3675 */ 3676 public ConditionalDeleteStatus getConditionalDelete() { 3677 return this.conditionalDelete == null ? null : this.conditionalDelete.getValue(); 3678 } 3679 3680 /** 3681 * @param value A code that indicates how the server supports conditional delete. 3682 */ 3683 public CapabilityStatementRestResourceComponent setConditionalDelete(ConditionalDeleteStatus value) { 3684 if (value == null) 3685 this.conditionalDelete = null; 3686 else { 3687 if (this.conditionalDelete == null) 3688 this.conditionalDelete = new Enumeration<ConditionalDeleteStatus>(new ConditionalDeleteStatusEnumFactory()); 3689 this.conditionalDelete.setValue(value); 3690 } 3691 return this; 3692 } 3693 3694 /** 3695 * @return {@link #referencePolicy} (A set of flags that defines how references are supported.) 3696 */ 3697 public List<Enumeration<ReferenceHandlingPolicy>> getReferencePolicy() { 3698 if (this.referencePolicy == null) 3699 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 3700 return this.referencePolicy; 3701 } 3702 3703 /** 3704 * @return Returns a reference to <code>this</code> for easy method chaining 3705 */ 3706 public CapabilityStatementRestResourceComponent setReferencePolicy(List<Enumeration<ReferenceHandlingPolicy>> theReferencePolicy) { 3707 this.referencePolicy = theReferencePolicy; 3708 return this; 3709 } 3710 3711 public boolean hasReferencePolicy() { 3712 if (this.referencePolicy == null) 3713 return false; 3714 for (Enumeration<ReferenceHandlingPolicy> item : this.referencePolicy) 3715 if (!item.isEmpty()) 3716 return true; 3717 return false; 3718 } 3719 3720 /** 3721 * @return {@link #referencePolicy} (A set of flags that defines how references are supported.) 3722 */ 3723 public Enumeration<ReferenceHandlingPolicy> addReferencePolicyElement() {//2 3724 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>(new ReferenceHandlingPolicyEnumFactory()); 3725 if (this.referencePolicy == null) 3726 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 3727 this.referencePolicy.add(t); 3728 return t; 3729 } 3730 3731 /** 3732 * @param value {@link #referencePolicy} (A set of flags that defines how references are supported.) 3733 */ 3734 public CapabilityStatementRestResourceComponent addReferencePolicy(ReferenceHandlingPolicy value) { //1 3735 Enumeration<ReferenceHandlingPolicy> t = new Enumeration<ReferenceHandlingPolicy>(new ReferenceHandlingPolicyEnumFactory()); 3736 t.setValue(value); 3737 if (this.referencePolicy == null) 3738 this.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 3739 this.referencePolicy.add(t); 3740 return this; 3741 } 3742 3743 /** 3744 * @param value {@link #referencePolicy} (A set of flags that defines how references are supported.) 3745 */ 3746 public boolean hasReferencePolicy(ReferenceHandlingPolicy value) { 3747 if (this.referencePolicy == null) 3748 return false; 3749 for (Enumeration<ReferenceHandlingPolicy> v : this.referencePolicy) 3750 if (v.getValue().equals(value)) // code 3751 return true; 3752 return false; 3753 } 3754 3755 /** 3756 * @return {@link #searchInclude} (A list of _include values supported by the server.) 3757 */ 3758 public List<StringType> getSearchInclude() { 3759 if (this.searchInclude == null) 3760 this.searchInclude = new ArrayList<StringType>(); 3761 return this.searchInclude; 3762 } 3763 3764 /** 3765 * @return Returns a reference to <code>this</code> for easy method chaining 3766 */ 3767 public CapabilityStatementRestResourceComponent setSearchInclude(List<StringType> theSearchInclude) { 3768 this.searchInclude = theSearchInclude; 3769 return this; 3770 } 3771 3772 public boolean hasSearchInclude() { 3773 if (this.searchInclude == null) 3774 return false; 3775 for (StringType item : this.searchInclude) 3776 if (!item.isEmpty()) 3777 return true; 3778 return false; 3779 } 3780 3781 /** 3782 * @return {@link #searchInclude} (A list of _include values supported by the server.) 3783 */ 3784 public StringType addSearchIncludeElement() {//2 3785 StringType t = new StringType(); 3786 if (this.searchInclude == null) 3787 this.searchInclude = new ArrayList<StringType>(); 3788 this.searchInclude.add(t); 3789 return t; 3790 } 3791 3792 /** 3793 * @param value {@link #searchInclude} (A list of _include values supported by the server.) 3794 */ 3795 public CapabilityStatementRestResourceComponent addSearchInclude(String value) { //1 3796 StringType t = new StringType(); 3797 t.setValue(value); 3798 if (this.searchInclude == null) 3799 this.searchInclude = new ArrayList<StringType>(); 3800 this.searchInclude.add(t); 3801 return this; 3802 } 3803 3804 /** 3805 * @param value {@link #searchInclude} (A list of _include values supported by the server.) 3806 */ 3807 public boolean hasSearchInclude(String value) { 3808 if (this.searchInclude == null) 3809 return false; 3810 for (StringType v : this.searchInclude) 3811 if (v.getValue().equals(value)) // string 3812 return true; 3813 return false; 3814 } 3815 3816 /** 3817 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 3818 */ 3819 public List<StringType> getSearchRevInclude() { 3820 if (this.searchRevInclude == null) 3821 this.searchRevInclude = new ArrayList<StringType>(); 3822 return this.searchRevInclude; 3823 } 3824 3825 /** 3826 * @return Returns a reference to <code>this</code> for easy method chaining 3827 */ 3828 public CapabilityStatementRestResourceComponent setSearchRevInclude(List<StringType> theSearchRevInclude) { 3829 this.searchRevInclude = theSearchRevInclude; 3830 return this; 3831 } 3832 3833 public boolean hasSearchRevInclude() { 3834 if (this.searchRevInclude == null) 3835 return false; 3836 for (StringType item : this.searchRevInclude) 3837 if (!item.isEmpty()) 3838 return true; 3839 return false; 3840 } 3841 3842 /** 3843 * @return {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 3844 */ 3845 public StringType addSearchRevIncludeElement() {//2 3846 StringType t = new StringType(); 3847 if (this.searchRevInclude == null) 3848 this.searchRevInclude = new ArrayList<StringType>(); 3849 this.searchRevInclude.add(t); 3850 return t; 3851 } 3852 3853 /** 3854 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 3855 */ 3856 public CapabilityStatementRestResourceComponent addSearchRevInclude(String value) { //1 3857 StringType t = new StringType(); 3858 t.setValue(value); 3859 if (this.searchRevInclude == null) 3860 this.searchRevInclude = new ArrayList<StringType>(); 3861 this.searchRevInclude.add(t); 3862 return this; 3863 } 3864 3865 /** 3866 * @param value {@link #searchRevInclude} (A list of _revinclude (reverse include) values supported by the server.) 3867 */ 3868 public boolean hasSearchRevInclude(String value) { 3869 if (this.searchRevInclude == null) 3870 return false; 3871 for (StringType v : this.searchRevInclude) 3872 if (v.getValue().equals(value)) // string 3873 return true; 3874 return false; 3875 } 3876 3877 /** 3878 * @return {@link #searchParam} (Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.) 3879 */ 3880 public List<CapabilityStatementRestResourceSearchParamComponent> getSearchParam() { 3881 if (this.searchParam == null) 3882 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 3883 return this.searchParam; 3884 } 3885 3886 /** 3887 * @return Returns a reference to <code>this</code> for easy method chaining 3888 */ 3889 public CapabilityStatementRestResourceComponent setSearchParam(List<CapabilityStatementRestResourceSearchParamComponent> theSearchParam) { 3890 this.searchParam = theSearchParam; 3891 return this; 3892 } 3893 3894 public boolean hasSearchParam() { 3895 if (this.searchParam == null) 3896 return false; 3897 for (CapabilityStatementRestResourceSearchParamComponent item : this.searchParam) 3898 if (!item.isEmpty()) 3899 return true; 3900 return false; 3901 } 3902 3903 public CapabilityStatementRestResourceSearchParamComponent addSearchParam() { //3 3904 CapabilityStatementRestResourceSearchParamComponent t = new CapabilityStatementRestResourceSearchParamComponent(); 3905 if (this.searchParam == null) 3906 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 3907 this.searchParam.add(t); 3908 return t; 3909 } 3910 3911 public CapabilityStatementRestResourceComponent addSearchParam(CapabilityStatementRestResourceSearchParamComponent t) { //3 3912 if (t == null) 3913 return this; 3914 if (this.searchParam == null) 3915 this.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 3916 this.searchParam.add(t); 3917 return this; 3918 } 3919 3920 /** 3921 * @return The first repetition of repeating field {@link #searchParam}, creating it if it does not already exist {3} 3922 */ 3923 public CapabilityStatementRestResourceSearchParamComponent getSearchParamFirstRep() { 3924 if (getSearchParam().isEmpty()) { 3925 addSearchParam(); 3926 } 3927 return getSearchParam().get(0); 3928 } 3929 3930 /** 3931 * @return {@link #operation} (Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.) 3932 */ 3933 public List<CapabilityStatementRestResourceOperationComponent> getOperation() { 3934 if (this.operation == null) 3935 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 3936 return this.operation; 3937 } 3938 3939 /** 3940 * @return Returns a reference to <code>this</code> for easy method chaining 3941 */ 3942 public CapabilityStatementRestResourceComponent setOperation(List<CapabilityStatementRestResourceOperationComponent> theOperation) { 3943 this.operation = theOperation; 3944 return this; 3945 } 3946 3947 public boolean hasOperation() { 3948 if (this.operation == null) 3949 return false; 3950 for (CapabilityStatementRestResourceOperationComponent item : this.operation) 3951 if (!item.isEmpty()) 3952 return true; 3953 return false; 3954 } 3955 3956 public CapabilityStatementRestResourceOperationComponent addOperation() { //3 3957 CapabilityStatementRestResourceOperationComponent t = new CapabilityStatementRestResourceOperationComponent(); 3958 if (this.operation == null) 3959 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 3960 this.operation.add(t); 3961 return t; 3962 } 3963 3964 public CapabilityStatementRestResourceComponent addOperation(CapabilityStatementRestResourceOperationComponent t) { //3 3965 if (t == null) 3966 return this; 3967 if (this.operation == null) 3968 this.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 3969 this.operation.add(t); 3970 return this; 3971 } 3972 3973 /** 3974 * @return The first repetition of repeating field {@link #operation}, creating it if it does not already exist {3} 3975 */ 3976 public CapabilityStatementRestResourceOperationComponent getOperationFirstRep() { 3977 if (getOperation().isEmpty()) { 3978 addOperation(); 3979 } 3980 return getOperation().get(0); 3981 } 3982 3983 protected void listChildren(List<Property> children) { 3984 super.listChildren(children); 3985 children.add(new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, type)); 3986 children.add(new Property("profile", "canonical(StructureDefinition)", "A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the \"superset\" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, 1, profile)); 3987 children.add(new Property("supportedProfile", "canonical(StructureDefinition)", "A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, java.lang.Integer.MAX_VALUE, supportedProfile)); 3988 children.add(new Property("documentation", "markdown", "Additional information about the resource type used by the system.", 0, 1, documentation)); 3989 children.add(new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, java.lang.Integer.MAX_VALUE, interaction)); 3990 children.add(new Property("versioning", "code", "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 0, 1, versioning)); 3991 children.add(new Property("readHistory", "boolean", "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, readHistory)); 3992 children.add(new Property("updateCreate", "boolean", "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 0, 1, updateCreate)); 3993 children.add(new Property("conditionalCreate", "boolean", "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate)); 3994 children.add(new Property("conditionalRead", "code", "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead)); 3995 children.add(new Property("conditionalUpdate", "boolean", "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate)); 3996 children.add(new Property("conditionalPatch", "boolean", "A flag that indicates that the server supports conditional patch.", 0, 1, conditionalPatch)); 3997 children.add(new Property("conditionalDelete", "code", "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete)); 3998 children.add(new Property("referencePolicy", "code", "A set of flags that defines how references are supported.", 0, java.lang.Integer.MAX_VALUE, referencePolicy)); 3999 children.add(new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchInclude)); 4000 children.add(new Property("searchRevInclude", "string", "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchRevInclude)); 4001 children.add(new Property("searchParam", "", "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 0, java.lang.Integer.MAX_VALUE, searchParam)); 4002 children.add(new Property("operation", "", "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.", 0, java.lang.Integer.MAX_VALUE, operation)); 4003 } 4004 4005 @Override 4006 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4007 switch (_hash) { 4008 case 3575610: /*type*/ return new Property("type", "code", "A type of resource exposed via the restful interface.", 0, 1, type); 4009 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A system-wide profile that is applied across *all* instances of the resource supported by the system. For example, if declared on Observation, this profile is the \"superset\" of capabilities for laboratory *and* vitals *and* other domains. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, 1, profile); 4010 case 1225477403: /*supportedProfile*/ return new Property("supportedProfile", "canonical(StructureDefinition)", "A list of profiles representing different use cases the system hosts/produces. A supported profile is a statement about the functionality of the data and services provided by the server (or the client) for supported use cases. For example, a system can define and declare multiple Observation profiles for laboratory observations, vital sign observations, etc. By declaring supported profiles, systems provide a way to determine whether individual resources are conformant. See further discussion in [Using Profiles](profiling.html#profile-uses).", 0, java.lang.Integer.MAX_VALUE, supportedProfile); 4011 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Additional information about the resource type used by the system.", 0, 1, documentation); 4012 case 1844104722: /*interaction*/ return new Property("interaction", "", "Identifies a restful operation supported by the solution.", 0, java.lang.Integer.MAX_VALUE, interaction); 4013 case -670487542: /*versioning*/ return new Property("versioning", "code", "This field is set to no-version to specify that the system does not support (server) or use (client) versioning for this resource type. If this has some other value, the server must at least correctly track and populate the versionId meta-property on resources. If the value is 'versioned-update', then the server supports all the versioning features, including using e-tags for version integrity in the API.", 0, 1, versioning); 4014 case 187518494: /*readHistory*/ return new Property("readHistory", "boolean", "A flag for whether the server is able to return past versions as part of the vRead operation.", 0, 1, readHistory); 4015 case -1400550619: /*updateCreate*/ return new Property("updateCreate", "boolean", "A flag to indicate that the server allows or needs to allow the client to create new identities on the server (that is, the client PUTs to a location where there is no existing resource). Allowing this operation means that the server allows the client to create new identities on the server.", 0, 1, updateCreate); 4016 case 6401826: /*conditionalCreate*/ return new Property("conditionalCreate", "boolean", "A flag that indicates that the server supports conditional create.", 0, 1, conditionalCreate); 4017 case 822786364: /*conditionalRead*/ return new Property("conditionalRead", "code", "A code that indicates how the server supports conditional read.", 0, 1, conditionalRead); 4018 case 519849711: /*conditionalUpdate*/ return new Property("conditionalUpdate", "boolean", "A flag that indicates that the server supports conditional update.", 0, 1, conditionalUpdate); 4019 case -265374366: /*conditionalPatch*/ return new Property("conditionalPatch", "boolean", "A flag that indicates that the server supports conditional patch.", 0, 1, conditionalPatch); 4020 case 23237585: /*conditionalDelete*/ return new Property("conditionalDelete", "code", "A code that indicates how the server supports conditional delete.", 0, 1, conditionalDelete); 4021 case 796257373: /*referencePolicy*/ return new Property("referencePolicy", "code", "A set of flags that defines how references are supported.", 0, java.lang.Integer.MAX_VALUE, referencePolicy); 4022 case -1035904544: /*searchInclude*/ return new Property("searchInclude", "string", "A list of _include values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchInclude); 4023 case -2123884979: /*searchRevInclude*/ return new Property("searchRevInclude", "string", "A list of _revinclude (reverse include) values supported by the server.", 0, java.lang.Integer.MAX_VALUE, searchRevInclude); 4024 case -553645115: /*searchParam*/ return new Property("searchParam", "", "Search parameters for implementations to support and/or make use of - either references to ones defined in the specification, or additional ones defined for/by the implementation.", 0, java.lang.Integer.MAX_VALUE, searchParam); 4025 case 1662702951: /*operation*/ return new Property("operation", "", "Definition of an operation or a named query together with its parameters and their meaning and type. Consult the definition of the operation for details about how to invoke the operation, and the parameters.", 0, java.lang.Integer.MAX_VALUE, operation); 4026 default: return super.getNamedProperty(_hash, _name, _checkValid); 4027 } 4028 4029 } 4030 4031 @Override 4032 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4033 switch (hash) { 4034 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 4035 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 4036 case 1225477403: /*supportedProfile*/ return this.supportedProfile == null ? new Base[0] : this.supportedProfile.toArray(new Base[this.supportedProfile.size()]); // CanonicalType 4037 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 4038 case 1844104722: /*interaction*/ return this.interaction == null ? new Base[0] : this.interaction.toArray(new Base[this.interaction.size()]); // ResourceInteractionComponent 4039 case -670487542: /*versioning*/ return this.versioning == null ? new Base[0] : new Base[] {this.versioning}; // Enumeration<ResourceVersionPolicy> 4040 case 187518494: /*readHistory*/ return this.readHistory == null ? new Base[0] : new Base[] {this.readHistory}; // BooleanType 4041 case -1400550619: /*updateCreate*/ return this.updateCreate == null ? new Base[0] : new Base[] {this.updateCreate}; // BooleanType 4042 case 6401826: /*conditionalCreate*/ return this.conditionalCreate == null ? new Base[0] : new Base[] {this.conditionalCreate}; // BooleanType 4043 case 822786364: /*conditionalRead*/ return this.conditionalRead == null ? new Base[0] : new Base[] {this.conditionalRead}; // Enumeration<ConditionalReadStatus> 4044 case 519849711: /*conditionalUpdate*/ return this.conditionalUpdate == null ? new Base[0] : new Base[] {this.conditionalUpdate}; // BooleanType 4045 case -265374366: /*conditionalPatch*/ return this.conditionalPatch == null ? new Base[0] : new Base[] {this.conditionalPatch}; // BooleanType 4046 case 23237585: /*conditionalDelete*/ return this.conditionalDelete == null ? new Base[0] : new Base[] {this.conditionalDelete}; // Enumeration<ConditionalDeleteStatus> 4047 case 796257373: /*referencePolicy*/ return this.referencePolicy == null ? new Base[0] : this.referencePolicy.toArray(new Base[this.referencePolicy.size()]); // Enumeration<ReferenceHandlingPolicy> 4048 case -1035904544: /*searchInclude*/ return this.searchInclude == null ? new Base[0] : this.searchInclude.toArray(new Base[this.searchInclude.size()]); // StringType 4049 case -2123884979: /*searchRevInclude*/ return this.searchRevInclude == null ? new Base[0] : this.searchRevInclude.toArray(new Base[this.searchRevInclude.size()]); // StringType 4050 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : this.searchParam.toArray(new Base[this.searchParam.size()]); // CapabilityStatementRestResourceSearchParamComponent 4051 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : this.operation.toArray(new Base[this.operation.size()]); // CapabilityStatementRestResourceOperationComponent 4052 default: return super.getProperty(hash, name, checkValid); 4053 } 4054 4055 } 4056 4057 @Override 4058 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4059 switch (hash) { 4060 case 3575610: // type 4061 this.type = TypeConvertor.castToCode(value); // CodeType 4062 return value; 4063 case -309425751: // profile 4064 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 4065 return value; 4066 case 1225477403: // supportedProfile 4067 this.getSupportedProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4068 return value; 4069 case 1587405498: // documentation 4070 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 4071 return value; 4072 case 1844104722: // interaction 4073 this.getInteraction().add((ResourceInteractionComponent) value); // ResourceInteractionComponent 4074 return value; 4075 case -670487542: // versioning 4076 value = new ResourceVersionPolicyEnumFactory().fromType(TypeConvertor.castToCode(value)); 4077 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 4078 return value; 4079 case 187518494: // readHistory 4080 this.readHistory = TypeConvertor.castToBoolean(value); // BooleanType 4081 return value; 4082 case -1400550619: // updateCreate 4083 this.updateCreate = TypeConvertor.castToBoolean(value); // BooleanType 4084 return value; 4085 case 6401826: // conditionalCreate 4086 this.conditionalCreate = TypeConvertor.castToBoolean(value); // BooleanType 4087 return value; 4088 case 822786364: // conditionalRead 4089 value = new ConditionalReadStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4090 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 4091 return value; 4092 case 519849711: // conditionalUpdate 4093 this.conditionalUpdate = TypeConvertor.castToBoolean(value); // BooleanType 4094 return value; 4095 case -265374366: // conditionalPatch 4096 this.conditionalPatch = TypeConvertor.castToBoolean(value); // BooleanType 4097 return value; 4098 case 23237585: // conditionalDelete 4099 value = new ConditionalDeleteStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4100 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 4101 return value; 4102 case 796257373: // referencePolicy 4103 value = new ReferenceHandlingPolicyEnumFactory().fromType(TypeConvertor.castToCode(value)); 4104 this.getReferencePolicy().add((Enumeration) value); // Enumeration<ReferenceHandlingPolicy> 4105 return value; 4106 case -1035904544: // searchInclude 4107 this.getSearchInclude().add(TypeConvertor.castToString(value)); // StringType 4108 return value; 4109 case -2123884979: // searchRevInclude 4110 this.getSearchRevInclude().add(TypeConvertor.castToString(value)); // StringType 4111 return value; 4112 case -553645115: // searchParam 4113 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); // CapabilityStatementRestResourceSearchParamComponent 4114 return value; 4115 case 1662702951: // operation 4116 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); // CapabilityStatementRestResourceOperationComponent 4117 return value; 4118 default: return super.setProperty(hash, name, value); 4119 } 4120 4121 } 4122 4123 @Override 4124 public Base setProperty(String name, Base value) throws FHIRException { 4125 if (name.equals("type")) { 4126 this.type = TypeConvertor.castToCode(value); // CodeType 4127 } else if (name.equals("profile")) { 4128 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 4129 } else if (name.equals("supportedProfile")) { 4130 this.getSupportedProfile().add(TypeConvertor.castToCanonical(value)); 4131 } else if (name.equals("documentation")) { 4132 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 4133 } else if (name.equals("interaction")) { 4134 this.getInteraction().add((ResourceInteractionComponent) value); 4135 } else if (name.equals("versioning")) { 4136 value = new ResourceVersionPolicyEnumFactory().fromType(TypeConvertor.castToCode(value)); 4137 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 4138 } else if (name.equals("readHistory")) { 4139 this.readHistory = TypeConvertor.castToBoolean(value); // BooleanType 4140 } else if (name.equals("updateCreate")) { 4141 this.updateCreate = TypeConvertor.castToBoolean(value); // BooleanType 4142 } else if (name.equals("conditionalCreate")) { 4143 this.conditionalCreate = TypeConvertor.castToBoolean(value); // BooleanType 4144 } else if (name.equals("conditionalRead")) { 4145 value = new ConditionalReadStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4146 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 4147 } else if (name.equals("conditionalUpdate")) { 4148 this.conditionalUpdate = TypeConvertor.castToBoolean(value); // BooleanType 4149 } else if (name.equals("conditionalPatch")) { 4150 this.conditionalPatch = TypeConvertor.castToBoolean(value); // BooleanType 4151 } else if (name.equals("conditionalDelete")) { 4152 value = new ConditionalDeleteStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4153 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 4154 } else if (name.equals("referencePolicy")) { 4155 value = new ReferenceHandlingPolicyEnumFactory().fromType(TypeConvertor.castToCode(value)); 4156 this.getReferencePolicy().add((Enumeration) value); 4157 } else if (name.equals("searchInclude")) { 4158 this.getSearchInclude().add(TypeConvertor.castToString(value)); 4159 } else if (name.equals("searchRevInclude")) { 4160 this.getSearchRevInclude().add(TypeConvertor.castToString(value)); 4161 } else if (name.equals("searchParam")) { 4162 this.getSearchParam().add((CapabilityStatementRestResourceSearchParamComponent) value); 4163 } else if (name.equals("operation")) { 4164 this.getOperation().add((CapabilityStatementRestResourceOperationComponent) value); 4165 } else 4166 return super.setProperty(name, value); 4167 return value; 4168 } 4169 4170 @Override 4171 public void removeChild(String name, Base value) throws FHIRException { 4172 if (name.equals("type")) { 4173 this.type = null; 4174 } else if (name.equals("profile")) { 4175 this.profile = null; 4176 } else if (name.equals("supportedProfile")) { 4177 this.getSupportedProfile().remove(value); 4178 } else if (name.equals("documentation")) { 4179 this.documentation = null; 4180 } else if (name.equals("interaction")) { 4181 this.getInteraction().remove((ResourceInteractionComponent) value); 4182 } else if (name.equals("versioning")) { 4183 value = new ResourceVersionPolicyEnumFactory().fromType(TypeConvertor.castToCode(value)); 4184 this.versioning = (Enumeration) value; // Enumeration<ResourceVersionPolicy> 4185 } else if (name.equals("readHistory")) { 4186 this.readHistory = null; 4187 } else if (name.equals("updateCreate")) { 4188 this.updateCreate = null; 4189 } else if (name.equals("conditionalCreate")) { 4190 this.conditionalCreate = null; 4191 } else if (name.equals("conditionalRead")) { 4192 value = new ConditionalReadStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4193 this.conditionalRead = (Enumeration) value; // Enumeration<ConditionalReadStatus> 4194 } else if (name.equals("conditionalUpdate")) { 4195 this.conditionalUpdate = null; 4196 } else if (name.equals("conditionalPatch")) { 4197 this.conditionalPatch = null; 4198 } else if (name.equals("conditionalDelete")) { 4199 value = new ConditionalDeleteStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4200 this.conditionalDelete = (Enumeration) value; // Enumeration<ConditionalDeleteStatus> 4201 } else if (name.equals("referencePolicy")) { 4202 value = new ReferenceHandlingPolicyEnumFactory().fromType(TypeConvertor.castToCode(value)); 4203 this.getReferencePolicy().remove((Enumeration) value); 4204 } else if (name.equals("searchInclude")) { 4205 this.getSearchInclude().remove(value); 4206 } else if (name.equals("searchRevInclude")) { 4207 this.getSearchRevInclude().remove(value); 4208 } else if (name.equals("searchParam")) { 4209 this.getSearchParam().remove((CapabilityStatementRestResourceSearchParamComponent) value); 4210 } else if (name.equals("operation")) { 4211 this.getOperation().remove((CapabilityStatementRestResourceOperationComponent) value); 4212 } else 4213 super.removeChild(name, value); 4214 4215 } 4216 4217 @Override 4218 public Base makeProperty(int hash, String name) throws FHIRException { 4219 switch (hash) { 4220 case 3575610: return getTypeElement(); 4221 case -309425751: return getProfileElement(); 4222 case 1225477403: return addSupportedProfileElement(); 4223 case 1587405498: return getDocumentationElement(); 4224 case 1844104722: return addInteraction(); 4225 case -670487542: return getVersioningElement(); 4226 case 187518494: return getReadHistoryElement(); 4227 case -1400550619: return getUpdateCreateElement(); 4228 case 6401826: return getConditionalCreateElement(); 4229 case 822786364: return getConditionalReadElement(); 4230 case 519849711: return getConditionalUpdateElement(); 4231 case -265374366: return getConditionalPatchElement(); 4232 case 23237585: return getConditionalDeleteElement(); 4233 case 796257373: return addReferencePolicyElement(); 4234 case -1035904544: return addSearchIncludeElement(); 4235 case -2123884979: return addSearchRevIncludeElement(); 4236 case -553645115: return addSearchParam(); 4237 case 1662702951: return addOperation(); 4238 default: return super.makeProperty(hash, name); 4239 } 4240 4241 } 4242 4243 @Override 4244 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4245 switch (hash) { 4246 case 3575610: /*type*/ return new String[] {"code"}; 4247 case -309425751: /*profile*/ return new String[] {"canonical"}; 4248 case 1225477403: /*supportedProfile*/ return new String[] {"canonical"}; 4249 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 4250 case 1844104722: /*interaction*/ return new String[] {}; 4251 case -670487542: /*versioning*/ return new String[] {"code"}; 4252 case 187518494: /*readHistory*/ return new String[] {"boolean"}; 4253 case -1400550619: /*updateCreate*/ return new String[] {"boolean"}; 4254 case 6401826: /*conditionalCreate*/ return new String[] {"boolean"}; 4255 case 822786364: /*conditionalRead*/ return new String[] {"code"}; 4256 case 519849711: /*conditionalUpdate*/ return new String[] {"boolean"}; 4257 case -265374366: /*conditionalPatch*/ return new String[] {"boolean"}; 4258 case 23237585: /*conditionalDelete*/ return new String[] {"code"}; 4259 case 796257373: /*referencePolicy*/ return new String[] {"code"}; 4260 case -1035904544: /*searchInclude*/ return new String[] {"string"}; 4261 case -2123884979: /*searchRevInclude*/ return new String[] {"string"}; 4262 case -553645115: /*searchParam*/ return new String[] {}; 4263 case 1662702951: /*operation*/ return new String[] {}; 4264 default: return super.getTypesForProperty(hash, name); 4265 } 4266 4267 } 4268 4269 @Override 4270 public Base addChild(String name) throws FHIRException { 4271 if (name.equals("type")) { 4272 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.type"); 4273 } 4274 else if (name.equals("profile")) { 4275 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.profile"); 4276 } 4277 else if (name.equals("supportedProfile")) { 4278 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.supportedProfile"); 4279 } 4280 else if (name.equals("documentation")) { 4281 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.documentation"); 4282 } 4283 else if (name.equals("interaction")) { 4284 return addInteraction(); 4285 } 4286 else if (name.equals("versioning")) { 4287 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.versioning"); 4288 } 4289 else if (name.equals("readHistory")) { 4290 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.readHistory"); 4291 } 4292 else if (name.equals("updateCreate")) { 4293 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.updateCreate"); 4294 } 4295 else if (name.equals("conditionalCreate")) { 4296 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.conditionalCreate"); 4297 } 4298 else if (name.equals("conditionalRead")) { 4299 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.conditionalRead"); 4300 } 4301 else if (name.equals("conditionalUpdate")) { 4302 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.conditionalUpdate"); 4303 } 4304 else if (name.equals("conditionalPatch")) { 4305 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.conditionalPatch"); 4306 } 4307 else if (name.equals("conditionalDelete")) { 4308 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.conditionalDelete"); 4309 } 4310 else if (name.equals("referencePolicy")) { 4311 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.referencePolicy"); 4312 } 4313 else if (name.equals("searchInclude")) { 4314 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.searchInclude"); 4315 } 4316 else if (name.equals("searchRevInclude")) { 4317 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.searchRevInclude"); 4318 } 4319 else if (name.equals("searchParam")) { 4320 return addSearchParam(); 4321 } 4322 else if (name.equals("operation")) { 4323 return addOperation(); 4324 } 4325 else 4326 return super.addChild(name); 4327 } 4328 4329 public CapabilityStatementRestResourceComponent copy() { 4330 CapabilityStatementRestResourceComponent dst = new CapabilityStatementRestResourceComponent(); 4331 copyValues(dst); 4332 return dst; 4333 } 4334 4335 public void copyValues(CapabilityStatementRestResourceComponent dst) { 4336 super.copyValues(dst); 4337 dst.type = type == null ? null : type.copy(); 4338 dst.profile = profile == null ? null : profile.copy(); 4339 if (supportedProfile != null) { 4340 dst.supportedProfile = new ArrayList<CanonicalType>(); 4341 for (CanonicalType i : supportedProfile) 4342 dst.supportedProfile.add(i.copy()); 4343 }; 4344 dst.documentation = documentation == null ? null : documentation.copy(); 4345 if (interaction != null) { 4346 dst.interaction = new ArrayList<ResourceInteractionComponent>(); 4347 for (ResourceInteractionComponent i : interaction) 4348 dst.interaction.add(i.copy()); 4349 }; 4350 dst.versioning = versioning == null ? null : versioning.copy(); 4351 dst.readHistory = readHistory == null ? null : readHistory.copy(); 4352 dst.updateCreate = updateCreate == null ? null : updateCreate.copy(); 4353 dst.conditionalCreate = conditionalCreate == null ? null : conditionalCreate.copy(); 4354 dst.conditionalRead = conditionalRead == null ? null : conditionalRead.copy(); 4355 dst.conditionalUpdate = conditionalUpdate == null ? null : conditionalUpdate.copy(); 4356 dst.conditionalPatch = conditionalPatch == null ? null : conditionalPatch.copy(); 4357 dst.conditionalDelete = conditionalDelete == null ? null : conditionalDelete.copy(); 4358 if (referencePolicy != null) { 4359 dst.referencePolicy = new ArrayList<Enumeration<ReferenceHandlingPolicy>>(); 4360 for (Enumeration<ReferenceHandlingPolicy> i : referencePolicy) 4361 dst.referencePolicy.add(i.copy()); 4362 }; 4363 if (searchInclude != null) { 4364 dst.searchInclude = new ArrayList<StringType>(); 4365 for (StringType i : searchInclude) 4366 dst.searchInclude.add(i.copy()); 4367 }; 4368 if (searchRevInclude != null) { 4369 dst.searchRevInclude = new ArrayList<StringType>(); 4370 for (StringType i : searchRevInclude) 4371 dst.searchRevInclude.add(i.copy()); 4372 }; 4373 if (searchParam != null) { 4374 dst.searchParam = new ArrayList<CapabilityStatementRestResourceSearchParamComponent>(); 4375 for (CapabilityStatementRestResourceSearchParamComponent i : searchParam) 4376 dst.searchParam.add(i.copy()); 4377 }; 4378 if (operation != null) { 4379 dst.operation = new ArrayList<CapabilityStatementRestResourceOperationComponent>(); 4380 for (CapabilityStatementRestResourceOperationComponent i : operation) 4381 dst.operation.add(i.copy()); 4382 }; 4383 } 4384 4385 @Override 4386 public boolean equalsDeep(Base other_) { 4387 if (!super.equalsDeep(other_)) 4388 return false; 4389 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 4390 return false; 4391 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 4392 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) && compareDeep(supportedProfile, o.supportedProfile, true) 4393 && compareDeep(documentation, o.documentation, true) && compareDeep(interaction, o.interaction, true) 4394 && compareDeep(versioning, o.versioning, true) && compareDeep(readHistory, o.readHistory, true) 4395 && compareDeep(updateCreate, o.updateCreate, true) && compareDeep(conditionalCreate, o.conditionalCreate, true) 4396 && compareDeep(conditionalRead, o.conditionalRead, true) && compareDeep(conditionalUpdate, o.conditionalUpdate, true) 4397 && compareDeep(conditionalPatch, o.conditionalPatch, true) && compareDeep(conditionalDelete, o.conditionalDelete, true) 4398 && compareDeep(referencePolicy, o.referencePolicy, true) && compareDeep(searchInclude, o.searchInclude, true) 4399 && compareDeep(searchRevInclude, o.searchRevInclude, true) && compareDeep(searchParam, o.searchParam, true) 4400 && compareDeep(operation, o.operation, true); 4401 } 4402 4403 @Override 4404 public boolean equalsShallow(Base other_) { 4405 if (!super.equalsShallow(other_)) 4406 return false; 4407 if (!(other_ instanceof CapabilityStatementRestResourceComponent)) 4408 return false; 4409 CapabilityStatementRestResourceComponent o = (CapabilityStatementRestResourceComponent) other_; 4410 return compareValues(type, o.type, true) && compareValues(profile, o.profile, true) && compareValues(supportedProfile, o.supportedProfile, true) 4411 && compareValues(documentation, o.documentation, true) && compareValues(versioning, o.versioning, true) 4412 && compareValues(readHistory, o.readHistory, true) && compareValues(updateCreate, o.updateCreate, true) 4413 && compareValues(conditionalCreate, o.conditionalCreate, true) && compareValues(conditionalRead, o.conditionalRead, true) 4414 && compareValues(conditionalUpdate, o.conditionalUpdate, true) && compareValues(conditionalPatch, o.conditionalPatch, true) 4415 && compareValues(conditionalDelete, o.conditionalDelete, true) && compareValues(referencePolicy, o.referencePolicy, true) 4416 && compareValues(searchInclude, o.searchInclude, true) && compareValues(searchRevInclude, o.searchRevInclude, true) 4417 ; 4418 } 4419 4420 public boolean isEmpty() { 4421 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, supportedProfile 4422 , documentation, interaction, versioning, readHistory, updateCreate, conditionalCreate 4423 , conditionalRead, conditionalUpdate, conditionalPatch, conditionalDelete, referencePolicy 4424 , searchInclude, searchRevInclude, searchParam, operation); 4425 } 4426 4427 public String fhirType() { 4428 return "CapabilityStatement.rest.resource"; 4429 4430 } 4431 4432 } 4433 4434 @Block() 4435 public static class ResourceInteractionComponent extends BackboneElement implements IBaseBackboneElement { 4436 /** 4437 * Coded identifier of the operation, supported by the system resource. 4438 */ 4439 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4440 @Description(shortDefinition="read | vread | update | patch | delete | history-instance | history-type | create | search-type", formalDefinition="Coded identifier of the operation, supported by the system resource." ) 4441 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/type-restful-interaction") 4442 protected Enumeration<TypeRestfulInteraction> code; 4443 4444 /** 4445 * Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'. 4446 */ 4447 @Child(name = "documentation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4448 @Description(shortDefinition="Anything special about operation behavior", formalDefinition="Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'." ) 4449 protected MarkdownType documentation; 4450 4451 private static final long serialVersionUID = 2128937796L; 4452 4453 /** 4454 * Constructor 4455 */ 4456 public ResourceInteractionComponent() { 4457 super(); 4458 } 4459 4460 /** 4461 * Constructor 4462 */ 4463 public ResourceInteractionComponent(TypeRestfulInteraction code) { 4464 super(); 4465 this.setCode(code); 4466 } 4467 4468 /** 4469 * @return {@link #code} (Coded identifier of the operation, supported by the system resource.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4470 */ 4471 public Enumeration<TypeRestfulInteraction> getCodeElement() { 4472 if (this.code == null) 4473 if (Configuration.errorOnAutoCreate()) 4474 throw new Error("Attempt to auto-create ResourceInteractionComponent.code"); 4475 else if (Configuration.doAutoCreate()) 4476 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); // bb 4477 return this.code; 4478 } 4479 4480 public boolean hasCodeElement() { 4481 return this.code != null && !this.code.isEmpty(); 4482 } 4483 4484 public boolean hasCode() { 4485 return this.code != null && !this.code.isEmpty(); 4486 } 4487 4488 /** 4489 * @param value {@link #code} (Coded identifier of the operation, supported by the system resource.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 4490 */ 4491 public ResourceInteractionComponent setCodeElement(Enumeration<TypeRestfulInteraction> value) { 4492 this.code = value; 4493 return this; 4494 } 4495 4496 /** 4497 * @return Coded identifier of the operation, supported by the system resource. 4498 */ 4499 public TypeRestfulInteraction getCode() { 4500 return this.code == null ? null : this.code.getValue(); 4501 } 4502 4503 /** 4504 * @param value Coded identifier of the operation, supported by the system resource. 4505 */ 4506 public ResourceInteractionComponent setCode(TypeRestfulInteraction value) { 4507 if (this.code == null) 4508 this.code = new Enumeration<TypeRestfulInteraction>(new TypeRestfulInteractionEnumFactory()); 4509 this.code.setValue(value); 4510 return this; 4511 } 4512 4513 /** 4514 * @return {@link #documentation} (Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 4515 */ 4516 public MarkdownType getDocumentationElement() { 4517 if (this.documentation == null) 4518 if (Configuration.errorOnAutoCreate()) 4519 throw new Error("Attempt to auto-create ResourceInteractionComponent.documentation"); 4520 else if (Configuration.doAutoCreate()) 4521 this.documentation = new MarkdownType(); // bb 4522 return this.documentation; 4523 } 4524 4525 public boolean hasDocumentationElement() { 4526 return this.documentation != null && !this.documentation.isEmpty(); 4527 } 4528 4529 public boolean hasDocumentation() { 4530 return this.documentation != null && !this.documentation.isEmpty(); 4531 } 4532 4533 /** 4534 * @param value {@link #documentation} (Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 4535 */ 4536 public ResourceInteractionComponent setDocumentationElement(MarkdownType value) { 4537 this.documentation = value; 4538 return this; 4539 } 4540 4541 /** 4542 * @return Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'. 4543 */ 4544 public String getDocumentation() { 4545 return this.documentation == null ? null : this.documentation.getValue(); 4546 } 4547 4548 /** 4549 * @param value Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'. 4550 */ 4551 public ResourceInteractionComponent setDocumentation(String value) { 4552 if (Utilities.noString(value)) 4553 this.documentation = null; 4554 else { 4555 if (this.documentation == null) 4556 this.documentation = new MarkdownType(); 4557 this.documentation.setValue(value); 4558 } 4559 return this; 4560 } 4561 4562 protected void listChildren(List<Property> children) { 4563 super.listChildren(children); 4564 children.add(new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 0, 1, code)); 4565 children.add(new Property("documentation", "markdown", "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 0, 1, documentation)); 4566 } 4567 4568 @Override 4569 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4570 switch (_hash) { 4571 case 3059181: /*code*/ return new Property("code", "code", "Coded identifier of the operation, supported by the system resource.", 0, 1, code); 4572 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Guidance specific to the implementation of this operation, such as 'delete is a logical delete' or 'updates are only allowed with version id' or 'creates permitted from pre-authorized certificates only'.", 0, 1, documentation); 4573 default: return super.getNamedProperty(_hash, _name, _checkValid); 4574 } 4575 4576 } 4577 4578 @Override 4579 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4580 switch (hash) { 4581 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<TypeRestfulInteraction> 4582 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 4583 default: return super.getProperty(hash, name, checkValid); 4584 } 4585 4586 } 4587 4588 @Override 4589 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4590 switch (hash) { 4591 case 3059181: // code 4592 value = new TypeRestfulInteractionEnumFactory().fromType(TypeConvertor.castToCode(value)); 4593 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 4594 return value; 4595 case 1587405498: // documentation 4596 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 4597 return value; 4598 default: return super.setProperty(hash, name, value); 4599 } 4600 4601 } 4602 4603 @Override 4604 public Base setProperty(String name, Base value) throws FHIRException { 4605 if (name.equals("code")) { 4606 value = new TypeRestfulInteractionEnumFactory().fromType(TypeConvertor.castToCode(value)); 4607 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 4608 } else if (name.equals("documentation")) { 4609 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 4610 } else 4611 return super.setProperty(name, value); 4612 return value; 4613 } 4614 4615 @Override 4616 public void removeChild(String name, Base value) throws FHIRException { 4617 if (name.equals("code")) { 4618 value = new TypeRestfulInteractionEnumFactory().fromType(TypeConvertor.castToCode(value)); 4619 this.code = (Enumeration) value; // Enumeration<TypeRestfulInteraction> 4620 } else if (name.equals("documentation")) { 4621 this.documentation = null; 4622 } else 4623 super.removeChild(name, value); 4624 4625 } 4626 4627 @Override 4628 public Base makeProperty(int hash, String name) throws FHIRException { 4629 switch (hash) { 4630 case 3059181: return getCodeElement(); 4631 case 1587405498: return getDocumentationElement(); 4632 default: return super.makeProperty(hash, name); 4633 } 4634 4635 } 4636 4637 @Override 4638 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4639 switch (hash) { 4640 case 3059181: /*code*/ return new String[] {"code"}; 4641 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 4642 default: return super.getTypesForProperty(hash, name); 4643 } 4644 4645 } 4646 4647 @Override 4648 public Base addChild(String name) throws FHIRException { 4649 if (name.equals("code")) { 4650 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.interaction.code"); 4651 } 4652 else if (name.equals("documentation")) { 4653 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.interaction.documentation"); 4654 } 4655 else 4656 return super.addChild(name); 4657 } 4658 4659 public ResourceInteractionComponent copy() { 4660 ResourceInteractionComponent dst = new ResourceInteractionComponent(); 4661 copyValues(dst); 4662 return dst; 4663 } 4664 4665 public void copyValues(ResourceInteractionComponent dst) { 4666 super.copyValues(dst); 4667 dst.code = code == null ? null : code.copy(); 4668 dst.documentation = documentation == null ? null : documentation.copy(); 4669 } 4670 4671 @Override 4672 public boolean equalsDeep(Base other_) { 4673 if (!super.equalsDeep(other_)) 4674 return false; 4675 if (!(other_ instanceof ResourceInteractionComponent)) 4676 return false; 4677 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 4678 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 4679 } 4680 4681 @Override 4682 public boolean equalsShallow(Base other_) { 4683 if (!super.equalsShallow(other_)) 4684 return false; 4685 if (!(other_ instanceof ResourceInteractionComponent)) 4686 return false; 4687 ResourceInteractionComponent o = (ResourceInteractionComponent) other_; 4688 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 4689 } 4690 4691 public boolean isEmpty() { 4692 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 4693 } 4694 4695 public String fhirType() { 4696 return "CapabilityStatement.rest.resource.interaction"; 4697 4698 } 4699 4700 } 4701 4702 @Block() 4703 public static class CapabilityStatementRestResourceSearchParamComponent extends BackboneElement implements IBaseBackboneElement { 4704 /** 4705 * The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code. 4706 */ 4707 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4708 @Description(shortDefinition="Name for parameter in search url", formalDefinition="The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code." ) 4709 protected StringType name; 4710 4711 /** 4712 * An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs. 4713 */ 4714 @Child(name = "definition", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4715 @Description(shortDefinition="Source of definition for parameter", formalDefinition="An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs." ) 4716 protected CanonicalType definition; 4717 4718 /** 4719 * The type of value a search parameter refers to, and how the content is interpreted. 4720 */ 4721 @Child(name = "type", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 4722 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri | special", formalDefinition="The type of value a search parameter refers to, and how the content is interpreted." ) 4723 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 4724 protected Enumeration<SearchParamType> type; 4725 4726 /** 4727 * This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms. 4728 */ 4729 @Child(name = "documentation", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4730 @Description(shortDefinition="Server-specific usage", formalDefinition="This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms." ) 4731 protected MarkdownType documentation; 4732 4733 private static final long serialVersionUID = -171123928L; 4734 4735 /** 4736 * Constructor 4737 */ 4738 public CapabilityStatementRestResourceSearchParamComponent() { 4739 super(); 4740 } 4741 4742 /** 4743 * Constructor 4744 */ 4745 public CapabilityStatementRestResourceSearchParamComponent(String name, SearchParamType type) { 4746 super(); 4747 this.setName(name); 4748 this.setType(type); 4749 } 4750 4751 /** 4752 * @return {@link #name} (The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4753 */ 4754 public StringType getNameElement() { 4755 if (this.name == null) 4756 if (Configuration.errorOnAutoCreate()) 4757 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.name"); 4758 else if (Configuration.doAutoCreate()) 4759 this.name = new StringType(); // bb 4760 return this.name; 4761 } 4762 4763 public boolean hasNameElement() { 4764 return this.name != null && !this.name.isEmpty(); 4765 } 4766 4767 public boolean hasName() { 4768 return this.name != null && !this.name.isEmpty(); 4769 } 4770 4771 /** 4772 * @param value {@link #name} (The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4773 */ 4774 public CapabilityStatementRestResourceSearchParamComponent setNameElement(StringType value) { 4775 this.name = value; 4776 return this; 4777 } 4778 4779 /** 4780 * @return The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code. 4781 */ 4782 public String getName() { 4783 return this.name == null ? null : this.name.getValue(); 4784 } 4785 4786 /** 4787 * @param value The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code. 4788 */ 4789 public CapabilityStatementRestResourceSearchParamComponent setName(String value) { 4790 if (this.name == null) 4791 this.name = new StringType(); 4792 this.name.setValue(value); 4793 return this; 4794 } 4795 4796 /** 4797 * @return {@link #definition} (An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 4798 */ 4799 public CanonicalType getDefinitionElement() { 4800 if (this.definition == null) 4801 if (Configuration.errorOnAutoCreate()) 4802 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.definition"); 4803 else if (Configuration.doAutoCreate()) 4804 this.definition = new CanonicalType(); // bb 4805 return this.definition; 4806 } 4807 4808 public boolean hasDefinitionElement() { 4809 return this.definition != null && !this.definition.isEmpty(); 4810 } 4811 4812 public boolean hasDefinition() { 4813 return this.definition != null && !this.definition.isEmpty(); 4814 } 4815 4816 /** 4817 * @param value {@link #definition} (An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 4818 */ 4819 public CapabilityStatementRestResourceSearchParamComponent setDefinitionElement(CanonicalType value) { 4820 this.definition = value; 4821 return this; 4822 } 4823 4824 /** 4825 * @return An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs. 4826 */ 4827 public String getDefinition() { 4828 return this.definition == null ? null : this.definition.getValue(); 4829 } 4830 4831 /** 4832 * @param value An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs. 4833 */ 4834 public CapabilityStatementRestResourceSearchParamComponent setDefinition(String value) { 4835 if (Utilities.noString(value)) 4836 this.definition = null; 4837 else { 4838 if (this.definition == null) 4839 this.definition = new CanonicalType(); 4840 this.definition.setValue(value); 4841 } 4842 return this; 4843 } 4844 4845 /** 4846 * @return {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4847 */ 4848 public Enumeration<SearchParamType> getTypeElement() { 4849 if (this.type == null) 4850 if (Configuration.errorOnAutoCreate()) 4851 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.type"); 4852 else if (Configuration.doAutoCreate()) 4853 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 4854 return this.type; 4855 } 4856 4857 public boolean hasTypeElement() { 4858 return this.type != null && !this.type.isEmpty(); 4859 } 4860 4861 public boolean hasType() { 4862 return this.type != null && !this.type.isEmpty(); 4863 } 4864 4865 /** 4866 * @param value {@link #type} (The type of value a search parameter refers to, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4867 */ 4868 public CapabilityStatementRestResourceSearchParamComponent setTypeElement(Enumeration<SearchParamType> value) { 4869 this.type = value; 4870 return this; 4871 } 4872 4873 /** 4874 * @return The type of value a search parameter refers to, and how the content is interpreted. 4875 */ 4876 public SearchParamType getType() { 4877 return this.type == null ? null : this.type.getValue(); 4878 } 4879 4880 /** 4881 * @param value The type of value a search parameter refers to, and how the content is interpreted. 4882 */ 4883 public CapabilityStatementRestResourceSearchParamComponent setType(SearchParamType value) { 4884 if (this.type == null) 4885 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 4886 this.type.setValue(value); 4887 return this; 4888 } 4889 4890 /** 4891 * @return {@link #documentation} (This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 4892 */ 4893 public MarkdownType getDocumentationElement() { 4894 if (this.documentation == null) 4895 if (Configuration.errorOnAutoCreate()) 4896 throw new Error("Attempt to auto-create CapabilityStatementRestResourceSearchParamComponent.documentation"); 4897 else if (Configuration.doAutoCreate()) 4898 this.documentation = new MarkdownType(); // bb 4899 return this.documentation; 4900 } 4901 4902 public boolean hasDocumentationElement() { 4903 return this.documentation != null && !this.documentation.isEmpty(); 4904 } 4905 4906 public boolean hasDocumentation() { 4907 return this.documentation != null && !this.documentation.isEmpty(); 4908 } 4909 4910 /** 4911 * @param value {@link #documentation} (This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 4912 */ 4913 public CapabilityStatementRestResourceSearchParamComponent setDocumentationElement(MarkdownType value) { 4914 this.documentation = value; 4915 return this; 4916 } 4917 4918 /** 4919 * @return This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms. 4920 */ 4921 public String getDocumentation() { 4922 return this.documentation == null ? null : this.documentation.getValue(); 4923 } 4924 4925 /** 4926 * @param value This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms. 4927 */ 4928 public CapabilityStatementRestResourceSearchParamComponent setDocumentation(String value) { 4929 if (Utilities.noString(value)) 4930 this.documentation = null; 4931 else { 4932 if (this.documentation == null) 4933 this.documentation = new MarkdownType(); 4934 this.documentation.setValue(value); 4935 } 4936 return this; 4937 } 4938 4939 protected void listChildren(List<Property> children) { 4940 super.listChildren(children); 4941 children.add(new Property("name", "string", "The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code.", 0, 1, name)); 4942 children.add(new Property("definition", "canonical(SearchParameter)", "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.", 0, 1, definition)); 4943 children.add(new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type)); 4944 children.add(new Property("documentation", "markdown", "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 0, 1, documentation)); 4945 } 4946 4947 @Override 4948 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4949 switch (_hash) { 4950 case 3373707: /*name*/ return new Property("name", "string", "The label used for the search parameter in this particular system's API - i.e. the 'name' portion of the name-value pair that will appear as part of the search URL. This SHOULD be the same as the SearchParameter.code of the defining SearchParameter. However, it can sometimes differ if necessary to disambiguate when a server supports multiple SearchParameters that happen to share the same code.", 0, 1, name); 4951 case -1014418093: /*definition*/ return new Property("definition", "canonical(SearchParameter)", "An absolute URI that is a formal reference to where this parameter was first defined, so that a client can be confident of the meaning of the search parameter (a reference to [SearchParameter.url](searchparameter-definitions.html#SearchParameter.url)). This element SHALL be populated if the search parameter refers to a SearchParameter defined by the FHIR core specification or externally defined IGs.", 0, 1, definition); 4952 case 3575610: /*type*/ return new Property("type", "code", "The type of value a search parameter refers to, and how the content is interpreted.", 0, 1, type); 4953 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "This allows documentation of any distinct behaviors about how the search parameter is used. For example, text matching algorithms.", 0, 1, documentation); 4954 default: return super.getNamedProperty(_hash, _name, _checkValid); 4955 } 4956 4957 } 4958 4959 @Override 4960 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4961 switch (hash) { 4962 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4963 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CanonicalType 4964 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SearchParamType> 4965 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 4966 default: return super.getProperty(hash, name, checkValid); 4967 } 4968 4969 } 4970 4971 @Override 4972 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4973 switch (hash) { 4974 case 3373707: // name 4975 this.name = TypeConvertor.castToString(value); // StringType 4976 return value; 4977 case -1014418093: // definition 4978 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 4979 return value; 4980 case 3575610: // type 4981 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4982 this.type = (Enumeration) value; // Enumeration<SearchParamType> 4983 return value; 4984 case 1587405498: // documentation 4985 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 4986 return value; 4987 default: return super.setProperty(hash, name, value); 4988 } 4989 4990 } 4991 4992 @Override 4993 public Base setProperty(String name, Base value) throws FHIRException { 4994 if (name.equals("name")) { 4995 this.name = TypeConvertor.castToString(value); // StringType 4996 } else if (name.equals("definition")) { 4997 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 4998 } else if (name.equals("type")) { 4999 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5000 this.type = (Enumeration) value; // Enumeration<SearchParamType> 5001 } else if (name.equals("documentation")) { 5002 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5003 } else 5004 return super.setProperty(name, value); 5005 return value; 5006 } 5007 5008 @Override 5009 public void removeChild(String name, Base value) throws FHIRException { 5010 if (name.equals("name")) { 5011 this.name = null; 5012 } else if (name.equals("definition")) { 5013 this.definition = null; 5014 } else if (name.equals("type")) { 5015 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5016 this.type = (Enumeration) value; // Enumeration<SearchParamType> 5017 } else if (name.equals("documentation")) { 5018 this.documentation = null; 5019 } else 5020 super.removeChild(name, value); 5021 5022 } 5023 5024 @Override 5025 public Base makeProperty(int hash, String name) throws FHIRException { 5026 switch (hash) { 5027 case 3373707: return getNameElement(); 5028 case -1014418093: return getDefinitionElement(); 5029 case 3575610: return getTypeElement(); 5030 case 1587405498: return getDocumentationElement(); 5031 default: return super.makeProperty(hash, name); 5032 } 5033 5034 } 5035 5036 @Override 5037 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5038 switch (hash) { 5039 case 3373707: /*name*/ return new String[] {"string"}; 5040 case -1014418093: /*definition*/ return new String[] {"canonical"}; 5041 case 3575610: /*type*/ return new String[] {"code"}; 5042 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 5043 default: return super.getTypesForProperty(hash, name); 5044 } 5045 5046 } 5047 5048 @Override 5049 public Base addChild(String name) throws FHIRException { 5050 if (name.equals("name")) { 5051 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.searchParam.name"); 5052 } 5053 else if (name.equals("definition")) { 5054 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.searchParam.definition"); 5055 } 5056 else if (name.equals("type")) { 5057 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.searchParam.type"); 5058 } 5059 else if (name.equals("documentation")) { 5060 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.searchParam.documentation"); 5061 } 5062 else 5063 return super.addChild(name); 5064 } 5065 5066 public CapabilityStatementRestResourceSearchParamComponent copy() { 5067 CapabilityStatementRestResourceSearchParamComponent dst = new CapabilityStatementRestResourceSearchParamComponent(); 5068 copyValues(dst); 5069 return dst; 5070 } 5071 5072 public void copyValues(CapabilityStatementRestResourceSearchParamComponent dst) { 5073 super.copyValues(dst); 5074 dst.name = name == null ? null : name.copy(); 5075 dst.definition = definition == null ? null : definition.copy(); 5076 dst.type = type == null ? null : type.copy(); 5077 dst.documentation = documentation == null ? null : documentation.copy(); 5078 } 5079 5080 @Override 5081 public boolean equalsDeep(Base other_) { 5082 if (!super.equalsDeep(other_)) 5083 return false; 5084 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 5085 return false; 5086 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 5087 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) && compareDeep(type, o.type, true) 5088 && compareDeep(documentation, o.documentation, true); 5089 } 5090 5091 @Override 5092 public boolean equalsShallow(Base other_) { 5093 if (!super.equalsShallow(other_)) 5094 return false; 5095 if (!(other_ instanceof CapabilityStatementRestResourceSearchParamComponent)) 5096 return false; 5097 CapabilityStatementRestResourceSearchParamComponent o = (CapabilityStatementRestResourceSearchParamComponent) other_; 5098 return compareValues(name, o.name, true) && compareValues(definition, o.definition, true) && compareValues(type, o.type, true) 5099 && compareValues(documentation, o.documentation, true); 5100 } 5101 5102 public boolean isEmpty() { 5103 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, type, documentation 5104 ); 5105 } 5106 5107 public String fhirType() { 5108 return "CapabilityStatement.rest.resource.searchParam"; 5109 5110 } 5111 5112 } 5113 5114 @Block() 5115 public static class CapabilityStatementRestResourceOperationComponent extends BackboneElement implements IBaseBackboneElement { 5116 /** 5117 * The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code. 5118 */ 5119 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5120 @Description(shortDefinition="Name by which the operation/query is invoked", formalDefinition="The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code." ) 5121 protected StringType name; 5122 5123 /** 5124 * Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported. 5125 */ 5126 @Child(name = "definition", type = {CanonicalType.class}, order=2, min=1, max=1, modifier=false, summary=true) 5127 @Description(shortDefinition="The defined operation/query", formalDefinition="Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported." ) 5128 protected CanonicalType definition; 5129 5130 /** 5131 * Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation. 5132 */ 5133 @Child(name = "documentation", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5134 @Description(shortDefinition="Specific details about operation behavior", formalDefinition="Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation." ) 5135 protected MarkdownType documentation; 5136 5137 private static final long serialVersionUID = -388608084L; 5138 5139 /** 5140 * Constructor 5141 */ 5142 public CapabilityStatementRestResourceOperationComponent() { 5143 super(); 5144 } 5145 5146 /** 5147 * Constructor 5148 */ 5149 public CapabilityStatementRestResourceOperationComponent(String name, String definition) { 5150 super(); 5151 this.setName(name); 5152 this.setDefinition(definition); 5153 } 5154 5155 /** 5156 * @return {@link #name} (The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5157 */ 5158 public StringType getNameElement() { 5159 if (this.name == null) 5160 if (Configuration.errorOnAutoCreate()) 5161 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.name"); 5162 else if (Configuration.doAutoCreate()) 5163 this.name = new StringType(); // bb 5164 return this.name; 5165 } 5166 5167 public boolean hasNameElement() { 5168 return this.name != null && !this.name.isEmpty(); 5169 } 5170 5171 public boolean hasName() { 5172 return this.name != null && !this.name.isEmpty(); 5173 } 5174 5175 /** 5176 * @param value {@link #name} (The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5177 */ 5178 public CapabilityStatementRestResourceOperationComponent setNameElement(StringType value) { 5179 this.name = value; 5180 return this; 5181 } 5182 5183 /** 5184 * @return The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code. 5185 */ 5186 public String getName() { 5187 return this.name == null ? null : this.name.getValue(); 5188 } 5189 5190 /** 5191 * @param value The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code. 5192 */ 5193 public CapabilityStatementRestResourceOperationComponent setName(String value) { 5194 if (this.name == null) 5195 this.name = new StringType(); 5196 this.name.setValue(value); 5197 return this; 5198 } 5199 5200 /** 5201 * @return {@link #definition} (Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 5202 */ 5203 public CanonicalType getDefinitionElement() { 5204 if (this.definition == null) 5205 if (Configuration.errorOnAutoCreate()) 5206 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.definition"); 5207 else if (Configuration.doAutoCreate()) 5208 this.definition = new CanonicalType(); // bb 5209 return this.definition; 5210 } 5211 5212 public boolean hasDefinitionElement() { 5213 return this.definition != null && !this.definition.isEmpty(); 5214 } 5215 5216 public boolean hasDefinition() { 5217 return this.definition != null && !this.definition.isEmpty(); 5218 } 5219 5220 /** 5221 * @param value {@link #definition} (Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 5222 */ 5223 public CapabilityStatementRestResourceOperationComponent setDefinitionElement(CanonicalType value) { 5224 this.definition = value; 5225 return this; 5226 } 5227 5228 /** 5229 * @return Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported. 5230 */ 5231 public String getDefinition() { 5232 return this.definition == null ? null : this.definition.getValue(); 5233 } 5234 5235 /** 5236 * @param value Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported. 5237 */ 5238 public CapabilityStatementRestResourceOperationComponent setDefinition(String value) { 5239 if (this.definition == null) 5240 this.definition = new CanonicalType(); 5241 this.definition.setValue(value); 5242 return this; 5243 } 5244 5245 /** 5246 * @return {@link #documentation} (Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5247 */ 5248 public MarkdownType getDocumentationElement() { 5249 if (this.documentation == null) 5250 if (Configuration.errorOnAutoCreate()) 5251 throw new Error("Attempt to auto-create CapabilityStatementRestResourceOperationComponent.documentation"); 5252 else if (Configuration.doAutoCreate()) 5253 this.documentation = new MarkdownType(); // bb 5254 return this.documentation; 5255 } 5256 5257 public boolean hasDocumentationElement() { 5258 return this.documentation != null && !this.documentation.isEmpty(); 5259 } 5260 5261 public boolean hasDocumentation() { 5262 return this.documentation != null && !this.documentation.isEmpty(); 5263 } 5264 5265 /** 5266 * @param value {@link #documentation} (Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5267 */ 5268 public CapabilityStatementRestResourceOperationComponent setDocumentationElement(MarkdownType value) { 5269 this.documentation = value; 5270 return this; 5271 } 5272 5273 /** 5274 * @return Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation. 5275 */ 5276 public String getDocumentation() { 5277 return this.documentation == null ? null : this.documentation.getValue(); 5278 } 5279 5280 /** 5281 * @param value Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation. 5282 */ 5283 public CapabilityStatementRestResourceOperationComponent setDocumentation(String value) { 5284 if (Utilities.noString(value)) 5285 this.documentation = null; 5286 else { 5287 if (this.documentation == null) 5288 this.documentation = new MarkdownType(); 5289 this.documentation.setValue(value); 5290 } 5291 return this; 5292 } 5293 5294 protected void listChildren(List<Property> children) { 5295 super.listChildren(children); 5296 children.add(new Property("name", "string", "The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code.", 0, 1, name)); 5297 children.add(new Property("definition", "canonical(OperationDefinition)", "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.", 0, 1, definition)); 5298 children.add(new Property("documentation", "markdown", "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.", 0, 1, documentation)); 5299 } 5300 5301 @Override 5302 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5303 switch (_hash) { 5304 case 3373707: /*name*/ return new Property("name", "string", "The name of the operation or query. For an operation, this name is prefixed with $ and used in the URL. For a query, this is the name used in the _query parameter when the query is called. This SHOULD be the same as the OperationDefinition.code of the defining OperationDefinition. However, it can sometimes differ if necessary to disambiguate when a server supports multiple OperationDefinition that happen to share the same code.", 0, 1, name); 5305 case -1014418093: /*definition*/ return new Property("definition", "canonical(OperationDefinition)", "Where the formal definition can be found. If a server references the base definition of an Operation (i.e. from the specification itself such as ```http://hl7.org/fhir/OperationDefinition/ValueSet-expand```), that means it supports the full capabilities of the operation - e.g. both GET and POST invocation. If it only supports a subset, it must define its own custom [OperationDefinition](operationdefinition.html#) with a 'base' of the original OperationDefinition. The custom definition would describe the specific subset of functionality supported.", 0, 1, definition); 5306 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Documentation that describes anything special about the operation behavior, possibly detailing different behavior for system, type and instance-level invocation of the operation.", 0, 1, documentation); 5307 default: return super.getNamedProperty(_hash, _name, _checkValid); 5308 } 5309 5310 } 5311 5312 @Override 5313 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5314 switch (hash) { 5315 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5316 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CanonicalType 5317 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 5318 default: return super.getProperty(hash, name, checkValid); 5319 } 5320 5321 } 5322 5323 @Override 5324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5325 switch (hash) { 5326 case 3373707: // name 5327 this.name = TypeConvertor.castToString(value); // StringType 5328 return value; 5329 case -1014418093: // definition 5330 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 5331 return value; 5332 case 1587405498: // documentation 5333 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5334 return value; 5335 default: return super.setProperty(hash, name, value); 5336 } 5337 5338 } 5339 5340 @Override 5341 public Base setProperty(String name, Base value) throws FHIRException { 5342 if (name.equals("name")) { 5343 this.name = TypeConvertor.castToString(value); // StringType 5344 } else if (name.equals("definition")) { 5345 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 5346 } else if (name.equals("documentation")) { 5347 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5348 } else 5349 return super.setProperty(name, value); 5350 return value; 5351 } 5352 5353 @Override 5354 public void removeChild(String name, Base value) throws FHIRException { 5355 if (name.equals("name")) { 5356 this.name = null; 5357 } else if (name.equals("definition")) { 5358 this.definition = null; 5359 } else if (name.equals("documentation")) { 5360 this.documentation = null; 5361 } else 5362 super.removeChild(name, value); 5363 5364 } 5365 5366 @Override 5367 public Base makeProperty(int hash, String name) throws FHIRException { 5368 switch (hash) { 5369 case 3373707: return getNameElement(); 5370 case -1014418093: return getDefinitionElement(); 5371 case 1587405498: return getDocumentationElement(); 5372 default: return super.makeProperty(hash, name); 5373 } 5374 5375 } 5376 5377 @Override 5378 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5379 switch (hash) { 5380 case 3373707: /*name*/ return new String[] {"string"}; 5381 case -1014418093: /*definition*/ return new String[] {"canonical"}; 5382 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 5383 default: return super.getTypesForProperty(hash, name); 5384 } 5385 5386 } 5387 5388 @Override 5389 public Base addChild(String name) throws FHIRException { 5390 if (name.equals("name")) { 5391 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.operation.name"); 5392 } 5393 else if (name.equals("definition")) { 5394 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.operation.definition"); 5395 } 5396 else if (name.equals("documentation")) { 5397 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.resource.operation.documentation"); 5398 } 5399 else 5400 return super.addChild(name); 5401 } 5402 5403 public CapabilityStatementRestResourceOperationComponent copy() { 5404 CapabilityStatementRestResourceOperationComponent dst = new CapabilityStatementRestResourceOperationComponent(); 5405 copyValues(dst); 5406 return dst; 5407 } 5408 5409 public void copyValues(CapabilityStatementRestResourceOperationComponent dst) { 5410 super.copyValues(dst); 5411 dst.name = name == null ? null : name.copy(); 5412 dst.definition = definition == null ? null : definition.copy(); 5413 dst.documentation = documentation == null ? null : documentation.copy(); 5414 } 5415 5416 @Override 5417 public boolean equalsDeep(Base other_) { 5418 if (!super.equalsDeep(other_)) 5419 return false; 5420 if (!(other_ instanceof CapabilityStatementRestResourceOperationComponent)) 5421 return false; 5422 CapabilityStatementRestResourceOperationComponent o = (CapabilityStatementRestResourceOperationComponent) other_; 5423 return compareDeep(name, o.name, true) && compareDeep(definition, o.definition, true) && compareDeep(documentation, o.documentation, true) 5424 ; 5425 } 5426 5427 @Override 5428 public boolean equalsShallow(Base other_) { 5429 if (!super.equalsShallow(other_)) 5430 return false; 5431 if (!(other_ instanceof CapabilityStatementRestResourceOperationComponent)) 5432 return false; 5433 CapabilityStatementRestResourceOperationComponent o = (CapabilityStatementRestResourceOperationComponent) other_; 5434 return compareValues(name, o.name, true) && compareValues(definition, o.definition, true) && compareValues(documentation, o.documentation, true) 5435 ; 5436 } 5437 5438 public boolean isEmpty() { 5439 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, definition, documentation 5440 ); 5441 } 5442 5443 public String fhirType() { 5444 return "CapabilityStatement.rest.resource.operation"; 5445 5446 } 5447 5448 } 5449 5450 @Block() 5451 public static class SystemInteractionComponent extends BackboneElement implements IBaseBackboneElement { 5452 /** 5453 * A coded identifier of the operation, supported by the system. 5454 */ 5455 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5456 @Description(shortDefinition="transaction | batch | search-system | history-system", formalDefinition="A coded identifier of the operation, supported by the system." ) 5457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/system-restful-interaction") 5458 protected Enumeration<SystemRestfulInteraction> code; 5459 5460 /** 5461 * Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented. 5462 */ 5463 @Child(name = "documentation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5464 @Description(shortDefinition="Anything special about operation behavior", formalDefinition="Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented." ) 5465 protected MarkdownType documentation; 5466 5467 private static final long serialVersionUID = -1495143879L; 5468 5469 /** 5470 * Constructor 5471 */ 5472 public SystemInteractionComponent() { 5473 super(); 5474 } 5475 5476 /** 5477 * Constructor 5478 */ 5479 public SystemInteractionComponent(SystemRestfulInteraction code) { 5480 super(); 5481 this.setCode(code); 5482 } 5483 5484 /** 5485 * @return {@link #code} (A coded identifier of the operation, supported by the system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 5486 */ 5487 public Enumeration<SystemRestfulInteraction> getCodeElement() { 5488 if (this.code == null) 5489 if (Configuration.errorOnAutoCreate()) 5490 throw new Error("Attempt to auto-create SystemInteractionComponent.code"); 5491 else if (Configuration.doAutoCreate()) 5492 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); // bb 5493 return this.code; 5494 } 5495 5496 public boolean hasCodeElement() { 5497 return this.code != null && !this.code.isEmpty(); 5498 } 5499 5500 public boolean hasCode() { 5501 return this.code != null && !this.code.isEmpty(); 5502 } 5503 5504 /** 5505 * @param value {@link #code} (A coded identifier of the operation, supported by the system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 5506 */ 5507 public SystemInteractionComponent setCodeElement(Enumeration<SystemRestfulInteraction> value) { 5508 this.code = value; 5509 return this; 5510 } 5511 5512 /** 5513 * @return A coded identifier of the operation, supported by the system. 5514 */ 5515 public SystemRestfulInteraction getCode() { 5516 return this.code == null ? null : this.code.getValue(); 5517 } 5518 5519 /** 5520 * @param value A coded identifier of the operation, supported by the system. 5521 */ 5522 public SystemInteractionComponent setCode(SystemRestfulInteraction value) { 5523 if (this.code == null) 5524 this.code = new Enumeration<SystemRestfulInteraction>(new SystemRestfulInteractionEnumFactory()); 5525 this.code.setValue(value); 5526 return this; 5527 } 5528 5529 /** 5530 * @return {@link #documentation} (Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5531 */ 5532 public MarkdownType getDocumentationElement() { 5533 if (this.documentation == null) 5534 if (Configuration.errorOnAutoCreate()) 5535 throw new Error("Attempt to auto-create SystemInteractionComponent.documentation"); 5536 else if (Configuration.doAutoCreate()) 5537 this.documentation = new MarkdownType(); // bb 5538 return this.documentation; 5539 } 5540 5541 public boolean hasDocumentationElement() { 5542 return this.documentation != null && !this.documentation.isEmpty(); 5543 } 5544 5545 public boolean hasDocumentation() { 5546 return this.documentation != null && !this.documentation.isEmpty(); 5547 } 5548 5549 /** 5550 * @param value {@link #documentation} (Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5551 */ 5552 public SystemInteractionComponent setDocumentationElement(MarkdownType value) { 5553 this.documentation = value; 5554 return this; 5555 } 5556 5557 /** 5558 * @return Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented. 5559 */ 5560 public String getDocumentation() { 5561 return this.documentation == null ? null : this.documentation.getValue(); 5562 } 5563 5564 /** 5565 * @param value Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented. 5566 */ 5567 public SystemInteractionComponent setDocumentation(String value) { 5568 if (Utilities.noString(value)) 5569 this.documentation = null; 5570 else { 5571 if (this.documentation == null) 5572 this.documentation = new MarkdownType(); 5573 this.documentation.setValue(value); 5574 } 5575 return this; 5576 } 5577 5578 protected void listChildren(List<Property> children) { 5579 super.listChildren(children); 5580 children.add(new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 1, code)); 5581 children.add(new Property("documentation", "markdown", "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 0, 1, documentation)); 5582 } 5583 5584 @Override 5585 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5586 switch (_hash) { 5587 case 3059181: /*code*/ return new Property("code", "code", "A coded identifier of the operation, supported by the system.", 0, 1, code); 5588 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Guidance specific to the implementation of this operation, such as limitations on the kind of transactions allowed, or information about system wide search is implemented.", 0, 1, documentation); 5589 default: return super.getNamedProperty(_hash, _name, _checkValid); 5590 } 5591 5592 } 5593 5594 @Override 5595 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5596 switch (hash) { 5597 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<SystemRestfulInteraction> 5598 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 5599 default: return super.getProperty(hash, name, checkValid); 5600 } 5601 5602 } 5603 5604 @Override 5605 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5606 switch (hash) { 5607 case 3059181: // code 5608 value = new SystemRestfulInteractionEnumFactory().fromType(TypeConvertor.castToCode(value)); 5609 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 5610 return value; 5611 case 1587405498: // documentation 5612 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5613 return value; 5614 default: return super.setProperty(hash, name, value); 5615 } 5616 5617 } 5618 5619 @Override 5620 public Base setProperty(String name, Base value) throws FHIRException { 5621 if (name.equals("code")) { 5622 value = new SystemRestfulInteractionEnumFactory().fromType(TypeConvertor.castToCode(value)); 5623 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 5624 } else if (name.equals("documentation")) { 5625 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5626 } else 5627 return super.setProperty(name, value); 5628 return value; 5629 } 5630 5631 @Override 5632 public void removeChild(String name, Base value) throws FHIRException { 5633 if (name.equals("code")) { 5634 value = new SystemRestfulInteractionEnumFactory().fromType(TypeConvertor.castToCode(value)); 5635 this.code = (Enumeration) value; // Enumeration<SystemRestfulInteraction> 5636 } else if (name.equals("documentation")) { 5637 this.documentation = null; 5638 } else 5639 super.removeChild(name, value); 5640 5641 } 5642 5643 @Override 5644 public Base makeProperty(int hash, String name) throws FHIRException { 5645 switch (hash) { 5646 case 3059181: return getCodeElement(); 5647 case 1587405498: return getDocumentationElement(); 5648 default: return super.makeProperty(hash, name); 5649 } 5650 5651 } 5652 5653 @Override 5654 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5655 switch (hash) { 5656 case 3059181: /*code*/ return new String[] {"code"}; 5657 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 5658 default: return super.getTypesForProperty(hash, name); 5659 } 5660 5661 } 5662 5663 @Override 5664 public Base addChild(String name) throws FHIRException { 5665 if (name.equals("code")) { 5666 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.interaction.code"); 5667 } 5668 else if (name.equals("documentation")) { 5669 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.rest.interaction.documentation"); 5670 } 5671 else 5672 return super.addChild(name); 5673 } 5674 5675 public SystemInteractionComponent copy() { 5676 SystemInteractionComponent dst = new SystemInteractionComponent(); 5677 copyValues(dst); 5678 return dst; 5679 } 5680 5681 public void copyValues(SystemInteractionComponent dst) { 5682 super.copyValues(dst); 5683 dst.code = code == null ? null : code.copy(); 5684 dst.documentation = documentation == null ? null : documentation.copy(); 5685 } 5686 5687 @Override 5688 public boolean equalsDeep(Base other_) { 5689 if (!super.equalsDeep(other_)) 5690 return false; 5691 if (!(other_ instanceof SystemInteractionComponent)) 5692 return false; 5693 SystemInteractionComponent o = (SystemInteractionComponent) other_; 5694 return compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true); 5695 } 5696 5697 @Override 5698 public boolean equalsShallow(Base other_) { 5699 if (!super.equalsShallow(other_)) 5700 return false; 5701 if (!(other_ instanceof SystemInteractionComponent)) 5702 return false; 5703 SystemInteractionComponent o = (SystemInteractionComponent) other_; 5704 return compareValues(code, o.code, true) && compareValues(documentation, o.documentation, true); 5705 } 5706 5707 public boolean isEmpty() { 5708 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, documentation); 5709 } 5710 5711 public String fhirType() { 5712 return "CapabilityStatement.rest.interaction"; 5713 5714 } 5715 5716 } 5717 5718 @Block() 5719 public static class CapabilityStatementMessagingComponent extends BackboneElement implements IBaseBackboneElement { 5720 /** 5721 * An endpoint (network accessible address) to which messages and/or replies are to be sent. 5722 */ 5723 @Child(name = "endpoint", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5724 @Description(shortDefinition="Where messages should be sent", formalDefinition="An endpoint (network accessible address) to which messages and/or replies are to be sent." ) 5725 protected List<CapabilityStatementMessagingEndpointComponent> endpoint; 5726 5727 /** 5728 * Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender). 5729 */ 5730 @Child(name = "reliableCache", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5731 @Description(shortDefinition="Reliable Message Cache Length (min)", formalDefinition="Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender)." ) 5732 protected UnsignedIntType reliableCache; 5733 5734 /** 5735 * Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner. 5736 */ 5737 @Child(name = "documentation", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5738 @Description(shortDefinition="Messaging interface behavior details", formalDefinition="Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner." ) 5739 protected MarkdownType documentation; 5740 5741 /** 5742 * References to message definitions for messages this system can send or receive. 5743 */ 5744 @Child(name = "supportedMessage", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5745 @Description(shortDefinition="Messages supported by this system", formalDefinition="References to message definitions for messages this system can send or receive." ) 5746 protected List<CapabilityStatementMessagingSupportedMessageComponent> supportedMessage; 5747 5748 private static final long serialVersionUID = 300411231L; 5749 5750 /** 5751 * Constructor 5752 */ 5753 public CapabilityStatementMessagingComponent() { 5754 super(); 5755 } 5756 5757 /** 5758 * @return {@link #endpoint} (An endpoint (network accessible address) to which messages and/or replies are to be sent.) 5759 */ 5760 public List<CapabilityStatementMessagingEndpointComponent> getEndpoint() { 5761 if (this.endpoint == null) 5762 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 5763 return this.endpoint; 5764 } 5765 5766 /** 5767 * @return Returns a reference to <code>this</code> for easy method chaining 5768 */ 5769 public CapabilityStatementMessagingComponent setEndpoint(List<CapabilityStatementMessagingEndpointComponent> theEndpoint) { 5770 this.endpoint = theEndpoint; 5771 return this; 5772 } 5773 5774 public boolean hasEndpoint() { 5775 if (this.endpoint == null) 5776 return false; 5777 for (CapabilityStatementMessagingEndpointComponent item : this.endpoint) 5778 if (!item.isEmpty()) 5779 return true; 5780 return false; 5781 } 5782 5783 public CapabilityStatementMessagingEndpointComponent addEndpoint() { //3 5784 CapabilityStatementMessagingEndpointComponent t = new CapabilityStatementMessagingEndpointComponent(); 5785 if (this.endpoint == null) 5786 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 5787 this.endpoint.add(t); 5788 return t; 5789 } 5790 5791 public CapabilityStatementMessagingComponent addEndpoint(CapabilityStatementMessagingEndpointComponent t) { //3 5792 if (t == null) 5793 return this; 5794 if (this.endpoint == null) 5795 this.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 5796 this.endpoint.add(t); 5797 return this; 5798 } 5799 5800 /** 5801 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 5802 */ 5803 public CapabilityStatementMessagingEndpointComponent getEndpointFirstRep() { 5804 if (getEndpoint().isEmpty()) { 5805 addEndpoint(); 5806 } 5807 return getEndpoint().get(0); 5808 } 5809 5810 /** 5811 * @return {@link #reliableCache} (Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).). This is the underlying object with id, value and extensions. The accessor "getReliableCache" gives direct access to the value 5812 */ 5813 public UnsignedIntType getReliableCacheElement() { 5814 if (this.reliableCache == null) 5815 if (Configuration.errorOnAutoCreate()) 5816 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.reliableCache"); 5817 else if (Configuration.doAutoCreate()) 5818 this.reliableCache = new UnsignedIntType(); // bb 5819 return this.reliableCache; 5820 } 5821 5822 public boolean hasReliableCacheElement() { 5823 return this.reliableCache != null && !this.reliableCache.isEmpty(); 5824 } 5825 5826 public boolean hasReliableCache() { 5827 return this.reliableCache != null && !this.reliableCache.isEmpty(); 5828 } 5829 5830 /** 5831 * @param value {@link #reliableCache} (Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).). This is the underlying object with id, value and extensions. The accessor "getReliableCache" gives direct access to the value 5832 */ 5833 public CapabilityStatementMessagingComponent setReliableCacheElement(UnsignedIntType value) { 5834 this.reliableCache = value; 5835 return this; 5836 } 5837 5838 /** 5839 * @return Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender). 5840 */ 5841 public int getReliableCache() { 5842 return this.reliableCache == null || this.reliableCache.isEmpty() ? 0 : this.reliableCache.getValue(); 5843 } 5844 5845 /** 5846 * @param value Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender). 5847 */ 5848 public CapabilityStatementMessagingComponent setReliableCache(int value) { 5849 if (this.reliableCache == null) 5850 this.reliableCache = new UnsignedIntType(); 5851 this.reliableCache.setValue(value); 5852 return this; 5853 } 5854 5855 /** 5856 * @return {@link #documentation} (Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5857 */ 5858 public MarkdownType getDocumentationElement() { 5859 if (this.documentation == null) 5860 if (Configuration.errorOnAutoCreate()) 5861 throw new Error("Attempt to auto-create CapabilityStatementMessagingComponent.documentation"); 5862 else if (Configuration.doAutoCreate()) 5863 this.documentation = new MarkdownType(); // bb 5864 return this.documentation; 5865 } 5866 5867 public boolean hasDocumentationElement() { 5868 return this.documentation != null && !this.documentation.isEmpty(); 5869 } 5870 5871 public boolean hasDocumentation() { 5872 return this.documentation != null && !this.documentation.isEmpty(); 5873 } 5874 5875 /** 5876 * @param value {@link #documentation} (Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5877 */ 5878 public CapabilityStatementMessagingComponent setDocumentationElement(MarkdownType value) { 5879 this.documentation = value; 5880 return this; 5881 } 5882 5883 /** 5884 * @return Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner. 5885 */ 5886 public String getDocumentation() { 5887 return this.documentation == null ? null : this.documentation.getValue(); 5888 } 5889 5890 /** 5891 * @param value Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner. 5892 */ 5893 public CapabilityStatementMessagingComponent setDocumentation(String value) { 5894 if (Utilities.noString(value)) 5895 this.documentation = null; 5896 else { 5897 if (this.documentation == null) 5898 this.documentation = new MarkdownType(); 5899 this.documentation.setValue(value); 5900 } 5901 return this; 5902 } 5903 5904 /** 5905 * @return {@link #supportedMessage} (References to message definitions for messages this system can send or receive.) 5906 */ 5907 public List<CapabilityStatementMessagingSupportedMessageComponent> getSupportedMessage() { 5908 if (this.supportedMessage == null) 5909 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 5910 return this.supportedMessage; 5911 } 5912 5913 /** 5914 * @return Returns a reference to <code>this</code> for easy method chaining 5915 */ 5916 public CapabilityStatementMessagingComponent setSupportedMessage(List<CapabilityStatementMessagingSupportedMessageComponent> theSupportedMessage) { 5917 this.supportedMessage = theSupportedMessage; 5918 return this; 5919 } 5920 5921 public boolean hasSupportedMessage() { 5922 if (this.supportedMessage == null) 5923 return false; 5924 for (CapabilityStatementMessagingSupportedMessageComponent item : this.supportedMessage) 5925 if (!item.isEmpty()) 5926 return true; 5927 return false; 5928 } 5929 5930 public CapabilityStatementMessagingSupportedMessageComponent addSupportedMessage() { //3 5931 CapabilityStatementMessagingSupportedMessageComponent t = new CapabilityStatementMessagingSupportedMessageComponent(); 5932 if (this.supportedMessage == null) 5933 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 5934 this.supportedMessage.add(t); 5935 return t; 5936 } 5937 5938 public CapabilityStatementMessagingComponent addSupportedMessage(CapabilityStatementMessagingSupportedMessageComponent t) { //3 5939 if (t == null) 5940 return this; 5941 if (this.supportedMessage == null) 5942 this.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 5943 this.supportedMessage.add(t); 5944 return this; 5945 } 5946 5947 /** 5948 * @return The first repetition of repeating field {@link #supportedMessage}, creating it if it does not already exist {3} 5949 */ 5950 public CapabilityStatementMessagingSupportedMessageComponent getSupportedMessageFirstRep() { 5951 if (getSupportedMessage().isEmpty()) { 5952 addSupportedMessage(); 5953 } 5954 return getSupportedMessage().get(0); 5955 } 5956 5957 protected void listChildren(List<Property> children) { 5958 super.listChildren(children); 5959 children.add(new Property("endpoint", "", "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 5960 children.add(new Property("reliableCache", "unsignedInt", "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 0, 1, reliableCache)); 5961 children.add(new Property("documentation", "markdown", "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 0, 1, documentation)); 5962 children.add(new Property("supportedMessage", "", "References to message definitions for messages this system can send or receive.", 0, java.lang.Integer.MAX_VALUE, supportedMessage)); 5963 } 5964 5965 @Override 5966 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5967 switch (_hash) { 5968 case 1741102485: /*endpoint*/ return new Property("endpoint", "", "An endpoint (network accessible address) to which messages and/or replies are to be sent.", 0, java.lang.Integer.MAX_VALUE, endpoint); 5969 case 897803608: /*reliableCache*/ return new Property("reliableCache", "unsignedInt", "Length if the receiver's reliable messaging cache in minutes (if a receiver) or how long the cache length on the receiver should be (if a sender).", 0, 1, reliableCache); 5970 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Documentation about the system's messaging capabilities for this endpoint not otherwise documented by the capability statement. For example, the process for becoming an authorized messaging exchange partner.", 0, 1, documentation); 5971 case -1805139079: /*supportedMessage*/ return new Property("supportedMessage", "", "References to message definitions for messages this system can send or receive.", 0, java.lang.Integer.MAX_VALUE, supportedMessage); 5972 default: return super.getNamedProperty(_hash, _name, _checkValid); 5973 } 5974 5975 } 5976 5977 @Override 5978 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5979 switch (hash) { 5980 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // CapabilityStatementMessagingEndpointComponent 5981 case 897803608: /*reliableCache*/ return this.reliableCache == null ? new Base[0] : new Base[] {this.reliableCache}; // UnsignedIntType 5982 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 5983 case -1805139079: /*supportedMessage*/ return this.supportedMessage == null ? new Base[0] : this.supportedMessage.toArray(new Base[this.supportedMessage.size()]); // CapabilityStatementMessagingSupportedMessageComponent 5984 default: return super.getProperty(hash, name, checkValid); 5985 } 5986 5987 } 5988 5989 @Override 5990 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5991 switch (hash) { 5992 case 1741102485: // endpoint 5993 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); // CapabilityStatementMessagingEndpointComponent 5994 return value; 5995 case 897803608: // reliableCache 5996 this.reliableCache = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 5997 return value; 5998 case 1587405498: // documentation 5999 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 6000 return value; 6001 case -1805139079: // supportedMessage 6002 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); // CapabilityStatementMessagingSupportedMessageComponent 6003 return value; 6004 default: return super.setProperty(hash, name, value); 6005 } 6006 6007 } 6008 6009 @Override 6010 public Base setProperty(String name, Base value) throws FHIRException { 6011 if (name.equals("endpoint")) { 6012 this.getEndpoint().add((CapabilityStatementMessagingEndpointComponent) value); 6013 } else if (name.equals("reliableCache")) { 6014 this.reliableCache = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 6015 } else if (name.equals("documentation")) { 6016 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 6017 } else if (name.equals("supportedMessage")) { 6018 this.getSupportedMessage().add((CapabilityStatementMessagingSupportedMessageComponent) value); 6019 } else 6020 return super.setProperty(name, value); 6021 return value; 6022 } 6023 6024 @Override 6025 public void removeChild(String name, Base value) throws FHIRException { 6026 if (name.equals("endpoint")) { 6027 this.getEndpoint().remove((CapabilityStatementMessagingEndpointComponent) value); 6028 } else if (name.equals("reliableCache")) { 6029 this.reliableCache = null; 6030 } else if (name.equals("documentation")) { 6031 this.documentation = null; 6032 } else if (name.equals("supportedMessage")) { 6033 this.getSupportedMessage().remove((CapabilityStatementMessagingSupportedMessageComponent) value); 6034 } else 6035 super.removeChild(name, value); 6036 6037 } 6038 6039 @Override 6040 public Base makeProperty(int hash, String name) throws FHIRException { 6041 switch (hash) { 6042 case 1741102485: return addEndpoint(); 6043 case 897803608: return getReliableCacheElement(); 6044 case 1587405498: return getDocumentationElement(); 6045 case -1805139079: return addSupportedMessage(); 6046 default: return super.makeProperty(hash, name); 6047 } 6048 6049 } 6050 6051 @Override 6052 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6053 switch (hash) { 6054 case 1741102485: /*endpoint*/ return new String[] {}; 6055 case 897803608: /*reliableCache*/ return new String[] {"unsignedInt"}; 6056 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 6057 case -1805139079: /*supportedMessage*/ return new String[] {}; 6058 default: return super.getTypesForProperty(hash, name); 6059 } 6060 6061 } 6062 6063 @Override 6064 public Base addChild(String name) throws FHIRException { 6065 if (name.equals("endpoint")) { 6066 return addEndpoint(); 6067 } 6068 else if (name.equals("reliableCache")) { 6069 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.messaging.reliableCache"); 6070 } 6071 else if (name.equals("documentation")) { 6072 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.messaging.documentation"); 6073 } 6074 else if (name.equals("supportedMessage")) { 6075 return addSupportedMessage(); 6076 } 6077 else 6078 return super.addChild(name); 6079 } 6080 6081 public CapabilityStatementMessagingComponent copy() { 6082 CapabilityStatementMessagingComponent dst = new CapabilityStatementMessagingComponent(); 6083 copyValues(dst); 6084 return dst; 6085 } 6086 6087 public void copyValues(CapabilityStatementMessagingComponent dst) { 6088 super.copyValues(dst); 6089 if (endpoint != null) { 6090 dst.endpoint = new ArrayList<CapabilityStatementMessagingEndpointComponent>(); 6091 for (CapabilityStatementMessagingEndpointComponent i : endpoint) 6092 dst.endpoint.add(i.copy()); 6093 }; 6094 dst.reliableCache = reliableCache == null ? null : reliableCache.copy(); 6095 dst.documentation = documentation == null ? null : documentation.copy(); 6096 if (supportedMessage != null) { 6097 dst.supportedMessage = new ArrayList<CapabilityStatementMessagingSupportedMessageComponent>(); 6098 for (CapabilityStatementMessagingSupportedMessageComponent i : supportedMessage) 6099 dst.supportedMessage.add(i.copy()); 6100 }; 6101 } 6102 6103 @Override 6104 public boolean equalsDeep(Base other_) { 6105 if (!super.equalsDeep(other_)) 6106 return false; 6107 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 6108 return false; 6109 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 6110 return compareDeep(endpoint, o.endpoint, true) && compareDeep(reliableCache, o.reliableCache, true) 6111 && compareDeep(documentation, o.documentation, true) && compareDeep(supportedMessage, o.supportedMessage, true) 6112 ; 6113 } 6114 6115 @Override 6116 public boolean equalsShallow(Base other_) { 6117 if (!super.equalsShallow(other_)) 6118 return false; 6119 if (!(other_ instanceof CapabilityStatementMessagingComponent)) 6120 return false; 6121 CapabilityStatementMessagingComponent o = (CapabilityStatementMessagingComponent) other_; 6122 return compareValues(reliableCache, o.reliableCache, true) && compareValues(documentation, o.documentation, true) 6123 ; 6124 } 6125 6126 public boolean isEmpty() { 6127 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(endpoint, reliableCache, documentation 6128 , supportedMessage); 6129 } 6130 6131 public String fhirType() { 6132 return "CapabilityStatement.messaging"; 6133 6134 } 6135 6136 } 6137 6138 @Block() 6139 public static class CapabilityStatementMessagingEndpointComponent extends BackboneElement implements IBaseBackboneElement { 6140 /** 6141 * A list of the messaging transport protocol(s) identifiers, supported by this endpoint. 6142 */ 6143 @Child(name = "protocol", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 6144 @Description(shortDefinition="http | ftp | mllp +", formalDefinition="A list of the messaging transport protocol(s) identifiers, supported by this endpoint." ) 6145 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-transport") 6146 protected Coding protocol; 6147 6148 /** 6149 * The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier. 6150 */ 6151 @Child(name = "address", type = {UrlType.class}, order=2, min=1, max=1, modifier=false, summary=false) 6152 @Description(shortDefinition="Network address or identifier of the end-point", formalDefinition="The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier." ) 6153 protected UrlType address; 6154 6155 private static final long serialVersionUID = -236946103L; 6156 6157 /** 6158 * Constructor 6159 */ 6160 public CapabilityStatementMessagingEndpointComponent() { 6161 super(); 6162 } 6163 6164 /** 6165 * Constructor 6166 */ 6167 public CapabilityStatementMessagingEndpointComponent(Coding protocol, String address) { 6168 super(); 6169 this.setProtocol(protocol); 6170 this.setAddress(address); 6171 } 6172 6173 /** 6174 * @return {@link #protocol} (A list of the messaging transport protocol(s) identifiers, supported by this endpoint.) 6175 */ 6176 public Coding getProtocol() { 6177 if (this.protocol == null) 6178 if (Configuration.errorOnAutoCreate()) 6179 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.protocol"); 6180 else if (Configuration.doAutoCreate()) 6181 this.protocol = new Coding(); // cc 6182 return this.protocol; 6183 } 6184 6185 public boolean hasProtocol() { 6186 return this.protocol != null && !this.protocol.isEmpty(); 6187 } 6188 6189 /** 6190 * @param value {@link #protocol} (A list of the messaging transport protocol(s) identifiers, supported by this endpoint.) 6191 */ 6192 public CapabilityStatementMessagingEndpointComponent setProtocol(Coding value) { 6193 this.protocol = value; 6194 return this; 6195 } 6196 6197 /** 6198 * @return {@link #address} (The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 6199 */ 6200 public UrlType getAddressElement() { 6201 if (this.address == null) 6202 if (Configuration.errorOnAutoCreate()) 6203 throw new Error("Attempt to auto-create CapabilityStatementMessagingEndpointComponent.address"); 6204 else if (Configuration.doAutoCreate()) 6205 this.address = new UrlType(); // bb 6206 return this.address; 6207 } 6208 6209 public boolean hasAddressElement() { 6210 return this.address != null && !this.address.isEmpty(); 6211 } 6212 6213 public boolean hasAddress() { 6214 return this.address != null && !this.address.isEmpty(); 6215 } 6216 6217 /** 6218 * @param value {@link #address} (The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 6219 */ 6220 public CapabilityStatementMessagingEndpointComponent setAddressElement(UrlType value) { 6221 this.address = value; 6222 return this; 6223 } 6224 6225 /** 6226 * @return The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier. 6227 */ 6228 public String getAddress() { 6229 return this.address == null ? null : this.address.getValue(); 6230 } 6231 6232 /** 6233 * @param value The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier. 6234 */ 6235 public CapabilityStatementMessagingEndpointComponent setAddress(String value) { 6236 if (this.address == null) 6237 this.address = new UrlType(); 6238 this.address.setValue(value); 6239 return this; 6240 } 6241 6242 protected void listChildren(List<Property> children) { 6243 super.listChildren(children); 6244 children.add(new Property("protocol", "Coding", "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol)); 6245 children.add(new Property("address", "url", "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.", 0, 1, address)); 6246 } 6247 6248 @Override 6249 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6250 switch (_hash) { 6251 case -989163880: /*protocol*/ return new Property("protocol", "Coding", "A list of the messaging transport protocol(s) identifiers, supported by this endpoint.", 0, 1, protocol); 6252 case -1147692044: /*address*/ return new Property("address", "url", "The network address of the endpoint. For solutions that do not use network addresses for routing, it can be just an identifier.", 0, 1, address); 6253 default: return super.getNamedProperty(_hash, _name, _checkValid); 6254 } 6255 6256 } 6257 6258 @Override 6259 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6260 switch (hash) { 6261 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : new Base[] {this.protocol}; // Coding 6262 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // UrlType 6263 default: return super.getProperty(hash, name, checkValid); 6264 } 6265 6266 } 6267 6268 @Override 6269 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6270 switch (hash) { 6271 case -989163880: // protocol 6272 this.protocol = TypeConvertor.castToCoding(value); // Coding 6273 return value; 6274 case -1147692044: // address 6275 this.address = TypeConvertor.castToUrl(value); // UrlType 6276 return value; 6277 default: return super.setProperty(hash, name, value); 6278 } 6279 6280 } 6281 6282 @Override 6283 public Base setProperty(String name, Base value) throws FHIRException { 6284 if (name.equals("protocol")) { 6285 this.protocol = TypeConvertor.castToCoding(value); // Coding 6286 } else if (name.equals("address")) { 6287 this.address = TypeConvertor.castToUrl(value); // UrlType 6288 } else 6289 return super.setProperty(name, value); 6290 return value; 6291 } 6292 6293 @Override 6294 public void removeChild(String name, Base value) throws FHIRException { 6295 if (name.equals("protocol")) { 6296 this.protocol = null; 6297 } else if (name.equals("address")) { 6298 this.address = null; 6299 } else 6300 super.removeChild(name, value); 6301 6302 } 6303 6304 @Override 6305 public Base makeProperty(int hash, String name) throws FHIRException { 6306 switch (hash) { 6307 case -989163880: return getProtocol(); 6308 case -1147692044: return getAddressElement(); 6309 default: return super.makeProperty(hash, name); 6310 } 6311 6312 } 6313 6314 @Override 6315 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6316 switch (hash) { 6317 case -989163880: /*protocol*/ return new String[] {"Coding"}; 6318 case -1147692044: /*address*/ return new String[] {"url"}; 6319 default: return super.getTypesForProperty(hash, name); 6320 } 6321 6322 } 6323 6324 @Override 6325 public Base addChild(String name) throws FHIRException { 6326 if (name.equals("protocol")) { 6327 this.protocol = new Coding(); 6328 return this.protocol; 6329 } 6330 else if (name.equals("address")) { 6331 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.messaging.endpoint.address"); 6332 } 6333 else 6334 return super.addChild(name); 6335 } 6336 6337 public CapabilityStatementMessagingEndpointComponent copy() { 6338 CapabilityStatementMessagingEndpointComponent dst = new CapabilityStatementMessagingEndpointComponent(); 6339 copyValues(dst); 6340 return dst; 6341 } 6342 6343 public void copyValues(CapabilityStatementMessagingEndpointComponent dst) { 6344 super.copyValues(dst); 6345 dst.protocol = protocol == null ? null : protocol.copy(); 6346 dst.address = address == null ? null : address.copy(); 6347 } 6348 6349 @Override 6350 public boolean equalsDeep(Base other_) { 6351 if (!super.equalsDeep(other_)) 6352 return false; 6353 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 6354 return false; 6355 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 6356 return compareDeep(protocol, o.protocol, true) && compareDeep(address, o.address, true); 6357 } 6358 6359 @Override 6360 public boolean equalsShallow(Base other_) { 6361 if (!super.equalsShallow(other_)) 6362 return false; 6363 if (!(other_ instanceof CapabilityStatementMessagingEndpointComponent)) 6364 return false; 6365 CapabilityStatementMessagingEndpointComponent o = (CapabilityStatementMessagingEndpointComponent) other_; 6366 return compareValues(address, o.address, true); 6367 } 6368 6369 public boolean isEmpty() { 6370 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(protocol, address); 6371 } 6372 6373 public String fhirType() { 6374 return "CapabilityStatement.messaging.endpoint"; 6375 6376 } 6377 6378 } 6379 6380 @Block() 6381 public static class CapabilityStatementMessagingSupportedMessageComponent extends BackboneElement implements IBaseBackboneElement { 6382 /** 6383 * The mode of this event declaration - whether application is sender or receiver. 6384 */ 6385 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 6386 @Description(shortDefinition="sender | receiver", formalDefinition="The mode of this event declaration - whether application is sender or receiver." ) 6387 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-capability-mode") 6388 protected Enumeration<EventCapabilityMode> mode; 6389 6390 /** 6391 * Points to a message definition that identifies the messaging event, message structure, allowed responses, etc. 6392 */ 6393 @Child(name = "definition", type = {CanonicalType.class}, order=2, min=1, max=1, modifier=false, summary=true) 6394 @Description(shortDefinition="Message supported by this system", formalDefinition="Points to a message definition that identifies the messaging event, message structure, allowed responses, etc." ) 6395 protected CanonicalType definition; 6396 6397 private static final long serialVersionUID = -1172840676L; 6398 6399 /** 6400 * Constructor 6401 */ 6402 public CapabilityStatementMessagingSupportedMessageComponent() { 6403 super(); 6404 } 6405 6406 /** 6407 * Constructor 6408 */ 6409 public CapabilityStatementMessagingSupportedMessageComponent(EventCapabilityMode mode, String definition) { 6410 super(); 6411 this.setMode(mode); 6412 this.setDefinition(definition); 6413 } 6414 6415 /** 6416 * @return {@link #mode} (The mode of this event declaration - whether application is sender or receiver.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6417 */ 6418 public Enumeration<EventCapabilityMode> getModeElement() { 6419 if (this.mode == null) 6420 if (Configuration.errorOnAutoCreate()) 6421 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.mode"); 6422 else if (Configuration.doAutoCreate()) 6423 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); // bb 6424 return this.mode; 6425 } 6426 6427 public boolean hasModeElement() { 6428 return this.mode != null && !this.mode.isEmpty(); 6429 } 6430 6431 public boolean hasMode() { 6432 return this.mode != null && !this.mode.isEmpty(); 6433 } 6434 6435 /** 6436 * @param value {@link #mode} (The mode of this event declaration - whether application is sender or receiver.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6437 */ 6438 public CapabilityStatementMessagingSupportedMessageComponent setModeElement(Enumeration<EventCapabilityMode> value) { 6439 this.mode = value; 6440 return this; 6441 } 6442 6443 /** 6444 * @return The mode of this event declaration - whether application is sender or receiver. 6445 */ 6446 public EventCapabilityMode getMode() { 6447 return this.mode == null ? null : this.mode.getValue(); 6448 } 6449 6450 /** 6451 * @param value The mode of this event declaration - whether application is sender or receiver. 6452 */ 6453 public CapabilityStatementMessagingSupportedMessageComponent setMode(EventCapabilityMode value) { 6454 if (this.mode == null) 6455 this.mode = new Enumeration<EventCapabilityMode>(new EventCapabilityModeEnumFactory()); 6456 this.mode.setValue(value); 6457 return this; 6458 } 6459 6460 /** 6461 * @return {@link #definition} (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 6462 */ 6463 public CanonicalType getDefinitionElement() { 6464 if (this.definition == null) 6465 if (Configuration.errorOnAutoCreate()) 6466 throw new Error("Attempt to auto-create CapabilityStatementMessagingSupportedMessageComponent.definition"); 6467 else if (Configuration.doAutoCreate()) 6468 this.definition = new CanonicalType(); // bb 6469 return this.definition; 6470 } 6471 6472 public boolean hasDefinitionElement() { 6473 return this.definition != null && !this.definition.isEmpty(); 6474 } 6475 6476 public boolean hasDefinition() { 6477 return this.definition != null && !this.definition.isEmpty(); 6478 } 6479 6480 /** 6481 * @param value {@link #definition} (Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 6482 */ 6483 public CapabilityStatementMessagingSupportedMessageComponent setDefinitionElement(CanonicalType value) { 6484 this.definition = value; 6485 return this; 6486 } 6487 6488 /** 6489 * @return Points to a message definition that identifies the messaging event, message structure, allowed responses, etc. 6490 */ 6491 public String getDefinition() { 6492 return this.definition == null ? null : this.definition.getValue(); 6493 } 6494 6495 /** 6496 * @param value Points to a message definition that identifies the messaging event, message structure, allowed responses, etc. 6497 */ 6498 public CapabilityStatementMessagingSupportedMessageComponent setDefinition(String value) { 6499 if (this.definition == null) 6500 this.definition = new CanonicalType(); 6501 this.definition.setValue(value); 6502 return this; 6503 } 6504 6505 protected void listChildren(List<Property> children) { 6506 super.listChildren(children); 6507 children.add(new Property("mode", "code", "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode)); 6508 children.add(new Property("definition", "canonical(MessageDefinition)", "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 0, 1, definition)); 6509 } 6510 6511 @Override 6512 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6513 switch (_hash) { 6514 case 3357091: /*mode*/ return new Property("mode", "code", "The mode of this event declaration - whether application is sender or receiver.", 0, 1, mode); 6515 case -1014418093: /*definition*/ return new Property("definition", "canonical(MessageDefinition)", "Points to a message definition that identifies the messaging event, message structure, allowed responses, etc.", 0, 1, definition); 6516 default: return super.getNamedProperty(_hash, _name, _checkValid); 6517 } 6518 6519 } 6520 6521 @Override 6522 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6523 switch (hash) { 6524 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<EventCapabilityMode> 6525 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CanonicalType 6526 default: return super.getProperty(hash, name, checkValid); 6527 } 6528 6529 } 6530 6531 @Override 6532 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6533 switch (hash) { 6534 case 3357091: // mode 6535 value = new EventCapabilityModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6536 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 6537 return value; 6538 case -1014418093: // definition 6539 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 6540 return value; 6541 default: return super.setProperty(hash, name, value); 6542 } 6543 6544 } 6545 6546 @Override 6547 public Base setProperty(String name, Base value) throws FHIRException { 6548 if (name.equals("mode")) { 6549 value = new EventCapabilityModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6550 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 6551 } else if (name.equals("definition")) { 6552 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 6553 } else 6554 return super.setProperty(name, value); 6555 return value; 6556 } 6557 6558 @Override 6559 public void removeChild(String name, Base value) throws FHIRException { 6560 if (name.equals("mode")) { 6561 value = new EventCapabilityModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6562 this.mode = (Enumeration) value; // Enumeration<EventCapabilityMode> 6563 } else if (name.equals("definition")) { 6564 this.definition = null; 6565 } else 6566 super.removeChild(name, value); 6567 6568 } 6569 6570 @Override 6571 public Base makeProperty(int hash, String name) throws FHIRException { 6572 switch (hash) { 6573 case 3357091: return getModeElement(); 6574 case -1014418093: return getDefinitionElement(); 6575 default: return super.makeProperty(hash, name); 6576 } 6577 6578 } 6579 6580 @Override 6581 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6582 switch (hash) { 6583 case 3357091: /*mode*/ return new String[] {"code"}; 6584 case -1014418093: /*definition*/ return new String[] {"canonical"}; 6585 default: return super.getTypesForProperty(hash, name); 6586 } 6587 6588 } 6589 6590 @Override 6591 public Base addChild(String name) throws FHIRException { 6592 if (name.equals("mode")) { 6593 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.messaging.supportedMessage.mode"); 6594 } 6595 else if (name.equals("definition")) { 6596 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.messaging.supportedMessage.definition"); 6597 } 6598 else 6599 return super.addChild(name); 6600 } 6601 6602 public CapabilityStatementMessagingSupportedMessageComponent copy() { 6603 CapabilityStatementMessagingSupportedMessageComponent dst = new CapabilityStatementMessagingSupportedMessageComponent(); 6604 copyValues(dst); 6605 return dst; 6606 } 6607 6608 public void copyValues(CapabilityStatementMessagingSupportedMessageComponent dst) { 6609 super.copyValues(dst); 6610 dst.mode = mode == null ? null : mode.copy(); 6611 dst.definition = definition == null ? null : definition.copy(); 6612 } 6613 6614 @Override 6615 public boolean equalsDeep(Base other_) { 6616 if (!super.equalsDeep(other_)) 6617 return false; 6618 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 6619 return false; 6620 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 6621 return compareDeep(mode, o.mode, true) && compareDeep(definition, o.definition, true); 6622 } 6623 6624 @Override 6625 public boolean equalsShallow(Base other_) { 6626 if (!super.equalsShallow(other_)) 6627 return false; 6628 if (!(other_ instanceof CapabilityStatementMessagingSupportedMessageComponent)) 6629 return false; 6630 CapabilityStatementMessagingSupportedMessageComponent o = (CapabilityStatementMessagingSupportedMessageComponent) other_; 6631 return compareValues(mode, o.mode, true) && compareValues(definition, o.definition, true); 6632 } 6633 6634 public boolean isEmpty() { 6635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, definition); 6636 } 6637 6638 public String fhirType() { 6639 return "CapabilityStatement.messaging.supportedMessage"; 6640 6641 } 6642 6643 } 6644 6645 @Block() 6646 public static class CapabilityStatementDocumentComponent extends BackboneElement implements IBaseBackboneElement { 6647 /** 6648 * Mode of this document declaration - whether an application is a producer or consumer. 6649 */ 6650 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 6651 @Description(shortDefinition="producer | consumer", formalDefinition="Mode of this document declaration - whether an application is a producer or consumer." ) 6652 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-mode") 6653 protected Enumeration<DocumentMode> mode; 6654 6655 /** 6656 * A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc. 6657 */ 6658 @Child(name = "documentation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 6659 @Description(shortDefinition="Description of document support", formalDefinition="A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc." ) 6660 protected MarkdownType documentation; 6661 6662 /** 6663 * A profile on the document Bundle that constrains which resources are present, and their contents. 6664 */ 6665 @Child(name = "profile", type = {CanonicalType.class}, order=3, min=1, max=1, modifier=false, summary=true) 6666 @Description(shortDefinition="Constraint on the resources used in the document", formalDefinition="A profile on the document Bundle that constrains which resources are present, and their contents." ) 6667 protected CanonicalType profile; 6668 6669 private static final long serialVersionUID = 18026632L; 6670 6671 /** 6672 * Constructor 6673 */ 6674 public CapabilityStatementDocumentComponent() { 6675 super(); 6676 } 6677 6678 /** 6679 * Constructor 6680 */ 6681 public CapabilityStatementDocumentComponent(DocumentMode mode, String profile) { 6682 super(); 6683 this.setMode(mode); 6684 this.setProfile(profile); 6685 } 6686 6687 /** 6688 * @return {@link #mode} (Mode of this document declaration - whether an application is a producer or consumer.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6689 */ 6690 public Enumeration<DocumentMode> getModeElement() { 6691 if (this.mode == null) 6692 if (Configuration.errorOnAutoCreate()) 6693 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.mode"); 6694 else if (Configuration.doAutoCreate()) 6695 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); // bb 6696 return this.mode; 6697 } 6698 6699 public boolean hasModeElement() { 6700 return this.mode != null && !this.mode.isEmpty(); 6701 } 6702 6703 public boolean hasMode() { 6704 return this.mode != null && !this.mode.isEmpty(); 6705 } 6706 6707 /** 6708 * @param value {@link #mode} (Mode of this document declaration - whether an application is a producer or consumer.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 6709 */ 6710 public CapabilityStatementDocumentComponent setModeElement(Enumeration<DocumentMode> value) { 6711 this.mode = value; 6712 return this; 6713 } 6714 6715 /** 6716 * @return Mode of this document declaration - whether an application is a producer or consumer. 6717 */ 6718 public DocumentMode getMode() { 6719 return this.mode == null ? null : this.mode.getValue(); 6720 } 6721 6722 /** 6723 * @param value Mode of this document declaration - whether an application is a producer or consumer. 6724 */ 6725 public CapabilityStatementDocumentComponent setMode(DocumentMode value) { 6726 if (this.mode == null) 6727 this.mode = new Enumeration<DocumentMode>(new DocumentModeEnumFactory()); 6728 this.mode.setValue(value); 6729 return this; 6730 } 6731 6732 /** 6733 * @return {@link #documentation} (A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 6734 */ 6735 public MarkdownType getDocumentationElement() { 6736 if (this.documentation == null) 6737 if (Configuration.errorOnAutoCreate()) 6738 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.documentation"); 6739 else if (Configuration.doAutoCreate()) 6740 this.documentation = new MarkdownType(); // bb 6741 return this.documentation; 6742 } 6743 6744 public boolean hasDocumentationElement() { 6745 return this.documentation != null && !this.documentation.isEmpty(); 6746 } 6747 6748 public boolean hasDocumentation() { 6749 return this.documentation != null && !this.documentation.isEmpty(); 6750 } 6751 6752 /** 6753 * @param value {@link #documentation} (A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 6754 */ 6755 public CapabilityStatementDocumentComponent setDocumentationElement(MarkdownType value) { 6756 this.documentation = value; 6757 return this; 6758 } 6759 6760 /** 6761 * @return A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc. 6762 */ 6763 public String getDocumentation() { 6764 return this.documentation == null ? null : this.documentation.getValue(); 6765 } 6766 6767 /** 6768 * @param value A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc. 6769 */ 6770 public CapabilityStatementDocumentComponent setDocumentation(String value) { 6771 if (Utilities.noString(value)) 6772 this.documentation = null; 6773 else { 6774 if (this.documentation == null) 6775 this.documentation = new MarkdownType(); 6776 this.documentation.setValue(value); 6777 } 6778 return this; 6779 } 6780 6781 /** 6782 * @return {@link #profile} (A profile on the document Bundle that constrains which resources are present, and their contents.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 6783 */ 6784 public CanonicalType getProfileElement() { 6785 if (this.profile == null) 6786 if (Configuration.errorOnAutoCreate()) 6787 throw new Error("Attempt to auto-create CapabilityStatementDocumentComponent.profile"); 6788 else if (Configuration.doAutoCreate()) 6789 this.profile = new CanonicalType(); // bb 6790 return this.profile; 6791 } 6792 6793 public boolean hasProfileElement() { 6794 return this.profile != null && !this.profile.isEmpty(); 6795 } 6796 6797 public boolean hasProfile() { 6798 return this.profile != null && !this.profile.isEmpty(); 6799 } 6800 6801 /** 6802 * @param value {@link #profile} (A profile on the document Bundle that constrains which resources are present, and their contents.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 6803 */ 6804 public CapabilityStatementDocumentComponent setProfileElement(CanonicalType value) { 6805 this.profile = value; 6806 return this; 6807 } 6808 6809 /** 6810 * @return A profile on the document Bundle that constrains which resources are present, and their contents. 6811 */ 6812 public String getProfile() { 6813 return this.profile == null ? null : this.profile.getValue(); 6814 } 6815 6816 /** 6817 * @param value A profile on the document Bundle that constrains which resources are present, and their contents. 6818 */ 6819 public CapabilityStatementDocumentComponent setProfile(String value) { 6820 if (this.profile == null) 6821 this.profile = new CanonicalType(); 6822 this.profile.setValue(value); 6823 return this; 6824 } 6825 6826 protected void listChildren(List<Property> children) { 6827 super.listChildren(children); 6828 children.add(new Property("mode", "code", "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode)); 6829 children.add(new Property("documentation", "markdown", "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 0, 1, documentation)); 6830 children.add(new Property("profile", "canonical(StructureDefinition)", "A profile on the document Bundle that constrains which resources are present, and their contents.", 0, 1, profile)); 6831 } 6832 6833 @Override 6834 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6835 switch (_hash) { 6836 case 3357091: /*mode*/ return new Property("mode", "code", "Mode of this document declaration - whether an application is a producer or consumer.", 0, 1, mode); 6837 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "A description of how the application supports or uses the specified document profile. For example, when documents are created, what action is taken with consumed documents, etc.", 0, 1, documentation); 6838 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A profile on the document Bundle that constrains which resources are present, and their contents.", 0, 1, profile); 6839 default: return super.getNamedProperty(_hash, _name, _checkValid); 6840 } 6841 6842 } 6843 6844 @Override 6845 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6846 switch (hash) { 6847 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<DocumentMode> 6848 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 6849 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 6850 default: return super.getProperty(hash, name, checkValid); 6851 } 6852 6853 } 6854 6855 @Override 6856 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6857 switch (hash) { 6858 case 3357091: // mode 6859 value = new DocumentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6860 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 6861 return value; 6862 case 1587405498: // documentation 6863 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 6864 return value; 6865 case -309425751: // profile 6866 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 6867 return value; 6868 default: return super.setProperty(hash, name, value); 6869 } 6870 6871 } 6872 6873 @Override 6874 public Base setProperty(String name, Base value) throws FHIRException { 6875 if (name.equals("mode")) { 6876 value = new DocumentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6877 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 6878 } else if (name.equals("documentation")) { 6879 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 6880 } else if (name.equals("profile")) { 6881 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 6882 } else 6883 return super.setProperty(name, value); 6884 return value; 6885 } 6886 6887 @Override 6888 public void removeChild(String name, Base value) throws FHIRException { 6889 if (name.equals("mode")) { 6890 value = new DocumentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6891 this.mode = (Enumeration) value; // Enumeration<DocumentMode> 6892 } else if (name.equals("documentation")) { 6893 this.documentation = null; 6894 } else if (name.equals("profile")) { 6895 this.profile = null; 6896 } else 6897 super.removeChild(name, value); 6898 6899 } 6900 6901 @Override 6902 public Base makeProperty(int hash, String name) throws FHIRException { 6903 switch (hash) { 6904 case 3357091: return getModeElement(); 6905 case 1587405498: return getDocumentationElement(); 6906 case -309425751: return getProfileElement(); 6907 default: return super.makeProperty(hash, name); 6908 } 6909 6910 } 6911 6912 @Override 6913 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6914 switch (hash) { 6915 case 3357091: /*mode*/ return new String[] {"code"}; 6916 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 6917 case -309425751: /*profile*/ return new String[] {"canonical"}; 6918 default: return super.getTypesForProperty(hash, name); 6919 } 6920 6921 } 6922 6923 @Override 6924 public Base addChild(String name) throws FHIRException { 6925 if (name.equals("mode")) { 6926 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.document.mode"); 6927 } 6928 else if (name.equals("documentation")) { 6929 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.document.documentation"); 6930 } 6931 else if (name.equals("profile")) { 6932 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.document.profile"); 6933 } 6934 else 6935 return super.addChild(name); 6936 } 6937 6938 public CapabilityStatementDocumentComponent copy() { 6939 CapabilityStatementDocumentComponent dst = new CapabilityStatementDocumentComponent(); 6940 copyValues(dst); 6941 return dst; 6942 } 6943 6944 public void copyValues(CapabilityStatementDocumentComponent dst) { 6945 super.copyValues(dst); 6946 dst.mode = mode == null ? null : mode.copy(); 6947 dst.documentation = documentation == null ? null : documentation.copy(); 6948 dst.profile = profile == null ? null : profile.copy(); 6949 } 6950 6951 @Override 6952 public boolean equalsDeep(Base other_) { 6953 if (!super.equalsDeep(other_)) 6954 return false; 6955 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 6956 return false; 6957 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 6958 return compareDeep(mode, o.mode, true) && compareDeep(documentation, o.documentation, true) && compareDeep(profile, o.profile, true) 6959 ; 6960 } 6961 6962 @Override 6963 public boolean equalsShallow(Base other_) { 6964 if (!super.equalsShallow(other_)) 6965 return false; 6966 if (!(other_ instanceof CapabilityStatementDocumentComponent)) 6967 return false; 6968 CapabilityStatementDocumentComponent o = (CapabilityStatementDocumentComponent) other_; 6969 return compareValues(mode, o.mode, true) && compareValues(documentation, o.documentation, true) && compareValues(profile, o.profile, true) 6970 ; 6971 } 6972 6973 public boolean isEmpty() { 6974 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, documentation, profile 6975 ); 6976 } 6977 6978 public String fhirType() { 6979 return "CapabilityStatement.document"; 6980 6981 } 6982 6983 } 6984 6985 /** 6986 * An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers. 6987 */ 6988 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 6989 @Description(shortDefinition="Canonical identifier for this capability statement, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers." ) 6990 protected UriType url; 6991 6992 /** 6993 * A formal identifier that is used to identify this CapabilityStatement when it is represented in other formats, or referenced in a specification, model, design or an instance. 6994 */ 6995 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6996 @Description(shortDefinition="Additional identifier for the CapabilityStatement (business identifier)", formalDefinition="A formal identifier that is used to identify this CapabilityStatement when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 6997 protected List<Identifier> identifier; 6998 6999 /** 7000 * The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 7001 */ 7002 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 7003 @Description(shortDefinition="Business version of the capability statement", formalDefinition="The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 7004 protected StringType version; 7005 7006 /** 7007 * Indicates the mechanism used to compare versions to determine which is more current. 7008 */ 7009 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 7010 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 7011 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 7012 protected DataType versionAlgorithm; 7013 7014 /** 7015 * A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation. 7016 */ 7017 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 7018 @Description(shortDefinition="Name for this capability statement (computer friendly)", formalDefinition="A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 7019 protected StringType name; 7020 7021 /** 7022 * A short, descriptive, user-friendly title for the capability statement. 7023 */ 7024 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 7025 @Description(shortDefinition="Name for this capability statement (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the capability statement." ) 7026 protected StringType title; 7027 7028 /** 7029 * The status of this capability statement. Enables tracking the life-cycle of the content. 7030 */ 7031 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 7032 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this capability statement. Enables tracking the life-cycle of the content." ) 7033 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 7034 protected Enumeration<PublicationStatus> status; 7035 7036 /** 7037 * A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 7038 */ 7039 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 7040 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 7041 protected BooleanType experimental; 7042 7043 /** 7044 * The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes. 7045 */ 7046 @Child(name = "date", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 7047 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes." ) 7048 protected DateTimeType date; 7049 7050 /** 7051 * The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement. 7052 */ 7053 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 7054 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement." ) 7055 protected StringType publisher; 7056 7057 /** 7058 * Contact details to assist a user in finding and communicating with the publisher. 7059 */ 7060 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7061 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 7062 protected List<ContactDetail> contact; 7063 7064 /** 7065 * A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 7066 */ 7067 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 7068 @Description(shortDefinition="Natural language description of the capability statement", formalDefinition="A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP." ) 7069 protected MarkdownType description; 7070 7071 /** 7072 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances. 7073 */ 7074 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7075 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances." ) 7076 protected List<UsageContext> useContext; 7077 7078 /** 7079 * A legal or geographic region in which the capability statement is intended to be used. 7080 */ 7081 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7082 @Description(shortDefinition="Intended jurisdiction for capability statement (if applicable)", formalDefinition="A legal or geographic region in which the capability statement is intended to be used." ) 7083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 7084 protected List<CodeableConcept> jurisdiction; 7085 7086 /** 7087 * Explanation of why this capability statement is needed and why it has been designed as it has. 7088 */ 7089 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 7090 @Description(shortDefinition="Why this capability statement is defined", formalDefinition="Explanation of why this capability statement is needed and why it has been designed as it has." ) 7091 protected MarkdownType purpose; 7092 7093 /** 7094 * A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement. 7095 */ 7096 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 7097 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement." ) 7098 protected MarkdownType copyright; 7099 7100 /** 7101 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 7102 */ 7103 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 7104 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 7105 protected StringType copyrightLabel; 7106 7107 /** 7108 * The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase). 7109 */ 7110 @Child(name = "kind", type = {CodeType.class}, order=17, min=1, max=1, modifier=false, summary=true) 7111 @Description(shortDefinition="instance | capability | requirements", formalDefinition="The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase)." ) 7112 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/capability-statement-kind") 7113 protected Enumeration<CapabilityStatementKind> kind; 7114 7115 /** 7116 * Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details. 7117 */ 7118 @Child(name = "instantiates", type = {CanonicalType.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7119 @Description(shortDefinition="Canonical URL of another capability statement this implements", formalDefinition="Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details." ) 7120 protected List<CanonicalType> instantiates; 7121 7122 /** 7123 * Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them. 7124 */ 7125 @Child(name = "imports", type = {CanonicalType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7126 @Description(shortDefinition="Canonical URL of another capability statement this adds to", formalDefinition="Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them." ) 7127 protected List<CanonicalType> imports; 7128 7129 /** 7130 * Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation. 7131 */ 7132 @Child(name = "software", type = {}, order=20, min=0, max=1, modifier=false, summary=true) 7133 @Description(shortDefinition="Software that is covered by this capability statement", formalDefinition="Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation." ) 7134 protected CapabilityStatementSoftwareComponent software; 7135 7136 /** 7137 * Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program. 7138 */ 7139 @Child(name = "implementation", type = {}, order=21, min=0, max=1, modifier=false, summary=true) 7140 @Description(shortDefinition="If this describes a specific instance", formalDefinition="Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program." ) 7141 protected CapabilityStatementImplementationComponent implementation; 7142 7143 /** 7144 * The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value. 7145 */ 7146 @Child(name = "fhirVersion", type = {CodeType.class}, order=22, min=1, max=1, modifier=false, summary=true) 7147 @Description(shortDefinition="FHIR Version the system supports", formalDefinition="The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value." ) 7148 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/FHIR-version") 7149 protected Enumeration<FHIRVersion> fhirVersion; 7150 7151 /** 7152 * A list of the formats supported by this implementation using their content types. 7153 */ 7154 @Child(name = "format", type = {CodeType.class}, order=23, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7155 @Description(shortDefinition="formats supported (xml | json | ttl | mime type)", formalDefinition="A list of the formats supported by this implementation using their content types." ) 7156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 7157 protected List<CodeType> format; 7158 7159 /** 7160 * A list of the patch formats supported by this implementation using their content types. 7161 */ 7162 @Child(name = "patchFormat", type = {CodeType.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7163 @Description(shortDefinition="Patch formats supported", formalDefinition="A list of the patch formats supported by this implementation using their content types." ) 7164 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 7165 protected List<CodeType> patchFormat; 7166 7167 /** 7168 * A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header. 7169 */ 7170 @Child(name = "acceptLanguage", type = {CodeType.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7171 @Description(shortDefinition="Languages supported", formalDefinition="A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header." ) 7172 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 7173 protected List<CodeType> acceptLanguage; 7174 7175 /** 7176 * A list of implementation guides that the server does (or should) support in their entirety. 7177 */ 7178 @Child(name = "implementationGuide", type = {CanonicalType.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7179 @Description(shortDefinition="Implementation guides supported", formalDefinition="A list of implementation guides that the server does (or should) support in their entirety." ) 7180 protected List<CanonicalType> implementationGuide; 7181 7182 /** 7183 * A definition of the restful capabilities of the solution, if any. 7184 */ 7185 @Child(name = "rest", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7186 @Description(shortDefinition="If the endpoint is a RESTful one", formalDefinition="A definition of the restful capabilities of the solution, if any." ) 7187 protected List<CapabilityStatementRestComponent> rest; 7188 7189 /** 7190 * A description of the messaging capabilities of the solution. 7191 */ 7192 @Child(name = "messaging", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7193 @Description(shortDefinition="If messaging is supported", formalDefinition="A description of the messaging capabilities of the solution." ) 7194 protected List<CapabilityStatementMessagingComponent> messaging; 7195 7196 /** 7197 * A document definition. 7198 */ 7199 @Child(name = "document", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7200 @Description(shortDefinition="Document definition", formalDefinition="A document definition." ) 7201 protected List<CapabilityStatementDocumentComponent> document; 7202 7203 private static final long serialVersionUID = -1432396321L; 7204 7205 /** 7206 * Constructor 7207 */ 7208 public CapabilityStatement() { 7209 super(); 7210 } 7211 7212 /** 7213 * Constructor 7214 */ 7215 public CapabilityStatement(PublicationStatus status, Date date, CapabilityStatementKind kind, FHIRVersion fhirVersion, String format) { 7216 super(); 7217 this.setStatus(status); 7218 this.setDate(date); 7219 this.setKind(kind); 7220 this.setFhirVersion(fhirVersion); 7221 this.addFormat(format); 7222 } 7223 7224 /** 7225 * @return {@link #url} (An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 7226 */ 7227 public UriType getUrlElement() { 7228 if (this.url == null) 7229 if (Configuration.errorOnAutoCreate()) 7230 throw new Error("Attempt to auto-create CapabilityStatement.url"); 7231 else if (Configuration.doAutoCreate()) 7232 this.url = new UriType(); // bb 7233 return this.url; 7234 } 7235 7236 public boolean hasUrlElement() { 7237 return this.url != null && !this.url.isEmpty(); 7238 } 7239 7240 public boolean hasUrl() { 7241 return this.url != null && !this.url.isEmpty(); 7242 } 7243 7244 /** 7245 * @param value {@link #url} (An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 7246 */ 7247 public CapabilityStatement setUrlElement(UriType value) { 7248 this.url = value; 7249 return this; 7250 } 7251 7252 /** 7253 * @return An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers. 7254 */ 7255 public String getUrl() { 7256 return this.url == null ? null : this.url.getValue(); 7257 } 7258 7259 /** 7260 * @param value An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers. 7261 */ 7262 public CapabilityStatement setUrl(String value) { 7263 if (Utilities.noString(value)) 7264 this.url = null; 7265 else { 7266 if (this.url == null) 7267 this.url = new UriType(); 7268 this.url.setValue(value); 7269 } 7270 return this; 7271 } 7272 7273 /** 7274 * @return {@link #identifier} (A formal identifier that is used to identify this CapabilityStatement when it is represented in other formats, or referenced in a specification, model, design or an instance.) 7275 */ 7276 public List<Identifier> getIdentifier() { 7277 if (this.identifier == null) 7278 this.identifier = new ArrayList<Identifier>(); 7279 return this.identifier; 7280 } 7281 7282 /** 7283 * @return Returns a reference to <code>this</code> for easy method chaining 7284 */ 7285 public CapabilityStatement setIdentifier(List<Identifier> theIdentifier) { 7286 this.identifier = theIdentifier; 7287 return this; 7288 } 7289 7290 public boolean hasIdentifier() { 7291 if (this.identifier == null) 7292 return false; 7293 for (Identifier item : this.identifier) 7294 if (!item.isEmpty()) 7295 return true; 7296 return false; 7297 } 7298 7299 public Identifier addIdentifier() { //3 7300 Identifier t = new Identifier(); 7301 if (this.identifier == null) 7302 this.identifier = new ArrayList<Identifier>(); 7303 this.identifier.add(t); 7304 return t; 7305 } 7306 7307 public CapabilityStatement addIdentifier(Identifier t) { //3 7308 if (t == null) 7309 return this; 7310 if (this.identifier == null) 7311 this.identifier = new ArrayList<Identifier>(); 7312 this.identifier.add(t); 7313 return this; 7314 } 7315 7316 /** 7317 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 7318 */ 7319 public Identifier getIdentifierFirstRep() { 7320 if (getIdentifier().isEmpty()) { 7321 addIdentifier(); 7322 } 7323 return getIdentifier().get(0); 7324 } 7325 7326 /** 7327 * @return {@link #version} (The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 7328 */ 7329 public StringType getVersionElement() { 7330 if (this.version == null) 7331 if (Configuration.errorOnAutoCreate()) 7332 throw new Error("Attempt to auto-create CapabilityStatement.version"); 7333 else if (Configuration.doAutoCreate()) 7334 this.version = new StringType(); // bb 7335 return this.version; 7336 } 7337 7338 public boolean hasVersionElement() { 7339 return this.version != null && !this.version.isEmpty(); 7340 } 7341 7342 public boolean hasVersion() { 7343 return this.version != null && !this.version.isEmpty(); 7344 } 7345 7346 /** 7347 * @param value {@link #version} (The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 7348 */ 7349 public CapabilityStatement setVersionElement(StringType value) { 7350 this.version = value; 7351 return this; 7352 } 7353 7354 /** 7355 * @return The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 7356 */ 7357 public String getVersion() { 7358 return this.version == null ? null : this.version.getValue(); 7359 } 7360 7361 /** 7362 * @param value The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 7363 */ 7364 public CapabilityStatement setVersion(String value) { 7365 if (Utilities.noString(value)) 7366 this.version = null; 7367 else { 7368 if (this.version == null) 7369 this.version = new StringType(); 7370 this.version.setValue(value); 7371 } 7372 return this; 7373 } 7374 7375 /** 7376 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 7377 */ 7378 public DataType getVersionAlgorithm() { 7379 return this.versionAlgorithm; 7380 } 7381 7382 /** 7383 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 7384 */ 7385 public StringType getVersionAlgorithmStringType() throws FHIRException { 7386 if (this.versionAlgorithm == null) 7387 this.versionAlgorithm = new StringType(); 7388 if (!(this.versionAlgorithm instanceof StringType)) 7389 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 7390 return (StringType) this.versionAlgorithm; 7391 } 7392 7393 public boolean hasVersionAlgorithmStringType() { 7394 return this != null && this.versionAlgorithm instanceof StringType; 7395 } 7396 7397 /** 7398 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 7399 */ 7400 public Coding getVersionAlgorithmCoding() throws FHIRException { 7401 if (this.versionAlgorithm == null) 7402 this.versionAlgorithm = new Coding(); 7403 if (!(this.versionAlgorithm instanceof Coding)) 7404 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 7405 return (Coding) this.versionAlgorithm; 7406 } 7407 7408 public boolean hasVersionAlgorithmCoding() { 7409 return this != null && this.versionAlgorithm instanceof Coding; 7410 } 7411 7412 public boolean hasVersionAlgorithm() { 7413 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 7414 } 7415 7416 /** 7417 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 7418 */ 7419 public CapabilityStatement setVersionAlgorithm(DataType value) { 7420 if (value != null && !(value instanceof StringType || value instanceof Coding)) 7421 throw new FHIRException("Not the right type for CapabilityStatement.versionAlgorithm[x]: "+value.fhirType()); 7422 this.versionAlgorithm = value; 7423 return this; 7424 } 7425 7426 /** 7427 * @return {@link #name} (A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 7428 */ 7429 public StringType getNameElement() { 7430 if (this.name == null) 7431 if (Configuration.errorOnAutoCreate()) 7432 throw new Error("Attempt to auto-create CapabilityStatement.name"); 7433 else if (Configuration.doAutoCreate()) 7434 this.name = new StringType(); // bb 7435 return this.name; 7436 } 7437 7438 public boolean hasNameElement() { 7439 return this.name != null && !this.name.isEmpty(); 7440 } 7441 7442 public boolean hasName() { 7443 return this.name != null && !this.name.isEmpty(); 7444 } 7445 7446 /** 7447 * @param value {@link #name} (A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 7448 */ 7449 public CapabilityStatement setNameElement(StringType value) { 7450 this.name = value; 7451 return this; 7452 } 7453 7454 /** 7455 * @return A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation. 7456 */ 7457 public String getName() { 7458 return this.name == null ? null : this.name.getValue(); 7459 } 7460 7461 /** 7462 * @param value A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation. 7463 */ 7464 public CapabilityStatement setName(String value) { 7465 if (Utilities.noString(value)) 7466 this.name = null; 7467 else { 7468 if (this.name == null) 7469 this.name = new StringType(); 7470 this.name.setValue(value); 7471 } 7472 return this; 7473 } 7474 7475 /** 7476 * @return {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 7477 */ 7478 public StringType getTitleElement() { 7479 if (this.title == null) 7480 if (Configuration.errorOnAutoCreate()) 7481 throw new Error("Attempt to auto-create CapabilityStatement.title"); 7482 else if (Configuration.doAutoCreate()) 7483 this.title = new StringType(); // bb 7484 return this.title; 7485 } 7486 7487 public boolean hasTitleElement() { 7488 return this.title != null && !this.title.isEmpty(); 7489 } 7490 7491 public boolean hasTitle() { 7492 return this.title != null && !this.title.isEmpty(); 7493 } 7494 7495 /** 7496 * @param value {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 7497 */ 7498 public CapabilityStatement setTitleElement(StringType value) { 7499 this.title = value; 7500 return this; 7501 } 7502 7503 /** 7504 * @return A short, descriptive, user-friendly title for the capability statement. 7505 */ 7506 public String getTitle() { 7507 return this.title == null ? null : this.title.getValue(); 7508 } 7509 7510 /** 7511 * @param value A short, descriptive, user-friendly title for the capability statement. 7512 */ 7513 public CapabilityStatement setTitle(String value) { 7514 if (Utilities.noString(value)) 7515 this.title = null; 7516 else { 7517 if (this.title == null) 7518 this.title = new StringType(); 7519 this.title.setValue(value); 7520 } 7521 return this; 7522 } 7523 7524 /** 7525 * @return {@link #status} (The status of this capability statement. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 7526 */ 7527 public Enumeration<PublicationStatus> getStatusElement() { 7528 if (this.status == null) 7529 if (Configuration.errorOnAutoCreate()) 7530 throw new Error("Attempt to auto-create CapabilityStatement.status"); 7531 else if (Configuration.doAutoCreate()) 7532 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 7533 return this.status; 7534 } 7535 7536 public boolean hasStatusElement() { 7537 return this.status != null && !this.status.isEmpty(); 7538 } 7539 7540 public boolean hasStatus() { 7541 return this.status != null && !this.status.isEmpty(); 7542 } 7543 7544 /** 7545 * @param value {@link #status} (The status of this capability statement. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 7546 */ 7547 public CapabilityStatement setStatusElement(Enumeration<PublicationStatus> value) { 7548 this.status = value; 7549 return this; 7550 } 7551 7552 /** 7553 * @return The status of this capability statement. Enables tracking the life-cycle of the content. 7554 */ 7555 public PublicationStatus getStatus() { 7556 return this.status == null ? null : this.status.getValue(); 7557 } 7558 7559 /** 7560 * @param value The status of this capability statement. Enables tracking the life-cycle of the content. 7561 */ 7562 public CapabilityStatement setStatus(PublicationStatus value) { 7563 if (this.status == null) 7564 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 7565 this.status.setValue(value); 7566 return this; 7567 } 7568 7569 /** 7570 * @return {@link #experimental} (A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 7571 */ 7572 public BooleanType getExperimentalElement() { 7573 if (this.experimental == null) 7574 if (Configuration.errorOnAutoCreate()) 7575 throw new Error("Attempt to auto-create CapabilityStatement.experimental"); 7576 else if (Configuration.doAutoCreate()) 7577 this.experimental = new BooleanType(); // bb 7578 return this.experimental; 7579 } 7580 7581 public boolean hasExperimentalElement() { 7582 return this.experimental != null && !this.experimental.isEmpty(); 7583 } 7584 7585 public boolean hasExperimental() { 7586 return this.experimental != null && !this.experimental.isEmpty(); 7587 } 7588 7589 /** 7590 * @param value {@link #experimental} (A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 7591 */ 7592 public CapabilityStatement setExperimentalElement(BooleanType value) { 7593 this.experimental = value; 7594 return this; 7595 } 7596 7597 /** 7598 * @return A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 7599 */ 7600 public boolean getExperimental() { 7601 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7602 } 7603 7604 /** 7605 * @param value A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 7606 */ 7607 public CapabilityStatement setExperimental(boolean value) { 7608 if (this.experimental == null) 7609 this.experimental = new BooleanType(); 7610 this.experimental.setValue(value); 7611 return this; 7612 } 7613 7614 /** 7615 * @return {@link #date} (The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 7616 */ 7617 public DateTimeType getDateElement() { 7618 if (this.date == null) 7619 if (Configuration.errorOnAutoCreate()) 7620 throw new Error("Attempt to auto-create CapabilityStatement.date"); 7621 else if (Configuration.doAutoCreate()) 7622 this.date = new DateTimeType(); // bb 7623 return this.date; 7624 } 7625 7626 public boolean hasDateElement() { 7627 return this.date != null && !this.date.isEmpty(); 7628 } 7629 7630 public boolean hasDate() { 7631 return this.date != null && !this.date.isEmpty(); 7632 } 7633 7634 /** 7635 * @param value {@link #date} (The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 7636 */ 7637 public CapabilityStatement setDateElement(DateTimeType value) { 7638 this.date = value; 7639 return this; 7640 } 7641 7642 /** 7643 * @return The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes. 7644 */ 7645 public Date getDate() { 7646 return this.date == null ? null : this.date.getValue(); 7647 } 7648 7649 /** 7650 * @param value The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes. 7651 */ 7652 public CapabilityStatement setDate(Date value) { 7653 if (this.date == null) 7654 this.date = new DateTimeType(); 7655 this.date.setValue(value); 7656 return this; 7657 } 7658 7659 /** 7660 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 7661 */ 7662 public StringType getPublisherElement() { 7663 if (this.publisher == null) 7664 if (Configuration.errorOnAutoCreate()) 7665 throw new Error("Attempt to auto-create CapabilityStatement.publisher"); 7666 else if (Configuration.doAutoCreate()) 7667 this.publisher = new StringType(); // bb 7668 return this.publisher; 7669 } 7670 7671 public boolean hasPublisherElement() { 7672 return this.publisher != null && !this.publisher.isEmpty(); 7673 } 7674 7675 public boolean hasPublisher() { 7676 return this.publisher != null && !this.publisher.isEmpty(); 7677 } 7678 7679 /** 7680 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 7681 */ 7682 public CapabilityStatement setPublisherElement(StringType value) { 7683 this.publisher = value; 7684 return this; 7685 } 7686 7687 /** 7688 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement. 7689 */ 7690 public String getPublisher() { 7691 return this.publisher == null ? null : this.publisher.getValue(); 7692 } 7693 7694 /** 7695 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement. 7696 */ 7697 public CapabilityStatement setPublisher(String value) { 7698 if (Utilities.noString(value)) 7699 this.publisher = null; 7700 else { 7701 if (this.publisher == null) 7702 this.publisher = new StringType(); 7703 this.publisher.setValue(value); 7704 } 7705 return this; 7706 } 7707 7708 /** 7709 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 7710 */ 7711 public List<ContactDetail> getContact() { 7712 if (this.contact == null) 7713 this.contact = new ArrayList<ContactDetail>(); 7714 return this.contact; 7715 } 7716 7717 /** 7718 * @return Returns a reference to <code>this</code> for easy method chaining 7719 */ 7720 public CapabilityStatement setContact(List<ContactDetail> theContact) { 7721 this.contact = theContact; 7722 return this; 7723 } 7724 7725 public boolean hasContact() { 7726 if (this.contact == null) 7727 return false; 7728 for (ContactDetail item : this.contact) 7729 if (!item.isEmpty()) 7730 return true; 7731 return false; 7732 } 7733 7734 public ContactDetail addContact() { //3 7735 ContactDetail t = new ContactDetail(); 7736 if (this.contact == null) 7737 this.contact = new ArrayList<ContactDetail>(); 7738 this.contact.add(t); 7739 return t; 7740 } 7741 7742 public CapabilityStatement addContact(ContactDetail t) { //3 7743 if (t == null) 7744 return this; 7745 if (this.contact == null) 7746 this.contact = new ArrayList<ContactDetail>(); 7747 this.contact.add(t); 7748 return this; 7749 } 7750 7751 /** 7752 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 7753 */ 7754 public ContactDetail getContactFirstRep() { 7755 if (getContact().isEmpty()) { 7756 addContact(); 7757 } 7758 return getContact().get(0); 7759 } 7760 7761 /** 7762 * @return {@link #description} (A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 7763 */ 7764 public MarkdownType getDescriptionElement() { 7765 if (this.description == null) 7766 if (Configuration.errorOnAutoCreate()) 7767 throw new Error("Attempt to auto-create CapabilityStatement.description"); 7768 else if (Configuration.doAutoCreate()) 7769 this.description = new MarkdownType(); // bb 7770 return this.description; 7771 } 7772 7773 public boolean hasDescriptionElement() { 7774 return this.description != null && !this.description.isEmpty(); 7775 } 7776 7777 public boolean hasDescription() { 7778 return this.description != null && !this.description.isEmpty(); 7779 } 7780 7781 /** 7782 * @param value {@link #description} (A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 7783 */ 7784 public CapabilityStatement setDescriptionElement(MarkdownType value) { 7785 this.description = value; 7786 return this; 7787 } 7788 7789 /** 7790 * @return A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 7791 */ 7792 public String getDescription() { 7793 return this.description == null ? null : this.description.getValue(); 7794 } 7795 7796 /** 7797 * @param value A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 7798 */ 7799 public CapabilityStatement setDescription(String value) { 7800 if (Utilities.noString(value)) 7801 this.description = null; 7802 else { 7803 if (this.description == null) 7804 this.description = new MarkdownType(); 7805 this.description.setValue(value); 7806 } 7807 return this; 7808 } 7809 7810 /** 7811 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.) 7812 */ 7813 public List<UsageContext> getUseContext() { 7814 if (this.useContext == null) 7815 this.useContext = new ArrayList<UsageContext>(); 7816 return this.useContext; 7817 } 7818 7819 /** 7820 * @return Returns a reference to <code>this</code> for easy method chaining 7821 */ 7822 public CapabilityStatement setUseContext(List<UsageContext> theUseContext) { 7823 this.useContext = theUseContext; 7824 return this; 7825 } 7826 7827 public boolean hasUseContext() { 7828 if (this.useContext == null) 7829 return false; 7830 for (UsageContext item : this.useContext) 7831 if (!item.isEmpty()) 7832 return true; 7833 return false; 7834 } 7835 7836 public UsageContext addUseContext() { //3 7837 UsageContext t = new UsageContext(); 7838 if (this.useContext == null) 7839 this.useContext = new ArrayList<UsageContext>(); 7840 this.useContext.add(t); 7841 return t; 7842 } 7843 7844 public CapabilityStatement addUseContext(UsageContext t) { //3 7845 if (t == null) 7846 return this; 7847 if (this.useContext == null) 7848 this.useContext = new ArrayList<UsageContext>(); 7849 this.useContext.add(t); 7850 return this; 7851 } 7852 7853 /** 7854 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 7855 */ 7856 public UsageContext getUseContextFirstRep() { 7857 if (getUseContext().isEmpty()) { 7858 addUseContext(); 7859 } 7860 return getUseContext().get(0); 7861 } 7862 7863 /** 7864 * @return {@link #jurisdiction} (A legal or geographic region in which the capability statement is intended to be used.) 7865 */ 7866 public List<CodeableConcept> getJurisdiction() { 7867 if (this.jurisdiction == null) 7868 this.jurisdiction = new ArrayList<CodeableConcept>(); 7869 return this.jurisdiction; 7870 } 7871 7872 /** 7873 * @return Returns a reference to <code>this</code> for easy method chaining 7874 */ 7875 public CapabilityStatement setJurisdiction(List<CodeableConcept> theJurisdiction) { 7876 this.jurisdiction = theJurisdiction; 7877 return this; 7878 } 7879 7880 public boolean hasJurisdiction() { 7881 if (this.jurisdiction == null) 7882 return false; 7883 for (CodeableConcept item : this.jurisdiction) 7884 if (!item.isEmpty()) 7885 return true; 7886 return false; 7887 } 7888 7889 public CodeableConcept addJurisdiction() { //3 7890 CodeableConcept t = new CodeableConcept(); 7891 if (this.jurisdiction == null) 7892 this.jurisdiction = new ArrayList<CodeableConcept>(); 7893 this.jurisdiction.add(t); 7894 return t; 7895 } 7896 7897 public CapabilityStatement addJurisdiction(CodeableConcept t) { //3 7898 if (t == null) 7899 return this; 7900 if (this.jurisdiction == null) 7901 this.jurisdiction = new ArrayList<CodeableConcept>(); 7902 this.jurisdiction.add(t); 7903 return this; 7904 } 7905 7906 /** 7907 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 7908 */ 7909 public CodeableConcept getJurisdictionFirstRep() { 7910 if (getJurisdiction().isEmpty()) { 7911 addJurisdiction(); 7912 } 7913 return getJurisdiction().get(0); 7914 } 7915 7916 /** 7917 * @return {@link #purpose} (Explanation of why this capability statement is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 7918 */ 7919 public MarkdownType getPurposeElement() { 7920 if (this.purpose == null) 7921 if (Configuration.errorOnAutoCreate()) 7922 throw new Error("Attempt to auto-create CapabilityStatement.purpose"); 7923 else if (Configuration.doAutoCreate()) 7924 this.purpose = new MarkdownType(); // bb 7925 return this.purpose; 7926 } 7927 7928 public boolean hasPurposeElement() { 7929 return this.purpose != null && !this.purpose.isEmpty(); 7930 } 7931 7932 public boolean hasPurpose() { 7933 return this.purpose != null && !this.purpose.isEmpty(); 7934 } 7935 7936 /** 7937 * @param value {@link #purpose} (Explanation of why this capability statement is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 7938 */ 7939 public CapabilityStatement setPurposeElement(MarkdownType value) { 7940 this.purpose = value; 7941 return this; 7942 } 7943 7944 /** 7945 * @return Explanation of why this capability statement is needed and why it has been designed as it has. 7946 */ 7947 public String getPurpose() { 7948 return this.purpose == null ? null : this.purpose.getValue(); 7949 } 7950 7951 /** 7952 * @param value Explanation of why this capability statement is needed and why it has been designed as it has. 7953 */ 7954 public CapabilityStatement setPurpose(String value) { 7955 if (Utilities.noString(value)) 7956 this.purpose = null; 7957 else { 7958 if (this.purpose == null) 7959 this.purpose = new MarkdownType(); 7960 this.purpose.setValue(value); 7961 } 7962 return this; 7963 } 7964 7965 /** 7966 * @return {@link #copyright} (A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 7967 */ 7968 public MarkdownType getCopyrightElement() { 7969 if (this.copyright == null) 7970 if (Configuration.errorOnAutoCreate()) 7971 throw new Error("Attempt to auto-create CapabilityStatement.copyright"); 7972 else if (Configuration.doAutoCreate()) 7973 this.copyright = new MarkdownType(); // bb 7974 return this.copyright; 7975 } 7976 7977 public boolean hasCopyrightElement() { 7978 return this.copyright != null && !this.copyright.isEmpty(); 7979 } 7980 7981 public boolean hasCopyright() { 7982 return this.copyright != null && !this.copyright.isEmpty(); 7983 } 7984 7985 /** 7986 * @param value {@link #copyright} (A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 7987 */ 7988 public CapabilityStatement setCopyrightElement(MarkdownType value) { 7989 this.copyright = value; 7990 return this; 7991 } 7992 7993 /** 7994 * @return A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement. 7995 */ 7996 public String getCopyright() { 7997 return this.copyright == null ? null : this.copyright.getValue(); 7998 } 7999 8000 /** 8001 * @param value A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement. 8002 */ 8003 public CapabilityStatement setCopyright(String value) { 8004 if (Utilities.noString(value)) 8005 this.copyright = null; 8006 else { 8007 if (this.copyright == null) 8008 this.copyright = new MarkdownType(); 8009 this.copyright.setValue(value); 8010 } 8011 return this; 8012 } 8013 8014 /** 8015 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 8016 */ 8017 public StringType getCopyrightLabelElement() { 8018 if (this.copyrightLabel == null) 8019 if (Configuration.errorOnAutoCreate()) 8020 throw new Error("Attempt to auto-create CapabilityStatement.copyrightLabel"); 8021 else if (Configuration.doAutoCreate()) 8022 this.copyrightLabel = new StringType(); // bb 8023 return this.copyrightLabel; 8024 } 8025 8026 public boolean hasCopyrightLabelElement() { 8027 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 8028 } 8029 8030 public boolean hasCopyrightLabel() { 8031 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 8032 } 8033 8034 /** 8035 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 8036 */ 8037 public CapabilityStatement setCopyrightLabelElement(StringType value) { 8038 this.copyrightLabel = value; 8039 return this; 8040 } 8041 8042 /** 8043 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 8044 */ 8045 public String getCopyrightLabel() { 8046 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 8047 } 8048 8049 /** 8050 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 8051 */ 8052 public CapabilityStatement setCopyrightLabel(String value) { 8053 if (Utilities.noString(value)) 8054 this.copyrightLabel = null; 8055 else { 8056 if (this.copyrightLabel == null) 8057 this.copyrightLabel = new StringType(); 8058 this.copyrightLabel.setValue(value); 8059 } 8060 return this; 8061 } 8062 8063 /** 8064 * @return {@link #kind} (The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 8065 */ 8066 public Enumeration<CapabilityStatementKind> getKindElement() { 8067 if (this.kind == null) 8068 if (Configuration.errorOnAutoCreate()) 8069 throw new Error("Attempt to auto-create CapabilityStatement.kind"); 8070 else if (Configuration.doAutoCreate()) 8071 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); // bb 8072 return this.kind; 8073 } 8074 8075 public boolean hasKindElement() { 8076 return this.kind != null && !this.kind.isEmpty(); 8077 } 8078 8079 public boolean hasKind() { 8080 return this.kind != null && !this.kind.isEmpty(); 8081 } 8082 8083 /** 8084 * @param value {@link #kind} (The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 8085 */ 8086 public CapabilityStatement setKindElement(Enumeration<CapabilityStatementKind> value) { 8087 this.kind = value; 8088 return this; 8089 } 8090 8091 /** 8092 * @return The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase). 8093 */ 8094 public CapabilityStatementKind getKind() { 8095 return this.kind == null ? null : this.kind.getValue(); 8096 } 8097 8098 /** 8099 * @param value The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase). 8100 */ 8101 public CapabilityStatement setKind(CapabilityStatementKind value) { 8102 if (this.kind == null) 8103 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); 8104 this.kind.setValue(value); 8105 return this; 8106 } 8107 8108 /** 8109 * @return {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.) 8110 */ 8111 public List<CanonicalType> getInstantiates() { 8112 if (this.instantiates == null) 8113 this.instantiates = new ArrayList<CanonicalType>(); 8114 return this.instantiates; 8115 } 8116 8117 /** 8118 * @return Returns a reference to <code>this</code> for easy method chaining 8119 */ 8120 public CapabilityStatement setInstantiates(List<CanonicalType> theInstantiates) { 8121 this.instantiates = theInstantiates; 8122 return this; 8123 } 8124 8125 public boolean hasInstantiates() { 8126 if (this.instantiates == null) 8127 return false; 8128 for (CanonicalType item : this.instantiates) 8129 if (!item.isEmpty()) 8130 return true; 8131 return false; 8132 } 8133 8134 /** 8135 * @return {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.) 8136 */ 8137 public CanonicalType addInstantiatesElement() {//2 8138 CanonicalType t = new CanonicalType(); 8139 if (this.instantiates == null) 8140 this.instantiates = new ArrayList<CanonicalType>(); 8141 this.instantiates.add(t); 8142 return t; 8143 } 8144 8145 /** 8146 * @param value {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.) 8147 */ 8148 public CapabilityStatement addInstantiates(String value) { //1 8149 CanonicalType t = new CanonicalType(); 8150 t.setValue(value); 8151 if (this.instantiates == null) 8152 this.instantiates = new ArrayList<CanonicalType>(); 8153 this.instantiates.add(t); 8154 return this; 8155 } 8156 8157 /** 8158 * @param value {@link #instantiates} (Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.) 8159 */ 8160 public boolean hasInstantiates(String value) { 8161 if (this.instantiates == null) 8162 return false; 8163 for (CanonicalType v : this.instantiates) 8164 if (v.getValue().equals(value)) // canonical 8165 return true; 8166 return false; 8167 } 8168 8169 /** 8170 * @return {@link #imports} (Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.) 8171 */ 8172 public List<CanonicalType> getImports() { 8173 if (this.imports == null) 8174 this.imports = new ArrayList<CanonicalType>(); 8175 return this.imports; 8176 } 8177 8178 /** 8179 * @return Returns a reference to <code>this</code> for easy method chaining 8180 */ 8181 public CapabilityStatement setImports(List<CanonicalType> theImports) { 8182 this.imports = theImports; 8183 return this; 8184 } 8185 8186 public boolean hasImports() { 8187 if (this.imports == null) 8188 return false; 8189 for (CanonicalType item : this.imports) 8190 if (!item.isEmpty()) 8191 return true; 8192 return false; 8193 } 8194 8195 /** 8196 * @return {@link #imports} (Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.) 8197 */ 8198 public CanonicalType addImportsElement() {//2 8199 CanonicalType t = new CanonicalType(); 8200 if (this.imports == null) 8201 this.imports = new ArrayList<CanonicalType>(); 8202 this.imports.add(t); 8203 return t; 8204 } 8205 8206 /** 8207 * @param value {@link #imports} (Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.) 8208 */ 8209 public CapabilityStatement addImports(String value) { //1 8210 CanonicalType t = new CanonicalType(); 8211 t.setValue(value); 8212 if (this.imports == null) 8213 this.imports = new ArrayList<CanonicalType>(); 8214 this.imports.add(t); 8215 return this; 8216 } 8217 8218 /** 8219 * @param value {@link #imports} (Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.) 8220 */ 8221 public boolean hasImports(String value) { 8222 if (this.imports == null) 8223 return false; 8224 for (CanonicalType v : this.imports) 8225 if (v.getValue().equals(value)) // canonical 8226 return true; 8227 return false; 8228 } 8229 8230 /** 8231 * @return {@link #software} (Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.) 8232 */ 8233 public CapabilityStatementSoftwareComponent getSoftware() { 8234 if (this.software == null) 8235 if (Configuration.errorOnAutoCreate()) 8236 throw new Error("Attempt to auto-create CapabilityStatement.software"); 8237 else if (Configuration.doAutoCreate()) 8238 this.software = new CapabilityStatementSoftwareComponent(); // cc 8239 return this.software; 8240 } 8241 8242 public boolean hasSoftware() { 8243 return this.software != null && !this.software.isEmpty(); 8244 } 8245 8246 /** 8247 * @param value {@link #software} (Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.) 8248 */ 8249 public CapabilityStatement setSoftware(CapabilityStatementSoftwareComponent value) { 8250 this.software = value; 8251 return this; 8252 } 8253 8254 /** 8255 * @return {@link #implementation} (Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.) 8256 */ 8257 public CapabilityStatementImplementationComponent getImplementation() { 8258 if (this.implementation == null) 8259 if (Configuration.errorOnAutoCreate()) 8260 throw new Error("Attempt to auto-create CapabilityStatement.implementation"); 8261 else if (Configuration.doAutoCreate()) 8262 this.implementation = new CapabilityStatementImplementationComponent(); // cc 8263 return this.implementation; 8264 } 8265 8266 public boolean hasImplementation() { 8267 return this.implementation != null && !this.implementation.isEmpty(); 8268 } 8269 8270 /** 8271 * @param value {@link #implementation} (Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.) 8272 */ 8273 public CapabilityStatement setImplementation(CapabilityStatementImplementationComponent value) { 8274 this.implementation = value; 8275 return this; 8276 } 8277 8278 /** 8279 * @return {@link #fhirVersion} (The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 8280 */ 8281 public Enumeration<FHIRVersion> getFhirVersionElement() { 8282 if (this.fhirVersion == null) 8283 if (Configuration.errorOnAutoCreate()) 8284 throw new Error("Attempt to auto-create CapabilityStatement.fhirVersion"); 8285 else if (Configuration.doAutoCreate()) 8286 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); // bb 8287 return this.fhirVersion; 8288 } 8289 8290 public boolean hasFhirVersionElement() { 8291 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8292 } 8293 8294 public boolean hasFhirVersion() { 8295 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 8296 } 8297 8298 /** 8299 * @param value {@link #fhirVersion} (The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 8300 */ 8301 public CapabilityStatement setFhirVersionElement(Enumeration<FHIRVersion> value) { 8302 this.fhirVersion = value; 8303 return this; 8304 } 8305 8306 /** 8307 * @return The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value. 8308 */ 8309 public FHIRVersion getFhirVersion() { 8310 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 8311 } 8312 8313 /** 8314 * @param value The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value. 8315 */ 8316 public CapabilityStatement setFhirVersion(FHIRVersion value) { 8317 if (this.fhirVersion == null) 8318 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 8319 this.fhirVersion.setValue(value); 8320 return this; 8321 } 8322 8323 /** 8324 * @return {@link #format} (A list of the formats supported by this implementation using their content types.) 8325 */ 8326 public List<CodeType> getFormat() { 8327 if (this.format == null) 8328 this.format = new ArrayList<CodeType>(); 8329 return this.format; 8330 } 8331 8332 /** 8333 * @return Returns a reference to <code>this</code> for easy method chaining 8334 */ 8335 public CapabilityStatement setFormat(List<CodeType> theFormat) { 8336 this.format = theFormat; 8337 return this; 8338 } 8339 8340 public boolean hasFormat() { 8341 if (this.format == null) 8342 return false; 8343 for (CodeType item : this.format) 8344 if (!item.isEmpty()) 8345 return true; 8346 return false; 8347 } 8348 8349 /** 8350 * @return {@link #format} (A list of the formats supported by this implementation using their content types.) 8351 */ 8352 public CodeType addFormatElement() {//2 8353 CodeType t = new CodeType(); 8354 if (this.format == null) 8355 this.format = new ArrayList<CodeType>(); 8356 this.format.add(t); 8357 return t; 8358 } 8359 8360 /** 8361 * @param value {@link #format} (A list of the formats supported by this implementation using their content types.) 8362 */ 8363 public CapabilityStatement addFormat(String value) { //1 8364 CodeType t = new CodeType(); 8365 t.setValue(value); 8366 if (this.format == null) 8367 this.format = new ArrayList<CodeType>(); 8368 this.format.add(t); 8369 return this; 8370 } 8371 8372 /** 8373 * @param value {@link #format} (A list of the formats supported by this implementation using their content types.) 8374 */ 8375 public boolean hasFormat(String value) { 8376 if (this.format == null) 8377 return false; 8378 for (CodeType v : this.format) 8379 if (v.getValue().equals(value)) // code 8380 return true; 8381 return false; 8382 } 8383 8384 /** 8385 * @return {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8386 */ 8387 public List<CodeType> getPatchFormat() { 8388 if (this.patchFormat == null) 8389 this.patchFormat = new ArrayList<CodeType>(); 8390 return this.patchFormat; 8391 } 8392 8393 /** 8394 * @return Returns a reference to <code>this</code> for easy method chaining 8395 */ 8396 public CapabilityStatement setPatchFormat(List<CodeType> thePatchFormat) { 8397 this.patchFormat = thePatchFormat; 8398 return this; 8399 } 8400 8401 public boolean hasPatchFormat() { 8402 if (this.patchFormat == null) 8403 return false; 8404 for (CodeType item : this.patchFormat) 8405 if (!item.isEmpty()) 8406 return true; 8407 return false; 8408 } 8409 8410 /** 8411 * @return {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8412 */ 8413 public CodeType addPatchFormatElement() {//2 8414 CodeType t = new CodeType(); 8415 if (this.patchFormat == null) 8416 this.patchFormat = new ArrayList<CodeType>(); 8417 this.patchFormat.add(t); 8418 return t; 8419 } 8420 8421 /** 8422 * @param value {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8423 */ 8424 public CapabilityStatement addPatchFormat(String value) { //1 8425 CodeType t = new CodeType(); 8426 t.setValue(value); 8427 if (this.patchFormat == null) 8428 this.patchFormat = new ArrayList<CodeType>(); 8429 this.patchFormat.add(t); 8430 return this; 8431 } 8432 8433 /** 8434 * @param value {@link #patchFormat} (A list of the patch formats supported by this implementation using their content types.) 8435 */ 8436 public boolean hasPatchFormat(String value) { 8437 if (this.patchFormat == null) 8438 return false; 8439 for (CodeType v : this.patchFormat) 8440 if (v.getValue().equals(value)) // code 8441 return true; 8442 return false; 8443 } 8444 8445 /** 8446 * @return {@link #acceptLanguage} (A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header.) 8447 */ 8448 public List<CodeType> getAcceptLanguage() { 8449 if (this.acceptLanguage == null) 8450 this.acceptLanguage = new ArrayList<CodeType>(); 8451 return this.acceptLanguage; 8452 } 8453 8454 /** 8455 * @return Returns a reference to <code>this</code> for easy method chaining 8456 */ 8457 public CapabilityStatement setAcceptLanguage(List<CodeType> theAcceptLanguage) { 8458 this.acceptLanguage = theAcceptLanguage; 8459 return this; 8460 } 8461 8462 public boolean hasAcceptLanguage() { 8463 if (this.acceptLanguage == null) 8464 return false; 8465 for (CodeType item : this.acceptLanguage) 8466 if (!item.isEmpty()) 8467 return true; 8468 return false; 8469 } 8470 8471 /** 8472 * @return {@link #acceptLanguage} (A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header.) 8473 */ 8474 public CodeType addAcceptLanguageElement() {//2 8475 CodeType t = new CodeType(); 8476 if (this.acceptLanguage == null) 8477 this.acceptLanguage = new ArrayList<CodeType>(); 8478 this.acceptLanguage.add(t); 8479 return t; 8480 } 8481 8482 /** 8483 * @param value {@link #acceptLanguage} (A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header.) 8484 */ 8485 public CapabilityStatement addAcceptLanguage(String value) { //1 8486 CodeType t = new CodeType(); 8487 t.setValue(value); 8488 if (this.acceptLanguage == null) 8489 this.acceptLanguage = new ArrayList<CodeType>(); 8490 this.acceptLanguage.add(t); 8491 return this; 8492 } 8493 8494 /** 8495 * @param value {@link #acceptLanguage} (A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header.) 8496 */ 8497 public boolean hasAcceptLanguage(String value) { 8498 if (this.acceptLanguage == null) 8499 return false; 8500 for (CodeType v : this.acceptLanguage) 8501 if (v.getValue().equals(value)) // code 8502 return true; 8503 return false; 8504 } 8505 8506 /** 8507 * @return {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8508 */ 8509 public List<CanonicalType> getImplementationGuide() { 8510 if (this.implementationGuide == null) 8511 this.implementationGuide = new ArrayList<CanonicalType>(); 8512 return this.implementationGuide; 8513 } 8514 8515 /** 8516 * @return Returns a reference to <code>this</code> for easy method chaining 8517 */ 8518 public CapabilityStatement setImplementationGuide(List<CanonicalType> theImplementationGuide) { 8519 this.implementationGuide = theImplementationGuide; 8520 return this; 8521 } 8522 8523 public boolean hasImplementationGuide() { 8524 if (this.implementationGuide == null) 8525 return false; 8526 for (CanonicalType item : this.implementationGuide) 8527 if (!item.isEmpty()) 8528 return true; 8529 return false; 8530 } 8531 8532 /** 8533 * @return {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8534 */ 8535 public CanonicalType addImplementationGuideElement() {//2 8536 CanonicalType t = new CanonicalType(); 8537 if (this.implementationGuide == null) 8538 this.implementationGuide = new ArrayList<CanonicalType>(); 8539 this.implementationGuide.add(t); 8540 return t; 8541 } 8542 8543 /** 8544 * @param value {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8545 */ 8546 public CapabilityStatement addImplementationGuide(String value) { //1 8547 CanonicalType t = new CanonicalType(); 8548 t.setValue(value); 8549 if (this.implementationGuide == null) 8550 this.implementationGuide = new ArrayList<CanonicalType>(); 8551 this.implementationGuide.add(t); 8552 return this; 8553 } 8554 8555 /** 8556 * @param value {@link #implementationGuide} (A list of implementation guides that the server does (or should) support in their entirety.) 8557 */ 8558 public boolean hasImplementationGuide(String value) { 8559 if (this.implementationGuide == null) 8560 return false; 8561 for (CanonicalType v : this.implementationGuide) 8562 if (v.getValue().equals(value)) // canonical 8563 return true; 8564 return false; 8565 } 8566 8567 /** 8568 * @return {@link #rest} (A definition of the restful capabilities of the solution, if any.) 8569 */ 8570 public List<CapabilityStatementRestComponent> getRest() { 8571 if (this.rest == null) 8572 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 8573 return this.rest; 8574 } 8575 8576 /** 8577 * @return Returns a reference to <code>this</code> for easy method chaining 8578 */ 8579 public CapabilityStatement setRest(List<CapabilityStatementRestComponent> theRest) { 8580 this.rest = theRest; 8581 return this; 8582 } 8583 8584 public boolean hasRest() { 8585 if (this.rest == null) 8586 return false; 8587 for (CapabilityStatementRestComponent item : this.rest) 8588 if (!item.isEmpty()) 8589 return true; 8590 return false; 8591 } 8592 8593 public CapabilityStatementRestComponent addRest() { //3 8594 CapabilityStatementRestComponent t = new CapabilityStatementRestComponent(); 8595 if (this.rest == null) 8596 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 8597 this.rest.add(t); 8598 return t; 8599 } 8600 8601 public CapabilityStatement addRest(CapabilityStatementRestComponent t) { //3 8602 if (t == null) 8603 return this; 8604 if (this.rest == null) 8605 this.rest = new ArrayList<CapabilityStatementRestComponent>(); 8606 this.rest.add(t); 8607 return this; 8608 } 8609 8610 /** 8611 * @return The first repetition of repeating field {@link #rest}, creating it if it does not already exist {3} 8612 */ 8613 public CapabilityStatementRestComponent getRestFirstRep() { 8614 if (getRest().isEmpty()) { 8615 addRest(); 8616 } 8617 return getRest().get(0); 8618 } 8619 8620 /** 8621 * @return {@link #messaging} (A description of the messaging capabilities of the solution.) 8622 */ 8623 public List<CapabilityStatementMessagingComponent> getMessaging() { 8624 if (this.messaging == null) 8625 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 8626 return this.messaging; 8627 } 8628 8629 /** 8630 * @return Returns a reference to <code>this</code> for easy method chaining 8631 */ 8632 public CapabilityStatement setMessaging(List<CapabilityStatementMessagingComponent> theMessaging) { 8633 this.messaging = theMessaging; 8634 return this; 8635 } 8636 8637 public boolean hasMessaging() { 8638 if (this.messaging == null) 8639 return false; 8640 for (CapabilityStatementMessagingComponent item : this.messaging) 8641 if (!item.isEmpty()) 8642 return true; 8643 return false; 8644 } 8645 8646 public CapabilityStatementMessagingComponent addMessaging() { //3 8647 CapabilityStatementMessagingComponent t = new CapabilityStatementMessagingComponent(); 8648 if (this.messaging == null) 8649 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 8650 this.messaging.add(t); 8651 return t; 8652 } 8653 8654 public CapabilityStatement addMessaging(CapabilityStatementMessagingComponent t) { //3 8655 if (t == null) 8656 return this; 8657 if (this.messaging == null) 8658 this.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 8659 this.messaging.add(t); 8660 return this; 8661 } 8662 8663 /** 8664 * @return The first repetition of repeating field {@link #messaging}, creating it if it does not already exist {3} 8665 */ 8666 public CapabilityStatementMessagingComponent getMessagingFirstRep() { 8667 if (getMessaging().isEmpty()) { 8668 addMessaging(); 8669 } 8670 return getMessaging().get(0); 8671 } 8672 8673 /** 8674 * @return {@link #document} (A document definition.) 8675 */ 8676 public List<CapabilityStatementDocumentComponent> getDocument() { 8677 if (this.document == null) 8678 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 8679 return this.document; 8680 } 8681 8682 /** 8683 * @return Returns a reference to <code>this</code> for easy method chaining 8684 */ 8685 public CapabilityStatement setDocument(List<CapabilityStatementDocumentComponent> theDocument) { 8686 this.document = theDocument; 8687 return this; 8688 } 8689 8690 public boolean hasDocument() { 8691 if (this.document == null) 8692 return false; 8693 for (CapabilityStatementDocumentComponent item : this.document) 8694 if (!item.isEmpty()) 8695 return true; 8696 return false; 8697 } 8698 8699 public CapabilityStatementDocumentComponent addDocument() { //3 8700 CapabilityStatementDocumentComponent t = new CapabilityStatementDocumentComponent(); 8701 if (this.document == null) 8702 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 8703 this.document.add(t); 8704 return t; 8705 } 8706 8707 public CapabilityStatement addDocument(CapabilityStatementDocumentComponent t) { //3 8708 if (t == null) 8709 return this; 8710 if (this.document == null) 8711 this.document = new ArrayList<CapabilityStatementDocumentComponent>(); 8712 this.document.add(t); 8713 return this; 8714 } 8715 8716 /** 8717 * @return The first repetition of repeating field {@link #document}, creating it if it does not already exist {3} 8718 */ 8719 public CapabilityStatementDocumentComponent getDocumentFirstRep() { 8720 if (getDocument().isEmpty()) { 8721 addDocument(); 8722 } 8723 return getDocument().get(0); 8724 } 8725 8726 protected void listChildren(List<Property> children) { 8727 super.listChildren(children); 8728 children.add(new Property("url", "uri", "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.", 0, 1, url)); 8729 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this CapabilityStatement when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 8730 children.add(new Property("version", "string", "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 8731 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 8732 children.add(new Property("name", "string", "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 8733 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title)); 8734 children.add(new Property("status", "code", "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status)); 8735 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 8736 children.add(new Property("date", "dateTime", "The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 0, 1, date)); 8737 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement.", 0, 1, publisher)); 8738 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 8739 children.add(new Property("description", "markdown", "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 0, 1, description)); 8740 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 8741 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the capability statement is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 8742 children.add(new Property("purpose", "markdown", "Explanation of why this capability statement is needed and why it has been designed as it has.", 0, 1, purpose)); 8743 children.add(new Property("copyright", "markdown", "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 0, 1, copyright)); 8744 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 8745 children.add(new Property("kind", "code", "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 0, 1, kind)); 8746 children.add(new Property("instantiates", "canonical(CapabilityStatement)", "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.", 0, java.lang.Integer.MAX_VALUE, instantiates)); 8747 children.add(new Property("imports", "canonical(CapabilityStatement)", "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.", 0, java.lang.Integer.MAX_VALUE, imports)); 8748 children.add(new Property("software", "", "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 0, 1, software)); 8749 children.add(new Property("implementation", "", "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 0, 1, implementation)); 8750 children.add(new Property("fhirVersion", "code", "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.", 0, 1, fhirVersion)); 8751 children.add(new Property("format", "code", "A list of the formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, format)); 8752 children.add(new Property("patchFormat", "code", "A list of the patch formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, patchFormat)); 8753 children.add(new Property("acceptLanguage", "code", "A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header.", 0, java.lang.Integer.MAX_VALUE, acceptLanguage)); 8754 children.add(new Property("implementationGuide", "canonical(ImplementationGuide)", "A list of implementation guides that the server does (or should) support in their entirety.", 0, java.lang.Integer.MAX_VALUE, implementationGuide)); 8755 children.add(new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, java.lang.Integer.MAX_VALUE, rest)); 8756 children.add(new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, java.lang.Integer.MAX_VALUE, messaging)); 8757 children.add(new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document)); 8758 } 8759 8760 @Override 8761 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8762 switch (_hash) { 8763 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this capability statement when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this capability statement is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the capability statement is stored on different servers.", 0, 1, url); 8764 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this CapabilityStatement when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 8765 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the capability statement when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the capability statement author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 8766 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8767 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8768 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8769 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 8770 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the capability statement. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 8771 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title); 8772 case -892481550: /*status*/ return new Property("status", "code", "The status of this capability statement. Enables tracking the life-cycle of the content.", 0, 1, status); 8773 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this capability statement is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 8774 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the capability statement was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the capability statement changes.", 0, 1, date); 8775 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the capability statement.", 0, 1, publisher); 8776 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 8777 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the capability statement from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 0, 1, description); 8778 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate capability statement instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 8779 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the capability statement is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 8780 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this capability statement is needed and why it has been designed as it has.", 0, 1, purpose); 8781 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the capability statement and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the capability statement.", 0, 1, copyright); 8782 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 8783 case 3292052: /*kind*/ return new Property("kind", "code", "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 0, 1, kind); 8784 case -246883639: /*instantiates*/ return new Property("instantiates", "canonical(CapabilityStatement)", "Reference to a canonical URL of another CapabilityStatement that this software implements. This capability statement is a published API description that corresponds to a business service. The server may actually implement a subset of the capability statement it claims to implement, so the capability statement must specify the full capability details.", 0, java.lang.Integer.MAX_VALUE, instantiates); 8785 case 1926037870: /*imports*/ return new Property("imports", "canonical(CapabilityStatement)", "Reference to a canonical URL of another CapabilityStatement that this software adds to. The capability statement automatically includes everything in the other statement, and it is not duplicated, though the server may repeat the same resources, interactions and operations to add additional details to them.", 0, java.lang.Integer.MAX_VALUE, imports); 8786 case 1319330215: /*software*/ return new Property("software", "", "Software that is covered by this capability statement. It is used when the capability statement describes the capabilities of a particular software version, independent of an installation.", 0, 1, software); 8787 case 1683336114: /*implementation*/ return new Property("implementation", "", "Identifies a specific implementation instance that is described by the capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 0, 1, implementation); 8788 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "code", "The version of the FHIR specification that this CapabilityStatement describes (which SHALL be the same as the FHIR version of the CapabilityStatement itself). There is no default value.", 0, 1, fhirVersion); 8789 case -1268779017: /*format*/ return new Property("format", "code", "A list of the formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, format); 8790 case 172338783: /*patchFormat*/ return new Property("patchFormat", "code", "A list of the patch formats supported by this implementation using their content types.", 0, java.lang.Integer.MAX_VALUE, patchFormat); 8791 case 1014178944: /*acceptLanguage*/ return new Property("acceptLanguage", "code", "A list of the languages supported by this implementation that are usefully supported in the ```Accept-Language``` header.", 0, java.lang.Integer.MAX_VALUE, acceptLanguage); 8792 case 156966506: /*implementationGuide*/ return new Property("implementationGuide", "canonical(ImplementationGuide)", "A list of implementation guides that the server does (or should) support in their entirety.", 0, java.lang.Integer.MAX_VALUE, implementationGuide); 8793 case 3496916: /*rest*/ return new Property("rest", "", "A definition of the restful capabilities of the solution, if any.", 0, java.lang.Integer.MAX_VALUE, rest); 8794 case -1440008444: /*messaging*/ return new Property("messaging", "", "A description of the messaging capabilities of the solution.", 0, java.lang.Integer.MAX_VALUE, messaging); 8795 case 861720859: /*document*/ return new Property("document", "", "A document definition.", 0, java.lang.Integer.MAX_VALUE, document); 8796 default: return super.getNamedProperty(_hash, _name, _checkValid); 8797 } 8798 8799 } 8800 8801 @Override 8802 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8803 switch (hash) { 8804 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 8805 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8806 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 8807 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 8808 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 8809 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 8810 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 8811 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 8812 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 8813 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 8814 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 8815 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 8816 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 8817 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 8818 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 8819 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 8820 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 8821 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<CapabilityStatementKind> 8822 case -246883639: /*instantiates*/ return this.instantiates == null ? new Base[0] : this.instantiates.toArray(new Base[this.instantiates.size()]); // CanonicalType 8823 case 1926037870: /*imports*/ return this.imports == null ? new Base[0] : this.imports.toArray(new Base[this.imports.size()]); // CanonicalType 8824 case 1319330215: /*software*/ return this.software == null ? new Base[0] : new Base[] {this.software}; // CapabilityStatementSoftwareComponent 8825 case 1683336114: /*implementation*/ return this.implementation == null ? new Base[0] : new Base[] {this.implementation}; // CapabilityStatementImplementationComponent 8826 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : new Base[] {this.fhirVersion}; // Enumeration<FHIRVersion> 8827 case -1268779017: /*format*/ return this.format == null ? new Base[0] : this.format.toArray(new Base[this.format.size()]); // CodeType 8828 case 172338783: /*patchFormat*/ return this.patchFormat == null ? new Base[0] : this.patchFormat.toArray(new Base[this.patchFormat.size()]); // CodeType 8829 case 1014178944: /*acceptLanguage*/ return this.acceptLanguage == null ? new Base[0] : this.acceptLanguage.toArray(new Base[this.acceptLanguage.size()]); // CodeType 8830 case 156966506: /*implementationGuide*/ return this.implementationGuide == null ? new Base[0] : this.implementationGuide.toArray(new Base[this.implementationGuide.size()]); // CanonicalType 8831 case 3496916: /*rest*/ return this.rest == null ? new Base[0] : this.rest.toArray(new Base[this.rest.size()]); // CapabilityStatementRestComponent 8832 case -1440008444: /*messaging*/ return this.messaging == null ? new Base[0] : this.messaging.toArray(new Base[this.messaging.size()]); // CapabilityStatementMessagingComponent 8833 case 861720859: /*document*/ return this.document == null ? new Base[0] : this.document.toArray(new Base[this.document.size()]); // CapabilityStatementDocumentComponent 8834 default: return super.getProperty(hash, name, checkValid); 8835 } 8836 8837 } 8838 8839 @Override 8840 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8841 switch (hash) { 8842 case 116079: // url 8843 this.url = TypeConvertor.castToUri(value); // UriType 8844 return value; 8845 case -1618432855: // identifier 8846 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 8847 return value; 8848 case 351608024: // version 8849 this.version = TypeConvertor.castToString(value); // StringType 8850 return value; 8851 case 1508158071: // versionAlgorithm 8852 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 8853 return value; 8854 case 3373707: // name 8855 this.name = TypeConvertor.castToString(value); // StringType 8856 return value; 8857 case 110371416: // title 8858 this.title = TypeConvertor.castToString(value); // StringType 8859 return value; 8860 case -892481550: // status 8861 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 8862 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8863 return value; 8864 case -404562712: // experimental 8865 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 8866 return value; 8867 case 3076014: // date 8868 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 8869 return value; 8870 case 1447404028: // publisher 8871 this.publisher = TypeConvertor.castToString(value); // StringType 8872 return value; 8873 case 951526432: // contact 8874 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 8875 return value; 8876 case -1724546052: // description 8877 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 8878 return value; 8879 case -669707736: // useContext 8880 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 8881 return value; 8882 case -507075711: // jurisdiction 8883 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8884 return value; 8885 case -220463842: // purpose 8886 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 8887 return value; 8888 case 1522889671: // copyright 8889 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 8890 return value; 8891 case 765157229: // copyrightLabel 8892 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 8893 return value; 8894 case 3292052: // kind 8895 value = new CapabilityStatementKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 8896 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 8897 return value; 8898 case -246883639: // instantiates 8899 this.getInstantiates().add(TypeConvertor.castToCanonical(value)); // CanonicalType 8900 return value; 8901 case 1926037870: // imports 8902 this.getImports().add(TypeConvertor.castToCanonical(value)); // CanonicalType 8903 return value; 8904 case 1319330215: // software 8905 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 8906 return value; 8907 case 1683336114: // implementation 8908 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 8909 return value; 8910 case 461006061: // fhirVersion 8911 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 8912 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 8913 return value; 8914 case -1268779017: // format 8915 this.getFormat().add(TypeConvertor.castToCode(value)); // CodeType 8916 return value; 8917 case 172338783: // patchFormat 8918 this.getPatchFormat().add(TypeConvertor.castToCode(value)); // CodeType 8919 return value; 8920 case 1014178944: // acceptLanguage 8921 this.getAcceptLanguage().add(TypeConvertor.castToCode(value)); // CodeType 8922 return value; 8923 case 156966506: // implementationGuide 8924 this.getImplementationGuide().add(TypeConvertor.castToCanonical(value)); // CanonicalType 8925 return value; 8926 case 3496916: // rest 8927 this.getRest().add((CapabilityStatementRestComponent) value); // CapabilityStatementRestComponent 8928 return value; 8929 case -1440008444: // messaging 8930 this.getMessaging().add((CapabilityStatementMessagingComponent) value); // CapabilityStatementMessagingComponent 8931 return value; 8932 case 861720859: // document 8933 this.getDocument().add((CapabilityStatementDocumentComponent) value); // CapabilityStatementDocumentComponent 8934 return value; 8935 default: return super.setProperty(hash, name, value); 8936 } 8937 8938 } 8939 8940 @Override 8941 public Base setProperty(String name, Base value) throws FHIRException { 8942 if (name.equals("url")) { 8943 this.url = TypeConvertor.castToUri(value); // UriType 8944 } else if (name.equals("identifier")) { 8945 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 8946 } else if (name.equals("version")) { 8947 this.version = TypeConvertor.castToString(value); // StringType 8948 } else if (name.equals("versionAlgorithm[x]")) { 8949 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 8950 } else if (name.equals("name")) { 8951 this.name = TypeConvertor.castToString(value); // StringType 8952 } else if (name.equals("title")) { 8953 this.title = TypeConvertor.castToString(value); // StringType 8954 } else if (name.equals("status")) { 8955 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 8956 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8957 } else if (name.equals("experimental")) { 8958 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 8959 } else if (name.equals("date")) { 8960 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 8961 } else if (name.equals("publisher")) { 8962 this.publisher = TypeConvertor.castToString(value); // StringType 8963 } else if (name.equals("contact")) { 8964 this.getContact().add(TypeConvertor.castToContactDetail(value)); 8965 } else if (name.equals("description")) { 8966 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 8967 } else if (name.equals("useContext")) { 8968 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 8969 } else if (name.equals("jurisdiction")) { 8970 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 8971 } else if (name.equals("purpose")) { 8972 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 8973 } else if (name.equals("copyright")) { 8974 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 8975 } else if (name.equals("copyrightLabel")) { 8976 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 8977 } else if (name.equals("kind")) { 8978 value = new CapabilityStatementKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 8979 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 8980 } else if (name.equals("instantiates")) { 8981 this.getInstantiates().add(TypeConvertor.castToCanonical(value)); 8982 } else if (name.equals("imports")) { 8983 this.getImports().add(TypeConvertor.castToCanonical(value)); 8984 } else if (name.equals("software")) { 8985 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 8986 } else if (name.equals("implementation")) { 8987 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 8988 } else if (name.equals("fhirVersion")) { 8989 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 8990 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 8991 } else if (name.equals("format")) { 8992 this.getFormat().add(TypeConvertor.castToCode(value)); 8993 } else if (name.equals("patchFormat")) { 8994 this.getPatchFormat().add(TypeConvertor.castToCode(value)); 8995 } else if (name.equals("acceptLanguage")) { 8996 this.getAcceptLanguage().add(TypeConvertor.castToCode(value)); 8997 } else if (name.equals("implementationGuide")) { 8998 this.getImplementationGuide().add(TypeConvertor.castToCanonical(value)); 8999 } else if (name.equals("rest")) { 9000 this.getRest().add((CapabilityStatementRestComponent) value); 9001 } else if (name.equals("messaging")) { 9002 this.getMessaging().add((CapabilityStatementMessagingComponent) value); 9003 } else if (name.equals("document")) { 9004 this.getDocument().add((CapabilityStatementDocumentComponent) value); 9005 } else 9006 return super.setProperty(name, value); 9007 return value; 9008 } 9009 9010 @Override 9011 public void removeChild(String name, Base value) throws FHIRException { 9012 if (name.equals("url")) { 9013 this.url = null; 9014 } else if (name.equals("identifier")) { 9015 this.getIdentifier().remove(value); 9016 } else if (name.equals("version")) { 9017 this.version = null; 9018 } else if (name.equals("versionAlgorithm[x]")) { 9019 this.versionAlgorithm = null; 9020 } else if (name.equals("name")) { 9021 this.name = null; 9022 } else if (name.equals("title")) { 9023 this.title = null; 9024 } else if (name.equals("status")) { 9025 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 9026 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 9027 } else if (name.equals("experimental")) { 9028 this.experimental = null; 9029 } else if (name.equals("date")) { 9030 this.date = null; 9031 } else if (name.equals("publisher")) { 9032 this.publisher = null; 9033 } else if (name.equals("contact")) { 9034 this.getContact().remove(value); 9035 } else if (name.equals("description")) { 9036 this.description = null; 9037 } else if (name.equals("useContext")) { 9038 this.getUseContext().remove(value); 9039 } else if (name.equals("jurisdiction")) { 9040 this.getJurisdiction().remove(value); 9041 } else if (name.equals("purpose")) { 9042 this.purpose = null; 9043 } else if (name.equals("copyright")) { 9044 this.copyright = null; 9045 } else if (name.equals("copyrightLabel")) { 9046 this.copyrightLabel = null; 9047 } else if (name.equals("kind")) { 9048 value = new CapabilityStatementKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 9049 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 9050 } else if (name.equals("instantiates")) { 9051 this.getInstantiates().remove(value); 9052 } else if (name.equals("imports")) { 9053 this.getImports().remove(value); 9054 } else if (name.equals("software")) { 9055 this.software = (CapabilityStatementSoftwareComponent) value; // CapabilityStatementSoftwareComponent 9056 } else if (name.equals("implementation")) { 9057 this.implementation = (CapabilityStatementImplementationComponent) value; // CapabilityStatementImplementationComponent 9058 } else if (name.equals("fhirVersion")) { 9059 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 9060 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 9061 } else if (name.equals("format")) { 9062 this.getFormat().remove(value); 9063 } else if (name.equals("patchFormat")) { 9064 this.getPatchFormat().remove(value); 9065 } else if (name.equals("acceptLanguage")) { 9066 this.getAcceptLanguage().remove(value); 9067 } else if (name.equals("implementationGuide")) { 9068 this.getImplementationGuide().remove(value); 9069 } else if (name.equals("rest")) { 9070 this.getRest().remove((CapabilityStatementRestComponent) value); 9071 } else if (name.equals("messaging")) { 9072 this.getMessaging().remove((CapabilityStatementMessagingComponent) value); 9073 } else if (name.equals("document")) { 9074 this.getDocument().remove((CapabilityStatementDocumentComponent) value); 9075 } else 9076 super.removeChild(name, value); 9077 9078 } 9079 9080 @Override 9081 public Base makeProperty(int hash, String name) throws FHIRException { 9082 switch (hash) { 9083 case 116079: return getUrlElement(); 9084 case -1618432855: return addIdentifier(); 9085 case 351608024: return getVersionElement(); 9086 case -115699031: return getVersionAlgorithm(); 9087 case 1508158071: return getVersionAlgorithm(); 9088 case 3373707: return getNameElement(); 9089 case 110371416: return getTitleElement(); 9090 case -892481550: return getStatusElement(); 9091 case -404562712: return getExperimentalElement(); 9092 case 3076014: return getDateElement(); 9093 case 1447404028: return getPublisherElement(); 9094 case 951526432: return addContact(); 9095 case -1724546052: return getDescriptionElement(); 9096 case -669707736: return addUseContext(); 9097 case -507075711: return addJurisdiction(); 9098 case -220463842: return getPurposeElement(); 9099 case 1522889671: return getCopyrightElement(); 9100 case 765157229: return getCopyrightLabelElement(); 9101 case 3292052: return getKindElement(); 9102 case -246883639: return addInstantiatesElement(); 9103 case 1926037870: return addImportsElement(); 9104 case 1319330215: return getSoftware(); 9105 case 1683336114: return getImplementation(); 9106 case 461006061: return getFhirVersionElement(); 9107 case -1268779017: return addFormatElement(); 9108 case 172338783: return addPatchFormatElement(); 9109 case 1014178944: return addAcceptLanguageElement(); 9110 case 156966506: return addImplementationGuideElement(); 9111 case 3496916: return addRest(); 9112 case -1440008444: return addMessaging(); 9113 case 861720859: return addDocument(); 9114 default: return super.makeProperty(hash, name); 9115 } 9116 9117 } 9118 9119 @Override 9120 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9121 switch (hash) { 9122 case 116079: /*url*/ return new String[] {"uri"}; 9123 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 9124 case 351608024: /*version*/ return new String[] {"string"}; 9125 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 9126 case 3373707: /*name*/ return new String[] {"string"}; 9127 case 110371416: /*title*/ return new String[] {"string"}; 9128 case -892481550: /*status*/ return new String[] {"code"}; 9129 case -404562712: /*experimental*/ return new String[] {"boolean"}; 9130 case 3076014: /*date*/ return new String[] {"dateTime"}; 9131 case 1447404028: /*publisher*/ return new String[] {"string"}; 9132 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 9133 case -1724546052: /*description*/ return new String[] {"markdown"}; 9134 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 9135 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 9136 case -220463842: /*purpose*/ return new String[] {"markdown"}; 9137 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 9138 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 9139 case 3292052: /*kind*/ return new String[] {"code"}; 9140 case -246883639: /*instantiates*/ return new String[] {"canonical"}; 9141 case 1926037870: /*imports*/ return new String[] {"canonical"}; 9142 case 1319330215: /*software*/ return new String[] {}; 9143 case 1683336114: /*implementation*/ return new String[] {}; 9144 case 461006061: /*fhirVersion*/ return new String[] {"code"}; 9145 case -1268779017: /*format*/ return new String[] {"code"}; 9146 case 172338783: /*patchFormat*/ return new String[] {"code"}; 9147 case 1014178944: /*acceptLanguage*/ return new String[] {"code"}; 9148 case 156966506: /*implementationGuide*/ return new String[] {"canonical"}; 9149 case 3496916: /*rest*/ return new String[] {}; 9150 case -1440008444: /*messaging*/ return new String[] {}; 9151 case 861720859: /*document*/ return new String[] {}; 9152 default: return super.getTypesForProperty(hash, name); 9153 } 9154 9155 } 9156 9157 @Override 9158 public Base addChild(String name) throws FHIRException { 9159 if (name.equals("url")) { 9160 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.url"); 9161 } 9162 else if (name.equals("identifier")) { 9163 return addIdentifier(); 9164 } 9165 else if (name.equals("version")) { 9166 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.version"); 9167 } 9168 else if (name.equals("versionAlgorithmString")) { 9169 this.versionAlgorithm = new StringType(); 9170 return this.versionAlgorithm; 9171 } 9172 else if (name.equals("versionAlgorithmCoding")) { 9173 this.versionAlgorithm = new Coding(); 9174 return this.versionAlgorithm; 9175 } 9176 else if (name.equals("name")) { 9177 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.name"); 9178 } 9179 else if (name.equals("title")) { 9180 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.title"); 9181 } 9182 else if (name.equals("status")) { 9183 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.status"); 9184 } 9185 else if (name.equals("experimental")) { 9186 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.experimental"); 9187 } 9188 else if (name.equals("date")) { 9189 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.date"); 9190 } 9191 else if (name.equals("publisher")) { 9192 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.publisher"); 9193 } 9194 else if (name.equals("contact")) { 9195 return addContact(); 9196 } 9197 else if (name.equals("description")) { 9198 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.description"); 9199 } 9200 else if (name.equals("useContext")) { 9201 return addUseContext(); 9202 } 9203 else if (name.equals("jurisdiction")) { 9204 return addJurisdiction(); 9205 } 9206 else if (name.equals("purpose")) { 9207 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.purpose"); 9208 } 9209 else if (name.equals("copyright")) { 9210 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.copyright"); 9211 } 9212 else if (name.equals("copyrightLabel")) { 9213 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.copyrightLabel"); 9214 } 9215 else if (name.equals("kind")) { 9216 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.kind"); 9217 } 9218 else if (name.equals("instantiates")) { 9219 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.instantiates"); 9220 } 9221 else if (name.equals("imports")) { 9222 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.imports"); 9223 } 9224 else if (name.equals("software")) { 9225 this.software = new CapabilityStatementSoftwareComponent(); 9226 return this.software; 9227 } 9228 else if (name.equals("implementation")) { 9229 this.implementation = new CapabilityStatementImplementationComponent(); 9230 return this.implementation; 9231 } 9232 else if (name.equals("fhirVersion")) { 9233 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.fhirVersion"); 9234 } 9235 else if (name.equals("format")) { 9236 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.format"); 9237 } 9238 else if (name.equals("patchFormat")) { 9239 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.patchFormat"); 9240 } 9241 else if (name.equals("acceptLanguage")) { 9242 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.acceptLanguage"); 9243 } 9244 else if (name.equals("implementationGuide")) { 9245 throw new FHIRException("Cannot call addChild on a singleton property CapabilityStatement.implementationGuide"); 9246 } 9247 else if (name.equals("rest")) { 9248 return addRest(); 9249 } 9250 else if (name.equals("messaging")) { 9251 return addMessaging(); 9252 } 9253 else if (name.equals("document")) { 9254 return addDocument(); 9255 } 9256 else 9257 return super.addChild(name); 9258 } 9259 9260 public String fhirType() { 9261 return "CapabilityStatement"; 9262 9263 } 9264 9265 public CapabilityStatement copy() { 9266 CapabilityStatement dst = new CapabilityStatement(); 9267 copyValues(dst); 9268 return dst; 9269 } 9270 9271 public void copyValues(CapabilityStatement dst) { 9272 super.copyValues(dst); 9273 dst.url = url == null ? null : url.copy(); 9274 if (identifier != null) { 9275 dst.identifier = new ArrayList<Identifier>(); 9276 for (Identifier i : identifier) 9277 dst.identifier.add(i.copy()); 9278 }; 9279 dst.version = version == null ? null : version.copy(); 9280 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 9281 dst.name = name == null ? null : name.copy(); 9282 dst.title = title == null ? null : title.copy(); 9283 dst.status = status == null ? null : status.copy(); 9284 dst.experimental = experimental == null ? null : experimental.copy(); 9285 dst.date = date == null ? null : date.copy(); 9286 dst.publisher = publisher == null ? null : publisher.copy(); 9287 if (contact != null) { 9288 dst.contact = new ArrayList<ContactDetail>(); 9289 for (ContactDetail i : contact) 9290 dst.contact.add(i.copy()); 9291 }; 9292 dst.description = description == null ? null : description.copy(); 9293 if (useContext != null) { 9294 dst.useContext = new ArrayList<UsageContext>(); 9295 for (UsageContext i : useContext) 9296 dst.useContext.add(i.copy()); 9297 }; 9298 if (jurisdiction != null) { 9299 dst.jurisdiction = new ArrayList<CodeableConcept>(); 9300 for (CodeableConcept i : jurisdiction) 9301 dst.jurisdiction.add(i.copy()); 9302 }; 9303 dst.purpose = purpose == null ? null : purpose.copy(); 9304 dst.copyright = copyright == null ? null : copyright.copy(); 9305 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 9306 dst.kind = kind == null ? null : kind.copy(); 9307 if (instantiates != null) { 9308 dst.instantiates = new ArrayList<CanonicalType>(); 9309 for (CanonicalType i : instantiates) 9310 dst.instantiates.add(i.copy()); 9311 }; 9312 if (imports != null) { 9313 dst.imports = new ArrayList<CanonicalType>(); 9314 for (CanonicalType i : imports) 9315 dst.imports.add(i.copy()); 9316 }; 9317 dst.software = software == null ? null : software.copy(); 9318 dst.implementation = implementation == null ? null : implementation.copy(); 9319 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 9320 if (format != null) { 9321 dst.format = new ArrayList<CodeType>(); 9322 for (CodeType i : format) 9323 dst.format.add(i.copy()); 9324 }; 9325 if (patchFormat != null) { 9326 dst.patchFormat = new ArrayList<CodeType>(); 9327 for (CodeType i : patchFormat) 9328 dst.patchFormat.add(i.copy()); 9329 }; 9330 if (acceptLanguage != null) { 9331 dst.acceptLanguage = new ArrayList<CodeType>(); 9332 for (CodeType i : acceptLanguage) 9333 dst.acceptLanguage.add(i.copy()); 9334 }; 9335 if (implementationGuide != null) { 9336 dst.implementationGuide = new ArrayList<CanonicalType>(); 9337 for (CanonicalType i : implementationGuide) 9338 dst.implementationGuide.add(i.copy()); 9339 }; 9340 if (rest != null) { 9341 dst.rest = new ArrayList<CapabilityStatementRestComponent>(); 9342 for (CapabilityStatementRestComponent i : rest) 9343 dst.rest.add(i.copy()); 9344 }; 9345 if (messaging != null) { 9346 dst.messaging = new ArrayList<CapabilityStatementMessagingComponent>(); 9347 for (CapabilityStatementMessagingComponent i : messaging) 9348 dst.messaging.add(i.copy()); 9349 }; 9350 if (document != null) { 9351 dst.document = new ArrayList<CapabilityStatementDocumentComponent>(); 9352 for (CapabilityStatementDocumentComponent i : document) 9353 dst.document.add(i.copy()); 9354 }; 9355 } 9356 9357 protected CapabilityStatement typedCopy() { 9358 return copy(); 9359 } 9360 9361 @Override 9362 public boolean equalsDeep(Base other_) { 9363 if (!super.equalsDeep(other_)) 9364 return false; 9365 if (!(other_ instanceof CapabilityStatement)) 9366 return false; 9367 CapabilityStatement o = (CapabilityStatement) other_; 9368 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 9369 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 9370 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 9371 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 9372 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 9373 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 9374 && compareDeep(kind, o.kind, true) && compareDeep(instantiates, o.instantiates, true) && compareDeep(imports, o.imports, true) 9375 && compareDeep(software, o.software, true) && compareDeep(implementation, o.implementation, true) 9376 && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(format, o.format, true) && compareDeep(patchFormat, o.patchFormat, true) 9377 && compareDeep(acceptLanguage, o.acceptLanguage, true) && compareDeep(implementationGuide, o.implementationGuide, true) 9378 && compareDeep(rest, o.rest, true) && compareDeep(messaging, o.messaging, true) && compareDeep(document, o.document, true) 9379 ; 9380 } 9381 9382 @Override 9383 public boolean equalsShallow(Base other_) { 9384 if (!super.equalsShallow(other_)) 9385 return false; 9386 if (!(other_ instanceof CapabilityStatement)) 9387 return false; 9388 CapabilityStatement o = (CapabilityStatement) other_; 9389 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 9390 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 9391 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 9392 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 9393 && compareValues(kind, o.kind, true) && compareValues(instantiates, o.instantiates, true) && compareValues(imports, o.imports, true) 9394 && compareValues(fhirVersion, o.fhirVersion, true) && compareValues(format, o.format, true) && compareValues(patchFormat, o.patchFormat, true) 9395 && compareValues(acceptLanguage, o.acceptLanguage, true) && compareValues(implementationGuide, o.implementationGuide, true) 9396 ; 9397 } 9398 9399 public boolean isEmpty() { 9400 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 9401 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 9402 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, kind 9403 , instantiates, imports, software, implementation, fhirVersion, format, patchFormat 9404 , acceptLanguage, implementationGuide, rest, messaging, document); 9405 } 9406 9407 @Override 9408 public ResourceType getResourceType() { 9409 return ResourceType.CapabilityStatement; 9410 } 9411 9412 /** 9413 * Search parameter: <b>context-quantity</b> 9414 * <p> 9415 * Description: <b>Multiple Resources: 9416 9417* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 9418* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 9419* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 9420* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 9421* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 9422* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 9423* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 9424* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 9425* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 9426* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 9427* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 9428* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 9429* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 9430* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 9431* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 9432* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 9433* [Library](library.html): A quantity- or range-valued use context assigned to the library 9434* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 9435* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 9436* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 9437* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 9438* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 9439* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 9440* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 9441* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 9442* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 9443* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 9444* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 9445* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 9446* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 9447</b><br> 9448 * Type: <b>quantity</b><br> 9449 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 9450 * </p> 9451 */ 9452 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 9453 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 9454 /** 9455 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 9456 * <p> 9457 * Description: <b>Multiple Resources: 9458 9459* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 9460* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 9461* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 9462* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 9463* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 9464* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 9465* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 9466* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 9467* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 9468* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 9469* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 9470* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 9471* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 9472* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 9473* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 9474* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 9475* [Library](library.html): A quantity- or range-valued use context assigned to the library 9476* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 9477* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 9478* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 9479* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 9480* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 9481* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 9482* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 9483* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 9484* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 9485* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 9486* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 9487* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 9488* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 9489</b><br> 9490 * Type: <b>quantity</b><br> 9491 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 9492 * </p> 9493 */ 9494 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 9495 9496 /** 9497 * Search parameter: <b>context-type-quantity</b> 9498 * <p> 9499 * Description: <b>Multiple Resources: 9500 9501* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 9502* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 9503* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 9504* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 9505* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 9506* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 9507* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 9508* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 9509* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 9510* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 9511* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 9512* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 9513* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 9514* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 9515* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 9516* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 9517* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 9518* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 9519* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 9520* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 9521* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 9522* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 9523* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 9524* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 9525* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 9526* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 9527* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 9528* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 9529* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 9530* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 9531</b><br> 9532 * Type: <b>composite</b><br> 9533 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9534 * </p> 9535 */ 9536 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 9537 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 9538 /** 9539 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 9540 * <p> 9541 * Description: <b>Multiple Resources: 9542 9543* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 9544* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 9545* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 9546* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 9547* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 9548* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 9549* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 9550* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 9551* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 9552* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 9553* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 9554* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 9555* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 9556* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 9557* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 9558* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 9559* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 9560* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 9561* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 9562* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 9563* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 9564* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 9565* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 9566* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 9567* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 9568* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 9569* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 9570* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 9571* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 9572* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 9573</b><br> 9574 * Type: <b>composite</b><br> 9575 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9576 * </p> 9577 */ 9578 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 9579 9580 /** 9581 * Search parameter: <b>context-type-value</b> 9582 * <p> 9583 * Description: <b>Multiple Resources: 9584 9585* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 9586* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 9587* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 9588* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 9589* [Citation](citation.html): A use context type and value assigned to the citation 9590* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 9591* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 9592* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 9593* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 9594* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 9595* [Evidence](evidence.html): A use context type and value assigned to the evidence 9596* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 9597* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 9598* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 9599* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 9600* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 9601* [Library](library.html): A use context type and value assigned to the library 9602* [Measure](measure.html): A use context type and value assigned to the measure 9603* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 9604* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 9605* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 9606* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 9607* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 9608* [Requirements](requirements.html): A use context type and value assigned to the requirements 9609* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 9610* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 9611* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 9612* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 9613* [TestScript](testscript.html): A use context type and value assigned to the test script 9614* [ValueSet](valueset.html): A use context type and value assigned to the value set 9615</b><br> 9616 * Type: <b>composite</b><br> 9617 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9618 * </p> 9619 */ 9620 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 9621 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 9622 /** 9623 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 9624 * <p> 9625 * Description: <b>Multiple Resources: 9626 9627* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 9628* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 9629* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 9630* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 9631* [Citation](citation.html): A use context type and value assigned to the citation 9632* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 9633* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 9634* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 9635* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 9636* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 9637* [Evidence](evidence.html): A use context type and value assigned to the evidence 9638* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 9639* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 9640* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 9641* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 9642* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 9643* [Library](library.html): A use context type and value assigned to the library 9644* [Measure](measure.html): A use context type and value assigned to the measure 9645* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 9646* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 9647* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 9648* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 9649* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 9650* [Requirements](requirements.html): A use context type and value assigned to the requirements 9651* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 9652* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 9653* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 9654* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 9655* [TestScript](testscript.html): A use context type and value assigned to the test script 9656* [ValueSet](valueset.html): A use context type and value assigned to the value set 9657</b><br> 9658 * Type: <b>composite</b><br> 9659 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 9660 * </p> 9661 */ 9662 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 9663 9664 /** 9665 * Search parameter: <b>context-type</b> 9666 * <p> 9667 * Description: <b>Multiple Resources: 9668 9669* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 9670* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 9671* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 9672* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 9673* [Citation](citation.html): A type of use context assigned to the citation 9674* [CodeSystem](codesystem.html): A type of use context assigned to the code system 9675* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 9676* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 9677* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 9678* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 9679* [Evidence](evidence.html): A type of use context assigned to the evidence 9680* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 9681* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 9682* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 9683* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 9684* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 9685* [Library](library.html): A type of use context assigned to the library 9686* [Measure](measure.html): A type of use context assigned to the measure 9687* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 9688* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 9689* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 9690* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 9691* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 9692* [Requirements](requirements.html): A type of use context assigned to the requirements 9693* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 9694* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 9695* [StructureMap](structuremap.html): A type of use context assigned to the structure map 9696* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 9697* [TestScript](testscript.html): A type of use context assigned to the test script 9698* [ValueSet](valueset.html): A type of use context assigned to the value set 9699</b><br> 9700 * Type: <b>token</b><br> 9701 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 9702 * </p> 9703 */ 9704 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 9705 public static final String SP_CONTEXT_TYPE = "context-type"; 9706 /** 9707 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 9708 * <p> 9709 * Description: <b>Multiple Resources: 9710 9711* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 9712* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 9713* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 9714* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 9715* [Citation](citation.html): A type of use context assigned to the citation 9716* [CodeSystem](codesystem.html): A type of use context assigned to the code system 9717* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 9718* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 9719* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 9720* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 9721* [Evidence](evidence.html): A type of use context assigned to the evidence 9722* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 9723* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 9724* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 9725* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 9726* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 9727* [Library](library.html): A type of use context assigned to the library 9728* [Measure](measure.html): A type of use context assigned to the measure 9729* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 9730* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 9731* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 9732* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 9733* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 9734* [Requirements](requirements.html): A type of use context assigned to the requirements 9735* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 9736* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 9737* [StructureMap](structuremap.html): A type of use context assigned to the structure map 9738* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 9739* [TestScript](testscript.html): A type of use context assigned to the test script 9740* [ValueSet](valueset.html): A type of use context assigned to the value set 9741</b><br> 9742 * Type: <b>token</b><br> 9743 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 9744 * </p> 9745 */ 9746 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 9747 9748 /** 9749 * Search parameter: <b>context</b> 9750 * <p> 9751 * Description: <b>Multiple Resources: 9752 9753* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 9754* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 9755* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 9756* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 9757* [Citation](citation.html): A use context assigned to the citation 9758* [CodeSystem](codesystem.html): A use context assigned to the code system 9759* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 9760* [ConceptMap](conceptmap.html): A use context assigned to the concept map 9761* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 9762* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 9763* [Evidence](evidence.html): A use context assigned to the evidence 9764* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 9765* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 9766* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 9767* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 9768* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 9769* [Library](library.html): A use context assigned to the library 9770* [Measure](measure.html): A use context assigned to the measure 9771* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 9772* [NamingSystem](namingsystem.html): A use context assigned to the naming system 9773* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 9774* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 9775* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 9776* [Requirements](requirements.html): A use context assigned to the requirements 9777* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 9778* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 9779* [StructureMap](structuremap.html): A use context assigned to the structure map 9780* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 9781* [TestScript](testscript.html): A use context assigned to the test script 9782* [ValueSet](valueset.html): A use context assigned to the value set 9783</b><br> 9784 * Type: <b>token</b><br> 9785 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 9786 * </p> 9787 */ 9788 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 9789 public static final String SP_CONTEXT = "context"; 9790 /** 9791 * <b>Fluent Client</b> search parameter constant for <b>context</b> 9792 * <p> 9793 * Description: <b>Multiple Resources: 9794 9795* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 9796* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 9797* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 9798* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 9799* [Citation](citation.html): A use context assigned to the citation 9800* [CodeSystem](codesystem.html): A use context assigned to the code system 9801* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 9802* [ConceptMap](conceptmap.html): A use context assigned to the concept map 9803* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 9804* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 9805* [Evidence](evidence.html): A use context assigned to the evidence 9806* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 9807* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 9808* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 9809* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 9810* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 9811* [Library](library.html): A use context assigned to the library 9812* [Measure](measure.html): A use context assigned to the measure 9813* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 9814* [NamingSystem](namingsystem.html): A use context assigned to the naming system 9815* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 9816* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 9817* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 9818* [Requirements](requirements.html): A use context assigned to the requirements 9819* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 9820* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 9821* [StructureMap](structuremap.html): A use context assigned to the structure map 9822* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 9823* [TestScript](testscript.html): A use context assigned to the test script 9824* [ValueSet](valueset.html): A use context assigned to the value set 9825</b><br> 9826 * Type: <b>token</b><br> 9827 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 9828 * </p> 9829 */ 9830 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 9831 9832 /** 9833 * Search parameter: <b>date</b> 9834 * <p> 9835 * Description: <b>Multiple Resources: 9836 9837* [ActivityDefinition](activitydefinition.html): The activity definition publication date 9838* [ActorDefinition](actordefinition.html): The Actor Definition publication date 9839* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 9840* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 9841* [Citation](citation.html): The citation publication date 9842* [CodeSystem](codesystem.html): The code system publication date 9843* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 9844* [ConceptMap](conceptmap.html): The concept map publication date 9845* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 9846* [EventDefinition](eventdefinition.html): The event definition publication date 9847* [Evidence](evidence.html): The evidence publication date 9848* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 9849* [ExampleScenario](examplescenario.html): The example scenario publication date 9850* [GraphDefinition](graphdefinition.html): The graph definition publication date 9851* [ImplementationGuide](implementationguide.html): The implementation guide publication date 9852* [Library](library.html): The library publication date 9853* [Measure](measure.html): The measure publication date 9854* [MessageDefinition](messagedefinition.html): The message definition publication date 9855* [NamingSystem](namingsystem.html): The naming system publication date 9856* [OperationDefinition](operationdefinition.html): The operation definition publication date 9857* [PlanDefinition](plandefinition.html): The plan definition publication date 9858* [Questionnaire](questionnaire.html): The questionnaire publication date 9859* [Requirements](requirements.html): The requirements publication date 9860* [SearchParameter](searchparameter.html): The search parameter publication date 9861* [StructureDefinition](structuredefinition.html): The structure definition publication date 9862* [StructureMap](structuremap.html): The structure map publication date 9863* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 9864* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 9865* [TestScript](testscript.html): The test script publication date 9866* [ValueSet](valueset.html): The value set publication date 9867</b><br> 9868 * Type: <b>date</b><br> 9869 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 9870 * </p> 9871 */ 9872 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 9873 public static final String SP_DATE = "date"; 9874 /** 9875 * <b>Fluent Client</b> search parameter constant for <b>date</b> 9876 * <p> 9877 * Description: <b>Multiple Resources: 9878 9879* [ActivityDefinition](activitydefinition.html): The activity definition publication date 9880* [ActorDefinition](actordefinition.html): The Actor Definition publication date 9881* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 9882* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 9883* [Citation](citation.html): The citation publication date 9884* [CodeSystem](codesystem.html): The code system publication date 9885* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 9886* [ConceptMap](conceptmap.html): The concept map publication date 9887* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 9888* [EventDefinition](eventdefinition.html): The event definition publication date 9889* [Evidence](evidence.html): The evidence publication date 9890* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 9891* [ExampleScenario](examplescenario.html): The example scenario publication date 9892* [GraphDefinition](graphdefinition.html): The graph definition publication date 9893* [ImplementationGuide](implementationguide.html): The implementation guide publication date 9894* [Library](library.html): The library publication date 9895* [Measure](measure.html): The measure publication date 9896* [MessageDefinition](messagedefinition.html): The message definition publication date 9897* [NamingSystem](namingsystem.html): The naming system publication date 9898* [OperationDefinition](operationdefinition.html): The operation definition publication date 9899* [PlanDefinition](plandefinition.html): The plan definition publication date 9900* [Questionnaire](questionnaire.html): The questionnaire publication date 9901* [Requirements](requirements.html): The requirements publication date 9902* [SearchParameter](searchparameter.html): The search parameter publication date 9903* [StructureDefinition](structuredefinition.html): The structure definition publication date 9904* [StructureMap](structuremap.html): The structure map publication date 9905* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 9906* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 9907* [TestScript](testscript.html): The test script publication date 9908* [ValueSet](valueset.html): The value set publication date 9909</b><br> 9910 * Type: <b>date</b><br> 9911 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 9912 * </p> 9913 */ 9914 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 9915 9916 /** 9917 * Search parameter: <b>description</b> 9918 * <p> 9919 * Description: <b>Multiple Resources: 9920 9921* [ActivityDefinition](activitydefinition.html): The description of the activity definition 9922* [ActorDefinition](actordefinition.html): The description of the Actor Definition 9923* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 9924* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 9925* [Citation](citation.html): The description of the citation 9926* [CodeSystem](codesystem.html): The description of the code system 9927* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 9928* [ConceptMap](conceptmap.html): The description of the concept map 9929* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 9930* [EventDefinition](eventdefinition.html): The description of the event definition 9931* [Evidence](evidence.html): The description of the evidence 9932* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 9933* [GraphDefinition](graphdefinition.html): The description of the graph definition 9934* [ImplementationGuide](implementationguide.html): The description of the implementation guide 9935* [Library](library.html): The description of the library 9936* [Measure](measure.html): The description of the measure 9937* [MessageDefinition](messagedefinition.html): The description of the message definition 9938* [NamingSystem](namingsystem.html): The description of the naming system 9939* [OperationDefinition](operationdefinition.html): The description of the operation definition 9940* [PlanDefinition](plandefinition.html): The description of the plan definition 9941* [Questionnaire](questionnaire.html): The description of the questionnaire 9942* [Requirements](requirements.html): The description of the requirements 9943* [SearchParameter](searchparameter.html): The description of the search parameter 9944* [StructureDefinition](structuredefinition.html): The description of the structure definition 9945* [StructureMap](structuremap.html): The description of the structure map 9946* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 9947* [TestScript](testscript.html): The description of the test script 9948* [ValueSet](valueset.html): The description of the value set 9949</b><br> 9950 * Type: <b>string</b><br> 9951 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 9952 * </p> 9953 */ 9954 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 9955 public static final String SP_DESCRIPTION = "description"; 9956 /** 9957 * <b>Fluent Client</b> search parameter constant for <b>description</b> 9958 * <p> 9959 * Description: <b>Multiple Resources: 9960 9961* [ActivityDefinition](activitydefinition.html): The description of the activity definition 9962* [ActorDefinition](actordefinition.html): The description of the Actor Definition 9963* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 9964* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 9965* [Citation](citation.html): The description of the citation 9966* [CodeSystem](codesystem.html): The description of the code system 9967* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 9968* [ConceptMap](conceptmap.html): The description of the concept map 9969* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 9970* [EventDefinition](eventdefinition.html): The description of the event definition 9971* [Evidence](evidence.html): The description of the evidence 9972* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 9973* [GraphDefinition](graphdefinition.html): The description of the graph definition 9974* [ImplementationGuide](implementationguide.html): The description of the implementation guide 9975* [Library](library.html): The description of the library 9976* [Measure](measure.html): The description of the measure 9977* [MessageDefinition](messagedefinition.html): The description of the message definition 9978* [NamingSystem](namingsystem.html): The description of the naming system 9979* [OperationDefinition](operationdefinition.html): The description of the operation definition 9980* [PlanDefinition](plandefinition.html): The description of the plan definition 9981* [Questionnaire](questionnaire.html): The description of the questionnaire 9982* [Requirements](requirements.html): The description of the requirements 9983* [SearchParameter](searchparameter.html): The description of the search parameter 9984* [StructureDefinition](structuredefinition.html): The description of the structure definition 9985* [StructureMap](structuremap.html): The description of the structure map 9986* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 9987* [TestScript](testscript.html): The description of the test script 9988* [ValueSet](valueset.html): The description of the value set 9989</b><br> 9990 * Type: <b>string</b><br> 9991 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 9992 * </p> 9993 */ 9994 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 9995 9996 /** 9997 * Search parameter: <b>identifier</b> 9998 * <p> 9999 * Description: <b>Multiple Resources: 10000 10001* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 10002* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 10003* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 10004* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 10005* [Citation](citation.html): External identifier for the citation 10006* [CodeSystem](codesystem.html): External identifier for the code system 10007* [ConceptMap](conceptmap.html): External identifier for the concept map 10008* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 10009* [EventDefinition](eventdefinition.html): External identifier for the event definition 10010* [Evidence](evidence.html): External identifier for the evidence 10011* [EvidenceReport](evidencereport.html): External identifier for the evidence report 10012* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 10013* [ExampleScenario](examplescenario.html): External identifier for the example scenario 10014* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 10015* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 10016* [Library](library.html): External identifier for the library 10017* [Measure](measure.html): External identifier for the measure 10018* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 10019* [MessageDefinition](messagedefinition.html): External identifier for the message definition 10020* [NamingSystem](namingsystem.html): External identifier for the naming system 10021* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 10022* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 10023* [PlanDefinition](plandefinition.html): External identifier for the plan definition 10024* [Questionnaire](questionnaire.html): External identifier for the questionnaire 10025* [Requirements](requirements.html): External identifier for the requirements 10026* [SearchParameter](searchparameter.html): External identifier for the search parameter 10027* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 10028* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 10029* [StructureMap](structuremap.html): External identifier for the structure map 10030* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 10031* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 10032* [TestPlan](testplan.html): An identifier for the test plan 10033* [TestScript](testscript.html): External identifier for the test script 10034* [ValueSet](valueset.html): External identifier for the value set 10035</b><br> 10036 * Type: <b>token</b><br> 10037 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 10038 * </p> 10039 */ 10040 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 10041 public static final String SP_IDENTIFIER = "identifier"; 10042 /** 10043 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10044 * <p> 10045 * Description: <b>Multiple Resources: 10046 10047* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 10048* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 10049* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 10050* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 10051* [Citation](citation.html): External identifier for the citation 10052* [CodeSystem](codesystem.html): External identifier for the code system 10053* [ConceptMap](conceptmap.html): External identifier for the concept map 10054* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 10055* [EventDefinition](eventdefinition.html): External identifier for the event definition 10056* [Evidence](evidence.html): External identifier for the evidence 10057* [EvidenceReport](evidencereport.html): External identifier for the evidence report 10058* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 10059* [ExampleScenario](examplescenario.html): External identifier for the example scenario 10060* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 10061* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 10062* [Library](library.html): External identifier for the library 10063* [Measure](measure.html): External identifier for the measure 10064* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 10065* [MessageDefinition](messagedefinition.html): External identifier for the message definition 10066* [NamingSystem](namingsystem.html): External identifier for the naming system 10067* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 10068* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 10069* [PlanDefinition](plandefinition.html): External identifier for the plan definition 10070* [Questionnaire](questionnaire.html): External identifier for the questionnaire 10071* [Requirements](requirements.html): External identifier for the requirements 10072* [SearchParameter](searchparameter.html): External identifier for the search parameter 10073* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 10074* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 10075* [StructureMap](structuremap.html): External identifier for the structure map 10076* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 10077* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 10078* [TestPlan](testplan.html): An identifier for the test plan 10079* [TestScript](testscript.html): External identifier for the test script 10080* [ValueSet](valueset.html): External identifier for the value set 10081</b><br> 10082 * Type: <b>token</b><br> 10083 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 10084 * </p> 10085 */ 10086 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 10087 10088 /** 10089 * Search parameter: <b>jurisdiction</b> 10090 * <p> 10091 * Description: <b>Multiple Resources: 10092 10093* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 10094* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 10095* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 10096* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 10097* [Citation](citation.html): Intended jurisdiction for the citation 10098* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 10099* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 10100* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 10101* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 10102* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 10103* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 10104* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 10105* [Library](library.html): Intended jurisdiction for the library 10106* [Measure](measure.html): Intended jurisdiction for the measure 10107* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 10108* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 10109* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 10110* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 10111* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 10112* [Requirements](requirements.html): Intended jurisdiction for the requirements 10113* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 10114* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 10115* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 10116* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 10117* [TestScript](testscript.html): Intended jurisdiction for the test script 10118* [ValueSet](valueset.html): Intended jurisdiction for the value set 10119</b><br> 10120 * Type: <b>token</b><br> 10121 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 10122 * </p> 10123 */ 10124 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 10125 public static final String SP_JURISDICTION = "jurisdiction"; 10126 /** 10127 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 10128 * <p> 10129 * Description: <b>Multiple Resources: 10130 10131* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 10132* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 10133* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 10134* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 10135* [Citation](citation.html): Intended jurisdiction for the citation 10136* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 10137* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 10138* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 10139* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 10140* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 10141* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 10142* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 10143* [Library](library.html): Intended jurisdiction for the library 10144* [Measure](measure.html): Intended jurisdiction for the measure 10145* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 10146* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 10147* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 10148* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 10149* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 10150* [Requirements](requirements.html): Intended jurisdiction for the requirements 10151* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 10152* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 10153* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 10154* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 10155* [TestScript](testscript.html): Intended jurisdiction for the test script 10156* [ValueSet](valueset.html): Intended jurisdiction for the value set 10157</b><br> 10158 * Type: <b>token</b><br> 10159 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 10160 * </p> 10161 */ 10162 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 10163 10164 /** 10165 * Search parameter: <b>name</b> 10166 * <p> 10167 * Description: <b>Multiple Resources: 10168 10169* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 10170* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 10171* [Citation](citation.html): Computationally friendly name of the citation 10172* [CodeSystem](codesystem.html): Computationally friendly name of the code system 10173* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 10174* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 10175* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 10176* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 10177* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 10178* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 10179* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 10180* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 10181* [Library](library.html): Computationally friendly name of the library 10182* [Measure](measure.html): Computationally friendly name of the measure 10183* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 10184* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 10185* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 10186* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 10187* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 10188* [Requirements](requirements.html): Computationally friendly name of the requirements 10189* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 10190* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 10191* [StructureMap](structuremap.html): Computationally friendly name of the structure map 10192* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 10193* [TestScript](testscript.html): Computationally friendly name of the test script 10194* [ValueSet](valueset.html): Computationally friendly name of the value set 10195</b><br> 10196 * Type: <b>string</b><br> 10197 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 10198 * </p> 10199 */ 10200 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 10201 public static final String SP_NAME = "name"; 10202 /** 10203 * <b>Fluent Client</b> search parameter constant for <b>name</b> 10204 * <p> 10205 * Description: <b>Multiple Resources: 10206 10207* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 10208* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 10209* [Citation](citation.html): Computationally friendly name of the citation 10210* [CodeSystem](codesystem.html): Computationally friendly name of the code system 10211* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 10212* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 10213* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 10214* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 10215* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 10216* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 10217* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 10218* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 10219* [Library](library.html): Computationally friendly name of the library 10220* [Measure](measure.html): Computationally friendly name of the measure 10221* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 10222* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 10223* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 10224* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 10225* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 10226* [Requirements](requirements.html): Computationally friendly name of the requirements 10227* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 10228* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 10229* [StructureMap](structuremap.html): Computationally friendly name of the structure map 10230* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 10231* [TestScript](testscript.html): Computationally friendly name of the test script 10232* [ValueSet](valueset.html): Computationally friendly name of the value set 10233</b><br> 10234 * Type: <b>string</b><br> 10235 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 10236 * </p> 10237 */ 10238 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 10239 10240 /** 10241 * Search parameter: <b>publisher</b> 10242 * <p> 10243 * Description: <b>Multiple Resources: 10244 10245* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 10246* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 10247* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 10248* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 10249* [Citation](citation.html): Name of the publisher of the citation 10250* [CodeSystem](codesystem.html): Name of the publisher of the code system 10251* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 10252* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 10253* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 10254* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 10255* [Evidence](evidence.html): Name of the publisher of the evidence 10256* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 10257* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 10258* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 10259* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 10260* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 10261* [Library](library.html): Name of the publisher of the library 10262* [Measure](measure.html): Name of the publisher of the measure 10263* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 10264* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 10265* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 10266* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 10267* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 10268* [Requirements](requirements.html): Name of the publisher of the requirements 10269* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 10270* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 10271* [StructureMap](structuremap.html): Name of the publisher of the structure map 10272* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 10273* [TestScript](testscript.html): Name of the publisher of the test script 10274* [ValueSet](valueset.html): Name of the publisher of the value set 10275</b><br> 10276 * Type: <b>string</b><br> 10277 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 10278 * </p> 10279 */ 10280 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 10281 public static final String SP_PUBLISHER = "publisher"; 10282 /** 10283 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 10284 * <p> 10285 * Description: <b>Multiple Resources: 10286 10287* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 10288* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 10289* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 10290* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 10291* [Citation](citation.html): Name of the publisher of the citation 10292* [CodeSystem](codesystem.html): Name of the publisher of the code system 10293* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 10294* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 10295* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 10296* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 10297* [Evidence](evidence.html): Name of the publisher of the evidence 10298* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 10299* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 10300* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 10301* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 10302* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 10303* [Library](library.html): Name of the publisher of the library 10304* [Measure](measure.html): Name of the publisher of the measure 10305* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 10306* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 10307* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 10308* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 10309* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 10310* [Requirements](requirements.html): Name of the publisher of the requirements 10311* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 10312* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 10313* [StructureMap](structuremap.html): Name of the publisher of the structure map 10314* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 10315* [TestScript](testscript.html): Name of the publisher of the test script 10316* [ValueSet](valueset.html): Name of the publisher of the value set 10317</b><br> 10318 * Type: <b>string</b><br> 10319 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 10320 * </p> 10321 */ 10322 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 10323 10324 /** 10325 * Search parameter: <b>status</b> 10326 * <p> 10327 * Description: <b>Multiple Resources: 10328 10329* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 10330* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 10331* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 10332* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 10333* [Citation](citation.html): The current status of the citation 10334* [CodeSystem](codesystem.html): The current status of the code system 10335* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 10336* [ConceptMap](conceptmap.html): The current status of the concept map 10337* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 10338* [EventDefinition](eventdefinition.html): The current status of the event definition 10339* [Evidence](evidence.html): The current status of the evidence 10340* [EvidenceReport](evidencereport.html): The current status of the evidence report 10341* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 10342* [ExampleScenario](examplescenario.html): The current status of the example scenario 10343* [GraphDefinition](graphdefinition.html): The current status of the graph definition 10344* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 10345* [Library](library.html): The current status of the library 10346* [Measure](measure.html): The current status of the measure 10347* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 10348* [MessageDefinition](messagedefinition.html): The current status of the message definition 10349* [NamingSystem](namingsystem.html): The current status of the naming system 10350* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 10351* [OperationDefinition](operationdefinition.html): The current status of the operation definition 10352* [PlanDefinition](plandefinition.html): The current status of the plan definition 10353* [Questionnaire](questionnaire.html): The current status of the questionnaire 10354* [Requirements](requirements.html): The current status of the requirements 10355* [SearchParameter](searchparameter.html): The current status of the search parameter 10356* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 10357* [StructureDefinition](structuredefinition.html): The current status of the structure definition 10358* [StructureMap](structuremap.html): The current status of the structure map 10359* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 10360* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 10361* [TestPlan](testplan.html): The current status of the test plan 10362* [TestScript](testscript.html): The current status of the test script 10363* [ValueSet](valueset.html): The current status of the value set 10364</b><br> 10365 * Type: <b>token</b><br> 10366 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 10367 * </p> 10368 */ 10369 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 10370 public static final String SP_STATUS = "status"; 10371 /** 10372 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10373 * <p> 10374 * Description: <b>Multiple Resources: 10375 10376* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 10377* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 10378* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 10379* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 10380* [Citation](citation.html): The current status of the citation 10381* [CodeSystem](codesystem.html): The current status of the code system 10382* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 10383* [ConceptMap](conceptmap.html): The current status of the concept map 10384* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 10385* [EventDefinition](eventdefinition.html): The current status of the event definition 10386* [Evidence](evidence.html): The current status of the evidence 10387* [EvidenceReport](evidencereport.html): The current status of the evidence report 10388* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 10389* [ExampleScenario](examplescenario.html): The current status of the example scenario 10390* [GraphDefinition](graphdefinition.html): The current status of the graph definition 10391* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 10392* [Library](library.html): The current status of the library 10393* [Measure](measure.html): The current status of the measure 10394* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 10395* [MessageDefinition](messagedefinition.html): The current status of the message definition 10396* [NamingSystem](namingsystem.html): The current status of the naming system 10397* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 10398* [OperationDefinition](operationdefinition.html): The current status of the operation definition 10399* [PlanDefinition](plandefinition.html): The current status of the plan definition 10400* [Questionnaire](questionnaire.html): The current status of the questionnaire 10401* [Requirements](requirements.html): The current status of the requirements 10402* [SearchParameter](searchparameter.html): The current status of the search parameter 10403* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 10404* [StructureDefinition](structuredefinition.html): The current status of the structure definition 10405* [StructureMap](structuremap.html): The current status of the structure map 10406* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 10407* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 10408* [TestPlan](testplan.html): The current status of the test plan 10409* [TestScript](testscript.html): The current status of the test script 10410* [ValueSet](valueset.html): The current status of the value set 10411</b><br> 10412 * Type: <b>token</b><br> 10413 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 10414 * </p> 10415 */ 10416 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 10417 10418 /** 10419 * Search parameter: <b>title</b> 10420 * <p> 10421 * Description: <b>Multiple Resources: 10422 10423* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 10424* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 10425* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 10426* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 10427* [Citation](citation.html): The human-friendly name of the citation 10428* [CodeSystem](codesystem.html): The human-friendly name of the code system 10429* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 10430* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 10431* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 10432* [Evidence](evidence.html): The human-friendly name of the evidence 10433* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 10434* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 10435* [Library](library.html): The human-friendly name of the library 10436* [Measure](measure.html): The human-friendly name of the measure 10437* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 10438* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 10439* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 10440* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 10441* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 10442* [Requirements](requirements.html): The human-friendly name of the requirements 10443* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 10444* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 10445* [StructureMap](structuremap.html): The human-friendly name of the structure map 10446* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 10447* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 10448* [TestScript](testscript.html): The human-friendly name of the test script 10449* [ValueSet](valueset.html): The human-friendly name of the value set 10450</b><br> 10451 * Type: <b>string</b><br> 10452 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 10453 * </p> 10454 */ 10455 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 10456 public static final String SP_TITLE = "title"; 10457 /** 10458 * <b>Fluent Client</b> search parameter constant for <b>title</b> 10459 * <p> 10460 * Description: <b>Multiple Resources: 10461 10462* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 10463* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 10464* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 10465* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 10466* [Citation](citation.html): The human-friendly name of the citation 10467* [CodeSystem](codesystem.html): The human-friendly name of the code system 10468* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 10469* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 10470* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 10471* [Evidence](evidence.html): The human-friendly name of the evidence 10472* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 10473* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 10474* [Library](library.html): The human-friendly name of the library 10475* [Measure](measure.html): The human-friendly name of the measure 10476* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 10477* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 10478* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 10479* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 10480* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 10481* [Requirements](requirements.html): The human-friendly name of the requirements 10482* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 10483* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 10484* [StructureMap](structuremap.html): The human-friendly name of the structure map 10485* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 10486* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 10487* [TestScript](testscript.html): The human-friendly name of the test script 10488* [ValueSet](valueset.html): The human-friendly name of the value set 10489</b><br> 10490 * Type: <b>string</b><br> 10491 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 10492 * </p> 10493 */ 10494 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 10495 10496 /** 10497 * Search parameter: <b>url</b> 10498 * <p> 10499 * Description: <b>Multiple Resources: 10500 10501* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 10502* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 10503* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 10504* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 10505* [Citation](citation.html): The uri that identifies the citation 10506* [CodeSystem](codesystem.html): The uri that identifies the code system 10507* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 10508* [ConceptMap](conceptmap.html): The URI that identifies the concept map 10509* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 10510* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 10511* [Evidence](evidence.html): The uri that identifies the evidence 10512* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 10513* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 10514* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 10515* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 10516* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 10517* [Library](library.html): The uri that identifies the library 10518* [Measure](measure.html): The uri that identifies the measure 10519* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 10520* [NamingSystem](namingsystem.html): The uri that identifies the naming system 10521* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 10522* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 10523* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 10524* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 10525* [Requirements](requirements.html): The uri that identifies the requirements 10526* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 10527* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 10528* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 10529* [StructureMap](structuremap.html): The uri that identifies the structure map 10530* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 10531* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 10532* [TestPlan](testplan.html): The uri that identifies the test plan 10533* [TestScript](testscript.html): The uri that identifies the test script 10534* [ValueSet](valueset.html): The uri that identifies the value set 10535</b><br> 10536 * Type: <b>uri</b><br> 10537 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 10538 * </p> 10539 */ 10540 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 10541 public static final String SP_URL = "url"; 10542 /** 10543 * <b>Fluent Client</b> search parameter constant for <b>url</b> 10544 * <p> 10545 * Description: <b>Multiple Resources: 10546 10547* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 10548* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 10549* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 10550* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 10551* [Citation](citation.html): The uri that identifies the citation 10552* [CodeSystem](codesystem.html): The uri that identifies the code system 10553* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 10554* [ConceptMap](conceptmap.html): The URI that identifies the concept map 10555* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 10556* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 10557* [Evidence](evidence.html): The uri that identifies the evidence 10558* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 10559* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 10560* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 10561* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 10562* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 10563* [Library](library.html): The uri that identifies the library 10564* [Measure](measure.html): The uri that identifies the measure 10565* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 10566* [NamingSystem](namingsystem.html): The uri that identifies the naming system 10567* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 10568* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 10569* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 10570* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 10571* [Requirements](requirements.html): The uri that identifies the requirements 10572* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 10573* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 10574* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 10575* [StructureMap](structuremap.html): The uri that identifies the structure map 10576* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 10577* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 10578* [TestPlan](testplan.html): The uri that identifies the test plan 10579* [TestScript](testscript.html): The uri that identifies the test script 10580* [ValueSet](valueset.html): The uri that identifies the value set 10581</b><br> 10582 * Type: <b>uri</b><br> 10583 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 10584 * </p> 10585 */ 10586 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 10587 10588 /** 10589 * Search parameter: <b>version</b> 10590 * <p> 10591 * Description: <b>Multiple Resources: 10592 10593* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 10594* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 10595* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 10596* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 10597* [Citation](citation.html): The business version of the citation 10598* [CodeSystem](codesystem.html): The business version of the code system 10599* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 10600* [ConceptMap](conceptmap.html): The business version of the concept map 10601* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 10602* [EventDefinition](eventdefinition.html): The business version of the event definition 10603* [Evidence](evidence.html): The business version of the evidence 10604* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 10605* [ExampleScenario](examplescenario.html): The business version of the example scenario 10606* [GraphDefinition](graphdefinition.html): The business version of the graph definition 10607* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 10608* [Library](library.html): The business version of the library 10609* [Measure](measure.html): The business version of the measure 10610* [MessageDefinition](messagedefinition.html): The business version of the message definition 10611* [NamingSystem](namingsystem.html): The business version of the naming system 10612* [OperationDefinition](operationdefinition.html): The business version of the operation definition 10613* [PlanDefinition](plandefinition.html): The business version of the plan definition 10614* [Questionnaire](questionnaire.html): The business version of the questionnaire 10615* [Requirements](requirements.html): The business version of the requirements 10616* [SearchParameter](searchparameter.html): The business version of the search parameter 10617* [StructureDefinition](structuredefinition.html): The business version of the structure definition 10618* [StructureMap](structuremap.html): The business version of the structure map 10619* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 10620* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 10621* [TestScript](testscript.html): The business version of the test script 10622* [ValueSet](valueset.html): The business version of the value set 10623</b><br> 10624 * Type: <b>token</b><br> 10625 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 10626 * </p> 10627 */ 10628 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 10629 public static final String SP_VERSION = "version"; 10630 /** 10631 * <b>Fluent Client</b> search parameter constant for <b>version</b> 10632 * <p> 10633 * Description: <b>Multiple Resources: 10634 10635* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 10636* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 10637* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 10638* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 10639* [Citation](citation.html): The business version of the citation 10640* [CodeSystem](codesystem.html): The business version of the code system 10641* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 10642* [ConceptMap](conceptmap.html): The business version of the concept map 10643* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 10644* [EventDefinition](eventdefinition.html): The business version of the event definition 10645* [Evidence](evidence.html): The business version of the evidence 10646* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 10647* [ExampleScenario](examplescenario.html): The business version of the example scenario 10648* [GraphDefinition](graphdefinition.html): The business version of the graph definition 10649* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 10650* [Library](library.html): The business version of the library 10651* [Measure](measure.html): The business version of the measure 10652* [MessageDefinition](messagedefinition.html): The business version of the message definition 10653* [NamingSystem](namingsystem.html): The business version of the naming system 10654* [OperationDefinition](operationdefinition.html): The business version of the operation definition 10655* [PlanDefinition](plandefinition.html): The business version of the plan definition 10656* [Questionnaire](questionnaire.html): The business version of the questionnaire 10657* [Requirements](requirements.html): The business version of the requirements 10658* [SearchParameter](searchparameter.html): The business version of the search parameter 10659* [StructureDefinition](structuredefinition.html): The business version of the structure definition 10660* [StructureMap](structuremap.html): The business version of the structure map 10661* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 10662* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 10663* [TestScript](testscript.html): The business version of the test script 10664* [ValueSet](valueset.html): The business version of the value set 10665</b><br> 10666 * Type: <b>token</b><br> 10667 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 10668 * </p> 10669 */ 10670 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 10671 10672 /** 10673 * Search parameter: <b>fhirversion</b> 10674 * <p> 10675 * Description: <b>The version of FHIR</b><br> 10676 * Type: <b>token</b><br> 10677 * Path: <b>CapabilityStatement.fhirVersion</b><br> 10678 * </p> 10679 */ 10680 @SearchParamDefinition(name="fhirversion", path="CapabilityStatement.fhirVersion", description="The version of FHIR", type="token" ) 10681 public static final String SP_FHIRVERSION = "fhirversion"; 10682 /** 10683 * <b>Fluent Client</b> search parameter constant for <b>fhirversion</b> 10684 * <p> 10685 * Description: <b>The version of FHIR</b><br> 10686 * Type: <b>token</b><br> 10687 * Path: <b>CapabilityStatement.fhirVersion</b><br> 10688 * </p> 10689 */ 10690 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FHIRVERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FHIRVERSION); 10691 10692 /** 10693 * Search parameter: <b>format</b> 10694 * <p> 10695 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 10696 * Type: <b>token</b><br> 10697 * Path: <b>CapabilityStatement.format</b><br> 10698 * </p> 10699 */ 10700 @SearchParamDefinition(name="format", path="CapabilityStatement.format", description="formats supported (xml | json | ttl | mime type)", type="token" ) 10701 public static final String SP_FORMAT = "format"; 10702 /** 10703 * <b>Fluent Client</b> search parameter constant for <b>format</b> 10704 * <p> 10705 * Description: <b>formats supported (xml | json | ttl | mime type)</b><br> 10706 * Type: <b>token</b><br> 10707 * Path: <b>CapabilityStatement.format</b><br> 10708 * </p> 10709 */ 10710 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORMAT); 10711 10712 /** 10713 * Search parameter: <b>guide</b> 10714 * <p> 10715 * Description: <b>Implementation guides supported</b><br> 10716 * Type: <b>reference</b><br> 10717 * Path: <b>CapabilityStatement.implementationGuide</b><br> 10718 * </p> 10719 */ 10720 @SearchParamDefinition(name="guide", path="CapabilityStatement.implementationGuide", description="Implementation guides supported", type="reference", target={ImplementationGuide.class } ) 10721 public static final String SP_GUIDE = "guide"; 10722 /** 10723 * <b>Fluent Client</b> search parameter constant for <b>guide</b> 10724 * <p> 10725 * Description: <b>Implementation guides supported</b><br> 10726 * Type: <b>reference</b><br> 10727 * Path: <b>CapabilityStatement.implementationGuide</b><br> 10728 * </p> 10729 */ 10730 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GUIDE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GUIDE); 10731 10732/** 10733 * Constant for fluent queries to be used to add include statements. Specifies 10734 * the path value of "<b>CapabilityStatement:guide</b>". 10735 */ 10736 public static final ca.uhn.fhir.model.api.Include INCLUDE_GUIDE = new ca.uhn.fhir.model.api.Include("CapabilityStatement:guide").toLocked(); 10737 10738 /** 10739 * Search parameter: <b>mode</b> 10740 * <p> 10741 * Description: <b>Mode - restful (server/client) or messaging (sender/receiver)</b><br> 10742 * Type: <b>token</b><br> 10743 * Path: <b>CapabilityStatement.rest.mode</b><br> 10744 * </p> 10745 */ 10746 @SearchParamDefinition(name="mode", path="CapabilityStatement.rest.mode", description="Mode - restful (server/client) or messaging (sender/receiver)", type="token" ) 10747 public static final String SP_MODE = "mode"; 10748 /** 10749 * <b>Fluent Client</b> search parameter constant for <b>mode</b> 10750 * <p> 10751 * Description: <b>Mode - restful (server/client) or messaging (sender/receiver)</b><br> 10752 * Type: <b>token</b><br> 10753 * Path: <b>CapabilityStatement.rest.mode</b><br> 10754 * </p> 10755 */ 10756 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MODE); 10757 10758 /** 10759 * Search parameter: <b>resource-profile</b> 10760 * <p> 10761 * Description: <b>A profile id invoked in a capability statement</b><br> 10762 * Type: <b>reference</b><br> 10763 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 10764 * </p> 10765 */ 10766 @SearchParamDefinition(name="resource-profile", path="CapabilityStatement.rest.resource.profile", description="A profile id invoked in a capability statement", type="reference", target={StructureDefinition.class } ) 10767 public static final String SP_RESOURCE_PROFILE = "resource-profile"; 10768 /** 10769 * <b>Fluent Client</b> search parameter constant for <b>resource-profile</b> 10770 * <p> 10771 * Description: <b>A profile id invoked in a capability statement</b><br> 10772 * Type: <b>reference</b><br> 10773 * Path: <b>CapabilityStatement.rest.resource.profile</b><br> 10774 * </p> 10775 */ 10776 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESOURCE_PROFILE); 10777 10778/** 10779 * Constant for fluent queries to be used to add include statements. Specifies 10780 * the path value of "<b>CapabilityStatement:resource-profile</b>". 10781 */ 10782 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE_PROFILE = new ca.uhn.fhir.model.api.Include("CapabilityStatement:resource-profile").toLocked(); 10783 10784 /** 10785 * Search parameter: <b>resource</b> 10786 * <p> 10787 * Description: <b>Name of a resource mentioned in a capability statement</b><br> 10788 * Type: <b>token</b><br> 10789 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 10790 * </p> 10791 */ 10792 @SearchParamDefinition(name="resource", path="CapabilityStatement.rest.resource.type", description="Name of a resource mentioned in a capability statement", type="token" ) 10793 public static final String SP_RESOURCE = "resource"; 10794 /** 10795 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 10796 * <p> 10797 * Description: <b>Name of a resource mentioned in a capability statement</b><br> 10798 * Type: <b>token</b><br> 10799 * Path: <b>CapabilityStatement.rest.resource.type</b><br> 10800 * </p> 10801 */ 10802 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESOURCE); 10803 10804 /** 10805 * Search parameter: <b>security-service</b> 10806 * <p> 10807 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates</b><br> 10808 * Type: <b>token</b><br> 10809 * Path: <b>CapabilityStatement.rest.security.service</b><br> 10810 * </p> 10811 */ 10812 @SearchParamDefinition(name="security-service", path="CapabilityStatement.rest.security.service", description="OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates", type="token" ) 10813 public static final String SP_SECURITY_SERVICE = "security-service"; 10814 /** 10815 * <b>Fluent Client</b> search parameter constant for <b>security-service</b> 10816 * <p> 10817 * Description: <b>OAuth | SMART-on-FHIR | NTLM | Basic | Kerberos | Certificates</b><br> 10818 * Type: <b>token</b><br> 10819 * Path: <b>CapabilityStatement.rest.security.service</b><br> 10820 * </p> 10821 */ 10822 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_SERVICE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITY_SERVICE); 10823 10824 /** 10825 * Search parameter: <b>software</b> 10826 * <p> 10827 * Description: <b>Part of the name of a software application</b><br> 10828 * Type: <b>string</b><br> 10829 * Path: <b>CapabilityStatement.software.name</b><br> 10830 * </p> 10831 */ 10832 @SearchParamDefinition(name="software", path="CapabilityStatement.software.name", description="Part of the name of a software application", type="string" ) 10833 public static final String SP_SOFTWARE = "software"; 10834 /** 10835 * <b>Fluent Client</b> search parameter constant for <b>software</b> 10836 * <p> 10837 * Description: <b>Part of the name of a software application</b><br> 10838 * Type: <b>string</b><br> 10839 * Path: <b>CapabilityStatement.software.name</b><br> 10840 * </p> 10841 */ 10842 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOFTWARE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SOFTWARE); 10843 10844 /** 10845 * Search parameter: <b>supported-profile</b> 10846 * <p> 10847 * Description: <b>Profiles for use cases supported</b><br> 10848 * Type: <b>reference</b><br> 10849 * Path: <b>CapabilityStatement.rest.resource.supportedProfile</b><br> 10850 * </p> 10851 */ 10852 @SearchParamDefinition(name="supported-profile", path="CapabilityStatement.rest.resource.supportedProfile", description="Profiles for use cases supported", type="reference", target={StructureDefinition.class } ) 10853 public static final String SP_SUPPORTED_PROFILE = "supported-profile"; 10854 /** 10855 * <b>Fluent Client</b> search parameter constant for <b>supported-profile</b> 10856 * <p> 10857 * Description: <b>Profiles for use cases supported</b><br> 10858 * Type: <b>reference</b><br> 10859 * Path: <b>CapabilityStatement.rest.resource.supportedProfile</b><br> 10860 * </p> 10861 */ 10862 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTED_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPORTED_PROFILE); 10863 10864/** 10865 * Constant for fluent queries to be used to add include statements. Specifies 10866 * the path value of "<b>CapabilityStatement:supported-profile</b>". 10867 */ 10868 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTED_PROFILE = new ca.uhn.fhir.model.api.Include("CapabilityStatement:supported-profile").toLocked(); 10869 10870// Manual code (from Configuration.txt): 10871 10872// end addition 10873 10874} 10875