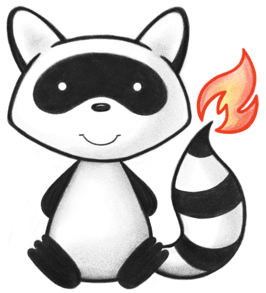
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 052 */ 053@ResourceDef(name="CarePlan", profile="http://hl7.org/fhir/StructureDefinition/CarePlan") 054public class CarePlan extends DomainResource { 055 056 public enum CarePlanIntent { 057 /** 058 * The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act. 059 */ 060 PROPOSAL, 061 /** 062 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 063 */ 064 PLAN, 065 /** 066 * The request represents a request/demand and authorization for action by the requestor. 067 */ 068 ORDER, 069 /** 070 * The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestOrchestration]]] for additional information on how this status is used. 071 */ 072 OPTION, 073 /** 074 * The request represents a legally binding instruction authored by a Patient or RelatedPerson. 075 */ 076 DIRECTIVE, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static CarePlanIntent fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("proposal".equals(codeString)) 085 return PROPOSAL; 086 if ("plan".equals(codeString)) 087 return PLAN; 088 if ("order".equals(codeString)) 089 return ORDER; 090 if ("option".equals(codeString)) 091 return OPTION; 092 if ("directive".equals(codeString)) 093 return DIRECTIVE; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown CarePlanIntent code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case PROPOSAL: return "proposal"; 102 case PLAN: return "plan"; 103 case ORDER: return "order"; 104 case OPTION: return "option"; 105 case DIRECTIVE: return "directive"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 113 case PLAN: return "http://hl7.org/fhir/request-intent"; 114 case ORDER: return "http://hl7.org/fhir/request-intent"; 115 case OPTION: return "http://hl7.org/fhir/request-intent"; 116 case DIRECTIVE: return "http://hl7.org/fhir/request-intent"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case PROPOSAL: return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 124 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 125 case ORDER: return "The request represents a request/demand and authorization for action by the requestor."; 126 case OPTION: return "The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestOrchestration]]] for additional information on how this status is used."; 127 case DIRECTIVE: return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case PROPOSAL: return "Proposal"; 135 case PLAN: return "Plan"; 136 case ORDER: return "Order"; 137 case OPTION: return "Option"; 138 case DIRECTIVE: return "Directive"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class CarePlanIntentEnumFactory implements EnumFactory<CarePlanIntent> { 146 public CarePlanIntent fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("proposal".equals(codeString)) 151 return CarePlanIntent.PROPOSAL; 152 if ("plan".equals(codeString)) 153 return CarePlanIntent.PLAN; 154 if ("order".equals(codeString)) 155 return CarePlanIntent.ORDER; 156 if ("option".equals(codeString)) 157 return CarePlanIntent.OPTION; 158 if ("directive".equals(codeString)) 159 return CarePlanIntent.DIRECTIVE; 160 throw new IllegalArgumentException("Unknown CarePlanIntent code '"+codeString+"'"); 161 } 162 public Enumeration<CarePlanIntent> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.NULL, code); 170 if ("proposal".equals(codeString)) 171 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PROPOSAL, code); 172 if ("plan".equals(codeString)) 173 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.PLAN, code); 174 if ("order".equals(codeString)) 175 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.ORDER, code); 176 if ("option".equals(codeString)) 177 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.OPTION, code); 178 if ("directive".equals(codeString)) 179 return new Enumeration<CarePlanIntent>(this, CarePlanIntent.DIRECTIVE, code); 180 throw new FHIRException("Unknown CarePlanIntent code '"+codeString+"'"); 181 } 182 public String toCode(CarePlanIntent code) { 183 if (code == CarePlanIntent.NULL) 184 return null; 185 if (code == CarePlanIntent.PROPOSAL) 186 return "proposal"; 187 if (code == CarePlanIntent.PLAN) 188 return "plan"; 189 if (code == CarePlanIntent.ORDER) 190 return "order"; 191 if (code == CarePlanIntent.OPTION) 192 return "option"; 193 if (code == CarePlanIntent.DIRECTIVE) 194 return "directive"; 195 return "?"; 196 } 197 public String toSystem(CarePlanIntent code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class CarePlanActivityComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * Identifies the activity that was performed. For example, an activity could be patient education, exercise, or a medication administration. The reference to an "event" resource, such as Procedure or Encounter or Observation, represents the activity that was performed. The requested activity can be conveyed using the CarePlan.activity.plannedActivityReference (a reference to a ?request? resource). 206 */ 207 @Child(name = "performedActivity", type = {CodeableReference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 208 @Description(shortDefinition="Results of the activity (concept, or Appointment, Encounter, Procedure, etc.)", formalDefinition="Identifies the activity that was performed. For example, an activity could be patient education, exercise, or a medication administration. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, represents the activity that was performed. The requested activity can be conveyed using the CarePlan.activity.plannedActivityReference (a reference to a ?request? resource)." ) 209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-activity-performed") 210 protected List<CodeableReference> performedActivity; 211 212 /** 213 * Notes about the adherence/status/progress of the activity. 214 */ 215 @Child(name = "progress", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 216 @Description(shortDefinition="Comments about the activity status/progress", formalDefinition="Notes about the adherence/status/progress of the activity." ) 217 protected List<Annotation> progress; 218 219 /** 220 * The details of the proposed activity represented in a specific resource. 221 */ 222 @Child(name = "plannedActivityReference", type = {Appointment.class, CommunicationRequest.class, DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, Task.class, ServiceRequest.class, VisionPrescription.class, RequestOrchestration.class, ImmunizationRecommendation.class, SupplyRequest.class}, order=3, min=0, max=1, modifier=false, summary=false) 223 @Description(shortDefinition="Activity that is intended to be part of the care plan", formalDefinition="The details of the proposed activity represented in a specific resource." ) 224 protected Reference plannedActivityReference; 225 226 private static final long serialVersionUID = 1416911432L; 227 228 /** 229 * Constructor 230 */ 231 public CarePlanActivityComponent() { 232 super(); 233 } 234 235 /** 236 * @return {@link #performedActivity} (Identifies the activity that was performed. For example, an activity could be patient education, exercise, or a medication administration. The reference to an "event" resource, such as Procedure or Encounter or Observation, represents the activity that was performed. The requested activity can be conveyed using the CarePlan.activity.plannedActivityReference (a reference to a ?request? resource).) 237 */ 238 public List<CodeableReference> getPerformedActivity() { 239 if (this.performedActivity == null) 240 this.performedActivity = new ArrayList<CodeableReference>(); 241 return this.performedActivity; 242 } 243 244 /** 245 * @return Returns a reference to <code>this</code> for easy method chaining 246 */ 247 public CarePlanActivityComponent setPerformedActivity(List<CodeableReference> thePerformedActivity) { 248 this.performedActivity = thePerformedActivity; 249 return this; 250 } 251 252 public boolean hasPerformedActivity() { 253 if (this.performedActivity == null) 254 return false; 255 for (CodeableReference item : this.performedActivity) 256 if (!item.isEmpty()) 257 return true; 258 return false; 259 } 260 261 public CodeableReference addPerformedActivity() { //3 262 CodeableReference t = new CodeableReference(); 263 if (this.performedActivity == null) 264 this.performedActivity = new ArrayList<CodeableReference>(); 265 this.performedActivity.add(t); 266 return t; 267 } 268 269 public CarePlanActivityComponent addPerformedActivity(CodeableReference t) { //3 270 if (t == null) 271 return this; 272 if (this.performedActivity == null) 273 this.performedActivity = new ArrayList<CodeableReference>(); 274 this.performedActivity.add(t); 275 return this; 276 } 277 278 /** 279 * @return The first repetition of repeating field {@link #performedActivity}, creating it if it does not already exist {3} 280 */ 281 public CodeableReference getPerformedActivityFirstRep() { 282 if (getPerformedActivity().isEmpty()) { 283 addPerformedActivity(); 284 } 285 return getPerformedActivity().get(0); 286 } 287 288 /** 289 * @return {@link #progress} (Notes about the adherence/status/progress of the activity.) 290 */ 291 public List<Annotation> getProgress() { 292 if (this.progress == null) 293 this.progress = new ArrayList<Annotation>(); 294 return this.progress; 295 } 296 297 /** 298 * @return Returns a reference to <code>this</code> for easy method chaining 299 */ 300 public CarePlanActivityComponent setProgress(List<Annotation> theProgress) { 301 this.progress = theProgress; 302 return this; 303 } 304 305 public boolean hasProgress() { 306 if (this.progress == null) 307 return false; 308 for (Annotation item : this.progress) 309 if (!item.isEmpty()) 310 return true; 311 return false; 312 } 313 314 public Annotation addProgress() { //3 315 Annotation t = new Annotation(); 316 if (this.progress == null) 317 this.progress = new ArrayList<Annotation>(); 318 this.progress.add(t); 319 return t; 320 } 321 322 public CarePlanActivityComponent addProgress(Annotation t) { //3 323 if (t == null) 324 return this; 325 if (this.progress == null) 326 this.progress = new ArrayList<Annotation>(); 327 this.progress.add(t); 328 return this; 329 } 330 331 /** 332 * @return The first repetition of repeating field {@link #progress}, creating it if it does not already exist {3} 333 */ 334 public Annotation getProgressFirstRep() { 335 if (getProgress().isEmpty()) { 336 addProgress(); 337 } 338 return getProgress().get(0); 339 } 340 341 /** 342 * @return {@link #plannedActivityReference} (The details of the proposed activity represented in a specific resource.) 343 */ 344 public Reference getPlannedActivityReference() { 345 if (this.plannedActivityReference == null) 346 if (Configuration.errorOnAutoCreate()) 347 throw new Error("Attempt to auto-create CarePlanActivityComponent.plannedActivityReference"); 348 else if (Configuration.doAutoCreate()) 349 this.plannedActivityReference = new Reference(); // cc 350 return this.plannedActivityReference; 351 } 352 353 public boolean hasPlannedActivityReference() { 354 return this.plannedActivityReference != null && !this.plannedActivityReference.isEmpty(); 355 } 356 357 /** 358 * @param value {@link #plannedActivityReference} (The details of the proposed activity represented in a specific resource.) 359 */ 360 public CarePlanActivityComponent setPlannedActivityReference(Reference value) { 361 this.plannedActivityReference = value; 362 return this; 363 } 364 365 protected void listChildren(List<Property> children) { 366 super.listChildren(children); 367 children.add(new Property("performedActivity", "CodeableReference(Any)", "Identifies the activity that was performed. For example, an activity could be patient education, exercise, or a medication administration. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, represents the activity that was performed. The requested activity can be conveyed using the CarePlan.activity.plannedActivityReference (a reference to a ?request? resource).", 0, java.lang.Integer.MAX_VALUE, performedActivity)); 368 children.add(new Property("progress", "Annotation", "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress)); 369 children.add(new Property("plannedActivityReference", "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ServiceRequest|VisionPrescription|RequestOrchestration|ImmunizationRecommendation|SupplyRequest)", "The details of the proposed activity represented in a specific resource.", 0, 1, plannedActivityReference)); 370 } 371 372 @Override 373 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 374 switch (_hash) { 375 case 1964521199: /*performedActivity*/ return new Property("performedActivity", "CodeableReference(Any)", "Identifies the activity that was performed. For example, an activity could be patient education, exercise, or a medication administration. The reference to an \"event\" resource, such as Procedure or Encounter or Observation, represents the activity that was performed. The requested activity can be conveyed using the CarePlan.activity.plannedActivityReference (a reference to a ?request? resource).", 0, java.lang.Integer.MAX_VALUE, performedActivity); 376 case -1001078227: /*progress*/ return new Property("progress", "Annotation", "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress); 377 case -1114371176: /*plannedActivityReference*/ return new Property("plannedActivityReference", "Reference(Appointment|CommunicationRequest|DeviceRequest|MedicationRequest|NutritionOrder|Task|ServiceRequest|VisionPrescription|RequestOrchestration|ImmunizationRecommendation|SupplyRequest)", "The details of the proposed activity represented in a specific resource.", 0, 1, plannedActivityReference); 378 default: return super.getNamedProperty(_hash, _name, _checkValid); 379 } 380 381 } 382 383 @Override 384 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 385 switch (hash) { 386 case 1964521199: /*performedActivity*/ return this.performedActivity == null ? new Base[0] : this.performedActivity.toArray(new Base[this.performedActivity.size()]); // CodeableReference 387 case -1001078227: /*progress*/ return this.progress == null ? new Base[0] : this.progress.toArray(new Base[this.progress.size()]); // Annotation 388 case -1114371176: /*plannedActivityReference*/ return this.plannedActivityReference == null ? new Base[0] : new Base[] {this.plannedActivityReference}; // Reference 389 default: return super.getProperty(hash, name, checkValid); 390 } 391 392 } 393 394 @Override 395 public Base setProperty(int hash, String name, Base value) throws FHIRException { 396 switch (hash) { 397 case 1964521199: // performedActivity 398 this.getPerformedActivity().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 399 return value; 400 case -1001078227: // progress 401 this.getProgress().add(TypeConvertor.castToAnnotation(value)); // Annotation 402 return value; 403 case -1114371176: // plannedActivityReference 404 this.plannedActivityReference = TypeConvertor.castToReference(value); // Reference 405 return value; 406 default: return super.setProperty(hash, name, value); 407 } 408 409 } 410 411 @Override 412 public Base setProperty(String name, Base value) throws FHIRException { 413 if (name.equals("performedActivity")) { 414 this.getPerformedActivity().add(TypeConvertor.castToCodeableReference(value)); 415 } else if (name.equals("progress")) { 416 this.getProgress().add(TypeConvertor.castToAnnotation(value)); 417 } else if (name.equals("plannedActivityReference")) { 418 this.plannedActivityReference = TypeConvertor.castToReference(value); // Reference 419 } else 420 return super.setProperty(name, value); 421 return value; 422 } 423 424 @Override 425 public void removeChild(String name, Base value) throws FHIRException { 426 if (name.equals("performedActivity")) { 427 this.getPerformedActivity().remove(value); 428 } else if (name.equals("progress")) { 429 this.getProgress().remove(value); 430 } else if (name.equals("plannedActivityReference")) { 431 this.plannedActivityReference = null; 432 } else 433 super.removeChild(name, value); 434 435 } 436 437 @Override 438 public Base makeProperty(int hash, String name) throws FHIRException { 439 switch (hash) { 440 case 1964521199: return addPerformedActivity(); 441 case -1001078227: return addProgress(); 442 case -1114371176: return getPlannedActivityReference(); 443 default: return super.makeProperty(hash, name); 444 } 445 446 } 447 448 @Override 449 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 450 switch (hash) { 451 case 1964521199: /*performedActivity*/ return new String[] {"CodeableReference"}; 452 case -1001078227: /*progress*/ return new String[] {"Annotation"}; 453 case -1114371176: /*plannedActivityReference*/ return new String[] {"Reference"}; 454 default: return super.getTypesForProperty(hash, name); 455 } 456 457 } 458 459 @Override 460 public Base addChild(String name) throws FHIRException { 461 if (name.equals("performedActivity")) { 462 return addPerformedActivity(); 463 } 464 else if (name.equals("progress")) { 465 return addProgress(); 466 } 467 else if (name.equals("plannedActivityReference")) { 468 this.plannedActivityReference = new Reference(); 469 return this.plannedActivityReference; 470 } 471 else 472 return super.addChild(name); 473 } 474 475 public CarePlanActivityComponent copy() { 476 CarePlanActivityComponent dst = new CarePlanActivityComponent(); 477 copyValues(dst); 478 return dst; 479 } 480 481 public void copyValues(CarePlanActivityComponent dst) { 482 super.copyValues(dst); 483 if (performedActivity != null) { 484 dst.performedActivity = new ArrayList<CodeableReference>(); 485 for (CodeableReference i : performedActivity) 486 dst.performedActivity.add(i.copy()); 487 }; 488 if (progress != null) { 489 dst.progress = new ArrayList<Annotation>(); 490 for (Annotation i : progress) 491 dst.progress.add(i.copy()); 492 }; 493 dst.plannedActivityReference = plannedActivityReference == null ? null : plannedActivityReference.copy(); 494 } 495 496 @Override 497 public boolean equalsDeep(Base other_) { 498 if (!super.equalsDeep(other_)) 499 return false; 500 if (!(other_ instanceof CarePlanActivityComponent)) 501 return false; 502 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 503 return compareDeep(performedActivity, o.performedActivity, true) && compareDeep(progress, o.progress, true) 504 && compareDeep(plannedActivityReference, o.plannedActivityReference, true); 505 } 506 507 @Override 508 public boolean equalsShallow(Base other_) { 509 if (!super.equalsShallow(other_)) 510 return false; 511 if (!(other_ instanceof CarePlanActivityComponent)) 512 return false; 513 CarePlanActivityComponent o = (CarePlanActivityComponent) other_; 514 return true; 515 } 516 517 public boolean isEmpty() { 518 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(performedActivity, progress 519 , plannedActivityReference); 520 } 521 522 public String fhirType() { 523 return "CarePlan.activity"; 524 525 } 526 527 } 528 529 /** 530 * Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 531 */ 532 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 533 @Description(shortDefinition="External Ids for this plan", formalDefinition="Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 534 protected List<Identifier> identifier; 535 536 /** 537 * The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan. 538 */ 539 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 540 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan." ) 541 protected List<CanonicalType> instantiatesCanonical; 542 543 /** 544 * The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan. 545 */ 546 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 547 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan." ) 548 protected List<UriType> instantiatesUri; 549 550 /** 551 * A higher-level request resource (i.e. a plan, proposal or order) that is fulfilled in whole or in part by this care plan. 552 */ 553 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class, RequestOrchestration.class, NutritionOrder.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 554 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A higher-level request resource (i.e. a plan, proposal or order) that is fulfilled in whole or in part by this care plan." ) 555 protected List<Reference> basedOn; 556 557 /** 558 * Completed or terminated care plan whose function is taken by this new care plan. 559 */ 560 @Child(name = "replaces", type = {CarePlan.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 561 @Description(shortDefinition="CarePlan replaced by this CarePlan", formalDefinition="Completed or terminated care plan whose function is taken by this new care plan." ) 562 protected List<Reference> replaces; 563 564 /** 565 * A larger care plan of which this particular care plan is a component or step. 566 */ 567 @Child(name = "partOf", type = {CarePlan.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 568 @Description(shortDefinition="Part of referenced CarePlan", formalDefinition="A larger care plan of which this particular care plan is a component or step." ) 569 protected List<Reference> partOf; 570 571 /** 572 * Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record. 573 */ 574 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 575 @Description(shortDefinition="draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition="Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record." ) 576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 577 protected Enumeration<RequestStatus> status; 578 579 /** 580 * Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain. 581 */ 582 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 583 @Description(shortDefinition="proposal | plan | order | option | directive", formalDefinition="Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain." ) 584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-intent") 585 protected Enumeration<CarePlanIntent> intent; 586 587 /** 588 * Identifies what "kind" of plan this is to support differentiation between multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", "disease management", "wellness plan", etc. 589 */ 590 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 591 @Description(shortDefinition="Type of plan", formalDefinition="Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc." ) 592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-plan-category") 593 protected List<CodeableConcept> category; 594 595 /** 596 * Human-friendly name for the care plan. 597 */ 598 @Child(name = "title", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 599 @Description(shortDefinition="Human-friendly name for the care plan", formalDefinition="Human-friendly name for the care plan." ) 600 protected StringType title; 601 602 /** 603 * A description of the scope and nature of the plan. 604 */ 605 @Child(name = "description", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 606 @Description(shortDefinition="Summary of nature of plan", formalDefinition="A description of the scope and nature of the plan." ) 607 protected StringType description; 608 609 /** 610 * Identifies the patient or group whose intended care is described by the plan. 611 */ 612 @Child(name = "subject", type = {Patient.class, Group.class}, order=11, min=1, max=1, modifier=false, summary=true) 613 @Description(shortDefinition="Who the care plan is for", formalDefinition="Identifies the patient or group whose intended care is described by the plan." ) 614 protected Reference subject; 615 616 /** 617 * The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated. 618 */ 619 @Child(name = "encounter", type = {Encounter.class}, order=12, min=0, max=1, modifier=false, summary=true) 620 @Description(shortDefinition="The Encounter during which this CarePlan was created", formalDefinition="The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated." ) 621 protected Reference encounter; 622 623 /** 624 * Indicates when the plan did (or is intended to) come into effect and end. 625 */ 626 @Child(name = "period", type = {Period.class}, order=13, min=0, max=1, modifier=false, summary=true) 627 @Description(shortDefinition="Time period plan covers", formalDefinition="Indicates when the plan did (or is intended to) come into effect and end." ) 628 protected Period period; 629 630 /** 631 * Represents when this particular CarePlan record was created in the system, which is often a system-generated date. 632 */ 633 @Child(name = "created", type = {DateTimeType.class}, order=14, min=0, max=1, modifier=false, summary=true) 634 @Description(shortDefinition="Date record was first recorded", formalDefinition="Represents when this particular CarePlan record was created in the system, which is often a system-generated date." ) 635 protected DateTimeType created; 636 637 /** 638 * When populated, the custodian is responsible for the care plan. The care plan is attributed to the custodian. 639 */ 640 @Child(name = "custodian", type = {Patient.class, Practitioner.class, PractitionerRole.class, Device.class, RelatedPerson.class, Organization.class, CareTeam.class}, order=15, min=0, max=1, modifier=false, summary=true) 641 @Description(shortDefinition="Who is the designated responsible party", formalDefinition="When populated, the custodian is responsible for the care plan. The care plan is attributed to the custodian." ) 642 protected Reference custodian; 643 644 /** 645 * Identifies the individual(s), organization or device who provided the contents of the care plan. 646 */ 647 @Child(name = "contributor", type = {Patient.class, Practitioner.class, PractitionerRole.class, Device.class, RelatedPerson.class, Organization.class, CareTeam.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 648 @Description(shortDefinition="Who provided the content of the care plan", formalDefinition="Identifies the individual(s), organization or device who provided the contents of the care plan." ) 649 protected List<Reference> contributor; 650 651 /** 652 * Identifies all people and organizations who are expected to be involved in the care envisioned by this plan. 653 */ 654 @Child(name = "careTeam", type = {CareTeam.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 655 @Description(shortDefinition="Who's involved in plan?", formalDefinition="Identifies all people and organizations who are expected to be involved in the care envisioned by this plan." ) 656 protected List<Reference> careTeam; 657 658 /** 659 * Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan. 660 */ 661 @Child(name = "addresses", type = {CodeableReference.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 662 @Description(shortDefinition="Health issues this plan addresses", formalDefinition="Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan." ) 663 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 664 protected List<CodeableReference> addresses; 665 666 /** 667 * Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc. 668 */ 669 @Child(name = "supportingInfo", type = {Reference.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 670 @Description(shortDefinition="Information considered as part of plan", formalDefinition="Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc." ) 671 protected List<Reference> supportingInfo; 672 673 /** 674 * Describes the intended objective(s) of carrying out the care plan. 675 */ 676 @Child(name = "goal", type = {Goal.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 677 @Description(shortDefinition="Desired outcome of plan", formalDefinition="Describes the intended objective(s) of carrying out the care plan." ) 678 protected List<Reference> goal; 679 680 /** 681 * Identifies an action that has occurred or is a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring that has occurred, education etc. 682 */ 683 @Child(name = "activity", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 684 @Description(shortDefinition="Action to occur or has occurred as part of plan", formalDefinition="Identifies an action that has occurred or is a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring that has occurred, education etc." ) 685 protected List<CarePlanActivityComponent> activity; 686 687 /** 688 * General notes about the care plan not covered elsewhere. 689 */ 690 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 691 @Description(shortDefinition="Comments about the plan", formalDefinition="General notes about the care plan not covered elsewhere." ) 692 protected List<Annotation> note; 693 694 private static final long serialVersionUID = -700769298L; 695 696 /** 697 * Constructor 698 */ 699 public CarePlan() { 700 super(); 701 } 702 703 /** 704 * Constructor 705 */ 706 public CarePlan(RequestStatus status, CarePlanIntent intent, Reference subject) { 707 super(); 708 this.setStatus(status); 709 this.setIntent(intent); 710 this.setSubject(subject); 711 } 712 713 /** 714 * @return {@link #identifier} (Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 715 */ 716 public List<Identifier> getIdentifier() { 717 if (this.identifier == null) 718 this.identifier = new ArrayList<Identifier>(); 719 return this.identifier; 720 } 721 722 /** 723 * @return Returns a reference to <code>this</code> for easy method chaining 724 */ 725 public CarePlan setIdentifier(List<Identifier> theIdentifier) { 726 this.identifier = theIdentifier; 727 return this; 728 } 729 730 public boolean hasIdentifier() { 731 if (this.identifier == null) 732 return false; 733 for (Identifier item : this.identifier) 734 if (!item.isEmpty()) 735 return true; 736 return false; 737 } 738 739 public Identifier addIdentifier() { //3 740 Identifier t = new Identifier(); 741 if (this.identifier == null) 742 this.identifier = new ArrayList<Identifier>(); 743 this.identifier.add(t); 744 return t; 745 } 746 747 public CarePlan addIdentifier(Identifier t) { //3 748 if (t == null) 749 return this; 750 if (this.identifier == null) 751 this.identifier = new ArrayList<Identifier>(); 752 this.identifier.add(t); 753 return this; 754 } 755 756 /** 757 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 758 */ 759 public Identifier getIdentifierFirstRep() { 760 if (getIdentifier().isEmpty()) { 761 addIdentifier(); 762 } 763 return getIdentifier().get(0); 764 } 765 766 /** 767 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 768 */ 769 public List<CanonicalType> getInstantiatesCanonical() { 770 if (this.instantiatesCanonical == null) 771 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 772 return this.instantiatesCanonical; 773 } 774 775 /** 776 * @return Returns a reference to <code>this</code> for easy method chaining 777 */ 778 public CarePlan setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 779 this.instantiatesCanonical = theInstantiatesCanonical; 780 return this; 781 } 782 783 public boolean hasInstantiatesCanonical() { 784 if (this.instantiatesCanonical == null) 785 return false; 786 for (CanonicalType item : this.instantiatesCanonical) 787 if (!item.isEmpty()) 788 return true; 789 return false; 790 } 791 792 /** 793 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 794 */ 795 public CanonicalType addInstantiatesCanonicalElement() {//2 796 CanonicalType t = new CanonicalType(); 797 if (this.instantiatesCanonical == null) 798 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 799 this.instantiatesCanonical.add(t); 800 return t; 801 } 802 803 /** 804 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 805 */ 806 public CarePlan addInstantiatesCanonical(String value) { //1 807 CanonicalType t = new CanonicalType(); 808 t.setValue(value); 809 if (this.instantiatesCanonical == null) 810 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 811 this.instantiatesCanonical.add(t); 812 return this; 813 } 814 815 /** 816 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 817 */ 818 public boolean hasInstantiatesCanonical(String value) { 819 if (this.instantiatesCanonical == null) 820 return false; 821 for (CanonicalType v : this.instantiatesCanonical) 822 if (v.getValue().equals(value)) // canonical 823 return true; 824 return false; 825 } 826 827 /** 828 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 829 */ 830 public List<UriType> getInstantiatesUri() { 831 if (this.instantiatesUri == null) 832 this.instantiatesUri = new ArrayList<UriType>(); 833 return this.instantiatesUri; 834 } 835 836 /** 837 * @return Returns a reference to <code>this</code> for easy method chaining 838 */ 839 public CarePlan setInstantiatesUri(List<UriType> theInstantiatesUri) { 840 this.instantiatesUri = theInstantiatesUri; 841 return this; 842 } 843 844 public boolean hasInstantiatesUri() { 845 if (this.instantiatesUri == null) 846 return false; 847 for (UriType item : this.instantiatesUri) 848 if (!item.isEmpty()) 849 return true; 850 return false; 851 } 852 853 /** 854 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 855 */ 856 public UriType addInstantiatesUriElement() {//2 857 UriType t = new UriType(); 858 if (this.instantiatesUri == null) 859 this.instantiatesUri = new ArrayList<UriType>(); 860 this.instantiatesUri.add(t); 861 return t; 862 } 863 864 /** 865 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 866 */ 867 public CarePlan addInstantiatesUri(String value) { //1 868 UriType t = new UriType(); 869 t.setValue(value); 870 if (this.instantiatesUri == null) 871 this.instantiatesUri = new ArrayList<UriType>(); 872 this.instantiatesUri.add(t); 873 return this; 874 } 875 876 /** 877 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.) 878 */ 879 public boolean hasInstantiatesUri(String value) { 880 if (this.instantiatesUri == null) 881 return false; 882 for (UriType v : this.instantiatesUri) 883 if (v.getValue().equals(value)) // uri 884 return true; 885 return false; 886 } 887 888 /** 889 * @return {@link #basedOn} (A higher-level request resource (i.e. a plan, proposal or order) that is fulfilled in whole or in part by this care plan.) 890 */ 891 public List<Reference> getBasedOn() { 892 if (this.basedOn == null) 893 this.basedOn = new ArrayList<Reference>(); 894 return this.basedOn; 895 } 896 897 /** 898 * @return Returns a reference to <code>this</code> for easy method chaining 899 */ 900 public CarePlan setBasedOn(List<Reference> theBasedOn) { 901 this.basedOn = theBasedOn; 902 return this; 903 } 904 905 public boolean hasBasedOn() { 906 if (this.basedOn == null) 907 return false; 908 for (Reference item : this.basedOn) 909 if (!item.isEmpty()) 910 return true; 911 return false; 912 } 913 914 public Reference addBasedOn() { //3 915 Reference t = new Reference(); 916 if (this.basedOn == null) 917 this.basedOn = new ArrayList<Reference>(); 918 this.basedOn.add(t); 919 return t; 920 } 921 922 public CarePlan addBasedOn(Reference t) { //3 923 if (t == null) 924 return this; 925 if (this.basedOn == null) 926 this.basedOn = new ArrayList<Reference>(); 927 this.basedOn.add(t); 928 return this; 929 } 930 931 /** 932 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 933 */ 934 public Reference getBasedOnFirstRep() { 935 if (getBasedOn().isEmpty()) { 936 addBasedOn(); 937 } 938 return getBasedOn().get(0); 939 } 940 941 /** 942 * @return {@link #replaces} (Completed or terminated care plan whose function is taken by this new care plan.) 943 */ 944 public List<Reference> getReplaces() { 945 if (this.replaces == null) 946 this.replaces = new ArrayList<Reference>(); 947 return this.replaces; 948 } 949 950 /** 951 * @return Returns a reference to <code>this</code> for easy method chaining 952 */ 953 public CarePlan setReplaces(List<Reference> theReplaces) { 954 this.replaces = theReplaces; 955 return this; 956 } 957 958 public boolean hasReplaces() { 959 if (this.replaces == null) 960 return false; 961 for (Reference item : this.replaces) 962 if (!item.isEmpty()) 963 return true; 964 return false; 965 } 966 967 public Reference addReplaces() { //3 968 Reference t = new Reference(); 969 if (this.replaces == null) 970 this.replaces = new ArrayList<Reference>(); 971 this.replaces.add(t); 972 return t; 973 } 974 975 public CarePlan addReplaces(Reference t) { //3 976 if (t == null) 977 return this; 978 if (this.replaces == null) 979 this.replaces = new ArrayList<Reference>(); 980 this.replaces.add(t); 981 return this; 982 } 983 984 /** 985 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist {3} 986 */ 987 public Reference getReplacesFirstRep() { 988 if (getReplaces().isEmpty()) { 989 addReplaces(); 990 } 991 return getReplaces().get(0); 992 } 993 994 /** 995 * @return {@link #partOf} (A larger care plan of which this particular care plan is a component or step.) 996 */ 997 public List<Reference> getPartOf() { 998 if (this.partOf == null) 999 this.partOf = new ArrayList<Reference>(); 1000 return this.partOf; 1001 } 1002 1003 /** 1004 * @return Returns a reference to <code>this</code> for easy method chaining 1005 */ 1006 public CarePlan setPartOf(List<Reference> thePartOf) { 1007 this.partOf = thePartOf; 1008 return this; 1009 } 1010 1011 public boolean hasPartOf() { 1012 if (this.partOf == null) 1013 return false; 1014 for (Reference item : this.partOf) 1015 if (!item.isEmpty()) 1016 return true; 1017 return false; 1018 } 1019 1020 public Reference addPartOf() { //3 1021 Reference t = new Reference(); 1022 if (this.partOf == null) 1023 this.partOf = new ArrayList<Reference>(); 1024 this.partOf.add(t); 1025 return t; 1026 } 1027 1028 public CarePlan addPartOf(Reference t) { //3 1029 if (t == null) 1030 return this; 1031 if (this.partOf == null) 1032 this.partOf = new ArrayList<Reference>(); 1033 this.partOf.add(t); 1034 return this; 1035 } 1036 1037 /** 1038 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1039 */ 1040 public Reference getPartOfFirstRep() { 1041 if (getPartOf().isEmpty()) { 1042 addPartOf(); 1043 } 1044 return getPartOf().get(0); 1045 } 1046 1047 /** 1048 * @return {@link #status} (Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1049 */ 1050 public Enumeration<RequestStatus> getStatusElement() { 1051 if (this.status == null) 1052 if (Configuration.errorOnAutoCreate()) 1053 throw new Error("Attempt to auto-create CarePlan.status"); 1054 else if (Configuration.doAutoCreate()) 1055 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 1056 return this.status; 1057 } 1058 1059 public boolean hasStatusElement() { 1060 return this.status != null && !this.status.isEmpty(); 1061 } 1062 1063 public boolean hasStatus() { 1064 return this.status != null && !this.status.isEmpty(); 1065 } 1066 1067 /** 1068 * @param value {@link #status} (Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1069 */ 1070 public CarePlan setStatusElement(Enumeration<RequestStatus> value) { 1071 this.status = value; 1072 return this; 1073 } 1074 1075 /** 1076 * @return Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record. 1077 */ 1078 public RequestStatus getStatus() { 1079 return this.status == null ? null : this.status.getValue(); 1080 } 1081 1082 /** 1083 * @param value Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record. 1084 */ 1085 public CarePlan setStatus(RequestStatus value) { 1086 if (this.status == null) 1087 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 1088 this.status.setValue(value); 1089 return this; 1090 } 1091 1092 /** 1093 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1094 */ 1095 public Enumeration<CarePlanIntent> getIntentElement() { 1096 if (this.intent == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create CarePlan.intent"); 1099 else if (Configuration.doAutoCreate()) 1100 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); // bb 1101 return this.intent; 1102 } 1103 1104 public boolean hasIntentElement() { 1105 return this.intent != null && !this.intent.isEmpty(); 1106 } 1107 1108 public boolean hasIntent() { 1109 return this.intent != null && !this.intent.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1114 */ 1115 public CarePlan setIntentElement(Enumeration<CarePlanIntent> value) { 1116 this.intent = value; 1117 return this; 1118 } 1119 1120 /** 1121 * @return Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain. 1122 */ 1123 public CarePlanIntent getIntent() { 1124 return this.intent == null ? null : this.intent.getValue(); 1125 } 1126 1127 /** 1128 * @param value Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain. 1129 */ 1130 public CarePlan setIntent(CarePlanIntent value) { 1131 if (this.intent == null) 1132 this.intent = new Enumeration<CarePlanIntent>(new CarePlanIntentEnumFactory()); 1133 this.intent.setValue(value); 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #category} (Identifies what "kind" of plan this is to support differentiation between multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", "disease management", "wellness plan", etc.) 1139 */ 1140 public List<CodeableConcept> getCategory() { 1141 if (this.category == null) 1142 this.category = new ArrayList<CodeableConcept>(); 1143 return this.category; 1144 } 1145 1146 /** 1147 * @return Returns a reference to <code>this</code> for easy method chaining 1148 */ 1149 public CarePlan setCategory(List<CodeableConcept> theCategory) { 1150 this.category = theCategory; 1151 return this; 1152 } 1153 1154 public boolean hasCategory() { 1155 if (this.category == null) 1156 return false; 1157 for (CodeableConcept item : this.category) 1158 if (!item.isEmpty()) 1159 return true; 1160 return false; 1161 } 1162 1163 public CodeableConcept addCategory() { //3 1164 CodeableConcept t = new CodeableConcept(); 1165 if (this.category == null) 1166 this.category = new ArrayList<CodeableConcept>(); 1167 this.category.add(t); 1168 return t; 1169 } 1170 1171 public CarePlan addCategory(CodeableConcept t) { //3 1172 if (t == null) 1173 return this; 1174 if (this.category == null) 1175 this.category = new ArrayList<CodeableConcept>(); 1176 this.category.add(t); 1177 return this; 1178 } 1179 1180 /** 1181 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1182 */ 1183 public CodeableConcept getCategoryFirstRep() { 1184 if (getCategory().isEmpty()) { 1185 addCategory(); 1186 } 1187 return getCategory().get(0); 1188 } 1189 1190 /** 1191 * @return {@link #title} (Human-friendly name for the care plan.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1192 */ 1193 public StringType getTitleElement() { 1194 if (this.title == null) 1195 if (Configuration.errorOnAutoCreate()) 1196 throw new Error("Attempt to auto-create CarePlan.title"); 1197 else if (Configuration.doAutoCreate()) 1198 this.title = new StringType(); // bb 1199 return this.title; 1200 } 1201 1202 public boolean hasTitleElement() { 1203 return this.title != null && !this.title.isEmpty(); 1204 } 1205 1206 public boolean hasTitle() { 1207 return this.title != null && !this.title.isEmpty(); 1208 } 1209 1210 /** 1211 * @param value {@link #title} (Human-friendly name for the care plan.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1212 */ 1213 public CarePlan setTitleElement(StringType value) { 1214 this.title = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return Human-friendly name for the care plan. 1220 */ 1221 public String getTitle() { 1222 return this.title == null ? null : this.title.getValue(); 1223 } 1224 1225 /** 1226 * @param value Human-friendly name for the care plan. 1227 */ 1228 public CarePlan setTitle(String value) { 1229 if (Utilities.noString(value)) 1230 this.title = null; 1231 else { 1232 if (this.title == null) 1233 this.title = new StringType(); 1234 this.title.setValue(value); 1235 } 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #description} (A description of the scope and nature of the plan.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1241 */ 1242 public StringType getDescriptionElement() { 1243 if (this.description == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create CarePlan.description"); 1246 else if (Configuration.doAutoCreate()) 1247 this.description = new StringType(); // bb 1248 return this.description; 1249 } 1250 1251 public boolean hasDescriptionElement() { 1252 return this.description != null && !this.description.isEmpty(); 1253 } 1254 1255 public boolean hasDescription() { 1256 return this.description != null && !this.description.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #description} (A description of the scope and nature of the plan.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1261 */ 1262 public CarePlan setDescriptionElement(StringType value) { 1263 this.description = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return A description of the scope and nature of the plan. 1269 */ 1270 public String getDescription() { 1271 return this.description == null ? null : this.description.getValue(); 1272 } 1273 1274 /** 1275 * @param value A description of the scope and nature of the plan. 1276 */ 1277 public CarePlan setDescription(String value) { 1278 if (Utilities.noString(value)) 1279 this.description = null; 1280 else { 1281 if (this.description == null) 1282 this.description = new StringType(); 1283 this.description.setValue(value); 1284 } 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #subject} (Identifies the patient or group whose intended care is described by the plan.) 1290 */ 1291 public Reference getSubject() { 1292 if (this.subject == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create CarePlan.subject"); 1295 else if (Configuration.doAutoCreate()) 1296 this.subject = new Reference(); // cc 1297 return this.subject; 1298 } 1299 1300 public boolean hasSubject() { 1301 return this.subject != null && !this.subject.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #subject} (Identifies the patient or group whose intended care is described by the plan.) 1306 */ 1307 public CarePlan setSubject(Reference value) { 1308 this.subject = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return {@link #encounter} (The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.) 1314 */ 1315 public Reference getEncounter() { 1316 if (this.encounter == null) 1317 if (Configuration.errorOnAutoCreate()) 1318 throw new Error("Attempt to auto-create CarePlan.encounter"); 1319 else if (Configuration.doAutoCreate()) 1320 this.encounter = new Reference(); // cc 1321 return this.encounter; 1322 } 1323 1324 public boolean hasEncounter() { 1325 return this.encounter != null && !this.encounter.isEmpty(); 1326 } 1327 1328 /** 1329 * @param value {@link #encounter} (The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.) 1330 */ 1331 public CarePlan setEncounter(Reference value) { 1332 this.encounter = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return {@link #period} (Indicates when the plan did (or is intended to) come into effect and end.) 1338 */ 1339 public Period getPeriod() { 1340 if (this.period == null) 1341 if (Configuration.errorOnAutoCreate()) 1342 throw new Error("Attempt to auto-create CarePlan.period"); 1343 else if (Configuration.doAutoCreate()) 1344 this.period = new Period(); // cc 1345 return this.period; 1346 } 1347 1348 public boolean hasPeriod() { 1349 return this.period != null && !this.period.isEmpty(); 1350 } 1351 1352 /** 1353 * @param value {@link #period} (Indicates when the plan did (or is intended to) come into effect and end.) 1354 */ 1355 public CarePlan setPeriod(Period value) { 1356 this.period = value; 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #created} (Represents when this particular CarePlan record was created in the system, which is often a system-generated date.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1362 */ 1363 public DateTimeType getCreatedElement() { 1364 if (this.created == null) 1365 if (Configuration.errorOnAutoCreate()) 1366 throw new Error("Attempt to auto-create CarePlan.created"); 1367 else if (Configuration.doAutoCreate()) 1368 this.created = new DateTimeType(); // bb 1369 return this.created; 1370 } 1371 1372 public boolean hasCreatedElement() { 1373 return this.created != null && !this.created.isEmpty(); 1374 } 1375 1376 public boolean hasCreated() { 1377 return this.created != null && !this.created.isEmpty(); 1378 } 1379 1380 /** 1381 * @param value {@link #created} (Represents when this particular CarePlan record was created in the system, which is often a system-generated date.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1382 */ 1383 public CarePlan setCreatedElement(DateTimeType value) { 1384 this.created = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return Represents when this particular CarePlan record was created in the system, which is often a system-generated date. 1390 */ 1391 public Date getCreated() { 1392 return this.created == null ? null : this.created.getValue(); 1393 } 1394 1395 /** 1396 * @param value Represents when this particular CarePlan record was created in the system, which is often a system-generated date. 1397 */ 1398 public CarePlan setCreated(Date value) { 1399 if (value == null) 1400 this.created = null; 1401 else { 1402 if (this.created == null) 1403 this.created = new DateTimeType(); 1404 this.created.setValue(value); 1405 } 1406 return this; 1407 } 1408 1409 /** 1410 * @return {@link #custodian} (When populated, the custodian is responsible for the care plan. The care plan is attributed to the custodian.) 1411 */ 1412 public Reference getCustodian() { 1413 if (this.custodian == null) 1414 if (Configuration.errorOnAutoCreate()) 1415 throw new Error("Attempt to auto-create CarePlan.custodian"); 1416 else if (Configuration.doAutoCreate()) 1417 this.custodian = new Reference(); // cc 1418 return this.custodian; 1419 } 1420 1421 public boolean hasCustodian() { 1422 return this.custodian != null && !this.custodian.isEmpty(); 1423 } 1424 1425 /** 1426 * @param value {@link #custodian} (When populated, the custodian is responsible for the care plan. The care plan is attributed to the custodian.) 1427 */ 1428 public CarePlan setCustodian(Reference value) { 1429 this.custodian = value; 1430 return this; 1431 } 1432 1433 /** 1434 * @return {@link #contributor} (Identifies the individual(s), organization or device who provided the contents of the care plan.) 1435 */ 1436 public List<Reference> getContributor() { 1437 if (this.contributor == null) 1438 this.contributor = new ArrayList<Reference>(); 1439 return this.contributor; 1440 } 1441 1442 /** 1443 * @return Returns a reference to <code>this</code> for easy method chaining 1444 */ 1445 public CarePlan setContributor(List<Reference> theContributor) { 1446 this.contributor = theContributor; 1447 return this; 1448 } 1449 1450 public boolean hasContributor() { 1451 if (this.contributor == null) 1452 return false; 1453 for (Reference item : this.contributor) 1454 if (!item.isEmpty()) 1455 return true; 1456 return false; 1457 } 1458 1459 public Reference addContributor() { //3 1460 Reference t = new Reference(); 1461 if (this.contributor == null) 1462 this.contributor = new ArrayList<Reference>(); 1463 this.contributor.add(t); 1464 return t; 1465 } 1466 1467 public CarePlan addContributor(Reference t) { //3 1468 if (t == null) 1469 return this; 1470 if (this.contributor == null) 1471 this.contributor = new ArrayList<Reference>(); 1472 this.contributor.add(t); 1473 return this; 1474 } 1475 1476 /** 1477 * @return The first repetition of repeating field {@link #contributor}, creating it if it does not already exist {3} 1478 */ 1479 public Reference getContributorFirstRep() { 1480 if (getContributor().isEmpty()) { 1481 addContributor(); 1482 } 1483 return getContributor().get(0); 1484 } 1485 1486 /** 1487 * @return {@link #careTeam} (Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.) 1488 */ 1489 public List<Reference> getCareTeam() { 1490 if (this.careTeam == null) 1491 this.careTeam = new ArrayList<Reference>(); 1492 return this.careTeam; 1493 } 1494 1495 /** 1496 * @return Returns a reference to <code>this</code> for easy method chaining 1497 */ 1498 public CarePlan setCareTeam(List<Reference> theCareTeam) { 1499 this.careTeam = theCareTeam; 1500 return this; 1501 } 1502 1503 public boolean hasCareTeam() { 1504 if (this.careTeam == null) 1505 return false; 1506 for (Reference item : this.careTeam) 1507 if (!item.isEmpty()) 1508 return true; 1509 return false; 1510 } 1511 1512 public Reference addCareTeam() { //3 1513 Reference t = new Reference(); 1514 if (this.careTeam == null) 1515 this.careTeam = new ArrayList<Reference>(); 1516 this.careTeam.add(t); 1517 return t; 1518 } 1519 1520 public CarePlan addCareTeam(Reference t) { //3 1521 if (t == null) 1522 return this; 1523 if (this.careTeam == null) 1524 this.careTeam = new ArrayList<Reference>(); 1525 this.careTeam.add(t); 1526 return this; 1527 } 1528 1529 /** 1530 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 1531 */ 1532 public Reference getCareTeamFirstRep() { 1533 if (getCareTeam().isEmpty()) { 1534 addCareTeam(); 1535 } 1536 return getCareTeam().get(0); 1537 } 1538 1539 /** 1540 * @return {@link #addresses} (Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.) 1541 */ 1542 public List<CodeableReference> getAddresses() { 1543 if (this.addresses == null) 1544 this.addresses = new ArrayList<CodeableReference>(); 1545 return this.addresses; 1546 } 1547 1548 /** 1549 * @return Returns a reference to <code>this</code> for easy method chaining 1550 */ 1551 public CarePlan setAddresses(List<CodeableReference> theAddresses) { 1552 this.addresses = theAddresses; 1553 return this; 1554 } 1555 1556 public boolean hasAddresses() { 1557 if (this.addresses == null) 1558 return false; 1559 for (CodeableReference item : this.addresses) 1560 if (!item.isEmpty()) 1561 return true; 1562 return false; 1563 } 1564 1565 public CodeableReference addAddresses() { //3 1566 CodeableReference t = new CodeableReference(); 1567 if (this.addresses == null) 1568 this.addresses = new ArrayList<CodeableReference>(); 1569 this.addresses.add(t); 1570 return t; 1571 } 1572 1573 public CarePlan addAddresses(CodeableReference t) { //3 1574 if (t == null) 1575 return this; 1576 if (this.addresses == null) 1577 this.addresses = new ArrayList<CodeableReference>(); 1578 this.addresses.add(t); 1579 return this; 1580 } 1581 1582 /** 1583 * @return The first repetition of repeating field {@link #addresses}, creating it if it does not already exist {3} 1584 */ 1585 public CodeableReference getAddressesFirstRep() { 1586 if (getAddresses().isEmpty()) { 1587 addAddresses(); 1588 } 1589 return getAddresses().get(0); 1590 } 1591 1592 /** 1593 * @return {@link #supportingInfo} (Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.) 1594 */ 1595 public List<Reference> getSupportingInfo() { 1596 if (this.supportingInfo == null) 1597 this.supportingInfo = new ArrayList<Reference>(); 1598 return this.supportingInfo; 1599 } 1600 1601 /** 1602 * @return Returns a reference to <code>this</code> for easy method chaining 1603 */ 1604 public CarePlan setSupportingInfo(List<Reference> theSupportingInfo) { 1605 this.supportingInfo = theSupportingInfo; 1606 return this; 1607 } 1608 1609 public boolean hasSupportingInfo() { 1610 if (this.supportingInfo == null) 1611 return false; 1612 for (Reference item : this.supportingInfo) 1613 if (!item.isEmpty()) 1614 return true; 1615 return false; 1616 } 1617 1618 public Reference addSupportingInfo() { //3 1619 Reference t = new Reference(); 1620 if (this.supportingInfo == null) 1621 this.supportingInfo = new ArrayList<Reference>(); 1622 this.supportingInfo.add(t); 1623 return t; 1624 } 1625 1626 public CarePlan addSupportingInfo(Reference t) { //3 1627 if (t == null) 1628 return this; 1629 if (this.supportingInfo == null) 1630 this.supportingInfo = new ArrayList<Reference>(); 1631 this.supportingInfo.add(t); 1632 return this; 1633 } 1634 1635 /** 1636 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 1637 */ 1638 public Reference getSupportingInfoFirstRep() { 1639 if (getSupportingInfo().isEmpty()) { 1640 addSupportingInfo(); 1641 } 1642 return getSupportingInfo().get(0); 1643 } 1644 1645 /** 1646 * @return {@link #goal} (Describes the intended objective(s) of carrying out the care plan.) 1647 */ 1648 public List<Reference> getGoal() { 1649 if (this.goal == null) 1650 this.goal = new ArrayList<Reference>(); 1651 return this.goal; 1652 } 1653 1654 /** 1655 * @return Returns a reference to <code>this</code> for easy method chaining 1656 */ 1657 public CarePlan setGoal(List<Reference> theGoal) { 1658 this.goal = theGoal; 1659 return this; 1660 } 1661 1662 public boolean hasGoal() { 1663 if (this.goal == null) 1664 return false; 1665 for (Reference item : this.goal) 1666 if (!item.isEmpty()) 1667 return true; 1668 return false; 1669 } 1670 1671 public Reference addGoal() { //3 1672 Reference t = new Reference(); 1673 if (this.goal == null) 1674 this.goal = new ArrayList<Reference>(); 1675 this.goal.add(t); 1676 return t; 1677 } 1678 1679 public CarePlan addGoal(Reference t) { //3 1680 if (t == null) 1681 return this; 1682 if (this.goal == null) 1683 this.goal = new ArrayList<Reference>(); 1684 this.goal.add(t); 1685 return this; 1686 } 1687 1688 /** 1689 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist {3} 1690 */ 1691 public Reference getGoalFirstRep() { 1692 if (getGoal().isEmpty()) { 1693 addGoal(); 1694 } 1695 return getGoal().get(0); 1696 } 1697 1698 /** 1699 * @return {@link #activity} (Identifies an action that has occurred or is a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring that has occurred, education etc.) 1700 */ 1701 public List<CarePlanActivityComponent> getActivity() { 1702 if (this.activity == null) 1703 this.activity = new ArrayList<CarePlanActivityComponent>(); 1704 return this.activity; 1705 } 1706 1707 /** 1708 * @return Returns a reference to <code>this</code> for easy method chaining 1709 */ 1710 public CarePlan setActivity(List<CarePlanActivityComponent> theActivity) { 1711 this.activity = theActivity; 1712 return this; 1713 } 1714 1715 public boolean hasActivity() { 1716 if (this.activity == null) 1717 return false; 1718 for (CarePlanActivityComponent item : this.activity) 1719 if (!item.isEmpty()) 1720 return true; 1721 return false; 1722 } 1723 1724 public CarePlanActivityComponent addActivity() { //3 1725 CarePlanActivityComponent t = new CarePlanActivityComponent(); 1726 if (this.activity == null) 1727 this.activity = new ArrayList<CarePlanActivityComponent>(); 1728 this.activity.add(t); 1729 return t; 1730 } 1731 1732 public CarePlan addActivity(CarePlanActivityComponent t) { //3 1733 if (t == null) 1734 return this; 1735 if (this.activity == null) 1736 this.activity = new ArrayList<CarePlanActivityComponent>(); 1737 this.activity.add(t); 1738 return this; 1739 } 1740 1741 /** 1742 * @return The first repetition of repeating field {@link #activity}, creating it if it does not already exist {3} 1743 */ 1744 public CarePlanActivityComponent getActivityFirstRep() { 1745 if (getActivity().isEmpty()) { 1746 addActivity(); 1747 } 1748 return getActivity().get(0); 1749 } 1750 1751 /** 1752 * @return {@link #note} (General notes about the care plan not covered elsewhere.) 1753 */ 1754 public List<Annotation> getNote() { 1755 if (this.note == null) 1756 this.note = new ArrayList<Annotation>(); 1757 return this.note; 1758 } 1759 1760 /** 1761 * @return Returns a reference to <code>this</code> for easy method chaining 1762 */ 1763 public CarePlan setNote(List<Annotation> theNote) { 1764 this.note = theNote; 1765 return this; 1766 } 1767 1768 public boolean hasNote() { 1769 if (this.note == null) 1770 return false; 1771 for (Annotation item : this.note) 1772 if (!item.isEmpty()) 1773 return true; 1774 return false; 1775 } 1776 1777 public Annotation addNote() { //3 1778 Annotation t = new Annotation(); 1779 if (this.note == null) 1780 this.note = new ArrayList<Annotation>(); 1781 this.note.add(t); 1782 return t; 1783 } 1784 1785 public CarePlan addNote(Annotation t) { //3 1786 if (t == null) 1787 return this; 1788 if (this.note == null) 1789 this.note = new ArrayList<Annotation>(); 1790 this.note.add(t); 1791 return this; 1792 } 1793 1794 /** 1795 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1796 */ 1797 public Annotation getNoteFirstRep() { 1798 if (getNote().isEmpty()) { 1799 addNote(); 1800 } 1801 return getNote().get(0); 1802 } 1803 1804 protected void listChildren(List<Property> children) { 1805 super.listChildren(children); 1806 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1807 children.add(new Property("instantiatesCanonical", "canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 1808 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 1809 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|RequestOrchestration|NutritionOrder)", "A higher-level request resource (i.e. a plan, proposal or order) that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1810 children.add(new Property("replaces", "Reference(CarePlan)", "Completed or terminated care plan whose function is taken by this new care plan.", 0, java.lang.Integer.MAX_VALUE, replaces)); 1811 children.add(new Property("partOf", "Reference(CarePlan)", "A larger care plan of which this particular care plan is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1812 children.add(new Property("status", "code", "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 0, 1, status)); 1813 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 0, 1, intent)); 1814 children.add(new Property("category", "CodeableConcept", "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 0, java.lang.Integer.MAX_VALUE, category)); 1815 children.add(new Property("title", "string", "Human-friendly name for the care plan.", 0, 1, title)); 1816 children.add(new Property("description", "string", "A description of the scope and nature of the plan.", 0, 1, description)); 1817 children.add(new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject)); 1818 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1819 children.add(new Property("period", "Period", "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period)); 1820 children.add(new Property("created", "dateTime", "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.", 0, 1, created)); 1821 children.add(new Property("custodian", "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", "When populated, the custodian is responsible for the care plan. The care plan is attributed to the custodian.", 0, 1, custodian)); 1822 children.add(new Property("contributor", "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", "Identifies the individual(s), organization or device who provided the contents of the care plan.", 0, java.lang.Integer.MAX_VALUE, contributor)); 1823 children.add(new Property("careTeam", "Reference(CareTeam)", "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 1824 children.add(new Property("addresses", "CodeableReference(Condition)", "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 0, java.lang.Integer.MAX_VALUE, addresses)); 1825 children.add(new Property("supportingInfo", "Reference(Any)", "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 1826 children.add(new Property("goal", "Reference(Goal)", "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal)); 1827 children.add(new Property("activity", "", "Identifies an action that has occurred or is a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring that has occurred, education etc.", 0, java.lang.Integer.MAX_VALUE, activity)); 1828 children.add(new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, java.lang.Integer.MAX_VALUE, note)); 1829 } 1830 1831 @Override 1832 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1833 switch (_hash) { 1834 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this care plan by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1835 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(PlanDefinition|Questionnaire|Measure|ActivityDefinition|OperationDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 1836 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, questionnaire or other definition that is adhered to in whole or in part by this CarePlan.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 1837 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest|RequestOrchestration|NutritionOrder)", "A higher-level request resource (i.e. a plan, proposal or order) that is fulfilled in whole or in part by this care plan.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1838 case -430332865: /*replaces*/ return new Property("replaces", "Reference(CarePlan)", "Completed or terminated care plan whose function is taken by this new care plan.", 0, java.lang.Integer.MAX_VALUE, replaces); 1839 case -995410646: /*partOf*/ return new Property("partOf", "Reference(CarePlan)", "A larger care plan of which this particular care plan is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1840 case -892481550: /*status*/ return new Property("status", "code", "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 0, 1, status); 1841 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the care plan and where the care plan fits into the workflow chain.", 0, 1, intent); 1842 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 0, java.lang.Integer.MAX_VALUE, category); 1843 case 110371416: /*title*/ return new Property("title", "string", "Human-friendly name for the care plan.", 0, 1, title); 1844 case -1724546052: /*description*/ return new Property("description", "string", "A description of the scope and nature of the plan.", 0, 1, description); 1845 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is described by the plan.", 0, 1, subject); 1846 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this CarePlan was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1847 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the plan did (or is intended to) come into effect and end.", 0, 1, period); 1848 case 1028554472: /*created*/ return new Property("created", "dateTime", "Represents when this particular CarePlan record was created in the system, which is often a system-generated date.", 0, 1, created); 1849 case 1611297262: /*custodian*/ return new Property("custodian", "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", "When populated, the custodian is responsible for the care plan. The care plan is attributed to the custodian.", 0, 1, custodian); 1850 case -1895276325: /*contributor*/ return new Property("contributor", "Reference(Patient|Practitioner|PractitionerRole|Device|RelatedPerson|Organization|CareTeam)", "Identifies the individual(s), organization or device who provided the contents of the care plan.", 0, java.lang.Integer.MAX_VALUE, contributor); 1851 case -7323378: /*careTeam*/ return new Property("careTeam", "Reference(CareTeam)", "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 0, java.lang.Integer.MAX_VALUE, careTeam); 1852 case 874544034: /*addresses*/ return new Property("addresses", "CodeableReference(Condition)", "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 0, java.lang.Integer.MAX_VALUE, addresses); 1853 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include comorbidities, recent procedures, limitations, recent assessments, etc.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 1854 case 3178259: /*goal*/ return new Property("goal", "Reference(Goal)", "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal); 1855 case -1655966961: /*activity*/ return new Property("activity", "", "Identifies an action that has occurred or is a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring that has occurred, education etc.", 0, java.lang.Integer.MAX_VALUE, activity); 1856 case 3387378: /*note*/ return new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, java.lang.Integer.MAX_VALUE, note); 1857 default: return super.getNamedProperty(_hash, _name, _checkValid); 1858 } 1859 1860 } 1861 1862 @Override 1863 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1864 switch (hash) { 1865 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1866 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 1867 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 1868 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1869 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 1870 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1871 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 1872 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<CarePlanIntent> 1873 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1874 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1875 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1876 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1877 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1878 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1879 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 1880 case 1611297262: /*custodian*/ return this.custodian == null ? new Base[0] : new Base[] {this.custodian}; // Reference 1881 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : this.contributor.toArray(new Base[this.contributor.size()]); // Reference 1882 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // Reference 1883 case 874544034: /*addresses*/ return this.addresses == null ? new Base[0] : this.addresses.toArray(new Base[this.addresses.size()]); // CodeableReference 1884 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 1885 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 1886 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : this.activity.toArray(new Base[this.activity.size()]); // CarePlanActivityComponent 1887 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1888 default: return super.getProperty(hash, name, checkValid); 1889 } 1890 1891 } 1892 1893 @Override 1894 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1895 switch (hash) { 1896 case -1618432855: // identifier 1897 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1898 return value; 1899 case 8911915: // instantiatesCanonical 1900 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1901 return value; 1902 case -1926393373: // instantiatesUri 1903 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 1904 return value; 1905 case -332612366: // basedOn 1906 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1907 return value; 1908 case -430332865: // replaces 1909 this.getReplaces().add(TypeConvertor.castToReference(value)); // Reference 1910 return value; 1911 case -995410646: // partOf 1912 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1913 return value; 1914 case -892481550: // status 1915 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1916 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1917 return value; 1918 case -1183762788: // intent 1919 value = new CarePlanIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1920 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 1921 return value; 1922 case 50511102: // category 1923 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1924 return value; 1925 case 110371416: // title 1926 this.title = TypeConvertor.castToString(value); // StringType 1927 return value; 1928 case -1724546052: // description 1929 this.description = TypeConvertor.castToString(value); // StringType 1930 return value; 1931 case -1867885268: // subject 1932 this.subject = TypeConvertor.castToReference(value); // Reference 1933 return value; 1934 case 1524132147: // encounter 1935 this.encounter = TypeConvertor.castToReference(value); // Reference 1936 return value; 1937 case -991726143: // period 1938 this.period = TypeConvertor.castToPeriod(value); // Period 1939 return value; 1940 case 1028554472: // created 1941 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 1942 return value; 1943 case 1611297262: // custodian 1944 this.custodian = TypeConvertor.castToReference(value); // Reference 1945 return value; 1946 case -1895276325: // contributor 1947 this.getContributor().add(TypeConvertor.castToReference(value)); // Reference 1948 return value; 1949 case -7323378: // careTeam 1950 this.getCareTeam().add(TypeConvertor.castToReference(value)); // Reference 1951 return value; 1952 case 874544034: // addresses 1953 this.getAddresses().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1954 return value; 1955 case 1922406657: // supportingInfo 1956 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); // Reference 1957 return value; 1958 case 3178259: // goal 1959 this.getGoal().add(TypeConvertor.castToReference(value)); // Reference 1960 return value; 1961 case -1655966961: // activity 1962 this.getActivity().add((CarePlanActivityComponent) value); // CarePlanActivityComponent 1963 return value; 1964 case 3387378: // note 1965 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1966 return value; 1967 default: return super.setProperty(hash, name, value); 1968 } 1969 1970 } 1971 1972 @Override 1973 public Base setProperty(String name, Base value) throws FHIRException { 1974 if (name.equals("identifier")) { 1975 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1976 } else if (name.equals("instantiatesCanonical")) { 1977 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 1978 } else if (name.equals("instantiatesUri")) { 1979 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 1980 } else if (name.equals("basedOn")) { 1981 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1982 } else if (name.equals("replaces")) { 1983 this.getReplaces().add(TypeConvertor.castToReference(value)); 1984 } else if (name.equals("partOf")) { 1985 this.getPartOf().add(TypeConvertor.castToReference(value)); 1986 } else if (name.equals("status")) { 1987 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1988 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1989 } else if (name.equals("intent")) { 1990 value = new CarePlanIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1991 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 1992 } else if (name.equals("category")) { 1993 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1994 } else if (name.equals("title")) { 1995 this.title = TypeConvertor.castToString(value); // StringType 1996 } else if (name.equals("description")) { 1997 this.description = TypeConvertor.castToString(value); // StringType 1998 } else if (name.equals("subject")) { 1999 this.subject = TypeConvertor.castToReference(value); // Reference 2000 } else if (name.equals("encounter")) { 2001 this.encounter = TypeConvertor.castToReference(value); // Reference 2002 } else if (name.equals("period")) { 2003 this.period = TypeConvertor.castToPeriod(value); // Period 2004 } else if (name.equals("created")) { 2005 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2006 } else if (name.equals("custodian")) { 2007 this.custodian = TypeConvertor.castToReference(value); // Reference 2008 } else if (name.equals("contributor")) { 2009 this.getContributor().add(TypeConvertor.castToReference(value)); 2010 } else if (name.equals("careTeam")) { 2011 this.getCareTeam().add(TypeConvertor.castToReference(value)); 2012 } else if (name.equals("addresses")) { 2013 this.getAddresses().add(TypeConvertor.castToCodeableReference(value)); 2014 } else if (name.equals("supportingInfo")) { 2015 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); 2016 } else if (name.equals("goal")) { 2017 this.getGoal().add(TypeConvertor.castToReference(value)); 2018 } else if (name.equals("activity")) { 2019 this.getActivity().add((CarePlanActivityComponent) value); 2020 } else if (name.equals("note")) { 2021 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2022 } else 2023 return super.setProperty(name, value); 2024 return value; 2025 } 2026 2027 @Override 2028 public void removeChild(String name, Base value) throws FHIRException { 2029 if (name.equals("identifier")) { 2030 this.getIdentifier().remove(value); 2031 } else if (name.equals("instantiatesCanonical")) { 2032 this.getInstantiatesCanonical().remove(value); 2033 } else if (name.equals("instantiatesUri")) { 2034 this.getInstantiatesUri().remove(value); 2035 } else if (name.equals("basedOn")) { 2036 this.getBasedOn().remove(value); 2037 } else if (name.equals("replaces")) { 2038 this.getReplaces().remove(value); 2039 } else if (name.equals("partOf")) { 2040 this.getPartOf().remove(value); 2041 } else if (name.equals("status")) { 2042 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2043 this.status = (Enumeration) value; // Enumeration<RequestStatus> 2044 } else if (name.equals("intent")) { 2045 value = new CarePlanIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 2046 this.intent = (Enumeration) value; // Enumeration<CarePlanIntent> 2047 } else if (name.equals("category")) { 2048 this.getCategory().remove(value); 2049 } else if (name.equals("title")) { 2050 this.title = null; 2051 } else if (name.equals("description")) { 2052 this.description = null; 2053 } else if (name.equals("subject")) { 2054 this.subject = null; 2055 } else if (name.equals("encounter")) { 2056 this.encounter = null; 2057 } else if (name.equals("period")) { 2058 this.period = null; 2059 } else if (name.equals("created")) { 2060 this.created = null; 2061 } else if (name.equals("custodian")) { 2062 this.custodian = null; 2063 } else if (name.equals("contributor")) { 2064 this.getContributor().remove(value); 2065 } else if (name.equals("careTeam")) { 2066 this.getCareTeam().remove(value); 2067 } else if (name.equals("addresses")) { 2068 this.getAddresses().remove(value); 2069 } else if (name.equals("supportingInfo")) { 2070 this.getSupportingInfo().remove(value); 2071 } else if (name.equals("goal")) { 2072 this.getGoal().remove(value); 2073 } else if (name.equals("activity")) { 2074 this.getActivity().remove((CarePlanActivityComponent) value); 2075 } else if (name.equals("note")) { 2076 this.getNote().remove(value); 2077 } else 2078 super.removeChild(name, value); 2079 2080 } 2081 2082 @Override 2083 public Base makeProperty(int hash, String name) throws FHIRException { 2084 switch (hash) { 2085 case -1618432855: return addIdentifier(); 2086 case 8911915: return addInstantiatesCanonicalElement(); 2087 case -1926393373: return addInstantiatesUriElement(); 2088 case -332612366: return addBasedOn(); 2089 case -430332865: return addReplaces(); 2090 case -995410646: return addPartOf(); 2091 case -892481550: return getStatusElement(); 2092 case -1183762788: return getIntentElement(); 2093 case 50511102: return addCategory(); 2094 case 110371416: return getTitleElement(); 2095 case -1724546052: return getDescriptionElement(); 2096 case -1867885268: return getSubject(); 2097 case 1524132147: return getEncounter(); 2098 case -991726143: return getPeriod(); 2099 case 1028554472: return getCreatedElement(); 2100 case 1611297262: return getCustodian(); 2101 case -1895276325: return addContributor(); 2102 case -7323378: return addCareTeam(); 2103 case 874544034: return addAddresses(); 2104 case 1922406657: return addSupportingInfo(); 2105 case 3178259: return addGoal(); 2106 case -1655966961: return addActivity(); 2107 case 3387378: return addNote(); 2108 default: return super.makeProperty(hash, name); 2109 } 2110 2111 } 2112 2113 @Override 2114 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2115 switch (hash) { 2116 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2117 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 2118 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 2119 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2120 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2121 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2122 case -892481550: /*status*/ return new String[] {"code"}; 2123 case -1183762788: /*intent*/ return new String[] {"code"}; 2124 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2125 case 110371416: /*title*/ return new String[] {"string"}; 2126 case -1724546052: /*description*/ return new String[] {"string"}; 2127 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2128 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2129 case -991726143: /*period*/ return new String[] {"Period"}; 2130 case 1028554472: /*created*/ return new String[] {"dateTime"}; 2131 case 1611297262: /*custodian*/ return new String[] {"Reference"}; 2132 case -1895276325: /*contributor*/ return new String[] {"Reference"}; 2133 case -7323378: /*careTeam*/ return new String[] {"Reference"}; 2134 case 874544034: /*addresses*/ return new String[] {"CodeableReference"}; 2135 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2136 case 3178259: /*goal*/ return new String[] {"Reference"}; 2137 case -1655966961: /*activity*/ return new String[] {}; 2138 case 3387378: /*note*/ return new String[] {"Annotation"}; 2139 default: return super.getTypesForProperty(hash, name); 2140 } 2141 2142 } 2143 2144 @Override 2145 public Base addChild(String name) throws FHIRException { 2146 if (name.equals("identifier")) { 2147 return addIdentifier(); 2148 } 2149 else if (name.equals("instantiatesCanonical")) { 2150 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesCanonical"); 2151 } 2152 else if (name.equals("instantiatesUri")) { 2153 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.instantiatesUri"); 2154 } 2155 else if (name.equals("basedOn")) { 2156 return addBasedOn(); 2157 } 2158 else if (name.equals("replaces")) { 2159 return addReplaces(); 2160 } 2161 else if (name.equals("partOf")) { 2162 return addPartOf(); 2163 } 2164 else if (name.equals("status")) { 2165 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 2166 } 2167 else if (name.equals("intent")) { 2168 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.intent"); 2169 } 2170 else if (name.equals("category")) { 2171 return addCategory(); 2172 } 2173 else if (name.equals("title")) { 2174 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.title"); 2175 } 2176 else if (name.equals("description")) { 2177 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 2178 } 2179 else if (name.equals("subject")) { 2180 this.subject = new Reference(); 2181 return this.subject; 2182 } 2183 else if (name.equals("encounter")) { 2184 this.encounter = new Reference(); 2185 return this.encounter; 2186 } 2187 else if (name.equals("period")) { 2188 this.period = new Period(); 2189 return this.period; 2190 } 2191 else if (name.equals("created")) { 2192 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.created"); 2193 } 2194 else if (name.equals("custodian")) { 2195 this.custodian = new Reference(); 2196 return this.custodian; 2197 } 2198 else if (name.equals("contributor")) { 2199 return addContributor(); 2200 } 2201 else if (name.equals("careTeam")) { 2202 return addCareTeam(); 2203 } 2204 else if (name.equals("addresses")) { 2205 return addAddresses(); 2206 } 2207 else if (name.equals("supportingInfo")) { 2208 return addSupportingInfo(); 2209 } 2210 else if (name.equals("goal")) { 2211 return addGoal(); 2212 } 2213 else if (name.equals("activity")) { 2214 return addActivity(); 2215 } 2216 else if (name.equals("note")) { 2217 return addNote(); 2218 } 2219 else 2220 return super.addChild(name); 2221 } 2222 2223 public String fhirType() { 2224 return "CarePlan"; 2225 2226 } 2227 2228 public CarePlan copy() { 2229 CarePlan dst = new CarePlan(); 2230 copyValues(dst); 2231 return dst; 2232 } 2233 2234 public void copyValues(CarePlan dst) { 2235 super.copyValues(dst); 2236 if (identifier != null) { 2237 dst.identifier = new ArrayList<Identifier>(); 2238 for (Identifier i : identifier) 2239 dst.identifier.add(i.copy()); 2240 }; 2241 if (instantiatesCanonical != null) { 2242 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2243 for (CanonicalType i : instantiatesCanonical) 2244 dst.instantiatesCanonical.add(i.copy()); 2245 }; 2246 if (instantiatesUri != null) { 2247 dst.instantiatesUri = new ArrayList<UriType>(); 2248 for (UriType i : instantiatesUri) 2249 dst.instantiatesUri.add(i.copy()); 2250 }; 2251 if (basedOn != null) { 2252 dst.basedOn = new ArrayList<Reference>(); 2253 for (Reference i : basedOn) 2254 dst.basedOn.add(i.copy()); 2255 }; 2256 if (replaces != null) { 2257 dst.replaces = new ArrayList<Reference>(); 2258 for (Reference i : replaces) 2259 dst.replaces.add(i.copy()); 2260 }; 2261 if (partOf != null) { 2262 dst.partOf = new ArrayList<Reference>(); 2263 for (Reference i : partOf) 2264 dst.partOf.add(i.copy()); 2265 }; 2266 dst.status = status == null ? null : status.copy(); 2267 dst.intent = intent == null ? null : intent.copy(); 2268 if (category != null) { 2269 dst.category = new ArrayList<CodeableConcept>(); 2270 for (CodeableConcept i : category) 2271 dst.category.add(i.copy()); 2272 }; 2273 dst.title = title == null ? null : title.copy(); 2274 dst.description = description == null ? null : description.copy(); 2275 dst.subject = subject == null ? null : subject.copy(); 2276 dst.encounter = encounter == null ? null : encounter.copy(); 2277 dst.period = period == null ? null : period.copy(); 2278 dst.created = created == null ? null : created.copy(); 2279 dst.custodian = custodian == null ? null : custodian.copy(); 2280 if (contributor != null) { 2281 dst.contributor = new ArrayList<Reference>(); 2282 for (Reference i : contributor) 2283 dst.contributor.add(i.copy()); 2284 }; 2285 if (careTeam != null) { 2286 dst.careTeam = new ArrayList<Reference>(); 2287 for (Reference i : careTeam) 2288 dst.careTeam.add(i.copy()); 2289 }; 2290 if (addresses != null) { 2291 dst.addresses = new ArrayList<CodeableReference>(); 2292 for (CodeableReference i : addresses) 2293 dst.addresses.add(i.copy()); 2294 }; 2295 if (supportingInfo != null) { 2296 dst.supportingInfo = new ArrayList<Reference>(); 2297 for (Reference i : supportingInfo) 2298 dst.supportingInfo.add(i.copy()); 2299 }; 2300 if (goal != null) { 2301 dst.goal = new ArrayList<Reference>(); 2302 for (Reference i : goal) 2303 dst.goal.add(i.copy()); 2304 }; 2305 if (activity != null) { 2306 dst.activity = new ArrayList<CarePlanActivityComponent>(); 2307 for (CarePlanActivityComponent i : activity) 2308 dst.activity.add(i.copy()); 2309 }; 2310 if (note != null) { 2311 dst.note = new ArrayList<Annotation>(); 2312 for (Annotation i : note) 2313 dst.note.add(i.copy()); 2314 }; 2315 } 2316 2317 protected CarePlan typedCopy() { 2318 return copy(); 2319 } 2320 2321 @Override 2322 public boolean equalsDeep(Base other_) { 2323 if (!super.equalsDeep(other_)) 2324 return false; 2325 if (!(other_ instanceof CarePlan)) 2326 return false; 2327 CarePlan o = (CarePlan) other_; 2328 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 2329 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 2330 && compareDeep(replaces, o.replaces, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 2331 && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) && compareDeep(title, o.title, true) 2332 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2333 && compareDeep(period, o.period, true) && compareDeep(created, o.created, true) && compareDeep(custodian, o.custodian, true) 2334 && compareDeep(contributor, o.contributor, true) && compareDeep(careTeam, o.careTeam, true) && compareDeep(addresses, o.addresses, true) 2335 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(goal, o.goal, true) && compareDeep(activity, o.activity, true) 2336 && compareDeep(note, o.note, true); 2337 } 2338 2339 @Override 2340 public boolean equalsShallow(Base other_) { 2341 if (!super.equalsShallow(other_)) 2342 return false; 2343 if (!(other_ instanceof CarePlan)) 2344 return false; 2345 CarePlan o = (CarePlan) other_; 2346 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 2347 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(title, o.title, true) 2348 && compareValues(description, o.description, true) && compareValues(created, o.created, true); 2349 } 2350 2351 public boolean isEmpty() { 2352 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 2353 , instantiatesUri, basedOn, replaces, partOf, status, intent, category, title 2354 , description, subject, encounter, period, created, custodian, contributor, careTeam 2355 , addresses, supportingInfo, goal, activity, note); 2356 } 2357 2358 @Override 2359 public ResourceType getResourceType() { 2360 return ResourceType.CarePlan; 2361 } 2362 2363 /** 2364 * Search parameter: <b>activity-reference</b> 2365 * <p> 2366 * Description: <b>Activity that is intended to be part of the care plan</b><br> 2367 * Type: <b>reference</b><br> 2368 * Path: <b>CarePlan.activity.plannedActivityReference</b><br> 2369 * </p> 2370 */ 2371 @SearchParamDefinition(name="activity-reference", path="CarePlan.activity.plannedActivityReference", description="Activity that is intended to be part of the care plan", type="reference", target={Appointment.class, CommunicationRequest.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, RequestOrchestration.class, ServiceRequest.class, SupplyRequest.class, Task.class, VisionPrescription.class } ) 2372 public static final String SP_ACTIVITY_REFERENCE = "activity-reference"; 2373 /** 2374 * <b>Fluent Client</b> search parameter constant for <b>activity-reference</b> 2375 * <p> 2376 * Description: <b>Activity that is intended to be part of the care plan</b><br> 2377 * Type: <b>reference</b><br> 2378 * Path: <b>CarePlan.activity.plannedActivityReference</b><br> 2379 * </p> 2380 */ 2381 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTIVITY_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTIVITY_REFERENCE); 2382 2383/** 2384 * Constant for fluent queries to be used to add include statements. Specifies 2385 * the path value of "<b>CarePlan:activity-reference</b>". 2386 */ 2387 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTIVITY_REFERENCE = new ca.uhn.fhir.model.api.Include("CarePlan:activity-reference").toLocked(); 2388 2389 /** 2390 * Search parameter: <b>based-on</b> 2391 * <p> 2392 * Description: <b>Fulfills CarePlan</b><br> 2393 * Type: <b>reference</b><br> 2394 * Path: <b>CarePlan.basedOn</b><br> 2395 * </p> 2396 */ 2397 @SearchParamDefinition(name="based-on", path="CarePlan.basedOn", description="Fulfills CarePlan", type="reference", target={CarePlan.class, NutritionOrder.class, RequestOrchestration.class, ServiceRequest.class } ) 2398 public static final String SP_BASED_ON = "based-on"; 2399 /** 2400 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2401 * <p> 2402 * Description: <b>Fulfills CarePlan</b><br> 2403 * Type: <b>reference</b><br> 2404 * Path: <b>CarePlan.basedOn</b><br> 2405 * </p> 2406 */ 2407 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2408 2409/** 2410 * Constant for fluent queries to be used to add include statements. Specifies 2411 * the path value of "<b>CarePlan:based-on</b>". 2412 */ 2413 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("CarePlan:based-on").toLocked(); 2414 2415 /** 2416 * Search parameter: <b>care-team</b> 2417 * <p> 2418 * Description: <b>Who's involved in plan?</b><br> 2419 * Type: <b>reference</b><br> 2420 * Path: <b>CarePlan.careTeam</b><br> 2421 * </p> 2422 */ 2423 @SearchParamDefinition(name="care-team", path="CarePlan.careTeam", description="Who's involved in plan?", type="reference", target={CareTeam.class } ) 2424 public static final String SP_CARE_TEAM = "care-team"; 2425 /** 2426 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 2427 * <p> 2428 * Description: <b>Who's involved in plan?</b><br> 2429 * Type: <b>reference</b><br> 2430 * Path: <b>CarePlan.careTeam</b><br> 2431 * </p> 2432 */ 2433 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 2434 2435/** 2436 * Constant for fluent queries to be used to add include statements. Specifies 2437 * the path value of "<b>CarePlan:care-team</b>". 2438 */ 2439 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("CarePlan:care-team").toLocked(); 2440 2441 /** 2442 * Search parameter: <b>category</b> 2443 * <p> 2444 * Description: <b>Type of plan</b><br> 2445 * Type: <b>token</b><br> 2446 * Path: <b>CarePlan.category</b><br> 2447 * </p> 2448 */ 2449 @SearchParamDefinition(name="category", path="CarePlan.category", description="Type of plan", type="token" ) 2450 public static final String SP_CATEGORY = "category"; 2451 /** 2452 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2453 * <p> 2454 * Description: <b>Type of plan</b><br> 2455 * Type: <b>token</b><br> 2456 * Path: <b>CarePlan.category</b><br> 2457 * </p> 2458 */ 2459 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2460 2461 /** 2462 * Search parameter: <b>condition</b> 2463 * <p> 2464 * Description: <b>Reference to a resource (by instance)</b><br> 2465 * Type: <b>reference</b><br> 2466 * Path: <b>CarePlan.addresses.reference</b><br> 2467 * </p> 2468 */ 2469 @SearchParamDefinition(name="condition", path="CarePlan.addresses.reference", description="Reference to a resource (by instance)", type="reference", target={Condition.class } ) 2470 public static final String SP_CONDITION = "condition"; 2471 /** 2472 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 2473 * <p> 2474 * Description: <b>Reference to a resource (by instance)</b><br> 2475 * Type: <b>reference</b><br> 2476 * Path: <b>CarePlan.addresses.reference</b><br> 2477 * </p> 2478 */ 2479 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONDITION); 2480 2481/** 2482 * Constant for fluent queries to be used to add include statements. Specifies 2483 * the path value of "<b>CarePlan:condition</b>". 2484 */ 2485 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include("CarePlan:condition").toLocked(); 2486 2487 /** 2488 * Search parameter: <b>custodian</b> 2489 * <p> 2490 * Description: <b>Who is the designated responsible party</b><br> 2491 * Type: <b>reference</b><br> 2492 * Path: <b>CarePlan.custodian</b><br> 2493 * </p> 2494 */ 2495 @SearchParamDefinition(name="custodian", path="CarePlan.custodian", description="Who is the designated responsible party", type="reference", target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2496 public static final String SP_CUSTODIAN = "custodian"; 2497 /** 2498 * <b>Fluent Client</b> search parameter constant for <b>custodian</b> 2499 * <p> 2500 * Description: <b>Who is the designated responsible party</b><br> 2501 * Type: <b>reference</b><br> 2502 * Path: <b>CarePlan.custodian</b><br> 2503 * </p> 2504 */ 2505 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CUSTODIAN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CUSTODIAN); 2506 2507/** 2508 * Constant for fluent queries to be used to add include statements. Specifies 2509 * the path value of "<b>CarePlan:custodian</b>". 2510 */ 2511 public static final ca.uhn.fhir.model.api.Include INCLUDE_CUSTODIAN = new ca.uhn.fhir.model.api.Include("CarePlan:custodian").toLocked(); 2512 2513 /** 2514 * Search parameter: <b>goal</b> 2515 * <p> 2516 * Description: <b>Desired outcome of plan</b><br> 2517 * Type: <b>reference</b><br> 2518 * Path: <b>CarePlan.goal</b><br> 2519 * </p> 2520 */ 2521 @SearchParamDefinition(name="goal", path="CarePlan.goal", description="Desired outcome of plan", type="reference", target={Goal.class } ) 2522 public static final String SP_GOAL = "goal"; 2523 /** 2524 * <b>Fluent Client</b> search parameter constant for <b>goal</b> 2525 * <p> 2526 * Description: <b>Desired outcome of plan</b><br> 2527 * Type: <b>reference</b><br> 2528 * Path: <b>CarePlan.goal</b><br> 2529 * </p> 2530 */ 2531 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GOAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GOAL); 2532 2533/** 2534 * Constant for fluent queries to be used to add include statements. Specifies 2535 * the path value of "<b>CarePlan:goal</b>". 2536 */ 2537 public static final ca.uhn.fhir.model.api.Include INCLUDE_GOAL = new ca.uhn.fhir.model.api.Include("CarePlan:goal").toLocked(); 2538 2539 /** 2540 * Search parameter: <b>instantiates-canonical</b> 2541 * <p> 2542 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2543 * Type: <b>reference</b><br> 2544 * Path: <b>CarePlan.instantiatesCanonical</b><br> 2545 * </p> 2546 */ 2547 @SearchParamDefinition(name="instantiates-canonical", path="CarePlan.instantiatesCanonical", description="Instantiates FHIR protocol or definition", type="reference", target={ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class } ) 2548 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 2549 /** 2550 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 2551 * <p> 2552 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2553 * Type: <b>reference</b><br> 2554 * Path: <b>CarePlan.instantiatesCanonical</b><br> 2555 * </p> 2556 */ 2557 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 2558 2559/** 2560 * Constant for fluent queries to be used to add include statements. Specifies 2561 * the path value of "<b>CarePlan:instantiates-canonical</b>". 2562 */ 2563 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("CarePlan:instantiates-canonical").toLocked(); 2564 2565 /** 2566 * Search parameter: <b>instantiates-uri</b> 2567 * <p> 2568 * Description: <b>Instantiates external protocol or definition</b><br> 2569 * Type: <b>uri</b><br> 2570 * Path: <b>CarePlan.instantiatesUri</b><br> 2571 * </p> 2572 */ 2573 @SearchParamDefinition(name="instantiates-uri", path="CarePlan.instantiatesUri", description="Instantiates external protocol or definition", type="uri" ) 2574 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 2575 /** 2576 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 2577 * <p> 2578 * Description: <b>Instantiates external protocol or definition</b><br> 2579 * Type: <b>uri</b><br> 2580 * Path: <b>CarePlan.instantiatesUri</b><br> 2581 * </p> 2582 */ 2583 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 2584 2585 /** 2586 * Search parameter: <b>intent</b> 2587 * <p> 2588 * Description: <b>proposal | plan | order | option | directive</b><br> 2589 * Type: <b>token</b><br> 2590 * Path: <b>CarePlan.intent</b><br> 2591 * </p> 2592 */ 2593 @SearchParamDefinition(name="intent", path="CarePlan.intent", description="proposal | plan | order | option | directive", type="token" ) 2594 public static final String SP_INTENT = "intent"; 2595 /** 2596 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 2597 * <p> 2598 * Description: <b>proposal | plan | order | option | directive</b><br> 2599 * Type: <b>token</b><br> 2600 * Path: <b>CarePlan.intent</b><br> 2601 * </p> 2602 */ 2603 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 2604 2605 /** 2606 * Search parameter: <b>part-of</b> 2607 * <p> 2608 * Description: <b>Part of referenced CarePlan</b><br> 2609 * Type: <b>reference</b><br> 2610 * Path: <b>CarePlan.partOf</b><br> 2611 * </p> 2612 */ 2613 @SearchParamDefinition(name="part-of", path="CarePlan.partOf", description="Part of referenced CarePlan", type="reference", target={CarePlan.class } ) 2614 public static final String SP_PART_OF = "part-of"; 2615 /** 2616 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2617 * <p> 2618 * Description: <b>Part of referenced CarePlan</b><br> 2619 * Type: <b>reference</b><br> 2620 * Path: <b>CarePlan.partOf</b><br> 2621 * </p> 2622 */ 2623 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2624 2625/** 2626 * Constant for fluent queries to be used to add include statements. Specifies 2627 * the path value of "<b>CarePlan:part-of</b>". 2628 */ 2629 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("CarePlan:part-of").toLocked(); 2630 2631 /** 2632 * Search parameter: <b>replaces</b> 2633 * <p> 2634 * Description: <b>CarePlan replaced by this CarePlan</b><br> 2635 * Type: <b>reference</b><br> 2636 * Path: <b>CarePlan.replaces</b><br> 2637 * </p> 2638 */ 2639 @SearchParamDefinition(name="replaces", path="CarePlan.replaces", description="CarePlan replaced by this CarePlan", type="reference", target={CarePlan.class } ) 2640 public static final String SP_REPLACES = "replaces"; 2641 /** 2642 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 2643 * <p> 2644 * Description: <b>CarePlan replaced by this CarePlan</b><br> 2645 * Type: <b>reference</b><br> 2646 * Path: <b>CarePlan.replaces</b><br> 2647 * </p> 2648 */ 2649 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 2650 2651/** 2652 * Constant for fluent queries to be used to add include statements. Specifies 2653 * the path value of "<b>CarePlan:replaces</b>". 2654 */ 2655 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("CarePlan:replaces").toLocked(); 2656 2657 /** 2658 * Search parameter: <b>status</b> 2659 * <p> 2660 * Description: <b>draft | active | on-hold | revoked | completed | entered-in-error | unknown</b><br> 2661 * Type: <b>token</b><br> 2662 * Path: <b>CarePlan.status</b><br> 2663 * </p> 2664 */ 2665 @SearchParamDefinition(name="status", path="CarePlan.status", description="draft | active | on-hold | revoked | completed | entered-in-error | unknown", type="token" ) 2666 public static final String SP_STATUS = "status"; 2667 /** 2668 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2669 * <p> 2670 * Description: <b>draft | active | on-hold | revoked | completed | entered-in-error | unknown</b><br> 2671 * Type: <b>token</b><br> 2672 * Path: <b>CarePlan.status</b><br> 2673 * </p> 2674 */ 2675 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2676 2677 /** 2678 * Search parameter: <b>subject</b> 2679 * <p> 2680 * Description: <b>Who the care plan is for</b><br> 2681 * Type: <b>reference</b><br> 2682 * Path: <b>CarePlan.subject</b><br> 2683 * </p> 2684 */ 2685 @SearchParamDefinition(name="subject", path="CarePlan.subject", description="Who the care plan is for", type="reference", target={Group.class, Patient.class } ) 2686 public static final String SP_SUBJECT = "subject"; 2687 /** 2688 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2689 * <p> 2690 * Description: <b>Who the care plan is for</b><br> 2691 * Type: <b>reference</b><br> 2692 * Path: <b>CarePlan.subject</b><br> 2693 * </p> 2694 */ 2695 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2696 2697/** 2698 * Constant for fluent queries to be used to add include statements. Specifies 2699 * the path value of "<b>CarePlan:subject</b>". 2700 */ 2701 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CarePlan:subject").toLocked(); 2702 2703 /** 2704 * Search parameter: <b>date</b> 2705 * <p> 2706 * Description: <b>Multiple Resources: 2707 2708* [AdverseEvent](adverseevent.html): When the event occurred 2709* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2710* [Appointment](appointment.html): Appointment date/time. 2711* [AuditEvent](auditevent.html): Time when the event was recorded 2712* [CarePlan](careplan.html): Time period plan covers 2713* [CareTeam](careteam.html): A date within the coverage time period. 2714* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2715* [Composition](composition.html): Composition editing time 2716* [Consent](consent.html): When consent was agreed to 2717* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2718* [DocumentReference](documentreference.html): When this document reference was created 2719* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2720* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2721* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2722* [Flag](flag.html): Time period when flag is active 2723* [Immunization](immunization.html): Vaccination (non)-Administration Date 2724* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2725* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2726* [Invoice](invoice.html): Invoice date / posting date 2727* [List](list.html): When the list was prepared 2728* [MeasureReport](measurereport.html): The date of the measure report 2729* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2730* [Observation](observation.html): Clinically relevant time/time-period for observation 2731* [Procedure](procedure.html): When the procedure occurred or is occurring 2732* [ResearchSubject](researchsubject.html): Start and end of participation 2733* [RiskAssessment](riskassessment.html): When was assessment made? 2734* [SupplyRequest](supplyrequest.html): When the request was made 2735</b><br> 2736 * Type: <b>date</b><br> 2737 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2738 * </p> 2739 */ 2740 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2741 public static final String SP_DATE = "date"; 2742 /** 2743 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2744 * <p> 2745 * Description: <b>Multiple Resources: 2746 2747* [AdverseEvent](adverseevent.html): When the event occurred 2748* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2749* [Appointment](appointment.html): Appointment date/time. 2750* [AuditEvent](auditevent.html): Time when the event was recorded 2751* [CarePlan](careplan.html): Time period plan covers 2752* [CareTeam](careteam.html): A date within the coverage time period. 2753* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2754* [Composition](composition.html): Composition editing time 2755* [Consent](consent.html): When consent was agreed to 2756* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2757* [DocumentReference](documentreference.html): When this document reference was created 2758* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2759* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2760* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2761* [Flag](flag.html): Time period when flag is active 2762* [Immunization](immunization.html): Vaccination (non)-Administration Date 2763* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2764* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2765* [Invoice](invoice.html): Invoice date / posting date 2766* [List](list.html): When the list was prepared 2767* [MeasureReport](measurereport.html): The date of the measure report 2768* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2769* [Observation](observation.html): Clinically relevant time/time-period for observation 2770* [Procedure](procedure.html): When the procedure occurred or is occurring 2771* [ResearchSubject](researchsubject.html): Start and end of participation 2772* [RiskAssessment](riskassessment.html): When was assessment made? 2773* [SupplyRequest](supplyrequest.html): When the request was made 2774</b><br> 2775 * Type: <b>date</b><br> 2776 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2777 * </p> 2778 */ 2779 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2780 2781 /** 2782 * Search parameter: <b>encounter</b> 2783 * <p> 2784 * Description: <b>Multiple Resources: 2785 2786* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2787* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2788* [ChargeItem](chargeitem.html): Encounter associated with event 2789* [Claim](claim.html): Encounters associated with a billed line item 2790* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2791* [Communication](communication.html): The Encounter during which this Communication was created 2792* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2793* [Composition](composition.html): Context of the Composition 2794* [Condition](condition.html): The Encounter during which this Condition was created 2795* [DeviceRequest](devicerequest.html): Encounter during which request was created 2796* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2797* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2798* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2799* [Flag](flag.html): Alert relevant during encounter 2800* [ImagingStudy](imagingstudy.html): The context of the study 2801* [List](list.html): Context in which list created 2802* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2803* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2804* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2805* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2806* [Observation](observation.html): Encounter related to the observation 2807* [Procedure](procedure.html): The Encounter during which this Procedure was created 2808* [Provenance](provenance.html): Encounter related to the Provenance 2809* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2810* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2811* [RiskAssessment](riskassessment.html): Where was assessment performed? 2812* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2813* [Task](task.html): Search by encounter 2814* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2815</b><br> 2816 * Type: <b>reference</b><br> 2817 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2818 * </p> 2819 */ 2820 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2821 public static final String SP_ENCOUNTER = "encounter"; 2822 /** 2823 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2824 * <p> 2825 * Description: <b>Multiple Resources: 2826 2827* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2828* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2829* [ChargeItem](chargeitem.html): Encounter associated with event 2830* [Claim](claim.html): Encounters associated with a billed line item 2831* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2832* [Communication](communication.html): The Encounter during which this Communication was created 2833* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2834* [Composition](composition.html): Context of the Composition 2835* [Condition](condition.html): The Encounter during which this Condition was created 2836* [DeviceRequest](devicerequest.html): Encounter during which request was created 2837* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2838* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2839* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2840* [Flag](flag.html): Alert relevant during encounter 2841* [ImagingStudy](imagingstudy.html): The context of the study 2842* [List](list.html): Context in which list created 2843* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2844* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2845* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2846* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2847* [Observation](observation.html): Encounter related to the observation 2848* [Procedure](procedure.html): The Encounter during which this Procedure was created 2849* [Provenance](provenance.html): Encounter related to the Provenance 2850* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2851* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2852* [RiskAssessment](riskassessment.html): Where was assessment performed? 2853* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2854* [Task](task.html): Search by encounter 2855* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2856</b><br> 2857 * Type: <b>reference</b><br> 2858 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2859 * </p> 2860 */ 2861 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2862 2863/** 2864 * Constant for fluent queries to be used to add include statements. Specifies 2865 * the path value of "<b>CarePlan:encounter</b>". 2866 */ 2867 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("CarePlan:encounter").toLocked(); 2868 2869 /** 2870 * Search parameter: <b>identifier</b> 2871 * <p> 2872 * Description: <b>Multiple Resources: 2873 2874* [Account](account.html): Account number 2875* [AdverseEvent](adverseevent.html): Business identifier for the event 2876* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2877* [Appointment](appointment.html): An Identifier of the Appointment 2878* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2879* [Basic](basic.html): Business identifier 2880* [BodyStructure](bodystructure.html): Bodystructure identifier 2881* [CarePlan](careplan.html): External Ids for this plan 2882* [CareTeam](careteam.html): External Ids for this team 2883* [ChargeItem](chargeitem.html): Business Identifier for item 2884* [Claim](claim.html): The primary identifier of the financial resource 2885* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2886* [ClinicalImpression](clinicalimpression.html): Business identifier 2887* [Communication](communication.html): Unique identifier 2888* [CommunicationRequest](communicationrequest.html): Unique identifier 2889* [Composition](composition.html): Version-independent identifier for the Composition 2890* [Condition](condition.html): A unique identifier of the condition record 2891* [Consent](consent.html): Identifier for this record (external references) 2892* [Contract](contract.html): The identity of the contract 2893* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2894* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2895* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2896* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2897* [DeviceRequest](devicerequest.html): Business identifier for request/order 2898* [DeviceUsage](deviceusage.html): Search by identifier 2899* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2900* [DocumentReference](documentreference.html): Identifier of the attachment binary 2901* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2902* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2903* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2904* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2905* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2906* [Flag](flag.html): Business identifier 2907* [Goal](goal.html): External Ids for this goal 2908* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2909* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2910* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2911* [Immunization](immunization.html): Business identifier 2912* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2913* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2914* [Invoice](invoice.html): Business Identifier for item 2915* [List](list.html): Business identifier 2916* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2917* [Medication](medication.html): Returns medications with this external identifier 2918* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2919* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2920* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2921* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2922* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2923* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2924* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2925* [Observation](observation.html): The unique id for a particular observation 2926* [Person](person.html): A person Identifier 2927* [Procedure](procedure.html): A unique identifier for a procedure 2928* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2929* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2930* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2931* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2932* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2933* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2934* [Specimen](specimen.html): The unique identifier associated with the specimen 2935* [SupplyDelivery](supplydelivery.html): External identifier 2936* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2937* [Task](task.html): Search for a task instance by its business identifier 2938* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2939</b><br> 2940 * Type: <b>token</b><br> 2941 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2942 * </p> 2943 */ 2944 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2945 public static final String SP_IDENTIFIER = "identifier"; 2946 /** 2947 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2948 * <p> 2949 * Description: <b>Multiple Resources: 2950 2951* [Account](account.html): Account number 2952* [AdverseEvent](adverseevent.html): Business identifier for the event 2953* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2954* [Appointment](appointment.html): An Identifier of the Appointment 2955* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2956* [Basic](basic.html): Business identifier 2957* [BodyStructure](bodystructure.html): Bodystructure identifier 2958* [CarePlan](careplan.html): External Ids for this plan 2959* [CareTeam](careteam.html): External Ids for this team 2960* [ChargeItem](chargeitem.html): Business Identifier for item 2961* [Claim](claim.html): The primary identifier of the financial resource 2962* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2963* [ClinicalImpression](clinicalimpression.html): Business identifier 2964* [Communication](communication.html): Unique identifier 2965* [CommunicationRequest](communicationrequest.html): Unique identifier 2966* [Composition](composition.html): Version-independent identifier for the Composition 2967* [Condition](condition.html): A unique identifier of the condition record 2968* [Consent](consent.html): Identifier for this record (external references) 2969* [Contract](contract.html): The identity of the contract 2970* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2971* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2972* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2973* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2974* [DeviceRequest](devicerequest.html): Business identifier for request/order 2975* [DeviceUsage](deviceusage.html): Search by identifier 2976* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2977* [DocumentReference](documentreference.html): Identifier of the attachment binary 2978* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2979* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2980* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2981* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2982* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2983* [Flag](flag.html): Business identifier 2984* [Goal](goal.html): External Ids for this goal 2985* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2986* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2987* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2988* [Immunization](immunization.html): Business identifier 2989* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2990* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2991* [Invoice](invoice.html): Business Identifier for item 2992* [List](list.html): Business identifier 2993* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2994* [Medication](medication.html): Returns medications with this external identifier 2995* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2996* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2997* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2998* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2999* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3000* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3001* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3002* [Observation](observation.html): The unique id for a particular observation 3003* [Person](person.html): A person Identifier 3004* [Procedure](procedure.html): A unique identifier for a procedure 3005* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3006* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3007* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3008* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3009* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3010* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3011* [Specimen](specimen.html): The unique identifier associated with the specimen 3012* [SupplyDelivery](supplydelivery.html): External identifier 3013* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3014* [Task](task.html): Search for a task instance by its business identifier 3015* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3016</b><br> 3017 * Type: <b>token</b><br> 3018 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3019 * </p> 3020 */ 3021 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3022 3023 /** 3024 * Search parameter: <b>patient</b> 3025 * <p> 3026 * Description: <b>Multiple Resources: 3027 3028* [Account](account.html): The entity that caused the expenses 3029* [AdverseEvent](adverseevent.html): Subject impacted by event 3030* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3031* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3032* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3033* [AuditEvent](auditevent.html): Where the activity involved patient data 3034* [Basic](basic.html): Identifies the focus of this resource 3035* [BodyStructure](bodystructure.html): Who this is about 3036* [CarePlan](careplan.html): Who the care plan is for 3037* [CareTeam](careteam.html): Who care team is for 3038* [ChargeItem](chargeitem.html): Individual service was done for/to 3039* [Claim](claim.html): Patient receiving the products or services 3040* [ClaimResponse](claimresponse.html): The subject of care 3041* [ClinicalImpression](clinicalimpression.html): Patient assessed 3042* [Communication](communication.html): Focus of message 3043* [CommunicationRequest](communicationrequest.html): Focus of message 3044* [Composition](composition.html): Who and/or what the composition is about 3045* [Condition](condition.html): Who has the condition? 3046* [Consent](consent.html): Who the consent applies to 3047* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3048* [Coverage](coverage.html): Retrieve coverages for a patient 3049* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3050* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3051* [DetectedIssue](detectedissue.html): Associated patient 3052* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3053* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3054* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3055* [DocumentReference](documentreference.html): Who/what is the subject of the document 3056* [Encounter](encounter.html): The patient present at the encounter 3057* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3058* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3059* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3060* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3061* [Flag](flag.html): The identity of a subject to list flags for 3062* [Goal](goal.html): Who this goal is intended for 3063* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3064* [ImagingSelection](imagingselection.html): Who the study is about 3065* [ImagingStudy](imagingstudy.html): Who the study is about 3066* [Immunization](immunization.html): The patient for the vaccination record 3067* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3068* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3069* [Invoice](invoice.html): Recipient(s) of goods and services 3070* [List](list.html): If all resources have the same subject 3071* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3072* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3073* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3074* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3075* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3076* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3077* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3078* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3079* [Observation](observation.html): The subject that the observation is about (if patient) 3080* [Person](person.html): The Person links to this Patient 3081* [Procedure](procedure.html): Search by subject - a patient 3082* [Provenance](provenance.html): Where the activity involved patient data 3083* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3084* [RelatedPerson](relatedperson.html): The patient this related person is related to 3085* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3086* [ResearchSubject](researchsubject.html): Who or what is part of study 3087* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3088* [ServiceRequest](servicerequest.html): Search by subject - a patient 3089* [Specimen](specimen.html): The patient the specimen comes from 3090* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3091* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3092* [Task](task.html): Search by patient 3093* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3094</b><br> 3095 * Type: <b>reference</b><br> 3096 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3097 * </p> 3098 */ 3099 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3100 public static final String SP_PATIENT = "patient"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3103 * <p> 3104 * Description: <b>Multiple Resources: 3105 3106* [Account](account.html): The entity that caused the expenses 3107* [AdverseEvent](adverseevent.html): Subject impacted by event 3108* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3109* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3110* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3111* [AuditEvent](auditevent.html): Where the activity involved patient data 3112* [Basic](basic.html): Identifies the focus of this resource 3113* [BodyStructure](bodystructure.html): Who this is about 3114* [CarePlan](careplan.html): Who the care plan is for 3115* [CareTeam](careteam.html): Who care team is for 3116* [ChargeItem](chargeitem.html): Individual service was done for/to 3117* [Claim](claim.html): Patient receiving the products or services 3118* [ClaimResponse](claimresponse.html): The subject of care 3119* [ClinicalImpression](clinicalimpression.html): Patient assessed 3120* [Communication](communication.html): Focus of message 3121* [CommunicationRequest](communicationrequest.html): Focus of message 3122* [Composition](composition.html): Who and/or what the composition is about 3123* [Condition](condition.html): Who has the condition? 3124* [Consent](consent.html): Who the consent applies to 3125* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3126* [Coverage](coverage.html): Retrieve coverages for a patient 3127* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3128* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3129* [DetectedIssue](detectedissue.html): Associated patient 3130* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3131* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3132* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3133* [DocumentReference](documentreference.html): Who/what is the subject of the document 3134* [Encounter](encounter.html): The patient present at the encounter 3135* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3136* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3137* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3138* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3139* [Flag](flag.html): The identity of a subject to list flags for 3140* [Goal](goal.html): Who this goal is intended for 3141* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3142* [ImagingSelection](imagingselection.html): Who the study is about 3143* [ImagingStudy](imagingstudy.html): Who the study is about 3144* [Immunization](immunization.html): The patient for the vaccination record 3145* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3146* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3147* [Invoice](invoice.html): Recipient(s) of goods and services 3148* [List](list.html): If all resources have the same subject 3149* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3150* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3151* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3152* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3153* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3154* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3155* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3156* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3157* [Observation](observation.html): The subject that the observation is about (if patient) 3158* [Person](person.html): The Person links to this Patient 3159* [Procedure](procedure.html): Search by subject - a patient 3160* [Provenance](provenance.html): Where the activity involved patient data 3161* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3162* [RelatedPerson](relatedperson.html): The patient this related person is related to 3163* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3164* [ResearchSubject](researchsubject.html): Who or what is part of study 3165* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3166* [ServiceRequest](servicerequest.html): Search by subject - a patient 3167* [Specimen](specimen.html): The patient the specimen comes from 3168* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3169* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3170* [Task](task.html): Search by patient 3171* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3172</b><br> 3173 * Type: <b>reference</b><br> 3174 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3175 * </p> 3176 */ 3177 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3178 3179/** 3180 * Constant for fluent queries to be used to add include statements. Specifies 3181 * the path value of "<b>CarePlan:patient</b>". 3182 */ 3183 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CarePlan:patient").toLocked(); 3184 3185 3186} 3187