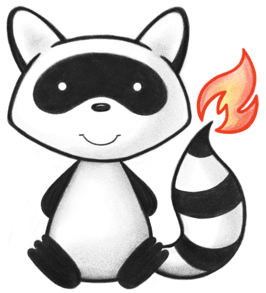
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care. 052 */ 053@ResourceDef(name="CareTeam", profile="http://hl7.org/fhir/StructureDefinition/CareTeam") 054public class CareTeam extends DomainResource { 055 056 public enum CareTeamStatus { 057 /** 058 * The care team has been drafted and proposed, but not yet participating in the coordination and delivery of patient care. 059 */ 060 PROPOSED, 061 /** 062 * The care team is currently participating in the coordination and delivery of care. 063 */ 064 ACTIVE, 065 /** 066 * The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care. 067 */ 068 SUSPENDED, 069 /** 070 * The care team was, but is no longer, participating in the coordination and delivery of care. 071 */ 072 INACTIVE, 073 /** 074 * The care team should have never existed. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static CareTeamStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("proposed".equals(codeString)) 085 return PROPOSED; 086 if ("active".equals(codeString)) 087 return ACTIVE; 088 if ("suspended".equals(codeString)) 089 return SUSPENDED; 090 if ("inactive".equals(codeString)) 091 return INACTIVE; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown CareTeamStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case PROPOSED: return "proposed"; 102 case ACTIVE: return "active"; 103 case SUSPENDED: return "suspended"; 104 case INACTIVE: return "inactive"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case PROPOSED: return "http://hl7.org/fhir/care-team-status"; 113 case ACTIVE: return "http://hl7.org/fhir/care-team-status"; 114 case SUSPENDED: return "http://hl7.org/fhir/care-team-status"; 115 case INACTIVE: return "http://hl7.org/fhir/care-team-status"; 116 case ENTEREDINERROR: return "http://hl7.org/fhir/care-team-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case PROPOSED: return "The care team has been drafted and proposed, but not yet participating in the coordination and delivery of patient care."; 124 case ACTIVE: return "The care team is currently participating in the coordination and delivery of care."; 125 case SUSPENDED: return "The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care."; 126 case INACTIVE: return "The care team was, but is no longer, participating in the coordination and delivery of care."; 127 case ENTEREDINERROR: return "The care team should have never existed."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case PROPOSED: return "Proposed"; 135 case ACTIVE: return "Active"; 136 case SUSPENDED: return "Suspended"; 137 case INACTIVE: return "Inactive"; 138 case ENTEREDINERROR: return "Entered in Error"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class CareTeamStatusEnumFactory implements EnumFactory<CareTeamStatus> { 146 public CareTeamStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("proposed".equals(codeString)) 151 return CareTeamStatus.PROPOSED; 152 if ("active".equals(codeString)) 153 return CareTeamStatus.ACTIVE; 154 if ("suspended".equals(codeString)) 155 return CareTeamStatus.SUSPENDED; 156 if ("inactive".equals(codeString)) 157 return CareTeamStatus.INACTIVE; 158 if ("entered-in-error".equals(codeString)) 159 return CareTeamStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown CareTeamStatus code '"+codeString+"'"); 161 } 162 public Enumeration<CareTeamStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 170 if ("proposed".equals(codeString)) 171 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.PROPOSED, code); 172 if ("active".equals(codeString)) 173 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ACTIVE, code); 174 if ("suspended".equals(codeString)) 175 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.SUSPENDED, code); 176 if ("inactive".equals(codeString)) 177 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.INACTIVE, code); 178 if ("entered-in-error".equals(codeString)) 179 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ENTEREDINERROR, code); 180 throw new FHIRException("Unknown CareTeamStatus code '"+codeString+"'"); 181 } 182 public String toCode(CareTeamStatus code) { 183 if (code == CareTeamStatus.NULL) 184 return null; 185 if (code == CareTeamStatus.PROPOSED) 186 return "proposed"; 187 if (code == CareTeamStatus.ACTIVE) 188 return "active"; 189 if (code == CareTeamStatus.SUSPENDED) 190 return "suspended"; 191 if (code == CareTeamStatus.INACTIVE) 192 return "inactive"; 193 if (code == CareTeamStatus.ENTEREDINERROR) 194 return "entered-in-error"; 195 return "?"; 196 } 197 public String toSystem(CareTeamStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class CareTeamParticipantComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc. 206 */ 207 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 208 @Description(shortDefinition="Type of involvement", formalDefinition="Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc." ) 209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 210 protected CodeableConcept role; 211 212 /** 213 * The specific person or organization who is participating/expected to participate in the care team. 214 */ 215 @Child(name = "member", type = {Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, Organization.class, CareTeam.class}, order=2, min=0, max=1, modifier=false, summary=true) 216 @Description(shortDefinition="Who is involved", formalDefinition="The specific person or organization who is participating/expected to participate in the care team." ) 217 protected Reference member; 218 219 /** 220 * The organization of the practitioner. 221 */ 222 @Child(name = "onBehalfOf", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 223 @Description(shortDefinition="Organization of the practitioner", formalDefinition="The organization of the practitioner." ) 224 protected Reference onBehalfOf; 225 226 /** 227 * When the member is generally available within this care team. 228 */ 229 @Child(name = "coverage", type = {Period.class, Timing.class}, order=4, min=0, max=1, modifier=false, summary=false) 230 @Description(shortDefinition="When the member is generally available within this care team", formalDefinition="When the member is generally available within this care team." ) 231 protected DataType coverage; 232 233 private static final long serialVersionUID = 192079749L; 234 235 /** 236 * Constructor 237 */ 238 public CareTeamParticipantComponent() { 239 super(); 240 } 241 242 /** 243 * @return {@link #role} (Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc.) 244 */ 245 public CodeableConcept getRole() { 246 if (this.role == null) 247 if (Configuration.errorOnAutoCreate()) 248 throw new Error("Attempt to auto-create CareTeamParticipantComponent.role"); 249 else if (Configuration.doAutoCreate()) 250 this.role = new CodeableConcept(); // cc 251 return this.role; 252 } 253 254 public boolean hasRole() { 255 return this.role != null && !this.role.isEmpty(); 256 } 257 258 /** 259 * @param value {@link #role} (Indicates specific responsibility of an individual within the care team, such as "Primary care physician", "Trained social worker counselor", "Caregiver", etc.) 260 */ 261 public CareTeamParticipantComponent setRole(CodeableConcept value) { 262 this.role = value; 263 return this; 264 } 265 266 /** 267 * @return {@link #member} (The specific person or organization who is participating/expected to participate in the care team.) 268 */ 269 public Reference getMember() { 270 if (this.member == null) 271 if (Configuration.errorOnAutoCreate()) 272 throw new Error("Attempt to auto-create CareTeamParticipantComponent.member"); 273 else if (Configuration.doAutoCreate()) 274 this.member = new Reference(); // cc 275 return this.member; 276 } 277 278 public boolean hasMember() { 279 return this.member != null && !this.member.isEmpty(); 280 } 281 282 /** 283 * @param value {@link #member} (The specific person or organization who is participating/expected to participate in the care team.) 284 */ 285 public CareTeamParticipantComponent setMember(Reference value) { 286 this.member = value; 287 return this; 288 } 289 290 /** 291 * @return {@link #onBehalfOf} (The organization of the practitioner.) 292 */ 293 public Reference getOnBehalfOf() { 294 if (this.onBehalfOf == null) 295 if (Configuration.errorOnAutoCreate()) 296 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 297 else if (Configuration.doAutoCreate()) 298 this.onBehalfOf = new Reference(); // cc 299 return this.onBehalfOf; 300 } 301 302 public boolean hasOnBehalfOf() { 303 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 304 } 305 306 /** 307 * @param value {@link #onBehalfOf} (The organization of the practitioner.) 308 */ 309 public CareTeamParticipantComponent setOnBehalfOf(Reference value) { 310 this.onBehalfOf = value; 311 return this; 312 } 313 314 /** 315 * @return {@link #coverage} (When the member is generally available within this care team.) 316 */ 317 public DataType getCoverage() { 318 return this.coverage; 319 } 320 321 /** 322 * @return {@link #coverage} (When the member is generally available within this care team.) 323 */ 324 public Period getCoveragePeriod() throws FHIRException { 325 if (this.coverage == null) 326 this.coverage = new Period(); 327 if (!(this.coverage instanceof Period)) 328 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.coverage.getClass().getName()+" was encountered"); 329 return (Period) this.coverage; 330 } 331 332 public boolean hasCoveragePeriod() { 333 return this != null && this.coverage instanceof Period; 334 } 335 336 /** 337 * @return {@link #coverage} (When the member is generally available within this care team.) 338 */ 339 public Timing getCoverageTiming() throws FHIRException { 340 if (this.coverage == null) 341 this.coverage = new Timing(); 342 if (!(this.coverage instanceof Timing)) 343 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.coverage.getClass().getName()+" was encountered"); 344 return (Timing) this.coverage; 345 } 346 347 public boolean hasCoverageTiming() { 348 return this != null && this.coverage instanceof Timing; 349 } 350 351 public boolean hasCoverage() { 352 return this.coverage != null && !this.coverage.isEmpty(); 353 } 354 355 /** 356 * @param value {@link #coverage} (When the member is generally available within this care team.) 357 */ 358 public CareTeamParticipantComponent setCoverage(DataType value) { 359 if (value != null && !(value instanceof Period || value instanceof Timing)) 360 throw new FHIRException("Not the right type for CareTeam.participant.coverage[x]: "+value.fhirType()); 361 this.coverage = value; 362 return this; 363 } 364 365 protected void listChildren(List<Property> children) { 366 super.listChildren(children); 367 children.add(new Property("role", "CodeableConcept", "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 0, 1, role)); 368 children.add(new Property("member", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, member)); 369 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, onBehalfOf)); 370 children.add(new Property("coverage[x]", "Period|Timing", "When the member is generally available within this care team.", 0, 1, coverage)); 371 } 372 373 @Override 374 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 375 switch (_hash) { 376 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 0, 1, role); 377 case -1077769574: /*member*/ return new Property("member", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, member); 378 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, onBehalfOf); 379 case 227689880: /*coverage[x]*/ return new Property("coverage[x]", "Period|Timing", "When the member is generally available within this care team.", 0, 1, coverage); 380 case -351767064: /*coverage*/ return new Property("coverage[x]", "Period|Timing", "When the member is generally available within this care team.", 0, 1, coverage); 381 case 1024117193: /*coveragePeriod*/ return new Property("coverage[x]", "Period", "When the member is generally available within this care team.", 0, 1, coverage); 382 case 1142178898: /*coverageTiming*/ return new Property("coverage[x]", "Timing", "When the member is generally available within this care team.", 0, 1, coverage); 383 default: return super.getNamedProperty(_hash, _name, _checkValid); 384 } 385 386 } 387 388 @Override 389 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 390 switch (hash) { 391 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 392 case -1077769574: /*member*/ return this.member == null ? new Base[0] : new Base[] {this.member}; // Reference 393 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 394 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // DataType 395 default: return super.getProperty(hash, name, checkValid); 396 } 397 398 } 399 400 @Override 401 public Base setProperty(int hash, String name, Base value) throws FHIRException { 402 switch (hash) { 403 case 3506294: // role 404 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 405 return value; 406 case -1077769574: // member 407 this.member = TypeConvertor.castToReference(value); // Reference 408 return value; 409 case -14402964: // onBehalfOf 410 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 411 return value; 412 case -351767064: // coverage 413 this.coverage = TypeConvertor.castToType(value); // DataType 414 return value; 415 default: return super.setProperty(hash, name, value); 416 } 417 418 } 419 420 @Override 421 public Base setProperty(String name, Base value) throws FHIRException { 422 if (name.equals("role")) { 423 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 424 } else if (name.equals("member")) { 425 this.member = TypeConvertor.castToReference(value); // Reference 426 } else if (name.equals("onBehalfOf")) { 427 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 428 } else if (name.equals("coverage[x]")) { 429 this.coverage = TypeConvertor.castToType(value); // DataType 430 } else 431 return super.setProperty(name, value); 432 return value; 433 } 434 435 @Override 436 public void removeChild(String name, Base value) throws FHIRException { 437 if (name.equals("role")) { 438 this.role = null; 439 } else if (name.equals("member")) { 440 this.member = null; 441 } else if (name.equals("onBehalfOf")) { 442 this.onBehalfOf = null; 443 } else if (name.equals("coverage[x]")) { 444 this.coverage = null; 445 } else 446 super.removeChild(name, value); 447 448 } 449 450 @Override 451 public Base makeProperty(int hash, String name) throws FHIRException { 452 switch (hash) { 453 case 3506294: return getRole(); 454 case -1077769574: return getMember(); 455 case -14402964: return getOnBehalfOf(); 456 case 227689880: return getCoverage(); 457 case -351767064: return getCoverage(); 458 default: return super.makeProperty(hash, name); 459 } 460 461 } 462 463 @Override 464 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 465 switch (hash) { 466 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 467 case -1077769574: /*member*/ return new String[] {"Reference"}; 468 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 469 case -351767064: /*coverage*/ return new String[] {"Period", "Timing"}; 470 default: return super.getTypesForProperty(hash, name); 471 } 472 473 } 474 475 @Override 476 public Base addChild(String name) throws FHIRException { 477 if (name.equals("role")) { 478 this.role = new CodeableConcept(); 479 return this.role; 480 } 481 else if (name.equals("member")) { 482 this.member = new Reference(); 483 return this.member; 484 } 485 else if (name.equals("onBehalfOf")) { 486 this.onBehalfOf = new Reference(); 487 return this.onBehalfOf; 488 } 489 else if (name.equals("coveragePeriod")) { 490 this.coverage = new Period(); 491 return this.coverage; 492 } 493 else if (name.equals("coverageTiming")) { 494 this.coverage = new Timing(); 495 return this.coverage; 496 } 497 else 498 return super.addChild(name); 499 } 500 501 public CareTeamParticipantComponent copy() { 502 CareTeamParticipantComponent dst = new CareTeamParticipantComponent(); 503 copyValues(dst); 504 return dst; 505 } 506 507 public void copyValues(CareTeamParticipantComponent dst) { 508 super.copyValues(dst); 509 dst.role = role == null ? null : role.copy(); 510 dst.member = member == null ? null : member.copy(); 511 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 512 dst.coverage = coverage == null ? null : coverage.copy(); 513 } 514 515 @Override 516 public boolean equalsDeep(Base other_) { 517 if (!super.equalsDeep(other_)) 518 return false; 519 if (!(other_ instanceof CareTeamParticipantComponent)) 520 return false; 521 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 522 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 523 && compareDeep(coverage, o.coverage, true); 524 } 525 526 @Override 527 public boolean equalsShallow(Base other_) { 528 if (!super.equalsShallow(other_)) 529 return false; 530 if (!(other_ instanceof CareTeamParticipantComponent)) 531 return false; 532 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 533 return true; 534 } 535 536 public boolean isEmpty() { 537 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, member, onBehalfOf 538 , coverage); 539 } 540 541 public String fhirType() { 542 return "CareTeam.participant"; 543 544 } 545 546 } 547 548 /** 549 * Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 550 */ 551 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 552 @Description(shortDefinition="External Ids for this team", formalDefinition="Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 553 protected List<Identifier> identifier; 554 555 /** 556 * Indicates the current state of the care team. 557 */ 558 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 559 @Description(shortDefinition="proposed | active | suspended | inactive | entered-in-error", formalDefinition="Indicates the current state of the care team." ) 560 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-team-status") 561 protected Enumeration<CareTeamStatus> status; 562 563 /** 564 * Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team. 565 */ 566 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 567 @Description(shortDefinition="Type of team", formalDefinition="Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team." ) 568 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/care-team-category") 569 protected List<CodeableConcept> category; 570 571 /** 572 * A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 573 */ 574 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 575 @Description(shortDefinition="Name of the team, such as crisis assessment team", formalDefinition="A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams." ) 576 protected StringType name; 577 578 /** 579 * Identifies the patient or group whose intended care is handled by the team. 580 */ 581 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 582 @Description(shortDefinition="Who care team is for", formalDefinition="Identifies the patient or group whose intended care is handled by the team." ) 583 protected Reference subject; 584 585 /** 586 * Indicates when the team did (or is intended to) come into effect and end. 587 */ 588 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 589 @Description(shortDefinition="Time period team covers", formalDefinition="Indicates when the team did (or is intended to) come into effect and end." ) 590 protected Period period; 591 592 /** 593 * Identifies all people and organizations who are expected to be involved in the care team. 594 */ 595 @Child(name = "participant", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 596 @Description(shortDefinition="Members of the team", formalDefinition="Identifies all people and organizations who are expected to be involved in the care team." ) 597 protected List<CareTeamParticipantComponent> participant; 598 599 /** 600 * Describes why the care team exists. 601 */ 602 @Child(name = "reason", type = {CodeableReference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 603 @Description(shortDefinition="Why the care team exists", formalDefinition="Describes why the care team exists." ) 604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 605 protected List<CodeableReference> reason; 606 607 /** 608 * The organization responsible for the care team. 609 */ 610 @Child(name = "managingOrganization", type = {Organization.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 611 @Description(shortDefinition="Organization responsible for the care team", formalDefinition="The organization responsible for the care team." ) 612 protected List<Reference> managingOrganization; 613 614 /** 615 * A central contact detail for the care team (that applies to all members). 616 */ 617 @Child(name = "telecom", type = {ContactPoint.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 618 @Description(shortDefinition="A contact detail for the care team (that applies to all members)", formalDefinition="A central contact detail for the care team (that applies to all members)." ) 619 protected List<ContactPoint> telecom; 620 621 /** 622 * Comments made about the CareTeam. 623 */ 624 @Child(name = "note", type = {Annotation.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 625 @Description(shortDefinition="Comments made about the CareTeam", formalDefinition="Comments made about the CareTeam." ) 626 protected List<Annotation> note; 627 628 private static final long serialVersionUID = 1147350970L; 629 630 /** 631 * Constructor 632 */ 633 public CareTeam() { 634 super(); 635 } 636 637 /** 638 * @return {@link #identifier} (Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 639 */ 640 public List<Identifier> getIdentifier() { 641 if (this.identifier == null) 642 this.identifier = new ArrayList<Identifier>(); 643 return this.identifier; 644 } 645 646 /** 647 * @return Returns a reference to <code>this</code> for easy method chaining 648 */ 649 public CareTeam setIdentifier(List<Identifier> theIdentifier) { 650 this.identifier = theIdentifier; 651 return this; 652 } 653 654 public boolean hasIdentifier() { 655 if (this.identifier == null) 656 return false; 657 for (Identifier item : this.identifier) 658 if (!item.isEmpty()) 659 return true; 660 return false; 661 } 662 663 public Identifier addIdentifier() { //3 664 Identifier t = new Identifier(); 665 if (this.identifier == null) 666 this.identifier = new ArrayList<Identifier>(); 667 this.identifier.add(t); 668 return t; 669 } 670 671 public CareTeam addIdentifier(Identifier t) { //3 672 if (t == null) 673 return this; 674 if (this.identifier == null) 675 this.identifier = new ArrayList<Identifier>(); 676 this.identifier.add(t); 677 return this; 678 } 679 680 /** 681 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 682 */ 683 public Identifier getIdentifierFirstRep() { 684 if (getIdentifier().isEmpty()) { 685 addIdentifier(); 686 } 687 return getIdentifier().get(0); 688 } 689 690 /** 691 * @return {@link #status} (Indicates the current state of the care team.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 692 */ 693 public Enumeration<CareTeamStatus> getStatusElement() { 694 if (this.status == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create CareTeam.status"); 697 else if (Configuration.doAutoCreate()) 698 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); // bb 699 return this.status; 700 } 701 702 public boolean hasStatusElement() { 703 return this.status != null && !this.status.isEmpty(); 704 } 705 706 public boolean hasStatus() { 707 return this.status != null && !this.status.isEmpty(); 708 } 709 710 /** 711 * @param value {@link #status} (Indicates the current state of the care team.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 712 */ 713 public CareTeam setStatusElement(Enumeration<CareTeamStatus> value) { 714 this.status = value; 715 return this; 716 } 717 718 /** 719 * @return Indicates the current state of the care team. 720 */ 721 public CareTeamStatus getStatus() { 722 return this.status == null ? null : this.status.getValue(); 723 } 724 725 /** 726 * @param value Indicates the current state of the care team. 727 */ 728 public CareTeam setStatus(CareTeamStatus value) { 729 if (value == null) 730 this.status = null; 731 else { 732 if (this.status == null) 733 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); 734 this.status.setValue(value); 735 } 736 return this; 737 } 738 739 /** 740 * @return {@link #category} (Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.) 741 */ 742 public List<CodeableConcept> getCategory() { 743 if (this.category == null) 744 this.category = new ArrayList<CodeableConcept>(); 745 return this.category; 746 } 747 748 /** 749 * @return Returns a reference to <code>this</code> for easy method chaining 750 */ 751 public CareTeam setCategory(List<CodeableConcept> theCategory) { 752 this.category = theCategory; 753 return this; 754 } 755 756 public boolean hasCategory() { 757 if (this.category == null) 758 return false; 759 for (CodeableConcept item : this.category) 760 if (!item.isEmpty()) 761 return true; 762 return false; 763 } 764 765 public CodeableConcept addCategory() { //3 766 CodeableConcept t = new CodeableConcept(); 767 if (this.category == null) 768 this.category = new ArrayList<CodeableConcept>(); 769 this.category.add(t); 770 return t; 771 } 772 773 public CareTeam addCategory(CodeableConcept t) { //3 774 if (t == null) 775 return this; 776 if (this.category == null) 777 this.category = new ArrayList<CodeableConcept>(); 778 this.category.add(t); 779 return this; 780 } 781 782 /** 783 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 784 */ 785 public CodeableConcept getCategoryFirstRep() { 786 if (getCategory().isEmpty()) { 787 addCategory(); 788 } 789 return getCategory().get(0); 790 } 791 792 /** 793 * @return {@link #name} (A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 794 */ 795 public StringType getNameElement() { 796 if (this.name == null) 797 if (Configuration.errorOnAutoCreate()) 798 throw new Error("Attempt to auto-create CareTeam.name"); 799 else if (Configuration.doAutoCreate()) 800 this.name = new StringType(); // bb 801 return this.name; 802 } 803 804 public boolean hasNameElement() { 805 return this.name != null && !this.name.isEmpty(); 806 } 807 808 public boolean hasName() { 809 return this.name != null && !this.name.isEmpty(); 810 } 811 812 /** 813 * @param value {@link #name} (A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 814 */ 815 public CareTeam setNameElement(StringType value) { 816 this.name = value; 817 return this; 818 } 819 820 /** 821 * @return A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 822 */ 823 public String getName() { 824 return this.name == null ? null : this.name.getValue(); 825 } 826 827 /** 828 * @param value A label for human use intended to distinguish like teams. E.g. the "red" vs. "green" trauma teams. 829 */ 830 public CareTeam setName(String value) { 831 if (Utilities.noString(value)) 832 this.name = null; 833 else { 834 if (this.name == null) 835 this.name = new StringType(); 836 this.name.setValue(value); 837 } 838 return this; 839 } 840 841 /** 842 * @return {@link #subject} (Identifies the patient or group whose intended care is handled by the team.) 843 */ 844 public Reference getSubject() { 845 if (this.subject == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create CareTeam.subject"); 848 else if (Configuration.doAutoCreate()) 849 this.subject = new Reference(); // cc 850 return this.subject; 851 } 852 853 public boolean hasSubject() { 854 return this.subject != null && !this.subject.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #subject} (Identifies the patient or group whose intended care is handled by the team.) 859 */ 860 public CareTeam setSubject(Reference value) { 861 this.subject = value; 862 return this; 863 } 864 865 /** 866 * @return {@link #period} (Indicates when the team did (or is intended to) come into effect and end.) 867 */ 868 public Period getPeriod() { 869 if (this.period == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create CareTeam.period"); 872 else if (Configuration.doAutoCreate()) 873 this.period = new Period(); // cc 874 return this.period; 875 } 876 877 public boolean hasPeriod() { 878 return this.period != null && !this.period.isEmpty(); 879 } 880 881 /** 882 * @param value {@link #period} (Indicates when the team did (or is intended to) come into effect and end.) 883 */ 884 public CareTeam setPeriod(Period value) { 885 this.period = value; 886 return this; 887 } 888 889 /** 890 * @return {@link #participant} (Identifies all people and organizations who are expected to be involved in the care team.) 891 */ 892 public List<CareTeamParticipantComponent> getParticipant() { 893 if (this.participant == null) 894 this.participant = new ArrayList<CareTeamParticipantComponent>(); 895 return this.participant; 896 } 897 898 /** 899 * @return Returns a reference to <code>this</code> for easy method chaining 900 */ 901 public CareTeam setParticipant(List<CareTeamParticipantComponent> theParticipant) { 902 this.participant = theParticipant; 903 return this; 904 } 905 906 public boolean hasParticipant() { 907 if (this.participant == null) 908 return false; 909 for (CareTeamParticipantComponent item : this.participant) 910 if (!item.isEmpty()) 911 return true; 912 return false; 913 } 914 915 public CareTeamParticipantComponent addParticipant() { //3 916 CareTeamParticipantComponent t = new CareTeamParticipantComponent(); 917 if (this.participant == null) 918 this.participant = new ArrayList<CareTeamParticipantComponent>(); 919 this.participant.add(t); 920 return t; 921 } 922 923 public CareTeam addParticipant(CareTeamParticipantComponent t) { //3 924 if (t == null) 925 return this; 926 if (this.participant == null) 927 this.participant = new ArrayList<CareTeamParticipantComponent>(); 928 this.participant.add(t); 929 return this; 930 } 931 932 /** 933 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 934 */ 935 public CareTeamParticipantComponent getParticipantFirstRep() { 936 if (getParticipant().isEmpty()) { 937 addParticipant(); 938 } 939 return getParticipant().get(0); 940 } 941 942 /** 943 * @return {@link #reason} (Describes why the care team exists.) 944 */ 945 public List<CodeableReference> getReason() { 946 if (this.reason == null) 947 this.reason = new ArrayList<CodeableReference>(); 948 return this.reason; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public CareTeam setReason(List<CodeableReference> theReason) { 955 this.reason = theReason; 956 return this; 957 } 958 959 public boolean hasReason() { 960 if (this.reason == null) 961 return false; 962 for (CodeableReference item : this.reason) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 public CodeableReference addReason() { //3 969 CodeableReference t = new CodeableReference(); 970 if (this.reason == null) 971 this.reason = new ArrayList<CodeableReference>(); 972 this.reason.add(t); 973 return t; 974 } 975 976 public CareTeam addReason(CodeableReference t) { //3 977 if (t == null) 978 return this; 979 if (this.reason == null) 980 this.reason = new ArrayList<CodeableReference>(); 981 this.reason.add(t); 982 return this; 983 } 984 985 /** 986 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 987 */ 988 public CodeableReference getReasonFirstRep() { 989 if (getReason().isEmpty()) { 990 addReason(); 991 } 992 return getReason().get(0); 993 } 994 995 /** 996 * @return {@link #managingOrganization} (The organization responsible for the care team.) 997 */ 998 public List<Reference> getManagingOrganization() { 999 if (this.managingOrganization == null) 1000 this.managingOrganization = new ArrayList<Reference>(); 1001 return this.managingOrganization; 1002 } 1003 1004 /** 1005 * @return Returns a reference to <code>this</code> for easy method chaining 1006 */ 1007 public CareTeam setManagingOrganization(List<Reference> theManagingOrganization) { 1008 this.managingOrganization = theManagingOrganization; 1009 return this; 1010 } 1011 1012 public boolean hasManagingOrganization() { 1013 if (this.managingOrganization == null) 1014 return false; 1015 for (Reference item : this.managingOrganization) 1016 if (!item.isEmpty()) 1017 return true; 1018 return false; 1019 } 1020 1021 public Reference addManagingOrganization() { //3 1022 Reference t = new Reference(); 1023 if (this.managingOrganization == null) 1024 this.managingOrganization = new ArrayList<Reference>(); 1025 this.managingOrganization.add(t); 1026 return t; 1027 } 1028 1029 public CareTeam addManagingOrganization(Reference t) { //3 1030 if (t == null) 1031 return this; 1032 if (this.managingOrganization == null) 1033 this.managingOrganization = new ArrayList<Reference>(); 1034 this.managingOrganization.add(t); 1035 return this; 1036 } 1037 1038 /** 1039 * @return The first repetition of repeating field {@link #managingOrganization}, creating it if it does not already exist {3} 1040 */ 1041 public Reference getManagingOrganizationFirstRep() { 1042 if (getManagingOrganization().isEmpty()) { 1043 addManagingOrganization(); 1044 } 1045 return getManagingOrganization().get(0); 1046 } 1047 1048 /** 1049 * @return {@link #telecom} (A central contact detail for the care team (that applies to all members).) 1050 */ 1051 public List<ContactPoint> getTelecom() { 1052 if (this.telecom == null) 1053 this.telecom = new ArrayList<ContactPoint>(); 1054 return this.telecom; 1055 } 1056 1057 /** 1058 * @return Returns a reference to <code>this</code> for easy method chaining 1059 */ 1060 public CareTeam setTelecom(List<ContactPoint> theTelecom) { 1061 this.telecom = theTelecom; 1062 return this; 1063 } 1064 1065 public boolean hasTelecom() { 1066 if (this.telecom == null) 1067 return false; 1068 for (ContactPoint item : this.telecom) 1069 if (!item.isEmpty()) 1070 return true; 1071 return false; 1072 } 1073 1074 public ContactPoint addTelecom() { //3 1075 ContactPoint t = new ContactPoint(); 1076 if (this.telecom == null) 1077 this.telecom = new ArrayList<ContactPoint>(); 1078 this.telecom.add(t); 1079 return t; 1080 } 1081 1082 public CareTeam addTelecom(ContactPoint t) { //3 1083 if (t == null) 1084 return this; 1085 if (this.telecom == null) 1086 this.telecom = new ArrayList<ContactPoint>(); 1087 this.telecom.add(t); 1088 return this; 1089 } 1090 1091 /** 1092 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 1093 */ 1094 public ContactPoint getTelecomFirstRep() { 1095 if (getTelecom().isEmpty()) { 1096 addTelecom(); 1097 } 1098 return getTelecom().get(0); 1099 } 1100 1101 /** 1102 * @return {@link #note} (Comments made about the CareTeam.) 1103 */ 1104 public List<Annotation> getNote() { 1105 if (this.note == null) 1106 this.note = new ArrayList<Annotation>(); 1107 return this.note; 1108 } 1109 1110 /** 1111 * @return Returns a reference to <code>this</code> for easy method chaining 1112 */ 1113 public CareTeam setNote(List<Annotation> theNote) { 1114 this.note = theNote; 1115 return this; 1116 } 1117 1118 public boolean hasNote() { 1119 if (this.note == null) 1120 return false; 1121 for (Annotation item : this.note) 1122 if (!item.isEmpty()) 1123 return true; 1124 return false; 1125 } 1126 1127 public Annotation addNote() { //3 1128 Annotation t = new Annotation(); 1129 if (this.note == null) 1130 this.note = new ArrayList<Annotation>(); 1131 this.note.add(t); 1132 return t; 1133 } 1134 1135 public CareTeam addNote(Annotation t) { //3 1136 if (t == null) 1137 return this; 1138 if (this.note == null) 1139 this.note = new ArrayList<Annotation>(); 1140 this.note.add(t); 1141 return this; 1142 } 1143 1144 /** 1145 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1146 */ 1147 public Annotation getNoteFirstRep() { 1148 if (getNote().isEmpty()) { 1149 addNote(); 1150 } 1151 return getNote().get(0); 1152 } 1153 1154 protected void listChildren(List<Property> children) { 1155 super.listChildren(children); 1156 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1157 children.add(new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status)); 1158 children.add(new Property("category", "CodeableConcept", "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 0, java.lang.Integer.MAX_VALUE, category)); 1159 children.add(new Property("name", "string", "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, name)); 1160 children.add(new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject)); 1161 children.add(new Property("period", "Period", "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period)); 1162 children.add(new Property("participant", "", "Identifies all people and organizations who are expected to be involved in the care team.", 0, java.lang.Integer.MAX_VALUE, participant)); 1163 children.add(new Property("reason", "CodeableReference(Condition)", "Describes why the care team exists.", 0, java.lang.Integer.MAX_VALUE, reason)); 1164 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization)); 1165 children.add(new Property("telecom", "ContactPoint", "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, telecom)); 1166 children.add(new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note)); 1167 } 1168 1169 @Override 1170 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1171 switch (_hash) { 1172 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1173 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status); 1174 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 0, java.lang.Integer.MAX_VALUE, category); 1175 case 3373707: /*name*/ return new Property("name", "string", "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, name); 1176 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject); 1177 case -991726143: /*period*/ return new Property("period", "Period", "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period); 1178 case 767422259: /*participant*/ return new Property("participant", "", "Identifies all people and organizations who are expected to be involved in the care team.", 0, java.lang.Integer.MAX_VALUE, participant); 1179 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition)", "Describes why the care team exists.", 0, java.lang.Integer.MAX_VALUE, reason); 1180 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization); 1181 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, telecom); 1182 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note); 1183 default: return super.getNamedProperty(_hash, _name, _checkValid); 1184 } 1185 1186 } 1187 1188 @Override 1189 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1190 switch (hash) { 1191 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1192 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CareTeamStatus> 1193 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1194 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1195 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1196 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1197 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // CareTeamParticipantComponent 1198 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1199 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : this.managingOrganization.toArray(new Base[this.managingOrganization.size()]); // Reference 1200 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1201 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1202 default: return super.getProperty(hash, name, checkValid); 1203 } 1204 1205 } 1206 1207 @Override 1208 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1209 switch (hash) { 1210 case -1618432855: // identifier 1211 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1212 return value; 1213 case -892481550: // status 1214 value = new CareTeamStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1215 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1216 return value; 1217 case 50511102: // category 1218 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1219 return value; 1220 case 3373707: // name 1221 this.name = TypeConvertor.castToString(value); // StringType 1222 return value; 1223 case -1867885268: // subject 1224 this.subject = TypeConvertor.castToReference(value); // Reference 1225 return value; 1226 case -991726143: // period 1227 this.period = TypeConvertor.castToPeriod(value); // Period 1228 return value; 1229 case 767422259: // participant 1230 this.getParticipant().add((CareTeamParticipantComponent) value); // CareTeamParticipantComponent 1231 return value; 1232 case -934964668: // reason 1233 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1234 return value; 1235 case -2058947787: // managingOrganization 1236 this.getManagingOrganization().add(TypeConvertor.castToReference(value)); // Reference 1237 return value; 1238 case -1429363305: // telecom 1239 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 1240 return value; 1241 case 3387378: // note 1242 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1243 return value; 1244 default: return super.setProperty(hash, name, value); 1245 } 1246 1247 } 1248 1249 @Override 1250 public Base setProperty(String name, Base value) throws FHIRException { 1251 if (name.equals("identifier")) { 1252 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1253 } else if (name.equals("status")) { 1254 value = new CareTeamStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1255 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1256 } else if (name.equals("category")) { 1257 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1258 } else if (name.equals("name")) { 1259 this.name = TypeConvertor.castToString(value); // StringType 1260 } else if (name.equals("subject")) { 1261 this.subject = TypeConvertor.castToReference(value); // Reference 1262 } else if (name.equals("period")) { 1263 this.period = TypeConvertor.castToPeriod(value); // Period 1264 } else if (name.equals("participant")) { 1265 this.getParticipant().add((CareTeamParticipantComponent) value); 1266 } else if (name.equals("reason")) { 1267 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1268 } else if (name.equals("managingOrganization")) { 1269 this.getManagingOrganization().add(TypeConvertor.castToReference(value)); 1270 } else if (name.equals("telecom")) { 1271 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 1272 } else if (name.equals("note")) { 1273 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1274 } else 1275 return super.setProperty(name, value); 1276 return value; 1277 } 1278 1279 @Override 1280 public void removeChild(String name, Base value) throws FHIRException { 1281 if (name.equals("identifier")) { 1282 this.getIdentifier().remove(value); 1283 } else if (name.equals("status")) { 1284 value = new CareTeamStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1285 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1286 } else if (name.equals("category")) { 1287 this.getCategory().remove(value); 1288 } else if (name.equals("name")) { 1289 this.name = null; 1290 } else if (name.equals("subject")) { 1291 this.subject = null; 1292 } else if (name.equals("period")) { 1293 this.period = null; 1294 } else if (name.equals("participant")) { 1295 this.getParticipant().remove((CareTeamParticipantComponent) value); 1296 } else if (name.equals("reason")) { 1297 this.getReason().remove(value); 1298 } else if (name.equals("managingOrganization")) { 1299 this.getManagingOrganization().remove(value); 1300 } else if (name.equals("telecom")) { 1301 this.getTelecom().remove(value); 1302 } else if (name.equals("note")) { 1303 this.getNote().remove(value); 1304 } else 1305 super.removeChild(name, value); 1306 1307 } 1308 1309 @Override 1310 public Base makeProperty(int hash, String name) throws FHIRException { 1311 switch (hash) { 1312 case -1618432855: return addIdentifier(); 1313 case -892481550: return getStatusElement(); 1314 case 50511102: return addCategory(); 1315 case 3373707: return getNameElement(); 1316 case -1867885268: return getSubject(); 1317 case -991726143: return getPeriod(); 1318 case 767422259: return addParticipant(); 1319 case -934964668: return addReason(); 1320 case -2058947787: return addManagingOrganization(); 1321 case -1429363305: return addTelecom(); 1322 case 3387378: return addNote(); 1323 default: return super.makeProperty(hash, name); 1324 } 1325 1326 } 1327 1328 @Override 1329 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1330 switch (hash) { 1331 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1332 case -892481550: /*status*/ return new String[] {"code"}; 1333 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1334 case 3373707: /*name*/ return new String[] {"string"}; 1335 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1336 case -991726143: /*period*/ return new String[] {"Period"}; 1337 case 767422259: /*participant*/ return new String[] {}; 1338 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1339 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1340 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1341 case 3387378: /*note*/ return new String[] {"Annotation"}; 1342 default: return super.getTypesForProperty(hash, name); 1343 } 1344 1345 } 1346 1347 @Override 1348 public Base addChild(String name) throws FHIRException { 1349 if (name.equals("identifier")) { 1350 return addIdentifier(); 1351 } 1352 else if (name.equals("status")) { 1353 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.status"); 1354 } 1355 else if (name.equals("category")) { 1356 return addCategory(); 1357 } 1358 else if (name.equals("name")) { 1359 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.name"); 1360 } 1361 else if (name.equals("subject")) { 1362 this.subject = new Reference(); 1363 return this.subject; 1364 } 1365 else if (name.equals("period")) { 1366 this.period = new Period(); 1367 return this.period; 1368 } 1369 else if (name.equals("participant")) { 1370 return addParticipant(); 1371 } 1372 else if (name.equals("reason")) { 1373 return addReason(); 1374 } 1375 else if (name.equals("managingOrganization")) { 1376 return addManagingOrganization(); 1377 } 1378 else if (name.equals("telecom")) { 1379 return addTelecom(); 1380 } 1381 else if (name.equals("note")) { 1382 return addNote(); 1383 } 1384 else 1385 return super.addChild(name); 1386 } 1387 1388 public String fhirType() { 1389 return "CareTeam"; 1390 1391 } 1392 1393 public CareTeam copy() { 1394 CareTeam dst = new CareTeam(); 1395 copyValues(dst); 1396 return dst; 1397 } 1398 1399 public void copyValues(CareTeam dst) { 1400 super.copyValues(dst); 1401 if (identifier != null) { 1402 dst.identifier = new ArrayList<Identifier>(); 1403 for (Identifier i : identifier) 1404 dst.identifier.add(i.copy()); 1405 }; 1406 dst.status = status == null ? null : status.copy(); 1407 if (category != null) { 1408 dst.category = new ArrayList<CodeableConcept>(); 1409 for (CodeableConcept i : category) 1410 dst.category.add(i.copy()); 1411 }; 1412 dst.name = name == null ? null : name.copy(); 1413 dst.subject = subject == null ? null : subject.copy(); 1414 dst.period = period == null ? null : period.copy(); 1415 if (participant != null) { 1416 dst.participant = new ArrayList<CareTeamParticipantComponent>(); 1417 for (CareTeamParticipantComponent i : participant) 1418 dst.participant.add(i.copy()); 1419 }; 1420 if (reason != null) { 1421 dst.reason = new ArrayList<CodeableReference>(); 1422 for (CodeableReference i : reason) 1423 dst.reason.add(i.copy()); 1424 }; 1425 if (managingOrganization != null) { 1426 dst.managingOrganization = new ArrayList<Reference>(); 1427 for (Reference i : managingOrganization) 1428 dst.managingOrganization.add(i.copy()); 1429 }; 1430 if (telecom != null) { 1431 dst.telecom = new ArrayList<ContactPoint>(); 1432 for (ContactPoint i : telecom) 1433 dst.telecom.add(i.copy()); 1434 }; 1435 if (note != null) { 1436 dst.note = new ArrayList<Annotation>(); 1437 for (Annotation i : note) 1438 dst.note.add(i.copy()); 1439 }; 1440 } 1441 1442 protected CareTeam typedCopy() { 1443 return copy(); 1444 } 1445 1446 @Override 1447 public boolean equalsDeep(Base other_) { 1448 if (!super.equalsDeep(other_)) 1449 return false; 1450 if (!(other_ instanceof CareTeam)) 1451 return false; 1452 CareTeam o = (CareTeam) other_; 1453 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1454 && compareDeep(name, o.name, true) && compareDeep(subject, o.subject, true) && compareDeep(period, o.period, true) 1455 && compareDeep(participant, o.participant, true) && compareDeep(reason, o.reason, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1456 && compareDeep(telecom, o.telecom, true) && compareDeep(note, o.note, true); 1457 } 1458 1459 @Override 1460 public boolean equalsShallow(Base other_) { 1461 if (!super.equalsShallow(other_)) 1462 return false; 1463 if (!(other_ instanceof CareTeam)) 1464 return false; 1465 CareTeam o = (CareTeam) other_; 1466 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 1467 } 1468 1469 public boolean isEmpty() { 1470 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1471 , name, subject, period, participant, reason, managingOrganization, telecom, note 1472 ); 1473 } 1474 1475 @Override 1476 public ResourceType getResourceType() { 1477 return ResourceType.CareTeam; 1478 } 1479 1480 /** 1481 * Search parameter: <b>category</b> 1482 * <p> 1483 * Description: <b>Type of team</b><br> 1484 * Type: <b>token</b><br> 1485 * Path: <b>CareTeam.category</b><br> 1486 * </p> 1487 */ 1488 @SearchParamDefinition(name="category", path="CareTeam.category", description="Type of team", type="token" ) 1489 public static final String SP_CATEGORY = "category"; 1490 /** 1491 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1492 * <p> 1493 * Description: <b>Type of team</b><br> 1494 * Type: <b>token</b><br> 1495 * Path: <b>CareTeam.category</b><br> 1496 * </p> 1497 */ 1498 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1499 1500 /** 1501 * Search parameter: <b>name</b> 1502 * <p> 1503 * Description: <b>Name of the team, such as crisis assessment team</b><br> 1504 * Type: <b>string</b><br> 1505 * Path: <b>CareTeam.name | CareTeam.extension('http://hl7.org/fhir/StructureDefinition/careteam-alias').value</b><br> 1506 * </p> 1507 */ 1508 @SearchParamDefinition(name="name", path="CareTeam.name | CareTeam.extension('http://hl7.org/fhir/StructureDefinition/careteam-alias').value", description="Name of the team, such as crisis assessment team", type="string" ) 1509 public static final String SP_NAME = "name"; 1510 /** 1511 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1512 * <p> 1513 * Description: <b>Name of the team, such as crisis assessment team</b><br> 1514 * Type: <b>string</b><br> 1515 * Path: <b>CareTeam.name | CareTeam.extension('http://hl7.org/fhir/StructureDefinition/careteam-alias').value</b><br> 1516 * </p> 1517 */ 1518 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1519 1520 /** 1521 * Search parameter: <b>participant</b> 1522 * <p> 1523 * Description: <b>Who is involved</b><br> 1524 * Type: <b>reference</b><br> 1525 * Path: <b>CareTeam.participant.member</b><br> 1526 * </p> 1527 */ 1528 @SearchParamDefinition(name="participant", path="CareTeam.participant.member", description="Who is involved", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1529 public static final String SP_PARTICIPANT = "participant"; 1530 /** 1531 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 1532 * <p> 1533 * Description: <b>Who is involved</b><br> 1534 * Type: <b>reference</b><br> 1535 * Path: <b>CareTeam.participant.member</b><br> 1536 * </p> 1537 */ 1538 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 1539 1540/** 1541 * Constant for fluent queries to be used to add include statements. Specifies 1542 * the path value of "<b>CareTeam:participant</b>". 1543 */ 1544 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("CareTeam:participant").toLocked(); 1545 1546 /** 1547 * Search parameter: <b>status</b> 1548 * <p> 1549 * Description: <b>proposed | active | suspended | inactive | entered-in-error</b><br> 1550 * Type: <b>token</b><br> 1551 * Path: <b>CareTeam.status</b><br> 1552 * </p> 1553 */ 1554 @SearchParamDefinition(name="status", path="CareTeam.status", description="proposed | active | suspended | inactive | entered-in-error", type="token" ) 1555 public static final String SP_STATUS = "status"; 1556 /** 1557 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1558 * <p> 1559 * Description: <b>proposed | active | suspended | inactive | entered-in-error</b><br> 1560 * Type: <b>token</b><br> 1561 * Path: <b>CareTeam.status</b><br> 1562 * </p> 1563 */ 1564 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1565 1566 /** 1567 * Search parameter: <b>subject</b> 1568 * <p> 1569 * Description: <b>Who care team is for</b><br> 1570 * Type: <b>reference</b><br> 1571 * Path: <b>CareTeam.subject</b><br> 1572 * </p> 1573 */ 1574 @SearchParamDefinition(name="subject", path="CareTeam.subject", description="Who care team is for", type="reference", target={Group.class, Patient.class } ) 1575 public static final String SP_SUBJECT = "subject"; 1576 /** 1577 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1578 * <p> 1579 * Description: <b>Who care team is for</b><br> 1580 * Type: <b>reference</b><br> 1581 * Path: <b>CareTeam.subject</b><br> 1582 * </p> 1583 */ 1584 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1585 1586/** 1587 * Constant for fluent queries to be used to add include statements. Specifies 1588 * the path value of "<b>CareTeam:subject</b>". 1589 */ 1590 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CareTeam:subject").toLocked(); 1591 1592 /** 1593 * Search parameter: <b>date</b> 1594 * <p> 1595 * Description: <b>Multiple Resources: 1596 1597* [AdverseEvent](adverseevent.html): When the event occurred 1598* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1599* [Appointment](appointment.html): Appointment date/time. 1600* [AuditEvent](auditevent.html): Time when the event was recorded 1601* [CarePlan](careplan.html): Time period plan covers 1602* [CareTeam](careteam.html): A date within the coverage time period. 1603* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1604* [Composition](composition.html): Composition editing time 1605* [Consent](consent.html): When consent was agreed to 1606* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1607* [DocumentReference](documentreference.html): When this document reference was created 1608* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1609* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1610* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1611* [Flag](flag.html): Time period when flag is active 1612* [Immunization](immunization.html): Vaccination (non)-Administration Date 1613* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1614* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1615* [Invoice](invoice.html): Invoice date / posting date 1616* [List](list.html): When the list was prepared 1617* [MeasureReport](measurereport.html): The date of the measure report 1618* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1619* [Observation](observation.html): Clinically relevant time/time-period for observation 1620* [Procedure](procedure.html): When the procedure occurred or is occurring 1621* [ResearchSubject](researchsubject.html): Start and end of participation 1622* [RiskAssessment](riskassessment.html): When was assessment made? 1623* [SupplyRequest](supplyrequest.html): When the request was made 1624</b><br> 1625 * Type: <b>date</b><br> 1626 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1627 * </p> 1628 */ 1629 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1630 public static final String SP_DATE = "date"; 1631 /** 1632 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1633 * <p> 1634 * Description: <b>Multiple Resources: 1635 1636* [AdverseEvent](adverseevent.html): When the event occurred 1637* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1638* [Appointment](appointment.html): Appointment date/time. 1639* [AuditEvent](auditevent.html): Time when the event was recorded 1640* [CarePlan](careplan.html): Time period plan covers 1641* [CareTeam](careteam.html): A date within the coverage time period. 1642* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1643* [Composition](composition.html): Composition editing time 1644* [Consent](consent.html): When consent was agreed to 1645* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1646* [DocumentReference](documentreference.html): When this document reference was created 1647* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1648* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1649* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1650* [Flag](flag.html): Time period when flag is active 1651* [Immunization](immunization.html): Vaccination (non)-Administration Date 1652* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1653* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1654* [Invoice](invoice.html): Invoice date / posting date 1655* [List](list.html): When the list was prepared 1656* [MeasureReport](measurereport.html): The date of the measure report 1657* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1658* [Observation](observation.html): Clinically relevant time/time-period for observation 1659* [Procedure](procedure.html): When the procedure occurred or is occurring 1660* [ResearchSubject](researchsubject.html): Start and end of participation 1661* [RiskAssessment](riskassessment.html): When was assessment made? 1662* [SupplyRequest](supplyrequest.html): When the request was made 1663</b><br> 1664 * Type: <b>date</b><br> 1665 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1666 * </p> 1667 */ 1668 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1669 1670 /** 1671 * Search parameter: <b>identifier</b> 1672 * <p> 1673 * Description: <b>Multiple Resources: 1674 1675* [Account](account.html): Account number 1676* [AdverseEvent](adverseevent.html): Business identifier for the event 1677* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1678* [Appointment](appointment.html): An Identifier of the Appointment 1679* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1680* [Basic](basic.html): Business identifier 1681* [BodyStructure](bodystructure.html): Bodystructure identifier 1682* [CarePlan](careplan.html): External Ids for this plan 1683* [CareTeam](careteam.html): External Ids for this team 1684* [ChargeItem](chargeitem.html): Business Identifier for item 1685* [Claim](claim.html): The primary identifier of the financial resource 1686* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1687* [ClinicalImpression](clinicalimpression.html): Business identifier 1688* [Communication](communication.html): Unique identifier 1689* [CommunicationRequest](communicationrequest.html): Unique identifier 1690* [Composition](composition.html): Version-independent identifier for the Composition 1691* [Condition](condition.html): A unique identifier of the condition record 1692* [Consent](consent.html): Identifier for this record (external references) 1693* [Contract](contract.html): The identity of the contract 1694* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1695* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1696* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1697* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1698* [DeviceRequest](devicerequest.html): Business identifier for request/order 1699* [DeviceUsage](deviceusage.html): Search by identifier 1700* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1701* [DocumentReference](documentreference.html): Identifier of the attachment binary 1702* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1703* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1704* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1705* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1706* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1707* [Flag](flag.html): Business identifier 1708* [Goal](goal.html): External Ids for this goal 1709* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1710* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1711* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1712* [Immunization](immunization.html): Business identifier 1713* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1714* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1715* [Invoice](invoice.html): Business Identifier for item 1716* [List](list.html): Business identifier 1717* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1718* [Medication](medication.html): Returns medications with this external identifier 1719* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1720* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1721* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1722* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1723* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1724* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1725* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1726* [Observation](observation.html): The unique id for a particular observation 1727* [Person](person.html): A person Identifier 1728* [Procedure](procedure.html): A unique identifier for a procedure 1729* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1730* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1731* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1732* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1733* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1734* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1735* [Specimen](specimen.html): The unique identifier associated with the specimen 1736* [SupplyDelivery](supplydelivery.html): External identifier 1737* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1738* [Task](task.html): Search for a task instance by its business identifier 1739* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1740</b><br> 1741 * Type: <b>token</b><br> 1742 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1743 * </p> 1744 */ 1745 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1746 public static final String SP_IDENTIFIER = "identifier"; 1747 /** 1748 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1749 * <p> 1750 * Description: <b>Multiple Resources: 1751 1752* [Account](account.html): Account number 1753* [AdverseEvent](adverseevent.html): Business identifier for the event 1754* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1755* [Appointment](appointment.html): An Identifier of the Appointment 1756* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1757* [Basic](basic.html): Business identifier 1758* [BodyStructure](bodystructure.html): Bodystructure identifier 1759* [CarePlan](careplan.html): External Ids for this plan 1760* [CareTeam](careteam.html): External Ids for this team 1761* [ChargeItem](chargeitem.html): Business Identifier for item 1762* [Claim](claim.html): The primary identifier of the financial resource 1763* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1764* [ClinicalImpression](clinicalimpression.html): Business identifier 1765* [Communication](communication.html): Unique identifier 1766* [CommunicationRequest](communicationrequest.html): Unique identifier 1767* [Composition](composition.html): Version-independent identifier for the Composition 1768* [Condition](condition.html): A unique identifier of the condition record 1769* [Consent](consent.html): Identifier for this record (external references) 1770* [Contract](contract.html): The identity of the contract 1771* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1772* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1773* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1774* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1775* [DeviceRequest](devicerequest.html): Business identifier for request/order 1776* [DeviceUsage](deviceusage.html): Search by identifier 1777* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1778* [DocumentReference](documentreference.html): Identifier of the attachment binary 1779* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1780* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1781* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1782* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1783* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1784* [Flag](flag.html): Business identifier 1785* [Goal](goal.html): External Ids for this goal 1786* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1787* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1788* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1789* [Immunization](immunization.html): Business identifier 1790* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1791* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1792* [Invoice](invoice.html): Business Identifier for item 1793* [List](list.html): Business identifier 1794* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1795* [Medication](medication.html): Returns medications with this external identifier 1796* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1797* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1798* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1799* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1800* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1801* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1802* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1803* [Observation](observation.html): The unique id for a particular observation 1804* [Person](person.html): A person Identifier 1805* [Procedure](procedure.html): A unique identifier for a procedure 1806* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1807* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1808* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1809* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1810* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1811* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1812* [Specimen](specimen.html): The unique identifier associated with the specimen 1813* [SupplyDelivery](supplydelivery.html): External identifier 1814* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1815* [Task](task.html): Search for a task instance by its business identifier 1816* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1817</b><br> 1818 * Type: <b>token</b><br> 1819 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1820 * </p> 1821 */ 1822 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1823 1824 /** 1825 * Search parameter: <b>patient</b> 1826 * <p> 1827 * Description: <b>Multiple Resources: 1828 1829* [Account](account.html): The entity that caused the expenses 1830* [AdverseEvent](adverseevent.html): Subject impacted by event 1831* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1832* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1833* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1834* [AuditEvent](auditevent.html): Where the activity involved patient data 1835* [Basic](basic.html): Identifies the focus of this resource 1836* [BodyStructure](bodystructure.html): Who this is about 1837* [CarePlan](careplan.html): Who the care plan is for 1838* [CareTeam](careteam.html): Who care team is for 1839* [ChargeItem](chargeitem.html): Individual service was done for/to 1840* [Claim](claim.html): Patient receiving the products or services 1841* [ClaimResponse](claimresponse.html): The subject of care 1842* [ClinicalImpression](clinicalimpression.html): Patient assessed 1843* [Communication](communication.html): Focus of message 1844* [CommunicationRequest](communicationrequest.html): Focus of message 1845* [Composition](composition.html): Who and/or what the composition is about 1846* [Condition](condition.html): Who has the condition? 1847* [Consent](consent.html): Who the consent applies to 1848* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1849* [Coverage](coverage.html): Retrieve coverages for a patient 1850* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1851* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1852* [DetectedIssue](detectedissue.html): Associated patient 1853* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1854* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1855* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1856* [DocumentReference](documentreference.html): Who/what is the subject of the document 1857* [Encounter](encounter.html): The patient present at the encounter 1858* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1859* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1860* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1861* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1862* [Flag](flag.html): The identity of a subject to list flags for 1863* [Goal](goal.html): Who this goal is intended for 1864* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1865* [ImagingSelection](imagingselection.html): Who the study is about 1866* [ImagingStudy](imagingstudy.html): Who the study is about 1867* [Immunization](immunization.html): The patient for the vaccination record 1868* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1869* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1870* [Invoice](invoice.html): Recipient(s) of goods and services 1871* [List](list.html): If all resources have the same subject 1872* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1873* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1874* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1875* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1876* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1877* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1878* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1879* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1880* [Observation](observation.html): The subject that the observation is about (if patient) 1881* [Person](person.html): The Person links to this Patient 1882* [Procedure](procedure.html): Search by subject - a patient 1883* [Provenance](provenance.html): Where the activity involved patient data 1884* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1885* [RelatedPerson](relatedperson.html): The patient this related person is related to 1886* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1887* [ResearchSubject](researchsubject.html): Who or what is part of study 1888* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1889* [ServiceRequest](servicerequest.html): Search by subject - a patient 1890* [Specimen](specimen.html): The patient the specimen comes from 1891* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1892* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1893* [Task](task.html): Search by patient 1894* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1895</b><br> 1896 * Type: <b>reference</b><br> 1897 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1898 * </p> 1899 */ 1900 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1901 public static final String SP_PATIENT = "patient"; 1902 /** 1903 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1904 * <p> 1905 * Description: <b>Multiple Resources: 1906 1907* [Account](account.html): The entity that caused the expenses 1908* [AdverseEvent](adverseevent.html): Subject impacted by event 1909* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1910* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1911* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1912* [AuditEvent](auditevent.html): Where the activity involved patient data 1913* [Basic](basic.html): Identifies the focus of this resource 1914* [BodyStructure](bodystructure.html): Who this is about 1915* [CarePlan](careplan.html): Who the care plan is for 1916* [CareTeam](careteam.html): Who care team is for 1917* [ChargeItem](chargeitem.html): Individual service was done for/to 1918* [Claim](claim.html): Patient receiving the products or services 1919* [ClaimResponse](claimresponse.html): The subject of care 1920* [ClinicalImpression](clinicalimpression.html): Patient assessed 1921* [Communication](communication.html): Focus of message 1922* [CommunicationRequest](communicationrequest.html): Focus of message 1923* [Composition](composition.html): Who and/or what the composition is about 1924* [Condition](condition.html): Who has the condition? 1925* [Consent](consent.html): Who the consent applies to 1926* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1927* [Coverage](coverage.html): Retrieve coverages for a patient 1928* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1929* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1930* [DetectedIssue](detectedissue.html): Associated patient 1931* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1932* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1933* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1934* [DocumentReference](documentreference.html): Who/what is the subject of the document 1935* [Encounter](encounter.html): The patient present at the encounter 1936* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1937* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1938* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1939* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1940* [Flag](flag.html): The identity of a subject to list flags for 1941* [Goal](goal.html): Who this goal is intended for 1942* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1943* [ImagingSelection](imagingselection.html): Who the study is about 1944* [ImagingStudy](imagingstudy.html): Who the study is about 1945* [Immunization](immunization.html): The patient for the vaccination record 1946* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1947* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1948* [Invoice](invoice.html): Recipient(s) of goods and services 1949* [List](list.html): If all resources have the same subject 1950* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1951* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1952* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1953* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1954* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1955* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1956* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1957* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1958* [Observation](observation.html): The subject that the observation is about (if patient) 1959* [Person](person.html): The Person links to this Patient 1960* [Procedure](procedure.html): Search by subject - a patient 1961* [Provenance](provenance.html): Where the activity involved patient data 1962* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1963* [RelatedPerson](relatedperson.html): The patient this related person is related to 1964* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1965* [ResearchSubject](researchsubject.html): Who or what is part of study 1966* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1967* [ServiceRequest](servicerequest.html): Search by subject - a patient 1968* [Specimen](specimen.html): The patient the specimen comes from 1969* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1970* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1971* [Task](task.html): Search by patient 1972* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1973</b><br> 1974 * Type: <b>reference</b><br> 1975 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1976 * </p> 1977 */ 1978 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1979 1980/** 1981 * Constant for fluent queries to be used to add include statements. Specifies 1982 * the path value of "<b>CareTeam:patient</b>". 1983 */ 1984 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CareTeam:patient").toLocked(); 1985 1986 1987} 1988