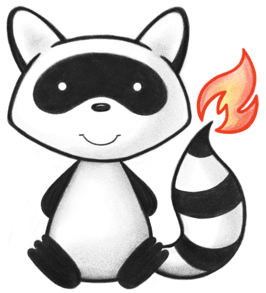
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 052 */ 053@ResourceDef(name="ChargeItem", profile="http://hl7.org/fhir/StructureDefinition/ChargeItem") 054public class ChargeItem extends DomainResource { 055 056 public enum ChargeItemStatus { 057 /** 058 * The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization. 059 */ 060 PLANNED, 061 /** 062 * The charge item is ready for billing. 063 */ 064 BILLABLE, 065 /** 066 * The charge item has been determined to be not billable (e.g. due to rules associated with the billing code). 067 */ 068 NOTBILLABLE, 069 /** 070 * The processing of the charge was aborted. 071 */ 072 ABORTED, 073 /** 074 * The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices. 075 */ 076 BILLED, 077 /** 078 * The charge item has been entered in error and should not be processed for billing. 079 */ 080 ENTEREDINERROR, 081 /** 082 * The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static ChargeItemStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("planned".equals(codeString)) 093 return PLANNED; 094 if ("billable".equals(codeString)) 095 return BILLABLE; 096 if ("not-billable".equals(codeString)) 097 return NOTBILLABLE; 098 if ("aborted".equals(codeString)) 099 return ABORTED; 100 if ("billed".equals(codeString)) 101 return BILLED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown ChargeItemStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case PLANNED: return "planned"; 114 case BILLABLE: return "billable"; 115 case NOTBILLABLE: return "not-billable"; 116 case ABORTED: return "aborted"; 117 case BILLED: return "billed"; 118 case ENTEREDINERROR: return "entered-in-error"; 119 case UNKNOWN: return "unknown"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case PLANNED: return "http://hl7.org/fhir/chargeitem-status"; 127 case BILLABLE: return "http://hl7.org/fhir/chargeitem-status"; 128 case NOTBILLABLE: return "http://hl7.org/fhir/chargeitem-status"; 129 case ABORTED: return "http://hl7.org/fhir/chargeitem-status"; 130 case BILLED: return "http://hl7.org/fhir/chargeitem-status"; 131 case ENTEREDINERROR: return "http://hl7.org/fhir/chargeitem-status"; 132 case UNKNOWN: return "http://hl7.org/fhir/chargeitem-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case PLANNED: return "The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization."; 140 case BILLABLE: return "The charge item is ready for billing."; 141 case NOTBILLABLE: return "The charge item has been determined to be not billable (e.g. due to rules associated with the billing code)."; 142 case ABORTED: return "The processing of the charge was aborted."; 143 case BILLED: return "The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices."; 144 case ENTEREDINERROR: return "The charge item has been entered in error and should not be processed for billing."; 145 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case PLANNED: return "Planned"; 153 case BILLABLE: return "Billable"; 154 case NOTBILLABLE: return "Not billable"; 155 case ABORTED: return "Aborted"; 156 case BILLED: return "Billed"; 157 case ENTEREDINERROR: return "Entered in Error"; 158 case UNKNOWN: return "Unknown"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class ChargeItemStatusEnumFactory implements EnumFactory<ChargeItemStatus> { 166 public ChargeItemStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("planned".equals(codeString)) 171 return ChargeItemStatus.PLANNED; 172 if ("billable".equals(codeString)) 173 return ChargeItemStatus.BILLABLE; 174 if ("not-billable".equals(codeString)) 175 return ChargeItemStatus.NOTBILLABLE; 176 if ("aborted".equals(codeString)) 177 return ChargeItemStatus.ABORTED; 178 if ("billed".equals(codeString)) 179 return ChargeItemStatus.BILLED; 180 if ("entered-in-error".equals(codeString)) 181 return ChargeItemStatus.ENTEREDINERROR; 182 if ("unknown".equals(codeString)) 183 return ChargeItemStatus.UNKNOWN; 184 throw new IllegalArgumentException("Unknown ChargeItemStatus code '"+codeString+"'"); 185 } 186 public Enumeration<ChargeItemStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NULL, code); 194 if ("planned".equals(codeString)) 195 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.PLANNED, code); 196 if ("billable".equals(codeString)) 197 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLABLE, code); 198 if ("not-billable".equals(codeString)) 199 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NOTBILLABLE, code); 200 if ("aborted".equals(codeString)) 201 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ABORTED, code); 202 if ("billed".equals(codeString)) 203 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLED, code); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ENTEREDINERROR, code); 206 if ("unknown".equals(codeString)) 207 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.UNKNOWN, code); 208 throw new FHIRException("Unknown ChargeItemStatus code '"+codeString+"'"); 209 } 210 public String toCode(ChargeItemStatus code) { 211 if (code == ChargeItemStatus.NULL) 212 return null; 213 if (code == ChargeItemStatus.PLANNED) 214 return "planned"; 215 if (code == ChargeItemStatus.BILLABLE) 216 return "billable"; 217 if (code == ChargeItemStatus.NOTBILLABLE) 218 return "not-billable"; 219 if (code == ChargeItemStatus.ABORTED) 220 return "aborted"; 221 if (code == ChargeItemStatus.BILLED) 222 return "billed"; 223 if (code == ChargeItemStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 if (code == ChargeItemStatus.UNKNOWN) 226 return "unknown"; 227 return "?"; 228 } 229 public String toSystem(ChargeItemStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class ChargeItemPerformerComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.). 238 */ 239 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="What type of performance was done", formalDefinition="Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.)." ) 241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 242 protected CodeableConcept function; 243 244 /** 245 * The device, practitioner, etc. who performed or participated in the service. 246 */ 247 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, HealthcareService.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 248 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed or participated in the service." ) 249 protected Reference actor; 250 251 private static final long serialVersionUID = -576943815L; 252 253 /** 254 * Constructor 255 */ 256 public ChargeItemPerformerComponent() { 257 super(); 258 } 259 260 /** 261 * Constructor 262 */ 263 public ChargeItemPerformerComponent(Reference actor) { 264 super(); 265 this.setActor(actor); 266 } 267 268 /** 269 * @return {@link #function} (Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).) 270 */ 271 public CodeableConcept getFunction() { 272 if (this.function == null) 273 if (Configuration.errorOnAutoCreate()) 274 throw new Error("Attempt to auto-create ChargeItemPerformerComponent.function"); 275 else if (Configuration.doAutoCreate()) 276 this.function = new CodeableConcept(); // cc 277 return this.function; 278 } 279 280 public boolean hasFunction() { 281 return this.function != null && !this.function.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #function} (Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).) 286 */ 287 public ChargeItemPerformerComponent setFunction(CodeableConcept value) { 288 this.function = value; 289 return this; 290 } 291 292 /** 293 * @return {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 294 */ 295 public Reference getActor() { 296 if (this.actor == null) 297 if (Configuration.errorOnAutoCreate()) 298 throw new Error("Attempt to auto-create ChargeItemPerformerComponent.actor"); 299 else if (Configuration.doAutoCreate()) 300 this.actor = new Reference(); // cc 301 return this.actor; 302 } 303 304 public boolean hasActor() { 305 return this.actor != null && !this.actor.isEmpty(); 306 } 307 308 /** 309 * @param value {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 310 */ 311 public ChargeItemPerformerComponent setActor(Reference value) { 312 this.actor = value; 313 return this; 314 } 315 316 protected void listChildren(List<Property> children) { 317 super.listChildren(children); 318 children.add(new Property("function", "CodeableConcept", "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).", 0, 1, function)); 319 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|HealthcareService|CareTeam|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 320 } 321 322 @Override 323 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 324 switch (_hash) { 325 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).", 0, 1, function); 326 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|HealthcareService|CareTeam|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 327 default: return super.getNamedProperty(_hash, _name, _checkValid); 328 } 329 330 } 331 332 @Override 333 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 334 switch (hash) { 335 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 336 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 337 default: return super.getProperty(hash, name, checkValid); 338 } 339 340 } 341 342 @Override 343 public Base setProperty(int hash, String name, Base value) throws FHIRException { 344 switch (hash) { 345 case 1380938712: // function 346 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 347 return value; 348 case 92645877: // actor 349 this.actor = TypeConvertor.castToReference(value); // Reference 350 return value; 351 default: return super.setProperty(hash, name, value); 352 } 353 354 } 355 356 @Override 357 public Base setProperty(String name, Base value) throws FHIRException { 358 if (name.equals("function")) { 359 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 360 } else if (name.equals("actor")) { 361 this.actor = TypeConvertor.castToReference(value); // Reference 362 } else 363 return super.setProperty(name, value); 364 return value; 365 } 366 367 @Override 368 public void removeChild(String name, Base value) throws FHIRException { 369 if (name.equals("function")) { 370 this.function = null; 371 } else if (name.equals("actor")) { 372 this.actor = null; 373 } else 374 super.removeChild(name, value); 375 376 } 377 378 @Override 379 public Base makeProperty(int hash, String name) throws FHIRException { 380 switch (hash) { 381 case 1380938712: return getFunction(); 382 case 92645877: return getActor(); 383 default: return super.makeProperty(hash, name); 384 } 385 386 } 387 388 @Override 389 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 390 switch (hash) { 391 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 392 case 92645877: /*actor*/ return new String[] {"Reference"}; 393 default: return super.getTypesForProperty(hash, name); 394 } 395 396 } 397 398 @Override 399 public Base addChild(String name) throws FHIRException { 400 if (name.equals("function")) { 401 this.function = new CodeableConcept(); 402 return this.function; 403 } 404 else if (name.equals("actor")) { 405 this.actor = new Reference(); 406 return this.actor; 407 } 408 else 409 return super.addChild(name); 410 } 411 412 public ChargeItemPerformerComponent copy() { 413 ChargeItemPerformerComponent dst = new ChargeItemPerformerComponent(); 414 copyValues(dst); 415 return dst; 416 } 417 418 public void copyValues(ChargeItemPerformerComponent dst) { 419 super.copyValues(dst); 420 dst.function = function == null ? null : function.copy(); 421 dst.actor = actor == null ? null : actor.copy(); 422 } 423 424 @Override 425 public boolean equalsDeep(Base other_) { 426 if (!super.equalsDeep(other_)) 427 return false; 428 if (!(other_ instanceof ChargeItemPerformerComponent)) 429 return false; 430 ChargeItemPerformerComponent o = (ChargeItemPerformerComponent) other_; 431 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 432 } 433 434 @Override 435 public boolean equalsShallow(Base other_) { 436 if (!super.equalsShallow(other_)) 437 return false; 438 if (!(other_ instanceof ChargeItemPerformerComponent)) 439 return false; 440 ChargeItemPerformerComponent o = (ChargeItemPerformerComponent) other_; 441 return true; 442 } 443 444 public boolean isEmpty() { 445 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 446 } 447 448 public String fhirType() { 449 return "ChargeItem.performer"; 450 451 } 452 453 } 454 455 /** 456 * Identifiers assigned to this event performer or other systems. 457 */ 458 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 459 @Description(shortDefinition="Business Identifier for item", formalDefinition="Identifiers assigned to this event performer or other systems." ) 460 protected List<Identifier> identifier; 461 462 /** 463 * References the (external) source of pricing information, rules of application for the code this ChargeItem uses. 464 */ 465 @Child(name = "definitionUri", type = {UriType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 466 @Description(shortDefinition="Defining information about the code of this charge item", formalDefinition="References the (external) source of pricing information, rules of application for the code this ChargeItem uses." ) 467 protected List<UriType> definitionUri; 468 469 /** 470 * References the source of pricing information, rules of application for the code this ChargeItem uses. 471 */ 472 @Child(name = "definitionCanonical", type = {CanonicalType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 473 @Description(shortDefinition="Resource defining the code of this ChargeItem", formalDefinition="References the source of pricing information, rules of application for the code this ChargeItem uses." ) 474 protected List<CanonicalType> definitionCanonical; 475 476 /** 477 * The current state of the ChargeItem. 478 */ 479 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 480 @Description(shortDefinition="planned | billable | not-billable | aborted | billed | entered-in-error | unknown", formalDefinition="The current state of the ChargeItem." ) 481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chargeitem-status") 482 protected Enumeration<ChargeItemStatus> status; 483 484 /** 485 * ChargeItems can be grouped to larger ChargeItems covering the whole set. 486 */ 487 @Child(name = "partOf", type = {ChargeItem.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 488 @Description(shortDefinition="Part of referenced ChargeItem", formalDefinition="ChargeItems can be grouped to larger ChargeItems covering the whole set." ) 489 protected List<Reference> partOf; 490 491 /** 492 * A code that identifies the charge, like a billing code. 493 */ 494 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=1, max=1, modifier=false, summary=true) 495 @Description(shortDefinition="A code that identifies the charge, like a billing code", formalDefinition="A code that identifies the charge, like a billing code." ) 496 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 497 protected CodeableConcept code; 498 499 /** 500 * The individual or set of individuals the action is being or was performed on. 501 */ 502 @Child(name = "subject", type = {Patient.class, Group.class}, order=6, min=1, max=1, modifier=false, summary=true) 503 @Description(shortDefinition="Individual service was done for/to", formalDefinition="The individual or set of individuals the action is being or was performed on." ) 504 protected Reference subject; 505 506 /** 507 * This ChargeItem has the details of how the associated Encounter should be billed or otherwise be handled by finance systems. 508 */ 509 @Child(name = "encounter", type = {Encounter.class}, order=7, min=0, max=1, modifier=false, summary=true) 510 @Description(shortDefinition="Encounter associated with this ChargeItem", formalDefinition="This ChargeItem has the details of how the associated Encounter should be billed or otherwise be handled by finance systems." ) 511 protected Reference encounter; 512 513 /** 514 * Date/time(s) or duration when the charged service was applied. 515 */ 516 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=8, min=0, max=1, modifier=false, summary=true) 517 @Description(shortDefinition="When the charged service was applied", formalDefinition="Date/time(s) or duration when the charged service was applied." ) 518 protected DataType occurrence; 519 520 /** 521 * Indicates who or what performed or participated in the charged service. 522 */ 523 @Child(name = "performer", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 524 @Description(shortDefinition="Who performed charged service", formalDefinition="Indicates who or what performed or participated in the charged service." ) 525 protected List<ChargeItemPerformerComponent> performer; 526 527 /** 528 * The organization performing the service. 529 */ 530 @Child(name = "performingOrganization", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 531 @Description(shortDefinition="Organization providing the charged service", formalDefinition="The organization performing the service." ) 532 protected Reference performingOrganization; 533 534 /** 535 * The organization requesting the service. 536 */ 537 @Child(name = "requestingOrganization", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=false) 538 @Description(shortDefinition="Organization requesting the charged service", formalDefinition="The organization requesting the service." ) 539 protected Reference requestingOrganization; 540 541 /** 542 * The financial cost center permits the tracking of charge attribution. 543 */ 544 @Child(name = "costCenter", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=false) 545 @Description(shortDefinition="Organization that has ownership of the (potential, future) revenue", formalDefinition="The financial cost center permits the tracking of charge attribution." ) 546 protected Reference costCenter; 547 548 /** 549 * Quantity of which the charge item has been serviced. 550 */ 551 @Child(name = "quantity", type = {Quantity.class}, order=13, min=0, max=1, modifier=false, summary=true) 552 @Description(shortDefinition="Quantity of which the charge item has been serviced", formalDefinition="Quantity of which the charge item has been serviced." ) 553 protected Quantity quantity; 554 555 /** 556 * The anatomical location where the related service has been applied. 557 */ 558 @Child(name = "bodysite", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 559 @Description(shortDefinition="Anatomical location, if relevant", formalDefinition="The anatomical location where the related service has been applied." ) 560 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 561 protected List<CodeableConcept> bodysite; 562 563 /** 564 * The unit price of the chargable item. 565 */ 566 @Child(name = "unitPriceComponent", type = {MonetaryComponent.class}, order=15, min=0, max=1, modifier=false, summary=false) 567 @Description(shortDefinition="Unit price overriding the associated rules", formalDefinition="The unit price of the chargable item." ) 568 protected MonetaryComponent unitPriceComponent; 569 570 /** 571 * The total price for the chargable item, accounting for the quantity. 572 */ 573 @Child(name = "totalPriceComponent", type = {MonetaryComponent.class}, order=16, min=0, max=1, modifier=false, summary=false) 574 @Description(shortDefinition="Total price overriding the associated rules", formalDefinition="The total price for the chargable item, accounting for the quantity." ) 575 protected MonetaryComponent totalPriceComponent; 576 577 /** 578 * If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action. 579 */ 580 @Child(name = "overrideReason", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=false) 581 @Description(shortDefinition="Reason for overriding the list price/factor", formalDefinition="If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action." ) 582 protected CodeableConcept overrideReason; 583 584 /** 585 * The device, practitioner, etc. who entered the charge item. 586 */ 587 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, Device.class, RelatedPerson.class}, order=18, min=0, max=1, modifier=false, summary=true) 588 @Description(shortDefinition="Individual who was entering", formalDefinition="The device, practitioner, etc. who entered the charge item." ) 589 protected Reference enterer; 590 591 /** 592 * Date the charge item was entered. 593 */ 594 @Child(name = "enteredDate", type = {DateTimeType.class}, order=19, min=0, max=1, modifier=false, summary=true) 595 @Description(shortDefinition="Date the charge item was entered", formalDefinition="Date the charge item was entered." ) 596 protected DateTimeType enteredDate; 597 598 /** 599 * Describes why the event occurred in coded or textual form. 600 */ 601 @Child(name = "reason", type = {CodeableConcept.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 602 @Description(shortDefinition="Why was the charged service rendered?", formalDefinition="Describes why the event occurred in coded or textual form." ) 603 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 604 protected List<CodeableConcept> reason; 605 606 /** 607 * Indicated the rendered service that caused this charge. 608 */ 609 @Child(name = "service", type = {CodeableReference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 610 @Description(shortDefinition="Which rendered service is being charged?", formalDefinition="Indicated the rendered service that caused this charge." ) 611 protected List<CodeableReference> service; 612 613 /** 614 * Identifies the device, food, drug or other product being charged either by type code or reference to an instance. 615 */ 616 @Child(name = "product", type = {CodeableReference.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 617 @Description(shortDefinition="Product charged", formalDefinition="Identifies the device, food, drug or other product being charged either by type code or reference to an instance." ) 618 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 619 protected List<CodeableReference> product; 620 621 /** 622 * Account into which this ChargeItems belongs. 623 */ 624 @Child(name = "account", type = {Account.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 625 @Description(shortDefinition="Account to place this charge", formalDefinition="Account into which this ChargeItems belongs." ) 626 protected List<Reference> account; 627 628 /** 629 * Comments made about the event by the performer, subject or other participants. 630 */ 631 @Child(name = "note", type = {Annotation.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 632 @Description(shortDefinition="Comments made about the ChargeItem", formalDefinition="Comments made about the event by the performer, subject or other participants." ) 633 protected List<Annotation> note; 634 635 /** 636 * Further information supporting this charge. 637 */ 638 @Child(name = "supportingInformation", type = {Reference.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 639 @Description(shortDefinition="Further information supporting this charge", formalDefinition="Further information supporting this charge." ) 640 protected List<Reference> supportingInformation; 641 642 private static final long serialVersionUID = -766813613L; 643 644 /** 645 * Constructor 646 */ 647 public ChargeItem() { 648 super(); 649 } 650 651 /** 652 * Constructor 653 */ 654 public ChargeItem(ChargeItemStatus status, CodeableConcept code, Reference subject) { 655 super(); 656 this.setStatus(status); 657 this.setCode(code); 658 this.setSubject(subject); 659 } 660 661 /** 662 * @return {@link #identifier} (Identifiers assigned to this event performer or other systems.) 663 */ 664 public List<Identifier> getIdentifier() { 665 if (this.identifier == null) 666 this.identifier = new ArrayList<Identifier>(); 667 return this.identifier; 668 } 669 670 /** 671 * @return Returns a reference to <code>this</code> for easy method chaining 672 */ 673 public ChargeItem setIdentifier(List<Identifier> theIdentifier) { 674 this.identifier = theIdentifier; 675 return this; 676 } 677 678 public boolean hasIdentifier() { 679 if (this.identifier == null) 680 return false; 681 for (Identifier item : this.identifier) 682 if (!item.isEmpty()) 683 return true; 684 return false; 685 } 686 687 public Identifier addIdentifier() { //3 688 Identifier t = new Identifier(); 689 if (this.identifier == null) 690 this.identifier = new ArrayList<Identifier>(); 691 this.identifier.add(t); 692 return t; 693 } 694 695 public ChargeItem addIdentifier(Identifier t) { //3 696 if (t == null) 697 return this; 698 if (this.identifier == null) 699 this.identifier = new ArrayList<Identifier>(); 700 this.identifier.add(t); 701 return this; 702 } 703 704 /** 705 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 706 */ 707 public Identifier getIdentifierFirstRep() { 708 if (getIdentifier().isEmpty()) { 709 addIdentifier(); 710 } 711 return getIdentifier().get(0); 712 } 713 714 /** 715 * @return {@link #definitionUri} (References the (external) source of pricing information, rules of application for the code this ChargeItem uses.) 716 */ 717 public List<UriType> getDefinitionUri() { 718 if (this.definitionUri == null) 719 this.definitionUri = new ArrayList<UriType>(); 720 return this.definitionUri; 721 } 722 723 /** 724 * @return Returns a reference to <code>this</code> for easy method chaining 725 */ 726 public ChargeItem setDefinitionUri(List<UriType> theDefinitionUri) { 727 this.definitionUri = theDefinitionUri; 728 return this; 729 } 730 731 public boolean hasDefinitionUri() { 732 if (this.definitionUri == null) 733 return false; 734 for (UriType item : this.definitionUri) 735 if (!item.isEmpty()) 736 return true; 737 return false; 738 } 739 740 /** 741 * @return {@link #definitionUri} (References the (external) source of pricing information, rules of application for the code this ChargeItem uses.) 742 */ 743 public UriType addDefinitionUriElement() {//2 744 UriType t = new UriType(); 745 if (this.definitionUri == null) 746 this.definitionUri = new ArrayList<UriType>(); 747 this.definitionUri.add(t); 748 return t; 749 } 750 751 /** 752 * @param value {@link #definitionUri} (References the (external) source of pricing information, rules of application for the code this ChargeItem uses.) 753 */ 754 public ChargeItem addDefinitionUri(String value) { //1 755 UriType t = new UriType(); 756 t.setValue(value); 757 if (this.definitionUri == null) 758 this.definitionUri = new ArrayList<UriType>(); 759 this.definitionUri.add(t); 760 return this; 761 } 762 763 /** 764 * @param value {@link #definitionUri} (References the (external) source of pricing information, rules of application for the code this ChargeItem uses.) 765 */ 766 public boolean hasDefinitionUri(String value) { 767 if (this.definitionUri == null) 768 return false; 769 for (UriType v : this.definitionUri) 770 if (v.getValue().equals(value)) // uri 771 return true; 772 return false; 773 } 774 775 /** 776 * @return {@link #definitionCanonical} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 777 */ 778 public List<CanonicalType> getDefinitionCanonical() { 779 if (this.definitionCanonical == null) 780 this.definitionCanonical = new ArrayList<CanonicalType>(); 781 return this.definitionCanonical; 782 } 783 784 /** 785 * @return Returns a reference to <code>this</code> for easy method chaining 786 */ 787 public ChargeItem setDefinitionCanonical(List<CanonicalType> theDefinitionCanonical) { 788 this.definitionCanonical = theDefinitionCanonical; 789 return this; 790 } 791 792 public boolean hasDefinitionCanonical() { 793 if (this.definitionCanonical == null) 794 return false; 795 for (CanonicalType item : this.definitionCanonical) 796 if (!item.isEmpty()) 797 return true; 798 return false; 799 } 800 801 /** 802 * @return {@link #definitionCanonical} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 803 */ 804 public CanonicalType addDefinitionCanonicalElement() {//2 805 CanonicalType t = new CanonicalType(); 806 if (this.definitionCanonical == null) 807 this.definitionCanonical = new ArrayList<CanonicalType>(); 808 this.definitionCanonical.add(t); 809 return t; 810 } 811 812 /** 813 * @param value {@link #definitionCanonical} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 814 */ 815 public ChargeItem addDefinitionCanonical(String value) { //1 816 CanonicalType t = new CanonicalType(); 817 t.setValue(value); 818 if (this.definitionCanonical == null) 819 this.definitionCanonical = new ArrayList<CanonicalType>(); 820 this.definitionCanonical.add(t); 821 return this; 822 } 823 824 /** 825 * @param value {@link #definitionCanonical} (References the source of pricing information, rules of application for the code this ChargeItem uses.) 826 */ 827 public boolean hasDefinitionCanonical(String value) { 828 if (this.definitionCanonical == null) 829 return false; 830 for (CanonicalType v : this.definitionCanonical) 831 if (v.getValue().equals(value)) // canonical 832 return true; 833 return false; 834 } 835 836 /** 837 * @return {@link #status} (The current state of the ChargeItem.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 838 */ 839 public Enumeration<ChargeItemStatus> getStatusElement() { 840 if (this.status == null) 841 if (Configuration.errorOnAutoCreate()) 842 throw new Error("Attempt to auto-create ChargeItem.status"); 843 else if (Configuration.doAutoCreate()) 844 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); // bb 845 return this.status; 846 } 847 848 public boolean hasStatusElement() { 849 return this.status != null && !this.status.isEmpty(); 850 } 851 852 public boolean hasStatus() { 853 return this.status != null && !this.status.isEmpty(); 854 } 855 856 /** 857 * @param value {@link #status} (The current state of the ChargeItem.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 858 */ 859 public ChargeItem setStatusElement(Enumeration<ChargeItemStatus> value) { 860 this.status = value; 861 return this; 862 } 863 864 /** 865 * @return The current state of the ChargeItem. 866 */ 867 public ChargeItemStatus getStatus() { 868 return this.status == null ? null : this.status.getValue(); 869 } 870 871 /** 872 * @param value The current state of the ChargeItem. 873 */ 874 public ChargeItem setStatus(ChargeItemStatus value) { 875 if (this.status == null) 876 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); 877 this.status.setValue(value); 878 return this; 879 } 880 881 /** 882 * @return {@link #partOf} (ChargeItems can be grouped to larger ChargeItems covering the whole set.) 883 */ 884 public List<Reference> getPartOf() { 885 if (this.partOf == null) 886 this.partOf = new ArrayList<Reference>(); 887 return this.partOf; 888 } 889 890 /** 891 * @return Returns a reference to <code>this</code> for easy method chaining 892 */ 893 public ChargeItem setPartOf(List<Reference> thePartOf) { 894 this.partOf = thePartOf; 895 return this; 896 } 897 898 public boolean hasPartOf() { 899 if (this.partOf == null) 900 return false; 901 for (Reference item : this.partOf) 902 if (!item.isEmpty()) 903 return true; 904 return false; 905 } 906 907 public Reference addPartOf() { //3 908 Reference t = new Reference(); 909 if (this.partOf == null) 910 this.partOf = new ArrayList<Reference>(); 911 this.partOf.add(t); 912 return t; 913 } 914 915 public ChargeItem addPartOf(Reference t) { //3 916 if (t == null) 917 return this; 918 if (this.partOf == null) 919 this.partOf = new ArrayList<Reference>(); 920 this.partOf.add(t); 921 return this; 922 } 923 924 /** 925 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 926 */ 927 public Reference getPartOfFirstRep() { 928 if (getPartOf().isEmpty()) { 929 addPartOf(); 930 } 931 return getPartOf().get(0); 932 } 933 934 /** 935 * @return {@link #code} (A code that identifies the charge, like a billing code.) 936 */ 937 public CodeableConcept getCode() { 938 if (this.code == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create ChargeItem.code"); 941 else if (Configuration.doAutoCreate()) 942 this.code = new CodeableConcept(); // cc 943 return this.code; 944 } 945 946 public boolean hasCode() { 947 return this.code != null && !this.code.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #code} (A code that identifies the charge, like a billing code.) 952 */ 953 public ChargeItem setCode(CodeableConcept value) { 954 this.code = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #subject} (The individual or set of individuals the action is being or was performed on.) 960 */ 961 public Reference getSubject() { 962 if (this.subject == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create ChargeItem.subject"); 965 else if (Configuration.doAutoCreate()) 966 this.subject = new Reference(); // cc 967 return this.subject; 968 } 969 970 public boolean hasSubject() { 971 return this.subject != null && !this.subject.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #subject} (The individual or set of individuals the action is being or was performed on.) 976 */ 977 public ChargeItem setSubject(Reference value) { 978 this.subject = value; 979 return this; 980 } 981 982 /** 983 * @return {@link #encounter} (This ChargeItem has the details of how the associated Encounter should be billed or otherwise be handled by finance systems.) 984 */ 985 public Reference getEncounter() { 986 if (this.encounter == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create ChargeItem.encounter"); 989 else if (Configuration.doAutoCreate()) 990 this.encounter = new Reference(); // cc 991 return this.encounter; 992 } 993 994 public boolean hasEncounter() { 995 return this.encounter != null && !this.encounter.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #encounter} (This ChargeItem has the details of how the associated Encounter should be billed or otherwise be handled by finance systems.) 1000 */ 1001 public ChargeItem setEncounter(Reference value) { 1002 this.encounter = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1008 */ 1009 public DataType getOccurrence() { 1010 return this.occurrence; 1011 } 1012 1013 /** 1014 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1015 */ 1016 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1017 if (this.occurrence == null) 1018 this.occurrence = new DateTimeType(); 1019 if (!(this.occurrence instanceof DateTimeType)) 1020 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1021 return (DateTimeType) this.occurrence; 1022 } 1023 1024 public boolean hasOccurrenceDateTimeType() { 1025 return this != null && this.occurrence instanceof DateTimeType; 1026 } 1027 1028 /** 1029 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1030 */ 1031 public Period getOccurrencePeriod() throws FHIRException { 1032 if (this.occurrence == null) 1033 this.occurrence = new Period(); 1034 if (!(this.occurrence instanceof Period)) 1035 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1036 return (Period) this.occurrence; 1037 } 1038 1039 public boolean hasOccurrencePeriod() { 1040 return this != null && this.occurrence instanceof Period; 1041 } 1042 1043 /** 1044 * @return {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1045 */ 1046 public Timing getOccurrenceTiming() throws FHIRException { 1047 if (this.occurrence == null) 1048 this.occurrence = new Timing(); 1049 if (!(this.occurrence instanceof Timing)) 1050 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1051 return (Timing) this.occurrence; 1052 } 1053 1054 public boolean hasOccurrenceTiming() { 1055 return this != null && this.occurrence instanceof Timing; 1056 } 1057 1058 public boolean hasOccurrence() { 1059 return this.occurrence != null && !this.occurrence.isEmpty(); 1060 } 1061 1062 /** 1063 * @param value {@link #occurrence} (Date/time(s) or duration when the charged service was applied.) 1064 */ 1065 public ChargeItem setOccurrence(DataType value) { 1066 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1067 throw new FHIRException("Not the right type for ChargeItem.occurrence[x]: "+value.fhirType()); 1068 this.occurrence = value; 1069 return this; 1070 } 1071 1072 /** 1073 * @return {@link #performer} (Indicates who or what performed or participated in the charged service.) 1074 */ 1075 public List<ChargeItemPerformerComponent> getPerformer() { 1076 if (this.performer == null) 1077 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1078 return this.performer; 1079 } 1080 1081 /** 1082 * @return Returns a reference to <code>this</code> for easy method chaining 1083 */ 1084 public ChargeItem setPerformer(List<ChargeItemPerformerComponent> thePerformer) { 1085 this.performer = thePerformer; 1086 return this; 1087 } 1088 1089 public boolean hasPerformer() { 1090 if (this.performer == null) 1091 return false; 1092 for (ChargeItemPerformerComponent item : this.performer) 1093 if (!item.isEmpty()) 1094 return true; 1095 return false; 1096 } 1097 1098 public ChargeItemPerformerComponent addPerformer() { //3 1099 ChargeItemPerformerComponent t = new ChargeItemPerformerComponent(); 1100 if (this.performer == null) 1101 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1102 this.performer.add(t); 1103 return t; 1104 } 1105 1106 public ChargeItem addPerformer(ChargeItemPerformerComponent t) { //3 1107 if (t == null) 1108 return this; 1109 if (this.performer == null) 1110 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1111 this.performer.add(t); 1112 return this; 1113 } 1114 1115 /** 1116 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1117 */ 1118 public ChargeItemPerformerComponent getPerformerFirstRep() { 1119 if (getPerformer().isEmpty()) { 1120 addPerformer(); 1121 } 1122 return getPerformer().get(0); 1123 } 1124 1125 /** 1126 * @return {@link #performingOrganization} (The organization performing the service.) 1127 */ 1128 public Reference getPerformingOrganization() { 1129 if (this.performingOrganization == null) 1130 if (Configuration.errorOnAutoCreate()) 1131 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1132 else if (Configuration.doAutoCreate()) 1133 this.performingOrganization = new Reference(); // cc 1134 return this.performingOrganization; 1135 } 1136 1137 public boolean hasPerformingOrganization() { 1138 return this.performingOrganization != null && !this.performingOrganization.isEmpty(); 1139 } 1140 1141 /** 1142 * @param value {@link #performingOrganization} (The organization performing the service.) 1143 */ 1144 public ChargeItem setPerformingOrganization(Reference value) { 1145 this.performingOrganization = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return {@link #requestingOrganization} (The organization requesting the service.) 1151 */ 1152 public Reference getRequestingOrganization() { 1153 if (this.requestingOrganization == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1156 else if (Configuration.doAutoCreate()) 1157 this.requestingOrganization = new Reference(); // cc 1158 return this.requestingOrganization; 1159 } 1160 1161 public boolean hasRequestingOrganization() { 1162 return this.requestingOrganization != null && !this.requestingOrganization.isEmpty(); 1163 } 1164 1165 /** 1166 * @param value {@link #requestingOrganization} (The organization requesting the service.) 1167 */ 1168 public ChargeItem setRequestingOrganization(Reference value) { 1169 this.requestingOrganization = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return {@link #costCenter} (The financial cost center permits the tracking of charge attribution.) 1175 */ 1176 public Reference getCostCenter() { 1177 if (this.costCenter == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create ChargeItem.costCenter"); 1180 else if (Configuration.doAutoCreate()) 1181 this.costCenter = new Reference(); // cc 1182 return this.costCenter; 1183 } 1184 1185 public boolean hasCostCenter() { 1186 return this.costCenter != null && !this.costCenter.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #costCenter} (The financial cost center permits the tracking of charge attribution.) 1191 */ 1192 public ChargeItem setCostCenter(Reference value) { 1193 this.costCenter = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return {@link #quantity} (Quantity of which the charge item has been serviced.) 1199 */ 1200 public Quantity getQuantity() { 1201 if (this.quantity == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create ChargeItem.quantity"); 1204 else if (Configuration.doAutoCreate()) 1205 this.quantity = new Quantity(); // cc 1206 return this.quantity; 1207 } 1208 1209 public boolean hasQuantity() { 1210 return this.quantity != null && !this.quantity.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #quantity} (Quantity of which the charge item has been serviced.) 1215 */ 1216 public ChargeItem setQuantity(Quantity value) { 1217 this.quantity = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #bodysite} (The anatomical location where the related service has been applied.) 1223 */ 1224 public List<CodeableConcept> getBodysite() { 1225 if (this.bodysite == null) 1226 this.bodysite = new ArrayList<CodeableConcept>(); 1227 return this.bodysite; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public ChargeItem setBodysite(List<CodeableConcept> theBodysite) { 1234 this.bodysite = theBodysite; 1235 return this; 1236 } 1237 1238 public boolean hasBodysite() { 1239 if (this.bodysite == null) 1240 return false; 1241 for (CodeableConcept item : this.bodysite) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 public CodeableConcept addBodysite() { //3 1248 CodeableConcept t = new CodeableConcept(); 1249 if (this.bodysite == null) 1250 this.bodysite = new ArrayList<CodeableConcept>(); 1251 this.bodysite.add(t); 1252 return t; 1253 } 1254 1255 public ChargeItem addBodysite(CodeableConcept t) { //3 1256 if (t == null) 1257 return this; 1258 if (this.bodysite == null) 1259 this.bodysite = new ArrayList<CodeableConcept>(); 1260 this.bodysite.add(t); 1261 return this; 1262 } 1263 1264 /** 1265 * @return The first repetition of repeating field {@link #bodysite}, creating it if it does not already exist {3} 1266 */ 1267 public CodeableConcept getBodysiteFirstRep() { 1268 if (getBodysite().isEmpty()) { 1269 addBodysite(); 1270 } 1271 return getBodysite().get(0); 1272 } 1273 1274 /** 1275 * @return {@link #unitPriceComponent} (The unit price of the chargable item.) 1276 */ 1277 public MonetaryComponent getUnitPriceComponent() { 1278 if (this.unitPriceComponent == null) 1279 if (Configuration.errorOnAutoCreate()) 1280 throw new Error("Attempt to auto-create ChargeItem.unitPriceComponent"); 1281 else if (Configuration.doAutoCreate()) 1282 this.unitPriceComponent = new MonetaryComponent(); // cc 1283 return this.unitPriceComponent; 1284 } 1285 1286 public boolean hasUnitPriceComponent() { 1287 return this.unitPriceComponent != null && !this.unitPriceComponent.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #unitPriceComponent} (The unit price of the chargable item.) 1292 */ 1293 public ChargeItem setUnitPriceComponent(MonetaryComponent value) { 1294 this.unitPriceComponent = value; 1295 return this; 1296 } 1297 1298 /** 1299 * @return {@link #totalPriceComponent} (The total price for the chargable item, accounting for the quantity.) 1300 */ 1301 public MonetaryComponent getTotalPriceComponent() { 1302 if (this.totalPriceComponent == null) 1303 if (Configuration.errorOnAutoCreate()) 1304 throw new Error("Attempt to auto-create ChargeItem.totalPriceComponent"); 1305 else if (Configuration.doAutoCreate()) 1306 this.totalPriceComponent = new MonetaryComponent(); // cc 1307 return this.totalPriceComponent; 1308 } 1309 1310 public boolean hasTotalPriceComponent() { 1311 return this.totalPriceComponent != null && !this.totalPriceComponent.isEmpty(); 1312 } 1313 1314 /** 1315 * @param value {@link #totalPriceComponent} (The total price for the chargable item, accounting for the quantity.) 1316 */ 1317 public ChargeItem setTotalPriceComponent(MonetaryComponent value) { 1318 this.totalPriceComponent = value; 1319 return this; 1320 } 1321 1322 /** 1323 * @return {@link #overrideReason} (If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.) 1324 */ 1325 public CodeableConcept getOverrideReason() { 1326 if (this.overrideReason == null) 1327 if (Configuration.errorOnAutoCreate()) 1328 throw new Error("Attempt to auto-create ChargeItem.overrideReason"); 1329 else if (Configuration.doAutoCreate()) 1330 this.overrideReason = new CodeableConcept(); // cc 1331 return this.overrideReason; 1332 } 1333 1334 public boolean hasOverrideReason() { 1335 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1336 } 1337 1338 /** 1339 * @param value {@link #overrideReason} (If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.) 1340 */ 1341 public ChargeItem setOverrideReason(CodeableConcept value) { 1342 this.overrideReason = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return {@link #enterer} (The device, practitioner, etc. who entered the charge item.) 1348 */ 1349 public Reference getEnterer() { 1350 if (this.enterer == null) 1351 if (Configuration.errorOnAutoCreate()) 1352 throw new Error("Attempt to auto-create ChargeItem.enterer"); 1353 else if (Configuration.doAutoCreate()) 1354 this.enterer = new Reference(); // cc 1355 return this.enterer; 1356 } 1357 1358 public boolean hasEnterer() { 1359 return this.enterer != null && !this.enterer.isEmpty(); 1360 } 1361 1362 /** 1363 * @param value {@link #enterer} (The device, practitioner, etc. who entered the charge item.) 1364 */ 1365 public ChargeItem setEnterer(Reference value) { 1366 this.enterer = value; 1367 return this; 1368 } 1369 1370 /** 1371 * @return {@link #enteredDate} (Date the charge item was entered.). This is the underlying object with id, value and extensions. The accessor "getEnteredDate" gives direct access to the value 1372 */ 1373 public DateTimeType getEnteredDateElement() { 1374 if (this.enteredDate == null) 1375 if (Configuration.errorOnAutoCreate()) 1376 throw new Error("Attempt to auto-create ChargeItem.enteredDate"); 1377 else if (Configuration.doAutoCreate()) 1378 this.enteredDate = new DateTimeType(); // bb 1379 return this.enteredDate; 1380 } 1381 1382 public boolean hasEnteredDateElement() { 1383 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1384 } 1385 1386 public boolean hasEnteredDate() { 1387 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1388 } 1389 1390 /** 1391 * @param value {@link #enteredDate} (Date the charge item was entered.). This is the underlying object with id, value and extensions. The accessor "getEnteredDate" gives direct access to the value 1392 */ 1393 public ChargeItem setEnteredDateElement(DateTimeType value) { 1394 this.enteredDate = value; 1395 return this; 1396 } 1397 1398 /** 1399 * @return Date the charge item was entered. 1400 */ 1401 public Date getEnteredDate() { 1402 return this.enteredDate == null ? null : this.enteredDate.getValue(); 1403 } 1404 1405 /** 1406 * @param value Date the charge item was entered. 1407 */ 1408 public ChargeItem setEnteredDate(Date value) { 1409 if (value == null) 1410 this.enteredDate = null; 1411 else { 1412 if (this.enteredDate == null) 1413 this.enteredDate = new DateTimeType(); 1414 this.enteredDate.setValue(value); 1415 } 1416 return this; 1417 } 1418 1419 /** 1420 * @return {@link #reason} (Describes why the event occurred in coded or textual form.) 1421 */ 1422 public List<CodeableConcept> getReason() { 1423 if (this.reason == null) 1424 this.reason = new ArrayList<CodeableConcept>(); 1425 return this.reason; 1426 } 1427 1428 /** 1429 * @return Returns a reference to <code>this</code> for easy method chaining 1430 */ 1431 public ChargeItem setReason(List<CodeableConcept> theReason) { 1432 this.reason = theReason; 1433 return this; 1434 } 1435 1436 public boolean hasReason() { 1437 if (this.reason == null) 1438 return false; 1439 for (CodeableConcept item : this.reason) 1440 if (!item.isEmpty()) 1441 return true; 1442 return false; 1443 } 1444 1445 public CodeableConcept addReason() { //3 1446 CodeableConcept t = new CodeableConcept(); 1447 if (this.reason == null) 1448 this.reason = new ArrayList<CodeableConcept>(); 1449 this.reason.add(t); 1450 return t; 1451 } 1452 1453 public ChargeItem addReason(CodeableConcept t) { //3 1454 if (t == null) 1455 return this; 1456 if (this.reason == null) 1457 this.reason = new ArrayList<CodeableConcept>(); 1458 this.reason.add(t); 1459 return this; 1460 } 1461 1462 /** 1463 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1464 */ 1465 public CodeableConcept getReasonFirstRep() { 1466 if (getReason().isEmpty()) { 1467 addReason(); 1468 } 1469 return getReason().get(0); 1470 } 1471 1472 /** 1473 * @return {@link #service} (Indicated the rendered service that caused this charge.) 1474 */ 1475 public List<CodeableReference> getService() { 1476 if (this.service == null) 1477 this.service = new ArrayList<CodeableReference>(); 1478 return this.service; 1479 } 1480 1481 /** 1482 * @return Returns a reference to <code>this</code> for easy method chaining 1483 */ 1484 public ChargeItem setService(List<CodeableReference> theService) { 1485 this.service = theService; 1486 return this; 1487 } 1488 1489 public boolean hasService() { 1490 if (this.service == null) 1491 return false; 1492 for (CodeableReference item : this.service) 1493 if (!item.isEmpty()) 1494 return true; 1495 return false; 1496 } 1497 1498 public CodeableReference addService() { //3 1499 CodeableReference t = new CodeableReference(); 1500 if (this.service == null) 1501 this.service = new ArrayList<CodeableReference>(); 1502 this.service.add(t); 1503 return t; 1504 } 1505 1506 public ChargeItem addService(CodeableReference t) { //3 1507 if (t == null) 1508 return this; 1509 if (this.service == null) 1510 this.service = new ArrayList<CodeableReference>(); 1511 this.service.add(t); 1512 return this; 1513 } 1514 1515 /** 1516 * @return The first repetition of repeating field {@link #service}, creating it if it does not already exist {3} 1517 */ 1518 public CodeableReference getServiceFirstRep() { 1519 if (getService().isEmpty()) { 1520 addService(); 1521 } 1522 return getService().get(0); 1523 } 1524 1525 /** 1526 * @return {@link #product} (Identifies the device, food, drug or other product being charged either by type code or reference to an instance.) 1527 */ 1528 public List<CodeableReference> getProduct() { 1529 if (this.product == null) 1530 this.product = new ArrayList<CodeableReference>(); 1531 return this.product; 1532 } 1533 1534 /** 1535 * @return Returns a reference to <code>this</code> for easy method chaining 1536 */ 1537 public ChargeItem setProduct(List<CodeableReference> theProduct) { 1538 this.product = theProduct; 1539 return this; 1540 } 1541 1542 public boolean hasProduct() { 1543 if (this.product == null) 1544 return false; 1545 for (CodeableReference item : this.product) 1546 if (!item.isEmpty()) 1547 return true; 1548 return false; 1549 } 1550 1551 public CodeableReference addProduct() { //3 1552 CodeableReference t = new CodeableReference(); 1553 if (this.product == null) 1554 this.product = new ArrayList<CodeableReference>(); 1555 this.product.add(t); 1556 return t; 1557 } 1558 1559 public ChargeItem addProduct(CodeableReference t) { //3 1560 if (t == null) 1561 return this; 1562 if (this.product == null) 1563 this.product = new ArrayList<CodeableReference>(); 1564 this.product.add(t); 1565 return this; 1566 } 1567 1568 /** 1569 * @return The first repetition of repeating field {@link #product}, creating it if it does not already exist {3} 1570 */ 1571 public CodeableReference getProductFirstRep() { 1572 if (getProduct().isEmpty()) { 1573 addProduct(); 1574 } 1575 return getProduct().get(0); 1576 } 1577 1578 /** 1579 * @return {@link #account} (Account into which this ChargeItems belongs.) 1580 */ 1581 public List<Reference> getAccount() { 1582 if (this.account == null) 1583 this.account = new ArrayList<Reference>(); 1584 return this.account; 1585 } 1586 1587 /** 1588 * @return Returns a reference to <code>this</code> for easy method chaining 1589 */ 1590 public ChargeItem setAccount(List<Reference> theAccount) { 1591 this.account = theAccount; 1592 return this; 1593 } 1594 1595 public boolean hasAccount() { 1596 if (this.account == null) 1597 return false; 1598 for (Reference item : this.account) 1599 if (!item.isEmpty()) 1600 return true; 1601 return false; 1602 } 1603 1604 public Reference addAccount() { //3 1605 Reference t = new Reference(); 1606 if (this.account == null) 1607 this.account = new ArrayList<Reference>(); 1608 this.account.add(t); 1609 return t; 1610 } 1611 1612 public ChargeItem addAccount(Reference t) { //3 1613 if (t == null) 1614 return this; 1615 if (this.account == null) 1616 this.account = new ArrayList<Reference>(); 1617 this.account.add(t); 1618 return this; 1619 } 1620 1621 /** 1622 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist {3} 1623 */ 1624 public Reference getAccountFirstRep() { 1625 if (getAccount().isEmpty()) { 1626 addAccount(); 1627 } 1628 return getAccount().get(0); 1629 } 1630 1631 /** 1632 * @return {@link #note} (Comments made about the event by the performer, subject or other participants.) 1633 */ 1634 public List<Annotation> getNote() { 1635 if (this.note == null) 1636 this.note = new ArrayList<Annotation>(); 1637 return this.note; 1638 } 1639 1640 /** 1641 * @return Returns a reference to <code>this</code> for easy method chaining 1642 */ 1643 public ChargeItem setNote(List<Annotation> theNote) { 1644 this.note = theNote; 1645 return this; 1646 } 1647 1648 public boolean hasNote() { 1649 if (this.note == null) 1650 return false; 1651 for (Annotation item : this.note) 1652 if (!item.isEmpty()) 1653 return true; 1654 return false; 1655 } 1656 1657 public Annotation addNote() { //3 1658 Annotation t = new Annotation(); 1659 if (this.note == null) 1660 this.note = new ArrayList<Annotation>(); 1661 this.note.add(t); 1662 return t; 1663 } 1664 1665 public ChargeItem addNote(Annotation t) { //3 1666 if (t == null) 1667 return this; 1668 if (this.note == null) 1669 this.note = new ArrayList<Annotation>(); 1670 this.note.add(t); 1671 return this; 1672 } 1673 1674 /** 1675 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1676 */ 1677 public Annotation getNoteFirstRep() { 1678 if (getNote().isEmpty()) { 1679 addNote(); 1680 } 1681 return getNote().get(0); 1682 } 1683 1684 /** 1685 * @return {@link #supportingInformation} (Further information supporting this charge.) 1686 */ 1687 public List<Reference> getSupportingInformation() { 1688 if (this.supportingInformation == null) 1689 this.supportingInformation = new ArrayList<Reference>(); 1690 return this.supportingInformation; 1691 } 1692 1693 /** 1694 * @return Returns a reference to <code>this</code> for easy method chaining 1695 */ 1696 public ChargeItem setSupportingInformation(List<Reference> theSupportingInformation) { 1697 this.supportingInformation = theSupportingInformation; 1698 return this; 1699 } 1700 1701 public boolean hasSupportingInformation() { 1702 if (this.supportingInformation == null) 1703 return false; 1704 for (Reference item : this.supportingInformation) 1705 if (!item.isEmpty()) 1706 return true; 1707 return false; 1708 } 1709 1710 public Reference addSupportingInformation() { //3 1711 Reference t = new Reference(); 1712 if (this.supportingInformation == null) 1713 this.supportingInformation = new ArrayList<Reference>(); 1714 this.supportingInformation.add(t); 1715 return t; 1716 } 1717 1718 public ChargeItem addSupportingInformation(Reference t) { //3 1719 if (t == null) 1720 return this; 1721 if (this.supportingInformation == null) 1722 this.supportingInformation = new ArrayList<Reference>(); 1723 this.supportingInformation.add(t); 1724 return this; 1725 } 1726 1727 /** 1728 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 1729 */ 1730 public Reference getSupportingInformationFirstRep() { 1731 if (getSupportingInformation().isEmpty()) { 1732 addSupportingInformation(); 1733 } 1734 return getSupportingInformation().get(0); 1735 } 1736 1737 protected void listChildren(List<Property> children) { 1738 super.listChildren(children); 1739 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this event performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1740 children.add(new Property("definitionUri", "uri", "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.", 0, java.lang.Integer.MAX_VALUE, definitionUri)); 1741 children.add(new Property("definitionCanonical", "canonical(ChargeItemDefinition)", "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, java.lang.Integer.MAX_VALUE, definitionCanonical)); 1742 children.add(new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status)); 1743 children.add(new Property("partOf", "Reference(ChargeItem)", "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1744 children.add(new Property("code", "CodeableConcept", "A code that identifies the charge, like a billing code.", 0, 1, code)); 1745 children.add(new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals the action is being or was performed on.", 0, 1, subject)); 1746 children.add(new Property("encounter", "Reference(Encounter)", "This ChargeItem has the details of how the associated Encounter should be billed or otherwise be handled by finance systems.", 0, 1, encounter)); 1747 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence)); 1748 children.add(new Property("performer", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, performer)); 1749 children.add(new Property("performingOrganization", "Reference(Organization)", "The organization performing the service.", 0, 1, performingOrganization)); 1750 children.add(new Property("requestingOrganization", "Reference(Organization)", "The organization requesting the service.", 0, 1, requestingOrganization)); 1751 children.add(new Property("costCenter", "Reference(Organization)", "The financial cost center permits the tracking of charge attribution.", 0, 1, costCenter)); 1752 children.add(new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 0, 1, quantity)); 1753 children.add(new Property("bodysite", "CodeableConcept", "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, bodysite)); 1754 children.add(new Property("unitPriceComponent", "MonetaryComponent", "The unit price of the chargable item.", 0, 1, unitPriceComponent)); 1755 children.add(new Property("totalPriceComponent", "MonetaryComponent", "The total price for the chargable item, accounting for the quantity.", 0, 1, totalPriceComponent)); 1756 children.add(new Property("overrideReason", "CodeableConcept", "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 0, 1, overrideReason)); 1757 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer)); 1758 children.add(new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, enteredDate)); 1759 children.add(new Property("reason", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason)); 1760 children.add(new Property("service", "CodeableReference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|MedicationRequest|Observation|Procedure|ServiceRequest|SupplyDelivery)", "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service)); 1761 children.add(new Property("product", "CodeableReference(Device|Medication|Substance)", "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 0, java.lang.Integer.MAX_VALUE, product)); 1762 children.add(new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 0, java.lang.Integer.MAX_VALUE, account)); 1763 children.add(new Property("note", "Annotation", "Comments made about the event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1764 children.add(new Property("supportingInformation", "Reference(Any)", "Further information supporting this charge.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 1765 } 1766 1767 @Override 1768 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1769 switch (_hash) { 1770 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this event performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1771 case -1139428583: /*definitionUri*/ return new Property("definitionUri", "uri", "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.", 0, java.lang.Integer.MAX_VALUE, definitionUri); 1772 case 933485793: /*definitionCanonical*/ return new Property("definitionCanonical", "canonical(ChargeItemDefinition)", "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, java.lang.Integer.MAX_VALUE, definitionCanonical); 1773 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status); 1774 case -995410646: /*partOf*/ return new Property("partOf", "Reference(ChargeItem)", "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, partOf); 1775 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies the charge, like a billing code.", 0, 1, code); 1776 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals the action is being or was performed on.", 0, 1, subject); 1777 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "This ChargeItem has the details of how the associated Encounter should be billed or otherwise be handled by finance systems.", 0, 1, encounter); 1778 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1779 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1780 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1781 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1782 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 1783 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, performer); 1784 case 1273192628: /*performingOrganization*/ return new Property("performingOrganization", "Reference(Organization)", "The organization performing the service.", 0, 1, performingOrganization); 1785 case 1279054790: /*requestingOrganization*/ return new Property("requestingOrganization", "Reference(Organization)", "The organization requesting the service.", 0, 1, requestingOrganization); 1786 case -593192318: /*costCenter*/ return new Property("costCenter", "Reference(Organization)", "The financial cost center permits the tracking of charge attribution.", 0, 1, costCenter); 1787 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 0, 1, quantity); 1788 case 1703573481: /*bodysite*/ return new Property("bodysite", "CodeableConcept", "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, bodysite); 1789 case -925197224: /*unitPriceComponent*/ return new Property("unitPriceComponent", "MonetaryComponent", "The unit price of the chargable item.", 0, 1, unitPriceComponent); 1790 case 1731497496: /*totalPriceComponent*/ return new Property("totalPriceComponent", "MonetaryComponent", "The total price for the chargable item, accounting for the quantity.", 0, 1, totalPriceComponent); 1791 case -742878928: /*overrideReason*/ return new Property("overrideReason", "CodeableConcept", "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 0, 1, overrideReason); 1792 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer); 1793 case 555978181: /*enteredDate*/ return new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, enteredDate); 1794 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason); 1795 case 1984153269: /*service*/ return new Property("service", "CodeableReference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|MedicationRequest|Observation|Procedure|ServiceRequest|SupplyDelivery)", "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service); 1796 case -309474065: /*product*/ return new Property("product", "CodeableReference(Device|Medication|Substance)", "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 0, java.lang.Integer.MAX_VALUE, product); 1797 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 0, java.lang.Integer.MAX_VALUE, account); 1798 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the event by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1799 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Further information supporting this charge.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 1800 default: return super.getNamedProperty(_hash, _name, _checkValid); 1801 } 1802 1803 } 1804 1805 @Override 1806 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1807 switch (hash) { 1808 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1809 case -1139428583: /*definitionUri*/ return this.definitionUri == null ? new Base[0] : this.definitionUri.toArray(new Base[this.definitionUri.size()]); // UriType 1810 case 933485793: /*definitionCanonical*/ return this.definitionCanonical == null ? new Base[0] : this.definitionCanonical.toArray(new Base[this.definitionCanonical.size()]); // CanonicalType 1811 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ChargeItemStatus> 1812 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1813 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1814 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1815 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1816 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1817 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ChargeItemPerformerComponent 1818 case 1273192628: /*performingOrganization*/ return this.performingOrganization == null ? new Base[0] : new Base[] {this.performingOrganization}; // Reference 1819 case 1279054790: /*requestingOrganization*/ return this.requestingOrganization == null ? new Base[0] : new Base[] {this.requestingOrganization}; // Reference 1820 case -593192318: /*costCenter*/ return this.costCenter == null ? new Base[0] : new Base[] {this.costCenter}; // Reference 1821 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1822 case 1703573481: /*bodysite*/ return this.bodysite == null ? new Base[0] : this.bodysite.toArray(new Base[this.bodysite.size()]); // CodeableConcept 1823 case -925197224: /*unitPriceComponent*/ return this.unitPriceComponent == null ? new Base[0] : new Base[] {this.unitPriceComponent}; // MonetaryComponent 1824 case 1731497496: /*totalPriceComponent*/ return this.totalPriceComponent == null ? new Base[0] : new Base[] {this.totalPriceComponent}; // MonetaryComponent 1825 case -742878928: /*overrideReason*/ return this.overrideReason == null ? new Base[0] : new Base[] {this.overrideReason}; // CodeableConcept 1826 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 1827 case 555978181: /*enteredDate*/ return this.enteredDate == null ? new Base[0] : new Base[] {this.enteredDate}; // DateTimeType 1828 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 1829 case 1984153269: /*service*/ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // CodeableReference 1830 case -309474065: /*product*/ return this.product == null ? new Base[0] : this.product.toArray(new Base[this.product.size()]); // CodeableReference 1831 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 1832 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1833 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 1834 default: return super.getProperty(hash, name, checkValid); 1835 } 1836 1837 } 1838 1839 @Override 1840 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1841 switch (hash) { 1842 case -1618432855: // identifier 1843 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1844 return value; 1845 case -1139428583: // definitionUri 1846 this.getDefinitionUri().add(TypeConvertor.castToUri(value)); // UriType 1847 return value; 1848 case 933485793: // definitionCanonical 1849 this.getDefinitionCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1850 return value; 1851 case -892481550: // status 1852 value = new ChargeItemStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1853 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 1854 return value; 1855 case -995410646: // partOf 1856 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1857 return value; 1858 case 3059181: // code 1859 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1860 return value; 1861 case -1867885268: // subject 1862 this.subject = TypeConvertor.castToReference(value); // Reference 1863 return value; 1864 case 1524132147: // encounter 1865 this.encounter = TypeConvertor.castToReference(value); // Reference 1866 return value; 1867 case 1687874001: // occurrence 1868 this.occurrence = TypeConvertor.castToType(value); // DataType 1869 return value; 1870 case 481140686: // performer 1871 this.getPerformer().add((ChargeItemPerformerComponent) value); // ChargeItemPerformerComponent 1872 return value; 1873 case 1273192628: // performingOrganization 1874 this.performingOrganization = TypeConvertor.castToReference(value); // Reference 1875 return value; 1876 case 1279054790: // requestingOrganization 1877 this.requestingOrganization = TypeConvertor.castToReference(value); // Reference 1878 return value; 1879 case -593192318: // costCenter 1880 this.costCenter = TypeConvertor.castToReference(value); // Reference 1881 return value; 1882 case -1285004149: // quantity 1883 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1884 return value; 1885 case 1703573481: // bodysite 1886 this.getBodysite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1887 return value; 1888 case -925197224: // unitPriceComponent 1889 this.unitPriceComponent = TypeConvertor.castToMonetaryComponent(value); // MonetaryComponent 1890 return value; 1891 case 1731497496: // totalPriceComponent 1892 this.totalPriceComponent = TypeConvertor.castToMonetaryComponent(value); // MonetaryComponent 1893 return value; 1894 case -742878928: // overrideReason 1895 this.overrideReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1896 return value; 1897 case -1591951995: // enterer 1898 this.enterer = TypeConvertor.castToReference(value); // Reference 1899 return value; 1900 case 555978181: // enteredDate 1901 this.enteredDate = TypeConvertor.castToDateTime(value); // DateTimeType 1902 return value; 1903 case -934964668: // reason 1904 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1905 return value; 1906 case 1984153269: // service 1907 this.getService().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1908 return value; 1909 case -309474065: // product 1910 this.getProduct().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1911 return value; 1912 case -1177318867: // account 1913 this.getAccount().add(TypeConvertor.castToReference(value)); // Reference 1914 return value; 1915 case 3387378: // note 1916 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1917 return value; 1918 case -1248768647: // supportingInformation 1919 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 1920 return value; 1921 default: return super.setProperty(hash, name, value); 1922 } 1923 1924 } 1925 1926 @Override 1927 public Base setProperty(String name, Base value) throws FHIRException { 1928 if (name.equals("identifier")) { 1929 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1930 } else if (name.equals("definitionUri")) { 1931 this.getDefinitionUri().add(TypeConvertor.castToUri(value)); 1932 } else if (name.equals("definitionCanonical")) { 1933 this.getDefinitionCanonical().add(TypeConvertor.castToCanonical(value)); 1934 } else if (name.equals("status")) { 1935 value = new ChargeItemStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1936 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 1937 } else if (name.equals("partOf")) { 1938 this.getPartOf().add(TypeConvertor.castToReference(value)); 1939 } else if (name.equals("code")) { 1940 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1941 } else if (name.equals("subject")) { 1942 this.subject = TypeConvertor.castToReference(value); // Reference 1943 } else if (name.equals("encounter")) { 1944 this.encounter = TypeConvertor.castToReference(value); // Reference 1945 } else if (name.equals("occurrence[x]")) { 1946 this.occurrence = TypeConvertor.castToType(value); // DataType 1947 } else if (name.equals("performer")) { 1948 this.getPerformer().add((ChargeItemPerformerComponent) value); 1949 } else if (name.equals("performingOrganization")) { 1950 this.performingOrganization = TypeConvertor.castToReference(value); // Reference 1951 } else if (name.equals("requestingOrganization")) { 1952 this.requestingOrganization = TypeConvertor.castToReference(value); // Reference 1953 } else if (name.equals("costCenter")) { 1954 this.costCenter = TypeConvertor.castToReference(value); // Reference 1955 } else if (name.equals("quantity")) { 1956 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1957 } else if (name.equals("bodysite")) { 1958 this.getBodysite().add(TypeConvertor.castToCodeableConcept(value)); 1959 } else if (name.equals("unitPriceComponent")) { 1960 this.unitPriceComponent = TypeConvertor.castToMonetaryComponent(value); // MonetaryComponent 1961 } else if (name.equals("totalPriceComponent")) { 1962 this.totalPriceComponent = TypeConvertor.castToMonetaryComponent(value); // MonetaryComponent 1963 } else if (name.equals("overrideReason")) { 1964 this.overrideReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1965 } else if (name.equals("enterer")) { 1966 this.enterer = TypeConvertor.castToReference(value); // Reference 1967 } else if (name.equals("enteredDate")) { 1968 this.enteredDate = TypeConvertor.castToDateTime(value); // DateTimeType 1969 } else if (name.equals("reason")) { 1970 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 1971 } else if (name.equals("service")) { 1972 this.getService().add(TypeConvertor.castToCodeableReference(value)); 1973 } else if (name.equals("product")) { 1974 this.getProduct().add(TypeConvertor.castToCodeableReference(value)); 1975 } else if (name.equals("account")) { 1976 this.getAccount().add(TypeConvertor.castToReference(value)); 1977 } else if (name.equals("note")) { 1978 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1979 } else if (name.equals("supportingInformation")) { 1980 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 1981 } else 1982 return super.setProperty(name, value); 1983 return value; 1984 } 1985 1986 @Override 1987 public void removeChild(String name, Base value) throws FHIRException { 1988 if (name.equals("identifier")) { 1989 this.getIdentifier().remove(value); 1990 } else if (name.equals("definitionUri")) { 1991 this.getDefinitionUri().remove(value); 1992 } else if (name.equals("definitionCanonical")) { 1993 this.getDefinitionCanonical().remove(value); 1994 } else if (name.equals("status")) { 1995 value = new ChargeItemStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1996 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 1997 } else if (name.equals("partOf")) { 1998 this.getPartOf().remove(value); 1999 } else if (name.equals("code")) { 2000 this.code = null; 2001 } else if (name.equals("subject")) { 2002 this.subject = null; 2003 } else if (name.equals("encounter")) { 2004 this.encounter = null; 2005 } else if (name.equals("occurrence[x]")) { 2006 this.occurrence = null; 2007 } else if (name.equals("performer")) { 2008 this.getPerformer().remove((ChargeItemPerformerComponent) value); 2009 } else if (name.equals("performingOrganization")) { 2010 this.performingOrganization = null; 2011 } else if (name.equals("requestingOrganization")) { 2012 this.requestingOrganization = null; 2013 } else if (name.equals("costCenter")) { 2014 this.costCenter = null; 2015 } else if (name.equals("quantity")) { 2016 this.quantity = null; 2017 } else if (name.equals("bodysite")) { 2018 this.getBodysite().remove(value); 2019 } else if (name.equals("unitPriceComponent")) { 2020 this.unitPriceComponent = null; 2021 } else if (name.equals("totalPriceComponent")) { 2022 this.totalPriceComponent = null; 2023 } else if (name.equals("overrideReason")) { 2024 this.overrideReason = null; 2025 } else if (name.equals("enterer")) { 2026 this.enterer = null; 2027 } else if (name.equals("enteredDate")) { 2028 this.enteredDate = null; 2029 } else if (name.equals("reason")) { 2030 this.getReason().remove(value); 2031 } else if (name.equals("service")) { 2032 this.getService().remove(value); 2033 } else if (name.equals("product")) { 2034 this.getProduct().remove(value); 2035 } else if (name.equals("account")) { 2036 this.getAccount().remove(value); 2037 } else if (name.equals("note")) { 2038 this.getNote().remove(value); 2039 } else if (name.equals("supportingInformation")) { 2040 this.getSupportingInformation().remove(value); 2041 } else 2042 super.removeChild(name, value); 2043 2044 } 2045 2046 @Override 2047 public Base makeProperty(int hash, String name) throws FHIRException { 2048 switch (hash) { 2049 case -1618432855: return addIdentifier(); 2050 case -1139428583: return addDefinitionUriElement(); 2051 case 933485793: return addDefinitionCanonicalElement(); 2052 case -892481550: return getStatusElement(); 2053 case -995410646: return addPartOf(); 2054 case 3059181: return getCode(); 2055 case -1867885268: return getSubject(); 2056 case 1524132147: return getEncounter(); 2057 case -2022646513: return getOccurrence(); 2058 case 1687874001: return getOccurrence(); 2059 case 481140686: return addPerformer(); 2060 case 1273192628: return getPerformingOrganization(); 2061 case 1279054790: return getRequestingOrganization(); 2062 case -593192318: return getCostCenter(); 2063 case -1285004149: return getQuantity(); 2064 case 1703573481: return addBodysite(); 2065 case -925197224: return getUnitPriceComponent(); 2066 case 1731497496: return getTotalPriceComponent(); 2067 case -742878928: return getOverrideReason(); 2068 case -1591951995: return getEnterer(); 2069 case 555978181: return getEnteredDateElement(); 2070 case -934964668: return addReason(); 2071 case 1984153269: return addService(); 2072 case -309474065: return addProduct(); 2073 case -1177318867: return addAccount(); 2074 case 3387378: return addNote(); 2075 case -1248768647: return addSupportingInformation(); 2076 default: return super.makeProperty(hash, name); 2077 } 2078 2079 } 2080 2081 @Override 2082 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2083 switch (hash) { 2084 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2085 case -1139428583: /*definitionUri*/ return new String[] {"uri"}; 2086 case 933485793: /*definitionCanonical*/ return new String[] {"canonical"}; 2087 case -892481550: /*status*/ return new String[] {"code"}; 2088 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2089 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2090 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2091 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2092 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2093 case 481140686: /*performer*/ return new String[] {}; 2094 case 1273192628: /*performingOrganization*/ return new String[] {"Reference"}; 2095 case 1279054790: /*requestingOrganization*/ return new String[] {"Reference"}; 2096 case -593192318: /*costCenter*/ return new String[] {"Reference"}; 2097 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 2098 case 1703573481: /*bodysite*/ return new String[] {"CodeableConcept"}; 2099 case -925197224: /*unitPriceComponent*/ return new String[] {"MonetaryComponent"}; 2100 case 1731497496: /*totalPriceComponent*/ return new String[] {"MonetaryComponent"}; 2101 case -742878928: /*overrideReason*/ return new String[] {"CodeableConcept"}; 2102 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 2103 case 555978181: /*enteredDate*/ return new String[] {"dateTime"}; 2104 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 2105 case 1984153269: /*service*/ return new String[] {"CodeableReference"}; 2106 case -309474065: /*product*/ return new String[] {"CodeableReference"}; 2107 case -1177318867: /*account*/ return new String[] {"Reference"}; 2108 case 3387378: /*note*/ return new String[] {"Annotation"}; 2109 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2110 default: return super.getTypesForProperty(hash, name); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base addChild(String name) throws FHIRException { 2117 if (name.equals("identifier")) { 2118 return addIdentifier(); 2119 } 2120 else if (name.equals("definitionUri")) { 2121 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definitionUri"); 2122 } 2123 else if (name.equals("definitionCanonical")) { 2124 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definitionCanonical"); 2125 } 2126 else if (name.equals("status")) { 2127 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.status"); 2128 } 2129 else if (name.equals("partOf")) { 2130 return addPartOf(); 2131 } 2132 else if (name.equals("code")) { 2133 this.code = new CodeableConcept(); 2134 return this.code; 2135 } 2136 else if (name.equals("subject")) { 2137 this.subject = new Reference(); 2138 return this.subject; 2139 } 2140 else if (name.equals("encounter")) { 2141 this.encounter = new Reference(); 2142 return this.encounter; 2143 } 2144 else if (name.equals("occurrenceDateTime")) { 2145 this.occurrence = new DateTimeType(); 2146 return this.occurrence; 2147 } 2148 else if (name.equals("occurrencePeriod")) { 2149 this.occurrence = new Period(); 2150 return this.occurrence; 2151 } 2152 else if (name.equals("occurrenceTiming")) { 2153 this.occurrence = new Timing(); 2154 return this.occurrence; 2155 } 2156 else if (name.equals("performer")) { 2157 return addPerformer(); 2158 } 2159 else if (name.equals("performingOrganization")) { 2160 this.performingOrganization = new Reference(); 2161 return this.performingOrganization; 2162 } 2163 else if (name.equals("requestingOrganization")) { 2164 this.requestingOrganization = new Reference(); 2165 return this.requestingOrganization; 2166 } 2167 else if (name.equals("costCenter")) { 2168 this.costCenter = new Reference(); 2169 return this.costCenter; 2170 } 2171 else if (name.equals("quantity")) { 2172 this.quantity = new Quantity(); 2173 return this.quantity; 2174 } 2175 else if (name.equals("bodysite")) { 2176 return addBodysite(); 2177 } 2178 else if (name.equals("unitPriceComponent")) { 2179 this.unitPriceComponent = new MonetaryComponent(); 2180 return this.unitPriceComponent; 2181 } 2182 else if (name.equals("totalPriceComponent")) { 2183 this.totalPriceComponent = new MonetaryComponent(); 2184 return this.totalPriceComponent; 2185 } 2186 else if (name.equals("overrideReason")) { 2187 this.overrideReason = new CodeableConcept(); 2188 return this.overrideReason; 2189 } 2190 else if (name.equals("enterer")) { 2191 this.enterer = new Reference(); 2192 return this.enterer; 2193 } 2194 else if (name.equals("enteredDate")) { 2195 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.enteredDate"); 2196 } 2197 else if (name.equals("reason")) { 2198 return addReason(); 2199 } 2200 else if (name.equals("service")) { 2201 return addService(); 2202 } 2203 else if (name.equals("product")) { 2204 return addProduct(); 2205 } 2206 else if (name.equals("account")) { 2207 return addAccount(); 2208 } 2209 else if (name.equals("note")) { 2210 return addNote(); 2211 } 2212 else if (name.equals("supportingInformation")) { 2213 return addSupportingInformation(); 2214 } 2215 else 2216 return super.addChild(name); 2217 } 2218 2219 public String fhirType() { 2220 return "ChargeItem"; 2221 2222 } 2223 2224 public ChargeItem copy() { 2225 ChargeItem dst = new ChargeItem(); 2226 copyValues(dst); 2227 return dst; 2228 } 2229 2230 public void copyValues(ChargeItem dst) { 2231 super.copyValues(dst); 2232 if (identifier != null) { 2233 dst.identifier = new ArrayList<Identifier>(); 2234 for (Identifier i : identifier) 2235 dst.identifier.add(i.copy()); 2236 }; 2237 if (definitionUri != null) { 2238 dst.definitionUri = new ArrayList<UriType>(); 2239 for (UriType i : definitionUri) 2240 dst.definitionUri.add(i.copy()); 2241 }; 2242 if (definitionCanonical != null) { 2243 dst.definitionCanonical = new ArrayList<CanonicalType>(); 2244 for (CanonicalType i : definitionCanonical) 2245 dst.definitionCanonical.add(i.copy()); 2246 }; 2247 dst.status = status == null ? null : status.copy(); 2248 if (partOf != null) { 2249 dst.partOf = new ArrayList<Reference>(); 2250 for (Reference i : partOf) 2251 dst.partOf.add(i.copy()); 2252 }; 2253 dst.code = code == null ? null : code.copy(); 2254 dst.subject = subject == null ? null : subject.copy(); 2255 dst.encounter = encounter == null ? null : encounter.copy(); 2256 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2257 if (performer != null) { 2258 dst.performer = new ArrayList<ChargeItemPerformerComponent>(); 2259 for (ChargeItemPerformerComponent i : performer) 2260 dst.performer.add(i.copy()); 2261 }; 2262 dst.performingOrganization = performingOrganization == null ? null : performingOrganization.copy(); 2263 dst.requestingOrganization = requestingOrganization == null ? null : requestingOrganization.copy(); 2264 dst.costCenter = costCenter == null ? null : costCenter.copy(); 2265 dst.quantity = quantity == null ? null : quantity.copy(); 2266 if (bodysite != null) { 2267 dst.bodysite = new ArrayList<CodeableConcept>(); 2268 for (CodeableConcept i : bodysite) 2269 dst.bodysite.add(i.copy()); 2270 }; 2271 dst.unitPriceComponent = unitPriceComponent == null ? null : unitPriceComponent.copy(); 2272 dst.totalPriceComponent = totalPriceComponent == null ? null : totalPriceComponent.copy(); 2273 dst.overrideReason = overrideReason == null ? null : overrideReason.copy(); 2274 dst.enterer = enterer == null ? null : enterer.copy(); 2275 dst.enteredDate = enteredDate == null ? null : enteredDate.copy(); 2276 if (reason != null) { 2277 dst.reason = new ArrayList<CodeableConcept>(); 2278 for (CodeableConcept i : reason) 2279 dst.reason.add(i.copy()); 2280 }; 2281 if (service != null) { 2282 dst.service = new ArrayList<CodeableReference>(); 2283 for (CodeableReference i : service) 2284 dst.service.add(i.copy()); 2285 }; 2286 if (product != null) { 2287 dst.product = new ArrayList<CodeableReference>(); 2288 for (CodeableReference i : product) 2289 dst.product.add(i.copy()); 2290 }; 2291 if (account != null) { 2292 dst.account = new ArrayList<Reference>(); 2293 for (Reference i : account) 2294 dst.account.add(i.copy()); 2295 }; 2296 if (note != null) { 2297 dst.note = new ArrayList<Annotation>(); 2298 for (Annotation i : note) 2299 dst.note.add(i.copy()); 2300 }; 2301 if (supportingInformation != null) { 2302 dst.supportingInformation = new ArrayList<Reference>(); 2303 for (Reference i : supportingInformation) 2304 dst.supportingInformation.add(i.copy()); 2305 }; 2306 } 2307 2308 protected ChargeItem typedCopy() { 2309 return copy(); 2310 } 2311 2312 @Override 2313 public boolean equalsDeep(Base other_) { 2314 if (!super.equalsDeep(other_)) 2315 return false; 2316 if (!(other_ instanceof ChargeItem)) 2317 return false; 2318 ChargeItem o = (ChargeItem) other_; 2319 return compareDeep(identifier, o.identifier, true) && compareDeep(definitionUri, o.definitionUri, true) 2320 && compareDeep(definitionCanonical, o.definitionCanonical, true) && compareDeep(status, o.status, true) 2321 && compareDeep(partOf, o.partOf, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2322 && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) && compareDeep(performer, o.performer, true) 2323 && compareDeep(performingOrganization, o.performingOrganization, true) && compareDeep(requestingOrganization, o.requestingOrganization, true) 2324 && compareDeep(costCenter, o.costCenter, true) && compareDeep(quantity, o.quantity, true) && compareDeep(bodysite, o.bodysite, true) 2325 && compareDeep(unitPriceComponent, o.unitPriceComponent, true) && compareDeep(totalPriceComponent, o.totalPriceComponent, true) 2326 && compareDeep(overrideReason, o.overrideReason, true) && compareDeep(enterer, o.enterer, true) 2327 && compareDeep(enteredDate, o.enteredDate, true) && compareDeep(reason, o.reason, true) && compareDeep(service, o.service, true) 2328 && compareDeep(product, o.product, true) && compareDeep(account, o.account, true) && compareDeep(note, o.note, true) 2329 && compareDeep(supportingInformation, o.supportingInformation, true); 2330 } 2331 2332 @Override 2333 public boolean equalsShallow(Base other_) { 2334 if (!super.equalsShallow(other_)) 2335 return false; 2336 if (!(other_ instanceof ChargeItem)) 2337 return false; 2338 ChargeItem o = (ChargeItem) other_; 2339 return compareValues(definitionUri, o.definitionUri, true) && compareValues(definitionCanonical, o.definitionCanonical, true) 2340 && compareValues(status, o.status, true) && compareValues(enteredDate, o.enteredDate, true); 2341 } 2342 2343 public boolean isEmpty() { 2344 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definitionUri 2345 , definitionCanonical, status, partOf, code, subject, encounter, occurrence, performer 2346 , performingOrganization, requestingOrganization, costCenter, quantity, bodysite, unitPriceComponent 2347 , totalPriceComponent, overrideReason, enterer, enteredDate, reason, service, product 2348 , account, note, supportingInformation); 2349 } 2350 2351 @Override 2352 public ResourceType getResourceType() { 2353 return ResourceType.ChargeItem; 2354 } 2355 2356 /** 2357 * Search parameter: <b>account</b> 2358 * <p> 2359 * Description: <b>Account to place this charge</b><br> 2360 * Type: <b>reference</b><br> 2361 * Path: <b>ChargeItem.account</b><br> 2362 * </p> 2363 */ 2364 @SearchParamDefinition(name="account", path="ChargeItem.account", description="Account to place this charge", type="reference", target={Account.class } ) 2365 public static final String SP_ACCOUNT = "account"; 2366 /** 2367 * <b>Fluent Client</b> search parameter constant for <b>account</b> 2368 * <p> 2369 * Description: <b>Account to place this charge</b><br> 2370 * Type: <b>reference</b><br> 2371 * Path: <b>ChargeItem.account</b><br> 2372 * </p> 2373 */ 2374 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACCOUNT); 2375 2376/** 2377 * Constant for fluent queries to be used to add include statements. Specifies 2378 * the path value of "<b>ChargeItem:account</b>". 2379 */ 2380 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include("ChargeItem:account").toLocked(); 2381 2382 /** 2383 * Search parameter: <b>entered-date</b> 2384 * <p> 2385 * Description: <b>Date the charge item was entered</b><br> 2386 * Type: <b>date</b><br> 2387 * Path: <b>ChargeItem.enteredDate</b><br> 2388 * </p> 2389 */ 2390 @SearchParamDefinition(name="entered-date", path="ChargeItem.enteredDate", description="Date the charge item was entered", type="date" ) 2391 public static final String SP_ENTERED_DATE = "entered-date"; 2392 /** 2393 * <b>Fluent Client</b> search parameter constant for <b>entered-date</b> 2394 * <p> 2395 * Description: <b>Date the charge item was entered</b><br> 2396 * Type: <b>date</b><br> 2397 * Path: <b>ChargeItem.enteredDate</b><br> 2398 * </p> 2399 */ 2400 public static final ca.uhn.fhir.rest.gclient.DateClientParam ENTERED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ENTERED_DATE); 2401 2402 /** 2403 * Search parameter: <b>enterer</b> 2404 * <p> 2405 * Description: <b>Individual who was entering</b><br> 2406 * Type: <b>reference</b><br> 2407 * Path: <b>ChargeItem.enterer</b><br> 2408 * </p> 2409 */ 2410 @SearchParamDefinition(name="enterer", path="ChargeItem.enterer", description="Individual who was entering", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2411 public static final String SP_ENTERER = "enterer"; 2412 /** 2413 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 2414 * <p> 2415 * Description: <b>Individual who was entering</b><br> 2416 * Type: <b>reference</b><br> 2417 * Path: <b>ChargeItem.enterer</b><br> 2418 * </p> 2419 */ 2420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 2421 2422/** 2423 * Constant for fluent queries to be used to add include statements. Specifies 2424 * the path value of "<b>ChargeItem:enterer</b>". 2425 */ 2426 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("ChargeItem:enterer").toLocked(); 2427 2428 /** 2429 * Search parameter: <b>factor-override</b> 2430 * <p> 2431 * Description: <b>Factor overriding the associated rules</b><br> 2432 * Type: <b>number</b><br> 2433 * Path: <b>ChargeItem.totalPriceComponent.factor</b><br> 2434 * </p> 2435 */ 2436 @SearchParamDefinition(name="factor-override", path="ChargeItem.totalPriceComponent.factor", description="Factor overriding the associated rules", type="number" ) 2437 public static final String SP_FACTOR_OVERRIDE = "factor-override"; 2438 /** 2439 * <b>Fluent Client</b> search parameter constant for <b>factor-override</b> 2440 * <p> 2441 * Description: <b>Factor overriding the associated rules</b><br> 2442 * Type: <b>number</b><br> 2443 * Path: <b>ChargeItem.totalPriceComponent.factor</b><br> 2444 * </p> 2445 */ 2446 public static final ca.uhn.fhir.rest.gclient.NumberClientParam FACTOR_OVERRIDE = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_FACTOR_OVERRIDE); 2447 2448 /** 2449 * Search parameter: <b>occurrence</b> 2450 * <p> 2451 * Description: <b>When the charged service was applied</b><br> 2452 * Type: <b>date</b><br> 2453 * Path: <b>ChargeItem.occurrence.ofType(dateTime) | ChargeItem.occurrence.ofType(Period) | ChargeItem.occurrence.ofType(Timing)</b><br> 2454 * </p> 2455 */ 2456 @SearchParamDefinition(name="occurrence", path="ChargeItem.occurrence.ofType(dateTime) | ChargeItem.occurrence.ofType(Period) | ChargeItem.occurrence.ofType(Timing)", description="When the charged service was applied", type="date" ) 2457 public static final String SP_OCCURRENCE = "occurrence"; 2458 /** 2459 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 2460 * <p> 2461 * Description: <b>When the charged service was applied</b><br> 2462 * Type: <b>date</b><br> 2463 * Path: <b>ChargeItem.occurrence.ofType(dateTime) | ChargeItem.occurrence.ofType(Period) | ChargeItem.occurrence.ofType(Timing)</b><br> 2464 * </p> 2465 */ 2466 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 2467 2468 /** 2469 * Search parameter: <b>performer-actor</b> 2470 * <p> 2471 * Description: <b>Individual who was performing</b><br> 2472 * Type: <b>reference</b><br> 2473 * Path: <b>ChargeItem.performer.actor</b><br> 2474 * </p> 2475 */ 2476 @SearchParamDefinition(name="performer-actor", path="ChargeItem.performer.actor", description="Individual who was performing", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2477 public static final String SP_PERFORMER_ACTOR = "performer-actor"; 2478 /** 2479 * <b>Fluent Client</b> search parameter constant for <b>performer-actor</b> 2480 * <p> 2481 * Description: <b>Individual who was performing</b><br> 2482 * Type: <b>reference</b><br> 2483 * Path: <b>ChargeItem.performer.actor</b><br> 2484 * </p> 2485 */ 2486 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER_ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER_ACTOR); 2487 2488/** 2489 * Constant for fluent queries to be used to add include statements. Specifies 2490 * the path value of "<b>ChargeItem:performer-actor</b>". 2491 */ 2492 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER_ACTOR = new ca.uhn.fhir.model.api.Include("ChargeItem:performer-actor").toLocked(); 2493 2494 /** 2495 * Search parameter: <b>performer-function</b> 2496 * <p> 2497 * Description: <b>What type of performance was done</b><br> 2498 * Type: <b>token</b><br> 2499 * Path: <b>ChargeItem.performer.function</b><br> 2500 * </p> 2501 */ 2502 @SearchParamDefinition(name="performer-function", path="ChargeItem.performer.function", description="What type of performance was done", type="token" ) 2503 public static final String SP_PERFORMER_FUNCTION = "performer-function"; 2504 /** 2505 * <b>Fluent Client</b> search parameter constant for <b>performer-function</b> 2506 * <p> 2507 * Description: <b>What type of performance was done</b><br> 2508 * Type: <b>token</b><br> 2509 * Path: <b>ChargeItem.performer.function</b><br> 2510 * </p> 2511 */ 2512 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_FUNCTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER_FUNCTION); 2513 2514 /** 2515 * Search parameter: <b>performing-organization</b> 2516 * <p> 2517 * Description: <b>Organization providing the charged service</b><br> 2518 * Type: <b>reference</b><br> 2519 * Path: <b>ChargeItem.performingOrganization</b><br> 2520 * </p> 2521 */ 2522 @SearchParamDefinition(name="performing-organization", path="ChargeItem.performingOrganization", description="Organization providing the charged service", type="reference", target={Organization.class } ) 2523 public static final String SP_PERFORMING_ORGANIZATION = "performing-organization"; 2524 /** 2525 * <b>Fluent Client</b> search parameter constant for <b>performing-organization</b> 2526 * <p> 2527 * Description: <b>Organization providing the charged service</b><br> 2528 * Type: <b>reference</b><br> 2529 * Path: <b>ChargeItem.performingOrganization</b><br> 2530 * </p> 2531 */ 2532 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMING_ORGANIZATION); 2533 2534/** 2535 * Constant for fluent queries to be used to add include statements. Specifies 2536 * the path value of "<b>ChargeItem:performing-organization</b>". 2537 */ 2538 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMING_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ChargeItem:performing-organization").toLocked(); 2539 2540 /** 2541 * Search parameter: <b>price-override</b> 2542 * <p> 2543 * Description: <b>Price overriding the associated rules</b><br> 2544 * Type: <b>quantity</b><br> 2545 * Path: <b>ChargeItem.totalPriceComponent.amount</b><br> 2546 * </p> 2547 */ 2548 @SearchParamDefinition(name="price-override", path="ChargeItem.totalPriceComponent.amount", description="Price overriding the associated rules", type="quantity" ) 2549 public static final String SP_PRICE_OVERRIDE = "price-override"; 2550 /** 2551 * <b>Fluent Client</b> search parameter constant for <b>price-override</b> 2552 * <p> 2553 * Description: <b>Price overriding the associated rules</b><br> 2554 * Type: <b>quantity</b><br> 2555 * Path: <b>ChargeItem.totalPriceComponent.amount</b><br> 2556 * </p> 2557 */ 2558 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam PRICE_OVERRIDE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_PRICE_OVERRIDE); 2559 2560 /** 2561 * Search parameter: <b>quantity</b> 2562 * <p> 2563 * Description: <b>Quantity of which the charge item has been serviced</b><br> 2564 * Type: <b>quantity</b><br> 2565 * Path: <b>ChargeItem.quantity</b><br> 2566 * </p> 2567 */ 2568 @SearchParamDefinition(name="quantity", path="ChargeItem.quantity", description="Quantity of which the charge item has been serviced", type="quantity" ) 2569 public static final String SP_QUANTITY = "quantity"; 2570 /** 2571 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 2572 * <p> 2573 * Description: <b>Quantity of which the charge item has been serviced</b><br> 2574 * Type: <b>quantity</b><br> 2575 * Path: <b>ChargeItem.quantity</b><br> 2576 * </p> 2577 */ 2578 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_QUANTITY); 2579 2580 /** 2581 * Search parameter: <b>requesting-organization</b> 2582 * <p> 2583 * Description: <b>Organization requesting the charged service</b><br> 2584 * Type: <b>reference</b><br> 2585 * Path: <b>ChargeItem.requestingOrganization</b><br> 2586 * </p> 2587 */ 2588 @SearchParamDefinition(name="requesting-organization", path="ChargeItem.requestingOrganization", description="Organization requesting the charged service", type="reference", target={Organization.class } ) 2589 public static final String SP_REQUESTING_ORGANIZATION = "requesting-organization"; 2590 /** 2591 * <b>Fluent Client</b> search parameter constant for <b>requesting-organization</b> 2592 * <p> 2593 * Description: <b>Organization requesting the charged service</b><br> 2594 * Type: <b>reference</b><br> 2595 * Path: <b>ChargeItem.requestingOrganization</b><br> 2596 * </p> 2597 */ 2598 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTING_ORGANIZATION); 2599 2600/** 2601 * Constant for fluent queries to be used to add include statements. Specifies 2602 * the path value of "<b>ChargeItem:requesting-organization</b>". 2603 */ 2604 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTING_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ChargeItem:requesting-organization").toLocked(); 2605 2606 /** 2607 * Search parameter: <b>service</b> 2608 * <p> 2609 * Description: <b>Which rendered service is being charged?</b><br> 2610 * Type: <b>reference</b><br> 2611 * Path: <b>ChargeItem.service.reference</b><br> 2612 * </p> 2613 */ 2614 @SearchParamDefinition(name="service", path="ChargeItem.service.reference", description="Which rendered service is being charged?", type="reference", target={DiagnosticReport.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, MedicationRequest.class, Observation.class, Procedure.class, ServiceRequest.class, SupplyDelivery.class } ) 2615 public static final String SP_SERVICE = "service"; 2616 /** 2617 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2618 * <p> 2619 * Description: <b>Which rendered service is being charged?</b><br> 2620 * Type: <b>reference</b><br> 2621 * Path: <b>ChargeItem.service.reference</b><br> 2622 * </p> 2623 */ 2624 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE); 2625 2626/** 2627 * Constant for fluent queries to be used to add include statements. Specifies 2628 * the path value of "<b>ChargeItem:service</b>". 2629 */ 2630 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include("ChargeItem:service").toLocked(); 2631 2632 /** 2633 * Search parameter: <b>status</b> 2634 * <p> 2635 * Description: <b>Is this charge item active</b><br> 2636 * Type: <b>token</b><br> 2637 * Path: <b>ChargeItem.status</b><br> 2638 * </p> 2639 */ 2640 @SearchParamDefinition(name="status", path="ChargeItem.status", description="Is this charge item active", type="token" ) 2641 public static final String SP_STATUS = "status"; 2642 /** 2643 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2644 * <p> 2645 * Description: <b>Is this charge item active</b><br> 2646 * Type: <b>token</b><br> 2647 * Path: <b>ChargeItem.status</b><br> 2648 * </p> 2649 */ 2650 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2651 2652 /** 2653 * Search parameter: <b>subject</b> 2654 * <p> 2655 * Description: <b>Individual service was done for/to</b><br> 2656 * Type: <b>reference</b><br> 2657 * Path: <b>ChargeItem.subject</b><br> 2658 * </p> 2659 */ 2660 @SearchParamDefinition(name="subject", path="ChargeItem.subject", description="Individual service was done for/to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2661 public static final String SP_SUBJECT = "subject"; 2662 /** 2663 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2664 * <p> 2665 * Description: <b>Individual service was done for/to</b><br> 2666 * Type: <b>reference</b><br> 2667 * Path: <b>ChargeItem.subject</b><br> 2668 * </p> 2669 */ 2670 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2671 2672/** 2673 * Constant for fluent queries to be used to add include statements. Specifies 2674 * the path value of "<b>ChargeItem:subject</b>". 2675 */ 2676 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ChargeItem:subject").toLocked(); 2677 2678 /** 2679 * Search parameter: <b>code</b> 2680 * <p> 2681 * Description: <b>Multiple Resources: 2682 2683* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2684* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2685* [AuditEvent](auditevent.html): More specific code for the event 2686* [Basic](basic.html): Kind of Resource 2687* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2688* [Condition](condition.html): Code for the condition 2689* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2690* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2691* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2692* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2693* [ImagingSelection](imagingselection.html): The imaging selection status 2694* [List](list.html): What the purpose of this list is 2695* [Medication](medication.html): Returns medications for a specific code 2696* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2697* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2698* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2699* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2700* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2701* [Observation](observation.html): The code of the observation type 2702* [Procedure](procedure.html): A code to identify a procedure 2703* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2704* [Task](task.html): Search by task code 2705</b><br> 2706 * Type: <b>token</b><br> 2707 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2708 * </p> 2709 */ 2710 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2711 public static final String SP_CODE = "code"; 2712 /** 2713 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2714 * <p> 2715 * Description: <b>Multiple Resources: 2716 2717* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2718* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2719* [AuditEvent](auditevent.html): More specific code for the event 2720* [Basic](basic.html): Kind of Resource 2721* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2722* [Condition](condition.html): Code for the condition 2723* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2724* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2725* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2726* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2727* [ImagingSelection](imagingselection.html): The imaging selection status 2728* [List](list.html): What the purpose of this list is 2729* [Medication](medication.html): Returns medications for a specific code 2730* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2731* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2732* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2733* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2734* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2735* [Observation](observation.html): The code of the observation type 2736* [Procedure](procedure.html): A code to identify a procedure 2737* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2738* [Task](task.html): Search by task code 2739</b><br> 2740 * Type: <b>token</b><br> 2741 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2742 * </p> 2743 */ 2744 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2745 2746 /** 2747 * Search parameter: <b>encounter</b> 2748 * <p> 2749 * Description: <b>Multiple Resources: 2750 2751* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2752* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2753* [ChargeItem](chargeitem.html): Encounter associated with event 2754* [Claim](claim.html): Encounters associated with a billed line item 2755* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2756* [Communication](communication.html): The Encounter during which this Communication was created 2757* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2758* [Composition](composition.html): Context of the Composition 2759* [Condition](condition.html): The Encounter during which this Condition was created 2760* [DeviceRequest](devicerequest.html): Encounter during which request was created 2761* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2762* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2763* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2764* [Flag](flag.html): Alert relevant during encounter 2765* [ImagingStudy](imagingstudy.html): The context of the study 2766* [List](list.html): Context in which list created 2767* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2768* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2769* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2770* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2771* [Observation](observation.html): Encounter related to the observation 2772* [Procedure](procedure.html): The Encounter during which this Procedure was created 2773* [Provenance](provenance.html): Encounter related to the Provenance 2774* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2775* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2776* [RiskAssessment](riskassessment.html): Where was assessment performed? 2777* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2778* [Task](task.html): Search by encounter 2779* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2780</b><br> 2781 * Type: <b>reference</b><br> 2782 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2783 * </p> 2784 */ 2785 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2786 public static final String SP_ENCOUNTER = "encounter"; 2787 /** 2788 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2789 * <p> 2790 * Description: <b>Multiple Resources: 2791 2792* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2793* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2794* [ChargeItem](chargeitem.html): Encounter associated with event 2795* [Claim](claim.html): Encounters associated with a billed line item 2796* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2797* [Communication](communication.html): The Encounter during which this Communication was created 2798* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2799* [Composition](composition.html): Context of the Composition 2800* [Condition](condition.html): The Encounter during which this Condition was created 2801* [DeviceRequest](devicerequest.html): Encounter during which request was created 2802* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2803* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2804* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2805* [Flag](flag.html): Alert relevant during encounter 2806* [ImagingStudy](imagingstudy.html): The context of the study 2807* [List](list.html): Context in which list created 2808* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2809* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2810* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2811* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2812* [Observation](observation.html): Encounter related to the observation 2813* [Procedure](procedure.html): The Encounter during which this Procedure was created 2814* [Provenance](provenance.html): Encounter related to the Provenance 2815* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2816* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2817* [RiskAssessment](riskassessment.html): Where was assessment performed? 2818* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2819* [Task](task.html): Search by encounter 2820* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2821</b><br> 2822 * Type: <b>reference</b><br> 2823 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2824 * </p> 2825 */ 2826 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2827 2828/** 2829 * Constant for fluent queries to be used to add include statements. Specifies 2830 * the path value of "<b>ChargeItem:encounter</b>". 2831 */ 2832 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ChargeItem:encounter").toLocked(); 2833 2834 /** 2835 * Search parameter: <b>identifier</b> 2836 * <p> 2837 * Description: <b>Multiple Resources: 2838 2839* [Account](account.html): Account number 2840* [AdverseEvent](adverseevent.html): Business identifier for the event 2841* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2842* [Appointment](appointment.html): An Identifier of the Appointment 2843* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2844* [Basic](basic.html): Business identifier 2845* [BodyStructure](bodystructure.html): Bodystructure identifier 2846* [CarePlan](careplan.html): External Ids for this plan 2847* [CareTeam](careteam.html): External Ids for this team 2848* [ChargeItem](chargeitem.html): Business Identifier for item 2849* [Claim](claim.html): The primary identifier of the financial resource 2850* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2851* [ClinicalImpression](clinicalimpression.html): Business identifier 2852* [Communication](communication.html): Unique identifier 2853* [CommunicationRequest](communicationrequest.html): Unique identifier 2854* [Composition](composition.html): Version-independent identifier for the Composition 2855* [Condition](condition.html): A unique identifier of the condition record 2856* [Consent](consent.html): Identifier for this record (external references) 2857* [Contract](contract.html): The identity of the contract 2858* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2859* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2860* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2861* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2862* [DeviceRequest](devicerequest.html): Business identifier for request/order 2863* [DeviceUsage](deviceusage.html): Search by identifier 2864* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2865* [DocumentReference](documentreference.html): Identifier of the attachment binary 2866* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2867* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2868* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2869* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2870* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2871* [Flag](flag.html): Business identifier 2872* [Goal](goal.html): External Ids for this goal 2873* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2874* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2875* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2876* [Immunization](immunization.html): Business identifier 2877* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2878* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2879* [Invoice](invoice.html): Business Identifier for item 2880* [List](list.html): Business identifier 2881* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2882* [Medication](medication.html): Returns medications with this external identifier 2883* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2884* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2885* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2886* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2887* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2888* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2889* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2890* [Observation](observation.html): The unique id for a particular observation 2891* [Person](person.html): A person Identifier 2892* [Procedure](procedure.html): A unique identifier for a procedure 2893* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2894* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2895* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2896* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2897* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2898* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2899* [Specimen](specimen.html): The unique identifier associated with the specimen 2900* [SupplyDelivery](supplydelivery.html): External identifier 2901* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2902* [Task](task.html): Search for a task instance by its business identifier 2903* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2904</b><br> 2905 * Type: <b>token</b><br> 2906 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2907 * </p> 2908 */ 2909 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2910 public static final String SP_IDENTIFIER = "identifier"; 2911 /** 2912 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2913 * <p> 2914 * Description: <b>Multiple Resources: 2915 2916* [Account](account.html): Account number 2917* [AdverseEvent](adverseevent.html): Business identifier for the event 2918* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2919* [Appointment](appointment.html): An Identifier of the Appointment 2920* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2921* [Basic](basic.html): Business identifier 2922* [BodyStructure](bodystructure.html): Bodystructure identifier 2923* [CarePlan](careplan.html): External Ids for this plan 2924* [CareTeam](careteam.html): External Ids for this team 2925* [ChargeItem](chargeitem.html): Business Identifier for item 2926* [Claim](claim.html): The primary identifier of the financial resource 2927* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2928* [ClinicalImpression](clinicalimpression.html): Business identifier 2929* [Communication](communication.html): Unique identifier 2930* [CommunicationRequest](communicationrequest.html): Unique identifier 2931* [Composition](composition.html): Version-independent identifier for the Composition 2932* [Condition](condition.html): A unique identifier of the condition record 2933* [Consent](consent.html): Identifier for this record (external references) 2934* [Contract](contract.html): The identity of the contract 2935* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2936* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2937* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2938* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2939* [DeviceRequest](devicerequest.html): Business identifier for request/order 2940* [DeviceUsage](deviceusage.html): Search by identifier 2941* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2942* [DocumentReference](documentreference.html): Identifier of the attachment binary 2943* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2944* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2945* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2946* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2947* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2948* [Flag](flag.html): Business identifier 2949* [Goal](goal.html): External Ids for this goal 2950* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2951* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2952* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2953* [Immunization](immunization.html): Business identifier 2954* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2955* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2956* [Invoice](invoice.html): Business Identifier for item 2957* [List](list.html): Business identifier 2958* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2959* [Medication](medication.html): Returns medications with this external identifier 2960* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2961* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2962* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2963* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2964* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2965* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2966* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2967* [Observation](observation.html): The unique id for a particular observation 2968* [Person](person.html): A person Identifier 2969* [Procedure](procedure.html): A unique identifier for a procedure 2970* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2971* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2972* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2973* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2974* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2975* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2976* [Specimen](specimen.html): The unique identifier associated with the specimen 2977* [SupplyDelivery](supplydelivery.html): External identifier 2978* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2979* [Task](task.html): Search for a task instance by its business identifier 2980* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2981</b><br> 2982 * Type: <b>token</b><br> 2983 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2984 * </p> 2985 */ 2986 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2987 2988 /** 2989 * Search parameter: <b>patient</b> 2990 * <p> 2991 * Description: <b>Multiple Resources: 2992 2993* [Account](account.html): The entity that caused the expenses 2994* [AdverseEvent](adverseevent.html): Subject impacted by event 2995* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2996* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2997* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2998* [AuditEvent](auditevent.html): Where the activity involved patient data 2999* [Basic](basic.html): Identifies the focus of this resource 3000* [BodyStructure](bodystructure.html): Who this is about 3001* [CarePlan](careplan.html): Who the care plan is for 3002* [CareTeam](careteam.html): Who care team is for 3003* [ChargeItem](chargeitem.html): Individual service was done for/to 3004* [Claim](claim.html): Patient receiving the products or services 3005* [ClaimResponse](claimresponse.html): The subject of care 3006* [ClinicalImpression](clinicalimpression.html): Patient assessed 3007* [Communication](communication.html): Focus of message 3008* [CommunicationRequest](communicationrequest.html): Focus of message 3009* [Composition](composition.html): Who and/or what the composition is about 3010* [Condition](condition.html): Who has the condition? 3011* [Consent](consent.html): Who the consent applies to 3012* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3013* [Coverage](coverage.html): Retrieve coverages for a patient 3014* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3015* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3016* [DetectedIssue](detectedissue.html): Associated patient 3017* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3018* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3019* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3020* [DocumentReference](documentreference.html): Who/what is the subject of the document 3021* [Encounter](encounter.html): The patient present at the encounter 3022* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3023* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3024* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3025* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3026* [Flag](flag.html): The identity of a subject to list flags for 3027* [Goal](goal.html): Who this goal is intended for 3028* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3029* [ImagingSelection](imagingselection.html): Who the study is about 3030* [ImagingStudy](imagingstudy.html): Who the study is about 3031* [Immunization](immunization.html): The patient for the vaccination record 3032* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3033* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3034* [Invoice](invoice.html): Recipient(s) of goods and services 3035* [List](list.html): If all resources have the same subject 3036* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3037* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3038* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3039* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3040* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3041* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3042* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3043* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3044* [Observation](observation.html): The subject that the observation is about (if patient) 3045* [Person](person.html): The Person links to this Patient 3046* [Procedure](procedure.html): Search by subject - a patient 3047* [Provenance](provenance.html): Where the activity involved patient data 3048* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3049* [RelatedPerson](relatedperson.html): The patient this related person is related to 3050* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3051* [ResearchSubject](researchsubject.html): Who or what is part of study 3052* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3053* [ServiceRequest](servicerequest.html): Search by subject - a patient 3054* [Specimen](specimen.html): The patient the specimen comes from 3055* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3056* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3057* [Task](task.html): Search by patient 3058* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3059</b><br> 3060 * Type: <b>reference</b><br> 3061 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3062 * </p> 3063 */ 3064 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3065 public static final String SP_PATIENT = "patient"; 3066 /** 3067 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3068 * <p> 3069 * Description: <b>Multiple Resources: 3070 3071* [Account](account.html): The entity that caused the expenses 3072* [AdverseEvent](adverseevent.html): Subject impacted by event 3073* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3074* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3075* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3076* [AuditEvent](auditevent.html): Where the activity involved patient data 3077* [Basic](basic.html): Identifies the focus of this resource 3078* [BodyStructure](bodystructure.html): Who this is about 3079* [CarePlan](careplan.html): Who the care plan is for 3080* [CareTeam](careteam.html): Who care team is for 3081* [ChargeItem](chargeitem.html): Individual service was done for/to 3082* [Claim](claim.html): Patient receiving the products or services 3083* [ClaimResponse](claimresponse.html): The subject of care 3084* [ClinicalImpression](clinicalimpression.html): Patient assessed 3085* [Communication](communication.html): Focus of message 3086* [CommunicationRequest](communicationrequest.html): Focus of message 3087* [Composition](composition.html): Who and/or what the composition is about 3088* [Condition](condition.html): Who has the condition? 3089* [Consent](consent.html): Who the consent applies to 3090* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3091* [Coverage](coverage.html): Retrieve coverages for a patient 3092* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3093* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3094* [DetectedIssue](detectedissue.html): Associated patient 3095* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3096* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3097* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3098* [DocumentReference](documentreference.html): Who/what is the subject of the document 3099* [Encounter](encounter.html): The patient present at the encounter 3100* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3101* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3102* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3103* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3104* [Flag](flag.html): The identity of a subject to list flags for 3105* [Goal](goal.html): Who this goal is intended for 3106* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3107* [ImagingSelection](imagingselection.html): Who the study is about 3108* [ImagingStudy](imagingstudy.html): Who the study is about 3109* [Immunization](immunization.html): The patient for the vaccination record 3110* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3111* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3112* [Invoice](invoice.html): Recipient(s) of goods and services 3113* [List](list.html): If all resources have the same subject 3114* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3115* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3116* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3117* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3118* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3119* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3120* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3121* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3122* [Observation](observation.html): The subject that the observation is about (if patient) 3123* [Person](person.html): The Person links to this Patient 3124* [Procedure](procedure.html): Search by subject - a patient 3125* [Provenance](provenance.html): Where the activity involved patient data 3126* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3127* [RelatedPerson](relatedperson.html): The patient this related person is related to 3128* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3129* [ResearchSubject](researchsubject.html): Who or what is part of study 3130* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3131* [ServiceRequest](servicerequest.html): Search by subject - a patient 3132* [Specimen](specimen.html): The patient the specimen comes from 3133* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3134* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3135* [Task](task.html): Search by patient 3136* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3137</b><br> 3138 * Type: <b>reference</b><br> 3139 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3140 * </p> 3141 */ 3142 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3143 3144/** 3145 * Constant for fluent queries to be used to add include statements. Specifies 3146 * the path value of "<b>ChargeItem:patient</b>". 3147 */ 3148 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ChargeItem:patient").toLocked(); 3149 3150 3151} 3152