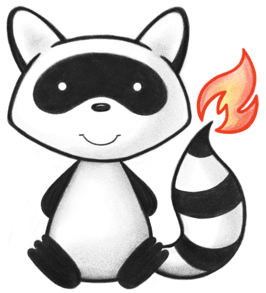
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system. 052 */ 053@ResourceDef(name="ChargeItemDefinition", profile="http://hl7.org/fhir/StructureDefinition/ChargeItemDefinition") 054public class ChargeItemDefinition extends MetadataResource { 055 056 @Block() 057 public static class ChargeItemDefinitionApplicabilityComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied. 060 */ 061 @Child(name = "condition", type = {Expression.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied." ) 063 protected Expression condition; 064 065 /** 066 * The period during which the charge item definition content was or is planned to be in active use. 067 */ 068 @Child(name = "effectivePeriod", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="When the charge item definition is expected to be used", formalDefinition="The period during which the charge item definition content was or is planned to be in active use." ) 070 protected Period effectivePeriod; 071 072 /** 073 * Reference to / quotation of the external source of the group of properties. 074 */ 075 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=3, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="Reference to / quotation of the external source of the group of properties", formalDefinition="Reference to / quotation of the external source of the group of properties." ) 077 protected RelatedArtifact relatedArtifact; 078 079 private static final long serialVersionUID = -1706427366L; 080 081 /** 082 * Constructor 083 */ 084 public ChargeItemDefinitionApplicabilityComponent() { 085 super(); 086 } 087 088 /** 089 * @return {@link #condition} (An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.) 090 */ 091 public Expression getCondition() { 092 if (this.condition == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.condition"); 095 else if (Configuration.doAutoCreate()) 096 this.condition = new Expression(); // cc 097 return this.condition; 098 } 099 100 public boolean hasCondition() { 101 return this.condition != null && !this.condition.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #condition} (An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.) 106 */ 107 public ChargeItemDefinitionApplicabilityComponent setCondition(Expression value) { 108 this.condition = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 114 */ 115 public Period getEffectivePeriod() { 116 if (this.effectivePeriod == null) 117 if (Configuration.errorOnAutoCreate()) 118 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.effectivePeriod"); 119 else if (Configuration.doAutoCreate()) 120 this.effectivePeriod = new Period(); // cc 121 return this.effectivePeriod; 122 } 123 124 public boolean hasEffectivePeriod() { 125 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 130 */ 131 public ChargeItemDefinitionApplicabilityComponent setEffectivePeriod(Period value) { 132 this.effectivePeriod = value; 133 return this; 134 } 135 136 /** 137 * @return {@link #relatedArtifact} (Reference to / quotation of the external source of the group of properties.) 138 */ 139 public RelatedArtifact getRelatedArtifact() { 140 if (this.relatedArtifact == null) 141 if (Configuration.errorOnAutoCreate()) 142 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.relatedArtifact"); 143 else if (Configuration.doAutoCreate()) 144 this.relatedArtifact = new RelatedArtifact(); // cc 145 return this.relatedArtifact; 146 } 147 148 public boolean hasRelatedArtifact() { 149 return this.relatedArtifact != null && !this.relatedArtifact.isEmpty(); 150 } 151 152 /** 153 * @param value {@link #relatedArtifact} (Reference to / quotation of the external source of the group of properties.) 154 */ 155 public ChargeItemDefinitionApplicabilityComponent setRelatedArtifact(RelatedArtifact value) { 156 this.relatedArtifact = value; 157 return this; 158 } 159 160 protected void listChildren(List<Property> children) { 161 super.listChildren(children); 162 children.add(new Property("condition", "Expression", "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 0, 1, condition)); 163 children.add(new Property("effectivePeriod", "Period", "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 164 children.add(new Property("relatedArtifact", "RelatedArtifact", "Reference to / quotation of the external source of the group of properties.", 0, 1, relatedArtifact)); 165 } 166 167 @Override 168 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 169 switch (_hash) { 170 case -861311717: /*condition*/ return new Property("condition", "Expression", "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 0, 1, condition); 171 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 172 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Reference to / quotation of the external source of the group of properties.", 0, 1, relatedArtifact); 173 default: return super.getNamedProperty(_hash, _name, _checkValid); 174 } 175 176 } 177 178 @Override 179 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 180 switch (hash) { 181 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Expression 182 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 183 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : new Base[] {this.relatedArtifact}; // RelatedArtifact 184 default: return super.getProperty(hash, name, checkValid); 185 } 186 187 } 188 189 @Override 190 public Base setProperty(int hash, String name, Base value) throws FHIRException { 191 switch (hash) { 192 case -861311717: // condition 193 this.condition = TypeConvertor.castToExpression(value); // Expression 194 return value; 195 case -403934648: // effectivePeriod 196 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 197 return value; 198 case 666807069: // relatedArtifact 199 this.relatedArtifact = TypeConvertor.castToRelatedArtifact(value); // RelatedArtifact 200 return value; 201 default: return super.setProperty(hash, name, value); 202 } 203 204 } 205 206 @Override 207 public Base setProperty(String name, Base value) throws FHIRException { 208 if (name.equals("condition")) { 209 this.condition = TypeConvertor.castToExpression(value); // Expression 210 } else if (name.equals("effectivePeriod")) { 211 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 212 } else if (name.equals("relatedArtifact")) { 213 this.relatedArtifact = TypeConvertor.castToRelatedArtifact(value); // RelatedArtifact 214 } else 215 return super.setProperty(name, value); 216 return value; 217 } 218 219 @Override 220 public Base makeProperty(int hash, String name) throws FHIRException { 221 switch (hash) { 222 case -861311717: return getCondition(); 223 case -403934648: return getEffectivePeriod(); 224 case 666807069: return getRelatedArtifact(); 225 default: return super.makeProperty(hash, name); 226 } 227 228 } 229 230 @Override 231 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 232 switch (hash) { 233 case -861311717: /*condition*/ return new String[] {"Expression"}; 234 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 235 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 236 default: return super.getTypesForProperty(hash, name); 237 } 238 239 } 240 241 @Override 242 public Base addChild(String name) throws FHIRException { 243 if (name.equals("condition")) { 244 this.condition = new Expression(); 245 return this.condition; 246 } 247 else if (name.equals("effectivePeriod")) { 248 this.effectivePeriod = new Period(); 249 return this.effectivePeriod; 250 } 251 else if (name.equals("relatedArtifact")) { 252 this.relatedArtifact = new RelatedArtifact(); 253 return this.relatedArtifact; 254 } 255 else 256 return super.addChild(name); 257 } 258 259 public ChargeItemDefinitionApplicabilityComponent copy() { 260 ChargeItemDefinitionApplicabilityComponent dst = new ChargeItemDefinitionApplicabilityComponent(); 261 copyValues(dst); 262 return dst; 263 } 264 265 public void copyValues(ChargeItemDefinitionApplicabilityComponent dst) { 266 super.copyValues(dst); 267 dst.condition = condition == null ? null : condition.copy(); 268 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 269 dst.relatedArtifact = relatedArtifact == null ? null : relatedArtifact.copy(); 270 } 271 272 @Override 273 public boolean equalsDeep(Base other_) { 274 if (!super.equalsDeep(other_)) 275 return false; 276 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 277 return false; 278 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 279 return compareDeep(condition, o.condition, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 280 && compareDeep(relatedArtifact, o.relatedArtifact, true); 281 } 282 283 @Override 284 public boolean equalsShallow(Base other_) { 285 if (!super.equalsShallow(other_)) 286 return false; 287 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 288 return false; 289 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 290 return true; 291 } 292 293 public boolean isEmpty() { 294 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, effectivePeriod 295 , relatedArtifact); 296 } 297 298 public String fhirType() { 299 return "ChargeItemDefinition.applicability"; 300 301 } 302 303 } 304 305 @Block() 306 public static class ChargeItemDefinitionPropertyGroupComponent extends BackboneElement implements IBaseBackboneElement { 307 /** 308 * Expressions that describe applicability criteria for the priceComponent. 309 */ 310 @Child(name = "applicability", type = {ChargeItemDefinitionApplicabilityComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 311 @Description(shortDefinition="Conditions under which the priceComponent is applicable", formalDefinition="Expressions that describe applicability criteria for the priceComponent." ) 312 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 313 314 /** 315 * The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated. 316 */ 317 @Child(name = "priceComponent", type = {MonetaryComponent.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 318 @Description(shortDefinition="Components of total line item price", formalDefinition="The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated." ) 319 protected List<MonetaryComponent> priceComponent; 320 321 private static final long serialVersionUID = -1829474901L; 322 323 /** 324 * Constructor 325 */ 326 public ChargeItemDefinitionPropertyGroupComponent() { 327 super(); 328 } 329 330 /** 331 * @return {@link #applicability} (Expressions that describe applicability criteria for the priceComponent.) 332 */ 333 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 334 if (this.applicability == null) 335 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 336 return this.applicability; 337 } 338 339 /** 340 * @return Returns a reference to <code>this</code> for easy method chaining 341 */ 342 public ChargeItemDefinitionPropertyGroupComponent setApplicability(List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 343 this.applicability = theApplicability; 344 return this; 345 } 346 347 public boolean hasApplicability() { 348 if (this.applicability == null) 349 return false; 350 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 351 if (!item.isEmpty()) 352 return true; 353 return false; 354 } 355 356 public ChargeItemDefinitionApplicabilityComponent addApplicability() { //3 357 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 358 if (this.applicability == null) 359 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 360 this.applicability.add(t); 361 return t; 362 } 363 364 public ChargeItemDefinitionPropertyGroupComponent addApplicability(ChargeItemDefinitionApplicabilityComponent t) { //3 365 if (t == null) 366 return this; 367 if (this.applicability == null) 368 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 369 this.applicability.add(t); 370 return this; 371 } 372 373 /** 374 * @return The first repetition of repeating field {@link #applicability}, creating it if it does not already exist {3} 375 */ 376 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 377 if (getApplicability().isEmpty()) { 378 addApplicability(); 379 } 380 return getApplicability().get(0); 381 } 382 383 /** 384 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.) 385 */ 386 public List<MonetaryComponent> getPriceComponent() { 387 if (this.priceComponent == null) 388 this.priceComponent = new ArrayList<MonetaryComponent>(); 389 return this.priceComponent; 390 } 391 392 /** 393 * @return Returns a reference to <code>this</code> for easy method chaining 394 */ 395 public ChargeItemDefinitionPropertyGroupComponent setPriceComponent(List<MonetaryComponent> thePriceComponent) { 396 this.priceComponent = thePriceComponent; 397 return this; 398 } 399 400 public boolean hasPriceComponent() { 401 if (this.priceComponent == null) 402 return false; 403 for (MonetaryComponent item : this.priceComponent) 404 if (!item.isEmpty()) 405 return true; 406 return false; 407 } 408 409 public MonetaryComponent addPriceComponent() { //3 410 MonetaryComponent t = new MonetaryComponent(); 411 if (this.priceComponent == null) 412 this.priceComponent = new ArrayList<MonetaryComponent>(); 413 this.priceComponent.add(t); 414 return t; 415 } 416 417 public ChargeItemDefinitionPropertyGroupComponent addPriceComponent(MonetaryComponent t) { //3 418 if (t == null) 419 return this; 420 if (this.priceComponent == null) 421 this.priceComponent = new ArrayList<MonetaryComponent>(); 422 this.priceComponent.add(t); 423 return this; 424 } 425 426 /** 427 * @return The first repetition of repeating field {@link #priceComponent}, creating it if it does not already exist {3} 428 */ 429 public MonetaryComponent getPriceComponentFirstRep() { 430 if (getPriceComponent().isEmpty()) { 431 addPriceComponent(); 432 } 433 return getPriceComponent().get(0); 434 } 435 436 protected void listChildren(List<Property> children) { 437 super.listChildren(children); 438 children.add(new Property("applicability", "@ChargeItemDefinition.applicability", "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, applicability)); 439 children.add(new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent)); 440 } 441 442 @Override 443 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 444 switch (_hash) { 445 case -1526770491: /*applicability*/ return new Property("applicability", "@ChargeItemDefinition.applicability", "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, applicability); 446 case 1219095988: /*priceComponent*/ return new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent); 447 default: return super.getNamedProperty(_hash, _name, _checkValid); 448 } 449 450 } 451 452 @Override 453 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 454 switch (hash) { 455 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 456 case 1219095988: /*priceComponent*/ return this.priceComponent == null ? new Base[0] : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // MonetaryComponent 457 default: return super.getProperty(hash, name, checkValid); 458 } 459 460 } 461 462 @Override 463 public Base setProperty(int hash, String name, Base value) throws FHIRException { 464 switch (hash) { 465 case -1526770491: // applicability 466 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 467 return value; 468 case 1219095988: // priceComponent 469 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); // MonetaryComponent 470 return value; 471 default: return super.setProperty(hash, name, value); 472 } 473 474 } 475 476 @Override 477 public Base setProperty(String name, Base value) throws FHIRException { 478 if (name.equals("applicability")) { 479 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 480 } else if (name.equals("priceComponent")) { 481 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); 482 } else 483 return super.setProperty(name, value); 484 return value; 485 } 486 487 @Override 488 public Base makeProperty(int hash, String name) throws FHIRException { 489 switch (hash) { 490 case -1526770491: return addApplicability(); 491 case 1219095988: return addPriceComponent(); 492 default: return super.makeProperty(hash, name); 493 } 494 495 } 496 497 @Override 498 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 499 switch (hash) { 500 case -1526770491: /*applicability*/ return new String[] {"@ChargeItemDefinition.applicability"}; 501 case 1219095988: /*priceComponent*/ return new String[] {"MonetaryComponent"}; 502 default: return super.getTypesForProperty(hash, name); 503 } 504 505 } 506 507 @Override 508 public Base addChild(String name) throws FHIRException { 509 if (name.equals("applicability")) { 510 return addApplicability(); 511 } 512 else if (name.equals("priceComponent")) { 513 return addPriceComponent(); 514 } 515 else 516 return super.addChild(name); 517 } 518 519 public ChargeItemDefinitionPropertyGroupComponent copy() { 520 ChargeItemDefinitionPropertyGroupComponent dst = new ChargeItemDefinitionPropertyGroupComponent(); 521 copyValues(dst); 522 return dst; 523 } 524 525 public void copyValues(ChargeItemDefinitionPropertyGroupComponent dst) { 526 super.copyValues(dst); 527 if (applicability != null) { 528 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 529 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 530 dst.applicability.add(i.copy()); 531 }; 532 if (priceComponent != null) { 533 dst.priceComponent = new ArrayList<MonetaryComponent>(); 534 for (MonetaryComponent i : priceComponent) 535 dst.priceComponent.add(i.copy()); 536 }; 537 } 538 539 @Override 540 public boolean equalsDeep(Base other_) { 541 if (!super.equalsDeep(other_)) 542 return false; 543 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 544 return false; 545 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 546 return compareDeep(applicability, o.applicability, true) && compareDeep(priceComponent, o.priceComponent, true) 547 ; 548 } 549 550 @Override 551 public boolean equalsShallow(Base other_) { 552 if (!super.equalsShallow(other_)) 553 return false; 554 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 555 return false; 556 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 557 return true; 558 } 559 560 public boolean isEmpty() { 561 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(applicability, priceComponent 562 ); 563 } 564 565 public String fhirType() { 566 return "ChargeItemDefinition.propertyGroup"; 567 568 } 569 570 } 571 572 /** 573 * An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers. 574 */ 575 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 576 @Description(shortDefinition="Canonical identifier for this charge item definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers." ) 577 protected UriType url; 578 579 /** 580 * A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 581 */ 582 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 583 @Description(shortDefinition="Additional identifier for the charge item definition", formalDefinition="A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 584 protected List<Identifier> identifier; 585 586 /** 587 * The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 588 */ 589 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 590 @Description(shortDefinition="Business version of the charge item definition", formalDefinition="The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets." ) 591 protected StringType version; 592 593 /** 594 * Indicates the mechanism used to compare versions to determine which is more current. 595 */ 596 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 597 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 598 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 599 protected DataType versionAlgorithm; 600 601 /** 602 * A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 603 */ 604 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 605 @Description(shortDefinition="Name for this charge item definition (computer friendly)", formalDefinition="A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 606 protected StringType name; 607 608 /** 609 * A short, descriptive, user-friendly title for the charge item definition. 610 */ 611 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 612 @Description(shortDefinition="Name for this charge item definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the charge item definition." ) 613 protected StringType title; 614 615 /** 616 * The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition. 617 */ 618 @Child(name = "derivedFromUri", type = {UriType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 619 @Description(shortDefinition="Underlying externally-defined charge item definition", formalDefinition="The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition." ) 620 protected List<UriType> derivedFromUri; 621 622 /** 623 * A larger definition of which this particular definition is a component or step. 624 */ 625 @Child(name = "partOf", type = {CanonicalType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 626 @Description(shortDefinition="A larger definition of which this particular definition is a component or step", formalDefinition="A larger definition of which this particular definition is a component or step." ) 627 protected List<CanonicalType> partOf; 628 629 /** 630 * As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance. 631 */ 632 @Child(name = "replaces", type = {CanonicalType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 633 @Description(shortDefinition="Completed or terminated request(s) whose function is taken by this new request", formalDefinition="As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance." ) 634 protected List<CanonicalType> replaces; 635 636 /** 637 * The current state of the ChargeItemDefinition. 638 */ 639 @Child(name = "status", type = {CodeType.class}, order=9, min=1, max=1, modifier=true, summary=true) 640 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the ChargeItemDefinition." ) 641 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 642 protected Enumeration<PublicationStatus> status; 643 644 /** 645 * A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 646 */ 647 @Child(name = "experimental", type = {BooleanType.class}, order=10, min=0, max=1, modifier=false, summary=true) 648 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 649 protected BooleanType experimental; 650 651 /** 652 * The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes. 653 */ 654 @Child(name = "date", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 655 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes." ) 656 protected DateTimeType date; 657 658 /** 659 * The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition. 660 */ 661 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 662 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition." ) 663 protected StringType publisher; 664 665 /** 666 * Contact details to assist a user in finding and communicating with the publisher. 667 */ 668 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 669 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 670 protected List<ContactDetail> contact; 671 672 /** 673 * A free text natural language description of the charge item definition from a consumer's perspective. 674 */ 675 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=true) 676 @Description(shortDefinition="Natural language description of the charge item definition", formalDefinition="A free text natural language description of the charge item definition from a consumer's perspective." ) 677 protected MarkdownType description; 678 679 /** 680 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances. 681 */ 682 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 683 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances." ) 684 protected List<UsageContext> useContext; 685 686 /** 687 * A legal or geographic region in which the charge item definition is intended to be used. 688 */ 689 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 690 @Description(shortDefinition="Intended jurisdiction for charge item definition (if applicable)", formalDefinition="A legal or geographic region in which the charge item definition is intended to be used." ) 691 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 692 protected List<CodeableConcept> jurisdiction; 693 694 /** 695 * Explanation of why this charge item definition is needed and why it has been designed as it has. 696 */ 697 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 698 @Description(shortDefinition="Why this charge item definition is defined", formalDefinition="Explanation of why this charge item definition is needed and why it has been designed as it has." ) 699 protected MarkdownType purpose; 700 701 /** 702 * A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition. 703 */ 704 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 705 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition." ) 706 protected MarkdownType copyright; 707 708 /** 709 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 710 */ 711 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 712 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 713 protected StringType copyrightLabel; 714 715 /** 716 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 717 */ 718 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 719 @Description(shortDefinition="When the charge item definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 720 protected DateType approvalDate; 721 722 /** 723 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 724 */ 725 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 726 @Description(shortDefinition="When the charge item definition was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 727 protected DateType lastReviewDate; 728 729 /** 730 * The defined billing details in this resource pertain to the given billing code. 731 */ 732 @Child(name = "code", type = {CodeableConcept.class}, order=22, min=0, max=1, modifier=false, summary=true) 733 @Description(shortDefinition="Billing code or product type this definition applies to", formalDefinition="The defined billing details in this resource pertain to the given billing code." ) 734 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 735 protected CodeableConcept code; 736 737 /** 738 * The defined billing details in this resource pertain to the given product instance(s). 739 */ 740 @Child(name = "instance", type = {Medication.class, Substance.class, Device.class, DeviceDefinition.class, ActivityDefinition.class, PlanDefinition.class, HealthcareService.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 741 @Description(shortDefinition="Instances this definition applies to", formalDefinition="The defined billing details in this resource pertain to the given product instance(s)." ) 742 protected List<Reference> instance; 743 744 /** 745 * Expressions that describe applicability criteria for the billing code. 746 */ 747 @Child(name = "applicability", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 748 @Description(shortDefinition="Whether or not the billing code is applicable", formalDefinition="Expressions that describe applicability criteria for the billing code." ) 749 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 750 751 /** 752 * Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply. 753 */ 754 @Child(name = "propertyGroup", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 755 @Description(shortDefinition="Group of properties which are applicable under the same conditions", formalDefinition="Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply." ) 756 protected List<ChargeItemDefinitionPropertyGroupComponent> propertyGroup; 757 758 private static final long serialVersionUID = 900671183L; 759 760 /** 761 * Constructor 762 */ 763 public ChargeItemDefinition() { 764 super(); 765 } 766 767 /** 768 * Constructor 769 */ 770 public ChargeItemDefinition(PublicationStatus status) { 771 super(); 772 this.setStatus(status); 773 } 774 775 /** 776 * @return {@link #url} (An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 777 */ 778 public UriType getUrlElement() { 779 if (this.url == null) 780 if (Configuration.errorOnAutoCreate()) 781 throw new Error("Attempt to auto-create ChargeItemDefinition.url"); 782 else if (Configuration.doAutoCreate()) 783 this.url = new UriType(); // bb 784 return this.url; 785 } 786 787 public boolean hasUrlElement() { 788 return this.url != null && !this.url.isEmpty(); 789 } 790 791 public boolean hasUrl() { 792 return this.url != null && !this.url.isEmpty(); 793 } 794 795 /** 796 * @param value {@link #url} (An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 797 */ 798 public ChargeItemDefinition setUrlElement(UriType value) { 799 this.url = value; 800 return this; 801 } 802 803 /** 804 * @return An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers. 805 */ 806 public String getUrl() { 807 return this.url == null ? null : this.url.getValue(); 808 } 809 810 /** 811 * @param value An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers. 812 */ 813 public ChargeItemDefinition setUrl(String value) { 814 if (Utilities.noString(value)) 815 this.url = null; 816 else { 817 if (this.url == null) 818 this.url = new UriType(); 819 this.url.setValue(value); 820 } 821 return this; 822 } 823 824 /** 825 * @return {@link #identifier} (A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 826 */ 827 public List<Identifier> getIdentifier() { 828 if (this.identifier == null) 829 this.identifier = new ArrayList<Identifier>(); 830 return this.identifier; 831 } 832 833 /** 834 * @return Returns a reference to <code>this</code> for easy method chaining 835 */ 836 public ChargeItemDefinition setIdentifier(List<Identifier> theIdentifier) { 837 this.identifier = theIdentifier; 838 return this; 839 } 840 841 public boolean hasIdentifier() { 842 if (this.identifier == null) 843 return false; 844 for (Identifier item : this.identifier) 845 if (!item.isEmpty()) 846 return true; 847 return false; 848 } 849 850 public Identifier addIdentifier() { //3 851 Identifier t = new Identifier(); 852 if (this.identifier == null) 853 this.identifier = new ArrayList<Identifier>(); 854 this.identifier.add(t); 855 return t; 856 } 857 858 public ChargeItemDefinition addIdentifier(Identifier t) { //3 859 if (t == null) 860 return this; 861 if (this.identifier == null) 862 this.identifier = new ArrayList<Identifier>(); 863 this.identifier.add(t); 864 return this; 865 } 866 867 /** 868 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 869 */ 870 public Identifier getIdentifierFirstRep() { 871 if (getIdentifier().isEmpty()) { 872 addIdentifier(); 873 } 874 return getIdentifier().get(0); 875 } 876 877 /** 878 * @return {@link #version} (The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 879 */ 880 public StringType getVersionElement() { 881 if (this.version == null) 882 if (Configuration.errorOnAutoCreate()) 883 throw new Error("Attempt to auto-create ChargeItemDefinition.version"); 884 else if (Configuration.doAutoCreate()) 885 this.version = new StringType(); // bb 886 return this.version; 887 } 888 889 public boolean hasVersionElement() { 890 return this.version != null && !this.version.isEmpty(); 891 } 892 893 public boolean hasVersion() { 894 return this.version != null && !this.version.isEmpty(); 895 } 896 897 /** 898 * @param value {@link #version} (The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 899 */ 900 public ChargeItemDefinition setVersionElement(StringType value) { 901 this.version = value; 902 return this; 903 } 904 905 /** 906 * @return The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 907 */ 908 public String getVersion() { 909 return this.version == null ? null : this.version.getValue(); 910 } 911 912 /** 913 * @param value The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 914 */ 915 public ChargeItemDefinition setVersion(String value) { 916 if (Utilities.noString(value)) 917 this.version = null; 918 else { 919 if (this.version == null) 920 this.version = new StringType(); 921 this.version.setValue(value); 922 } 923 return this; 924 } 925 926 /** 927 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 928 */ 929 public DataType getVersionAlgorithm() { 930 return this.versionAlgorithm; 931 } 932 933 /** 934 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 935 */ 936 public StringType getVersionAlgorithmStringType() throws FHIRException { 937 if (this.versionAlgorithm == null) 938 this.versionAlgorithm = new StringType(); 939 if (!(this.versionAlgorithm instanceof StringType)) 940 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 941 return (StringType) this.versionAlgorithm; 942 } 943 944 public boolean hasVersionAlgorithmStringType() { 945 return this != null && this.versionAlgorithm instanceof StringType; 946 } 947 948 /** 949 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 950 */ 951 public Coding getVersionAlgorithmCoding() throws FHIRException { 952 if (this.versionAlgorithm == null) 953 this.versionAlgorithm = new Coding(); 954 if (!(this.versionAlgorithm instanceof Coding)) 955 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 956 return (Coding) this.versionAlgorithm; 957 } 958 959 public boolean hasVersionAlgorithmCoding() { 960 return this != null && this.versionAlgorithm instanceof Coding; 961 } 962 963 public boolean hasVersionAlgorithm() { 964 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 965 } 966 967 /** 968 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 969 */ 970 public ChargeItemDefinition setVersionAlgorithm(DataType value) { 971 if (value != null && !(value instanceof StringType || value instanceof Coding)) 972 throw new FHIRException("Not the right type for ChargeItemDefinition.versionAlgorithm[x]: "+value.fhirType()); 973 this.versionAlgorithm = value; 974 return this; 975 } 976 977 /** 978 * @return {@link #name} (A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 979 */ 980 public StringType getNameElement() { 981 if (this.name == null) 982 if (Configuration.errorOnAutoCreate()) 983 throw new Error("Attempt to auto-create ChargeItemDefinition.name"); 984 else if (Configuration.doAutoCreate()) 985 this.name = new StringType(); // bb 986 return this.name; 987 } 988 989 public boolean hasNameElement() { 990 return this.name != null && !this.name.isEmpty(); 991 } 992 993 public boolean hasName() { 994 return this.name != null && !this.name.isEmpty(); 995 } 996 997 /** 998 * @param value {@link #name} (A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 999 */ 1000 public ChargeItemDefinition setNameElement(StringType value) { 1001 this.name = value; 1002 return this; 1003 } 1004 1005 /** 1006 * @return A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1007 */ 1008 public String getName() { 1009 return this.name == null ? null : this.name.getValue(); 1010 } 1011 1012 /** 1013 * @param value A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1014 */ 1015 public ChargeItemDefinition setName(String value) { 1016 if (Utilities.noString(value)) 1017 this.name = null; 1018 else { 1019 if (this.name == null) 1020 this.name = new StringType(); 1021 this.name.setValue(value); 1022 } 1023 return this; 1024 } 1025 1026 /** 1027 * @return {@link #title} (A short, descriptive, user-friendly title for the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1028 */ 1029 public StringType getTitleElement() { 1030 if (this.title == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create ChargeItemDefinition.title"); 1033 else if (Configuration.doAutoCreate()) 1034 this.title = new StringType(); // bb 1035 return this.title; 1036 } 1037 1038 public boolean hasTitleElement() { 1039 return this.title != null && !this.title.isEmpty(); 1040 } 1041 1042 public boolean hasTitle() { 1043 return this.title != null && !this.title.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #title} (A short, descriptive, user-friendly title for the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1048 */ 1049 public ChargeItemDefinition setTitleElement(StringType value) { 1050 this.title = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return A short, descriptive, user-friendly title for the charge item definition. 1056 */ 1057 public String getTitle() { 1058 return this.title == null ? null : this.title.getValue(); 1059 } 1060 1061 /** 1062 * @param value A short, descriptive, user-friendly title for the charge item definition. 1063 */ 1064 public ChargeItemDefinition setTitle(String value) { 1065 if (Utilities.noString(value)) 1066 this.title = null; 1067 else { 1068 if (this.title == null) 1069 this.title = new StringType(); 1070 this.title.setValue(value); 1071 } 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1077 */ 1078 public List<UriType> getDerivedFromUri() { 1079 if (this.derivedFromUri == null) 1080 this.derivedFromUri = new ArrayList<UriType>(); 1081 return this.derivedFromUri; 1082 } 1083 1084 /** 1085 * @return Returns a reference to <code>this</code> for easy method chaining 1086 */ 1087 public ChargeItemDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 1088 this.derivedFromUri = theDerivedFromUri; 1089 return this; 1090 } 1091 1092 public boolean hasDerivedFromUri() { 1093 if (this.derivedFromUri == null) 1094 return false; 1095 for (UriType item : this.derivedFromUri) 1096 if (!item.isEmpty()) 1097 return true; 1098 return false; 1099 } 1100 1101 /** 1102 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1103 */ 1104 public UriType addDerivedFromUriElement() {//2 1105 UriType t = new UriType(); 1106 if (this.derivedFromUri == null) 1107 this.derivedFromUri = new ArrayList<UriType>(); 1108 this.derivedFromUri.add(t); 1109 return t; 1110 } 1111 1112 /** 1113 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1114 */ 1115 public ChargeItemDefinition addDerivedFromUri(String value) { //1 1116 UriType t = new UriType(); 1117 t.setValue(value); 1118 if (this.derivedFromUri == null) 1119 this.derivedFromUri = new ArrayList<UriType>(); 1120 this.derivedFromUri.add(t); 1121 return this; 1122 } 1123 1124 /** 1125 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1126 */ 1127 public boolean hasDerivedFromUri(String value) { 1128 if (this.derivedFromUri == null) 1129 return false; 1130 for (UriType v : this.derivedFromUri) 1131 if (v.getValue().equals(value)) // uri 1132 return true; 1133 return false; 1134 } 1135 1136 /** 1137 * @return {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1138 */ 1139 public List<CanonicalType> getPartOf() { 1140 if (this.partOf == null) 1141 this.partOf = new ArrayList<CanonicalType>(); 1142 return this.partOf; 1143 } 1144 1145 /** 1146 * @return Returns a reference to <code>this</code> for easy method chaining 1147 */ 1148 public ChargeItemDefinition setPartOf(List<CanonicalType> thePartOf) { 1149 this.partOf = thePartOf; 1150 return this; 1151 } 1152 1153 public boolean hasPartOf() { 1154 if (this.partOf == null) 1155 return false; 1156 for (CanonicalType item : this.partOf) 1157 if (!item.isEmpty()) 1158 return true; 1159 return false; 1160 } 1161 1162 /** 1163 * @return {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1164 */ 1165 public CanonicalType addPartOfElement() {//2 1166 CanonicalType t = new CanonicalType(); 1167 if (this.partOf == null) 1168 this.partOf = new ArrayList<CanonicalType>(); 1169 this.partOf.add(t); 1170 return t; 1171 } 1172 1173 /** 1174 * @param value {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1175 */ 1176 public ChargeItemDefinition addPartOf(String value) { //1 1177 CanonicalType t = new CanonicalType(); 1178 t.setValue(value); 1179 if (this.partOf == null) 1180 this.partOf = new ArrayList<CanonicalType>(); 1181 this.partOf.add(t); 1182 return this; 1183 } 1184 1185 /** 1186 * @param value {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1187 */ 1188 public boolean hasPartOf(String value) { 1189 if (this.partOf == null) 1190 return false; 1191 for (CanonicalType v : this.partOf) 1192 if (v.getValue().equals(value)) // canonical 1193 return true; 1194 return false; 1195 } 1196 1197 /** 1198 * @return {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1199 */ 1200 public List<CanonicalType> getReplaces() { 1201 if (this.replaces == null) 1202 this.replaces = new ArrayList<CanonicalType>(); 1203 return this.replaces; 1204 } 1205 1206 /** 1207 * @return Returns a reference to <code>this</code> for easy method chaining 1208 */ 1209 public ChargeItemDefinition setReplaces(List<CanonicalType> theReplaces) { 1210 this.replaces = theReplaces; 1211 return this; 1212 } 1213 1214 public boolean hasReplaces() { 1215 if (this.replaces == null) 1216 return false; 1217 for (CanonicalType item : this.replaces) 1218 if (!item.isEmpty()) 1219 return true; 1220 return false; 1221 } 1222 1223 /** 1224 * @return {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1225 */ 1226 public CanonicalType addReplacesElement() {//2 1227 CanonicalType t = new CanonicalType(); 1228 if (this.replaces == null) 1229 this.replaces = new ArrayList<CanonicalType>(); 1230 this.replaces.add(t); 1231 return t; 1232 } 1233 1234 /** 1235 * @param value {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1236 */ 1237 public ChargeItemDefinition addReplaces(String value) { //1 1238 CanonicalType t = new CanonicalType(); 1239 t.setValue(value); 1240 if (this.replaces == null) 1241 this.replaces = new ArrayList<CanonicalType>(); 1242 this.replaces.add(t); 1243 return this; 1244 } 1245 1246 /** 1247 * @param value {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1248 */ 1249 public boolean hasReplaces(String value) { 1250 if (this.replaces == null) 1251 return false; 1252 for (CanonicalType v : this.replaces) 1253 if (v.getValue().equals(value)) // canonical 1254 return true; 1255 return false; 1256 } 1257 1258 /** 1259 * @return {@link #status} (The current state of the ChargeItemDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1260 */ 1261 public Enumeration<PublicationStatus> getStatusElement() { 1262 if (this.status == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create ChargeItemDefinition.status"); 1265 else if (Configuration.doAutoCreate()) 1266 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1267 return this.status; 1268 } 1269 1270 public boolean hasStatusElement() { 1271 return this.status != null && !this.status.isEmpty(); 1272 } 1273 1274 public boolean hasStatus() { 1275 return this.status != null && !this.status.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #status} (The current state of the ChargeItemDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1280 */ 1281 public ChargeItemDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1282 this.status = value; 1283 return this; 1284 } 1285 1286 /** 1287 * @return The current state of the ChargeItemDefinition. 1288 */ 1289 public PublicationStatus getStatus() { 1290 return this.status == null ? null : this.status.getValue(); 1291 } 1292 1293 /** 1294 * @param value The current state of the ChargeItemDefinition. 1295 */ 1296 public ChargeItemDefinition setStatus(PublicationStatus value) { 1297 if (this.status == null) 1298 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1299 this.status.setValue(value); 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #experimental} (A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1305 */ 1306 public BooleanType getExperimentalElement() { 1307 if (this.experimental == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create ChargeItemDefinition.experimental"); 1310 else if (Configuration.doAutoCreate()) 1311 this.experimental = new BooleanType(); // bb 1312 return this.experimental; 1313 } 1314 1315 public boolean hasExperimentalElement() { 1316 return this.experimental != null && !this.experimental.isEmpty(); 1317 } 1318 1319 public boolean hasExperimental() { 1320 return this.experimental != null && !this.experimental.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #experimental} (A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1325 */ 1326 public ChargeItemDefinition setExperimentalElement(BooleanType value) { 1327 this.experimental = value; 1328 return this; 1329 } 1330 1331 /** 1332 * @return A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1333 */ 1334 public boolean getExperimental() { 1335 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1336 } 1337 1338 /** 1339 * @param value A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1340 */ 1341 public ChargeItemDefinition setExperimental(boolean value) { 1342 if (this.experimental == null) 1343 this.experimental = new BooleanType(); 1344 this.experimental.setValue(value); 1345 return this; 1346 } 1347 1348 /** 1349 * @return {@link #date} (The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1350 */ 1351 public DateTimeType getDateElement() { 1352 if (this.date == null) 1353 if (Configuration.errorOnAutoCreate()) 1354 throw new Error("Attempt to auto-create ChargeItemDefinition.date"); 1355 else if (Configuration.doAutoCreate()) 1356 this.date = new DateTimeType(); // bb 1357 return this.date; 1358 } 1359 1360 public boolean hasDateElement() { 1361 return this.date != null && !this.date.isEmpty(); 1362 } 1363 1364 public boolean hasDate() { 1365 return this.date != null && !this.date.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #date} (The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1370 */ 1371 public ChargeItemDefinition setDateElement(DateTimeType value) { 1372 this.date = value; 1373 return this; 1374 } 1375 1376 /** 1377 * @return The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes. 1378 */ 1379 public Date getDate() { 1380 return this.date == null ? null : this.date.getValue(); 1381 } 1382 1383 /** 1384 * @param value The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes. 1385 */ 1386 public ChargeItemDefinition setDate(Date value) { 1387 if (value == null) 1388 this.date = null; 1389 else { 1390 if (this.date == null) 1391 this.date = new DateTimeType(); 1392 this.date.setValue(value); 1393 } 1394 return this; 1395 } 1396 1397 /** 1398 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1399 */ 1400 public StringType getPublisherElement() { 1401 if (this.publisher == null) 1402 if (Configuration.errorOnAutoCreate()) 1403 throw new Error("Attempt to auto-create ChargeItemDefinition.publisher"); 1404 else if (Configuration.doAutoCreate()) 1405 this.publisher = new StringType(); // bb 1406 return this.publisher; 1407 } 1408 1409 public boolean hasPublisherElement() { 1410 return this.publisher != null && !this.publisher.isEmpty(); 1411 } 1412 1413 public boolean hasPublisher() { 1414 return this.publisher != null && !this.publisher.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1419 */ 1420 public ChargeItemDefinition setPublisherElement(StringType value) { 1421 this.publisher = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition. 1427 */ 1428 public String getPublisher() { 1429 return this.publisher == null ? null : this.publisher.getValue(); 1430 } 1431 1432 /** 1433 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition. 1434 */ 1435 public ChargeItemDefinition setPublisher(String value) { 1436 if (Utilities.noString(value)) 1437 this.publisher = null; 1438 else { 1439 if (this.publisher == null) 1440 this.publisher = new StringType(); 1441 this.publisher.setValue(value); 1442 } 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1448 */ 1449 public List<ContactDetail> getContact() { 1450 if (this.contact == null) 1451 this.contact = new ArrayList<ContactDetail>(); 1452 return this.contact; 1453 } 1454 1455 /** 1456 * @return Returns a reference to <code>this</code> for easy method chaining 1457 */ 1458 public ChargeItemDefinition setContact(List<ContactDetail> theContact) { 1459 this.contact = theContact; 1460 return this; 1461 } 1462 1463 public boolean hasContact() { 1464 if (this.contact == null) 1465 return false; 1466 for (ContactDetail item : this.contact) 1467 if (!item.isEmpty()) 1468 return true; 1469 return false; 1470 } 1471 1472 public ContactDetail addContact() { //3 1473 ContactDetail t = new ContactDetail(); 1474 if (this.contact == null) 1475 this.contact = new ArrayList<ContactDetail>(); 1476 this.contact.add(t); 1477 return t; 1478 } 1479 1480 public ChargeItemDefinition addContact(ContactDetail t) { //3 1481 if (t == null) 1482 return this; 1483 if (this.contact == null) 1484 this.contact = new ArrayList<ContactDetail>(); 1485 this.contact.add(t); 1486 return this; 1487 } 1488 1489 /** 1490 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1491 */ 1492 public ContactDetail getContactFirstRep() { 1493 if (getContact().isEmpty()) { 1494 addContact(); 1495 } 1496 return getContact().get(0); 1497 } 1498 1499 /** 1500 * @return {@link #description} (A free text natural language description of the charge item definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1501 */ 1502 public MarkdownType getDescriptionElement() { 1503 if (this.description == null) 1504 if (Configuration.errorOnAutoCreate()) 1505 throw new Error("Attempt to auto-create ChargeItemDefinition.description"); 1506 else if (Configuration.doAutoCreate()) 1507 this.description = new MarkdownType(); // bb 1508 return this.description; 1509 } 1510 1511 public boolean hasDescriptionElement() { 1512 return this.description != null && !this.description.isEmpty(); 1513 } 1514 1515 public boolean hasDescription() { 1516 return this.description != null && !this.description.isEmpty(); 1517 } 1518 1519 /** 1520 * @param value {@link #description} (A free text natural language description of the charge item definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1521 */ 1522 public ChargeItemDefinition setDescriptionElement(MarkdownType value) { 1523 this.description = value; 1524 return this; 1525 } 1526 1527 /** 1528 * @return A free text natural language description of the charge item definition from a consumer's perspective. 1529 */ 1530 public String getDescription() { 1531 return this.description == null ? null : this.description.getValue(); 1532 } 1533 1534 /** 1535 * @param value A free text natural language description of the charge item definition from a consumer's perspective. 1536 */ 1537 public ChargeItemDefinition setDescription(String value) { 1538 if (Utilities.noString(value)) 1539 this.description = null; 1540 else { 1541 if (this.description == null) 1542 this.description = new MarkdownType(); 1543 this.description.setValue(value); 1544 } 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.) 1550 */ 1551 public List<UsageContext> getUseContext() { 1552 if (this.useContext == null) 1553 this.useContext = new ArrayList<UsageContext>(); 1554 return this.useContext; 1555 } 1556 1557 /** 1558 * @return Returns a reference to <code>this</code> for easy method chaining 1559 */ 1560 public ChargeItemDefinition setUseContext(List<UsageContext> theUseContext) { 1561 this.useContext = theUseContext; 1562 return this; 1563 } 1564 1565 public boolean hasUseContext() { 1566 if (this.useContext == null) 1567 return false; 1568 for (UsageContext item : this.useContext) 1569 if (!item.isEmpty()) 1570 return true; 1571 return false; 1572 } 1573 1574 public UsageContext addUseContext() { //3 1575 UsageContext t = new UsageContext(); 1576 if (this.useContext == null) 1577 this.useContext = new ArrayList<UsageContext>(); 1578 this.useContext.add(t); 1579 return t; 1580 } 1581 1582 public ChargeItemDefinition addUseContext(UsageContext t) { //3 1583 if (t == null) 1584 return this; 1585 if (this.useContext == null) 1586 this.useContext = new ArrayList<UsageContext>(); 1587 this.useContext.add(t); 1588 return this; 1589 } 1590 1591 /** 1592 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1593 */ 1594 public UsageContext getUseContextFirstRep() { 1595 if (getUseContext().isEmpty()) { 1596 addUseContext(); 1597 } 1598 return getUseContext().get(0); 1599 } 1600 1601 /** 1602 * @return {@link #jurisdiction} (A legal or geographic region in which the charge item definition is intended to be used.) 1603 */ 1604 public List<CodeableConcept> getJurisdiction() { 1605 if (this.jurisdiction == null) 1606 this.jurisdiction = new ArrayList<CodeableConcept>(); 1607 return this.jurisdiction; 1608 } 1609 1610 /** 1611 * @return Returns a reference to <code>this</code> for easy method chaining 1612 */ 1613 public ChargeItemDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1614 this.jurisdiction = theJurisdiction; 1615 return this; 1616 } 1617 1618 public boolean hasJurisdiction() { 1619 if (this.jurisdiction == null) 1620 return false; 1621 for (CodeableConcept item : this.jurisdiction) 1622 if (!item.isEmpty()) 1623 return true; 1624 return false; 1625 } 1626 1627 public CodeableConcept addJurisdiction() { //3 1628 CodeableConcept t = new CodeableConcept(); 1629 if (this.jurisdiction == null) 1630 this.jurisdiction = new ArrayList<CodeableConcept>(); 1631 this.jurisdiction.add(t); 1632 return t; 1633 } 1634 1635 public ChargeItemDefinition addJurisdiction(CodeableConcept t) { //3 1636 if (t == null) 1637 return this; 1638 if (this.jurisdiction == null) 1639 this.jurisdiction = new ArrayList<CodeableConcept>(); 1640 this.jurisdiction.add(t); 1641 return this; 1642 } 1643 1644 /** 1645 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1646 */ 1647 public CodeableConcept getJurisdictionFirstRep() { 1648 if (getJurisdiction().isEmpty()) { 1649 addJurisdiction(); 1650 } 1651 return getJurisdiction().get(0); 1652 } 1653 1654 /** 1655 * @return {@link #purpose} (Explanation of why this charge item definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1656 */ 1657 public MarkdownType getPurposeElement() { 1658 if (this.purpose == null) 1659 if (Configuration.errorOnAutoCreate()) 1660 throw new Error("Attempt to auto-create ChargeItemDefinition.purpose"); 1661 else if (Configuration.doAutoCreate()) 1662 this.purpose = new MarkdownType(); // bb 1663 return this.purpose; 1664 } 1665 1666 public boolean hasPurposeElement() { 1667 return this.purpose != null && !this.purpose.isEmpty(); 1668 } 1669 1670 public boolean hasPurpose() { 1671 return this.purpose != null && !this.purpose.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #purpose} (Explanation of why this charge item definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1676 */ 1677 public ChargeItemDefinition setPurposeElement(MarkdownType value) { 1678 this.purpose = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return Explanation of why this charge item definition is needed and why it has been designed as it has. 1684 */ 1685 public String getPurpose() { 1686 return this.purpose == null ? null : this.purpose.getValue(); 1687 } 1688 1689 /** 1690 * @param value Explanation of why this charge item definition is needed and why it has been designed as it has. 1691 */ 1692 public ChargeItemDefinition setPurpose(String value) { 1693 if (Utilities.noString(value)) 1694 this.purpose = null; 1695 else { 1696 if (this.purpose == null) 1697 this.purpose = new MarkdownType(); 1698 this.purpose.setValue(value); 1699 } 1700 return this; 1701 } 1702 1703 /** 1704 * @return {@link #copyright} (A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1705 */ 1706 public MarkdownType getCopyrightElement() { 1707 if (this.copyright == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create ChargeItemDefinition.copyright"); 1710 else if (Configuration.doAutoCreate()) 1711 this.copyright = new MarkdownType(); // bb 1712 return this.copyright; 1713 } 1714 1715 public boolean hasCopyrightElement() { 1716 return this.copyright != null && !this.copyright.isEmpty(); 1717 } 1718 1719 public boolean hasCopyright() { 1720 return this.copyright != null && !this.copyright.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #copyright} (A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1725 */ 1726 public ChargeItemDefinition setCopyrightElement(MarkdownType value) { 1727 this.copyright = value; 1728 return this; 1729 } 1730 1731 /** 1732 * @return A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition. 1733 */ 1734 public String getCopyright() { 1735 return this.copyright == null ? null : this.copyright.getValue(); 1736 } 1737 1738 /** 1739 * @param value A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition. 1740 */ 1741 public ChargeItemDefinition setCopyright(String value) { 1742 if (Utilities.noString(value)) 1743 this.copyright = null; 1744 else { 1745 if (this.copyright == null) 1746 this.copyright = new MarkdownType(); 1747 this.copyright.setValue(value); 1748 } 1749 return this; 1750 } 1751 1752 /** 1753 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1754 */ 1755 public StringType getCopyrightLabelElement() { 1756 if (this.copyrightLabel == null) 1757 if (Configuration.errorOnAutoCreate()) 1758 throw new Error("Attempt to auto-create ChargeItemDefinition.copyrightLabel"); 1759 else if (Configuration.doAutoCreate()) 1760 this.copyrightLabel = new StringType(); // bb 1761 return this.copyrightLabel; 1762 } 1763 1764 public boolean hasCopyrightLabelElement() { 1765 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1766 } 1767 1768 public boolean hasCopyrightLabel() { 1769 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1770 } 1771 1772 /** 1773 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1774 */ 1775 public ChargeItemDefinition setCopyrightLabelElement(StringType value) { 1776 this.copyrightLabel = value; 1777 return this; 1778 } 1779 1780 /** 1781 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1782 */ 1783 public String getCopyrightLabel() { 1784 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1785 } 1786 1787 /** 1788 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1789 */ 1790 public ChargeItemDefinition setCopyrightLabel(String value) { 1791 if (Utilities.noString(value)) 1792 this.copyrightLabel = null; 1793 else { 1794 if (this.copyrightLabel == null) 1795 this.copyrightLabel = new StringType(); 1796 this.copyrightLabel.setValue(value); 1797 } 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1803 */ 1804 public DateType getApprovalDateElement() { 1805 if (this.approvalDate == null) 1806 if (Configuration.errorOnAutoCreate()) 1807 throw new Error("Attempt to auto-create ChargeItemDefinition.approvalDate"); 1808 else if (Configuration.doAutoCreate()) 1809 this.approvalDate = new DateType(); // bb 1810 return this.approvalDate; 1811 } 1812 1813 public boolean hasApprovalDateElement() { 1814 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1815 } 1816 1817 public boolean hasApprovalDate() { 1818 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1819 } 1820 1821 /** 1822 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1823 */ 1824 public ChargeItemDefinition setApprovalDateElement(DateType value) { 1825 this.approvalDate = value; 1826 return this; 1827 } 1828 1829 /** 1830 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1831 */ 1832 public Date getApprovalDate() { 1833 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1834 } 1835 1836 /** 1837 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1838 */ 1839 public ChargeItemDefinition setApprovalDate(Date value) { 1840 if (value == null) 1841 this.approvalDate = null; 1842 else { 1843 if (this.approvalDate == null) 1844 this.approvalDate = new DateType(); 1845 this.approvalDate.setValue(value); 1846 } 1847 return this; 1848 } 1849 1850 /** 1851 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1852 */ 1853 public DateType getLastReviewDateElement() { 1854 if (this.lastReviewDate == null) 1855 if (Configuration.errorOnAutoCreate()) 1856 throw new Error("Attempt to auto-create ChargeItemDefinition.lastReviewDate"); 1857 else if (Configuration.doAutoCreate()) 1858 this.lastReviewDate = new DateType(); // bb 1859 return this.lastReviewDate; 1860 } 1861 1862 public boolean hasLastReviewDateElement() { 1863 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1864 } 1865 1866 public boolean hasLastReviewDate() { 1867 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1868 } 1869 1870 /** 1871 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1872 */ 1873 public ChargeItemDefinition setLastReviewDateElement(DateType value) { 1874 this.lastReviewDate = value; 1875 return this; 1876 } 1877 1878 /** 1879 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1880 */ 1881 public Date getLastReviewDate() { 1882 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1883 } 1884 1885 /** 1886 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1887 */ 1888 public ChargeItemDefinition setLastReviewDate(Date value) { 1889 if (value == null) 1890 this.lastReviewDate = null; 1891 else { 1892 if (this.lastReviewDate == null) 1893 this.lastReviewDate = new DateType(); 1894 this.lastReviewDate.setValue(value); 1895 } 1896 return this; 1897 } 1898 1899 /** 1900 * @return {@link #code} (The defined billing details in this resource pertain to the given billing code.) 1901 */ 1902 public CodeableConcept getCode() { 1903 if (this.code == null) 1904 if (Configuration.errorOnAutoCreate()) 1905 throw new Error("Attempt to auto-create ChargeItemDefinition.code"); 1906 else if (Configuration.doAutoCreate()) 1907 this.code = new CodeableConcept(); // cc 1908 return this.code; 1909 } 1910 1911 public boolean hasCode() { 1912 return this.code != null && !this.code.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #code} (The defined billing details in this resource pertain to the given billing code.) 1917 */ 1918 public ChargeItemDefinition setCode(CodeableConcept value) { 1919 this.code = value; 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #instance} (The defined billing details in this resource pertain to the given product instance(s).) 1925 */ 1926 public List<Reference> getInstance() { 1927 if (this.instance == null) 1928 this.instance = new ArrayList<Reference>(); 1929 return this.instance; 1930 } 1931 1932 /** 1933 * @return Returns a reference to <code>this</code> for easy method chaining 1934 */ 1935 public ChargeItemDefinition setInstance(List<Reference> theInstance) { 1936 this.instance = theInstance; 1937 return this; 1938 } 1939 1940 public boolean hasInstance() { 1941 if (this.instance == null) 1942 return false; 1943 for (Reference item : this.instance) 1944 if (!item.isEmpty()) 1945 return true; 1946 return false; 1947 } 1948 1949 public Reference addInstance() { //3 1950 Reference t = new Reference(); 1951 if (this.instance == null) 1952 this.instance = new ArrayList<Reference>(); 1953 this.instance.add(t); 1954 return t; 1955 } 1956 1957 public ChargeItemDefinition addInstance(Reference t) { //3 1958 if (t == null) 1959 return this; 1960 if (this.instance == null) 1961 this.instance = new ArrayList<Reference>(); 1962 this.instance.add(t); 1963 return this; 1964 } 1965 1966 /** 1967 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 1968 */ 1969 public Reference getInstanceFirstRep() { 1970 if (getInstance().isEmpty()) { 1971 addInstance(); 1972 } 1973 return getInstance().get(0); 1974 } 1975 1976 /** 1977 * @return {@link #applicability} (Expressions that describe applicability criteria for the billing code.) 1978 */ 1979 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 1980 if (this.applicability == null) 1981 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 1982 return this.applicability; 1983 } 1984 1985 /** 1986 * @return Returns a reference to <code>this</code> for easy method chaining 1987 */ 1988 public ChargeItemDefinition setApplicability(List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 1989 this.applicability = theApplicability; 1990 return this; 1991 } 1992 1993 public boolean hasApplicability() { 1994 if (this.applicability == null) 1995 return false; 1996 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 1997 if (!item.isEmpty()) 1998 return true; 1999 return false; 2000 } 2001 2002 public ChargeItemDefinitionApplicabilityComponent addApplicability() { //3 2003 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 2004 if (this.applicability == null) 2005 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2006 this.applicability.add(t); 2007 return t; 2008 } 2009 2010 public ChargeItemDefinition addApplicability(ChargeItemDefinitionApplicabilityComponent t) { //3 2011 if (t == null) 2012 return this; 2013 if (this.applicability == null) 2014 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2015 this.applicability.add(t); 2016 return this; 2017 } 2018 2019 /** 2020 * @return The first repetition of repeating field {@link #applicability}, creating it if it does not already exist {3} 2021 */ 2022 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 2023 if (getApplicability().isEmpty()) { 2024 addApplicability(); 2025 } 2026 return getApplicability().get(0); 2027 } 2028 2029 /** 2030 * @return {@link #propertyGroup} (Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.) 2031 */ 2032 public List<ChargeItemDefinitionPropertyGroupComponent> getPropertyGroup() { 2033 if (this.propertyGroup == null) 2034 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2035 return this.propertyGroup; 2036 } 2037 2038 /** 2039 * @return Returns a reference to <code>this</code> for easy method chaining 2040 */ 2041 public ChargeItemDefinition setPropertyGroup(List<ChargeItemDefinitionPropertyGroupComponent> thePropertyGroup) { 2042 this.propertyGroup = thePropertyGroup; 2043 return this; 2044 } 2045 2046 public boolean hasPropertyGroup() { 2047 if (this.propertyGroup == null) 2048 return false; 2049 for (ChargeItemDefinitionPropertyGroupComponent item : this.propertyGroup) 2050 if (!item.isEmpty()) 2051 return true; 2052 return false; 2053 } 2054 2055 public ChargeItemDefinitionPropertyGroupComponent addPropertyGroup() { //3 2056 ChargeItemDefinitionPropertyGroupComponent t = new ChargeItemDefinitionPropertyGroupComponent(); 2057 if (this.propertyGroup == null) 2058 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2059 this.propertyGroup.add(t); 2060 return t; 2061 } 2062 2063 public ChargeItemDefinition addPropertyGroup(ChargeItemDefinitionPropertyGroupComponent t) { //3 2064 if (t == null) 2065 return this; 2066 if (this.propertyGroup == null) 2067 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2068 this.propertyGroup.add(t); 2069 return this; 2070 } 2071 2072 /** 2073 * @return The first repetition of repeating field {@link #propertyGroup}, creating it if it does not already exist {3} 2074 */ 2075 public ChargeItemDefinitionPropertyGroupComponent getPropertyGroupFirstRep() { 2076 if (getPropertyGroup().isEmpty()) { 2077 addPropertyGroup(); 2078 } 2079 return getPropertyGroup().get(0); 2080 } 2081 2082 /** 2083 * not supported on this implementation 2084 */ 2085 @Override 2086 public int getEffectivePeriodMax() { 2087 return 0; 2088 } 2089 /** 2090 * @return {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 2091 */ 2092 public Period getEffectivePeriod() { 2093 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"effectivePeriod\""); 2094 } 2095 public boolean hasEffectivePeriod() { 2096 return false; 2097 } 2098 /** 2099 * @param value {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 2100 */ 2101 public ChargeItemDefinition setEffectivePeriod(Period value) { 2102 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"effectivePeriod\""); 2103 } 2104 2105 /** 2106 * not supported on this implementation 2107 */ 2108 @Override 2109 public int getTopicMax() { 2110 return 0; 2111 } 2112 /** 2113 * @return {@link #topic} (Descriptive topics related to the content of the charge item definition. Topics provide a high-level categorization as well as keywords for the charge item definition that can be useful for filtering and searching.) 2114 */ 2115 public List<CodeableConcept> getTopic() { 2116 return new ArrayList<>(); 2117 } 2118 /** 2119 * @return Returns a reference to <code>this</code> for easy method chaining 2120 */ 2121 public ChargeItemDefinition setTopic(List<CodeableConcept> theTopic) { 2122 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2123 } 2124 public boolean hasTopic() { 2125 return false; 2126 } 2127 2128 public CodeableConcept addTopic() { //3 2129 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2130 } 2131 public ChargeItemDefinition addTopic(CodeableConcept t) { //3 2132 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2133 } 2134 /** 2135 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 2136 */ 2137 public CodeableConcept getTopicFirstRep() { 2138 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2139 } 2140 /** 2141 * not supported on this implementation 2142 */ 2143 @Override 2144 public int getAuthorMax() { 2145 return 0; 2146 } 2147 /** 2148 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the charge item definition.) 2149 */ 2150 public List<ContactDetail> getAuthor() { 2151 return new ArrayList<>(); 2152 } 2153 /** 2154 * @return Returns a reference to <code>this</code> for easy method chaining 2155 */ 2156 public ChargeItemDefinition setAuthor(List<ContactDetail> theAuthor) { 2157 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2158 } 2159 public boolean hasAuthor() { 2160 return false; 2161 } 2162 2163 public ContactDetail addAuthor() { //3 2164 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2165 } 2166 public ChargeItemDefinition addAuthor(ContactDetail t) { //3 2167 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2168 } 2169 /** 2170 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {2} 2171 */ 2172 public ContactDetail getAuthorFirstRep() { 2173 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2174 } 2175 /** 2176 * not supported on this implementation 2177 */ 2178 @Override 2179 public int getEditorMax() { 2180 return 0; 2181 } 2182 /** 2183 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the charge item definition.) 2184 */ 2185 public List<ContactDetail> getEditor() { 2186 return new ArrayList<>(); 2187 } 2188 /** 2189 * @return Returns a reference to <code>this</code> for easy method chaining 2190 */ 2191 public ChargeItemDefinition setEditor(List<ContactDetail> theEditor) { 2192 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2193 } 2194 public boolean hasEditor() { 2195 return false; 2196 } 2197 2198 public ContactDetail addEditor() { //3 2199 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2200 } 2201 public ChargeItemDefinition addEditor(ContactDetail t) { //3 2202 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2203 } 2204 /** 2205 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {2} 2206 */ 2207 public ContactDetail getEditorFirstRep() { 2208 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2209 } 2210 /** 2211 * not supported on this implementation 2212 */ 2213 @Override 2214 public int getReviewerMax() { 2215 return 0; 2216 } 2217 /** 2218 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the charge item definition.) 2219 */ 2220 public List<ContactDetail> getReviewer() { 2221 return new ArrayList<>(); 2222 } 2223 /** 2224 * @return Returns a reference to <code>this</code> for easy method chaining 2225 */ 2226 public ChargeItemDefinition setReviewer(List<ContactDetail> theReviewer) { 2227 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2228 } 2229 public boolean hasReviewer() { 2230 return false; 2231 } 2232 2233 public ContactDetail addReviewer() { //3 2234 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2235 } 2236 public ChargeItemDefinition addReviewer(ContactDetail t) { //3 2237 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2238 } 2239 /** 2240 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {2} 2241 */ 2242 public ContactDetail getReviewerFirstRep() { 2243 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2244 } 2245 /** 2246 * not supported on this implementation 2247 */ 2248 @Override 2249 public int getEndorserMax() { 2250 return 0; 2251 } 2252 /** 2253 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the charge item definition for use in some setting.) 2254 */ 2255 public List<ContactDetail> getEndorser() { 2256 return new ArrayList<>(); 2257 } 2258 /** 2259 * @return Returns a reference to <code>this</code> for easy method chaining 2260 */ 2261 public ChargeItemDefinition setEndorser(List<ContactDetail> theEndorser) { 2262 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2263 } 2264 public boolean hasEndorser() { 2265 return false; 2266 } 2267 2268 public ContactDetail addEndorser() { //3 2269 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2270 } 2271 public ChargeItemDefinition addEndorser(ContactDetail t) { //3 2272 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2273 } 2274 /** 2275 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {2} 2276 */ 2277 public ContactDetail getEndorserFirstRep() { 2278 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2279 } 2280 /** 2281 * not supported on this implementation 2282 */ 2283 @Override 2284 public int getRelatedArtifactMax() { 2285 return 0; 2286 } 2287 /** 2288 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 2289 */ 2290 public List<RelatedArtifact> getRelatedArtifact() { 2291 return new ArrayList<>(); 2292 } 2293 /** 2294 * @return Returns a reference to <code>this</code> for easy method chaining 2295 */ 2296 public ChargeItemDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2297 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2298 } 2299 public boolean hasRelatedArtifact() { 2300 return false; 2301 } 2302 2303 public RelatedArtifact addRelatedArtifact() { //3 2304 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2305 } 2306 public ChargeItemDefinition addRelatedArtifact(RelatedArtifact t) { //3 2307 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2308 } 2309 /** 2310 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {2} 2311 */ 2312 public RelatedArtifact getRelatedArtifactFirstRep() { 2313 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2314 } 2315 protected void listChildren(List<Property> children) { 2316 super.listChildren(children); 2317 children.add(new Property("url", "uri", "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 0, 1, url)); 2318 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2319 children.add(new Property("version", "string", "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version)); 2320 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2321 children.add(new Property("name", "string", "A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2322 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title)); 2323 children.add(new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 2324 children.add(new Property("partOf", "canonical(ChargeItemDefinition)", "A larger definition of which this particular definition is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2325 children.add(new Property("replaces", "canonical(ChargeItemDefinition)", "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2326 children.add(new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, status)); 2327 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2328 children.add(new Property("date", "dateTime", "The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 0, 1, date)); 2329 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.", 0, 1, publisher)); 2330 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2331 children.add(new Property("description", "markdown", "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, description)); 2332 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2333 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the charge item definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2334 children.add(new Property("purpose", "markdown", "Explanation of why this charge item definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2335 children.add(new Property("copyright", "markdown", "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 0, 1, copyright)); 2336 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2337 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 2338 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 2339 children.add(new Property("code", "CodeableConcept", "The defined billing details in this resource pertain to the given billing code.", 0, 1, code)); 2340 children.add(new Property("instance", "Reference(Medication|Substance|Device|DeviceDefinition|ActivityDefinition|PlanDefinition|HealthcareService)", "The defined billing details in this resource pertain to the given product instance(s).", 0, java.lang.Integer.MAX_VALUE, instance)); 2341 children.add(new Property("applicability", "", "Expressions that describe applicability criteria for the billing code.", 0, java.lang.Integer.MAX_VALUE, applicability)); 2342 children.add(new Property("propertyGroup", "", "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 0, java.lang.Integer.MAX_VALUE, propertyGroup)); 2343 } 2344 2345 @Override 2346 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2347 switch (_hash) { 2348 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 0, 1, url); 2349 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2350 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version); 2351 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2352 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2353 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2354 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2355 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2356 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title); 2357 case -1076333435: /*derivedFromUri*/ return new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 2358 case -995410646: /*partOf*/ return new Property("partOf", "canonical(ChargeItemDefinition)", "A larger definition of which this particular definition is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2359 case -430332865: /*replaces*/ return new Property("replaces", "canonical(ChargeItemDefinition)", "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 0, java.lang.Integer.MAX_VALUE, replaces); 2360 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, status); 2361 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2362 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 0, 1, date); 2363 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.", 0, 1, publisher); 2364 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2365 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, description); 2366 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2367 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the charge item definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2368 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this charge item definition is needed and why it has been designed as it has.", 0, 1, purpose); 2369 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 0, 1, copyright); 2370 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2371 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 2372 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 2373 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The defined billing details in this resource pertain to the given billing code.", 0, 1, code); 2374 case 555127957: /*instance*/ return new Property("instance", "Reference(Medication|Substance|Device|DeviceDefinition|ActivityDefinition|PlanDefinition|HealthcareService)", "The defined billing details in this resource pertain to the given product instance(s).", 0, java.lang.Integer.MAX_VALUE, instance); 2375 case -1526770491: /*applicability*/ return new Property("applicability", "", "Expressions that describe applicability criteria for the billing code.", 0, java.lang.Integer.MAX_VALUE, applicability); 2376 case -1041594966: /*propertyGroup*/ return new Property("propertyGroup", "", "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 0, java.lang.Integer.MAX_VALUE, propertyGroup); 2377 default: return super.getNamedProperty(_hash, _name, _checkValid); 2378 } 2379 2380 } 2381 2382 @Override 2383 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2384 switch (hash) { 2385 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2386 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2387 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2388 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2389 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2390 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2391 case -1076333435: /*derivedFromUri*/ return this.derivedFromUri == null ? new Base[0] : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 2392 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // CanonicalType 2393 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 2394 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2395 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2396 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2397 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2398 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2399 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2400 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2401 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2402 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2403 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2404 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2405 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 2406 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 2407 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2408 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // Reference 2409 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 2410 case -1041594966: /*propertyGroup*/ return this.propertyGroup == null ? new Base[0] : this.propertyGroup.toArray(new Base[this.propertyGroup.size()]); // ChargeItemDefinitionPropertyGroupComponent 2411 default: return super.getProperty(hash, name, checkValid); 2412 } 2413 2414 } 2415 2416 @Override 2417 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2418 switch (hash) { 2419 case 116079: // url 2420 this.url = TypeConvertor.castToUri(value); // UriType 2421 return value; 2422 case -1618432855: // identifier 2423 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2424 return value; 2425 case 351608024: // version 2426 this.version = TypeConvertor.castToString(value); // StringType 2427 return value; 2428 case 1508158071: // versionAlgorithm 2429 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2430 return value; 2431 case 3373707: // name 2432 this.name = TypeConvertor.castToString(value); // StringType 2433 return value; 2434 case 110371416: // title 2435 this.title = TypeConvertor.castToString(value); // StringType 2436 return value; 2437 case -1076333435: // derivedFromUri 2438 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); // UriType 2439 return value; 2440 case -995410646: // partOf 2441 this.getPartOf().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2442 return value; 2443 case -430332865: // replaces 2444 this.getReplaces().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2445 return value; 2446 case -892481550: // status 2447 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2448 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2449 return value; 2450 case -404562712: // experimental 2451 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2452 return value; 2453 case 3076014: // date 2454 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2455 return value; 2456 case 1447404028: // publisher 2457 this.publisher = TypeConvertor.castToString(value); // StringType 2458 return value; 2459 case 951526432: // contact 2460 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2461 return value; 2462 case -1724546052: // description 2463 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2464 return value; 2465 case -669707736: // useContext 2466 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2467 return value; 2468 case -507075711: // jurisdiction 2469 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2470 return value; 2471 case -220463842: // purpose 2472 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2473 return value; 2474 case 1522889671: // copyright 2475 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2476 return value; 2477 case 765157229: // copyrightLabel 2478 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2479 return value; 2480 case 223539345: // approvalDate 2481 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2482 return value; 2483 case -1687512484: // lastReviewDate 2484 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2485 return value; 2486 case 3059181: // code 2487 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2488 return value; 2489 case 555127957: // instance 2490 this.getInstance().add(TypeConvertor.castToReference(value)); // Reference 2491 return value; 2492 case -1526770491: // applicability 2493 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 2494 return value; 2495 case -1041594966: // propertyGroup 2496 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); // ChargeItemDefinitionPropertyGroupComponent 2497 return value; 2498 default: return super.setProperty(hash, name, value); 2499 } 2500 2501 } 2502 2503 @Override 2504 public Base setProperty(String name, Base value) throws FHIRException { 2505 if (name.equals("url")) { 2506 this.url = TypeConvertor.castToUri(value); // UriType 2507 } else if (name.equals("identifier")) { 2508 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2509 } else if (name.equals("version")) { 2510 this.version = TypeConvertor.castToString(value); // StringType 2511 } else if (name.equals("versionAlgorithm[x]")) { 2512 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2513 } else if (name.equals("name")) { 2514 this.name = TypeConvertor.castToString(value); // StringType 2515 } else if (name.equals("title")) { 2516 this.title = TypeConvertor.castToString(value); // StringType 2517 } else if (name.equals("derivedFromUri")) { 2518 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); 2519 } else if (name.equals("partOf")) { 2520 this.getPartOf().add(TypeConvertor.castToCanonical(value)); 2521 } else if (name.equals("replaces")) { 2522 this.getReplaces().add(TypeConvertor.castToCanonical(value)); 2523 } else if (name.equals("status")) { 2524 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2525 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2526 } else if (name.equals("experimental")) { 2527 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2528 } else if (name.equals("date")) { 2529 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2530 } else if (name.equals("publisher")) { 2531 this.publisher = TypeConvertor.castToString(value); // StringType 2532 } else if (name.equals("contact")) { 2533 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2534 } else if (name.equals("description")) { 2535 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2536 } else if (name.equals("useContext")) { 2537 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2538 } else if (name.equals("jurisdiction")) { 2539 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2540 } else if (name.equals("purpose")) { 2541 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2542 } else if (name.equals("copyright")) { 2543 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2544 } else if (name.equals("copyrightLabel")) { 2545 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2546 } else if (name.equals("approvalDate")) { 2547 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2548 } else if (name.equals("lastReviewDate")) { 2549 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2550 } else if (name.equals("code")) { 2551 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2552 } else if (name.equals("instance")) { 2553 this.getInstance().add(TypeConvertor.castToReference(value)); 2554 } else if (name.equals("applicability")) { 2555 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 2556 } else if (name.equals("propertyGroup")) { 2557 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); 2558 } else 2559 return super.setProperty(name, value); 2560 return value; 2561 } 2562 2563 @Override 2564 public Base makeProperty(int hash, String name) throws FHIRException { 2565 switch (hash) { 2566 case 116079: return getUrlElement(); 2567 case -1618432855: return addIdentifier(); 2568 case 351608024: return getVersionElement(); 2569 case -115699031: return getVersionAlgorithm(); 2570 case 1508158071: return getVersionAlgorithm(); 2571 case 3373707: return getNameElement(); 2572 case 110371416: return getTitleElement(); 2573 case -1076333435: return addDerivedFromUriElement(); 2574 case -995410646: return addPartOfElement(); 2575 case -430332865: return addReplacesElement(); 2576 case -892481550: return getStatusElement(); 2577 case -404562712: return getExperimentalElement(); 2578 case 3076014: return getDateElement(); 2579 case 1447404028: return getPublisherElement(); 2580 case 951526432: return addContact(); 2581 case -1724546052: return getDescriptionElement(); 2582 case -669707736: return addUseContext(); 2583 case -507075711: return addJurisdiction(); 2584 case -220463842: return getPurposeElement(); 2585 case 1522889671: return getCopyrightElement(); 2586 case 765157229: return getCopyrightLabelElement(); 2587 case 223539345: return getApprovalDateElement(); 2588 case -1687512484: return getLastReviewDateElement(); 2589 case 3059181: return getCode(); 2590 case 555127957: return addInstance(); 2591 case -1526770491: return addApplicability(); 2592 case -1041594966: return addPropertyGroup(); 2593 default: return super.makeProperty(hash, name); 2594 } 2595 2596 } 2597 2598 @Override 2599 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2600 switch (hash) { 2601 case 116079: /*url*/ return new String[] {"uri"}; 2602 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2603 case 351608024: /*version*/ return new String[] {"string"}; 2604 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2605 case 3373707: /*name*/ return new String[] {"string"}; 2606 case 110371416: /*title*/ return new String[] {"string"}; 2607 case -1076333435: /*derivedFromUri*/ return new String[] {"uri"}; 2608 case -995410646: /*partOf*/ return new String[] {"canonical"}; 2609 case -430332865: /*replaces*/ return new String[] {"canonical"}; 2610 case -892481550: /*status*/ return new String[] {"code"}; 2611 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2612 case 3076014: /*date*/ return new String[] {"dateTime"}; 2613 case 1447404028: /*publisher*/ return new String[] {"string"}; 2614 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2615 case -1724546052: /*description*/ return new String[] {"markdown"}; 2616 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2617 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2618 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2619 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2620 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2621 case 223539345: /*approvalDate*/ return new String[] {"date"}; 2622 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 2623 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2624 case 555127957: /*instance*/ return new String[] {"Reference"}; 2625 case -1526770491: /*applicability*/ return new String[] {}; 2626 case -1041594966: /*propertyGroup*/ return new String[] {}; 2627 default: return super.getTypesForProperty(hash, name); 2628 } 2629 2630 } 2631 2632 @Override 2633 public Base addChild(String name) throws FHIRException { 2634 if (name.equals("url")) { 2635 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.url"); 2636 } 2637 else if (name.equals("identifier")) { 2638 return addIdentifier(); 2639 } 2640 else if (name.equals("version")) { 2641 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.version"); 2642 } 2643 else if (name.equals("versionAlgorithmString")) { 2644 this.versionAlgorithm = new StringType(); 2645 return this.versionAlgorithm; 2646 } 2647 else if (name.equals("versionAlgorithmCoding")) { 2648 this.versionAlgorithm = new Coding(); 2649 return this.versionAlgorithm; 2650 } 2651 else if (name.equals("name")) { 2652 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.name"); 2653 } 2654 else if (name.equals("title")) { 2655 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.title"); 2656 } 2657 else if (name.equals("derivedFromUri")) { 2658 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.derivedFromUri"); 2659 } 2660 else if (name.equals("partOf")) { 2661 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.partOf"); 2662 } 2663 else if (name.equals("replaces")) { 2664 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.replaces"); 2665 } 2666 else if (name.equals("status")) { 2667 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.status"); 2668 } 2669 else if (name.equals("experimental")) { 2670 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.experimental"); 2671 } 2672 else if (name.equals("date")) { 2673 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.date"); 2674 } 2675 else if (name.equals("publisher")) { 2676 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.publisher"); 2677 } 2678 else if (name.equals("contact")) { 2679 return addContact(); 2680 } 2681 else if (name.equals("description")) { 2682 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.description"); 2683 } 2684 else if (name.equals("useContext")) { 2685 return addUseContext(); 2686 } 2687 else if (name.equals("jurisdiction")) { 2688 return addJurisdiction(); 2689 } 2690 else if (name.equals("purpose")) { 2691 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.purpose"); 2692 } 2693 else if (name.equals("copyright")) { 2694 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.copyright"); 2695 } 2696 else if (name.equals("copyrightLabel")) { 2697 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.copyrightLabel"); 2698 } 2699 else if (name.equals("approvalDate")) { 2700 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.approvalDate"); 2701 } 2702 else if (name.equals("lastReviewDate")) { 2703 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.lastReviewDate"); 2704 } 2705 else if (name.equals("code")) { 2706 this.code = new CodeableConcept(); 2707 return this.code; 2708 } 2709 else if (name.equals("instance")) { 2710 return addInstance(); 2711 } 2712 else if (name.equals("applicability")) { 2713 return addApplicability(); 2714 } 2715 else if (name.equals("propertyGroup")) { 2716 return addPropertyGroup(); 2717 } 2718 else 2719 return super.addChild(name); 2720 } 2721 2722 public String fhirType() { 2723 return "ChargeItemDefinition"; 2724 2725 } 2726 2727 public ChargeItemDefinition copy() { 2728 ChargeItemDefinition dst = new ChargeItemDefinition(); 2729 copyValues(dst); 2730 return dst; 2731 } 2732 2733 public void copyValues(ChargeItemDefinition dst) { 2734 super.copyValues(dst); 2735 dst.url = url == null ? null : url.copy(); 2736 if (identifier != null) { 2737 dst.identifier = new ArrayList<Identifier>(); 2738 for (Identifier i : identifier) 2739 dst.identifier.add(i.copy()); 2740 }; 2741 dst.version = version == null ? null : version.copy(); 2742 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2743 dst.name = name == null ? null : name.copy(); 2744 dst.title = title == null ? null : title.copy(); 2745 if (derivedFromUri != null) { 2746 dst.derivedFromUri = new ArrayList<UriType>(); 2747 for (UriType i : derivedFromUri) 2748 dst.derivedFromUri.add(i.copy()); 2749 }; 2750 if (partOf != null) { 2751 dst.partOf = new ArrayList<CanonicalType>(); 2752 for (CanonicalType i : partOf) 2753 dst.partOf.add(i.copy()); 2754 }; 2755 if (replaces != null) { 2756 dst.replaces = new ArrayList<CanonicalType>(); 2757 for (CanonicalType i : replaces) 2758 dst.replaces.add(i.copy()); 2759 }; 2760 dst.status = status == null ? null : status.copy(); 2761 dst.experimental = experimental == null ? null : experimental.copy(); 2762 dst.date = date == null ? null : date.copy(); 2763 dst.publisher = publisher == null ? null : publisher.copy(); 2764 if (contact != null) { 2765 dst.contact = new ArrayList<ContactDetail>(); 2766 for (ContactDetail i : contact) 2767 dst.contact.add(i.copy()); 2768 }; 2769 dst.description = description == null ? null : description.copy(); 2770 if (useContext != null) { 2771 dst.useContext = new ArrayList<UsageContext>(); 2772 for (UsageContext i : useContext) 2773 dst.useContext.add(i.copy()); 2774 }; 2775 if (jurisdiction != null) { 2776 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2777 for (CodeableConcept i : jurisdiction) 2778 dst.jurisdiction.add(i.copy()); 2779 }; 2780 dst.purpose = purpose == null ? null : purpose.copy(); 2781 dst.copyright = copyright == null ? null : copyright.copy(); 2782 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2783 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2784 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2785 dst.code = code == null ? null : code.copy(); 2786 if (instance != null) { 2787 dst.instance = new ArrayList<Reference>(); 2788 for (Reference i : instance) 2789 dst.instance.add(i.copy()); 2790 }; 2791 if (applicability != null) { 2792 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2793 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 2794 dst.applicability.add(i.copy()); 2795 }; 2796 if (propertyGroup != null) { 2797 dst.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2798 for (ChargeItemDefinitionPropertyGroupComponent i : propertyGroup) 2799 dst.propertyGroup.add(i.copy()); 2800 }; 2801 } 2802 2803 protected ChargeItemDefinition typedCopy() { 2804 return copy(); 2805 } 2806 2807 @Override 2808 public boolean equalsDeep(Base other_) { 2809 if (!super.equalsDeep(other_)) 2810 return false; 2811 if (!(other_ instanceof ChargeItemDefinition)) 2812 return false; 2813 ChargeItemDefinition o = (ChargeItemDefinition) other_; 2814 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2815 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2816 && compareDeep(derivedFromUri, o.derivedFromUri, true) && compareDeep(partOf, o.partOf, true) && compareDeep(replaces, o.replaces, true) 2817 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 2818 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 2819 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 2820 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 2821 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 2822 && compareDeep(code, o.code, true) && compareDeep(instance, o.instance, true) && compareDeep(applicability, o.applicability, true) 2823 && compareDeep(propertyGroup, o.propertyGroup, true); 2824 } 2825 2826 @Override 2827 public boolean equalsShallow(Base other_) { 2828 if (!super.equalsShallow(other_)) 2829 return false; 2830 if (!(other_ instanceof ChargeItemDefinition)) 2831 return false; 2832 ChargeItemDefinition o = (ChargeItemDefinition) other_; 2833 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2834 && compareValues(title, o.title, true) && compareValues(derivedFromUri, o.derivedFromUri, true) && compareValues(partOf, o.partOf, true) 2835 && compareValues(replaces, o.replaces, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 2836 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 2837 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 2838 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 2839 ; 2840 } 2841 2842 public boolean isEmpty() { 2843 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2844 , versionAlgorithm, name, title, derivedFromUri, partOf, replaces, status, experimental 2845 , date, publisher, contact, description, useContext, jurisdiction, purpose, copyright 2846 , copyrightLabel, approvalDate, lastReviewDate, code, instance, applicability, propertyGroup 2847 ); 2848 } 2849 2850 @Override 2851 public ResourceType getResourceType() { 2852 return ResourceType.ChargeItemDefinition; 2853 } 2854 2855 /** 2856 * Search parameter: <b>context-quantity</b> 2857 * <p> 2858 * Description: <b>Multiple Resources: 2859 2860* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2861* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2862* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2863* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2864* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2865* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2866* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2867* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2868* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2869* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2870* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2871* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2872* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2873* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2874* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2875* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2876* [Library](library.html): A quantity- or range-valued use context assigned to the library 2877* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2878* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2879* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2880* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2881* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2882* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2883* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2884* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2885* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2886* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2887* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2888* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2889* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2890</b><br> 2891 * Type: <b>quantity</b><br> 2892 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2893 * </p> 2894 */ 2895 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2896 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2897 /** 2898 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2899 * <p> 2900 * Description: <b>Multiple Resources: 2901 2902* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2903* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2904* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2905* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2906* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2907* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2908* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2909* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2910* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2911* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2912* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2913* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2914* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2915* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2916* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2917* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2918* [Library](library.html): A quantity- or range-valued use context assigned to the library 2919* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2920* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2921* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2922* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2923* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2924* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2925* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2926* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2927* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2928* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2929* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2930* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2931* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2932</b><br> 2933 * Type: <b>quantity</b><br> 2934 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2935 * </p> 2936 */ 2937 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2938 2939 /** 2940 * Search parameter: <b>context-type-quantity</b> 2941 * <p> 2942 * Description: <b>Multiple Resources: 2943 2944* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2945* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2946* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2947* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2948* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2949* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2950* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2951* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2952* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2953* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2954* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2955* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2956* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2957* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2958* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2959* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2960* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2961* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2962* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2963* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2964* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2965* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2966* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2967* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2968* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2969* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2970* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2971* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2972* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2973* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2974</b><br> 2975 * Type: <b>composite</b><br> 2976 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2977 * </p> 2978 */ 2979 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2980 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2981 /** 2982 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2983 * <p> 2984 * Description: <b>Multiple Resources: 2985 2986* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2987* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2988* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2989* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2990* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2991* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2992* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2993* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2994* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2995* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2996* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2997* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2998* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2999* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3000* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3001* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3002* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3003* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3004* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3005* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3006* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3007* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3008* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3009* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3010* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3011* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3012* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3013* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3014* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3015* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3016</b><br> 3017 * Type: <b>composite</b><br> 3018 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3019 * </p> 3020 */ 3021 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3022 3023 /** 3024 * Search parameter: <b>context-type-value</b> 3025 * <p> 3026 * Description: <b>Multiple Resources: 3027 3028* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3029* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3030* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3031* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3032* [Citation](citation.html): A use context type and value assigned to the citation 3033* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3034* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3035* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3036* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3037* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3038* [Evidence](evidence.html): A use context type and value assigned to the evidence 3039* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3040* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3041* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3042* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3043* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3044* [Library](library.html): A use context type and value assigned to the library 3045* [Measure](measure.html): A use context type and value assigned to the measure 3046* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3047* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3048* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3049* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3050* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3051* [Requirements](requirements.html): A use context type and value assigned to the requirements 3052* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3053* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3054* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3055* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3056* [TestScript](testscript.html): A use context type and value assigned to the test script 3057* [ValueSet](valueset.html): A use context type and value assigned to the value set 3058</b><br> 3059 * Type: <b>composite</b><br> 3060 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3061 * </p> 3062 */ 3063 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3064 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3065 /** 3066 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3067 * <p> 3068 * Description: <b>Multiple Resources: 3069 3070* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3071* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3072* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3073* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3074* [Citation](citation.html): A use context type and value assigned to the citation 3075* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3076* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3077* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3078* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3079* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3080* [Evidence](evidence.html): A use context type and value assigned to the evidence 3081* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3082* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3083* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3084* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3085* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3086* [Library](library.html): A use context type and value assigned to the library 3087* [Measure](measure.html): A use context type and value assigned to the measure 3088* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3089* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3090* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3091* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3092* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3093* [Requirements](requirements.html): A use context type and value assigned to the requirements 3094* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3095* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3096* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3097* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3098* [TestScript](testscript.html): A use context type and value assigned to the test script 3099* [ValueSet](valueset.html): A use context type and value assigned to the value set 3100</b><br> 3101 * Type: <b>composite</b><br> 3102 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3103 * </p> 3104 */ 3105 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3106 3107 /** 3108 * Search parameter: <b>context-type</b> 3109 * <p> 3110 * Description: <b>Multiple Resources: 3111 3112* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3113* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3114* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3115* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3116* [Citation](citation.html): A type of use context assigned to the citation 3117* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3118* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3119* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3120* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3121* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3122* [Evidence](evidence.html): A type of use context assigned to the evidence 3123* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3124* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3125* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3126* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3127* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3128* [Library](library.html): A type of use context assigned to the library 3129* [Measure](measure.html): A type of use context assigned to the measure 3130* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3131* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3132* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3133* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3134* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3135* [Requirements](requirements.html): A type of use context assigned to the requirements 3136* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3137* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3138* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3139* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3140* [TestScript](testscript.html): A type of use context assigned to the test script 3141* [ValueSet](valueset.html): A type of use context assigned to the value set 3142</b><br> 3143 * Type: <b>token</b><br> 3144 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3145 * </p> 3146 */ 3147 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3148 public static final String SP_CONTEXT_TYPE = "context-type"; 3149 /** 3150 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3151 * <p> 3152 * Description: <b>Multiple Resources: 3153 3154* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3155* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3156* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3157* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3158* [Citation](citation.html): A type of use context assigned to the citation 3159* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3160* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3161* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3162* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3163* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3164* [Evidence](evidence.html): A type of use context assigned to the evidence 3165* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3166* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3167* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3168* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3169* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3170* [Library](library.html): A type of use context assigned to the library 3171* [Measure](measure.html): A type of use context assigned to the measure 3172* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3173* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3174* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3175* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3176* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3177* [Requirements](requirements.html): A type of use context assigned to the requirements 3178* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3179* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3180* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3181* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3182* [TestScript](testscript.html): A type of use context assigned to the test script 3183* [ValueSet](valueset.html): A type of use context assigned to the value set 3184</b><br> 3185 * Type: <b>token</b><br> 3186 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3187 * </p> 3188 */ 3189 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3190 3191 /** 3192 * Search parameter: <b>context</b> 3193 * <p> 3194 * Description: <b>Multiple Resources: 3195 3196* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3197* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3198* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3199* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3200* [Citation](citation.html): A use context assigned to the citation 3201* [CodeSystem](codesystem.html): A use context assigned to the code system 3202* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3203* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3204* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3205* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3206* [Evidence](evidence.html): A use context assigned to the evidence 3207* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3208* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3209* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3210* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3211* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3212* [Library](library.html): A use context assigned to the library 3213* [Measure](measure.html): A use context assigned to the measure 3214* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3215* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3216* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3217* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3218* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3219* [Requirements](requirements.html): A use context assigned to the requirements 3220* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3221* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3222* [StructureMap](structuremap.html): A use context assigned to the structure map 3223* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3224* [TestScript](testscript.html): A use context assigned to the test script 3225* [ValueSet](valueset.html): A use context assigned to the value set 3226</b><br> 3227 * Type: <b>token</b><br> 3228 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3229 * </p> 3230 */ 3231 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3232 public static final String SP_CONTEXT = "context"; 3233 /** 3234 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3235 * <p> 3236 * Description: <b>Multiple Resources: 3237 3238* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3239* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3240* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3241* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3242* [Citation](citation.html): A use context assigned to the citation 3243* [CodeSystem](codesystem.html): A use context assigned to the code system 3244* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3245* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3246* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3247* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3248* [Evidence](evidence.html): A use context assigned to the evidence 3249* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3250* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3251* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3252* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3253* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3254* [Library](library.html): A use context assigned to the library 3255* [Measure](measure.html): A use context assigned to the measure 3256* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3257* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3258* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3259* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3260* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3261* [Requirements](requirements.html): A use context assigned to the requirements 3262* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3263* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3264* [StructureMap](structuremap.html): A use context assigned to the structure map 3265* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3266* [TestScript](testscript.html): A use context assigned to the test script 3267* [ValueSet](valueset.html): A use context assigned to the value set 3268</b><br> 3269 * Type: <b>token</b><br> 3270 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3271 * </p> 3272 */ 3273 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3274 3275 /** 3276 * Search parameter: <b>date</b> 3277 * <p> 3278 * Description: <b>Multiple Resources: 3279 3280* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3281* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3282* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3283* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3284* [Citation](citation.html): The citation publication date 3285* [CodeSystem](codesystem.html): The code system publication date 3286* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3287* [ConceptMap](conceptmap.html): The concept map publication date 3288* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3289* [EventDefinition](eventdefinition.html): The event definition publication date 3290* [Evidence](evidence.html): The evidence publication date 3291* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3292* [ExampleScenario](examplescenario.html): The example scenario publication date 3293* [GraphDefinition](graphdefinition.html): The graph definition publication date 3294* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3295* [Library](library.html): The library publication date 3296* [Measure](measure.html): The measure publication date 3297* [MessageDefinition](messagedefinition.html): The message definition publication date 3298* [NamingSystem](namingsystem.html): The naming system publication date 3299* [OperationDefinition](operationdefinition.html): The operation definition publication date 3300* [PlanDefinition](plandefinition.html): The plan definition publication date 3301* [Questionnaire](questionnaire.html): The questionnaire publication date 3302* [Requirements](requirements.html): The requirements publication date 3303* [SearchParameter](searchparameter.html): The search parameter publication date 3304* [StructureDefinition](structuredefinition.html): The structure definition publication date 3305* [StructureMap](structuremap.html): The structure map publication date 3306* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3307* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3308* [TestScript](testscript.html): The test script publication date 3309* [ValueSet](valueset.html): The value set publication date 3310</b><br> 3311 * Type: <b>date</b><br> 3312 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3313 * </p> 3314 */ 3315 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3316 public static final String SP_DATE = "date"; 3317 /** 3318 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3319 * <p> 3320 * Description: <b>Multiple Resources: 3321 3322* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3323* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3324* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3325* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3326* [Citation](citation.html): The citation publication date 3327* [CodeSystem](codesystem.html): The code system publication date 3328* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3329* [ConceptMap](conceptmap.html): The concept map publication date 3330* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3331* [EventDefinition](eventdefinition.html): The event definition publication date 3332* [Evidence](evidence.html): The evidence publication date 3333* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3334* [ExampleScenario](examplescenario.html): The example scenario publication date 3335* [GraphDefinition](graphdefinition.html): The graph definition publication date 3336* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3337* [Library](library.html): The library publication date 3338* [Measure](measure.html): The measure publication date 3339* [MessageDefinition](messagedefinition.html): The message definition publication date 3340* [NamingSystem](namingsystem.html): The naming system publication date 3341* [OperationDefinition](operationdefinition.html): The operation definition publication date 3342* [PlanDefinition](plandefinition.html): The plan definition publication date 3343* [Questionnaire](questionnaire.html): The questionnaire publication date 3344* [Requirements](requirements.html): The requirements publication date 3345* [SearchParameter](searchparameter.html): The search parameter publication date 3346* [StructureDefinition](structuredefinition.html): The structure definition publication date 3347* [StructureMap](structuremap.html): The structure map publication date 3348* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3349* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3350* [TestScript](testscript.html): The test script publication date 3351* [ValueSet](valueset.html): The value set publication date 3352</b><br> 3353 * Type: <b>date</b><br> 3354 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3355 * </p> 3356 */ 3357 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3358 3359 /** 3360 * Search parameter: <b>description</b> 3361 * <p> 3362 * Description: <b>Multiple Resources: 3363 3364* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3365* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3366* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3367* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3368* [Citation](citation.html): The description of the citation 3369* [CodeSystem](codesystem.html): The description of the code system 3370* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3371* [ConceptMap](conceptmap.html): The description of the concept map 3372* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3373* [EventDefinition](eventdefinition.html): The description of the event definition 3374* [Evidence](evidence.html): The description of the evidence 3375* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3376* [GraphDefinition](graphdefinition.html): The description of the graph definition 3377* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3378* [Library](library.html): The description of the library 3379* [Measure](measure.html): The description of the measure 3380* [MessageDefinition](messagedefinition.html): The description of the message definition 3381* [NamingSystem](namingsystem.html): The description of the naming system 3382* [OperationDefinition](operationdefinition.html): The description of the operation definition 3383* [PlanDefinition](plandefinition.html): The description of the plan definition 3384* [Questionnaire](questionnaire.html): The description of the questionnaire 3385* [Requirements](requirements.html): The description of the requirements 3386* [SearchParameter](searchparameter.html): The description of the search parameter 3387* [StructureDefinition](structuredefinition.html): The description of the structure definition 3388* [StructureMap](structuremap.html): The description of the structure map 3389* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3390* [TestScript](testscript.html): The description of the test script 3391* [ValueSet](valueset.html): The description of the value set 3392</b><br> 3393 * Type: <b>string</b><br> 3394 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3395 * </p> 3396 */ 3397 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3398 public static final String SP_DESCRIPTION = "description"; 3399 /** 3400 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3401 * <p> 3402 * Description: <b>Multiple Resources: 3403 3404* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3405* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3406* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3407* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3408* [Citation](citation.html): The description of the citation 3409* [CodeSystem](codesystem.html): The description of the code system 3410* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3411* [ConceptMap](conceptmap.html): The description of the concept map 3412* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3413* [EventDefinition](eventdefinition.html): The description of the event definition 3414* [Evidence](evidence.html): The description of the evidence 3415* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3416* [GraphDefinition](graphdefinition.html): The description of the graph definition 3417* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3418* [Library](library.html): The description of the library 3419* [Measure](measure.html): The description of the measure 3420* [MessageDefinition](messagedefinition.html): The description of the message definition 3421* [NamingSystem](namingsystem.html): The description of the naming system 3422* [OperationDefinition](operationdefinition.html): The description of the operation definition 3423* [PlanDefinition](plandefinition.html): The description of the plan definition 3424* [Questionnaire](questionnaire.html): The description of the questionnaire 3425* [Requirements](requirements.html): The description of the requirements 3426* [SearchParameter](searchparameter.html): The description of the search parameter 3427* [StructureDefinition](structuredefinition.html): The description of the structure definition 3428* [StructureMap](structuremap.html): The description of the structure map 3429* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3430* [TestScript](testscript.html): The description of the test script 3431* [ValueSet](valueset.html): The description of the value set 3432</b><br> 3433 * Type: <b>string</b><br> 3434 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3435 * </p> 3436 */ 3437 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3438 3439 /** 3440 * Search parameter: <b>identifier</b> 3441 * <p> 3442 * Description: <b>Multiple Resources: 3443 3444* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3445* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3446* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3447* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3448* [Citation](citation.html): External identifier for the citation 3449* [CodeSystem](codesystem.html): External identifier for the code system 3450* [ConceptMap](conceptmap.html): External identifier for the concept map 3451* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3452* [EventDefinition](eventdefinition.html): External identifier for the event definition 3453* [Evidence](evidence.html): External identifier for the evidence 3454* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3455* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3456* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3457* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3458* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3459* [Library](library.html): External identifier for the library 3460* [Measure](measure.html): External identifier for the measure 3461* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3462* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3463* [NamingSystem](namingsystem.html): External identifier for the naming system 3464* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3465* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3466* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3467* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3468* [Requirements](requirements.html): External identifier for the requirements 3469* [SearchParameter](searchparameter.html): External identifier for the search parameter 3470* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3471* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3472* [StructureMap](structuremap.html): External identifier for the structure map 3473* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3474* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3475* [TestPlan](testplan.html): An identifier for the test plan 3476* [TestScript](testscript.html): External identifier for the test script 3477* [ValueSet](valueset.html): External identifier for the value set 3478</b><br> 3479 * Type: <b>token</b><br> 3480 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3481 * </p> 3482 */ 3483 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3484 public static final String SP_IDENTIFIER = "identifier"; 3485 /** 3486 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3487 * <p> 3488 * Description: <b>Multiple Resources: 3489 3490* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3491* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3492* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3493* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3494* [Citation](citation.html): External identifier for the citation 3495* [CodeSystem](codesystem.html): External identifier for the code system 3496* [ConceptMap](conceptmap.html): External identifier for the concept map 3497* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3498* [EventDefinition](eventdefinition.html): External identifier for the event definition 3499* [Evidence](evidence.html): External identifier for the evidence 3500* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3501* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3502* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3503* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3504* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3505* [Library](library.html): External identifier for the library 3506* [Measure](measure.html): External identifier for the measure 3507* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3508* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3509* [NamingSystem](namingsystem.html): External identifier for the naming system 3510* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3511* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3512* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3513* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3514* [Requirements](requirements.html): External identifier for the requirements 3515* [SearchParameter](searchparameter.html): External identifier for the search parameter 3516* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3517* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3518* [StructureMap](structuremap.html): External identifier for the structure map 3519* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3520* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3521* [TestPlan](testplan.html): An identifier for the test plan 3522* [TestScript](testscript.html): External identifier for the test script 3523* [ValueSet](valueset.html): External identifier for the value set 3524</b><br> 3525 * Type: <b>token</b><br> 3526 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3527 * </p> 3528 */ 3529 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3530 3531 /** 3532 * Search parameter: <b>jurisdiction</b> 3533 * <p> 3534 * Description: <b>Multiple Resources: 3535 3536* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3537* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3538* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3539* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3540* [Citation](citation.html): Intended jurisdiction for the citation 3541* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3542* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3543* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3544* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3545* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3546* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3547* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3548* [Library](library.html): Intended jurisdiction for the library 3549* [Measure](measure.html): Intended jurisdiction for the measure 3550* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3551* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3552* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3553* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3554* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3555* [Requirements](requirements.html): Intended jurisdiction for the requirements 3556* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3557* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3558* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3559* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3560* [TestScript](testscript.html): Intended jurisdiction for the test script 3561* [ValueSet](valueset.html): Intended jurisdiction for the value set 3562</b><br> 3563 * Type: <b>token</b><br> 3564 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3565 * </p> 3566 */ 3567 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3568 public static final String SP_JURISDICTION = "jurisdiction"; 3569 /** 3570 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3571 * <p> 3572 * Description: <b>Multiple Resources: 3573 3574* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3575* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3576* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3577* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3578* [Citation](citation.html): Intended jurisdiction for the citation 3579* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3580* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3581* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3582* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3583* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3584* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3585* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3586* [Library](library.html): Intended jurisdiction for the library 3587* [Measure](measure.html): Intended jurisdiction for the measure 3588* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3589* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3590* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3591* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3592* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3593* [Requirements](requirements.html): Intended jurisdiction for the requirements 3594* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3595* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3596* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3597* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3598* [TestScript](testscript.html): Intended jurisdiction for the test script 3599* [ValueSet](valueset.html): Intended jurisdiction for the value set 3600</b><br> 3601 * Type: <b>token</b><br> 3602 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3603 * </p> 3604 */ 3605 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3606 3607 /** 3608 * Search parameter: <b>publisher</b> 3609 * <p> 3610 * Description: <b>Multiple Resources: 3611 3612* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3613* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3614* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3615* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3616* [Citation](citation.html): Name of the publisher of the citation 3617* [CodeSystem](codesystem.html): Name of the publisher of the code system 3618* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3619* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3620* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3621* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3622* [Evidence](evidence.html): Name of the publisher of the evidence 3623* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3624* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3625* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3626* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3627* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3628* [Library](library.html): Name of the publisher of the library 3629* [Measure](measure.html): Name of the publisher of the measure 3630* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3631* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3632* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3633* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3634* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3635* [Requirements](requirements.html): Name of the publisher of the requirements 3636* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3637* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3638* [StructureMap](structuremap.html): Name of the publisher of the structure map 3639* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3640* [TestScript](testscript.html): Name of the publisher of the test script 3641* [ValueSet](valueset.html): Name of the publisher of the value set 3642</b><br> 3643 * Type: <b>string</b><br> 3644 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3645 * </p> 3646 */ 3647 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3648 public static final String SP_PUBLISHER = "publisher"; 3649 /** 3650 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3651 * <p> 3652 * Description: <b>Multiple Resources: 3653 3654* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3655* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3656* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3657* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3658* [Citation](citation.html): Name of the publisher of the citation 3659* [CodeSystem](codesystem.html): Name of the publisher of the code system 3660* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3661* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3662* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3663* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3664* [Evidence](evidence.html): Name of the publisher of the evidence 3665* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3666* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3667* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3668* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3669* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3670* [Library](library.html): Name of the publisher of the library 3671* [Measure](measure.html): Name of the publisher of the measure 3672* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3673* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3674* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3675* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3676* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3677* [Requirements](requirements.html): Name of the publisher of the requirements 3678* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3679* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3680* [StructureMap](structuremap.html): Name of the publisher of the structure map 3681* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3682* [TestScript](testscript.html): Name of the publisher of the test script 3683* [ValueSet](valueset.html): Name of the publisher of the value set 3684</b><br> 3685 * Type: <b>string</b><br> 3686 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3687 * </p> 3688 */ 3689 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3690 3691 /** 3692 * Search parameter: <b>status</b> 3693 * <p> 3694 * Description: <b>Multiple Resources: 3695 3696* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3697* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3698* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3699* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3700* [Citation](citation.html): The current status of the citation 3701* [CodeSystem](codesystem.html): The current status of the code system 3702* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3703* [ConceptMap](conceptmap.html): The current status of the concept map 3704* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3705* [EventDefinition](eventdefinition.html): The current status of the event definition 3706* [Evidence](evidence.html): The current status of the evidence 3707* [EvidenceReport](evidencereport.html): The current status of the evidence report 3708* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3709* [ExampleScenario](examplescenario.html): The current status of the example scenario 3710* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3711* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3712* [Library](library.html): The current status of the library 3713* [Measure](measure.html): The current status of the measure 3714* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3715* [MessageDefinition](messagedefinition.html): The current status of the message definition 3716* [NamingSystem](namingsystem.html): The current status of the naming system 3717* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3718* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3719* [PlanDefinition](plandefinition.html): The current status of the plan definition 3720* [Questionnaire](questionnaire.html): The current status of the questionnaire 3721* [Requirements](requirements.html): The current status of the requirements 3722* [SearchParameter](searchparameter.html): The current status of the search parameter 3723* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3724* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3725* [StructureMap](structuremap.html): The current status of the structure map 3726* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3727* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3728* [TestPlan](testplan.html): The current status of the test plan 3729* [TestScript](testscript.html): The current status of the test script 3730* [ValueSet](valueset.html): The current status of the value set 3731</b><br> 3732 * Type: <b>token</b><br> 3733 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3734 * </p> 3735 */ 3736 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3737 public static final String SP_STATUS = "status"; 3738 /** 3739 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3740 * <p> 3741 * Description: <b>Multiple Resources: 3742 3743* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3744* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3745* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3746* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3747* [Citation](citation.html): The current status of the citation 3748* [CodeSystem](codesystem.html): The current status of the code system 3749* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3750* [ConceptMap](conceptmap.html): The current status of the concept map 3751* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3752* [EventDefinition](eventdefinition.html): The current status of the event definition 3753* [Evidence](evidence.html): The current status of the evidence 3754* [EvidenceReport](evidencereport.html): The current status of the evidence report 3755* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3756* [ExampleScenario](examplescenario.html): The current status of the example scenario 3757* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3758* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3759* [Library](library.html): The current status of the library 3760* [Measure](measure.html): The current status of the measure 3761* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3762* [MessageDefinition](messagedefinition.html): The current status of the message definition 3763* [NamingSystem](namingsystem.html): The current status of the naming system 3764* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3765* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3766* [PlanDefinition](plandefinition.html): The current status of the plan definition 3767* [Questionnaire](questionnaire.html): The current status of the questionnaire 3768* [Requirements](requirements.html): The current status of the requirements 3769* [SearchParameter](searchparameter.html): The current status of the search parameter 3770* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3771* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3772* [StructureMap](structuremap.html): The current status of the structure map 3773* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3774* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3775* [TestPlan](testplan.html): The current status of the test plan 3776* [TestScript](testscript.html): The current status of the test script 3777* [ValueSet](valueset.html): The current status of the value set 3778</b><br> 3779 * Type: <b>token</b><br> 3780 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3781 * </p> 3782 */ 3783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3784 3785 /** 3786 * Search parameter: <b>title</b> 3787 * <p> 3788 * Description: <b>Multiple Resources: 3789 3790* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3791* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3792* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3793* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3794* [Citation](citation.html): The human-friendly name of the citation 3795* [CodeSystem](codesystem.html): The human-friendly name of the code system 3796* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3797* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3798* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3799* [Evidence](evidence.html): The human-friendly name of the evidence 3800* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3801* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3802* [Library](library.html): The human-friendly name of the library 3803* [Measure](measure.html): The human-friendly name of the measure 3804* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3805* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3806* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3807* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3808* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3809* [Requirements](requirements.html): The human-friendly name of the requirements 3810* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3811* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3812* [StructureMap](structuremap.html): The human-friendly name of the structure map 3813* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3814* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3815* [TestScript](testscript.html): The human-friendly name of the test script 3816* [ValueSet](valueset.html): The human-friendly name of the value set 3817</b><br> 3818 * Type: <b>string</b><br> 3819 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3820 * </p> 3821 */ 3822 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 3823 public static final String SP_TITLE = "title"; 3824 /** 3825 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3826 * <p> 3827 * Description: <b>Multiple Resources: 3828 3829* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3830* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3831* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3832* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3833* [Citation](citation.html): The human-friendly name of the citation 3834* [CodeSystem](codesystem.html): The human-friendly name of the code system 3835* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3836* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3837* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3838* [Evidence](evidence.html): The human-friendly name of the evidence 3839* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3840* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3841* [Library](library.html): The human-friendly name of the library 3842* [Measure](measure.html): The human-friendly name of the measure 3843* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3844* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3845* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3846* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3847* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3848* [Requirements](requirements.html): The human-friendly name of the requirements 3849* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3850* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3851* [StructureMap](structuremap.html): The human-friendly name of the structure map 3852* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3853* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3854* [TestScript](testscript.html): The human-friendly name of the test script 3855* [ValueSet](valueset.html): The human-friendly name of the value set 3856</b><br> 3857 * Type: <b>string</b><br> 3858 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3859 * </p> 3860 */ 3861 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3862 3863 /** 3864 * Search parameter: <b>url</b> 3865 * <p> 3866 * Description: <b>Multiple Resources: 3867 3868* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3869* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3870* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3871* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3872* [Citation](citation.html): The uri that identifies the citation 3873* [CodeSystem](codesystem.html): The uri that identifies the code system 3874* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3875* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3876* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3877* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3878* [Evidence](evidence.html): The uri that identifies the evidence 3879* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3880* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3881* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3882* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3883* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3884* [Library](library.html): The uri that identifies the library 3885* [Measure](measure.html): The uri that identifies the measure 3886* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3887* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3888* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3889* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3890* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3891* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3892* [Requirements](requirements.html): The uri that identifies the requirements 3893* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3894* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3895* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3896* [StructureMap](structuremap.html): The uri that identifies the structure map 3897* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3898* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3899* [TestPlan](testplan.html): The uri that identifies the test plan 3900* [TestScript](testscript.html): The uri that identifies the test script 3901* [ValueSet](valueset.html): The uri that identifies the value set 3902</b><br> 3903 * Type: <b>uri</b><br> 3904 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3905 * </p> 3906 */ 3907 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3908 public static final String SP_URL = "url"; 3909 /** 3910 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3911 * <p> 3912 * Description: <b>Multiple Resources: 3913 3914* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3915* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3916* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3917* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3918* [Citation](citation.html): The uri that identifies the citation 3919* [CodeSystem](codesystem.html): The uri that identifies the code system 3920* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3921* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3922* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3923* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3924* [Evidence](evidence.html): The uri that identifies the evidence 3925* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3926* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3927* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3928* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3929* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3930* [Library](library.html): The uri that identifies the library 3931* [Measure](measure.html): The uri that identifies the measure 3932* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3933* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3934* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3935* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3936* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3937* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3938* [Requirements](requirements.html): The uri that identifies the requirements 3939* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3940* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3941* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3942* [StructureMap](structuremap.html): The uri that identifies the structure map 3943* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3944* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3945* [TestPlan](testplan.html): The uri that identifies the test plan 3946* [TestScript](testscript.html): The uri that identifies the test script 3947* [ValueSet](valueset.html): The uri that identifies the value set 3948</b><br> 3949 * Type: <b>uri</b><br> 3950 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3951 * </p> 3952 */ 3953 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3954 3955 /** 3956 * Search parameter: <b>version</b> 3957 * <p> 3958 * Description: <b>Multiple Resources: 3959 3960* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3961* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3962* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3963* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3964* [Citation](citation.html): The business version of the citation 3965* [CodeSystem](codesystem.html): The business version of the code system 3966* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3967* [ConceptMap](conceptmap.html): The business version of the concept map 3968* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3969* [EventDefinition](eventdefinition.html): The business version of the event definition 3970* [Evidence](evidence.html): The business version of the evidence 3971* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3972* [ExampleScenario](examplescenario.html): The business version of the example scenario 3973* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3974* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3975* [Library](library.html): The business version of the library 3976* [Measure](measure.html): The business version of the measure 3977* [MessageDefinition](messagedefinition.html): The business version of the message definition 3978* [NamingSystem](namingsystem.html): The business version of the naming system 3979* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3980* [PlanDefinition](plandefinition.html): The business version of the plan definition 3981* [Questionnaire](questionnaire.html): The business version of the questionnaire 3982* [Requirements](requirements.html): The business version of the requirements 3983* [SearchParameter](searchparameter.html): The business version of the search parameter 3984* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3985* [StructureMap](structuremap.html): The business version of the structure map 3986* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3987* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3988* [TestScript](testscript.html): The business version of the test script 3989* [ValueSet](valueset.html): The business version of the value set 3990</b><br> 3991 * Type: <b>token</b><br> 3992 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3993 * </p> 3994 */ 3995 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 3996 public static final String SP_VERSION = "version"; 3997 /** 3998 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3999 * <p> 4000 * Description: <b>Multiple Resources: 4001 4002* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4003* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4004* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4005* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4006* [Citation](citation.html): The business version of the citation 4007* [CodeSystem](codesystem.html): The business version of the code system 4008* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4009* [ConceptMap](conceptmap.html): The business version of the concept map 4010* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4011* [EventDefinition](eventdefinition.html): The business version of the event definition 4012* [Evidence](evidence.html): The business version of the evidence 4013* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4014* [ExampleScenario](examplescenario.html): The business version of the example scenario 4015* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4016* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4017* [Library](library.html): The business version of the library 4018* [Measure](measure.html): The business version of the measure 4019* [MessageDefinition](messagedefinition.html): The business version of the message definition 4020* [NamingSystem](namingsystem.html): The business version of the naming system 4021* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4022* [PlanDefinition](plandefinition.html): The business version of the plan definition 4023* [Questionnaire](questionnaire.html): The business version of the questionnaire 4024* [Requirements](requirements.html): The business version of the requirements 4025* [SearchParameter](searchparameter.html): The business version of the search parameter 4026* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4027* [StructureMap](structuremap.html): The business version of the structure map 4028* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4029* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4030* [TestScript](testscript.html): The business version of the test script 4031* [ValueSet](valueset.html): The business version of the value set 4032</b><br> 4033 * Type: <b>token</b><br> 4034 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4035 * </p> 4036 */ 4037 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4038 4039 /** 4040 * Search parameter: <b>effective</b> 4041 * <p> 4042 * Description: <b>Multiple Resources: 4043 4044* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4045* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4046* [Citation](citation.html): The time during which the citation is intended to be in use 4047* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4048* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4049* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4050* [Library](library.html): The time during which the library is intended to be in use 4051* [Measure](measure.html): The time during which the measure is intended to be in use 4052* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4053* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4054* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4055* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4056</b><br> 4057 * Type: <b>date</b><br> 4058 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4059 * </p> 4060 */ 4061 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 4062 public static final String SP_EFFECTIVE = "effective"; 4063 /** 4064 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4065 * <p> 4066 * Description: <b>Multiple Resources: 4067 4068* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4069* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4070* [Citation](citation.html): The time during which the citation is intended to be in use 4071* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4072* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4073* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4074* [Library](library.html): The time during which the library is intended to be in use 4075* [Measure](measure.html): The time during which the measure is intended to be in use 4076* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4077* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4078* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4079* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4080</b><br> 4081 * Type: <b>date</b><br> 4082 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4083 * </p> 4084 */ 4085 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4086 4087 4088} 4089