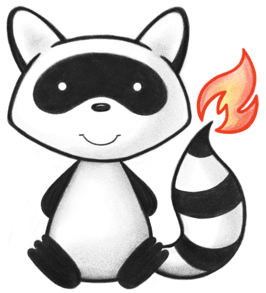
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system. 052 */ 053@ResourceDef(name="ChargeItemDefinition", profile="http://hl7.org/fhir/StructureDefinition/ChargeItemDefinition") 054public class ChargeItemDefinition extends MetadataResource { 055 056 @Block() 057 public static class ChargeItemDefinitionApplicabilityComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied. 060 */ 061 @Child(name = "condition", type = {Expression.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied." ) 063 protected Expression condition; 064 065 /** 066 * The period during which the charge item definition content was or is planned to be in active use. 067 */ 068 @Child(name = "effectivePeriod", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="When the charge item definition is expected to be used", formalDefinition="The period during which the charge item definition content was or is planned to be in active use." ) 070 protected Period effectivePeriod; 071 072 /** 073 * Reference to / quotation of the external source of the group of properties. 074 */ 075 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=3, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="Reference to / quotation of the external source of the group of properties", formalDefinition="Reference to / quotation of the external source of the group of properties." ) 077 protected RelatedArtifact relatedArtifact; 078 079 private static final long serialVersionUID = -1706427366L; 080 081 /** 082 * Constructor 083 */ 084 public ChargeItemDefinitionApplicabilityComponent() { 085 super(); 086 } 087 088 /** 089 * @return {@link #condition} (An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.) 090 */ 091 public Expression getCondition() { 092 if (this.condition == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.condition"); 095 else if (Configuration.doAutoCreate()) 096 this.condition = new Expression(); // cc 097 return this.condition; 098 } 099 100 public boolean hasCondition() { 101 return this.condition != null && !this.condition.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #condition} (An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.) 106 */ 107 public ChargeItemDefinitionApplicabilityComponent setCondition(Expression value) { 108 this.condition = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 114 */ 115 public Period getEffectivePeriod() { 116 if (this.effectivePeriod == null) 117 if (Configuration.errorOnAutoCreate()) 118 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.effectivePeriod"); 119 else if (Configuration.doAutoCreate()) 120 this.effectivePeriod = new Period(); // cc 121 return this.effectivePeriod; 122 } 123 124 public boolean hasEffectivePeriod() { 125 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 130 */ 131 public ChargeItemDefinitionApplicabilityComponent setEffectivePeriod(Period value) { 132 this.effectivePeriod = value; 133 return this; 134 } 135 136 /** 137 * @return {@link #relatedArtifact} (Reference to / quotation of the external source of the group of properties.) 138 */ 139 public RelatedArtifact getRelatedArtifact() { 140 if (this.relatedArtifact == null) 141 if (Configuration.errorOnAutoCreate()) 142 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.relatedArtifact"); 143 else if (Configuration.doAutoCreate()) 144 this.relatedArtifact = new RelatedArtifact(); // cc 145 return this.relatedArtifact; 146 } 147 148 public boolean hasRelatedArtifact() { 149 return this.relatedArtifact != null && !this.relatedArtifact.isEmpty(); 150 } 151 152 /** 153 * @param value {@link #relatedArtifact} (Reference to / quotation of the external source of the group of properties.) 154 */ 155 public ChargeItemDefinitionApplicabilityComponent setRelatedArtifact(RelatedArtifact value) { 156 this.relatedArtifact = value; 157 return this; 158 } 159 160 protected void listChildren(List<Property> children) { 161 super.listChildren(children); 162 children.add(new Property("condition", "Expression", "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 0, 1, condition)); 163 children.add(new Property("effectivePeriod", "Period", "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 164 children.add(new Property("relatedArtifact", "RelatedArtifact", "Reference to / quotation of the external source of the group of properties.", 0, 1, relatedArtifact)); 165 } 166 167 @Override 168 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 169 switch (_hash) { 170 case -861311717: /*condition*/ return new Property("condition", "Expression", "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 0, 1, condition); 171 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 172 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Reference to / quotation of the external source of the group of properties.", 0, 1, relatedArtifact); 173 default: return super.getNamedProperty(_hash, _name, _checkValid); 174 } 175 176 } 177 178 @Override 179 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 180 switch (hash) { 181 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Expression 182 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 183 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : new Base[] {this.relatedArtifact}; // RelatedArtifact 184 default: return super.getProperty(hash, name, checkValid); 185 } 186 187 } 188 189 @Override 190 public Base setProperty(int hash, String name, Base value) throws FHIRException { 191 switch (hash) { 192 case -861311717: // condition 193 this.condition = TypeConvertor.castToExpression(value); // Expression 194 return value; 195 case -403934648: // effectivePeriod 196 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 197 return value; 198 case 666807069: // relatedArtifact 199 this.relatedArtifact = TypeConvertor.castToRelatedArtifact(value); // RelatedArtifact 200 return value; 201 default: return super.setProperty(hash, name, value); 202 } 203 204 } 205 206 @Override 207 public Base setProperty(String name, Base value) throws FHIRException { 208 if (name.equals("condition")) { 209 this.condition = TypeConvertor.castToExpression(value); // Expression 210 } else if (name.equals("effectivePeriod")) { 211 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 212 } else if (name.equals("relatedArtifact")) { 213 this.relatedArtifact = TypeConvertor.castToRelatedArtifact(value); // RelatedArtifact 214 } else 215 return super.setProperty(name, value); 216 return value; 217 } 218 219 @Override 220 public void removeChild(String name, Base value) throws FHIRException { 221 if (name.equals("condition")) { 222 this.condition = null; 223 } else if (name.equals("effectivePeriod")) { 224 this.effectivePeriod = null; 225 } else if (name.equals("relatedArtifact")) { 226 this.relatedArtifact = null; 227 } else 228 super.removeChild(name, value); 229 230 } 231 232 @Override 233 public Base makeProperty(int hash, String name) throws FHIRException { 234 switch (hash) { 235 case -861311717: return getCondition(); 236 case -403934648: return getEffectivePeriod(); 237 case 666807069: return getRelatedArtifact(); 238 default: return super.makeProperty(hash, name); 239 } 240 241 } 242 243 @Override 244 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 245 switch (hash) { 246 case -861311717: /*condition*/ return new String[] {"Expression"}; 247 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 248 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 249 default: return super.getTypesForProperty(hash, name); 250 } 251 252 } 253 254 @Override 255 public Base addChild(String name) throws FHIRException { 256 if (name.equals("condition")) { 257 this.condition = new Expression(); 258 return this.condition; 259 } 260 else if (name.equals("effectivePeriod")) { 261 this.effectivePeriod = new Period(); 262 return this.effectivePeriod; 263 } 264 else if (name.equals("relatedArtifact")) { 265 this.relatedArtifact = new RelatedArtifact(); 266 return this.relatedArtifact; 267 } 268 else 269 return super.addChild(name); 270 } 271 272 public ChargeItemDefinitionApplicabilityComponent copy() { 273 ChargeItemDefinitionApplicabilityComponent dst = new ChargeItemDefinitionApplicabilityComponent(); 274 copyValues(dst); 275 return dst; 276 } 277 278 public void copyValues(ChargeItemDefinitionApplicabilityComponent dst) { 279 super.copyValues(dst); 280 dst.condition = condition == null ? null : condition.copy(); 281 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 282 dst.relatedArtifact = relatedArtifact == null ? null : relatedArtifact.copy(); 283 } 284 285 @Override 286 public boolean equalsDeep(Base other_) { 287 if (!super.equalsDeep(other_)) 288 return false; 289 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 290 return false; 291 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 292 return compareDeep(condition, o.condition, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 293 && compareDeep(relatedArtifact, o.relatedArtifact, true); 294 } 295 296 @Override 297 public boolean equalsShallow(Base other_) { 298 if (!super.equalsShallow(other_)) 299 return false; 300 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 301 return false; 302 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 303 return true; 304 } 305 306 public boolean isEmpty() { 307 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, effectivePeriod 308 , relatedArtifact); 309 } 310 311 public String fhirType() { 312 return "ChargeItemDefinition.applicability"; 313 314 } 315 316 } 317 318 @Block() 319 public static class ChargeItemDefinitionPropertyGroupComponent extends BackboneElement implements IBaseBackboneElement { 320 /** 321 * Expressions that describe applicability criteria for the priceComponent. 322 */ 323 @Child(name = "applicability", type = {ChargeItemDefinitionApplicabilityComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 324 @Description(shortDefinition="Conditions under which the priceComponent is applicable", formalDefinition="Expressions that describe applicability criteria for the priceComponent." ) 325 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 326 327 /** 328 * The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated. 329 */ 330 @Child(name = "priceComponent", type = {MonetaryComponent.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 331 @Description(shortDefinition="Components of total line item price", formalDefinition="The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated." ) 332 protected List<MonetaryComponent> priceComponent; 333 334 private static final long serialVersionUID = -1829474901L; 335 336 /** 337 * Constructor 338 */ 339 public ChargeItemDefinitionPropertyGroupComponent() { 340 super(); 341 } 342 343 /** 344 * @return {@link #applicability} (Expressions that describe applicability criteria for the priceComponent.) 345 */ 346 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 347 if (this.applicability == null) 348 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 349 return this.applicability; 350 } 351 352 /** 353 * @return Returns a reference to <code>this</code> for easy method chaining 354 */ 355 public ChargeItemDefinitionPropertyGroupComponent setApplicability(List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 356 this.applicability = theApplicability; 357 return this; 358 } 359 360 public boolean hasApplicability() { 361 if (this.applicability == null) 362 return false; 363 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 364 if (!item.isEmpty()) 365 return true; 366 return false; 367 } 368 369 public ChargeItemDefinitionApplicabilityComponent addApplicability() { //3 370 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 371 if (this.applicability == null) 372 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 373 this.applicability.add(t); 374 return t; 375 } 376 377 public ChargeItemDefinitionPropertyGroupComponent addApplicability(ChargeItemDefinitionApplicabilityComponent t) { //3 378 if (t == null) 379 return this; 380 if (this.applicability == null) 381 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 382 this.applicability.add(t); 383 return this; 384 } 385 386 /** 387 * @return The first repetition of repeating field {@link #applicability}, creating it if it does not already exist {3} 388 */ 389 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 390 if (getApplicability().isEmpty()) { 391 addApplicability(); 392 } 393 return getApplicability().get(0); 394 } 395 396 /** 397 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.) 398 */ 399 public List<MonetaryComponent> getPriceComponent() { 400 if (this.priceComponent == null) 401 this.priceComponent = new ArrayList<MonetaryComponent>(); 402 return this.priceComponent; 403 } 404 405 /** 406 * @return Returns a reference to <code>this</code> for easy method chaining 407 */ 408 public ChargeItemDefinitionPropertyGroupComponent setPriceComponent(List<MonetaryComponent> thePriceComponent) { 409 this.priceComponent = thePriceComponent; 410 return this; 411 } 412 413 public boolean hasPriceComponent() { 414 if (this.priceComponent == null) 415 return false; 416 for (MonetaryComponent item : this.priceComponent) 417 if (!item.isEmpty()) 418 return true; 419 return false; 420 } 421 422 public MonetaryComponent addPriceComponent() { //3 423 MonetaryComponent t = new MonetaryComponent(); 424 if (this.priceComponent == null) 425 this.priceComponent = new ArrayList<MonetaryComponent>(); 426 this.priceComponent.add(t); 427 return t; 428 } 429 430 public ChargeItemDefinitionPropertyGroupComponent addPriceComponent(MonetaryComponent t) { //3 431 if (t == null) 432 return this; 433 if (this.priceComponent == null) 434 this.priceComponent = new ArrayList<MonetaryComponent>(); 435 this.priceComponent.add(t); 436 return this; 437 } 438 439 /** 440 * @return The first repetition of repeating field {@link #priceComponent}, creating it if it does not already exist {3} 441 */ 442 public MonetaryComponent getPriceComponentFirstRep() { 443 if (getPriceComponent().isEmpty()) { 444 addPriceComponent(); 445 } 446 return getPriceComponent().get(0); 447 } 448 449 protected void listChildren(List<Property> children) { 450 super.listChildren(children); 451 children.add(new Property("applicability", "@ChargeItemDefinition.applicability", "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, applicability)); 452 children.add(new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent)); 453 } 454 455 @Override 456 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 457 switch (_hash) { 458 case -1526770491: /*applicability*/ return new Property("applicability", "@ChargeItemDefinition.applicability", "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, applicability); 459 case 1219095988: /*priceComponent*/ return new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent); 460 default: return super.getNamedProperty(_hash, _name, _checkValid); 461 } 462 463 } 464 465 @Override 466 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 467 switch (hash) { 468 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 469 case 1219095988: /*priceComponent*/ return this.priceComponent == null ? new Base[0] : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // MonetaryComponent 470 default: return super.getProperty(hash, name, checkValid); 471 } 472 473 } 474 475 @Override 476 public Base setProperty(int hash, String name, Base value) throws FHIRException { 477 switch (hash) { 478 case -1526770491: // applicability 479 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 480 return value; 481 case 1219095988: // priceComponent 482 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); // MonetaryComponent 483 return value; 484 default: return super.setProperty(hash, name, value); 485 } 486 487 } 488 489 @Override 490 public Base setProperty(String name, Base value) throws FHIRException { 491 if (name.equals("applicability")) { 492 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 493 } else if (name.equals("priceComponent")) { 494 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); 495 } else 496 return super.setProperty(name, value); 497 return value; 498 } 499 500 @Override 501 public void removeChild(String name, Base value) throws FHIRException { 502 if (name.equals("applicability")) { 503 this.getApplicability().remove((ChargeItemDefinitionApplicabilityComponent) value); 504 } else if (name.equals("priceComponent")) { 505 this.getPriceComponent().remove(value); 506 } else 507 super.removeChild(name, value); 508 509 } 510 511 @Override 512 public Base makeProperty(int hash, String name) throws FHIRException { 513 switch (hash) { 514 case -1526770491: return addApplicability(); 515 case 1219095988: return addPriceComponent(); 516 default: return super.makeProperty(hash, name); 517 } 518 519 } 520 521 @Override 522 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 523 switch (hash) { 524 case -1526770491: /*applicability*/ return new String[] {"@ChargeItemDefinition.applicability"}; 525 case 1219095988: /*priceComponent*/ return new String[] {"MonetaryComponent"}; 526 default: return super.getTypesForProperty(hash, name); 527 } 528 529 } 530 531 @Override 532 public Base addChild(String name) throws FHIRException { 533 if (name.equals("applicability")) { 534 return addApplicability(); 535 } 536 else if (name.equals("priceComponent")) { 537 return addPriceComponent(); 538 } 539 else 540 return super.addChild(name); 541 } 542 543 public ChargeItemDefinitionPropertyGroupComponent copy() { 544 ChargeItemDefinitionPropertyGroupComponent dst = new ChargeItemDefinitionPropertyGroupComponent(); 545 copyValues(dst); 546 return dst; 547 } 548 549 public void copyValues(ChargeItemDefinitionPropertyGroupComponent dst) { 550 super.copyValues(dst); 551 if (applicability != null) { 552 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 553 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 554 dst.applicability.add(i.copy()); 555 }; 556 if (priceComponent != null) { 557 dst.priceComponent = new ArrayList<MonetaryComponent>(); 558 for (MonetaryComponent i : priceComponent) 559 dst.priceComponent.add(i.copy()); 560 }; 561 } 562 563 @Override 564 public boolean equalsDeep(Base other_) { 565 if (!super.equalsDeep(other_)) 566 return false; 567 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 568 return false; 569 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 570 return compareDeep(applicability, o.applicability, true) && compareDeep(priceComponent, o.priceComponent, true) 571 ; 572 } 573 574 @Override 575 public boolean equalsShallow(Base other_) { 576 if (!super.equalsShallow(other_)) 577 return false; 578 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 579 return false; 580 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 581 return true; 582 } 583 584 public boolean isEmpty() { 585 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(applicability, priceComponent 586 ); 587 } 588 589 public String fhirType() { 590 return "ChargeItemDefinition.propertyGroup"; 591 592 } 593 594 } 595 596 /** 597 * An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers. 598 */ 599 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 600 @Description(shortDefinition="Canonical identifier for this charge item definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers." ) 601 protected UriType url; 602 603 /** 604 * A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 605 */ 606 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 607 @Description(shortDefinition="Additional identifier for the charge item definition", formalDefinition="A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 608 protected List<Identifier> identifier; 609 610 /** 611 * The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 612 */ 613 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 614 @Description(shortDefinition="Business version of the charge item definition", formalDefinition="The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets." ) 615 protected StringType version; 616 617 /** 618 * Indicates the mechanism used to compare versions to determine which is more current. 619 */ 620 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 621 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 622 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 623 protected DataType versionAlgorithm; 624 625 /** 626 * A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 627 */ 628 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 629 @Description(shortDefinition="Name for this charge item definition (computer friendly)", formalDefinition="A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 630 protected StringType name; 631 632 /** 633 * A short, descriptive, user-friendly title for the charge item definition. 634 */ 635 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 636 @Description(shortDefinition="Name for this charge item definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the charge item definition." ) 637 protected StringType title; 638 639 /** 640 * The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition. 641 */ 642 @Child(name = "derivedFromUri", type = {UriType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 643 @Description(shortDefinition="Underlying externally-defined charge item definition", formalDefinition="The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition." ) 644 protected List<UriType> derivedFromUri; 645 646 /** 647 * A larger definition of which this particular definition is a component or step. 648 */ 649 @Child(name = "partOf", type = {CanonicalType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 650 @Description(shortDefinition="A larger definition of which this particular definition is a component or step", formalDefinition="A larger definition of which this particular definition is a component or step." ) 651 protected List<CanonicalType> partOf; 652 653 /** 654 * As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance. 655 */ 656 @Child(name = "replaces", type = {CanonicalType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 657 @Description(shortDefinition="Completed or terminated request(s) whose function is taken by this new request", formalDefinition="As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance." ) 658 protected List<CanonicalType> replaces; 659 660 /** 661 * The current state of the ChargeItemDefinition. 662 */ 663 @Child(name = "status", type = {CodeType.class}, order=9, min=1, max=1, modifier=true, summary=true) 664 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the ChargeItemDefinition." ) 665 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 666 protected Enumeration<PublicationStatus> status; 667 668 /** 669 * A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 670 */ 671 @Child(name = "experimental", type = {BooleanType.class}, order=10, min=0, max=1, modifier=false, summary=true) 672 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 673 protected BooleanType experimental; 674 675 /** 676 * The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes. 677 */ 678 @Child(name = "date", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 679 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes." ) 680 protected DateTimeType date; 681 682 /** 683 * The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition. 684 */ 685 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 686 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition." ) 687 protected StringType publisher; 688 689 /** 690 * Contact details to assist a user in finding and communicating with the publisher. 691 */ 692 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 693 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 694 protected List<ContactDetail> contact; 695 696 /** 697 * A free text natural language description of the charge item definition from a consumer's perspective. 698 */ 699 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=true) 700 @Description(shortDefinition="Natural language description of the charge item definition", formalDefinition="A free text natural language description of the charge item definition from a consumer's perspective." ) 701 protected MarkdownType description; 702 703 /** 704 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances. 705 */ 706 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 707 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances." ) 708 protected List<UsageContext> useContext; 709 710 /** 711 * A legal or geographic region in which the charge item definition is intended to be used. 712 */ 713 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 714 @Description(shortDefinition="Intended jurisdiction for charge item definition (if applicable)", formalDefinition="A legal or geographic region in which the charge item definition is intended to be used." ) 715 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 716 protected List<CodeableConcept> jurisdiction; 717 718 /** 719 * Explanation of why this charge item definition is needed and why it has been designed as it has. 720 */ 721 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 722 @Description(shortDefinition="Why this charge item definition is defined", formalDefinition="Explanation of why this charge item definition is needed and why it has been designed as it has." ) 723 protected MarkdownType purpose; 724 725 /** 726 * A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition. 727 */ 728 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 729 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition." ) 730 protected MarkdownType copyright; 731 732 /** 733 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 734 */ 735 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 736 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 737 protected StringType copyrightLabel; 738 739 /** 740 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 741 */ 742 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 743 @Description(shortDefinition="When the charge item definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 744 protected DateType approvalDate; 745 746 /** 747 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 748 */ 749 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 750 @Description(shortDefinition="When the charge item definition was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 751 protected DateType lastReviewDate; 752 753 /** 754 * The defined billing details in this resource pertain to the given billing code. 755 */ 756 @Child(name = "code", type = {CodeableConcept.class}, order=22, min=0, max=1, modifier=false, summary=true) 757 @Description(shortDefinition="Billing code or product type this definition applies to", formalDefinition="The defined billing details in this resource pertain to the given billing code." ) 758 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 759 protected CodeableConcept code; 760 761 /** 762 * The defined billing details in this resource pertain to the given product instance(s). 763 */ 764 @Child(name = "instance", type = {Medication.class, Substance.class, Device.class, DeviceDefinition.class, ActivityDefinition.class, PlanDefinition.class, HealthcareService.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 765 @Description(shortDefinition="Instances this definition applies to", formalDefinition="The defined billing details in this resource pertain to the given product instance(s)." ) 766 protected List<Reference> instance; 767 768 /** 769 * Expressions that describe applicability criteria for the billing code. 770 */ 771 @Child(name = "applicability", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 772 @Description(shortDefinition="Whether or not the billing code is applicable", formalDefinition="Expressions that describe applicability criteria for the billing code." ) 773 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 774 775 /** 776 * Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply. 777 */ 778 @Child(name = "propertyGroup", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 779 @Description(shortDefinition="Group of properties which are applicable under the same conditions", formalDefinition="Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply." ) 780 protected List<ChargeItemDefinitionPropertyGroupComponent> propertyGroup; 781 782 private static final long serialVersionUID = 900671183L; 783 784 /** 785 * Constructor 786 */ 787 public ChargeItemDefinition() { 788 super(); 789 } 790 791 /** 792 * Constructor 793 */ 794 public ChargeItemDefinition(PublicationStatus status) { 795 super(); 796 this.setStatus(status); 797 } 798 799 /** 800 * @return {@link #url} (An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 801 */ 802 public UriType getUrlElement() { 803 if (this.url == null) 804 if (Configuration.errorOnAutoCreate()) 805 throw new Error("Attempt to auto-create ChargeItemDefinition.url"); 806 else if (Configuration.doAutoCreate()) 807 this.url = new UriType(); // bb 808 return this.url; 809 } 810 811 public boolean hasUrlElement() { 812 return this.url != null && !this.url.isEmpty(); 813 } 814 815 public boolean hasUrl() { 816 return this.url != null && !this.url.isEmpty(); 817 } 818 819 /** 820 * @param value {@link #url} (An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 821 */ 822 public ChargeItemDefinition setUrlElement(UriType value) { 823 this.url = value; 824 return this; 825 } 826 827 /** 828 * @return An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers. 829 */ 830 public String getUrl() { 831 return this.url == null ? null : this.url.getValue(); 832 } 833 834 /** 835 * @param value An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers. 836 */ 837 public ChargeItemDefinition setUrl(String value) { 838 if (Utilities.noString(value)) 839 this.url = null; 840 else { 841 if (this.url == null) 842 this.url = new UriType(); 843 this.url.setValue(value); 844 } 845 return this; 846 } 847 848 /** 849 * @return {@link #identifier} (A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 850 */ 851 public List<Identifier> getIdentifier() { 852 if (this.identifier == null) 853 this.identifier = new ArrayList<Identifier>(); 854 return this.identifier; 855 } 856 857 /** 858 * @return Returns a reference to <code>this</code> for easy method chaining 859 */ 860 public ChargeItemDefinition setIdentifier(List<Identifier> theIdentifier) { 861 this.identifier = theIdentifier; 862 return this; 863 } 864 865 public boolean hasIdentifier() { 866 if (this.identifier == null) 867 return false; 868 for (Identifier item : this.identifier) 869 if (!item.isEmpty()) 870 return true; 871 return false; 872 } 873 874 public Identifier addIdentifier() { //3 875 Identifier t = new Identifier(); 876 if (this.identifier == null) 877 this.identifier = new ArrayList<Identifier>(); 878 this.identifier.add(t); 879 return t; 880 } 881 882 public ChargeItemDefinition addIdentifier(Identifier t) { //3 883 if (t == null) 884 return this; 885 if (this.identifier == null) 886 this.identifier = new ArrayList<Identifier>(); 887 this.identifier.add(t); 888 return this; 889 } 890 891 /** 892 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 893 */ 894 public Identifier getIdentifierFirstRep() { 895 if (getIdentifier().isEmpty()) { 896 addIdentifier(); 897 } 898 return getIdentifier().get(0); 899 } 900 901 /** 902 * @return {@link #version} (The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 903 */ 904 public StringType getVersionElement() { 905 if (this.version == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create ChargeItemDefinition.version"); 908 else if (Configuration.doAutoCreate()) 909 this.version = new StringType(); // bb 910 return this.version; 911 } 912 913 public boolean hasVersionElement() { 914 return this.version != null && !this.version.isEmpty(); 915 } 916 917 public boolean hasVersion() { 918 return this.version != null && !this.version.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #version} (The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 923 */ 924 public ChargeItemDefinition setVersionElement(StringType value) { 925 this.version = value; 926 return this; 927 } 928 929 /** 930 * @return The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 931 */ 932 public String getVersion() { 933 return this.version == null ? null : this.version.getValue(); 934 } 935 936 /** 937 * @param value The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets. 938 */ 939 public ChargeItemDefinition setVersion(String value) { 940 if (Utilities.noString(value)) 941 this.version = null; 942 else { 943 if (this.version == null) 944 this.version = new StringType(); 945 this.version.setValue(value); 946 } 947 return this; 948 } 949 950 /** 951 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 952 */ 953 public DataType getVersionAlgorithm() { 954 return this.versionAlgorithm; 955 } 956 957 /** 958 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 959 */ 960 public StringType getVersionAlgorithmStringType() throws FHIRException { 961 if (this.versionAlgorithm == null) 962 this.versionAlgorithm = new StringType(); 963 if (!(this.versionAlgorithm instanceof StringType)) 964 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 965 return (StringType) this.versionAlgorithm; 966 } 967 968 public boolean hasVersionAlgorithmStringType() { 969 return this != null && this.versionAlgorithm instanceof StringType; 970 } 971 972 /** 973 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 974 */ 975 public Coding getVersionAlgorithmCoding() throws FHIRException { 976 if (this.versionAlgorithm == null) 977 this.versionAlgorithm = new Coding(); 978 if (!(this.versionAlgorithm instanceof Coding)) 979 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 980 return (Coding) this.versionAlgorithm; 981 } 982 983 public boolean hasVersionAlgorithmCoding() { 984 return this != null && this.versionAlgorithm instanceof Coding; 985 } 986 987 public boolean hasVersionAlgorithm() { 988 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 989 } 990 991 /** 992 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 993 */ 994 public ChargeItemDefinition setVersionAlgorithm(DataType value) { 995 if (value != null && !(value instanceof StringType || value instanceof Coding)) 996 throw new FHIRException("Not the right type for ChargeItemDefinition.versionAlgorithm[x]: "+value.fhirType()); 997 this.versionAlgorithm = value; 998 return this; 999 } 1000 1001 /** 1002 * @return {@link #name} (A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1003 */ 1004 public StringType getNameElement() { 1005 if (this.name == null) 1006 if (Configuration.errorOnAutoCreate()) 1007 throw new Error("Attempt to auto-create ChargeItemDefinition.name"); 1008 else if (Configuration.doAutoCreate()) 1009 this.name = new StringType(); // bb 1010 return this.name; 1011 } 1012 1013 public boolean hasNameElement() { 1014 return this.name != null && !this.name.isEmpty(); 1015 } 1016 1017 public boolean hasName() { 1018 return this.name != null && !this.name.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #name} (A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1023 */ 1024 public ChargeItemDefinition setNameElement(StringType value) { 1025 this.name = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1031 */ 1032 public String getName() { 1033 return this.name == null ? null : this.name.getValue(); 1034 } 1035 1036 /** 1037 * @param value A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1038 */ 1039 public ChargeItemDefinition setName(String value) { 1040 if (Utilities.noString(value)) 1041 this.name = null; 1042 else { 1043 if (this.name == null) 1044 this.name = new StringType(); 1045 this.name.setValue(value); 1046 } 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #title} (A short, descriptive, user-friendly title for the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1052 */ 1053 public StringType getTitleElement() { 1054 if (this.title == null) 1055 if (Configuration.errorOnAutoCreate()) 1056 throw new Error("Attempt to auto-create ChargeItemDefinition.title"); 1057 else if (Configuration.doAutoCreate()) 1058 this.title = new StringType(); // bb 1059 return this.title; 1060 } 1061 1062 public boolean hasTitleElement() { 1063 return this.title != null && !this.title.isEmpty(); 1064 } 1065 1066 public boolean hasTitle() { 1067 return this.title != null && !this.title.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #title} (A short, descriptive, user-friendly title for the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1072 */ 1073 public ChargeItemDefinition setTitleElement(StringType value) { 1074 this.title = value; 1075 return this; 1076 } 1077 1078 /** 1079 * @return A short, descriptive, user-friendly title for the charge item definition. 1080 */ 1081 public String getTitle() { 1082 return this.title == null ? null : this.title.getValue(); 1083 } 1084 1085 /** 1086 * @param value A short, descriptive, user-friendly title for the charge item definition. 1087 */ 1088 public ChargeItemDefinition setTitle(String value) { 1089 if (Utilities.noString(value)) 1090 this.title = null; 1091 else { 1092 if (this.title == null) 1093 this.title = new StringType(); 1094 this.title.setValue(value); 1095 } 1096 return this; 1097 } 1098 1099 /** 1100 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1101 */ 1102 public List<UriType> getDerivedFromUri() { 1103 if (this.derivedFromUri == null) 1104 this.derivedFromUri = new ArrayList<UriType>(); 1105 return this.derivedFromUri; 1106 } 1107 1108 /** 1109 * @return Returns a reference to <code>this</code> for easy method chaining 1110 */ 1111 public ChargeItemDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 1112 this.derivedFromUri = theDerivedFromUri; 1113 return this; 1114 } 1115 1116 public boolean hasDerivedFromUri() { 1117 if (this.derivedFromUri == null) 1118 return false; 1119 for (UriType item : this.derivedFromUri) 1120 if (!item.isEmpty()) 1121 return true; 1122 return false; 1123 } 1124 1125 /** 1126 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1127 */ 1128 public UriType addDerivedFromUriElement() {//2 1129 UriType t = new UriType(); 1130 if (this.derivedFromUri == null) 1131 this.derivedFromUri = new ArrayList<UriType>(); 1132 this.derivedFromUri.add(t); 1133 return t; 1134 } 1135 1136 /** 1137 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1138 */ 1139 public ChargeItemDefinition addDerivedFromUri(String value) { //1 1140 UriType t = new UriType(); 1141 t.setValue(value); 1142 if (this.derivedFromUri == null) 1143 this.derivedFromUri = new ArrayList<UriType>(); 1144 this.derivedFromUri.add(t); 1145 return this; 1146 } 1147 1148 /** 1149 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.) 1150 */ 1151 public boolean hasDerivedFromUri(String value) { 1152 if (this.derivedFromUri == null) 1153 return false; 1154 for (UriType v : this.derivedFromUri) 1155 if (v.getValue().equals(value)) // uri 1156 return true; 1157 return false; 1158 } 1159 1160 /** 1161 * @return {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1162 */ 1163 public List<CanonicalType> getPartOf() { 1164 if (this.partOf == null) 1165 this.partOf = new ArrayList<CanonicalType>(); 1166 return this.partOf; 1167 } 1168 1169 /** 1170 * @return Returns a reference to <code>this</code> for easy method chaining 1171 */ 1172 public ChargeItemDefinition setPartOf(List<CanonicalType> thePartOf) { 1173 this.partOf = thePartOf; 1174 return this; 1175 } 1176 1177 public boolean hasPartOf() { 1178 if (this.partOf == null) 1179 return false; 1180 for (CanonicalType item : this.partOf) 1181 if (!item.isEmpty()) 1182 return true; 1183 return false; 1184 } 1185 1186 /** 1187 * @return {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1188 */ 1189 public CanonicalType addPartOfElement() {//2 1190 CanonicalType t = new CanonicalType(); 1191 if (this.partOf == null) 1192 this.partOf = new ArrayList<CanonicalType>(); 1193 this.partOf.add(t); 1194 return t; 1195 } 1196 1197 /** 1198 * @param value {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1199 */ 1200 public ChargeItemDefinition addPartOf(String value) { //1 1201 CanonicalType t = new CanonicalType(); 1202 t.setValue(value); 1203 if (this.partOf == null) 1204 this.partOf = new ArrayList<CanonicalType>(); 1205 this.partOf.add(t); 1206 return this; 1207 } 1208 1209 /** 1210 * @param value {@link #partOf} (A larger definition of which this particular definition is a component or step.) 1211 */ 1212 public boolean hasPartOf(String value) { 1213 if (this.partOf == null) 1214 return false; 1215 for (CanonicalType v : this.partOf) 1216 if (v.getValue().equals(value)) // canonical 1217 return true; 1218 return false; 1219 } 1220 1221 /** 1222 * @return {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1223 */ 1224 public List<CanonicalType> getReplaces() { 1225 if (this.replaces == null) 1226 this.replaces = new ArrayList<CanonicalType>(); 1227 return this.replaces; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public ChargeItemDefinition setReplaces(List<CanonicalType> theReplaces) { 1234 this.replaces = theReplaces; 1235 return this; 1236 } 1237 1238 public boolean hasReplaces() { 1239 if (this.replaces == null) 1240 return false; 1241 for (CanonicalType item : this.replaces) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 /** 1248 * @return {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1249 */ 1250 public CanonicalType addReplacesElement() {//2 1251 CanonicalType t = new CanonicalType(); 1252 if (this.replaces == null) 1253 this.replaces = new ArrayList<CanonicalType>(); 1254 this.replaces.add(t); 1255 return t; 1256 } 1257 1258 /** 1259 * @param value {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1260 */ 1261 public ChargeItemDefinition addReplaces(String value) { //1 1262 CanonicalType t = new CanonicalType(); 1263 t.setValue(value); 1264 if (this.replaces == null) 1265 this.replaces = new ArrayList<CanonicalType>(); 1266 this.replaces.add(t); 1267 return this; 1268 } 1269 1270 /** 1271 * @param value {@link #replaces} (As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.) 1272 */ 1273 public boolean hasReplaces(String value) { 1274 if (this.replaces == null) 1275 return false; 1276 for (CanonicalType v : this.replaces) 1277 if (v.getValue().equals(value)) // canonical 1278 return true; 1279 return false; 1280 } 1281 1282 /** 1283 * @return {@link #status} (The current state of the ChargeItemDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1284 */ 1285 public Enumeration<PublicationStatus> getStatusElement() { 1286 if (this.status == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create ChargeItemDefinition.status"); 1289 else if (Configuration.doAutoCreate()) 1290 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1291 return this.status; 1292 } 1293 1294 public boolean hasStatusElement() { 1295 return this.status != null && !this.status.isEmpty(); 1296 } 1297 1298 public boolean hasStatus() { 1299 return this.status != null && !this.status.isEmpty(); 1300 } 1301 1302 /** 1303 * @param value {@link #status} (The current state of the ChargeItemDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1304 */ 1305 public ChargeItemDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1306 this.status = value; 1307 return this; 1308 } 1309 1310 /** 1311 * @return The current state of the ChargeItemDefinition. 1312 */ 1313 public PublicationStatus getStatus() { 1314 return this.status == null ? null : this.status.getValue(); 1315 } 1316 1317 /** 1318 * @param value The current state of the ChargeItemDefinition. 1319 */ 1320 public ChargeItemDefinition setStatus(PublicationStatus value) { 1321 if (this.status == null) 1322 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1323 this.status.setValue(value); 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #experimental} (A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1329 */ 1330 public BooleanType getExperimentalElement() { 1331 if (this.experimental == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create ChargeItemDefinition.experimental"); 1334 else if (Configuration.doAutoCreate()) 1335 this.experimental = new BooleanType(); // bb 1336 return this.experimental; 1337 } 1338 1339 public boolean hasExperimentalElement() { 1340 return this.experimental != null && !this.experimental.isEmpty(); 1341 } 1342 1343 public boolean hasExperimental() { 1344 return this.experimental != null && !this.experimental.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #experimental} (A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1349 */ 1350 public ChargeItemDefinition setExperimentalElement(BooleanType value) { 1351 this.experimental = value; 1352 return this; 1353 } 1354 1355 /** 1356 * @return A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1357 */ 1358 public boolean getExperimental() { 1359 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1360 } 1361 1362 /** 1363 * @param value A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1364 */ 1365 public ChargeItemDefinition setExperimental(boolean value) { 1366 if (this.experimental == null) 1367 this.experimental = new BooleanType(); 1368 this.experimental.setValue(value); 1369 return this; 1370 } 1371 1372 /** 1373 * @return {@link #date} (The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1374 */ 1375 public DateTimeType getDateElement() { 1376 if (this.date == null) 1377 if (Configuration.errorOnAutoCreate()) 1378 throw new Error("Attempt to auto-create ChargeItemDefinition.date"); 1379 else if (Configuration.doAutoCreate()) 1380 this.date = new DateTimeType(); // bb 1381 return this.date; 1382 } 1383 1384 public boolean hasDateElement() { 1385 return this.date != null && !this.date.isEmpty(); 1386 } 1387 1388 public boolean hasDate() { 1389 return this.date != null && !this.date.isEmpty(); 1390 } 1391 1392 /** 1393 * @param value {@link #date} (The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1394 */ 1395 public ChargeItemDefinition setDateElement(DateTimeType value) { 1396 this.date = value; 1397 return this; 1398 } 1399 1400 /** 1401 * @return The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes. 1402 */ 1403 public Date getDate() { 1404 return this.date == null ? null : this.date.getValue(); 1405 } 1406 1407 /** 1408 * @param value The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes. 1409 */ 1410 public ChargeItemDefinition setDate(Date value) { 1411 if (value == null) 1412 this.date = null; 1413 else { 1414 if (this.date == null) 1415 this.date = new DateTimeType(); 1416 this.date.setValue(value); 1417 } 1418 return this; 1419 } 1420 1421 /** 1422 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1423 */ 1424 public StringType getPublisherElement() { 1425 if (this.publisher == null) 1426 if (Configuration.errorOnAutoCreate()) 1427 throw new Error("Attempt to auto-create ChargeItemDefinition.publisher"); 1428 else if (Configuration.doAutoCreate()) 1429 this.publisher = new StringType(); // bb 1430 return this.publisher; 1431 } 1432 1433 public boolean hasPublisherElement() { 1434 return this.publisher != null && !this.publisher.isEmpty(); 1435 } 1436 1437 public boolean hasPublisher() { 1438 return this.publisher != null && !this.publisher.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1443 */ 1444 public ChargeItemDefinition setPublisherElement(StringType value) { 1445 this.publisher = value; 1446 return this; 1447 } 1448 1449 /** 1450 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition. 1451 */ 1452 public String getPublisher() { 1453 return this.publisher == null ? null : this.publisher.getValue(); 1454 } 1455 1456 /** 1457 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition. 1458 */ 1459 public ChargeItemDefinition setPublisher(String value) { 1460 if (Utilities.noString(value)) 1461 this.publisher = null; 1462 else { 1463 if (this.publisher == null) 1464 this.publisher = new StringType(); 1465 this.publisher.setValue(value); 1466 } 1467 return this; 1468 } 1469 1470 /** 1471 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1472 */ 1473 public List<ContactDetail> getContact() { 1474 if (this.contact == null) 1475 this.contact = new ArrayList<ContactDetail>(); 1476 return this.contact; 1477 } 1478 1479 /** 1480 * @return Returns a reference to <code>this</code> for easy method chaining 1481 */ 1482 public ChargeItemDefinition setContact(List<ContactDetail> theContact) { 1483 this.contact = theContact; 1484 return this; 1485 } 1486 1487 public boolean hasContact() { 1488 if (this.contact == null) 1489 return false; 1490 for (ContactDetail item : this.contact) 1491 if (!item.isEmpty()) 1492 return true; 1493 return false; 1494 } 1495 1496 public ContactDetail addContact() { //3 1497 ContactDetail t = new ContactDetail(); 1498 if (this.contact == null) 1499 this.contact = new ArrayList<ContactDetail>(); 1500 this.contact.add(t); 1501 return t; 1502 } 1503 1504 public ChargeItemDefinition addContact(ContactDetail t) { //3 1505 if (t == null) 1506 return this; 1507 if (this.contact == null) 1508 this.contact = new ArrayList<ContactDetail>(); 1509 this.contact.add(t); 1510 return this; 1511 } 1512 1513 /** 1514 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1515 */ 1516 public ContactDetail getContactFirstRep() { 1517 if (getContact().isEmpty()) { 1518 addContact(); 1519 } 1520 return getContact().get(0); 1521 } 1522 1523 /** 1524 * @return {@link #description} (A free text natural language description of the charge item definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1525 */ 1526 public MarkdownType getDescriptionElement() { 1527 if (this.description == null) 1528 if (Configuration.errorOnAutoCreate()) 1529 throw new Error("Attempt to auto-create ChargeItemDefinition.description"); 1530 else if (Configuration.doAutoCreate()) 1531 this.description = new MarkdownType(); // bb 1532 return this.description; 1533 } 1534 1535 public boolean hasDescriptionElement() { 1536 return this.description != null && !this.description.isEmpty(); 1537 } 1538 1539 public boolean hasDescription() { 1540 return this.description != null && !this.description.isEmpty(); 1541 } 1542 1543 /** 1544 * @param value {@link #description} (A free text natural language description of the charge item definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1545 */ 1546 public ChargeItemDefinition setDescriptionElement(MarkdownType value) { 1547 this.description = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return A free text natural language description of the charge item definition from a consumer's perspective. 1553 */ 1554 public String getDescription() { 1555 return this.description == null ? null : this.description.getValue(); 1556 } 1557 1558 /** 1559 * @param value A free text natural language description of the charge item definition from a consumer's perspective. 1560 */ 1561 public ChargeItemDefinition setDescription(String value) { 1562 if (Utilities.noString(value)) 1563 this.description = null; 1564 else { 1565 if (this.description == null) 1566 this.description = new MarkdownType(); 1567 this.description.setValue(value); 1568 } 1569 return this; 1570 } 1571 1572 /** 1573 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.) 1574 */ 1575 public List<UsageContext> getUseContext() { 1576 if (this.useContext == null) 1577 this.useContext = new ArrayList<UsageContext>(); 1578 return this.useContext; 1579 } 1580 1581 /** 1582 * @return Returns a reference to <code>this</code> for easy method chaining 1583 */ 1584 public ChargeItemDefinition setUseContext(List<UsageContext> theUseContext) { 1585 this.useContext = theUseContext; 1586 return this; 1587 } 1588 1589 public boolean hasUseContext() { 1590 if (this.useContext == null) 1591 return false; 1592 for (UsageContext item : this.useContext) 1593 if (!item.isEmpty()) 1594 return true; 1595 return false; 1596 } 1597 1598 public UsageContext addUseContext() { //3 1599 UsageContext t = new UsageContext(); 1600 if (this.useContext == null) 1601 this.useContext = new ArrayList<UsageContext>(); 1602 this.useContext.add(t); 1603 return t; 1604 } 1605 1606 public ChargeItemDefinition addUseContext(UsageContext t) { //3 1607 if (t == null) 1608 return this; 1609 if (this.useContext == null) 1610 this.useContext = new ArrayList<UsageContext>(); 1611 this.useContext.add(t); 1612 return this; 1613 } 1614 1615 /** 1616 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1617 */ 1618 public UsageContext getUseContextFirstRep() { 1619 if (getUseContext().isEmpty()) { 1620 addUseContext(); 1621 } 1622 return getUseContext().get(0); 1623 } 1624 1625 /** 1626 * @return {@link #jurisdiction} (A legal or geographic region in which the charge item definition is intended to be used.) 1627 */ 1628 public List<CodeableConcept> getJurisdiction() { 1629 if (this.jurisdiction == null) 1630 this.jurisdiction = new ArrayList<CodeableConcept>(); 1631 return this.jurisdiction; 1632 } 1633 1634 /** 1635 * @return Returns a reference to <code>this</code> for easy method chaining 1636 */ 1637 public ChargeItemDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1638 this.jurisdiction = theJurisdiction; 1639 return this; 1640 } 1641 1642 public boolean hasJurisdiction() { 1643 if (this.jurisdiction == null) 1644 return false; 1645 for (CodeableConcept item : this.jurisdiction) 1646 if (!item.isEmpty()) 1647 return true; 1648 return false; 1649 } 1650 1651 public CodeableConcept addJurisdiction() { //3 1652 CodeableConcept t = new CodeableConcept(); 1653 if (this.jurisdiction == null) 1654 this.jurisdiction = new ArrayList<CodeableConcept>(); 1655 this.jurisdiction.add(t); 1656 return t; 1657 } 1658 1659 public ChargeItemDefinition addJurisdiction(CodeableConcept t) { //3 1660 if (t == null) 1661 return this; 1662 if (this.jurisdiction == null) 1663 this.jurisdiction = new ArrayList<CodeableConcept>(); 1664 this.jurisdiction.add(t); 1665 return this; 1666 } 1667 1668 /** 1669 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1670 */ 1671 public CodeableConcept getJurisdictionFirstRep() { 1672 if (getJurisdiction().isEmpty()) { 1673 addJurisdiction(); 1674 } 1675 return getJurisdiction().get(0); 1676 } 1677 1678 /** 1679 * @return {@link #purpose} (Explanation of why this charge item definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1680 */ 1681 public MarkdownType getPurposeElement() { 1682 if (this.purpose == null) 1683 if (Configuration.errorOnAutoCreate()) 1684 throw new Error("Attempt to auto-create ChargeItemDefinition.purpose"); 1685 else if (Configuration.doAutoCreate()) 1686 this.purpose = new MarkdownType(); // bb 1687 return this.purpose; 1688 } 1689 1690 public boolean hasPurposeElement() { 1691 return this.purpose != null && !this.purpose.isEmpty(); 1692 } 1693 1694 public boolean hasPurpose() { 1695 return this.purpose != null && !this.purpose.isEmpty(); 1696 } 1697 1698 /** 1699 * @param value {@link #purpose} (Explanation of why this charge item definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1700 */ 1701 public ChargeItemDefinition setPurposeElement(MarkdownType value) { 1702 this.purpose = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return Explanation of why this charge item definition is needed and why it has been designed as it has. 1708 */ 1709 public String getPurpose() { 1710 return this.purpose == null ? null : this.purpose.getValue(); 1711 } 1712 1713 /** 1714 * @param value Explanation of why this charge item definition is needed and why it has been designed as it has. 1715 */ 1716 public ChargeItemDefinition setPurpose(String value) { 1717 if (Utilities.noString(value)) 1718 this.purpose = null; 1719 else { 1720 if (this.purpose == null) 1721 this.purpose = new MarkdownType(); 1722 this.purpose.setValue(value); 1723 } 1724 return this; 1725 } 1726 1727 /** 1728 * @return {@link #copyright} (A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1729 */ 1730 public MarkdownType getCopyrightElement() { 1731 if (this.copyright == null) 1732 if (Configuration.errorOnAutoCreate()) 1733 throw new Error("Attempt to auto-create ChargeItemDefinition.copyright"); 1734 else if (Configuration.doAutoCreate()) 1735 this.copyright = new MarkdownType(); // bb 1736 return this.copyright; 1737 } 1738 1739 public boolean hasCopyrightElement() { 1740 return this.copyright != null && !this.copyright.isEmpty(); 1741 } 1742 1743 public boolean hasCopyright() { 1744 return this.copyright != null && !this.copyright.isEmpty(); 1745 } 1746 1747 /** 1748 * @param value {@link #copyright} (A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1749 */ 1750 public ChargeItemDefinition setCopyrightElement(MarkdownType value) { 1751 this.copyright = value; 1752 return this; 1753 } 1754 1755 /** 1756 * @return A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition. 1757 */ 1758 public String getCopyright() { 1759 return this.copyright == null ? null : this.copyright.getValue(); 1760 } 1761 1762 /** 1763 * @param value A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition. 1764 */ 1765 public ChargeItemDefinition setCopyright(String value) { 1766 if (Utilities.noString(value)) 1767 this.copyright = null; 1768 else { 1769 if (this.copyright == null) 1770 this.copyright = new MarkdownType(); 1771 this.copyright.setValue(value); 1772 } 1773 return this; 1774 } 1775 1776 /** 1777 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1778 */ 1779 public StringType getCopyrightLabelElement() { 1780 if (this.copyrightLabel == null) 1781 if (Configuration.errorOnAutoCreate()) 1782 throw new Error("Attempt to auto-create ChargeItemDefinition.copyrightLabel"); 1783 else if (Configuration.doAutoCreate()) 1784 this.copyrightLabel = new StringType(); // bb 1785 return this.copyrightLabel; 1786 } 1787 1788 public boolean hasCopyrightLabelElement() { 1789 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1790 } 1791 1792 public boolean hasCopyrightLabel() { 1793 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1794 } 1795 1796 /** 1797 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1798 */ 1799 public ChargeItemDefinition setCopyrightLabelElement(StringType value) { 1800 this.copyrightLabel = value; 1801 return this; 1802 } 1803 1804 /** 1805 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1806 */ 1807 public String getCopyrightLabel() { 1808 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1809 } 1810 1811 /** 1812 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1813 */ 1814 public ChargeItemDefinition setCopyrightLabel(String value) { 1815 if (Utilities.noString(value)) 1816 this.copyrightLabel = null; 1817 else { 1818 if (this.copyrightLabel == null) 1819 this.copyrightLabel = new StringType(); 1820 this.copyrightLabel.setValue(value); 1821 } 1822 return this; 1823 } 1824 1825 /** 1826 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1827 */ 1828 public DateType getApprovalDateElement() { 1829 if (this.approvalDate == null) 1830 if (Configuration.errorOnAutoCreate()) 1831 throw new Error("Attempt to auto-create ChargeItemDefinition.approvalDate"); 1832 else if (Configuration.doAutoCreate()) 1833 this.approvalDate = new DateType(); // bb 1834 return this.approvalDate; 1835 } 1836 1837 public boolean hasApprovalDateElement() { 1838 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1839 } 1840 1841 public boolean hasApprovalDate() { 1842 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1843 } 1844 1845 /** 1846 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1847 */ 1848 public ChargeItemDefinition setApprovalDateElement(DateType value) { 1849 this.approvalDate = value; 1850 return this; 1851 } 1852 1853 /** 1854 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1855 */ 1856 public Date getApprovalDate() { 1857 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1858 } 1859 1860 /** 1861 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1862 */ 1863 public ChargeItemDefinition setApprovalDate(Date value) { 1864 if (value == null) 1865 this.approvalDate = null; 1866 else { 1867 if (this.approvalDate == null) 1868 this.approvalDate = new DateType(); 1869 this.approvalDate.setValue(value); 1870 } 1871 return this; 1872 } 1873 1874 /** 1875 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1876 */ 1877 public DateType getLastReviewDateElement() { 1878 if (this.lastReviewDate == null) 1879 if (Configuration.errorOnAutoCreate()) 1880 throw new Error("Attempt to auto-create ChargeItemDefinition.lastReviewDate"); 1881 else if (Configuration.doAutoCreate()) 1882 this.lastReviewDate = new DateType(); // bb 1883 return this.lastReviewDate; 1884 } 1885 1886 public boolean hasLastReviewDateElement() { 1887 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1888 } 1889 1890 public boolean hasLastReviewDate() { 1891 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1892 } 1893 1894 /** 1895 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1896 */ 1897 public ChargeItemDefinition setLastReviewDateElement(DateType value) { 1898 this.lastReviewDate = value; 1899 return this; 1900 } 1901 1902 /** 1903 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1904 */ 1905 public Date getLastReviewDate() { 1906 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1907 } 1908 1909 /** 1910 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1911 */ 1912 public ChargeItemDefinition setLastReviewDate(Date value) { 1913 if (value == null) 1914 this.lastReviewDate = null; 1915 else { 1916 if (this.lastReviewDate == null) 1917 this.lastReviewDate = new DateType(); 1918 this.lastReviewDate.setValue(value); 1919 } 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #code} (The defined billing details in this resource pertain to the given billing code.) 1925 */ 1926 public CodeableConcept getCode() { 1927 if (this.code == null) 1928 if (Configuration.errorOnAutoCreate()) 1929 throw new Error("Attempt to auto-create ChargeItemDefinition.code"); 1930 else if (Configuration.doAutoCreate()) 1931 this.code = new CodeableConcept(); // cc 1932 return this.code; 1933 } 1934 1935 public boolean hasCode() { 1936 return this.code != null && !this.code.isEmpty(); 1937 } 1938 1939 /** 1940 * @param value {@link #code} (The defined billing details in this resource pertain to the given billing code.) 1941 */ 1942 public ChargeItemDefinition setCode(CodeableConcept value) { 1943 this.code = value; 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #instance} (The defined billing details in this resource pertain to the given product instance(s).) 1949 */ 1950 public List<Reference> getInstance() { 1951 if (this.instance == null) 1952 this.instance = new ArrayList<Reference>(); 1953 return this.instance; 1954 } 1955 1956 /** 1957 * @return Returns a reference to <code>this</code> for easy method chaining 1958 */ 1959 public ChargeItemDefinition setInstance(List<Reference> theInstance) { 1960 this.instance = theInstance; 1961 return this; 1962 } 1963 1964 public boolean hasInstance() { 1965 if (this.instance == null) 1966 return false; 1967 for (Reference item : this.instance) 1968 if (!item.isEmpty()) 1969 return true; 1970 return false; 1971 } 1972 1973 public Reference addInstance() { //3 1974 Reference t = new Reference(); 1975 if (this.instance == null) 1976 this.instance = new ArrayList<Reference>(); 1977 this.instance.add(t); 1978 return t; 1979 } 1980 1981 public ChargeItemDefinition addInstance(Reference t) { //3 1982 if (t == null) 1983 return this; 1984 if (this.instance == null) 1985 this.instance = new ArrayList<Reference>(); 1986 this.instance.add(t); 1987 return this; 1988 } 1989 1990 /** 1991 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 1992 */ 1993 public Reference getInstanceFirstRep() { 1994 if (getInstance().isEmpty()) { 1995 addInstance(); 1996 } 1997 return getInstance().get(0); 1998 } 1999 2000 /** 2001 * @return {@link #applicability} (Expressions that describe applicability criteria for the billing code.) 2002 */ 2003 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 2004 if (this.applicability == null) 2005 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2006 return this.applicability; 2007 } 2008 2009 /** 2010 * @return Returns a reference to <code>this</code> for easy method chaining 2011 */ 2012 public ChargeItemDefinition setApplicability(List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 2013 this.applicability = theApplicability; 2014 return this; 2015 } 2016 2017 public boolean hasApplicability() { 2018 if (this.applicability == null) 2019 return false; 2020 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 2021 if (!item.isEmpty()) 2022 return true; 2023 return false; 2024 } 2025 2026 public ChargeItemDefinitionApplicabilityComponent addApplicability() { //3 2027 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 2028 if (this.applicability == null) 2029 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2030 this.applicability.add(t); 2031 return t; 2032 } 2033 2034 public ChargeItemDefinition addApplicability(ChargeItemDefinitionApplicabilityComponent t) { //3 2035 if (t == null) 2036 return this; 2037 if (this.applicability == null) 2038 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2039 this.applicability.add(t); 2040 return this; 2041 } 2042 2043 /** 2044 * @return The first repetition of repeating field {@link #applicability}, creating it if it does not already exist {3} 2045 */ 2046 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 2047 if (getApplicability().isEmpty()) { 2048 addApplicability(); 2049 } 2050 return getApplicability().get(0); 2051 } 2052 2053 /** 2054 * @return {@link #propertyGroup} (Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.) 2055 */ 2056 public List<ChargeItemDefinitionPropertyGroupComponent> getPropertyGroup() { 2057 if (this.propertyGroup == null) 2058 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2059 return this.propertyGroup; 2060 } 2061 2062 /** 2063 * @return Returns a reference to <code>this</code> for easy method chaining 2064 */ 2065 public ChargeItemDefinition setPropertyGroup(List<ChargeItemDefinitionPropertyGroupComponent> thePropertyGroup) { 2066 this.propertyGroup = thePropertyGroup; 2067 return this; 2068 } 2069 2070 public boolean hasPropertyGroup() { 2071 if (this.propertyGroup == null) 2072 return false; 2073 for (ChargeItemDefinitionPropertyGroupComponent item : this.propertyGroup) 2074 if (!item.isEmpty()) 2075 return true; 2076 return false; 2077 } 2078 2079 public ChargeItemDefinitionPropertyGroupComponent addPropertyGroup() { //3 2080 ChargeItemDefinitionPropertyGroupComponent t = new ChargeItemDefinitionPropertyGroupComponent(); 2081 if (this.propertyGroup == null) 2082 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2083 this.propertyGroup.add(t); 2084 return t; 2085 } 2086 2087 public ChargeItemDefinition addPropertyGroup(ChargeItemDefinitionPropertyGroupComponent t) { //3 2088 if (t == null) 2089 return this; 2090 if (this.propertyGroup == null) 2091 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2092 this.propertyGroup.add(t); 2093 return this; 2094 } 2095 2096 /** 2097 * @return The first repetition of repeating field {@link #propertyGroup}, creating it if it does not already exist {3} 2098 */ 2099 public ChargeItemDefinitionPropertyGroupComponent getPropertyGroupFirstRep() { 2100 if (getPropertyGroup().isEmpty()) { 2101 addPropertyGroup(); 2102 } 2103 return getPropertyGroup().get(0); 2104 } 2105 2106 /** 2107 * not supported on this implementation 2108 */ 2109 @Override 2110 public int getEffectivePeriodMax() { 2111 return 0; 2112 } 2113 /** 2114 * @return {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 2115 */ 2116 public Period getEffectivePeriod() { 2117 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"effectivePeriod\""); 2118 } 2119 public boolean hasEffectivePeriod() { 2120 return false; 2121 } 2122 /** 2123 * @param value {@link #effectivePeriod} (The period during which the charge item definition content was or is planned to be in active use.) 2124 */ 2125 public ChargeItemDefinition setEffectivePeriod(Period value) { 2126 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"effectivePeriod\""); 2127 } 2128 2129 /** 2130 * not supported on this implementation 2131 */ 2132 @Override 2133 public int getTopicMax() { 2134 return 0; 2135 } 2136 /** 2137 * @return {@link #topic} (Descriptive topics related to the content of the charge item definition. Topics provide a high-level categorization as well as keywords for the charge item definition that can be useful for filtering and searching.) 2138 */ 2139 public List<CodeableConcept> getTopic() { 2140 return new ArrayList<>(); 2141 } 2142 /** 2143 * @return Returns a reference to <code>this</code> for easy method chaining 2144 */ 2145 public ChargeItemDefinition setTopic(List<CodeableConcept> theTopic) { 2146 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2147 } 2148 public boolean hasTopic() { 2149 return false; 2150 } 2151 2152 public CodeableConcept addTopic() { //3 2153 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2154 } 2155 public ChargeItemDefinition addTopic(CodeableConcept t) { //3 2156 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2157 } 2158 /** 2159 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 2160 */ 2161 public CodeableConcept getTopicFirstRep() { 2162 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"topic\""); 2163 } 2164 /** 2165 * not supported on this implementation 2166 */ 2167 @Override 2168 public int getAuthorMax() { 2169 return 0; 2170 } 2171 /** 2172 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the charge item definition.) 2173 */ 2174 public List<ContactDetail> getAuthor() { 2175 return new ArrayList<>(); 2176 } 2177 /** 2178 * @return Returns a reference to <code>this</code> for easy method chaining 2179 */ 2180 public ChargeItemDefinition setAuthor(List<ContactDetail> theAuthor) { 2181 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2182 } 2183 public boolean hasAuthor() { 2184 return false; 2185 } 2186 2187 public ContactDetail addAuthor() { //3 2188 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2189 } 2190 public ChargeItemDefinition addAuthor(ContactDetail t) { //3 2191 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2192 } 2193 /** 2194 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {2} 2195 */ 2196 public ContactDetail getAuthorFirstRep() { 2197 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"author\""); 2198 } 2199 /** 2200 * not supported on this implementation 2201 */ 2202 @Override 2203 public int getEditorMax() { 2204 return 0; 2205 } 2206 /** 2207 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the charge item definition.) 2208 */ 2209 public List<ContactDetail> getEditor() { 2210 return new ArrayList<>(); 2211 } 2212 /** 2213 * @return Returns a reference to <code>this</code> for easy method chaining 2214 */ 2215 public ChargeItemDefinition setEditor(List<ContactDetail> theEditor) { 2216 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2217 } 2218 public boolean hasEditor() { 2219 return false; 2220 } 2221 2222 public ContactDetail addEditor() { //3 2223 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2224 } 2225 public ChargeItemDefinition addEditor(ContactDetail t) { //3 2226 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2227 } 2228 /** 2229 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {2} 2230 */ 2231 public ContactDetail getEditorFirstRep() { 2232 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"editor\""); 2233 } 2234 /** 2235 * not supported on this implementation 2236 */ 2237 @Override 2238 public int getReviewerMax() { 2239 return 0; 2240 } 2241 /** 2242 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the charge item definition.) 2243 */ 2244 public List<ContactDetail> getReviewer() { 2245 return new ArrayList<>(); 2246 } 2247 /** 2248 * @return Returns a reference to <code>this</code> for easy method chaining 2249 */ 2250 public ChargeItemDefinition setReviewer(List<ContactDetail> theReviewer) { 2251 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2252 } 2253 public boolean hasReviewer() { 2254 return false; 2255 } 2256 2257 public ContactDetail addReviewer() { //3 2258 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2259 } 2260 public ChargeItemDefinition addReviewer(ContactDetail t) { //3 2261 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2262 } 2263 /** 2264 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {2} 2265 */ 2266 public ContactDetail getReviewerFirstRep() { 2267 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"reviewer\""); 2268 } 2269 /** 2270 * not supported on this implementation 2271 */ 2272 @Override 2273 public int getEndorserMax() { 2274 return 0; 2275 } 2276 /** 2277 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the charge item definition for use in some setting.) 2278 */ 2279 public List<ContactDetail> getEndorser() { 2280 return new ArrayList<>(); 2281 } 2282 /** 2283 * @return Returns a reference to <code>this</code> for easy method chaining 2284 */ 2285 public ChargeItemDefinition setEndorser(List<ContactDetail> theEndorser) { 2286 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2287 } 2288 public boolean hasEndorser() { 2289 return false; 2290 } 2291 2292 public ContactDetail addEndorser() { //3 2293 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2294 } 2295 public ChargeItemDefinition addEndorser(ContactDetail t) { //3 2296 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2297 } 2298 /** 2299 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {2} 2300 */ 2301 public ContactDetail getEndorserFirstRep() { 2302 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"endorser\""); 2303 } 2304 /** 2305 * not supported on this implementation 2306 */ 2307 @Override 2308 public int getRelatedArtifactMax() { 2309 return 0; 2310 } 2311 /** 2312 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 2313 */ 2314 public List<RelatedArtifact> getRelatedArtifact() { 2315 return new ArrayList<>(); 2316 } 2317 /** 2318 * @return Returns a reference to <code>this</code> for easy method chaining 2319 */ 2320 public ChargeItemDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2321 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2322 } 2323 public boolean hasRelatedArtifact() { 2324 return false; 2325 } 2326 2327 public RelatedArtifact addRelatedArtifact() { //3 2328 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2329 } 2330 public ChargeItemDefinition addRelatedArtifact(RelatedArtifact t) { //3 2331 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2332 } 2333 /** 2334 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {2} 2335 */ 2336 public RelatedArtifact getRelatedArtifactFirstRep() { 2337 throw new Error("The resource type \"ChargeItemDefinition\" does not implement the property \"relatedArtifact\""); 2338 } 2339 protected void listChildren(List<Property> children) { 2340 super.listChildren(children); 2341 children.add(new Property("url", "uri", "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 0, 1, url)); 2342 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2343 children.add(new Property("version", "string", "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version)); 2344 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2345 children.add(new Property("name", "string", "A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2346 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title)); 2347 children.add(new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 2348 children.add(new Property("partOf", "canonical(ChargeItemDefinition)", "A larger definition of which this particular definition is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2349 children.add(new Property("replaces", "canonical(ChargeItemDefinition)", "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2350 children.add(new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, status)); 2351 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2352 children.add(new Property("date", "dateTime", "The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 0, 1, date)); 2353 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.", 0, 1, publisher)); 2354 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2355 children.add(new Property("description", "markdown", "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, description)); 2356 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2357 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the charge item definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2358 children.add(new Property("purpose", "markdown", "Explanation of why this charge item definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2359 children.add(new Property("copyright", "markdown", "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 0, 1, copyright)); 2360 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2361 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 2362 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 2363 children.add(new Property("code", "CodeableConcept", "The defined billing details in this resource pertain to the given billing code.", 0, 1, code)); 2364 children.add(new Property("instance", "Reference(Medication|Substance|Device|DeviceDefinition|ActivityDefinition|PlanDefinition|HealthcareService)", "The defined billing details in this resource pertain to the given product instance(s).", 0, java.lang.Integer.MAX_VALUE, instance)); 2365 children.add(new Property("applicability", "", "Expressions that describe applicability criteria for the billing code.", 0, java.lang.Integer.MAX_VALUE, applicability)); 2366 children.add(new Property("propertyGroup", "", "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 0, java.lang.Integer.MAX_VALUE, propertyGroup)); 2367 } 2368 2369 @Override 2370 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2371 switch (_hash) { 2372 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 0, 1, url); 2373 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2374 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 0, 1, version); 2375 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2376 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2377 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2378 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2379 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the ChargeItemDefinition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2380 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title); 2381 case -1076333435: /*derivedFromUri*/ return new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 2382 case -995410646: /*partOf*/ return new Property("partOf", "canonical(ChargeItemDefinition)", "A larger definition of which this particular definition is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2383 case -430332865: /*replaces*/ return new Property("replaces", "canonical(ChargeItemDefinition)", "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 0, java.lang.Integer.MAX_VALUE, replaces); 2384 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, status); 2385 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2386 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the charge item definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 0, 1, date); 2387 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the charge item definition.", 0, 1, publisher); 2388 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2389 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, description); 2390 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2391 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the charge item definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2392 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this charge item definition is needed and why it has been designed as it has.", 0, 1, purpose); 2393 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 0, 1, copyright); 2394 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2395 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 2396 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 2397 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The defined billing details in this resource pertain to the given billing code.", 0, 1, code); 2398 case 555127957: /*instance*/ return new Property("instance", "Reference(Medication|Substance|Device|DeviceDefinition|ActivityDefinition|PlanDefinition|HealthcareService)", "The defined billing details in this resource pertain to the given product instance(s).", 0, java.lang.Integer.MAX_VALUE, instance); 2399 case -1526770491: /*applicability*/ return new Property("applicability", "", "Expressions that describe applicability criteria for the billing code.", 0, java.lang.Integer.MAX_VALUE, applicability); 2400 case -1041594966: /*propertyGroup*/ return new Property("propertyGroup", "", "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 0, java.lang.Integer.MAX_VALUE, propertyGroup); 2401 default: return super.getNamedProperty(_hash, _name, _checkValid); 2402 } 2403 2404 } 2405 2406 @Override 2407 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2408 switch (hash) { 2409 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2410 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2411 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2412 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2413 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2414 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2415 case -1076333435: /*derivedFromUri*/ return this.derivedFromUri == null ? new Base[0] : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 2416 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // CanonicalType 2417 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 2418 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2419 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2420 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2421 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2422 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2423 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2424 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2425 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2426 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2427 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2428 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2429 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 2430 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 2431 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2432 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // Reference 2433 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 2434 case -1041594966: /*propertyGroup*/ return this.propertyGroup == null ? new Base[0] : this.propertyGroup.toArray(new Base[this.propertyGroup.size()]); // ChargeItemDefinitionPropertyGroupComponent 2435 default: return super.getProperty(hash, name, checkValid); 2436 } 2437 2438 } 2439 2440 @Override 2441 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2442 switch (hash) { 2443 case 116079: // url 2444 this.url = TypeConvertor.castToUri(value); // UriType 2445 return value; 2446 case -1618432855: // identifier 2447 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2448 return value; 2449 case 351608024: // version 2450 this.version = TypeConvertor.castToString(value); // StringType 2451 return value; 2452 case 1508158071: // versionAlgorithm 2453 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2454 return value; 2455 case 3373707: // name 2456 this.name = TypeConvertor.castToString(value); // StringType 2457 return value; 2458 case 110371416: // title 2459 this.title = TypeConvertor.castToString(value); // StringType 2460 return value; 2461 case -1076333435: // derivedFromUri 2462 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); // UriType 2463 return value; 2464 case -995410646: // partOf 2465 this.getPartOf().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2466 return value; 2467 case -430332865: // replaces 2468 this.getReplaces().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2469 return value; 2470 case -892481550: // status 2471 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2472 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2473 return value; 2474 case -404562712: // experimental 2475 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2476 return value; 2477 case 3076014: // date 2478 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2479 return value; 2480 case 1447404028: // publisher 2481 this.publisher = TypeConvertor.castToString(value); // StringType 2482 return value; 2483 case 951526432: // contact 2484 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2485 return value; 2486 case -1724546052: // description 2487 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2488 return value; 2489 case -669707736: // useContext 2490 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2491 return value; 2492 case -507075711: // jurisdiction 2493 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2494 return value; 2495 case -220463842: // purpose 2496 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2497 return value; 2498 case 1522889671: // copyright 2499 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2500 return value; 2501 case 765157229: // copyrightLabel 2502 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2503 return value; 2504 case 223539345: // approvalDate 2505 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2506 return value; 2507 case -1687512484: // lastReviewDate 2508 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2509 return value; 2510 case 3059181: // code 2511 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2512 return value; 2513 case 555127957: // instance 2514 this.getInstance().add(TypeConvertor.castToReference(value)); // Reference 2515 return value; 2516 case -1526770491: // applicability 2517 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 2518 return value; 2519 case -1041594966: // propertyGroup 2520 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); // ChargeItemDefinitionPropertyGroupComponent 2521 return value; 2522 default: return super.setProperty(hash, name, value); 2523 } 2524 2525 } 2526 2527 @Override 2528 public Base setProperty(String name, Base value) throws FHIRException { 2529 if (name.equals("url")) { 2530 this.url = TypeConvertor.castToUri(value); // UriType 2531 } else if (name.equals("identifier")) { 2532 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2533 } else if (name.equals("version")) { 2534 this.version = TypeConvertor.castToString(value); // StringType 2535 } else if (name.equals("versionAlgorithm[x]")) { 2536 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2537 } else if (name.equals("name")) { 2538 this.name = TypeConvertor.castToString(value); // StringType 2539 } else if (name.equals("title")) { 2540 this.title = TypeConvertor.castToString(value); // StringType 2541 } else if (name.equals("derivedFromUri")) { 2542 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); 2543 } else if (name.equals("partOf")) { 2544 this.getPartOf().add(TypeConvertor.castToCanonical(value)); 2545 } else if (name.equals("replaces")) { 2546 this.getReplaces().add(TypeConvertor.castToCanonical(value)); 2547 } else if (name.equals("status")) { 2548 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2549 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2550 } else if (name.equals("experimental")) { 2551 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2552 } else if (name.equals("date")) { 2553 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2554 } else if (name.equals("publisher")) { 2555 this.publisher = TypeConvertor.castToString(value); // StringType 2556 } else if (name.equals("contact")) { 2557 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2558 } else if (name.equals("description")) { 2559 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2560 } else if (name.equals("useContext")) { 2561 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2562 } else if (name.equals("jurisdiction")) { 2563 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2564 } else if (name.equals("purpose")) { 2565 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2566 } else if (name.equals("copyright")) { 2567 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2568 } else if (name.equals("copyrightLabel")) { 2569 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2570 } else if (name.equals("approvalDate")) { 2571 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2572 } else if (name.equals("lastReviewDate")) { 2573 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2574 } else if (name.equals("code")) { 2575 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2576 } else if (name.equals("instance")) { 2577 this.getInstance().add(TypeConvertor.castToReference(value)); 2578 } else if (name.equals("applicability")) { 2579 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 2580 } else if (name.equals("propertyGroup")) { 2581 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); 2582 } else 2583 return super.setProperty(name, value); 2584 return value; 2585 } 2586 2587 @Override 2588 public void removeChild(String name, Base value) throws FHIRException { 2589 if (name.equals("url")) { 2590 this.url = null; 2591 } else if (name.equals("identifier")) { 2592 this.getIdentifier().remove(value); 2593 } else if (name.equals("version")) { 2594 this.version = null; 2595 } else if (name.equals("versionAlgorithm[x]")) { 2596 this.versionAlgorithm = null; 2597 } else if (name.equals("name")) { 2598 this.name = null; 2599 } else if (name.equals("title")) { 2600 this.title = null; 2601 } else if (name.equals("derivedFromUri")) { 2602 this.getDerivedFromUri().remove(value); 2603 } else if (name.equals("partOf")) { 2604 this.getPartOf().remove(value); 2605 } else if (name.equals("replaces")) { 2606 this.getReplaces().remove(value); 2607 } else if (name.equals("status")) { 2608 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2609 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2610 } else if (name.equals("experimental")) { 2611 this.experimental = null; 2612 } else if (name.equals("date")) { 2613 this.date = null; 2614 } else if (name.equals("publisher")) { 2615 this.publisher = null; 2616 } else if (name.equals("contact")) { 2617 this.getContact().remove(value); 2618 } else if (name.equals("description")) { 2619 this.description = null; 2620 } else if (name.equals("useContext")) { 2621 this.getUseContext().remove(value); 2622 } else if (name.equals("jurisdiction")) { 2623 this.getJurisdiction().remove(value); 2624 } else if (name.equals("purpose")) { 2625 this.purpose = null; 2626 } else if (name.equals("copyright")) { 2627 this.copyright = null; 2628 } else if (name.equals("copyrightLabel")) { 2629 this.copyrightLabel = null; 2630 } else if (name.equals("approvalDate")) { 2631 this.approvalDate = null; 2632 } else if (name.equals("lastReviewDate")) { 2633 this.lastReviewDate = null; 2634 } else if (name.equals("code")) { 2635 this.code = null; 2636 } else if (name.equals("instance")) { 2637 this.getInstance().remove(value); 2638 } else if (name.equals("applicability")) { 2639 this.getApplicability().remove((ChargeItemDefinitionApplicabilityComponent) value); 2640 } else if (name.equals("propertyGroup")) { 2641 this.getPropertyGroup().remove((ChargeItemDefinitionPropertyGroupComponent) value); 2642 } else 2643 super.removeChild(name, value); 2644 2645 } 2646 2647 @Override 2648 public Base makeProperty(int hash, String name) throws FHIRException { 2649 switch (hash) { 2650 case 116079: return getUrlElement(); 2651 case -1618432855: return addIdentifier(); 2652 case 351608024: return getVersionElement(); 2653 case -115699031: return getVersionAlgorithm(); 2654 case 1508158071: return getVersionAlgorithm(); 2655 case 3373707: return getNameElement(); 2656 case 110371416: return getTitleElement(); 2657 case -1076333435: return addDerivedFromUriElement(); 2658 case -995410646: return addPartOfElement(); 2659 case -430332865: return addReplacesElement(); 2660 case -892481550: return getStatusElement(); 2661 case -404562712: return getExperimentalElement(); 2662 case 3076014: return getDateElement(); 2663 case 1447404028: return getPublisherElement(); 2664 case 951526432: return addContact(); 2665 case -1724546052: return getDescriptionElement(); 2666 case -669707736: return addUseContext(); 2667 case -507075711: return addJurisdiction(); 2668 case -220463842: return getPurposeElement(); 2669 case 1522889671: return getCopyrightElement(); 2670 case 765157229: return getCopyrightLabelElement(); 2671 case 223539345: return getApprovalDateElement(); 2672 case -1687512484: return getLastReviewDateElement(); 2673 case 3059181: return getCode(); 2674 case 555127957: return addInstance(); 2675 case -1526770491: return addApplicability(); 2676 case -1041594966: return addPropertyGroup(); 2677 default: return super.makeProperty(hash, name); 2678 } 2679 2680 } 2681 2682 @Override 2683 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2684 switch (hash) { 2685 case 116079: /*url*/ return new String[] {"uri"}; 2686 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2687 case 351608024: /*version*/ return new String[] {"string"}; 2688 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2689 case 3373707: /*name*/ return new String[] {"string"}; 2690 case 110371416: /*title*/ return new String[] {"string"}; 2691 case -1076333435: /*derivedFromUri*/ return new String[] {"uri"}; 2692 case -995410646: /*partOf*/ return new String[] {"canonical"}; 2693 case -430332865: /*replaces*/ return new String[] {"canonical"}; 2694 case -892481550: /*status*/ return new String[] {"code"}; 2695 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2696 case 3076014: /*date*/ return new String[] {"dateTime"}; 2697 case 1447404028: /*publisher*/ return new String[] {"string"}; 2698 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2699 case -1724546052: /*description*/ return new String[] {"markdown"}; 2700 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2701 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2702 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2703 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2704 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2705 case 223539345: /*approvalDate*/ return new String[] {"date"}; 2706 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 2707 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2708 case 555127957: /*instance*/ return new String[] {"Reference"}; 2709 case -1526770491: /*applicability*/ return new String[] {}; 2710 case -1041594966: /*propertyGroup*/ return new String[] {}; 2711 default: return super.getTypesForProperty(hash, name); 2712 } 2713 2714 } 2715 2716 @Override 2717 public Base addChild(String name) throws FHIRException { 2718 if (name.equals("url")) { 2719 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.url"); 2720 } 2721 else if (name.equals("identifier")) { 2722 return addIdentifier(); 2723 } 2724 else if (name.equals("version")) { 2725 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.version"); 2726 } 2727 else if (name.equals("versionAlgorithmString")) { 2728 this.versionAlgorithm = new StringType(); 2729 return this.versionAlgorithm; 2730 } 2731 else if (name.equals("versionAlgorithmCoding")) { 2732 this.versionAlgorithm = new Coding(); 2733 return this.versionAlgorithm; 2734 } 2735 else if (name.equals("name")) { 2736 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.name"); 2737 } 2738 else if (name.equals("title")) { 2739 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.title"); 2740 } 2741 else if (name.equals("derivedFromUri")) { 2742 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.derivedFromUri"); 2743 } 2744 else if (name.equals("partOf")) { 2745 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.partOf"); 2746 } 2747 else if (name.equals("replaces")) { 2748 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.replaces"); 2749 } 2750 else if (name.equals("status")) { 2751 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.status"); 2752 } 2753 else if (name.equals("experimental")) { 2754 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.experimental"); 2755 } 2756 else if (name.equals("date")) { 2757 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.date"); 2758 } 2759 else if (name.equals("publisher")) { 2760 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.publisher"); 2761 } 2762 else if (name.equals("contact")) { 2763 return addContact(); 2764 } 2765 else if (name.equals("description")) { 2766 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.description"); 2767 } 2768 else if (name.equals("useContext")) { 2769 return addUseContext(); 2770 } 2771 else if (name.equals("jurisdiction")) { 2772 return addJurisdiction(); 2773 } 2774 else if (name.equals("purpose")) { 2775 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.purpose"); 2776 } 2777 else if (name.equals("copyright")) { 2778 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.copyright"); 2779 } 2780 else if (name.equals("copyrightLabel")) { 2781 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.copyrightLabel"); 2782 } 2783 else if (name.equals("approvalDate")) { 2784 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.approvalDate"); 2785 } 2786 else if (name.equals("lastReviewDate")) { 2787 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.lastReviewDate"); 2788 } 2789 else if (name.equals("code")) { 2790 this.code = new CodeableConcept(); 2791 return this.code; 2792 } 2793 else if (name.equals("instance")) { 2794 return addInstance(); 2795 } 2796 else if (name.equals("applicability")) { 2797 return addApplicability(); 2798 } 2799 else if (name.equals("propertyGroup")) { 2800 return addPropertyGroup(); 2801 } 2802 else 2803 return super.addChild(name); 2804 } 2805 2806 public String fhirType() { 2807 return "ChargeItemDefinition"; 2808 2809 } 2810 2811 public ChargeItemDefinition copy() { 2812 ChargeItemDefinition dst = new ChargeItemDefinition(); 2813 copyValues(dst); 2814 return dst; 2815 } 2816 2817 public void copyValues(ChargeItemDefinition dst) { 2818 super.copyValues(dst); 2819 dst.url = url == null ? null : url.copy(); 2820 if (identifier != null) { 2821 dst.identifier = new ArrayList<Identifier>(); 2822 for (Identifier i : identifier) 2823 dst.identifier.add(i.copy()); 2824 }; 2825 dst.version = version == null ? null : version.copy(); 2826 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2827 dst.name = name == null ? null : name.copy(); 2828 dst.title = title == null ? null : title.copy(); 2829 if (derivedFromUri != null) { 2830 dst.derivedFromUri = new ArrayList<UriType>(); 2831 for (UriType i : derivedFromUri) 2832 dst.derivedFromUri.add(i.copy()); 2833 }; 2834 if (partOf != null) { 2835 dst.partOf = new ArrayList<CanonicalType>(); 2836 for (CanonicalType i : partOf) 2837 dst.partOf.add(i.copy()); 2838 }; 2839 if (replaces != null) { 2840 dst.replaces = new ArrayList<CanonicalType>(); 2841 for (CanonicalType i : replaces) 2842 dst.replaces.add(i.copy()); 2843 }; 2844 dst.status = status == null ? null : status.copy(); 2845 dst.experimental = experimental == null ? null : experimental.copy(); 2846 dst.date = date == null ? null : date.copy(); 2847 dst.publisher = publisher == null ? null : publisher.copy(); 2848 if (contact != null) { 2849 dst.contact = new ArrayList<ContactDetail>(); 2850 for (ContactDetail i : contact) 2851 dst.contact.add(i.copy()); 2852 }; 2853 dst.description = description == null ? null : description.copy(); 2854 if (useContext != null) { 2855 dst.useContext = new ArrayList<UsageContext>(); 2856 for (UsageContext i : useContext) 2857 dst.useContext.add(i.copy()); 2858 }; 2859 if (jurisdiction != null) { 2860 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2861 for (CodeableConcept i : jurisdiction) 2862 dst.jurisdiction.add(i.copy()); 2863 }; 2864 dst.purpose = purpose == null ? null : purpose.copy(); 2865 dst.copyright = copyright == null ? null : copyright.copy(); 2866 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2867 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2868 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2869 dst.code = code == null ? null : code.copy(); 2870 if (instance != null) { 2871 dst.instance = new ArrayList<Reference>(); 2872 for (Reference i : instance) 2873 dst.instance.add(i.copy()); 2874 }; 2875 if (applicability != null) { 2876 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2877 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 2878 dst.applicability.add(i.copy()); 2879 }; 2880 if (propertyGroup != null) { 2881 dst.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2882 for (ChargeItemDefinitionPropertyGroupComponent i : propertyGroup) 2883 dst.propertyGroup.add(i.copy()); 2884 }; 2885 } 2886 2887 protected ChargeItemDefinition typedCopy() { 2888 return copy(); 2889 } 2890 2891 @Override 2892 public boolean equalsDeep(Base other_) { 2893 if (!super.equalsDeep(other_)) 2894 return false; 2895 if (!(other_ instanceof ChargeItemDefinition)) 2896 return false; 2897 ChargeItemDefinition o = (ChargeItemDefinition) other_; 2898 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2899 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2900 && compareDeep(derivedFromUri, o.derivedFromUri, true) && compareDeep(partOf, o.partOf, true) && compareDeep(replaces, o.replaces, true) 2901 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 2902 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 2903 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 2904 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 2905 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 2906 && compareDeep(code, o.code, true) && compareDeep(instance, o.instance, true) && compareDeep(applicability, o.applicability, true) 2907 && compareDeep(propertyGroup, o.propertyGroup, true); 2908 } 2909 2910 @Override 2911 public boolean equalsShallow(Base other_) { 2912 if (!super.equalsShallow(other_)) 2913 return false; 2914 if (!(other_ instanceof ChargeItemDefinition)) 2915 return false; 2916 ChargeItemDefinition o = (ChargeItemDefinition) other_; 2917 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2918 && compareValues(title, o.title, true) && compareValues(derivedFromUri, o.derivedFromUri, true) && compareValues(partOf, o.partOf, true) 2919 && compareValues(replaces, o.replaces, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 2920 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 2921 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 2922 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 2923 ; 2924 } 2925 2926 public boolean isEmpty() { 2927 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2928 , versionAlgorithm, name, title, derivedFromUri, partOf, replaces, status, experimental 2929 , date, publisher, contact, description, useContext, jurisdiction, purpose, copyright 2930 , copyrightLabel, approvalDate, lastReviewDate, code, instance, applicability, propertyGroup 2931 ); 2932 } 2933 2934 @Override 2935 public ResourceType getResourceType() { 2936 return ResourceType.ChargeItemDefinition; 2937 } 2938 2939 /** 2940 * Search parameter: <b>context-quantity</b> 2941 * <p> 2942 * Description: <b>Multiple Resources: 2943 2944* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2945* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2946* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2947* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2948* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2949* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2950* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2951* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2952* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2953* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2954* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2955* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2956* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2957* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2958* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2959* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2960* [Library](library.html): A quantity- or range-valued use context assigned to the library 2961* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2962* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2963* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2964* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2965* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2966* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2967* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2968* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2969* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2970* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2971* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2972* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2973* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2974</b><br> 2975 * Type: <b>quantity</b><br> 2976 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2977 * </p> 2978 */ 2979 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2980 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2981 /** 2982 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2983 * <p> 2984 * Description: <b>Multiple Resources: 2985 2986* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2987* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2988* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2989* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2990* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2991* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2992* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2993* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2994* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2995* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2996* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2997* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2998* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2999* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3000* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3001* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3002* [Library](library.html): A quantity- or range-valued use context assigned to the library 3003* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3004* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3005* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3006* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3007* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3008* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3009* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3010* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3011* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3012* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3013* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3014* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3015* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3016</b><br> 3017 * Type: <b>quantity</b><br> 3018 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3019 * </p> 3020 */ 3021 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3022 3023 /** 3024 * Search parameter: <b>context-type-quantity</b> 3025 * <p> 3026 * Description: <b>Multiple Resources: 3027 3028* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3029* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3030* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3031* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3032* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3033* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3034* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3035* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3036* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3037* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3038* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3039* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3040* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3041* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3042* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3043* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3044* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3045* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3046* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3047* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3048* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3049* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3050* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3051* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3052* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3053* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3054* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3055* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3056* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3057* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3058</b><br> 3059 * Type: <b>composite</b><br> 3060 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3061 * </p> 3062 */ 3063 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3064 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3065 /** 3066 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3067 * <p> 3068 * Description: <b>Multiple Resources: 3069 3070* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3071* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3072* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3073* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3074* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3075* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3076* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3077* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3078* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3079* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3080* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3081* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3082* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3083* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3084* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3085* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3086* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3087* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3088* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3089* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3090* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3091* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3092* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3093* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3094* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3095* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3096* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3097* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3098* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3099* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3100</b><br> 3101 * Type: <b>composite</b><br> 3102 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3103 * </p> 3104 */ 3105 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3106 3107 /** 3108 * Search parameter: <b>context-type-value</b> 3109 * <p> 3110 * Description: <b>Multiple Resources: 3111 3112* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3113* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3114* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3115* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3116* [Citation](citation.html): A use context type and value assigned to the citation 3117* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3118* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3119* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3120* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3121* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3122* [Evidence](evidence.html): A use context type and value assigned to the evidence 3123* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3124* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3125* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3126* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3127* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3128* [Library](library.html): A use context type and value assigned to the library 3129* [Measure](measure.html): A use context type and value assigned to the measure 3130* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3131* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3132* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3133* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3134* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3135* [Requirements](requirements.html): A use context type and value assigned to the requirements 3136* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3137* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3138* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3139* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3140* [TestScript](testscript.html): A use context type and value assigned to the test script 3141* [ValueSet](valueset.html): A use context type and value assigned to the value set 3142</b><br> 3143 * Type: <b>composite</b><br> 3144 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3145 * </p> 3146 */ 3147 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3148 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3149 /** 3150 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3151 * <p> 3152 * Description: <b>Multiple Resources: 3153 3154* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3155* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3156* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3157* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3158* [Citation](citation.html): A use context type and value assigned to the citation 3159* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3160* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3161* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3162* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3163* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3164* [Evidence](evidence.html): A use context type and value assigned to the evidence 3165* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3166* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3167* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3168* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3169* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3170* [Library](library.html): A use context type and value assigned to the library 3171* [Measure](measure.html): A use context type and value assigned to the measure 3172* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3173* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3174* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3175* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3176* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3177* [Requirements](requirements.html): A use context type and value assigned to the requirements 3178* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3179* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3180* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3181* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3182* [TestScript](testscript.html): A use context type and value assigned to the test script 3183* [ValueSet](valueset.html): A use context type and value assigned to the value set 3184</b><br> 3185 * Type: <b>composite</b><br> 3186 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3187 * </p> 3188 */ 3189 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3190 3191 /** 3192 * Search parameter: <b>context-type</b> 3193 * <p> 3194 * Description: <b>Multiple Resources: 3195 3196* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3197* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3198* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3199* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3200* [Citation](citation.html): A type of use context assigned to the citation 3201* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3202* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3203* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3204* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3205* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3206* [Evidence](evidence.html): A type of use context assigned to the evidence 3207* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3208* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3209* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3210* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3211* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3212* [Library](library.html): A type of use context assigned to the library 3213* [Measure](measure.html): A type of use context assigned to the measure 3214* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3215* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3216* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3217* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3218* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3219* [Requirements](requirements.html): A type of use context assigned to the requirements 3220* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3221* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3222* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3223* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3224* [TestScript](testscript.html): A type of use context assigned to the test script 3225* [ValueSet](valueset.html): A type of use context assigned to the value set 3226</b><br> 3227 * Type: <b>token</b><br> 3228 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3229 * </p> 3230 */ 3231 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3232 public static final String SP_CONTEXT_TYPE = "context-type"; 3233 /** 3234 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3235 * <p> 3236 * Description: <b>Multiple Resources: 3237 3238* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3239* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3240* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3241* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3242* [Citation](citation.html): A type of use context assigned to the citation 3243* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3244* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3245* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3246* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3247* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3248* [Evidence](evidence.html): A type of use context assigned to the evidence 3249* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3250* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3251* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3252* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3253* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3254* [Library](library.html): A type of use context assigned to the library 3255* [Measure](measure.html): A type of use context assigned to the measure 3256* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3257* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3258* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3259* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3260* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3261* [Requirements](requirements.html): A type of use context assigned to the requirements 3262* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3263* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3264* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3265* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3266* [TestScript](testscript.html): A type of use context assigned to the test script 3267* [ValueSet](valueset.html): A type of use context assigned to the value set 3268</b><br> 3269 * Type: <b>token</b><br> 3270 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3271 * </p> 3272 */ 3273 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3274 3275 /** 3276 * Search parameter: <b>context</b> 3277 * <p> 3278 * Description: <b>Multiple Resources: 3279 3280* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3281* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3282* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3283* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3284* [Citation](citation.html): A use context assigned to the citation 3285* [CodeSystem](codesystem.html): A use context assigned to the code system 3286* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3287* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3288* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3289* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3290* [Evidence](evidence.html): A use context assigned to the evidence 3291* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3292* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3293* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3294* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3295* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3296* [Library](library.html): A use context assigned to the library 3297* [Measure](measure.html): A use context assigned to the measure 3298* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3299* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3300* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3301* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3302* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3303* [Requirements](requirements.html): A use context assigned to the requirements 3304* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3305* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3306* [StructureMap](structuremap.html): A use context assigned to the structure map 3307* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3308* [TestScript](testscript.html): A use context assigned to the test script 3309* [ValueSet](valueset.html): A use context assigned to the value set 3310</b><br> 3311 * Type: <b>token</b><br> 3312 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3313 * </p> 3314 */ 3315 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3316 public static final String SP_CONTEXT = "context"; 3317 /** 3318 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3319 * <p> 3320 * Description: <b>Multiple Resources: 3321 3322* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3323* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3324* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3325* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3326* [Citation](citation.html): A use context assigned to the citation 3327* [CodeSystem](codesystem.html): A use context assigned to the code system 3328* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3329* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3330* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3331* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3332* [Evidence](evidence.html): A use context assigned to the evidence 3333* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3334* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3335* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3336* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3337* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3338* [Library](library.html): A use context assigned to the library 3339* [Measure](measure.html): A use context assigned to the measure 3340* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3341* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3342* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3343* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3344* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3345* [Requirements](requirements.html): A use context assigned to the requirements 3346* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3347* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3348* [StructureMap](structuremap.html): A use context assigned to the structure map 3349* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3350* [TestScript](testscript.html): A use context assigned to the test script 3351* [ValueSet](valueset.html): A use context assigned to the value set 3352</b><br> 3353 * Type: <b>token</b><br> 3354 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3355 * </p> 3356 */ 3357 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3358 3359 /** 3360 * Search parameter: <b>date</b> 3361 * <p> 3362 * Description: <b>Multiple Resources: 3363 3364* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3365* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3366* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3367* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3368* [Citation](citation.html): The citation publication date 3369* [CodeSystem](codesystem.html): The code system publication date 3370* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3371* [ConceptMap](conceptmap.html): The concept map publication date 3372* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3373* [EventDefinition](eventdefinition.html): The event definition publication date 3374* [Evidence](evidence.html): The evidence publication date 3375* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3376* [ExampleScenario](examplescenario.html): The example scenario publication date 3377* [GraphDefinition](graphdefinition.html): The graph definition publication date 3378* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3379* [Library](library.html): The library publication date 3380* [Measure](measure.html): The measure publication date 3381* [MessageDefinition](messagedefinition.html): The message definition publication date 3382* [NamingSystem](namingsystem.html): The naming system publication date 3383* [OperationDefinition](operationdefinition.html): The operation definition publication date 3384* [PlanDefinition](plandefinition.html): The plan definition publication date 3385* [Questionnaire](questionnaire.html): The questionnaire publication date 3386* [Requirements](requirements.html): The requirements publication date 3387* [SearchParameter](searchparameter.html): The search parameter publication date 3388* [StructureDefinition](structuredefinition.html): The structure definition publication date 3389* [StructureMap](structuremap.html): The structure map publication date 3390* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3391* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3392* [TestScript](testscript.html): The test script publication date 3393* [ValueSet](valueset.html): The value set publication date 3394</b><br> 3395 * Type: <b>date</b><br> 3396 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3397 * </p> 3398 */ 3399 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3400 public static final String SP_DATE = "date"; 3401 /** 3402 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3403 * <p> 3404 * Description: <b>Multiple Resources: 3405 3406* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3407* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3408* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3409* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3410* [Citation](citation.html): The citation publication date 3411* [CodeSystem](codesystem.html): The code system publication date 3412* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3413* [ConceptMap](conceptmap.html): The concept map publication date 3414* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3415* [EventDefinition](eventdefinition.html): The event definition publication date 3416* [Evidence](evidence.html): The evidence publication date 3417* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3418* [ExampleScenario](examplescenario.html): The example scenario publication date 3419* [GraphDefinition](graphdefinition.html): The graph definition publication date 3420* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3421* [Library](library.html): The library publication date 3422* [Measure](measure.html): The measure publication date 3423* [MessageDefinition](messagedefinition.html): The message definition publication date 3424* [NamingSystem](namingsystem.html): The naming system publication date 3425* [OperationDefinition](operationdefinition.html): The operation definition publication date 3426* [PlanDefinition](plandefinition.html): The plan definition publication date 3427* [Questionnaire](questionnaire.html): The questionnaire publication date 3428* [Requirements](requirements.html): The requirements publication date 3429* [SearchParameter](searchparameter.html): The search parameter publication date 3430* [StructureDefinition](structuredefinition.html): The structure definition publication date 3431* [StructureMap](structuremap.html): The structure map publication date 3432* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3433* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3434* [TestScript](testscript.html): The test script publication date 3435* [ValueSet](valueset.html): The value set publication date 3436</b><br> 3437 * Type: <b>date</b><br> 3438 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3439 * </p> 3440 */ 3441 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3442 3443 /** 3444 * Search parameter: <b>description</b> 3445 * <p> 3446 * Description: <b>Multiple Resources: 3447 3448* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3449* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3450* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3451* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3452* [Citation](citation.html): The description of the citation 3453* [CodeSystem](codesystem.html): The description of the code system 3454* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3455* [ConceptMap](conceptmap.html): The description of the concept map 3456* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3457* [EventDefinition](eventdefinition.html): The description of the event definition 3458* [Evidence](evidence.html): The description of the evidence 3459* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3460* [GraphDefinition](graphdefinition.html): The description of the graph definition 3461* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3462* [Library](library.html): The description of the library 3463* [Measure](measure.html): The description of the measure 3464* [MessageDefinition](messagedefinition.html): The description of the message definition 3465* [NamingSystem](namingsystem.html): The description of the naming system 3466* [OperationDefinition](operationdefinition.html): The description of the operation definition 3467* [PlanDefinition](plandefinition.html): The description of the plan definition 3468* [Questionnaire](questionnaire.html): The description of the questionnaire 3469* [Requirements](requirements.html): The description of the requirements 3470* [SearchParameter](searchparameter.html): The description of the search parameter 3471* [StructureDefinition](structuredefinition.html): The description of the structure definition 3472* [StructureMap](structuremap.html): The description of the structure map 3473* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3474* [TestScript](testscript.html): The description of the test script 3475* [ValueSet](valueset.html): The description of the value set 3476</b><br> 3477 * Type: <b>string</b><br> 3478 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3479 * </p> 3480 */ 3481 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3482 public static final String SP_DESCRIPTION = "description"; 3483 /** 3484 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3485 * <p> 3486 * Description: <b>Multiple Resources: 3487 3488* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3489* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3490* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3491* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3492* [Citation](citation.html): The description of the citation 3493* [CodeSystem](codesystem.html): The description of the code system 3494* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3495* [ConceptMap](conceptmap.html): The description of the concept map 3496* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3497* [EventDefinition](eventdefinition.html): The description of the event definition 3498* [Evidence](evidence.html): The description of the evidence 3499* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3500* [GraphDefinition](graphdefinition.html): The description of the graph definition 3501* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3502* [Library](library.html): The description of the library 3503* [Measure](measure.html): The description of the measure 3504* [MessageDefinition](messagedefinition.html): The description of the message definition 3505* [NamingSystem](namingsystem.html): The description of the naming system 3506* [OperationDefinition](operationdefinition.html): The description of the operation definition 3507* [PlanDefinition](plandefinition.html): The description of the plan definition 3508* [Questionnaire](questionnaire.html): The description of the questionnaire 3509* [Requirements](requirements.html): The description of the requirements 3510* [SearchParameter](searchparameter.html): The description of the search parameter 3511* [StructureDefinition](structuredefinition.html): The description of the structure definition 3512* [StructureMap](structuremap.html): The description of the structure map 3513* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3514* [TestScript](testscript.html): The description of the test script 3515* [ValueSet](valueset.html): The description of the value set 3516</b><br> 3517 * Type: <b>string</b><br> 3518 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3519 * </p> 3520 */ 3521 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3522 3523 /** 3524 * Search parameter: <b>identifier</b> 3525 * <p> 3526 * Description: <b>Multiple Resources: 3527 3528* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3529* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3530* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3531* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3532* [Citation](citation.html): External identifier for the citation 3533* [CodeSystem](codesystem.html): External identifier for the code system 3534* [ConceptMap](conceptmap.html): External identifier for the concept map 3535* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3536* [EventDefinition](eventdefinition.html): External identifier for the event definition 3537* [Evidence](evidence.html): External identifier for the evidence 3538* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3539* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3540* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3541* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3542* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3543* [Library](library.html): External identifier for the library 3544* [Measure](measure.html): External identifier for the measure 3545* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3546* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3547* [NamingSystem](namingsystem.html): External identifier for the naming system 3548* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3549* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3550* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3551* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3552* [Requirements](requirements.html): External identifier for the requirements 3553* [SearchParameter](searchparameter.html): External identifier for the search parameter 3554* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3555* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3556* [StructureMap](structuremap.html): External identifier for the structure map 3557* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3558* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3559* [TestPlan](testplan.html): An identifier for the test plan 3560* [TestScript](testscript.html): External identifier for the test script 3561* [ValueSet](valueset.html): External identifier for the value set 3562</b><br> 3563 * Type: <b>token</b><br> 3564 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3565 * </p> 3566 */ 3567 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3568 public static final String SP_IDENTIFIER = "identifier"; 3569 /** 3570 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3571 * <p> 3572 * Description: <b>Multiple Resources: 3573 3574* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3575* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3576* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3577* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3578* [Citation](citation.html): External identifier for the citation 3579* [CodeSystem](codesystem.html): External identifier for the code system 3580* [ConceptMap](conceptmap.html): External identifier for the concept map 3581* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3582* [EventDefinition](eventdefinition.html): External identifier for the event definition 3583* [Evidence](evidence.html): External identifier for the evidence 3584* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3585* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3586* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3587* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3588* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3589* [Library](library.html): External identifier for the library 3590* [Measure](measure.html): External identifier for the measure 3591* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3592* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3593* [NamingSystem](namingsystem.html): External identifier for the naming system 3594* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3595* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3596* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3597* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3598* [Requirements](requirements.html): External identifier for the requirements 3599* [SearchParameter](searchparameter.html): External identifier for the search parameter 3600* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3601* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3602* [StructureMap](structuremap.html): External identifier for the structure map 3603* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3604* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3605* [TestPlan](testplan.html): An identifier for the test plan 3606* [TestScript](testscript.html): External identifier for the test script 3607* [ValueSet](valueset.html): External identifier for the value set 3608</b><br> 3609 * Type: <b>token</b><br> 3610 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3611 * </p> 3612 */ 3613 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3614 3615 /** 3616 * Search parameter: <b>jurisdiction</b> 3617 * <p> 3618 * Description: <b>Multiple Resources: 3619 3620* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3621* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3622* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3623* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3624* [Citation](citation.html): Intended jurisdiction for the citation 3625* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3626* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3627* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3628* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3629* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3630* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3631* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3632* [Library](library.html): Intended jurisdiction for the library 3633* [Measure](measure.html): Intended jurisdiction for the measure 3634* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3635* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3636* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3637* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3638* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3639* [Requirements](requirements.html): Intended jurisdiction for the requirements 3640* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3641* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3642* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3643* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3644* [TestScript](testscript.html): Intended jurisdiction for the test script 3645* [ValueSet](valueset.html): Intended jurisdiction for the value set 3646</b><br> 3647 * Type: <b>token</b><br> 3648 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3649 * </p> 3650 */ 3651 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3652 public static final String SP_JURISDICTION = "jurisdiction"; 3653 /** 3654 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3655 * <p> 3656 * Description: <b>Multiple Resources: 3657 3658* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3659* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3660* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3661* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3662* [Citation](citation.html): Intended jurisdiction for the citation 3663* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3664* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3665* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3666* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3667* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3668* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3669* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3670* [Library](library.html): Intended jurisdiction for the library 3671* [Measure](measure.html): Intended jurisdiction for the measure 3672* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3673* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3674* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3675* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3676* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3677* [Requirements](requirements.html): Intended jurisdiction for the requirements 3678* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3679* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3680* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3681* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3682* [TestScript](testscript.html): Intended jurisdiction for the test script 3683* [ValueSet](valueset.html): Intended jurisdiction for the value set 3684</b><br> 3685 * Type: <b>token</b><br> 3686 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3687 * </p> 3688 */ 3689 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3690 3691 /** 3692 * Search parameter: <b>publisher</b> 3693 * <p> 3694 * Description: <b>Multiple Resources: 3695 3696* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3697* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3698* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3699* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3700* [Citation](citation.html): Name of the publisher of the citation 3701* [CodeSystem](codesystem.html): Name of the publisher of the code system 3702* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3703* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3704* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3705* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3706* [Evidence](evidence.html): Name of the publisher of the evidence 3707* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3708* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3709* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3710* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3711* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3712* [Library](library.html): Name of the publisher of the library 3713* [Measure](measure.html): Name of the publisher of the measure 3714* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3715* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3716* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3717* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3718* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3719* [Requirements](requirements.html): Name of the publisher of the requirements 3720* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3721* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3722* [StructureMap](structuremap.html): Name of the publisher of the structure map 3723* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3724* [TestScript](testscript.html): Name of the publisher of the test script 3725* [ValueSet](valueset.html): Name of the publisher of the value set 3726</b><br> 3727 * Type: <b>string</b><br> 3728 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3729 * </p> 3730 */ 3731 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3732 public static final String SP_PUBLISHER = "publisher"; 3733 /** 3734 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3735 * <p> 3736 * Description: <b>Multiple Resources: 3737 3738* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3739* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3740* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3741* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3742* [Citation](citation.html): Name of the publisher of the citation 3743* [CodeSystem](codesystem.html): Name of the publisher of the code system 3744* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3745* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3746* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3747* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3748* [Evidence](evidence.html): Name of the publisher of the evidence 3749* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3750* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3751* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3752* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3753* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3754* [Library](library.html): Name of the publisher of the library 3755* [Measure](measure.html): Name of the publisher of the measure 3756* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3757* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3758* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3759* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3760* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3761* [Requirements](requirements.html): Name of the publisher of the requirements 3762* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3763* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3764* [StructureMap](structuremap.html): Name of the publisher of the structure map 3765* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3766* [TestScript](testscript.html): Name of the publisher of the test script 3767* [ValueSet](valueset.html): Name of the publisher of the value set 3768</b><br> 3769 * Type: <b>string</b><br> 3770 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3771 * </p> 3772 */ 3773 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3774 3775 /** 3776 * Search parameter: <b>status</b> 3777 * <p> 3778 * Description: <b>Multiple Resources: 3779 3780* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3781* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3782* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3783* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3784* [Citation](citation.html): The current status of the citation 3785* [CodeSystem](codesystem.html): The current status of the code system 3786* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3787* [ConceptMap](conceptmap.html): The current status of the concept map 3788* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3789* [EventDefinition](eventdefinition.html): The current status of the event definition 3790* [Evidence](evidence.html): The current status of the evidence 3791* [EvidenceReport](evidencereport.html): The current status of the evidence report 3792* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3793* [ExampleScenario](examplescenario.html): The current status of the example scenario 3794* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3795* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3796* [Library](library.html): The current status of the library 3797* [Measure](measure.html): The current status of the measure 3798* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3799* [MessageDefinition](messagedefinition.html): The current status of the message definition 3800* [NamingSystem](namingsystem.html): The current status of the naming system 3801* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3802* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3803* [PlanDefinition](plandefinition.html): The current status of the plan definition 3804* [Questionnaire](questionnaire.html): The current status of the questionnaire 3805* [Requirements](requirements.html): The current status of the requirements 3806* [SearchParameter](searchparameter.html): The current status of the search parameter 3807* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3808* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3809* [StructureMap](structuremap.html): The current status of the structure map 3810* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3811* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3812* [TestPlan](testplan.html): The current status of the test plan 3813* [TestScript](testscript.html): The current status of the test script 3814* [ValueSet](valueset.html): The current status of the value set 3815</b><br> 3816 * Type: <b>token</b><br> 3817 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3818 * </p> 3819 */ 3820 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3821 public static final String SP_STATUS = "status"; 3822 /** 3823 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3824 * <p> 3825 * Description: <b>Multiple Resources: 3826 3827* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3828* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3829* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3830* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3831* [Citation](citation.html): The current status of the citation 3832* [CodeSystem](codesystem.html): The current status of the code system 3833* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3834* [ConceptMap](conceptmap.html): The current status of the concept map 3835* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3836* [EventDefinition](eventdefinition.html): The current status of the event definition 3837* [Evidence](evidence.html): The current status of the evidence 3838* [EvidenceReport](evidencereport.html): The current status of the evidence report 3839* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3840* [ExampleScenario](examplescenario.html): The current status of the example scenario 3841* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3842* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3843* [Library](library.html): The current status of the library 3844* [Measure](measure.html): The current status of the measure 3845* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3846* [MessageDefinition](messagedefinition.html): The current status of the message definition 3847* [NamingSystem](namingsystem.html): The current status of the naming system 3848* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3849* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3850* [PlanDefinition](plandefinition.html): The current status of the plan definition 3851* [Questionnaire](questionnaire.html): The current status of the questionnaire 3852* [Requirements](requirements.html): The current status of the requirements 3853* [SearchParameter](searchparameter.html): The current status of the search parameter 3854* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3855* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3856* [StructureMap](structuremap.html): The current status of the structure map 3857* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3858* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3859* [TestPlan](testplan.html): The current status of the test plan 3860* [TestScript](testscript.html): The current status of the test script 3861* [ValueSet](valueset.html): The current status of the value set 3862</b><br> 3863 * Type: <b>token</b><br> 3864 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3865 * </p> 3866 */ 3867 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3868 3869 /** 3870 * Search parameter: <b>title</b> 3871 * <p> 3872 * Description: <b>Multiple Resources: 3873 3874* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3875* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3876* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3877* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3878* [Citation](citation.html): The human-friendly name of the citation 3879* [CodeSystem](codesystem.html): The human-friendly name of the code system 3880* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3881* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3882* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3883* [Evidence](evidence.html): The human-friendly name of the evidence 3884* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3885* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3886* [Library](library.html): The human-friendly name of the library 3887* [Measure](measure.html): The human-friendly name of the measure 3888* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3889* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3890* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3891* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3892* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3893* [Requirements](requirements.html): The human-friendly name of the requirements 3894* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3895* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3896* [StructureMap](structuremap.html): The human-friendly name of the structure map 3897* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3898* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3899* [TestScript](testscript.html): The human-friendly name of the test script 3900* [ValueSet](valueset.html): The human-friendly name of the value set 3901</b><br> 3902 * Type: <b>string</b><br> 3903 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3904 * </p> 3905 */ 3906 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 3907 public static final String SP_TITLE = "title"; 3908 /** 3909 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3910 * <p> 3911 * Description: <b>Multiple Resources: 3912 3913* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3914* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3915* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3916* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3917* [Citation](citation.html): The human-friendly name of the citation 3918* [CodeSystem](codesystem.html): The human-friendly name of the code system 3919* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3920* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3921* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3922* [Evidence](evidence.html): The human-friendly name of the evidence 3923* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3924* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3925* [Library](library.html): The human-friendly name of the library 3926* [Measure](measure.html): The human-friendly name of the measure 3927* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3928* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3929* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3930* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3931* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3932* [Requirements](requirements.html): The human-friendly name of the requirements 3933* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3934* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3935* [StructureMap](structuremap.html): The human-friendly name of the structure map 3936* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3937* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3938* [TestScript](testscript.html): The human-friendly name of the test script 3939* [ValueSet](valueset.html): The human-friendly name of the value set 3940</b><br> 3941 * Type: <b>string</b><br> 3942 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3943 * </p> 3944 */ 3945 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3946 3947 /** 3948 * Search parameter: <b>url</b> 3949 * <p> 3950 * Description: <b>Multiple Resources: 3951 3952* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3953* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3954* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3955* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3956* [Citation](citation.html): The uri that identifies the citation 3957* [CodeSystem](codesystem.html): The uri that identifies the code system 3958* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3959* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3960* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3961* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3962* [Evidence](evidence.html): The uri that identifies the evidence 3963* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3964* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3965* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3966* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3967* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3968* [Library](library.html): The uri that identifies the library 3969* [Measure](measure.html): The uri that identifies the measure 3970* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3971* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3972* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3973* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3974* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3975* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3976* [Requirements](requirements.html): The uri that identifies the requirements 3977* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3978* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3979* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3980* [StructureMap](structuremap.html): The uri that identifies the structure map 3981* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3982* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3983* [TestPlan](testplan.html): The uri that identifies the test plan 3984* [TestScript](testscript.html): The uri that identifies the test script 3985* [ValueSet](valueset.html): The uri that identifies the value set 3986</b><br> 3987 * Type: <b>uri</b><br> 3988 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3989 * </p> 3990 */ 3991 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3992 public static final String SP_URL = "url"; 3993 /** 3994 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3995 * <p> 3996 * Description: <b>Multiple Resources: 3997 3998* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3999* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4000* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4001* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4002* [Citation](citation.html): The uri that identifies the citation 4003* [CodeSystem](codesystem.html): The uri that identifies the code system 4004* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4005* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4006* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4007* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4008* [Evidence](evidence.html): The uri that identifies the evidence 4009* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4010* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4011* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4012* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4013* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4014* [Library](library.html): The uri that identifies the library 4015* [Measure](measure.html): The uri that identifies the measure 4016* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4017* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4018* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4019* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4020* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4021* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4022* [Requirements](requirements.html): The uri that identifies the requirements 4023* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4024* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4025* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4026* [StructureMap](structuremap.html): The uri that identifies the structure map 4027* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4028* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4029* [TestPlan](testplan.html): The uri that identifies the test plan 4030* [TestScript](testscript.html): The uri that identifies the test script 4031* [ValueSet](valueset.html): The uri that identifies the value set 4032</b><br> 4033 * Type: <b>uri</b><br> 4034 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4035 * </p> 4036 */ 4037 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4038 4039 /** 4040 * Search parameter: <b>version</b> 4041 * <p> 4042 * Description: <b>Multiple Resources: 4043 4044* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4045* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4046* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4047* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4048* [Citation](citation.html): The business version of the citation 4049* [CodeSystem](codesystem.html): The business version of the code system 4050* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4051* [ConceptMap](conceptmap.html): The business version of the concept map 4052* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4053* [EventDefinition](eventdefinition.html): The business version of the event definition 4054* [Evidence](evidence.html): The business version of the evidence 4055* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4056* [ExampleScenario](examplescenario.html): The business version of the example scenario 4057* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4058* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4059* [Library](library.html): The business version of the library 4060* [Measure](measure.html): The business version of the measure 4061* [MessageDefinition](messagedefinition.html): The business version of the message definition 4062* [NamingSystem](namingsystem.html): The business version of the naming system 4063* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4064* [PlanDefinition](plandefinition.html): The business version of the plan definition 4065* [Questionnaire](questionnaire.html): The business version of the questionnaire 4066* [Requirements](requirements.html): The business version of the requirements 4067* [SearchParameter](searchparameter.html): The business version of the search parameter 4068* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4069* [StructureMap](structuremap.html): The business version of the structure map 4070* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4071* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4072* [TestScript](testscript.html): The business version of the test script 4073* [ValueSet](valueset.html): The business version of the value set 4074</b><br> 4075 * Type: <b>token</b><br> 4076 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4077 * </p> 4078 */ 4079 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4080 public static final String SP_VERSION = "version"; 4081 /** 4082 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4083 * <p> 4084 * Description: <b>Multiple Resources: 4085 4086* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4087* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4088* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4089* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4090* [Citation](citation.html): The business version of the citation 4091* [CodeSystem](codesystem.html): The business version of the code system 4092* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4093* [ConceptMap](conceptmap.html): The business version of the concept map 4094* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4095* [EventDefinition](eventdefinition.html): The business version of the event definition 4096* [Evidence](evidence.html): The business version of the evidence 4097* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4098* [ExampleScenario](examplescenario.html): The business version of the example scenario 4099* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4100* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4101* [Library](library.html): The business version of the library 4102* [Measure](measure.html): The business version of the measure 4103* [MessageDefinition](messagedefinition.html): The business version of the message definition 4104* [NamingSystem](namingsystem.html): The business version of the naming system 4105* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4106* [PlanDefinition](plandefinition.html): The business version of the plan definition 4107* [Questionnaire](questionnaire.html): The business version of the questionnaire 4108* [Requirements](requirements.html): The business version of the requirements 4109* [SearchParameter](searchparameter.html): The business version of the search parameter 4110* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4111* [StructureMap](structuremap.html): The business version of the structure map 4112* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4113* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4114* [TestScript](testscript.html): The business version of the test script 4115* [ValueSet](valueset.html): The business version of the value set 4116</b><br> 4117 * Type: <b>token</b><br> 4118 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4119 * </p> 4120 */ 4121 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4122 4123 /** 4124 * Search parameter: <b>effective</b> 4125 * <p> 4126 * Description: <b>Multiple Resources: 4127 4128* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4129* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4130* [Citation](citation.html): The time during which the citation is intended to be in use 4131* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4132* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4133* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4134* [Library](library.html): The time during which the library is intended to be in use 4135* [Measure](measure.html): The time during which the measure is intended to be in use 4136* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4137* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4138* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4139* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4140</b><br> 4141 * Type: <b>date</b><br> 4142 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4143 * </p> 4144 */ 4145 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 4146 public static final String SP_EFFECTIVE = "effective"; 4147 /** 4148 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4149 * <p> 4150 * Description: <b>Multiple Resources: 4151 4152* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4153* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4154* [Citation](citation.html): The time during which the citation is intended to be in use 4155* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4156* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4157* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4158* [Library](library.html): The time during which the library is intended to be in use 4159* [Measure](measure.html): The time during which the measure is intended to be in use 4160* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4161* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4162* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4163* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4164</b><br> 4165 * Type: <b>date</b><br> 4166 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4167 * </p> 4168 */ 4169 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4170 4171 4172} 4173