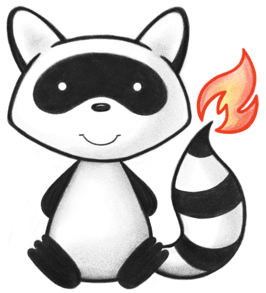
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The Citation Resource enables reference to any knowledge artifact for purposes of identification and attribution. The Citation Resource supports existing reference structures and developing publication practices such as versioning, expressing complex contributorship roles, and referencing computable resources. 052 */ 053@ResourceDef(name="Citation", profile="http://hl7.org/fhir/StructureDefinition/Citation") 054public class Citation extends MetadataResource { 055 056 public enum RelatedArtifactTypeExpanded { 057 /** 058 * Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness. 059 */ 060 DOCUMENTATION, 061 /** 062 * The target artifact is a summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource. 063 */ 064 JUSTIFICATION, 065 /** 066 * Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource. 067 */ 068 CITATION, 069 /** 070 * The previous version of the knowledge artifact, used to establish an ordering of versions of an artifact, independent of the status of each version. 071 */ 072 PREDECESSOR, 073 /** 074 * The subsequent version of the knowledge artfact, used to establish an ordering of versions of an artifact, independent of the status of each version. 075 */ 076 SUCCESSOR, 077 /** 078 * This artifact is derived from the target artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting. The artifact may be derived from one or more target artifacts. 079 */ 080 DERIVEDFROM, 081 /** 082 * This artifact depends on the target artifact. There is a requirement to use the target artifact in the creation or interpretation of this artifact. 083 */ 084 DEPENDSON, 085 /** 086 * This artifact is composed of the target artifact. This artifact is constructed with the target artifact as a component. The target artifact is a part of this artifact. (A dataset is composed of data.). 087 */ 088 COMPOSEDOF, 089 /** 090 * This artifact is a part of the target artifact. The target artifact is composed of this artifact (and possibly other artifacts). 091 */ 092 PARTOF, 093 /** 094 * This artifact amends or changes the target artifact. This artifact adds additional information that is functionally expected to replace information in the target artifact. This artifact replaces a part but not all of the target artifact. 095 */ 096 AMENDS, 097 /** 098 * This artifact is amended with or changed by the target artifact. There is information in this artifact that should be functionally replaced with information in the target artifact. 099 */ 100 AMENDEDWITH, 101 /** 102 * This artifact adds additional information to the target artifact. The additional information does not replace or change information in the target artifact. 103 */ 104 APPENDS, 105 /** 106 * This artifact has additional information in the target artifact. 107 */ 108 APPENDEDWITH, 109 /** 110 * This artifact cites the target artifact. This may be a bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource. 111 */ 112 CITES, 113 /** 114 * This artifact is cited by the target artifact. 115 */ 116 CITEDBY, 117 /** 118 * This artifact contains comments about the target artifact. 119 */ 120 COMMENTSON, 121 /** 122 * This artifact has comments about it in the target artifact. The type of comments may be expressed in the targetClassifier element such as reply, review, editorial, feedback, solicited, unsolicited, structured, unstructured. 123 */ 124 COMMENTIN, 125 /** 126 * This artifact is a container in which the target artifact is contained. A container is a data structure whose instances are collections of other objects. (A database contains the dataset.). 127 */ 128 CONTAINS, 129 /** 130 * This artifact is contained in the target artifact. The target artifact is a data structure whose instances are collections of other objects. 131 */ 132 CONTAINEDIN, 133 /** 134 * This artifact identifies errors and replacement content for the target artifact. 135 */ 136 CORRECTS, 137 /** 138 * This artifact has corrections to it in the target artifact. The target artifact identifies errors and replacement content for this artifact. 139 */ 140 CORRECTIONIN, 141 /** 142 * This artifact replaces or supersedes the target artifact. The target artifact may be considered deprecated. 143 */ 144 REPLACES, 145 /** 146 * This artifact is replaced with or superseded by the target artifact. This artifact may be considered deprecated. 147 */ 148 REPLACEDWITH, 149 /** 150 * This artifact retracts the target artifact. The content that was published in the target artifact should be considered removed from publication and should no longer be considered part of the public record. 151 */ 152 RETRACTS, 153 /** 154 * This artifact is retracted by the target artifact. The content that was published in this artifact should be considered removed from publication and should no longer be considered part of the public record. 155 */ 156 RETRACTEDBY, 157 /** 158 * This artifact is a signature of the target artifact. 159 */ 160 SIGNS, 161 /** 162 * This artifact has characteristics in common with the target artifact. This relationship may be used in systems to ?deduplicate? knowledge artifacts from different sources, or in systems to show ?similar items?. 163 */ 164 SIMILARTO, 165 /** 166 * This artifact provides additional support for the target artifact. The type of support is not documentation as it does not describe, explain, or instruct regarding the target artifact. 167 */ 168 SUPPORTS, 169 /** 170 * The target artifact contains additional information related to the knowledge artifact but is not documentation as the additional information does not describe, explain, or instruct regarding the knowledge artifact content or application. This could include an associated dataset. 171 */ 172 SUPPORTEDWITH, 173 /** 174 * This artifact was generated by transforming the target artifact (e.g., format or language conversion). This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but changes are only apparent in form and there is only one target artifact with the ?transforms? relationship type. 175 */ 176 TRANSFORMS, 177 /** 178 * This artifact was transformed into the target artifact (e.g., by format or language conversion). 179 */ 180 TRANSFORMEDINTO, 181 /** 182 * This artifact was generated by transforming a related artifact (e.g., format or language conversion), noted separately with the ?transforms? relationship type. This transformation used the target artifact to inform the transformation. The target artifact may be a conversion script or translation guide. 183 */ 184 TRANSFORMEDWITH, 185 /** 186 * This artifact provides additional documentation for the target artifact. This could include additional instructions on usage as well as additional information on clinical context or appropriateness. 187 */ 188 DOCUMENTS, 189 /** 190 * The target artifact is a precise description of a concept in this artifact. This may be used when the RelatedArtifact datatype is used in elements contained in this artifact. 191 */ 192 SPECIFICATIONOF, 193 /** 194 * This artifact was created with the target artifact. The target artifact is a tool or support material used in the creation of the artifact, and not content that the artifact was derived from. 195 */ 196 CREATEDWITH, 197 /** 198 * The related artifact is the citation for this artifact. 199 */ 200 CITEAS, 201 /** 202 * A copy of the artifact in a publication with a different artifact identifier. 203 */ 204 REPRINT, 205 /** 206 * The original version of record for which the current artifact is a copy. 207 */ 208 REPRINTOF, 209 /** 210 * added to help the parsers with the generic types 211 */ 212 NULL; 213 public static RelatedArtifactTypeExpanded fromCode(String codeString) throws FHIRException { 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("documentation".equals(codeString)) 217 return DOCUMENTATION; 218 if ("justification".equals(codeString)) 219 return JUSTIFICATION; 220 if ("citation".equals(codeString)) 221 return CITATION; 222 if ("predecessor".equals(codeString)) 223 return PREDECESSOR; 224 if ("successor".equals(codeString)) 225 return SUCCESSOR; 226 if ("derived-from".equals(codeString)) 227 return DERIVEDFROM; 228 if ("depends-on".equals(codeString)) 229 return DEPENDSON; 230 if ("composed-of".equals(codeString)) 231 return COMPOSEDOF; 232 if ("part-of".equals(codeString)) 233 return PARTOF; 234 if ("amends".equals(codeString)) 235 return AMENDS; 236 if ("amended-with".equals(codeString)) 237 return AMENDEDWITH; 238 if ("appends".equals(codeString)) 239 return APPENDS; 240 if ("appended-with".equals(codeString)) 241 return APPENDEDWITH; 242 if ("cites".equals(codeString)) 243 return CITES; 244 if ("cited-by".equals(codeString)) 245 return CITEDBY; 246 if ("comments-on".equals(codeString)) 247 return COMMENTSON; 248 if ("comment-in".equals(codeString)) 249 return COMMENTIN; 250 if ("contains".equals(codeString)) 251 return CONTAINS; 252 if ("contained-in".equals(codeString)) 253 return CONTAINEDIN; 254 if ("corrects".equals(codeString)) 255 return CORRECTS; 256 if ("correction-in".equals(codeString)) 257 return CORRECTIONIN; 258 if ("replaces".equals(codeString)) 259 return REPLACES; 260 if ("replaced-with".equals(codeString)) 261 return REPLACEDWITH; 262 if ("retracts".equals(codeString)) 263 return RETRACTS; 264 if ("retracted-by".equals(codeString)) 265 return RETRACTEDBY; 266 if ("signs".equals(codeString)) 267 return SIGNS; 268 if ("similar-to".equals(codeString)) 269 return SIMILARTO; 270 if ("supports".equals(codeString)) 271 return SUPPORTS; 272 if ("supported-with".equals(codeString)) 273 return SUPPORTEDWITH; 274 if ("transforms".equals(codeString)) 275 return TRANSFORMS; 276 if ("transformed-into".equals(codeString)) 277 return TRANSFORMEDINTO; 278 if ("transformed-with".equals(codeString)) 279 return TRANSFORMEDWITH; 280 if ("documents".equals(codeString)) 281 return DOCUMENTS; 282 if ("specification-of".equals(codeString)) 283 return SPECIFICATIONOF; 284 if ("created-with".equals(codeString)) 285 return CREATEDWITH; 286 if ("cite-as".equals(codeString)) 287 return CITEAS; 288 if ("reprint".equals(codeString)) 289 return REPRINT; 290 if ("reprint-of".equals(codeString)) 291 return REPRINTOF; 292 if (Configuration.isAcceptInvalidEnums()) 293 return null; 294 else 295 throw new FHIRException("Unknown RelatedArtifactTypeExpanded code '"+codeString+"'"); 296 } 297 public String toCode() { 298 switch (this) { 299 case DOCUMENTATION: return "documentation"; 300 case JUSTIFICATION: return "justification"; 301 case CITATION: return "citation"; 302 case PREDECESSOR: return "predecessor"; 303 case SUCCESSOR: return "successor"; 304 case DERIVEDFROM: return "derived-from"; 305 case DEPENDSON: return "depends-on"; 306 case COMPOSEDOF: return "composed-of"; 307 case PARTOF: return "part-of"; 308 case AMENDS: return "amends"; 309 case AMENDEDWITH: return "amended-with"; 310 case APPENDS: return "appends"; 311 case APPENDEDWITH: return "appended-with"; 312 case CITES: return "cites"; 313 case CITEDBY: return "cited-by"; 314 case COMMENTSON: return "comments-on"; 315 case COMMENTIN: return "comment-in"; 316 case CONTAINS: return "contains"; 317 case CONTAINEDIN: return "contained-in"; 318 case CORRECTS: return "corrects"; 319 case CORRECTIONIN: return "correction-in"; 320 case REPLACES: return "replaces"; 321 case REPLACEDWITH: return "replaced-with"; 322 case RETRACTS: return "retracts"; 323 case RETRACTEDBY: return "retracted-by"; 324 case SIGNS: return "signs"; 325 case SIMILARTO: return "similar-to"; 326 case SUPPORTS: return "supports"; 327 case SUPPORTEDWITH: return "supported-with"; 328 case TRANSFORMS: return "transforms"; 329 case TRANSFORMEDINTO: return "transformed-into"; 330 case TRANSFORMEDWITH: return "transformed-with"; 331 case DOCUMENTS: return "documents"; 332 case SPECIFICATIONOF: return "specification-of"; 333 case CREATEDWITH: return "created-with"; 334 case CITEAS: return "cite-as"; 335 case REPRINT: return "reprint"; 336 case REPRINTOF: return "reprint-of"; 337 case NULL: return null; 338 default: return "?"; 339 } 340 } 341 public String getSystem() { 342 switch (this) { 343 case DOCUMENTATION: return "http://hl7.org/fhir/related-artifact-type"; 344 case JUSTIFICATION: return "http://hl7.org/fhir/related-artifact-type"; 345 case CITATION: return "http://hl7.org/fhir/related-artifact-type"; 346 case PREDECESSOR: return "http://hl7.org/fhir/related-artifact-type"; 347 case SUCCESSOR: return "http://hl7.org/fhir/related-artifact-type"; 348 case DERIVEDFROM: return "http://hl7.org/fhir/related-artifact-type"; 349 case DEPENDSON: return "http://hl7.org/fhir/related-artifact-type"; 350 case COMPOSEDOF: return "http://hl7.org/fhir/related-artifact-type"; 351 case PARTOF: return "http://hl7.org/fhir/related-artifact-type"; 352 case AMENDS: return "http://hl7.org/fhir/related-artifact-type"; 353 case AMENDEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 354 case APPENDS: return "http://hl7.org/fhir/related-artifact-type"; 355 case APPENDEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 356 case CITES: return "http://hl7.org/fhir/related-artifact-type"; 357 case CITEDBY: return "http://hl7.org/fhir/related-artifact-type"; 358 case COMMENTSON: return "http://hl7.org/fhir/related-artifact-type"; 359 case COMMENTIN: return "http://hl7.org/fhir/related-artifact-type"; 360 case CONTAINS: return "http://hl7.org/fhir/related-artifact-type"; 361 case CONTAINEDIN: return "http://hl7.org/fhir/related-artifact-type"; 362 case CORRECTS: return "http://hl7.org/fhir/related-artifact-type"; 363 case CORRECTIONIN: return "http://hl7.org/fhir/related-artifact-type"; 364 case REPLACES: return "http://hl7.org/fhir/related-artifact-type"; 365 case REPLACEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 366 case RETRACTS: return "http://hl7.org/fhir/related-artifact-type"; 367 case RETRACTEDBY: return "http://hl7.org/fhir/related-artifact-type"; 368 case SIGNS: return "http://hl7.org/fhir/related-artifact-type"; 369 case SIMILARTO: return "http://hl7.org/fhir/related-artifact-type"; 370 case SUPPORTS: return "http://hl7.org/fhir/related-artifact-type"; 371 case SUPPORTEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 372 case TRANSFORMS: return "http://hl7.org/fhir/related-artifact-type"; 373 case TRANSFORMEDINTO: return "http://hl7.org/fhir/related-artifact-type"; 374 case TRANSFORMEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 375 case DOCUMENTS: return "http://hl7.org/fhir/related-artifact-type"; 376 case SPECIFICATIONOF: return "http://hl7.org/fhir/related-artifact-type"; 377 case CREATEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 378 case CITEAS: return "http://hl7.org/fhir/related-artifact-type"; 379 case REPRINT: return "http://hl7.org/fhir/related-artifact-type-expanded"; 380 case REPRINTOF: return "http://hl7.org/fhir/related-artifact-type-expanded"; 381 case NULL: return null; 382 default: return "?"; 383 } 384 } 385 public String getDefinition() { 386 switch (this) { 387 case DOCUMENTATION: return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness."; 388 case JUSTIFICATION: return "The target artifact is a summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource."; 389 case CITATION: return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 390 case PREDECESSOR: return "The previous version of the knowledge artifact, used to establish an ordering of versions of an artifact, independent of the status of each version."; 391 case SUCCESSOR: return "The subsequent version of the knowledge artfact, used to establish an ordering of versions of an artifact, independent of the status of each version."; 392 case DERIVEDFROM: return "This artifact is derived from the target artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting. The artifact may be derived from one or more target artifacts."; 393 case DEPENDSON: return "This artifact depends on the target artifact. There is a requirement to use the target artifact in the creation or interpretation of this artifact."; 394 case COMPOSEDOF: return "This artifact is composed of the target artifact. This artifact is constructed with the target artifact as a component. The target artifact is a part of this artifact. (A dataset is composed of data.)."; 395 case PARTOF: return "This artifact is a part of the target artifact. The target artifact is composed of this artifact (and possibly other artifacts)."; 396 case AMENDS: return "This artifact amends or changes the target artifact. This artifact adds additional information that is functionally expected to replace information in the target artifact. This artifact replaces a part but not all of the target artifact."; 397 case AMENDEDWITH: return "This artifact is amended with or changed by the target artifact. There is information in this artifact that should be functionally replaced with information in the target artifact."; 398 case APPENDS: return "This artifact adds additional information to the target artifact. The additional information does not replace or change information in the target artifact."; 399 case APPENDEDWITH: return "This artifact has additional information in the target artifact."; 400 case CITES: return "This artifact cites the target artifact. This may be a bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 401 case CITEDBY: return "This artifact is cited by the target artifact."; 402 case COMMENTSON: return "This artifact contains comments about the target artifact."; 403 case COMMENTIN: return "This artifact has comments about it in the target artifact. The type of comments may be expressed in the targetClassifier element such as reply, review, editorial, feedback, solicited, unsolicited, structured, unstructured."; 404 case CONTAINS: return "This artifact is a container in which the target artifact is contained. A container is a data structure whose instances are collections of other objects. (A database contains the dataset.)."; 405 case CONTAINEDIN: return "This artifact is contained in the target artifact. The target artifact is a data structure whose instances are collections of other objects."; 406 case CORRECTS: return "This artifact identifies errors and replacement content for the target artifact."; 407 case CORRECTIONIN: return "This artifact has corrections to it in the target artifact. The target artifact identifies errors and replacement content for this artifact."; 408 case REPLACES: return "This artifact replaces or supersedes the target artifact. The target artifact may be considered deprecated."; 409 case REPLACEDWITH: return "This artifact is replaced with or superseded by the target artifact. This artifact may be considered deprecated."; 410 case RETRACTS: return "This artifact retracts the target artifact. The content that was published in the target artifact should be considered removed from publication and should no longer be considered part of the public record."; 411 case RETRACTEDBY: return "This artifact is retracted by the target artifact. The content that was published in this artifact should be considered removed from publication and should no longer be considered part of the public record."; 412 case SIGNS: return "This artifact is a signature of the target artifact."; 413 case SIMILARTO: return "This artifact has characteristics in common with the target artifact. This relationship may be used in systems to ?deduplicate? knowledge artifacts from different sources, or in systems to show ?similar items?."; 414 case SUPPORTS: return "This artifact provides additional support for the target artifact. The type of support is not documentation as it does not describe, explain, or instruct regarding the target artifact."; 415 case SUPPORTEDWITH: return "The target artifact contains additional information related to the knowledge artifact but is not documentation as the additional information does not describe, explain, or instruct regarding the knowledge artifact content or application. This could include an associated dataset."; 416 case TRANSFORMS: return "This artifact was generated by transforming the target artifact (e.g., format or language conversion). This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but changes are only apparent in form and there is only one target artifact with the ?transforms? relationship type."; 417 case TRANSFORMEDINTO: return "This artifact was transformed into the target artifact (e.g., by format or language conversion)."; 418 case TRANSFORMEDWITH: return "This artifact was generated by transforming a related artifact (e.g., format or language conversion), noted separately with the ?transforms? relationship type. This transformation used the target artifact to inform the transformation. The target artifact may be a conversion script or translation guide."; 419 case DOCUMENTS: return "This artifact provides additional documentation for the target artifact. This could include additional instructions on usage as well as additional information on clinical context or appropriateness."; 420 case SPECIFICATIONOF: return "The target artifact is a precise description of a concept in this artifact. This may be used when the RelatedArtifact datatype is used in elements contained in this artifact."; 421 case CREATEDWITH: return "This artifact was created with the target artifact. The target artifact is a tool or support material used in the creation of the artifact, and not content that the artifact was derived from."; 422 case CITEAS: return "The related artifact is the citation for this artifact."; 423 case REPRINT: return "A copy of the artifact in a publication with a different artifact identifier."; 424 case REPRINTOF: return "The original version of record for which the current artifact is a copy."; 425 case NULL: return null; 426 default: return "?"; 427 } 428 } 429 public String getDisplay() { 430 switch (this) { 431 case DOCUMENTATION: return "Documentation"; 432 case JUSTIFICATION: return "Justification"; 433 case CITATION: return "Citation"; 434 case PREDECESSOR: return "Predecessor"; 435 case SUCCESSOR: return "Successor"; 436 case DERIVEDFROM: return "Derived From"; 437 case DEPENDSON: return "Depends On"; 438 case COMPOSEDOF: return "Composed Of"; 439 case PARTOF: return "Part Of"; 440 case AMENDS: return "Amends"; 441 case AMENDEDWITH: return "Amended With"; 442 case APPENDS: return "Appends"; 443 case APPENDEDWITH: return "Appended With"; 444 case CITES: return "Cites"; 445 case CITEDBY: return "Cited By"; 446 case COMMENTSON: return "Is Comment On"; 447 case COMMENTIN: return "Has Comment In"; 448 case CONTAINS: return "Contains"; 449 case CONTAINEDIN: return "Contained In"; 450 case CORRECTS: return "Corrects"; 451 case CORRECTIONIN: return "Correction In"; 452 case REPLACES: return "Replaces"; 453 case REPLACEDWITH: return "Replaced With"; 454 case RETRACTS: return "Retracts"; 455 case RETRACTEDBY: return "Retracted By"; 456 case SIGNS: return "Signs"; 457 case SIMILARTO: return "Similar To"; 458 case SUPPORTS: return "Supports"; 459 case SUPPORTEDWITH: return "Supported With"; 460 case TRANSFORMS: return "Transforms"; 461 case TRANSFORMEDINTO: return "Transformed Into"; 462 case TRANSFORMEDWITH: return "Transformed With"; 463 case DOCUMENTS: return "Documents"; 464 case SPECIFICATIONOF: return "Specification Of"; 465 case CREATEDWITH: return "Created With"; 466 case CITEAS: return "Cite As"; 467 case REPRINT: return "Reprint"; 468 case REPRINTOF: return "Reprint Of"; 469 case NULL: return null; 470 default: return "?"; 471 } 472 } 473 } 474 475 public static class RelatedArtifactTypeExpandedEnumFactory implements EnumFactory<RelatedArtifactTypeExpanded> { 476 public RelatedArtifactTypeExpanded fromCode(String codeString) throws IllegalArgumentException { 477 if (codeString == null || "".equals(codeString)) 478 if (codeString == null || "".equals(codeString)) 479 return null; 480 if ("documentation".equals(codeString)) 481 return RelatedArtifactTypeExpanded.DOCUMENTATION; 482 if ("justification".equals(codeString)) 483 return RelatedArtifactTypeExpanded.JUSTIFICATION; 484 if ("citation".equals(codeString)) 485 return RelatedArtifactTypeExpanded.CITATION; 486 if ("predecessor".equals(codeString)) 487 return RelatedArtifactTypeExpanded.PREDECESSOR; 488 if ("successor".equals(codeString)) 489 return RelatedArtifactTypeExpanded.SUCCESSOR; 490 if ("derived-from".equals(codeString)) 491 return RelatedArtifactTypeExpanded.DERIVEDFROM; 492 if ("depends-on".equals(codeString)) 493 return RelatedArtifactTypeExpanded.DEPENDSON; 494 if ("composed-of".equals(codeString)) 495 return RelatedArtifactTypeExpanded.COMPOSEDOF; 496 if ("part-of".equals(codeString)) 497 return RelatedArtifactTypeExpanded.PARTOF; 498 if ("amends".equals(codeString)) 499 return RelatedArtifactTypeExpanded.AMENDS; 500 if ("amended-with".equals(codeString)) 501 return RelatedArtifactTypeExpanded.AMENDEDWITH; 502 if ("appends".equals(codeString)) 503 return RelatedArtifactTypeExpanded.APPENDS; 504 if ("appended-with".equals(codeString)) 505 return RelatedArtifactTypeExpanded.APPENDEDWITH; 506 if ("cites".equals(codeString)) 507 return RelatedArtifactTypeExpanded.CITES; 508 if ("cited-by".equals(codeString)) 509 return RelatedArtifactTypeExpanded.CITEDBY; 510 if ("comments-on".equals(codeString)) 511 return RelatedArtifactTypeExpanded.COMMENTSON; 512 if ("comment-in".equals(codeString)) 513 return RelatedArtifactTypeExpanded.COMMENTIN; 514 if ("contains".equals(codeString)) 515 return RelatedArtifactTypeExpanded.CONTAINS; 516 if ("contained-in".equals(codeString)) 517 return RelatedArtifactTypeExpanded.CONTAINEDIN; 518 if ("corrects".equals(codeString)) 519 return RelatedArtifactTypeExpanded.CORRECTS; 520 if ("correction-in".equals(codeString)) 521 return RelatedArtifactTypeExpanded.CORRECTIONIN; 522 if ("replaces".equals(codeString)) 523 return RelatedArtifactTypeExpanded.REPLACES; 524 if ("replaced-with".equals(codeString)) 525 return RelatedArtifactTypeExpanded.REPLACEDWITH; 526 if ("retracts".equals(codeString)) 527 return RelatedArtifactTypeExpanded.RETRACTS; 528 if ("retracted-by".equals(codeString)) 529 return RelatedArtifactTypeExpanded.RETRACTEDBY; 530 if ("signs".equals(codeString)) 531 return RelatedArtifactTypeExpanded.SIGNS; 532 if ("similar-to".equals(codeString)) 533 return RelatedArtifactTypeExpanded.SIMILARTO; 534 if ("supports".equals(codeString)) 535 return RelatedArtifactTypeExpanded.SUPPORTS; 536 if ("supported-with".equals(codeString)) 537 return RelatedArtifactTypeExpanded.SUPPORTEDWITH; 538 if ("transforms".equals(codeString)) 539 return RelatedArtifactTypeExpanded.TRANSFORMS; 540 if ("transformed-into".equals(codeString)) 541 return RelatedArtifactTypeExpanded.TRANSFORMEDINTO; 542 if ("transformed-with".equals(codeString)) 543 return RelatedArtifactTypeExpanded.TRANSFORMEDWITH; 544 if ("documents".equals(codeString)) 545 return RelatedArtifactTypeExpanded.DOCUMENTS; 546 if ("specification-of".equals(codeString)) 547 return RelatedArtifactTypeExpanded.SPECIFICATIONOF; 548 if ("created-with".equals(codeString)) 549 return RelatedArtifactTypeExpanded.CREATEDWITH; 550 if ("cite-as".equals(codeString)) 551 return RelatedArtifactTypeExpanded.CITEAS; 552 if ("reprint".equals(codeString)) 553 return RelatedArtifactTypeExpanded.REPRINT; 554 if ("reprint-of".equals(codeString)) 555 return RelatedArtifactTypeExpanded.REPRINTOF; 556 throw new IllegalArgumentException("Unknown RelatedArtifactTypeExpanded code '"+codeString+"'"); 557 } 558 public Enumeration<RelatedArtifactTypeExpanded> fromType(PrimitiveType<?> code) throws FHIRException { 559 if (code == null) 560 return null; 561 if (code.isEmpty()) 562 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.NULL, code); 563 String codeString = ((PrimitiveType) code).asStringValue(); 564 if (codeString == null || "".equals(codeString)) 565 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.NULL, code); 566 if ("documentation".equals(codeString)) 567 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.DOCUMENTATION, code); 568 if ("justification".equals(codeString)) 569 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.JUSTIFICATION, code); 570 if ("citation".equals(codeString)) 571 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CITATION, code); 572 if ("predecessor".equals(codeString)) 573 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.PREDECESSOR, code); 574 if ("successor".equals(codeString)) 575 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.SUCCESSOR, code); 576 if ("derived-from".equals(codeString)) 577 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.DERIVEDFROM, code); 578 if ("depends-on".equals(codeString)) 579 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.DEPENDSON, code); 580 if ("composed-of".equals(codeString)) 581 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.COMPOSEDOF, code); 582 if ("part-of".equals(codeString)) 583 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.PARTOF, code); 584 if ("amends".equals(codeString)) 585 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.AMENDS, code); 586 if ("amended-with".equals(codeString)) 587 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.AMENDEDWITH, code); 588 if ("appends".equals(codeString)) 589 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.APPENDS, code); 590 if ("appended-with".equals(codeString)) 591 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.APPENDEDWITH, code); 592 if ("cites".equals(codeString)) 593 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CITES, code); 594 if ("cited-by".equals(codeString)) 595 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CITEDBY, code); 596 if ("comments-on".equals(codeString)) 597 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.COMMENTSON, code); 598 if ("comment-in".equals(codeString)) 599 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.COMMENTIN, code); 600 if ("contains".equals(codeString)) 601 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CONTAINS, code); 602 if ("contained-in".equals(codeString)) 603 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CONTAINEDIN, code); 604 if ("corrects".equals(codeString)) 605 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CORRECTS, code); 606 if ("correction-in".equals(codeString)) 607 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CORRECTIONIN, code); 608 if ("replaces".equals(codeString)) 609 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.REPLACES, code); 610 if ("replaced-with".equals(codeString)) 611 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.REPLACEDWITH, code); 612 if ("retracts".equals(codeString)) 613 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.RETRACTS, code); 614 if ("retracted-by".equals(codeString)) 615 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.RETRACTEDBY, code); 616 if ("signs".equals(codeString)) 617 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.SIGNS, code); 618 if ("similar-to".equals(codeString)) 619 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.SIMILARTO, code); 620 if ("supports".equals(codeString)) 621 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.SUPPORTS, code); 622 if ("supported-with".equals(codeString)) 623 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.SUPPORTEDWITH, code); 624 if ("transforms".equals(codeString)) 625 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.TRANSFORMS, code); 626 if ("transformed-into".equals(codeString)) 627 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.TRANSFORMEDINTO, code); 628 if ("transformed-with".equals(codeString)) 629 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.TRANSFORMEDWITH, code); 630 if ("documents".equals(codeString)) 631 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.DOCUMENTS, code); 632 if ("specification-of".equals(codeString)) 633 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.SPECIFICATIONOF, code); 634 if ("created-with".equals(codeString)) 635 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CREATEDWITH, code); 636 if ("cite-as".equals(codeString)) 637 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.CITEAS, code); 638 if ("reprint".equals(codeString)) 639 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.REPRINT, code); 640 if ("reprint-of".equals(codeString)) 641 return new Enumeration<RelatedArtifactTypeExpanded>(this, RelatedArtifactTypeExpanded.REPRINTOF, code); 642 throw new FHIRException("Unknown RelatedArtifactTypeExpanded code '"+codeString+"'"); 643 } 644 public String toCode(RelatedArtifactTypeExpanded code) { 645 if (code == RelatedArtifactTypeExpanded.NULL) 646 return null; 647 if (code == RelatedArtifactTypeExpanded.DOCUMENTATION) 648 return "documentation"; 649 if (code == RelatedArtifactTypeExpanded.JUSTIFICATION) 650 return "justification"; 651 if (code == RelatedArtifactTypeExpanded.CITATION) 652 return "citation"; 653 if (code == RelatedArtifactTypeExpanded.PREDECESSOR) 654 return "predecessor"; 655 if (code == RelatedArtifactTypeExpanded.SUCCESSOR) 656 return "successor"; 657 if (code == RelatedArtifactTypeExpanded.DERIVEDFROM) 658 return "derived-from"; 659 if (code == RelatedArtifactTypeExpanded.DEPENDSON) 660 return "depends-on"; 661 if (code == RelatedArtifactTypeExpanded.COMPOSEDOF) 662 return "composed-of"; 663 if (code == RelatedArtifactTypeExpanded.PARTOF) 664 return "part-of"; 665 if (code == RelatedArtifactTypeExpanded.AMENDS) 666 return "amends"; 667 if (code == RelatedArtifactTypeExpanded.AMENDEDWITH) 668 return "amended-with"; 669 if (code == RelatedArtifactTypeExpanded.APPENDS) 670 return "appends"; 671 if (code == RelatedArtifactTypeExpanded.APPENDEDWITH) 672 return "appended-with"; 673 if (code == RelatedArtifactTypeExpanded.CITES) 674 return "cites"; 675 if (code == RelatedArtifactTypeExpanded.CITEDBY) 676 return "cited-by"; 677 if (code == RelatedArtifactTypeExpanded.COMMENTSON) 678 return "comments-on"; 679 if (code == RelatedArtifactTypeExpanded.COMMENTIN) 680 return "comment-in"; 681 if (code == RelatedArtifactTypeExpanded.CONTAINS) 682 return "contains"; 683 if (code == RelatedArtifactTypeExpanded.CONTAINEDIN) 684 return "contained-in"; 685 if (code == RelatedArtifactTypeExpanded.CORRECTS) 686 return "corrects"; 687 if (code == RelatedArtifactTypeExpanded.CORRECTIONIN) 688 return "correction-in"; 689 if (code == RelatedArtifactTypeExpanded.REPLACES) 690 return "replaces"; 691 if (code == RelatedArtifactTypeExpanded.REPLACEDWITH) 692 return "replaced-with"; 693 if (code == RelatedArtifactTypeExpanded.RETRACTS) 694 return "retracts"; 695 if (code == RelatedArtifactTypeExpanded.RETRACTEDBY) 696 return "retracted-by"; 697 if (code == RelatedArtifactTypeExpanded.SIGNS) 698 return "signs"; 699 if (code == RelatedArtifactTypeExpanded.SIMILARTO) 700 return "similar-to"; 701 if (code == RelatedArtifactTypeExpanded.SUPPORTS) 702 return "supports"; 703 if (code == RelatedArtifactTypeExpanded.SUPPORTEDWITH) 704 return "supported-with"; 705 if (code == RelatedArtifactTypeExpanded.TRANSFORMS) 706 return "transforms"; 707 if (code == RelatedArtifactTypeExpanded.TRANSFORMEDINTO) 708 return "transformed-into"; 709 if (code == RelatedArtifactTypeExpanded.TRANSFORMEDWITH) 710 return "transformed-with"; 711 if (code == RelatedArtifactTypeExpanded.DOCUMENTS) 712 return "documents"; 713 if (code == RelatedArtifactTypeExpanded.SPECIFICATIONOF) 714 return "specification-of"; 715 if (code == RelatedArtifactTypeExpanded.CREATEDWITH) 716 return "created-with"; 717 if (code == RelatedArtifactTypeExpanded.CITEAS) 718 return "cite-as"; 719 if (code == RelatedArtifactTypeExpanded.REPRINT) 720 return "reprint"; 721 if (code == RelatedArtifactTypeExpanded.REPRINTOF) 722 return "reprint-of"; 723 return "?"; 724 } 725 public String toSystem(RelatedArtifactTypeExpanded code) { 726 return code.getSystem(); 727 } 728 } 729 730 @Block() 731 public static class CitationSummaryComponent extends BackboneElement implements IBaseBackboneElement { 732 /** 733 * Format for display of the citation summary. 734 */ 735 @Child(name = "style", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 736 @Description(shortDefinition="Format for display of the citation summary", formalDefinition="Format for display of the citation summary." ) 737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-summary-style") 738 protected CodeableConcept style; 739 740 /** 741 * The human-readable display of the citation summary. 742 */ 743 @Child(name = "text", type = {MarkdownType.class}, order=2, min=1, max=1, modifier=false, summary=true) 744 @Description(shortDefinition="The human-readable display of the citation summary", formalDefinition="The human-readable display of the citation summary." ) 745 protected MarkdownType text; 746 747 private static final long serialVersionUID = 123416446L; 748 749 /** 750 * Constructor 751 */ 752 public CitationSummaryComponent() { 753 super(); 754 } 755 756 /** 757 * Constructor 758 */ 759 public CitationSummaryComponent(String text) { 760 super(); 761 this.setText(text); 762 } 763 764 /** 765 * @return {@link #style} (Format for display of the citation summary.) 766 */ 767 public CodeableConcept getStyle() { 768 if (this.style == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create CitationSummaryComponent.style"); 771 else if (Configuration.doAutoCreate()) 772 this.style = new CodeableConcept(); // cc 773 return this.style; 774 } 775 776 public boolean hasStyle() { 777 return this.style != null && !this.style.isEmpty(); 778 } 779 780 /** 781 * @param value {@link #style} (Format for display of the citation summary.) 782 */ 783 public CitationSummaryComponent setStyle(CodeableConcept value) { 784 this.style = value; 785 return this; 786 } 787 788 /** 789 * @return {@link #text} (The human-readable display of the citation summary.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 790 */ 791 public MarkdownType getTextElement() { 792 if (this.text == null) 793 if (Configuration.errorOnAutoCreate()) 794 throw new Error("Attempt to auto-create CitationSummaryComponent.text"); 795 else if (Configuration.doAutoCreate()) 796 this.text = new MarkdownType(); // bb 797 return this.text; 798 } 799 800 public boolean hasTextElement() { 801 return this.text != null && !this.text.isEmpty(); 802 } 803 804 public boolean hasText() { 805 return this.text != null && !this.text.isEmpty(); 806 } 807 808 /** 809 * @param value {@link #text} (The human-readable display of the citation summary.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 810 */ 811 public CitationSummaryComponent setTextElement(MarkdownType value) { 812 this.text = value; 813 return this; 814 } 815 816 /** 817 * @return The human-readable display of the citation summary. 818 */ 819 public String getText() { 820 return this.text == null ? null : this.text.getValue(); 821 } 822 823 /** 824 * @param value The human-readable display of the citation summary. 825 */ 826 public CitationSummaryComponent setText(String value) { 827 if (this.text == null) 828 this.text = new MarkdownType(); 829 this.text.setValue(value); 830 return this; 831 } 832 833 protected void listChildren(List<Property> children) { 834 super.listChildren(children); 835 children.add(new Property("style", "CodeableConcept", "Format for display of the citation summary.", 0, 1, style)); 836 children.add(new Property("text", "markdown", "The human-readable display of the citation summary.", 0, 1, text)); 837 } 838 839 @Override 840 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 841 switch (_hash) { 842 case 109780401: /*style*/ return new Property("style", "CodeableConcept", "Format for display of the citation summary.", 0, 1, style); 843 case 3556653: /*text*/ return new Property("text", "markdown", "The human-readable display of the citation summary.", 0, 1, text); 844 default: return super.getNamedProperty(_hash, _name, _checkValid); 845 } 846 847 } 848 849 @Override 850 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 851 switch (hash) { 852 case 109780401: /*style*/ return this.style == null ? new Base[0] : new Base[] {this.style}; // CodeableConcept 853 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // MarkdownType 854 default: return super.getProperty(hash, name, checkValid); 855 } 856 857 } 858 859 @Override 860 public Base setProperty(int hash, String name, Base value) throws FHIRException { 861 switch (hash) { 862 case 109780401: // style 863 this.style = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 864 return value; 865 case 3556653: // text 866 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 867 return value; 868 default: return super.setProperty(hash, name, value); 869 } 870 871 } 872 873 @Override 874 public Base setProperty(String name, Base value) throws FHIRException { 875 if (name.equals("style")) { 876 this.style = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 877 } else if (name.equals("text")) { 878 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 879 } else 880 return super.setProperty(name, value); 881 return value; 882 } 883 884 @Override 885 public void removeChild(String name, Base value) throws FHIRException { 886 if (name.equals("style")) { 887 this.style = null; 888 } else if (name.equals("text")) { 889 this.text = null; 890 } else 891 super.removeChild(name, value); 892 893 } 894 895 @Override 896 public Base makeProperty(int hash, String name) throws FHIRException { 897 switch (hash) { 898 case 109780401: return getStyle(); 899 case 3556653: return getTextElement(); 900 default: return super.makeProperty(hash, name); 901 } 902 903 } 904 905 @Override 906 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 907 switch (hash) { 908 case 109780401: /*style*/ return new String[] {"CodeableConcept"}; 909 case 3556653: /*text*/ return new String[] {"markdown"}; 910 default: return super.getTypesForProperty(hash, name); 911 } 912 913 } 914 915 @Override 916 public Base addChild(String name) throws FHIRException { 917 if (name.equals("style")) { 918 this.style = new CodeableConcept(); 919 return this.style; 920 } 921 else if (name.equals("text")) { 922 throw new FHIRException("Cannot call addChild on a singleton property Citation.summary.text"); 923 } 924 else 925 return super.addChild(name); 926 } 927 928 public CitationSummaryComponent copy() { 929 CitationSummaryComponent dst = new CitationSummaryComponent(); 930 copyValues(dst); 931 return dst; 932 } 933 934 public void copyValues(CitationSummaryComponent dst) { 935 super.copyValues(dst); 936 dst.style = style == null ? null : style.copy(); 937 dst.text = text == null ? null : text.copy(); 938 } 939 940 @Override 941 public boolean equalsDeep(Base other_) { 942 if (!super.equalsDeep(other_)) 943 return false; 944 if (!(other_ instanceof CitationSummaryComponent)) 945 return false; 946 CitationSummaryComponent o = (CitationSummaryComponent) other_; 947 return compareDeep(style, o.style, true) && compareDeep(text, o.text, true); 948 } 949 950 @Override 951 public boolean equalsShallow(Base other_) { 952 if (!super.equalsShallow(other_)) 953 return false; 954 if (!(other_ instanceof CitationSummaryComponent)) 955 return false; 956 CitationSummaryComponent o = (CitationSummaryComponent) other_; 957 return compareValues(text, o.text, true); 958 } 959 960 public boolean isEmpty() { 961 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(style, text); 962 } 963 964 public String fhirType() { 965 return "Citation.summary"; 966 967 } 968 969 } 970 971 @Block() 972 public static class CitationClassificationComponent extends BackboneElement implements IBaseBackboneElement { 973 /** 974 * The kind of classifier (e.g. publication type, keyword). 975 */ 976 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 977 @Description(shortDefinition="The kind of classifier (e.g. publication type, keyword)", formalDefinition="The kind of classifier (e.g. publication type, keyword)." ) 978 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-classification-type") 979 protected CodeableConcept type; 980 981 /** 982 * The specific classification value. 983 */ 984 @Child(name = "classifier", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 985 @Description(shortDefinition="The specific classification value", formalDefinition="The specific classification value." ) 986 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-artifact-classifier") 987 protected List<CodeableConcept> classifier; 988 989 private static final long serialVersionUID = -283121869L; 990 991 /** 992 * Constructor 993 */ 994 public CitationClassificationComponent() { 995 super(); 996 } 997 998 /** 999 * @return {@link #type} (The kind of classifier (e.g. publication type, keyword).) 1000 */ 1001 public CodeableConcept getType() { 1002 if (this.type == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create CitationClassificationComponent.type"); 1005 else if (Configuration.doAutoCreate()) 1006 this.type = new CodeableConcept(); // cc 1007 return this.type; 1008 } 1009 1010 public boolean hasType() { 1011 return this.type != null && !this.type.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #type} (The kind of classifier (e.g. publication type, keyword).) 1016 */ 1017 public CitationClassificationComponent setType(CodeableConcept value) { 1018 this.type = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #classifier} (The specific classification value.) 1024 */ 1025 public List<CodeableConcept> getClassifier() { 1026 if (this.classifier == null) 1027 this.classifier = new ArrayList<CodeableConcept>(); 1028 return this.classifier; 1029 } 1030 1031 /** 1032 * @return Returns a reference to <code>this</code> for easy method chaining 1033 */ 1034 public CitationClassificationComponent setClassifier(List<CodeableConcept> theClassifier) { 1035 this.classifier = theClassifier; 1036 return this; 1037 } 1038 1039 public boolean hasClassifier() { 1040 if (this.classifier == null) 1041 return false; 1042 for (CodeableConcept item : this.classifier) 1043 if (!item.isEmpty()) 1044 return true; 1045 return false; 1046 } 1047 1048 public CodeableConcept addClassifier() { //3 1049 CodeableConcept t = new CodeableConcept(); 1050 if (this.classifier == null) 1051 this.classifier = new ArrayList<CodeableConcept>(); 1052 this.classifier.add(t); 1053 return t; 1054 } 1055 1056 public CitationClassificationComponent addClassifier(CodeableConcept t) { //3 1057 if (t == null) 1058 return this; 1059 if (this.classifier == null) 1060 this.classifier = new ArrayList<CodeableConcept>(); 1061 this.classifier.add(t); 1062 return this; 1063 } 1064 1065 /** 1066 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 1067 */ 1068 public CodeableConcept getClassifierFirstRep() { 1069 if (getClassifier().isEmpty()) { 1070 addClassifier(); 1071 } 1072 return getClassifier().get(0); 1073 } 1074 1075 protected void listChildren(List<Property> children) { 1076 super.listChildren(children); 1077 children.add(new Property("type", "CodeableConcept", "The kind of classifier (e.g. publication type, keyword).", 0, 1, type)); 1078 children.add(new Property("classifier", "CodeableConcept", "The specific classification value.", 0, java.lang.Integer.MAX_VALUE, classifier)); 1079 } 1080 1081 @Override 1082 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1083 switch (_hash) { 1084 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of classifier (e.g. publication type, keyword).", 0, 1, type); 1085 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "The specific classification value.", 0, java.lang.Integer.MAX_VALUE, classifier); 1086 default: return super.getNamedProperty(_hash, _name, _checkValid); 1087 } 1088 1089 } 1090 1091 @Override 1092 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1093 switch (hash) { 1094 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1095 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 1096 default: return super.getProperty(hash, name, checkValid); 1097 } 1098 1099 } 1100 1101 @Override 1102 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1103 switch (hash) { 1104 case 3575610: // type 1105 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1106 return value; 1107 case -281470431: // classifier 1108 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1109 return value; 1110 default: return super.setProperty(hash, name, value); 1111 } 1112 1113 } 1114 1115 @Override 1116 public Base setProperty(String name, Base value) throws FHIRException { 1117 if (name.equals("type")) { 1118 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1119 } else if (name.equals("classifier")) { 1120 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 1121 } else 1122 return super.setProperty(name, value); 1123 return value; 1124 } 1125 1126 @Override 1127 public void removeChild(String name, Base value) throws FHIRException { 1128 if (name.equals("type")) { 1129 this.type = null; 1130 } else if (name.equals("classifier")) { 1131 this.getClassifier().remove(value); 1132 } else 1133 super.removeChild(name, value); 1134 1135 } 1136 1137 @Override 1138 public Base makeProperty(int hash, String name) throws FHIRException { 1139 switch (hash) { 1140 case 3575610: return getType(); 1141 case -281470431: return addClassifier(); 1142 default: return super.makeProperty(hash, name); 1143 } 1144 1145 } 1146 1147 @Override 1148 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1149 switch (hash) { 1150 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1151 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 1152 default: return super.getTypesForProperty(hash, name); 1153 } 1154 1155 } 1156 1157 @Override 1158 public Base addChild(String name) throws FHIRException { 1159 if (name.equals("type")) { 1160 this.type = new CodeableConcept(); 1161 return this.type; 1162 } 1163 else if (name.equals("classifier")) { 1164 return addClassifier(); 1165 } 1166 else 1167 return super.addChild(name); 1168 } 1169 1170 public CitationClassificationComponent copy() { 1171 CitationClassificationComponent dst = new CitationClassificationComponent(); 1172 copyValues(dst); 1173 return dst; 1174 } 1175 1176 public void copyValues(CitationClassificationComponent dst) { 1177 super.copyValues(dst); 1178 dst.type = type == null ? null : type.copy(); 1179 if (classifier != null) { 1180 dst.classifier = new ArrayList<CodeableConcept>(); 1181 for (CodeableConcept i : classifier) 1182 dst.classifier.add(i.copy()); 1183 }; 1184 } 1185 1186 @Override 1187 public boolean equalsDeep(Base other_) { 1188 if (!super.equalsDeep(other_)) 1189 return false; 1190 if (!(other_ instanceof CitationClassificationComponent)) 1191 return false; 1192 CitationClassificationComponent o = (CitationClassificationComponent) other_; 1193 return compareDeep(type, o.type, true) && compareDeep(classifier, o.classifier, true); 1194 } 1195 1196 @Override 1197 public boolean equalsShallow(Base other_) { 1198 if (!super.equalsShallow(other_)) 1199 return false; 1200 if (!(other_ instanceof CitationClassificationComponent)) 1201 return false; 1202 CitationClassificationComponent o = (CitationClassificationComponent) other_; 1203 return true; 1204 } 1205 1206 public boolean isEmpty() { 1207 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, classifier); 1208 } 1209 1210 public String fhirType() { 1211 return "Citation.classification"; 1212 1213 } 1214 1215 } 1216 1217 @Block() 1218 public static class CitationStatusDateComponent extends BackboneElement implements IBaseBackboneElement { 1219 /** 1220 * The state or status of the citation record (that will be paired with the period). 1221 */ 1222 @Child(name = "activity", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1223 @Description(shortDefinition="Classification of the status", formalDefinition="The state or status of the citation record (that will be paired with the period)." ) 1224 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-status-type") 1225 protected CodeableConcept activity; 1226 1227 /** 1228 * Whether the status date is actual (has occurred) or expected (estimated or anticipated). 1229 */ 1230 @Child(name = "actual", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1231 @Description(shortDefinition="Either occurred or expected", formalDefinition="Whether the status date is actual (has occurred) or expected (estimated or anticipated)." ) 1232 protected BooleanType actual; 1233 1234 /** 1235 * When the status started and/or ended. 1236 */ 1237 @Child(name = "period", type = {Period.class}, order=3, min=1, max=1, modifier=false, summary=false) 1238 @Description(shortDefinition="When the status started and/or ended", formalDefinition="When the status started and/or ended." ) 1239 protected Period period; 1240 1241 private static final long serialVersionUID = 1123586924L; 1242 1243 /** 1244 * Constructor 1245 */ 1246 public CitationStatusDateComponent() { 1247 super(); 1248 } 1249 1250 /** 1251 * Constructor 1252 */ 1253 public CitationStatusDateComponent(CodeableConcept activity, Period period) { 1254 super(); 1255 this.setActivity(activity); 1256 this.setPeriod(period); 1257 } 1258 1259 /** 1260 * @return {@link #activity} (The state or status of the citation record (that will be paired with the period).) 1261 */ 1262 public CodeableConcept getActivity() { 1263 if (this.activity == null) 1264 if (Configuration.errorOnAutoCreate()) 1265 throw new Error("Attempt to auto-create CitationStatusDateComponent.activity"); 1266 else if (Configuration.doAutoCreate()) 1267 this.activity = new CodeableConcept(); // cc 1268 return this.activity; 1269 } 1270 1271 public boolean hasActivity() { 1272 return this.activity != null && !this.activity.isEmpty(); 1273 } 1274 1275 /** 1276 * @param value {@link #activity} (The state or status of the citation record (that will be paired with the period).) 1277 */ 1278 public CitationStatusDateComponent setActivity(CodeableConcept value) { 1279 this.activity = value; 1280 return this; 1281 } 1282 1283 /** 1284 * @return {@link #actual} (Whether the status date is actual (has occurred) or expected (estimated or anticipated).). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 1285 */ 1286 public BooleanType getActualElement() { 1287 if (this.actual == null) 1288 if (Configuration.errorOnAutoCreate()) 1289 throw new Error("Attempt to auto-create CitationStatusDateComponent.actual"); 1290 else if (Configuration.doAutoCreate()) 1291 this.actual = new BooleanType(); // bb 1292 return this.actual; 1293 } 1294 1295 public boolean hasActualElement() { 1296 return this.actual != null && !this.actual.isEmpty(); 1297 } 1298 1299 public boolean hasActual() { 1300 return this.actual != null && !this.actual.isEmpty(); 1301 } 1302 1303 /** 1304 * @param value {@link #actual} (Whether the status date is actual (has occurred) or expected (estimated or anticipated).). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 1305 */ 1306 public CitationStatusDateComponent setActualElement(BooleanType value) { 1307 this.actual = value; 1308 return this; 1309 } 1310 1311 /** 1312 * @return Whether the status date is actual (has occurred) or expected (estimated or anticipated). 1313 */ 1314 public boolean getActual() { 1315 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 1316 } 1317 1318 /** 1319 * @param value Whether the status date is actual (has occurred) or expected (estimated or anticipated). 1320 */ 1321 public CitationStatusDateComponent setActual(boolean value) { 1322 if (this.actual == null) 1323 this.actual = new BooleanType(); 1324 this.actual.setValue(value); 1325 return this; 1326 } 1327 1328 /** 1329 * @return {@link #period} (When the status started and/or ended.) 1330 */ 1331 public Period getPeriod() { 1332 if (this.period == null) 1333 if (Configuration.errorOnAutoCreate()) 1334 throw new Error("Attempt to auto-create CitationStatusDateComponent.period"); 1335 else if (Configuration.doAutoCreate()) 1336 this.period = new Period(); // cc 1337 return this.period; 1338 } 1339 1340 public boolean hasPeriod() { 1341 return this.period != null && !this.period.isEmpty(); 1342 } 1343 1344 /** 1345 * @param value {@link #period} (When the status started and/or ended.) 1346 */ 1347 public CitationStatusDateComponent setPeriod(Period value) { 1348 this.period = value; 1349 return this; 1350 } 1351 1352 protected void listChildren(List<Property> children) { 1353 super.listChildren(children); 1354 children.add(new Property("activity", "CodeableConcept", "The state or status of the citation record (that will be paired with the period).", 0, 1, activity)); 1355 children.add(new Property("actual", "boolean", "Whether the status date is actual (has occurred) or expected (estimated or anticipated).", 0, 1, actual)); 1356 children.add(new Property("period", "Period", "When the status started and/or ended.", 0, 1, period)); 1357 } 1358 1359 @Override 1360 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1361 switch (_hash) { 1362 case -1655966961: /*activity*/ return new Property("activity", "CodeableConcept", "The state or status of the citation record (that will be paired with the period).", 0, 1, activity); 1363 case -1422939762: /*actual*/ return new Property("actual", "boolean", "Whether the status date is actual (has occurred) or expected (estimated or anticipated).", 0, 1, actual); 1364 case -991726143: /*period*/ return new Property("period", "Period", "When the status started and/or ended.", 0, 1, period); 1365 default: return super.getNamedProperty(_hash, _name, _checkValid); 1366 } 1367 1368 } 1369 1370 @Override 1371 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1372 switch (hash) { 1373 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : new Base[] {this.activity}; // CodeableConcept 1374 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 1375 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1376 default: return super.getProperty(hash, name, checkValid); 1377 } 1378 1379 } 1380 1381 @Override 1382 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1383 switch (hash) { 1384 case -1655966961: // activity 1385 this.activity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1386 return value; 1387 case -1422939762: // actual 1388 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 1389 return value; 1390 case -991726143: // period 1391 this.period = TypeConvertor.castToPeriod(value); // Period 1392 return value; 1393 default: return super.setProperty(hash, name, value); 1394 } 1395 1396 } 1397 1398 @Override 1399 public Base setProperty(String name, Base value) throws FHIRException { 1400 if (name.equals("activity")) { 1401 this.activity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1402 } else if (name.equals("actual")) { 1403 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 1404 } else if (name.equals("period")) { 1405 this.period = TypeConvertor.castToPeriod(value); // Period 1406 } else 1407 return super.setProperty(name, value); 1408 return value; 1409 } 1410 1411 @Override 1412 public void removeChild(String name, Base value) throws FHIRException { 1413 if (name.equals("activity")) { 1414 this.activity = null; 1415 } else if (name.equals("actual")) { 1416 this.actual = null; 1417 } else if (name.equals("period")) { 1418 this.period = null; 1419 } else 1420 super.removeChild(name, value); 1421 1422 } 1423 1424 @Override 1425 public Base makeProperty(int hash, String name) throws FHIRException { 1426 switch (hash) { 1427 case -1655966961: return getActivity(); 1428 case -1422939762: return getActualElement(); 1429 case -991726143: return getPeriod(); 1430 default: return super.makeProperty(hash, name); 1431 } 1432 1433 } 1434 1435 @Override 1436 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1437 switch (hash) { 1438 case -1655966961: /*activity*/ return new String[] {"CodeableConcept"}; 1439 case -1422939762: /*actual*/ return new String[] {"boolean"}; 1440 case -991726143: /*period*/ return new String[] {"Period"}; 1441 default: return super.getTypesForProperty(hash, name); 1442 } 1443 1444 } 1445 1446 @Override 1447 public Base addChild(String name) throws FHIRException { 1448 if (name.equals("activity")) { 1449 this.activity = new CodeableConcept(); 1450 return this.activity; 1451 } 1452 else if (name.equals("actual")) { 1453 throw new FHIRException("Cannot call addChild on a singleton property Citation.statusDate.actual"); 1454 } 1455 else if (name.equals("period")) { 1456 this.period = new Period(); 1457 return this.period; 1458 } 1459 else 1460 return super.addChild(name); 1461 } 1462 1463 public CitationStatusDateComponent copy() { 1464 CitationStatusDateComponent dst = new CitationStatusDateComponent(); 1465 copyValues(dst); 1466 return dst; 1467 } 1468 1469 public void copyValues(CitationStatusDateComponent dst) { 1470 super.copyValues(dst); 1471 dst.activity = activity == null ? null : activity.copy(); 1472 dst.actual = actual == null ? null : actual.copy(); 1473 dst.period = period == null ? null : period.copy(); 1474 } 1475 1476 @Override 1477 public boolean equalsDeep(Base other_) { 1478 if (!super.equalsDeep(other_)) 1479 return false; 1480 if (!(other_ instanceof CitationStatusDateComponent)) 1481 return false; 1482 CitationStatusDateComponent o = (CitationStatusDateComponent) other_; 1483 return compareDeep(activity, o.activity, true) && compareDeep(actual, o.actual, true) && compareDeep(period, o.period, true) 1484 ; 1485 } 1486 1487 @Override 1488 public boolean equalsShallow(Base other_) { 1489 if (!super.equalsShallow(other_)) 1490 return false; 1491 if (!(other_ instanceof CitationStatusDateComponent)) 1492 return false; 1493 CitationStatusDateComponent o = (CitationStatusDateComponent) other_; 1494 return compareValues(actual, o.actual, true); 1495 } 1496 1497 public boolean isEmpty() { 1498 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(activity, actual, period 1499 ); 1500 } 1501 1502 public String fhirType() { 1503 return "Citation.statusDate"; 1504 1505 } 1506 1507 } 1508 1509 @Block() 1510 public static class CitationCitedArtifactComponent extends BackboneElement implements IBaseBackboneElement { 1511 /** 1512 * A formal identifier that is used to identify the cited artifact when it is represented in other formats, or referenced in a specification, model, design or an instance. 1513 */ 1514 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1515 @Description(shortDefinition="Unique identifier. May include DOI, PMID, PMCID, etc", formalDefinition="A formal identifier that is used to identify the cited artifact when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1516 protected List<Identifier> identifier; 1517 1518 /** 1519 * A formal identifier that is used to identify things closely related to the cited artifact. 1520 */ 1521 @Child(name = "relatedIdentifier", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1522 @Description(shortDefinition="Identifier not unique to the cited artifact. May include trial registry identifiers", formalDefinition="A formal identifier that is used to identify things closely related to the cited artifact." ) 1523 protected List<Identifier> relatedIdentifier; 1524 1525 /** 1526 * When the cited artifact was accessed. 1527 */ 1528 @Child(name = "dateAccessed", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1529 @Description(shortDefinition="When the cited artifact was accessed", formalDefinition="When the cited artifact was accessed." ) 1530 protected DateTimeType dateAccessed; 1531 1532 /** 1533 * The defined version of the cited artifact. 1534 */ 1535 @Child(name = "version", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 1536 @Description(shortDefinition="The defined version of the cited artifact", formalDefinition="The defined version of the cited artifact." ) 1537 protected CitationCitedArtifactVersionComponent version; 1538 1539 /** 1540 * The status of the cited artifact. 1541 */ 1542 @Child(name = "currentState", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1543 @Description(shortDefinition="The status of the cited artifact", formalDefinition="The status of the cited artifact." ) 1544 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/cited-artifact-status-type") 1545 protected List<CodeableConcept> currentState; 1546 1547 /** 1548 * An effective date or period, historical or future, actual or expected, for a status of the cited artifact. 1549 */ 1550 @Child(name = "statusDate", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1551 @Description(shortDefinition="An effective date or period for a status of the cited artifact", formalDefinition="An effective date or period, historical or future, actual or expected, for a status of the cited artifact." ) 1552 protected List<CitationCitedArtifactStatusDateComponent> statusDate; 1553 1554 /** 1555 * The title details of the article or artifact. 1556 */ 1557 @Child(name = "title", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1558 @Description(shortDefinition="The title details of the article or artifact", formalDefinition="The title details of the article or artifact." ) 1559 protected List<CitationCitedArtifactTitleComponent> title; 1560 1561 /** 1562 * The abstract may be used to convey article-contained abstracts, externally-created abstracts, or other descriptive summaries. 1563 */ 1564 @Child(name = "abstract", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1565 @Description(shortDefinition="Summary of the article or artifact", formalDefinition="The abstract may be used to convey article-contained abstracts, externally-created abstracts, or other descriptive summaries." ) 1566 protected List<CitationCitedArtifactAbstractComponent> abstract_; 1567 1568 /** 1569 * The component of the article or artifact. 1570 */ 1571 @Child(name = "part", type = {}, order=9, min=0, max=1, modifier=false, summary=false) 1572 @Description(shortDefinition="The component of the article or artifact", formalDefinition="The component of the article or artifact." ) 1573 protected CitationCitedArtifactPartComponent part; 1574 1575 /** 1576 * The artifact related to the cited artifact. 1577 */ 1578 @Child(name = "relatesTo", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1579 @Description(shortDefinition="The artifact related to the cited artifact", formalDefinition="The artifact related to the cited artifact." ) 1580 protected List<CitationCitedArtifactRelatesToComponent> relatesTo; 1581 1582 /** 1583 * If multiple, used to represent alternative forms of the article that are not separate citations. 1584 */ 1585 @Child(name = "publicationForm", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1586 @Description(shortDefinition="If multiple, used to represent alternative forms of the article that are not separate citations", formalDefinition="If multiple, used to represent alternative forms of the article that are not separate citations." ) 1587 protected List<CitationCitedArtifactPublicationFormComponent> publicationForm; 1588 1589 /** 1590 * Used for any URL for the article or artifact cited. 1591 */ 1592 @Child(name = "webLocation", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1593 @Description(shortDefinition="Used for any URL for the article or artifact cited", formalDefinition="Used for any URL for the article or artifact cited." ) 1594 protected List<CitationCitedArtifactWebLocationComponent> webLocation; 1595 1596 /** 1597 * The assignment to an organizing scheme. 1598 */ 1599 @Child(name = "classification", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1600 @Description(shortDefinition="The assignment to an organizing scheme", formalDefinition="The assignment to an organizing scheme." ) 1601 protected List<CitationCitedArtifactClassificationComponent> classification; 1602 1603 /** 1604 * This element is used to list authors and other contributors, their contact information, specific contributions, and summary statements. 1605 */ 1606 @Child(name = "contributorship", type = {}, order=14, min=0, max=1, modifier=false, summary=false) 1607 @Description(shortDefinition="Attribution of authors and other contributors", formalDefinition="This element is used to list authors and other contributors, their contact information, specific contributions, and summary statements." ) 1608 protected CitationCitedArtifactContributorshipComponent contributorship; 1609 1610 /** 1611 * Any additional information or content for the article or artifact. 1612 */ 1613 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1614 @Description(shortDefinition="Any additional information or content for the article or artifact", formalDefinition="Any additional information or content for the article or artifact." ) 1615 protected List<Annotation> note; 1616 1617 private static final long serialVersionUID = -1685890486L; 1618 1619 /** 1620 * Constructor 1621 */ 1622 public CitationCitedArtifactComponent() { 1623 super(); 1624 } 1625 1626 /** 1627 * @return {@link #identifier} (A formal identifier that is used to identify the cited artifact when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1628 */ 1629 public List<Identifier> getIdentifier() { 1630 if (this.identifier == null) 1631 this.identifier = new ArrayList<Identifier>(); 1632 return this.identifier; 1633 } 1634 1635 /** 1636 * @return Returns a reference to <code>this</code> for easy method chaining 1637 */ 1638 public CitationCitedArtifactComponent setIdentifier(List<Identifier> theIdentifier) { 1639 this.identifier = theIdentifier; 1640 return this; 1641 } 1642 1643 public boolean hasIdentifier() { 1644 if (this.identifier == null) 1645 return false; 1646 for (Identifier item : this.identifier) 1647 if (!item.isEmpty()) 1648 return true; 1649 return false; 1650 } 1651 1652 public Identifier addIdentifier() { //3 1653 Identifier t = new Identifier(); 1654 if (this.identifier == null) 1655 this.identifier = new ArrayList<Identifier>(); 1656 this.identifier.add(t); 1657 return t; 1658 } 1659 1660 public CitationCitedArtifactComponent addIdentifier(Identifier t) { //3 1661 if (t == null) 1662 return this; 1663 if (this.identifier == null) 1664 this.identifier = new ArrayList<Identifier>(); 1665 this.identifier.add(t); 1666 return this; 1667 } 1668 1669 /** 1670 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1671 */ 1672 public Identifier getIdentifierFirstRep() { 1673 if (getIdentifier().isEmpty()) { 1674 addIdentifier(); 1675 } 1676 return getIdentifier().get(0); 1677 } 1678 1679 /** 1680 * @return {@link #relatedIdentifier} (A formal identifier that is used to identify things closely related to the cited artifact.) 1681 */ 1682 public List<Identifier> getRelatedIdentifier() { 1683 if (this.relatedIdentifier == null) 1684 this.relatedIdentifier = new ArrayList<Identifier>(); 1685 return this.relatedIdentifier; 1686 } 1687 1688 /** 1689 * @return Returns a reference to <code>this</code> for easy method chaining 1690 */ 1691 public CitationCitedArtifactComponent setRelatedIdentifier(List<Identifier> theRelatedIdentifier) { 1692 this.relatedIdentifier = theRelatedIdentifier; 1693 return this; 1694 } 1695 1696 public boolean hasRelatedIdentifier() { 1697 if (this.relatedIdentifier == null) 1698 return false; 1699 for (Identifier item : this.relatedIdentifier) 1700 if (!item.isEmpty()) 1701 return true; 1702 return false; 1703 } 1704 1705 public Identifier addRelatedIdentifier() { //3 1706 Identifier t = new Identifier(); 1707 if (this.relatedIdentifier == null) 1708 this.relatedIdentifier = new ArrayList<Identifier>(); 1709 this.relatedIdentifier.add(t); 1710 return t; 1711 } 1712 1713 public CitationCitedArtifactComponent addRelatedIdentifier(Identifier t) { //3 1714 if (t == null) 1715 return this; 1716 if (this.relatedIdentifier == null) 1717 this.relatedIdentifier = new ArrayList<Identifier>(); 1718 this.relatedIdentifier.add(t); 1719 return this; 1720 } 1721 1722 /** 1723 * @return The first repetition of repeating field {@link #relatedIdentifier}, creating it if it does not already exist {3} 1724 */ 1725 public Identifier getRelatedIdentifierFirstRep() { 1726 if (getRelatedIdentifier().isEmpty()) { 1727 addRelatedIdentifier(); 1728 } 1729 return getRelatedIdentifier().get(0); 1730 } 1731 1732 /** 1733 * @return {@link #dateAccessed} (When the cited artifact was accessed.). This is the underlying object with id, value and extensions. The accessor "getDateAccessed" gives direct access to the value 1734 */ 1735 public DateTimeType getDateAccessedElement() { 1736 if (this.dateAccessed == null) 1737 if (Configuration.errorOnAutoCreate()) 1738 throw new Error("Attempt to auto-create CitationCitedArtifactComponent.dateAccessed"); 1739 else if (Configuration.doAutoCreate()) 1740 this.dateAccessed = new DateTimeType(); // bb 1741 return this.dateAccessed; 1742 } 1743 1744 public boolean hasDateAccessedElement() { 1745 return this.dateAccessed != null && !this.dateAccessed.isEmpty(); 1746 } 1747 1748 public boolean hasDateAccessed() { 1749 return this.dateAccessed != null && !this.dateAccessed.isEmpty(); 1750 } 1751 1752 /** 1753 * @param value {@link #dateAccessed} (When the cited artifact was accessed.). This is the underlying object with id, value and extensions. The accessor "getDateAccessed" gives direct access to the value 1754 */ 1755 public CitationCitedArtifactComponent setDateAccessedElement(DateTimeType value) { 1756 this.dateAccessed = value; 1757 return this; 1758 } 1759 1760 /** 1761 * @return When the cited artifact was accessed. 1762 */ 1763 public Date getDateAccessed() { 1764 return this.dateAccessed == null ? null : this.dateAccessed.getValue(); 1765 } 1766 1767 /** 1768 * @param value When the cited artifact was accessed. 1769 */ 1770 public CitationCitedArtifactComponent setDateAccessed(Date value) { 1771 if (value == null) 1772 this.dateAccessed = null; 1773 else { 1774 if (this.dateAccessed == null) 1775 this.dateAccessed = new DateTimeType(); 1776 this.dateAccessed.setValue(value); 1777 } 1778 return this; 1779 } 1780 1781 /** 1782 * @return {@link #version} (The defined version of the cited artifact.) 1783 */ 1784 public CitationCitedArtifactVersionComponent getVersion() { 1785 if (this.version == null) 1786 if (Configuration.errorOnAutoCreate()) 1787 throw new Error("Attempt to auto-create CitationCitedArtifactComponent.version"); 1788 else if (Configuration.doAutoCreate()) 1789 this.version = new CitationCitedArtifactVersionComponent(); // cc 1790 return this.version; 1791 } 1792 1793 public boolean hasVersion() { 1794 return this.version != null && !this.version.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #version} (The defined version of the cited artifact.) 1799 */ 1800 public CitationCitedArtifactComponent setVersion(CitationCitedArtifactVersionComponent value) { 1801 this.version = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #currentState} (The status of the cited artifact.) 1807 */ 1808 public List<CodeableConcept> getCurrentState() { 1809 if (this.currentState == null) 1810 this.currentState = new ArrayList<CodeableConcept>(); 1811 return this.currentState; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public CitationCitedArtifactComponent setCurrentState(List<CodeableConcept> theCurrentState) { 1818 this.currentState = theCurrentState; 1819 return this; 1820 } 1821 1822 public boolean hasCurrentState() { 1823 if (this.currentState == null) 1824 return false; 1825 for (CodeableConcept item : this.currentState) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 public CodeableConcept addCurrentState() { //3 1832 CodeableConcept t = new CodeableConcept(); 1833 if (this.currentState == null) 1834 this.currentState = new ArrayList<CodeableConcept>(); 1835 this.currentState.add(t); 1836 return t; 1837 } 1838 1839 public CitationCitedArtifactComponent addCurrentState(CodeableConcept t) { //3 1840 if (t == null) 1841 return this; 1842 if (this.currentState == null) 1843 this.currentState = new ArrayList<CodeableConcept>(); 1844 this.currentState.add(t); 1845 return this; 1846 } 1847 1848 /** 1849 * @return The first repetition of repeating field {@link #currentState}, creating it if it does not already exist {3} 1850 */ 1851 public CodeableConcept getCurrentStateFirstRep() { 1852 if (getCurrentState().isEmpty()) { 1853 addCurrentState(); 1854 } 1855 return getCurrentState().get(0); 1856 } 1857 1858 /** 1859 * @return {@link #statusDate} (An effective date or period, historical or future, actual or expected, for a status of the cited artifact.) 1860 */ 1861 public List<CitationCitedArtifactStatusDateComponent> getStatusDate() { 1862 if (this.statusDate == null) 1863 this.statusDate = new ArrayList<CitationCitedArtifactStatusDateComponent>(); 1864 return this.statusDate; 1865 } 1866 1867 /** 1868 * @return Returns a reference to <code>this</code> for easy method chaining 1869 */ 1870 public CitationCitedArtifactComponent setStatusDate(List<CitationCitedArtifactStatusDateComponent> theStatusDate) { 1871 this.statusDate = theStatusDate; 1872 return this; 1873 } 1874 1875 public boolean hasStatusDate() { 1876 if (this.statusDate == null) 1877 return false; 1878 for (CitationCitedArtifactStatusDateComponent item : this.statusDate) 1879 if (!item.isEmpty()) 1880 return true; 1881 return false; 1882 } 1883 1884 public CitationCitedArtifactStatusDateComponent addStatusDate() { //3 1885 CitationCitedArtifactStatusDateComponent t = new CitationCitedArtifactStatusDateComponent(); 1886 if (this.statusDate == null) 1887 this.statusDate = new ArrayList<CitationCitedArtifactStatusDateComponent>(); 1888 this.statusDate.add(t); 1889 return t; 1890 } 1891 1892 public CitationCitedArtifactComponent addStatusDate(CitationCitedArtifactStatusDateComponent t) { //3 1893 if (t == null) 1894 return this; 1895 if (this.statusDate == null) 1896 this.statusDate = new ArrayList<CitationCitedArtifactStatusDateComponent>(); 1897 this.statusDate.add(t); 1898 return this; 1899 } 1900 1901 /** 1902 * @return The first repetition of repeating field {@link #statusDate}, creating it if it does not already exist {3} 1903 */ 1904 public CitationCitedArtifactStatusDateComponent getStatusDateFirstRep() { 1905 if (getStatusDate().isEmpty()) { 1906 addStatusDate(); 1907 } 1908 return getStatusDate().get(0); 1909 } 1910 1911 /** 1912 * @return {@link #title} (The title details of the article or artifact.) 1913 */ 1914 public List<CitationCitedArtifactTitleComponent> getTitle() { 1915 if (this.title == null) 1916 this.title = new ArrayList<CitationCitedArtifactTitleComponent>(); 1917 return this.title; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public CitationCitedArtifactComponent setTitle(List<CitationCitedArtifactTitleComponent> theTitle) { 1924 this.title = theTitle; 1925 return this; 1926 } 1927 1928 public boolean hasTitle() { 1929 if (this.title == null) 1930 return false; 1931 for (CitationCitedArtifactTitleComponent item : this.title) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public CitationCitedArtifactTitleComponent addTitle() { //3 1938 CitationCitedArtifactTitleComponent t = new CitationCitedArtifactTitleComponent(); 1939 if (this.title == null) 1940 this.title = new ArrayList<CitationCitedArtifactTitleComponent>(); 1941 this.title.add(t); 1942 return t; 1943 } 1944 1945 public CitationCitedArtifactComponent addTitle(CitationCitedArtifactTitleComponent t) { //3 1946 if (t == null) 1947 return this; 1948 if (this.title == null) 1949 this.title = new ArrayList<CitationCitedArtifactTitleComponent>(); 1950 this.title.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field {@link #title}, creating it if it does not already exist {3} 1956 */ 1957 public CitationCitedArtifactTitleComponent getTitleFirstRep() { 1958 if (getTitle().isEmpty()) { 1959 addTitle(); 1960 } 1961 return getTitle().get(0); 1962 } 1963 1964 /** 1965 * @return {@link #abstract_} (The abstract may be used to convey article-contained abstracts, externally-created abstracts, or other descriptive summaries.) 1966 */ 1967 public List<CitationCitedArtifactAbstractComponent> getAbstract() { 1968 if (this.abstract_ == null) 1969 this.abstract_ = new ArrayList<CitationCitedArtifactAbstractComponent>(); 1970 return this.abstract_; 1971 } 1972 1973 /** 1974 * @return Returns a reference to <code>this</code> for easy method chaining 1975 */ 1976 public CitationCitedArtifactComponent setAbstract(List<CitationCitedArtifactAbstractComponent> theAbstract) { 1977 this.abstract_ = theAbstract; 1978 return this; 1979 } 1980 1981 public boolean hasAbstract() { 1982 if (this.abstract_ == null) 1983 return false; 1984 for (CitationCitedArtifactAbstractComponent item : this.abstract_) 1985 if (!item.isEmpty()) 1986 return true; 1987 return false; 1988 } 1989 1990 public CitationCitedArtifactAbstractComponent addAbstract() { //3 1991 CitationCitedArtifactAbstractComponent t = new CitationCitedArtifactAbstractComponent(); 1992 if (this.abstract_ == null) 1993 this.abstract_ = new ArrayList<CitationCitedArtifactAbstractComponent>(); 1994 this.abstract_.add(t); 1995 return t; 1996 } 1997 1998 public CitationCitedArtifactComponent addAbstract(CitationCitedArtifactAbstractComponent t) { //3 1999 if (t == null) 2000 return this; 2001 if (this.abstract_ == null) 2002 this.abstract_ = new ArrayList<CitationCitedArtifactAbstractComponent>(); 2003 this.abstract_.add(t); 2004 return this; 2005 } 2006 2007 /** 2008 * @return The first repetition of repeating field {@link #abstract_}, creating it if it does not already exist {3} 2009 */ 2010 public CitationCitedArtifactAbstractComponent getAbstractFirstRep() { 2011 if (getAbstract().isEmpty()) { 2012 addAbstract(); 2013 } 2014 return getAbstract().get(0); 2015 } 2016 2017 /** 2018 * @return {@link #part} (The component of the article or artifact.) 2019 */ 2020 public CitationCitedArtifactPartComponent getPart() { 2021 if (this.part == null) 2022 if (Configuration.errorOnAutoCreate()) 2023 throw new Error("Attempt to auto-create CitationCitedArtifactComponent.part"); 2024 else if (Configuration.doAutoCreate()) 2025 this.part = new CitationCitedArtifactPartComponent(); // cc 2026 return this.part; 2027 } 2028 2029 public boolean hasPart() { 2030 return this.part != null && !this.part.isEmpty(); 2031 } 2032 2033 /** 2034 * @param value {@link #part} (The component of the article or artifact.) 2035 */ 2036 public CitationCitedArtifactComponent setPart(CitationCitedArtifactPartComponent value) { 2037 this.part = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return {@link #relatesTo} (The artifact related to the cited artifact.) 2043 */ 2044 public List<CitationCitedArtifactRelatesToComponent> getRelatesTo() { 2045 if (this.relatesTo == null) 2046 this.relatesTo = new ArrayList<CitationCitedArtifactRelatesToComponent>(); 2047 return this.relatesTo; 2048 } 2049 2050 /** 2051 * @return Returns a reference to <code>this</code> for easy method chaining 2052 */ 2053 public CitationCitedArtifactComponent setRelatesTo(List<CitationCitedArtifactRelatesToComponent> theRelatesTo) { 2054 this.relatesTo = theRelatesTo; 2055 return this; 2056 } 2057 2058 public boolean hasRelatesTo() { 2059 if (this.relatesTo == null) 2060 return false; 2061 for (CitationCitedArtifactRelatesToComponent item : this.relatesTo) 2062 if (!item.isEmpty()) 2063 return true; 2064 return false; 2065 } 2066 2067 public CitationCitedArtifactRelatesToComponent addRelatesTo() { //3 2068 CitationCitedArtifactRelatesToComponent t = new CitationCitedArtifactRelatesToComponent(); 2069 if (this.relatesTo == null) 2070 this.relatesTo = new ArrayList<CitationCitedArtifactRelatesToComponent>(); 2071 this.relatesTo.add(t); 2072 return t; 2073 } 2074 2075 public CitationCitedArtifactComponent addRelatesTo(CitationCitedArtifactRelatesToComponent t) { //3 2076 if (t == null) 2077 return this; 2078 if (this.relatesTo == null) 2079 this.relatesTo = new ArrayList<CitationCitedArtifactRelatesToComponent>(); 2080 this.relatesTo.add(t); 2081 return this; 2082 } 2083 2084 /** 2085 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist {3} 2086 */ 2087 public CitationCitedArtifactRelatesToComponent getRelatesToFirstRep() { 2088 if (getRelatesTo().isEmpty()) { 2089 addRelatesTo(); 2090 } 2091 return getRelatesTo().get(0); 2092 } 2093 2094 /** 2095 * @return {@link #publicationForm} (If multiple, used to represent alternative forms of the article that are not separate citations.) 2096 */ 2097 public List<CitationCitedArtifactPublicationFormComponent> getPublicationForm() { 2098 if (this.publicationForm == null) 2099 this.publicationForm = new ArrayList<CitationCitedArtifactPublicationFormComponent>(); 2100 return this.publicationForm; 2101 } 2102 2103 /** 2104 * @return Returns a reference to <code>this</code> for easy method chaining 2105 */ 2106 public CitationCitedArtifactComponent setPublicationForm(List<CitationCitedArtifactPublicationFormComponent> thePublicationForm) { 2107 this.publicationForm = thePublicationForm; 2108 return this; 2109 } 2110 2111 public boolean hasPublicationForm() { 2112 if (this.publicationForm == null) 2113 return false; 2114 for (CitationCitedArtifactPublicationFormComponent item : this.publicationForm) 2115 if (!item.isEmpty()) 2116 return true; 2117 return false; 2118 } 2119 2120 public CitationCitedArtifactPublicationFormComponent addPublicationForm() { //3 2121 CitationCitedArtifactPublicationFormComponent t = new CitationCitedArtifactPublicationFormComponent(); 2122 if (this.publicationForm == null) 2123 this.publicationForm = new ArrayList<CitationCitedArtifactPublicationFormComponent>(); 2124 this.publicationForm.add(t); 2125 return t; 2126 } 2127 2128 public CitationCitedArtifactComponent addPublicationForm(CitationCitedArtifactPublicationFormComponent t) { //3 2129 if (t == null) 2130 return this; 2131 if (this.publicationForm == null) 2132 this.publicationForm = new ArrayList<CitationCitedArtifactPublicationFormComponent>(); 2133 this.publicationForm.add(t); 2134 return this; 2135 } 2136 2137 /** 2138 * @return The first repetition of repeating field {@link #publicationForm}, creating it if it does not already exist {3} 2139 */ 2140 public CitationCitedArtifactPublicationFormComponent getPublicationFormFirstRep() { 2141 if (getPublicationForm().isEmpty()) { 2142 addPublicationForm(); 2143 } 2144 return getPublicationForm().get(0); 2145 } 2146 2147 /** 2148 * @return {@link #webLocation} (Used for any URL for the article or artifact cited.) 2149 */ 2150 public List<CitationCitedArtifactWebLocationComponent> getWebLocation() { 2151 if (this.webLocation == null) 2152 this.webLocation = new ArrayList<CitationCitedArtifactWebLocationComponent>(); 2153 return this.webLocation; 2154 } 2155 2156 /** 2157 * @return Returns a reference to <code>this</code> for easy method chaining 2158 */ 2159 public CitationCitedArtifactComponent setWebLocation(List<CitationCitedArtifactWebLocationComponent> theWebLocation) { 2160 this.webLocation = theWebLocation; 2161 return this; 2162 } 2163 2164 public boolean hasWebLocation() { 2165 if (this.webLocation == null) 2166 return false; 2167 for (CitationCitedArtifactWebLocationComponent item : this.webLocation) 2168 if (!item.isEmpty()) 2169 return true; 2170 return false; 2171 } 2172 2173 public CitationCitedArtifactWebLocationComponent addWebLocation() { //3 2174 CitationCitedArtifactWebLocationComponent t = new CitationCitedArtifactWebLocationComponent(); 2175 if (this.webLocation == null) 2176 this.webLocation = new ArrayList<CitationCitedArtifactWebLocationComponent>(); 2177 this.webLocation.add(t); 2178 return t; 2179 } 2180 2181 public CitationCitedArtifactComponent addWebLocation(CitationCitedArtifactWebLocationComponent t) { //3 2182 if (t == null) 2183 return this; 2184 if (this.webLocation == null) 2185 this.webLocation = new ArrayList<CitationCitedArtifactWebLocationComponent>(); 2186 this.webLocation.add(t); 2187 return this; 2188 } 2189 2190 /** 2191 * @return The first repetition of repeating field {@link #webLocation}, creating it if it does not already exist {3} 2192 */ 2193 public CitationCitedArtifactWebLocationComponent getWebLocationFirstRep() { 2194 if (getWebLocation().isEmpty()) { 2195 addWebLocation(); 2196 } 2197 return getWebLocation().get(0); 2198 } 2199 2200 /** 2201 * @return {@link #classification} (The assignment to an organizing scheme.) 2202 */ 2203 public List<CitationCitedArtifactClassificationComponent> getClassification() { 2204 if (this.classification == null) 2205 this.classification = new ArrayList<CitationCitedArtifactClassificationComponent>(); 2206 return this.classification; 2207 } 2208 2209 /** 2210 * @return Returns a reference to <code>this</code> for easy method chaining 2211 */ 2212 public CitationCitedArtifactComponent setClassification(List<CitationCitedArtifactClassificationComponent> theClassification) { 2213 this.classification = theClassification; 2214 return this; 2215 } 2216 2217 public boolean hasClassification() { 2218 if (this.classification == null) 2219 return false; 2220 for (CitationCitedArtifactClassificationComponent item : this.classification) 2221 if (!item.isEmpty()) 2222 return true; 2223 return false; 2224 } 2225 2226 public CitationCitedArtifactClassificationComponent addClassification() { //3 2227 CitationCitedArtifactClassificationComponent t = new CitationCitedArtifactClassificationComponent(); 2228 if (this.classification == null) 2229 this.classification = new ArrayList<CitationCitedArtifactClassificationComponent>(); 2230 this.classification.add(t); 2231 return t; 2232 } 2233 2234 public CitationCitedArtifactComponent addClassification(CitationCitedArtifactClassificationComponent t) { //3 2235 if (t == null) 2236 return this; 2237 if (this.classification == null) 2238 this.classification = new ArrayList<CitationCitedArtifactClassificationComponent>(); 2239 this.classification.add(t); 2240 return this; 2241 } 2242 2243 /** 2244 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 2245 */ 2246 public CitationCitedArtifactClassificationComponent getClassificationFirstRep() { 2247 if (getClassification().isEmpty()) { 2248 addClassification(); 2249 } 2250 return getClassification().get(0); 2251 } 2252 2253 /** 2254 * @return {@link #contributorship} (This element is used to list authors and other contributors, their contact information, specific contributions, and summary statements.) 2255 */ 2256 public CitationCitedArtifactContributorshipComponent getContributorship() { 2257 if (this.contributorship == null) 2258 if (Configuration.errorOnAutoCreate()) 2259 throw new Error("Attempt to auto-create CitationCitedArtifactComponent.contributorship"); 2260 else if (Configuration.doAutoCreate()) 2261 this.contributorship = new CitationCitedArtifactContributorshipComponent(); // cc 2262 return this.contributorship; 2263 } 2264 2265 public boolean hasContributorship() { 2266 return this.contributorship != null && !this.contributorship.isEmpty(); 2267 } 2268 2269 /** 2270 * @param value {@link #contributorship} (This element is used to list authors and other contributors, their contact information, specific contributions, and summary statements.) 2271 */ 2272 public CitationCitedArtifactComponent setContributorship(CitationCitedArtifactContributorshipComponent value) { 2273 this.contributorship = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return {@link #note} (Any additional information or content for the article or artifact.) 2279 */ 2280 public List<Annotation> getNote() { 2281 if (this.note == null) 2282 this.note = new ArrayList<Annotation>(); 2283 return this.note; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public CitationCitedArtifactComponent setNote(List<Annotation> theNote) { 2290 this.note = theNote; 2291 return this; 2292 } 2293 2294 public boolean hasNote() { 2295 if (this.note == null) 2296 return false; 2297 for (Annotation item : this.note) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public Annotation addNote() { //3 2304 Annotation t = new Annotation(); 2305 if (this.note == null) 2306 this.note = new ArrayList<Annotation>(); 2307 this.note.add(t); 2308 return t; 2309 } 2310 2311 public CitationCitedArtifactComponent addNote(Annotation t) { //3 2312 if (t == null) 2313 return this; 2314 if (this.note == null) 2315 this.note = new ArrayList<Annotation>(); 2316 this.note.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2322 */ 2323 public Annotation getNoteFirstRep() { 2324 if (getNote().isEmpty()) { 2325 addNote(); 2326 } 2327 return getNote().get(0); 2328 } 2329 2330 protected void listChildren(List<Property> children) { 2331 super.listChildren(children); 2332 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify the cited artifact when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2333 children.add(new Property("relatedIdentifier", "Identifier", "A formal identifier that is used to identify things closely related to the cited artifact.", 0, java.lang.Integer.MAX_VALUE, relatedIdentifier)); 2334 children.add(new Property("dateAccessed", "dateTime", "When the cited artifact was accessed.", 0, 1, dateAccessed)); 2335 children.add(new Property("version", "", "The defined version of the cited artifact.", 0, 1, version)); 2336 children.add(new Property("currentState", "CodeableConcept", "The status of the cited artifact.", 0, java.lang.Integer.MAX_VALUE, currentState)); 2337 children.add(new Property("statusDate", "", "An effective date or period, historical or future, actual or expected, for a status of the cited artifact.", 0, java.lang.Integer.MAX_VALUE, statusDate)); 2338 children.add(new Property("title", "", "The title details of the article or artifact.", 0, java.lang.Integer.MAX_VALUE, title)); 2339 children.add(new Property("abstract", "", "The abstract may be used to convey article-contained abstracts, externally-created abstracts, or other descriptive summaries.", 0, java.lang.Integer.MAX_VALUE, abstract_)); 2340 children.add(new Property("part", "", "The component of the article or artifact.", 0, 1, part)); 2341 children.add(new Property("relatesTo", "", "The artifact related to the cited artifact.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 2342 children.add(new Property("publicationForm", "", "If multiple, used to represent alternative forms of the article that are not separate citations.", 0, java.lang.Integer.MAX_VALUE, publicationForm)); 2343 children.add(new Property("webLocation", "", "Used for any URL for the article or artifact cited.", 0, java.lang.Integer.MAX_VALUE, webLocation)); 2344 children.add(new Property("classification", "", "The assignment to an organizing scheme.", 0, java.lang.Integer.MAX_VALUE, classification)); 2345 children.add(new Property("contributorship", "", "This element is used to list authors and other contributors, their contact information, specific contributions, and summary statements.", 0, 1, contributorship)); 2346 children.add(new Property("note", "Annotation", "Any additional information or content for the article or artifact.", 0, java.lang.Integer.MAX_VALUE, note)); 2347 } 2348 2349 @Override 2350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2351 switch (_hash) { 2352 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify the cited artifact when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2353 case -1007604940: /*relatedIdentifier*/ return new Property("relatedIdentifier", "Identifier", "A formal identifier that is used to identify things closely related to the cited artifact.", 0, java.lang.Integer.MAX_VALUE, relatedIdentifier); 2354 case 540917457: /*dateAccessed*/ return new Property("dateAccessed", "dateTime", "When the cited artifact was accessed.", 0, 1, dateAccessed); 2355 case 351608024: /*version*/ return new Property("version", "", "The defined version of the cited artifact.", 0, 1, version); 2356 case 1457822360: /*currentState*/ return new Property("currentState", "CodeableConcept", "The status of the cited artifact.", 0, java.lang.Integer.MAX_VALUE, currentState); 2357 case 247524032: /*statusDate*/ return new Property("statusDate", "", "An effective date or period, historical or future, actual or expected, for a status of the cited artifact.", 0, java.lang.Integer.MAX_VALUE, statusDate); 2358 case 110371416: /*title*/ return new Property("title", "", "The title details of the article or artifact.", 0, java.lang.Integer.MAX_VALUE, title); 2359 case 1732898850: /*abstract*/ return new Property("abstract", "", "The abstract may be used to convey article-contained abstracts, externally-created abstracts, or other descriptive summaries.", 0, java.lang.Integer.MAX_VALUE, abstract_); 2360 case 3433459: /*part*/ return new Property("part", "", "The component of the article or artifact.", 0, 1, part); 2361 case -7765931: /*relatesTo*/ return new Property("relatesTo", "", "The artifact related to the cited artifact.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 2362 case 1470639376: /*publicationForm*/ return new Property("publicationForm", "", "If multiple, used to represent alternative forms of the article that are not separate citations.", 0, java.lang.Integer.MAX_VALUE, publicationForm); 2363 case -828032215: /*webLocation*/ return new Property("webLocation", "", "Used for any URL for the article or artifact cited.", 0, java.lang.Integer.MAX_VALUE, webLocation); 2364 case 382350310: /*classification*/ return new Property("classification", "", "The assignment to an organizing scheme.", 0, java.lang.Integer.MAX_VALUE, classification); 2365 case 538727831: /*contributorship*/ return new Property("contributorship", "", "This element is used to list authors and other contributors, their contact information, specific contributions, and summary statements.", 0, 1, contributorship); 2366 case 3387378: /*note*/ return new Property("note", "Annotation", "Any additional information or content for the article or artifact.", 0, java.lang.Integer.MAX_VALUE, note); 2367 default: return super.getNamedProperty(_hash, _name, _checkValid); 2368 } 2369 2370 } 2371 2372 @Override 2373 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2374 switch (hash) { 2375 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2376 case -1007604940: /*relatedIdentifier*/ return this.relatedIdentifier == null ? new Base[0] : this.relatedIdentifier.toArray(new Base[this.relatedIdentifier.size()]); // Identifier 2377 case 540917457: /*dateAccessed*/ return this.dateAccessed == null ? new Base[0] : new Base[] {this.dateAccessed}; // DateTimeType 2378 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // CitationCitedArtifactVersionComponent 2379 case 1457822360: /*currentState*/ return this.currentState == null ? new Base[0] : this.currentState.toArray(new Base[this.currentState.size()]); // CodeableConcept 2380 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : this.statusDate.toArray(new Base[this.statusDate.size()]); // CitationCitedArtifactStatusDateComponent 2381 case 110371416: /*title*/ return this.title == null ? new Base[0] : this.title.toArray(new Base[this.title.size()]); // CitationCitedArtifactTitleComponent 2382 case 1732898850: /*abstract*/ return this.abstract_ == null ? new Base[0] : this.abstract_.toArray(new Base[this.abstract_.size()]); // CitationCitedArtifactAbstractComponent 2383 case 3433459: /*part*/ return this.part == null ? new Base[0] : new Base[] {this.part}; // CitationCitedArtifactPartComponent 2384 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // CitationCitedArtifactRelatesToComponent 2385 case 1470639376: /*publicationForm*/ return this.publicationForm == null ? new Base[0] : this.publicationForm.toArray(new Base[this.publicationForm.size()]); // CitationCitedArtifactPublicationFormComponent 2386 case -828032215: /*webLocation*/ return this.webLocation == null ? new Base[0] : this.webLocation.toArray(new Base[this.webLocation.size()]); // CitationCitedArtifactWebLocationComponent 2387 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // CitationCitedArtifactClassificationComponent 2388 case 538727831: /*contributorship*/ return this.contributorship == null ? new Base[0] : new Base[] {this.contributorship}; // CitationCitedArtifactContributorshipComponent 2389 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2390 default: return super.getProperty(hash, name, checkValid); 2391 } 2392 2393 } 2394 2395 @Override 2396 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2397 switch (hash) { 2398 case -1618432855: // identifier 2399 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2400 return value; 2401 case -1007604940: // relatedIdentifier 2402 this.getRelatedIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2403 return value; 2404 case 540917457: // dateAccessed 2405 this.dateAccessed = TypeConvertor.castToDateTime(value); // DateTimeType 2406 return value; 2407 case 351608024: // version 2408 this.version = (CitationCitedArtifactVersionComponent) value; // CitationCitedArtifactVersionComponent 2409 return value; 2410 case 1457822360: // currentState 2411 this.getCurrentState().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2412 return value; 2413 case 247524032: // statusDate 2414 this.getStatusDate().add((CitationCitedArtifactStatusDateComponent) value); // CitationCitedArtifactStatusDateComponent 2415 return value; 2416 case 110371416: // title 2417 this.getTitle().add((CitationCitedArtifactTitleComponent) value); // CitationCitedArtifactTitleComponent 2418 return value; 2419 case 1732898850: // abstract 2420 this.getAbstract().add((CitationCitedArtifactAbstractComponent) value); // CitationCitedArtifactAbstractComponent 2421 return value; 2422 case 3433459: // part 2423 this.part = (CitationCitedArtifactPartComponent) value; // CitationCitedArtifactPartComponent 2424 return value; 2425 case -7765931: // relatesTo 2426 this.getRelatesTo().add((CitationCitedArtifactRelatesToComponent) value); // CitationCitedArtifactRelatesToComponent 2427 return value; 2428 case 1470639376: // publicationForm 2429 this.getPublicationForm().add((CitationCitedArtifactPublicationFormComponent) value); // CitationCitedArtifactPublicationFormComponent 2430 return value; 2431 case -828032215: // webLocation 2432 this.getWebLocation().add((CitationCitedArtifactWebLocationComponent) value); // CitationCitedArtifactWebLocationComponent 2433 return value; 2434 case 382350310: // classification 2435 this.getClassification().add((CitationCitedArtifactClassificationComponent) value); // CitationCitedArtifactClassificationComponent 2436 return value; 2437 case 538727831: // contributorship 2438 this.contributorship = (CitationCitedArtifactContributorshipComponent) value; // CitationCitedArtifactContributorshipComponent 2439 return value; 2440 case 3387378: // note 2441 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2442 return value; 2443 default: return super.setProperty(hash, name, value); 2444 } 2445 2446 } 2447 2448 @Override 2449 public Base setProperty(String name, Base value) throws FHIRException { 2450 if (name.equals("identifier")) { 2451 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2452 } else if (name.equals("relatedIdentifier")) { 2453 this.getRelatedIdentifier().add(TypeConvertor.castToIdentifier(value)); 2454 } else if (name.equals("dateAccessed")) { 2455 this.dateAccessed = TypeConvertor.castToDateTime(value); // DateTimeType 2456 } else if (name.equals("version")) { 2457 this.version = (CitationCitedArtifactVersionComponent) value; // CitationCitedArtifactVersionComponent 2458 } else if (name.equals("currentState")) { 2459 this.getCurrentState().add(TypeConvertor.castToCodeableConcept(value)); 2460 } else if (name.equals("statusDate")) { 2461 this.getStatusDate().add((CitationCitedArtifactStatusDateComponent) value); 2462 } else if (name.equals("title")) { 2463 this.getTitle().add((CitationCitedArtifactTitleComponent) value); 2464 } else if (name.equals("abstract")) { 2465 this.getAbstract().add((CitationCitedArtifactAbstractComponent) value); 2466 } else if (name.equals("part")) { 2467 this.part = (CitationCitedArtifactPartComponent) value; // CitationCitedArtifactPartComponent 2468 } else if (name.equals("relatesTo")) { 2469 this.getRelatesTo().add((CitationCitedArtifactRelatesToComponent) value); 2470 } else if (name.equals("publicationForm")) { 2471 this.getPublicationForm().add((CitationCitedArtifactPublicationFormComponent) value); 2472 } else if (name.equals("webLocation")) { 2473 this.getWebLocation().add((CitationCitedArtifactWebLocationComponent) value); 2474 } else if (name.equals("classification")) { 2475 this.getClassification().add((CitationCitedArtifactClassificationComponent) value); 2476 } else if (name.equals("contributorship")) { 2477 this.contributorship = (CitationCitedArtifactContributorshipComponent) value; // CitationCitedArtifactContributorshipComponent 2478 } else if (name.equals("note")) { 2479 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2480 } else 2481 return super.setProperty(name, value); 2482 return value; 2483 } 2484 2485 @Override 2486 public void removeChild(String name, Base value) throws FHIRException { 2487 if (name.equals("identifier")) { 2488 this.getIdentifier().remove(value); 2489 } else if (name.equals("relatedIdentifier")) { 2490 this.getRelatedIdentifier().remove(value); 2491 } else if (name.equals("dateAccessed")) { 2492 this.dateAccessed = null; 2493 } else if (name.equals("version")) { 2494 this.version = (CitationCitedArtifactVersionComponent) value; // CitationCitedArtifactVersionComponent 2495 } else if (name.equals("currentState")) { 2496 this.getCurrentState().remove(value); 2497 } else if (name.equals("statusDate")) { 2498 this.getStatusDate().remove((CitationCitedArtifactStatusDateComponent) value); 2499 } else if (name.equals("title")) { 2500 this.getTitle().remove((CitationCitedArtifactTitleComponent) value); 2501 } else if (name.equals("abstract")) { 2502 this.getAbstract().remove((CitationCitedArtifactAbstractComponent) value); 2503 } else if (name.equals("part")) { 2504 this.part = (CitationCitedArtifactPartComponent) value; // CitationCitedArtifactPartComponent 2505 } else if (name.equals("relatesTo")) { 2506 this.getRelatesTo().remove((CitationCitedArtifactRelatesToComponent) value); 2507 } else if (name.equals("publicationForm")) { 2508 this.getPublicationForm().remove((CitationCitedArtifactPublicationFormComponent) value); 2509 } else if (name.equals("webLocation")) { 2510 this.getWebLocation().remove((CitationCitedArtifactWebLocationComponent) value); 2511 } else if (name.equals("classification")) { 2512 this.getClassification().remove((CitationCitedArtifactClassificationComponent) value); 2513 } else if (name.equals("contributorship")) { 2514 this.contributorship = (CitationCitedArtifactContributorshipComponent) value; // CitationCitedArtifactContributorshipComponent 2515 } else if (name.equals("note")) { 2516 this.getNote().remove(value); 2517 } else 2518 super.removeChild(name, value); 2519 2520 } 2521 2522 @Override 2523 public Base makeProperty(int hash, String name) throws FHIRException { 2524 switch (hash) { 2525 case -1618432855: return addIdentifier(); 2526 case -1007604940: return addRelatedIdentifier(); 2527 case 540917457: return getDateAccessedElement(); 2528 case 351608024: return getVersion(); 2529 case 1457822360: return addCurrentState(); 2530 case 247524032: return addStatusDate(); 2531 case 110371416: return addTitle(); 2532 case 1732898850: return addAbstract(); 2533 case 3433459: return getPart(); 2534 case -7765931: return addRelatesTo(); 2535 case 1470639376: return addPublicationForm(); 2536 case -828032215: return addWebLocation(); 2537 case 382350310: return addClassification(); 2538 case 538727831: return getContributorship(); 2539 case 3387378: return addNote(); 2540 default: return super.makeProperty(hash, name); 2541 } 2542 2543 } 2544 2545 @Override 2546 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2547 switch (hash) { 2548 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2549 case -1007604940: /*relatedIdentifier*/ return new String[] {"Identifier"}; 2550 case 540917457: /*dateAccessed*/ return new String[] {"dateTime"}; 2551 case 351608024: /*version*/ return new String[] {}; 2552 case 1457822360: /*currentState*/ return new String[] {"CodeableConcept"}; 2553 case 247524032: /*statusDate*/ return new String[] {}; 2554 case 110371416: /*title*/ return new String[] {}; 2555 case 1732898850: /*abstract*/ return new String[] {}; 2556 case 3433459: /*part*/ return new String[] {}; 2557 case -7765931: /*relatesTo*/ return new String[] {}; 2558 case 1470639376: /*publicationForm*/ return new String[] {}; 2559 case -828032215: /*webLocation*/ return new String[] {}; 2560 case 382350310: /*classification*/ return new String[] {}; 2561 case 538727831: /*contributorship*/ return new String[] {}; 2562 case 3387378: /*note*/ return new String[] {"Annotation"}; 2563 default: return super.getTypesForProperty(hash, name); 2564 } 2565 2566 } 2567 2568 @Override 2569 public Base addChild(String name) throws FHIRException { 2570 if (name.equals("identifier")) { 2571 return addIdentifier(); 2572 } 2573 else if (name.equals("relatedIdentifier")) { 2574 return addRelatedIdentifier(); 2575 } 2576 else if (name.equals("dateAccessed")) { 2577 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.dateAccessed"); 2578 } 2579 else if (name.equals("version")) { 2580 this.version = new CitationCitedArtifactVersionComponent(); 2581 return this.version; 2582 } 2583 else if (name.equals("currentState")) { 2584 return addCurrentState(); 2585 } 2586 else if (name.equals("statusDate")) { 2587 return addStatusDate(); 2588 } 2589 else if (name.equals("title")) { 2590 return addTitle(); 2591 } 2592 else if (name.equals("abstract")) { 2593 return addAbstract(); 2594 } 2595 else if (name.equals("part")) { 2596 this.part = new CitationCitedArtifactPartComponent(); 2597 return this.part; 2598 } 2599 else if (name.equals("relatesTo")) { 2600 return addRelatesTo(); 2601 } 2602 else if (name.equals("publicationForm")) { 2603 return addPublicationForm(); 2604 } 2605 else if (name.equals("webLocation")) { 2606 return addWebLocation(); 2607 } 2608 else if (name.equals("classification")) { 2609 return addClassification(); 2610 } 2611 else if (name.equals("contributorship")) { 2612 this.contributorship = new CitationCitedArtifactContributorshipComponent(); 2613 return this.contributorship; 2614 } 2615 else if (name.equals("note")) { 2616 return addNote(); 2617 } 2618 else 2619 return super.addChild(name); 2620 } 2621 2622 public CitationCitedArtifactComponent copy() { 2623 CitationCitedArtifactComponent dst = new CitationCitedArtifactComponent(); 2624 copyValues(dst); 2625 return dst; 2626 } 2627 2628 public void copyValues(CitationCitedArtifactComponent dst) { 2629 super.copyValues(dst); 2630 if (identifier != null) { 2631 dst.identifier = new ArrayList<Identifier>(); 2632 for (Identifier i : identifier) 2633 dst.identifier.add(i.copy()); 2634 }; 2635 if (relatedIdentifier != null) { 2636 dst.relatedIdentifier = new ArrayList<Identifier>(); 2637 for (Identifier i : relatedIdentifier) 2638 dst.relatedIdentifier.add(i.copy()); 2639 }; 2640 dst.dateAccessed = dateAccessed == null ? null : dateAccessed.copy(); 2641 dst.version = version == null ? null : version.copy(); 2642 if (currentState != null) { 2643 dst.currentState = new ArrayList<CodeableConcept>(); 2644 for (CodeableConcept i : currentState) 2645 dst.currentState.add(i.copy()); 2646 }; 2647 if (statusDate != null) { 2648 dst.statusDate = new ArrayList<CitationCitedArtifactStatusDateComponent>(); 2649 for (CitationCitedArtifactStatusDateComponent i : statusDate) 2650 dst.statusDate.add(i.copy()); 2651 }; 2652 if (title != null) { 2653 dst.title = new ArrayList<CitationCitedArtifactTitleComponent>(); 2654 for (CitationCitedArtifactTitleComponent i : title) 2655 dst.title.add(i.copy()); 2656 }; 2657 if (abstract_ != null) { 2658 dst.abstract_ = new ArrayList<CitationCitedArtifactAbstractComponent>(); 2659 for (CitationCitedArtifactAbstractComponent i : abstract_) 2660 dst.abstract_.add(i.copy()); 2661 }; 2662 dst.part = part == null ? null : part.copy(); 2663 if (relatesTo != null) { 2664 dst.relatesTo = new ArrayList<CitationCitedArtifactRelatesToComponent>(); 2665 for (CitationCitedArtifactRelatesToComponent i : relatesTo) 2666 dst.relatesTo.add(i.copy()); 2667 }; 2668 if (publicationForm != null) { 2669 dst.publicationForm = new ArrayList<CitationCitedArtifactPublicationFormComponent>(); 2670 for (CitationCitedArtifactPublicationFormComponent i : publicationForm) 2671 dst.publicationForm.add(i.copy()); 2672 }; 2673 if (webLocation != null) { 2674 dst.webLocation = new ArrayList<CitationCitedArtifactWebLocationComponent>(); 2675 for (CitationCitedArtifactWebLocationComponent i : webLocation) 2676 dst.webLocation.add(i.copy()); 2677 }; 2678 if (classification != null) { 2679 dst.classification = new ArrayList<CitationCitedArtifactClassificationComponent>(); 2680 for (CitationCitedArtifactClassificationComponent i : classification) 2681 dst.classification.add(i.copy()); 2682 }; 2683 dst.contributorship = contributorship == null ? null : contributorship.copy(); 2684 if (note != null) { 2685 dst.note = new ArrayList<Annotation>(); 2686 for (Annotation i : note) 2687 dst.note.add(i.copy()); 2688 }; 2689 } 2690 2691 @Override 2692 public boolean equalsDeep(Base other_) { 2693 if (!super.equalsDeep(other_)) 2694 return false; 2695 if (!(other_ instanceof CitationCitedArtifactComponent)) 2696 return false; 2697 CitationCitedArtifactComponent o = (CitationCitedArtifactComponent) other_; 2698 return compareDeep(identifier, o.identifier, true) && compareDeep(relatedIdentifier, o.relatedIdentifier, true) 2699 && compareDeep(dateAccessed, o.dateAccessed, true) && compareDeep(version, o.version, true) && compareDeep(currentState, o.currentState, true) 2700 && compareDeep(statusDate, o.statusDate, true) && compareDeep(title, o.title, true) && compareDeep(abstract_, o.abstract_, true) 2701 && compareDeep(part, o.part, true) && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(publicationForm, o.publicationForm, true) 2702 && compareDeep(webLocation, o.webLocation, true) && compareDeep(classification, o.classification, true) 2703 && compareDeep(contributorship, o.contributorship, true) && compareDeep(note, o.note, true); 2704 } 2705 2706 @Override 2707 public boolean equalsShallow(Base other_) { 2708 if (!super.equalsShallow(other_)) 2709 return false; 2710 if (!(other_ instanceof CitationCitedArtifactComponent)) 2711 return false; 2712 CitationCitedArtifactComponent o = (CitationCitedArtifactComponent) other_; 2713 return compareValues(dateAccessed, o.dateAccessed, true); 2714 } 2715 2716 public boolean isEmpty() { 2717 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, relatedIdentifier 2718 , dateAccessed, version, currentState, statusDate, title, abstract_, part, relatesTo 2719 , publicationForm, webLocation, classification, contributorship, note); 2720 } 2721 2722 public String fhirType() { 2723 return "Citation.citedArtifact"; 2724 2725 } 2726 2727 } 2728 2729 @Block() 2730 public static class CitationCitedArtifactVersionComponent extends BackboneElement implements IBaseBackboneElement { 2731 /** 2732 * The version number or other version identifier. 2733 */ 2734 @Child(name = "value", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2735 @Description(shortDefinition="The version number or other version identifier", formalDefinition="The version number or other version identifier." ) 2736 protected StringType value; 2737 2738 /** 2739 * Citation for the main version of the cited artifact. 2740 */ 2741 @Child(name = "baseCitation", type = {Citation.class}, order=2, min=0, max=1, modifier=false, summary=false) 2742 @Description(shortDefinition="Citation for the main version of the cited artifact", formalDefinition="Citation for the main version of the cited artifact." ) 2743 protected Reference baseCitation; 2744 2745 private static final long serialVersionUID = 1437090319L; 2746 2747 /** 2748 * Constructor 2749 */ 2750 public CitationCitedArtifactVersionComponent() { 2751 super(); 2752 } 2753 2754 /** 2755 * Constructor 2756 */ 2757 public CitationCitedArtifactVersionComponent(String value) { 2758 super(); 2759 this.setValue(value); 2760 } 2761 2762 /** 2763 * @return {@link #value} (The version number or other version identifier.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2764 */ 2765 public StringType getValueElement() { 2766 if (this.value == null) 2767 if (Configuration.errorOnAutoCreate()) 2768 throw new Error("Attempt to auto-create CitationCitedArtifactVersionComponent.value"); 2769 else if (Configuration.doAutoCreate()) 2770 this.value = new StringType(); // bb 2771 return this.value; 2772 } 2773 2774 public boolean hasValueElement() { 2775 return this.value != null && !this.value.isEmpty(); 2776 } 2777 2778 public boolean hasValue() { 2779 return this.value != null && !this.value.isEmpty(); 2780 } 2781 2782 /** 2783 * @param value {@link #value} (The version number or other version identifier.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 2784 */ 2785 public CitationCitedArtifactVersionComponent setValueElement(StringType value) { 2786 this.value = value; 2787 return this; 2788 } 2789 2790 /** 2791 * @return The version number or other version identifier. 2792 */ 2793 public String getValue() { 2794 return this.value == null ? null : this.value.getValue(); 2795 } 2796 2797 /** 2798 * @param value The version number or other version identifier. 2799 */ 2800 public CitationCitedArtifactVersionComponent setValue(String value) { 2801 if (this.value == null) 2802 this.value = new StringType(); 2803 this.value.setValue(value); 2804 return this; 2805 } 2806 2807 /** 2808 * @return {@link #baseCitation} (Citation for the main version of the cited artifact.) 2809 */ 2810 public Reference getBaseCitation() { 2811 if (this.baseCitation == null) 2812 if (Configuration.errorOnAutoCreate()) 2813 throw new Error("Attempt to auto-create CitationCitedArtifactVersionComponent.baseCitation"); 2814 else if (Configuration.doAutoCreate()) 2815 this.baseCitation = new Reference(); // cc 2816 return this.baseCitation; 2817 } 2818 2819 public boolean hasBaseCitation() { 2820 return this.baseCitation != null && !this.baseCitation.isEmpty(); 2821 } 2822 2823 /** 2824 * @param value {@link #baseCitation} (Citation for the main version of the cited artifact.) 2825 */ 2826 public CitationCitedArtifactVersionComponent setBaseCitation(Reference value) { 2827 this.baseCitation = value; 2828 return this; 2829 } 2830 2831 protected void listChildren(List<Property> children) { 2832 super.listChildren(children); 2833 children.add(new Property("value", "string", "The version number or other version identifier.", 0, 1, value)); 2834 children.add(new Property("baseCitation", "Reference(Citation)", "Citation for the main version of the cited artifact.", 0, 1, baseCitation)); 2835 } 2836 2837 @Override 2838 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2839 switch (_hash) { 2840 case 111972721: /*value*/ return new Property("value", "string", "The version number or other version identifier.", 0, 1, value); 2841 case 1182995672: /*baseCitation*/ return new Property("baseCitation", "Reference(Citation)", "Citation for the main version of the cited artifact.", 0, 1, baseCitation); 2842 default: return super.getNamedProperty(_hash, _name, _checkValid); 2843 } 2844 2845 } 2846 2847 @Override 2848 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2849 switch (hash) { 2850 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 2851 case 1182995672: /*baseCitation*/ return this.baseCitation == null ? new Base[0] : new Base[] {this.baseCitation}; // Reference 2852 default: return super.getProperty(hash, name, checkValid); 2853 } 2854 2855 } 2856 2857 @Override 2858 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2859 switch (hash) { 2860 case 111972721: // value 2861 this.value = TypeConvertor.castToString(value); // StringType 2862 return value; 2863 case 1182995672: // baseCitation 2864 this.baseCitation = TypeConvertor.castToReference(value); // Reference 2865 return value; 2866 default: return super.setProperty(hash, name, value); 2867 } 2868 2869 } 2870 2871 @Override 2872 public Base setProperty(String name, Base value) throws FHIRException { 2873 if (name.equals("value")) { 2874 this.value = TypeConvertor.castToString(value); // StringType 2875 } else if (name.equals("baseCitation")) { 2876 this.baseCitation = TypeConvertor.castToReference(value); // Reference 2877 } else 2878 return super.setProperty(name, value); 2879 return value; 2880 } 2881 2882 @Override 2883 public void removeChild(String name, Base value) throws FHIRException { 2884 if (name.equals("value")) { 2885 this.value = null; 2886 } else if (name.equals("baseCitation")) { 2887 this.baseCitation = null; 2888 } else 2889 super.removeChild(name, value); 2890 2891 } 2892 2893 @Override 2894 public Base makeProperty(int hash, String name) throws FHIRException { 2895 switch (hash) { 2896 case 111972721: return getValueElement(); 2897 case 1182995672: return getBaseCitation(); 2898 default: return super.makeProperty(hash, name); 2899 } 2900 2901 } 2902 2903 @Override 2904 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2905 switch (hash) { 2906 case 111972721: /*value*/ return new String[] {"string"}; 2907 case 1182995672: /*baseCitation*/ return new String[] {"Reference"}; 2908 default: return super.getTypesForProperty(hash, name); 2909 } 2910 2911 } 2912 2913 @Override 2914 public Base addChild(String name) throws FHIRException { 2915 if (name.equals("value")) { 2916 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.version.value"); 2917 } 2918 else if (name.equals("baseCitation")) { 2919 this.baseCitation = new Reference(); 2920 return this.baseCitation; 2921 } 2922 else 2923 return super.addChild(name); 2924 } 2925 2926 public CitationCitedArtifactVersionComponent copy() { 2927 CitationCitedArtifactVersionComponent dst = new CitationCitedArtifactVersionComponent(); 2928 copyValues(dst); 2929 return dst; 2930 } 2931 2932 public void copyValues(CitationCitedArtifactVersionComponent dst) { 2933 super.copyValues(dst); 2934 dst.value = value == null ? null : value.copy(); 2935 dst.baseCitation = baseCitation == null ? null : baseCitation.copy(); 2936 } 2937 2938 @Override 2939 public boolean equalsDeep(Base other_) { 2940 if (!super.equalsDeep(other_)) 2941 return false; 2942 if (!(other_ instanceof CitationCitedArtifactVersionComponent)) 2943 return false; 2944 CitationCitedArtifactVersionComponent o = (CitationCitedArtifactVersionComponent) other_; 2945 return compareDeep(value, o.value, true) && compareDeep(baseCitation, o.baseCitation, true); 2946 } 2947 2948 @Override 2949 public boolean equalsShallow(Base other_) { 2950 if (!super.equalsShallow(other_)) 2951 return false; 2952 if (!(other_ instanceof CitationCitedArtifactVersionComponent)) 2953 return false; 2954 CitationCitedArtifactVersionComponent o = (CitationCitedArtifactVersionComponent) other_; 2955 return compareValues(value, o.value, true); 2956 } 2957 2958 public boolean isEmpty() { 2959 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, baseCitation); 2960 } 2961 2962 public String fhirType() { 2963 return "Citation.citedArtifact.version"; 2964 2965 } 2966 2967 } 2968 2969 @Block() 2970 public static class CitationCitedArtifactStatusDateComponent extends BackboneElement implements IBaseBackboneElement { 2971 /** 2972 * A definition of the status associated with a date or period. 2973 */ 2974 @Child(name = "activity", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2975 @Description(shortDefinition="Classification of the status", formalDefinition="A definition of the status associated with a date or period." ) 2976 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/cited-artifact-status-type") 2977 protected CodeableConcept activity; 2978 2979 /** 2980 * Either occurred or expected. 2981 */ 2982 @Child(name = "actual", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2983 @Description(shortDefinition="Either occurred or expected", formalDefinition="Either occurred or expected." ) 2984 protected BooleanType actual; 2985 2986 /** 2987 * When the status started and/or ended. 2988 */ 2989 @Child(name = "period", type = {Period.class}, order=3, min=1, max=1, modifier=false, summary=false) 2990 @Description(shortDefinition="When the status started and/or ended", formalDefinition="When the status started and/or ended." ) 2991 protected Period period; 2992 2993 private static final long serialVersionUID = 1123586924L; 2994 2995 /** 2996 * Constructor 2997 */ 2998 public CitationCitedArtifactStatusDateComponent() { 2999 super(); 3000 } 3001 3002 /** 3003 * Constructor 3004 */ 3005 public CitationCitedArtifactStatusDateComponent(CodeableConcept activity, Period period) { 3006 super(); 3007 this.setActivity(activity); 3008 this.setPeriod(period); 3009 } 3010 3011 /** 3012 * @return {@link #activity} (A definition of the status associated with a date or period.) 3013 */ 3014 public CodeableConcept getActivity() { 3015 if (this.activity == null) 3016 if (Configuration.errorOnAutoCreate()) 3017 throw new Error("Attempt to auto-create CitationCitedArtifactStatusDateComponent.activity"); 3018 else if (Configuration.doAutoCreate()) 3019 this.activity = new CodeableConcept(); // cc 3020 return this.activity; 3021 } 3022 3023 public boolean hasActivity() { 3024 return this.activity != null && !this.activity.isEmpty(); 3025 } 3026 3027 /** 3028 * @param value {@link #activity} (A definition of the status associated with a date or period.) 3029 */ 3030 public CitationCitedArtifactStatusDateComponent setActivity(CodeableConcept value) { 3031 this.activity = value; 3032 return this; 3033 } 3034 3035 /** 3036 * @return {@link #actual} (Either occurred or expected.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 3037 */ 3038 public BooleanType getActualElement() { 3039 if (this.actual == null) 3040 if (Configuration.errorOnAutoCreate()) 3041 throw new Error("Attempt to auto-create CitationCitedArtifactStatusDateComponent.actual"); 3042 else if (Configuration.doAutoCreate()) 3043 this.actual = new BooleanType(); // bb 3044 return this.actual; 3045 } 3046 3047 public boolean hasActualElement() { 3048 return this.actual != null && !this.actual.isEmpty(); 3049 } 3050 3051 public boolean hasActual() { 3052 return this.actual != null && !this.actual.isEmpty(); 3053 } 3054 3055 /** 3056 * @param value {@link #actual} (Either occurred or expected.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 3057 */ 3058 public CitationCitedArtifactStatusDateComponent setActualElement(BooleanType value) { 3059 this.actual = value; 3060 return this; 3061 } 3062 3063 /** 3064 * @return Either occurred or expected. 3065 */ 3066 public boolean getActual() { 3067 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 3068 } 3069 3070 /** 3071 * @param value Either occurred or expected. 3072 */ 3073 public CitationCitedArtifactStatusDateComponent setActual(boolean value) { 3074 if (this.actual == null) 3075 this.actual = new BooleanType(); 3076 this.actual.setValue(value); 3077 return this; 3078 } 3079 3080 /** 3081 * @return {@link #period} (When the status started and/or ended.) 3082 */ 3083 public Period getPeriod() { 3084 if (this.period == null) 3085 if (Configuration.errorOnAutoCreate()) 3086 throw new Error("Attempt to auto-create CitationCitedArtifactStatusDateComponent.period"); 3087 else if (Configuration.doAutoCreate()) 3088 this.period = new Period(); // cc 3089 return this.period; 3090 } 3091 3092 public boolean hasPeriod() { 3093 return this.period != null && !this.period.isEmpty(); 3094 } 3095 3096 /** 3097 * @param value {@link #period} (When the status started and/or ended.) 3098 */ 3099 public CitationCitedArtifactStatusDateComponent setPeriod(Period value) { 3100 this.period = value; 3101 return this; 3102 } 3103 3104 protected void listChildren(List<Property> children) { 3105 super.listChildren(children); 3106 children.add(new Property("activity", "CodeableConcept", "A definition of the status associated with a date or period.", 0, 1, activity)); 3107 children.add(new Property("actual", "boolean", "Either occurred or expected.", 0, 1, actual)); 3108 children.add(new Property("period", "Period", "When the status started and/or ended.", 0, 1, period)); 3109 } 3110 3111 @Override 3112 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3113 switch (_hash) { 3114 case -1655966961: /*activity*/ return new Property("activity", "CodeableConcept", "A definition of the status associated with a date or period.", 0, 1, activity); 3115 case -1422939762: /*actual*/ return new Property("actual", "boolean", "Either occurred or expected.", 0, 1, actual); 3116 case -991726143: /*period*/ return new Property("period", "Period", "When the status started and/or ended.", 0, 1, period); 3117 default: return super.getNamedProperty(_hash, _name, _checkValid); 3118 } 3119 3120 } 3121 3122 @Override 3123 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3124 switch (hash) { 3125 case -1655966961: /*activity*/ return this.activity == null ? new Base[0] : new Base[] {this.activity}; // CodeableConcept 3126 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 3127 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3128 default: return super.getProperty(hash, name, checkValid); 3129 } 3130 3131 } 3132 3133 @Override 3134 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3135 switch (hash) { 3136 case -1655966961: // activity 3137 this.activity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3138 return value; 3139 case -1422939762: // actual 3140 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 3141 return value; 3142 case -991726143: // period 3143 this.period = TypeConvertor.castToPeriod(value); // Period 3144 return value; 3145 default: return super.setProperty(hash, name, value); 3146 } 3147 3148 } 3149 3150 @Override 3151 public Base setProperty(String name, Base value) throws FHIRException { 3152 if (name.equals("activity")) { 3153 this.activity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3154 } else if (name.equals("actual")) { 3155 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 3156 } else if (name.equals("period")) { 3157 this.period = TypeConvertor.castToPeriod(value); // Period 3158 } else 3159 return super.setProperty(name, value); 3160 return value; 3161 } 3162 3163 @Override 3164 public void removeChild(String name, Base value) throws FHIRException { 3165 if (name.equals("activity")) { 3166 this.activity = null; 3167 } else if (name.equals("actual")) { 3168 this.actual = null; 3169 } else if (name.equals("period")) { 3170 this.period = null; 3171 } else 3172 super.removeChild(name, value); 3173 3174 } 3175 3176 @Override 3177 public Base makeProperty(int hash, String name) throws FHIRException { 3178 switch (hash) { 3179 case -1655966961: return getActivity(); 3180 case -1422939762: return getActualElement(); 3181 case -991726143: return getPeriod(); 3182 default: return super.makeProperty(hash, name); 3183 } 3184 3185 } 3186 3187 @Override 3188 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3189 switch (hash) { 3190 case -1655966961: /*activity*/ return new String[] {"CodeableConcept"}; 3191 case -1422939762: /*actual*/ return new String[] {"boolean"}; 3192 case -991726143: /*period*/ return new String[] {"Period"}; 3193 default: return super.getTypesForProperty(hash, name); 3194 } 3195 3196 } 3197 3198 @Override 3199 public Base addChild(String name) throws FHIRException { 3200 if (name.equals("activity")) { 3201 this.activity = new CodeableConcept(); 3202 return this.activity; 3203 } 3204 else if (name.equals("actual")) { 3205 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.statusDate.actual"); 3206 } 3207 else if (name.equals("period")) { 3208 this.period = new Period(); 3209 return this.period; 3210 } 3211 else 3212 return super.addChild(name); 3213 } 3214 3215 public CitationCitedArtifactStatusDateComponent copy() { 3216 CitationCitedArtifactStatusDateComponent dst = new CitationCitedArtifactStatusDateComponent(); 3217 copyValues(dst); 3218 return dst; 3219 } 3220 3221 public void copyValues(CitationCitedArtifactStatusDateComponent dst) { 3222 super.copyValues(dst); 3223 dst.activity = activity == null ? null : activity.copy(); 3224 dst.actual = actual == null ? null : actual.copy(); 3225 dst.period = period == null ? null : period.copy(); 3226 } 3227 3228 @Override 3229 public boolean equalsDeep(Base other_) { 3230 if (!super.equalsDeep(other_)) 3231 return false; 3232 if (!(other_ instanceof CitationCitedArtifactStatusDateComponent)) 3233 return false; 3234 CitationCitedArtifactStatusDateComponent o = (CitationCitedArtifactStatusDateComponent) other_; 3235 return compareDeep(activity, o.activity, true) && compareDeep(actual, o.actual, true) && compareDeep(period, o.period, true) 3236 ; 3237 } 3238 3239 @Override 3240 public boolean equalsShallow(Base other_) { 3241 if (!super.equalsShallow(other_)) 3242 return false; 3243 if (!(other_ instanceof CitationCitedArtifactStatusDateComponent)) 3244 return false; 3245 CitationCitedArtifactStatusDateComponent o = (CitationCitedArtifactStatusDateComponent) other_; 3246 return compareValues(actual, o.actual, true); 3247 } 3248 3249 public boolean isEmpty() { 3250 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(activity, actual, period 3251 ); 3252 } 3253 3254 public String fhirType() { 3255 return "Citation.citedArtifact.statusDate"; 3256 3257 } 3258 3259 } 3260 3261 @Block() 3262 public static class CitationCitedArtifactTitleComponent extends BackboneElement implements IBaseBackboneElement { 3263 /** 3264 * Used to express the reason for or classification of the title. 3265 */ 3266 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3267 @Description(shortDefinition="The kind of title", formalDefinition="Used to express the reason for or classification of the title." ) 3268 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/title-type") 3269 protected List<CodeableConcept> type; 3270 3271 /** 3272 * Used to express the specific language of the title. 3273 */ 3274 @Child(name = "language", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3275 @Description(shortDefinition="Used to express the specific language", formalDefinition="Used to express the specific language of the title." ) 3276 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 3277 protected CodeableConcept language; 3278 3279 /** 3280 * The title of the article or artifact. 3281 */ 3282 @Child(name = "text", type = {MarkdownType.class}, order=3, min=1, max=1, modifier=false, summary=false) 3283 @Description(shortDefinition="The title of the article or artifact", formalDefinition="The title of the article or artifact." ) 3284 protected MarkdownType text; 3285 3286 private static final long serialVersionUID = 1526221998L; 3287 3288 /** 3289 * Constructor 3290 */ 3291 public CitationCitedArtifactTitleComponent() { 3292 super(); 3293 } 3294 3295 /** 3296 * Constructor 3297 */ 3298 public CitationCitedArtifactTitleComponent(String text) { 3299 super(); 3300 this.setText(text); 3301 } 3302 3303 /** 3304 * @return {@link #type} (Used to express the reason for or classification of the title.) 3305 */ 3306 public List<CodeableConcept> getType() { 3307 if (this.type == null) 3308 this.type = new ArrayList<CodeableConcept>(); 3309 return this.type; 3310 } 3311 3312 /** 3313 * @return Returns a reference to <code>this</code> for easy method chaining 3314 */ 3315 public CitationCitedArtifactTitleComponent setType(List<CodeableConcept> theType) { 3316 this.type = theType; 3317 return this; 3318 } 3319 3320 public boolean hasType() { 3321 if (this.type == null) 3322 return false; 3323 for (CodeableConcept item : this.type) 3324 if (!item.isEmpty()) 3325 return true; 3326 return false; 3327 } 3328 3329 public CodeableConcept addType() { //3 3330 CodeableConcept t = new CodeableConcept(); 3331 if (this.type == null) 3332 this.type = new ArrayList<CodeableConcept>(); 3333 this.type.add(t); 3334 return t; 3335 } 3336 3337 public CitationCitedArtifactTitleComponent addType(CodeableConcept t) { //3 3338 if (t == null) 3339 return this; 3340 if (this.type == null) 3341 this.type = new ArrayList<CodeableConcept>(); 3342 this.type.add(t); 3343 return this; 3344 } 3345 3346 /** 3347 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 3348 */ 3349 public CodeableConcept getTypeFirstRep() { 3350 if (getType().isEmpty()) { 3351 addType(); 3352 } 3353 return getType().get(0); 3354 } 3355 3356 /** 3357 * @return {@link #language} (Used to express the specific language of the title.) 3358 */ 3359 public CodeableConcept getLanguage() { 3360 if (this.language == null) 3361 if (Configuration.errorOnAutoCreate()) 3362 throw new Error("Attempt to auto-create CitationCitedArtifactTitleComponent.language"); 3363 else if (Configuration.doAutoCreate()) 3364 this.language = new CodeableConcept(); // cc 3365 return this.language; 3366 } 3367 3368 public boolean hasLanguage() { 3369 return this.language != null && !this.language.isEmpty(); 3370 } 3371 3372 /** 3373 * @param value {@link #language} (Used to express the specific language of the title.) 3374 */ 3375 public CitationCitedArtifactTitleComponent setLanguage(CodeableConcept value) { 3376 this.language = value; 3377 return this; 3378 } 3379 3380 /** 3381 * @return {@link #text} (The title of the article or artifact.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 3382 */ 3383 public MarkdownType getTextElement() { 3384 if (this.text == null) 3385 if (Configuration.errorOnAutoCreate()) 3386 throw new Error("Attempt to auto-create CitationCitedArtifactTitleComponent.text"); 3387 else if (Configuration.doAutoCreate()) 3388 this.text = new MarkdownType(); // bb 3389 return this.text; 3390 } 3391 3392 public boolean hasTextElement() { 3393 return this.text != null && !this.text.isEmpty(); 3394 } 3395 3396 public boolean hasText() { 3397 return this.text != null && !this.text.isEmpty(); 3398 } 3399 3400 /** 3401 * @param value {@link #text} (The title of the article or artifact.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 3402 */ 3403 public CitationCitedArtifactTitleComponent setTextElement(MarkdownType value) { 3404 this.text = value; 3405 return this; 3406 } 3407 3408 /** 3409 * @return The title of the article or artifact. 3410 */ 3411 public String getText() { 3412 return this.text == null ? null : this.text.getValue(); 3413 } 3414 3415 /** 3416 * @param value The title of the article or artifact. 3417 */ 3418 public CitationCitedArtifactTitleComponent setText(String value) { 3419 if (this.text == null) 3420 this.text = new MarkdownType(); 3421 this.text.setValue(value); 3422 return this; 3423 } 3424 3425 protected void listChildren(List<Property> children) { 3426 super.listChildren(children); 3427 children.add(new Property("type", "CodeableConcept", "Used to express the reason for or classification of the title.", 0, java.lang.Integer.MAX_VALUE, type)); 3428 children.add(new Property("language", "CodeableConcept", "Used to express the specific language of the title.", 0, 1, language)); 3429 children.add(new Property("text", "markdown", "The title of the article or artifact.", 0, 1, text)); 3430 } 3431 3432 @Override 3433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3434 switch (_hash) { 3435 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Used to express the reason for or classification of the title.", 0, java.lang.Integer.MAX_VALUE, type); 3436 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "Used to express the specific language of the title.", 0, 1, language); 3437 case 3556653: /*text*/ return new Property("text", "markdown", "The title of the article or artifact.", 0, 1, text); 3438 default: return super.getNamedProperty(_hash, _name, _checkValid); 3439 } 3440 3441 } 3442 3443 @Override 3444 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3445 switch (hash) { 3446 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3447 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 3448 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // MarkdownType 3449 default: return super.getProperty(hash, name, checkValid); 3450 } 3451 3452 } 3453 3454 @Override 3455 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3456 switch (hash) { 3457 case 3575610: // type 3458 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3459 return value; 3460 case -1613589672: // language 3461 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3462 return value; 3463 case 3556653: // text 3464 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 3465 return value; 3466 default: return super.setProperty(hash, name, value); 3467 } 3468 3469 } 3470 3471 @Override 3472 public Base setProperty(String name, Base value) throws FHIRException { 3473 if (name.equals("type")) { 3474 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 3475 } else if (name.equals("language")) { 3476 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3477 } else if (name.equals("text")) { 3478 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 3479 } else 3480 return super.setProperty(name, value); 3481 return value; 3482 } 3483 3484 @Override 3485 public void removeChild(String name, Base value) throws FHIRException { 3486 if (name.equals("type")) { 3487 this.getType().remove(value); 3488 } else if (name.equals("language")) { 3489 this.language = null; 3490 } else if (name.equals("text")) { 3491 this.text = null; 3492 } else 3493 super.removeChild(name, value); 3494 3495 } 3496 3497 @Override 3498 public Base makeProperty(int hash, String name) throws FHIRException { 3499 switch (hash) { 3500 case 3575610: return addType(); 3501 case -1613589672: return getLanguage(); 3502 case 3556653: return getTextElement(); 3503 default: return super.makeProperty(hash, name); 3504 } 3505 3506 } 3507 3508 @Override 3509 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3510 switch (hash) { 3511 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3512 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 3513 case 3556653: /*text*/ return new String[] {"markdown"}; 3514 default: return super.getTypesForProperty(hash, name); 3515 } 3516 3517 } 3518 3519 @Override 3520 public Base addChild(String name) throws FHIRException { 3521 if (name.equals("type")) { 3522 return addType(); 3523 } 3524 else if (name.equals("language")) { 3525 this.language = new CodeableConcept(); 3526 return this.language; 3527 } 3528 else if (name.equals("text")) { 3529 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.title.text"); 3530 } 3531 else 3532 return super.addChild(name); 3533 } 3534 3535 public CitationCitedArtifactTitleComponent copy() { 3536 CitationCitedArtifactTitleComponent dst = new CitationCitedArtifactTitleComponent(); 3537 copyValues(dst); 3538 return dst; 3539 } 3540 3541 public void copyValues(CitationCitedArtifactTitleComponent dst) { 3542 super.copyValues(dst); 3543 if (type != null) { 3544 dst.type = new ArrayList<CodeableConcept>(); 3545 for (CodeableConcept i : type) 3546 dst.type.add(i.copy()); 3547 }; 3548 dst.language = language == null ? null : language.copy(); 3549 dst.text = text == null ? null : text.copy(); 3550 } 3551 3552 @Override 3553 public boolean equalsDeep(Base other_) { 3554 if (!super.equalsDeep(other_)) 3555 return false; 3556 if (!(other_ instanceof CitationCitedArtifactTitleComponent)) 3557 return false; 3558 CitationCitedArtifactTitleComponent o = (CitationCitedArtifactTitleComponent) other_; 3559 return compareDeep(type, o.type, true) && compareDeep(language, o.language, true) && compareDeep(text, o.text, true) 3560 ; 3561 } 3562 3563 @Override 3564 public boolean equalsShallow(Base other_) { 3565 if (!super.equalsShallow(other_)) 3566 return false; 3567 if (!(other_ instanceof CitationCitedArtifactTitleComponent)) 3568 return false; 3569 CitationCitedArtifactTitleComponent o = (CitationCitedArtifactTitleComponent) other_; 3570 return compareValues(text, o.text, true); 3571 } 3572 3573 public boolean isEmpty() { 3574 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, language, text); 3575 } 3576 3577 public String fhirType() { 3578 return "Citation.citedArtifact.title"; 3579 3580 } 3581 3582 } 3583 3584 @Block() 3585 public static class CitationCitedArtifactAbstractComponent extends BackboneElement implements IBaseBackboneElement { 3586 /** 3587 * Used to express the reason for or classification of the abstract. 3588 */ 3589 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 3590 @Description(shortDefinition="The kind of abstract", formalDefinition="Used to express the reason for or classification of the abstract." ) 3591 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/cited-artifact-abstract-type") 3592 protected CodeableConcept type; 3593 3594 /** 3595 * Used to express the specific language of the abstract. 3596 */ 3597 @Child(name = "language", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3598 @Description(shortDefinition="Used to express the specific language", formalDefinition="Used to express the specific language of the abstract." ) 3599 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 3600 protected CodeableConcept language; 3601 3602 /** 3603 * Abstract content. 3604 */ 3605 @Child(name = "text", type = {MarkdownType.class}, order=3, min=1, max=1, modifier=false, summary=false) 3606 @Description(shortDefinition="Abstract content", formalDefinition="Abstract content." ) 3607 protected MarkdownType text; 3608 3609 /** 3610 * Copyright notice for the abstract. 3611 */ 3612 @Child(name = "copyright", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3613 @Description(shortDefinition="Copyright notice for the abstract", formalDefinition="Copyright notice for the abstract." ) 3614 protected MarkdownType copyright; 3615 3616 private static final long serialVersionUID = -1882363442L; 3617 3618 /** 3619 * Constructor 3620 */ 3621 public CitationCitedArtifactAbstractComponent() { 3622 super(); 3623 } 3624 3625 /** 3626 * Constructor 3627 */ 3628 public CitationCitedArtifactAbstractComponent(String text) { 3629 super(); 3630 this.setText(text); 3631 } 3632 3633 /** 3634 * @return {@link #type} (Used to express the reason for or classification of the abstract.) 3635 */ 3636 public CodeableConcept getType() { 3637 if (this.type == null) 3638 if (Configuration.errorOnAutoCreate()) 3639 throw new Error("Attempt to auto-create CitationCitedArtifactAbstractComponent.type"); 3640 else if (Configuration.doAutoCreate()) 3641 this.type = new CodeableConcept(); // cc 3642 return this.type; 3643 } 3644 3645 public boolean hasType() { 3646 return this.type != null && !this.type.isEmpty(); 3647 } 3648 3649 /** 3650 * @param value {@link #type} (Used to express the reason for or classification of the abstract.) 3651 */ 3652 public CitationCitedArtifactAbstractComponent setType(CodeableConcept value) { 3653 this.type = value; 3654 return this; 3655 } 3656 3657 /** 3658 * @return {@link #language} (Used to express the specific language of the abstract.) 3659 */ 3660 public CodeableConcept getLanguage() { 3661 if (this.language == null) 3662 if (Configuration.errorOnAutoCreate()) 3663 throw new Error("Attempt to auto-create CitationCitedArtifactAbstractComponent.language"); 3664 else if (Configuration.doAutoCreate()) 3665 this.language = new CodeableConcept(); // cc 3666 return this.language; 3667 } 3668 3669 public boolean hasLanguage() { 3670 return this.language != null && !this.language.isEmpty(); 3671 } 3672 3673 /** 3674 * @param value {@link #language} (Used to express the specific language of the abstract.) 3675 */ 3676 public CitationCitedArtifactAbstractComponent setLanguage(CodeableConcept value) { 3677 this.language = value; 3678 return this; 3679 } 3680 3681 /** 3682 * @return {@link #text} (Abstract content.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 3683 */ 3684 public MarkdownType getTextElement() { 3685 if (this.text == null) 3686 if (Configuration.errorOnAutoCreate()) 3687 throw new Error("Attempt to auto-create CitationCitedArtifactAbstractComponent.text"); 3688 else if (Configuration.doAutoCreate()) 3689 this.text = new MarkdownType(); // bb 3690 return this.text; 3691 } 3692 3693 public boolean hasTextElement() { 3694 return this.text != null && !this.text.isEmpty(); 3695 } 3696 3697 public boolean hasText() { 3698 return this.text != null && !this.text.isEmpty(); 3699 } 3700 3701 /** 3702 * @param value {@link #text} (Abstract content.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 3703 */ 3704 public CitationCitedArtifactAbstractComponent setTextElement(MarkdownType value) { 3705 this.text = value; 3706 return this; 3707 } 3708 3709 /** 3710 * @return Abstract content. 3711 */ 3712 public String getText() { 3713 return this.text == null ? null : this.text.getValue(); 3714 } 3715 3716 /** 3717 * @param value Abstract content. 3718 */ 3719 public CitationCitedArtifactAbstractComponent setText(String value) { 3720 if (this.text == null) 3721 this.text = new MarkdownType(); 3722 this.text.setValue(value); 3723 return this; 3724 } 3725 3726 /** 3727 * @return {@link #copyright} (Copyright notice for the abstract.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3728 */ 3729 public MarkdownType getCopyrightElement() { 3730 if (this.copyright == null) 3731 if (Configuration.errorOnAutoCreate()) 3732 throw new Error("Attempt to auto-create CitationCitedArtifactAbstractComponent.copyright"); 3733 else if (Configuration.doAutoCreate()) 3734 this.copyright = new MarkdownType(); // bb 3735 return this.copyright; 3736 } 3737 3738 public boolean hasCopyrightElement() { 3739 return this.copyright != null && !this.copyright.isEmpty(); 3740 } 3741 3742 public boolean hasCopyright() { 3743 return this.copyright != null && !this.copyright.isEmpty(); 3744 } 3745 3746 /** 3747 * @param value {@link #copyright} (Copyright notice for the abstract.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3748 */ 3749 public CitationCitedArtifactAbstractComponent setCopyrightElement(MarkdownType value) { 3750 this.copyright = value; 3751 return this; 3752 } 3753 3754 /** 3755 * @return Copyright notice for the abstract. 3756 */ 3757 public String getCopyright() { 3758 return this.copyright == null ? null : this.copyright.getValue(); 3759 } 3760 3761 /** 3762 * @param value Copyright notice for the abstract. 3763 */ 3764 public CitationCitedArtifactAbstractComponent setCopyright(String value) { 3765 if (Utilities.noString(value)) 3766 this.copyright = null; 3767 else { 3768 if (this.copyright == null) 3769 this.copyright = new MarkdownType(); 3770 this.copyright.setValue(value); 3771 } 3772 return this; 3773 } 3774 3775 protected void listChildren(List<Property> children) { 3776 super.listChildren(children); 3777 children.add(new Property("type", "CodeableConcept", "Used to express the reason for or classification of the abstract.", 0, 1, type)); 3778 children.add(new Property("language", "CodeableConcept", "Used to express the specific language of the abstract.", 0, 1, language)); 3779 children.add(new Property("text", "markdown", "Abstract content.", 0, 1, text)); 3780 children.add(new Property("copyright", "markdown", "Copyright notice for the abstract.", 0, 1, copyright)); 3781 } 3782 3783 @Override 3784 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3785 switch (_hash) { 3786 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Used to express the reason for or classification of the abstract.", 0, 1, type); 3787 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "Used to express the specific language of the abstract.", 0, 1, language); 3788 case 3556653: /*text*/ return new Property("text", "markdown", "Abstract content.", 0, 1, text); 3789 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "Copyright notice for the abstract.", 0, 1, copyright); 3790 default: return super.getNamedProperty(_hash, _name, _checkValid); 3791 } 3792 3793 } 3794 3795 @Override 3796 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3797 switch (hash) { 3798 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3799 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 3800 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // MarkdownType 3801 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3802 default: return super.getProperty(hash, name, checkValid); 3803 } 3804 3805 } 3806 3807 @Override 3808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3809 switch (hash) { 3810 case 3575610: // type 3811 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3812 return value; 3813 case -1613589672: // language 3814 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3815 return value; 3816 case 3556653: // text 3817 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 3818 return value; 3819 case 1522889671: // copyright 3820 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3821 return value; 3822 default: return super.setProperty(hash, name, value); 3823 } 3824 3825 } 3826 3827 @Override 3828 public Base setProperty(String name, Base value) throws FHIRException { 3829 if (name.equals("type")) { 3830 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3831 } else if (name.equals("language")) { 3832 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3833 } else if (name.equals("text")) { 3834 this.text = TypeConvertor.castToMarkdown(value); // MarkdownType 3835 } else if (name.equals("copyright")) { 3836 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3837 } else 3838 return super.setProperty(name, value); 3839 return value; 3840 } 3841 3842 @Override 3843 public void removeChild(String name, Base value) throws FHIRException { 3844 if (name.equals("type")) { 3845 this.type = null; 3846 } else if (name.equals("language")) { 3847 this.language = null; 3848 } else if (name.equals("text")) { 3849 this.text = null; 3850 } else if (name.equals("copyright")) { 3851 this.copyright = null; 3852 } else 3853 super.removeChild(name, value); 3854 3855 } 3856 3857 @Override 3858 public Base makeProperty(int hash, String name) throws FHIRException { 3859 switch (hash) { 3860 case 3575610: return getType(); 3861 case -1613589672: return getLanguage(); 3862 case 3556653: return getTextElement(); 3863 case 1522889671: return getCopyrightElement(); 3864 default: return super.makeProperty(hash, name); 3865 } 3866 3867 } 3868 3869 @Override 3870 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3871 switch (hash) { 3872 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3873 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 3874 case 3556653: /*text*/ return new String[] {"markdown"}; 3875 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3876 default: return super.getTypesForProperty(hash, name); 3877 } 3878 3879 } 3880 3881 @Override 3882 public Base addChild(String name) throws FHIRException { 3883 if (name.equals("type")) { 3884 this.type = new CodeableConcept(); 3885 return this.type; 3886 } 3887 else if (name.equals("language")) { 3888 this.language = new CodeableConcept(); 3889 return this.language; 3890 } 3891 else if (name.equals("text")) { 3892 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.abstract.text"); 3893 } 3894 else if (name.equals("copyright")) { 3895 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.abstract.copyright"); 3896 } 3897 else 3898 return super.addChild(name); 3899 } 3900 3901 public CitationCitedArtifactAbstractComponent copy() { 3902 CitationCitedArtifactAbstractComponent dst = new CitationCitedArtifactAbstractComponent(); 3903 copyValues(dst); 3904 return dst; 3905 } 3906 3907 public void copyValues(CitationCitedArtifactAbstractComponent dst) { 3908 super.copyValues(dst); 3909 dst.type = type == null ? null : type.copy(); 3910 dst.language = language == null ? null : language.copy(); 3911 dst.text = text == null ? null : text.copy(); 3912 dst.copyright = copyright == null ? null : copyright.copy(); 3913 } 3914 3915 @Override 3916 public boolean equalsDeep(Base other_) { 3917 if (!super.equalsDeep(other_)) 3918 return false; 3919 if (!(other_ instanceof CitationCitedArtifactAbstractComponent)) 3920 return false; 3921 CitationCitedArtifactAbstractComponent o = (CitationCitedArtifactAbstractComponent) other_; 3922 return compareDeep(type, o.type, true) && compareDeep(language, o.language, true) && compareDeep(text, o.text, true) 3923 && compareDeep(copyright, o.copyright, true); 3924 } 3925 3926 @Override 3927 public boolean equalsShallow(Base other_) { 3928 if (!super.equalsShallow(other_)) 3929 return false; 3930 if (!(other_ instanceof CitationCitedArtifactAbstractComponent)) 3931 return false; 3932 CitationCitedArtifactAbstractComponent o = (CitationCitedArtifactAbstractComponent) other_; 3933 return compareValues(text, o.text, true) && compareValues(copyright, o.copyright, true); 3934 } 3935 3936 public boolean isEmpty() { 3937 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, language, text, copyright 3938 ); 3939 } 3940 3941 public String fhirType() { 3942 return "Citation.citedArtifact.abstract"; 3943 3944 } 3945 3946 } 3947 3948 @Block() 3949 public static class CitationCitedArtifactPartComponent extends BackboneElement implements IBaseBackboneElement { 3950 /** 3951 * The kind of component. 3952 */ 3953 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 3954 @Description(shortDefinition="The kind of component", formalDefinition="The kind of component." ) 3955 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/cited-artifact-part-type") 3956 protected CodeableConcept type; 3957 3958 /** 3959 * The specification of the component. 3960 */ 3961 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3962 @Description(shortDefinition="The specification of the component", formalDefinition="The specification of the component." ) 3963 protected StringType value; 3964 3965 /** 3966 * The citation for the full article or artifact. 3967 */ 3968 @Child(name = "baseCitation", type = {Citation.class}, order=3, min=0, max=1, modifier=false, summary=false) 3969 @Description(shortDefinition="The citation for the full article or artifact", formalDefinition="The citation for the full article or artifact." ) 3970 protected Reference baseCitation; 3971 3972 private static final long serialVersionUID = -765350500L; 3973 3974 /** 3975 * Constructor 3976 */ 3977 public CitationCitedArtifactPartComponent() { 3978 super(); 3979 } 3980 3981 /** 3982 * @return {@link #type} (The kind of component.) 3983 */ 3984 public CodeableConcept getType() { 3985 if (this.type == null) 3986 if (Configuration.errorOnAutoCreate()) 3987 throw new Error("Attempt to auto-create CitationCitedArtifactPartComponent.type"); 3988 else if (Configuration.doAutoCreate()) 3989 this.type = new CodeableConcept(); // cc 3990 return this.type; 3991 } 3992 3993 public boolean hasType() { 3994 return this.type != null && !this.type.isEmpty(); 3995 } 3996 3997 /** 3998 * @param value {@link #type} (The kind of component.) 3999 */ 4000 public CitationCitedArtifactPartComponent setType(CodeableConcept value) { 4001 this.type = value; 4002 return this; 4003 } 4004 4005 /** 4006 * @return {@link #value} (The specification of the component.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4007 */ 4008 public StringType getValueElement() { 4009 if (this.value == null) 4010 if (Configuration.errorOnAutoCreate()) 4011 throw new Error("Attempt to auto-create CitationCitedArtifactPartComponent.value"); 4012 else if (Configuration.doAutoCreate()) 4013 this.value = new StringType(); // bb 4014 return this.value; 4015 } 4016 4017 public boolean hasValueElement() { 4018 return this.value != null && !this.value.isEmpty(); 4019 } 4020 4021 public boolean hasValue() { 4022 return this.value != null && !this.value.isEmpty(); 4023 } 4024 4025 /** 4026 * @param value {@link #value} (The specification of the component.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4027 */ 4028 public CitationCitedArtifactPartComponent setValueElement(StringType value) { 4029 this.value = value; 4030 return this; 4031 } 4032 4033 /** 4034 * @return The specification of the component. 4035 */ 4036 public String getValue() { 4037 return this.value == null ? null : this.value.getValue(); 4038 } 4039 4040 /** 4041 * @param value The specification of the component. 4042 */ 4043 public CitationCitedArtifactPartComponent setValue(String value) { 4044 if (Utilities.noString(value)) 4045 this.value = null; 4046 else { 4047 if (this.value == null) 4048 this.value = new StringType(); 4049 this.value.setValue(value); 4050 } 4051 return this; 4052 } 4053 4054 /** 4055 * @return {@link #baseCitation} (The citation for the full article or artifact.) 4056 */ 4057 public Reference getBaseCitation() { 4058 if (this.baseCitation == null) 4059 if (Configuration.errorOnAutoCreate()) 4060 throw new Error("Attempt to auto-create CitationCitedArtifactPartComponent.baseCitation"); 4061 else if (Configuration.doAutoCreate()) 4062 this.baseCitation = new Reference(); // cc 4063 return this.baseCitation; 4064 } 4065 4066 public boolean hasBaseCitation() { 4067 return this.baseCitation != null && !this.baseCitation.isEmpty(); 4068 } 4069 4070 /** 4071 * @param value {@link #baseCitation} (The citation for the full article or artifact.) 4072 */ 4073 public CitationCitedArtifactPartComponent setBaseCitation(Reference value) { 4074 this.baseCitation = value; 4075 return this; 4076 } 4077 4078 protected void listChildren(List<Property> children) { 4079 super.listChildren(children); 4080 children.add(new Property("type", "CodeableConcept", "The kind of component.", 0, 1, type)); 4081 children.add(new Property("value", "string", "The specification of the component.", 0, 1, value)); 4082 children.add(new Property("baseCitation", "Reference(Citation)", "The citation for the full article or artifact.", 0, 1, baseCitation)); 4083 } 4084 4085 @Override 4086 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4087 switch (_hash) { 4088 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of component.", 0, 1, type); 4089 case 111972721: /*value*/ return new Property("value", "string", "The specification of the component.", 0, 1, value); 4090 case 1182995672: /*baseCitation*/ return new Property("baseCitation", "Reference(Citation)", "The citation for the full article or artifact.", 0, 1, baseCitation); 4091 default: return super.getNamedProperty(_hash, _name, _checkValid); 4092 } 4093 4094 } 4095 4096 @Override 4097 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4098 switch (hash) { 4099 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 4100 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 4101 case 1182995672: /*baseCitation*/ return this.baseCitation == null ? new Base[0] : new Base[] {this.baseCitation}; // Reference 4102 default: return super.getProperty(hash, name, checkValid); 4103 } 4104 4105 } 4106 4107 @Override 4108 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4109 switch (hash) { 4110 case 3575610: // type 4111 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4112 return value; 4113 case 111972721: // value 4114 this.value = TypeConvertor.castToString(value); // StringType 4115 return value; 4116 case 1182995672: // baseCitation 4117 this.baseCitation = TypeConvertor.castToReference(value); // Reference 4118 return value; 4119 default: return super.setProperty(hash, name, value); 4120 } 4121 4122 } 4123 4124 @Override 4125 public Base setProperty(String name, Base value) throws FHIRException { 4126 if (name.equals("type")) { 4127 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4128 } else if (name.equals("value")) { 4129 this.value = TypeConvertor.castToString(value); // StringType 4130 } else if (name.equals("baseCitation")) { 4131 this.baseCitation = TypeConvertor.castToReference(value); // Reference 4132 } else 4133 return super.setProperty(name, value); 4134 return value; 4135 } 4136 4137 @Override 4138 public void removeChild(String name, Base value) throws FHIRException { 4139 if (name.equals("type")) { 4140 this.type = null; 4141 } else if (name.equals("value")) { 4142 this.value = null; 4143 } else if (name.equals("baseCitation")) { 4144 this.baseCitation = null; 4145 } else 4146 super.removeChild(name, value); 4147 4148 } 4149 4150 @Override 4151 public Base makeProperty(int hash, String name) throws FHIRException { 4152 switch (hash) { 4153 case 3575610: return getType(); 4154 case 111972721: return getValueElement(); 4155 case 1182995672: return getBaseCitation(); 4156 default: return super.makeProperty(hash, name); 4157 } 4158 4159 } 4160 4161 @Override 4162 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4163 switch (hash) { 4164 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4165 case 111972721: /*value*/ return new String[] {"string"}; 4166 case 1182995672: /*baseCitation*/ return new String[] {"Reference"}; 4167 default: return super.getTypesForProperty(hash, name); 4168 } 4169 4170 } 4171 4172 @Override 4173 public Base addChild(String name) throws FHIRException { 4174 if (name.equals("type")) { 4175 this.type = new CodeableConcept(); 4176 return this.type; 4177 } 4178 else if (name.equals("value")) { 4179 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.part.value"); 4180 } 4181 else if (name.equals("baseCitation")) { 4182 this.baseCitation = new Reference(); 4183 return this.baseCitation; 4184 } 4185 else 4186 return super.addChild(name); 4187 } 4188 4189 public CitationCitedArtifactPartComponent copy() { 4190 CitationCitedArtifactPartComponent dst = new CitationCitedArtifactPartComponent(); 4191 copyValues(dst); 4192 return dst; 4193 } 4194 4195 public void copyValues(CitationCitedArtifactPartComponent dst) { 4196 super.copyValues(dst); 4197 dst.type = type == null ? null : type.copy(); 4198 dst.value = value == null ? null : value.copy(); 4199 dst.baseCitation = baseCitation == null ? null : baseCitation.copy(); 4200 } 4201 4202 @Override 4203 public boolean equalsDeep(Base other_) { 4204 if (!super.equalsDeep(other_)) 4205 return false; 4206 if (!(other_ instanceof CitationCitedArtifactPartComponent)) 4207 return false; 4208 CitationCitedArtifactPartComponent o = (CitationCitedArtifactPartComponent) other_; 4209 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(baseCitation, o.baseCitation, true) 4210 ; 4211 } 4212 4213 @Override 4214 public boolean equalsShallow(Base other_) { 4215 if (!super.equalsShallow(other_)) 4216 return false; 4217 if (!(other_ instanceof CitationCitedArtifactPartComponent)) 4218 return false; 4219 CitationCitedArtifactPartComponent o = (CitationCitedArtifactPartComponent) other_; 4220 return compareValues(value, o.value, true); 4221 } 4222 4223 public boolean isEmpty() { 4224 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, baseCitation 4225 ); 4226 } 4227 4228 public String fhirType() { 4229 return "Citation.citedArtifact.part"; 4230 4231 } 4232 4233 } 4234 4235 @Block() 4236 public static class CitationCitedArtifactRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 4237 /** 4238 * The type of relationship to the related artifact. 4239 */ 4240 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4241 @Description(shortDefinition="documentation | justification | citation | predecessor | successor | derived-from | depends-on | composed-of | part-of | amends | amended-with | appends | appended-with | cites | cited-by | comments-on | comment-in | contains | contained-in | corrects | correction-in | replaces | replaced-with | retracts | retracted-by | signs | similar-to | supports | supported-with | transforms | transformed-into | transformed-with | documents | specification-of | created-with | cite-as | reprint | reprint-of", formalDefinition="The type of relationship to the related artifact." ) 4242 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-artifact-type-all") 4243 protected Enumeration<RelatedArtifactTypeExpanded> type; 4244 4245 /** 4246 * Provides additional classifiers of the related artifact. 4247 */ 4248 @Child(name = "classifier", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4249 @Description(shortDefinition="Additional classifiers", formalDefinition="Provides additional classifiers of the related artifact." ) 4250 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-artifact-classifier") 4251 protected List<CodeableConcept> classifier; 4252 4253 /** 4254 * A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index. 4255 */ 4256 @Child(name = "label", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4257 @Description(shortDefinition="Short label", formalDefinition="A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index." ) 4258 protected StringType label; 4259 4260 /** 4261 * A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 4262 */ 4263 @Child(name = "display", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4264 @Description(shortDefinition="Brief description of the related artifact", formalDefinition="A brief description of the document or knowledge resource being referenced, suitable for display to a consumer." ) 4265 protected StringType display; 4266 4267 /** 4268 * A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 4269 */ 4270 @Child(name = "citation", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 4271 @Description(shortDefinition="Bibliographic citation for the artifact", formalDefinition="A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format." ) 4272 protected MarkdownType citation; 4273 4274 /** 4275 * The document being referenced, represented as an attachment. Do not use this element if using the resource element to provide the canonical to the related artifact. 4276 */ 4277 @Child(name = "document", type = {Attachment.class}, order=6, min=0, max=1, modifier=false, summary=false) 4278 @Description(shortDefinition="What document is being referenced", formalDefinition="The document being referenced, represented as an attachment. Do not use this element if using the resource element to provide the canonical to the related artifact." ) 4279 protected Attachment document; 4280 4281 /** 4282 * The related artifact, such as a library, value set, profile, or other knowledge resource. 4283 */ 4284 @Child(name = "resource", type = {CanonicalType.class}, order=7, min=0, max=1, modifier=false, summary=false) 4285 @Description(shortDefinition="What artifact is being referenced", formalDefinition="The related artifact, such as a library, value set, profile, or other knowledge resource." ) 4286 protected CanonicalType resource; 4287 4288 /** 4289 * The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource. 4290 */ 4291 @Child(name = "resourceReference", type = {Reference.class}, order=8, min=0, max=1, modifier=false, summary=false) 4292 @Description(shortDefinition="What artifact, if not a conformance resource", formalDefinition="The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource." ) 4293 protected Reference resourceReference; 4294 4295 private static final long serialVersionUID = 1537406923L; 4296 4297 /** 4298 * Constructor 4299 */ 4300 public CitationCitedArtifactRelatesToComponent() { 4301 super(); 4302 } 4303 4304 /** 4305 * Constructor 4306 */ 4307 public CitationCitedArtifactRelatesToComponent(RelatedArtifactTypeExpanded type) { 4308 super(); 4309 this.setType(type); 4310 } 4311 4312 /** 4313 * @return {@link #type} (The type of relationship to the related artifact.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4314 */ 4315 public Enumeration<RelatedArtifactTypeExpanded> getTypeElement() { 4316 if (this.type == null) 4317 if (Configuration.errorOnAutoCreate()) 4318 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.type"); 4319 else if (Configuration.doAutoCreate()) 4320 this.type = new Enumeration<RelatedArtifactTypeExpanded>(new RelatedArtifactTypeExpandedEnumFactory()); // bb 4321 return this.type; 4322 } 4323 4324 public boolean hasTypeElement() { 4325 return this.type != null && !this.type.isEmpty(); 4326 } 4327 4328 public boolean hasType() { 4329 return this.type != null && !this.type.isEmpty(); 4330 } 4331 4332 /** 4333 * @param value {@link #type} (The type of relationship to the related artifact.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 4334 */ 4335 public CitationCitedArtifactRelatesToComponent setTypeElement(Enumeration<RelatedArtifactTypeExpanded> value) { 4336 this.type = value; 4337 return this; 4338 } 4339 4340 /** 4341 * @return The type of relationship to the related artifact. 4342 */ 4343 public RelatedArtifactTypeExpanded getType() { 4344 return this.type == null ? null : this.type.getValue(); 4345 } 4346 4347 /** 4348 * @param value The type of relationship to the related artifact. 4349 */ 4350 public CitationCitedArtifactRelatesToComponent setType(RelatedArtifactTypeExpanded value) { 4351 if (this.type == null) 4352 this.type = new Enumeration<RelatedArtifactTypeExpanded>(new RelatedArtifactTypeExpandedEnumFactory()); 4353 this.type.setValue(value); 4354 return this; 4355 } 4356 4357 /** 4358 * @return {@link #classifier} (Provides additional classifiers of the related artifact.) 4359 */ 4360 public List<CodeableConcept> getClassifier() { 4361 if (this.classifier == null) 4362 this.classifier = new ArrayList<CodeableConcept>(); 4363 return this.classifier; 4364 } 4365 4366 /** 4367 * @return Returns a reference to <code>this</code> for easy method chaining 4368 */ 4369 public CitationCitedArtifactRelatesToComponent setClassifier(List<CodeableConcept> theClassifier) { 4370 this.classifier = theClassifier; 4371 return this; 4372 } 4373 4374 public boolean hasClassifier() { 4375 if (this.classifier == null) 4376 return false; 4377 for (CodeableConcept item : this.classifier) 4378 if (!item.isEmpty()) 4379 return true; 4380 return false; 4381 } 4382 4383 public CodeableConcept addClassifier() { //3 4384 CodeableConcept t = new CodeableConcept(); 4385 if (this.classifier == null) 4386 this.classifier = new ArrayList<CodeableConcept>(); 4387 this.classifier.add(t); 4388 return t; 4389 } 4390 4391 public CitationCitedArtifactRelatesToComponent addClassifier(CodeableConcept t) { //3 4392 if (t == null) 4393 return this; 4394 if (this.classifier == null) 4395 this.classifier = new ArrayList<CodeableConcept>(); 4396 this.classifier.add(t); 4397 return this; 4398 } 4399 4400 /** 4401 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 4402 */ 4403 public CodeableConcept getClassifierFirstRep() { 4404 if (getClassifier().isEmpty()) { 4405 addClassifier(); 4406 } 4407 return getClassifier().get(0); 4408 } 4409 4410 /** 4411 * @return {@link #label} (A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 4412 */ 4413 public StringType getLabelElement() { 4414 if (this.label == null) 4415 if (Configuration.errorOnAutoCreate()) 4416 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.label"); 4417 else if (Configuration.doAutoCreate()) 4418 this.label = new StringType(); // bb 4419 return this.label; 4420 } 4421 4422 public boolean hasLabelElement() { 4423 return this.label != null && !this.label.isEmpty(); 4424 } 4425 4426 public boolean hasLabel() { 4427 return this.label != null && !this.label.isEmpty(); 4428 } 4429 4430 /** 4431 * @param value {@link #label} (A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 4432 */ 4433 public CitationCitedArtifactRelatesToComponent setLabelElement(StringType value) { 4434 this.label = value; 4435 return this; 4436 } 4437 4438 /** 4439 * @return A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index. 4440 */ 4441 public String getLabel() { 4442 return this.label == null ? null : this.label.getValue(); 4443 } 4444 4445 /** 4446 * @param value A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index. 4447 */ 4448 public CitationCitedArtifactRelatesToComponent setLabel(String value) { 4449 if (Utilities.noString(value)) 4450 this.label = null; 4451 else { 4452 if (this.label == null) 4453 this.label = new StringType(); 4454 this.label.setValue(value); 4455 } 4456 return this; 4457 } 4458 4459 /** 4460 * @return {@link #display} (A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 4461 */ 4462 public StringType getDisplayElement() { 4463 if (this.display == null) 4464 if (Configuration.errorOnAutoCreate()) 4465 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.display"); 4466 else if (Configuration.doAutoCreate()) 4467 this.display = new StringType(); // bb 4468 return this.display; 4469 } 4470 4471 public boolean hasDisplayElement() { 4472 return this.display != null && !this.display.isEmpty(); 4473 } 4474 4475 public boolean hasDisplay() { 4476 return this.display != null && !this.display.isEmpty(); 4477 } 4478 4479 /** 4480 * @param value {@link #display} (A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 4481 */ 4482 public CitationCitedArtifactRelatesToComponent setDisplayElement(StringType value) { 4483 this.display = value; 4484 return this; 4485 } 4486 4487 /** 4488 * @return A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 4489 */ 4490 public String getDisplay() { 4491 return this.display == null ? null : this.display.getValue(); 4492 } 4493 4494 /** 4495 * @param value A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 4496 */ 4497 public CitationCitedArtifactRelatesToComponent setDisplay(String value) { 4498 if (Utilities.noString(value)) 4499 this.display = null; 4500 else { 4501 if (this.display == null) 4502 this.display = new StringType(); 4503 this.display.setValue(value); 4504 } 4505 return this; 4506 } 4507 4508 /** 4509 * @return {@link #citation} (A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.). This is the underlying object with id, value and extensions. The accessor "getCitation" gives direct access to the value 4510 */ 4511 public MarkdownType getCitationElement() { 4512 if (this.citation == null) 4513 if (Configuration.errorOnAutoCreate()) 4514 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.citation"); 4515 else if (Configuration.doAutoCreate()) 4516 this.citation = new MarkdownType(); // bb 4517 return this.citation; 4518 } 4519 4520 public boolean hasCitationElement() { 4521 return this.citation != null && !this.citation.isEmpty(); 4522 } 4523 4524 public boolean hasCitation() { 4525 return this.citation != null && !this.citation.isEmpty(); 4526 } 4527 4528 /** 4529 * @param value {@link #citation} (A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.). This is the underlying object with id, value and extensions. The accessor "getCitation" gives direct access to the value 4530 */ 4531 public CitationCitedArtifactRelatesToComponent setCitationElement(MarkdownType value) { 4532 this.citation = value; 4533 return this; 4534 } 4535 4536 /** 4537 * @return A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 4538 */ 4539 public String getCitation() { 4540 return this.citation == null ? null : this.citation.getValue(); 4541 } 4542 4543 /** 4544 * @param value A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 4545 */ 4546 public CitationCitedArtifactRelatesToComponent setCitation(String value) { 4547 if (Utilities.noString(value)) 4548 this.citation = null; 4549 else { 4550 if (this.citation == null) 4551 this.citation = new MarkdownType(); 4552 this.citation.setValue(value); 4553 } 4554 return this; 4555 } 4556 4557 /** 4558 * @return {@link #document} (The document being referenced, represented as an attachment. Do not use this element if using the resource element to provide the canonical to the related artifact.) 4559 */ 4560 public Attachment getDocument() { 4561 if (this.document == null) 4562 if (Configuration.errorOnAutoCreate()) 4563 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.document"); 4564 else if (Configuration.doAutoCreate()) 4565 this.document = new Attachment(); // cc 4566 return this.document; 4567 } 4568 4569 public boolean hasDocument() { 4570 return this.document != null && !this.document.isEmpty(); 4571 } 4572 4573 /** 4574 * @param value {@link #document} (The document being referenced, represented as an attachment. Do not use this element if using the resource element to provide the canonical to the related artifact.) 4575 */ 4576 public CitationCitedArtifactRelatesToComponent setDocument(Attachment value) { 4577 this.document = value; 4578 return this; 4579 } 4580 4581 /** 4582 * @return {@link #resource} (The related artifact, such as a library, value set, profile, or other knowledge resource.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 4583 */ 4584 public CanonicalType getResourceElement() { 4585 if (this.resource == null) 4586 if (Configuration.errorOnAutoCreate()) 4587 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.resource"); 4588 else if (Configuration.doAutoCreate()) 4589 this.resource = new CanonicalType(); // bb 4590 return this.resource; 4591 } 4592 4593 public boolean hasResourceElement() { 4594 return this.resource != null && !this.resource.isEmpty(); 4595 } 4596 4597 public boolean hasResource() { 4598 return this.resource != null && !this.resource.isEmpty(); 4599 } 4600 4601 /** 4602 * @param value {@link #resource} (The related artifact, such as a library, value set, profile, or other knowledge resource.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 4603 */ 4604 public CitationCitedArtifactRelatesToComponent setResourceElement(CanonicalType value) { 4605 this.resource = value; 4606 return this; 4607 } 4608 4609 /** 4610 * @return The related artifact, such as a library, value set, profile, or other knowledge resource. 4611 */ 4612 public String getResource() { 4613 return this.resource == null ? null : this.resource.getValue(); 4614 } 4615 4616 /** 4617 * @param value The related artifact, such as a library, value set, profile, or other knowledge resource. 4618 */ 4619 public CitationCitedArtifactRelatesToComponent setResource(String value) { 4620 if (Utilities.noString(value)) 4621 this.resource = null; 4622 else { 4623 if (this.resource == null) 4624 this.resource = new CanonicalType(); 4625 this.resource.setValue(value); 4626 } 4627 return this; 4628 } 4629 4630 /** 4631 * @return {@link #resourceReference} (The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.) 4632 */ 4633 public Reference getResourceReference() { 4634 if (this.resourceReference == null) 4635 if (Configuration.errorOnAutoCreate()) 4636 throw new Error("Attempt to auto-create CitationCitedArtifactRelatesToComponent.resourceReference"); 4637 else if (Configuration.doAutoCreate()) 4638 this.resourceReference = new Reference(); // cc 4639 return this.resourceReference; 4640 } 4641 4642 public boolean hasResourceReference() { 4643 return this.resourceReference != null && !this.resourceReference.isEmpty(); 4644 } 4645 4646 /** 4647 * @param value {@link #resourceReference} (The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.) 4648 */ 4649 public CitationCitedArtifactRelatesToComponent setResourceReference(Reference value) { 4650 this.resourceReference = value; 4651 return this; 4652 } 4653 4654 protected void listChildren(List<Property> children) { 4655 super.listChildren(children); 4656 children.add(new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type)); 4657 children.add(new Property("classifier", "CodeableConcept", "Provides additional classifiers of the related artifact.", 0, java.lang.Integer.MAX_VALUE, classifier)); 4658 children.add(new Property("label", "string", "A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index.", 0, 1, label)); 4659 children.add(new Property("display", "string", "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 0, 1, display)); 4660 children.add(new Property("citation", "markdown", "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 0, 1, citation)); 4661 children.add(new Property("document", "Attachment", "The document being referenced, represented as an attachment. Do not use this element if using the resource element to provide the canonical to the related artifact.", 0, 1, document)); 4662 children.add(new Property("resource", "canonical", "The related artifact, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource)); 4663 children.add(new Property("resourceReference", "Reference", "The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.", 0, 1, resourceReference)); 4664 } 4665 4666 @Override 4667 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4668 switch (_hash) { 4669 case 3575610: /*type*/ return new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type); 4670 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "Provides additional classifiers of the related artifact.", 0, java.lang.Integer.MAX_VALUE, classifier); 4671 case 102727412: /*label*/ return new Property("label", "string", "A short label that can be used to reference the related artifact from elsewhere in the containing artifact, such as a footnote index.", 0, 1, label); 4672 case 1671764162: /*display*/ return new Property("display", "string", "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 0, 1, display); 4673 case -1442706713: /*citation*/ return new Property("citation", "markdown", "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 0, 1, citation); 4674 case 861720859: /*document*/ return new Property("document", "Attachment", "The document being referenced, represented as an attachment. Do not use this element if using the resource element to provide the canonical to the related artifact.", 0, 1, document); 4675 case -341064690: /*resource*/ return new Property("resource", "canonical", "The related artifact, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource); 4676 case -610120995: /*resourceReference*/ return new Property("resourceReference", "Reference", "The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.", 0, 1, resourceReference); 4677 default: return super.getNamedProperty(_hash, _name, _checkValid); 4678 } 4679 4680 } 4681 4682 @Override 4683 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4684 switch (hash) { 4685 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<RelatedArtifactTypeExpanded> 4686 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 4687 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 4688 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 4689 case -1442706713: /*citation*/ return this.citation == null ? new Base[0] : new Base[] {this.citation}; // MarkdownType 4690 case 861720859: /*document*/ return this.document == null ? new Base[0] : new Base[] {this.document}; // Attachment 4691 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // CanonicalType 4692 case -610120995: /*resourceReference*/ return this.resourceReference == null ? new Base[0] : new Base[] {this.resourceReference}; // Reference 4693 default: return super.getProperty(hash, name, checkValid); 4694 } 4695 4696 } 4697 4698 @Override 4699 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4700 switch (hash) { 4701 case 3575610: // type 4702 value = new RelatedArtifactTypeExpandedEnumFactory().fromType(TypeConvertor.castToCode(value)); 4703 this.type = (Enumeration) value; // Enumeration<RelatedArtifactTypeExpanded> 4704 return value; 4705 case -281470431: // classifier 4706 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4707 return value; 4708 case 102727412: // label 4709 this.label = TypeConvertor.castToString(value); // StringType 4710 return value; 4711 case 1671764162: // display 4712 this.display = TypeConvertor.castToString(value); // StringType 4713 return value; 4714 case -1442706713: // citation 4715 this.citation = TypeConvertor.castToMarkdown(value); // MarkdownType 4716 return value; 4717 case 861720859: // document 4718 this.document = TypeConvertor.castToAttachment(value); // Attachment 4719 return value; 4720 case -341064690: // resource 4721 this.resource = TypeConvertor.castToCanonical(value); // CanonicalType 4722 return value; 4723 case -610120995: // resourceReference 4724 this.resourceReference = TypeConvertor.castToReference(value); // Reference 4725 return value; 4726 default: return super.setProperty(hash, name, value); 4727 } 4728 4729 } 4730 4731 @Override 4732 public Base setProperty(String name, Base value) throws FHIRException { 4733 if (name.equals("type")) { 4734 value = new RelatedArtifactTypeExpandedEnumFactory().fromType(TypeConvertor.castToCode(value)); 4735 this.type = (Enumeration) value; // Enumeration<RelatedArtifactTypeExpanded> 4736 } else if (name.equals("classifier")) { 4737 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 4738 } else if (name.equals("label")) { 4739 this.label = TypeConvertor.castToString(value); // StringType 4740 } else if (name.equals("display")) { 4741 this.display = TypeConvertor.castToString(value); // StringType 4742 } else if (name.equals("citation")) { 4743 this.citation = TypeConvertor.castToMarkdown(value); // MarkdownType 4744 } else if (name.equals("document")) { 4745 this.document = TypeConvertor.castToAttachment(value); // Attachment 4746 } else if (name.equals("resource")) { 4747 this.resource = TypeConvertor.castToCanonical(value); // CanonicalType 4748 } else if (name.equals("resourceReference")) { 4749 this.resourceReference = TypeConvertor.castToReference(value); // Reference 4750 } else 4751 return super.setProperty(name, value); 4752 return value; 4753 } 4754 4755 @Override 4756 public void removeChild(String name, Base value) throws FHIRException { 4757 if (name.equals("type")) { 4758 value = new RelatedArtifactTypeExpandedEnumFactory().fromType(TypeConvertor.castToCode(value)); 4759 this.type = (Enumeration) value; // Enumeration<RelatedArtifactTypeExpanded> 4760 } else if (name.equals("classifier")) { 4761 this.getClassifier().remove(value); 4762 } else if (name.equals("label")) { 4763 this.label = null; 4764 } else if (name.equals("display")) { 4765 this.display = null; 4766 } else if (name.equals("citation")) { 4767 this.citation = null; 4768 } else if (name.equals("document")) { 4769 this.document = null; 4770 } else if (name.equals("resource")) { 4771 this.resource = null; 4772 } else if (name.equals("resourceReference")) { 4773 this.resourceReference = null; 4774 } else 4775 super.removeChild(name, value); 4776 4777 } 4778 4779 @Override 4780 public Base makeProperty(int hash, String name) throws FHIRException { 4781 switch (hash) { 4782 case 3575610: return getTypeElement(); 4783 case -281470431: return addClassifier(); 4784 case 102727412: return getLabelElement(); 4785 case 1671764162: return getDisplayElement(); 4786 case -1442706713: return getCitationElement(); 4787 case 861720859: return getDocument(); 4788 case -341064690: return getResourceElement(); 4789 case -610120995: return getResourceReference(); 4790 default: return super.makeProperty(hash, name); 4791 } 4792 4793 } 4794 4795 @Override 4796 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4797 switch (hash) { 4798 case 3575610: /*type*/ return new String[] {"code"}; 4799 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 4800 case 102727412: /*label*/ return new String[] {"string"}; 4801 case 1671764162: /*display*/ return new String[] {"string"}; 4802 case -1442706713: /*citation*/ return new String[] {"markdown"}; 4803 case 861720859: /*document*/ return new String[] {"Attachment"}; 4804 case -341064690: /*resource*/ return new String[] {"canonical"}; 4805 case -610120995: /*resourceReference*/ return new String[] {"Reference"}; 4806 default: return super.getTypesForProperty(hash, name); 4807 } 4808 4809 } 4810 4811 @Override 4812 public Base addChild(String name) throws FHIRException { 4813 if (name.equals("type")) { 4814 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.relatesTo.type"); 4815 } 4816 else if (name.equals("classifier")) { 4817 return addClassifier(); 4818 } 4819 else if (name.equals("label")) { 4820 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.relatesTo.label"); 4821 } 4822 else if (name.equals("display")) { 4823 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.relatesTo.display"); 4824 } 4825 else if (name.equals("citation")) { 4826 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.relatesTo.citation"); 4827 } 4828 else if (name.equals("document")) { 4829 this.document = new Attachment(); 4830 return this.document; 4831 } 4832 else if (name.equals("resource")) { 4833 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.relatesTo.resource"); 4834 } 4835 else if (name.equals("resourceReference")) { 4836 this.resourceReference = new Reference(); 4837 return this.resourceReference; 4838 } 4839 else 4840 return super.addChild(name); 4841 } 4842 4843 public CitationCitedArtifactRelatesToComponent copy() { 4844 CitationCitedArtifactRelatesToComponent dst = new CitationCitedArtifactRelatesToComponent(); 4845 copyValues(dst); 4846 return dst; 4847 } 4848 4849 public void copyValues(CitationCitedArtifactRelatesToComponent dst) { 4850 super.copyValues(dst); 4851 dst.type = type == null ? null : type.copy(); 4852 if (classifier != null) { 4853 dst.classifier = new ArrayList<CodeableConcept>(); 4854 for (CodeableConcept i : classifier) 4855 dst.classifier.add(i.copy()); 4856 }; 4857 dst.label = label == null ? null : label.copy(); 4858 dst.display = display == null ? null : display.copy(); 4859 dst.citation = citation == null ? null : citation.copy(); 4860 dst.document = document == null ? null : document.copy(); 4861 dst.resource = resource == null ? null : resource.copy(); 4862 dst.resourceReference = resourceReference == null ? null : resourceReference.copy(); 4863 } 4864 4865 @Override 4866 public boolean equalsDeep(Base other_) { 4867 if (!super.equalsDeep(other_)) 4868 return false; 4869 if (!(other_ instanceof CitationCitedArtifactRelatesToComponent)) 4870 return false; 4871 CitationCitedArtifactRelatesToComponent o = (CitationCitedArtifactRelatesToComponent) other_; 4872 return compareDeep(type, o.type, true) && compareDeep(classifier, o.classifier, true) && compareDeep(label, o.label, true) 4873 && compareDeep(display, o.display, true) && compareDeep(citation, o.citation, true) && compareDeep(document, o.document, true) 4874 && compareDeep(resource, o.resource, true) && compareDeep(resourceReference, o.resourceReference, true) 4875 ; 4876 } 4877 4878 @Override 4879 public boolean equalsShallow(Base other_) { 4880 if (!super.equalsShallow(other_)) 4881 return false; 4882 if (!(other_ instanceof CitationCitedArtifactRelatesToComponent)) 4883 return false; 4884 CitationCitedArtifactRelatesToComponent o = (CitationCitedArtifactRelatesToComponent) other_; 4885 return compareValues(type, o.type, true) && compareValues(label, o.label, true) && compareValues(display, o.display, true) 4886 && compareValues(citation, o.citation, true) && compareValues(resource, o.resource, true); 4887 } 4888 4889 public boolean isEmpty() { 4890 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, classifier, label 4891 , display, citation, document, resource, resourceReference); 4892 } 4893 4894 public String fhirType() { 4895 return "Citation.citedArtifact.relatesTo"; 4896 4897 } 4898 4899 } 4900 4901 @Block() 4902 public static class CitationCitedArtifactPublicationFormComponent extends BackboneElement implements IBaseBackboneElement { 4903 /** 4904 * The collection the cited article or artifact is published in. 4905 */ 4906 @Child(name = "publishedIn", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 4907 @Description(shortDefinition="The collection the cited article or artifact is published in", formalDefinition="The collection the cited article or artifact is published in." ) 4908 protected CitationCitedArtifactPublicationFormPublishedInComponent publishedIn; 4909 4910 /** 4911 * Describes the form of the medium cited. Common codes are "Internet" or "Print". The CitedMedium value set has 6 codes. The codes internet, print, and offline-digital-storage are the common codes for a typical publication form, though internet and print are more common for study citations. Three additional codes (each appending one of the primary codes with "-without-issue" are used for situations when a study is published both within an issue (of a periodical release as commonly done for journals) AND is published separately from the issue (as commonly done with early online publication), to represent specific identification of the publication form not associated with the issue. 4912 */ 4913 @Child(name = "citedMedium", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4914 @Description(shortDefinition="Internet or Print", formalDefinition="Describes the form of the medium cited. Common codes are \"Internet\" or \"Print\". The CitedMedium value set has 6 codes. The codes internet, print, and offline-digital-storage are the common codes for a typical publication form, though internet and print are more common for study citations. Three additional codes (each appending one of the primary codes with \"-without-issue\" are used for situations when a study is published both within an issue (of a periodical release as commonly done for journals) AND is published separately from the issue (as commonly done with early online publication), to represent specific identification of the publication form not associated with the issue." ) 4915 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/cited-medium") 4916 protected CodeableConcept citedMedium; 4917 4918 /** 4919 * Volume number of journal or other collection in which the article is published. 4920 */ 4921 @Child(name = "volume", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4922 @Description(shortDefinition="Volume number of journal or other collection in which the article is published", formalDefinition="Volume number of journal or other collection in which the article is published." ) 4923 protected StringType volume; 4924 4925 /** 4926 * Issue, part or supplement of journal or other collection in which the article is published. 4927 */ 4928 @Child(name = "issue", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4929 @Description(shortDefinition="Issue, part or supplement of journal or other collection in which the article is published", formalDefinition="Issue, part or supplement of journal or other collection in which the article is published." ) 4930 protected StringType issue; 4931 4932 /** 4933 * The date the article was added to the database, or the date the article was released. 4934 */ 4935 @Child(name = "articleDate", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 4936 @Description(shortDefinition="The date the article was added to the database, or the date the article was released", formalDefinition="The date the article was added to the database, or the date the article was released." ) 4937 protected DateTimeType articleDate; 4938 4939 /** 4940 * Text representation of the date on which the issue of the cited artifact was published. 4941 */ 4942 @Child(name = "publicationDateText", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 4943 @Description(shortDefinition="Text representation of the date on which the issue of the cited artifact was published", formalDefinition="Text representation of the date on which the issue of the cited artifact was published." ) 4944 protected StringType publicationDateText; 4945 4946 /** 4947 * Spring, Summer, Fall/Autumn, Winter. 4948 */ 4949 @Child(name = "publicationDateSeason", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 4950 @Description(shortDefinition="Season in which the cited artifact was published", formalDefinition="Spring, Summer, Fall/Autumn, Winter." ) 4951 protected StringType publicationDateSeason; 4952 4953 /** 4954 * The date the article was last revised or updated in the database. 4955 */ 4956 @Child(name = "lastRevisionDate", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 4957 @Description(shortDefinition="The date the article was last revised or updated in the database", formalDefinition="The date the article was last revised or updated in the database." ) 4958 protected DateTimeType lastRevisionDate; 4959 4960 /** 4961 * The language or languages in which this form of the article is published. 4962 */ 4963 @Child(name = "language", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4964 @Description(shortDefinition="Language(s) in which this form of the article is published", formalDefinition="The language or languages in which this form of the article is published." ) 4965 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 4966 protected List<CodeableConcept> language; 4967 4968 /** 4969 * Entry number or identifier for inclusion in a database. 4970 */ 4971 @Child(name = "accessionNumber", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 4972 @Description(shortDefinition="Entry number or identifier for inclusion in a database", formalDefinition="Entry number or identifier for inclusion in a database." ) 4973 protected StringType accessionNumber; 4974 4975 /** 4976 * Used for full display of pagination. 4977 */ 4978 @Child(name = "pageString", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 4979 @Description(shortDefinition="Used for full display of pagination", formalDefinition="Used for full display of pagination." ) 4980 protected StringType pageString; 4981 4982 /** 4983 * Used for isolated representation of first page. 4984 */ 4985 @Child(name = "firstPage", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 4986 @Description(shortDefinition="Used for isolated representation of first page", formalDefinition="Used for isolated representation of first page." ) 4987 protected StringType firstPage; 4988 4989 /** 4990 * Used for isolated representation of last page. 4991 */ 4992 @Child(name = "lastPage", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 4993 @Description(shortDefinition="Used for isolated representation of last page", formalDefinition="Used for isolated representation of last page." ) 4994 protected StringType lastPage; 4995 4996 /** 4997 * Actual or approximate number of pages or screens. Distinct from reporting the page numbers. 4998 */ 4999 @Child(name = "pageCount", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 5000 @Description(shortDefinition="Number of pages or screens", formalDefinition="Actual or approximate number of pages or screens. Distinct from reporting the page numbers." ) 5001 protected StringType pageCount; 5002 5003 /** 5004 * Copyright notice for the full article or artifact. 5005 */ 5006 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 5007 @Description(shortDefinition="Copyright notice for the full article or artifact", formalDefinition="Copyright notice for the full article or artifact." ) 5008 protected MarkdownType copyright; 5009 5010 private static final long serialVersionUID = 1791622597L; 5011 5012 /** 5013 * Constructor 5014 */ 5015 public CitationCitedArtifactPublicationFormComponent() { 5016 super(); 5017 } 5018 5019 /** 5020 * @return {@link #publishedIn} (The collection the cited article or artifact is published in.) 5021 */ 5022 public CitationCitedArtifactPublicationFormPublishedInComponent getPublishedIn() { 5023 if (this.publishedIn == null) 5024 if (Configuration.errorOnAutoCreate()) 5025 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.publishedIn"); 5026 else if (Configuration.doAutoCreate()) 5027 this.publishedIn = new CitationCitedArtifactPublicationFormPublishedInComponent(); // cc 5028 return this.publishedIn; 5029 } 5030 5031 public boolean hasPublishedIn() { 5032 return this.publishedIn != null && !this.publishedIn.isEmpty(); 5033 } 5034 5035 /** 5036 * @param value {@link #publishedIn} (The collection the cited article or artifact is published in.) 5037 */ 5038 public CitationCitedArtifactPublicationFormComponent setPublishedIn(CitationCitedArtifactPublicationFormPublishedInComponent value) { 5039 this.publishedIn = value; 5040 return this; 5041 } 5042 5043 /** 5044 * @return {@link #citedMedium} (Describes the form of the medium cited. Common codes are "Internet" or "Print". The CitedMedium value set has 6 codes. The codes internet, print, and offline-digital-storage are the common codes for a typical publication form, though internet and print are more common for study citations. Three additional codes (each appending one of the primary codes with "-without-issue" are used for situations when a study is published both within an issue (of a periodical release as commonly done for journals) AND is published separately from the issue (as commonly done with early online publication), to represent specific identification of the publication form not associated with the issue.) 5045 */ 5046 public CodeableConcept getCitedMedium() { 5047 if (this.citedMedium == null) 5048 if (Configuration.errorOnAutoCreate()) 5049 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.citedMedium"); 5050 else if (Configuration.doAutoCreate()) 5051 this.citedMedium = new CodeableConcept(); // cc 5052 return this.citedMedium; 5053 } 5054 5055 public boolean hasCitedMedium() { 5056 return this.citedMedium != null && !this.citedMedium.isEmpty(); 5057 } 5058 5059 /** 5060 * @param value {@link #citedMedium} (Describes the form of the medium cited. Common codes are "Internet" or "Print". The CitedMedium value set has 6 codes. The codes internet, print, and offline-digital-storage are the common codes for a typical publication form, though internet and print are more common for study citations. Three additional codes (each appending one of the primary codes with "-without-issue" are used for situations when a study is published both within an issue (of a periodical release as commonly done for journals) AND is published separately from the issue (as commonly done with early online publication), to represent specific identification of the publication form not associated with the issue.) 5061 */ 5062 public CitationCitedArtifactPublicationFormComponent setCitedMedium(CodeableConcept value) { 5063 this.citedMedium = value; 5064 return this; 5065 } 5066 5067 /** 5068 * @return {@link #volume} (Volume number of journal or other collection in which the article is published.). This is the underlying object with id, value and extensions. The accessor "getVolume" gives direct access to the value 5069 */ 5070 public StringType getVolumeElement() { 5071 if (this.volume == null) 5072 if (Configuration.errorOnAutoCreate()) 5073 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.volume"); 5074 else if (Configuration.doAutoCreate()) 5075 this.volume = new StringType(); // bb 5076 return this.volume; 5077 } 5078 5079 public boolean hasVolumeElement() { 5080 return this.volume != null && !this.volume.isEmpty(); 5081 } 5082 5083 public boolean hasVolume() { 5084 return this.volume != null && !this.volume.isEmpty(); 5085 } 5086 5087 /** 5088 * @param value {@link #volume} (Volume number of journal or other collection in which the article is published.). This is the underlying object with id, value and extensions. The accessor "getVolume" gives direct access to the value 5089 */ 5090 public CitationCitedArtifactPublicationFormComponent setVolumeElement(StringType value) { 5091 this.volume = value; 5092 return this; 5093 } 5094 5095 /** 5096 * @return Volume number of journal or other collection in which the article is published. 5097 */ 5098 public String getVolume() { 5099 return this.volume == null ? null : this.volume.getValue(); 5100 } 5101 5102 /** 5103 * @param value Volume number of journal or other collection in which the article is published. 5104 */ 5105 public CitationCitedArtifactPublicationFormComponent setVolume(String value) { 5106 if (Utilities.noString(value)) 5107 this.volume = null; 5108 else { 5109 if (this.volume == null) 5110 this.volume = new StringType(); 5111 this.volume.setValue(value); 5112 } 5113 return this; 5114 } 5115 5116 /** 5117 * @return {@link #issue} (Issue, part or supplement of journal or other collection in which the article is published.). This is the underlying object with id, value and extensions. The accessor "getIssue" gives direct access to the value 5118 */ 5119 public StringType getIssueElement() { 5120 if (this.issue == null) 5121 if (Configuration.errorOnAutoCreate()) 5122 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.issue"); 5123 else if (Configuration.doAutoCreate()) 5124 this.issue = new StringType(); // bb 5125 return this.issue; 5126 } 5127 5128 public boolean hasIssueElement() { 5129 return this.issue != null && !this.issue.isEmpty(); 5130 } 5131 5132 public boolean hasIssue() { 5133 return this.issue != null && !this.issue.isEmpty(); 5134 } 5135 5136 /** 5137 * @param value {@link #issue} (Issue, part or supplement of journal or other collection in which the article is published.). This is the underlying object with id, value and extensions. The accessor "getIssue" gives direct access to the value 5138 */ 5139 public CitationCitedArtifactPublicationFormComponent setIssueElement(StringType value) { 5140 this.issue = value; 5141 return this; 5142 } 5143 5144 /** 5145 * @return Issue, part or supplement of journal or other collection in which the article is published. 5146 */ 5147 public String getIssue() { 5148 return this.issue == null ? null : this.issue.getValue(); 5149 } 5150 5151 /** 5152 * @param value Issue, part or supplement of journal or other collection in which the article is published. 5153 */ 5154 public CitationCitedArtifactPublicationFormComponent setIssue(String value) { 5155 if (Utilities.noString(value)) 5156 this.issue = null; 5157 else { 5158 if (this.issue == null) 5159 this.issue = new StringType(); 5160 this.issue.setValue(value); 5161 } 5162 return this; 5163 } 5164 5165 /** 5166 * @return {@link #articleDate} (The date the article was added to the database, or the date the article was released.). This is the underlying object with id, value and extensions. The accessor "getArticleDate" gives direct access to the value 5167 */ 5168 public DateTimeType getArticleDateElement() { 5169 if (this.articleDate == null) 5170 if (Configuration.errorOnAutoCreate()) 5171 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.articleDate"); 5172 else if (Configuration.doAutoCreate()) 5173 this.articleDate = new DateTimeType(); // bb 5174 return this.articleDate; 5175 } 5176 5177 public boolean hasArticleDateElement() { 5178 return this.articleDate != null && !this.articleDate.isEmpty(); 5179 } 5180 5181 public boolean hasArticleDate() { 5182 return this.articleDate != null && !this.articleDate.isEmpty(); 5183 } 5184 5185 /** 5186 * @param value {@link #articleDate} (The date the article was added to the database, or the date the article was released.). This is the underlying object with id, value and extensions. The accessor "getArticleDate" gives direct access to the value 5187 */ 5188 public CitationCitedArtifactPublicationFormComponent setArticleDateElement(DateTimeType value) { 5189 this.articleDate = value; 5190 return this; 5191 } 5192 5193 /** 5194 * @return The date the article was added to the database, or the date the article was released. 5195 */ 5196 public Date getArticleDate() { 5197 return this.articleDate == null ? null : this.articleDate.getValue(); 5198 } 5199 5200 /** 5201 * @param value The date the article was added to the database, or the date the article was released. 5202 */ 5203 public CitationCitedArtifactPublicationFormComponent setArticleDate(Date value) { 5204 if (value == null) 5205 this.articleDate = null; 5206 else { 5207 if (this.articleDate == null) 5208 this.articleDate = new DateTimeType(); 5209 this.articleDate.setValue(value); 5210 } 5211 return this; 5212 } 5213 5214 /** 5215 * @return {@link #publicationDateText} (Text representation of the date on which the issue of the cited artifact was published.). This is the underlying object with id, value and extensions. The accessor "getPublicationDateText" gives direct access to the value 5216 */ 5217 public StringType getPublicationDateTextElement() { 5218 if (this.publicationDateText == null) 5219 if (Configuration.errorOnAutoCreate()) 5220 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.publicationDateText"); 5221 else if (Configuration.doAutoCreate()) 5222 this.publicationDateText = new StringType(); // bb 5223 return this.publicationDateText; 5224 } 5225 5226 public boolean hasPublicationDateTextElement() { 5227 return this.publicationDateText != null && !this.publicationDateText.isEmpty(); 5228 } 5229 5230 public boolean hasPublicationDateText() { 5231 return this.publicationDateText != null && !this.publicationDateText.isEmpty(); 5232 } 5233 5234 /** 5235 * @param value {@link #publicationDateText} (Text representation of the date on which the issue of the cited artifact was published.). This is the underlying object with id, value and extensions. The accessor "getPublicationDateText" gives direct access to the value 5236 */ 5237 public CitationCitedArtifactPublicationFormComponent setPublicationDateTextElement(StringType value) { 5238 this.publicationDateText = value; 5239 return this; 5240 } 5241 5242 /** 5243 * @return Text representation of the date on which the issue of the cited artifact was published. 5244 */ 5245 public String getPublicationDateText() { 5246 return this.publicationDateText == null ? null : this.publicationDateText.getValue(); 5247 } 5248 5249 /** 5250 * @param value Text representation of the date on which the issue of the cited artifact was published. 5251 */ 5252 public CitationCitedArtifactPublicationFormComponent setPublicationDateText(String value) { 5253 if (Utilities.noString(value)) 5254 this.publicationDateText = null; 5255 else { 5256 if (this.publicationDateText == null) 5257 this.publicationDateText = new StringType(); 5258 this.publicationDateText.setValue(value); 5259 } 5260 return this; 5261 } 5262 5263 /** 5264 * @return {@link #publicationDateSeason} (Spring, Summer, Fall/Autumn, Winter.). This is the underlying object with id, value and extensions. The accessor "getPublicationDateSeason" gives direct access to the value 5265 */ 5266 public StringType getPublicationDateSeasonElement() { 5267 if (this.publicationDateSeason == null) 5268 if (Configuration.errorOnAutoCreate()) 5269 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.publicationDateSeason"); 5270 else if (Configuration.doAutoCreate()) 5271 this.publicationDateSeason = new StringType(); // bb 5272 return this.publicationDateSeason; 5273 } 5274 5275 public boolean hasPublicationDateSeasonElement() { 5276 return this.publicationDateSeason != null && !this.publicationDateSeason.isEmpty(); 5277 } 5278 5279 public boolean hasPublicationDateSeason() { 5280 return this.publicationDateSeason != null && !this.publicationDateSeason.isEmpty(); 5281 } 5282 5283 /** 5284 * @param value {@link #publicationDateSeason} (Spring, Summer, Fall/Autumn, Winter.). This is the underlying object with id, value and extensions. The accessor "getPublicationDateSeason" gives direct access to the value 5285 */ 5286 public CitationCitedArtifactPublicationFormComponent setPublicationDateSeasonElement(StringType value) { 5287 this.publicationDateSeason = value; 5288 return this; 5289 } 5290 5291 /** 5292 * @return Spring, Summer, Fall/Autumn, Winter. 5293 */ 5294 public String getPublicationDateSeason() { 5295 return this.publicationDateSeason == null ? null : this.publicationDateSeason.getValue(); 5296 } 5297 5298 /** 5299 * @param value Spring, Summer, Fall/Autumn, Winter. 5300 */ 5301 public CitationCitedArtifactPublicationFormComponent setPublicationDateSeason(String value) { 5302 if (Utilities.noString(value)) 5303 this.publicationDateSeason = null; 5304 else { 5305 if (this.publicationDateSeason == null) 5306 this.publicationDateSeason = new StringType(); 5307 this.publicationDateSeason.setValue(value); 5308 } 5309 return this; 5310 } 5311 5312 /** 5313 * @return {@link #lastRevisionDate} (The date the article was last revised or updated in the database.). This is the underlying object with id, value and extensions. The accessor "getLastRevisionDate" gives direct access to the value 5314 */ 5315 public DateTimeType getLastRevisionDateElement() { 5316 if (this.lastRevisionDate == null) 5317 if (Configuration.errorOnAutoCreate()) 5318 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.lastRevisionDate"); 5319 else if (Configuration.doAutoCreate()) 5320 this.lastRevisionDate = new DateTimeType(); // bb 5321 return this.lastRevisionDate; 5322 } 5323 5324 public boolean hasLastRevisionDateElement() { 5325 return this.lastRevisionDate != null && !this.lastRevisionDate.isEmpty(); 5326 } 5327 5328 public boolean hasLastRevisionDate() { 5329 return this.lastRevisionDate != null && !this.lastRevisionDate.isEmpty(); 5330 } 5331 5332 /** 5333 * @param value {@link #lastRevisionDate} (The date the article was last revised or updated in the database.). This is the underlying object with id, value and extensions. The accessor "getLastRevisionDate" gives direct access to the value 5334 */ 5335 public CitationCitedArtifactPublicationFormComponent setLastRevisionDateElement(DateTimeType value) { 5336 this.lastRevisionDate = value; 5337 return this; 5338 } 5339 5340 /** 5341 * @return The date the article was last revised or updated in the database. 5342 */ 5343 public Date getLastRevisionDate() { 5344 return this.lastRevisionDate == null ? null : this.lastRevisionDate.getValue(); 5345 } 5346 5347 /** 5348 * @param value The date the article was last revised or updated in the database. 5349 */ 5350 public CitationCitedArtifactPublicationFormComponent setLastRevisionDate(Date value) { 5351 if (value == null) 5352 this.lastRevisionDate = null; 5353 else { 5354 if (this.lastRevisionDate == null) 5355 this.lastRevisionDate = new DateTimeType(); 5356 this.lastRevisionDate.setValue(value); 5357 } 5358 return this; 5359 } 5360 5361 /** 5362 * @return {@link #language} (The language or languages in which this form of the article is published.) 5363 */ 5364 public List<CodeableConcept> getLanguage() { 5365 if (this.language == null) 5366 this.language = new ArrayList<CodeableConcept>(); 5367 return this.language; 5368 } 5369 5370 /** 5371 * @return Returns a reference to <code>this</code> for easy method chaining 5372 */ 5373 public CitationCitedArtifactPublicationFormComponent setLanguage(List<CodeableConcept> theLanguage) { 5374 this.language = theLanguage; 5375 return this; 5376 } 5377 5378 public boolean hasLanguage() { 5379 if (this.language == null) 5380 return false; 5381 for (CodeableConcept item : this.language) 5382 if (!item.isEmpty()) 5383 return true; 5384 return false; 5385 } 5386 5387 public CodeableConcept addLanguage() { //3 5388 CodeableConcept t = new CodeableConcept(); 5389 if (this.language == null) 5390 this.language = new ArrayList<CodeableConcept>(); 5391 this.language.add(t); 5392 return t; 5393 } 5394 5395 public CitationCitedArtifactPublicationFormComponent addLanguage(CodeableConcept t) { //3 5396 if (t == null) 5397 return this; 5398 if (this.language == null) 5399 this.language = new ArrayList<CodeableConcept>(); 5400 this.language.add(t); 5401 return this; 5402 } 5403 5404 /** 5405 * @return The first repetition of repeating field {@link #language}, creating it if it does not already exist {3} 5406 */ 5407 public CodeableConcept getLanguageFirstRep() { 5408 if (getLanguage().isEmpty()) { 5409 addLanguage(); 5410 } 5411 return getLanguage().get(0); 5412 } 5413 5414 /** 5415 * @return {@link #accessionNumber} (Entry number or identifier for inclusion in a database.). This is the underlying object with id, value and extensions. The accessor "getAccessionNumber" gives direct access to the value 5416 */ 5417 public StringType getAccessionNumberElement() { 5418 if (this.accessionNumber == null) 5419 if (Configuration.errorOnAutoCreate()) 5420 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.accessionNumber"); 5421 else if (Configuration.doAutoCreate()) 5422 this.accessionNumber = new StringType(); // bb 5423 return this.accessionNumber; 5424 } 5425 5426 public boolean hasAccessionNumberElement() { 5427 return this.accessionNumber != null && !this.accessionNumber.isEmpty(); 5428 } 5429 5430 public boolean hasAccessionNumber() { 5431 return this.accessionNumber != null && !this.accessionNumber.isEmpty(); 5432 } 5433 5434 /** 5435 * @param value {@link #accessionNumber} (Entry number or identifier for inclusion in a database.). This is the underlying object with id, value and extensions. The accessor "getAccessionNumber" gives direct access to the value 5436 */ 5437 public CitationCitedArtifactPublicationFormComponent setAccessionNumberElement(StringType value) { 5438 this.accessionNumber = value; 5439 return this; 5440 } 5441 5442 /** 5443 * @return Entry number or identifier for inclusion in a database. 5444 */ 5445 public String getAccessionNumber() { 5446 return this.accessionNumber == null ? null : this.accessionNumber.getValue(); 5447 } 5448 5449 /** 5450 * @param value Entry number or identifier for inclusion in a database. 5451 */ 5452 public CitationCitedArtifactPublicationFormComponent setAccessionNumber(String value) { 5453 if (Utilities.noString(value)) 5454 this.accessionNumber = null; 5455 else { 5456 if (this.accessionNumber == null) 5457 this.accessionNumber = new StringType(); 5458 this.accessionNumber.setValue(value); 5459 } 5460 return this; 5461 } 5462 5463 /** 5464 * @return {@link #pageString} (Used for full display of pagination.). This is the underlying object with id, value and extensions. The accessor "getPageString" gives direct access to the value 5465 */ 5466 public StringType getPageStringElement() { 5467 if (this.pageString == null) 5468 if (Configuration.errorOnAutoCreate()) 5469 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.pageString"); 5470 else if (Configuration.doAutoCreate()) 5471 this.pageString = new StringType(); // bb 5472 return this.pageString; 5473 } 5474 5475 public boolean hasPageStringElement() { 5476 return this.pageString != null && !this.pageString.isEmpty(); 5477 } 5478 5479 public boolean hasPageString() { 5480 return this.pageString != null && !this.pageString.isEmpty(); 5481 } 5482 5483 /** 5484 * @param value {@link #pageString} (Used for full display of pagination.). This is the underlying object with id, value and extensions. The accessor "getPageString" gives direct access to the value 5485 */ 5486 public CitationCitedArtifactPublicationFormComponent setPageStringElement(StringType value) { 5487 this.pageString = value; 5488 return this; 5489 } 5490 5491 /** 5492 * @return Used for full display of pagination. 5493 */ 5494 public String getPageString() { 5495 return this.pageString == null ? null : this.pageString.getValue(); 5496 } 5497 5498 /** 5499 * @param value Used for full display of pagination. 5500 */ 5501 public CitationCitedArtifactPublicationFormComponent setPageString(String value) { 5502 if (Utilities.noString(value)) 5503 this.pageString = null; 5504 else { 5505 if (this.pageString == null) 5506 this.pageString = new StringType(); 5507 this.pageString.setValue(value); 5508 } 5509 return this; 5510 } 5511 5512 /** 5513 * @return {@link #firstPage} (Used for isolated representation of first page.). This is the underlying object with id, value and extensions. The accessor "getFirstPage" gives direct access to the value 5514 */ 5515 public StringType getFirstPageElement() { 5516 if (this.firstPage == null) 5517 if (Configuration.errorOnAutoCreate()) 5518 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.firstPage"); 5519 else if (Configuration.doAutoCreate()) 5520 this.firstPage = new StringType(); // bb 5521 return this.firstPage; 5522 } 5523 5524 public boolean hasFirstPageElement() { 5525 return this.firstPage != null && !this.firstPage.isEmpty(); 5526 } 5527 5528 public boolean hasFirstPage() { 5529 return this.firstPage != null && !this.firstPage.isEmpty(); 5530 } 5531 5532 /** 5533 * @param value {@link #firstPage} (Used for isolated representation of first page.). This is the underlying object with id, value and extensions. The accessor "getFirstPage" gives direct access to the value 5534 */ 5535 public CitationCitedArtifactPublicationFormComponent setFirstPageElement(StringType value) { 5536 this.firstPage = value; 5537 return this; 5538 } 5539 5540 /** 5541 * @return Used for isolated representation of first page. 5542 */ 5543 public String getFirstPage() { 5544 return this.firstPage == null ? null : this.firstPage.getValue(); 5545 } 5546 5547 /** 5548 * @param value Used for isolated representation of first page. 5549 */ 5550 public CitationCitedArtifactPublicationFormComponent setFirstPage(String value) { 5551 if (Utilities.noString(value)) 5552 this.firstPage = null; 5553 else { 5554 if (this.firstPage == null) 5555 this.firstPage = new StringType(); 5556 this.firstPage.setValue(value); 5557 } 5558 return this; 5559 } 5560 5561 /** 5562 * @return {@link #lastPage} (Used for isolated representation of last page.). This is the underlying object with id, value and extensions. The accessor "getLastPage" gives direct access to the value 5563 */ 5564 public StringType getLastPageElement() { 5565 if (this.lastPage == null) 5566 if (Configuration.errorOnAutoCreate()) 5567 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.lastPage"); 5568 else if (Configuration.doAutoCreate()) 5569 this.lastPage = new StringType(); // bb 5570 return this.lastPage; 5571 } 5572 5573 public boolean hasLastPageElement() { 5574 return this.lastPage != null && !this.lastPage.isEmpty(); 5575 } 5576 5577 public boolean hasLastPage() { 5578 return this.lastPage != null && !this.lastPage.isEmpty(); 5579 } 5580 5581 /** 5582 * @param value {@link #lastPage} (Used for isolated representation of last page.). This is the underlying object with id, value and extensions. The accessor "getLastPage" gives direct access to the value 5583 */ 5584 public CitationCitedArtifactPublicationFormComponent setLastPageElement(StringType value) { 5585 this.lastPage = value; 5586 return this; 5587 } 5588 5589 /** 5590 * @return Used for isolated representation of last page. 5591 */ 5592 public String getLastPage() { 5593 return this.lastPage == null ? null : this.lastPage.getValue(); 5594 } 5595 5596 /** 5597 * @param value Used for isolated representation of last page. 5598 */ 5599 public CitationCitedArtifactPublicationFormComponent setLastPage(String value) { 5600 if (Utilities.noString(value)) 5601 this.lastPage = null; 5602 else { 5603 if (this.lastPage == null) 5604 this.lastPage = new StringType(); 5605 this.lastPage.setValue(value); 5606 } 5607 return this; 5608 } 5609 5610 /** 5611 * @return {@link #pageCount} (Actual or approximate number of pages or screens. Distinct from reporting the page numbers.). This is the underlying object with id, value and extensions. The accessor "getPageCount" gives direct access to the value 5612 */ 5613 public StringType getPageCountElement() { 5614 if (this.pageCount == null) 5615 if (Configuration.errorOnAutoCreate()) 5616 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.pageCount"); 5617 else if (Configuration.doAutoCreate()) 5618 this.pageCount = new StringType(); // bb 5619 return this.pageCount; 5620 } 5621 5622 public boolean hasPageCountElement() { 5623 return this.pageCount != null && !this.pageCount.isEmpty(); 5624 } 5625 5626 public boolean hasPageCount() { 5627 return this.pageCount != null && !this.pageCount.isEmpty(); 5628 } 5629 5630 /** 5631 * @param value {@link #pageCount} (Actual or approximate number of pages or screens. Distinct from reporting the page numbers.). This is the underlying object with id, value and extensions. The accessor "getPageCount" gives direct access to the value 5632 */ 5633 public CitationCitedArtifactPublicationFormComponent setPageCountElement(StringType value) { 5634 this.pageCount = value; 5635 return this; 5636 } 5637 5638 /** 5639 * @return Actual or approximate number of pages or screens. Distinct from reporting the page numbers. 5640 */ 5641 public String getPageCount() { 5642 return this.pageCount == null ? null : this.pageCount.getValue(); 5643 } 5644 5645 /** 5646 * @param value Actual or approximate number of pages or screens. Distinct from reporting the page numbers. 5647 */ 5648 public CitationCitedArtifactPublicationFormComponent setPageCount(String value) { 5649 if (Utilities.noString(value)) 5650 this.pageCount = null; 5651 else { 5652 if (this.pageCount == null) 5653 this.pageCount = new StringType(); 5654 this.pageCount.setValue(value); 5655 } 5656 return this; 5657 } 5658 5659 /** 5660 * @return {@link #copyright} (Copyright notice for the full article or artifact.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5661 */ 5662 public MarkdownType getCopyrightElement() { 5663 if (this.copyright == null) 5664 if (Configuration.errorOnAutoCreate()) 5665 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormComponent.copyright"); 5666 else if (Configuration.doAutoCreate()) 5667 this.copyright = new MarkdownType(); // bb 5668 return this.copyright; 5669 } 5670 5671 public boolean hasCopyrightElement() { 5672 return this.copyright != null && !this.copyright.isEmpty(); 5673 } 5674 5675 public boolean hasCopyright() { 5676 return this.copyright != null && !this.copyright.isEmpty(); 5677 } 5678 5679 /** 5680 * @param value {@link #copyright} (Copyright notice for the full article or artifact.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5681 */ 5682 public CitationCitedArtifactPublicationFormComponent setCopyrightElement(MarkdownType value) { 5683 this.copyright = value; 5684 return this; 5685 } 5686 5687 /** 5688 * @return Copyright notice for the full article or artifact. 5689 */ 5690 public String getCopyright() { 5691 return this.copyright == null ? null : this.copyright.getValue(); 5692 } 5693 5694 /** 5695 * @param value Copyright notice for the full article or artifact. 5696 */ 5697 public CitationCitedArtifactPublicationFormComponent setCopyright(String value) { 5698 if (Utilities.noString(value)) 5699 this.copyright = null; 5700 else { 5701 if (this.copyright == null) 5702 this.copyright = new MarkdownType(); 5703 this.copyright.setValue(value); 5704 } 5705 return this; 5706 } 5707 5708 protected void listChildren(List<Property> children) { 5709 super.listChildren(children); 5710 children.add(new Property("publishedIn", "", "The collection the cited article or artifact is published in.", 0, 1, publishedIn)); 5711 children.add(new Property("citedMedium", "CodeableConcept", "Describes the form of the medium cited. Common codes are \"Internet\" or \"Print\". The CitedMedium value set has 6 codes. The codes internet, print, and offline-digital-storage are the common codes for a typical publication form, though internet and print are more common for study citations. Three additional codes (each appending one of the primary codes with \"-without-issue\" are used for situations when a study is published both within an issue (of a periodical release as commonly done for journals) AND is published separately from the issue (as commonly done with early online publication), to represent specific identification of the publication form not associated with the issue.", 0, 1, citedMedium)); 5712 children.add(new Property("volume", "string", "Volume number of journal or other collection in which the article is published.", 0, 1, volume)); 5713 children.add(new Property("issue", "string", "Issue, part or supplement of journal or other collection in which the article is published.", 0, 1, issue)); 5714 children.add(new Property("articleDate", "dateTime", "The date the article was added to the database, or the date the article was released.", 0, 1, articleDate)); 5715 children.add(new Property("publicationDateText", "string", "Text representation of the date on which the issue of the cited artifact was published.", 0, 1, publicationDateText)); 5716 children.add(new Property("publicationDateSeason", "string", "Spring, Summer, Fall/Autumn, Winter.", 0, 1, publicationDateSeason)); 5717 children.add(new Property("lastRevisionDate", "dateTime", "The date the article was last revised or updated in the database.", 0, 1, lastRevisionDate)); 5718 children.add(new Property("language", "CodeableConcept", "The language or languages in which this form of the article is published.", 0, java.lang.Integer.MAX_VALUE, language)); 5719 children.add(new Property("accessionNumber", "string", "Entry number or identifier for inclusion in a database.", 0, 1, accessionNumber)); 5720 children.add(new Property("pageString", "string", "Used for full display of pagination.", 0, 1, pageString)); 5721 children.add(new Property("firstPage", "string", "Used for isolated representation of first page.", 0, 1, firstPage)); 5722 children.add(new Property("lastPage", "string", "Used for isolated representation of last page.", 0, 1, lastPage)); 5723 children.add(new Property("pageCount", "string", "Actual or approximate number of pages or screens. Distinct from reporting the page numbers.", 0, 1, pageCount)); 5724 children.add(new Property("copyright", "markdown", "Copyright notice for the full article or artifact.", 0, 1, copyright)); 5725 } 5726 5727 @Override 5728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5729 switch (_hash) { 5730 case -614144077: /*publishedIn*/ return new Property("publishedIn", "", "The collection the cited article or artifact is published in.", 0, 1, publishedIn); 5731 case 612116418: /*citedMedium*/ return new Property("citedMedium", "CodeableConcept", "Describes the form of the medium cited. Common codes are \"Internet\" or \"Print\". The CitedMedium value set has 6 codes. The codes internet, print, and offline-digital-storage are the common codes for a typical publication form, though internet and print are more common for study citations. Three additional codes (each appending one of the primary codes with \"-without-issue\" are used for situations when a study is published both within an issue (of a periodical release as commonly done for journals) AND is published separately from the issue (as commonly done with early online publication), to represent specific identification of the publication form not associated with the issue.", 0, 1, citedMedium); 5732 case -810883302: /*volume*/ return new Property("volume", "string", "Volume number of journal or other collection in which the article is published.", 0, 1, volume); 5733 case 100509913: /*issue*/ return new Property("issue", "string", "Issue, part or supplement of journal or other collection in which the article is published.", 0, 1, issue); 5734 case 817743300: /*articleDate*/ return new Property("articleDate", "dateTime", "The date the article was added to the database, or the date the article was released.", 0, 1, articleDate); 5735 case 225590343: /*publicationDateText*/ return new Property("publicationDateText", "string", "Text representation of the date on which the issue of the cited artifact was published.", 0, 1, publicationDateText); 5736 case 2014643069: /*publicationDateSeason*/ return new Property("publicationDateSeason", "string", "Spring, Summer, Fall/Autumn, Winter.", 0, 1, publicationDateSeason); 5737 case 2129161183: /*lastRevisionDate*/ return new Property("lastRevisionDate", "dateTime", "The date the article was last revised or updated in the database.", 0, 1, lastRevisionDate); 5738 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The language or languages in which this form of the article is published.", 0, java.lang.Integer.MAX_VALUE, language); 5739 case 1807963277: /*accessionNumber*/ return new Property("accessionNumber", "string", "Entry number or identifier for inclusion in a database.", 0, 1, accessionNumber); 5740 case 1287145344: /*pageString*/ return new Property("pageString", "string", "Used for full display of pagination.", 0, 1, pageString); 5741 case 132895071: /*firstPage*/ return new Property("firstPage", "string", "Used for isolated representation of first page.", 0, 1, firstPage); 5742 case -1459540411: /*lastPage*/ return new Property("lastPage", "string", "Used for isolated representation of last page.", 0, 1, lastPage); 5743 case 857882560: /*pageCount*/ return new Property("pageCount", "string", "Actual or approximate number of pages or screens. Distinct from reporting the page numbers.", 0, 1, pageCount); 5744 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "Copyright notice for the full article or artifact.", 0, 1, copyright); 5745 default: return super.getNamedProperty(_hash, _name, _checkValid); 5746 } 5747 5748 } 5749 5750 @Override 5751 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5752 switch (hash) { 5753 case -614144077: /*publishedIn*/ return this.publishedIn == null ? new Base[0] : new Base[] {this.publishedIn}; // CitationCitedArtifactPublicationFormPublishedInComponent 5754 case 612116418: /*citedMedium*/ return this.citedMedium == null ? new Base[0] : new Base[] {this.citedMedium}; // CodeableConcept 5755 case -810883302: /*volume*/ return this.volume == null ? new Base[0] : new Base[] {this.volume}; // StringType 5756 case 100509913: /*issue*/ return this.issue == null ? new Base[0] : new Base[] {this.issue}; // StringType 5757 case 817743300: /*articleDate*/ return this.articleDate == null ? new Base[0] : new Base[] {this.articleDate}; // DateTimeType 5758 case 225590343: /*publicationDateText*/ return this.publicationDateText == null ? new Base[0] : new Base[] {this.publicationDateText}; // StringType 5759 case 2014643069: /*publicationDateSeason*/ return this.publicationDateSeason == null ? new Base[0] : new Base[] {this.publicationDateSeason}; // StringType 5760 case 2129161183: /*lastRevisionDate*/ return this.lastRevisionDate == null ? new Base[0] : new Base[] {this.lastRevisionDate}; // DateTimeType 5761 case -1613589672: /*language*/ return this.language == null ? new Base[0] : this.language.toArray(new Base[this.language.size()]); // CodeableConcept 5762 case 1807963277: /*accessionNumber*/ return this.accessionNumber == null ? new Base[0] : new Base[] {this.accessionNumber}; // StringType 5763 case 1287145344: /*pageString*/ return this.pageString == null ? new Base[0] : new Base[] {this.pageString}; // StringType 5764 case 132895071: /*firstPage*/ return this.firstPage == null ? new Base[0] : new Base[] {this.firstPage}; // StringType 5765 case -1459540411: /*lastPage*/ return this.lastPage == null ? new Base[0] : new Base[] {this.lastPage}; // StringType 5766 case 857882560: /*pageCount*/ return this.pageCount == null ? new Base[0] : new Base[] {this.pageCount}; // StringType 5767 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 5768 default: return super.getProperty(hash, name, checkValid); 5769 } 5770 5771 } 5772 5773 @Override 5774 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5775 switch (hash) { 5776 case -614144077: // publishedIn 5777 this.publishedIn = (CitationCitedArtifactPublicationFormPublishedInComponent) value; // CitationCitedArtifactPublicationFormPublishedInComponent 5778 return value; 5779 case 612116418: // citedMedium 5780 this.citedMedium = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5781 return value; 5782 case -810883302: // volume 5783 this.volume = TypeConvertor.castToString(value); // StringType 5784 return value; 5785 case 100509913: // issue 5786 this.issue = TypeConvertor.castToString(value); // StringType 5787 return value; 5788 case 817743300: // articleDate 5789 this.articleDate = TypeConvertor.castToDateTime(value); // DateTimeType 5790 return value; 5791 case 225590343: // publicationDateText 5792 this.publicationDateText = TypeConvertor.castToString(value); // StringType 5793 return value; 5794 case 2014643069: // publicationDateSeason 5795 this.publicationDateSeason = TypeConvertor.castToString(value); // StringType 5796 return value; 5797 case 2129161183: // lastRevisionDate 5798 this.lastRevisionDate = TypeConvertor.castToDateTime(value); // DateTimeType 5799 return value; 5800 case -1613589672: // language 5801 this.getLanguage().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5802 return value; 5803 case 1807963277: // accessionNumber 5804 this.accessionNumber = TypeConvertor.castToString(value); // StringType 5805 return value; 5806 case 1287145344: // pageString 5807 this.pageString = TypeConvertor.castToString(value); // StringType 5808 return value; 5809 case 132895071: // firstPage 5810 this.firstPage = TypeConvertor.castToString(value); // StringType 5811 return value; 5812 case -1459540411: // lastPage 5813 this.lastPage = TypeConvertor.castToString(value); // StringType 5814 return value; 5815 case 857882560: // pageCount 5816 this.pageCount = TypeConvertor.castToString(value); // StringType 5817 return value; 5818 case 1522889671: // copyright 5819 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5820 return value; 5821 default: return super.setProperty(hash, name, value); 5822 } 5823 5824 } 5825 5826 @Override 5827 public Base setProperty(String name, Base value) throws FHIRException { 5828 if (name.equals("publishedIn")) { 5829 this.publishedIn = (CitationCitedArtifactPublicationFormPublishedInComponent) value; // CitationCitedArtifactPublicationFormPublishedInComponent 5830 } else if (name.equals("citedMedium")) { 5831 this.citedMedium = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5832 } else if (name.equals("volume")) { 5833 this.volume = TypeConvertor.castToString(value); // StringType 5834 } else if (name.equals("issue")) { 5835 this.issue = TypeConvertor.castToString(value); // StringType 5836 } else if (name.equals("articleDate")) { 5837 this.articleDate = TypeConvertor.castToDateTime(value); // DateTimeType 5838 } else if (name.equals("publicationDateText")) { 5839 this.publicationDateText = TypeConvertor.castToString(value); // StringType 5840 } else if (name.equals("publicationDateSeason")) { 5841 this.publicationDateSeason = TypeConvertor.castToString(value); // StringType 5842 } else if (name.equals("lastRevisionDate")) { 5843 this.lastRevisionDate = TypeConvertor.castToDateTime(value); // DateTimeType 5844 } else if (name.equals("language")) { 5845 this.getLanguage().add(TypeConvertor.castToCodeableConcept(value)); 5846 } else if (name.equals("accessionNumber")) { 5847 this.accessionNumber = TypeConvertor.castToString(value); // StringType 5848 } else if (name.equals("pageString")) { 5849 this.pageString = TypeConvertor.castToString(value); // StringType 5850 } else if (name.equals("firstPage")) { 5851 this.firstPage = TypeConvertor.castToString(value); // StringType 5852 } else if (name.equals("lastPage")) { 5853 this.lastPage = TypeConvertor.castToString(value); // StringType 5854 } else if (name.equals("pageCount")) { 5855 this.pageCount = TypeConvertor.castToString(value); // StringType 5856 } else if (name.equals("copyright")) { 5857 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5858 } else 5859 return super.setProperty(name, value); 5860 return value; 5861 } 5862 5863 @Override 5864 public void removeChild(String name, Base value) throws FHIRException { 5865 if (name.equals("publishedIn")) { 5866 this.publishedIn = (CitationCitedArtifactPublicationFormPublishedInComponent) value; // CitationCitedArtifactPublicationFormPublishedInComponent 5867 } else if (name.equals("citedMedium")) { 5868 this.citedMedium = null; 5869 } else if (name.equals("volume")) { 5870 this.volume = null; 5871 } else if (name.equals("issue")) { 5872 this.issue = null; 5873 } else if (name.equals("articleDate")) { 5874 this.articleDate = null; 5875 } else if (name.equals("publicationDateText")) { 5876 this.publicationDateText = null; 5877 } else if (name.equals("publicationDateSeason")) { 5878 this.publicationDateSeason = null; 5879 } else if (name.equals("lastRevisionDate")) { 5880 this.lastRevisionDate = null; 5881 } else if (name.equals("language")) { 5882 this.getLanguage().remove(value); 5883 } else if (name.equals("accessionNumber")) { 5884 this.accessionNumber = null; 5885 } else if (name.equals("pageString")) { 5886 this.pageString = null; 5887 } else if (name.equals("firstPage")) { 5888 this.firstPage = null; 5889 } else if (name.equals("lastPage")) { 5890 this.lastPage = null; 5891 } else if (name.equals("pageCount")) { 5892 this.pageCount = null; 5893 } else if (name.equals("copyright")) { 5894 this.copyright = null; 5895 } else 5896 super.removeChild(name, value); 5897 5898 } 5899 5900 @Override 5901 public Base makeProperty(int hash, String name) throws FHIRException { 5902 switch (hash) { 5903 case -614144077: return getPublishedIn(); 5904 case 612116418: return getCitedMedium(); 5905 case -810883302: return getVolumeElement(); 5906 case 100509913: return getIssueElement(); 5907 case 817743300: return getArticleDateElement(); 5908 case 225590343: return getPublicationDateTextElement(); 5909 case 2014643069: return getPublicationDateSeasonElement(); 5910 case 2129161183: return getLastRevisionDateElement(); 5911 case -1613589672: return addLanguage(); 5912 case 1807963277: return getAccessionNumberElement(); 5913 case 1287145344: return getPageStringElement(); 5914 case 132895071: return getFirstPageElement(); 5915 case -1459540411: return getLastPageElement(); 5916 case 857882560: return getPageCountElement(); 5917 case 1522889671: return getCopyrightElement(); 5918 default: return super.makeProperty(hash, name); 5919 } 5920 5921 } 5922 5923 @Override 5924 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5925 switch (hash) { 5926 case -614144077: /*publishedIn*/ return new String[] {}; 5927 case 612116418: /*citedMedium*/ return new String[] {"CodeableConcept"}; 5928 case -810883302: /*volume*/ return new String[] {"string"}; 5929 case 100509913: /*issue*/ return new String[] {"string"}; 5930 case 817743300: /*articleDate*/ return new String[] {"dateTime"}; 5931 case 225590343: /*publicationDateText*/ return new String[] {"string"}; 5932 case 2014643069: /*publicationDateSeason*/ return new String[] {"string"}; 5933 case 2129161183: /*lastRevisionDate*/ return new String[] {"dateTime"}; 5934 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 5935 case 1807963277: /*accessionNumber*/ return new String[] {"string"}; 5936 case 1287145344: /*pageString*/ return new String[] {"string"}; 5937 case 132895071: /*firstPage*/ return new String[] {"string"}; 5938 case -1459540411: /*lastPage*/ return new String[] {"string"}; 5939 case 857882560: /*pageCount*/ return new String[] {"string"}; 5940 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5941 default: return super.getTypesForProperty(hash, name); 5942 } 5943 5944 } 5945 5946 @Override 5947 public Base addChild(String name) throws FHIRException { 5948 if (name.equals("publishedIn")) { 5949 this.publishedIn = new CitationCitedArtifactPublicationFormPublishedInComponent(); 5950 return this.publishedIn; 5951 } 5952 else if (name.equals("citedMedium")) { 5953 this.citedMedium = new CodeableConcept(); 5954 return this.citedMedium; 5955 } 5956 else if (name.equals("volume")) { 5957 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.volume"); 5958 } 5959 else if (name.equals("issue")) { 5960 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.issue"); 5961 } 5962 else if (name.equals("articleDate")) { 5963 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.articleDate"); 5964 } 5965 else if (name.equals("publicationDateText")) { 5966 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.publicationDateText"); 5967 } 5968 else if (name.equals("publicationDateSeason")) { 5969 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.publicationDateSeason"); 5970 } 5971 else if (name.equals("lastRevisionDate")) { 5972 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.lastRevisionDate"); 5973 } 5974 else if (name.equals("language")) { 5975 return addLanguage(); 5976 } 5977 else if (name.equals("accessionNumber")) { 5978 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.accessionNumber"); 5979 } 5980 else if (name.equals("pageString")) { 5981 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.pageString"); 5982 } 5983 else if (name.equals("firstPage")) { 5984 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.firstPage"); 5985 } 5986 else if (name.equals("lastPage")) { 5987 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.lastPage"); 5988 } 5989 else if (name.equals("pageCount")) { 5990 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.pageCount"); 5991 } 5992 else if (name.equals("copyright")) { 5993 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.copyright"); 5994 } 5995 else 5996 return super.addChild(name); 5997 } 5998 5999 public CitationCitedArtifactPublicationFormComponent copy() { 6000 CitationCitedArtifactPublicationFormComponent dst = new CitationCitedArtifactPublicationFormComponent(); 6001 copyValues(dst); 6002 return dst; 6003 } 6004 6005 public void copyValues(CitationCitedArtifactPublicationFormComponent dst) { 6006 super.copyValues(dst); 6007 dst.publishedIn = publishedIn == null ? null : publishedIn.copy(); 6008 dst.citedMedium = citedMedium == null ? null : citedMedium.copy(); 6009 dst.volume = volume == null ? null : volume.copy(); 6010 dst.issue = issue == null ? null : issue.copy(); 6011 dst.articleDate = articleDate == null ? null : articleDate.copy(); 6012 dst.publicationDateText = publicationDateText == null ? null : publicationDateText.copy(); 6013 dst.publicationDateSeason = publicationDateSeason == null ? null : publicationDateSeason.copy(); 6014 dst.lastRevisionDate = lastRevisionDate == null ? null : lastRevisionDate.copy(); 6015 if (language != null) { 6016 dst.language = new ArrayList<CodeableConcept>(); 6017 for (CodeableConcept i : language) 6018 dst.language.add(i.copy()); 6019 }; 6020 dst.accessionNumber = accessionNumber == null ? null : accessionNumber.copy(); 6021 dst.pageString = pageString == null ? null : pageString.copy(); 6022 dst.firstPage = firstPage == null ? null : firstPage.copy(); 6023 dst.lastPage = lastPage == null ? null : lastPage.copy(); 6024 dst.pageCount = pageCount == null ? null : pageCount.copy(); 6025 dst.copyright = copyright == null ? null : copyright.copy(); 6026 } 6027 6028 @Override 6029 public boolean equalsDeep(Base other_) { 6030 if (!super.equalsDeep(other_)) 6031 return false; 6032 if (!(other_ instanceof CitationCitedArtifactPublicationFormComponent)) 6033 return false; 6034 CitationCitedArtifactPublicationFormComponent o = (CitationCitedArtifactPublicationFormComponent) other_; 6035 return compareDeep(publishedIn, o.publishedIn, true) && compareDeep(citedMedium, o.citedMedium, true) 6036 && compareDeep(volume, o.volume, true) && compareDeep(issue, o.issue, true) && compareDeep(articleDate, o.articleDate, true) 6037 && compareDeep(publicationDateText, o.publicationDateText, true) && compareDeep(publicationDateSeason, o.publicationDateSeason, true) 6038 && compareDeep(lastRevisionDate, o.lastRevisionDate, true) && compareDeep(language, o.language, true) 6039 && compareDeep(accessionNumber, o.accessionNumber, true) && compareDeep(pageString, o.pageString, true) 6040 && compareDeep(firstPage, o.firstPage, true) && compareDeep(lastPage, o.lastPage, true) && compareDeep(pageCount, o.pageCount, true) 6041 && compareDeep(copyright, o.copyright, true); 6042 } 6043 6044 @Override 6045 public boolean equalsShallow(Base other_) { 6046 if (!super.equalsShallow(other_)) 6047 return false; 6048 if (!(other_ instanceof CitationCitedArtifactPublicationFormComponent)) 6049 return false; 6050 CitationCitedArtifactPublicationFormComponent o = (CitationCitedArtifactPublicationFormComponent) other_; 6051 return compareValues(volume, o.volume, true) && compareValues(issue, o.issue, true) && compareValues(articleDate, o.articleDate, true) 6052 && compareValues(publicationDateText, o.publicationDateText, true) && compareValues(publicationDateSeason, o.publicationDateSeason, true) 6053 && compareValues(lastRevisionDate, o.lastRevisionDate, true) && compareValues(accessionNumber, o.accessionNumber, true) 6054 && compareValues(pageString, o.pageString, true) && compareValues(firstPage, o.firstPage, true) && compareValues(lastPage, o.lastPage, true) 6055 && compareValues(pageCount, o.pageCount, true) && compareValues(copyright, o.copyright, true); 6056 } 6057 6058 public boolean isEmpty() { 6059 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(publishedIn, citedMedium, volume 6060 , issue, articleDate, publicationDateText, publicationDateSeason, lastRevisionDate 6061 , language, accessionNumber, pageString, firstPage, lastPage, pageCount, copyright 6062 ); 6063 } 6064 6065 public String fhirType() { 6066 return "Citation.citedArtifact.publicationForm"; 6067 6068 } 6069 6070 } 6071 6072 @Block() 6073 public static class CitationCitedArtifactPublicationFormPublishedInComponent extends BackboneElement implements IBaseBackboneElement { 6074 /** 6075 * Kind of container (e.g. Periodical, database, or book). 6076 */ 6077 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 6078 @Description(shortDefinition="Kind of container (e.g. Periodical, database, or book)", formalDefinition="Kind of container (e.g. Periodical, database, or book)." ) 6079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/published-in-type") 6080 protected CodeableConcept type; 6081 6082 /** 6083 * Journal identifiers include ISSN, ISO Abbreviation and NLMuniqueID; Book identifiers include ISBN. 6084 */ 6085 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6086 @Description(shortDefinition="Journal identifiers include ISSN, ISO Abbreviation and NLMuniqueID; Book identifiers include ISBN", formalDefinition="Journal identifiers include ISSN, ISO Abbreviation and NLMuniqueID; Book identifiers include ISBN." ) 6087 protected List<Identifier> identifier; 6088 6089 /** 6090 * Name of the database or title of the book or journal. 6091 */ 6092 @Child(name = "title", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 6093 @Description(shortDefinition="Name of the database or title of the book or journal", formalDefinition="Name of the database or title of the book or journal." ) 6094 protected StringType title; 6095 6096 /** 6097 * Name of or resource describing the publisher. 6098 */ 6099 @Child(name = "publisher", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 6100 @Description(shortDefinition="Name of or resource describing the publisher", formalDefinition="Name of or resource describing the publisher." ) 6101 protected Reference publisher; 6102 6103 /** 6104 * Geographic location of the publisher. 6105 */ 6106 @Child(name = "publisherLocation", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 6107 @Description(shortDefinition="Geographic location of the publisher", formalDefinition="Geographic location of the publisher." ) 6108 protected StringType publisherLocation; 6109 6110 private static final long serialVersionUID = 1440066953L; 6111 6112 /** 6113 * Constructor 6114 */ 6115 public CitationCitedArtifactPublicationFormPublishedInComponent() { 6116 super(); 6117 } 6118 6119 /** 6120 * @return {@link #type} (Kind of container (e.g. Periodical, database, or book).) 6121 */ 6122 public CodeableConcept getType() { 6123 if (this.type == null) 6124 if (Configuration.errorOnAutoCreate()) 6125 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormPublishedInComponent.type"); 6126 else if (Configuration.doAutoCreate()) 6127 this.type = new CodeableConcept(); // cc 6128 return this.type; 6129 } 6130 6131 public boolean hasType() { 6132 return this.type != null && !this.type.isEmpty(); 6133 } 6134 6135 /** 6136 * @param value {@link #type} (Kind of container (e.g. Periodical, database, or book).) 6137 */ 6138 public CitationCitedArtifactPublicationFormPublishedInComponent setType(CodeableConcept value) { 6139 this.type = value; 6140 return this; 6141 } 6142 6143 /** 6144 * @return {@link #identifier} (Journal identifiers include ISSN, ISO Abbreviation and NLMuniqueID; Book identifiers include ISBN.) 6145 */ 6146 public List<Identifier> getIdentifier() { 6147 if (this.identifier == null) 6148 this.identifier = new ArrayList<Identifier>(); 6149 return this.identifier; 6150 } 6151 6152 /** 6153 * @return Returns a reference to <code>this</code> for easy method chaining 6154 */ 6155 public CitationCitedArtifactPublicationFormPublishedInComponent setIdentifier(List<Identifier> theIdentifier) { 6156 this.identifier = theIdentifier; 6157 return this; 6158 } 6159 6160 public boolean hasIdentifier() { 6161 if (this.identifier == null) 6162 return false; 6163 for (Identifier item : this.identifier) 6164 if (!item.isEmpty()) 6165 return true; 6166 return false; 6167 } 6168 6169 public Identifier addIdentifier() { //3 6170 Identifier t = new Identifier(); 6171 if (this.identifier == null) 6172 this.identifier = new ArrayList<Identifier>(); 6173 this.identifier.add(t); 6174 return t; 6175 } 6176 6177 public CitationCitedArtifactPublicationFormPublishedInComponent addIdentifier(Identifier t) { //3 6178 if (t == null) 6179 return this; 6180 if (this.identifier == null) 6181 this.identifier = new ArrayList<Identifier>(); 6182 this.identifier.add(t); 6183 return this; 6184 } 6185 6186 /** 6187 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 6188 */ 6189 public Identifier getIdentifierFirstRep() { 6190 if (getIdentifier().isEmpty()) { 6191 addIdentifier(); 6192 } 6193 return getIdentifier().get(0); 6194 } 6195 6196 /** 6197 * @return {@link #title} (Name of the database or title of the book or journal.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 6198 */ 6199 public StringType getTitleElement() { 6200 if (this.title == null) 6201 if (Configuration.errorOnAutoCreate()) 6202 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormPublishedInComponent.title"); 6203 else if (Configuration.doAutoCreate()) 6204 this.title = new StringType(); // bb 6205 return this.title; 6206 } 6207 6208 public boolean hasTitleElement() { 6209 return this.title != null && !this.title.isEmpty(); 6210 } 6211 6212 public boolean hasTitle() { 6213 return this.title != null && !this.title.isEmpty(); 6214 } 6215 6216 /** 6217 * @param value {@link #title} (Name of the database or title of the book or journal.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 6218 */ 6219 public CitationCitedArtifactPublicationFormPublishedInComponent setTitleElement(StringType value) { 6220 this.title = value; 6221 return this; 6222 } 6223 6224 /** 6225 * @return Name of the database or title of the book or journal. 6226 */ 6227 public String getTitle() { 6228 return this.title == null ? null : this.title.getValue(); 6229 } 6230 6231 /** 6232 * @param value Name of the database or title of the book or journal. 6233 */ 6234 public CitationCitedArtifactPublicationFormPublishedInComponent setTitle(String value) { 6235 if (Utilities.noString(value)) 6236 this.title = null; 6237 else { 6238 if (this.title == null) 6239 this.title = new StringType(); 6240 this.title.setValue(value); 6241 } 6242 return this; 6243 } 6244 6245 /** 6246 * @return {@link #publisher} (Name of or resource describing the publisher.) 6247 */ 6248 public Reference getPublisher() { 6249 if (this.publisher == null) 6250 if (Configuration.errorOnAutoCreate()) 6251 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormPublishedInComponent.publisher"); 6252 else if (Configuration.doAutoCreate()) 6253 this.publisher = new Reference(); // cc 6254 return this.publisher; 6255 } 6256 6257 public boolean hasPublisher() { 6258 return this.publisher != null && !this.publisher.isEmpty(); 6259 } 6260 6261 /** 6262 * @param value {@link #publisher} (Name of or resource describing the publisher.) 6263 */ 6264 public CitationCitedArtifactPublicationFormPublishedInComponent setPublisher(Reference value) { 6265 this.publisher = value; 6266 return this; 6267 } 6268 6269 /** 6270 * @return {@link #publisherLocation} (Geographic location of the publisher.). This is the underlying object with id, value and extensions. The accessor "getPublisherLocation" gives direct access to the value 6271 */ 6272 public StringType getPublisherLocationElement() { 6273 if (this.publisherLocation == null) 6274 if (Configuration.errorOnAutoCreate()) 6275 throw new Error("Attempt to auto-create CitationCitedArtifactPublicationFormPublishedInComponent.publisherLocation"); 6276 else if (Configuration.doAutoCreate()) 6277 this.publisherLocation = new StringType(); // bb 6278 return this.publisherLocation; 6279 } 6280 6281 public boolean hasPublisherLocationElement() { 6282 return this.publisherLocation != null && !this.publisherLocation.isEmpty(); 6283 } 6284 6285 public boolean hasPublisherLocation() { 6286 return this.publisherLocation != null && !this.publisherLocation.isEmpty(); 6287 } 6288 6289 /** 6290 * @param value {@link #publisherLocation} (Geographic location of the publisher.). This is the underlying object with id, value and extensions. The accessor "getPublisherLocation" gives direct access to the value 6291 */ 6292 public CitationCitedArtifactPublicationFormPublishedInComponent setPublisherLocationElement(StringType value) { 6293 this.publisherLocation = value; 6294 return this; 6295 } 6296 6297 /** 6298 * @return Geographic location of the publisher. 6299 */ 6300 public String getPublisherLocation() { 6301 return this.publisherLocation == null ? null : this.publisherLocation.getValue(); 6302 } 6303 6304 /** 6305 * @param value Geographic location of the publisher. 6306 */ 6307 public CitationCitedArtifactPublicationFormPublishedInComponent setPublisherLocation(String value) { 6308 if (Utilities.noString(value)) 6309 this.publisherLocation = null; 6310 else { 6311 if (this.publisherLocation == null) 6312 this.publisherLocation = new StringType(); 6313 this.publisherLocation.setValue(value); 6314 } 6315 return this; 6316 } 6317 6318 protected void listChildren(List<Property> children) { 6319 super.listChildren(children); 6320 children.add(new Property("type", "CodeableConcept", "Kind of container (e.g. Periodical, database, or book).", 0, 1, type)); 6321 children.add(new Property("identifier", "Identifier", "Journal identifiers include ISSN, ISO Abbreviation and NLMuniqueID; Book identifiers include ISBN.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6322 children.add(new Property("title", "string", "Name of the database or title of the book or journal.", 0, 1, title)); 6323 children.add(new Property("publisher", "Reference(Organization)", "Name of or resource describing the publisher.", 0, 1, publisher)); 6324 children.add(new Property("publisherLocation", "string", "Geographic location of the publisher.", 0, 1, publisherLocation)); 6325 } 6326 6327 @Override 6328 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6329 switch (_hash) { 6330 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Kind of container (e.g. Periodical, database, or book).", 0, 1, type); 6331 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Journal identifiers include ISSN, ISO Abbreviation and NLMuniqueID; Book identifiers include ISBN.", 0, java.lang.Integer.MAX_VALUE, identifier); 6332 case 110371416: /*title*/ return new Property("title", "string", "Name of the database or title of the book or journal.", 0, 1, title); 6333 case 1447404028: /*publisher*/ return new Property("publisher", "Reference(Organization)", "Name of or resource describing the publisher.", 0, 1, publisher); 6334 case -1281627695: /*publisherLocation*/ return new Property("publisherLocation", "string", "Geographic location of the publisher.", 0, 1, publisherLocation); 6335 default: return super.getNamedProperty(_hash, _name, _checkValid); 6336 } 6337 6338 } 6339 6340 @Override 6341 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6342 switch (hash) { 6343 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 6344 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6345 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 6346 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // Reference 6347 case -1281627695: /*publisherLocation*/ return this.publisherLocation == null ? new Base[0] : new Base[] {this.publisherLocation}; // StringType 6348 default: return super.getProperty(hash, name, checkValid); 6349 } 6350 6351 } 6352 6353 @Override 6354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6355 switch (hash) { 6356 case 3575610: // type 6357 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6358 return value; 6359 case -1618432855: // identifier 6360 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6361 return value; 6362 case 110371416: // title 6363 this.title = TypeConvertor.castToString(value); // StringType 6364 return value; 6365 case 1447404028: // publisher 6366 this.publisher = TypeConvertor.castToReference(value); // Reference 6367 return value; 6368 case -1281627695: // publisherLocation 6369 this.publisherLocation = TypeConvertor.castToString(value); // StringType 6370 return value; 6371 default: return super.setProperty(hash, name, value); 6372 } 6373 6374 } 6375 6376 @Override 6377 public Base setProperty(String name, Base value) throws FHIRException { 6378 if (name.equals("type")) { 6379 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6380 } else if (name.equals("identifier")) { 6381 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6382 } else if (name.equals("title")) { 6383 this.title = TypeConvertor.castToString(value); // StringType 6384 } else if (name.equals("publisher")) { 6385 this.publisher = TypeConvertor.castToReference(value); // Reference 6386 } else if (name.equals("publisherLocation")) { 6387 this.publisherLocation = TypeConvertor.castToString(value); // StringType 6388 } else 6389 return super.setProperty(name, value); 6390 return value; 6391 } 6392 6393 @Override 6394 public void removeChild(String name, Base value) throws FHIRException { 6395 if (name.equals("type")) { 6396 this.type = null; 6397 } else if (name.equals("identifier")) { 6398 this.getIdentifier().remove(value); 6399 } else if (name.equals("title")) { 6400 this.title = null; 6401 } else if (name.equals("publisher")) { 6402 this.publisher = null; 6403 } else if (name.equals("publisherLocation")) { 6404 this.publisherLocation = null; 6405 } else 6406 super.removeChild(name, value); 6407 6408 } 6409 6410 @Override 6411 public Base makeProperty(int hash, String name) throws FHIRException { 6412 switch (hash) { 6413 case 3575610: return getType(); 6414 case -1618432855: return addIdentifier(); 6415 case 110371416: return getTitleElement(); 6416 case 1447404028: return getPublisher(); 6417 case -1281627695: return getPublisherLocationElement(); 6418 default: return super.makeProperty(hash, name); 6419 } 6420 6421 } 6422 6423 @Override 6424 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6425 switch (hash) { 6426 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 6427 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6428 case 110371416: /*title*/ return new String[] {"string"}; 6429 case 1447404028: /*publisher*/ return new String[] {"Reference"}; 6430 case -1281627695: /*publisherLocation*/ return new String[] {"string"}; 6431 default: return super.getTypesForProperty(hash, name); 6432 } 6433 6434 } 6435 6436 @Override 6437 public Base addChild(String name) throws FHIRException { 6438 if (name.equals("type")) { 6439 this.type = new CodeableConcept(); 6440 return this.type; 6441 } 6442 else if (name.equals("identifier")) { 6443 return addIdentifier(); 6444 } 6445 else if (name.equals("title")) { 6446 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.publishedIn.title"); 6447 } 6448 else if (name.equals("publisher")) { 6449 this.publisher = new Reference(); 6450 return this.publisher; 6451 } 6452 else if (name.equals("publisherLocation")) { 6453 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.publicationForm.publishedIn.publisherLocation"); 6454 } 6455 else 6456 return super.addChild(name); 6457 } 6458 6459 public CitationCitedArtifactPublicationFormPublishedInComponent copy() { 6460 CitationCitedArtifactPublicationFormPublishedInComponent dst = new CitationCitedArtifactPublicationFormPublishedInComponent(); 6461 copyValues(dst); 6462 return dst; 6463 } 6464 6465 public void copyValues(CitationCitedArtifactPublicationFormPublishedInComponent dst) { 6466 super.copyValues(dst); 6467 dst.type = type == null ? null : type.copy(); 6468 if (identifier != null) { 6469 dst.identifier = new ArrayList<Identifier>(); 6470 for (Identifier i : identifier) 6471 dst.identifier.add(i.copy()); 6472 }; 6473 dst.title = title == null ? null : title.copy(); 6474 dst.publisher = publisher == null ? null : publisher.copy(); 6475 dst.publisherLocation = publisherLocation == null ? null : publisherLocation.copy(); 6476 } 6477 6478 @Override 6479 public boolean equalsDeep(Base other_) { 6480 if (!super.equalsDeep(other_)) 6481 return false; 6482 if (!(other_ instanceof CitationCitedArtifactPublicationFormPublishedInComponent)) 6483 return false; 6484 CitationCitedArtifactPublicationFormPublishedInComponent o = (CitationCitedArtifactPublicationFormPublishedInComponent) other_; 6485 return compareDeep(type, o.type, true) && compareDeep(identifier, o.identifier, true) && compareDeep(title, o.title, true) 6486 && compareDeep(publisher, o.publisher, true) && compareDeep(publisherLocation, o.publisherLocation, true) 6487 ; 6488 } 6489 6490 @Override 6491 public boolean equalsShallow(Base other_) { 6492 if (!super.equalsShallow(other_)) 6493 return false; 6494 if (!(other_ instanceof CitationCitedArtifactPublicationFormPublishedInComponent)) 6495 return false; 6496 CitationCitedArtifactPublicationFormPublishedInComponent o = (CitationCitedArtifactPublicationFormPublishedInComponent) other_; 6497 return compareValues(title, o.title, true) && compareValues(publisherLocation, o.publisherLocation, true) 6498 ; 6499 } 6500 6501 public boolean isEmpty() { 6502 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, identifier, title 6503 , publisher, publisherLocation); 6504 } 6505 6506 public String fhirType() { 6507 return "Citation.citedArtifact.publicationForm.publishedIn"; 6508 6509 } 6510 6511 } 6512 6513 @Block() 6514 public static class CitationCitedArtifactWebLocationComponent extends BackboneElement implements IBaseBackboneElement { 6515 /** 6516 * A characterization of the object expected at the web location. 6517 */ 6518 @Child(name = "classifier", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6519 @Description(shortDefinition="Code the reason for different URLs, e.g. abstract and full-text", formalDefinition="A characterization of the object expected at the web location." ) 6520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/artifact-url-classifier") 6521 protected List<CodeableConcept> classifier; 6522 6523 /** 6524 * The specific URL. 6525 */ 6526 @Child(name = "url", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 6527 @Description(shortDefinition="The specific URL", formalDefinition="The specific URL." ) 6528 protected UriType url; 6529 6530 private static final long serialVersionUID = -1300703403L; 6531 6532 /** 6533 * Constructor 6534 */ 6535 public CitationCitedArtifactWebLocationComponent() { 6536 super(); 6537 } 6538 6539 /** 6540 * @return {@link #classifier} (A characterization of the object expected at the web location.) 6541 */ 6542 public List<CodeableConcept> getClassifier() { 6543 if (this.classifier == null) 6544 this.classifier = new ArrayList<CodeableConcept>(); 6545 return this.classifier; 6546 } 6547 6548 /** 6549 * @return Returns a reference to <code>this</code> for easy method chaining 6550 */ 6551 public CitationCitedArtifactWebLocationComponent setClassifier(List<CodeableConcept> theClassifier) { 6552 this.classifier = theClassifier; 6553 return this; 6554 } 6555 6556 public boolean hasClassifier() { 6557 if (this.classifier == null) 6558 return false; 6559 for (CodeableConcept item : this.classifier) 6560 if (!item.isEmpty()) 6561 return true; 6562 return false; 6563 } 6564 6565 public CodeableConcept addClassifier() { //3 6566 CodeableConcept t = new CodeableConcept(); 6567 if (this.classifier == null) 6568 this.classifier = new ArrayList<CodeableConcept>(); 6569 this.classifier.add(t); 6570 return t; 6571 } 6572 6573 public CitationCitedArtifactWebLocationComponent addClassifier(CodeableConcept t) { //3 6574 if (t == null) 6575 return this; 6576 if (this.classifier == null) 6577 this.classifier = new ArrayList<CodeableConcept>(); 6578 this.classifier.add(t); 6579 return this; 6580 } 6581 6582 /** 6583 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 6584 */ 6585 public CodeableConcept getClassifierFirstRep() { 6586 if (getClassifier().isEmpty()) { 6587 addClassifier(); 6588 } 6589 return getClassifier().get(0); 6590 } 6591 6592 /** 6593 * @return {@link #url} (The specific URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 6594 */ 6595 public UriType getUrlElement() { 6596 if (this.url == null) 6597 if (Configuration.errorOnAutoCreate()) 6598 throw new Error("Attempt to auto-create CitationCitedArtifactWebLocationComponent.url"); 6599 else if (Configuration.doAutoCreate()) 6600 this.url = new UriType(); // bb 6601 return this.url; 6602 } 6603 6604 public boolean hasUrlElement() { 6605 return this.url != null && !this.url.isEmpty(); 6606 } 6607 6608 public boolean hasUrl() { 6609 return this.url != null && !this.url.isEmpty(); 6610 } 6611 6612 /** 6613 * @param value {@link #url} (The specific URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 6614 */ 6615 public CitationCitedArtifactWebLocationComponent setUrlElement(UriType value) { 6616 this.url = value; 6617 return this; 6618 } 6619 6620 /** 6621 * @return The specific URL. 6622 */ 6623 public String getUrl() { 6624 return this.url == null ? null : this.url.getValue(); 6625 } 6626 6627 /** 6628 * @param value The specific URL. 6629 */ 6630 public CitationCitedArtifactWebLocationComponent setUrl(String value) { 6631 if (Utilities.noString(value)) 6632 this.url = null; 6633 else { 6634 if (this.url == null) 6635 this.url = new UriType(); 6636 this.url.setValue(value); 6637 } 6638 return this; 6639 } 6640 6641 protected void listChildren(List<Property> children) { 6642 super.listChildren(children); 6643 children.add(new Property("classifier", "CodeableConcept", "A characterization of the object expected at the web location.", 0, java.lang.Integer.MAX_VALUE, classifier)); 6644 children.add(new Property("url", "uri", "The specific URL.", 0, 1, url)); 6645 } 6646 6647 @Override 6648 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6649 switch (_hash) { 6650 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "A characterization of the object expected at the web location.", 0, java.lang.Integer.MAX_VALUE, classifier); 6651 case 116079: /*url*/ return new Property("url", "uri", "The specific URL.", 0, 1, url); 6652 default: return super.getNamedProperty(_hash, _name, _checkValid); 6653 } 6654 6655 } 6656 6657 @Override 6658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6659 switch (hash) { 6660 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 6661 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 6662 default: return super.getProperty(hash, name, checkValid); 6663 } 6664 6665 } 6666 6667 @Override 6668 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6669 switch (hash) { 6670 case -281470431: // classifier 6671 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6672 return value; 6673 case 116079: // url 6674 this.url = TypeConvertor.castToUri(value); // UriType 6675 return value; 6676 default: return super.setProperty(hash, name, value); 6677 } 6678 6679 } 6680 6681 @Override 6682 public Base setProperty(String name, Base value) throws FHIRException { 6683 if (name.equals("classifier")) { 6684 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 6685 } else if (name.equals("url")) { 6686 this.url = TypeConvertor.castToUri(value); // UriType 6687 } else 6688 return super.setProperty(name, value); 6689 return value; 6690 } 6691 6692 @Override 6693 public void removeChild(String name, Base value) throws FHIRException { 6694 if (name.equals("classifier")) { 6695 this.getClassifier().remove(value); 6696 } else if (name.equals("url")) { 6697 this.url = null; 6698 } else 6699 super.removeChild(name, value); 6700 6701 } 6702 6703 @Override 6704 public Base makeProperty(int hash, String name) throws FHIRException { 6705 switch (hash) { 6706 case -281470431: return addClassifier(); 6707 case 116079: return getUrlElement(); 6708 default: return super.makeProperty(hash, name); 6709 } 6710 6711 } 6712 6713 @Override 6714 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6715 switch (hash) { 6716 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 6717 case 116079: /*url*/ return new String[] {"uri"}; 6718 default: return super.getTypesForProperty(hash, name); 6719 } 6720 6721 } 6722 6723 @Override 6724 public Base addChild(String name) throws FHIRException { 6725 if (name.equals("classifier")) { 6726 return addClassifier(); 6727 } 6728 else if (name.equals("url")) { 6729 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.webLocation.url"); 6730 } 6731 else 6732 return super.addChild(name); 6733 } 6734 6735 public CitationCitedArtifactWebLocationComponent copy() { 6736 CitationCitedArtifactWebLocationComponent dst = new CitationCitedArtifactWebLocationComponent(); 6737 copyValues(dst); 6738 return dst; 6739 } 6740 6741 public void copyValues(CitationCitedArtifactWebLocationComponent dst) { 6742 super.copyValues(dst); 6743 if (classifier != null) { 6744 dst.classifier = new ArrayList<CodeableConcept>(); 6745 for (CodeableConcept i : classifier) 6746 dst.classifier.add(i.copy()); 6747 }; 6748 dst.url = url == null ? null : url.copy(); 6749 } 6750 6751 @Override 6752 public boolean equalsDeep(Base other_) { 6753 if (!super.equalsDeep(other_)) 6754 return false; 6755 if (!(other_ instanceof CitationCitedArtifactWebLocationComponent)) 6756 return false; 6757 CitationCitedArtifactWebLocationComponent o = (CitationCitedArtifactWebLocationComponent) other_; 6758 return compareDeep(classifier, o.classifier, true) && compareDeep(url, o.url, true); 6759 } 6760 6761 @Override 6762 public boolean equalsShallow(Base other_) { 6763 if (!super.equalsShallow(other_)) 6764 return false; 6765 if (!(other_ instanceof CitationCitedArtifactWebLocationComponent)) 6766 return false; 6767 CitationCitedArtifactWebLocationComponent o = (CitationCitedArtifactWebLocationComponent) other_; 6768 return compareValues(url, o.url, true); 6769 } 6770 6771 public boolean isEmpty() { 6772 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(classifier, url); 6773 } 6774 6775 public String fhirType() { 6776 return "Citation.citedArtifact.webLocation"; 6777 6778 } 6779 6780 } 6781 6782 @Block() 6783 public static class CitationCitedArtifactClassificationComponent extends BackboneElement implements IBaseBackboneElement { 6784 /** 6785 * The kind of classifier (e.g. publication type, keyword). 6786 */ 6787 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 6788 @Description(shortDefinition="The kind of classifier (e.g. publication type, keyword)", formalDefinition="The kind of classifier (e.g. publication type, keyword)." ) 6789 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/cited-artifact-classification-type") 6790 protected CodeableConcept type; 6791 6792 /** 6793 * The specific classification value. 6794 */ 6795 @Child(name = "classifier", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6796 @Description(shortDefinition="The specific classification value", formalDefinition="The specific classification value." ) 6797 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-artifact-classifier") 6798 protected List<CodeableConcept> classifier; 6799 6800 /** 6801 * Complex or externally created classification. 6802 */ 6803 @Child(name = "artifactAssessment", type = {ArtifactAssessment.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6804 @Description(shortDefinition="Complex or externally created classification", formalDefinition="Complex or externally created classification." ) 6805 protected List<Reference> artifactAssessment; 6806 6807 private static final long serialVersionUID = 394554928L; 6808 6809 /** 6810 * Constructor 6811 */ 6812 public CitationCitedArtifactClassificationComponent() { 6813 super(); 6814 } 6815 6816 /** 6817 * @return {@link #type} (The kind of classifier (e.g. publication type, keyword).) 6818 */ 6819 public CodeableConcept getType() { 6820 if (this.type == null) 6821 if (Configuration.errorOnAutoCreate()) 6822 throw new Error("Attempt to auto-create CitationCitedArtifactClassificationComponent.type"); 6823 else if (Configuration.doAutoCreate()) 6824 this.type = new CodeableConcept(); // cc 6825 return this.type; 6826 } 6827 6828 public boolean hasType() { 6829 return this.type != null && !this.type.isEmpty(); 6830 } 6831 6832 /** 6833 * @param value {@link #type} (The kind of classifier (e.g. publication type, keyword).) 6834 */ 6835 public CitationCitedArtifactClassificationComponent setType(CodeableConcept value) { 6836 this.type = value; 6837 return this; 6838 } 6839 6840 /** 6841 * @return {@link #classifier} (The specific classification value.) 6842 */ 6843 public List<CodeableConcept> getClassifier() { 6844 if (this.classifier == null) 6845 this.classifier = new ArrayList<CodeableConcept>(); 6846 return this.classifier; 6847 } 6848 6849 /** 6850 * @return Returns a reference to <code>this</code> for easy method chaining 6851 */ 6852 public CitationCitedArtifactClassificationComponent setClassifier(List<CodeableConcept> theClassifier) { 6853 this.classifier = theClassifier; 6854 return this; 6855 } 6856 6857 public boolean hasClassifier() { 6858 if (this.classifier == null) 6859 return false; 6860 for (CodeableConcept item : this.classifier) 6861 if (!item.isEmpty()) 6862 return true; 6863 return false; 6864 } 6865 6866 public CodeableConcept addClassifier() { //3 6867 CodeableConcept t = new CodeableConcept(); 6868 if (this.classifier == null) 6869 this.classifier = new ArrayList<CodeableConcept>(); 6870 this.classifier.add(t); 6871 return t; 6872 } 6873 6874 public CitationCitedArtifactClassificationComponent addClassifier(CodeableConcept t) { //3 6875 if (t == null) 6876 return this; 6877 if (this.classifier == null) 6878 this.classifier = new ArrayList<CodeableConcept>(); 6879 this.classifier.add(t); 6880 return this; 6881 } 6882 6883 /** 6884 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 6885 */ 6886 public CodeableConcept getClassifierFirstRep() { 6887 if (getClassifier().isEmpty()) { 6888 addClassifier(); 6889 } 6890 return getClassifier().get(0); 6891 } 6892 6893 /** 6894 * @return {@link #artifactAssessment} (Complex or externally created classification.) 6895 */ 6896 public List<Reference> getArtifactAssessment() { 6897 if (this.artifactAssessment == null) 6898 this.artifactAssessment = new ArrayList<Reference>(); 6899 return this.artifactAssessment; 6900 } 6901 6902 /** 6903 * @return Returns a reference to <code>this</code> for easy method chaining 6904 */ 6905 public CitationCitedArtifactClassificationComponent setArtifactAssessment(List<Reference> theArtifactAssessment) { 6906 this.artifactAssessment = theArtifactAssessment; 6907 return this; 6908 } 6909 6910 public boolean hasArtifactAssessment() { 6911 if (this.artifactAssessment == null) 6912 return false; 6913 for (Reference item : this.artifactAssessment) 6914 if (!item.isEmpty()) 6915 return true; 6916 return false; 6917 } 6918 6919 public Reference addArtifactAssessment() { //3 6920 Reference t = new Reference(); 6921 if (this.artifactAssessment == null) 6922 this.artifactAssessment = new ArrayList<Reference>(); 6923 this.artifactAssessment.add(t); 6924 return t; 6925 } 6926 6927 public CitationCitedArtifactClassificationComponent addArtifactAssessment(Reference t) { //3 6928 if (t == null) 6929 return this; 6930 if (this.artifactAssessment == null) 6931 this.artifactAssessment = new ArrayList<Reference>(); 6932 this.artifactAssessment.add(t); 6933 return this; 6934 } 6935 6936 /** 6937 * @return The first repetition of repeating field {@link #artifactAssessment}, creating it if it does not already exist {3} 6938 */ 6939 public Reference getArtifactAssessmentFirstRep() { 6940 if (getArtifactAssessment().isEmpty()) { 6941 addArtifactAssessment(); 6942 } 6943 return getArtifactAssessment().get(0); 6944 } 6945 6946 protected void listChildren(List<Property> children) { 6947 super.listChildren(children); 6948 children.add(new Property("type", "CodeableConcept", "The kind of classifier (e.g. publication type, keyword).", 0, 1, type)); 6949 children.add(new Property("classifier", "CodeableConcept", "The specific classification value.", 0, java.lang.Integer.MAX_VALUE, classifier)); 6950 children.add(new Property("artifactAssessment", "Reference(ArtifactAssessment)", "Complex or externally created classification.", 0, java.lang.Integer.MAX_VALUE, artifactAssessment)); 6951 } 6952 6953 @Override 6954 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6955 switch (_hash) { 6956 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of classifier (e.g. publication type, keyword).", 0, 1, type); 6957 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "The specific classification value.", 0, java.lang.Integer.MAX_VALUE, classifier); 6958 case 1014987316: /*artifactAssessment*/ return new Property("artifactAssessment", "Reference(ArtifactAssessment)", "Complex or externally created classification.", 0, java.lang.Integer.MAX_VALUE, artifactAssessment); 6959 default: return super.getNamedProperty(_hash, _name, _checkValid); 6960 } 6961 6962 } 6963 6964 @Override 6965 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6966 switch (hash) { 6967 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 6968 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 6969 case 1014987316: /*artifactAssessment*/ return this.artifactAssessment == null ? new Base[0] : this.artifactAssessment.toArray(new Base[this.artifactAssessment.size()]); // Reference 6970 default: return super.getProperty(hash, name, checkValid); 6971 } 6972 6973 } 6974 6975 @Override 6976 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6977 switch (hash) { 6978 case 3575610: // type 6979 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6980 return value; 6981 case -281470431: // classifier 6982 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6983 return value; 6984 case 1014987316: // artifactAssessment 6985 this.getArtifactAssessment().add(TypeConvertor.castToReference(value)); // Reference 6986 return value; 6987 default: return super.setProperty(hash, name, value); 6988 } 6989 6990 } 6991 6992 @Override 6993 public Base setProperty(String name, Base value) throws FHIRException { 6994 if (name.equals("type")) { 6995 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6996 } else if (name.equals("classifier")) { 6997 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 6998 } else if (name.equals("artifactAssessment")) { 6999 this.getArtifactAssessment().add(TypeConvertor.castToReference(value)); 7000 } else 7001 return super.setProperty(name, value); 7002 return value; 7003 } 7004 7005 @Override 7006 public void removeChild(String name, Base value) throws FHIRException { 7007 if (name.equals("type")) { 7008 this.type = null; 7009 } else if (name.equals("classifier")) { 7010 this.getClassifier().remove(value); 7011 } else if (name.equals("artifactAssessment")) { 7012 this.getArtifactAssessment().remove(value); 7013 } else 7014 super.removeChild(name, value); 7015 7016 } 7017 7018 @Override 7019 public Base makeProperty(int hash, String name) throws FHIRException { 7020 switch (hash) { 7021 case 3575610: return getType(); 7022 case -281470431: return addClassifier(); 7023 case 1014987316: return addArtifactAssessment(); 7024 default: return super.makeProperty(hash, name); 7025 } 7026 7027 } 7028 7029 @Override 7030 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7031 switch (hash) { 7032 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 7033 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 7034 case 1014987316: /*artifactAssessment*/ return new String[] {"Reference"}; 7035 default: return super.getTypesForProperty(hash, name); 7036 } 7037 7038 } 7039 7040 @Override 7041 public Base addChild(String name) throws FHIRException { 7042 if (name.equals("type")) { 7043 this.type = new CodeableConcept(); 7044 return this.type; 7045 } 7046 else if (name.equals("classifier")) { 7047 return addClassifier(); 7048 } 7049 else if (name.equals("artifactAssessment")) { 7050 return addArtifactAssessment(); 7051 } 7052 else 7053 return super.addChild(name); 7054 } 7055 7056 public CitationCitedArtifactClassificationComponent copy() { 7057 CitationCitedArtifactClassificationComponent dst = new CitationCitedArtifactClassificationComponent(); 7058 copyValues(dst); 7059 return dst; 7060 } 7061 7062 public void copyValues(CitationCitedArtifactClassificationComponent dst) { 7063 super.copyValues(dst); 7064 dst.type = type == null ? null : type.copy(); 7065 if (classifier != null) { 7066 dst.classifier = new ArrayList<CodeableConcept>(); 7067 for (CodeableConcept i : classifier) 7068 dst.classifier.add(i.copy()); 7069 }; 7070 if (artifactAssessment != null) { 7071 dst.artifactAssessment = new ArrayList<Reference>(); 7072 for (Reference i : artifactAssessment) 7073 dst.artifactAssessment.add(i.copy()); 7074 }; 7075 } 7076 7077 @Override 7078 public boolean equalsDeep(Base other_) { 7079 if (!super.equalsDeep(other_)) 7080 return false; 7081 if (!(other_ instanceof CitationCitedArtifactClassificationComponent)) 7082 return false; 7083 CitationCitedArtifactClassificationComponent o = (CitationCitedArtifactClassificationComponent) other_; 7084 return compareDeep(type, o.type, true) && compareDeep(classifier, o.classifier, true) && compareDeep(artifactAssessment, o.artifactAssessment, true) 7085 ; 7086 } 7087 7088 @Override 7089 public boolean equalsShallow(Base other_) { 7090 if (!super.equalsShallow(other_)) 7091 return false; 7092 if (!(other_ instanceof CitationCitedArtifactClassificationComponent)) 7093 return false; 7094 CitationCitedArtifactClassificationComponent o = (CitationCitedArtifactClassificationComponent) other_; 7095 return true; 7096 } 7097 7098 public boolean isEmpty() { 7099 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, classifier, artifactAssessment 7100 ); 7101 } 7102 7103 public String fhirType() { 7104 return "Citation.citedArtifact.classification"; 7105 7106 } 7107 7108 } 7109 7110 @Block() 7111 public static class CitationCitedArtifactContributorshipComponent extends BackboneElement implements IBaseBackboneElement { 7112 /** 7113 * Indicates if the list includes all authors and/or contributors. 7114 */ 7115 @Child(name = "complete", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 7116 @Description(shortDefinition="Indicates if the list includes all authors and/or contributors", formalDefinition="Indicates if the list includes all authors and/or contributors." ) 7117 protected BooleanType complete; 7118 7119 /** 7120 * An individual entity named as a contributor, for example in the author list or contributor list. 7121 */ 7122 @Child(name = "entry", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7123 @Description(shortDefinition="An individual entity named as a contributor", formalDefinition="An individual entity named as a contributor, for example in the author list or contributor list." ) 7124 protected List<CitationCitedArtifactContributorshipEntryComponent> entry; 7125 7126 /** 7127 * Used to record a display of the author/contributor list without separate data element for each list member. 7128 */ 7129 @Child(name = "summary", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7130 @Description(shortDefinition="Used to record a display of the author/contributor list without separate data element for each list member", formalDefinition="Used to record a display of the author/contributor list without separate data element for each list member." ) 7131 protected List<ContributorshipSummaryComponent> summary; 7132 7133 private static final long serialVersionUID = 662810405L; 7134 7135 /** 7136 * Constructor 7137 */ 7138 public CitationCitedArtifactContributorshipComponent() { 7139 super(); 7140 } 7141 7142 /** 7143 * @return {@link #complete} (Indicates if the list includes all authors and/or contributors.). This is the underlying object with id, value and extensions. The accessor "getComplete" gives direct access to the value 7144 */ 7145 public BooleanType getCompleteElement() { 7146 if (this.complete == null) 7147 if (Configuration.errorOnAutoCreate()) 7148 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipComponent.complete"); 7149 else if (Configuration.doAutoCreate()) 7150 this.complete = new BooleanType(); // bb 7151 return this.complete; 7152 } 7153 7154 public boolean hasCompleteElement() { 7155 return this.complete != null && !this.complete.isEmpty(); 7156 } 7157 7158 public boolean hasComplete() { 7159 return this.complete != null && !this.complete.isEmpty(); 7160 } 7161 7162 /** 7163 * @param value {@link #complete} (Indicates if the list includes all authors and/or contributors.). This is the underlying object with id, value and extensions. The accessor "getComplete" gives direct access to the value 7164 */ 7165 public CitationCitedArtifactContributorshipComponent setCompleteElement(BooleanType value) { 7166 this.complete = value; 7167 return this; 7168 } 7169 7170 /** 7171 * @return Indicates if the list includes all authors and/or contributors. 7172 */ 7173 public boolean getComplete() { 7174 return this.complete == null || this.complete.isEmpty() ? false : this.complete.getValue(); 7175 } 7176 7177 /** 7178 * @param value Indicates if the list includes all authors and/or contributors. 7179 */ 7180 public CitationCitedArtifactContributorshipComponent setComplete(boolean value) { 7181 if (this.complete == null) 7182 this.complete = new BooleanType(); 7183 this.complete.setValue(value); 7184 return this; 7185 } 7186 7187 /** 7188 * @return {@link #entry} (An individual entity named as a contributor, for example in the author list or contributor list.) 7189 */ 7190 public List<CitationCitedArtifactContributorshipEntryComponent> getEntry() { 7191 if (this.entry == null) 7192 this.entry = new ArrayList<CitationCitedArtifactContributorshipEntryComponent>(); 7193 return this.entry; 7194 } 7195 7196 /** 7197 * @return Returns a reference to <code>this</code> for easy method chaining 7198 */ 7199 public CitationCitedArtifactContributorshipComponent setEntry(List<CitationCitedArtifactContributorshipEntryComponent> theEntry) { 7200 this.entry = theEntry; 7201 return this; 7202 } 7203 7204 public boolean hasEntry() { 7205 if (this.entry == null) 7206 return false; 7207 for (CitationCitedArtifactContributorshipEntryComponent item : this.entry) 7208 if (!item.isEmpty()) 7209 return true; 7210 return false; 7211 } 7212 7213 public CitationCitedArtifactContributorshipEntryComponent addEntry() { //3 7214 CitationCitedArtifactContributorshipEntryComponent t = new CitationCitedArtifactContributorshipEntryComponent(); 7215 if (this.entry == null) 7216 this.entry = new ArrayList<CitationCitedArtifactContributorshipEntryComponent>(); 7217 this.entry.add(t); 7218 return t; 7219 } 7220 7221 public CitationCitedArtifactContributorshipComponent addEntry(CitationCitedArtifactContributorshipEntryComponent t) { //3 7222 if (t == null) 7223 return this; 7224 if (this.entry == null) 7225 this.entry = new ArrayList<CitationCitedArtifactContributorshipEntryComponent>(); 7226 this.entry.add(t); 7227 return this; 7228 } 7229 7230 /** 7231 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist {3} 7232 */ 7233 public CitationCitedArtifactContributorshipEntryComponent getEntryFirstRep() { 7234 if (getEntry().isEmpty()) { 7235 addEntry(); 7236 } 7237 return getEntry().get(0); 7238 } 7239 7240 /** 7241 * @return {@link #summary} (Used to record a display of the author/contributor list without separate data element for each list member.) 7242 */ 7243 public List<ContributorshipSummaryComponent> getSummary() { 7244 if (this.summary == null) 7245 this.summary = new ArrayList<ContributorshipSummaryComponent>(); 7246 return this.summary; 7247 } 7248 7249 /** 7250 * @return Returns a reference to <code>this</code> for easy method chaining 7251 */ 7252 public CitationCitedArtifactContributorshipComponent setSummary(List<ContributorshipSummaryComponent> theSummary) { 7253 this.summary = theSummary; 7254 return this; 7255 } 7256 7257 public boolean hasSummary() { 7258 if (this.summary == null) 7259 return false; 7260 for (ContributorshipSummaryComponent item : this.summary) 7261 if (!item.isEmpty()) 7262 return true; 7263 return false; 7264 } 7265 7266 public ContributorshipSummaryComponent addSummary() { //3 7267 ContributorshipSummaryComponent t = new ContributorshipSummaryComponent(); 7268 if (this.summary == null) 7269 this.summary = new ArrayList<ContributorshipSummaryComponent>(); 7270 this.summary.add(t); 7271 return t; 7272 } 7273 7274 public CitationCitedArtifactContributorshipComponent addSummary(ContributorshipSummaryComponent t) { //3 7275 if (t == null) 7276 return this; 7277 if (this.summary == null) 7278 this.summary = new ArrayList<ContributorshipSummaryComponent>(); 7279 this.summary.add(t); 7280 return this; 7281 } 7282 7283 /** 7284 * @return The first repetition of repeating field {@link #summary}, creating it if it does not already exist {3} 7285 */ 7286 public ContributorshipSummaryComponent getSummaryFirstRep() { 7287 if (getSummary().isEmpty()) { 7288 addSummary(); 7289 } 7290 return getSummary().get(0); 7291 } 7292 7293 protected void listChildren(List<Property> children) { 7294 super.listChildren(children); 7295 children.add(new Property("complete", "boolean", "Indicates if the list includes all authors and/or contributors.", 0, 1, complete)); 7296 children.add(new Property("entry", "", "An individual entity named as a contributor, for example in the author list or contributor list.", 0, java.lang.Integer.MAX_VALUE, entry)); 7297 children.add(new Property("summary", "", "Used to record a display of the author/contributor list without separate data element for each list member.", 0, java.lang.Integer.MAX_VALUE, summary)); 7298 } 7299 7300 @Override 7301 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7302 switch (_hash) { 7303 case -599445191: /*complete*/ return new Property("complete", "boolean", "Indicates if the list includes all authors and/or contributors.", 0, 1, complete); 7304 case 96667762: /*entry*/ return new Property("entry", "", "An individual entity named as a contributor, for example in the author list or contributor list.", 0, java.lang.Integer.MAX_VALUE, entry); 7305 case -1857640538: /*summary*/ return new Property("summary", "", "Used to record a display of the author/contributor list without separate data element for each list member.", 0, java.lang.Integer.MAX_VALUE, summary); 7306 default: return super.getNamedProperty(_hash, _name, _checkValid); 7307 } 7308 7309 } 7310 7311 @Override 7312 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7313 switch (hash) { 7314 case -599445191: /*complete*/ return this.complete == null ? new Base[0] : new Base[] {this.complete}; // BooleanType 7315 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // CitationCitedArtifactContributorshipEntryComponent 7316 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : this.summary.toArray(new Base[this.summary.size()]); // ContributorshipSummaryComponent 7317 default: return super.getProperty(hash, name, checkValid); 7318 } 7319 7320 } 7321 7322 @Override 7323 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7324 switch (hash) { 7325 case -599445191: // complete 7326 this.complete = TypeConvertor.castToBoolean(value); // BooleanType 7327 return value; 7328 case 96667762: // entry 7329 this.getEntry().add((CitationCitedArtifactContributorshipEntryComponent) value); // CitationCitedArtifactContributorshipEntryComponent 7330 return value; 7331 case -1857640538: // summary 7332 this.getSummary().add((ContributorshipSummaryComponent) value); // ContributorshipSummaryComponent 7333 return value; 7334 default: return super.setProperty(hash, name, value); 7335 } 7336 7337 } 7338 7339 @Override 7340 public Base setProperty(String name, Base value) throws FHIRException { 7341 if (name.equals("complete")) { 7342 this.complete = TypeConvertor.castToBoolean(value); // BooleanType 7343 } else if (name.equals("entry")) { 7344 this.getEntry().add((CitationCitedArtifactContributorshipEntryComponent) value); 7345 } else if (name.equals("summary")) { 7346 this.getSummary().add((ContributorshipSummaryComponent) value); 7347 } else 7348 return super.setProperty(name, value); 7349 return value; 7350 } 7351 7352 @Override 7353 public void removeChild(String name, Base value) throws FHIRException { 7354 if (name.equals("complete")) { 7355 this.complete = null; 7356 } else if (name.equals("entry")) { 7357 this.getEntry().remove((CitationCitedArtifactContributorshipEntryComponent) value); 7358 } else if (name.equals("summary")) { 7359 this.getSummary().remove((ContributorshipSummaryComponent) value); 7360 } else 7361 super.removeChild(name, value); 7362 7363 } 7364 7365 @Override 7366 public Base makeProperty(int hash, String name) throws FHIRException { 7367 switch (hash) { 7368 case -599445191: return getCompleteElement(); 7369 case 96667762: return addEntry(); 7370 case -1857640538: return addSummary(); 7371 default: return super.makeProperty(hash, name); 7372 } 7373 7374 } 7375 7376 @Override 7377 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7378 switch (hash) { 7379 case -599445191: /*complete*/ return new String[] {"boolean"}; 7380 case 96667762: /*entry*/ return new String[] {}; 7381 case -1857640538: /*summary*/ return new String[] {}; 7382 default: return super.getTypesForProperty(hash, name); 7383 } 7384 7385 } 7386 7387 @Override 7388 public Base addChild(String name) throws FHIRException { 7389 if (name.equals("complete")) { 7390 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.contributorship.complete"); 7391 } 7392 else if (name.equals("entry")) { 7393 return addEntry(); 7394 } 7395 else if (name.equals("summary")) { 7396 return addSummary(); 7397 } 7398 else 7399 return super.addChild(name); 7400 } 7401 7402 public CitationCitedArtifactContributorshipComponent copy() { 7403 CitationCitedArtifactContributorshipComponent dst = new CitationCitedArtifactContributorshipComponent(); 7404 copyValues(dst); 7405 return dst; 7406 } 7407 7408 public void copyValues(CitationCitedArtifactContributorshipComponent dst) { 7409 super.copyValues(dst); 7410 dst.complete = complete == null ? null : complete.copy(); 7411 if (entry != null) { 7412 dst.entry = new ArrayList<CitationCitedArtifactContributorshipEntryComponent>(); 7413 for (CitationCitedArtifactContributorshipEntryComponent i : entry) 7414 dst.entry.add(i.copy()); 7415 }; 7416 if (summary != null) { 7417 dst.summary = new ArrayList<ContributorshipSummaryComponent>(); 7418 for (ContributorshipSummaryComponent i : summary) 7419 dst.summary.add(i.copy()); 7420 }; 7421 } 7422 7423 @Override 7424 public boolean equalsDeep(Base other_) { 7425 if (!super.equalsDeep(other_)) 7426 return false; 7427 if (!(other_ instanceof CitationCitedArtifactContributorshipComponent)) 7428 return false; 7429 CitationCitedArtifactContributorshipComponent o = (CitationCitedArtifactContributorshipComponent) other_; 7430 return compareDeep(complete, o.complete, true) && compareDeep(entry, o.entry, true) && compareDeep(summary, o.summary, true) 7431 ; 7432 } 7433 7434 @Override 7435 public boolean equalsShallow(Base other_) { 7436 if (!super.equalsShallow(other_)) 7437 return false; 7438 if (!(other_ instanceof CitationCitedArtifactContributorshipComponent)) 7439 return false; 7440 CitationCitedArtifactContributorshipComponent o = (CitationCitedArtifactContributorshipComponent) other_; 7441 return compareValues(complete, o.complete, true); 7442 } 7443 7444 public boolean isEmpty() { 7445 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(complete, entry, summary 7446 ); 7447 } 7448 7449 public String fhirType() { 7450 return "Citation.citedArtifact.contributorship"; 7451 7452 } 7453 7454 } 7455 7456 @Block() 7457 public static class CitationCitedArtifactContributorshipEntryComponent extends BackboneElement implements IBaseBackboneElement { 7458 /** 7459 * The identity of the individual contributor. 7460 */ 7461 @Child(name = "contributor", type = {Practitioner.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=false) 7462 @Description(shortDefinition="The identity of the individual contributor", formalDefinition="The identity of the individual contributor." ) 7463 protected Reference contributor; 7464 7465 /** 7466 * For citation styles that use initials. 7467 */ 7468 @Child(name = "forenameInitials", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 7469 @Description(shortDefinition="For citation styles that use initials", formalDefinition="For citation styles that use initials." ) 7470 protected StringType forenameInitials; 7471 7472 /** 7473 * Organization affiliated with the contributor. 7474 */ 7475 @Child(name = "affiliation", type = {Organization.class, PractitionerRole.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7476 @Description(shortDefinition="Organizational affiliation", formalDefinition="Organization affiliated with the contributor." ) 7477 protected List<Reference> affiliation; 7478 7479 /** 7480 * This element identifies the specific nature of an individual?s contribution with respect to the cited work. 7481 */ 7482 @Child(name = "contributionType", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7483 @Description(shortDefinition="The specific contribution", formalDefinition="This element identifies the specific nature of an individual?s contribution with respect to the cited work." ) 7484 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/artifact-contribution-type") 7485 protected List<CodeableConcept> contributionType; 7486 7487 /** 7488 * The role of the contributor (e.g. author, editor, reviewer, funder). 7489 */ 7490 @Child(name = "role", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 7491 @Description(shortDefinition="The role of the contributor (e.g. author, editor, reviewer, funder)", formalDefinition="The role of the contributor (e.g. author, editor, reviewer, funder)." ) 7492 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contributor-role") 7493 protected CodeableConcept role; 7494 7495 /** 7496 * Contributions with accounting for time or number. 7497 */ 7498 @Child(name = "contributionInstance", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7499 @Description(shortDefinition="Contributions with accounting for time or number", formalDefinition="Contributions with accounting for time or number." ) 7500 protected List<CitationCitedArtifactContributorshipEntryContributionInstanceComponent> contributionInstance; 7501 7502 /** 7503 * Whether the contributor is the corresponding contributor for the role. 7504 */ 7505 @Child(name = "correspondingContact", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=false) 7506 @Description(shortDefinition="Whether the contributor is the corresponding contributor for the role", formalDefinition="Whether the contributor is the corresponding contributor for the role." ) 7507 protected BooleanType correspondingContact; 7508 7509 /** 7510 * Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author. 7511 */ 7512 @Child(name = "rankingOrder", type = {PositiveIntType.class}, order=8, min=0, max=1, modifier=false, summary=false) 7513 @Description(shortDefinition="Ranked order of contribution", formalDefinition="Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author." ) 7514 protected PositiveIntType rankingOrder; 7515 7516 private static final long serialVersionUID = 1654594857L; 7517 7518 /** 7519 * Constructor 7520 */ 7521 public CitationCitedArtifactContributorshipEntryComponent() { 7522 super(); 7523 } 7524 7525 /** 7526 * Constructor 7527 */ 7528 public CitationCitedArtifactContributorshipEntryComponent(Reference contributor) { 7529 super(); 7530 this.setContributor(contributor); 7531 } 7532 7533 /** 7534 * @return {@link #contributor} (The identity of the individual contributor.) 7535 */ 7536 public Reference getContributor() { 7537 if (this.contributor == null) 7538 if (Configuration.errorOnAutoCreate()) 7539 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryComponent.contributor"); 7540 else if (Configuration.doAutoCreate()) 7541 this.contributor = new Reference(); // cc 7542 return this.contributor; 7543 } 7544 7545 public boolean hasContributor() { 7546 return this.contributor != null && !this.contributor.isEmpty(); 7547 } 7548 7549 /** 7550 * @param value {@link #contributor} (The identity of the individual contributor.) 7551 */ 7552 public CitationCitedArtifactContributorshipEntryComponent setContributor(Reference value) { 7553 this.contributor = value; 7554 return this; 7555 } 7556 7557 /** 7558 * @return {@link #forenameInitials} (For citation styles that use initials.). This is the underlying object with id, value and extensions. The accessor "getForenameInitials" gives direct access to the value 7559 */ 7560 public StringType getForenameInitialsElement() { 7561 if (this.forenameInitials == null) 7562 if (Configuration.errorOnAutoCreate()) 7563 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryComponent.forenameInitials"); 7564 else if (Configuration.doAutoCreate()) 7565 this.forenameInitials = new StringType(); // bb 7566 return this.forenameInitials; 7567 } 7568 7569 public boolean hasForenameInitialsElement() { 7570 return this.forenameInitials != null && !this.forenameInitials.isEmpty(); 7571 } 7572 7573 public boolean hasForenameInitials() { 7574 return this.forenameInitials != null && !this.forenameInitials.isEmpty(); 7575 } 7576 7577 /** 7578 * @param value {@link #forenameInitials} (For citation styles that use initials.). This is the underlying object with id, value and extensions. The accessor "getForenameInitials" gives direct access to the value 7579 */ 7580 public CitationCitedArtifactContributorshipEntryComponent setForenameInitialsElement(StringType value) { 7581 this.forenameInitials = value; 7582 return this; 7583 } 7584 7585 /** 7586 * @return For citation styles that use initials. 7587 */ 7588 public String getForenameInitials() { 7589 return this.forenameInitials == null ? null : this.forenameInitials.getValue(); 7590 } 7591 7592 /** 7593 * @param value For citation styles that use initials. 7594 */ 7595 public CitationCitedArtifactContributorshipEntryComponent setForenameInitials(String value) { 7596 if (Utilities.noString(value)) 7597 this.forenameInitials = null; 7598 else { 7599 if (this.forenameInitials == null) 7600 this.forenameInitials = new StringType(); 7601 this.forenameInitials.setValue(value); 7602 } 7603 return this; 7604 } 7605 7606 /** 7607 * @return {@link #affiliation} (Organization affiliated with the contributor.) 7608 */ 7609 public List<Reference> getAffiliation() { 7610 if (this.affiliation == null) 7611 this.affiliation = new ArrayList<Reference>(); 7612 return this.affiliation; 7613 } 7614 7615 /** 7616 * @return Returns a reference to <code>this</code> for easy method chaining 7617 */ 7618 public CitationCitedArtifactContributorshipEntryComponent setAffiliation(List<Reference> theAffiliation) { 7619 this.affiliation = theAffiliation; 7620 return this; 7621 } 7622 7623 public boolean hasAffiliation() { 7624 if (this.affiliation == null) 7625 return false; 7626 for (Reference item : this.affiliation) 7627 if (!item.isEmpty()) 7628 return true; 7629 return false; 7630 } 7631 7632 public Reference addAffiliation() { //3 7633 Reference t = new Reference(); 7634 if (this.affiliation == null) 7635 this.affiliation = new ArrayList<Reference>(); 7636 this.affiliation.add(t); 7637 return t; 7638 } 7639 7640 public CitationCitedArtifactContributorshipEntryComponent addAffiliation(Reference t) { //3 7641 if (t == null) 7642 return this; 7643 if (this.affiliation == null) 7644 this.affiliation = new ArrayList<Reference>(); 7645 this.affiliation.add(t); 7646 return this; 7647 } 7648 7649 /** 7650 * @return The first repetition of repeating field {@link #affiliation}, creating it if it does not already exist {3} 7651 */ 7652 public Reference getAffiliationFirstRep() { 7653 if (getAffiliation().isEmpty()) { 7654 addAffiliation(); 7655 } 7656 return getAffiliation().get(0); 7657 } 7658 7659 /** 7660 * @return {@link #contributionType} (This element identifies the specific nature of an individual?s contribution with respect to the cited work.) 7661 */ 7662 public List<CodeableConcept> getContributionType() { 7663 if (this.contributionType == null) 7664 this.contributionType = new ArrayList<CodeableConcept>(); 7665 return this.contributionType; 7666 } 7667 7668 /** 7669 * @return Returns a reference to <code>this</code> for easy method chaining 7670 */ 7671 public CitationCitedArtifactContributorshipEntryComponent setContributionType(List<CodeableConcept> theContributionType) { 7672 this.contributionType = theContributionType; 7673 return this; 7674 } 7675 7676 public boolean hasContributionType() { 7677 if (this.contributionType == null) 7678 return false; 7679 for (CodeableConcept item : this.contributionType) 7680 if (!item.isEmpty()) 7681 return true; 7682 return false; 7683 } 7684 7685 public CodeableConcept addContributionType() { //3 7686 CodeableConcept t = new CodeableConcept(); 7687 if (this.contributionType == null) 7688 this.contributionType = new ArrayList<CodeableConcept>(); 7689 this.contributionType.add(t); 7690 return t; 7691 } 7692 7693 public CitationCitedArtifactContributorshipEntryComponent addContributionType(CodeableConcept t) { //3 7694 if (t == null) 7695 return this; 7696 if (this.contributionType == null) 7697 this.contributionType = new ArrayList<CodeableConcept>(); 7698 this.contributionType.add(t); 7699 return this; 7700 } 7701 7702 /** 7703 * @return The first repetition of repeating field {@link #contributionType}, creating it if it does not already exist {3} 7704 */ 7705 public CodeableConcept getContributionTypeFirstRep() { 7706 if (getContributionType().isEmpty()) { 7707 addContributionType(); 7708 } 7709 return getContributionType().get(0); 7710 } 7711 7712 /** 7713 * @return {@link #role} (The role of the contributor (e.g. author, editor, reviewer, funder).) 7714 */ 7715 public CodeableConcept getRole() { 7716 if (this.role == null) 7717 if (Configuration.errorOnAutoCreate()) 7718 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryComponent.role"); 7719 else if (Configuration.doAutoCreate()) 7720 this.role = new CodeableConcept(); // cc 7721 return this.role; 7722 } 7723 7724 public boolean hasRole() { 7725 return this.role != null && !this.role.isEmpty(); 7726 } 7727 7728 /** 7729 * @param value {@link #role} (The role of the contributor (e.g. author, editor, reviewer, funder).) 7730 */ 7731 public CitationCitedArtifactContributorshipEntryComponent setRole(CodeableConcept value) { 7732 this.role = value; 7733 return this; 7734 } 7735 7736 /** 7737 * @return {@link #contributionInstance} (Contributions with accounting for time or number.) 7738 */ 7739 public List<CitationCitedArtifactContributorshipEntryContributionInstanceComponent> getContributionInstance() { 7740 if (this.contributionInstance == null) 7741 this.contributionInstance = new ArrayList<CitationCitedArtifactContributorshipEntryContributionInstanceComponent>(); 7742 return this.contributionInstance; 7743 } 7744 7745 /** 7746 * @return Returns a reference to <code>this</code> for easy method chaining 7747 */ 7748 public CitationCitedArtifactContributorshipEntryComponent setContributionInstance(List<CitationCitedArtifactContributorshipEntryContributionInstanceComponent> theContributionInstance) { 7749 this.contributionInstance = theContributionInstance; 7750 return this; 7751 } 7752 7753 public boolean hasContributionInstance() { 7754 if (this.contributionInstance == null) 7755 return false; 7756 for (CitationCitedArtifactContributorshipEntryContributionInstanceComponent item : this.contributionInstance) 7757 if (!item.isEmpty()) 7758 return true; 7759 return false; 7760 } 7761 7762 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent addContributionInstance() { //3 7763 CitationCitedArtifactContributorshipEntryContributionInstanceComponent t = new CitationCitedArtifactContributorshipEntryContributionInstanceComponent(); 7764 if (this.contributionInstance == null) 7765 this.contributionInstance = new ArrayList<CitationCitedArtifactContributorshipEntryContributionInstanceComponent>(); 7766 this.contributionInstance.add(t); 7767 return t; 7768 } 7769 7770 public CitationCitedArtifactContributorshipEntryComponent addContributionInstance(CitationCitedArtifactContributorshipEntryContributionInstanceComponent t) { //3 7771 if (t == null) 7772 return this; 7773 if (this.contributionInstance == null) 7774 this.contributionInstance = new ArrayList<CitationCitedArtifactContributorshipEntryContributionInstanceComponent>(); 7775 this.contributionInstance.add(t); 7776 return this; 7777 } 7778 7779 /** 7780 * @return The first repetition of repeating field {@link #contributionInstance}, creating it if it does not already exist {3} 7781 */ 7782 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent getContributionInstanceFirstRep() { 7783 if (getContributionInstance().isEmpty()) { 7784 addContributionInstance(); 7785 } 7786 return getContributionInstance().get(0); 7787 } 7788 7789 /** 7790 * @return {@link #correspondingContact} (Whether the contributor is the corresponding contributor for the role.). This is the underlying object with id, value and extensions. The accessor "getCorrespondingContact" gives direct access to the value 7791 */ 7792 public BooleanType getCorrespondingContactElement() { 7793 if (this.correspondingContact == null) 7794 if (Configuration.errorOnAutoCreate()) 7795 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryComponent.correspondingContact"); 7796 else if (Configuration.doAutoCreate()) 7797 this.correspondingContact = new BooleanType(); // bb 7798 return this.correspondingContact; 7799 } 7800 7801 public boolean hasCorrespondingContactElement() { 7802 return this.correspondingContact != null && !this.correspondingContact.isEmpty(); 7803 } 7804 7805 public boolean hasCorrespondingContact() { 7806 return this.correspondingContact != null && !this.correspondingContact.isEmpty(); 7807 } 7808 7809 /** 7810 * @param value {@link #correspondingContact} (Whether the contributor is the corresponding contributor for the role.). This is the underlying object with id, value and extensions. The accessor "getCorrespondingContact" gives direct access to the value 7811 */ 7812 public CitationCitedArtifactContributorshipEntryComponent setCorrespondingContactElement(BooleanType value) { 7813 this.correspondingContact = value; 7814 return this; 7815 } 7816 7817 /** 7818 * @return Whether the contributor is the corresponding contributor for the role. 7819 */ 7820 public boolean getCorrespondingContact() { 7821 return this.correspondingContact == null || this.correspondingContact.isEmpty() ? false : this.correspondingContact.getValue(); 7822 } 7823 7824 /** 7825 * @param value Whether the contributor is the corresponding contributor for the role. 7826 */ 7827 public CitationCitedArtifactContributorshipEntryComponent setCorrespondingContact(boolean value) { 7828 if (this.correspondingContact == null) 7829 this.correspondingContact = new BooleanType(); 7830 this.correspondingContact.setValue(value); 7831 return this; 7832 } 7833 7834 /** 7835 * @return {@link #rankingOrder} (Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author.). This is the underlying object with id, value and extensions. The accessor "getRankingOrder" gives direct access to the value 7836 */ 7837 public PositiveIntType getRankingOrderElement() { 7838 if (this.rankingOrder == null) 7839 if (Configuration.errorOnAutoCreate()) 7840 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryComponent.rankingOrder"); 7841 else if (Configuration.doAutoCreate()) 7842 this.rankingOrder = new PositiveIntType(); // bb 7843 return this.rankingOrder; 7844 } 7845 7846 public boolean hasRankingOrderElement() { 7847 return this.rankingOrder != null && !this.rankingOrder.isEmpty(); 7848 } 7849 7850 public boolean hasRankingOrder() { 7851 return this.rankingOrder != null && !this.rankingOrder.isEmpty(); 7852 } 7853 7854 /** 7855 * @param value {@link #rankingOrder} (Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author.). This is the underlying object with id, value and extensions. The accessor "getRankingOrder" gives direct access to the value 7856 */ 7857 public CitationCitedArtifactContributorshipEntryComponent setRankingOrderElement(PositiveIntType value) { 7858 this.rankingOrder = value; 7859 return this; 7860 } 7861 7862 /** 7863 * @return Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author. 7864 */ 7865 public int getRankingOrder() { 7866 return this.rankingOrder == null || this.rankingOrder.isEmpty() ? 0 : this.rankingOrder.getValue(); 7867 } 7868 7869 /** 7870 * @param value Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author. 7871 */ 7872 public CitationCitedArtifactContributorshipEntryComponent setRankingOrder(int value) { 7873 if (this.rankingOrder == null) 7874 this.rankingOrder = new PositiveIntType(); 7875 this.rankingOrder.setValue(value); 7876 return this; 7877 } 7878 7879 protected void listChildren(List<Property> children) { 7880 super.listChildren(children); 7881 children.add(new Property("contributor", "Reference(Practitioner|Organization)", "The identity of the individual contributor.", 0, 1, contributor)); 7882 children.add(new Property("forenameInitials", "string", "For citation styles that use initials.", 0, 1, forenameInitials)); 7883 children.add(new Property("affiliation", "Reference(Organization|PractitionerRole)", "Organization affiliated with the contributor.", 0, java.lang.Integer.MAX_VALUE, affiliation)); 7884 children.add(new Property("contributionType", "CodeableConcept", "This element identifies the specific nature of an individual?s contribution with respect to the cited work.", 0, java.lang.Integer.MAX_VALUE, contributionType)); 7885 children.add(new Property("role", "CodeableConcept", "The role of the contributor (e.g. author, editor, reviewer, funder).", 0, 1, role)); 7886 children.add(new Property("contributionInstance", "", "Contributions with accounting for time or number.", 0, java.lang.Integer.MAX_VALUE, contributionInstance)); 7887 children.add(new Property("correspondingContact", "boolean", "Whether the contributor is the corresponding contributor for the role.", 0, 1, correspondingContact)); 7888 children.add(new Property("rankingOrder", "positiveInt", "Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author.", 0, 1, rankingOrder)); 7889 } 7890 7891 @Override 7892 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7893 switch (_hash) { 7894 case -1895276325: /*contributor*/ return new Property("contributor", "Reference(Practitioner|Organization)", "The identity of the individual contributor.", 0, 1, contributor); 7895 case -740521962: /*forenameInitials*/ return new Property("forenameInitials", "string", "For citation styles that use initials.", 0, 1, forenameInitials); 7896 case 2019918576: /*affiliation*/ return new Property("affiliation", "Reference(Organization|PractitionerRole)", "Organization affiliated with the contributor.", 0, java.lang.Integer.MAX_VALUE, affiliation); 7897 case -1600446614: /*contributionType*/ return new Property("contributionType", "CodeableConcept", "This element identifies the specific nature of an individual?s contribution with respect to the cited work.", 0, java.lang.Integer.MAX_VALUE, contributionType); 7898 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role of the contributor (e.g. author, editor, reviewer, funder).", 0, 1, role); 7899 case -547910459: /*contributionInstance*/ return new Property("contributionInstance", "", "Contributions with accounting for time or number.", 0, java.lang.Integer.MAX_VALUE, contributionInstance); 7900 case -1816008851: /*correspondingContact*/ return new Property("correspondingContact", "boolean", "Whether the contributor is the corresponding contributor for the role.", 0, 1, correspondingContact); 7901 case -762905416: /*rankingOrder*/ return new Property("rankingOrder", "positiveInt", "Provides a numerical ranking to represent the degree of contributorship relative to other contributors, such as 1 for first author and 2 for second author.", 0, 1, rankingOrder); 7902 default: return super.getNamedProperty(_hash, _name, _checkValid); 7903 } 7904 7905 } 7906 7907 @Override 7908 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7909 switch (hash) { 7910 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : new Base[] {this.contributor}; // Reference 7911 case -740521962: /*forenameInitials*/ return this.forenameInitials == null ? new Base[0] : new Base[] {this.forenameInitials}; // StringType 7912 case 2019918576: /*affiliation*/ return this.affiliation == null ? new Base[0] : this.affiliation.toArray(new Base[this.affiliation.size()]); // Reference 7913 case -1600446614: /*contributionType*/ return this.contributionType == null ? new Base[0] : this.contributionType.toArray(new Base[this.contributionType.size()]); // CodeableConcept 7914 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 7915 case -547910459: /*contributionInstance*/ return this.contributionInstance == null ? new Base[0] : this.contributionInstance.toArray(new Base[this.contributionInstance.size()]); // CitationCitedArtifactContributorshipEntryContributionInstanceComponent 7916 case -1816008851: /*correspondingContact*/ return this.correspondingContact == null ? new Base[0] : new Base[] {this.correspondingContact}; // BooleanType 7917 case -762905416: /*rankingOrder*/ return this.rankingOrder == null ? new Base[0] : new Base[] {this.rankingOrder}; // PositiveIntType 7918 default: return super.getProperty(hash, name, checkValid); 7919 } 7920 7921 } 7922 7923 @Override 7924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7925 switch (hash) { 7926 case -1895276325: // contributor 7927 this.contributor = TypeConvertor.castToReference(value); // Reference 7928 return value; 7929 case -740521962: // forenameInitials 7930 this.forenameInitials = TypeConvertor.castToString(value); // StringType 7931 return value; 7932 case 2019918576: // affiliation 7933 this.getAffiliation().add(TypeConvertor.castToReference(value)); // Reference 7934 return value; 7935 case -1600446614: // contributionType 7936 this.getContributionType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7937 return value; 7938 case 3506294: // role 7939 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7940 return value; 7941 case -547910459: // contributionInstance 7942 this.getContributionInstance().add((CitationCitedArtifactContributorshipEntryContributionInstanceComponent) value); // CitationCitedArtifactContributorshipEntryContributionInstanceComponent 7943 return value; 7944 case -1816008851: // correspondingContact 7945 this.correspondingContact = TypeConvertor.castToBoolean(value); // BooleanType 7946 return value; 7947 case -762905416: // rankingOrder 7948 this.rankingOrder = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7949 return value; 7950 default: return super.setProperty(hash, name, value); 7951 } 7952 7953 } 7954 7955 @Override 7956 public Base setProperty(String name, Base value) throws FHIRException { 7957 if (name.equals("contributor")) { 7958 this.contributor = TypeConvertor.castToReference(value); // Reference 7959 } else if (name.equals("forenameInitials")) { 7960 this.forenameInitials = TypeConvertor.castToString(value); // StringType 7961 } else if (name.equals("affiliation")) { 7962 this.getAffiliation().add(TypeConvertor.castToReference(value)); 7963 } else if (name.equals("contributionType")) { 7964 this.getContributionType().add(TypeConvertor.castToCodeableConcept(value)); 7965 } else if (name.equals("role")) { 7966 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7967 } else if (name.equals("contributionInstance")) { 7968 this.getContributionInstance().add((CitationCitedArtifactContributorshipEntryContributionInstanceComponent) value); 7969 } else if (name.equals("correspondingContact")) { 7970 this.correspondingContact = TypeConvertor.castToBoolean(value); // BooleanType 7971 } else if (name.equals("rankingOrder")) { 7972 this.rankingOrder = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7973 } else 7974 return super.setProperty(name, value); 7975 return value; 7976 } 7977 7978 @Override 7979 public void removeChild(String name, Base value) throws FHIRException { 7980 if (name.equals("contributor")) { 7981 this.contributor = null; 7982 } else if (name.equals("forenameInitials")) { 7983 this.forenameInitials = null; 7984 } else if (name.equals("affiliation")) { 7985 this.getAffiliation().remove(value); 7986 } else if (name.equals("contributionType")) { 7987 this.getContributionType().remove(value); 7988 } else if (name.equals("role")) { 7989 this.role = null; 7990 } else if (name.equals("contributionInstance")) { 7991 this.getContributionInstance().remove((CitationCitedArtifactContributorshipEntryContributionInstanceComponent) value); 7992 } else if (name.equals("correspondingContact")) { 7993 this.correspondingContact = null; 7994 } else if (name.equals("rankingOrder")) { 7995 this.rankingOrder = null; 7996 } else 7997 super.removeChild(name, value); 7998 7999 } 8000 8001 @Override 8002 public Base makeProperty(int hash, String name) throws FHIRException { 8003 switch (hash) { 8004 case -1895276325: return getContributor(); 8005 case -740521962: return getForenameInitialsElement(); 8006 case 2019918576: return addAffiliation(); 8007 case -1600446614: return addContributionType(); 8008 case 3506294: return getRole(); 8009 case -547910459: return addContributionInstance(); 8010 case -1816008851: return getCorrespondingContactElement(); 8011 case -762905416: return getRankingOrderElement(); 8012 default: return super.makeProperty(hash, name); 8013 } 8014 8015 } 8016 8017 @Override 8018 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8019 switch (hash) { 8020 case -1895276325: /*contributor*/ return new String[] {"Reference"}; 8021 case -740521962: /*forenameInitials*/ return new String[] {"string"}; 8022 case 2019918576: /*affiliation*/ return new String[] {"Reference"}; 8023 case -1600446614: /*contributionType*/ return new String[] {"CodeableConcept"}; 8024 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 8025 case -547910459: /*contributionInstance*/ return new String[] {}; 8026 case -1816008851: /*correspondingContact*/ return new String[] {"boolean"}; 8027 case -762905416: /*rankingOrder*/ return new String[] {"positiveInt"}; 8028 default: return super.getTypesForProperty(hash, name); 8029 } 8030 8031 } 8032 8033 @Override 8034 public Base addChild(String name) throws FHIRException { 8035 if (name.equals("contributor")) { 8036 this.contributor = new Reference(); 8037 return this.contributor; 8038 } 8039 else if (name.equals("forenameInitials")) { 8040 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.contributorship.entry.forenameInitials"); 8041 } 8042 else if (name.equals("affiliation")) { 8043 return addAffiliation(); 8044 } 8045 else if (name.equals("contributionType")) { 8046 return addContributionType(); 8047 } 8048 else if (name.equals("role")) { 8049 this.role = new CodeableConcept(); 8050 return this.role; 8051 } 8052 else if (name.equals("contributionInstance")) { 8053 return addContributionInstance(); 8054 } 8055 else if (name.equals("correspondingContact")) { 8056 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.contributorship.entry.correspondingContact"); 8057 } 8058 else if (name.equals("rankingOrder")) { 8059 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.contributorship.entry.rankingOrder"); 8060 } 8061 else 8062 return super.addChild(name); 8063 } 8064 8065 public CitationCitedArtifactContributorshipEntryComponent copy() { 8066 CitationCitedArtifactContributorshipEntryComponent dst = new CitationCitedArtifactContributorshipEntryComponent(); 8067 copyValues(dst); 8068 return dst; 8069 } 8070 8071 public void copyValues(CitationCitedArtifactContributorshipEntryComponent dst) { 8072 super.copyValues(dst); 8073 dst.contributor = contributor == null ? null : contributor.copy(); 8074 dst.forenameInitials = forenameInitials == null ? null : forenameInitials.copy(); 8075 if (affiliation != null) { 8076 dst.affiliation = new ArrayList<Reference>(); 8077 for (Reference i : affiliation) 8078 dst.affiliation.add(i.copy()); 8079 }; 8080 if (contributionType != null) { 8081 dst.contributionType = new ArrayList<CodeableConcept>(); 8082 for (CodeableConcept i : contributionType) 8083 dst.contributionType.add(i.copy()); 8084 }; 8085 dst.role = role == null ? null : role.copy(); 8086 if (contributionInstance != null) { 8087 dst.contributionInstance = new ArrayList<CitationCitedArtifactContributorshipEntryContributionInstanceComponent>(); 8088 for (CitationCitedArtifactContributorshipEntryContributionInstanceComponent i : contributionInstance) 8089 dst.contributionInstance.add(i.copy()); 8090 }; 8091 dst.correspondingContact = correspondingContact == null ? null : correspondingContact.copy(); 8092 dst.rankingOrder = rankingOrder == null ? null : rankingOrder.copy(); 8093 } 8094 8095 @Override 8096 public boolean equalsDeep(Base other_) { 8097 if (!super.equalsDeep(other_)) 8098 return false; 8099 if (!(other_ instanceof CitationCitedArtifactContributorshipEntryComponent)) 8100 return false; 8101 CitationCitedArtifactContributorshipEntryComponent o = (CitationCitedArtifactContributorshipEntryComponent) other_; 8102 return compareDeep(contributor, o.contributor, true) && compareDeep(forenameInitials, o.forenameInitials, true) 8103 && compareDeep(affiliation, o.affiliation, true) && compareDeep(contributionType, o.contributionType, true) 8104 && compareDeep(role, o.role, true) && compareDeep(contributionInstance, o.contributionInstance, true) 8105 && compareDeep(correspondingContact, o.correspondingContact, true) && compareDeep(rankingOrder, o.rankingOrder, true) 8106 ; 8107 } 8108 8109 @Override 8110 public boolean equalsShallow(Base other_) { 8111 if (!super.equalsShallow(other_)) 8112 return false; 8113 if (!(other_ instanceof CitationCitedArtifactContributorshipEntryComponent)) 8114 return false; 8115 CitationCitedArtifactContributorshipEntryComponent o = (CitationCitedArtifactContributorshipEntryComponent) other_; 8116 return compareValues(forenameInitials, o.forenameInitials, true) && compareValues(correspondingContact, o.correspondingContact, true) 8117 && compareValues(rankingOrder, o.rankingOrder, true); 8118 } 8119 8120 public boolean isEmpty() { 8121 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(contributor, forenameInitials 8122 , affiliation, contributionType, role, contributionInstance, correspondingContact 8123 , rankingOrder); 8124 } 8125 8126 public String fhirType() { 8127 return "Citation.citedArtifact.contributorship.entry"; 8128 8129 } 8130 8131 } 8132 8133 @Block() 8134 public static class CitationCitedArtifactContributorshipEntryContributionInstanceComponent extends BackboneElement implements IBaseBackboneElement { 8135 /** 8136 * The specific contribution. 8137 */ 8138 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 8139 @Description(shortDefinition="The specific contribution", formalDefinition="The specific contribution." ) 8140 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/artifact-contribution-instance-type") 8141 protected CodeableConcept type; 8142 8143 /** 8144 * The time that the contribution was made. 8145 */ 8146 @Child(name = "time", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 8147 @Description(shortDefinition="The time that the contribution was made", formalDefinition="The time that the contribution was made." ) 8148 protected DateTimeType time; 8149 8150 private static final long serialVersionUID = -196837729L; 8151 8152 /** 8153 * Constructor 8154 */ 8155 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent() { 8156 super(); 8157 } 8158 8159 /** 8160 * Constructor 8161 */ 8162 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent(CodeableConcept type) { 8163 super(); 8164 this.setType(type); 8165 } 8166 8167 /** 8168 * @return {@link #type} (The specific contribution.) 8169 */ 8170 public CodeableConcept getType() { 8171 if (this.type == null) 8172 if (Configuration.errorOnAutoCreate()) 8173 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryContributionInstanceComponent.type"); 8174 else if (Configuration.doAutoCreate()) 8175 this.type = new CodeableConcept(); // cc 8176 return this.type; 8177 } 8178 8179 public boolean hasType() { 8180 return this.type != null && !this.type.isEmpty(); 8181 } 8182 8183 /** 8184 * @param value {@link #type} (The specific contribution.) 8185 */ 8186 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent setType(CodeableConcept value) { 8187 this.type = value; 8188 return this; 8189 } 8190 8191 /** 8192 * @return {@link #time} (The time that the contribution was made.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 8193 */ 8194 public DateTimeType getTimeElement() { 8195 if (this.time == null) 8196 if (Configuration.errorOnAutoCreate()) 8197 throw new Error("Attempt to auto-create CitationCitedArtifactContributorshipEntryContributionInstanceComponent.time"); 8198 else if (Configuration.doAutoCreate()) 8199 this.time = new DateTimeType(); // bb 8200 return this.time; 8201 } 8202 8203 public boolean hasTimeElement() { 8204 return this.time != null && !this.time.isEmpty(); 8205 } 8206 8207 public boolean hasTime() { 8208 return this.time != null && !this.time.isEmpty(); 8209 } 8210 8211 /** 8212 * @param value {@link #time} (The time that the contribution was made.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 8213 */ 8214 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent setTimeElement(DateTimeType value) { 8215 this.time = value; 8216 return this; 8217 } 8218 8219 /** 8220 * @return The time that the contribution was made. 8221 */ 8222 public Date getTime() { 8223 return this.time == null ? null : this.time.getValue(); 8224 } 8225 8226 /** 8227 * @param value The time that the contribution was made. 8228 */ 8229 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent setTime(Date value) { 8230 if (value == null) 8231 this.time = null; 8232 else { 8233 if (this.time == null) 8234 this.time = new DateTimeType(); 8235 this.time.setValue(value); 8236 } 8237 return this; 8238 } 8239 8240 protected void listChildren(List<Property> children) { 8241 super.listChildren(children); 8242 children.add(new Property("type", "CodeableConcept", "The specific contribution.", 0, 1, type)); 8243 children.add(new Property("time", "dateTime", "The time that the contribution was made.", 0, 1, time)); 8244 } 8245 8246 @Override 8247 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8248 switch (_hash) { 8249 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The specific contribution.", 0, 1, type); 8250 case 3560141: /*time*/ return new Property("time", "dateTime", "The time that the contribution was made.", 0, 1, time); 8251 default: return super.getNamedProperty(_hash, _name, _checkValid); 8252 } 8253 8254 } 8255 8256 @Override 8257 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8258 switch (hash) { 8259 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8260 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DateTimeType 8261 default: return super.getProperty(hash, name, checkValid); 8262 } 8263 8264 } 8265 8266 @Override 8267 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8268 switch (hash) { 8269 case 3575610: // type 8270 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8271 return value; 8272 case 3560141: // time 8273 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 8274 return value; 8275 default: return super.setProperty(hash, name, value); 8276 } 8277 8278 } 8279 8280 @Override 8281 public Base setProperty(String name, Base value) throws FHIRException { 8282 if (name.equals("type")) { 8283 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8284 } else if (name.equals("time")) { 8285 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 8286 } else 8287 return super.setProperty(name, value); 8288 return value; 8289 } 8290 8291 @Override 8292 public void removeChild(String name, Base value) throws FHIRException { 8293 if (name.equals("type")) { 8294 this.type = null; 8295 } else if (name.equals("time")) { 8296 this.time = null; 8297 } else 8298 super.removeChild(name, value); 8299 8300 } 8301 8302 @Override 8303 public Base makeProperty(int hash, String name) throws FHIRException { 8304 switch (hash) { 8305 case 3575610: return getType(); 8306 case 3560141: return getTimeElement(); 8307 default: return super.makeProperty(hash, name); 8308 } 8309 8310 } 8311 8312 @Override 8313 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8314 switch (hash) { 8315 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8316 case 3560141: /*time*/ return new String[] {"dateTime"}; 8317 default: return super.getTypesForProperty(hash, name); 8318 } 8319 8320 } 8321 8322 @Override 8323 public Base addChild(String name) throws FHIRException { 8324 if (name.equals("type")) { 8325 this.type = new CodeableConcept(); 8326 return this.type; 8327 } 8328 else if (name.equals("time")) { 8329 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.contributorship.entry.contributionInstance.time"); 8330 } 8331 else 8332 return super.addChild(name); 8333 } 8334 8335 public CitationCitedArtifactContributorshipEntryContributionInstanceComponent copy() { 8336 CitationCitedArtifactContributorshipEntryContributionInstanceComponent dst = new CitationCitedArtifactContributorshipEntryContributionInstanceComponent(); 8337 copyValues(dst); 8338 return dst; 8339 } 8340 8341 public void copyValues(CitationCitedArtifactContributorshipEntryContributionInstanceComponent dst) { 8342 super.copyValues(dst); 8343 dst.type = type == null ? null : type.copy(); 8344 dst.time = time == null ? null : time.copy(); 8345 } 8346 8347 @Override 8348 public boolean equalsDeep(Base other_) { 8349 if (!super.equalsDeep(other_)) 8350 return false; 8351 if (!(other_ instanceof CitationCitedArtifactContributorshipEntryContributionInstanceComponent)) 8352 return false; 8353 CitationCitedArtifactContributorshipEntryContributionInstanceComponent o = (CitationCitedArtifactContributorshipEntryContributionInstanceComponent) other_; 8354 return compareDeep(type, o.type, true) && compareDeep(time, o.time, true); 8355 } 8356 8357 @Override 8358 public boolean equalsShallow(Base other_) { 8359 if (!super.equalsShallow(other_)) 8360 return false; 8361 if (!(other_ instanceof CitationCitedArtifactContributorshipEntryContributionInstanceComponent)) 8362 return false; 8363 CitationCitedArtifactContributorshipEntryContributionInstanceComponent o = (CitationCitedArtifactContributorshipEntryContributionInstanceComponent) other_; 8364 return compareValues(time, o.time, true); 8365 } 8366 8367 public boolean isEmpty() { 8368 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, time); 8369 } 8370 8371 public String fhirType() { 8372 return "Citation.citedArtifact.contributorship.entry.contributionInstance"; 8373 8374 } 8375 8376 } 8377 8378 @Block() 8379 public static class ContributorshipSummaryComponent extends BackboneElement implements IBaseBackboneElement { 8380 /** 8381 * Used most commonly to express an author list or a contributorship statement. 8382 */ 8383 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 8384 @Description(shortDefinition="Such as author list, contributorship statement, funding statement, acknowledgements statement, or conflicts of interest statement", formalDefinition="Used most commonly to express an author list or a contributorship statement." ) 8385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contributor-summary-type") 8386 protected CodeableConcept type; 8387 8388 /** 8389 * The format for the display string, such as author last name with first letter capitalized followed by forename initials. 8390 */ 8391 @Child(name = "style", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 8392 @Description(shortDefinition="The format for the display string", formalDefinition="The format for the display string, such as author last name with first letter capitalized followed by forename initials." ) 8393 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contributor-summary-style") 8394 protected CodeableConcept style; 8395 8396 /** 8397 * Used to code the producer or rule for creating the display string. 8398 */ 8399 @Child(name = "source", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 8400 @Description(shortDefinition="Used to code the producer or rule for creating the display string", formalDefinition="Used to code the producer or rule for creating the display string." ) 8401 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contributor-summary-source") 8402 protected CodeableConcept source; 8403 8404 /** 8405 * The display string for the author list, contributor list, or contributorship statement. 8406 */ 8407 @Child(name = "value", type = {MarkdownType.class}, order=4, min=1, max=1, modifier=false, summary=false) 8408 @Description(shortDefinition="The display string for the author list, contributor list, or contributorship statement", formalDefinition="The display string for the author list, contributor list, or contributorship statement." ) 8409 protected MarkdownType value; 8410 8411 private static final long serialVersionUID = 1353383781L; 8412 8413 /** 8414 * Constructor 8415 */ 8416 public ContributorshipSummaryComponent() { 8417 super(); 8418 } 8419 8420 /** 8421 * Constructor 8422 */ 8423 public ContributorshipSummaryComponent(String value) { 8424 super(); 8425 this.setValue(value); 8426 } 8427 8428 /** 8429 * @return {@link #type} (Used most commonly to express an author list or a contributorship statement.) 8430 */ 8431 public CodeableConcept getType() { 8432 if (this.type == null) 8433 if (Configuration.errorOnAutoCreate()) 8434 throw new Error("Attempt to auto-create ContributorshipSummaryComponent.type"); 8435 else if (Configuration.doAutoCreate()) 8436 this.type = new CodeableConcept(); // cc 8437 return this.type; 8438 } 8439 8440 public boolean hasType() { 8441 return this.type != null && !this.type.isEmpty(); 8442 } 8443 8444 /** 8445 * @param value {@link #type} (Used most commonly to express an author list or a contributorship statement.) 8446 */ 8447 public ContributorshipSummaryComponent setType(CodeableConcept value) { 8448 this.type = value; 8449 return this; 8450 } 8451 8452 /** 8453 * @return {@link #style} (The format for the display string, such as author last name with first letter capitalized followed by forename initials.) 8454 */ 8455 public CodeableConcept getStyle() { 8456 if (this.style == null) 8457 if (Configuration.errorOnAutoCreate()) 8458 throw new Error("Attempt to auto-create ContributorshipSummaryComponent.style"); 8459 else if (Configuration.doAutoCreate()) 8460 this.style = new CodeableConcept(); // cc 8461 return this.style; 8462 } 8463 8464 public boolean hasStyle() { 8465 return this.style != null && !this.style.isEmpty(); 8466 } 8467 8468 /** 8469 * @param value {@link #style} (The format for the display string, such as author last name with first letter capitalized followed by forename initials.) 8470 */ 8471 public ContributorshipSummaryComponent setStyle(CodeableConcept value) { 8472 this.style = value; 8473 return this; 8474 } 8475 8476 /** 8477 * @return {@link #source} (Used to code the producer or rule for creating the display string.) 8478 */ 8479 public CodeableConcept getSource() { 8480 if (this.source == null) 8481 if (Configuration.errorOnAutoCreate()) 8482 throw new Error("Attempt to auto-create ContributorshipSummaryComponent.source"); 8483 else if (Configuration.doAutoCreate()) 8484 this.source = new CodeableConcept(); // cc 8485 return this.source; 8486 } 8487 8488 public boolean hasSource() { 8489 return this.source != null && !this.source.isEmpty(); 8490 } 8491 8492 /** 8493 * @param value {@link #source} (Used to code the producer or rule for creating the display string.) 8494 */ 8495 public ContributorshipSummaryComponent setSource(CodeableConcept value) { 8496 this.source = value; 8497 return this; 8498 } 8499 8500 /** 8501 * @return {@link #value} (The display string for the author list, contributor list, or contributorship statement.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 8502 */ 8503 public MarkdownType getValueElement() { 8504 if (this.value == null) 8505 if (Configuration.errorOnAutoCreate()) 8506 throw new Error("Attempt to auto-create ContributorshipSummaryComponent.value"); 8507 else if (Configuration.doAutoCreate()) 8508 this.value = new MarkdownType(); // bb 8509 return this.value; 8510 } 8511 8512 public boolean hasValueElement() { 8513 return this.value != null && !this.value.isEmpty(); 8514 } 8515 8516 public boolean hasValue() { 8517 return this.value != null && !this.value.isEmpty(); 8518 } 8519 8520 /** 8521 * @param value {@link #value} (The display string for the author list, contributor list, or contributorship statement.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 8522 */ 8523 public ContributorshipSummaryComponent setValueElement(MarkdownType value) { 8524 this.value = value; 8525 return this; 8526 } 8527 8528 /** 8529 * @return The display string for the author list, contributor list, or contributorship statement. 8530 */ 8531 public String getValue() { 8532 return this.value == null ? null : this.value.getValue(); 8533 } 8534 8535 /** 8536 * @param value The display string for the author list, contributor list, or contributorship statement. 8537 */ 8538 public ContributorshipSummaryComponent setValue(String value) { 8539 if (this.value == null) 8540 this.value = new MarkdownType(); 8541 this.value.setValue(value); 8542 return this; 8543 } 8544 8545 protected void listChildren(List<Property> children) { 8546 super.listChildren(children); 8547 children.add(new Property("type", "CodeableConcept", "Used most commonly to express an author list or a contributorship statement.", 0, 1, type)); 8548 children.add(new Property("style", "CodeableConcept", "The format for the display string, such as author last name with first letter capitalized followed by forename initials.", 0, 1, style)); 8549 children.add(new Property("source", "CodeableConcept", "Used to code the producer or rule for creating the display string.", 0, 1, source)); 8550 children.add(new Property("value", "markdown", "The display string for the author list, contributor list, or contributorship statement.", 0, 1, value)); 8551 } 8552 8553 @Override 8554 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8555 switch (_hash) { 8556 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Used most commonly to express an author list or a contributorship statement.", 0, 1, type); 8557 case 109780401: /*style*/ return new Property("style", "CodeableConcept", "The format for the display string, such as author last name with first letter capitalized followed by forename initials.", 0, 1, style); 8558 case -896505829: /*source*/ return new Property("source", "CodeableConcept", "Used to code the producer or rule for creating the display string.", 0, 1, source); 8559 case 111972721: /*value*/ return new Property("value", "markdown", "The display string for the author list, contributor list, or contributorship statement.", 0, 1, value); 8560 default: return super.getNamedProperty(_hash, _name, _checkValid); 8561 } 8562 8563 } 8564 8565 @Override 8566 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8567 switch (hash) { 8568 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8569 case 109780401: /*style*/ return this.style == null ? new Base[0] : new Base[] {this.style}; // CodeableConcept 8570 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // CodeableConcept 8571 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // MarkdownType 8572 default: return super.getProperty(hash, name, checkValid); 8573 } 8574 8575 } 8576 8577 @Override 8578 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8579 switch (hash) { 8580 case 3575610: // type 8581 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8582 return value; 8583 case 109780401: // style 8584 this.style = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8585 return value; 8586 case -896505829: // source 8587 this.source = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8588 return value; 8589 case 111972721: // value 8590 this.value = TypeConvertor.castToMarkdown(value); // MarkdownType 8591 return value; 8592 default: return super.setProperty(hash, name, value); 8593 } 8594 8595 } 8596 8597 @Override 8598 public Base setProperty(String name, Base value) throws FHIRException { 8599 if (name.equals("type")) { 8600 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8601 } else if (name.equals("style")) { 8602 this.style = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8603 } else if (name.equals("source")) { 8604 this.source = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8605 } else if (name.equals("value")) { 8606 this.value = TypeConvertor.castToMarkdown(value); // MarkdownType 8607 } else 8608 return super.setProperty(name, value); 8609 return value; 8610 } 8611 8612 @Override 8613 public void removeChild(String name, Base value) throws FHIRException { 8614 if (name.equals("type")) { 8615 this.type = null; 8616 } else if (name.equals("style")) { 8617 this.style = null; 8618 } else if (name.equals("source")) { 8619 this.source = null; 8620 } else if (name.equals("value")) { 8621 this.value = null; 8622 } else 8623 super.removeChild(name, value); 8624 8625 } 8626 8627 @Override 8628 public Base makeProperty(int hash, String name) throws FHIRException { 8629 switch (hash) { 8630 case 3575610: return getType(); 8631 case 109780401: return getStyle(); 8632 case -896505829: return getSource(); 8633 case 111972721: return getValueElement(); 8634 default: return super.makeProperty(hash, name); 8635 } 8636 8637 } 8638 8639 @Override 8640 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8641 switch (hash) { 8642 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8643 case 109780401: /*style*/ return new String[] {"CodeableConcept"}; 8644 case -896505829: /*source*/ return new String[] {"CodeableConcept"}; 8645 case 111972721: /*value*/ return new String[] {"markdown"}; 8646 default: return super.getTypesForProperty(hash, name); 8647 } 8648 8649 } 8650 8651 @Override 8652 public Base addChild(String name) throws FHIRException { 8653 if (name.equals("type")) { 8654 this.type = new CodeableConcept(); 8655 return this.type; 8656 } 8657 else if (name.equals("style")) { 8658 this.style = new CodeableConcept(); 8659 return this.style; 8660 } 8661 else if (name.equals("source")) { 8662 this.source = new CodeableConcept(); 8663 return this.source; 8664 } 8665 else if (name.equals("value")) { 8666 throw new FHIRException("Cannot call addChild on a singleton property Citation.citedArtifact.contributorship.summary.value"); 8667 } 8668 else 8669 return super.addChild(name); 8670 } 8671 8672 public ContributorshipSummaryComponent copy() { 8673 ContributorshipSummaryComponent dst = new ContributorshipSummaryComponent(); 8674 copyValues(dst); 8675 return dst; 8676 } 8677 8678 public void copyValues(ContributorshipSummaryComponent dst) { 8679 super.copyValues(dst); 8680 dst.type = type == null ? null : type.copy(); 8681 dst.style = style == null ? null : style.copy(); 8682 dst.source = source == null ? null : source.copy(); 8683 dst.value = value == null ? null : value.copy(); 8684 } 8685 8686 @Override 8687 public boolean equalsDeep(Base other_) { 8688 if (!super.equalsDeep(other_)) 8689 return false; 8690 if (!(other_ instanceof ContributorshipSummaryComponent)) 8691 return false; 8692 ContributorshipSummaryComponent o = (ContributorshipSummaryComponent) other_; 8693 return compareDeep(type, o.type, true) && compareDeep(style, o.style, true) && compareDeep(source, o.source, true) 8694 && compareDeep(value, o.value, true); 8695 } 8696 8697 @Override 8698 public boolean equalsShallow(Base other_) { 8699 if (!super.equalsShallow(other_)) 8700 return false; 8701 if (!(other_ instanceof ContributorshipSummaryComponent)) 8702 return false; 8703 ContributorshipSummaryComponent o = (ContributorshipSummaryComponent) other_; 8704 return compareValues(value, o.value, true); 8705 } 8706 8707 public boolean isEmpty() { 8708 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, style, source, value 8709 ); 8710 } 8711 8712 public String fhirType() { 8713 return "Citation.citedArtifact.contributorship.summary"; 8714 8715 } 8716 8717 } 8718 8719 /** 8720 * An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 8721 */ 8722 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 8723 @Description(shortDefinition="Canonical identifier for this citation record, represented as a globally unique URI", formalDefinition="An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers." ) 8724 protected UriType url; 8725 8726 /** 8727 * A formal identifier that is used to identify this citation record when it is represented in other formats, or referenced in a specification, model, design or an instance. 8728 */ 8729 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8730 @Description(shortDefinition="Identifier for the citation record itself", formalDefinition="A formal identifier that is used to identify this citation record when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 8731 protected List<Identifier> identifier; 8732 8733 /** 8734 * The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 8735 */ 8736 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 8737 @Description(shortDefinition="Business version of the citation record", formalDefinition="The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 8738 protected StringType version; 8739 8740 /** 8741 * Indicates the mechanism used to compare versions to determine which is more current. 8742 */ 8743 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 8744 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 8745 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 8746 protected DataType versionAlgorithm; 8747 8748 /** 8749 * A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation. 8750 */ 8751 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 8752 @Description(shortDefinition="Name for this citation record (computer friendly)", formalDefinition="A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 8753 protected StringType name; 8754 8755 /** 8756 * A short, descriptive, user-friendly title for the citation record. 8757 */ 8758 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 8759 @Description(shortDefinition="Name for this citation record (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the citation record." ) 8760 protected StringType title; 8761 8762 /** 8763 * The status of this summary. Enables tracking the life-cycle of the content. 8764 */ 8765 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 8766 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this summary. Enables tracking the life-cycle of the content." ) 8767 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 8768 protected Enumeration<PublicationStatus> status; 8769 8770 /** 8771 * A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 8772 */ 8773 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 8774 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 8775 protected BooleanType experimental; 8776 8777 /** 8778 * The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes. 8779 */ 8780 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 8781 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes." ) 8782 protected DateTimeType date; 8783 8784 /** 8785 * The name of the organization or individual that published the citation record. 8786 */ 8787 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 8788 @Description(shortDefinition="The publisher of the citation record, not the publisher of the article or artifact being cited", formalDefinition="The name of the organization or individual that published the citation record." ) 8789 protected StringType publisher; 8790 8791 /** 8792 * Contact details to assist a user in finding and communicating with the publisher. 8793 */ 8794 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8795 @Description(shortDefinition="Contact details for the publisher of the citation record", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 8796 protected List<ContactDetail> contact; 8797 8798 /** 8799 * A free text natural language description of the citation from a consumer's perspective. 8800 */ 8801 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 8802 @Description(shortDefinition="Natural language description of the citation", formalDefinition="A free text natural language description of the citation from a consumer's perspective." ) 8803 protected MarkdownType description; 8804 8805 /** 8806 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate citation record instances. 8807 */ 8808 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8809 @Description(shortDefinition="The context that the citation record content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate citation record instances." ) 8810 protected List<UsageContext> useContext; 8811 8812 /** 8813 * A legal or geographic region in which the citation record is intended to be used. 8814 */ 8815 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8816 @Description(shortDefinition="Intended jurisdiction for citation record (if applicable)", formalDefinition="A legal or geographic region in which the citation record is intended to be used." ) 8817 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 8818 protected List<CodeableConcept> jurisdiction; 8819 8820 /** 8821 * Explanation of why this citation is needed and why it has been designed as it has. 8822 */ 8823 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 8824 @Description(shortDefinition="Why this citation is defined", formalDefinition="Explanation of why this citation is needed and why it has been designed as it has." ) 8825 protected MarkdownType purpose; 8826 8827 /** 8828 * Use and/or publishing restrictions for the citation record, not for the cited artifact. 8829 */ 8830 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 8831 @Description(shortDefinition="Use and/or publishing restrictions for the citation record, not for the cited artifact", formalDefinition="Use and/or publishing restrictions for the citation record, not for the cited artifact." ) 8832 protected MarkdownType copyright; 8833 8834 /** 8835 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 8836 */ 8837 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 8838 @Description(shortDefinition="Copyright holder and year(s) for the ciation record, not for the cited artifact", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 8839 protected StringType copyrightLabel; 8840 8841 /** 8842 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 8843 */ 8844 @Child(name = "approvalDate", type = {DateType.class}, order=17, min=0, max=1, modifier=false, summary=false) 8845 @Description(shortDefinition="When the citation record was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 8846 protected DateType approvalDate; 8847 8848 /** 8849 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 8850 */ 8851 @Child(name = "lastReviewDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 8852 @Description(shortDefinition="When the citation record was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 8853 protected DateType lastReviewDate; 8854 8855 /** 8856 * The period during which the citation record content was or is planned to be in active use. 8857 */ 8858 @Child(name = "effectivePeriod", type = {Period.class}, order=19, min=0, max=1, modifier=false, summary=true) 8859 @Description(shortDefinition="When the citation record is expected to be used", formalDefinition="The period during which the citation record content was or is planned to be in active use." ) 8860 protected Period effectivePeriod; 8861 8862 /** 8863 * Who authored or created the citation record. 8864 */ 8865 @Child(name = "author", type = {ContactDetail.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8866 @Description(shortDefinition="Who authored the citation record", formalDefinition="Who authored or created the citation record." ) 8867 protected List<ContactDetail> author; 8868 8869 /** 8870 * Who edited or revised the citation record. 8871 */ 8872 @Child(name = "editor", type = {ContactDetail.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8873 @Description(shortDefinition="Who edited the citation record", formalDefinition="Who edited or revised the citation record." ) 8874 protected List<ContactDetail> editor; 8875 8876 /** 8877 * Who reviewed the citation record. 8878 */ 8879 @Child(name = "reviewer", type = {ContactDetail.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8880 @Description(shortDefinition="Who reviewed the citation record", formalDefinition="Who reviewed the citation record." ) 8881 protected List<ContactDetail> reviewer; 8882 8883 /** 8884 * Who endorsed the citation record. 8885 */ 8886 @Child(name = "endorser", type = {ContactDetail.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8887 @Description(shortDefinition="Who endorsed the citation record", formalDefinition="Who endorsed the citation record." ) 8888 protected List<ContactDetail> endorser; 8889 8890 /** 8891 * A human-readable display of key concepts to represent the citation. 8892 */ 8893 @Child(name = "summary", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8894 @Description(shortDefinition="A human-readable display of key concepts to represent the citation", formalDefinition="A human-readable display of key concepts to represent the citation." ) 8895 protected List<CitationSummaryComponent> summary; 8896 8897 /** 8898 * The assignment to an organizing scheme. 8899 */ 8900 @Child(name = "classification", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8901 @Description(shortDefinition="The assignment to an organizing scheme", formalDefinition="The assignment to an organizing scheme." ) 8902 protected List<CitationClassificationComponent> classification; 8903 8904 /** 8905 * Used for general notes and annotations not coded elsewhere. 8906 */ 8907 @Child(name = "note", type = {Annotation.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8908 @Description(shortDefinition="Used for general notes and annotations not coded elsewhere", formalDefinition="Used for general notes and annotations not coded elsewhere." ) 8909 protected List<Annotation> note; 8910 8911 /** 8912 * The status of the citation record. 8913 */ 8914 @Child(name = "currentState", type = {CodeableConcept.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8915 @Description(shortDefinition="The status of the citation record", formalDefinition="The status of the citation record." ) 8916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-status-type") 8917 protected List<CodeableConcept> currentState; 8918 8919 /** 8920 * The state or status of the citation record paired with an effective date or period for that state. 8921 */ 8922 @Child(name = "statusDate", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8923 @Description(shortDefinition="An effective date or period for a status of the citation record", formalDefinition="The state or status of the citation record paired with an effective date or period for that state." ) 8924 protected List<CitationStatusDateComponent> statusDate; 8925 8926 /** 8927 * Artifact related to the citation record. 8928 */ 8929 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8930 @Description(shortDefinition="Artifact related to the citation record", formalDefinition="Artifact related to the citation record." ) 8931 protected List<RelatedArtifact> relatedArtifact; 8932 8933 /** 8934 * The article or artifact being described. 8935 */ 8936 @Child(name = "citedArtifact", type = {}, order=30, min=0, max=1, modifier=false, summary=false) 8937 @Description(shortDefinition="The article or artifact being described", formalDefinition="The article or artifact being described." ) 8938 protected CitationCitedArtifactComponent citedArtifact; 8939 8940 private static final long serialVersionUID = 717016163L; 8941 8942 /** 8943 * Constructor 8944 */ 8945 public Citation() { 8946 super(); 8947 } 8948 8949 /** 8950 * Constructor 8951 */ 8952 public Citation(PublicationStatus status) { 8953 super(); 8954 this.setStatus(status); 8955 } 8956 8957 /** 8958 * @return {@link #url} (An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 8959 */ 8960 public UriType getUrlElement() { 8961 if (this.url == null) 8962 if (Configuration.errorOnAutoCreate()) 8963 throw new Error("Attempt to auto-create Citation.url"); 8964 else if (Configuration.doAutoCreate()) 8965 this.url = new UriType(); // bb 8966 return this.url; 8967 } 8968 8969 public boolean hasUrlElement() { 8970 return this.url != null && !this.url.isEmpty(); 8971 } 8972 8973 public boolean hasUrl() { 8974 return this.url != null && !this.url.isEmpty(); 8975 } 8976 8977 /** 8978 * @param value {@link #url} (An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 8979 */ 8980 public Citation setUrlElement(UriType value) { 8981 this.url = value; 8982 return this; 8983 } 8984 8985 /** 8986 * @return An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 8987 */ 8988 public String getUrl() { 8989 return this.url == null ? null : this.url.getValue(); 8990 } 8991 8992 /** 8993 * @param value An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 8994 */ 8995 public Citation setUrl(String value) { 8996 if (Utilities.noString(value)) 8997 this.url = null; 8998 else { 8999 if (this.url == null) 9000 this.url = new UriType(); 9001 this.url.setValue(value); 9002 } 9003 return this; 9004 } 9005 9006 /** 9007 * @return {@link #identifier} (A formal identifier that is used to identify this citation record when it is represented in other formats, or referenced in a specification, model, design or an instance.) 9008 */ 9009 public List<Identifier> getIdentifier() { 9010 if (this.identifier == null) 9011 this.identifier = new ArrayList<Identifier>(); 9012 return this.identifier; 9013 } 9014 9015 /** 9016 * @return Returns a reference to <code>this</code> for easy method chaining 9017 */ 9018 public Citation setIdentifier(List<Identifier> theIdentifier) { 9019 this.identifier = theIdentifier; 9020 return this; 9021 } 9022 9023 public boolean hasIdentifier() { 9024 if (this.identifier == null) 9025 return false; 9026 for (Identifier item : this.identifier) 9027 if (!item.isEmpty()) 9028 return true; 9029 return false; 9030 } 9031 9032 public Identifier addIdentifier() { //3 9033 Identifier t = new Identifier(); 9034 if (this.identifier == null) 9035 this.identifier = new ArrayList<Identifier>(); 9036 this.identifier.add(t); 9037 return t; 9038 } 9039 9040 public Citation addIdentifier(Identifier t) { //3 9041 if (t == null) 9042 return this; 9043 if (this.identifier == null) 9044 this.identifier = new ArrayList<Identifier>(); 9045 this.identifier.add(t); 9046 return this; 9047 } 9048 9049 /** 9050 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 9051 */ 9052 public Identifier getIdentifierFirstRep() { 9053 if (getIdentifier().isEmpty()) { 9054 addIdentifier(); 9055 } 9056 return getIdentifier().get(0); 9057 } 9058 9059 /** 9060 * @return {@link #version} (The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 9061 */ 9062 public StringType getVersionElement() { 9063 if (this.version == null) 9064 if (Configuration.errorOnAutoCreate()) 9065 throw new Error("Attempt to auto-create Citation.version"); 9066 else if (Configuration.doAutoCreate()) 9067 this.version = new StringType(); // bb 9068 return this.version; 9069 } 9070 9071 public boolean hasVersionElement() { 9072 return this.version != null && !this.version.isEmpty(); 9073 } 9074 9075 public boolean hasVersion() { 9076 return this.version != null && !this.version.isEmpty(); 9077 } 9078 9079 /** 9080 * @param value {@link #version} (The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 9081 */ 9082 public Citation setVersionElement(StringType value) { 9083 this.version = value; 9084 return this; 9085 } 9086 9087 /** 9088 * @return The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 9089 */ 9090 public String getVersion() { 9091 return this.version == null ? null : this.version.getValue(); 9092 } 9093 9094 /** 9095 * @param value The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 9096 */ 9097 public Citation setVersion(String value) { 9098 if (Utilities.noString(value)) 9099 this.version = null; 9100 else { 9101 if (this.version == null) 9102 this.version = new StringType(); 9103 this.version.setValue(value); 9104 } 9105 return this; 9106 } 9107 9108 /** 9109 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 9110 */ 9111 public DataType getVersionAlgorithm() { 9112 return this.versionAlgorithm; 9113 } 9114 9115 /** 9116 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 9117 */ 9118 public StringType getVersionAlgorithmStringType() throws FHIRException { 9119 if (this.versionAlgorithm == null) 9120 this.versionAlgorithm = new StringType(); 9121 if (!(this.versionAlgorithm instanceof StringType)) 9122 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 9123 return (StringType) this.versionAlgorithm; 9124 } 9125 9126 public boolean hasVersionAlgorithmStringType() { 9127 return this != null && this.versionAlgorithm instanceof StringType; 9128 } 9129 9130 /** 9131 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 9132 */ 9133 public Coding getVersionAlgorithmCoding() throws FHIRException { 9134 if (this.versionAlgorithm == null) 9135 this.versionAlgorithm = new Coding(); 9136 if (!(this.versionAlgorithm instanceof Coding)) 9137 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 9138 return (Coding) this.versionAlgorithm; 9139 } 9140 9141 public boolean hasVersionAlgorithmCoding() { 9142 return this != null && this.versionAlgorithm instanceof Coding; 9143 } 9144 9145 public boolean hasVersionAlgorithm() { 9146 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 9147 } 9148 9149 /** 9150 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 9151 */ 9152 public Citation setVersionAlgorithm(DataType value) { 9153 if (value != null && !(value instanceof StringType || value instanceof Coding)) 9154 throw new FHIRException("Not the right type for Citation.versionAlgorithm[x]: "+value.fhirType()); 9155 this.versionAlgorithm = value; 9156 return this; 9157 } 9158 9159 /** 9160 * @return {@link #name} (A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9161 */ 9162 public StringType getNameElement() { 9163 if (this.name == null) 9164 if (Configuration.errorOnAutoCreate()) 9165 throw new Error("Attempt to auto-create Citation.name"); 9166 else if (Configuration.doAutoCreate()) 9167 this.name = new StringType(); // bb 9168 return this.name; 9169 } 9170 9171 public boolean hasNameElement() { 9172 return this.name != null && !this.name.isEmpty(); 9173 } 9174 9175 public boolean hasName() { 9176 return this.name != null && !this.name.isEmpty(); 9177 } 9178 9179 /** 9180 * @param value {@link #name} (A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9181 */ 9182 public Citation setNameElement(StringType value) { 9183 this.name = value; 9184 return this; 9185 } 9186 9187 /** 9188 * @return A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation. 9189 */ 9190 public String getName() { 9191 return this.name == null ? null : this.name.getValue(); 9192 } 9193 9194 /** 9195 * @param value A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation. 9196 */ 9197 public Citation setName(String value) { 9198 if (Utilities.noString(value)) 9199 this.name = null; 9200 else { 9201 if (this.name == null) 9202 this.name = new StringType(); 9203 this.name.setValue(value); 9204 } 9205 return this; 9206 } 9207 9208 /** 9209 * @return {@link #title} (A short, descriptive, user-friendly title for the citation record.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 9210 */ 9211 public StringType getTitleElement() { 9212 if (this.title == null) 9213 if (Configuration.errorOnAutoCreate()) 9214 throw new Error("Attempt to auto-create Citation.title"); 9215 else if (Configuration.doAutoCreate()) 9216 this.title = new StringType(); // bb 9217 return this.title; 9218 } 9219 9220 public boolean hasTitleElement() { 9221 return this.title != null && !this.title.isEmpty(); 9222 } 9223 9224 public boolean hasTitle() { 9225 return this.title != null && !this.title.isEmpty(); 9226 } 9227 9228 /** 9229 * @param value {@link #title} (A short, descriptive, user-friendly title for the citation record.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 9230 */ 9231 public Citation setTitleElement(StringType value) { 9232 this.title = value; 9233 return this; 9234 } 9235 9236 /** 9237 * @return A short, descriptive, user-friendly title for the citation record. 9238 */ 9239 public String getTitle() { 9240 return this.title == null ? null : this.title.getValue(); 9241 } 9242 9243 /** 9244 * @param value A short, descriptive, user-friendly title for the citation record. 9245 */ 9246 public Citation setTitle(String value) { 9247 if (Utilities.noString(value)) 9248 this.title = null; 9249 else { 9250 if (this.title == null) 9251 this.title = new StringType(); 9252 this.title.setValue(value); 9253 } 9254 return this; 9255 } 9256 9257 /** 9258 * @return {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 9259 */ 9260 public Enumeration<PublicationStatus> getStatusElement() { 9261 if (this.status == null) 9262 if (Configuration.errorOnAutoCreate()) 9263 throw new Error("Attempt to auto-create Citation.status"); 9264 else if (Configuration.doAutoCreate()) 9265 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 9266 return this.status; 9267 } 9268 9269 public boolean hasStatusElement() { 9270 return this.status != null && !this.status.isEmpty(); 9271 } 9272 9273 public boolean hasStatus() { 9274 return this.status != null && !this.status.isEmpty(); 9275 } 9276 9277 /** 9278 * @param value {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 9279 */ 9280 public Citation setStatusElement(Enumeration<PublicationStatus> value) { 9281 this.status = value; 9282 return this; 9283 } 9284 9285 /** 9286 * @return The status of this summary. Enables tracking the life-cycle of the content. 9287 */ 9288 public PublicationStatus getStatus() { 9289 return this.status == null ? null : this.status.getValue(); 9290 } 9291 9292 /** 9293 * @param value The status of this summary. Enables tracking the life-cycle of the content. 9294 */ 9295 public Citation setStatus(PublicationStatus value) { 9296 if (this.status == null) 9297 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 9298 this.status.setValue(value); 9299 return this; 9300 } 9301 9302 /** 9303 * @return {@link #experimental} (A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 9304 */ 9305 public BooleanType getExperimentalElement() { 9306 if (this.experimental == null) 9307 if (Configuration.errorOnAutoCreate()) 9308 throw new Error("Attempt to auto-create Citation.experimental"); 9309 else if (Configuration.doAutoCreate()) 9310 this.experimental = new BooleanType(); // bb 9311 return this.experimental; 9312 } 9313 9314 public boolean hasExperimentalElement() { 9315 return this.experimental != null && !this.experimental.isEmpty(); 9316 } 9317 9318 public boolean hasExperimental() { 9319 return this.experimental != null && !this.experimental.isEmpty(); 9320 } 9321 9322 /** 9323 * @param value {@link #experimental} (A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 9324 */ 9325 public Citation setExperimentalElement(BooleanType value) { 9326 this.experimental = value; 9327 return this; 9328 } 9329 9330 /** 9331 * @return A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 9332 */ 9333 public boolean getExperimental() { 9334 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 9335 } 9336 9337 /** 9338 * @param value A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 9339 */ 9340 public Citation setExperimental(boolean value) { 9341 if (this.experimental == null) 9342 this.experimental = new BooleanType(); 9343 this.experimental.setValue(value); 9344 return this; 9345 } 9346 9347 /** 9348 * @return {@link #date} (The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 9349 */ 9350 public DateTimeType getDateElement() { 9351 if (this.date == null) 9352 if (Configuration.errorOnAutoCreate()) 9353 throw new Error("Attempt to auto-create Citation.date"); 9354 else if (Configuration.doAutoCreate()) 9355 this.date = new DateTimeType(); // bb 9356 return this.date; 9357 } 9358 9359 public boolean hasDateElement() { 9360 return this.date != null && !this.date.isEmpty(); 9361 } 9362 9363 public boolean hasDate() { 9364 return this.date != null && !this.date.isEmpty(); 9365 } 9366 9367 /** 9368 * @param value {@link #date} (The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 9369 */ 9370 public Citation setDateElement(DateTimeType value) { 9371 this.date = value; 9372 return this; 9373 } 9374 9375 /** 9376 * @return The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes. 9377 */ 9378 public Date getDate() { 9379 return this.date == null ? null : this.date.getValue(); 9380 } 9381 9382 /** 9383 * @param value The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes. 9384 */ 9385 public Citation setDate(Date value) { 9386 if (value == null) 9387 this.date = null; 9388 else { 9389 if (this.date == null) 9390 this.date = new DateTimeType(); 9391 this.date.setValue(value); 9392 } 9393 return this; 9394 } 9395 9396 /** 9397 * @return {@link #publisher} (The name of the organization or individual that published the citation record.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 9398 */ 9399 public StringType getPublisherElement() { 9400 if (this.publisher == null) 9401 if (Configuration.errorOnAutoCreate()) 9402 throw new Error("Attempt to auto-create Citation.publisher"); 9403 else if (Configuration.doAutoCreate()) 9404 this.publisher = new StringType(); // bb 9405 return this.publisher; 9406 } 9407 9408 public boolean hasPublisherElement() { 9409 return this.publisher != null && !this.publisher.isEmpty(); 9410 } 9411 9412 public boolean hasPublisher() { 9413 return this.publisher != null && !this.publisher.isEmpty(); 9414 } 9415 9416 /** 9417 * @param value {@link #publisher} (The name of the organization or individual that published the citation record.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 9418 */ 9419 public Citation setPublisherElement(StringType value) { 9420 this.publisher = value; 9421 return this; 9422 } 9423 9424 /** 9425 * @return The name of the organization or individual that published the citation record. 9426 */ 9427 public String getPublisher() { 9428 return this.publisher == null ? null : this.publisher.getValue(); 9429 } 9430 9431 /** 9432 * @param value The name of the organization or individual that published the citation record. 9433 */ 9434 public Citation setPublisher(String value) { 9435 if (Utilities.noString(value)) 9436 this.publisher = null; 9437 else { 9438 if (this.publisher == null) 9439 this.publisher = new StringType(); 9440 this.publisher.setValue(value); 9441 } 9442 return this; 9443 } 9444 9445 /** 9446 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 9447 */ 9448 public List<ContactDetail> getContact() { 9449 if (this.contact == null) 9450 this.contact = new ArrayList<ContactDetail>(); 9451 return this.contact; 9452 } 9453 9454 /** 9455 * @return Returns a reference to <code>this</code> for easy method chaining 9456 */ 9457 public Citation setContact(List<ContactDetail> theContact) { 9458 this.contact = theContact; 9459 return this; 9460 } 9461 9462 public boolean hasContact() { 9463 if (this.contact == null) 9464 return false; 9465 for (ContactDetail item : this.contact) 9466 if (!item.isEmpty()) 9467 return true; 9468 return false; 9469 } 9470 9471 public ContactDetail addContact() { //3 9472 ContactDetail t = new ContactDetail(); 9473 if (this.contact == null) 9474 this.contact = new ArrayList<ContactDetail>(); 9475 this.contact.add(t); 9476 return t; 9477 } 9478 9479 public Citation addContact(ContactDetail t) { //3 9480 if (t == null) 9481 return this; 9482 if (this.contact == null) 9483 this.contact = new ArrayList<ContactDetail>(); 9484 this.contact.add(t); 9485 return this; 9486 } 9487 9488 /** 9489 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 9490 */ 9491 public ContactDetail getContactFirstRep() { 9492 if (getContact().isEmpty()) { 9493 addContact(); 9494 } 9495 return getContact().get(0); 9496 } 9497 9498 /** 9499 * @return {@link #description} (A free text natural language description of the citation from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9500 */ 9501 public MarkdownType getDescriptionElement() { 9502 if (this.description == null) 9503 if (Configuration.errorOnAutoCreate()) 9504 throw new Error("Attempt to auto-create Citation.description"); 9505 else if (Configuration.doAutoCreate()) 9506 this.description = new MarkdownType(); // bb 9507 return this.description; 9508 } 9509 9510 public boolean hasDescriptionElement() { 9511 return this.description != null && !this.description.isEmpty(); 9512 } 9513 9514 public boolean hasDescription() { 9515 return this.description != null && !this.description.isEmpty(); 9516 } 9517 9518 /** 9519 * @param value {@link #description} (A free text natural language description of the citation from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9520 */ 9521 public Citation setDescriptionElement(MarkdownType value) { 9522 this.description = value; 9523 return this; 9524 } 9525 9526 /** 9527 * @return A free text natural language description of the citation from a consumer's perspective. 9528 */ 9529 public String getDescription() { 9530 return this.description == null ? null : this.description.getValue(); 9531 } 9532 9533 /** 9534 * @param value A free text natural language description of the citation from a consumer's perspective. 9535 */ 9536 public Citation setDescription(String value) { 9537 if (Utilities.noString(value)) 9538 this.description = null; 9539 else { 9540 if (this.description == null) 9541 this.description = new MarkdownType(); 9542 this.description.setValue(value); 9543 } 9544 return this; 9545 } 9546 9547 /** 9548 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate citation record instances.) 9549 */ 9550 public List<UsageContext> getUseContext() { 9551 if (this.useContext == null) 9552 this.useContext = new ArrayList<UsageContext>(); 9553 return this.useContext; 9554 } 9555 9556 /** 9557 * @return Returns a reference to <code>this</code> for easy method chaining 9558 */ 9559 public Citation setUseContext(List<UsageContext> theUseContext) { 9560 this.useContext = theUseContext; 9561 return this; 9562 } 9563 9564 public boolean hasUseContext() { 9565 if (this.useContext == null) 9566 return false; 9567 for (UsageContext item : this.useContext) 9568 if (!item.isEmpty()) 9569 return true; 9570 return false; 9571 } 9572 9573 public UsageContext addUseContext() { //3 9574 UsageContext t = new UsageContext(); 9575 if (this.useContext == null) 9576 this.useContext = new ArrayList<UsageContext>(); 9577 this.useContext.add(t); 9578 return t; 9579 } 9580 9581 public Citation addUseContext(UsageContext t) { //3 9582 if (t == null) 9583 return this; 9584 if (this.useContext == null) 9585 this.useContext = new ArrayList<UsageContext>(); 9586 this.useContext.add(t); 9587 return this; 9588 } 9589 9590 /** 9591 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 9592 */ 9593 public UsageContext getUseContextFirstRep() { 9594 if (getUseContext().isEmpty()) { 9595 addUseContext(); 9596 } 9597 return getUseContext().get(0); 9598 } 9599 9600 /** 9601 * @return {@link #jurisdiction} (A legal or geographic region in which the citation record is intended to be used.) 9602 */ 9603 public List<CodeableConcept> getJurisdiction() { 9604 if (this.jurisdiction == null) 9605 this.jurisdiction = new ArrayList<CodeableConcept>(); 9606 return this.jurisdiction; 9607 } 9608 9609 /** 9610 * @return Returns a reference to <code>this</code> for easy method chaining 9611 */ 9612 public Citation setJurisdiction(List<CodeableConcept> theJurisdiction) { 9613 this.jurisdiction = theJurisdiction; 9614 return this; 9615 } 9616 9617 public boolean hasJurisdiction() { 9618 if (this.jurisdiction == null) 9619 return false; 9620 for (CodeableConcept item : this.jurisdiction) 9621 if (!item.isEmpty()) 9622 return true; 9623 return false; 9624 } 9625 9626 public CodeableConcept addJurisdiction() { //3 9627 CodeableConcept t = new CodeableConcept(); 9628 if (this.jurisdiction == null) 9629 this.jurisdiction = new ArrayList<CodeableConcept>(); 9630 this.jurisdiction.add(t); 9631 return t; 9632 } 9633 9634 public Citation addJurisdiction(CodeableConcept t) { //3 9635 if (t == null) 9636 return this; 9637 if (this.jurisdiction == null) 9638 this.jurisdiction = new ArrayList<CodeableConcept>(); 9639 this.jurisdiction.add(t); 9640 return this; 9641 } 9642 9643 /** 9644 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 9645 */ 9646 public CodeableConcept getJurisdictionFirstRep() { 9647 if (getJurisdiction().isEmpty()) { 9648 addJurisdiction(); 9649 } 9650 return getJurisdiction().get(0); 9651 } 9652 9653 /** 9654 * @return {@link #purpose} (Explanation of why this citation is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 9655 */ 9656 public MarkdownType getPurposeElement() { 9657 if (this.purpose == null) 9658 if (Configuration.errorOnAutoCreate()) 9659 throw new Error("Attempt to auto-create Citation.purpose"); 9660 else if (Configuration.doAutoCreate()) 9661 this.purpose = new MarkdownType(); // bb 9662 return this.purpose; 9663 } 9664 9665 public boolean hasPurposeElement() { 9666 return this.purpose != null && !this.purpose.isEmpty(); 9667 } 9668 9669 public boolean hasPurpose() { 9670 return this.purpose != null && !this.purpose.isEmpty(); 9671 } 9672 9673 /** 9674 * @param value {@link #purpose} (Explanation of why this citation is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 9675 */ 9676 public Citation setPurposeElement(MarkdownType value) { 9677 this.purpose = value; 9678 return this; 9679 } 9680 9681 /** 9682 * @return Explanation of why this citation is needed and why it has been designed as it has. 9683 */ 9684 public String getPurpose() { 9685 return this.purpose == null ? null : this.purpose.getValue(); 9686 } 9687 9688 /** 9689 * @param value Explanation of why this citation is needed and why it has been designed as it has. 9690 */ 9691 public Citation setPurpose(String value) { 9692 if (Utilities.noString(value)) 9693 this.purpose = null; 9694 else { 9695 if (this.purpose == null) 9696 this.purpose = new MarkdownType(); 9697 this.purpose.setValue(value); 9698 } 9699 return this; 9700 } 9701 9702 /** 9703 * @return {@link #copyright} (Use and/or publishing restrictions for the citation record, not for the cited artifact.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 9704 */ 9705 public MarkdownType getCopyrightElement() { 9706 if (this.copyright == null) 9707 if (Configuration.errorOnAutoCreate()) 9708 throw new Error("Attempt to auto-create Citation.copyright"); 9709 else if (Configuration.doAutoCreate()) 9710 this.copyright = new MarkdownType(); // bb 9711 return this.copyright; 9712 } 9713 9714 public boolean hasCopyrightElement() { 9715 return this.copyright != null && !this.copyright.isEmpty(); 9716 } 9717 9718 public boolean hasCopyright() { 9719 return this.copyright != null && !this.copyright.isEmpty(); 9720 } 9721 9722 /** 9723 * @param value {@link #copyright} (Use and/or publishing restrictions for the citation record, not for the cited artifact.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 9724 */ 9725 public Citation setCopyrightElement(MarkdownType value) { 9726 this.copyright = value; 9727 return this; 9728 } 9729 9730 /** 9731 * @return Use and/or publishing restrictions for the citation record, not for the cited artifact. 9732 */ 9733 public String getCopyright() { 9734 return this.copyright == null ? null : this.copyright.getValue(); 9735 } 9736 9737 /** 9738 * @param value Use and/or publishing restrictions for the citation record, not for the cited artifact. 9739 */ 9740 public Citation setCopyright(String value) { 9741 if (Utilities.noString(value)) 9742 this.copyright = null; 9743 else { 9744 if (this.copyright == null) 9745 this.copyright = new MarkdownType(); 9746 this.copyright.setValue(value); 9747 } 9748 return this; 9749 } 9750 9751 /** 9752 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 9753 */ 9754 public StringType getCopyrightLabelElement() { 9755 if (this.copyrightLabel == null) 9756 if (Configuration.errorOnAutoCreate()) 9757 throw new Error("Attempt to auto-create Citation.copyrightLabel"); 9758 else if (Configuration.doAutoCreate()) 9759 this.copyrightLabel = new StringType(); // bb 9760 return this.copyrightLabel; 9761 } 9762 9763 public boolean hasCopyrightLabelElement() { 9764 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 9765 } 9766 9767 public boolean hasCopyrightLabel() { 9768 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 9769 } 9770 9771 /** 9772 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 9773 */ 9774 public Citation setCopyrightLabelElement(StringType value) { 9775 this.copyrightLabel = value; 9776 return this; 9777 } 9778 9779 /** 9780 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 9781 */ 9782 public String getCopyrightLabel() { 9783 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 9784 } 9785 9786 /** 9787 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 9788 */ 9789 public Citation setCopyrightLabel(String value) { 9790 if (Utilities.noString(value)) 9791 this.copyrightLabel = null; 9792 else { 9793 if (this.copyrightLabel == null) 9794 this.copyrightLabel = new StringType(); 9795 this.copyrightLabel.setValue(value); 9796 } 9797 return this; 9798 } 9799 9800 /** 9801 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 9802 */ 9803 public DateType getApprovalDateElement() { 9804 if (this.approvalDate == null) 9805 if (Configuration.errorOnAutoCreate()) 9806 throw new Error("Attempt to auto-create Citation.approvalDate"); 9807 else if (Configuration.doAutoCreate()) 9808 this.approvalDate = new DateType(); // bb 9809 return this.approvalDate; 9810 } 9811 9812 public boolean hasApprovalDateElement() { 9813 return this.approvalDate != null && !this.approvalDate.isEmpty(); 9814 } 9815 9816 public boolean hasApprovalDate() { 9817 return this.approvalDate != null && !this.approvalDate.isEmpty(); 9818 } 9819 9820 /** 9821 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 9822 */ 9823 public Citation setApprovalDateElement(DateType value) { 9824 this.approvalDate = value; 9825 return this; 9826 } 9827 9828 /** 9829 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 9830 */ 9831 public Date getApprovalDate() { 9832 return this.approvalDate == null ? null : this.approvalDate.getValue(); 9833 } 9834 9835 /** 9836 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 9837 */ 9838 public Citation setApprovalDate(Date value) { 9839 if (value == null) 9840 this.approvalDate = null; 9841 else { 9842 if (this.approvalDate == null) 9843 this.approvalDate = new DateType(); 9844 this.approvalDate.setValue(value); 9845 } 9846 return this; 9847 } 9848 9849 /** 9850 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 9851 */ 9852 public DateType getLastReviewDateElement() { 9853 if (this.lastReviewDate == null) 9854 if (Configuration.errorOnAutoCreate()) 9855 throw new Error("Attempt to auto-create Citation.lastReviewDate"); 9856 else if (Configuration.doAutoCreate()) 9857 this.lastReviewDate = new DateType(); // bb 9858 return this.lastReviewDate; 9859 } 9860 9861 public boolean hasLastReviewDateElement() { 9862 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 9863 } 9864 9865 public boolean hasLastReviewDate() { 9866 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 9867 } 9868 9869 /** 9870 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 9871 */ 9872 public Citation setLastReviewDateElement(DateType value) { 9873 this.lastReviewDate = value; 9874 return this; 9875 } 9876 9877 /** 9878 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 9879 */ 9880 public Date getLastReviewDate() { 9881 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 9882 } 9883 9884 /** 9885 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 9886 */ 9887 public Citation setLastReviewDate(Date value) { 9888 if (value == null) 9889 this.lastReviewDate = null; 9890 else { 9891 if (this.lastReviewDate == null) 9892 this.lastReviewDate = new DateType(); 9893 this.lastReviewDate.setValue(value); 9894 } 9895 return this; 9896 } 9897 9898 /** 9899 * @return {@link #effectivePeriod} (The period during which the citation record content was or is planned to be in active use.) 9900 */ 9901 public Period getEffectivePeriod() { 9902 if (this.effectivePeriod == null) 9903 if (Configuration.errorOnAutoCreate()) 9904 throw new Error("Attempt to auto-create Citation.effectivePeriod"); 9905 else if (Configuration.doAutoCreate()) 9906 this.effectivePeriod = new Period(); // cc 9907 return this.effectivePeriod; 9908 } 9909 9910 public boolean hasEffectivePeriod() { 9911 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 9912 } 9913 9914 /** 9915 * @param value {@link #effectivePeriod} (The period during which the citation record content was or is planned to be in active use.) 9916 */ 9917 public Citation setEffectivePeriod(Period value) { 9918 this.effectivePeriod = value; 9919 return this; 9920 } 9921 9922 /** 9923 * @return {@link #author} (Who authored or created the citation record.) 9924 */ 9925 public List<ContactDetail> getAuthor() { 9926 if (this.author == null) 9927 this.author = new ArrayList<ContactDetail>(); 9928 return this.author; 9929 } 9930 9931 /** 9932 * @return Returns a reference to <code>this</code> for easy method chaining 9933 */ 9934 public Citation setAuthor(List<ContactDetail> theAuthor) { 9935 this.author = theAuthor; 9936 return this; 9937 } 9938 9939 public boolean hasAuthor() { 9940 if (this.author == null) 9941 return false; 9942 for (ContactDetail item : this.author) 9943 if (!item.isEmpty()) 9944 return true; 9945 return false; 9946 } 9947 9948 public ContactDetail addAuthor() { //3 9949 ContactDetail t = new ContactDetail(); 9950 if (this.author == null) 9951 this.author = new ArrayList<ContactDetail>(); 9952 this.author.add(t); 9953 return t; 9954 } 9955 9956 public Citation addAuthor(ContactDetail t) { //3 9957 if (t == null) 9958 return this; 9959 if (this.author == null) 9960 this.author = new ArrayList<ContactDetail>(); 9961 this.author.add(t); 9962 return this; 9963 } 9964 9965 /** 9966 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 9967 */ 9968 public ContactDetail getAuthorFirstRep() { 9969 if (getAuthor().isEmpty()) { 9970 addAuthor(); 9971 } 9972 return getAuthor().get(0); 9973 } 9974 9975 /** 9976 * @return {@link #editor} (Who edited or revised the citation record.) 9977 */ 9978 public List<ContactDetail> getEditor() { 9979 if (this.editor == null) 9980 this.editor = new ArrayList<ContactDetail>(); 9981 return this.editor; 9982 } 9983 9984 /** 9985 * @return Returns a reference to <code>this</code> for easy method chaining 9986 */ 9987 public Citation setEditor(List<ContactDetail> theEditor) { 9988 this.editor = theEditor; 9989 return this; 9990 } 9991 9992 public boolean hasEditor() { 9993 if (this.editor == null) 9994 return false; 9995 for (ContactDetail item : this.editor) 9996 if (!item.isEmpty()) 9997 return true; 9998 return false; 9999 } 10000 10001 public ContactDetail addEditor() { //3 10002 ContactDetail t = new ContactDetail(); 10003 if (this.editor == null) 10004 this.editor = new ArrayList<ContactDetail>(); 10005 this.editor.add(t); 10006 return t; 10007 } 10008 10009 public Citation addEditor(ContactDetail t) { //3 10010 if (t == null) 10011 return this; 10012 if (this.editor == null) 10013 this.editor = new ArrayList<ContactDetail>(); 10014 this.editor.add(t); 10015 return this; 10016 } 10017 10018 /** 10019 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 10020 */ 10021 public ContactDetail getEditorFirstRep() { 10022 if (getEditor().isEmpty()) { 10023 addEditor(); 10024 } 10025 return getEditor().get(0); 10026 } 10027 10028 /** 10029 * @return {@link #reviewer} (Who reviewed the citation record.) 10030 */ 10031 public List<ContactDetail> getReviewer() { 10032 if (this.reviewer == null) 10033 this.reviewer = new ArrayList<ContactDetail>(); 10034 return this.reviewer; 10035 } 10036 10037 /** 10038 * @return Returns a reference to <code>this</code> for easy method chaining 10039 */ 10040 public Citation setReviewer(List<ContactDetail> theReviewer) { 10041 this.reviewer = theReviewer; 10042 return this; 10043 } 10044 10045 public boolean hasReviewer() { 10046 if (this.reviewer == null) 10047 return false; 10048 for (ContactDetail item : this.reviewer) 10049 if (!item.isEmpty()) 10050 return true; 10051 return false; 10052 } 10053 10054 public ContactDetail addReviewer() { //3 10055 ContactDetail t = new ContactDetail(); 10056 if (this.reviewer == null) 10057 this.reviewer = new ArrayList<ContactDetail>(); 10058 this.reviewer.add(t); 10059 return t; 10060 } 10061 10062 public Citation addReviewer(ContactDetail t) { //3 10063 if (t == null) 10064 return this; 10065 if (this.reviewer == null) 10066 this.reviewer = new ArrayList<ContactDetail>(); 10067 this.reviewer.add(t); 10068 return this; 10069 } 10070 10071 /** 10072 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 10073 */ 10074 public ContactDetail getReviewerFirstRep() { 10075 if (getReviewer().isEmpty()) { 10076 addReviewer(); 10077 } 10078 return getReviewer().get(0); 10079 } 10080 10081 /** 10082 * @return {@link #endorser} (Who endorsed the citation record.) 10083 */ 10084 public List<ContactDetail> getEndorser() { 10085 if (this.endorser == null) 10086 this.endorser = new ArrayList<ContactDetail>(); 10087 return this.endorser; 10088 } 10089 10090 /** 10091 * @return Returns a reference to <code>this</code> for easy method chaining 10092 */ 10093 public Citation setEndorser(List<ContactDetail> theEndorser) { 10094 this.endorser = theEndorser; 10095 return this; 10096 } 10097 10098 public boolean hasEndorser() { 10099 if (this.endorser == null) 10100 return false; 10101 for (ContactDetail item : this.endorser) 10102 if (!item.isEmpty()) 10103 return true; 10104 return false; 10105 } 10106 10107 public ContactDetail addEndorser() { //3 10108 ContactDetail t = new ContactDetail(); 10109 if (this.endorser == null) 10110 this.endorser = new ArrayList<ContactDetail>(); 10111 this.endorser.add(t); 10112 return t; 10113 } 10114 10115 public Citation addEndorser(ContactDetail t) { //3 10116 if (t == null) 10117 return this; 10118 if (this.endorser == null) 10119 this.endorser = new ArrayList<ContactDetail>(); 10120 this.endorser.add(t); 10121 return this; 10122 } 10123 10124 /** 10125 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 10126 */ 10127 public ContactDetail getEndorserFirstRep() { 10128 if (getEndorser().isEmpty()) { 10129 addEndorser(); 10130 } 10131 return getEndorser().get(0); 10132 } 10133 10134 /** 10135 * @return {@link #summary} (A human-readable display of key concepts to represent the citation.) 10136 */ 10137 public List<CitationSummaryComponent> getSummary() { 10138 if (this.summary == null) 10139 this.summary = new ArrayList<CitationSummaryComponent>(); 10140 return this.summary; 10141 } 10142 10143 /** 10144 * @return Returns a reference to <code>this</code> for easy method chaining 10145 */ 10146 public Citation setSummary(List<CitationSummaryComponent> theSummary) { 10147 this.summary = theSummary; 10148 return this; 10149 } 10150 10151 public boolean hasSummary() { 10152 if (this.summary == null) 10153 return false; 10154 for (CitationSummaryComponent item : this.summary) 10155 if (!item.isEmpty()) 10156 return true; 10157 return false; 10158 } 10159 10160 public CitationSummaryComponent addSummary() { //3 10161 CitationSummaryComponent t = new CitationSummaryComponent(); 10162 if (this.summary == null) 10163 this.summary = new ArrayList<CitationSummaryComponent>(); 10164 this.summary.add(t); 10165 return t; 10166 } 10167 10168 public Citation addSummary(CitationSummaryComponent t) { //3 10169 if (t == null) 10170 return this; 10171 if (this.summary == null) 10172 this.summary = new ArrayList<CitationSummaryComponent>(); 10173 this.summary.add(t); 10174 return this; 10175 } 10176 10177 /** 10178 * @return The first repetition of repeating field {@link #summary}, creating it if it does not already exist {3} 10179 */ 10180 public CitationSummaryComponent getSummaryFirstRep() { 10181 if (getSummary().isEmpty()) { 10182 addSummary(); 10183 } 10184 return getSummary().get(0); 10185 } 10186 10187 /** 10188 * @return {@link #classification} (The assignment to an organizing scheme.) 10189 */ 10190 public List<CitationClassificationComponent> getClassification() { 10191 if (this.classification == null) 10192 this.classification = new ArrayList<CitationClassificationComponent>(); 10193 return this.classification; 10194 } 10195 10196 /** 10197 * @return Returns a reference to <code>this</code> for easy method chaining 10198 */ 10199 public Citation setClassification(List<CitationClassificationComponent> theClassification) { 10200 this.classification = theClassification; 10201 return this; 10202 } 10203 10204 public boolean hasClassification() { 10205 if (this.classification == null) 10206 return false; 10207 for (CitationClassificationComponent item : this.classification) 10208 if (!item.isEmpty()) 10209 return true; 10210 return false; 10211 } 10212 10213 public CitationClassificationComponent addClassification() { //3 10214 CitationClassificationComponent t = new CitationClassificationComponent(); 10215 if (this.classification == null) 10216 this.classification = new ArrayList<CitationClassificationComponent>(); 10217 this.classification.add(t); 10218 return t; 10219 } 10220 10221 public Citation addClassification(CitationClassificationComponent t) { //3 10222 if (t == null) 10223 return this; 10224 if (this.classification == null) 10225 this.classification = new ArrayList<CitationClassificationComponent>(); 10226 this.classification.add(t); 10227 return this; 10228 } 10229 10230 /** 10231 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 10232 */ 10233 public CitationClassificationComponent getClassificationFirstRep() { 10234 if (getClassification().isEmpty()) { 10235 addClassification(); 10236 } 10237 return getClassification().get(0); 10238 } 10239 10240 /** 10241 * @return {@link #note} (Used for general notes and annotations not coded elsewhere.) 10242 */ 10243 public List<Annotation> getNote() { 10244 if (this.note == null) 10245 this.note = new ArrayList<Annotation>(); 10246 return this.note; 10247 } 10248 10249 /** 10250 * @return Returns a reference to <code>this</code> for easy method chaining 10251 */ 10252 public Citation setNote(List<Annotation> theNote) { 10253 this.note = theNote; 10254 return this; 10255 } 10256 10257 public boolean hasNote() { 10258 if (this.note == null) 10259 return false; 10260 for (Annotation item : this.note) 10261 if (!item.isEmpty()) 10262 return true; 10263 return false; 10264 } 10265 10266 public Annotation addNote() { //3 10267 Annotation t = new Annotation(); 10268 if (this.note == null) 10269 this.note = new ArrayList<Annotation>(); 10270 this.note.add(t); 10271 return t; 10272 } 10273 10274 public Citation addNote(Annotation t) { //3 10275 if (t == null) 10276 return this; 10277 if (this.note == null) 10278 this.note = new ArrayList<Annotation>(); 10279 this.note.add(t); 10280 return this; 10281 } 10282 10283 /** 10284 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 10285 */ 10286 public Annotation getNoteFirstRep() { 10287 if (getNote().isEmpty()) { 10288 addNote(); 10289 } 10290 return getNote().get(0); 10291 } 10292 10293 /** 10294 * @return {@link #currentState} (The status of the citation record.) 10295 */ 10296 public List<CodeableConcept> getCurrentState() { 10297 if (this.currentState == null) 10298 this.currentState = new ArrayList<CodeableConcept>(); 10299 return this.currentState; 10300 } 10301 10302 /** 10303 * @return Returns a reference to <code>this</code> for easy method chaining 10304 */ 10305 public Citation setCurrentState(List<CodeableConcept> theCurrentState) { 10306 this.currentState = theCurrentState; 10307 return this; 10308 } 10309 10310 public boolean hasCurrentState() { 10311 if (this.currentState == null) 10312 return false; 10313 for (CodeableConcept item : this.currentState) 10314 if (!item.isEmpty()) 10315 return true; 10316 return false; 10317 } 10318 10319 public CodeableConcept addCurrentState() { //3 10320 CodeableConcept t = new CodeableConcept(); 10321 if (this.currentState == null) 10322 this.currentState = new ArrayList<CodeableConcept>(); 10323 this.currentState.add(t); 10324 return t; 10325 } 10326 10327 public Citation addCurrentState(CodeableConcept t) { //3 10328 if (t == null) 10329 return this; 10330 if (this.currentState == null) 10331 this.currentState = new ArrayList<CodeableConcept>(); 10332 this.currentState.add(t); 10333 return this; 10334 } 10335 10336 /** 10337 * @return The first repetition of repeating field {@link #currentState}, creating it if it does not already exist {3} 10338 */ 10339 public CodeableConcept getCurrentStateFirstRep() { 10340 if (getCurrentState().isEmpty()) { 10341 addCurrentState(); 10342 } 10343 return getCurrentState().get(0); 10344 } 10345 10346 /** 10347 * @return {@link #statusDate} (The state or status of the citation record paired with an effective date or period for that state.) 10348 */ 10349 public List<CitationStatusDateComponent> getStatusDate() { 10350 if (this.statusDate == null) 10351 this.statusDate = new ArrayList<CitationStatusDateComponent>(); 10352 return this.statusDate; 10353 } 10354 10355 /** 10356 * @return Returns a reference to <code>this</code> for easy method chaining 10357 */ 10358 public Citation setStatusDate(List<CitationStatusDateComponent> theStatusDate) { 10359 this.statusDate = theStatusDate; 10360 return this; 10361 } 10362 10363 public boolean hasStatusDate() { 10364 if (this.statusDate == null) 10365 return false; 10366 for (CitationStatusDateComponent item : this.statusDate) 10367 if (!item.isEmpty()) 10368 return true; 10369 return false; 10370 } 10371 10372 public CitationStatusDateComponent addStatusDate() { //3 10373 CitationStatusDateComponent t = new CitationStatusDateComponent(); 10374 if (this.statusDate == null) 10375 this.statusDate = new ArrayList<CitationStatusDateComponent>(); 10376 this.statusDate.add(t); 10377 return t; 10378 } 10379 10380 public Citation addStatusDate(CitationStatusDateComponent t) { //3 10381 if (t == null) 10382 return this; 10383 if (this.statusDate == null) 10384 this.statusDate = new ArrayList<CitationStatusDateComponent>(); 10385 this.statusDate.add(t); 10386 return this; 10387 } 10388 10389 /** 10390 * @return The first repetition of repeating field {@link #statusDate}, creating it if it does not already exist {3} 10391 */ 10392 public CitationStatusDateComponent getStatusDateFirstRep() { 10393 if (getStatusDate().isEmpty()) { 10394 addStatusDate(); 10395 } 10396 return getStatusDate().get(0); 10397 } 10398 10399 /** 10400 * @return {@link #relatedArtifact} (Artifact related to the citation record.) 10401 */ 10402 public List<RelatedArtifact> getRelatedArtifact() { 10403 if (this.relatedArtifact == null) 10404 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 10405 return this.relatedArtifact; 10406 } 10407 10408 /** 10409 * @return Returns a reference to <code>this</code> for easy method chaining 10410 */ 10411 public Citation setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 10412 this.relatedArtifact = theRelatedArtifact; 10413 return this; 10414 } 10415 10416 public boolean hasRelatedArtifact() { 10417 if (this.relatedArtifact == null) 10418 return false; 10419 for (RelatedArtifact item : this.relatedArtifact) 10420 if (!item.isEmpty()) 10421 return true; 10422 return false; 10423 } 10424 10425 public RelatedArtifact addRelatedArtifact() { //3 10426 RelatedArtifact t = new RelatedArtifact(); 10427 if (this.relatedArtifact == null) 10428 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 10429 this.relatedArtifact.add(t); 10430 return t; 10431 } 10432 10433 public Citation addRelatedArtifact(RelatedArtifact t) { //3 10434 if (t == null) 10435 return this; 10436 if (this.relatedArtifact == null) 10437 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 10438 this.relatedArtifact.add(t); 10439 return this; 10440 } 10441 10442 /** 10443 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 10444 */ 10445 public RelatedArtifact getRelatedArtifactFirstRep() { 10446 if (getRelatedArtifact().isEmpty()) { 10447 addRelatedArtifact(); 10448 } 10449 return getRelatedArtifact().get(0); 10450 } 10451 10452 /** 10453 * @return {@link #citedArtifact} (The article or artifact being described.) 10454 */ 10455 public CitationCitedArtifactComponent getCitedArtifact() { 10456 if (this.citedArtifact == null) 10457 if (Configuration.errorOnAutoCreate()) 10458 throw new Error("Attempt to auto-create Citation.citedArtifact"); 10459 else if (Configuration.doAutoCreate()) 10460 this.citedArtifact = new CitationCitedArtifactComponent(); // cc 10461 return this.citedArtifact; 10462 } 10463 10464 public boolean hasCitedArtifact() { 10465 return this.citedArtifact != null && !this.citedArtifact.isEmpty(); 10466 } 10467 10468 /** 10469 * @param value {@link #citedArtifact} (The article or artifact being described.) 10470 */ 10471 public Citation setCitedArtifact(CitationCitedArtifactComponent value) { 10472 this.citedArtifact = value; 10473 return this; 10474 } 10475 10476 /** 10477 * not supported on this implementation 10478 */ 10479 @Override 10480 public int getTopicMax() { 10481 return 0; 10482 } 10483 /** 10484 * @return {@link #topic} (Descriptive topics related to the content of the citation. Topics provide a high-level categorization as well as keywords for the citation that can be useful for filtering and searching.) 10485 */ 10486 public List<CodeableConcept> getTopic() { 10487 return new ArrayList<>(); 10488 } 10489 /** 10490 * @return Returns a reference to <code>this</code> for easy method chaining 10491 */ 10492 public Citation setTopic(List<CodeableConcept> theTopic) { 10493 throw new Error("The resource type \"Citation\" does not implement the property \"topic\""); 10494 } 10495 public boolean hasTopic() { 10496 return false; 10497 } 10498 10499 public CodeableConcept addTopic() { //3 10500 throw new Error("The resource type \"Citation\" does not implement the property \"topic\""); 10501 } 10502 public Citation addTopic(CodeableConcept t) { //3 10503 throw new Error("The resource type \"Citation\" does not implement the property \"topic\""); 10504 } 10505 /** 10506 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 10507 */ 10508 public CodeableConcept getTopicFirstRep() { 10509 throw new Error("The resource type \"Citation\" does not implement the property \"topic\""); 10510 } 10511 protected void listChildren(List<Property> children) { 10512 super.listChildren(children); 10513 children.add(new Property("url", "uri", "An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url)); 10514 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this citation record when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 10515 children.add(new Property("version", "string", "The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 10516 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 10517 children.add(new Property("name", "string", "A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 10518 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the citation record.", 0, 1, title)); 10519 children.add(new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status)); 10520 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 10521 children.add(new Property("date", "dateTime", "The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes.", 0, 1, date)); 10522 children.add(new Property("publisher", "string", "The name of the organization or individual that published the citation record.", 0, 1, publisher)); 10523 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 10524 children.add(new Property("description", "markdown", "A free text natural language description of the citation from a consumer's perspective.", 0, 1, description)); 10525 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate citation record instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 10526 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the citation record is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 10527 children.add(new Property("purpose", "markdown", "Explanation of why this citation is needed and why it has been designed as it has.", 0, 1, purpose)); 10528 children.add(new Property("copyright", "markdown", "Use and/or publishing restrictions for the citation record, not for the cited artifact.", 0, 1, copyright)); 10529 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 10530 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 10531 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 10532 children.add(new Property("effectivePeriod", "Period", "The period during which the citation record content was or is planned to be in active use.", 0, 1, effectivePeriod)); 10533 children.add(new Property("author", "ContactDetail", "Who authored or created the citation record.", 0, java.lang.Integer.MAX_VALUE, author)); 10534 children.add(new Property("editor", "ContactDetail", "Who edited or revised the citation record.", 0, java.lang.Integer.MAX_VALUE, editor)); 10535 children.add(new Property("reviewer", "ContactDetail", "Who reviewed the citation record.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 10536 children.add(new Property("endorser", "ContactDetail", "Who endorsed the citation record.", 0, java.lang.Integer.MAX_VALUE, endorser)); 10537 children.add(new Property("summary", "", "A human-readable display of key concepts to represent the citation.", 0, java.lang.Integer.MAX_VALUE, summary)); 10538 children.add(new Property("classification", "", "The assignment to an organizing scheme.", 0, java.lang.Integer.MAX_VALUE, classification)); 10539 children.add(new Property("note", "Annotation", "Used for general notes and annotations not coded elsewhere.", 0, java.lang.Integer.MAX_VALUE, note)); 10540 children.add(new Property("currentState", "CodeableConcept", "The status of the citation record.", 0, java.lang.Integer.MAX_VALUE, currentState)); 10541 children.add(new Property("statusDate", "", "The state or status of the citation record paired with an effective date or period for that state.", 0, java.lang.Integer.MAX_VALUE, statusDate)); 10542 children.add(new Property("relatedArtifact", "RelatedArtifact", "Artifact related to the citation record.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 10543 children.add(new Property("citedArtifact", "", "The article or artifact being described.", 0, 1, citedArtifact)); 10544 } 10545 10546 @Override 10547 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10548 switch (_hash) { 10549 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this citation record when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url); 10550 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this citation record when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 10551 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the citation record when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the citation record author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 10552 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 10553 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 10554 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 10555 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 10556 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the citation record. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 10557 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the citation record.", 0, 1, title); 10558 case -892481550: /*status*/ return new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status); 10559 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this citation record is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 10560 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the citation record was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the citation record changes.", 0, 1, date); 10561 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual that published the citation record.", 0, 1, publisher); 10562 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 10563 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the citation from a consumer's perspective.", 0, 1, description); 10564 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate citation record instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 10565 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the citation record is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 10566 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this citation is needed and why it has been designed as it has.", 0, 1, purpose); 10567 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "Use and/or publishing restrictions for the citation record, not for the cited artifact.", 0, 1, copyright); 10568 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 10569 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 10570 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 10571 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the citation record content was or is planned to be in active use.", 0, 1, effectivePeriod); 10572 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "Who authored or created the citation record.", 0, java.lang.Integer.MAX_VALUE, author); 10573 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "Who edited or revised the citation record.", 0, java.lang.Integer.MAX_VALUE, editor); 10574 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "Who reviewed the citation record.", 0, java.lang.Integer.MAX_VALUE, reviewer); 10575 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "Who endorsed the citation record.", 0, java.lang.Integer.MAX_VALUE, endorser); 10576 case -1857640538: /*summary*/ return new Property("summary", "", "A human-readable display of key concepts to represent the citation.", 0, java.lang.Integer.MAX_VALUE, summary); 10577 case 382350310: /*classification*/ return new Property("classification", "", "The assignment to an organizing scheme.", 0, java.lang.Integer.MAX_VALUE, classification); 10578 case 3387378: /*note*/ return new Property("note", "Annotation", "Used for general notes and annotations not coded elsewhere.", 0, java.lang.Integer.MAX_VALUE, note); 10579 case 1457822360: /*currentState*/ return new Property("currentState", "CodeableConcept", "The status of the citation record.", 0, java.lang.Integer.MAX_VALUE, currentState); 10580 case 247524032: /*statusDate*/ return new Property("statusDate", "", "The state or status of the citation record paired with an effective date or period for that state.", 0, java.lang.Integer.MAX_VALUE, statusDate); 10581 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Artifact related to the citation record.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 10582 case -495272225: /*citedArtifact*/ return new Property("citedArtifact", "", "The article or artifact being described.", 0, 1, citedArtifact); 10583 default: return super.getNamedProperty(_hash, _name, _checkValid); 10584 } 10585 10586 } 10587 10588 @Override 10589 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10590 switch (hash) { 10591 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 10592 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 10593 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 10594 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 10595 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 10596 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 10597 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 10598 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 10599 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 10600 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 10601 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 10602 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 10603 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 10604 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 10605 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 10606 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 10607 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 10608 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 10609 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 10610 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 10611 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 10612 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 10613 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 10614 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 10615 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : this.summary.toArray(new Base[this.summary.size()]); // CitationSummaryComponent 10616 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // CitationClassificationComponent 10617 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 10618 case 1457822360: /*currentState*/ return this.currentState == null ? new Base[0] : this.currentState.toArray(new Base[this.currentState.size()]); // CodeableConcept 10619 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : this.statusDate.toArray(new Base[this.statusDate.size()]); // CitationStatusDateComponent 10620 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 10621 case -495272225: /*citedArtifact*/ return this.citedArtifact == null ? new Base[0] : new Base[] {this.citedArtifact}; // CitationCitedArtifactComponent 10622 default: return super.getProperty(hash, name, checkValid); 10623 } 10624 10625 } 10626 10627 @Override 10628 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10629 switch (hash) { 10630 case 116079: // url 10631 this.url = TypeConvertor.castToUri(value); // UriType 10632 return value; 10633 case -1618432855: // identifier 10634 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 10635 return value; 10636 case 351608024: // version 10637 this.version = TypeConvertor.castToString(value); // StringType 10638 return value; 10639 case 1508158071: // versionAlgorithm 10640 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 10641 return value; 10642 case 3373707: // name 10643 this.name = TypeConvertor.castToString(value); // StringType 10644 return value; 10645 case 110371416: // title 10646 this.title = TypeConvertor.castToString(value); // StringType 10647 return value; 10648 case -892481550: // status 10649 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 10650 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10651 return value; 10652 case -404562712: // experimental 10653 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 10654 return value; 10655 case 3076014: // date 10656 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 10657 return value; 10658 case 1447404028: // publisher 10659 this.publisher = TypeConvertor.castToString(value); // StringType 10660 return value; 10661 case 951526432: // contact 10662 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 10663 return value; 10664 case -1724546052: // description 10665 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 10666 return value; 10667 case -669707736: // useContext 10668 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 10669 return value; 10670 case -507075711: // jurisdiction 10671 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10672 return value; 10673 case -220463842: // purpose 10674 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 10675 return value; 10676 case 1522889671: // copyright 10677 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 10678 return value; 10679 case 765157229: // copyrightLabel 10680 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 10681 return value; 10682 case 223539345: // approvalDate 10683 this.approvalDate = TypeConvertor.castToDate(value); // DateType 10684 return value; 10685 case -1687512484: // lastReviewDate 10686 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 10687 return value; 10688 case -403934648: // effectivePeriod 10689 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 10690 return value; 10691 case -1406328437: // author 10692 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 10693 return value; 10694 case -1307827859: // editor 10695 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 10696 return value; 10697 case -261190139: // reviewer 10698 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 10699 return value; 10700 case 1740277666: // endorser 10701 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 10702 return value; 10703 case -1857640538: // summary 10704 this.getSummary().add((CitationSummaryComponent) value); // CitationSummaryComponent 10705 return value; 10706 case 382350310: // classification 10707 this.getClassification().add((CitationClassificationComponent) value); // CitationClassificationComponent 10708 return value; 10709 case 3387378: // note 10710 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 10711 return value; 10712 case 1457822360: // currentState 10713 this.getCurrentState().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10714 return value; 10715 case 247524032: // statusDate 10716 this.getStatusDate().add((CitationStatusDateComponent) value); // CitationStatusDateComponent 10717 return value; 10718 case 666807069: // relatedArtifact 10719 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 10720 return value; 10721 case -495272225: // citedArtifact 10722 this.citedArtifact = (CitationCitedArtifactComponent) value; // CitationCitedArtifactComponent 10723 return value; 10724 default: return super.setProperty(hash, name, value); 10725 } 10726 10727 } 10728 10729 @Override 10730 public Base setProperty(String name, Base value) throws FHIRException { 10731 if (name.equals("url")) { 10732 this.url = TypeConvertor.castToUri(value); // UriType 10733 } else if (name.equals("identifier")) { 10734 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 10735 } else if (name.equals("version")) { 10736 this.version = TypeConvertor.castToString(value); // StringType 10737 } else if (name.equals("versionAlgorithm[x]")) { 10738 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 10739 } else if (name.equals("name")) { 10740 this.name = TypeConvertor.castToString(value); // StringType 10741 } else if (name.equals("title")) { 10742 this.title = TypeConvertor.castToString(value); // StringType 10743 } else if (name.equals("status")) { 10744 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 10745 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10746 } else if (name.equals("experimental")) { 10747 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 10748 } else if (name.equals("date")) { 10749 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 10750 } else if (name.equals("publisher")) { 10751 this.publisher = TypeConvertor.castToString(value); // StringType 10752 } else if (name.equals("contact")) { 10753 this.getContact().add(TypeConvertor.castToContactDetail(value)); 10754 } else if (name.equals("description")) { 10755 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 10756 } else if (name.equals("useContext")) { 10757 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 10758 } else if (name.equals("jurisdiction")) { 10759 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 10760 } else if (name.equals("purpose")) { 10761 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 10762 } else if (name.equals("copyright")) { 10763 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 10764 } else if (name.equals("copyrightLabel")) { 10765 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 10766 } else if (name.equals("approvalDate")) { 10767 this.approvalDate = TypeConvertor.castToDate(value); // DateType 10768 } else if (name.equals("lastReviewDate")) { 10769 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 10770 } else if (name.equals("effectivePeriod")) { 10771 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 10772 } else if (name.equals("author")) { 10773 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 10774 } else if (name.equals("editor")) { 10775 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 10776 } else if (name.equals("reviewer")) { 10777 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 10778 } else if (name.equals("endorser")) { 10779 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 10780 } else if (name.equals("summary")) { 10781 this.getSummary().add((CitationSummaryComponent) value); 10782 } else if (name.equals("classification")) { 10783 this.getClassification().add((CitationClassificationComponent) value); 10784 } else if (name.equals("note")) { 10785 this.getNote().add(TypeConvertor.castToAnnotation(value)); 10786 } else if (name.equals("currentState")) { 10787 this.getCurrentState().add(TypeConvertor.castToCodeableConcept(value)); 10788 } else if (name.equals("statusDate")) { 10789 this.getStatusDate().add((CitationStatusDateComponent) value); 10790 } else if (name.equals("relatedArtifact")) { 10791 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 10792 } else if (name.equals("citedArtifact")) { 10793 this.citedArtifact = (CitationCitedArtifactComponent) value; // CitationCitedArtifactComponent 10794 } else 10795 return super.setProperty(name, value); 10796 return value; 10797 } 10798 10799 @Override 10800 public void removeChild(String name, Base value) throws FHIRException { 10801 if (name.equals("url")) { 10802 this.url = null; 10803 } else if (name.equals("identifier")) { 10804 this.getIdentifier().remove(value); 10805 } else if (name.equals("version")) { 10806 this.version = null; 10807 } else if (name.equals("versionAlgorithm[x]")) { 10808 this.versionAlgorithm = null; 10809 } else if (name.equals("name")) { 10810 this.name = null; 10811 } else if (name.equals("title")) { 10812 this.title = null; 10813 } else if (name.equals("status")) { 10814 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 10815 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 10816 } else if (name.equals("experimental")) { 10817 this.experimental = null; 10818 } else if (name.equals("date")) { 10819 this.date = null; 10820 } else if (name.equals("publisher")) { 10821 this.publisher = null; 10822 } else if (name.equals("contact")) { 10823 this.getContact().remove(value); 10824 } else if (name.equals("description")) { 10825 this.description = null; 10826 } else if (name.equals("useContext")) { 10827 this.getUseContext().remove(value); 10828 } else if (name.equals("jurisdiction")) { 10829 this.getJurisdiction().remove(value); 10830 } else if (name.equals("purpose")) { 10831 this.purpose = null; 10832 } else if (name.equals("copyright")) { 10833 this.copyright = null; 10834 } else if (name.equals("copyrightLabel")) { 10835 this.copyrightLabel = null; 10836 } else if (name.equals("approvalDate")) { 10837 this.approvalDate = null; 10838 } else if (name.equals("lastReviewDate")) { 10839 this.lastReviewDate = null; 10840 } else if (name.equals("effectivePeriod")) { 10841 this.effectivePeriod = null; 10842 } else if (name.equals("author")) { 10843 this.getAuthor().remove(value); 10844 } else if (name.equals("editor")) { 10845 this.getEditor().remove(value); 10846 } else if (name.equals("reviewer")) { 10847 this.getReviewer().remove(value); 10848 } else if (name.equals("endorser")) { 10849 this.getEndorser().remove(value); 10850 } else if (name.equals("summary")) { 10851 this.getSummary().remove((CitationSummaryComponent) value); 10852 } else if (name.equals("classification")) { 10853 this.getClassification().remove((CitationClassificationComponent) value); 10854 } else if (name.equals("note")) { 10855 this.getNote().remove(value); 10856 } else if (name.equals("currentState")) { 10857 this.getCurrentState().remove(value); 10858 } else if (name.equals("statusDate")) { 10859 this.getStatusDate().remove((CitationStatusDateComponent) value); 10860 } else if (name.equals("relatedArtifact")) { 10861 this.getRelatedArtifact().remove(value); 10862 } else if (name.equals("citedArtifact")) { 10863 this.citedArtifact = (CitationCitedArtifactComponent) value; // CitationCitedArtifactComponent 10864 } else 10865 super.removeChild(name, value); 10866 10867 } 10868 10869 @Override 10870 public Base makeProperty(int hash, String name) throws FHIRException { 10871 switch (hash) { 10872 case 116079: return getUrlElement(); 10873 case -1618432855: return addIdentifier(); 10874 case 351608024: return getVersionElement(); 10875 case -115699031: return getVersionAlgorithm(); 10876 case 1508158071: return getVersionAlgorithm(); 10877 case 3373707: return getNameElement(); 10878 case 110371416: return getTitleElement(); 10879 case -892481550: return getStatusElement(); 10880 case -404562712: return getExperimentalElement(); 10881 case 3076014: return getDateElement(); 10882 case 1447404028: return getPublisherElement(); 10883 case 951526432: return addContact(); 10884 case -1724546052: return getDescriptionElement(); 10885 case -669707736: return addUseContext(); 10886 case -507075711: return addJurisdiction(); 10887 case -220463842: return getPurposeElement(); 10888 case 1522889671: return getCopyrightElement(); 10889 case 765157229: return getCopyrightLabelElement(); 10890 case 223539345: return getApprovalDateElement(); 10891 case -1687512484: return getLastReviewDateElement(); 10892 case -403934648: return getEffectivePeriod(); 10893 case -1406328437: return addAuthor(); 10894 case -1307827859: return addEditor(); 10895 case -261190139: return addReviewer(); 10896 case 1740277666: return addEndorser(); 10897 case -1857640538: return addSummary(); 10898 case 382350310: return addClassification(); 10899 case 3387378: return addNote(); 10900 case 1457822360: return addCurrentState(); 10901 case 247524032: return addStatusDate(); 10902 case 666807069: return addRelatedArtifact(); 10903 case -495272225: return getCitedArtifact(); 10904 default: return super.makeProperty(hash, name); 10905 } 10906 10907 } 10908 10909 @Override 10910 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10911 switch (hash) { 10912 case 116079: /*url*/ return new String[] {"uri"}; 10913 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 10914 case 351608024: /*version*/ return new String[] {"string"}; 10915 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 10916 case 3373707: /*name*/ return new String[] {"string"}; 10917 case 110371416: /*title*/ return new String[] {"string"}; 10918 case -892481550: /*status*/ return new String[] {"code"}; 10919 case -404562712: /*experimental*/ return new String[] {"boolean"}; 10920 case 3076014: /*date*/ return new String[] {"dateTime"}; 10921 case 1447404028: /*publisher*/ return new String[] {"string"}; 10922 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 10923 case -1724546052: /*description*/ return new String[] {"markdown"}; 10924 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 10925 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 10926 case -220463842: /*purpose*/ return new String[] {"markdown"}; 10927 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 10928 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 10929 case 223539345: /*approvalDate*/ return new String[] {"date"}; 10930 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 10931 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 10932 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 10933 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 10934 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 10935 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 10936 case -1857640538: /*summary*/ return new String[] {}; 10937 case 382350310: /*classification*/ return new String[] {}; 10938 case 3387378: /*note*/ return new String[] {"Annotation"}; 10939 case 1457822360: /*currentState*/ return new String[] {"CodeableConcept"}; 10940 case 247524032: /*statusDate*/ return new String[] {}; 10941 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 10942 case -495272225: /*citedArtifact*/ return new String[] {}; 10943 default: return super.getTypesForProperty(hash, name); 10944 } 10945 10946 } 10947 10948 @Override 10949 public Base addChild(String name) throws FHIRException { 10950 if (name.equals("url")) { 10951 throw new FHIRException("Cannot call addChild on a singleton property Citation.url"); 10952 } 10953 else if (name.equals("identifier")) { 10954 return addIdentifier(); 10955 } 10956 else if (name.equals("version")) { 10957 throw new FHIRException("Cannot call addChild on a singleton property Citation.version"); 10958 } 10959 else if (name.equals("versionAlgorithmString")) { 10960 this.versionAlgorithm = new StringType(); 10961 return this.versionAlgorithm; 10962 } 10963 else if (name.equals("versionAlgorithmCoding")) { 10964 this.versionAlgorithm = new Coding(); 10965 return this.versionAlgorithm; 10966 } 10967 else if (name.equals("name")) { 10968 throw new FHIRException("Cannot call addChild on a singleton property Citation.name"); 10969 } 10970 else if (name.equals("title")) { 10971 throw new FHIRException("Cannot call addChild on a singleton property Citation.title"); 10972 } 10973 else if (name.equals("status")) { 10974 throw new FHIRException("Cannot call addChild on a singleton property Citation.status"); 10975 } 10976 else if (name.equals("experimental")) { 10977 throw new FHIRException("Cannot call addChild on a singleton property Citation.experimental"); 10978 } 10979 else if (name.equals("date")) { 10980 throw new FHIRException("Cannot call addChild on a singleton property Citation.date"); 10981 } 10982 else if (name.equals("publisher")) { 10983 throw new FHIRException("Cannot call addChild on a singleton property Citation.publisher"); 10984 } 10985 else if (name.equals("contact")) { 10986 return addContact(); 10987 } 10988 else if (name.equals("description")) { 10989 throw new FHIRException("Cannot call addChild on a singleton property Citation.description"); 10990 } 10991 else if (name.equals("useContext")) { 10992 return addUseContext(); 10993 } 10994 else if (name.equals("jurisdiction")) { 10995 return addJurisdiction(); 10996 } 10997 else if (name.equals("purpose")) { 10998 throw new FHIRException("Cannot call addChild on a singleton property Citation.purpose"); 10999 } 11000 else if (name.equals("copyright")) { 11001 throw new FHIRException("Cannot call addChild on a singleton property Citation.copyright"); 11002 } 11003 else if (name.equals("copyrightLabel")) { 11004 throw new FHIRException("Cannot call addChild on a singleton property Citation.copyrightLabel"); 11005 } 11006 else if (name.equals("approvalDate")) { 11007 throw new FHIRException("Cannot call addChild on a singleton property Citation.approvalDate"); 11008 } 11009 else if (name.equals("lastReviewDate")) { 11010 throw new FHIRException("Cannot call addChild on a singleton property Citation.lastReviewDate"); 11011 } 11012 else if (name.equals("effectivePeriod")) { 11013 this.effectivePeriod = new Period(); 11014 return this.effectivePeriod; 11015 } 11016 else if (name.equals("author")) { 11017 return addAuthor(); 11018 } 11019 else if (name.equals("editor")) { 11020 return addEditor(); 11021 } 11022 else if (name.equals("reviewer")) { 11023 return addReviewer(); 11024 } 11025 else if (name.equals("endorser")) { 11026 return addEndorser(); 11027 } 11028 else if (name.equals("summary")) { 11029 return addSummary(); 11030 } 11031 else if (name.equals("classification")) { 11032 return addClassification(); 11033 } 11034 else if (name.equals("note")) { 11035 return addNote(); 11036 } 11037 else if (name.equals("currentState")) { 11038 return addCurrentState(); 11039 } 11040 else if (name.equals("statusDate")) { 11041 return addStatusDate(); 11042 } 11043 else if (name.equals("relatedArtifact")) { 11044 return addRelatedArtifact(); 11045 } 11046 else if (name.equals("citedArtifact")) { 11047 this.citedArtifact = new CitationCitedArtifactComponent(); 11048 return this.citedArtifact; 11049 } 11050 else 11051 return super.addChild(name); 11052 } 11053 11054 public String fhirType() { 11055 return "Citation"; 11056 11057 } 11058 11059 public Citation copy() { 11060 Citation dst = new Citation(); 11061 copyValues(dst); 11062 return dst; 11063 } 11064 11065 public void copyValues(Citation dst) { 11066 super.copyValues(dst); 11067 dst.url = url == null ? null : url.copy(); 11068 if (identifier != null) { 11069 dst.identifier = new ArrayList<Identifier>(); 11070 for (Identifier i : identifier) 11071 dst.identifier.add(i.copy()); 11072 }; 11073 dst.version = version == null ? null : version.copy(); 11074 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 11075 dst.name = name == null ? null : name.copy(); 11076 dst.title = title == null ? null : title.copy(); 11077 dst.status = status == null ? null : status.copy(); 11078 dst.experimental = experimental == null ? null : experimental.copy(); 11079 dst.date = date == null ? null : date.copy(); 11080 dst.publisher = publisher == null ? null : publisher.copy(); 11081 if (contact != null) { 11082 dst.contact = new ArrayList<ContactDetail>(); 11083 for (ContactDetail i : contact) 11084 dst.contact.add(i.copy()); 11085 }; 11086 dst.description = description == null ? null : description.copy(); 11087 if (useContext != null) { 11088 dst.useContext = new ArrayList<UsageContext>(); 11089 for (UsageContext i : useContext) 11090 dst.useContext.add(i.copy()); 11091 }; 11092 if (jurisdiction != null) { 11093 dst.jurisdiction = new ArrayList<CodeableConcept>(); 11094 for (CodeableConcept i : jurisdiction) 11095 dst.jurisdiction.add(i.copy()); 11096 }; 11097 dst.purpose = purpose == null ? null : purpose.copy(); 11098 dst.copyright = copyright == null ? null : copyright.copy(); 11099 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 11100 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 11101 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 11102 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 11103 if (author != null) { 11104 dst.author = new ArrayList<ContactDetail>(); 11105 for (ContactDetail i : author) 11106 dst.author.add(i.copy()); 11107 }; 11108 if (editor != null) { 11109 dst.editor = new ArrayList<ContactDetail>(); 11110 for (ContactDetail i : editor) 11111 dst.editor.add(i.copy()); 11112 }; 11113 if (reviewer != null) { 11114 dst.reviewer = new ArrayList<ContactDetail>(); 11115 for (ContactDetail i : reviewer) 11116 dst.reviewer.add(i.copy()); 11117 }; 11118 if (endorser != null) { 11119 dst.endorser = new ArrayList<ContactDetail>(); 11120 for (ContactDetail i : endorser) 11121 dst.endorser.add(i.copy()); 11122 }; 11123 if (summary != null) { 11124 dst.summary = new ArrayList<CitationSummaryComponent>(); 11125 for (CitationSummaryComponent i : summary) 11126 dst.summary.add(i.copy()); 11127 }; 11128 if (classification != null) { 11129 dst.classification = new ArrayList<CitationClassificationComponent>(); 11130 for (CitationClassificationComponent i : classification) 11131 dst.classification.add(i.copy()); 11132 }; 11133 if (note != null) { 11134 dst.note = new ArrayList<Annotation>(); 11135 for (Annotation i : note) 11136 dst.note.add(i.copy()); 11137 }; 11138 if (currentState != null) { 11139 dst.currentState = new ArrayList<CodeableConcept>(); 11140 for (CodeableConcept i : currentState) 11141 dst.currentState.add(i.copy()); 11142 }; 11143 if (statusDate != null) { 11144 dst.statusDate = new ArrayList<CitationStatusDateComponent>(); 11145 for (CitationStatusDateComponent i : statusDate) 11146 dst.statusDate.add(i.copy()); 11147 }; 11148 if (relatedArtifact != null) { 11149 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 11150 for (RelatedArtifact i : relatedArtifact) 11151 dst.relatedArtifact.add(i.copy()); 11152 }; 11153 dst.citedArtifact = citedArtifact == null ? null : citedArtifact.copy(); 11154 } 11155 11156 protected Citation typedCopy() { 11157 return copy(); 11158 } 11159 11160 @Override 11161 public boolean equalsDeep(Base other_) { 11162 if (!super.equalsDeep(other_)) 11163 return false; 11164 if (!(other_ instanceof Citation)) 11165 return false; 11166 Citation o = (Citation) other_; 11167 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 11168 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 11169 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 11170 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 11171 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 11172 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 11173 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 11174 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(author, o.author, true) 11175 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 11176 && compareDeep(summary, o.summary, true) && compareDeep(classification, o.classification, true) 11177 && compareDeep(note, o.note, true) && compareDeep(currentState, o.currentState, true) && compareDeep(statusDate, o.statusDate, true) 11178 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(citedArtifact, o.citedArtifact, true) 11179 ; 11180 } 11181 11182 @Override 11183 public boolean equalsShallow(Base other_) { 11184 if (!super.equalsShallow(other_)) 11185 return false; 11186 if (!(other_ instanceof Citation)) 11187 return false; 11188 Citation o = (Citation) other_; 11189 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 11190 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 11191 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 11192 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 11193 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 11194 ; 11195 } 11196 11197 public boolean isEmpty() { 11198 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 11199 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 11200 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, approvalDate 11201 , lastReviewDate, effectivePeriod, author, editor, reviewer, endorser, summary 11202 , classification, note, currentState, statusDate, relatedArtifact, citedArtifact 11203 ); 11204 } 11205 11206 @Override 11207 public ResourceType getResourceType() { 11208 return ResourceType.Citation; 11209 } 11210 11211 /** 11212 * Search parameter: <b>context-quantity</b> 11213 * <p> 11214 * Description: <b>Multiple Resources: 11215 11216* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 11217* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 11218* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 11219* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 11220* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 11221* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 11222* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 11223* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 11224* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 11225* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 11226* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 11227* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 11228* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 11229* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 11230* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 11231* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 11232* [Library](library.html): A quantity- or range-valued use context assigned to the library 11233* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 11234* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 11235* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 11236* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 11237* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 11238* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 11239* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 11240* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 11241* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 11242* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 11243* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 11244* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 11245* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 11246</b><br> 11247 * Type: <b>quantity</b><br> 11248 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 11249 * </p> 11250 */ 11251 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 11252 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 11253 /** 11254 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 11255 * <p> 11256 * Description: <b>Multiple Resources: 11257 11258* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 11259* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 11260* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 11261* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 11262* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 11263* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 11264* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 11265* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 11266* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 11267* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 11268* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 11269* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 11270* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 11271* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 11272* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 11273* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 11274* [Library](library.html): A quantity- or range-valued use context assigned to the library 11275* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 11276* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 11277* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 11278* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 11279* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 11280* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 11281* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 11282* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 11283* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 11284* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 11285* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 11286* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 11287* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 11288</b><br> 11289 * Type: <b>quantity</b><br> 11290 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 11291 * </p> 11292 */ 11293 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 11294 11295 /** 11296 * Search parameter: <b>context-type-quantity</b> 11297 * <p> 11298 * Description: <b>Multiple Resources: 11299 11300* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 11301* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 11302* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 11303* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 11304* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 11305* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 11306* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 11307* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 11308* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 11309* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 11310* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 11311* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 11312* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 11313* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 11314* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 11315* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 11316* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 11317* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 11318* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 11319* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 11320* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 11321* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 11322* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 11323* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 11324* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 11325* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 11326* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 11327* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 11328* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 11329* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 11330</b><br> 11331 * Type: <b>composite</b><br> 11332 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 11333 * </p> 11334 */ 11335 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 11336 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 11337 /** 11338 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 11339 * <p> 11340 * Description: <b>Multiple Resources: 11341 11342* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 11343* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 11344* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 11345* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 11346* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 11347* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 11348* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 11349* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 11350* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 11351* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 11352* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 11353* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 11354* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 11355* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 11356* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 11357* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 11358* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 11359* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 11360* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 11361* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 11362* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 11363* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 11364* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 11365* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 11366* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 11367* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 11368* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 11369* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 11370* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 11371* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 11372</b><br> 11373 * Type: <b>composite</b><br> 11374 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 11375 * </p> 11376 */ 11377 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 11378 11379 /** 11380 * Search parameter: <b>context-type-value</b> 11381 * <p> 11382 * Description: <b>Multiple Resources: 11383 11384* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 11385* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 11386* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 11387* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 11388* [Citation](citation.html): A use context type and value assigned to the citation 11389* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 11390* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 11391* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 11392* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 11393* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 11394* [Evidence](evidence.html): A use context type and value assigned to the evidence 11395* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 11396* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 11397* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 11398* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 11399* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 11400* [Library](library.html): A use context type and value assigned to the library 11401* [Measure](measure.html): A use context type and value assigned to the measure 11402* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 11403* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 11404* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 11405* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 11406* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 11407* [Requirements](requirements.html): A use context type and value assigned to the requirements 11408* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 11409* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 11410* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 11411* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 11412* [TestScript](testscript.html): A use context type and value assigned to the test script 11413* [ValueSet](valueset.html): A use context type and value assigned to the value set 11414</b><br> 11415 * Type: <b>composite</b><br> 11416 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 11417 * </p> 11418 */ 11419 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 11420 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 11421 /** 11422 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 11423 * <p> 11424 * Description: <b>Multiple Resources: 11425 11426* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 11427* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 11428* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 11429* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 11430* [Citation](citation.html): A use context type and value assigned to the citation 11431* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 11432* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 11433* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 11434* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 11435* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 11436* [Evidence](evidence.html): A use context type and value assigned to the evidence 11437* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 11438* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 11439* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 11440* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 11441* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 11442* [Library](library.html): A use context type and value assigned to the library 11443* [Measure](measure.html): A use context type and value assigned to the measure 11444* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 11445* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 11446* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 11447* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 11448* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 11449* [Requirements](requirements.html): A use context type and value assigned to the requirements 11450* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 11451* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 11452* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 11453* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 11454* [TestScript](testscript.html): A use context type and value assigned to the test script 11455* [ValueSet](valueset.html): A use context type and value assigned to the value set 11456</b><br> 11457 * Type: <b>composite</b><br> 11458 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 11459 * </p> 11460 */ 11461 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 11462 11463 /** 11464 * Search parameter: <b>context-type</b> 11465 * <p> 11466 * Description: <b>Multiple Resources: 11467 11468* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 11469* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 11470* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 11471* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 11472* [Citation](citation.html): A type of use context assigned to the citation 11473* [CodeSystem](codesystem.html): A type of use context assigned to the code system 11474* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 11475* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 11476* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 11477* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 11478* [Evidence](evidence.html): A type of use context assigned to the evidence 11479* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 11480* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 11481* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 11482* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 11483* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 11484* [Library](library.html): A type of use context assigned to the library 11485* [Measure](measure.html): A type of use context assigned to the measure 11486* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 11487* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 11488* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 11489* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 11490* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 11491* [Requirements](requirements.html): A type of use context assigned to the requirements 11492* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 11493* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 11494* [StructureMap](structuremap.html): A type of use context assigned to the structure map 11495* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 11496* [TestScript](testscript.html): A type of use context assigned to the test script 11497* [ValueSet](valueset.html): A type of use context assigned to the value set 11498</b><br> 11499 * Type: <b>token</b><br> 11500 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 11501 * </p> 11502 */ 11503 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 11504 public static final String SP_CONTEXT_TYPE = "context-type"; 11505 /** 11506 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 11507 * <p> 11508 * Description: <b>Multiple Resources: 11509 11510* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 11511* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 11512* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 11513* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 11514* [Citation](citation.html): A type of use context assigned to the citation 11515* [CodeSystem](codesystem.html): A type of use context assigned to the code system 11516* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 11517* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 11518* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 11519* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 11520* [Evidence](evidence.html): A type of use context assigned to the evidence 11521* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 11522* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 11523* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 11524* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 11525* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 11526* [Library](library.html): A type of use context assigned to the library 11527* [Measure](measure.html): A type of use context assigned to the measure 11528* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 11529* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 11530* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 11531* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 11532* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 11533* [Requirements](requirements.html): A type of use context assigned to the requirements 11534* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 11535* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 11536* [StructureMap](structuremap.html): A type of use context assigned to the structure map 11537* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 11538* [TestScript](testscript.html): A type of use context assigned to the test script 11539* [ValueSet](valueset.html): A type of use context assigned to the value set 11540</b><br> 11541 * Type: <b>token</b><br> 11542 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 11543 * </p> 11544 */ 11545 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 11546 11547 /** 11548 * Search parameter: <b>context</b> 11549 * <p> 11550 * Description: <b>Multiple Resources: 11551 11552* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 11553* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 11554* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 11555* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 11556* [Citation](citation.html): A use context assigned to the citation 11557* [CodeSystem](codesystem.html): A use context assigned to the code system 11558* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 11559* [ConceptMap](conceptmap.html): A use context assigned to the concept map 11560* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 11561* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 11562* [Evidence](evidence.html): A use context assigned to the evidence 11563* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 11564* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 11565* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 11566* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 11567* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 11568* [Library](library.html): A use context assigned to the library 11569* [Measure](measure.html): A use context assigned to the measure 11570* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 11571* [NamingSystem](namingsystem.html): A use context assigned to the naming system 11572* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 11573* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 11574* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 11575* [Requirements](requirements.html): A use context assigned to the requirements 11576* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 11577* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 11578* [StructureMap](structuremap.html): A use context assigned to the structure map 11579* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 11580* [TestScript](testscript.html): A use context assigned to the test script 11581* [ValueSet](valueset.html): A use context assigned to the value set 11582</b><br> 11583 * Type: <b>token</b><br> 11584 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 11585 * </p> 11586 */ 11587 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 11588 public static final String SP_CONTEXT = "context"; 11589 /** 11590 * <b>Fluent Client</b> search parameter constant for <b>context</b> 11591 * <p> 11592 * Description: <b>Multiple Resources: 11593 11594* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 11595* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 11596* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 11597* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 11598* [Citation](citation.html): A use context assigned to the citation 11599* [CodeSystem](codesystem.html): A use context assigned to the code system 11600* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 11601* [ConceptMap](conceptmap.html): A use context assigned to the concept map 11602* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 11603* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 11604* [Evidence](evidence.html): A use context assigned to the evidence 11605* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 11606* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 11607* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 11608* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 11609* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 11610* [Library](library.html): A use context assigned to the library 11611* [Measure](measure.html): A use context assigned to the measure 11612* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 11613* [NamingSystem](namingsystem.html): A use context assigned to the naming system 11614* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 11615* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 11616* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 11617* [Requirements](requirements.html): A use context assigned to the requirements 11618* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 11619* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 11620* [StructureMap](structuremap.html): A use context assigned to the structure map 11621* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 11622* [TestScript](testscript.html): A use context assigned to the test script 11623* [ValueSet](valueset.html): A use context assigned to the value set 11624</b><br> 11625 * Type: <b>token</b><br> 11626 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 11627 * </p> 11628 */ 11629 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 11630 11631 /** 11632 * Search parameter: <b>date</b> 11633 * <p> 11634 * Description: <b>Multiple Resources: 11635 11636* [ActivityDefinition](activitydefinition.html): The activity definition publication date 11637* [ActorDefinition](actordefinition.html): The Actor Definition publication date 11638* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 11639* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 11640* [Citation](citation.html): The citation publication date 11641* [CodeSystem](codesystem.html): The code system publication date 11642* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 11643* [ConceptMap](conceptmap.html): The concept map publication date 11644* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 11645* [EventDefinition](eventdefinition.html): The event definition publication date 11646* [Evidence](evidence.html): The evidence publication date 11647* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 11648* [ExampleScenario](examplescenario.html): The example scenario publication date 11649* [GraphDefinition](graphdefinition.html): The graph definition publication date 11650* [ImplementationGuide](implementationguide.html): The implementation guide publication date 11651* [Library](library.html): The library publication date 11652* [Measure](measure.html): The measure publication date 11653* [MessageDefinition](messagedefinition.html): The message definition publication date 11654* [NamingSystem](namingsystem.html): The naming system publication date 11655* [OperationDefinition](operationdefinition.html): The operation definition publication date 11656* [PlanDefinition](plandefinition.html): The plan definition publication date 11657* [Questionnaire](questionnaire.html): The questionnaire publication date 11658* [Requirements](requirements.html): The requirements publication date 11659* [SearchParameter](searchparameter.html): The search parameter publication date 11660* [StructureDefinition](structuredefinition.html): The structure definition publication date 11661* [StructureMap](structuremap.html): The structure map publication date 11662* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 11663* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 11664* [TestScript](testscript.html): The test script publication date 11665* [ValueSet](valueset.html): The value set publication date 11666</b><br> 11667 * Type: <b>date</b><br> 11668 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 11669 * </p> 11670 */ 11671 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 11672 public static final String SP_DATE = "date"; 11673 /** 11674 * <b>Fluent Client</b> search parameter constant for <b>date</b> 11675 * <p> 11676 * Description: <b>Multiple Resources: 11677 11678* [ActivityDefinition](activitydefinition.html): The activity definition publication date 11679* [ActorDefinition](actordefinition.html): The Actor Definition publication date 11680* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 11681* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 11682* [Citation](citation.html): The citation publication date 11683* [CodeSystem](codesystem.html): The code system publication date 11684* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 11685* [ConceptMap](conceptmap.html): The concept map publication date 11686* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 11687* [EventDefinition](eventdefinition.html): The event definition publication date 11688* [Evidence](evidence.html): The evidence publication date 11689* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 11690* [ExampleScenario](examplescenario.html): The example scenario publication date 11691* [GraphDefinition](graphdefinition.html): The graph definition publication date 11692* [ImplementationGuide](implementationguide.html): The implementation guide publication date 11693* [Library](library.html): The library publication date 11694* [Measure](measure.html): The measure publication date 11695* [MessageDefinition](messagedefinition.html): The message definition publication date 11696* [NamingSystem](namingsystem.html): The naming system publication date 11697* [OperationDefinition](operationdefinition.html): The operation definition publication date 11698* [PlanDefinition](plandefinition.html): The plan definition publication date 11699* [Questionnaire](questionnaire.html): The questionnaire publication date 11700* [Requirements](requirements.html): The requirements publication date 11701* [SearchParameter](searchparameter.html): The search parameter publication date 11702* [StructureDefinition](structuredefinition.html): The structure definition publication date 11703* [StructureMap](structuremap.html): The structure map publication date 11704* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 11705* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 11706* [TestScript](testscript.html): The test script publication date 11707* [ValueSet](valueset.html): The value set publication date 11708</b><br> 11709 * Type: <b>date</b><br> 11710 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 11711 * </p> 11712 */ 11713 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 11714 11715 /** 11716 * Search parameter: <b>description</b> 11717 * <p> 11718 * Description: <b>Multiple Resources: 11719 11720* [ActivityDefinition](activitydefinition.html): The description of the activity definition 11721* [ActorDefinition](actordefinition.html): The description of the Actor Definition 11722* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 11723* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 11724* [Citation](citation.html): The description of the citation 11725* [CodeSystem](codesystem.html): The description of the code system 11726* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 11727* [ConceptMap](conceptmap.html): The description of the concept map 11728* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 11729* [EventDefinition](eventdefinition.html): The description of the event definition 11730* [Evidence](evidence.html): The description of the evidence 11731* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 11732* [GraphDefinition](graphdefinition.html): The description of the graph definition 11733* [ImplementationGuide](implementationguide.html): The description of the implementation guide 11734* [Library](library.html): The description of the library 11735* [Measure](measure.html): The description of the measure 11736* [MessageDefinition](messagedefinition.html): The description of the message definition 11737* [NamingSystem](namingsystem.html): The description of the naming system 11738* [OperationDefinition](operationdefinition.html): The description of the operation definition 11739* [PlanDefinition](plandefinition.html): The description of the plan definition 11740* [Questionnaire](questionnaire.html): The description of the questionnaire 11741* [Requirements](requirements.html): The description of the requirements 11742* [SearchParameter](searchparameter.html): The description of the search parameter 11743* [StructureDefinition](structuredefinition.html): The description of the structure definition 11744* [StructureMap](structuremap.html): The description of the structure map 11745* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 11746* [TestScript](testscript.html): The description of the test script 11747* [ValueSet](valueset.html): The description of the value set 11748</b><br> 11749 * Type: <b>string</b><br> 11750 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 11751 * </p> 11752 */ 11753 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 11754 public static final String SP_DESCRIPTION = "description"; 11755 /** 11756 * <b>Fluent Client</b> search parameter constant for <b>description</b> 11757 * <p> 11758 * Description: <b>Multiple Resources: 11759 11760* [ActivityDefinition](activitydefinition.html): The description of the activity definition 11761* [ActorDefinition](actordefinition.html): The description of the Actor Definition 11762* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 11763* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 11764* [Citation](citation.html): The description of the citation 11765* [CodeSystem](codesystem.html): The description of the code system 11766* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 11767* [ConceptMap](conceptmap.html): The description of the concept map 11768* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 11769* [EventDefinition](eventdefinition.html): The description of the event definition 11770* [Evidence](evidence.html): The description of the evidence 11771* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 11772* [GraphDefinition](graphdefinition.html): The description of the graph definition 11773* [ImplementationGuide](implementationguide.html): The description of the implementation guide 11774* [Library](library.html): The description of the library 11775* [Measure](measure.html): The description of the measure 11776* [MessageDefinition](messagedefinition.html): The description of the message definition 11777* [NamingSystem](namingsystem.html): The description of the naming system 11778* [OperationDefinition](operationdefinition.html): The description of the operation definition 11779* [PlanDefinition](plandefinition.html): The description of the plan definition 11780* [Questionnaire](questionnaire.html): The description of the questionnaire 11781* [Requirements](requirements.html): The description of the requirements 11782* [SearchParameter](searchparameter.html): The description of the search parameter 11783* [StructureDefinition](structuredefinition.html): The description of the structure definition 11784* [StructureMap](structuremap.html): The description of the structure map 11785* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 11786* [TestScript](testscript.html): The description of the test script 11787* [ValueSet](valueset.html): The description of the value set 11788</b><br> 11789 * Type: <b>string</b><br> 11790 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 11791 * </p> 11792 */ 11793 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 11794 11795 /** 11796 * Search parameter: <b>identifier</b> 11797 * <p> 11798 * Description: <b>Multiple Resources: 11799 11800* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 11801* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 11802* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 11803* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 11804* [Citation](citation.html): External identifier for the citation 11805* [CodeSystem](codesystem.html): External identifier for the code system 11806* [ConceptMap](conceptmap.html): External identifier for the concept map 11807* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 11808* [EventDefinition](eventdefinition.html): External identifier for the event definition 11809* [Evidence](evidence.html): External identifier for the evidence 11810* [EvidenceReport](evidencereport.html): External identifier for the evidence report 11811* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 11812* [ExampleScenario](examplescenario.html): External identifier for the example scenario 11813* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 11814* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 11815* [Library](library.html): External identifier for the library 11816* [Measure](measure.html): External identifier for the measure 11817* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 11818* [MessageDefinition](messagedefinition.html): External identifier for the message definition 11819* [NamingSystem](namingsystem.html): External identifier for the naming system 11820* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 11821* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 11822* [PlanDefinition](plandefinition.html): External identifier for the plan definition 11823* [Questionnaire](questionnaire.html): External identifier for the questionnaire 11824* [Requirements](requirements.html): External identifier for the requirements 11825* [SearchParameter](searchparameter.html): External identifier for the search parameter 11826* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 11827* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 11828* [StructureMap](structuremap.html): External identifier for the structure map 11829* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 11830* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 11831* [TestPlan](testplan.html): An identifier for the test plan 11832* [TestScript](testscript.html): External identifier for the test script 11833* [ValueSet](valueset.html): External identifier for the value set 11834</b><br> 11835 * Type: <b>token</b><br> 11836 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 11837 * </p> 11838 */ 11839 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 11840 public static final String SP_IDENTIFIER = "identifier"; 11841 /** 11842 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 11843 * <p> 11844 * Description: <b>Multiple Resources: 11845 11846* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 11847* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 11848* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 11849* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 11850* [Citation](citation.html): External identifier for the citation 11851* [CodeSystem](codesystem.html): External identifier for the code system 11852* [ConceptMap](conceptmap.html): External identifier for the concept map 11853* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 11854* [EventDefinition](eventdefinition.html): External identifier for the event definition 11855* [Evidence](evidence.html): External identifier for the evidence 11856* [EvidenceReport](evidencereport.html): External identifier for the evidence report 11857* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 11858* [ExampleScenario](examplescenario.html): External identifier for the example scenario 11859* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 11860* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 11861* [Library](library.html): External identifier for the library 11862* [Measure](measure.html): External identifier for the measure 11863* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 11864* [MessageDefinition](messagedefinition.html): External identifier for the message definition 11865* [NamingSystem](namingsystem.html): External identifier for the naming system 11866* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 11867* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 11868* [PlanDefinition](plandefinition.html): External identifier for the plan definition 11869* [Questionnaire](questionnaire.html): External identifier for the questionnaire 11870* [Requirements](requirements.html): External identifier for the requirements 11871* [SearchParameter](searchparameter.html): External identifier for the search parameter 11872* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 11873* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 11874* [StructureMap](structuremap.html): External identifier for the structure map 11875* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 11876* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 11877* [TestPlan](testplan.html): An identifier for the test plan 11878* [TestScript](testscript.html): External identifier for the test script 11879* [ValueSet](valueset.html): External identifier for the value set 11880</b><br> 11881 * Type: <b>token</b><br> 11882 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 11883 * </p> 11884 */ 11885 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 11886 11887 /** 11888 * Search parameter: <b>jurisdiction</b> 11889 * <p> 11890 * Description: <b>Multiple Resources: 11891 11892* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 11893* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 11894* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 11895* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 11896* [Citation](citation.html): Intended jurisdiction for the citation 11897* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 11898* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 11899* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 11900* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 11901* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 11902* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 11903* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 11904* [Library](library.html): Intended jurisdiction for the library 11905* [Measure](measure.html): Intended jurisdiction for the measure 11906* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 11907* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 11908* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 11909* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 11910* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 11911* [Requirements](requirements.html): Intended jurisdiction for the requirements 11912* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 11913* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 11914* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 11915* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 11916* [TestScript](testscript.html): Intended jurisdiction for the test script 11917* [ValueSet](valueset.html): Intended jurisdiction for the value set 11918</b><br> 11919 * Type: <b>token</b><br> 11920 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 11921 * </p> 11922 */ 11923 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 11924 public static final String SP_JURISDICTION = "jurisdiction"; 11925 /** 11926 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 11927 * <p> 11928 * Description: <b>Multiple Resources: 11929 11930* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 11931* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 11932* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 11933* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 11934* [Citation](citation.html): Intended jurisdiction for the citation 11935* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 11936* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 11937* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 11938* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 11939* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 11940* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 11941* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 11942* [Library](library.html): Intended jurisdiction for the library 11943* [Measure](measure.html): Intended jurisdiction for the measure 11944* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 11945* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 11946* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 11947* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 11948* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 11949* [Requirements](requirements.html): Intended jurisdiction for the requirements 11950* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 11951* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 11952* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 11953* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 11954* [TestScript](testscript.html): Intended jurisdiction for the test script 11955* [ValueSet](valueset.html): Intended jurisdiction for the value set 11956</b><br> 11957 * Type: <b>token</b><br> 11958 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 11959 * </p> 11960 */ 11961 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 11962 11963 /** 11964 * Search parameter: <b>name</b> 11965 * <p> 11966 * Description: <b>Multiple Resources: 11967 11968* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 11969* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 11970* [Citation](citation.html): Computationally friendly name of the citation 11971* [CodeSystem](codesystem.html): Computationally friendly name of the code system 11972* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 11973* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 11974* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 11975* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 11976* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 11977* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 11978* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 11979* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 11980* [Library](library.html): Computationally friendly name of the library 11981* [Measure](measure.html): Computationally friendly name of the measure 11982* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 11983* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 11984* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 11985* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 11986* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 11987* [Requirements](requirements.html): Computationally friendly name of the requirements 11988* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 11989* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 11990* [StructureMap](structuremap.html): Computationally friendly name of the structure map 11991* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 11992* [TestScript](testscript.html): Computationally friendly name of the test script 11993* [ValueSet](valueset.html): Computationally friendly name of the value set 11994</b><br> 11995 * Type: <b>string</b><br> 11996 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 11997 * </p> 11998 */ 11999 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 12000 public static final String SP_NAME = "name"; 12001 /** 12002 * <b>Fluent Client</b> search parameter constant for <b>name</b> 12003 * <p> 12004 * Description: <b>Multiple Resources: 12005 12006* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 12007* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 12008* [Citation](citation.html): Computationally friendly name of the citation 12009* [CodeSystem](codesystem.html): Computationally friendly name of the code system 12010* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 12011* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 12012* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 12013* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 12014* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 12015* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 12016* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 12017* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 12018* [Library](library.html): Computationally friendly name of the library 12019* [Measure](measure.html): Computationally friendly name of the measure 12020* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 12021* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 12022* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 12023* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 12024* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 12025* [Requirements](requirements.html): Computationally friendly name of the requirements 12026* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 12027* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 12028* [StructureMap](structuremap.html): Computationally friendly name of the structure map 12029* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 12030* [TestScript](testscript.html): Computationally friendly name of the test script 12031* [ValueSet](valueset.html): Computationally friendly name of the value set 12032</b><br> 12033 * Type: <b>string</b><br> 12034 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 12035 * </p> 12036 */ 12037 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 12038 12039 /** 12040 * Search parameter: <b>publisher</b> 12041 * <p> 12042 * Description: <b>Multiple Resources: 12043 12044* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 12045* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 12046* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 12047* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 12048* [Citation](citation.html): Name of the publisher of the citation 12049* [CodeSystem](codesystem.html): Name of the publisher of the code system 12050* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 12051* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 12052* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 12053* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 12054* [Evidence](evidence.html): Name of the publisher of the evidence 12055* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 12056* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 12057* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 12058* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 12059* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 12060* [Library](library.html): Name of the publisher of the library 12061* [Measure](measure.html): Name of the publisher of the measure 12062* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 12063* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 12064* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 12065* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 12066* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 12067* [Requirements](requirements.html): Name of the publisher of the requirements 12068* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 12069* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 12070* [StructureMap](structuremap.html): Name of the publisher of the structure map 12071* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 12072* [TestScript](testscript.html): Name of the publisher of the test script 12073* [ValueSet](valueset.html): Name of the publisher of the value set 12074</b><br> 12075 * Type: <b>string</b><br> 12076 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 12077 * </p> 12078 */ 12079 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 12080 public static final String SP_PUBLISHER = "publisher"; 12081 /** 12082 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 12083 * <p> 12084 * Description: <b>Multiple Resources: 12085 12086* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 12087* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 12088* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 12089* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 12090* [Citation](citation.html): Name of the publisher of the citation 12091* [CodeSystem](codesystem.html): Name of the publisher of the code system 12092* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 12093* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 12094* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 12095* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 12096* [Evidence](evidence.html): Name of the publisher of the evidence 12097* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 12098* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 12099* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 12100* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 12101* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 12102* [Library](library.html): Name of the publisher of the library 12103* [Measure](measure.html): Name of the publisher of the measure 12104* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 12105* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 12106* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 12107* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 12108* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 12109* [Requirements](requirements.html): Name of the publisher of the requirements 12110* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 12111* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 12112* [StructureMap](structuremap.html): Name of the publisher of the structure map 12113* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 12114* [TestScript](testscript.html): Name of the publisher of the test script 12115* [ValueSet](valueset.html): Name of the publisher of the value set 12116</b><br> 12117 * Type: <b>string</b><br> 12118 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 12119 * </p> 12120 */ 12121 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 12122 12123 /** 12124 * Search parameter: <b>status</b> 12125 * <p> 12126 * Description: <b>Multiple Resources: 12127 12128* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 12129* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 12130* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 12131* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 12132* [Citation](citation.html): The current status of the citation 12133* [CodeSystem](codesystem.html): The current status of the code system 12134* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 12135* [ConceptMap](conceptmap.html): The current status of the concept map 12136* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 12137* [EventDefinition](eventdefinition.html): The current status of the event definition 12138* [Evidence](evidence.html): The current status of the evidence 12139* [EvidenceReport](evidencereport.html): The current status of the evidence report 12140* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 12141* [ExampleScenario](examplescenario.html): The current status of the example scenario 12142* [GraphDefinition](graphdefinition.html): The current status of the graph definition 12143* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 12144* [Library](library.html): The current status of the library 12145* [Measure](measure.html): The current status of the measure 12146* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 12147* [MessageDefinition](messagedefinition.html): The current status of the message definition 12148* [NamingSystem](namingsystem.html): The current status of the naming system 12149* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 12150* [OperationDefinition](operationdefinition.html): The current status of the operation definition 12151* [PlanDefinition](plandefinition.html): The current status of the plan definition 12152* [Questionnaire](questionnaire.html): The current status of the questionnaire 12153* [Requirements](requirements.html): The current status of the requirements 12154* [SearchParameter](searchparameter.html): The current status of the search parameter 12155* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 12156* [StructureDefinition](structuredefinition.html): The current status of the structure definition 12157* [StructureMap](structuremap.html): The current status of the structure map 12158* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 12159* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 12160* [TestPlan](testplan.html): The current status of the test plan 12161* [TestScript](testscript.html): The current status of the test script 12162* [ValueSet](valueset.html): The current status of the value set 12163</b><br> 12164 * Type: <b>token</b><br> 12165 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 12166 * </p> 12167 */ 12168 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 12169 public static final String SP_STATUS = "status"; 12170 /** 12171 * <b>Fluent Client</b> search parameter constant for <b>status</b> 12172 * <p> 12173 * Description: <b>Multiple Resources: 12174 12175* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 12176* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 12177* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 12178* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 12179* [Citation](citation.html): The current status of the citation 12180* [CodeSystem](codesystem.html): The current status of the code system 12181* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 12182* [ConceptMap](conceptmap.html): The current status of the concept map 12183* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 12184* [EventDefinition](eventdefinition.html): The current status of the event definition 12185* [Evidence](evidence.html): The current status of the evidence 12186* [EvidenceReport](evidencereport.html): The current status of the evidence report 12187* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 12188* [ExampleScenario](examplescenario.html): The current status of the example scenario 12189* [GraphDefinition](graphdefinition.html): The current status of the graph definition 12190* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 12191* [Library](library.html): The current status of the library 12192* [Measure](measure.html): The current status of the measure 12193* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 12194* [MessageDefinition](messagedefinition.html): The current status of the message definition 12195* [NamingSystem](namingsystem.html): The current status of the naming system 12196* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 12197* [OperationDefinition](operationdefinition.html): The current status of the operation definition 12198* [PlanDefinition](plandefinition.html): The current status of the plan definition 12199* [Questionnaire](questionnaire.html): The current status of the questionnaire 12200* [Requirements](requirements.html): The current status of the requirements 12201* [SearchParameter](searchparameter.html): The current status of the search parameter 12202* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 12203* [StructureDefinition](structuredefinition.html): The current status of the structure definition 12204* [StructureMap](structuremap.html): The current status of the structure map 12205* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 12206* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 12207* [TestPlan](testplan.html): The current status of the test plan 12208* [TestScript](testscript.html): The current status of the test script 12209* [ValueSet](valueset.html): The current status of the value set 12210</b><br> 12211 * Type: <b>token</b><br> 12212 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 12213 * </p> 12214 */ 12215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 12216 12217 /** 12218 * Search parameter: <b>title</b> 12219 * <p> 12220 * Description: <b>Multiple Resources: 12221 12222* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 12223* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 12224* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 12225* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 12226* [Citation](citation.html): The human-friendly name of the citation 12227* [CodeSystem](codesystem.html): The human-friendly name of the code system 12228* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 12229* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 12230* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 12231* [Evidence](evidence.html): The human-friendly name of the evidence 12232* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 12233* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 12234* [Library](library.html): The human-friendly name of the library 12235* [Measure](measure.html): The human-friendly name of the measure 12236* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 12237* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 12238* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 12239* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 12240* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 12241* [Requirements](requirements.html): The human-friendly name of the requirements 12242* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 12243* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 12244* [StructureMap](structuremap.html): The human-friendly name of the structure map 12245* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 12246* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 12247* [TestScript](testscript.html): The human-friendly name of the test script 12248* [ValueSet](valueset.html): The human-friendly name of the value set 12249</b><br> 12250 * Type: <b>string</b><br> 12251 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 12252 * </p> 12253 */ 12254 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 12255 public static final String SP_TITLE = "title"; 12256 /** 12257 * <b>Fluent Client</b> search parameter constant for <b>title</b> 12258 * <p> 12259 * Description: <b>Multiple Resources: 12260 12261* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 12262* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 12263* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 12264* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 12265* [Citation](citation.html): The human-friendly name of the citation 12266* [CodeSystem](codesystem.html): The human-friendly name of the code system 12267* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 12268* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 12269* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 12270* [Evidence](evidence.html): The human-friendly name of the evidence 12271* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 12272* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 12273* [Library](library.html): The human-friendly name of the library 12274* [Measure](measure.html): The human-friendly name of the measure 12275* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 12276* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 12277* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 12278* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 12279* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 12280* [Requirements](requirements.html): The human-friendly name of the requirements 12281* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 12282* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 12283* [StructureMap](structuremap.html): The human-friendly name of the structure map 12284* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 12285* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 12286* [TestScript](testscript.html): The human-friendly name of the test script 12287* [ValueSet](valueset.html): The human-friendly name of the value set 12288</b><br> 12289 * Type: <b>string</b><br> 12290 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 12291 * </p> 12292 */ 12293 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 12294 12295 /** 12296 * Search parameter: <b>url</b> 12297 * <p> 12298 * Description: <b>Multiple Resources: 12299 12300* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 12301* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 12302* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 12303* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 12304* [Citation](citation.html): The uri that identifies the citation 12305* [CodeSystem](codesystem.html): The uri that identifies the code system 12306* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 12307* [ConceptMap](conceptmap.html): The URI that identifies the concept map 12308* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 12309* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 12310* [Evidence](evidence.html): The uri that identifies the evidence 12311* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 12312* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 12313* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 12314* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 12315* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 12316* [Library](library.html): The uri that identifies the library 12317* [Measure](measure.html): The uri that identifies the measure 12318* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 12319* [NamingSystem](namingsystem.html): The uri that identifies the naming system 12320* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 12321* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 12322* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 12323* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 12324* [Requirements](requirements.html): The uri that identifies the requirements 12325* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 12326* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 12327* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 12328* [StructureMap](structuremap.html): The uri that identifies the structure map 12329* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 12330* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 12331* [TestPlan](testplan.html): The uri that identifies the test plan 12332* [TestScript](testscript.html): The uri that identifies the test script 12333* [ValueSet](valueset.html): The uri that identifies the value set 12334</b><br> 12335 * Type: <b>uri</b><br> 12336 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 12337 * </p> 12338 */ 12339 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 12340 public static final String SP_URL = "url"; 12341 /** 12342 * <b>Fluent Client</b> search parameter constant for <b>url</b> 12343 * <p> 12344 * Description: <b>Multiple Resources: 12345 12346* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 12347* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 12348* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 12349* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 12350* [Citation](citation.html): The uri that identifies the citation 12351* [CodeSystem](codesystem.html): The uri that identifies the code system 12352* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 12353* [ConceptMap](conceptmap.html): The URI that identifies the concept map 12354* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 12355* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 12356* [Evidence](evidence.html): The uri that identifies the evidence 12357* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 12358* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 12359* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 12360* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 12361* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 12362* [Library](library.html): The uri that identifies the library 12363* [Measure](measure.html): The uri that identifies the measure 12364* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 12365* [NamingSystem](namingsystem.html): The uri that identifies the naming system 12366* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 12367* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 12368* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 12369* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 12370* [Requirements](requirements.html): The uri that identifies the requirements 12371* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 12372* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 12373* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 12374* [StructureMap](structuremap.html): The uri that identifies the structure map 12375* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 12376* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 12377* [TestPlan](testplan.html): The uri that identifies the test plan 12378* [TestScript](testscript.html): The uri that identifies the test script 12379* [ValueSet](valueset.html): The uri that identifies the value set 12380</b><br> 12381 * Type: <b>uri</b><br> 12382 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 12383 * </p> 12384 */ 12385 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 12386 12387 /** 12388 * Search parameter: <b>version</b> 12389 * <p> 12390 * Description: <b>Multiple Resources: 12391 12392* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 12393* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 12394* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 12395* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 12396* [Citation](citation.html): The business version of the citation 12397* [CodeSystem](codesystem.html): The business version of the code system 12398* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 12399* [ConceptMap](conceptmap.html): The business version of the concept map 12400* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 12401* [EventDefinition](eventdefinition.html): The business version of the event definition 12402* [Evidence](evidence.html): The business version of the evidence 12403* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 12404* [ExampleScenario](examplescenario.html): The business version of the example scenario 12405* [GraphDefinition](graphdefinition.html): The business version of the graph definition 12406* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 12407* [Library](library.html): The business version of the library 12408* [Measure](measure.html): The business version of the measure 12409* [MessageDefinition](messagedefinition.html): The business version of the message definition 12410* [NamingSystem](namingsystem.html): The business version of the naming system 12411* [OperationDefinition](operationdefinition.html): The business version of the operation definition 12412* [PlanDefinition](plandefinition.html): The business version of the plan definition 12413* [Questionnaire](questionnaire.html): The business version of the questionnaire 12414* [Requirements](requirements.html): The business version of the requirements 12415* [SearchParameter](searchparameter.html): The business version of the search parameter 12416* [StructureDefinition](structuredefinition.html): The business version of the structure definition 12417* [StructureMap](structuremap.html): The business version of the structure map 12418* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 12419* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 12420* [TestScript](testscript.html): The business version of the test script 12421* [ValueSet](valueset.html): The business version of the value set 12422</b><br> 12423 * Type: <b>token</b><br> 12424 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 12425 * </p> 12426 */ 12427 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 12428 public static final String SP_VERSION = "version"; 12429 /** 12430 * <b>Fluent Client</b> search parameter constant for <b>version</b> 12431 * <p> 12432 * Description: <b>Multiple Resources: 12433 12434* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 12435* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 12436* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 12437* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 12438* [Citation](citation.html): The business version of the citation 12439* [CodeSystem](codesystem.html): The business version of the code system 12440* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 12441* [ConceptMap](conceptmap.html): The business version of the concept map 12442* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 12443* [EventDefinition](eventdefinition.html): The business version of the event definition 12444* [Evidence](evidence.html): The business version of the evidence 12445* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 12446* [ExampleScenario](examplescenario.html): The business version of the example scenario 12447* [GraphDefinition](graphdefinition.html): The business version of the graph definition 12448* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 12449* [Library](library.html): The business version of the library 12450* [Measure](measure.html): The business version of the measure 12451* [MessageDefinition](messagedefinition.html): The business version of the message definition 12452* [NamingSystem](namingsystem.html): The business version of the naming system 12453* [OperationDefinition](operationdefinition.html): The business version of the operation definition 12454* [PlanDefinition](plandefinition.html): The business version of the plan definition 12455* [Questionnaire](questionnaire.html): The business version of the questionnaire 12456* [Requirements](requirements.html): The business version of the requirements 12457* [SearchParameter](searchparameter.html): The business version of the search parameter 12458* [StructureDefinition](structuredefinition.html): The business version of the structure definition 12459* [StructureMap](structuremap.html): The business version of the structure map 12460* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 12461* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 12462* [TestScript](testscript.html): The business version of the test script 12463* [ValueSet](valueset.html): The business version of the value set 12464</b><br> 12465 * Type: <b>token</b><br> 12466 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 12467 * </p> 12468 */ 12469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 12470 12471 /** 12472 * Search parameter: <b>classification-type</b> 12473 * <p> 12474 * Description: <b>A type of classification assigned to the citation</b><br> 12475 * Type: <b>token</b><br> 12476 * Path: <b>(Citation.classification.type)</b><br> 12477 * </p> 12478 */ 12479 @SearchParamDefinition(name="classification-type", path="(Citation.classification.type)", description="A type of classification assigned to the citation", type="token" ) 12480 public static final String SP_CLASSIFICATION_TYPE = "classification-type"; 12481 /** 12482 * <b>Fluent Client</b> search parameter constant for <b>classification-type</b> 12483 * <p> 12484 * Description: <b>A type of classification assigned to the citation</b><br> 12485 * Type: <b>token</b><br> 12486 * Path: <b>(Citation.classification.type)</b><br> 12487 * </p> 12488 */ 12489 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFICATION_TYPE); 12490 12491 /** 12492 * Search parameter: <b>classification</b> 12493 * <p> 12494 * Description: <b>A classification type and value assigned to the citation</b><br> 12495 * Type: <b>composite</b><br> 12496 * Path: <b>Citation.classification</b><br> 12497 * </p> 12498 */ 12499 @SearchParamDefinition(name="classification", path="Citation.classification", description="A classification type and value assigned to the citation", type="composite", compositeOf={"classification-type", "classifier"} ) 12500 public static final String SP_CLASSIFICATION = "classification"; 12501 /** 12502 * <b>Fluent Client</b> search parameter constant for <b>classification</b> 12503 * <p> 12504 * Description: <b>A classification type and value assigned to the citation</b><br> 12505 * Type: <b>composite</b><br> 12506 * Path: <b>Citation.classification</b><br> 12507 * </p> 12508 */ 12509 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CLASSIFICATION = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CLASSIFICATION); 12510 12511 /** 12512 * Search parameter: <b>classifier</b> 12513 * <p> 12514 * Description: <b>A classifier assigned to the citation</b><br> 12515 * Type: <b>token</b><br> 12516 * Path: <b>(Citation.classification.classifier)</b><br> 12517 * </p> 12518 */ 12519 @SearchParamDefinition(name="classifier", path="(Citation.classification.classifier)", description="A classifier assigned to the citation", type="token" ) 12520 public static final String SP_CLASSIFIER = "classifier"; 12521 /** 12522 * <b>Fluent Client</b> search parameter constant for <b>classifier</b> 12523 * <p> 12524 * Description: <b>A classifier assigned to the citation</b><br> 12525 * Type: <b>token</b><br> 12526 * Path: <b>(Citation.classification.classifier)</b><br> 12527 * </p> 12528 */ 12529 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFIER); 12530 12531 /** 12532 * Search parameter: <b>effective</b> 12533 * <p> 12534 * Description: <b>Multiple Resources: 12535 12536* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 12537* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 12538* [Citation](citation.html): The time during which the citation is intended to be in use 12539* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 12540* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 12541* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 12542* [Library](library.html): The time during which the library is intended to be in use 12543* [Measure](measure.html): The time during which the measure is intended to be in use 12544* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 12545* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 12546* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 12547* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 12548</b><br> 12549 * Type: <b>date</b><br> 12550 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 12551 * </p> 12552 */ 12553 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 12554 public static final String SP_EFFECTIVE = "effective"; 12555 /** 12556 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 12557 * <p> 12558 * Description: <b>Multiple Resources: 12559 12560* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 12561* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 12562* [Citation](citation.html): The time during which the citation is intended to be in use 12563* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 12564* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 12565* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 12566* [Library](library.html): The time during which the library is intended to be in use 12567* [Measure](measure.html): The time during which the measure is intended to be in use 12568* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 12569* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 12570* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 12571* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 12572</b><br> 12573 * Type: <b>date</b><br> 12574 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 12575 * </p> 12576 */ 12577 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 12578 12579 12580} 12581