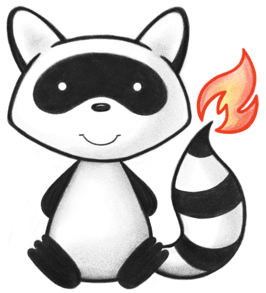
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement. 053 */ 054@ResourceDef(name="Claim", profile="http://hl7.org/fhir/StructureDefinition/Claim") 055public class Claim extends DomainResource { 056 057 @Block() 058 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * Reference to a related claim. 061 */ 062 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 063 @Description(shortDefinition="Reference to the related claim", formalDefinition="Reference to a related claim." ) 064 protected Reference claim; 065 066 /** 067 * A code to convey how the claims are related. 068 */ 069 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="How the reference claim is related", formalDefinition="A code to convey how the claims are related." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 072 protected CodeableConcept relationship; 073 074 /** 075 * An alternate organizational reference to the case or file to which this particular claim pertains. 076 */ 077 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 078 @Description(shortDefinition="File or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains." ) 079 protected Identifier reference; 080 081 private static final long serialVersionUID = 1047077926L; 082 083 /** 084 * Constructor 085 */ 086 public RelatedClaimComponent() { 087 super(); 088 } 089 090 /** 091 * @return {@link #claim} (Reference to a related claim.) 092 */ 093 public Reference getClaim() { 094 if (this.claim == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 097 else if (Configuration.doAutoCreate()) 098 this.claim = new Reference(); // cc 099 return this.claim; 100 } 101 102 public boolean hasClaim() { 103 return this.claim != null && !this.claim.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #claim} (Reference to a related claim.) 108 */ 109 public RelatedClaimComponent setClaim(Reference value) { 110 this.claim = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #relationship} (A code to convey how the claims are related.) 116 */ 117 public CodeableConcept getRelationship() { 118 if (this.relationship == null) 119 if (Configuration.errorOnAutoCreate()) 120 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 121 else if (Configuration.doAutoCreate()) 122 this.relationship = new CodeableConcept(); // cc 123 return this.relationship; 124 } 125 126 public boolean hasRelationship() { 127 return this.relationship != null && !this.relationship.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #relationship} (A code to convey how the claims are related.) 132 */ 133 public RelatedClaimComponent setRelationship(CodeableConcept value) { 134 this.relationship = value; 135 return this; 136 } 137 138 /** 139 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 140 */ 141 public Identifier getReference() { 142 if (this.reference == null) 143 if (Configuration.errorOnAutoCreate()) 144 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 145 else if (Configuration.doAutoCreate()) 146 this.reference = new Identifier(); // cc 147 return this.reference; 148 } 149 150 public boolean hasReference() { 151 return this.reference != null && !this.reference.isEmpty(); 152 } 153 154 /** 155 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 156 */ 157 public RelatedClaimComponent setReference(Identifier value) { 158 this.reference = value; 159 return this; 160 } 161 162 protected void listChildren(List<Property> children) { 163 super.listChildren(children); 164 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 165 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship)); 166 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference)); 167 } 168 169 @Override 170 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 171 switch (_hash) { 172 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 173 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship); 174 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference); 175 default: return super.getNamedProperty(_hash, _name, _checkValid); 176 } 177 178 } 179 180 @Override 181 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 182 switch (hash) { 183 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 184 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 185 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 186 default: return super.getProperty(hash, name, checkValid); 187 } 188 189 } 190 191 @Override 192 public Base setProperty(int hash, String name, Base value) throws FHIRException { 193 switch (hash) { 194 case 94742588: // claim 195 this.claim = TypeConvertor.castToReference(value); // Reference 196 return value; 197 case -261851592: // relationship 198 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 199 return value; 200 case -925155509: // reference 201 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 202 return value; 203 default: return super.setProperty(hash, name, value); 204 } 205 206 } 207 208 @Override 209 public Base setProperty(String name, Base value) throws FHIRException { 210 if (name.equals("claim")) { 211 this.claim = TypeConvertor.castToReference(value); // Reference 212 } else if (name.equals("relationship")) { 213 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 214 } else if (name.equals("reference")) { 215 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 216 } else 217 return super.setProperty(name, value); 218 return value; 219 } 220 221 @Override 222 public Base makeProperty(int hash, String name) throws FHIRException { 223 switch (hash) { 224 case 94742588: return getClaim(); 225 case -261851592: return getRelationship(); 226 case -925155509: return getReference(); 227 default: return super.makeProperty(hash, name); 228 } 229 230 } 231 232 @Override 233 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 234 switch (hash) { 235 case 94742588: /*claim*/ return new String[] {"Reference"}; 236 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 237 case -925155509: /*reference*/ return new String[] {"Identifier"}; 238 default: return super.getTypesForProperty(hash, name); 239 } 240 241 } 242 243 @Override 244 public Base addChild(String name) throws FHIRException { 245 if (name.equals("claim")) { 246 this.claim = new Reference(); 247 return this.claim; 248 } 249 else if (name.equals("relationship")) { 250 this.relationship = new CodeableConcept(); 251 return this.relationship; 252 } 253 else if (name.equals("reference")) { 254 this.reference = new Identifier(); 255 return this.reference; 256 } 257 else 258 return super.addChild(name); 259 } 260 261 public RelatedClaimComponent copy() { 262 RelatedClaimComponent dst = new RelatedClaimComponent(); 263 copyValues(dst); 264 return dst; 265 } 266 267 public void copyValues(RelatedClaimComponent dst) { 268 super.copyValues(dst); 269 dst.claim = claim == null ? null : claim.copy(); 270 dst.relationship = relationship == null ? null : relationship.copy(); 271 dst.reference = reference == null ? null : reference.copy(); 272 } 273 274 @Override 275 public boolean equalsDeep(Base other_) { 276 if (!super.equalsDeep(other_)) 277 return false; 278 if (!(other_ instanceof RelatedClaimComponent)) 279 return false; 280 RelatedClaimComponent o = (RelatedClaimComponent) other_; 281 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 282 ; 283 } 284 285 @Override 286 public boolean equalsShallow(Base other_) { 287 if (!super.equalsShallow(other_)) 288 return false; 289 if (!(other_ instanceof RelatedClaimComponent)) 290 return false; 291 RelatedClaimComponent o = (RelatedClaimComponent) other_; 292 return true; 293 } 294 295 public boolean isEmpty() { 296 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 297 ); 298 } 299 300 public String fhirType() { 301 return "Claim.related"; 302 303 } 304 305 } 306 307 @Block() 308 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 309 /** 310 * Type of Party to be reimbursed: subscriber, provider, other. 311 */ 312 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 313 @Description(shortDefinition="Category of recipient", formalDefinition="Type of Party to be reimbursed: subscriber, provider, other." ) 314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 315 protected CodeableConcept type; 316 317 /** 318 * Reference to the individual or organization to whom any payment will be made. 319 */ 320 @Child(name = "party", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class}, order=2, min=0, max=1, modifier=false, summary=false) 321 @Description(shortDefinition="Recipient reference", formalDefinition="Reference to the individual or organization to whom any payment will be made." ) 322 protected Reference party; 323 324 private static final long serialVersionUID = -1948897146L; 325 326 /** 327 * Constructor 328 */ 329 public PayeeComponent() { 330 super(); 331 } 332 333 /** 334 * Constructor 335 */ 336 public PayeeComponent(CodeableConcept type) { 337 super(); 338 this.setType(type); 339 } 340 341 /** 342 * @return {@link #type} (Type of Party to be reimbursed: subscriber, provider, other.) 343 */ 344 public CodeableConcept getType() { 345 if (this.type == null) 346 if (Configuration.errorOnAutoCreate()) 347 throw new Error("Attempt to auto-create PayeeComponent.type"); 348 else if (Configuration.doAutoCreate()) 349 this.type = new CodeableConcept(); // cc 350 return this.type; 351 } 352 353 public boolean hasType() { 354 return this.type != null && !this.type.isEmpty(); 355 } 356 357 /** 358 * @param value {@link #type} (Type of Party to be reimbursed: subscriber, provider, other.) 359 */ 360 public PayeeComponent setType(CodeableConcept value) { 361 this.type = value; 362 return this; 363 } 364 365 /** 366 * @return {@link #party} (Reference to the individual or organization to whom any payment will be made.) 367 */ 368 public Reference getParty() { 369 if (this.party == null) 370 if (Configuration.errorOnAutoCreate()) 371 throw new Error("Attempt to auto-create PayeeComponent.party"); 372 else if (Configuration.doAutoCreate()) 373 this.party = new Reference(); // cc 374 return this.party; 375 } 376 377 public boolean hasParty() { 378 return this.party != null && !this.party.isEmpty(); 379 } 380 381 /** 382 * @param value {@link #party} (Reference to the individual or organization to whom any payment will be made.) 383 */ 384 public PayeeComponent setParty(Reference value) { 385 this.party = value; 386 return this; 387 } 388 389 protected void listChildren(List<Property> children) { 390 super.listChildren(children); 391 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type)); 392 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 393 } 394 395 @Override 396 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 397 switch (_hash) { 398 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type); 399 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 400 default: return super.getNamedProperty(_hash, _name, _checkValid); 401 } 402 403 } 404 405 @Override 406 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 407 switch (hash) { 408 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 409 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 410 default: return super.getProperty(hash, name, checkValid); 411 } 412 413 } 414 415 @Override 416 public Base setProperty(int hash, String name, Base value) throws FHIRException { 417 switch (hash) { 418 case 3575610: // type 419 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 420 return value; 421 case 106437350: // party 422 this.party = TypeConvertor.castToReference(value); // Reference 423 return value; 424 default: return super.setProperty(hash, name, value); 425 } 426 427 } 428 429 @Override 430 public Base setProperty(String name, Base value) throws FHIRException { 431 if (name.equals("type")) { 432 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 433 } else if (name.equals("party")) { 434 this.party = TypeConvertor.castToReference(value); // Reference 435 } else 436 return super.setProperty(name, value); 437 return value; 438 } 439 440 @Override 441 public Base makeProperty(int hash, String name) throws FHIRException { 442 switch (hash) { 443 case 3575610: return getType(); 444 case 106437350: return getParty(); 445 default: return super.makeProperty(hash, name); 446 } 447 448 } 449 450 @Override 451 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 452 switch (hash) { 453 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 454 case 106437350: /*party*/ return new String[] {"Reference"}; 455 default: return super.getTypesForProperty(hash, name); 456 } 457 458 } 459 460 @Override 461 public Base addChild(String name) throws FHIRException { 462 if (name.equals("type")) { 463 this.type = new CodeableConcept(); 464 return this.type; 465 } 466 else if (name.equals("party")) { 467 this.party = new Reference(); 468 return this.party; 469 } 470 else 471 return super.addChild(name); 472 } 473 474 public PayeeComponent copy() { 475 PayeeComponent dst = new PayeeComponent(); 476 copyValues(dst); 477 return dst; 478 } 479 480 public void copyValues(PayeeComponent dst) { 481 super.copyValues(dst); 482 dst.type = type == null ? null : type.copy(); 483 dst.party = party == null ? null : party.copy(); 484 } 485 486 @Override 487 public boolean equalsDeep(Base other_) { 488 if (!super.equalsDeep(other_)) 489 return false; 490 if (!(other_ instanceof PayeeComponent)) 491 return false; 492 PayeeComponent o = (PayeeComponent) other_; 493 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 494 } 495 496 @Override 497 public boolean equalsShallow(Base other_) { 498 if (!super.equalsShallow(other_)) 499 return false; 500 if (!(other_ instanceof PayeeComponent)) 501 return false; 502 PayeeComponent o = (PayeeComponent) other_; 503 return true; 504 } 505 506 public boolean isEmpty() { 507 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 508 } 509 510 public String fhirType() { 511 return "Claim.payee"; 512 513 } 514 515 } 516 517 @Block() 518 public static class ClaimEventComponent extends BackboneElement implements IBaseBackboneElement { 519 /** 520 * A coded event such as when a service is expected or a card printed. 521 */ 522 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 523 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 524 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 525 protected CodeableConcept type; 526 527 /** 528 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 529 */ 530 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 531 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 532 protected DataType when; 533 534 private static final long serialVersionUID = -634897375L; 535 536 /** 537 * Constructor 538 */ 539 public ClaimEventComponent() { 540 super(); 541 } 542 543 /** 544 * Constructor 545 */ 546 public ClaimEventComponent(CodeableConcept type, DataType when) { 547 super(); 548 this.setType(type); 549 this.setWhen(when); 550 } 551 552 /** 553 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 554 */ 555 public CodeableConcept getType() { 556 if (this.type == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create ClaimEventComponent.type"); 559 else if (Configuration.doAutoCreate()) 560 this.type = new CodeableConcept(); // cc 561 return this.type; 562 } 563 564 public boolean hasType() { 565 return this.type != null && !this.type.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 570 */ 571 public ClaimEventComponent setType(CodeableConcept value) { 572 this.type = value; 573 return this; 574 } 575 576 /** 577 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 578 */ 579 public DataType getWhen() { 580 return this.when; 581 } 582 583 /** 584 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 585 */ 586 public DateTimeType getWhenDateTimeType() throws FHIRException { 587 if (this.when == null) 588 this.when = new DateTimeType(); 589 if (!(this.when instanceof DateTimeType)) 590 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 591 return (DateTimeType) this.when; 592 } 593 594 public boolean hasWhenDateTimeType() { 595 return this != null && this.when instanceof DateTimeType; 596 } 597 598 /** 599 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 600 */ 601 public Period getWhenPeriod() throws FHIRException { 602 if (this.when == null) 603 this.when = new Period(); 604 if (!(this.when instanceof Period)) 605 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 606 return (Period) this.when; 607 } 608 609 public boolean hasWhenPeriod() { 610 return this != null && this.when instanceof Period; 611 } 612 613 public boolean hasWhen() { 614 return this.when != null && !this.when.isEmpty(); 615 } 616 617 /** 618 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 619 */ 620 public ClaimEventComponent setWhen(DataType value) { 621 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 622 throw new FHIRException("Not the right type for Claim.event.when[x]: "+value.fhirType()); 623 this.when = value; 624 return this; 625 } 626 627 protected void listChildren(List<Property> children) { 628 super.listChildren(children); 629 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 630 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 631 } 632 633 @Override 634 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 635 switch (_hash) { 636 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 637 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 638 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 639 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 640 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 641 default: return super.getNamedProperty(_hash, _name, _checkValid); 642 } 643 644 } 645 646 @Override 647 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 648 switch (hash) { 649 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 650 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 651 default: return super.getProperty(hash, name, checkValid); 652 } 653 654 } 655 656 @Override 657 public Base setProperty(int hash, String name, Base value) throws FHIRException { 658 switch (hash) { 659 case 3575610: // type 660 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 661 return value; 662 case 3648314: // when 663 this.when = TypeConvertor.castToType(value); // DataType 664 return value; 665 default: return super.setProperty(hash, name, value); 666 } 667 668 } 669 670 @Override 671 public Base setProperty(String name, Base value) throws FHIRException { 672 if (name.equals("type")) { 673 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 674 } else if (name.equals("when[x]")) { 675 this.when = TypeConvertor.castToType(value); // DataType 676 } else 677 return super.setProperty(name, value); 678 return value; 679 } 680 681 @Override 682 public Base makeProperty(int hash, String name) throws FHIRException { 683 switch (hash) { 684 case 3575610: return getType(); 685 case 1312831238: return getWhen(); 686 case 3648314: return getWhen(); 687 default: return super.makeProperty(hash, name); 688 } 689 690 } 691 692 @Override 693 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 694 switch (hash) { 695 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 696 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 697 default: return super.getTypesForProperty(hash, name); 698 } 699 700 } 701 702 @Override 703 public Base addChild(String name) throws FHIRException { 704 if (name.equals("type")) { 705 this.type = new CodeableConcept(); 706 return this.type; 707 } 708 else if (name.equals("whenDateTime")) { 709 this.when = new DateTimeType(); 710 return this.when; 711 } 712 else if (name.equals("whenPeriod")) { 713 this.when = new Period(); 714 return this.when; 715 } 716 else 717 return super.addChild(name); 718 } 719 720 public ClaimEventComponent copy() { 721 ClaimEventComponent dst = new ClaimEventComponent(); 722 copyValues(dst); 723 return dst; 724 } 725 726 public void copyValues(ClaimEventComponent dst) { 727 super.copyValues(dst); 728 dst.type = type == null ? null : type.copy(); 729 dst.when = when == null ? null : when.copy(); 730 } 731 732 @Override 733 public boolean equalsDeep(Base other_) { 734 if (!super.equalsDeep(other_)) 735 return false; 736 if (!(other_ instanceof ClaimEventComponent)) 737 return false; 738 ClaimEventComponent o = (ClaimEventComponent) other_; 739 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 740 } 741 742 @Override 743 public boolean equalsShallow(Base other_) { 744 if (!super.equalsShallow(other_)) 745 return false; 746 if (!(other_ instanceof ClaimEventComponent)) 747 return false; 748 ClaimEventComponent o = (ClaimEventComponent) other_; 749 return true; 750 } 751 752 public boolean isEmpty() { 753 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 754 } 755 756 public String fhirType() { 757 return "Claim.event"; 758 759 } 760 761 } 762 763 @Block() 764 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 765 /** 766 * A number to uniquely identify care team entries. 767 */ 768 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 769 @Description(shortDefinition="Order of care team", formalDefinition="A number to uniquely identify care team entries." ) 770 protected PositiveIntType sequence; 771 772 /** 773 * Member of the team who provided the product or service. 774 */ 775 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 776 @Description(shortDefinition="Practitioner or organization", formalDefinition="Member of the team who provided the product or service." ) 777 protected Reference provider; 778 779 /** 780 * The party who is billing and/or responsible for the claimed products or services. 781 */ 782 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 783 @Description(shortDefinition="Indicator of the lead practitioner", formalDefinition="The party who is billing and/or responsible for the claimed products or services." ) 784 protected BooleanType responsible; 785 786 /** 787 * The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team. 788 */ 789 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 790 @Description(shortDefinition="Function within the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team." ) 791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 792 protected CodeableConcept role; 793 794 /** 795 * The specialization of the practitioner or provider which is applicable for this service. 796 */ 797 @Child(name = "specialty", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 798 @Description(shortDefinition="Practitioner or provider specialization", formalDefinition="The specialization of the practitioner or provider which is applicable for this service." ) 799 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 800 protected CodeableConcept specialty; 801 802 private static final long serialVersionUID = 1238813503L; 803 804 /** 805 * Constructor 806 */ 807 public CareTeamComponent() { 808 super(); 809 } 810 811 /** 812 * Constructor 813 */ 814 public CareTeamComponent(int sequence, Reference provider) { 815 super(); 816 this.setSequence(sequence); 817 this.setProvider(provider); 818 } 819 820 /** 821 * @return {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 822 */ 823 public PositiveIntType getSequenceElement() { 824 if (this.sequence == null) 825 if (Configuration.errorOnAutoCreate()) 826 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 827 else if (Configuration.doAutoCreate()) 828 this.sequence = new PositiveIntType(); // bb 829 return this.sequence; 830 } 831 832 public boolean hasSequenceElement() { 833 return this.sequence != null && !this.sequence.isEmpty(); 834 } 835 836 public boolean hasSequence() { 837 return this.sequence != null && !this.sequence.isEmpty(); 838 } 839 840 /** 841 * @param value {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 842 */ 843 public CareTeamComponent setSequenceElement(PositiveIntType value) { 844 this.sequence = value; 845 return this; 846 } 847 848 /** 849 * @return A number to uniquely identify care team entries. 850 */ 851 public int getSequence() { 852 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 853 } 854 855 /** 856 * @param value A number to uniquely identify care team entries. 857 */ 858 public CareTeamComponent setSequence(int value) { 859 if (this.sequence == null) 860 this.sequence = new PositiveIntType(); 861 this.sequence.setValue(value); 862 return this; 863 } 864 865 /** 866 * @return {@link #provider} (Member of the team who provided the product or service.) 867 */ 868 public Reference getProvider() { 869 if (this.provider == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 872 else if (Configuration.doAutoCreate()) 873 this.provider = new Reference(); // cc 874 return this.provider; 875 } 876 877 public boolean hasProvider() { 878 return this.provider != null && !this.provider.isEmpty(); 879 } 880 881 /** 882 * @param value {@link #provider} (Member of the team who provided the product or service.) 883 */ 884 public CareTeamComponent setProvider(Reference value) { 885 this.provider = value; 886 return this; 887 } 888 889 /** 890 * @return {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 891 */ 892 public BooleanType getResponsibleElement() { 893 if (this.responsible == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 896 else if (Configuration.doAutoCreate()) 897 this.responsible = new BooleanType(); // bb 898 return this.responsible; 899 } 900 901 public boolean hasResponsibleElement() { 902 return this.responsible != null && !this.responsible.isEmpty(); 903 } 904 905 public boolean hasResponsible() { 906 return this.responsible != null && !this.responsible.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 911 */ 912 public CareTeamComponent setResponsibleElement(BooleanType value) { 913 this.responsible = value; 914 return this; 915 } 916 917 /** 918 * @return The party who is billing and/or responsible for the claimed products or services. 919 */ 920 public boolean getResponsible() { 921 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 922 } 923 924 /** 925 * @param value The party who is billing and/or responsible for the claimed products or services. 926 */ 927 public CareTeamComponent setResponsible(boolean value) { 928 if (this.responsible == null) 929 this.responsible = new BooleanType(); 930 this.responsible.setValue(value); 931 return this; 932 } 933 934 /** 935 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 936 */ 937 public CodeableConcept getRole() { 938 if (this.role == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create CareTeamComponent.role"); 941 else if (Configuration.doAutoCreate()) 942 this.role = new CodeableConcept(); // cc 943 return this.role; 944 } 945 946 public boolean hasRole() { 947 return this.role != null && !this.role.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 952 */ 953 public CareTeamComponent setRole(CodeableConcept value) { 954 this.role = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 960 */ 961 public CodeableConcept getSpecialty() { 962 if (this.specialty == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create CareTeamComponent.specialty"); 965 else if (Configuration.doAutoCreate()) 966 this.specialty = new CodeableConcept(); // cc 967 return this.specialty; 968 } 969 970 public boolean hasSpecialty() { 971 return this.specialty != null && !this.specialty.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 976 */ 977 public CareTeamComponent setSpecialty(CodeableConcept value) { 978 this.specialty = value; 979 return this; 980 } 981 982 protected void listChildren(List<Property> children) { 983 super.listChildren(children); 984 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 985 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider)); 986 children.add(new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 987 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role)); 988 children.add(new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty)); 989 } 990 991 @Override 992 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 993 switch (_hash) { 994 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence); 995 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider); 996 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 997 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role); 998 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty); 999 default: return super.getNamedProperty(_hash, _name, _checkValid); 1000 } 1001 1002 } 1003 1004 @Override 1005 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1006 switch (hash) { 1007 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1008 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1009 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1010 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1011 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : new Base[] {this.specialty}; // CodeableConcept 1012 default: return super.getProperty(hash, name, checkValid); 1013 } 1014 1015 } 1016 1017 @Override 1018 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1019 switch (hash) { 1020 case 1349547969: // sequence 1021 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1022 return value; 1023 case -987494927: // provider 1024 this.provider = TypeConvertor.castToReference(value); // Reference 1025 return value; 1026 case 1847674614: // responsible 1027 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1028 return value; 1029 case 3506294: // role 1030 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1031 return value; 1032 case -1694759682: // specialty 1033 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1034 return value; 1035 default: return super.setProperty(hash, name, value); 1036 } 1037 1038 } 1039 1040 @Override 1041 public Base setProperty(String name, Base value) throws FHIRException { 1042 if (name.equals("sequence")) { 1043 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1044 } else if (name.equals("provider")) { 1045 this.provider = TypeConvertor.castToReference(value); // Reference 1046 } else if (name.equals("responsible")) { 1047 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1048 } else if (name.equals("role")) { 1049 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1050 } else if (name.equals("specialty")) { 1051 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1052 } else 1053 return super.setProperty(name, value); 1054 return value; 1055 } 1056 1057 @Override 1058 public Base makeProperty(int hash, String name) throws FHIRException { 1059 switch (hash) { 1060 case 1349547969: return getSequenceElement(); 1061 case -987494927: return getProvider(); 1062 case 1847674614: return getResponsibleElement(); 1063 case 3506294: return getRole(); 1064 case -1694759682: return getSpecialty(); 1065 default: return super.makeProperty(hash, name); 1066 } 1067 1068 } 1069 1070 @Override 1071 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1072 switch (hash) { 1073 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1074 case -987494927: /*provider*/ return new String[] {"Reference"}; 1075 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1076 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1077 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1078 default: return super.getTypesForProperty(hash, name); 1079 } 1080 1081 } 1082 1083 @Override 1084 public Base addChild(String name) throws FHIRException { 1085 if (name.equals("sequence")) { 1086 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeam.sequence"); 1087 } 1088 else if (name.equals("provider")) { 1089 this.provider = new Reference(); 1090 return this.provider; 1091 } 1092 else if (name.equals("responsible")) { 1093 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeam.responsible"); 1094 } 1095 else if (name.equals("role")) { 1096 this.role = new CodeableConcept(); 1097 return this.role; 1098 } 1099 else if (name.equals("specialty")) { 1100 this.specialty = new CodeableConcept(); 1101 return this.specialty; 1102 } 1103 else 1104 return super.addChild(name); 1105 } 1106 1107 public CareTeamComponent copy() { 1108 CareTeamComponent dst = new CareTeamComponent(); 1109 copyValues(dst); 1110 return dst; 1111 } 1112 1113 public void copyValues(CareTeamComponent dst) { 1114 super.copyValues(dst); 1115 dst.sequence = sequence == null ? null : sequence.copy(); 1116 dst.provider = provider == null ? null : provider.copy(); 1117 dst.responsible = responsible == null ? null : responsible.copy(); 1118 dst.role = role == null ? null : role.copy(); 1119 dst.specialty = specialty == null ? null : specialty.copy(); 1120 } 1121 1122 @Override 1123 public boolean equalsDeep(Base other_) { 1124 if (!super.equalsDeep(other_)) 1125 return false; 1126 if (!(other_ instanceof CareTeamComponent)) 1127 return false; 1128 CareTeamComponent o = (CareTeamComponent) other_; 1129 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1130 && compareDeep(role, o.role, true) && compareDeep(specialty, o.specialty, true); 1131 } 1132 1133 @Override 1134 public boolean equalsShallow(Base other_) { 1135 if (!super.equalsShallow(other_)) 1136 return false; 1137 if (!(other_ instanceof CareTeamComponent)) 1138 return false; 1139 CareTeamComponent o = (CareTeamComponent) other_; 1140 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1141 ; 1142 } 1143 1144 public boolean isEmpty() { 1145 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1146 , role, specialty); 1147 } 1148 1149 public String fhirType() { 1150 return "Claim.careTeam"; 1151 1152 } 1153 1154 } 1155 1156 @Block() 1157 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1158 /** 1159 * A number to uniquely identify supporting information entries. 1160 */ 1161 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1162 @Description(shortDefinition="Information instance identifier", formalDefinition="A number to uniquely identify supporting information entries." ) 1163 protected PositiveIntType sequence; 1164 1165 /** 1166 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 1167 */ 1168 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1169 @Description(shortDefinition="Classification of the supplied information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 1170 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 1171 protected CodeableConcept category; 1172 1173 /** 1174 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought. 1175 */ 1176 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1177 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought." ) 1178 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 1179 protected CodeableConcept code; 1180 1181 /** 1182 * The date when or period to which this information refers. 1183 */ 1184 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1185 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 1186 protected DataType timing; 1187 1188 /** 1189 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 1190 */ 1191 @Child(name = "value", type = {BooleanType.class, StringType.class, Quantity.class, Attachment.class, Reference.class, Identifier.class}, order=5, min=0, max=1, modifier=false, summary=false) 1192 @Description(shortDefinition="Data to be provided", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 1193 protected DataType value; 1194 1195 /** 1196 * Provides the reason in the situation where a reason code is required in addition to the content. 1197 */ 1198 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1199 @Description(shortDefinition="Explanation for the information", formalDefinition="Provides the reason in the situation where a reason code is required in addition to the content." ) 1200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1201 protected CodeableConcept reason; 1202 1203 private static final long serialVersionUID = 2028146236L; 1204 1205 /** 1206 * Constructor 1207 */ 1208 public SupportingInformationComponent() { 1209 super(); 1210 } 1211 1212 /** 1213 * Constructor 1214 */ 1215 public SupportingInformationComponent(int sequence, CodeableConcept category) { 1216 super(); 1217 this.setSequence(sequence); 1218 this.setCategory(category); 1219 } 1220 1221 /** 1222 * @return {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1223 */ 1224 public PositiveIntType getSequenceElement() { 1225 if (this.sequence == null) 1226 if (Configuration.errorOnAutoCreate()) 1227 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1228 else if (Configuration.doAutoCreate()) 1229 this.sequence = new PositiveIntType(); // bb 1230 return this.sequence; 1231 } 1232 1233 public boolean hasSequenceElement() { 1234 return this.sequence != null && !this.sequence.isEmpty(); 1235 } 1236 1237 public boolean hasSequence() { 1238 return this.sequence != null && !this.sequence.isEmpty(); 1239 } 1240 1241 /** 1242 * @param value {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1243 */ 1244 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1245 this.sequence = value; 1246 return this; 1247 } 1248 1249 /** 1250 * @return A number to uniquely identify supporting information entries. 1251 */ 1252 public int getSequence() { 1253 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1254 } 1255 1256 /** 1257 * @param value A number to uniquely identify supporting information entries. 1258 */ 1259 public SupportingInformationComponent setSequence(int value) { 1260 if (this.sequence == null) 1261 this.sequence = new PositiveIntType(); 1262 this.sequence.setValue(value); 1263 return this; 1264 } 1265 1266 /** 1267 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1268 */ 1269 public CodeableConcept getCategory() { 1270 if (this.category == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1273 else if (Configuration.doAutoCreate()) 1274 this.category = new CodeableConcept(); // cc 1275 return this.category; 1276 } 1277 1278 public boolean hasCategory() { 1279 return this.category != null && !this.category.isEmpty(); 1280 } 1281 1282 /** 1283 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1284 */ 1285 public SupportingInformationComponent setCategory(CodeableConcept value) { 1286 this.category = value; 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1292 */ 1293 public CodeableConcept getCode() { 1294 if (this.code == null) 1295 if (Configuration.errorOnAutoCreate()) 1296 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1297 else if (Configuration.doAutoCreate()) 1298 this.code = new CodeableConcept(); // cc 1299 return this.code; 1300 } 1301 1302 public boolean hasCode() { 1303 return this.code != null && !this.code.isEmpty(); 1304 } 1305 1306 /** 1307 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1308 */ 1309 public SupportingInformationComponent setCode(CodeableConcept value) { 1310 this.code = value; 1311 return this; 1312 } 1313 1314 /** 1315 * @return {@link #timing} (The date when or period to which this information refers.) 1316 */ 1317 public DataType getTiming() { 1318 return this.timing; 1319 } 1320 1321 /** 1322 * @return {@link #timing} (The date when or period to which this information refers.) 1323 */ 1324 public DateType getTimingDateType() throws FHIRException { 1325 if (this.timing == null) 1326 this.timing = new DateType(); 1327 if (!(this.timing instanceof DateType)) 1328 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 1329 return (DateType) this.timing; 1330 } 1331 1332 public boolean hasTimingDateType() { 1333 return this != null && this.timing instanceof DateType; 1334 } 1335 1336 /** 1337 * @return {@link #timing} (The date when or period to which this information refers.) 1338 */ 1339 public Period getTimingPeriod() throws FHIRException { 1340 if (this.timing == null) 1341 this.timing = new Period(); 1342 if (!(this.timing instanceof Period)) 1343 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 1344 return (Period) this.timing; 1345 } 1346 1347 public boolean hasTimingPeriod() { 1348 return this != null && this.timing instanceof Period; 1349 } 1350 1351 public boolean hasTiming() { 1352 return this.timing != null && !this.timing.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #timing} (The date when or period to which this information refers.) 1357 */ 1358 public SupportingInformationComponent setTiming(DataType value) { 1359 if (value != null && !(value instanceof DateType || value instanceof Period)) 1360 throw new FHIRException("Not the right type for Claim.supportingInfo.timing[x]: "+value.fhirType()); 1361 this.timing = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1367 */ 1368 public DataType getValue() { 1369 return this.value; 1370 } 1371 1372 /** 1373 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1374 */ 1375 public BooleanType getValueBooleanType() throws FHIRException { 1376 if (this.value == null) 1377 this.value = new BooleanType(); 1378 if (!(this.value instanceof BooleanType)) 1379 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1380 return (BooleanType) this.value; 1381 } 1382 1383 public boolean hasValueBooleanType() { 1384 return this != null && this.value instanceof BooleanType; 1385 } 1386 1387 /** 1388 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1389 */ 1390 public StringType getValueStringType() throws FHIRException { 1391 if (this.value == null) 1392 this.value = new StringType(); 1393 if (!(this.value instanceof StringType)) 1394 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1395 return (StringType) this.value; 1396 } 1397 1398 public boolean hasValueStringType() { 1399 return this != null && this.value instanceof StringType; 1400 } 1401 1402 /** 1403 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1404 */ 1405 public Quantity getValueQuantity() throws FHIRException { 1406 if (this.value == null) 1407 this.value = new Quantity(); 1408 if (!(this.value instanceof Quantity)) 1409 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1410 return (Quantity) this.value; 1411 } 1412 1413 public boolean hasValueQuantity() { 1414 return this != null && this.value instanceof Quantity; 1415 } 1416 1417 /** 1418 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1419 */ 1420 public Attachment getValueAttachment() throws FHIRException { 1421 if (this.value == null) 1422 this.value = new Attachment(); 1423 if (!(this.value instanceof Attachment)) 1424 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1425 return (Attachment) this.value; 1426 } 1427 1428 public boolean hasValueAttachment() { 1429 return this != null && this.value instanceof Attachment; 1430 } 1431 1432 /** 1433 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1434 */ 1435 public Reference getValueReference() throws FHIRException { 1436 if (this.value == null) 1437 this.value = new Reference(); 1438 if (!(this.value instanceof Reference)) 1439 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1440 return (Reference) this.value; 1441 } 1442 1443 public boolean hasValueReference() { 1444 return this != null && this.value instanceof Reference; 1445 } 1446 1447 /** 1448 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1449 */ 1450 public Identifier getValueIdentifier() throws FHIRException { 1451 if (this.value == null) 1452 this.value = new Identifier(); 1453 if (!(this.value instanceof Identifier)) 1454 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 1455 return (Identifier) this.value; 1456 } 1457 1458 public boolean hasValueIdentifier() { 1459 return this != null && this.value instanceof Identifier; 1460 } 1461 1462 public boolean hasValue() { 1463 return this.value != null && !this.value.isEmpty(); 1464 } 1465 1466 /** 1467 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1468 */ 1469 public SupportingInformationComponent setValue(DataType value) { 1470 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference || value instanceof Identifier)) 1471 throw new FHIRException("Not the right type for Claim.supportingInfo.value[x]: "+value.fhirType()); 1472 this.value = value; 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1478 */ 1479 public CodeableConcept getReason() { 1480 if (this.reason == null) 1481 if (Configuration.errorOnAutoCreate()) 1482 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1483 else if (Configuration.doAutoCreate()) 1484 this.reason = new CodeableConcept(); // cc 1485 return this.reason; 1486 } 1487 1488 public boolean hasReason() { 1489 return this.reason != null && !this.reason.isEmpty(); 1490 } 1491 1492 /** 1493 * @param value {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1494 */ 1495 public SupportingInformationComponent setReason(CodeableConcept value) { 1496 this.reason = value; 1497 return this; 1498 } 1499 1500 protected void listChildren(List<Property> children) { 1501 super.listChildren(children); 1502 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1503 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1504 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code)); 1505 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1506 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1507 children.add(new Property("reason", "CodeableConcept", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason)); 1508 } 1509 1510 @Override 1511 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1512 switch (_hash) { 1513 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence); 1514 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1515 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code); 1516 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1517 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1518 case 807935768: /*timingDate*/ return new Property("timing[x]", "date", "The date when or period to which this information refers.", 0, 1, timing); 1519 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Period", "The date when or period to which this information refers.", 0, 1, timing); 1520 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1521 case 111972721: /*value*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1522 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1523 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1524 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1525 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1526 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1527 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1528 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason); 1529 default: return super.getNamedProperty(_hash, _name, _checkValid); 1530 } 1531 1532 } 1533 1534 @Override 1535 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1536 switch (hash) { 1537 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1538 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1539 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1540 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 1541 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1542 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1543 default: return super.getProperty(hash, name, checkValid); 1544 } 1545 1546 } 1547 1548 @Override 1549 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1550 switch (hash) { 1551 case 1349547969: // sequence 1552 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1553 return value; 1554 case 50511102: // category 1555 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1556 return value; 1557 case 3059181: // code 1558 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1559 return value; 1560 case -873664438: // timing 1561 this.timing = TypeConvertor.castToType(value); // DataType 1562 return value; 1563 case 111972721: // value 1564 this.value = TypeConvertor.castToType(value); // DataType 1565 return value; 1566 case -934964668: // reason 1567 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1568 return value; 1569 default: return super.setProperty(hash, name, value); 1570 } 1571 1572 } 1573 1574 @Override 1575 public Base setProperty(String name, Base value) throws FHIRException { 1576 if (name.equals("sequence")) { 1577 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1578 } else if (name.equals("category")) { 1579 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1580 } else if (name.equals("code")) { 1581 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1582 } else if (name.equals("timing[x]")) { 1583 this.timing = TypeConvertor.castToType(value); // DataType 1584 } else if (name.equals("value[x]")) { 1585 this.value = TypeConvertor.castToType(value); // DataType 1586 } else if (name.equals("reason")) { 1587 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1588 } else 1589 return super.setProperty(name, value); 1590 return value; 1591 } 1592 1593 @Override 1594 public Base makeProperty(int hash, String name) throws FHIRException { 1595 switch (hash) { 1596 case 1349547969: return getSequenceElement(); 1597 case 50511102: return getCategory(); 1598 case 3059181: return getCode(); 1599 case 164632566: return getTiming(); 1600 case -873664438: return getTiming(); 1601 case -1410166417: return getValue(); 1602 case 111972721: return getValue(); 1603 case -934964668: return getReason(); 1604 default: return super.makeProperty(hash, name); 1605 } 1606 1607 } 1608 1609 @Override 1610 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1611 switch (hash) { 1612 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1613 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1614 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1615 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1616 case 111972721: /*value*/ return new String[] {"boolean", "string", "Quantity", "Attachment", "Reference", "Identifier"}; 1617 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1618 default: return super.getTypesForProperty(hash, name); 1619 } 1620 1621 } 1622 1623 @Override 1624 public Base addChild(String name) throws FHIRException { 1625 if (name.equals("sequence")) { 1626 throw new FHIRException("Cannot call addChild on a singleton property Claim.supportingInfo.sequence"); 1627 } 1628 else if (name.equals("category")) { 1629 this.category = new CodeableConcept(); 1630 return this.category; 1631 } 1632 else if (name.equals("code")) { 1633 this.code = new CodeableConcept(); 1634 return this.code; 1635 } 1636 else if (name.equals("timingDate")) { 1637 this.timing = new DateType(); 1638 return this.timing; 1639 } 1640 else if (name.equals("timingPeriod")) { 1641 this.timing = new Period(); 1642 return this.timing; 1643 } 1644 else if (name.equals("valueBoolean")) { 1645 this.value = new BooleanType(); 1646 return this.value; 1647 } 1648 else if (name.equals("valueString")) { 1649 this.value = new StringType(); 1650 return this.value; 1651 } 1652 else if (name.equals("valueQuantity")) { 1653 this.value = new Quantity(); 1654 return this.value; 1655 } 1656 else if (name.equals("valueAttachment")) { 1657 this.value = new Attachment(); 1658 return this.value; 1659 } 1660 else if (name.equals("valueReference")) { 1661 this.value = new Reference(); 1662 return this.value; 1663 } 1664 else if (name.equals("valueIdentifier")) { 1665 this.value = new Identifier(); 1666 return this.value; 1667 } 1668 else if (name.equals("reason")) { 1669 this.reason = new CodeableConcept(); 1670 return this.reason; 1671 } 1672 else 1673 return super.addChild(name); 1674 } 1675 1676 public SupportingInformationComponent copy() { 1677 SupportingInformationComponent dst = new SupportingInformationComponent(); 1678 copyValues(dst); 1679 return dst; 1680 } 1681 1682 public void copyValues(SupportingInformationComponent dst) { 1683 super.copyValues(dst); 1684 dst.sequence = sequence == null ? null : sequence.copy(); 1685 dst.category = category == null ? null : category.copy(); 1686 dst.code = code == null ? null : code.copy(); 1687 dst.timing = timing == null ? null : timing.copy(); 1688 dst.value = value == null ? null : value.copy(); 1689 dst.reason = reason == null ? null : reason.copy(); 1690 } 1691 1692 @Override 1693 public boolean equalsDeep(Base other_) { 1694 if (!super.equalsDeep(other_)) 1695 return false; 1696 if (!(other_ instanceof SupportingInformationComponent)) 1697 return false; 1698 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1699 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1700 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1701 ; 1702 } 1703 1704 @Override 1705 public boolean equalsShallow(Base other_) { 1706 if (!super.equalsShallow(other_)) 1707 return false; 1708 if (!(other_ instanceof SupportingInformationComponent)) 1709 return false; 1710 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1711 return compareValues(sequence, o.sequence, true); 1712 } 1713 1714 public boolean isEmpty() { 1715 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1716 , timing, value, reason); 1717 } 1718 1719 public String fhirType() { 1720 return "Claim.supportingInfo"; 1721 1722 } 1723 1724 } 1725 1726 @Block() 1727 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1728 /** 1729 * A number to uniquely identify diagnosis entries. 1730 */ 1731 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1732 @Description(shortDefinition="Diagnosis instance identifier", formalDefinition="A number to uniquely identify diagnosis entries." ) 1733 protected PositiveIntType sequence; 1734 1735 /** 1736 * The nature of illness or problem in a coded form or as a reference to an external defined Condition. 1737 */ 1738 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1739 @Description(shortDefinition="Nature of illness or problem", formalDefinition="The nature of illness or problem in a coded form or as a reference to an external defined Condition." ) 1740 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1741 protected DataType diagnosis; 1742 1743 /** 1744 * When the condition was observed or the relative ranking. 1745 */ 1746 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1747 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="When the condition was observed or the relative ranking." ) 1748 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1749 protected List<CodeableConcept> type; 1750 1751 /** 1752 * Indication of whether the diagnosis was present on admission to a facility. 1753 */ 1754 @Child(name = "onAdmission", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1755 @Description(shortDefinition="Present on admission", formalDefinition="Indication of whether the diagnosis was present on admission to a facility." ) 1756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 1757 protected CodeableConcept onAdmission; 1758 1759 private static final long serialVersionUID = -320261526L; 1760 1761 /** 1762 * Constructor 1763 */ 1764 public DiagnosisComponent() { 1765 super(); 1766 } 1767 1768 /** 1769 * Constructor 1770 */ 1771 public DiagnosisComponent(int sequence, DataType diagnosis) { 1772 super(); 1773 this.setSequence(sequence); 1774 this.setDiagnosis(diagnosis); 1775 } 1776 1777 /** 1778 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1779 */ 1780 public PositiveIntType getSequenceElement() { 1781 if (this.sequence == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1784 else if (Configuration.doAutoCreate()) 1785 this.sequence = new PositiveIntType(); // bb 1786 return this.sequence; 1787 } 1788 1789 public boolean hasSequenceElement() { 1790 return this.sequence != null && !this.sequence.isEmpty(); 1791 } 1792 1793 public boolean hasSequence() { 1794 return this.sequence != null && !this.sequence.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1799 */ 1800 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1801 this.sequence = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return A number to uniquely identify diagnosis entries. 1807 */ 1808 public int getSequence() { 1809 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1810 } 1811 1812 /** 1813 * @param value A number to uniquely identify diagnosis entries. 1814 */ 1815 public DiagnosisComponent setSequence(int value) { 1816 if (this.sequence == null) 1817 this.sequence = new PositiveIntType(); 1818 this.sequence.setValue(value); 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1824 */ 1825 public DataType getDiagnosis() { 1826 return this.diagnosis; 1827 } 1828 1829 /** 1830 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1831 */ 1832 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1833 if (this.diagnosis == null) 1834 this.diagnosis = new CodeableConcept(); 1835 if (!(this.diagnosis instanceof CodeableConcept)) 1836 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1837 return (CodeableConcept) this.diagnosis; 1838 } 1839 1840 public boolean hasDiagnosisCodeableConcept() { 1841 return this != null && this.diagnosis instanceof CodeableConcept; 1842 } 1843 1844 /** 1845 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1846 */ 1847 public Reference getDiagnosisReference() throws FHIRException { 1848 if (this.diagnosis == null) 1849 this.diagnosis = new Reference(); 1850 if (!(this.diagnosis instanceof Reference)) 1851 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1852 return (Reference) this.diagnosis; 1853 } 1854 1855 public boolean hasDiagnosisReference() { 1856 return this != null && this.diagnosis instanceof Reference; 1857 } 1858 1859 public boolean hasDiagnosis() { 1860 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1861 } 1862 1863 /** 1864 * @param value {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1865 */ 1866 public DiagnosisComponent setDiagnosis(DataType value) { 1867 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1868 throw new FHIRException("Not the right type for Claim.diagnosis.diagnosis[x]: "+value.fhirType()); 1869 this.diagnosis = value; 1870 return this; 1871 } 1872 1873 /** 1874 * @return {@link #type} (When the condition was observed or the relative ranking.) 1875 */ 1876 public List<CodeableConcept> getType() { 1877 if (this.type == null) 1878 this.type = new ArrayList<CodeableConcept>(); 1879 return this.type; 1880 } 1881 1882 /** 1883 * @return Returns a reference to <code>this</code> for easy method chaining 1884 */ 1885 public DiagnosisComponent setType(List<CodeableConcept> theType) { 1886 this.type = theType; 1887 return this; 1888 } 1889 1890 public boolean hasType() { 1891 if (this.type == null) 1892 return false; 1893 for (CodeableConcept item : this.type) 1894 if (!item.isEmpty()) 1895 return true; 1896 return false; 1897 } 1898 1899 public CodeableConcept addType() { //3 1900 CodeableConcept t = new CodeableConcept(); 1901 if (this.type == null) 1902 this.type = new ArrayList<CodeableConcept>(); 1903 this.type.add(t); 1904 return t; 1905 } 1906 1907 public DiagnosisComponent addType(CodeableConcept t) { //3 1908 if (t == null) 1909 return this; 1910 if (this.type == null) 1911 this.type = new ArrayList<CodeableConcept>(); 1912 this.type.add(t); 1913 return this; 1914 } 1915 1916 /** 1917 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1918 */ 1919 public CodeableConcept getTypeFirstRep() { 1920 if (getType().isEmpty()) { 1921 addType(); 1922 } 1923 return getType().get(0); 1924 } 1925 1926 /** 1927 * @return {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 1928 */ 1929 public CodeableConcept getOnAdmission() { 1930 if (this.onAdmission == null) 1931 if (Configuration.errorOnAutoCreate()) 1932 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 1933 else if (Configuration.doAutoCreate()) 1934 this.onAdmission = new CodeableConcept(); // cc 1935 return this.onAdmission; 1936 } 1937 1938 public boolean hasOnAdmission() { 1939 return this.onAdmission != null && !this.onAdmission.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 1944 */ 1945 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 1946 this.onAdmission = value; 1947 return this; 1948 } 1949 1950 protected void listChildren(List<Property> children) { 1951 super.listChildren(children); 1952 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 1953 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis)); 1954 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 1955 children.add(new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 1956 } 1957 1958 @Override 1959 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1960 switch (_hash) { 1961 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 1962 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1963 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1964 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1965 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1966 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 1967 case -3386134: /*onAdmission*/ return new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 1968 default: return super.getNamedProperty(_hash, _name, _checkValid); 1969 } 1970 1971 } 1972 1973 @Override 1974 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1975 switch (hash) { 1976 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1977 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // DataType 1978 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1979 case -3386134: /*onAdmission*/ return this.onAdmission == null ? new Base[0] : new Base[] {this.onAdmission}; // CodeableConcept 1980 default: return super.getProperty(hash, name, checkValid); 1981 } 1982 1983 } 1984 1985 @Override 1986 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1987 switch (hash) { 1988 case 1349547969: // sequence 1989 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1990 return value; 1991 case 1196993265: // diagnosis 1992 this.diagnosis = TypeConvertor.castToType(value); // DataType 1993 return value; 1994 case 3575610: // type 1995 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1996 return value; 1997 case -3386134: // onAdmission 1998 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1999 return value; 2000 default: return super.setProperty(hash, name, value); 2001 } 2002 2003 } 2004 2005 @Override 2006 public Base setProperty(String name, Base value) throws FHIRException { 2007 if (name.equals("sequence")) { 2008 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2009 } else if (name.equals("diagnosis[x]")) { 2010 this.diagnosis = TypeConvertor.castToType(value); // DataType 2011 } else if (name.equals("type")) { 2012 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2013 } else if (name.equals("onAdmission")) { 2014 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2015 } else 2016 return super.setProperty(name, value); 2017 return value; 2018 } 2019 2020 @Override 2021 public Base makeProperty(int hash, String name) throws FHIRException { 2022 switch (hash) { 2023 case 1349547969: return getSequenceElement(); 2024 case -1487009809: return getDiagnosis(); 2025 case 1196993265: return getDiagnosis(); 2026 case 3575610: return addType(); 2027 case -3386134: return getOnAdmission(); 2028 default: return super.makeProperty(hash, name); 2029 } 2030 2031 } 2032 2033 @Override 2034 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2035 switch (hash) { 2036 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2037 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 2038 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2039 case -3386134: /*onAdmission*/ return new String[] {"CodeableConcept"}; 2040 default: return super.getTypesForProperty(hash, name); 2041 } 2042 2043 } 2044 2045 @Override 2046 public Base addChild(String name) throws FHIRException { 2047 if (name.equals("sequence")) { 2048 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosis.sequence"); 2049 } 2050 else if (name.equals("diagnosisCodeableConcept")) { 2051 this.diagnosis = new CodeableConcept(); 2052 return this.diagnosis; 2053 } 2054 else if (name.equals("diagnosisReference")) { 2055 this.diagnosis = new Reference(); 2056 return this.diagnosis; 2057 } 2058 else if (name.equals("type")) { 2059 return addType(); 2060 } 2061 else if (name.equals("onAdmission")) { 2062 this.onAdmission = new CodeableConcept(); 2063 return this.onAdmission; 2064 } 2065 else 2066 return super.addChild(name); 2067 } 2068 2069 public DiagnosisComponent copy() { 2070 DiagnosisComponent dst = new DiagnosisComponent(); 2071 copyValues(dst); 2072 return dst; 2073 } 2074 2075 public void copyValues(DiagnosisComponent dst) { 2076 super.copyValues(dst); 2077 dst.sequence = sequence == null ? null : sequence.copy(); 2078 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2079 if (type != null) { 2080 dst.type = new ArrayList<CodeableConcept>(); 2081 for (CodeableConcept i : type) 2082 dst.type.add(i.copy()); 2083 }; 2084 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2085 } 2086 2087 @Override 2088 public boolean equalsDeep(Base other_) { 2089 if (!super.equalsDeep(other_)) 2090 return false; 2091 if (!(other_ instanceof DiagnosisComponent)) 2092 return false; 2093 DiagnosisComponent o = (DiagnosisComponent) other_; 2094 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2095 && compareDeep(onAdmission, o.onAdmission, true); 2096 } 2097 2098 @Override 2099 public boolean equalsShallow(Base other_) { 2100 if (!super.equalsShallow(other_)) 2101 return false; 2102 if (!(other_ instanceof DiagnosisComponent)) 2103 return false; 2104 DiagnosisComponent o = (DiagnosisComponent) other_; 2105 return compareValues(sequence, o.sequence, true); 2106 } 2107 2108 public boolean isEmpty() { 2109 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2110 , onAdmission); 2111 } 2112 2113 public String fhirType() { 2114 return "Claim.diagnosis"; 2115 2116 } 2117 2118 } 2119 2120 @Block() 2121 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2122 /** 2123 * A number to uniquely identify procedure entries. 2124 */ 2125 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2126 @Description(shortDefinition="Procedure instance identifier", formalDefinition="A number to uniquely identify procedure entries." ) 2127 protected PositiveIntType sequence; 2128 2129 /** 2130 * When the condition was observed or the relative ranking. 2131 */ 2132 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2133 @Description(shortDefinition="Category of Procedure", formalDefinition="When the condition was observed or the relative ranking." ) 2134 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-procedure-type") 2135 protected List<CodeableConcept> type; 2136 2137 /** 2138 * Date and optionally time the procedure was performed. 2139 */ 2140 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2141 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed." ) 2142 protected DateTimeType date; 2143 2144 /** 2145 * The code or reference to a Procedure resource which identifies the clinical intervention performed. 2146 */ 2147 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=4, min=1, max=1, modifier=false, summary=false) 2148 @Description(shortDefinition="Specific clinical procedure", formalDefinition="The code or reference to a Procedure resource which identifies the clinical intervention performed." ) 2149 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2150 protected DataType procedure; 2151 2152 /** 2153 * Unique Device Identifiers associated with this line item. 2154 */ 2155 @Child(name = "udi", type = {Device.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2156 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 2157 protected List<Reference> udi; 2158 2159 private static final long serialVersionUID = 1165684715L; 2160 2161 /** 2162 * Constructor 2163 */ 2164 public ProcedureComponent() { 2165 super(); 2166 } 2167 2168 /** 2169 * Constructor 2170 */ 2171 public ProcedureComponent(int sequence, DataType procedure) { 2172 super(); 2173 this.setSequence(sequence); 2174 this.setProcedure(procedure); 2175 } 2176 2177 /** 2178 * @return {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2179 */ 2180 public PositiveIntType getSequenceElement() { 2181 if (this.sequence == null) 2182 if (Configuration.errorOnAutoCreate()) 2183 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2184 else if (Configuration.doAutoCreate()) 2185 this.sequence = new PositiveIntType(); // bb 2186 return this.sequence; 2187 } 2188 2189 public boolean hasSequenceElement() { 2190 return this.sequence != null && !this.sequence.isEmpty(); 2191 } 2192 2193 public boolean hasSequence() { 2194 return this.sequence != null && !this.sequence.isEmpty(); 2195 } 2196 2197 /** 2198 * @param value {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2199 */ 2200 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2201 this.sequence = value; 2202 return this; 2203 } 2204 2205 /** 2206 * @return A number to uniquely identify procedure entries. 2207 */ 2208 public int getSequence() { 2209 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2210 } 2211 2212 /** 2213 * @param value A number to uniquely identify procedure entries. 2214 */ 2215 public ProcedureComponent setSequence(int value) { 2216 if (this.sequence == null) 2217 this.sequence = new PositiveIntType(); 2218 this.sequence.setValue(value); 2219 return this; 2220 } 2221 2222 /** 2223 * @return {@link #type} (When the condition was observed or the relative ranking.) 2224 */ 2225 public List<CodeableConcept> getType() { 2226 if (this.type == null) 2227 this.type = new ArrayList<CodeableConcept>(); 2228 return this.type; 2229 } 2230 2231 /** 2232 * @return Returns a reference to <code>this</code> for easy method chaining 2233 */ 2234 public ProcedureComponent setType(List<CodeableConcept> theType) { 2235 this.type = theType; 2236 return this; 2237 } 2238 2239 public boolean hasType() { 2240 if (this.type == null) 2241 return false; 2242 for (CodeableConcept item : this.type) 2243 if (!item.isEmpty()) 2244 return true; 2245 return false; 2246 } 2247 2248 public CodeableConcept addType() { //3 2249 CodeableConcept t = new CodeableConcept(); 2250 if (this.type == null) 2251 this.type = new ArrayList<CodeableConcept>(); 2252 this.type.add(t); 2253 return t; 2254 } 2255 2256 public ProcedureComponent addType(CodeableConcept t) { //3 2257 if (t == null) 2258 return this; 2259 if (this.type == null) 2260 this.type = new ArrayList<CodeableConcept>(); 2261 this.type.add(t); 2262 return this; 2263 } 2264 2265 /** 2266 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2267 */ 2268 public CodeableConcept getTypeFirstRep() { 2269 if (getType().isEmpty()) { 2270 addType(); 2271 } 2272 return getType().get(0); 2273 } 2274 2275 /** 2276 * @return {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2277 */ 2278 public DateTimeType getDateElement() { 2279 if (this.date == null) 2280 if (Configuration.errorOnAutoCreate()) 2281 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2282 else if (Configuration.doAutoCreate()) 2283 this.date = new DateTimeType(); // bb 2284 return this.date; 2285 } 2286 2287 public boolean hasDateElement() { 2288 return this.date != null && !this.date.isEmpty(); 2289 } 2290 2291 public boolean hasDate() { 2292 return this.date != null && !this.date.isEmpty(); 2293 } 2294 2295 /** 2296 * @param value {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2297 */ 2298 public ProcedureComponent setDateElement(DateTimeType value) { 2299 this.date = value; 2300 return this; 2301 } 2302 2303 /** 2304 * @return Date and optionally time the procedure was performed. 2305 */ 2306 public Date getDate() { 2307 return this.date == null ? null : this.date.getValue(); 2308 } 2309 2310 /** 2311 * @param value Date and optionally time the procedure was performed. 2312 */ 2313 public ProcedureComponent setDate(Date value) { 2314 if (value == null) 2315 this.date = null; 2316 else { 2317 if (this.date == null) 2318 this.date = new DateTimeType(); 2319 this.date.setValue(value); 2320 } 2321 return this; 2322 } 2323 2324 /** 2325 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2326 */ 2327 public DataType getProcedure() { 2328 return this.procedure; 2329 } 2330 2331 /** 2332 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2333 */ 2334 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2335 if (this.procedure == null) 2336 this.procedure = new CodeableConcept(); 2337 if (!(this.procedure instanceof CodeableConcept)) 2338 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2339 return (CodeableConcept) this.procedure; 2340 } 2341 2342 public boolean hasProcedureCodeableConcept() { 2343 return this != null && this.procedure instanceof CodeableConcept; 2344 } 2345 2346 /** 2347 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2348 */ 2349 public Reference getProcedureReference() throws FHIRException { 2350 if (this.procedure == null) 2351 this.procedure = new Reference(); 2352 if (!(this.procedure instanceof Reference)) 2353 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2354 return (Reference) this.procedure; 2355 } 2356 2357 public boolean hasProcedureReference() { 2358 return this != null && this.procedure instanceof Reference; 2359 } 2360 2361 public boolean hasProcedure() { 2362 return this.procedure != null && !this.procedure.isEmpty(); 2363 } 2364 2365 /** 2366 * @param value {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2367 */ 2368 public ProcedureComponent setProcedure(DataType value) { 2369 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2370 throw new FHIRException("Not the right type for Claim.procedure.procedure[x]: "+value.fhirType()); 2371 this.procedure = value; 2372 return this; 2373 } 2374 2375 /** 2376 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 2377 */ 2378 public List<Reference> getUdi() { 2379 if (this.udi == null) 2380 this.udi = new ArrayList<Reference>(); 2381 return this.udi; 2382 } 2383 2384 /** 2385 * @return Returns a reference to <code>this</code> for easy method chaining 2386 */ 2387 public ProcedureComponent setUdi(List<Reference> theUdi) { 2388 this.udi = theUdi; 2389 return this; 2390 } 2391 2392 public boolean hasUdi() { 2393 if (this.udi == null) 2394 return false; 2395 for (Reference item : this.udi) 2396 if (!item.isEmpty()) 2397 return true; 2398 return false; 2399 } 2400 2401 public Reference addUdi() { //3 2402 Reference t = new Reference(); 2403 if (this.udi == null) 2404 this.udi = new ArrayList<Reference>(); 2405 this.udi.add(t); 2406 return t; 2407 } 2408 2409 public ProcedureComponent addUdi(Reference t) { //3 2410 if (t == null) 2411 return this; 2412 if (this.udi == null) 2413 this.udi = new ArrayList<Reference>(); 2414 this.udi.add(t); 2415 return this; 2416 } 2417 2418 /** 2419 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 2420 */ 2421 public Reference getUdiFirstRep() { 2422 if (getUdi().isEmpty()) { 2423 addUdi(); 2424 } 2425 return getUdi().get(0); 2426 } 2427 2428 protected void listChildren(List<Property> children) { 2429 super.listChildren(children); 2430 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 2431 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2432 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 2433 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure)); 2434 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 2435 } 2436 2437 @Override 2438 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2439 switch (_hash) { 2440 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence); 2441 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2442 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date); 2443 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2444 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2445 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2446 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2447 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 2448 default: return super.getNamedProperty(_hash, _name, _checkValid); 2449 } 2450 2451 } 2452 2453 @Override 2454 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2455 switch (hash) { 2456 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2457 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2458 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2459 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // DataType 2460 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 2461 default: return super.getProperty(hash, name, checkValid); 2462 } 2463 2464 } 2465 2466 @Override 2467 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2468 switch (hash) { 2469 case 1349547969: // sequence 2470 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2471 return value; 2472 case 3575610: // type 2473 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2474 return value; 2475 case 3076014: // date 2476 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2477 return value; 2478 case -1095204141: // procedure 2479 this.procedure = TypeConvertor.castToType(value); // DataType 2480 return value; 2481 case 115642: // udi 2482 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 2483 return value; 2484 default: return super.setProperty(hash, name, value); 2485 } 2486 2487 } 2488 2489 @Override 2490 public Base setProperty(String name, Base value) throws FHIRException { 2491 if (name.equals("sequence")) { 2492 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2493 } else if (name.equals("type")) { 2494 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2495 } else if (name.equals("date")) { 2496 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2497 } else if (name.equals("procedure[x]")) { 2498 this.procedure = TypeConvertor.castToType(value); // DataType 2499 } else if (name.equals("udi")) { 2500 this.getUdi().add(TypeConvertor.castToReference(value)); 2501 } else 2502 return super.setProperty(name, value); 2503 return value; 2504 } 2505 2506 @Override 2507 public Base makeProperty(int hash, String name) throws FHIRException { 2508 switch (hash) { 2509 case 1349547969: return getSequenceElement(); 2510 case 3575610: return addType(); 2511 case 3076014: return getDateElement(); 2512 case 1640074445: return getProcedure(); 2513 case -1095204141: return getProcedure(); 2514 case 115642: return addUdi(); 2515 default: return super.makeProperty(hash, name); 2516 } 2517 2518 } 2519 2520 @Override 2521 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2522 switch (hash) { 2523 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2524 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2525 case 3076014: /*date*/ return new String[] {"dateTime"}; 2526 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2527 case 115642: /*udi*/ return new String[] {"Reference"}; 2528 default: return super.getTypesForProperty(hash, name); 2529 } 2530 2531 } 2532 2533 @Override 2534 public Base addChild(String name) throws FHIRException { 2535 if (name.equals("sequence")) { 2536 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedure.sequence"); 2537 } 2538 else if (name.equals("type")) { 2539 return addType(); 2540 } 2541 else if (name.equals("date")) { 2542 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedure.date"); 2543 } 2544 else if (name.equals("procedureCodeableConcept")) { 2545 this.procedure = new CodeableConcept(); 2546 return this.procedure; 2547 } 2548 else if (name.equals("procedureReference")) { 2549 this.procedure = new Reference(); 2550 return this.procedure; 2551 } 2552 else if (name.equals("udi")) { 2553 return addUdi(); 2554 } 2555 else 2556 return super.addChild(name); 2557 } 2558 2559 public ProcedureComponent copy() { 2560 ProcedureComponent dst = new ProcedureComponent(); 2561 copyValues(dst); 2562 return dst; 2563 } 2564 2565 public void copyValues(ProcedureComponent dst) { 2566 super.copyValues(dst); 2567 dst.sequence = sequence == null ? null : sequence.copy(); 2568 if (type != null) { 2569 dst.type = new ArrayList<CodeableConcept>(); 2570 for (CodeableConcept i : type) 2571 dst.type.add(i.copy()); 2572 }; 2573 dst.date = date == null ? null : date.copy(); 2574 dst.procedure = procedure == null ? null : procedure.copy(); 2575 if (udi != null) { 2576 dst.udi = new ArrayList<Reference>(); 2577 for (Reference i : udi) 2578 dst.udi.add(i.copy()); 2579 }; 2580 } 2581 2582 @Override 2583 public boolean equalsDeep(Base other_) { 2584 if (!super.equalsDeep(other_)) 2585 return false; 2586 if (!(other_ instanceof ProcedureComponent)) 2587 return false; 2588 ProcedureComponent o = (ProcedureComponent) other_; 2589 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(date, o.date, true) 2590 && compareDeep(procedure, o.procedure, true) && compareDeep(udi, o.udi, true); 2591 } 2592 2593 @Override 2594 public boolean equalsShallow(Base other_) { 2595 if (!super.equalsShallow(other_)) 2596 return false; 2597 if (!(other_ instanceof ProcedureComponent)) 2598 return false; 2599 ProcedureComponent o = (ProcedureComponent) other_; 2600 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2601 } 2602 2603 public boolean isEmpty() { 2604 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure 2605 , udi); 2606 } 2607 2608 public String fhirType() { 2609 return "Claim.procedure"; 2610 2611 } 2612 2613 } 2614 2615 @Block() 2616 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2617 /** 2618 * A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 2619 */ 2620 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2621 @Description(shortDefinition="Insurance instance identifier", formalDefinition="A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order." ) 2622 protected PositiveIntType sequence; 2623 2624 /** 2625 * A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2626 */ 2627 @Child(name = "focal", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=true) 2628 @Description(shortDefinition="Coverage to be used for adjudication", formalDefinition="A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true." ) 2629 protected BooleanType focal; 2630 2631 /** 2632 * The business identifier to be used when the claim is sent for adjudication against this insurance policy. 2633 */ 2634 @Child(name = "identifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 2635 @Description(shortDefinition="Pre-assigned Claim number", formalDefinition="The business identifier to be used when the claim is sent for adjudication against this insurance policy." ) 2636 protected Identifier identifier; 2637 2638 /** 2639 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 2640 */ 2641 @Child(name = "coverage", type = {Coverage.class}, order=4, min=1, max=1, modifier=false, summary=true) 2642 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 2643 protected Reference coverage; 2644 2645 /** 2646 * A business agreement number established between the provider and the insurer for special business processing purposes. 2647 */ 2648 @Child(name = "businessArrangement", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2649 @Description(shortDefinition="Additional provider contract number", formalDefinition="A business agreement number established between the provider and the insurer for special business processing purposes." ) 2650 protected StringType businessArrangement; 2651 2652 /** 2653 * Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization. 2654 */ 2655 @Child(name = "preAuthRef", type = {StringType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2656 @Description(shortDefinition="Prior authorization reference number", formalDefinition="Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization." ) 2657 protected List<StringType> preAuthRef; 2658 2659 /** 2660 * The result of the adjudication of the line items for the Coverage specified in this insurance. 2661 */ 2662 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=7, min=0, max=1, modifier=false, summary=false) 2663 @Description(shortDefinition="Adjudication results", formalDefinition="The result of the adjudication of the line items for the Coverage specified in this insurance." ) 2664 protected Reference claimResponse; 2665 2666 private static final long serialVersionUID = 481628099L; 2667 2668 /** 2669 * Constructor 2670 */ 2671 public InsuranceComponent() { 2672 super(); 2673 } 2674 2675 /** 2676 * Constructor 2677 */ 2678 public InsuranceComponent(int sequence, boolean focal, Reference coverage) { 2679 super(); 2680 this.setSequence(sequence); 2681 this.setFocal(focal); 2682 this.setCoverage(coverage); 2683 } 2684 2685 /** 2686 * @return {@link #sequence} (A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2687 */ 2688 public PositiveIntType getSequenceElement() { 2689 if (this.sequence == null) 2690 if (Configuration.errorOnAutoCreate()) 2691 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 2692 else if (Configuration.doAutoCreate()) 2693 this.sequence = new PositiveIntType(); // bb 2694 return this.sequence; 2695 } 2696 2697 public boolean hasSequenceElement() { 2698 return this.sequence != null && !this.sequence.isEmpty(); 2699 } 2700 2701 public boolean hasSequence() { 2702 return this.sequence != null && !this.sequence.isEmpty(); 2703 } 2704 2705 /** 2706 * @param value {@link #sequence} (A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2707 */ 2708 public InsuranceComponent setSequenceElement(PositiveIntType value) { 2709 this.sequence = value; 2710 return this; 2711 } 2712 2713 /** 2714 * @return A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 2715 */ 2716 public int getSequence() { 2717 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2718 } 2719 2720 /** 2721 * @param value A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 2722 */ 2723 public InsuranceComponent setSequence(int value) { 2724 if (this.sequence == null) 2725 this.sequence = new PositiveIntType(); 2726 this.sequence.setValue(value); 2727 return this; 2728 } 2729 2730 /** 2731 * @return {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2732 */ 2733 public BooleanType getFocalElement() { 2734 if (this.focal == null) 2735 if (Configuration.errorOnAutoCreate()) 2736 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 2737 else if (Configuration.doAutoCreate()) 2738 this.focal = new BooleanType(); // bb 2739 return this.focal; 2740 } 2741 2742 public boolean hasFocalElement() { 2743 return this.focal != null && !this.focal.isEmpty(); 2744 } 2745 2746 public boolean hasFocal() { 2747 return this.focal != null && !this.focal.isEmpty(); 2748 } 2749 2750 /** 2751 * @param value {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2752 */ 2753 public InsuranceComponent setFocalElement(BooleanType value) { 2754 this.focal = value; 2755 return this; 2756 } 2757 2758 /** 2759 * @return A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2760 */ 2761 public boolean getFocal() { 2762 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 2763 } 2764 2765 /** 2766 * @param value A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2767 */ 2768 public InsuranceComponent setFocal(boolean value) { 2769 if (this.focal == null) 2770 this.focal = new BooleanType(); 2771 this.focal.setValue(value); 2772 return this; 2773 } 2774 2775 /** 2776 * @return {@link #identifier} (The business identifier to be used when the claim is sent for adjudication against this insurance policy.) 2777 */ 2778 public Identifier getIdentifier() { 2779 if (this.identifier == null) 2780 if (Configuration.errorOnAutoCreate()) 2781 throw new Error("Attempt to auto-create InsuranceComponent.identifier"); 2782 else if (Configuration.doAutoCreate()) 2783 this.identifier = new Identifier(); // cc 2784 return this.identifier; 2785 } 2786 2787 public boolean hasIdentifier() { 2788 return this.identifier != null && !this.identifier.isEmpty(); 2789 } 2790 2791 /** 2792 * @param value {@link #identifier} (The business identifier to be used when the claim is sent for adjudication against this insurance policy.) 2793 */ 2794 public InsuranceComponent setIdentifier(Identifier value) { 2795 this.identifier = value; 2796 return this; 2797 } 2798 2799 /** 2800 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2801 */ 2802 public Reference getCoverage() { 2803 if (this.coverage == null) 2804 if (Configuration.errorOnAutoCreate()) 2805 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2806 else if (Configuration.doAutoCreate()) 2807 this.coverage = new Reference(); // cc 2808 return this.coverage; 2809 } 2810 2811 public boolean hasCoverage() { 2812 return this.coverage != null && !this.coverage.isEmpty(); 2813 } 2814 2815 /** 2816 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2817 */ 2818 public InsuranceComponent setCoverage(Reference value) { 2819 this.coverage = value; 2820 return this; 2821 } 2822 2823 /** 2824 * @return {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2825 */ 2826 public StringType getBusinessArrangementElement() { 2827 if (this.businessArrangement == null) 2828 if (Configuration.errorOnAutoCreate()) 2829 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 2830 else if (Configuration.doAutoCreate()) 2831 this.businessArrangement = new StringType(); // bb 2832 return this.businessArrangement; 2833 } 2834 2835 public boolean hasBusinessArrangementElement() { 2836 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2837 } 2838 2839 public boolean hasBusinessArrangement() { 2840 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2841 } 2842 2843 /** 2844 * @param value {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2845 */ 2846 public InsuranceComponent setBusinessArrangementElement(StringType value) { 2847 this.businessArrangement = value; 2848 return this; 2849 } 2850 2851 /** 2852 * @return A business agreement number established between the provider and the insurer for special business processing purposes. 2853 */ 2854 public String getBusinessArrangement() { 2855 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 2856 } 2857 2858 /** 2859 * @param value A business agreement number established between the provider and the insurer for special business processing purposes. 2860 */ 2861 public InsuranceComponent setBusinessArrangement(String value) { 2862 if (Utilities.noString(value)) 2863 this.businessArrangement = null; 2864 else { 2865 if (this.businessArrangement == null) 2866 this.businessArrangement = new StringType(); 2867 this.businessArrangement.setValue(value); 2868 } 2869 return this; 2870 } 2871 2872 /** 2873 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2874 */ 2875 public List<StringType> getPreAuthRef() { 2876 if (this.preAuthRef == null) 2877 this.preAuthRef = new ArrayList<StringType>(); 2878 return this.preAuthRef; 2879 } 2880 2881 /** 2882 * @return Returns a reference to <code>this</code> for easy method chaining 2883 */ 2884 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2885 this.preAuthRef = thePreAuthRef; 2886 return this; 2887 } 2888 2889 public boolean hasPreAuthRef() { 2890 if (this.preAuthRef == null) 2891 return false; 2892 for (StringType item : this.preAuthRef) 2893 if (!item.isEmpty()) 2894 return true; 2895 return false; 2896 } 2897 2898 /** 2899 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2900 */ 2901 public StringType addPreAuthRefElement() {//2 2902 StringType t = new StringType(); 2903 if (this.preAuthRef == null) 2904 this.preAuthRef = new ArrayList<StringType>(); 2905 this.preAuthRef.add(t); 2906 return t; 2907 } 2908 2909 /** 2910 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2911 */ 2912 public InsuranceComponent addPreAuthRef(String value) { //1 2913 StringType t = new StringType(); 2914 t.setValue(value); 2915 if (this.preAuthRef == null) 2916 this.preAuthRef = new ArrayList<StringType>(); 2917 this.preAuthRef.add(t); 2918 return this; 2919 } 2920 2921 /** 2922 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2923 */ 2924 public boolean hasPreAuthRef(String value) { 2925 if (this.preAuthRef == null) 2926 return false; 2927 for (StringType v : this.preAuthRef) 2928 if (v.getValue().equals(value)) // string 2929 return true; 2930 return false; 2931 } 2932 2933 /** 2934 * @return {@link #claimResponse} (The result of the adjudication of the line items for the Coverage specified in this insurance.) 2935 */ 2936 public Reference getClaimResponse() { 2937 if (this.claimResponse == null) 2938 if (Configuration.errorOnAutoCreate()) 2939 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 2940 else if (Configuration.doAutoCreate()) 2941 this.claimResponse = new Reference(); // cc 2942 return this.claimResponse; 2943 } 2944 2945 public boolean hasClaimResponse() { 2946 return this.claimResponse != null && !this.claimResponse.isEmpty(); 2947 } 2948 2949 /** 2950 * @param value {@link #claimResponse} (The result of the adjudication of the line items for the Coverage specified in this insurance.) 2951 */ 2952 public InsuranceComponent setClaimResponse(Reference value) { 2953 this.claimResponse = value; 2954 return this; 2955 } 2956 2957 protected void listChildren(List<Property> children) { 2958 super.listChildren(children); 2959 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 0, 1, sequence)); 2960 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal)); 2961 children.add(new Property("identifier", "Identifier", "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 0, 1, identifier)); 2962 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 2963 children.add(new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement)); 2964 children.add(new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 2965 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, claimResponse)); 2966 } 2967 2968 @Override 2969 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2970 switch (_hash) { 2971 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 0, 1, sequence); 2972 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal); 2973 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 0, 1, identifier); 2974 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 2975 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement); 2976 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 2977 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, claimResponse); 2978 default: return super.getNamedProperty(_hash, _name, _checkValid); 2979 } 2980 2981 } 2982 2983 @Override 2984 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2985 switch (hash) { 2986 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2987 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 2988 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2989 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 2990 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 2991 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 2992 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 2993 default: return super.getProperty(hash, name, checkValid); 2994 } 2995 2996 } 2997 2998 @Override 2999 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3000 switch (hash) { 3001 case 1349547969: // sequence 3002 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 3003 return value; 3004 case 97604197: // focal 3005 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 3006 return value; 3007 case -1618432855: // identifier 3008 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3009 return value; 3010 case -351767064: // coverage 3011 this.coverage = TypeConvertor.castToReference(value); // Reference 3012 return value; 3013 case 259920682: // businessArrangement 3014 this.businessArrangement = TypeConvertor.castToString(value); // StringType 3015 return value; 3016 case 522246568: // preAuthRef 3017 this.getPreAuthRef().add(TypeConvertor.castToString(value)); // StringType 3018 return value; 3019 case 689513629: // claimResponse 3020 this.claimResponse = TypeConvertor.castToReference(value); // Reference 3021 return value; 3022 default: return super.setProperty(hash, name, value); 3023 } 3024 3025 } 3026 3027 @Override 3028 public Base setProperty(String name, Base value) throws FHIRException { 3029 if (name.equals("sequence")) { 3030 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 3031 } else if (name.equals("focal")) { 3032 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 3033 } else if (name.equals("identifier")) { 3034 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3035 } else if (name.equals("coverage")) { 3036 this.coverage = TypeConvertor.castToReference(value); // Reference 3037 } else if (name.equals("businessArrangement")) { 3038 this.businessArrangement = TypeConvertor.castToString(value); // StringType 3039 } else if (name.equals("preAuthRef")) { 3040 this.getPreAuthRef().add(TypeConvertor.castToString(value)); 3041 } else if (name.equals("claimResponse")) { 3042 this.claimResponse = TypeConvertor.castToReference(value); // Reference 3043 } else 3044 return super.setProperty(name, value); 3045 return value; 3046 } 3047 3048 @Override 3049 public Base makeProperty(int hash, String name) throws FHIRException { 3050 switch (hash) { 3051 case 1349547969: return getSequenceElement(); 3052 case 97604197: return getFocalElement(); 3053 case -1618432855: return getIdentifier(); 3054 case -351767064: return getCoverage(); 3055 case 259920682: return getBusinessArrangementElement(); 3056 case 522246568: return addPreAuthRefElement(); 3057 case 689513629: return getClaimResponse(); 3058 default: return super.makeProperty(hash, name); 3059 } 3060 3061 } 3062 3063 @Override 3064 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3065 switch (hash) { 3066 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 3067 case 97604197: /*focal*/ return new String[] {"boolean"}; 3068 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3069 case -351767064: /*coverage*/ return new String[] {"Reference"}; 3070 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 3071 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 3072 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 3073 default: return super.getTypesForProperty(hash, name); 3074 } 3075 3076 } 3077 3078 @Override 3079 public Base addChild(String name) throws FHIRException { 3080 if (name.equals("sequence")) { 3081 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.sequence"); 3082 } 3083 else if (name.equals("focal")) { 3084 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.focal"); 3085 } 3086 else if (name.equals("identifier")) { 3087 this.identifier = new Identifier(); 3088 return this.identifier; 3089 } 3090 else if (name.equals("coverage")) { 3091 this.coverage = new Reference(); 3092 return this.coverage; 3093 } 3094 else if (name.equals("businessArrangement")) { 3095 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.businessArrangement"); 3096 } 3097 else if (name.equals("preAuthRef")) { 3098 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.preAuthRef"); 3099 } 3100 else if (name.equals("claimResponse")) { 3101 this.claimResponse = new Reference(); 3102 return this.claimResponse; 3103 } 3104 else 3105 return super.addChild(name); 3106 } 3107 3108 public InsuranceComponent copy() { 3109 InsuranceComponent dst = new InsuranceComponent(); 3110 copyValues(dst); 3111 return dst; 3112 } 3113 3114 public void copyValues(InsuranceComponent dst) { 3115 super.copyValues(dst); 3116 dst.sequence = sequence == null ? null : sequence.copy(); 3117 dst.focal = focal == null ? null : focal.copy(); 3118 dst.identifier = identifier == null ? null : identifier.copy(); 3119 dst.coverage = coverage == null ? null : coverage.copy(); 3120 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3121 if (preAuthRef != null) { 3122 dst.preAuthRef = new ArrayList<StringType>(); 3123 for (StringType i : preAuthRef) 3124 dst.preAuthRef.add(i.copy()); 3125 }; 3126 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3127 } 3128 3129 @Override 3130 public boolean equalsDeep(Base other_) { 3131 if (!super.equalsDeep(other_)) 3132 return false; 3133 if (!(other_ instanceof InsuranceComponent)) 3134 return false; 3135 InsuranceComponent o = (InsuranceComponent) other_; 3136 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) && compareDeep(identifier, o.identifier, true) 3137 && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 3138 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(claimResponse, o.claimResponse, true) 3139 ; 3140 } 3141 3142 @Override 3143 public boolean equalsShallow(Base other_) { 3144 if (!super.equalsShallow(other_)) 3145 return false; 3146 if (!(other_ instanceof InsuranceComponent)) 3147 return false; 3148 InsuranceComponent o = (InsuranceComponent) other_; 3149 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 3150 && compareValues(preAuthRef, o.preAuthRef, true); 3151 } 3152 3153 public boolean isEmpty() { 3154 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, identifier 3155 , coverage, businessArrangement, preAuthRef, claimResponse); 3156 } 3157 3158 public String fhirType() { 3159 return "Claim.insurance"; 3160 3161 } 3162 3163 } 3164 3165 @Block() 3166 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3167 /** 3168 * Date of an accident event related to the products and services contained in the claim. 3169 */ 3170 @Child(name = "date", type = {DateType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3171 @Description(shortDefinition="When the incident occurred", formalDefinition="Date of an accident event related to the products and services contained in the claim." ) 3172 protected DateType date; 3173 3174 /** 3175 * The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers. 3176 */ 3177 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3178 @Description(shortDefinition="The nature of the accident", formalDefinition="The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers." ) 3179 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 3180 protected CodeableConcept type; 3181 3182 /** 3183 * The physical location of the accident event. 3184 */ 3185 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 3186 @Description(shortDefinition="Where the event occurred", formalDefinition="The physical location of the accident event." ) 3187 protected DataType location; 3188 3189 private static final long serialVersionUID = 11882722L; 3190 3191 /** 3192 * Constructor 3193 */ 3194 public AccidentComponent() { 3195 super(); 3196 } 3197 3198 /** 3199 * Constructor 3200 */ 3201 public AccidentComponent(Date date) { 3202 super(); 3203 this.setDate(date); 3204 } 3205 3206 /** 3207 * @return {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3208 */ 3209 public DateType getDateElement() { 3210 if (this.date == null) 3211 if (Configuration.errorOnAutoCreate()) 3212 throw new Error("Attempt to auto-create AccidentComponent.date"); 3213 else if (Configuration.doAutoCreate()) 3214 this.date = new DateType(); // bb 3215 return this.date; 3216 } 3217 3218 public boolean hasDateElement() { 3219 return this.date != null && !this.date.isEmpty(); 3220 } 3221 3222 public boolean hasDate() { 3223 return this.date != null && !this.date.isEmpty(); 3224 } 3225 3226 /** 3227 * @param value {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3228 */ 3229 public AccidentComponent setDateElement(DateType value) { 3230 this.date = value; 3231 return this; 3232 } 3233 3234 /** 3235 * @return Date of an accident event related to the products and services contained in the claim. 3236 */ 3237 public Date getDate() { 3238 return this.date == null ? null : this.date.getValue(); 3239 } 3240 3241 /** 3242 * @param value Date of an accident event related to the products and services contained in the claim. 3243 */ 3244 public AccidentComponent setDate(Date value) { 3245 if (this.date == null) 3246 this.date = new DateType(); 3247 this.date.setValue(value); 3248 return this; 3249 } 3250 3251 /** 3252 * @return {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3253 */ 3254 public CodeableConcept getType() { 3255 if (this.type == null) 3256 if (Configuration.errorOnAutoCreate()) 3257 throw new Error("Attempt to auto-create AccidentComponent.type"); 3258 else if (Configuration.doAutoCreate()) 3259 this.type = new CodeableConcept(); // cc 3260 return this.type; 3261 } 3262 3263 public boolean hasType() { 3264 return this.type != null && !this.type.isEmpty(); 3265 } 3266 3267 /** 3268 * @param value {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3269 */ 3270 public AccidentComponent setType(CodeableConcept value) { 3271 this.type = value; 3272 return this; 3273 } 3274 3275 /** 3276 * @return {@link #location} (The physical location of the accident event.) 3277 */ 3278 public DataType getLocation() { 3279 return this.location; 3280 } 3281 3282 /** 3283 * @return {@link #location} (The physical location of the accident event.) 3284 */ 3285 public Address getLocationAddress() throws FHIRException { 3286 if (this.location == null) 3287 this.location = new Address(); 3288 if (!(this.location instanceof Address)) 3289 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3290 return (Address) this.location; 3291 } 3292 3293 public boolean hasLocationAddress() { 3294 return this != null && this.location instanceof Address; 3295 } 3296 3297 /** 3298 * @return {@link #location} (The physical location of the accident event.) 3299 */ 3300 public Reference getLocationReference() throws FHIRException { 3301 if (this.location == null) 3302 this.location = new Reference(); 3303 if (!(this.location instanceof Reference)) 3304 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3305 return (Reference) this.location; 3306 } 3307 3308 public boolean hasLocationReference() { 3309 return this != null && this.location instanceof Reference; 3310 } 3311 3312 public boolean hasLocation() { 3313 return this.location != null && !this.location.isEmpty(); 3314 } 3315 3316 /** 3317 * @param value {@link #location} (The physical location of the accident event.) 3318 */ 3319 public AccidentComponent setLocation(DataType value) { 3320 if (value != null && !(value instanceof Address || value instanceof Reference)) 3321 throw new FHIRException("Not the right type for Claim.accident.location[x]: "+value.fhirType()); 3322 this.location = value; 3323 return this; 3324 } 3325 3326 protected void listChildren(List<Property> children) { 3327 super.listChildren(children); 3328 children.add(new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 3329 children.add(new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type)); 3330 children.add(new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location)); 3331 } 3332 3333 @Override 3334 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3335 switch (_hash) { 3336 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 3337 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type); 3338 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3339 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3340 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "The physical location of the accident event.", 0, 1, location); 3341 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3342 default: return super.getNamedProperty(_hash, _name, _checkValid); 3343 } 3344 3345 } 3346 3347 @Override 3348 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3349 switch (hash) { 3350 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3351 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3352 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 3353 default: return super.getProperty(hash, name, checkValid); 3354 } 3355 3356 } 3357 3358 @Override 3359 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3360 switch (hash) { 3361 case 3076014: // date 3362 this.date = TypeConvertor.castToDate(value); // DateType 3363 return value; 3364 case 3575610: // type 3365 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3366 return value; 3367 case 1901043637: // location 3368 this.location = TypeConvertor.castToType(value); // DataType 3369 return value; 3370 default: return super.setProperty(hash, name, value); 3371 } 3372 3373 } 3374 3375 @Override 3376 public Base setProperty(String name, Base value) throws FHIRException { 3377 if (name.equals("date")) { 3378 this.date = TypeConvertor.castToDate(value); // DateType 3379 } else if (name.equals("type")) { 3380 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3381 } else if (name.equals("location[x]")) { 3382 this.location = TypeConvertor.castToType(value); // DataType 3383 } else 3384 return super.setProperty(name, value); 3385 return value; 3386 } 3387 3388 @Override 3389 public Base makeProperty(int hash, String name) throws FHIRException { 3390 switch (hash) { 3391 case 3076014: return getDateElement(); 3392 case 3575610: return getType(); 3393 case 552316075: return getLocation(); 3394 case 1901043637: return getLocation(); 3395 default: return super.makeProperty(hash, name); 3396 } 3397 3398 } 3399 3400 @Override 3401 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3402 switch (hash) { 3403 case 3076014: /*date*/ return new String[] {"date"}; 3404 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3405 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 3406 default: return super.getTypesForProperty(hash, name); 3407 } 3408 3409 } 3410 3411 @Override 3412 public Base addChild(String name) throws FHIRException { 3413 if (name.equals("date")) { 3414 throw new FHIRException("Cannot call addChild on a singleton property Claim.accident.date"); 3415 } 3416 else if (name.equals("type")) { 3417 this.type = new CodeableConcept(); 3418 return this.type; 3419 } 3420 else if (name.equals("locationAddress")) { 3421 this.location = new Address(); 3422 return this.location; 3423 } 3424 else if (name.equals("locationReference")) { 3425 this.location = new Reference(); 3426 return this.location; 3427 } 3428 else 3429 return super.addChild(name); 3430 } 3431 3432 public AccidentComponent copy() { 3433 AccidentComponent dst = new AccidentComponent(); 3434 copyValues(dst); 3435 return dst; 3436 } 3437 3438 public void copyValues(AccidentComponent dst) { 3439 super.copyValues(dst); 3440 dst.date = date == null ? null : date.copy(); 3441 dst.type = type == null ? null : type.copy(); 3442 dst.location = location == null ? null : location.copy(); 3443 } 3444 3445 @Override 3446 public boolean equalsDeep(Base other_) { 3447 if (!super.equalsDeep(other_)) 3448 return false; 3449 if (!(other_ instanceof AccidentComponent)) 3450 return false; 3451 AccidentComponent o = (AccidentComponent) other_; 3452 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 3453 ; 3454 } 3455 3456 @Override 3457 public boolean equalsShallow(Base other_) { 3458 if (!super.equalsShallow(other_)) 3459 return false; 3460 if (!(other_ instanceof AccidentComponent)) 3461 return false; 3462 AccidentComponent o = (AccidentComponent) other_; 3463 return compareValues(date, o.date, true); 3464 } 3465 3466 public boolean isEmpty() { 3467 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 3468 } 3469 3470 public String fhirType() { 3471 return "Claim.accident"; 3472 3473 } 3474 3475 } 3476 3477 @Block() 3478 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 3479 /** 3480 * A number to uniquely identify item entries. 3481 */ 3482 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3483 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 3484 protected PositiveIntType sequence; 3485 3486 /** 3487 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 3488 */ 3489 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3490 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 3491 protected List<Identifier> traceNumber; 3492 3493 /** 3494 * CareTeam members related to this service or product. 3495 */ 3496 @Child(name = "careTeamSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3497 @Description(shortDefinition="Applicable careTeam members", formalDefinition="CareTeam members related to this service or product." ) 3498 protected List<PositiveIntType> careTeamSequence; 3499 3500 /** 3501 * Diagnosis applicable for this service or product. 3502 */ 3503 @Child(name = "diagnosisSequence", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3504 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnosis applicable for this service or product." ) 3505 protected List<PositiveIntType> diagnosisSequence; 3506 3507 /** 3508 * Procedures applicable for this service or product. 3509 */ 3510 @Child(name = "procedureSequence", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3511 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product." ) 3512 protected List<PositiveIntType> procedureSequence; 3513 3514 /** 3515 * Exceptions, special conditions and supporting information applicable for this service or product. 3516 */ 3517 @Child(name = "informationSequence", type = {PositiveIntType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3518 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information applicable for this service or product." ) 3519 protected List<PositiveIntType> informationSequence; 3520 3521 /** 3522 * The type of revenue or cost center providing the product and/or service. 3523 */ 3524 @Child(name = "revenue", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 3525 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 3526 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 3527 protected CodeableConcept revenue; 3528 3529 /** 3530 * Code to identify the general type of benefits under which products and services are provided. 3531 */ 3532 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3533 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 3534 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 3535 protected CodeableConcept category; 3536 3537 /** 3538 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 3539 */ 3540 @Child(name = "productOrService", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 3541 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 3542 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3543 protected CodeableConcept productOrService; 3544 3545 /** 3546 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 3547 */ 3548 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 3549 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 3550 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3551 protected CodeableConcept productOrServiceEnd; 3552 3553 /** 3554 * Request or Referral for Goods or Service to be rendered. 3555 */ 3556 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3557 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 3558 protected List<Reference> request; 3559 3560 /** 3561 * Item typification or modifiers codes to convey additional context for the product or service. 3562 */ 3563 @Child(name = "modifier", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3564 @Description(shortDefinition="Product or service billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 3565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3566 protected List<CodeableConcept> modifier; 3567 3568 /** 3569 * Identifies the program under which this may be recovered. 3570 */ 3571 @Child(name = "programCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3572 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 3573 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3574 protected List<CodeableConcept> programCode; 3575 3576 /** 3577 * The date or dates when the service or product was supplied, performed or completed. 3578 */ 3579 @Child(name = "serviced", type = {DateType.class, Period.class}, order=14, min=0, max=1, modifier=false, summary=false) 3580 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 3581 protected DataType serviced; 3582 3583 /** 3584 * Where the product or service was provided. 3585 */ 3586 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=15, min=0, max=1, modifier=false, summary=false) 3587 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 3588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3589 protected DataType location; 3590 3591 /** 3592 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 3593 */ 3594 @Child(name = "patientPaid", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3595 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 3596 protected Money patientPaid; 3597 3598 /** 3599 * The number of repetitions of a service or product. 3600 */ 3601 @Child(name = "quantity", type = {Quantity.class}, order=17, min=0, max=1, modifier=false, summary=false) 3602 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 3603 protected Quantity quantity; 3604 3605 /** 3606 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 3607 */ 3608 @Child(name = "unitPrice", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 3609 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 3610 protected Money unitPrice; 3611 3612 /** 3613 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3614 */ 3615 @Child(name = "factor", type = {DecimalType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3616 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3617 protected DecimalType factor; 3618 3619 /** 3620 * The total of taxes applicable for this product or service. 3621 */ 3622 @Child(name = "tax", type = {Money.class}, order=20, min=0, max=1, modifier=false, summary=false) 3623 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 3624 protected Money tax; 3625 3626 /** 3627 * The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor. 3628 */ 3629 @Child(name = "net", type = {Money.class}, order=21, min=0, max=1, modifier=false, summary=false) 3630 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor." ) 3631 protected Money net; 3632 3633 /** 3634 * Unique Device Identifiers associated with this line item. 3635 */ 3636 @Child(name = "udi", type = {Device.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3637 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 3638 protected List<Reference> udi; 3639 3640 /** 3641 * Physical location where the service is performed or applies. 3642 */ 3643 @Child(name = "bodySite", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3644 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 3645 protected List<BodySiteComponent> bodySite; 3646 3647 /** 3648 * Healthcare encounters related to this claim. 3649 */ 3650 @Child(name = "encounter", type = {Encounter.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3651 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 3652 protected List<Reference> encounter; 3653 3654 /** 3655 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 3656 */ 3657 @Child(name = "detail", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3658 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 3659 protected List<DetailComponent> detail; 3660 3661 private static final long serialVersionUID = -1174144448L; 3662 3663 /** 3664 * Constructor 3665 */ 3666 public ItemComponent() { 3667 super(); 3668 } 3669 3670 /** 3671 * Constructor 3672 */ 3673 public ItemComponent(int sequence) { 3674 super(); 3675 this.setSequence(sequence); 3676 } 3677 3678 /** 3679 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3680 */ 3681 public PositiveIntType getSequenceElement() { 3682 if (this.sequence == null) 3683 if (Configuration.errorOnAutoCreate()) 3684 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3685 else if (Configuration.doAutoCreate()) 3686 this.sequence = new PositiveIntType(); // bb 3687 return this.sequence; 3688 } 3689 3690 public boolean hasSequenceElement() { 3691 return this.sequence != null && !this.sequence.isEmpty(); 3692 } 3693 3694 public boolean hasSequence() { 3695 return this.sequence != null && !this.sequence.isEmpty(); 3696 } 3697 3698 /** 3699 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3700 */ 3701 public ItemComponent setSequenceElement(PositiveIntType value) { 3702 this.sequence = value; 3703 return this; 3704 } 3705 3706 /** 3707 * @return A number to uniquely identify item entries. 3708 */ 3709 public int getSequence() { 3710 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3711 } 3712 3713 /** 3714 * @param value A number to uniquely identify item entries. 3715 */ 3716 public ItemComponent setSequence(int value) { 3717 if (this.sequence == null) 3718 this.sequence = new PositiveIntType(); 3719 this.sequence.setValue(value); 3720 return this; 3721 } 3722 3723 /** 3724 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 3725 */ 3726 public List<Identifier> getTraceNumber() { 3727 if (this.traceNumber == null) 3728 this.traceNumber = new ArrayList<Identifier>(); 3729 return this.traceNumber; 3730 } 3731 3732 /** 3733 * @return Returns a reference to <code>this</code> for easy method chaining 3734 */ 3735 public ItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 3736 this.traceNumber = theTraceNumber; 3737 return this; 3738 } 3739 3740 public boolean hasTraceNumber() { 3741 if (this.traceNumber == null) 3742 return false; 3743 for (Identifier item : this.traceNumber) 3744 if (!item.isEmpty()) 3745 return true; 3746 return false; 3747 } 3748 3749 public Identifier addTraceNumber() { //3 3750 Identifier t = new Identifier(); 3751 if (this.traceNumber == null) 3752 this.traceNumber = new ArrayList<Identifier>(); 3753 this.traceNumber.add(t); 3754 return t; 3755 } 3756 3757 public ItemComponent addTraceNumber(Identifier t) { //3 3758 if (t == null) 3759 return this; 3760 if (this.traceNumber == null) 3761 this.traceNumber = new ArrayList<Identifier>(); 3762 this.traceNumber.add(t); 3763 return this; 3764 } 3765 3766 /** 3767 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 3768 */ 3769 public Identifier getTraceNumberFirstRep() { 3770 if (getTraceNumber().isEmpty()) { 3771 addTraceNumber(); 3772 } 3773 return getTraceNumber().get(0); 3774 } 3775 3776 /** 3777 * @return {@link #careTeamSequence} (CareTeam members related to this service or product.) 3778 */ 3779 public List<PositiveIntType> getCareTeamSequence() { 3780 if (this.careTeamSequence == null) 3781 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3782 return this.careTeamSequence; 3783 } 3784 3785 /** 3786 * @return Returns a reference to <code>this</code> for easy method chaining 3787 */ 3788 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 3789 this.careTeamSequence = theCareTeamSequence; 3790 return this; 3791 } 3792 3793 public boolean hasCareTeamSequence() { 3794 if (this.careTeamSequence == null) 3795 return false; 3796 for (PositiveIntType item : this.careTeamSequence) 3797 if (!item.isEmpty()) 3798 return true; 3799 return false; 3800 } 3801 3802 /** 3803 * @return {@link #careTeamSequence} (CareTeam members related to this service or product.) 3804 */ 3805 public PositiveIntType addCareTeamSequenceElement() {//2 3806 PositiveIntType t = new PositiveIntType(); 3807 if (this.careTeamSequence == null) 3808 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3809 this.careTeamSequence.add(t); 3810 return t; 3811 } 3812 3813 /** 3814 * @param value {@link #careTeamSequence} (CareTeam members related to this service or product.) 3815 */ 3816 public ItemComponent addCareTeamSequence(int value) { //1 3817 PositiveIntType t = new PositiveIntType(); 3818 t.setValue(value); 3819 if (this.careTeamSequence == null) 3820 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3821 this.careTeamSequence.add(t); 3822 return this; 3823 } 3824 3825 /** 3826 * @param value {@link #careTeamSequence} (CareTeam members related to this service or product.) 3827 */ 3828 public boolean hasCareTeamSequence(int value) { 3829 if (this.careTeamSequence == null) 3830 return false; 3831 for (PositiveIntType v : this.careTeamSequence) 3832 if (v.getValue().equals(value)) // positiveInt 3833 return true; 3834 return false; 3835 } 3836 3837 /** 3838 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 3839 */ 3840 public List<PositiveIntType> getDiagnosisSequence() { 3841 if (this.diagnosisSequence == null) 3842 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3843 return this.diagnosisSequence; 3844 } 3845 3846 /** 3847 * @return Returns a reference to <code>this</code> for easy method chaining 3848 */ 3849 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 3850 this.diagnosisSequence = theDiagnosisSequence; 3851 return this; 3852 } 3853 3854 public boolean hasDiagnosisSequence() { 3855 if (this.diagnosisSequence == null) 3856 return false; 3857 for (PositiveIntType item : this.diagnosisSequence) 3858 if (!item.isEmpty()) 3859 return true; 3860 return false; 3861 } 3862 3863 /** 3864 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 3865 */ 3866 public PositiveIntType addDiagnosisSequenceElement() {//2 3867 PositiveIntType t = new PositiveIntType(); 3868 if (this.diagnosisSequence == null) 3869 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3870 this.diagnosisSequence.add(t); 3871 return t; 3872 } 3873 3874 /** 3875 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 3876 */ 3877 public ItemComponent addDiagnosisSequence(int value) { //1 3878 PositiveIntType t = new PositiveIntType(); 3879 t.setValue(value); 3880 if (this.diagnosisSequence == null) 3881 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3882 this.diagnosisSequence.add(t); 3883 return this; 3884 } 3885 3886 /** 3887 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 3888 */ 3889 public boolean hasDiagnosisSequence(int value) { 3890 if (this.diagnosisSequence == null) 3891 return false; 3892 for (PositiveIntType v : this.diagnosisSequence) 3893 if (v.getValue().equals(value)) // positiveInt 3894 return true; 3895 return false; 3896 } 3897 3898 /** 3899 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 3900 */ 3901 public List<PositiveIntType> getProcedureSequence() { 3902 if (this.procedureSequence == null) 3903 this.procedureSequence = new ArrayList<PositiveIntType>(); 3904 return this.procedureSequence; 3905 } 3906 3907 /** 3908 * @return Returns a reference to <code>this</code> for easy method chaining 3909 */ 3910 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 3911 this.procedureSequence = theProcedureSequence; 3912 return this; 3913 } 3914 3915 public boolean hasProcedureSequence() { 3916 if (this.procedureSequence == null) 3917 return false; 3918 for (PositiveIntType item : this.procedureSequence) 3919 if (!item.isEmpty()) 3920 return true; 3921 return false; 3922 } 3923 3924 /** 3925 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 3926 */ 3927 public PositiveIntType addProcedureSequenceElement() {//2 3928 PositiveIntType t = new PositiveIntType(); 3929 if (this.procedureSequence == null) 3930 this.procedureSequence = new ArrayList<PositiveIntType>(); 3931 this.procedureSequence.add(t); 3932 return t; 3933 } 3934 3935 /** 3936 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 3937 */ 3938 public ItemComponent addProcedureSequence(int value) { //1 3939 PositiveIntType t = new PositiveIntType(); 3940 t.setValue(value); 3941 if (this.procedureSequence == null) 3942 this.procedureSequence = new ArrayList<PositiveIntType>(); 3943 this.procedureSequence.add(t); 3944 return this; 3945 } 3946 3947 /** 3948 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 3949 */ 3950 public boolean hasProcedureSequence(int value) { 3951 if (this.procedureSequence == null) 3952 return false; 3953 for (PositiveIntType v : this.procedureSequence) 3954 if (v.getValue().equals(value)) // positiveInt 3955 return true; 3956 return false; 3957 } 3958 3959 /** 3960 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3961 */ 3962 public List<PositiveIntType> getInformationSequence() { 3963 if (this.informationSequence == null) 3964 this.informationSequence = new ArrayList<PositiveIntType>(); 3965 return this.informationSequence; 3966 } 3967 3968 /** 3969 * @return Returns a reference to <code>this</code> for easy method chaining 3970 */ 3971 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 3972 this.informationSequence = theInformationSequence; 3973 return this; 3974 } 3975 3976 public boolean hasInformationSequence() { 3977 if (this.informationSequence == null) 3978 return false; 3979 for (PositiveIntType item : this.informationSequence) 3980 if (!item.isEmpty()) 3981 return true; 3982 return false; 3983 } 3984 3985 /** 3986 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3987 */ 3988 public PositiveIntType addInformationSequenceElement() {//2 3989 PositiveIntType t = new PositiveIntType(); 3990 if (this.informationSequence == null) 3991 this.informationSequence = new ArrayList<PositiveIntType>(); 3992 this.informationSequence.add(t); 3993 return t; 3994 } 3995 3996 /** 3997 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3998 */ 3999 public ItemComponent addInformationSequence(int value) { //1 4000 PositiveIntType t = new PositiveIntType(); 4001 t.setValue(value); 4002 if (this.informationSequence == null) 4003 this.informationSequence = new ArrayList<PositiveIntType>(); 4004 this.informationSequence.add(t); 4005 return this; 4006 } 4007 4008 /** 4009 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 4010 */ 4011 public boolean hasInformationSequence(int value) { 4012 if (this.informationSequence == null) 4013 return false; 4014 for (PositiveIntType v : this.informationSequence) 4015 if (v.getValue().equals(value)) // positiveInt 4016 return true; 4017 return false; 4018 } 4019 4020 /** 4021 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4022 */ 4023 public CodeableConcept getRevenue() { 4024 if (this.revenue == null) 4025 if (Configuration.errorOnAutoCreate()) 4026 throw new Error("Attempt to auto-create ItemComponent.revenue"); 4027 else if (Configuration.doAutoCreate()) 4028 this.revenue = new CodeableConcept(); // cc 4029 return this.revenue; 4030 } 4031 4032 public boolean hasRevenue() { 4033 return this.revenue != null && !this.revenue.isEmpty(); 4034 } 4035 4036 /** 4037 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4038 */ 4039 public ItemComponent setRevenue(CodeableConcept value) { 4040 this.revenue = value; 4041 return this; 4042 } 4043 4044 /** 4045 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 4046 */ 4047 public CodeableConcept getCategory() { 4048 if (this.category == null) 4049 if (Configuration.errorOnAutoCreate()) 4050 throw new Error("Attempt to auto-create ItemComponent.category"); 4051 else if (Configuration.doAutoCreate()) 4052 this.category = new CodeableConcept(); // cc 4053 return this.category; 4054 } 4055 4056 public boolean hasCategory() { 4057 return this.category != null && !this.category.isEmpty(); 4058 } 4059 4060 /** 4061 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 4062 */ 4063 public ItemComponent setCategory(CodeableConcept value) { 4064 this.category = value; 4065 return this; 4066 } 4067 4068 /** 4069 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4070 */ 4071 public CodeableConcept getProductOrService() { 4072 if (this.productOrService == null) 4073 if (Configuration.errorOnAutoCreate()) 4074 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 4075 else if (Configuration.doAutoCreate()) 4076 this.productOrService = new CodeableConcept(); // cc 4077 return this.productOrService; 4078 } 4079 4080 public boolean hasProductOrService() { 4081 return this.productOrService != null && !this.productOrService.isEmpty(); 4082 } 4083 4084 /** 4085 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4086 */ 4087 public ItemComponent setProductOrService(CodeableConcept value) { 4088 this.productOrService = value; 4089 return this; 4090 } 4091 4092 /** 4093 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4094 */ 4095 public CodeableConcept getProductOrServiceEnd() { 4096 if (this.productOrServiceEnd == null) 4097 if (Configuration.errorOnAutoCreate()) 4098 throw new Error("Attempt to auto-create ItemComponent.productOrServiceEnd"); 4099 else if (Configuration.doAutoCreate()) 4100 this.productOrServiceEnd = new CodeableConcept(); // cc 4101 return this.productOrServiceEnd; 4102 } 4103 4104 public boolean hasProductOrServiceEnd() { 4105 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 4106 } 4107 4108 /** 4109 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4110 */ 4111 public ItemComponent setProductOrServiceEnd(CodeableConcept value) { 4112 this.productOrServiceEnd = value; 4113 return this; 4114 } 4115 4116 /** 4117 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 4118 */ 4119 public List<Reference> getRequest() { 4120 if (this.request == null) 4121 this.request = new ArrayList<Reference>(); 4122 return this.request; 4123 } 4124 4125 /** 4126 * @return Returns a reference to <code>this</code> for easy method chaining 4127 */ 4128 public ItemComponent setRequest(List<Reference> theRequest) { 4129 this.request = theRequest; 4130 return this; 4131 } 4132 4133 public boolean hasRequest() { 4134 if (this.request == null) 4135 return false; 4136 for (Reference item : this.request) 4137 if (!item.isEmpty()) 4138 return true; 4139 return false; 4140 } 4141 4142 public Reference addRequest() { //3 4143 Reference t = new Reference(); 4144 if (this.request == null) 4145 this.request = new ArrayList<Reference>(); 4146 this.request.add(t); 4147 return t; 4148 } 4149 4150 public ItemComponent addRequest(Reference t) { //3 4151 if (t == null) 4152 return this; 4153 if (this.request == null) 4154 this.request = new ArrayList<Reference>(); 4155 this.request.add(t); 4156 return this; 4157 } 4158 4159 /** 4160 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 4161 */ 4162 public Reference getRequestFirstRep() { 4163 if (getRequest().isEmpty()) { 4164 addRequest(); 4165 } 4166 return getRequest().get(0); 4167 } 4168 4169 /** 4170 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 4171 */ 4172 public List<CodeableConcept> getModifier() { 4173 if (this.modifier == null) 4174 this.modifier = new ArrayList<CodeableConcept>(); 4175 return this.modifier; 4176 } 4177 4178 /** 4179 * @return Returns a reference to <code>this</code> for easy method chaining 4180 */ 4181 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 4182 this.modifier = theModifier; 4183 return this; 4184 } 4185 4186 public boolean hasModifier() { 4187 if (this.modifier == null) 4188 return false; 4189 for (CodeableConcept item : this.modifier) 4190 if (!item.isEmpty()) 4191 return true; 4192 return false; 4193 } 4194 4195 public CodeableConcept addModifier() { //3 4196 CodeableConcept t = new CodeableConcept(); 4197 if (this.modifier == null) 4198 this.modifier = new ArrayList<CodeableConcept>(); 4199 this.modifier.add(t); 4200 return t; 4201 } 4202 4203 public ItemComponent addModifier(CodeableConcept t) { //3 4204 if (t == null) 4205 return this; 4206 if (this.modifier == null) 4207 this.modifier = new ArrayList<CodeableConcept>(); 4208 this.modifier.add(t); 4209 return this; 4210 } 4211 4212 /** 4213 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 4214 */ 4215 public CodeableConcept getModifierFirstRep() { 4216 if (getModifier().isEmpty()) { 4217 addModifier(); 4218 } 4219 return getModifier().get(0); 4220 } 4221 4222 /** 4223 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 4224 */ 4225 public List<CodeableConcept> getProgramCode() { 4226 if (this.programCode == null) 4227 this.programCode = new ArrayList<CodeableConcept>(); 4228 return this.programCode; 4229 } 4230 4231 /** 4232 * @return Returns a reference to <code>this</code> for easy method chaining 4233 */ 4234 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 4235 this.programCode = theProgramCode; 4236 return this; 4237 } 4238 4239 public boolean hasProgramCode() { 4240 if (this.programCode == null) 4241 return false; 4242 for (CodeableConcept item : this.programCode) 4243 if (!item.isEmpty()) 4244 return true; 4245 return false; 4246 } 4247 4248 public CodeableConcept addProgramCode() { //3 4249 CodeableConcept t = new CodeableConcept(); 4250 if (this.programCode == null) 4251 this.programCode = new ArrayList<CodeableConcept>(); 4252 this.programCode.add(t); 4253 return t; 4254 } 4255 4256 public ItemComponent addProgramCode(CodeableConcept t) { //3 4257 if (t == null) 4258 return this; 4259 if (this.programCode == null) 4260 this.programCode = new ArrayList<CodeableConcept>(); 4261 this.programCode.add(t); 4262 return this; 4263 } 4264 4265 /** 4266 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 4267 */ 4268 public CodeableConcept getProgramCodeFirstRep() { 4269 if (getProgramCode().isEmpty()) { 4270 addProgramCode(); 4271 } 4272 return getProgramCode().get(0); 4273 } 4274 4275 /** 4276 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4277 */ 4278 public DataType getServiced() { 4279 return this.serviced; 4280 } 4281 4282 /** 4283 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4284 */ 4285 public DateType getServicedDateType() throws FHIRException { 4286 if (this.serviced == null) 4287 this.serviced = new DateType(); 4288 if (!(this.serviced instanceof DateType)) 4289 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4290 return (DateType) this.serviced; 4291 } 4292 4293 public boolean hasServicedDateType() { 4294 return this != null && this.serviced instanceof DateType; 4295 } 4296 4297 /** 4298 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4299 */ 4300 public Period getServicedPeriod() throws FHIRException { 4301 if (this.serviced == null) 4302 this.serviced = new Period(); 4303 if (!(this.serviced instanceof Period)) 4304 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4305 return (Period) this.serviced; 4306 } 4307 4308 public boolean hasServicedPeriod() { 4309 return this != null && this.serviced instanceof Period; 4310 } 4311 4312 public boolean hasServiced() { 4313 return this.serviced != null && !this.serviced.isEmpty(); 4314 } 4315 4316 /** 4317 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4318 */ 4319 public ItemComponent setServiced(DataType value) { 4320 if (value != null && !(value instanceof DateType || value instanceof Period)) 4321 throw new FHIRException("Not the right type for Claim.item.serviced[x]: "+value.fhirType()); 4322 this.serviced = value; 4323 return this; 4324 } 4325 4326 /** 4327 * @return {@link #location} (Where the product or service was provided.) 4328 */ 4329 public DataType getLocation() { 4330 return this.location; 4331 } 4332 4333 /** 4334 * @return {@link #location} (Where the product or service was provided.) 4335 */ 4336 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 4337 if (this.location == null) 4338 this.location = new CodeableConcept(); 4339 if (!(this.location instanceof CodeableConcept)) 4340 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 4341 return (CodeableConcept) this.location; 4342 } 4343 4344 public boolean hasLocationCodeableConcept() { 4345 return this != null && this.location instanceof CodeableConcept; 4346 } 4347 4348 /** 4349 * @return {@link #location} (Where the product or service was provided.) 4350 */ 4351 public Address getLocationAddress() throws FHIRException { 4352 if (this.location == null) 4353 this.location = new Address(); 4354 if (!(this.location instanceof Address)) 4355 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 4356 return (Address) this.location; 4357 } 4358 4359 public boolean hasLocationAddress() { 4360 return this != null && this.location instanceof Address; 4361 } 4362 4363 /** 4364 * @return {@link #location} (Where the product or service was provided.) 4365 */ 4366 public Reference getLocationReference() throws FHIRException { 4367 if (this.location == null) 4368 this.location = new Reference(); 4369 if (!(this.location instanceof Reference)) 4370 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 4371 return (Reference) this.location; 4372 } 4373 4374 public boolean hasLocationReference() { 4375 return this != null && this.location instanceof Reference; 4376 } 4377 4378 public boolean hasLocation() { 4379 return this.location != null && !this.location.isEmpty(); 4380 } 4381 4382 /** 4383 * @param value {@link #location} (Where the product or service was provided.) 4384 */ 4385 public ItemComponent setLocation(DataType value) { 4386 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 4387 throw new FHIRException("Not the right type for Claim.item.location[x]: "+value.fhirType()); 4388 this.location = value; 4389 return this; 4390 } 4391 4392 /** 4393 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4394 */ 4395 public Money getPatientPaid() { 4396 if (this.patientPaid == null) 4397 if (Configuration.errorOnAutoCreate()) 4398 throw new Error("Attempt to auto-create ItemComponent.patientPaid"); 4399 else if (Configuration.doAutoCreate()) 4400 this.patientPaid = new Money(); // cc 4401 return this.patientPaid; 4402 } 4403 4404 public boolean hasPatientPaid() { 4405 return this.patientPaid != null && !this.patientPaid.isEmpty(); 4406 } 4407 4408 /** 4409 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4410 */ 4411 public ItemComponent setPatientPaid(Money value) { 4412 this.patientPaid = value; 4413 return this; 4414 } 4415 4416 /** 4417 * @return {@link #quantity} (The number of repetitions of a service or product.) 4418 */ 4419 public Quantity getQuantity() { 4420 if (this.quantity == null) 4421 if (Configuration.errorOnAutoCreate()) 4422 throw new Error("Attempt to auto-create ItemComponent.quantity"); 4423 else if (Configuration.doAutoCreate()) 4424 this.quantity = new Quantity(); // cc 4425 return this.quantity; 4426 } 4427 4428 public boolean hasQuantity() { 4429 return this.quantity != null && !this.quantity.isEmpty(); 4430 } 4431 4432 /** 4433 * @param value {@link #quantity} (The number of repetitions of a service or product.) 4434 */ 4435 public ItemComponent setQuantity(Quantity value) { 4436 this.quantity = value; 4437 return this; 4438 } 4439 4440 /** 4441 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4442 */ 4443 public Money getUnitPrice() { 4444 if (this.unitPrice == null) 4445 if (Configuration.errorOnAutoCreate()) 4446 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 4447 else if (Configuration.doAutoCreate()) 4448 this.unitPrice = new Money(); // cc 4449 return this.unitPrice; 4450 } 4451 4452 public boolean hasUnitPrice() { 4453 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4454 } 4455 4456 /** 4457 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4458 */ 4459 public ItemComponent setUnitPrice(Money value) { 4460 this.unitPrice = value; 4461 return this; 4462 } 4463 4464 /** 4465 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4466 */ 4467 public DecimalType getFactorElement() { 4468 if (this.factor == null) 4469 if (Configuration.errorOnAutoCreate()) 4470 throw new Error("Attempt to auto-create ItemComponent.factor"); 4471 else if (Configuration.doAutoCreate()) 4472 this.factor = new DecimalType(); // bb 4473 return this.factor; 4474 } 4475 4476 public boolean hasFactorElement() { 4477 return this.factor != null && !this.factor.isEmpty(); 4478 } 4479 4480 public boolean hasFactor() { 4481 return this.factor != null && !this.factor.isEmpty(); 4482 } 4483 4484 /** 4485 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4486 */ 4487 public ItemComponent setFactorElement(DecimalType value) { 4488 this.factor = value; 4489 return this; 4490 } 4491 4492 /** 4493 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4494 */ 4495 public BigDecimal getFactor() { 4496 return this.factor == null ? null : this.factor.getValue(); 4497 } 4498 4499 /** 4500 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4501 */ 4502 public ItemComponent setFactor(BigDecimal value) { 4503 if (value == null) 4504 this.factor = null; 4505 else { 4506 if (this.factor == null) 4507 this.factor = new DecimalType(); 4508 this.factor.setValue(value); 4509 } 4510 return this; 4511 } 4512 4513 /** 4514 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4515 */ 4516 public ItemComponent setFactor(long value) { 4517 this.factor = new DecimalType(); 4518 this.factor.setValue(value); 4519 return this; 4520 } 4521 4522 /** 4523 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4524 */ 4525 public ItemComponent setFactor(double value) { 4526 this.factor = new DecimalType(); 4527 this.factor.setValue(value); 4528 return this; 4529 } 4530 4531 /** 4532 * @return {@link #tax} (The total of taxes applicable for this product or service.) 4533 */ 4534 public Money getTax() { 4535 if (this.tax == null) 4536 if (Configuration.errorOnAutoCreate()) 4537 throw new Error("Attempt to auto-create ItemComponent.tax"); 4538 else if (Configuration.doAutoCreate()) 4539 this.tax = new Money(); // cc 4540 return this.tax; 4541 } 4542 4543 public boolean hasTax() { 4544 return this.tax != null && !this.tax.isEmpty(); 4545 } 4546 4547 /** 4548 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 4549 */ 4550 public ItemComponent setTax(Money value) { 4551 this.tax = value; 4552 return this; 4553 } 4554 4555 /** 4556 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4557 */ 4558 public Money getNet() { 4559 if (this.net == null) 4560 if (Configuration.errorOnAutoCreate()) 4561 throw new Error("Attempt to auto-create ItemComponent.net"); 4562 else if (Configuration.doAutoCreate()) 4563 this.net = new Money(); // cc 4564 return this.net; 4565 } 4566 4567 public boolean hasNet() { 4568 return this.net != null && !this.net.isEmpty(); 4569 } 4570 4571 /** 4572 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4573 */ 4574 public ItemComponent setNet(Money value) { 4575 this.net = value; 4576 return this; 4577 } 4578 4579 /** 4580 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 4581 */ 4582 public List<Reference> getUdi() { 4583 if (this.udi == null) 4584 this.udi = new ArrayList<Reference>(); 4585 return this.udi; 4586 } 4587 4588 /** 4589 * @return Returns a reference to <code>this</code> for easy method chaining 4590 */ 4591 public ItemComponent setUdi(List<Reference> theUdi) { 4592 this.udi = theUdi; 4593 return this; 4594 } 4595 4596 public boolean hasUdi() { 4597 if (this.udi == null) 4598 return false; 4599 for (Reference item : this.udi) 4600 if (!item.isEmpty()) 4601 return true; 4602 return false; 4603 } 4604 4605 public Reference addUdi() { //3 4606 Reference t = new Reference(); 4607 if (this.udi == null) 4608 this.udi = new ArrayList<Reference>(); 4609 this.udi.add(t); 4610 return t; 4611 } 4612 4613 public ItemComponent addUdi(Reference t) { //3 4614 if (t == null) 4615 return this; 4616 if (this.udi == null) 4617 this.udi = new ArrayList<Reference>(); 4618 this.udi.add(t); 4619 return this; 4620 } 4621 4622 /** 4623 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 4624 */ 4625 public Reference getUdiFirstRep() { 4626 if (getUdi().isEmpty()) { 4627 addUdi(); 4628 } 4629 return getUdi().get(0); 4630 } 4631 4632 /** 4633 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 4634 */ 4635 public List<BodySiteComponent> getBodySite() { 4636 if (this.bodySite == null) 4637 this.bodySite = new ArrayList<BodySiteComponent>(); 4638 return this.bodySite; 4639 } 4640 4641 /** 4642 * @return Returns a reference to <code>this</code> for easy method chaining 4643 */ 4644 public ItemComponent setBodySite(List<BodySiteComponent> theBodySite) { 4645 this.bodySite = theBodySite; 4646 return this; 4647 } 4648 4649 public boolean hasBodySite() { 4650 if (this.bodySite == null) 4651 return false; 4652 for (BodySiteComponent item : this.bodySite) 4653 if (!item.isEmpty()) 4654 return true; 4655 return false; 4656 } 4657 4658 public BodySiteComponent addBodySite() { //3 4659 BodySiteComponent t = new BodySiteComponent(); 4660 if (this.bodySite == null) 4661 this.bodySite = new ArrayList<BodySiteComponent>(); 4662 this.bodySite.add(t); 4663 return t; 4664 } 4665 4666 public ItemComponent addBodySite(BodySiteComponent t) { //3 4667 if (t == null) 4668 return this; 4669 if (this.bodySite == null) 4670 this.bodySite = new ArrayList<BodySiteComponent>(); 4671 this.bodySite.add(t); 4672 return this; 4673 } 4674 4675 /** 4676 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 4677 */ 4678 public BodySiteComponent getBodySiteFirstRep() { 4679 if (getBodySite().isEmpty()) { 4680 addBodySite(); 4681 } 4682 return getBodySite().get(0); 4683 } 4684 4685 /** 4686 * @return {@link #encounter} (Healthcare encounters related to this claim.) 4687 */ 4688 public List<Reference> getEncounter() { 4689 if (this.encounter == null) 4690 this.encounter = new ArrayList<Reference>(); 4691 return this.encounter; 4692 } 4693 4694 /** 4695 * @return Returns a reference to <code>this</code> for easy method chaining 4696 */ 4697 public ItemComponent setEncounter(List<Reference> theEncounter) { 4698 this.encounter = theEncounter; 4699 return this; 4700 } 4701 4702 public boolean hasEncounter() { 4703 if (this.encounter == null) 4704 return false; 4705 for (Reference item : this.encounter) 4706 if (!item.isEmpty()) 4707 return true; 4708 return false; 4709 } 4710 4711 public Reference addEncounter() { //3 4712 Reference t = new Reference(); 4713 if (this.encounter == null) 4714 this.encounter = new ArrayList<Reference>(); 4715 this.encounter.add(t); 4716 return t; 4717 } 4718 4719 public ItemComponent addEncounter(Reference t) { //3 4720 if (t == null) 4721 return this; 4722 if (this.encounter == null) 4723 this.encounter = new ArrayList<Reference>(); 4724 this.encounter.add(t); 4725 return this; 4726 } 4727 4728 /** 4729 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 4730 */ 4731 public Reference getEncounterFirstRep() { 4732 if (getEncounter().isEmpty()) { 4733 addEncounter(); 4734 } 4735 return getEncounter().get(0); 4736 } 4737 4738 /** 4739 * @return {@link #detail} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.) 4740 */ 4741 public List<DetailComponent> getDetail() { 4742 if (this.detail == null) 4743 this.detail = new ArrayList<DetailComponent>(); 4744 return this.detail; 4745 } 4746 4747 /** 4748 * @return Returns a reference to <code>this</code> for easy method chaining 4749 */ 4750 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4751 this.detail = theDetail; 4752 return this; 4753 } 4754 4755 public boolean hasDetail() { 4756 if (this.detail == null) 4757 return false; 4758 for (DetailComponent item : this.detail) 4759 if (!item.isEmpty()) 4760 return true; 4761 return false; 4762 } 4763 4764 public DetailComponent addDetail() { //3 4765 DetailComponent t = new DetailComponent(); 4766 if (this.detail == null) 4767 this.detail = new ArrayList<DetailComponent>(); 4768 this.detail.add(t); 4769 return t; 4770 } 4771 4772 public ItemComponent addDetail(DetailComponent t) { //3 4773 if (t == null) 4774 return this; 4775 if (this.detail == null) 4776 this.detail = new ArrayList<DetailComponent>(); 4777 this.detail.add(t); 4778 return this; 4779 } 4780 4781 /** 4782 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 4783 */ 4784 public DetailComponent getDetailFirstRep() { 4785 if (getDetail().isEmpty()) { 4786 addDetail(); 4787 } 4788 return getDetail().get(0); 4789 } 4790 4791 protected void listChildren(List<Property> children) { 4792 super.listChildren(children); 4793 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 4794 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 4795 children.add(new Property("careTeamSequence", "positiveInt", "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 4796 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnosis applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 4797 children.add(new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 4798 children.add(new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence)); 4799 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 4800 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 4801 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 4802 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 4803 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 4804 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4805 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4806 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 4807 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 4808 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 4809 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4810 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 4811 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4812 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 4813 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net)); 4814 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4815 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 4816 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4817 children.add(new Property("detail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, detail)); 4818 } 4819 4820 @Override 4821 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4822 switch (_hash) { 4823 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 4824 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 4825 case 1070083823: /*careTeamSequence*/ return new Property("careTeamSequence", "positiveInt", "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 4826 case -909769262: /*diagnosisSequence*/ return new Property("diagnosisSequence", "positiveInt", "Diagnosis applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 4827 case -808920140: /*procedureSequence*/ return new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 4828 case -702585587: /*informationSequence*/ return new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence); 4829 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 4830 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 4831 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 4832 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 4833 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 4834 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 4835 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 4836 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4837 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4838 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4839 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4840 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4841 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4842 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 4843 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 4844 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4845 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 4846 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4847 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 4848 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4849 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 4850 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net); 4851 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4852 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 4853 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 4854 case -1335224239: /*detail*/ return new Property("detail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, detail); 4855 default: return super.getNamedProperty(_hash, _name, _checkValid); 4856 } 4857 4858 } 4859 4860 @Override 4861 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4862 switch (hash) { 4863 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4864 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 4865 case 1070083823: /*careTeamSequence*/ return this.careTeamSequence == null ? new Base[0] : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 4866 case -909769262: /*diagnosisSequence*/ return this.diagnosisSequence == null ? new Base[0] : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 4867 case -808920140: /*procedureSequence*/ return this.procedureSequence == null ? new Base[0] : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 4868 case -702585587: /*informationSequence*/ return this.informationSequence == null ? new Base[0] : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 4869 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 4870 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4871 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 4872 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 4873 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 4874 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4875 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 4876 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 4877 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 4878 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 4879 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4880 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 4881 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 4882 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 4883 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 4884 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 4885 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // BodySiteComponent 4886 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 4887 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 4888 default: return super.getProperty(hash, name, checkValid); 4889 } 4890 4891 } 4892 4893 @Override 4894 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4895 switch (hash) { 4896 case 1349547969: // sequence 4897 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4898 return value; 4899 case 82505966: // traceNumber 4900 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 4901 return value; 4902 case 1070083823: // careTeamSequence 4903 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4904 return value; 4905 case -909769262: // diagnosisSequence 4906 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4907 return value; 4908 case -808920140: // procedureSequence 4909 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4910 return value; 4911 case -702585587: // informationSequence 4912 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4913 return value; 4914 case 1099842588: // revenue 4915 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4916 return value; 4917 case 50511102: // category 4918 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4919 return value; 4920 case 1957227299: // productOrService 4921 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4922 return value; 4923 case -717476168: // productOrServiceEnd 4924 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4925 return value; 4926 case 1095692943: // request 4927 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 4928 return value; 4929 case -615513385: // modifier 4930 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4931 return value; 4932 case 1010065041: // programCode 4933 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4934 return value; 4935 case 1379209295: // serviced 4936 this.serviced = TypeConvertor.castToType(value); // DataType 4937 return value; 4938 case 1901043637: // location 4939 this.location = TypeConvertor.castToType(value); // DataType 4940 return value; 4941 case 525514609: // patientPaid 4942 this.patientPaid = TypeConvertor.castToMoney(value); // Money 4943 return value; 4944 case -1285004149: // quantity 4945 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4946 return value; 4947 case -486196699: // unitPrice 4948 this.unitPrice = TypeConvertor.castToMoney(value); // Money 4949 return value; 4950 case -1282148017: // factor 4951 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 4952 return value; 4953 case 114603: // tax 4954 this.tax = TypeConvertor.castToMoney(value); // Money 4955 return value; 4956 case 108957: // net 4957 this.net = TypeConvertor.castToMoney(value); // Money 4958 return value; 4959 case 115642: // udi 4960 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 4961 return value; 4962 case 1702620169: // bodySite 4963 this.getBodySite().add((BodySiteComponent) value); // BodySiteComponent 4964 return value; 4965 case 1524132147: // encounter 4966 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 4967 return value; 4968 case -1335224239: // detail 4969 this.getDetail().add((DetailComponent) value); // DetailComponent 4970 return value; 4971 default: return super.setProperty(hash, name, value); 4972 } 4973 4974 } 4975 4976 @Override 4977 public Base setProperty(String name, Base value) throws FHIRException { 4978 if (name.equals("sequence")) { 4979 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4980 } else if (name.equals("traceNumber")) { 4981 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 4982 } else if (name.equals("careTeamSequence")) { 4983 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); 4984 } else if (name.equals("diagnosisSequence")) { 4985 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); 4986 } else if (name.equals("procedureSequence")) { 4987 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); 4988 } else if (name.equals("informationSequence")) { 4989 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); 4990 } else if (name.equals("revenue")) { 4991 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4992 } else if (name.equals("category")) { 4993 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4994 } else if (name.equals("productOrService")) { 4995 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4996 } else if (name.equals("productOrServiceEnd")) { 4997 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4998 } else if (name.equals("request")) { 4999 this.getRequest().add(TypeConvertor.castToReference(value)); 5000 } else if (name.equals("modifier")) { 5001 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 5002 } else if (name.equals("programCode")) { 5003 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 5004 } else if (name.equals("serviced[x]")) { 5005 this.serviced = TypeConvertor.castToType(value); // DataType 5006 } else if (name.equals("location[x]")) { 5007 this.location = TypeConvertor.castToType(value); // DataType 5008 } else if (name.equals("patientPaid")) { 5009 this.patientPaid = TypeConvertor.castToMoney(value); // Money 5010 } else if (name.equals("quantity")) { 5011 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5012 } else if (name.equals("unitPrice")) { 5013 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5014 } else if (name.equals("factor")) { 5015 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5016 } else if (name.equals("tax")) { 5017 this.tax = TypeConvertor.castToMoney(value); // Money 5018 } else if (name.equals("net")) { 5019 this.net = TypeConvertor.castToMoney(value); // Money 5020 } else if (name.equals("udi")) { 5021 this.getUdi().add(TypeConvertor.castToReference(value)); 5022 } else if (name.equals("bodySite")) { 5023 this.getBodySite().add((BodySiteComponent) value); 5024 } else if (name.equals("encounter")) { 5025 this.getEncounter().add(TypeConvertor.castToReference(value)); 5026 } else if (name.equals("detail")) { 5027 this.getDetail().add((DetailComponent) value); 5028 } else 5029 return super.setProperty(name, value); 5030 return value; 5031 } 5032 5033 @Override 5034 public Base makeProperty(int hash, String name) throws FHIRException { 5035 switch (hash) { 5036 case 1349547969: return getSequenceElement(); 5037 case 82505966: return addTraceNumber(); 5038 case 1070083823: return addCareTeamSequenceElement(); 5039 case -909769262: return addDiagnosisSequenceElement(); 5040 case -808920140: return addProcedureSequenceElement(); 5041 case -702585587: return addInformationSequenceElement(); 5042 case 1099842588: return getRevenue(); 5043 case 50511102: return getCategory(); 5044 case 1957227299: return getProductOrService(); 5045 case -717476168: return getProductOrServiceEnd(); 5046 case 1095692943: return addRequest(); 5047 case -615513385: return addModifier(); 5048 case 1010065041: return addProgramCode(); 5049 case -1927922223: return getServiced(); 5050 case 1379209295: return getServiced(); 5051 case 552316075: return getLocation(); 5052 case 1901043637: return getLocation(); 5053 case 525514609: return getPatientPaid(); 5054 case -1285004149: return getQuantity(); 5055 case -486196699: return getUnitPrice(); 5056 case -1282148017: return getFactorElement(); 5057 case 114603: return getTax(); 5058 case 108957: return getNet(); 5059 case 115642: return addUdi(); 5060 case 1702620169: return addBodySite(); 5061 case 1524132147: return addEncounter(); 5062 case -1335224239: return addDetail(); 5063 default: return super.makeProperty(hash, name); 5064 } 5065 5066 } 5067 5068 @Override 5069 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5070 switch (hash) { 5071 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 5072 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 5073 case 1070083823: /*careTeamSequence*/ return new String[] {"positiveInt"}; 5074 case -909769262: /*diagnosisSequence*/ return new String[] {"positiveInt"}; 5075 case -808920140: /*procedureSequence*/ return new String[] {"positiveInt"}; 5076 case -702585587: /*informationSequence*/ return new String[] {"positiveInt"}; 5077 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5078 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5079 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 5080 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 5081 case 1095692943: /*request*/ return new String[] {"Reference"}; 5082 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5083 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 5084 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 5085 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 5086 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 5087 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 5088 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5089 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5090 case 114603: /*tax*/ return new String[] {"Money"}; 5091 case 108957: /*net*/ return new String[] {"Money"}; 5092 case 115642: /*udi*/ return new String[] {"Reference"}; 5093 case 1702620169: /*bodySite*/ return new String[] {}; 5094 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5095 case -1335224239: /*detail*/ return new String[] {}; 5096 default: return super.getTypesForProperty(hash, name); 5097 } 5098 5099 } 5100 5101 @Override 5102 public Base addChild(String name) throws FHIRException { 5103 if (name.equals("sequence")) { 5104 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.sequence"); 5105 } 5106 else if (name.equals("traceNumber")) { 5107 return addTraceNumber(); 5108 } 5109 else if (name.equals("careTeamSequence")) { 5110 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.careTeamSequence"); 5111 } 5112 else if (name.equals("diagnosisSequence")) { 5113 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.diagnosisSequence"); 5114 } 5115 else if (name.equals("procedureSequence")) { 5116 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.procedureSequence"); 5117 } 5118 else if (name.equals("informationSequence")) { 5119 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.informationSequence"); 5120 } 5121 else if (name.equals("revenue")) { 5122 this.revenue = new CodeableConcept(); 5123 return this.revenue; 5124 } 5125 else if (name.equals("category")) { 5126 this.category = new CodeableConcept(); 5127 return this.category; 5128 } 5129 else if (name.equals("productOrService")) { 5130 this.productOrService = new CodeableConcept(); 5131 return this.productOrService; 5132 } 5133 else if (name.equals("productOrServiceEnd")) { 5134 this.productOrServiceEnd = new CodeableConcept(); 5135 return this.productOrServiceEnd; 5136 } 5137 else if (name.equals("request")) { 5138 return addRequest(); 5139 } 5140 else if (name.equals("modifier")) { 5141 return addModifier(); 5142 } 5143 else if (name.equals("programCode")) { 5144 return addProgramCode(); 5145 } 5146 else if (name.equals("servicedDate")) { 5147 this.serviced = new DateType(); 5148 return this.serviced; 5149 } 5150 else if (name.equals("servicedPeriod")) { 5151 this.serviced = new Period(); 5152 return this.serviced; 5153 } 5154 else if (name.equals("locationCodeableConcept")) { 5155 this.location = new CodeableConcept(); 5156 return this.location; 5157 } 5158 else if (name.equals("locationAddress")) { 5159 this.location = new Address(); 5160 return this.location; 5161 } 5162 else if (name.equals("locationReference")) { 5163 this.location = new Reference(); 5164 return this.location; 5165 } 5166 else if (name.equals("patientPaid")) { 5167 this.patientPaid = new Money(); 5168 return this.patientPaid; 5169 } 5170 else if (name.equals("quantity")) { 5171 this.quantity = new Quantity(); 5172 return this.quantity; 5173 } 5174 else if (name.equals("unitPrice")) { 5175 this.unitPrice = new Money(); 5176 return this.unitPrice; 5177 } 5178 else if (name.equals("factor")) { 5179 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.factor"); 5180 } 5181 else if (name.equals("tax")) { 5182 this.tax = new Money(); 5183 return this.tax; 5184 } 5185 else if (name.equals("net")) { 5186 this.net = new Money(); 5187 return this.net; 5188 } 5189 else if (name.equals("udi")) { 5190 return addUdi(); 5191 } 5192 else if (name.equals("bodySite")) { 5193 return addBodySite(); 5194 } 5195 else if (name.equals("encounter")) { 5196 return addEncounter(); 5197 } 5198 else if (name.equals("detail")) { 5199 return addDetail(); 5200 } 5201 else 5202 return super.addChild(name); 5203 } 5204 5205 public ItemComponent copy() { 5206 ItemComponent dst = new ItemComponent(); 5207 copyValues(dst); 5208 return dst; 5209 } 5210 5211 public void copyValues(ItemComponent dst) { 5212 super.copyValues(dst); 5213 dst.sequence = sequence == null ? null : sequence.copy(); 5214 if (traceNumber != null) { 5215 dst.traceNumber = new ArrayList<Identifier>(); 5216 for (Identifier i : traceNumber) 5217 dst.traceNumber.add(i.copy()); 5218 }; 5219 if (careTeamSequence != null) { 5220 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 5221 for (PositiveIntType i : careTeamSequence) 5222 dst.careTeamSequence.add(i.copy()); 5223 }; 5224 if (diagnosisSequence != null) { 5225 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 5226 for (PositiveIntType i : diagnosisSequence) 5227 dst.diagnosisSequence.add(i.copy()); 5228 }; 5229 if (procedureSequence != null) { 5230 dst.procedureSequence = new ArrayList<PositiveIntType>(); 5231 for (PositiveIntType i : procedureSequence) 5232 dst.procedureSequence.add(i.copy()); 5233 }; 5234 if (informationSequence != null) { 5235 dst.informationSequence = new ArrayList<PositiveIntType>(); 5236 for (PositiveIntType i : informationSequence) 5237 dst.informationSequence.add(i.copy()); 5238 }; 5239 dst.revenue = revenue == null ? null : revenue.copy(); 5240 dst.category = category == null ? null : category.copy(); 5241 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5242 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 5243 if (request != null) { 5244 dst.request = new ArrayList<Reference>(); 5245 for (Reference i : request) 5246 dst.request.add(i.copy()); 5247 }; 5248 if (modifier != null) { 5249 dst.modifier = new ArrayList<CodeableConcept>(); 5250 for (CodeableConcept i : modifier) 5251 dst.modifier.add(i.copy()); 5252 }; 5253 if (programCode != null) { 5254 dst.programCode = new ArrayList<CodeableConcept>(); 5255 for (CodeableConcept i : programCode) 5256 dst.programCode.add(i.copy()); 5257 }; 5258 dst.serviced = serviced == null ? null : serviced.copy(); 5259 dst.location = location == null ? null : location.copy(); 5260 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 5261 dst.quantity = quantity == null ? null : quantity.copy(); 5262 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5263 dst.factor = factor == null ? null : factor.copy(); 5264 dst.tax = tax == null ? null : tax.copy(); 5265 dst.net = net == null ? null : net.copy(); 5266 if (udi != null) { 5267 dst.udi = new ArrayList<Reference>(); 5268 for (Reference i : udi) 5269 dst.udi.add(i.copy()); 5270 }; 5271 if (bodySite != null) { 5272 dst.bodySite = new ArrayList<BodySiteComponent>(); 5273 for (BodySiteComponent i : bodySite) 5274 dst.bodySite.add(i.copy()); 5275 }; 5276 if (encounter != null) { 5277 dst.encounter = new ArrayList<Reference>(); 5278 for (Reference i : encounter) 5279 dst.encounter.add(i.copy()); 5280 }; 5281 if (detail != null) { 5282 dst.detail = new ArrayList<DetailComponent>(); 5283 for (DetailComponent i : detail) 5284 dst.detail.add(i.copy()); 5285 }; 5286 } 5287 5288 @Override 5289 public boolean equalsDeep(Base other_) { 5290 if (!super.equalsDeep(other_)) 5291 return false; 5292 if (!(other_ instanceof ItemComponent)) 5293 return false; 5294 ItemComponent o = (ItemComponent) other_; 5295 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 5296 && compareDeep(careTeamSequence, o.careTeamSequence, true) && compareDeep(diagnosisSequence, o.diagnosisSequence, true) 5297 && compareDeep(procedureSequence, o.procedureSequence, true) && compareDeep(informationSequence, o.informationSequence, true) 5298 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 5299 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 5300 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 5301 && compareDeep(location, o.location, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) 5302 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) 5303 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 5304 && compareDeep(encounter, o.encounter, true) && compareDeep(detail, o.detail, true); 5305 } 5306 5307 @Override 5308 public boolean equalsShallow(Base other_) { 5309 if (!super.equalsShallow(other_)) 5310 return false; 5311 if (!(other_ instanceof ItemComponent)) 5312 return false; 5313 ItemComponent o = (ItemComponent) other_; 5314 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 5315 && compareValues(diagnosisSequence, o.diagnosisSequence, true) && compareValues(procedureSequence, o.procedureSequence, true) 5316 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true) 5317 ; 5318 } 5319 5320 public boolean isEmpty() { 5321 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, careTeamSequence 5322 , diagnosisSequence, procedureSequence, informationSequence, revenue, category, productOrService 5323 , productOrServiceEnd, request, modifier, programCode, serviced, location, patientPaid 5324 , quantity, unitPrice, factor, tax, net, udi, bodySite, encounter, detail 5325 ); 5326 } 5327 5328 public String fhirType() { 5329 return "Claim.item"; 5330 5331 } 5332 5333 } 5334 5335 @Block() 5336 public static class BodySiteComponent extends BackboneElement implements IBaseBackboneElement { 5337 /** 5338 * Physical service site on the patient (limb, tooth, etc.). 5339 */ 5340 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5341 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 5342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 5343 protected List<CodeableReference> site; 5344 5345 /** 5346 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 5347 */ 5348 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5349 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 5350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 5351 protected List<CodeableConcept> subSite; 5352 5353 private static final long serialVersionUID = 1190632415L; 5354 5355 /** 5356 * Constructor 5357 */ 5358 public BodySiteComponent() { 5359 super(); 5360 } 5361 5362 /** 5363 * Constructor 5364 */ 5365 public BodySiteComponent(CodeableReference site) { 5366 super(); 5367 this.addSite(site); 5368 } 5369 5370 /** 5371 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 5372 */ 5373 public List<CodeableReference> getSite() { 5374 if (this.site == null) 5375 this.site = new ArrayList<CodeableReference>(); 5376 return this.site; 5377 } 5378 5379 /** 5380 * @return Returns a reference to <code>this</code> for easy method chaining 5381 */ 5382 public BodySiteComponent setSite(List<CodeableReference> theSite) { 5383 this.site = theSite; 5384 return this; 5385 } 5386 5387 public boolean hasSite() { 5388 if (this.site == null) 5389 return false; 5390 for (CodeableReference item : this.site) 5391 if (!item.isEmpty()) 5392 return true; 5393 return false; 5394 } 5395 5396 public CodeableReference addSite() { //3 5397 CodeableReference t = new CodeableReference(); 5398 if (this.site == null) 5399 this.site = new ArrayList<CodeableReference>(); 5400 this.site.add(t); 5401 return t; 5402 } 5403 5404 public BodySiteComponent addSite(CodeableReference t) { //3 5405 if (t == null) 5406 return this; 5407 if (this.site == null) 5408 this.site = new ArrayList<CodeableReference>(); 5409 this.site.add(t); 5410 return this; 5411 } 5412 5413 /** 5414 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 5415 */ 5416 public CodeableReference getSiteFirstRep() { 5417 if (getSite().isEmpty()) { 5418 addSite(); 5419 } 5420 return getSite().get(0); 5421 } 5422 5423 /** 5424 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 5425 */ 5426 public List<CodeableConcept> getSubSite() { 5427 if (this.subSite == null) 5428 this.subSite = new ArrayList<CodeableConcept>(); 5429 return this.subSite; 5430 } 5431 5432 /** 5433 * @return Returns a reference to <code>this</code> for easy method chaining 5434 */ 5435 public BodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 5436 this.subSite = theSubSite; 5437 return this; 5438 } 5439 5440 public boolean hasSubSite() { 5441 if (this.subSite == null) 5442 return false; 5443 for (CodeableConcept item : this.subSite) 5444 if (!item.isEmpty()) 5445 return true; 5446 return false; 5447 } 5448 5449 public CodeableConcept addSubSite() { //3 5450 CodeableConcept t = new CodeableConcept(); 5451 if (this.subSite == null) 5452 this.subSite = new ArrayList<CodeableConcept>(); 5453 this.subSite.add(t); 5454 return t; 5455 } 5456 5457 public BodySiteComponent addSubSite(CodeableConcept t) { //3 5458 if (t == null) 5459 return this; 5460 if (this.subSite == null) 5461 this.subSite = new ArrayList<CodeableConcept>(); 5462 this.subSite.add(t); 5463 return this; 5464 } 5465 5466 /** 5467 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 5468 */ 5469 public CodeableConcept getSubSiteFirstRep() { 5470 if (getSubSite().isEmpty()) { 5471 addSubSite(); 5472 } 5473 return getSubSite().get(0); 5474 } 5475 5476 protected void listChildren(List<Property> children) { 5477 super.listChildren(children); 5478 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 5479 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 5480 } 5481 5482 @Override 5483 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5484 switch (_hash) { 5485 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 5486 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 5487 default: return super.getNamedProperty(_hash, _name, _checkValid); 5488 } 5489 5490 } 5491 5492 @Override 5493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5494 switch (hash) { 5495 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 5496 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5497 default: return super.getProperty(hash, name, checkValid); 5498 } 5499 5500 } 5501 5502 @Override 5503 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5504 switch (hash) { 5505 case 3530567: // site 5506 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5507 return value; 5508 case -1868566105: // subSite 5509 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5510 return value; 5511 default: return super.setProperty(hash, name, value); 5512 } 5513 5514 } 5515 5516 @Override 5517 public Base setProperty(String name, Base value) throws FHIRException { 5518 if (name.equals("site")) { 5519 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 5520 } else if (name.equals("subSite")) { 5521 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 5522 } else 5523 return super.setProperty(name, value); 5524 return value; 5525 } 5526 5527 @Override 5528 public Base makeProperty(int hash, String name) throws FHIRException { 5529 switch (hash) { 5530 case 3530567: return addSite(); 5531 case -1868566105: return addSubSite(); 5532 default: return super.makeProperty(hash, name); 5533 } 5534 5535 } 5536 5537 @Override 5538 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5539 switch (hash) { 5540 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 5541 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 5542 default: return super.getTypesForProperty(hash, name); 5543 } 5544 5545 } 5546 5547 @Override 5548 public Base addChild(String name) throws FHIRException { 5549 if (name.equals("site")) { 5550 return addSite(); 5551 } 5552 else if (name.equals("subSite")) { 5553 return addSubSite(); 5554 } 5555 else 5556 return super.addChild(name); 5557 } 5558 5559 public BodySiteComponent copy() { 5560 BodySiteComponent dst = new BodySiteComponent(); 5561 copyValues(dst); 5562 return dst; 5563 } 5564 5565 public void copyValues(BodySiteComponent dst) { 5566 super.copyValues(dst); 5567 if (site != null) { 5568 dst.site = new ArrayList<CodeableReference>(); 5569 for (CodeableReference i : site) 5570 dst.site.add(i.copy()); 5571 }; 5572 if (subSite != null) { 5573 dst.subSite = new ArrayList<CodeableConcept>(); 5574 for (CodeableConcept i : subSite) 5575 dst.subSite.add(i.copy()); 5576 }; 5577 } 5578 5579 @Override 5580 public boolean equalsDeep(Base other_) { 5581 if (!super.equalsDeep(other_)) 5582 return false; 5583 if (!(other_ instanceof BodySiteComponent)) 5584 return false; 5585 BodySiteComponent o = (BodySiteComponent) other_; 5586 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 5587 } 5588 5589 @Override 5590 public boolean equalsShallow(Base other_) { 5591 if (!super.equalsShallow(other_)) 5592 return false; 5593 if (!(other_ instanceof BodySiteComponent)) 5594 return false; 5595 BodySiteComponent o = (BodySiteComponent) other_; 5596 return true; 5597 } 5598 5599 public boolean isEmpty() { 5600 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 5601 } 5602 5603 public String fhirType() { 5604 return "Claim.item.bodySite"; 5605 5606 } 5607 5608 } 5609 5610 @Block() 5611 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 5612 /** 5613 * A number to uniquely identify item entries. 5614 */ 5615 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5616 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 5617 protected PositiveIntType sequence; 5618 5619 /** 5620 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 5621 */ 5622 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5623 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 5624 protected List<Identifier> traceNumber; 5625 5626 /** 5627 * The type of revenue or cost center providing the product and/or service. 5628 */ 5629 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5630 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 5631 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5632 protected CodeableConcept revenue; 5633 5634 /** 5635 * Code to identify the general type of benefits under which products and services are provided. 5636 */ 5637 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5638 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 5639 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 5640 protected CodeableConcept category; 5641 5642 /** 5643 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 5644 */ 5645 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 5646 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 5647 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5648 protected CodeableConcept productOrService; 5649 5650 /** 5651 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 5652 */ 5653 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 5654 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 5655 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5656 protected CodeableConcept productOrServiceEnd; 5657 5658 /** 5659 * Item typification or modifiers codes to convey additional context for the product or service. 5660 */ 5661 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5662 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 5663 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5664 protected List<CodeableConcept> modifier; 5665 5666 /** 5667 * Identifies the program under which this may be recovered. 5668 */ 5669 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5670 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 5671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5672 protected List<CodeableConcept> programCode; 5673 5674 /** 5675 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 5676 */ 5677 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 5678 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 5679 protected Money patientPaid; 5680 5681 /** 5682 * The number of repetitions of a service or product. 5683 */ 5684 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 5685 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 5686 protected Quantity quantity; 5687 5688 /** 5689 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 5690 */ 5691 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 5692 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 5693 protected Money unitPrice; 5694 5695 /** 5696 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5697 */ 5698 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 5699 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5700 protected DecimalType factor; 5701 5702 /** 5703 * The total of taxes applicable for this product or service. 5704 */ 5705 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 5706 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 5707 protected Money tax; 5708 5709 /** 5710 * The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor. 5711 */ 5712 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 5713 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor." ) 5714 protected Money net; 5715 5716 /** 5717 * Unique Device Identifiers associated with this line item. 5718 */ 5719 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5720 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 5721 protected List<Reference> udi; 5722 5723 /** 5724 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 5725 */ 5726 @Child(name = "subDetail", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5727 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 5728 protected List<SubDetailComponent> subDetail; 5729 5730 private static final long serialVersionUID = -733030497L; 5731 5732 /** 5733 * Constructor 5734 */ 5735 public DetailComponent() { 5736 super(); 5737 } 5738 5739 /** 5740 * Constructor 5741 */ 5742 public DetailComponent(int sequence) { 5743 super(); 5744 this.setSequence(sequence); 5745 } 5746 5747 /** 5748 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5749 */ 5750 public PositiveIntType getSequenceElement() { 5751 if (this.sequence == null) 5752 if (Configuration.errorOnAutoCreate()) 5753 throw new Error("Attempt to auto-create DetailComponent.sequence"); 5754 else if (Configuration.doAutoCreate()) 5755 this.sequence = new PositiveIntType(); // bb 5756 return this.sequence; 5757 } 5758 5759 public boolean hasSequenceElement() { 5760 return this.sequence != null && !this.sequence.isEmpty(); 5761 } 5762 5763 public boolean hasSequence() { 5764 return this.sequence != null && !this.sequence.isEmpty(); 5765 } 5766 5767 /** 5768 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5769 */ 5770 public DetailComponent setSequenceElement(PositiveIntType value) { 5771 this.sequence = value; 5772 return this; 5773 } 5774 5775 /** 5776 * @return A number to uniquely identify item entries. 5777 */ 5778 public int getSequence() { 5779 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 5780 } 5781 5782 /** 5783 * @param value A number to uniquely identify item entries. 5784 */ 5785 public DetailComponent setSequence(int value) { 5786 if (this.sequence == null) 5787 this.sequence = new PositiveIntType(); 5788 this.sequence.setValue(value); 5789 return this; 5790 } 5791 5792 /** 5793 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 5794 */ 5795 public List<Identifier> getTraceNumber() { 5796 if (this.traceNumber == null) 5797 this.traceNumber = new ArrayList<Identifier>(); 5798 return this.traceNumber; 5799 } 5800 5801 /** 5802 * @return Returns a reference to <code>this</code> for easy method chaining 5803 */ 5804 public DetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 5805 this.traceNumber = theTraceNumber; 5806 return this; 5807 } 5808 5809 public boolean hasTraceNumber() { 5810 if (this.traceNumber == null) 5811 return false; 5812 for (Identifier item : this.traceNumber) 5813 if (!item.isEmpty()) 5814 return true; 5815 return false; 5816 } 5817 5818 public Identifier addTraceNumber() { //3 5819 Identifier t = new Identifier(); 5820 if (this.traceNumber == null) 5821 this.traceNumber = new ArrayList<Identifier>(); 5822 this.traceNumber.add(t); 5823 return t; 5824 } 5825 5826 public DetailComponent addTraceNumber(Identifier t) { //3 5827 if (t == null) 5828 return this; 5829 if (this.traceNumber == null) 5830 this.traceNumber = new ArrayList<Identifier>(); 5831 this.traceNumber.add(t); 5832 return this; 5833 } 5834 5835 /** 5836 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 5837 */ 5838 public Identifier getTraceNumberFirstRep() { 5839 if (getTraceNumber().isEmpty()) { 5840 addTraceNumber(); 5841 } 5842 return getTraceNumber().get(0); 5843 } 5844 5845 /** 5846 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 5847 */ 5848 public CodeableConcept getRevenue() { 5849 if (this.revenue == null) 5850 if (Configuration.errorOnAutoCreate()) 5851 throw new Error("Attempt to auto-create DetailComponent.revenue"); 5852 else if (Configuration.doAutoCreate()) 5853 this.revenue = new CodeableConcept(); // cc 5854 return this.revenue; 5855 } 5856 5857 public boolean hasRevenue() { 5858 return this.revenue != null && !this.revenue.isEmpty(); 5859 } 5860 5861 /** 5862 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 5863 */ 5864 public DetailComponent setRevenue(CodeableConcept value) { 5865 this.revenue = value; 5866 return this; 5867 } 5868 5869 /** 5870 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 5871 */ 5872 public CodeableConcept getCategory() { 5873 if (this.category == null) 5874 if (Configuration.errorOnAutoCreate()) 5875 throw new Error("Attempt to auto-create DetailComponent.category"); 5876 else if (Configuration.doAutoCreate()) 5877 this.category = new CodeableConcept(); // cc 5878 return this.category; 5879 } 5880 5881 public boolean hasCategory() { 5882 return this.category != null && !this.category.isEmpty(); 5883 } 5884 5885 /** 5886 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 5887 */ 5888 public DetailComponent setCategory(CodeableConcept value) { 5889 this.category = value; 5890 return this; 5891 } 5892 5893 /** 5894 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 5895 */ 5896 public CodeableConcept getProductOrService() { 5897 if (this.productOrService == null) 5898 if (Configuration.errorOnAutoCreate()) 5899 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 5900 else if (Configuration.doAutoCreate()) 5901 this.productOrService = new CodeableConcept(); // cc 5902 return this.productOrService; 5903 } 5904 5905 public boolean hasProductOrService() { 5906 return this.productOrService != null && !this.productOrService.isEmpty(); 5907 } 5908 5909 /** 5910 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 5911 */ 5912 public DetailComponent setProductOrService(CodeableConcept value) { 5913 this.productOrService = value; 5914 return this; 5915 } 5916 5917 /** 5918 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 5919 */ 5920 public CodeableConcept getProductOrServiceEnd() { 5921 if (this.productOrServiceEnd == null) 5922 if (Configuration.errorOnAutoCreate()) 5923 throw new Error("Attempt to auto-create DetailComponent.productOrServiceEnd"); 5924 else if (Configuration.doAutoCreate()) 5925 this.productOrServiceEnd = new CodeableConcept(); // cc 5926 return this.productOrServiceEnd; 5927 } 5928 5929 public boolean hasProductOrServiceEnd() { 5930 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 5931 } 5932 5933 /** 5934 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 5935 */ 5936 public DetailComponent setProductOrServiceEnd(CodeableConcept value) { 5937 this.productOrServiceEnd = value; 5938 return this; 5939 } 5940 5941 /** 5942 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 5943 */ 5944 public List<CodeableConcept> getModifier() { 5945 if (this.modifier == null) 5946 this.modifier = new ArrayList<CodeableConcept>(); 5947 return this.modifier; 5948 } 5949 5950 /** 5951 * @return Returns a reference to <code>this</code> for easy method chaining 5952 */ 5953 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 5954 this.modifier = theModifier; 5955 return this; 5956 } 5957 5958 public boolean hasModifier() { 5959 if (this.modifier == null) 5960 return false; 5961 for (CodeableConcept item : this.modifier) 5962 if (!item.isEmpty()) 5963 return true; 5964 return false; 5965 } 5966 5967 public CodeableConcept addModifier() { //3 5968 CodeableConcept t = new CodeableConcept(); 5969 if (this.modifier == null) 5970 this.modifier = new ArrayList<CodeableConcept>(); 5971 this.modifier.add(t); 5972 return t; 5973 } 5974 5975 public DetailComponent addModifier(CodeableConcept t) { //3 5976 if (t == null) 5977 return this; 5978 if (this.modifier == null) 5979 this.modifier = new ArrayList<CodeableConcept>(); 5980 this.modifier.add(t); 5981 return this; 5982 } 5983 5984 /** 5985 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 5986 */ 5987 public CodeableConcept getModifierFirstRep() { 5988 if (getModifier().isEmpty()) { 5989 addModifier(); 5990 } 5991 return getModifier().get(0); 5992 } 5993 5994 /** 5995 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 5996 */ 5997 public List<CodeableConcept> getProgramCode() { 5998 if (this.programCode == null) 5999 this.programCode = new ArrayList<CodeableConcept>(); 6000 return this.programCode; 6001 } 6002 6003 /** 6004 * @return Returns a reference to <code>this</code> for easy method chaining 6005 */ 6006 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6007 this.programCode = theProgramCode; 6008 return this; 6009 } 6010 6011 public boolean hasProgramCode() { 6012 if (this.programCode == null) 6013 return false; 6014 for (CodeableConcept item : this.programCode) 6015 if (!item.isEmpty()) 6016 return true; 6017 return false; 6018 } 6019 6020 public CodeableConcept addProgramCode() { //3 6021 CodeableConcept t = new CodeableConcept(); 6022 if (this.programCode == null) 6023 this.programCode = new ArrayList<CodeableConcept>(); 6024 this.programCode.add(t); 6025 return t; 6026 } 6027 6028 public DetailComponent addProgramCode(CodeableConcept t) { //3 6029 if (t == null) 6030 return this; 6031 if (this.programCode == null) 6032 this.programCode = new ArrayList<CodeableConcept>(); 6033 this.programCode.add(t); 6034 return this; 6035 } 6036 6037 /** 6038 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 6039 */ 6040 public CodeableConcept getProgramCodeFirstRep() { 6041 if (getProgramCode().isEmpty()) { 6042 addProgramCode(); 6043 } 6044 return getProgramCode().get(0); 6045 } 6046 6047 /** 6048 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 6049 */ 6050 public Money getPatientPaid() { 6051 if (this.patientPaid == null) 6052 if (Configuration.errorOnAutoCreate()) 6053 throw new Error("Attempt to auto-create DetailComponent.patientPaid"); 6054 else if (Configuration.doAutoCreate()) 6055 this.patientPaid = new Money(); // cc 6056 return this.patientPaid; 6057 } 6058 6059 public boolean hasPatientPaid() { 6060 return this.patientPaid != null && !this.patientPaid.isEmpty(); 6061 } 6062 6063 /** 6064 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 6065 */ 6066 public DetailComponent setPatientPaid(Money value) { 6067 this.patientPaid = value; 6068 return this; 6069 } 6070 6071 /** 6072 * @return {@link #quantity} (The number of repetitions of a service or product.) 6073 */ 6074 public Quantity getQuantity() { 6075 if (this.quantity == null) 6076 if (Configuration.errorOnAutoCreate()) 6077 throw new Error("Attempt to auto-create DetailComponent.quantity"); 6078 else if (Configuration.doAutoCreate()) 6079 this.quantity = new Quantity(); // cc 6080 return this.quantity; 6081 } 6082 6083 public boolean hasQuantity() { 6084 return this.quantity != null && !this.quantity.isEmpty(); 6085 } 6086 6087 /** 6088 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6089 */ 6090 public DetailComponent setQuantity(Quantity value) { 6091 this.quantity = value; 6092 return this; 6093 } 6094 6095 /** 6096 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6097 */ 6098 public Money getUnitPrice() { 6099 if (this.unitPrice == null) 6100 if (Configuration.errorOnAutoCreate()) 6101 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 6102 else if (Configuration.doAutoCreate()) 6103 this.unitPrice = new Money(); // cc 6104 return this.unitPrice; 6105 } 6106 6107 public boolean hasUnitPrice() { 6108 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6109 } 6110 6111 /** 6112 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6113 */ 6114 public DetailComponent setUnitPrice(Money value) { 6115 this.unitPrice = value; 6116 return this; 6117 } 6118 6119 /** 6120 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6121 */ 6122 public DecimalType getFactorElement() { 6123 if (this.factor == null) 6124 if (Configuration.errorOnAutoCreate()) 6125 throw new Error("Attempt to auto-create DetailComponent.factor"); 6126 else if (Configuration.doAutoCreate()) 6127 this.factor = new DecimalType(); // bb 6128 return this.factor; 6129 } 6130 6131 public boolean hasFactorElement() { 6132 return this.factor != null && !this.factor.isEmpty(); 6133 } 6134 6135 public boolean hasFactor() { 6136 return this.factor != null && !this.factor.isEmpty(); 6137 } 6138 6139 /** 6140 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6141 */ 6142 public DetailComponent setFactorElement(DecimalType value) { 6143 this.factor = value; 6144 return this; 6145 } 6146 6147 /** 6148 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6149 */ 6150 public BigDecimal getFactor() { 6151 return this.factor == null ? null : this.factor.getValue(); 6152 } 6153 6154 /** 6155 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6156 */ 6157 public DetailComponent setFactor(BigDecimal value) { 6158 if (value == null) 6159 this.factor = null; 6160 else { 6161 if (this.factor == null) 6162 this.factor = new DecimalType(); 6163 this.factor.setValue(value); 6164 } 6165 return this; 6166 } 6167 6168 /** 6169 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6170 */ 6171 public DetailComponent setFactor(long value) { 6172 this.factor = new DecimalType(); 6173 this.factor.setValue(value); 6174 return this; 6175 } 6176 6177 /** 6178 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6179 */ 6180 public DetailComponent setFactor(double value) { 6181 this.factor = new DecimalType(); 6182 this.factor.setValue(value); 6183 return this; 6184 } 6185 6186 /** 6187 * @return {@link #tax} (The total of taxes applicable for this product or service.) 6188 */ 6189 public Money getTax() { 6190 if (this.tax == null) 6191 if (Configuration.errorOnAutoCreate()) 6192 throw new Error("Attempt to auto-create DetailComponent.tax"); 6193 else if (Configuration.doAutoCreate()) 6194 this.tax = new Money(); // cc 6195 return this.tax; 6196 } 6197 6198 public boolean hasTax() { 6199 return this.tax != null && !this.tax.isEmpty(); 6200 } 6201 6202 /** 6203 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 6204 */ 6205 public DetailComponent setTax(Money value) { 6206 this.tax = value; 6207 return this; 6208 } 6209 6210 /** 6211 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 6212 */ 6213 public Money getNet() { 6214 if (this.net == null) 6215 if (Configuration.errorOnAutoCreate()) 6216 throw new Error("Attempt to auto-create DetailComponent.net"); 6217 else if (Configuration.doAutoCreate()) 6218 this.net = new Money(); // cc 6219 return this.net; 6220 } 6221 6222 public boolean hasNet() { 6223 return this.net != null && !this.net.isEmpty(); 6224 } 6225 6226 /** 6227 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 6228 */ 6229 public DetailComponent setNet(Money value) { 6230 this.net = value; 6231 return this; 6232 } 6233 6234 /** 6235 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 6236 */ 6237 public List<Reference> getUdi() { 6238 if (this.udi == null) 6239 this.udi = new ArrayList<Reference>(); 6240 return this.udi; 6241 } 6242 6243 /** 6244 * @return Returns a reference to <code>this</code> for easy method chaining 6245 */ 6246 public DetailComponent setUdi(List<Reference> theUdi) { 6247 this.udi = theUdi; 6248 return this; 6249 } 6250 6251 public boolean hasUdi() { 6252 if (this.udi == null) 6253 return false; 6254 for (Reference item : this.udi) 6255 if (!item.isEmpty()) 6256 return true; 6257 return false; 6258 } 6259 6260 public Reference addUdi() { //3 6261 Reference t = new Reference(); 6262 if (this.udi == null) 6263 this.udi = new ArrayList<Reference>(); 6264 this.udi.add(t); 6265 return t; 6266 } 6267 6268 public DetailComponent addUdi(Reference t) { //3 6269 if (t == null) 6270 return this; 6271 if (this.udi == null) 6272 this.udi = new ArrayList<Reference>(); 6273 this.udi.add(t); 6274 return this; 6275 } 6276 6277 /** 6278 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 6279 */ 6280 public Reference getUdiFirstRep() { 6281 if (getUdi().isEmpty()) { 6282 addUdi(); 6283 } 6284 return getUdi().get(0); 6285 } 6286 6287 /** 6288 * @return {@link #subDetail} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.) 6289 */ 6290 public List<SubDetailComponent> getSubDetail() { 6291 if (this.subDetail == null) 6292 this.subDetail = new ArrayList<SubDetailComponent>(); 6293 return this.subDetail; 6294 } 6295 6296 /** 6297 * @return Returns a reference to <code>this</code> for easy method chaining 6298 */ 6299 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 6300 this.subDetail = theSubDetail; 6301 return this; 6302 } 6303 6304 public boolean hasSubDetail() { 6305 if (this.subDetail == null) 6306 return false; 6307 for (SubDetailComponent item : this.subDetail) 6308 if (!item.isEmpty()) 6309 return true; 6310 return false; 6311 } 6312 6313 public SubDetailComponent addSubDetail() { //3 6314 SubDetailComponent t = new SubDetailComponent(); 6315 if (this.subDetail == null) 6316 this.subDetail = new ArrayList<SubDetailComponent>(); 6317 this.subDetail.add(t); 6318 return t; 6319 } 6320 6321 public DetailComponent addSubDetail(SubDetailComponent t) { //3 6322 if (t == null) 6323 return this; 6324 if (this.subDetail == null) 6325 this.subDetail = new ArrayList<SubDetailComponent>(); 6326 this.subDetail.add(t); 6327 return this; 6328 } 6329 6330 /** 6331 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 6332 */ 6333 public SubDetailComponent getSubDetailFirstRep() { 6334 if (getSubDetail().isEmpty()) { 6335 addSubDetail(); 6336 } 6337 return getSubDetail().get(0); 6338 } 6339 6340 protected void listChildren(List<Property> children) { 6341 super.listChildren(children); 6342 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 6343 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 6344 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 6345 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 6346 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 6347 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 6348 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6349 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 6350 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 6351 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6352 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 6353 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6354 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 6355 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net)); 6356 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 6357 children.add(new Property("subDetail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 6358 } 6359 6360 @Override 6361 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6362 switch (_hash) { 6363 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 6364 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 6365 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 6366 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 6367 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 6368 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 6369 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 6370 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 6371 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 6372 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6373 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 6374 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6375 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 6376 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net); 6377 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 6378 case -828829007: /*subDetail*/ return new Property("subDetail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, subDetail); 6379 default: return super.getNamedProperty(_hash, _name, _checkValid); 6380 } 6381 6382 } 6383 6384 @Override 6385 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6386 switch (hash) { 6387 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 6388 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 6389 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6390 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6391 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 6392 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 6393 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6394 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 6395 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 6396 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 6397 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6398 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6399 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 6400 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6401 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 6402 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 6403 default: return super.getProperty(hash, name, checkValid); 6404 } 6405 6406 } 6407 6408 @Override 6409 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6410 switch (hash) { 6411 case 1349547969: // sequence 6412 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 6413 return value; 6414 case 82505966: // traceNumber 6415 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 6416 return value; 6417 case 1099842588: // revenue 6418 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6419 return value; 6420 case 50511102: // category 6421 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6422 return value; 6423 case 1957227299: // productOrService 6424 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6425 return value; 6426 case -717476168: // productOrServiceEnd 6427 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6428 return value; 6429 case -615513385: // modifier 6430 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6431 return value; 6432 case 1010065041: // programCode 6433 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6434 return value; 6435 case 525514609: // patientPaid 6436 this.patientPaid = TypeConvertor.castToMoney(value); // Money 6437 return value; 6438 case -1285004149: // quantity 6439 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6440 return value; 6441 case -486196699: // unitPrice 6442 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6443 return value; 6444 case -1282148017: // factor 6445 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6446 return value; 6447 case 114603: // tax 6448 this.tax = TypeConvertor.castToMoney(value); // Money 6449 return value; 6450 case 108957: // net 6451 this.net = TypeConvertor.castToMoney(value); // Money 6452 return value; 6453 case 115642: // udi 6454 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 6455 return value; 6456 case -828829007: // subDetail 6457 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 6458 return value; 6459 default: return super.setProperty(hash, name, value); 6460 } 6461 6462 } 6463 6464 @Override 6465 public Base setProperty(String name, Base value) throws FHIRException { 6466 if (name.equals("sequence")) { 6467 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 6468 } else if (name.equals("traceNumber")) { 6469 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 6470 } else if (name.equals("revenue")) { 6471 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6472 } else if (name.equals("category")) { 6473 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6474 } else if (name.equals("productOrService")) { 6475 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6476 } else if (name.equals("productOrServiceEnd")) { 6477 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6478 } else if (name.equals("modifier")) { 6479 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 6480 } else if (name.equals("programCode")) { 6481 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 6482 } else if (name.equals("patientPaid")) { 6483 this.patientPaid = TypeConvertor.castToMoney(value); // Money 6484 } else if (name.equals("quantity")) { 6485 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6486 } else if (name.equals("unitPrice")) { 6487 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6488 } else if (name.equals("factor")) { 6489 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6490 } else if (name.equals("tax")) { 6491 this.tax = TypeConvertor.castToMoney(value); // Money 6492 } else if (name.equals("net")) { 6493 this.net = TypeConvertor.castToMoney(value); // Money 6494 } else if (name.equals("udi")) { 6495 this.getUdi().add(TypeConvertor.castToReference(value)); 6496 } else if (name.equals("subDetail")) { 6497 this.getSubDetail().add((SubDetailComponent) value); 6498 } else 6499 return super.setProperty(name, value); 6500 return value; 6501 } 6502 6503 @Override 6504 public Base makeProperty(int hash, String name) throws FHIRException { 6505 switch (hash) { 6506 case 1349547969: return getSequenceElement(); 6507 case 82505966: return addTraceNumber(); 6508 case 1099842588: return getRevenue(); 6509 case 50511102: return getCategory(); 6510 case 1957227299: return getProductOrService(); 6511 case -717476168: return getProductOrServiceEnd(); 6512 case -615513385: return addModifier(); 6513 case 1010065041: return addProgramCode(); 6514 case 525514609: return getPatientPaid(); 6515 case -1285004149: return getQuantity(); 6516 case -486196699: return getUnitPrice(); 6517 case -1282148017: return getFactorElement(); 6518 case 114603: return getTax(); 6519 case 108957: return getNet(); 6520 case 115642: return addUdi(); 6521 case -828829007: return addSubDetail(); 6522 default: return super.makeProperty(hash, name); 6523 } 6524 6525 } 6526 6527 @Override 6528 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6529 switch (hash) { 6530 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 6531 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 6532 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6533 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6534 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 6535 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 6536 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6537 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 6538 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 6539 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 6540 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6541 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6542 case 114603: /*tax*/ return new String[] {"Money"}; 6543 case 108957: /*net*/ return new String[] {"Money"}; 6544 case 115642: /*udi*/ return new String[] {"Reference"}; 6545 case -828829007: /*subDetail*/ return new String[] {}; 6546 default: return super.getTypesForProperty(hash, name); 6547 } 6548 6549 } 6550 6551 @Override 6552 public Base addChild(String name) throws FHIRException { 6553 if (name.equals("sequence")) { 6554 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.sequence"); 6555 } 6556 else if (name.equals("traceNumber")) { 6557 return addTraceNumber(); 6558 } 6559 else if (name.equals("revenue")) { 6560 this.revenue = new CodeableConcept(); 6561 return this.revenue; 6562 } 6563 else if (name.equals("category")) { 6564 this.category = new CodeableConcept(); 6565 return this.category; 6566 } 6567 else if (name.equals("productOrService")) { 6568 this.productOrService = new CodeableConcept(); 6569 return this.productOrService; 6570 } 6571 else if (name.equals("productOrServiceEnd")) { 6572 this.productOrServiceEnd = new CodeableConcept(); 6573 return this.productOrServiceEnd; 6574 } 6575 else if (name.equals("modifier")) { 6576 return addModifier(); 6577 } 6578 else if (name.equals("programCode")) { 6579 return addProgramCode(); 6580 } 6581 else if (name.equals("patientPaid")) { 6582 this.patientPaid = new Money(); 6583 return this.patientPaid; 6584 } 6585 else if (name.equals("quantity")) { 6586 this.quantity = new Quantity(); 6587 return this.quantity; 6588 } 6589 else if (name.equals("unitPrice")) { 6590 this.unitPrice = new Money(); 6591 return this.unitPrice; 6592 } 6593 else if (name.equals("factor")) { 6594 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.factor"); 6595 } 6596 else if (name.equals("tax")) { 6597 this.tax = new Money(); 6598 return this.tax; 6599 } 6600 else if (name.equals("net")) { 6601 this.net = new Money(); 6602 return this.net; 6603 } 6604 else if (name.equals("udi")) { 6605 return addUdi(); 6606 } 6607 else if (name.equals("subDetail")) { 6608 return addSubDetail(); 6609 } 6610 else 6611 return super.addChild(name); 6612 } 6613 6614 public DetailComponent copy() { 6615 DetailComponent dst = new DetailComponent(); 6616 copyValues(dst); 6617 return dst; 6618 } 6619 6620 public void copyValues(DetailComponent dst) { 6621 super.copyValues(dst); 6622 dst.sequence = sequence == null ? null : sequence.copy(); 6623 if (traceNumber != null) { 6624 dst.traceNumber = new ArrayList<Identifier>(); 6625 for (Identifier i : traceNumber) 6626 dst.traceNumber.add(i.copy()); 6627 }; 6628 dst.revenue = revenue == null ? null : revenue.copy(); 6629 dst.category = category == null ? null : category.copy(); 6630 dst.productOrService = productOrService == null ? null : productOrService.copy(); 6631 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 6632 if (modifier != null) { 6633 dst.modifier = new ArrayList<CodeableConcept>(); 6634 for (CodeableConcept i : modifier) 6635 dst.modifier.add(i.copy()); 6636 }; 6637 if (programCode != null) { 6638 dst.programCode = new ArrayList<CodeableConcept>(); 6639 for (CodeableConcept i : programCode) 6640 dst.programCode.add(i.copy()); 6641 }; 6642 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 6643 dst.quantity = quantity == null ? null : quantity.copy(); 6644 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6645 dst.factor = factor == null ? null : factor.copy(); 6646 dst.tax = tax == null ? null : tax.copy(); 6647 dst.net = net == null ? null : net.copy(); 6648 if (udi != null) { 6649 dst.udi = new ArrayList<Reference>(); 6650 for (Reference i : udi) 6651 dst.udi.add(i.copy()); 6652 }; 6653 if (subDetail != null) { 6654 dst.subDetail = new ArrayList<SubDetailComponent>(); 6655 for (SubDetailComponent i : subDetail) 6656 dst.subDetail.add(i.copy()); 6657 }; 6658 } 6659 6660 @Override 6661 public boolean equalsDeep(Base other_) { 6662 if (!super.equalsDeep(other_)) 6663 return false; 6664 if (!(other_ instanceof DetailComponent)) 6665 return false; 6666 DetailComponent o = (DetailComponent) other_; 6667 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 6668 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 6669 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 6670 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 6671 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 6672 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6673 && compareDeep(subDetail, o.subDetail, true); 6674 } 6675 6676 @Override 6677 public boolean equalsShallow(Base other_) { 6678 if (!super.equalsShallow(other_)) 6679 return false; 6680 if (!(other_ instanceof DetailComponent)) 6681 return false; 6682 DetailComponent o = (DetailComponent) other_; 6683 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 6684 } 6685 6686 public boolean isEmpty() { 6687 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 6688 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 6689 , quantity, unitPrice, factor, tax, net, udi, subDetail); 6690 } 6691 6692 public String fhirType() { 6693 return "Claim.item.detail"; 6694 6695 } 6696 6697 } 6698 6699 @Block() 6700 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 6701 /** 6702 * A number to uniquely identify item entries. 6703 */ 6704 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6705 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 6706 protected PositiveIntType sequence; 6707 6708 /** 6709 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 6710 */ 6711 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6712 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 6713 protected List<Identifier> traceNumber; 6714 6715 /** 6716 * The type of revenue or cost center providing the product and/or service. 6717 */ 6718 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6719 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 6720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 6721 protected CodeableConcept revenue; 6722 6723 /** 6724 * Code to identify the general type of benefits under which products and services are provided. 6725 */ 6726 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 6727 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 6728 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6729 protected CodeableConcept category; 6730 6731 /** 6732 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 6733 */ 6734 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 6735 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 6736 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6737 protected CodeableConcept productOrService; 6738 6739 /** 6740 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 6741 */ 6742 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 6743 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 6744 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6745 protected CodeableConcept productOrServiceEnd; 6746 6747 /** 6748 * Item typification or modifiers codes to convey additional context for the product or service. 6749 */ 6750 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6751 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 6752 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 6753 protected List<CodeableConcept> modifier; 6754 6755 /** 6756 * Identifies the program under which this may be recovered. 6757 */ 6758 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6759 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 6760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 6761 protected List<CodeableConcept> programCode; 6762 6763 /** 6764 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 6765 */ 6766 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 6767 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 6768 protected Money patientPaid; 6769 6770 /** 6771 * The number of repetitions of a service or product. 6772 */ 6773 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 6774 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 6775 protected Quantity quantity; 6776 6777 /** 6778 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 6779 */ 6780 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 6781 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 6782 protected Money unitPrice; 6783 6784 /** 6785 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6786 */ 6787 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 6788 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 6789 protected DecimalType factor; 6790 6791 /** 6792 * The total of taxes applicable for this product or service. 6793 */ 6794 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 6795 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 6796 protected Money tax; 6797 6798 /** 6799 * The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor. 6800 */ 6801 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 6802 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor." ) 6803 protected Money net; 6804 6805 /** 6806 * Unique Device Identifiers associated with this line item. 6807 */ 6808 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6809 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 6810 protected List<Reference> udi; 6811 6812 private static final long serialVersionUID = -2049468862L; 6813 6814 /** 6815 * Constructor 6816 */ 6817 public SubDetailComponent() { 6818 super(); 6819 } 6820 6821 /** 6822 * Constructor 6823 */ 6824 public SubDetailComponent(int sequence) { 6825 super(); 6826 this.setSequence(sequence); 6827 } 6828 6829 /** 6830 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6831 */ 6832 public PositiveIntType getSequenceElement() { 6833 if (this.sequence == null) 6834 if (Configuration.errorOnAutoCreate()) 6835 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 6836 else if (Configuration.doAutoCreate()) 6837 this.sequence = new PositiveIntType(); // bb 6838 return this.sequence; 6839 } 6840 6841 public boolean hasSequenceElement() { 6842 return this.sequence != null && !this.sequence.isEmpty(); 6843 } 6844 6845 public boolean hasSequence() { 6846 return this.sequence != null && !this.sequence.isEmpty(); 6847 } 6848 6849 /** 6850 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6851 */ 6852 public SubDetailComponent setSequenceElement(PositiveIntType value) { 6853 this.sequence = value; 6854 return this; 6855 } 6856 6857 /** 6858 * @return A number to uniquely identify item entries. 6859 */ 6860 public int getSequence() { 6861 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6862 } 6863 6864 /** 6865 * @param value A number to uniquely identify item entries. 6866 */ 6867 public SubDetailComponent setSequence(int value) { 6868 if (this.sequence == null) 6869 this.sequence = new PositiveIntType(); 6870 this.sequence.setValue(value); 6871 return this; 6872 } 6873 6874 /** 6875 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 6876 */ 6877 public List<Identifier> getTraceNumber() { 6878 if (this.traceNumber == null) 6879 this.traceNumber = new ArrayList<Identifier>(); 6880 return this.traceNumber; 6881 } 6882 6883 /** 6884 * @return Returns a reference to <code>this</code> for easy method chaining 6885 */ 6886 public SubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 6887 this.traceNumber = theTraceNumber; 6888 return this; 6889 } 6890 6891 public boolean hasTraceNumber() { 6892 if (this.traceNumber == null) 6893 return false; 6894 for (Identifier item : this.traceNumber) 6895 if (!item.isEmpty()) 6896 return true; 6897 return false; 6898 } 6899 6900 public Identifier addTraceNumber() { //3 6901 Identifier t = new Identifier(); 6902 if (this.traceNumber == null) 6903 this.traceNumber = new ArrayList<Identifier>(); 6904 this.traceNumber.add(t); 6905 return t; 6906 } 6907 6908 public SubDetailComponent addTraceNumber(Identifier t) { //3 6909 if (t == null) 6910 return this; 6911 if (this.traceNumber == null) 6912 this.traceNumber = new ArrayList<Identifier>(); 6913 this.traceNumber.add(t); 6914 return this; 6915 } 6916 6917 /** 6918 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 6919 */ 6920 public Identifier getTraceNumberFirstRep() { 6921 if (getTraceNumber().isEmpty()) { 6922 addTraceNumber(); 6923 } 6924 return getTraceNumber().get(0); 6925 } 6926 6927 /** 6928 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6929 */ 6930 public CodeableConcept getRevenue() { 6931 if (this.revenue == null) 6932 if (Configuration.errorOnAutoCreate()) 6933 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 6934 else if (Configuration.doAutoCreate()) 6935 this.revenue = new CodeableConcept(); // cc 6936 return this.revenue; 6937 } 6938 6939 public boolean hasRevenue() { 6940 return this.revenue != null && !this.revenue.isEmpty(); 6941 } 6942 6943 /** 6944 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6945 */ 6946 public SubDetailComponent setRevenue(CodeableConcept value) { 6947 this.revenue = value; 6948 return this; 6949 } 6950 6951 /** 6952 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6953 */ 6954 public CodeableConcept getCategory() { 6955 if (this.category == null) 6956 if (Configuration.errorOnAutoCreate()) 6957 throw new Error("Attempt to auto-create SubDetailComponent.category"); 6958 else if (Configuration.doAutoCreate()) 6959 this.category = new CodeableConcept(); // cc 6960 return this.category; 6961 } 6962 6963 public boolean hasCategory() { 6964 return this.category != null && !this.category.isEmpty(); 6965 } 6966 6967 /** 6968 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6969 */ 6970 public SubDetailComponent setCategory(CodeableConcept value) { 6971 this.category = value; 6972 return this; 6973 } 6974 6975 /** 6976 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6977 */ 6978 public CodeableConcept getProductOrService() { 6979 if (this.productOrService == null) 6980 if (Configuration.errorOnAutoCreate()) 6981 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 6982 else if (Configuration.doAutoCreate()) 6983 this.productOrService = new CodeableConcept(); // cc 6984 return this.productOrService; 6985 } 6986 6987 public boolean hasProductOrService() { 6988 return this.productOrService != null && !this.productOrService.isEmpty(); 6989 } 6990 6991 /** 6992 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6993 */ 6994 public SubDetailComponent setProductOrService(CodeableConcept value) { 6995 this.productOrService = value; 6996 return this; 6997 } 6998 6999 /** 7000 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 7001 */ 7002 public CodeableConcept getProductOrServiceEnd() { 7003 if (this.productOrServiceEnd == null) 7004 if (Configuration.errorOnAutoCreate()) 7005 throw new Error("Attempt to auto-create SubDetailComponent.productOrServiceEnd"); 7006 else if (Configuration.doAutoCreate()) 7007 this.productOrServiceEnd = new CodeableConcept(); // cc 7008 return this.productOrServiceEnd; 7009 } 7010 7011 public boolean hasProductOrServiceEnd() { 7012 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 7013 } 7014 7015 /** 7016 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 7017 */ 7018 public SubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 7019 this.productOrServiceEnd = value; 7020 return this; 7021 } 7022 7023 /** 7024 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 7025 */ 7026 public List<CodeableConcept> getModifier() { 7027 if (this.modifier == null) 7028 this.modifier = new ArrayList<CodeableConcept>(); 7029 return this.modifier; 7030 } 7031 7032 /** 7033 * @return Returns a reference to <code>this</code> for easy method chaining 7034 */ 7035 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 7036 this.modifier = theModifier; 7037 return this; 7038 } 7039 7040 public boolean hasModifier() { 7041 if (this.modifier == null) 7042 return false; 7043 for (CodeableConcept item : this.modifier) 7044 if (!item.isEmpty()) 7045 return true; 7046 return false; 7047 } 7048 7049 public CodeableConcept addModifier() { //3 7050 CodeableConcept t = new CodeableConcept(); 7051 if (this.modifier == null) 7052 this.modifier = new ArrayList<CodeableConcept>(); 7053 this.modifier.add(t); 7054 return t; 7055 } 7056 7057 public SubDetailComponent addModifier(CodeableConcept t) { //3 7058 if (t == null) 7059 return this; 7060 if (this.modifier == null) 7061 this.modifier = new ArrayList<CodeableConcept>(); 7062 this.modifier.add(t); 7063 return this; 7064 } 7065 7066 /** 7067 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 7068 */ 7069 public CodeableConcept getModifierFirstRep() { 7070 if (getModifier().isEmpty()) { 7071 addModifier(); 7072 } 7073 return getModifier().get(0); 7074 } 7075 7076 /** 7077 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 7078 */ 7079 public List<CodeableConcept> getProgramCode() { 7080 if (this.programCode == null) 7081 this.programCode = new ArrayList<CodeableConcept>(); 7082 return this.programCode; 7083 } 7084 7085 /** 7086 * @return Returns a reference to <code>this</code> for easy method chaining 7087 */ 7088 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 7089 this.programCode = theProgramCode; 7090 return this; 7091 } 7092 7093 public boolean hasProgramCode() { 7094 if (this.programCode == null) 7095 return false; 7096 for (CodeableConcept item : this.programCode) 7097 if (!item.isEmpty()) 7098 return true; 7099 return false; 7100 } 7101 7102 public CodeableConcept addProgramCode() { //3 7103 CodeableConcept t = new CodeableConcept(); 7104 if (this.programCode == null) 7105 this.programCode = new ArrayList<CodeableConcept>(); 7106 this.programCode.add(t); 7107 return t; 7108 } 7109 7110 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 7111 if (t == null) 7112 return this; 7113 if (this.programCode == null) 7114 this.programCode = new ArrayList<CodeableConcept>(); 7115 this.programCode.add(t); 7116 return this; 7117 } 7118 7119 /** 7120 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 7121 */ 7122 public CodeableConcept getProgramCodeFirstRep() { 7123 if (getProgramCode().isEmpty()) { 7124 addProgramCode(); 7125 } 7126 return getProgramCode().get(0); 7127 } 7128 7129 /** 7130 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 7131 */ 7132 public Money getPatientPaid() { 7133 if (this.patientPaid == null) 7134 if (Configuration.errorOnAutoCreate()) 7135 throw new Error("Attempt to auto-create SubDetailComponent.patientPaid"); 7136 else if (Configuration.doAutoCreate()) 7137 this.patientPaid = new Money(); // cc 7138 return this.patientPaid; 7139 } 7140 7141 public boolean hasPatientPaid() { 7142 return this.patientPaid != null && !this.patientPaid.isEmpty(); 7143 } 7144 7145 /** 7146 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 7147 */ 7148 public SubDetailComponent setPatientPaid(Money value) { 7149 this.patientPaid = value; 7150 return this; 7151 } 7152 7153 /** 7154 * @return {@link #quantity} (The number of repetitions of a service or product.) 7155 */ 7156 public Quantity getQuantity() { 7157 if (this.quantity == null) 7158 if (Configuration.errorOnAutoCreate()) 7159 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 7160 else if (Configuration.doAutoCreate()) 7161 this.quantity = new Quantity(); // cc 7162 return this.quantity; 7163 } 7164 7165 public boolean hasQuantity() { 7166 return this.quantity != null && !this.quantity.isEmpty(); 7167 } 7168 7169 /** 7170 * @param value {@link #quantity} (The number of repetitions of a service or product.) 7171 */ 7172 public SubDetailComponent setQuantity(Quantity value) { 7173 this.quantity = value; 7174 return this; 7175 } 7176 7177 /** 7178 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 7179 */ 7180 public Money getUnitPrice() { 7181 if (this.unitPrice == null) 7182 if (Configuration.errorOnAutoCreate()) 7183 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 7184 else if (Configuration.doAutoCreate()) 7185 this.unitPrice = new Money(); // cc 7186 return this.unitPrice; 7187 } 7188 7189 public boolean hasUnitPrice() { 7190 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7191 } 7192 7193 /** 7194 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 7195 */ 7196 public SubDetailComponent setUnitPrice(Money value) { 7197 this.unitPrice = value; 7198 return this; 7199 } 7200 7201 /** 7202 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 7203 */ 7204 public DecimalType getFactorElement() { 7205 if (this.factor == null) 7206 if (Configuration.errorOnAutoCreate()) 7207 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 7208 else if (Configuration.doAutoCreate()) 7209 this.factor = new DecimalType(); // bb 7210 return this.factor; 7211 } 7212 7213 public boolean hasFactorElement() { 7214 return this.factor != null && !this.factor.isEmpty(); 7215 } 7216 7217 public boolean hasFactor() { 7218 return this.factor != null && !this.factor.isEmpty(); 7219 } 7220 7221 /** 7222 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 7223 */ 7224 public SubDetailComponent setFactorElement(DecimalType value) { 7225 this.factor = value; 7226 return this; 7227 } 7228 7229 /** 7230 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7231 */ 7232 public BigDecimal getFactor() { 7233 return this.factor == null ? null : this.factor.getValue(); 7234 } 7235 7236 /** 7237 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7238 */ 7239 public SubDetailComponent setFactor(BigDecimal value) { 7240 if (value == null) 7241 this.factor = null; 7242 else { 7243 if (this.factor == null) 7244 this.factor = new DecimalType(); 7245 this.factor.setValue(value); 7246 } 7247 return this; 7248 } 7249 7250 /** 7251 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7252 */ 7253 public SubDetailComponent setFactor(long value) { 7254 this.factor = new DecimalType(); 7255 this.factor.setValue(value); 7256 return this; 7257 } 7258 7259 /** 7260 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7261 */ 7262 public SubDetailComponent setFactor(double value) { 7263 this.factor = new DecimalType(); 7264 this.factor.setValue(value); 7265 return this; 7266 } 7267 7268 /** 7269 * @return {@link #tax} (The total of taxes applicable for this product or service.) 7270 */ 7271 public Money getTax() { 7272 if (this.tax == null) 7273 if (Configuration.errorOnAutoCreate()) 7274 throw new Error("Attempt to auto-create SubDetailComponent.tax"); 7275 else if (Configuration.doAutoCreate()) 7276 this.tax = new Money(); // cc 7277 return this.tax; 7278 } 7279 7280 public boolean hasTax() { 7281 return this.tax != null && !this.tax.isEmpty(); 7282 } 7283 7284 /** 7285 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 7286 */ 7287 public SubDetailComponent setTax(Money value) { 7288 this.tax = value; 7289 return this; 7290 } 7291 7292 /** 7293 * @return {@link #net} (The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.) 7294 */ 7295 public Money getNet() { 7296 if (this.net == null) 7297 if (Configuration.errorOnAutoCreate()) 7298 throw new Error("Attempt to auto-create SubDetailComponent.net"); 7299 else if (Configuration.doAutoCreate()) 7300 this.net = new Money(); // cc 7301 return this.net; 7302 } 7303 7304 public boolean hasNet() { 7305 return this.net != null && !this.net.isEmpty(); 7306 } 7307 7308 /** 7309 * @param value {@link #net} (The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.) 7310 */ 7311 public SubDetailComponent setNet(Money value) { 7312 this.net = value; 7313 return this; 7314 } 7315 7316 /** 7317 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 7318 */ 7319 public List<Reference> getUdi() { 7320 if (this.udi == null) 7321 this.udi = new ArrayList<Reference>(); 7322 return this.udi; 7323 } 7324 7325 /** 7326 * @return Returns a reference to <code>this</code> for easy method chaining 7327 */ 7328 public SubDetailComponent setUdi(List<Reference> theUdi) { 7329 this.udi = theUdi; 7330 return this; 7331 } 7332 7333 public boolean hasUdi() { 7334 if (this.udi == null) 7335 return false; 7336 for (Reference item : this.udi) 7337 if (!item.isEmpty()) 7338 return true; 7339 return false; 7340 } 7341 7342 public Reference addUdi() { //3 7343 Reference t = new Reference(); 7344 if (this.udi == null) 7345 this.udi = new ArrayList<Reference>(); 7346 this.udi.add(t); 7347 return t; 7348 } 7349 7350 public SubDetailComponent addUdi(Reference t) { //3 7351 if (t == null) 7352 return this; 7353 if (this.udi == null) 7354 this.udi = new ArrayList<Reference>(); 7355 this.udi.add(t); 7356 return this; 7357 } 7358 7359 /** 7360 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 7361 */ 7362 public Reference getUdiFirstRep() { 7363 if (getUdi().isEmpty()) { 7364 addUdi(); 7365 } 7366 return getUdi().get(0); 7367 } 7368 7369 protected void listChildren(List<Property> children) { 7370 super.listChildren(children); 7371 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 7372 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 7373 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7374 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 7375 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 7376 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 7377 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 7378 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7379 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 7380 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 7381 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 7382 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 7383 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 7384 children.add(new Property("net", "Money", "The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 7385 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 7386 } 7387 7388 @Override 7389 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7390 switch (_hash) { 7391 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 7392 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 7393 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7394 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 7395 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 7396 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 7397 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 7398 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7399 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 7400 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 7401 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 7402 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 7403 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 7404 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 7405 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7406 default: return super.getNamedProperty(_hash, _name, _checkValid); 7407 } 7408 7409 } 7410 7411 @Override 7412 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7413 switch (hash) { 7414 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 7415 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 7416 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 7417 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 7418 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 7419 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 7420 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7421 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7422 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 7423 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 7424 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 7425 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 7426 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 7427 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 7428 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7429 default: return super.getProperty(hash, name, checkValid); 7430 } 7431 7432 } 7433 7434 @Override 7435 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7436 switch (hash) { 7437 case 1349547969: // sequence 7438 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7439 return value; 7440 case 82505966: // traceNumber 7441 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 7442 return value; 7443 case 1099842588: // revenue 7444 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7445 return value; 7446 case 50511102: // category 7447 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7448 return value; 7449 case 1957227299: // productOrService 7450 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7451 return value; 7452 case -717476168: // productOrServiceEnd 7453 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7454 return value; 7455 case -615513385: // modifier 7456 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7457 return value; 7458 case 1010065041: // programCode 7459 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7460 return value; 7461 case 525514609: // patientPaid 7462 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7463 return value; 7464 case -1285004149: // quantity 7465 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7466 return value; 7467 case -486196699: // unitPrice 7468 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7469 return value; 7470 case -1282148017: // factor 7471 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7472 return value; 7473 case 114603: // tax 7474 this.tax = TypeConvertor.castToMoney(value); // Money 7475 return value; 7476 case 108957: // net 7477 this.net = TypeConvertor.castToMoney(value); // Money 7478 return value; 7479 case 115642: // udi 7480 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 7481 return value; 7482 default: return super.setProperty(hash, name, value); 7483 } 7484 7485 } 7486 7487 @Override 7488 public Base setProperty(String name, Base value) throws FHIRException { 7489 if (name.equals("sequence")) { 7490 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7491 } else if (name.equals("traceNumber")) { 7492 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 7493 } else if (name.equals("revenue")) { 7494 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7495 } else if (name.equals("category")) { 7496 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7497 } else if (name.equals("productOrService")) { 7498 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7499 } else if (name.equals("productOrServiceEnd")) { 7500 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7501 } else if (name.equals("modifier")) { 7502 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 7503 } else if (name.equals("programCode")) { 7504 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 7505 } else if (name.equals("patientPaid")) { 7506 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7507 } else if (name.equals("quantity")) { 7508 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7509 } else if (name.equals("unitPrice")) { 7510 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7511 } else if (name.equals("factor")) { 7512 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7513 } else if (name.equals("tax")) { 7514 this.tax = TypeConvertor.castToMoney(value); // Money 7515 } else if (name.equals("net")) { 7516 this.net = TypeConvertor.castToMoney(value); // Money 7517 } else if (name.equals("udi")) { 7518 this.getUdi().add(TypeConvertor.castToReference(value)); 7519 } else 7520 return super.setProperty(name, value); 7521 return value; 7522 } 7523 7524 @Override 7525 public Base makeProperty(int hash, String name) throws FHIRException { 7526 switch (hash) { 7527 case 1349547969: return getSequenceElement(); 7528 case 82505966: return addTraceNumber(); 7529 case 1099842588: return getRevenue(); 7530 case 50511102: return getCategory(); 7531 case 1957227299: return getProductOrService(); 7532 case -717476168: return getProductOrServiceEnd(); 7533 case -615513385: return addModifier(); 7534 case 1010065041: return addProgramCode(); 7535 case 525514609: return getPatientPaid(); 7536 case -1285004149: return getQuantity(); 7537 case -486196699: return getUnitPrice(); 7538 case -1282148017: return getFactorElement(); 7539 case 114603: return getTax(); 7540 case 108957: return getNet(); 7541 case 115642: return addUdi(); 7542 default: return super.makeProperty(hash, name); 7543 } 7544 7545 } 7546 7547 @Override 7548 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7549 switch (hash) { 7550 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 7551 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 7552 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7553 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7554 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 7555 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 7556 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7557 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 7558 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 7559 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 7560 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 7561 case -1282148017: /*factor*/ return new String[] {"decimal"}; 7562 case 114603: /*tax*/ return new String[] {"Money"}; 7563 case 108957: /*net*/ return new String[] {"Money"}; 7564 case 115642: /*udi*/ return new String[] {"Reference"}; 7565 default: return super.getTypesForProperty(hash, name); 7566 } 7567 7568 } 7569 7570 @Override 7571 public Base addChild(String name) throws FHIRException { 7572 if (name.equals("sequence")) { 7573 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.subDetail.sequence"); 7574 } 7575 else if (name.equals("traceNumber")) { 7576 return addTraceNumber(); 7577 } 7578 else if (name.equals("revenue")) { 7579 this.revenue = new CodeableConcept(); 7580 return this.revenue; 7581 } 7582 else if (name.equals("category")) { 7583 this.category = new CodeableConcept(); 7584 return this.category; 7585 } 7586 else if (name.equals("productOrService")) { 7587 this.productOrService = new CodeableConcept(); 7588 return this.productOrService; 7589 } 7590 else if (name.equals("productOrServiceEnd")) { 7591 this.productOrServiceEnd = new CodeableConcept(); 7592 return this.productOrServiceEnd; 7593 } 7594 else if (name.equals("modifier")) { 7595 return addModifier(); 7596 } 7597 else if (name.equals("programCode")) { 7598 return addProgramCode(); 7599 } 7600 else if (name.equals("patientPaid")) { 7601 this.patientPaid = new Money(); 7602 return this.patientPaid; 7603 } 7604 else if (name.equals("quantity")) { 7605 this.quantity = new Quantity(); 7606 return this.quantity; 7607 } 7608 else if (name.equals("unitPrice")) { 7609 this.unitPrice = new Money(); 7610 return this.unitPrice; 7611 } 7612 else if (name.equals("factor")) { 7613 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.subDetail.factor"); 7614 } 7615 else if (name.equals("tax")) { 7616 this.tax = new Money(); 7617 return this.tax; 7618 } 7619 else if (name.equals("net")) { 7620 this.net = new Money(); 7621 return this.net; 7622 } 7623 else if (name.equals("udi")) { 7624 return addUdi(); 7625 } 7626 else 7627 return super.addChild(name); 7628 } 7629 7630 public SubDetailComponent copy() { 7631 SubDetailComponent dst = new SubDetailComponent(); 7632 copyValues(dst); 7633 return dst; 7634 } 7635 7636 public void copyValues(SubDetailComponent dst) { 7637 super.copyValues(dst); 7638 dst.sequence = sequence == null ? null : sequence.copy(); 7639 if (traceNumber != null) { 7640 dst.traceNumber = new ArrayList<Identifier>(); 7641 for (Identifier i : traceNumber) 7642 dst.traceNumber.add(i.copy()); 7643 }; 7644 dst.revenue = revenue == null ? null : revenue.copy(); 7645 dst.category = category == null ? null : category.copy(); 7646 dst.productOrService = productOrService == null ? null : productOrService.copy(); 7647 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 7648 if (modifier != null) { 7649 dst.modifier = new ArrayList<CodeableConcept>(); 7650 for (CodeableConcept i : modifier) 7651 dst.modifier.add(i.copy()); 7652 }; 7653 if (programCode != null) { 7654 dst.programCode = new ArrayList<CodeableConcept>(); 7655 for (CodeableConcept i : programCode) 7656 dst.programCode.add(i.copy()); 7657 }; 7658 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 7659 dst.quantity = quantity == null ? null : quantity.copy(); 7660 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7661 dst.factor = factor == null ? null : factor.copy(); 7662 dst.tax = tax == null ? null : tax.copy(); 7663 dst.net = net == null ? null : net.copy(); 7664 if (udi != null) { 7665 dst.udi = new ArrayList<Reference>(); 7666 for (Reference i : udi) 7667 dst.udi.add(i.copy()); 7668 }; 7669 } 7670 7671 @Override 7672 public boolean equalsDeep(Base other_) { 7673 if (!super.equalsDeep(other_)) 7674 return false; 7675 if (!(other_ instanceof SubDetailComponent)) 7676 return false; 7677 SubDetailComponent o = (SubDetailComponent) other_; 7678 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 7679 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 7680 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 7681 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 7682 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 7683 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7684 ; 7685 } 7686 7687 @Override 7688 public boolean equalsShallow(Base other_) { 7689 if (!super.equalsShallow(other_)) 7690 return false; 7691 if (!(other_ instanceof SubDetailComponent)) 7692 return false; 7693 SubDetailComponent o = (SubDetailComponent) other_; 7694 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 7695 } 7696 7697 public boolean isEmpty() { 7698 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 7699 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 7700 , quantity, unitPrice, factor, tax, net, udi); 7701 } 7702 7703 public String fhirType() { 7704 return "Claim.item.detail.subDetail"; 7705 7706 } 7707 7708 } 7709 7710 /** 7711 * A unique identifier assigned to this claim. 7712 */ 7713 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7714 @Description(shortDefinition="Business Identifier for claim", formalDefinition="A unique identifier assigned to this claim." ) 7715 protected List<Identifier> identifier; 7716 7717 /** 7718 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 7719 */ 7720 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7721 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 7722 protected List<Identifier> traceNumber; 7723 7724 /** 7725 * The status of the resource instance. 7726 */ 7727 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 7728 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 7729 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 7730 protected Enumeration<FinancialResourceStatusCodes> status; 7731 7732 /** 7733 * The category of claim, e.g. oral, pharmacy, vision, institutional, professional. 7734 */ 7735 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 7736 @Description(shortDefinition="Category or discipline", formalDefinition="The category of claim, e.g. oral, pharmacy, vision, institutional, professional." ) 7737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 7738 protected CodeableConcept type; 7739 7740 /** 7741 * A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service. 7742 */ 7743 @Child(name = "subType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 7744 @Description(shortDefinition="More granular claim type", formalDefinition="A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service." ) 7745 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 7746 protected CodeableConcept subType; 7747 7748 /** 7749 * A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 7750 */ 7751 @Child(name = "use", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 7752 @Description(shortDefinition="claim | preauthorization | predetermination", formalDefinition="A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided." ) 7753 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 7754 protected Enumeration<Use> use; 7755 7756 /** 7757 * The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought. 7758 */ 7759 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 7760 @Description(shortDefinition="The recipient of the products and services", formalDefinition="The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought." ) 7761 protected Reference patient; 7762 7763 /** 7764 * The period for which charges are being submitted. 7765 */ 7766 @Child(name = "billablePeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 7767 @Description(shortDefinition="Relevant time frame for the claim", formalDefinition="The period for which charges are being submitted." ) 7768 protected Period billablePeriod; 7769 7770 /** 7771 * The date this resource was created. 7772 */ 7773 @Child(name = "created", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 7774 @Description(shortDefinition="Resource creation date", formalDefinition="The date this resource was created." ) 7775 protected DateTimeType created; 7776 7777 /** 7778 * Individual who created the claim, predetermination or preauthorization. 7779 */ 7780 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=false) 7781 @Description(shortDefinition="Author of the claim", formalDefinition="Individual who created the claim, predetermination or preauthorization." ) 7782 protected Reference enterer; 7783 7784 /** 7785 * The Insurer who is target of the request. 7786 */ 7787 @Child(name = "insurer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=true) 7788 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 7789 protected Reference insurer; 7790 7791 /** 7792 * The provider which is responsible for the claim, predetermination or preauthorization. 7793 */ 7794 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 7795 @Description(shortDefinition="Party responsible for the claim", formalDefinition="The provider which is responsible for the claim, predetermination or preauthorization." ) 7796 protected Reference provider; 7797 7798 /** 7799 * The provider-required urgency of processing the request. Typical values include: stat, normal, deferred. 7800 */ 7801 @Child(name = "priority", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 7802 @Description(shortDefinition="Desired processing urgency", formalDefinition="The provider-required urgency of processing the request. Typical values include: stat, normal, deferred." ) 7803 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 7804 protected CodeableConcept priority; 7805 7806 /** 7807 * A code to indicate whether and for whom funds are to be reserved for future claims. 7808 */ 7809 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 7810 @Description(shortDefinition="For whom to reserve funds", formalDefinition="A code to indicate whether and for whom funds are to be reserved for future claims." ) 7811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 7812 protected CodeableConcept fundsReserve; 7813 7814 /** 7815 * Other claims which are related to this claim such as prior submissions or claims for related services or for the same event. 7816 */ 7817 @Child(name = "related", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7818 @Description(shortDefinition="Prior or corollary claims", formalDefinition="Other claims which are related to this claim such as prior submissions or claims for related services or for the same event." ) 7819 protected List<RelatedClaimComponent> related; 7820 7821 /** 7822 * Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments. 7823 */ 7824 @Child(name = "prescription", type = {DeviceRequest.class, MedicationRequest.class, VisionPrescription.class}, order=15, min=0, max=1, modifier=false, summary=false) 7825 @Description(shortDefinition="Prescription authorizing services and products", formalDefinition="Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments." ) 7826 protected Reference prescription; 7827 7828 /** 7829 * Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products. 7830 */ 7831 @Child(name = "originalPrescription", type = {DeviceRequest.class, MedicationRequest.class, VisionPrescription.class}, order=16, min=0, max=1, modifier=false, summary=false) 7832 @Description(shortDefinition="Original prescription if superseded by fulfiller", formalDefinition="Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products." ) 7833 protected Reference originalPrescription; 7834 7835 /** 7836 * The party to be reimbursed for cost of the products and services according to the terms of the policy. 7837 */ 7838 @Child(name = "payee", type = {}, order=17, min=0, max=1, modifier=false, summary=false) 7839 @Description(shortDefinition="Recipient of benefits payable", formalDefinition="The party to be reimbursed for cost of the products and services according to the terms of the policy." ) 7840 protected PayeeComponent payee; 7841 7842 /** 7843 * The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services. 7844 */ 7845 @Child(name = "referral", type = {ServiceRequest.class}, order=18, min=0, max=1, modifier=false, summary=false) 7846 @Description(shortDefinition="Treatment referral", formalDefinition="The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services." ) 7847 protected Reference referral; 7848 7849 /** 7850 * Healthcare encounters related to this claim. 7851 */ 7852 @Child(name = "encounter", type = {Encounter.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7853 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 7854 protected List<Reference> encounter; 7855 7856 /** 7857 * Facility where the services were provided. 7858 */ 7859 @Child(name = "facility", type = {Location.class, Organization.class}, order=20, min=0, max=1, modifier=false, summary=false) 7860 @Description(shortDefinition="Servicing facility", formalDefinition="Facility where the services were provided." ) 7861 protected Reference facility; 7862 7863 /** 7864 * A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system. 7865 */ 7866 @Child(name = "diagnosisRelatedGroup", type = {CodeableConcept.class}, order=21, min=0, max=1, modifier=false, summary=false) 7867 @Description(shortDefinition="Package billing code", formalDefinition="A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system." ) 7868 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 7869 protected CodeableConcept diagnosisRelatedGroup; 7870 7871 /** 7872 * Information code for an event with a corresponding date or period. 7873 */ 7874 @Child(name = "event", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7875 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 7876 protected List<ClaimEventComponent> event; 7877 7878 /** 7879 * The members of the team who provided the products and services. 7880 */ 7881 @Child(name = "careTeam", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7882 @Description(shortDefinition="Members of the care team", formalDefinition="The members of the team who provided the products and services." ) 7883 protected List<CareTeamComponent> careTeam; 7884 7885 /** 7886 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. 7887 */ 7888 @Child(name = "supportingInfo", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7889 @Description(shortDefinition="Supporting information", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues." ) 7890 protected List<SupportingInformationComponent> supportingInfo; 7891 7892 /** 7893 * Information about diagnoses relevant to the claim items. 7894 */ 7895 @Child(name = "diagnosis", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7896 @Description(shortDefinition="Pertinent diagnosis information", formalDefinition="Information about diagnoses relevant to the claim items." ) 7897 protected List<DiagnosisComponent> diagnosis; 7898 7899 /** 7900 * Procedures performed on the patient relevant to the billing items with the claim. 7901 */ 7902 @Child(name = "procedure", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7903 @Description(shortDefinition="Clinical procedures performed", formalDefinition="Procedures performed on the patient relevant to the billing items with the claim." ) 7904 protected List<ProcedureComponent> procedure; 7905 7906 /** 7907 * Financial instruments for reimbursement for the health care products and services specified on the claim. 7908 */ 7909 @Child(name = "insurance", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 7910 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services specified on the claim." ) 7911 protected List<InsuranceComponent> insurance; 7912 7913 /** 7914 * Details of an accident which resulted in injuries which required the products and services listed in the claim. 7915 */ 7916 @Child(name = "accident", type = {}, order=28, min=0, max=1, modifier=false, summary=false) 7917 @Description(shortDefinition="Details of the event", formalDefinition="Details of an accident which resulted in injuries which required the products and services listed in the claim." ) 7918 protected AccidentComponent accident; 7919 7920 /** 7921 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 7922 */ 7923 @Child(name = "patientPaid", type = {Money.class}, order=29, min=0, max=1, modifier=false, summary=false) 7924 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 7925 protected Money patientPaid; 7926 7927 /** 7928 * A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details. 7929 */ 7930 @Child(name = "item", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7931 @Description(shortDefinition="Product or service provided", formalDefinition="A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details." ) 7932 protected List<ItemComponent> item; 7933 7934 /** 7935 * The total value of the all the items in the claim. 7936 */ 7937 @Child(name = "total", type = {Money.class}, order=31, min=0, max=1, modifier=false, summary=false) 7938 @Description(shortDefinition="Total claim cost", formalDefinition="The total value of the all the items in the claim." ) 7939 protected Money total; 7940 7941 private static final long serialVersionUID = -64601265L; 7942 7943 /** 7944 * Constructor 7945 */ 7946 public Claim() { 7947 super(); 7948 } 7949 7950 /** 7951 * Constructor 7952 */ 7953 public Claim(FinancialResourceStatusCodes status, CodeableConcept type, Use use, Reference patient, Date created) { 7954 super(); 7955 this.setStatus(status); 7956 this.setType(type); 7957 this.setUse(use); 7958 this.setPatient(patient); 7959 this.setCreated(created); 7960 } 7961 7962 /** 7963 * @return {@link #identifier} (A unique identifier assigned to this claim.) 7964 */ 7965 public List<Identifier> getIdentifier() { 7966 if (this.identifier == null) 7967 this.identifier = new ArrayList<Identifier>(); 7968 return this.identifier; 7969 } 7970 7971 /** 7972 * @return Returns a reference to <code>this</code> for easy method chaining 7973 */ 7974 public Claim setIdentifier(List<Identifier> theIdentifier) { 7975 this.identifier = theIdentifier; 7976 return this; 7977 } 7978 7979 public boolean hasIdentifier() { 7980 if (this.identifier == null) 7981 return false; 7982 for (Identifier item : this.identifier) 7983 if (!item.isEmpty()) 7984 return true; 7985 return false; 7986 } 7987 7988 public Identifier addIdentifier() { //3 7989 Identifier t = new Identifier(); 7990 if (this.identifier == null) 7991 this.identifier = new ArrayList<Identifier>(); 7992 this.identifier.add(t); 7993 return t; 7994 } 7995 7996 public Claim addIdentifier(Identifier t) { //3 7997 if (t == null) 7998 return this; 7999 if (this.identifier == null) 8000 this.identifier = new ArrayList<Identifier>(); 8001 this.identifier.add(t); 8002 return this; 8003 } 8004 8005 /** 8006 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 8007 */ 8008 public Identifier getIdentifierFirstRep() { 8009 if (getIdentifier().isEmpty()) { 8010 addIdentifier(); 8011 } 8012 return getIdentifier().get(0); 8013 } 8014 8015 /** 8016 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 8017 */ 8018 public List<Identifier> getTraceNumber() { 8019 if (this.traceNumber == null) 8020 this.traceNumber = new ArrayList<Identifier>(); 8021 return this.traceNumber; 8022 } 8023 8024 /** 8025 * @return Returns a reference to <code>this</code> for easy method chaining 8026 */ 8027 public Claim setTraceNumber(List<Identifier> theTraceNumber) { 8028 this.traceNumber = theTraceNumber; 8029 return this; 8030 } 8031 8032 public boolean hasTraceNumber() { 8033 if (this.traceNumber == null) 8034 return false; 8035 for (Identifier item : this.traceNumber) 8036 if (!item.isEmpty()) 8037 return true; 8038 return false; 8039 } 8040 8041 public Identifier addTraceNumber() { //3 8042 Identifier t = new Identifier(); 8043 if (this.traceNumber == null) 8044 this.traceNumber = new ArrayList<Identifier>(); 8045 this.traceNumber.add(t); 8046 return t; 8047 } 8048 8049 public Claim addTraceNumber(Identifier t) { //3 8050 if (t == null) 8051 return this; 8052 if (this.traceNumber == null) 8053 this.traceNumber = new ArrayList<Identifier>(); 8054 this.traceNumber.add(t); 8055 return this; 8056 } 8057 8058 /** 8059 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 8060 */ 8061 public Identifier getTraceNumberFirstRep() { 8062 if (getTraceNumber().isEmpty()) { 8063 addTraceNumber(); 8064 } 8065 return getTraceNumber().get(0); 8066 } 8067 8068 /** 8069 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8070 */ 8071 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 8072 if (this.status == null) 8073 if (Configuration.errorOnAutoCreate()) 8074 throw new Error("Attempt to auto-create Claim.status"); 8075 else if (Configuration.doAutoCreate()) 8076 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 8077 return this.status; 8078 } 8079 8080 public boolean hasStatusElement() { 8081 return this.status != null && !this.status.isEmpty(); 8082 } 8083 8084 public boolean hasStatus() { 8085 return this.status != null && !this.status.isEmpty(); 8086 } 8087 8088 /** 8089 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8090 */ 8091 public Claim setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 8092 this.status = value; 8093 return this; 8094 } 8095 8096 /** 8097 * @return The status of the resource instance. 8098 */ 8099 public FinancialResourceStatusCodes getStatus() { 8100 return this.status == null ? null : this.status.getValue(); 8101 } 8102 8103 /** 8104 * @param value The status of the resource instance. 8105 */ 8106 public Claim setStatus(FinancialResourceStatusCodes value) { 8107 if (this.status == null) 8108 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 8109 this.status.setValue(value); 8110 return this; 8111 } 8112 8113 /** 8114 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 8115 */ 8116 public CodeableConcept getType() { 8117 if (this.type == null) 8118 if (Configuration.errorOnAutoCreate()) 8119 throw new Error("Attempt to auto-create Claim.type"); 8120 else if (Configuration.doAutoCreate()) 8121 this.type = new CodeableConcept(); // cc 8122 return this.type; 8123 } 8124 8125 public boolean hasType() { 8126 return this.type != null && !this.type.isEmpty(); 8127 } 8128 8129 /** 8130 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 8131 */ 8132 public Claim setType(CodeableConcept value) { 8133 this.type = value; 8134 return this; 8135 } 8136 8137 /** 8138 * @return {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 8139 */ 8140 public CodeableConcept getSubType() { 8141 if (this.subType == null) 8142 if (Configuration.errorOnAutoCreate()) 8143 throw new Error("Attempt to auto-create Claim.subType"); 8144 else if (Configuration.doAutoCreate()) 8145 this.subType = new CodeableConcept(); // cc 8146 return this.subType; 8147 } 8148 8149 public boolean hasSubType() { 8150 return this.subType != null && !this.subType.isEmpty(); 8151 } 8152 8153 /** 8154 * @param value {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 8155 */ 8156 public Claim setSubType(CodeableConcept value) { 8157 this.subType = value; 8158 return this; 8159 } 8160 8161 /** 8162 * @return {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 8163 */ 8164 public Enumeration<Use> getUseElement() { 8165 if (this.use == null) 8166 if (Configuration.errorOnAutoCreate()) 8167 throw new Error("Attempt to auto-create Claim.use"); 8168 else if (Configuration.doAutoCreate()) 8169 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 8170 return this.use; 8171 } 8172 8173 public boolean hasUseElement() { 8174 return this.use != null && !this.use.isEmpty(); 8175 } 8176 8177 public boolean hasUse() { 8178 return this.use != null && !this.use.isEmpty(); 8179 } 8180 8181 /** 8182 * @param value {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 8183 */ 8184 public Claim setUseElement(Enumeration<Use> value) { 8185 this.use = value; 8186 return this; 8187 } 8188 8189 /** 8190 * @return A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 8191 */ 8192 public Use getUse() { 8193 return this.use == null ? null : this.use.getValue(); 8194 } 8195 8196 /** 8197 * @param value A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 8198 */ 8199 public Claim setUse(Use value) { 8200 if (this.use == null) 8201 this.use = new Enumeration<Use>(new UseEnumFactory()); 8202 this.use.setValue(value); 8203 return this; 8204 } 8205 8206 /** 8207 * @return {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.) 8208 */ 8209 public Reference getPatient() { 8210 if (this.patient == null) 8211 if (Configuration.errorOnAutoCreate()) 8212 throw new Error("Attempt to auto-create Claim.patient"); 8213 else if (Configuration.doAutoCreate()) 8214 this.patient = new Reference(); // cc 8215 return this.patient; 8216 } 8217 8218 public boolean hasPatient() { 8219 return this.patient != null && !this.patient.isEmpty(); 8220 } 8221 8222 /** 8223 * @param value {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.) 8224 */ 8225 public Claim setPatient(Reference value) { 8226 this.patient = value; 8227 return this; 8228 } 8229 8230 /** 8231 * @return {@link #billablePeriod} (The period for which charges are being submitted.) 8232 */ 8233 public Period getBillablePeriod() { 8234 if (this.billablePeriod == null) 8235 if (Configuration.errorOnAutoCreate()) 8236 throw new Error("Attempt to auto-create Claim.billablePeriod"); 8237 else if (Configuration.doAutoCreate()) 8238 this.billablePeriod = new Period(); // cc 8239 return this.billablePeriod; 8240 } 8241 8242 public boolean hasBillablePeriod() { 8243 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 8244 } 8245 8246 /** 8247 * @param value {@link #billablePeriod} (The period for which charges are being submitted.) 8248 */ 8249 public Claim setBillablePeriod(Period value) { 8250 this.billablePeriod = value; 8251 return this; 8252 } 8253 8254 /** 8255 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 8256 */ 8257 public DateTimeType getCreatedElement() { 8258 if (this.created == null) 8259 if (Configuration.errorOnAutoCreate()) 8260 throw new Error("Attempt to auto-create Claim.created"); 8261 else if (Configuration.doAutoCreate()) 8262 this.created = new DateTimeType(); // bb 8263 return this.created; 8264 } 8265 8266 public boolean hasCreatedElement() { 8267 return this.created != null && !this.created.isEmpty(); 8268 } 8269 8270 public boolean hasCreated() { 8271 return this.created != null && !this.created.isEmpty(); 8272 } 8273 8274 /** 8275 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 8276 */ 8277 public Claim setCreatedElement(DateTimeType value) { 8278 this.created = value; 8279 return this; 8280 } 8281 8282 /** 8283 * @return The date this resource was created. 8284 */ 8285 public Date getCreated() { 8286 return this.created == null ? null : this.created.getValue(); 8287 } 8288 8289 /** 8290 * @param value The date this resource was created. 8291 */ 8292 public Claim setCreated(Date value) { 8293 if (this.created == null) 8294 this.created = new DateTimeType(); 8295 this.created.setValue(value); 8296 return this; 8297 } 8298 8299 /** 8300 * @return {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 8301 */ 8302 public Reference getEnterer() { 8303 if (this.enterer == null) 8304 if (Configuration.errorOnAutoCreate()) 8305 throw new Error("Attempt to auto-create Claim.enterer"); 8306 else if (Configuration.doAutoCreate()) 8307 this.enterer = new Reference(); // cc 8308 return this.enterer; 8309 } 8310 8311 public boolean hasEnterer() { 8312 return this.enterer != null && !this.enterer.isEmpty(); 8313 } 8314 8315 /** 8316 * @param value {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 8317 */ 8318 public Claim setEnterer(Reference value) { 8319 this.enterer = value; 8320 return this; 8321 } 8322 8323 /** 8324 * @return {@link #insurer} (The Insurer who is target of the request.) 8325 */ 8326 public Reference getInsurer() { 8327 if (this.insurer == null) 8328 if (Configuration.errorOnAutoCreate()) 8329 throw new Error("Attempt to auto-create Claim.insurer"); 8330 else if (Configuration.doAutoCreate()) 8331 this.insurer = new Reference(); // cc 8332 return this.insurer; 8333 } 8334 8335 public boolean hasInsurer() { 8336 return this.insurer != null && !this.insurer.isEmpty(); 8337 } 8338 8339 /** 8340 * @param value {@link #insurer} (The Insurer who is target of the request.) 8341 */ 8342 public Claim setInsurer(Reference value) { 8343 this.insurer = value; 8344 return this; 8345 } 8346 8347 /** 8348 * @return {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 8349 */ 8350 public Reference getProvider() { 8351 if (this.provider == null) 8352 if (Configuration.errorOnAutoCreate()) 8353 throw new Error("Attempt to auto-create Claim.provider"); 8354 else if (Configuration.doAutoCreate()) 8355 this.provider = new Reference(); // cc 8356 return this.provider; 8357 } 8358 8359 public boolean hasProvider() { 8360 return this.provider != null && !this.provider.isEmpty(); 8361 } 8362 8363 /** 8364 * @param value {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 8365 */ 8366 public Claim setProvider(Reference value) { 8367 this.provider = value; 8368 return this; 8369 } 8370 8371 /** 8372 * @return {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.) 8373 */ 8374 public CodeableConcept getPriority() { 8375 if (this.priority == null) 8376 if (Configuration.errorOnAutoCreate()) 8377 throw new Error("Attempt to auto-create Claim.priority"); 8378 else if (Configuration.doAutoCreate()) 8379 this.priority = new CodeableConcept(); // cc 8380 return this.priority; 8381 } 8382 8383 public boolean hasPriority() { 8384 return this.priority != null && !this.priority.isEmpty(); 8385 } 8386 8387 /** 8388 * @param value {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.) 8389 */ 8390 public Claim setPriority(CodeableConcept value) { 8391 this.priority = value; 8392 return this; 8393 } 8394 8395 /** 8396 * @return {@link #fundsReserve} (A code to indicate whether and for whom funds are to be reserved for future claims.) 8397 */ 8398 public CodeableConcept getFundsReserve() { 8399 if (this.fundsReserve == null) 8400 if (Configuration.errorOnAutoCreate()) 8401 throw new Error("Attempt to auto-create Claim.fundsReserve"); 8402 else if (Configuration.doAutoCreate()) 8403 this.fundsReserve = new CodeableConcept(); // cc 8404 return this.fundsReserve; 8405 } 8406 8407 public boolean hasFundsReserve() { 8408 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 8409 } 8410 8411 /** 8412 * @param value {@link #fundsReserve} (A code to indicate whether and for whom funds are to be reserved for future claims.) 8413 */ 8414 public Claim setFundsReserve(CodeableConcept value) { 8415 this.fundsReserve = value; 8416 return this; 8417 } 8418 8419 /** 8420 * @return {@link #related} (Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.) 8421 */ 8422 public List<RelatedClaimComponent> getRelated() { 8423 if (this.related == null) 8424 this.related = new ArrayList<RelatedClaimComponent>(); 8425 return this.related; 8426 } 8427 8428 /** 8429 * @return Returns a reference to <code>this</code> for easy method chaining 8430 */ 8431 public Claim setRelated(List<RelatedClaimComponent> theRelated) { 8432 this.related = theRelated; 8433 return this; 8434 } 8435 8436 public boolean hasRelated() { 8437 if (this.related == null) 8438 return false; 8439 for (RelatedClaimComponent item : this.related) 8440 if (!item.isEmpty()) 8441 return true; 8442 return false; 8443 } 8444 8445 public RelatedClaimComponent addRelated() { //3 8446 RelatedClaimComponent t = new RelatedClaimComponent(); 8447 if (this.related == null) 8448 this.related = new ArrayList<RelatedClaimComponent>(); 8449 this.related.add(t); 8450 return t; 8451 } 8452 8453 public Claim addRelated(RelatedClaimComponent t) { //3 8454 if (t == null) 8455 return this; 8456 if (this.related == null) 8457 this.related = new ArrayList<RelatedClaimComponent>(); 8458 this.related.add(t); 8459 return this; 8460 } 8461 8462 /** 8463 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist {3} 8464 */ 8465 public RelatedClaimComponent getRelatedFirstRep() { 8466 if (getRelated().isEmpty()) { 8467 addRelated(); 8468 } 8469 return getRelated().get(0); 8470 } 8471 8472 /** 8473 * @return {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 8474 */ 8475 public Reference getPrescription() { 8476 if (this.prescription == null) 8477 if (Configuration.errorOnAutoCreate()) 8478 throw new Error("Attempt to auto-create Claim.prescription"); 8479 else if (Configuration.doAutoCreate()) 8480 this.prescription = new Reference(); // cc 8481 return this.prescription; 8482 } 8483 8484 public boolean hasPrescription() { 8485 return this.prescription != null && !this.prescription.isEmpty(); 8486 } 8487 8488 /** 8489 * @param value {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 8490 */ 8491 public Claim setPrescription(Reference value) { 8492 this.prescription = value; 8493 return this; 8494 } 8495 8496 /** 8497 * @return {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 8498 */ 8499 public Reference getOriginalPrescription() { 8500 if (this.originalPrescription == null) 8501 if (Configuration.errorOnAutoCreate()) 8502 throw new Error("Attempt to auto-create Claim.originalPrescription"); 8503 else if (Configuration.doAutoCreate()) 8504 this.originalPrescription = new Reference(); // cc 8505 return this.originalPrescription; 8506 } 8507 8508 public boolean hasOriginalPrescription() { 8509 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 8510 } 8511 8512 /** 8513 * @param value {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 8514 */ 8515 public Claim setOriginalPrescription(Reference value) { 8516 this.originalPrescription = value; 8517 return this; 8518 } 8519 8520 /** 8521 * @return {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 8522 */ 8523 public PayeeComponent getPayee() { 8524 if (this.payee == null) 8525 if (Configuration.errorOnAutoCreate()) 8526 throw new Error("Attempt to auto-create Claim.payee"); 8527 else if (Configuration.doAutoCreate()) 8528 this.payee = new PayeeComponent(); // cc 8529 return this.payee; 8530 } 8531 8532 public boolean hasPayee() { 8533 return this.payee != null && !this.payee.isEmpty(); 8534 } 8535 8536 /** 8537 * @param value {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 8538 */ 8539 public Claim setPayee(PayeeComponent value) { 8540 this.payee = value; 8541 return this; 8542 } 8543 8544 /** 8545 * @return {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 8546 */ 8547 public Reference getReferral() { 8548 if (this.referral == null) 8549 if (Configuration.errorOnAutoCreate()) 8550 throw new Error("Attempt to auto-create Claim.referral"); 8551 else if (Configuration.doAutoCreate()) 8552 this.referral = new Reference(); // cc 8553 return this.referral; 8554 } 8555 8556 public boolean hasReferral() { 8557 return this.referral != null && !this.referral.isEmpty(); 8558 } 8559 8560 /** 8561 * @param value {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 8562 */ 8563 public Claim setReferral(Reference value) { 8564 this.referral = value; 8565 return this; 8566 } 8567 8568 /** 8569 * @return {@link #encounter} (Healthcare encounters related to this claim.) 8570 */ 8571 public List<Reference> getEncounter() { 8572 if (this.encounter == null) 8573 this.encounter = new ArrayList<Reference>(); 8574 return this.encounter; 8575 } 8576 8577 /** 8578 * @return Returns a reference to <code>this</code> for easy method chaining 8579 */ 8580 public Claim setEncounter(List<Reference> theEncounter) { 8581 this.encounter = theEncounter; 8582 return this; 8583 } 8584 8585 public boolean hasEncounter() { 8586 if (this.encounter == null) 8587 return false; 8588 for (Reference item : this.encounter) 8589 if (!item.isEmpty()) 8590 return true; 8591 return false; 8592 } 8593 8594 public Reference addEncounter() { //3 8595 Reference t = new Reference(); 8596 if (this.encounter == null) 8597 this.encounter = new ArrayList<Reference>(); 8598 this.encounter.add(t); 8599 return t; 8600 } 8601 8602 public Claim addEncounter(Reference t) { //3 8603 if (t == null) 8604 return this; 8605 if (this.encounter == null) 8606 this.encounter = new ArrayList<Reference>(); 8607 this.encounter.add(t); 8608 return this; 8609 } 8610 8611 /** 8612 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 8613 */ 8614 public Reference getEncounterFirstRep() { 8615 if (getEncounter().isEmpty()) { 8616 addEncounter(); 8617 } 8618 return getEncounter().get(0); 8619 } 8620 8621 /** 8622 * @return {@link #facility} (Facility where the services were provided.) 8623 */ 8624 public Reference getFacility() { 8625 if (this.facility == null) 8626 if (Configuration.errorOnAutoCreate()) 8627 throw new Error("Attempt to auto-create Claim.facility"); 8628 else if (Configuration.doAutoCreate()) 8629 this.facility = new Reference(); // cc 8630 return this.facility; 8631 } 8632 8633 public boolean hasFacility() { 8634 return this.facility != null && !this.facility.isEmpty(); 8635 } 8636 8637 /** 8638 * @param value {@link #facility} (Facility where the services were provided.) 8639 */ 8640 public Claim setFacility(Reference value) { 8641 this.facility = value; 8642 return this; 8643 } 8644 8645 /** 8646 * @return {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 8647 */ 8648 public CodeableConcept getDiagnosisRelatedGroup() { 8649 if (this.diagnosisRelatedGroup == null) 8650 if (Configuration.errorOnAutoCreate()) 8651 throw new Error("Attempt to auto-create Claim.diagnosisRelatedGroup"); 8652 else if (Configuration.doAutoCreate()) 8653 this.diagnosisRelatedGroup = new CodeableConcept(); // cc 8654 return this.diagnosisRelatedGroup; 8655 } 8656 8657 public boolean hasDiagnosisRelatedGroup() { 8658 return this.diagnosisRelatedGroup != null && !this.diagnosisRelatedGroup.isEmpty(); 8659 } 8660 8661 /** 8662 * @param value {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 8663 */ 8664 public Claim setDiagnosisRelatedGroup(CodeableConcept value) { 8665 this.diagnosisRelatedGroup = value; 8666 return this; 8667 } 8668 8669 /** 8670 * @return {@link #event} (Information code for an event with a corresponding date or period.) 8671 */ 8672 public List<ClaimEventComponent> getEvent() { 8673 if (this.event == null) 8674 this.event = new ArrayList<ClaimEventComponent>(); 8675 return this.event; 8676 } 8677 8678 /** 8679 * @return Returns a reference to <code>this</code> for easy method chaining 8680 */ 8681 public Claim setEvent(List<ClaimEventComponent> theEvent) { 8682 this.event = theEvent; 8683 return this; 8684 } 8685 8686 public boolean hasEvent() { 8687 if (this.event == null) 8688 return false; 8689 for (ClaimEventComponent item : this.event) 8690 if (!item.isEmpty()) 8691 return true; 8692 return false; 8693 } 8694 8695 public ClaimEventComponent addEvent() { //3 8696 ClaimEventComponent t = new ClaimEventComponent(); 8697 if (this.event == null) 8698 this.event = new ArrayList<ClaimEventComponent>(); 8699 this.event.add(t); 8700 return t; 8701 } 8702 8703 public Claim addEvent(ClaimEventComponent t) { //3 8704 if (t == null) 8705 return this; 8706 if (this.event == null) 8707 this.event = new ArrayList<ClaimEventComponent>(); 8708 this.event.add(t); 8709 return this; 8710 } 8711 8712 /** 8713 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 8714 */ 8715 public ClaimEventComponent getEventFirstRep() { 8716 if (getEvent().isEmpty()) { 8717 addEvent(); 8718 } 8719 return getEvent().get(0); 8720 } 8721 8722 /** 8723 * @return {@link #careTeam} (The members of the team who provided the products and services.) 8724 */ 8725 public List<CareTeamComponent> getCareTeam() { 8726 if (this.careTeam == null) 8727 this.careTeam = new ArrayList<CareTeamComponent>(); 8728 return this.careTeam; 8729 } 8730 8731 /** 8732 * @return Returns a reference to <code>this</code> for easy method chaining 8733 */ 8734 public Claim setCareTeam(List<CareTeamComponent> theCareTeam) { 8735 this.careTeam = theCareTeam; 8736 return this; 8737 } 8738 8739 public boolean hasCareTeam() { 8740 if (this.careTeam == null) 8741 return false; 8742 for (CareTeamComponent item : this.careTeam) 8743 if (!item.isEmpty()) 8744 return true; 8745 return false; 8746 } 8747 8748 public CareTeamComponent addCareTeam() { //3 8749 CareTeamComponent t = new CareTeamComponent(); 8750 if (this.careTeam == null) 8751 this.careTeam = new ArrayList<CareTeamComponent>(); 8752 this.careTeam.add(t); 8753 return t; 8754 } 8755 8756 public Claim addCareTeam(CareTeamComponent t) { //3 8757 if (t == null) 8758 return this; 8759 if (this.careTeam == null) 8760 this.careTeam = new ArrayList<CareTeamComponent>(); 8761 this.careTeam.add(t); 8762 return this; 8763 } 8764 8765 /** 8766 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 8767 */ 8768 public CareTeamComponent getCareTeamFirstRep() { 8769 if (getCareTeam().isEmpty()) { 8770 addCareTeam(); 8771 } 8772 return getCareTeam().get(0); 8773 } 8774 8775 /** 8776 * @return {@link #supportingInfo} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.) 8777 */ 8778 public List<SupportingInformationComponent> getSupportingInfo() { 8779 if (this.supportingInfo == null) 8780 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 8781 return this.supportingInfo; 8782 } 8783 8784 /** 8785 * @return Returns a reference to <code>this</code> for easy method chaining 8786 */ 8787 public Claim setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 8788 this.supportingInfo = theSupportingInfo; 8789 return this; 8790 } 8791 8792 public boolean hasSupportingInfo() { 8793 if (this.supportingInfo == null) 8794 return false; 8795 for (SupportingInformationComponent item : this.supportingInfo) 8796 if (!item.isEmpty()) 8797 return true; 8798 return false; 8799 } 8800 8801 public SupportingInformationComponent addSupportingInfo() { //3 8802 SupportingInformationComponent t = new SupportingInformationComponent(); 8803 if (this.supportingInfo == null) 8804 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 8805 this.supportingInfo.add(t); 8806 return t; 8807 } 8808 8809 public Claim addSupportingInfo(SupportingInformationComponent t) { //3 8810 if (t == null) 8811 return this; 8812 if (this.supportingInfo == null) 8813 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 8814 this.supportingInfo.add(t); 8815 return this; 8816 } 8817 8818 /** 8819 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 8820 */ 8821 public SupportingInformationComponent getSupportingInfoFirstRep() { 8822 if (getSupportingInfo().isEmpty()) { 8823 addSupportingInfo(); 8824 } 8825 return getSupportingInfo().get(0); 8826 } 8827 8828 /** 8829 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim items.) 8830 */ 8831 public List<DiagnosisComponent> getDiagnosis() { 8832 if (this.diagnosis == null) 8833 this.diagnosis = new ArrayList<DiagnosisComponent>(); 8834 return this.diagnosis; 8835 } 8836 8837 /** 8838 * @return Returns a reference to <code>this</code> for easy method chaining 8839 */ 8840 public Claim setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 8841 this.diagnosis = theDiagnosis; 8842 return this; 8843 } 8844 8845 public boolean hasDiagnosis() { 8846 if (this.diagnosis == null) 8847 return false; 8848 for (DiagnosisComponent item : this.diagnosis) 8849 if (!item.isEmpty()) 8850 return true; 8851 return false; 8852 } 8853 8854 public DiagnosisComponent addDiagnosis() { //3 8855 DiagnosisComponent t = new DiagnosisComponent(); 8856 if (this.diagnosis == null) 8857 this.diagnosis = new ArrayList<DiagnosisComponent>(); 8858 this.diagnosis.add(t); 8859 return t; 8860 } 8861 8862 public Claim addDiagnosis(DiagnosisComponent t) { //3 8863 if (t == null) 8864 return this; 8865 if (this.diagnosis == null) 8866 this.diagnosis = new ArrayList<DiagnosisComponent>(); 8867 this.diagnosis.add(t); 8868 return this; 8869 } 8870 8871 /** 8872 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 8873 */ 8874 public DiagnosisComponent getDiagnosisFirstRep() { 8875 if (getDiagnosis().isEmpty()) { 8876 addDiagnosis(); 8877 } 8878 return getDiagnosis().get(0); 8879 } 8880 8881 /** 8882 * @return {@link #procedure} (Procedures performed on the patient relevant to the billing items with the claim.) 8883 */ 8884 public List<ProcedureComponent> getProcedure() { 8885 if (this.procedure == null) 8886 this.procedure = new ArrayList<ProcedureComponent>(); 8887 return this.procedure; 8888 } 8889 8890 /** 8891 * @return Returns a reference to <code>this</code> for easy method chaining 8892 */ 8893 public Claim setProcedure(List<ProcedureComponent> theProcedure) { 8894 this.procedure = theProcedure; 8895 return this; 8896 } 8897 8898 public boolean hasProcedure() { 8899 if (this.procedure == null) 8900 return false; 8901 for (ProcedureComponent item : this.procedure) 8902 if (!item.isEmpty()) 8903 return true; 8904 return false; 8905 } 8906 8907 public ProcedureComponent addProcedure() { //3 8908 ProcedureComponent t = new ProcedureComponent(); 8909 if (this.procedure == null) 8910 this.procedure = new ArrayList<ProcedureComponent>(); 8911 this.procedure.add(t); 8912 return t; 8913 } 8914 8915 public Claim addProcedure(ProcedureComponent t) { //3 8916 if (t == null) 8917 return this; 8918 if (this.procedure == null) 8919 this.procedure = new ArrayList<ProcedureComponent>(); 8920 this.procedure.add(t); 8921 return this; 8922 } 8923 8924 /** 8925 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 8926 */ 8927 public ProcedureComponent getProcedureFirstRep() { 8928 if (getProcedure().isEmpty()) { 8929 addProcedure(); 8930 } 8931 return getProcedure().get(0); 8932 } 8933 8934 /** 8935 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services specified on the claim.) 8936 */ 8937 public List<InsuranceComponent> getInsurance() { 8938 if (this.insurance == null) 8939 this.insurance = new ArrayList<InsuranceComponent>(); 8940 return this.insurance; 8941 } 8942 8943 /** 8944 * @return Returns a reference to <code>this</code> for easy method chaining 8945 */ 8946 public Claim setInsurance(List<InsuranceComponent> theInsurance) { 8947 this.insurance = theInsurance; 8948 return this; 8949 } 8950 8951 public boolean hasInsurance() { 8952 if (this.insurance == null) 8953 return false; 8954 for (InsuranceComponent item : this.insurance) 8955 if (!item.isEmpty()) 8956 return true; 8957 return false; 8958 } 8959 8960 public InsuranceComponent addInsurance() { //3 8961 InsuranceComponent t = new InsuranceComponent(); 8962 if (this.insurance == null) 8963 this.insurance = new ArrayList<InsuranceComponent>(); 8964 this.insurance.add(t); 8965 return t; 8966 } 8967 8968 public Claim addInsurance(InsuranceComponent t) { //3 8969 if (t == null) 8970 return this; 8971 if (this.insurance == null) 8972 this.insurance = new ArrayList<InsuranceComponent>(); 8973 this.insurance.add(t); 8974 return this; 8975 } 8976 8977 /** 8978 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 8979 */ 8980 public InsuranceComponent getInsuranceFirstRep() { 8981 if (getInsurance().isEmpty()) { 8982 addInsurance(); 8983 } 8984 return getInsurance().get(0); 8985 } 8986 8987 /** 8988 * @return {@link #accident} (Details of an accident which resulted in injuries which required the products and services listed in the claim.) 8989 */ 8990 public AccidentComponent getAccident() { 8991 if (this.accident == null) 8992 if (Configuration.errorOnAutoCreate()) 8993 throw new Error("Attempt to auto-create Claim.accident"); 8994 else if (Configuration.doAutoCreate()) 8995 this.accident = new AccidentComponent(); // cc 8996 return this.accident; 8997 } 8998 8999 public boolean hasAccident() { 9000 return this.accident != null && !this.accident.isEmpty(); 9001 } 9002 9003 /** 9004 * @param value {@link #accident} (Details of an accident which resulted in injuries which required the products and services listed in the claim.) 9005 */ 9006 public Claim setAccident(AccidentComponent value) { 9007 this.accident = value; 9008 return this; 9009 } 9010 9011 /** 9012 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 9013 */ 9014 public Money getPatientPaid() { 9015 if (this.patientPaid == null) 9016 if (Configuration.errorOnAutoCreate()) 9017 throw new Error("Attempt to auto-create Claim.patientPaid"); 9018 else if (Configuration.doAutoCreate()) 9019 this.patientPaid = new Money(); // cc 9020 return this.patientPaid; 9021 } 9022 9023 public boolean hasPatientPaid() { 9024 return this.patientPaid != null && !this.patientPaid.isEmpty(); 9025 } 9026 9027 /** 9028 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 9029 */ 9030 public Claim setPatientPaid(Money value) { 9031 this.patientPaid = value; 9032 return this; 9033 } 9034 9035 /** 9036 * @return {@link #item} (A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.) 9037 */ 9038 public List<ItemComponent> getItem() { 9039 if (this.item == null) 9040 this.item = new ArrayList<ItemComponent>(); 9041 return this.item; 9042 } 9043 9044 /** 9045 * @return Returns a reference to <code>this</code> for easy method chaining 9046 */ 9047 public Claim setItem(List<ItemComponent> theItem) { 9048 this.item = theItem; 9049 return this; 9050 } 9051 9052 public boolean hasItem() { 9053 if (this.item == null) 9054 return false; 9055 for (ItemComponent item : this.item) 9056 if (!item.isEmpty()) 9057 return true; 9058 return false; 9059 } 9060 9061 public ItemComponent addItem() { //3 9062 ItemComponent t = new ItemComponent(); 9063 if (this.item == null) 9064 this.item = new ArrayList<ItemComponent>(); 9065 this.item.add(t); 9066 return t; 9067 } 9068 9069 public Claim addItem(ItemComponent t) { //3 9070 if (t == null) 9071 return this; 9072 if (this.item == null) 9073 this.item = new ArrayList<ItemComponent>(); 9074 this.item.add(t); 9075 return this; 9076 } 9077 9078 /** 9079 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 9080 */ 9081 public ItemComponent getItemFirstRep() { 9082 if (getItem().isEmpty()) { 9083 addItem(); 9084 } 9085 return getItem().get(0); 9086 } 9087 9088 /** 9089 * @return {@link #total} (The total value of the all the items in the claim.) 9090 */ 9091 public Money getTotal() { 9092 if (this.total == null) 9093 if (Configuration.errorOnAutoCreate()) 9094 throw new Error("Attempt to auto-create Claim.total"); 9095 else if (Configuration.doAutoCreate()) 9096 this.total = new Money(); // cc 9097 return this.total; 9098 } 9099 9100 public boolean hasTotal() { 9101 return this.total != null && !this.total.isEmpty(); 9102 } 9103 9104 /** 9105 * @param value {@link #total} (The total value of the all the items in the claim.) 9106 */ 9107 public Claim setTotal(Money value) { 9108 this.total = value; 9109 return this; 9110 } 9111 9112 protected void listChildren(List<Property> children) { 9113 super.listChildren(children); 9114 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, java.lang.Integer.MAX_VALUE, identifier)); 9115 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 9116 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 9117 children.add(new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 9118 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType)); 9119 children.add(new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use)); 9120 children.add(new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 0, 1, patient)); 9121 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod)); 9122 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 9123 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 9124 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 9125 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 9126 children.add(new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.", 0, 1, priority)); 9127 children.add(new Property("fundsReserve", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve)); 9128 children.add(new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related)); 9129 children.add(new Property("prescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription)); 9130 children.add(new Property("originalPrescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription)); 9131 children.add(new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee)); 9132 children.add(new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral)); 9133 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 9134 children.add(new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility)); 9135 children.add(new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup)); 9136 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 9137 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 9138 children.add(new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 9139 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 9140 children.add(new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure)); 9141 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance)); 9142 children.add(new Property("accident", "", "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident)); 9143 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 9144 children.add(new Property("item", "", "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item)); 9145 children.add(new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, total)); 9146 } 9147 9148 @Override 9149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9150 switch (_hash) { 9151 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, java.lang.Integer.MAX_VALUE, identifier); 9152 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 9153 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 9154 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 9155 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType); 9156 case 116103: /*use*/ return new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use); 9157 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 0, 1, patient); 9158 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod); 9159 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 9160 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 9161 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 9162 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 9163 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.", 0, 1, priority); 9164 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve); 9165 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related); 9166 case 460301338: /*prescription*/ return new Property("prescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription); 9167 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription); 9168 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee); 9169 case -722568291: /*referral*/ return new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral); 9170 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 9171 case 501116579: /*facility*/ return new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility); 9172 case -1599182171: /*diagnosisRelatedGroup*/ return new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup); 9173 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 9174 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 9175 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 9176 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 9177 case -1095204141: /*procedure*/ return new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure); 9178 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance); 9179 case -2143202801: /*accident*/ return new Property("accident", "", "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident); 9180 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 9181 case 3242771: /*item*/ return new Property("item", "", "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item); 9182 case 110549828: /*total*/ return new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, total); 9183 default: return super.getNamedProperty(_hash, _name, _checkValid); 9184 } 9185 9186 } 9187 9188 @Override 9189 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9190 switch (hash) { 9191 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 9192 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 9193 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 9194 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 9195 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 9196 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 9197 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 9198 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 9199 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 9200 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 9201 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 9202 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 9203 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 9204 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 9205 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 9206 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 9207 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 9208 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 9209 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 9210 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 9211 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 9212 case -1599182171: /*diagnosisRelatedGroup*/ return this.diagnosisRelatedGroup == null ? new Base[0] : new Base[] {this.diagnosisRelatedGroup}; // CodeableConcept 9213 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // ClaimEventComponent 9214 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 9215 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 9216 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 9217 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 9218 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 9219 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 9220 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 9221 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 9222 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // Money 9223 default: return super.getProperty(hash, name, checkValid); 9224 } 9225 9226 } 9227 9228 @Override 9229 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9230 switch (hash) { 9231 case -1618432855: // identifier 9232 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 9233 return value; 9234 case 82505966: // traceNumber 9235 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 9236 return value; 9237 case -892481550: // status 9238 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 9239 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 9240 return value; 9241 case 3575610: // type 9242 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9243 return value; 9244 case -1868521062: // subType 9245 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9246 return value; 9247 case 116103: // use 9248 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 9249 this.use = (Enumeration) value; // Enumeration<Use> 9250 return value; 9251 case -791418107: // patient 9252 this.patient = TypeConvertor.castToReference(value); // Reference 9253 return value; 9254 case -332066046: // billablePeriod 9255 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 9256 return value; 9257 case 1028554472: // created 9258 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 9259 return value; 9260 case -1591951995: // enterer 9261 this.enterer = TypeConvertor.castToReference(value); // Reference 9262 return value; 9263 case 1957615864: // insurer 9264 this.insurer = TypeConvertor.castToReference(value); // Reference 9265 return value; 9266 case -987494927: // provider 9267 this.provider = TypeConvertor.castToReference(value); // Reference 9268 return value; 9269 case -1165461084: // priority 9270 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9271 return value; 9272 case 1314609806: // fundsReserve 9273 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9274 return value; 9275 case 1090493483: // related 9276 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 9277 return value; 9278 case 460301338: // prescription 9279 this.prescription = TypeConvertor.castToReference(value); // Reference 9280 return value; 9281 case -1814015861: // originalPrescription 9282 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 9283 return value; 9284 case 106443592: // payee 9285 this.payee = (PayeeComponent) value; // PayeeComponent 9286 return value; 9287 case -722568291: // referral 9288 this.referral = TypeConvertor.castToReference(value); // Reference 9289 return value; 9290 case 1524132147: // encounter 9291 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 9292 return value; 9293 case 501116579: // facility 9294 this.facility = TypeConvertor.castToReference(value); // Reference 9295 return value; 9296 case -1599182171: // diagnosisRelatedGroup 9297 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9298 return value; 9299 case 96891546: // event 9300 this.getEvent().add((ClaimEventComponent) value); // ClaimEventComponent 9301 return value; 9302 case -7323378: // careTeam 9303 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 9304 return value; 9305 case 1922406657: // supportingInfo 9306 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 9307 return value; 9308 case 1196993265: // diagnosis 9309 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 9310 return value; 9311 case -1095204141: // procedure 9312 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 9313 return value; 9314 case 73049818: // insurance 9315 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 9316 return value; 9317 case -2143202801: // accident 9318 this.accident = (AccidentComponent) value; // AccidentComponent 9319 return value; 9320 case 525514609: // patientPaid 9321 this.patientPaid = TypeConvertor.castToMoney(value); // Money 9322 return value; 9323 case 3242771: // item 9324 this.getItem().add((ItemComponent) value); // ItemComponent 9325 return value; 9326 case 110549828: // total 9327 this.total = TypeConvertor.castToMoney(value); // Money 9328 return value; 9329 default: return super.setProperty(hash, name, value); 9330 } 9331 9332 } 9333 9334 @Override 9335 public Base setProperty(String name, Base value) throws FHIRException { 9336 if (name.equals("identifier")) { 9337 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 9338 } else if (name.equals("traceNumber")) { 9339 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 9340 } else if (name.equals("status")) { 9341 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 9342 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 9343 } else if (name.equals("type")) { 9344 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9345 } else if (name.equals("subType")) { 9346 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9347 } else if (name.equals("use")) { 9348 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 9349 this.use = (Enumeration) value; // Enumeration<Use> 9350 } else if (name.equals("patient")) { 9351 this.patient = TypeConvertor.castToReference(value); // Reference 9352 } else if (name.equals("billablePeriod")) { 9353 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 9354 } else if (name.equals("created")) { 9355 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 9356 } else if (name.equals("enterer")) { 9357 this.enterer = TypeConvertor.castToReference(value); // Reference 9358 } else if (name.equals("insurer")) { 9359 this.insurer = TypeConvertor.castToReference(value); // Reference 9360 } else if (name.equals("provider")) { 9361 this.provider = TypeConvertor.castToReference(value); // Reference 9362 } else if (name.equals("priority")) { 9363 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9364 } else if (name.equals("fundsReserve")) { 9365 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9366 } else if (name.equals("related")) { 9367 this.getRelated().add((RelatedClaimComponent) value); 9368 } else if (name.equals("prescription")) { 9369 this.prescription = TypeConvertor.castToReference(value); // Reference 9370 } else if (name.equals("originalPrescription")) { 9371 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 9372 } else if (name.equals("payee")) { 9373 this.payee = (PayeeComponent) value; // PayeeComponent 9374 } else if (name.equals("referral")) { 9375 this.referral = TypeConvertor.castToReference(value); // Reference 9376 } else if (name.equals("encounter")) { 9377 this.getEncounter().add(TypeConvertor.castToReference(value)); 9378 } else if (name.equals("facility")) { 9379 this.facility = TypeConvertor.castToReference(value); // Reference 9380 } else if (name.equals("diagnosisRelatedGroup")) { 9381 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9382 } else if (name.equals("event")) { 9383 this.getEvent().add((ClaimEventComponent) value); 9384 } else if (name.equals("careTeam")) { 9385 this.getCareTeam().add((CareTeamComponent) value); 9386 } else if (name.equals("supportingInfo")) { 9387 this.getSupportingInfo().add((SupportingInformationComponent) value); 9388 } else if (name.equals("diagnosis")) { 9389 this.getDiagnosis().add((DiagnosisComponent) value); 9390 } else if (name.equals("procedure")) { 9391 this.getProcedure().add((ProcedureComponent) value); 9392 } else if (name.equals("insurance")) { 9393 this.getInsurance().add((InsuranceComponent) value); 9394 } else if (name.equals("accident")) { 9395 this.accident = (AccidentComponent) value; // AccidentComponent 9396 } else if (name.equals("patientPaid")) { 9397 this.patientPaid = TypeConvertor.castToMoney(value); // Money 9398 } else if (name.equals("item")) { 9399 this.getItem().add((ItemComponent) value); 9400 } else if (name.equals("total")) { 9401 this.total = TypeConvertor.castToMoney(value); // Money 9402 } else 9403 return super.setProperty(name, value); 9404 return value; 9405 } 9406 9407 @Override 9408 public Base makeProperty(int hash, String name) throws FHIRException { 9409 switch (hash) { 9410 case -1618432855: return addIdentifier(); 9411 case 82505966: return addTraceNumber(); 9412 case -892481550: return getStatusElement(); 9413 case 3575610: return getType(); 9414 case -1868521062: return getSubType(); 9415 case 116103: return getUseElement(); 9416 case -791418107: return getPatient(); 9417 case -332066046: return getBillablePeriod(); 9418 case 1028554472: return getCreatedElement(); 9419 case -1591951995: return getEnterer(); 9420 case 1957615864: return getInsurer(); 9421 case -987494927: return getProvider(); 9422 case -1165461084: return getPriority(); 9423 case 1314609806: return getFundsReserve(); 9424 case 1090493483: return addRelated(); 9425 case 460301338: return getPrescription(); 9426 case -1814015861: return getOriginalPrescription(); 9427 case 106443592: return getPayee(); 9428 case -722568291: return getReferral(); 9429 case 1524132147: return addEncounter(); 9430 case 501116579: return getFacility(); 9431 case -1599182171: return getDiagnosisRelatedGroup(); 9432 case 96891546: return addEvent(); 9433 case -7323378: return addCareTeam(); 9434 case 1922406657: return addSupportingInfo(); 9435 case 1196993265: return addDiagnosis(); 9436 case -1095204141: return addProcedure(); 9437 case 73049818: return addInsurance(); 9438 case -2143202801: return getAccident(); 9439 case 525514609: return getPatientPaid(); 9440 case 3242771: return addItem(); 9441 case 110549828: return getTotal(); 9442 default: return super.makeProperty(hash, name); 9443 } 9444 9445 } 9446 9447 @Override 9448 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9449 switch (hash) { 9450 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 9451 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 9452 case -892481550: /*status*/ return new String[] {"code"}; 9453 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 9454 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 9455 case 116103: /*use*/ return new String[] {"code"}; 9456 case -791418107: /*patient*/ return new String[] {"Reference"}; 9457 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 9458 case 1028554472: /*created*/ return new String[] {"dateTime"}; 9459 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 9460 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 9461 case -987494927: /*provider*/ return new String[] {"Reference"}; 9462 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 9463 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 9464 case 1090493483: /*related*/ return new String[] {}; 9465 case 460301338: /*prescription*/ return new String[] {"Reference"}; 9466 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 9467 case 106443592: /*payee*/ return new String[] {}; 9468 case -722568291: /*referral*/ return new String[] {"Reference"}; 9469 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 9470 case 501116579: /*facility*/ return new String[] {"Reference"}; 9471 case -1599182171: /*diagnosisRelatedGroup*/ return new String[] {"CodeableConcept"}; 9472 case 96891546: /*event*/ return new String[] {}; 9473 case -7323378: /*careTeam*/ return new String[] {}; 9474 case 1922406657: /*supportingInfo*/ return new String[] {}; 9475 case 1196993265: /*diagnosis*/ return new String[] {}; 9476 case -1095204141: /*procedure*/ return new String[] {}; 9477 case 73049818: /*insurance*/ return new String[] {}; 9478 case -2143202801: /*accident*/ return new String[] {}; 9479 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 9480 case 3242771: /*item*/ return new String[] {}; 9481 case 110549828: /*total*/ return new String[] {"Money"}; 9482 default: return super.getTypesForProperty(hash, name); 9483 } 9484 9485 } 9486 9487 @Override 9488 public Base addChild(String name) throws FHIRException { 9489 if (name.equals("identifier")) { 9490 return addIdentifier(); 9491 } 9492 else if (name.equals("traceNumber")) { 9493 return addTraceNumber(); 9494 } 9495 else if (name.equals("status")) { 9496 throw new FHIRException("Cannot call addChild on a singleton property Claim.status"); 9497 } 9498 else if (name.equals("type")) { 9499 this.type = new CodeableConcept(); 9500 return this.type; 9501 } 9502 else if (name.equals("subType")) { 9503 this.subType = new CodeableConcept(); 9504 return this.subType; 9505 } 9506 else if (name.equals("use")) { 9507 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 9508 } 9509 else if (name.equals("patient")) { 9510 this.patient = new Reference(); 9511 return this.patient; 9512 } 9513 else if (name.equals("billablePeriod")) { 9514 this.billablePeriod = new Period(); 9515 return this.billablePeriod; 9516 } 9517 else if (name.equals("created")) { 9518 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 9519 } 9520 else if (name.equals("enterer")) { 9521 this.enterer = new Reference(); 9522 return this.enterer; 9523 } 9524 else if (name.equals("insurer")) { 9525 this.insurer = new Reference(); 9526 return this.insurer; 9527 } 9528 else if (name.equals("provider")) { 9529 this.provider = new Reference(); 9530 return this.provider; 9531 } 9532 else if (name.equals("priority")) { 9533 this.priority = new CodeableConcept(); 9534 return this.priority; 9535 } 9536 else if (name.equals("fundsReserve")) { 9537 this.fundsReserve = new CodeableConcept(); 9538 return this.fundsReserve; 9539 } 9540 else if (name.equals("related")) { 9541 return addRelated(); 9542 } 9543 else if (name.equals("prescription")) { 9544 this.prescription = new Reference(); 9545 return this.prescription; 9546 } 9547 else if (name.equals("originalPrescription")) { 9548 this.originalPrescription = new Reference(); 9549 return this.originalPrescription; 9550 } 9551 else if (name.equals("payee")) { 9552 this.payee = new PayeeComponent(); 9553 return this.payee; 9554 } 9555 else if (name.equals("referral")) { 9556 this.referral = new Reference(); 9557 return this.referral; 9558 } 9559 else if (name.equals("encounter")) { 9560 return addEncounter(); 9561 } 9562 else if (name.equals("facility")) { 9563 this.facility = new Reference(); 9564 return this.facility; 9565 } 9566 else if (name.equals("diagnosisRelatedGroup")) { 9567 this.diagnosisRelatedGroup = new CodeableConcept(); 9568 return this.diagnosisRelatedGroup; 9569 } 9570 else if (name.equals("event")) { 9571 return addEvent(); 9572 } 9573 else if (name.equals("careTeam")) { 9574 return addCareTeam(); 9575 } 9576 else if (name.equals("supportingInfo")) { 9577 return addSupportingInfo(); 9578 } 9579 else if (name.equals("diagnosis")) { 9580 return addDiagnosis(); 9581 } 9582 else if (name.equals("procedure")) { 9583 return addProcedure(); 9584 } 9585 else if (name.equals("insurance")) { 9586 return addInsurance(); 9587 } 9588 else if (name.equals("accident")) { 9589 this.accident = new AccidentComponent(); 9590 return this.accident; 9591 } 9592 else if (name.equals("patientPaid")) { 9593 this.patientPaid = new Money(); 9594 return this.patientPaid; 9595 } 9596 else if (name.equals("item")) { 9597 return addItem(); 9598 } 9599 else if (name.equals("total")) { 9600 this.total = new Money(); 9601 return this.total; 9602 } 9603 else 9604 return super.addChild(name); 9605 } 9606 9607 public String fhirType() { 9608 return "Claim"; 9609 9610 } 9611 9612 public Claim copy() { 9613 Claim dst = new Claim(); 9614 copyValues(dst); 9615 return dst; 9616 } 9617 9618 public void copyValues(Claim dst) { 9619 super.copyValues(dst); 9620 if (identifier != null) { 9621 dst.identifier = new ArrayList<Identifier>(); 9622 for (Identifier i : identifier) 9623 dst.identifier.add(i.copy()); 9624 }; 9625 if (traceNumber != null) { 9626 dst.traceNumber = new ArrayList<Identifier>(); 9627 for (Identifier i : traceNumber) 9628 dst.traceNumber.add(i.copy()); 9629 }; 9630 dst.status = status == null ? null : status.copy(); 9631 dst.type = type == null ? null : type.copy(); 9632 dst.subType = subType == null ? null : subType.copy(); 9633 dst.use = use == null ? null : use.copy(); 9634 dst.patient = patient == null ? null : patient.copy(); 9635 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 9636 dst.created = created == null ? null : created.copy(); 9637 dst.enterer = enterer == null ? null : enterer.copy(); 9638 dst.insurer = insurer == null ? null : insurer.copy(); 9639 dst.provider = provider == null ? null : provider.copy(); 9640 dst.priority = priority == null ? null : priority.copy(); 9641 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 9642 if (related != null) { 9643 dst.related = new ArrayList<RelatedClaimComponent>(); 9644 for (RelatedClaimComponent i : related) 9645 dst.related.add(i.copy()); 9646 }; 9647 dst.prescription = prescription == null ? null : prescription.copy(); 9648 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 9649 dst.payee = payee == null ? null : payee.copy(); 9650 dst.referral = referral == null ? null : referral.copy(); 9651 if (encounter != null) { 9652 dst.encounter = new ArrayList<Reference>(); 9653 for (Reference i : encounter) 9654 dst.encounter.add(i.copy()); 9655 }; 9656 dst.facility = facility == null ? null : facility.copy(); 9657 dst.diagnosisRelatedGroup = diagnosisRelatedGroup == null ? null : diagnosisRelatedGroup.copy(); 9658 if (event != null) { 9659 dst.event = new ArrayList<ClaimEventComponent>(); 9660 for (ClaimEventComponent i : event) 9661 dst.event.add(i.copy()); 9662 }; 9663 if (careTeam != null) { 9664 dst.careTeam = new ArrayList<CareTeamComponent>(); 9665 for (CareTeamComponent i : careTeam) 9666 dst.careTeam.add(i.copy()); 9667 }; 9668 if (supportingInfo != null) { 9669 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9670 for (SupportingInformationComponent i : supportingInfo) 9671 dst.supportingInfo.add(i.copy()); 9672 }; 9673 if (diagnosis != null) { 9674 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 9675 for (DiagnosisComponent i : diagnosis) 9676 dst.diagnosis.add(i.copy()); 9677 }; 9678 if (procedure != null) { 9679 dst.procedure = new ArrayList<ProcedureComponent>(); 9680 for (ProcedureComponent i : procedure) 9681 dst.procedure.add(i.copy()); 9682 }; 9683 if (insurance != null) { 9684 dst.insurance = new ArrayList<InsuranceComponent>(); 9685 for (InsuranceComponent i : insurance) 9686 dst.insurance.add(i.copy()); 9687 }; 9688 dst.accident = accident == null ? null : accident.copy(); 9689 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 9690 if (item != null) { 9691 dst.item = new ArrayList<ItemComponent>(); 9692 for (ItemComponent i : item) 9693 dst.item.add(i.copy()); 9694 }; 9695 dst.total = total == null ? null : total.copy(); 9696 } 9697 9698 protected Claim typedCopy() { 9699 return copy(); 9700 } 9701 9702 @Override 9703 public boolean equalsDeep(Base other_) { 9704 if (!super.equalsDeep(other_)) 9705 return false; 9706 if (!(other_ instanceof Claim)) 9707 return false; 9708 Claim o = (Claim) other_; 9709 return compareDeep(identifier, o.identifier, true) && compareDeep(traceNumber, o.traceNumber, true) 9710 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 9711 && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 9712 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) 9713 && compareDeep(provider, o.provider, true) && compareDeep(priority, o.priority, true) && compareDeep(fundsReserve, o.fundsReserve, true) 9714 && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) && compareDeep(originalPrescription, o.originalPrescription, true) 9715 && compareDeep(payee, o.payee, true) && compareDeep(referral, o.referral, true) && compareDeep(encounter, o.encounter, true) 9716 && compareDeep(facility, o.facility, true) && compareDeep(diagnosisRelatedGroup, o.diagnosisRelatedGroup, true) 9717 && compareDeep(event, o.event, true) && compareDeep(careTeam, o.careTeam, true) && compareDeep(supportingInfo, o.supportingInfo, true) 9718 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) && compareDeep(insurance, o.insurance, true) 9719 && compareDeep(accident, o.accident, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(item, o.item, true) 9720 && compareDeep(total, o.total, true); 9721 } 9722 9723 @Override 9724 public boolean equalsShallow(Base other_) { 9725 if (!super.equalsShallow(other_)) 9726 return false; 9727 if (!(other_ instanceof Claim)) 9728 return false; 9729 Claim o = (Claim) other_; 9730 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 9731 ; 9732 } 9733 9734 public boolean isEmpty() { 9735 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, traceNumber, status 9736 , type, subType, use, patient, billablePeriod, created, enterer, insurer, provider 9737 , priority, fundsReserve, related, prescription, originalPrescription, payee, referral 9738 , encounter, facility, diagnosisRelatedGroup, event, careTeam, supportingInfo, diagnosis 9739 , procedure, insurance, accident, patientPaid, item, total); 9740 } 9741 9742 @Override 9743 public ResourceType getResourceType() { 9744 return ResourceType.Claim; 9745 } 9746 9747 /** 9748 * Search parameter: <b>care-team</b> 9749 * <p> 9750 * Description: <b>Member of the CareTeam</b><br> 9751 * Type: <b>reference</b><br> 9752 * Path: <b>Claim.careTeam.provider</b><br> 9753 * </p> 9754 */ 9755 @SearchParamDefinition(name="care-team", path="Claim.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 9756 public static final String SP_CARE_TEAM = "care-team"; 9757 /** 9758 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 9759 * <p> 9760 * Description: <b>Member of the CareTeam</b><br> 9761 * Type: <b>reference</b><br> 9762 * Path: <b>Claim.careTeam.provider</b><br> 9763 * </p> 9764 */ 9765 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 9766 9767/** 9768 * Constant for fluent queries to be used to add include statements. Specifies 9769 * the path value of "<b>Claim:care-team</b>". 9770 */ 9771 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("Claim:care-team").toLocked(); 9772 9773 /** 9774 * Search parameter: <b>created</b> 9775 * <p> 9776 * Description: <b>The creation date for the Claim</b><br> 9777 * Type: <b>date</b><br> 9778 * Path: <b>Claim.created</b><br> 9779 * </p> 9780 */ 9781 @SearchParamDefinition(name="created", path="Claim.created", description="The creation date for the Claim", type="date" ) 9782 public static final String SP_CREATED = "created"; 9783 /** 9784 * <b>Fluent Client</b> search parameter constant for <b>created</b> 9785 * <p> 9786 * Description: <b>The creation date for the Claim</b><br> 9787 * Type: <b>date</b><br> 9788 * Path: <b>Claim.created</b><br> 9789 * </p> 9790 */ 9791 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 9792 9793 /** 9794 * Search parameter: <b>detail-udi</b> 9795 * <p> 9796 * Description: <b>UDI associated with a line item, detail product or service</b><br> 9797 * Type: <b>reference</b><br> 9798 * Path: <b>Claim.item.detail.udi</b><br> 9799 * </p> 9800 */ 9801 @SearchParamDefinition(name="detail-udi", path="Claim.item.detail.udi", description="UDI associated with a line item, detail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 9802 public static final String SP_DETAIL_UDI = "detail-udi"; 9803 /** 9804 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 9805 * <p> 9806 * Description: <b>UDI associated with a line item, detail product or service</b><br> 9807 * Type: <b>reference</b><br> 9808 * Path: <b>Claim.item.detail.udi</b><br> 9809 * </p> 9810 */ 9811 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DETAIL_UDI); 9812 9813/** 9814 * Constant for fluent queries to be used to add include statements. Specifies 9815 * the path value of "<b>Claim:detail-udi</b>". 9816 */ 9817 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include("Claim:detail-udi").toLocked(); 9818 9819 /** 9820 * Search parameter: <b>enterer</b> 9821 * <p> 9822 * Description: <b>The party responsible for the entry of the Claim</b><br> 9823 * Type: <b>reference</b><br> 9824 * Path: <b>Claim.enterer</b><br> 9825 * </p> 9826 */ 9827 @SearchParamDefinition(name="enterer", path="Claim.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 9828 public static final String SP_ENTERER = "enterer"; 9829 /** 9830 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 9831 * <p> 9832 * Description: <b>The party responsible for the entry of the Claim</b><br> 9833 * Type: <b>reference</b><br> 9834 * Path: <b>Claim.enterer</b><br> 9835 * </p> 9836 */ 9837 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 9838 9839/** 9840 * Constant for fluent queries to be used to add include statements. Specifies 9841 * the path value of "<b>Claim:enterer</b>". 9842 */ 9843 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("Claim:enterer").toLocked(); 9844 9845 /** 9846 * Search parameter: <b>facility</b> 9847 * <p> 9848 * Description: <b>Facility where the products or services have been or will be provided</b><br> 9849 * Type: <b>reference</b><br> 9850 * Path: <b>Claim.facility</b><br> 9851 * </p> 9852 */ 9853 @SearchParamDefinition(name="facility", path="Claim.facility", description="Facility where the products or services have been or will be provided", type="reference", target={Location.class, Organization.class } ) 9854 public static final String SP_FACILITY = "facility"; 9855 /** 9856 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 9857 * <p> 9858 * Description: <b>Facility where the products or services have been or will be provided</b><br> 9859 * Type: <b>reference</b><br> 9860 * Path: <b>Claim.facility</b><br> 9861 * </p> 9862 */ 9863 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 9864 9865/** 9866 * Constant for fluent queries to be used to add include statements. Specifies 9867 * the path value of "<b>Claim:facility</b>". 9868 */ 9869 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("Claim:facility").toLocked(); 9870 9871 /** 9872 * Search parameter: <b>insurer</b> 9873 * <p> 9874 * Description: <b>The target payor/insurer for the Claim</b><br> 9875 * Type: <b>reference</b><br> 9876 * Path: <b>Claim.insurer</b><br> 9877 * </p> 9878 */ 9879 @SearchParamDefinition(name="insurer", path="Claim.insurer", description="The target payor/insurer for the Claim", type="reference", target={Organization.class } ) 9880 public static final String SP_INSURER = "insurer"; 9881 /** 9882 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 9883 * <p> 9884 * Description: <b>The target payor/insurer for the Claim</b><br> 9885 * Type: <b>reference</b><br> 9886 * Path: <b>Claim.insurer</b><br> 9887 * </p> 9888 */ 9889 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 9890 9891/** 9892 * Constant for fluent queries to be used to add include statements. Specifies 9893 * the path value of "<b>Claim:insurer</b>". 9894 */ 9895 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Claim:insurer").toLocked(); 9896 9897 /** 9898 * Search parameter: <b>item-udi</b> 9899 * <p> 9900 * Description: <b>UDI associated with a line item product or service</b><br> 9901 * Type: <b>reference</b><br> 9902 * Path: <b>Claim.item.udi</b><br> 9903 * </p> 9904 */ 9905 @SearchParamDefinition(name="item-udi", path="Claim.item.udi", description="UDI associated with a line item product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 9906 public static final String SP_ITEM_UDI = "item-udi"; 9907 /** 9908 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 9909 * <p> 9910 * Description: <b>UDI associated with a line item product or service</b><br> 9911 * Type: <b>reference</b><br> 9912 * Path: <b>Claim.item.udi</b><br> 9913 * </p> 9914 */ 9915 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_UDI); 9916 9917/** 9918 * Constant for fluent queries to be used to add include statements. Specifies 9919 * the path value of "<b>Claim:item-udi</b>". 9920 */ 9921 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include("Claim:item-udi").toLocked(); 9922 9923 /** 9924 * Search parameter: <b>payee</b> 9925 * <p> 9926 * Description: <b>The party receiving any payment for the Claim</b><br> 9927 * Type: <b>reference</b><br> 9928 * Path: <b>Claim.payee.party</b><br> 9929 * </p> 9930 */ 9931 @SearchParamDefinition(name="payee", path="Claim.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 9932 public static final String SP_PAYEE = "payee"; 9933 /** 9934 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 9935 * <p> 9936 * Description: <b>The party receiving any payment for the Claim</b><br> 9937 * Type: <b>reference</b><br> 9938 * Path: <b>Claim.payee.party</b><br> 9939 * </p> 9940 */ 9941 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 9942 9943/** 9944 * Constant for fluent queries to be used to add include statements. Specifies 9945 * the path value of "<b>Claim:payee</b>". 9946 */ 9947 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("Claim:payee").toLocked(); 9948 9949 /** 9950 * Search parameter: <b>priority</b> 9951 * <p> 9952 * Description: <b>Processing priority requested</b><br> 9953 * Type: <b>token</b><br> 9954 * Path: <b>Claim.priority</b><br> 9955 * </p> 9956 */ 9957 @SearchParamDefinition(name="priority", path="Claim.priority", description="Processing priority requested", type="token" ) 9958 public static final String SP_PRIORITY = "priority"; 9959 /** 9960 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 9961 * <p> 9962 * Description: <b>Processing priority requested</b><br> 9963 * Type: <b>token</b><br> 9964 * Path: <b>Claim.priority</b><br> 9965 * </p> 9966 */ 9967 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 9968 9969 /** 9970 * Search parameter: <b>procedure-udi</b> 9971 * <p> 9972 * Description: <b>UDI associated with a procedure</b><br> 9973 * Type: <b>reference</b><br> 9974 * Path: <b>Claim.procedure.udi</b><br> 9975 * </p> 9976 */ 9977 @SearchParamDefinition(name="procedure-udi", path="Claim.procedure.udi", description="UDI associated with a procedure", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 9978 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 9979 /** 9980 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 9981 * <p> 9982 * Description: <b>UDI associated with a procedure</b><br> 9983 * Type: <b>reference</b><br> 9984 * Path: <b>Claim.procedure.udi</b><br> 9985 * </p> 9986 */ 9987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROCEDURE_UDI); 9988 9989/** 9990 * Constant for fluent queries to be used to add include statements. Specifies 9991 * the path value of "<b>Claim:procedure-udi</b>". 9992 */ 9993 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include("Claim:procedure-udi").toLocked(); 9994 9995 /** 9996 * Search parameter: <b>provider</b> 9997 * <p> 9998 * Description: <b>Provider responsible for the Claim</b><br> 9999 * Type: <b>reference</b><br> 10000 * Path: <b>Claim.provider</b><br> 10001 * </p> 10002 */ 10003 @SearchParamDefinition(name="provider", path="Claim.provider", description="Provider responsible for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 10004 public static final String SP_PROVIDER = "provider"; 10005 /** 10006 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 10007 * <p> 10008 * Description: <b>Provider responsible for the Claim</b><br> 10009 * Type: <b>reference</b><br> 10010 * Path: <b>Claim.provider</b><br> 10011 * </p> 10012 */ 10013 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 10014 10015/** 10016 * Constant for fluent queries to be used to add include statements. Specifies 10017 * the path value of "<b>Claim:provider</b>". 10018 */ 10019 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("Claim:provider").toLocked(); 10020 10021 /** 10022 * Search parameter: <b>status</b> 10023 * <p> 10024 * Description: <b>The status of the Claim instance.</b><br> 10025 * Type: <b>token</b><br> 10026 * Path: <b>Claim.status</b><br> 10027 * </p> 10028 */ 10029 @SearchParamDefinition(name="status", path="Claim.status", description="The status of the Claim instance.", type="token" ) 10030 public static final String SP_STATUS = "status"; 10031 /** 10032 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10033 * <p> 10034 * Description: <b>The status of the Claim instance.</b><br> 10035 * Type: <b>token</b><br> 10036 * Path: <b>Claim.status</b><br> 10037 * </p> 10038 */ 10039 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 10040 10041 /** 10042 * Search parameter: <b>subdetail-udi</b> 10043 * <p> 10044 * Description: <b>UDI associated with a line item, detail, subdetail product or service</b><br> 10045 * Type: <b>reference</b><br> 10046 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 10047 * </p> 10048 */ 10049 @SearchParamDefinition(name="subdetail-udi", path="Claim.item.detail.subDetail.udi", description="UDI associated with a line item, detail, subdetail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 10050 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 10051 /** 10052 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 10053 * <p> 10054 * Description: <b>UDI associated with a line item, detail, subdetail product or service</b><br> 10055 * Type: <b>reference</b><br> 10056 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 10057 * </p> 10058 */ 10059 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBDETAIL_UDI); 10060 10061/** 10062 * Constant for fluent queries to be used to add include statements. Specifies 10063 * the path value of "<b>Claim:subdetail-udi</b>". 10064 */ 10065 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include("Claim:subdetail-udi").toLocked(); 10066 10067 /** 10068 * Search parameter: <b>use</b> 10069 * <p> 10070 * Description: <b>The kind of financial resource</b><br> 10071 * Type: <b>token</b><br> 10072 * Path: <b>Claim.use</b><br> 10073 * </p> 10074 */ 10075 @SearchParamDefinition(name="use", path="Claim.use", description="The kind of financial resource", type="token" ) 10076 public static final String SP_USE = "use"; 10077 /** 10078 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10079 * <p> 10080 * Description: <b>The kind of financial resource</b><br> 10081 * Type: <b>token</b><br> 10082 * Path: <b>Claim.use</b><br> 10083 * </p> 10084 */ 10085 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_USE); 10086 10087 /** 10088 * Search parameter: <b>encounter</b> 10089 * <p> 10090 * Description: <b>Multiple Resources: 10091 10092* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 10093* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 10094* [ChargeItem](chargeitem.html): Encounter associated with event 10095* [Claim](claim.html): Encounters associated with a billed line item 10096* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 10097* [Communication](communication.html): The Encounter during which this Communication was created 10098* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 10099* [Composition](composition.html): Context of the Composition 10100* [Condition](condition.html): The Encounter during which this Condition was created 10101* [DeviceRequest](devicerequest.html): Encounter during which request was created 10102* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 10103* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 10104* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 10105* [Flag](flag.html): Alert relevant during encounter 10106* [ImagingStudy](imagingstudy.html): The context of the study 10107* [List](list.html): Context in which list created 10108* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 10109* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 10110* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 10111* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 10112* [Observation](observation.html): Encounter related to the observation 10113* [Procedure](procedure.html): The Encounter during which this Procedure was created 10114* [Provenance](provenance.html): Encounter related to the Provenance 10115* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 10116* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 10117* [RiskAssessment](riskassessment.html): Where was assessment performed? 10118* [ServiceRequest](servicerequest.html): An encounter in which this request is made 10119* [Task](task.html): Search by encounter 10120* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 10121</b><br> 10122 * Type: <b>reference</b><br> 10123 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 10124 * </p> 10125 */ 10126 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 10127 public static final String SP_ENCOUNTER = "encounter"; 10128 /** 10129 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 10130 * <p> 10131 * Description: <b>Multiple Resources: 10132 10133* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 10134* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 10135* [ChargeItem](chargeitem.html): Encounter associated with event 10136* [Claim](claim.html): Encounters associated with a billed line item 10137* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 10138* [Communication](communication.html): The Encounter during which this Communication was created 10139* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 10140* [Composition](composition.html): Context of the Composition 10141* [Condition](condition.html): The Encounter during which this Condition was created 10142* [DeviceRequest](devicerequest.html): Encounter during which request was created 10143* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 10144* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 10145* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 10146* [Flag](flag.html): Alert relevant during encounter 10147* [ImagingStudy](imagingstudy.html): The context of the study 10148* [List](list.html): Context in which list created 10149* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 10150* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 10151* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 10152* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 10153* [Observation](observation.html): Encounter related to the observation 10154* [Procedure](procedure.html): The Encounter during which this Procedure was created 10155* [Provenance](provenance.html): Encounter related to the Provenance 10156* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 10157* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 10158* [RiskAssessment](riskassessment.html): Where was assessment performed? 10159* [ServiceRequest](servicerequest.html): An encounter in which this request is made 10160* [Task](task.html): Search by encounter 10161* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 10162</b><br> 10163 * Type: <b>reference</b><br> 10164 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 10165 * </p> 10166 */ 10167 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 10168 10169/** 10170 * Constant for fluent queries to be used to add include statements. Specifies 10171 * the path value of "<b>Claim:encounter</b>". 10172 */ 10173 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Claim:encounter").toLocked(); 10174 10175 /** 10176 * Search parameter: <b>identifier</b> 10177 * <p> 10178 * Description: <b>Multiple Resources: 10179 10180* [Account](account.html): Account number 10181* [AdverseEvent](adverseevent.html): Business identifier for the event 10182* [AllergyIntolerance](allergyintolerance.html): External ids for this item 10183* [Appointment](appointment.html): An Identifier of the Appointment 10184* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 10185* [Basic](basic.html): Business identifier 10186* [BodyStructure](bodystructure.html): Bodystructure identifier 10187* [CarePlan](careplan.html): External Ids for this plan 10188* [CareTeam](careteam.html): External Ids for this team 10189* [ChargeItem](chargeitem.html): Business Identifier for item 10190* [Claim](claim.html): The primary identifier of the financial resource 10191* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 10192* [ClinicalImpression](clinicalimpression.html): Business identifier 10193* [Communication](communication.html): Unique identifier 10194* [CommunicationRequest](communicationrequest.html): Unique identifier 10195* [Composition](composition.html): Version-independent identifier for the Composition 10196* [Condition](condition.html): A unique identifier of the condition record 10197* [Consent](consent.html): Identifier for this record (external references) 10198* [Contract](contract.html): The identity of the contract 10199* [Coverage](coverage.html): The primary identifier of the insured and the coverage 10200* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 10201* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 10202* [DetectedIssue](detectedissue.html): Unique id for the detected issue 10203* [DeviceRequest](devicerequest.html): Business identifier for request/order 10204* [DeviceUsage](deviceusage.html): Search by identifier 10205* [DiagnosticReport](diagnosticreport.html): An identifier for the report 10206* [DocumentReference](documentreference.html): Identifier of the attachment binary 10207* [Encounter](encounter.html): Identifier(s) by which this encounter is known 10208* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 10209* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 10210* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 10211* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 10212* [Flag](flag.html): Business identifier 10213* [Goal](goal.html): External Ids for this goal 10214* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 10215* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 10216* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 10217* [Immunization](immunization.html): Business identifier 10218* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 10219* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 10220* [Invoice](invoice.html): Business Identifier for item 10221* [List](list.html): Business identifier 10222* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 10223* [Medication](medication.html): Returns medications with this external identifier 10224* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 10225* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 10226* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 10227* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 10228* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 10229* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 10230* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 10231* [Observation](observation.html): The unique id for a particular observation 10232* [Person](person.html): A person Identifier 10233* [Procedure](procedure.html): A unique identifier for a procedure 10234* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 10235* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 10236* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 10237* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 10238* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 10239* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 10240* [Specimen](specimen.html): The unique identifier associated with the specimen 10241* [SupplyDelivery](supplydelivery.html): External identifier 10242* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 10243* [Task](task.html): Search for a task instance by its business identifier 10244* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 10245</b><br> 10246 * Type: <b>token</b><br> 10247 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 10248 * </p> 10249 */ 10250 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 10251 public static final String SP_IDENTIFIER = "identifier"; 10252 /** 10253 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10254 * <p> 10255 * Description: <b>Multiple Resources: 10256 10257* [Account](account.html): Account number 10258* [AdverseEvent](adverseevent.html): Business identifier for the event 10259* [AllergyIntolerance](allergyintolerance.html): External ids for this item 10260* [Appointment](appointment.html): An Identifier of the Appointment 10261* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 10262* [Basic](basic.html): Business identifier 10263* [BodyStructure](bodystructure.html): Bodystructure identifier 10264* [CarePlan](careplan.html): External Ids for this plan 10265* [CareTeam](careteam.html): External Ids for this team 10266* [ChargeItem](chargeitem.html): Business Identifier for item 10267* [Claim](claim.html): The primary identifier of the financial resource 10268* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 10269* [ClinicalImpression](clinicalimpression.html): Business identifier 10270* [Communication](communication.html): Unique identifier 10271* [CommunicationRequest](communicationrequest.html): Unique identifier 10272* [Composition](composition.html): Version-independent identifier for the Composition 10273* [Condition](condition.html): A unique identifier of the condition record 10274* [Consent](consent.html): Identifier for this record (external references) 10275* [Contract](contract.html): The identity of the contract 10276* [Coverage](coverage.html): The primary identifier of the insured and the coverage 10277* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 10278* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 10279* [DetectedIssue](detectedissue.html): Unique id for the detected issue 10280* [DeviceRequest](devicerequest.html): Business identifier for request/order 10281* [DeviceUsage](deviceusage.html): Search by identifier 10282* [DiagnosticReport](diagnosticreport.html): An identifier for the report 10283* [DocumentReference](documentreference.html): Identifier of the attachment binary 10284* [Encounter](encounter.html): Identifier(s) by which this encounter is known 10285* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 10286* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 10287* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 10288* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 10289* [Flag](flag.html): Business identifier 10290* [Goal](goal.html): External Ids for this goal 10291* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 10292* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 10293* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 10294* [Immunization](immunization.html): Business identifier 10295* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 10296* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 10297* [Invoice](invoice.html): Business Identifier for item 10298* [List](list.html): Business identifier 10299* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 10300* [Medication](medication.html): Returns medications with this external identifier 10301* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 10302* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 10303* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 10304* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 10305* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 10306* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 10307* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 10308* [Observation](observation.html): The unique id for a particular observation 10309* [Person](person.html): A person Identifier 10310* [Procedure](procedure.html): A unique identifier for a procedure 10311* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 10312* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 10313* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 10314* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 10315* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 10316* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 10317* [Specimen](specimen.html): The unique identifier associated with the specimen 10318* [SupplyDelivery](supplydelivery.html): External identifier 10319* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 10320* [Task](task.html): Search for a task instance by its business identifier 10321* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 10322</b><br> 10323 * Type: <b>token</b><br> 10324 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 10325 * </p> 10326 */ 10327 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 10328 10329 /** 10330 * Search parameter: <b>patient</b> 10331 * <p> 10332 * Description: <b>Multiple Resources: 10333 10334* [Account](account.html): The entity that caused the expenses 10335* [AdverseEvent](adverseevent.html): Subject impacted by event 10336* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 10337* [Appointment](appointment.html): One of the individuals of the appointment is this patient 10338* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 10339* [AuditEvent](auditevent.html): Where the activity involved patient data 10340* [Basic](basic.html): Identifies the focus of this resource 10341* [BodyStructure](bodystructure.html): Who this is about 10342* [CarePlan](careplan.html): Who the care plan is for 10343* [CareTeam](careteam.html): Who care team is for 10344* [ChargeItem](chargeitem.html): Individual service was done for/to 10345* [Claim](claim.html): Patient receiving the products or services 10346* [ClaimResponse](claimresponse.html): The subject of care 10347* [ClinicalImpression](clinicalimpression.html): Patient assessed 10348* [Communication](communication.html): Focus of message 10349* [CommunicationRequest](communicationrequest.html): Focus of message 10350* [Composition](composition.html): Who and/or what the composition is about 10351* [Condition](condition.html): Who has the condition? 10352* [Consent](consent.html): Who the consent applies to 10353* [Contract](contract.html): The identity of the subject of the contract (if a patient) 10354* [Coverage](coverage.html): Retrieve coverages for a patient 10355* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 10356* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 10357* [DetectedIssue](detectedissue.html): Associated patient 10358* [DeviceRequest](devicerequest.html): Individual the service is ordered for 10359* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 10360* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 10361* [DocumentReference](documentreference.html): Who/what is the subject of the document 10362* [Encounter](encounter.html): The patient present at the encounter 10363* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 10364* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 10365* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 10366* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 10367* [Flag](flag.html): The identity of a subject to list flags for 10368* [Goal](goal.html): Who this goal is intended for 10369* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 10370* [ImagingSelection](imagingselection.html): Who the study is about 10371* [ImagingStudy](imagingstudy.html): Who the study is about 10372* [Immunization](immunization.html): The patient for the vaccination record 10373* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 10374* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 10375* [Invoice](invoice.html): Recipient(s) of goods and services 10376* [List](list.html): If all resources have the same subject 10377* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 10378* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 10379* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 10380* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 10381* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 10382* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 10383* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 10384* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 10385* [Observation](observation.html): The subject that the observation is about (if patient) 10386* [Person](person.html): The Person links to this Patient 10387* [Procedure](procedure.html): Search by subject - a patient 10388* [Provenance](provenance.html): Where the activity involved patient data 10389* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 10390* [RelatedPerson](relatedperson.html): The patient this related person is related to 10391* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 10392* [ResearchSubject](researchsubject.html): Who or what is part of study 10393* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 10394* [ServiceRequest](servicerequest.html): Search by subject - a patient 10395* [Specimen](specimen.html): The patient the specimen comes from 10396* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 10397* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 10398* [Task](task.html): Search by patient 10399* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 10400</b><br> 10401 * Type: <b>reference</b><br> 10402 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 10403 * </p> 10404 */ 10405 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 10406 public static final String SP_PATIENT = "patient"; 10407 /** 10408 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 10409 * <p> 10410 * Description: <b>Multiple Resources: 10411 10412* [Account](account.html): The entity that caused the expenses 10413* [AdverseEvent](adverseevent.html): Subject impacted by event 10414* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 10415* [Appointment](appointment.html): One of the individuals of the appointment is this patient 10416* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 10417* [AuditEvent](auditevent.html): Where the activity involved patient data 10418* [Basic](basic.html): Identifies the focus of this resource 10419* [BodyStructure](bodystructure.html): Who this is about 10420* [CarePlan](careplan.html): Who the care plan is for 10421* [CareTeam](careteam.html): Who care team is for 10422* [ChargeItem](chargeitem.html): Individual service was done for/to 10423* [Claim](claim.html): Patient receiving the products or services 10424* [ClaimResponse](claimresponse.html): The subject of care 10425* [ClinicalImpression](clinicalimpression.html): Patient assessed 10426* [Communication](communication.html): Focus of message 10427* [CommunicationRequest](communicationrequest.html): Focus of message 10428* [Composition](composition.html): Who and/or what the composition is about 10429* [Condition](condition.html): Who has the condition? 10430* [Consent](consent.html): Who the consent applies to 10431* [Contract](contract.html): The identity of the subject of the contract (if a patient) 10432* [Coverage](coverage.html): Retrieve coverages for a patient 10433* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 10434* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 10435* [DetectedIssue](detectedissue.html): Associated patient 10436* [DeviceRequest](devicerequest.html): Individual the service is ordered for 10437* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 10438* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 10439* [DocumentReference](documentreference.html): Who/what is the subject of the document 10440* [Encounter](encounter.html): The patient present at the encounter 10441* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 10442* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 10443* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 10444* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 10445* [Flag](flag.html): The identity of a subject to list flags for 10446* [Goal](goal.html): Who this goal is intended for 10447* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 10448* [ImagingSelection](imagingselection.html): Who the study is about 10449* [ImagingStudy](imagingstudy.html): Who the study is about 10450* [Immunization](immunization.html): The patient for the vaccination record 10451* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 10452* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 10453* [Invoice](invoice.html): Recipient(s) of goods and services 10454* [List](list.html): If all resources have the same subject 10455* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 10456* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 10457* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 10458* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 10459* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 10460* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 10461* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 10462* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 10463* [Observation](observation.html): The subject that the observation is about (if patient) 10464* [Person](person.html): The Person links to this Patient 10465* [Procedure](procedure.html): Search by subject - a patient 10466* [Provenance](provenance.html): Where the activity involved patient data 10467* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 10468* [RelatedPerson](relatedperson.html): The patient this related person is related to 10469* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 10470* [ResearchSubject](researchsubject.html): Who or what is part of study 10471* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 10472* [ServiceRequest](servicerequest.html): Search by subject - a patient 10473* [Specimen](specimen.html): The patient the specimen comes from 10474* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 10475* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 10476* [Task](task.html): Search by patient 10477* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 10478</b><br> 10479 * Type: <b>reference</b><br> 10480 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 10481 * </p> 10482 */ 10483 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 10484 10485/** 10486 * Constant for fluent queries to be used to add include statements. Specifies 10487 * the path value of "<b>Claim:patient</b>". 10488 */ 10489 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Claim:patient").toLocked(); 10490 10491 10492} 10493