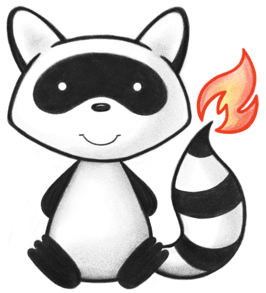
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement. 053 */ 054@ResourceDef(name="Claim", profile="http://hl7.org/fhir/StructureDefinition/Claim") 055public class Claim extends DomainResource { 056 057 @Block() 058 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * Reference to a related claim. 061 */ 062 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 063 @Description(shortDefinition="Reference to the related claim", formalDefinition="Reference to a related claim." ) 064 protected Reference claim; 065 066 /** 067 * A code to convey how the claims are related. 068 */ 069 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="How the reference claim is related", formalDefinition="A code to convey how the claims are related." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 072 protected CodeableConcept relationship; 073 074 /** 075 * An alternate organizational reference to the case or file to which this particular claim pertains. 076 */ 077 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 078 @Description(shortDefinition="File or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains." ) 079 protected Identifier reference; 080 081 private static final long serialVersionUID = 1047077926L; 082 083 /** 084 * Constructor 085 */ 086 public RelatedClaimComponent() { 087 super(); 088 } 089 090 /** 091 * @return {@link #claim} (Reference to a related claim.) 092 */ 093 public Reference getClaim() { 094 if (this.claim == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 097 else if (Configuration.doAutoCreate()) 098 this.claim = new Reference(); // cc 099 return this.claim; 100 } 101 102 public boolean hasClaim() { 103 return this.claim != null && !this.claim.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #claim} (Reference to a related claim.) 108 */ 109 public RelatedClaimComponent setClaim(Reference value) { 110 this.claim = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #relationship} (A code to convey how the claims are related.) 116 */ 117 public CodeableConcept getRelationship() { 118 if (this.relationship == null) 119 if (Configuration.errorOnAutoCreate()) 120 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 121 else if (Configuration.doAutoCreate()) 122 this.relationship = new CodeableConcept(); // cc 123 return this.relationship; 124 } 125 126 public boolean hasRelationship() { 127 return this.relationship != null && !this.relationship.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #relationship} (A code to convey how the claims are related.) 132 */ 133 public RelatedClaimComponent setRelationship(CodeableConcept value) { 134 this.relationship = value; 135 return this; 136 } 137 138 /** 139 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 140 */ 141 public Identifier getReference() { 142 if (this.reference == null) 143 if (Configuration.errorOnAutoCreate()) 144 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 145 else if (Configuration.doAutoCreate()) 146 this.reference = new Identifier(); // cc 147 return this.reference; 148 } 149 150 public boolean hasReference() { 151 return this.reference != null && !this.reference.isEmpty(); 152 } 153 154 /** 155 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 156 */ 157 public RelatedClaimComponent setReference(Identifier value) { 158 this.reference = value; 159 return this; 160 } 161 162 protected void listChildren(List<Property> children) { 163 super.listChildren(children); 164 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 165 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship)); 166 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference)); 167 } 168 169 @Override 170 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 171 switch (_hash) { 172 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 173 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship); 174 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference); 175 default: return super.getNamedProperty(_hash, _name, _checkValid); 176 } 177 178 } 179 180 @Override 181 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 182 switch (hash) { 183 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 184 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 185 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 186 default: return super.getProperty(hash, name, checkValid); 187 } 188 189 } 190 191 @Override 192 public Base setProperty(int hash, String name, Base value) throws FHIRException { 193 switch (hash) { 194 case 94742588: // claim 195 this.claim = TypeConvertor.castToReference(value); // Reference 196 return value; 197 case -261851592: // relationship 198 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 199 return value; 200 case -925155509: // reference 201 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 202 return value; 203 default: return super.setProperty(hash, name, value); 204 } 205 206 } 207 208 @Override 209 public Base setProperty(String name, Base value) throws FHIRException { 210 if (name.equals("claim")) { 211 this.claim = TypeConvertor.castToReference(value); // Reference 212 } else if (name.equals("relationship")) { 213 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 214 } else if (name.equals("reference")) { 215 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 216 } else 217 return super.setProperty(name, value); 218 return value; 219 } 220 221 @Override 222 public void removeChild(String name, Base value) throws FHIRException { 223 if (name.equals("claim")) { 224 this.claim = null; 225 } else if (name.equals("relationship")) { 226 this.relationship = null; 227 } else if (name.equals("reference")) { 228 this.reference = null; 229 } else 230 super.removeChild(name, value); 231 232 } 233 234 @Override 235 public Base makeProperty(int hash, String name) throws FHIRException { 236 switch (hash) { 237 case 94742588: return getClaim(); 238 case -261851592: return getRelationship(); 239 case -925155509: return getReference(); 240 default: return super.makeProperty(hash, name); 241 } 242 243 } 244 245 @Override 246 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 247 switch (hash) { 248 case 94742588: /*claim*/ return new String[] {"Reference"}; 249 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 250 case -925155509: /*reference*/ return new String[] {"Identifier"}; 251 default: return super.getTypesForProperty(hash, name); 252 } 253 254 } 255 256 @Override 257 public Base addChild(String name) throws FHIRException { 258 if (name.equals("claim")) { 259 this.claim = new Reference(); 260 return this.claim; 261 } 262 else if (name.equals("relationship")) { 263 this.relationship = new CodeableConcept(); 264 return this.relationship; 265 } 266 else if (name.equals("reference")) { 267 this.reference = new Identifier(); 268 return this.reference; 269 } 270 else 271 return super.addChild(name); 272 } 273 274 public RelatedClaimComponent copy() { 275 RelatedClaimComponent dst = new RelatedClaimComponent(); 276 copyValues(dst); 277 return dst; 278 } 279 280 public void copyValues(RelatedClaimComponent dst) { 281 super.copyValues(dst); 282 dst.claim = claim == null ? null : claim.copy(); 283 dst.relationship = relationship == null ? null : relationship.copy(); 284 dst.reference = reference == null ? null : reference.copy(); 285 } 286 287 @Override 288 public boolean equalsDeep(Base other_) { 289 if (!super.equalsDeep(other_)) 290 return false; 291 if (!(other_ instanceof RelatedClaimComponent)) 292 return false; 293 RelatedClaimComponent o = (RelatedClaimComponent) other_; 294 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 295 ; 296 } 297 298 @Override 299 public boolean equalsShallow(Base other_) { 300 if (!super.equalsShallow(other_)) 301 return false; 302 if (!(other_ instanceof RelatedClaimComponent)) 303 return false; 304 RelatedClaimComponent o = (RelatedClaimComponent) other_; 305 return true; 306 } 307 308 public boolean isEmpty() { 309 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 310 ); 311 } 312 313 public String fhirType() { 314 return "Claim.related"; 315 316 } 317 318 } 319 320 @Block() 321 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 322 /** 323 * Type of Party to be reimbursed: subscriber, provider, other. 324 */ 325 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 326 @Description(shortDefinition="Category of recipient", formalDefinition="Type of Party to be reimbursed: subscriber, provider, other." ) 327 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 328 protected CodeableConcept type; 329 330 /** 331 * Reference to the individual or organization to whom any payment will be made. 332 */ 333 @Child(name = "party", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class}, order=2, min=0, max=1, modifier=false, summary=false) 334 @Description(shortDefinition="Recipient reference", formalDefinition="Reference to the individual or organization to whom any payment will be made." ) 335 protected Reference party; 336 337 private static final long serialVersionUID = -1948897146L; 338 339 /** 340 * Constructor 341 */ 342 public PayeeComponent() { 343 super(); 344 } 345 346 /** 347 * Constructor 348 */ 349 public PayeeComponent(CodeableConcept type) { 350 super(); 351 this.setType(type); 352 } 353 354 /** 355 * @return {@link #type} (Type of Party to be reimbursed: subscriber, provider, other.) 356 */ 357 public CodeableConcept getType() { 358 if (this.type == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create PayeeComponent.type"); 361 else if (Configuration.doAutoCreate()) 362 this.type = new CodeableConcept(); // cc 363 return this.type; 364 } 365 366 public boolean hasType() { 367 return this.type != null && !this.type.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #type} (Type of Party to be reimbursed: subscriber, provider, other.) 372 */ 373 public PayeeComponent setType(CodeableConcept value) { 374 this.type = value; 375 return this; 376 } 377 378 /** 379 * @return {@link #party} (Reference to the individual or organization to whom any payment will be made.) 380 */ 381 public Reference getParty() { 382 if (this.party == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create PayeeComponent.party"); 385 else if (Configuration.doAutoCreate()) 386 this.party = new Reference(); // cc 387 return this.party; 388 } 389 390 public boolean hasParty() { 391 return this.party != null && !this.party.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #party} (Reference to the individual or organization to whom any payment will be made.) 396 */ 397 public PayeeComponent setParty(Reference value) { 398 this.party = value; 399 return this; 400 } 401 402 protected void listChildren(List<Property> children) { 403 super.listChildren(children); 404 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type)); 405 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 406 } 407 408 @Override 409 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 410 switch (_hash) { 411 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, type); 412 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 413 default: return super.getNamedProperty(_hash, _name, _checkValid); 414 } 415 416 } 417 418 @Override 419 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 420 switch (hash) { 421 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 422 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 423 default: return super.getProperty(hash, name, checkValid); 424 } 425 426 } 427 428 @Override 429 public Base setProperty(int hash, String name, Base value) throws FHIRException { 430 switch (hash) { 431 case 3575610: // type 432 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 433 return value; 434 case 106437350: // party 435 this.party = TypeConvertor.castToReference(value); // Reference 436 return value; 437 default: return super.setProperty(hash, name, value); 438 } 439 440 } 441 442 @Override 443 public Base setProperty(String name, Base value) throws FHIRException { 444 if (name.equals("type")) { 445 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 446 } else if (name.equals("party")) { 447 this.party = TypeConvertor.castToReference(value); // Reference 448 } else 449 return super.setProperty(name, value); 450 return value; 451 } 452 453 @Override 454 public void removeChild(String name, Base value) throws FHIRException { 455 if (name.equals("type")) { 456 this.type = null; 457 } else if (name.equals("party")) { 458 this.party = null; 459 } else 460 super.removeChild(name, value); 461 462 } 463 464 @Override 465 public Base makeProperty(int hash, String name) throws FHIRException { 466 switch (hash) { 467 case 3575610: return getType(); 468 case 106437350: return getParty(); 469 default: return super.makeProperty(hash, name); 470 } 471 472 } 473 474 @Override 475 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 476 switch (hash) { 477 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 478 case 106437350: /*party*/ return new String[] {"Reference"}; 479 default: return super.getTypesForProperty(hash, name); 480 } 481 482 } 483 484 @Override 485 public Base addChild(String name) throws FHIRException { 486 if (name.equals("type")) { 487 this.type = new CodeableConcept(); 488 return this.type; 489 } 490 else if (name.equals("party")) { 491 this.party = new Reference(); 492 return this.party; 493 } 494 else 495 return super.addChild(name); 496 } 497 498 public PayeeComponent copy() { 499 PayeeComponent dst = new PayeeComponent(); 500 copyValues(dst); 501 return dst; 502 } 503 504 public void copyValues(PayeeComponent dst) { 505 super.copyValues(dst); 506 dst.type = type == null ? null : type.copy(); 507 dst.party = party == null ? null : party.copy(); 508 } 509 510 @Override 511 public boolean equalsDeep(Base other_) { 512 if (!super.equalsDeep(other_)) 513 return false; 514 if (!(other_ instanceof PayeeComponent)) 515 return false; 516 PayeeComponent o = (PayeeComponent) other_; 517 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 518 } 519 520 @Override 521 public boolean equalsShallow(Base other_) { 522 if (!super.equalsShallow(other_)) 523 return false; 524 if (!(other_ instanceof PayeeComponent)) 525 return false; 526 PayeeComponent o = (PayeeComponent) other_; 527 return true; 528 } 529 530 public boolean isEmpty() { 531 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 532 } 533 534 public String fhirType() { 535 return "Claim.payee"; 536 537 } 538 539 } 540 541 @Block() 542 public static class ClaimEventComponent extends BackboneElement implements IBaseBackboneElement { 543 /** 544 * A coded event such as when a service is expected or a card printed. 545 */ 546 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 547 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 548 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 549 protected CodeableConcept type; 550 551 /** 552 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 553 */ 554 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 555 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 556 protected DataType when; 557 558 private static final long serialVersionUID = -634897375L; 559 560 /** 561 * Constructor 562 */ 563 public ClaimEventComponent() { 564 super(); 565 } 566 567 /** 568 * Constructor 569 */ 570 public ClaimEventComponent(CodeableConcept type, DataType when) { 571 super(); 572 this.setType(type); 573 this.setWhen(when); 574 } 575 576 /** 577 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 578 */ 579 public CodeableConcept getType() { 580 if (this.type == null) 581 if (Configuration.errorOnAutoCreate()) 582 throw new Error("Attempt to auto-create ClaimEventComponent.type"); 583 else if (Configuration.doAutoCreate()) 584 this.type = new CodeableConcept(); // cc 585 return this.type; 586 } 587 588 public boolean hasType() { 589 return this.type != null && !this.type.isEmpty(); 590 } 591 592 /** 593 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 594 */ 595 public ClaimEventComponent setType(CodeableConcept value) { 596 this.type = value; 597 return this; 598 } 599 600 /** 601 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 602 */ 603 public DataType getWhen() { 604 return this.when; 605 } 606 607 /** 608 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 609 */ 610 public DateTimeType getWhenDateTimeType() throws FHIRException { 611 if (this.when == null) 612 this.when = new DateTimeType(); 613 if (!(this.when instanceof DateTimeType)) 614 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 615 return (DateTimeType) this.when; 616 } 617 618 public boolean hasWhenDateTimeType() { 619 return this != null && this.when instanceof DateTimeType; 620 } 621 622 /** 623 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 624 */ 625 public Period getWhenPeriod() throws FHIRException { 626 if (this.when == null) 627 this.when = new Period(); 628 if (!(this.when instanceof Period)) 629 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 630 return (Period) this.when; 631 } 632 633 public boolean hasWhenPeriod() { 634 return this != null && this.when instanceof Period; 635 } 636 637 public boolean hasWhen() { 638 return this.when != null && !this.when.isEmpty(); 639 } 640 641 /** 642 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 643 */ 644 public ClaimEventComponent setWhen(DataType value) { 645 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 646 throw new FHIRException("Not the right type for Claim.event.when[x]: "+value.fhirType()); 647 this.when = value; 648 return this; 649 } 650 651 protected void listChildren(List<Property> children) { 652 super.listChildren(children); 653 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 654 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 655 } 656 657 @Override 658 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 659 switch (_hash) { 660 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 661 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 662 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 663 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 664 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 665 default: return super.getNamedProperty(_hash, _name, _checkValid); 666 } 667 668 } 669 670 @Override 671 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 672 switch (hash) { 673 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 674 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 675 default: return super.getProperty(hash, name, checkValid); 676 } 677 678 } 679 680 @Override 681 public Base setProperty(int hash, String name, Base value) throws FHIRException { 682 switch (hash) { 683 case 3575610: // type 684 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 685 return value; 686 case 3648314: // when 687 this.when = TypeConvertor.castToType(value); // DataType 688 return value; 689 default: return super.setProperty(hash, name, value); 690 } 691 692 } 693 694 @Override 695 public Base setProperty(String name, Base value) throws FHIRException { 696 if (name.equals("type")) { 697 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 698 } else if (name.equals("when[x]")) { 699 this.when = TypeConvertor.castToType(value); // DataType 700 } else 701 return super.setProperty(name, value); 702 return value; 703 } 704 705 @Override 706 public void removeChild(String name, Base value) throws FHIRException { 707 if (name.equals("type")) { 708 this.type = null; 709 } else if (name.equals("when[x]")) { 710 this.when = null; 711 } else 712 super.removeChild(name, value); 713 714 } 715 716 @Override 717 public Base makeProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case 3575610: return getType(); 720 case 1312831238: return getWhen(); 721 case 3648314: return getWhen(); 722 default: return super.makeProperty(hash, name); 723 } 724 725 } 726 727 @Override 728 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 729 switch (hash) { 730 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 731 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 732 default: return super.getTypesForProperty(hash, name); 733 } 734 735 } 736 737 @Override 738 public Base addChild(String name) throws FHIRException { 739 if (name.equals("type")) { 740 this.type = new CodeableConcept(); 741 return this.type; 742 } 743 else if (name.equals("whenDateTime")) { 744 this.when = new DateTimeType(); 745 return this.when; 746 } 747 else if (name.equals("whenPeriod")) { 748 this.when = new Period(); 749 return this.when; 750 } 751 else 752 return super.addChild(name); 753 } 754 755 public ClaimEventComponent copy() { 756 ClaimEventComponent dst = new ClaimEventComponent(); 757 copyValues(dst); 758 return dst; 759 } 760 761 public void copyValues(ClaimEventComponent dst) { 762 super.copyValues(dst); 763 dst.type = type == null ? null : type.copy(); 764 dst.when = when == null ? null : when.copy(); 765 } 766 767 @Override 768 public boolean equalsDeep(Base other_) { 769 if (!super.equalsDeep(other_)) 770 return false; 771 if (!(other_ instanceof ClaimEventComponent)) 772 return false; 773 ClaimEventComponent o = (ClaimEventComponent) other_; 774 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 775 } 776 777 @Override 778 public boolean equalsShallow(Base other_) { 779 if (!super.equalsShallow(other_)) 780 return false; 781 if (!(other_ instanceof ClaimEventComponent)) 782 return false; 783 ClaimEventComponent o = (ClaimEventComponent) other_; 784 return true; 785 } 786 787 public boolean isEmpty() { 788 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 789 } 790 791 public String fhirType() { 792 return "Claim.event"; 793 794 } 795 796 } 797 798 @Block() 799 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 800 /** 801 * A number to uniquely identify care team entries. 802 */ 803 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 804 @Description(shortDefinition="Order of care team", formalDefinition="A number to uniquely identify care team entries." ) 805 protected PositiveIntType sequence; 806 807 /** 808 * Member of the team who provided the product or service. 809 */ 810 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 811 @Description(shortDefinition="Practitioner or organization", formalDefinition="Member of the team who provided the product or service." ) 812 protected Reference provider; 813 814 /** 815 * The party who is billing and/or responsible for the claimed products or services. 816 */ 817 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 818 @Description(shortDefinition="Indicator of the lead practitioner", formalDefinition="The party who is billing and/or responsible for the claimed products or services." ) 819 protected BooleanType responsible; 820 821 /** 822 * The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team. 823 */ 824 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 825 @Description(shortDefinition="Function within the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team." ) 826 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 827 protected CodeableConcept role; 828 829 /** 830 * The specialization of the practitioner or provider which is applicable for this service. 831 */ 832 @Child(name = "specialty", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 833 @Description(shortDefinition="Practitioner or provider specialization", formalDefinition="The specialization of the practitioner or provider which is applicable for this service." ) 834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 835 protected CodeableConcept specialty; 836 837 private static final long serialVersionUID = 1238813503L; 838 839 /** 840 * Constructor 841 */ 842 public CareTeamComponent() { 843 super(); 844 } 845 846 /** 847 * Constructor 848 */ 849 public CareTeamComponent(int sequence, Reference provider) { 850 super(); 851 this.setSequence(sequence); 852 this.setProvider(provider); 853 } 854 855 /** 856 * @return {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 857 */ 858 public PositiveIntType getSequenceElement() { 859 if (this.sequence == null) 860 if (Configuration.errorOnAutoCreate()) 861 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 862 else if (Configuration.doAutoCreate()) 863 this.sequence = new PositiveIntType(); // bb 864 return this.sequence; 865 } 866 867 public boolean hasSequenceElement() { 868 return this.sequence != null && !this.sequence.isEmpty(); 869 } 870 871 public boolean hasSequence() { 872 return this.sequence != null && !this.sequence.isEmpty(); 873 } 874 875 /** 876 * @param value {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 877 */ 878 public CareTeamComponent setSequenceElement(PositiveIntType value) { 879 this.sequence = value; 880 return this; 881 } 882 883 /** 884 * @return A number to uniquely identify care team entries. 885 */ 886 public int getSequence() { 887 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 888 } 889 890 /** 891 * @param value A number to uniquely identify care team entries. 892 */ 893 public CareTeamComponent setSequence(int value) { 894 if (this.sequence == null) 895 this.sequence = new PositiveIntType(); 896 this.sequence.setValue(value); 897 return this; 898 } 899 900 /** 901 * @return {@link #provider} (Member of the team who provided the product or service.) 902 */ 903 public Reference getProvider() { 904 if (this.provider == null) 905 if (Configuration.errorOnAutoCreate()) 906 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 907 else if (Configuration.doAutoCreate()) 908 this.provider = new Reference(); // cc 909 return this.provider; 910 } 911 912 public boolean hasProvider() { 913 return this.provider != null && !this.provider.isEmpty(); 914 } 915 916 /** 917 * @param value {@link #provider} (Member of the team who provided the product or service.) 918 */ 919 public CareTeamComponent setProvider(Reference value) { 920 this.provider = value; 921 return this; 922 } 923 924 /** 925 * @return {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 926 */ 927 public BooleanType getResponsibleElement() { 928 if (this.responsible == null) 929 if (Configuration.errorOnAutoCreate()) 930 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 931 else if (Configuration.doAutoCreate()) 932 this.responsible = new BooleanType(); // bb 933 return this.responsible; 934 } 935 936 public boolean hasResponsibleElement() { 937 return this.responsible != null && !this.responsible.isEmpty(); 938 } 939 940 public boolean hasResponsible() { 941 return this.responsible != null && !this.responsible.isEmpty(); 942 } 943 944 /** 945 * @param value {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 946 */ 947 public CareTeamComponent setResponsibleElement(BooleanType value) { 948 this.responsible = value; 949 return this; 950 } 951 952 /** 953 * @return The party who is billing and/or responsible for the claimed products or services. 954 */ 955 public boolean getResponsible() { 956 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 957 } 958 959 /** 960 * @param value The party who is billing and/or responsible for the claimed products or services. 961 */ 962 public CareTeamComponent setResponsible(boolean value) { 963 if (this.responsible == null) 964 this.responsible = new BooleanType(); 965 this.responsible.setValue(value); 966 return this; 967 } 968 969 /** 970 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 971 */ 972 public CodeableConcept getRole() { 973 if (this.role == null) 974 if (Configuration.errorOnAutoCreate()) 975 throw new Error("Attempt to auto-create CareTeamComponent.role"); 976 else if (Configuration.doAutoCreate()) 977 this.role = new CodeableConcept(); // cc 978 return this.role; 979 } 980 981 public boolean hasRole() { 982 return this.role != null && !this.role.isEmpty(); 983 } 984 985 /** 986 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 987 */ 988 public CareTeamComponent setRole(CodeableConcept value) { 989 this.role = value; 990 return this; 991 } 992 993 /** 994 * @return {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 995 */ 996 public CodeableConcept getSpecialty() { 997 if (this.specialty == null) 998 if (Configuration.errorOnAutoCreate()) 999 throw new Error("Attempt to auto-create CareTeamComponent.specialty"); 1000 else if (Configuration.doAutoCreate()) 1001 this.specialty = new CodeableConcept(); // cc 1002 return this.specialty; 1003 } 1004 1005 public boolean hasSpecialty() { 1006 return this.specialty != null && !this.specialty.isEmpty(); 1007 } 1008 1009 /** 1010 * @param value {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 1011 */ 1012 public CareTeamComponent setSpecialty(CodeableConcept value) { 1013 this.specialty = value; 1014 return this; 1015 } 1016 1017 protected void listChildren(List<Property> children) { 1018 super.listChildren(children); 1019 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 1020 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider)); 1021 children.add(new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 1022 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role)); 1023 children.add(new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty)); 1024 } 1025 1026 @Override 1027 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1028 switch (_hash) { 1029 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence); 1030 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider); 1031 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 1032 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role); 1033 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty); 1034 default: return super.getNamedProperty(_hash, _name, _checkValid); 1035 } 1036 1037 } 1038 1039 @Override 1040 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1041 switch (hash) { 1042 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1043 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1044 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1045 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1046 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : new Base[] {this.specialty}; // CodeableConcept 1047 default: return super.getProperty(hash, name, checkValid); 1048 } 1049 1050 } 1051 1052 @Override 1053 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1054 switch (hash) { 1055 case 1349547969: // sequence 1056 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1057 return value; 1058 case -987494927: // provider 1059 this.provider = TypeConvertor.castToReference(value); // Reference 1060 return value; 1061 case 1847674614: // responsible 1062 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1063 return value; 1064 case 3506294: // role 1065 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1066 return value; 1067 case -1694759682: // specialty 1068 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1069 return value; 1070 default: return super.setProperty(hash, name, value); 1071 } 1072 1073 } 1074 1075 @Override 1076 public Base setProperty(String name, Base value) throws FHIRException { 1077 if (name.equals("sequence")) { 1078 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1079 } else if (name.equals("provider")) { 1080 this.provider = TypeConvertor.castToReference(value); // Reference 1081 } else if (name.equals("responsible")) { 1082 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1083 } else if (name.equals("role")) { 1084 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1085 } else if (name.equals("specialty")) { 1086 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1087 } else 1088 return super.setProperty(name, value); 1089 return value; 1090 } 1091 1092 @Override 1093 public void removeChild(String name, Base value) throws FHIRException { 1094 if (name.equals("sequence")) { 1095 this.sequence = null; 1096 } else if (name.equals("provider")) { 1097 this.provider = null; 1098 } else if (name.equals("responsible")) { 1099 this.responsible = null; 1100 } else if (name.equals("role")) { 1101 this.role = null; 1102 } else if (name.equals("specialty")) { 1103 this.specialty = null; 1104 } else 1105 super.removeChild(name, value); 1106 1107 } 1108 1109 @Override 1110 public Base makeProperty(int hash, String name) throws FHIRException { 1111 switch (hash) { 1112 case 1349547969: return getSequenceElement(); 1113 case -987494927: return getProvider(); 1114 case 1847674614: return getResponsibleElement(); 1115 case 3506294: return getRole(); 1116 case -1694759682: return getSpecialty(); 1117 default: return super.makeProperty(hash, name); 1118 } 1119 1120 } 1121 1122 @Override 1123 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1124 switch (hash) { 1125 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1126 case -987494927: /*provider*/ return new String[] {"Reference"}; 1127 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1128 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1129 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1130 default: return super.getTypesForProperty(hash, name); 1131 } 1132 1133 } 1134 1135 @Override 1136 public Base addChild(String name) throws FHIRException { 1137 if (name.equals("sequence")) { 1138 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeam.sequence"); 1139 } 1140 else if (name.equals("provider")) { 1141 this.provider = new Reference(); 1142 return this.provider; 1143 } 1144 else if (name.equals("responsible")) { 1145 throw new FHIRException("Cannot call addChild on a singleton property Claim.careTeam.responsible"); 1146 } 1147 else if (name.equals("role")) { 1148 this.role = new CodeableConcept(); 1149 return this.role; 1150 } 1151 else if (name.equals("specialty")) { 1152 this.specialty = new CodeableConcept(); 1153 return this.specialty; 1154 } 1155 else 1156 return super.addChild(name); 1157 } 1158 1159 public CareTeamComponent copy() { 1160 CareTeamComponent dst = new CareTeamComponent(); 1161 copyValues(dst); 1162 return dst; 1163 } 1164 1165 public void copyValues(CareTeamComponent dst) { 1166 super.copyValues(dst); 1167 dst.sequence = sequence == null ? null : sequence.copy(); 1168 dst.provider = provider == null ? null : provider.copy(); 1169 dst.responsible = responsible == null ? null : responsible.copy(); 1170 dst.role = role == null ? null : role.copy(); 1171 dst.specialty = specialty == null ? null : specialty.copy(); 1172 } 1173 1174 @Override 1175 public boolean equalsDeep(Base other_) { 1176 if (!super.equalsDeep(other_)) 1177 return false; 1178 if (!(other_ instanceof CareTeamComponent)) 1179 return false; 1180 CareTeamComponent o = (CareTeamComponent) other_; 1181 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1182 && compareDeep(role, o.role, true) && compareDeep(specialty, o.specialty, true); 1183 } 1184 1185 @Override 1186 public boolean equalsShallow(Base other_) { 1187 if (!super.equalsShallow(other_)) 1188 return false; 1189 if (!(other_ instanceof CareTeamComponent)) 1190 return false; 1191 CareTeamComponent o = (CareTeamComponent) other_; 1192 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1193 ; 1194 } 1195 1196 public boolean isEmpty() { 1197 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1198 , role, specialty); 1199 } 1200 1201 public String fhirType() { 1202 return "Claim.careTeam"; 1203 1204 } 1205 1206 } 1207 1208 @Block() 1209 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1210 /** 1211 * A number to uniquely identify supporting information entries. 1212 */ 1213 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1214 @Description(shortDefinition="Information instance identifier", formalDefinition="A number to uniquely identify supporting information entries." ) 1215 protected PositiveIntType sequence; 1216 1217 /** 1218 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 1219 */ 1220 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1221 @Description(shortDefinition="Classification of the supplied information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 1222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 1223 protected CodeableConcept category; 1224 1225 /** 1226 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought. 1227 */ 1228 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1229 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought." ) 1230 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 1231 protected CodeableConcept code; 1232 1233 /** 1234 * The date when or period to which this information refers. 1235 */ 1236 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1237 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 1238 protected DataType timing; 1239 1240 /** 1241 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 1242 */ 1243 @Child(name = "value", type = {BooleanType.class, StringType.class, Quantity.class, Attachment.class, Reference.class, Identifier.class}, order=5, min=0, max=1, modifier=false, summary=false) 1244 @Description(shortDefinition="Data to be provided", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 1245 protected DataType value; 1246 1247 /** 1248 * Provides the reason in the situation where a reason code is required in addition to the content. 1249 */ 1250 @Child(name = "reason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1251 @Description(shortDefinition="Explanation for the information", formalDefinition="Provides the reason in the situation where a reason code is required in addition to the content." ) 1252 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1253 protected CodeableConcept reason; 1254 1255 private static final long serialVersionUID = 2028146236L; 1256 1257 /** 1258 * Constructor 1259 */ 1260 public SupportingInformationComponent() { 1261 super(); 1262 } 1263 1264 /** 1265 * Constructor 1266 */ 1267 public SupportingInformationComponent(int sequence, CodeableConcept category) { 1268 super(); 1269 this.setSequence(sequence); 1270 this.setCategory(category); 1271 } 1272 1273 /** 1274 * @return {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1275 */ 1276 public PositiveIntType getSequenceElement() { 1277 if (this.sequence == null) 1278 if (Configuration.errorOnAutoCreate()) 1279 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1280 else if (Configuration.doAutoCreate()) 1281 this.sequence = new PositiveIntType(); // bb 1282 return this.sequence; 1283 } 1284 1285 public boolean hasSequenceElement() { 1286 return this.sequence != null && !this.sequence.isEmpty(); 1287 } 1288 1289 public boolean hasSequence() { 1290 return this.sequence != null && !this.sequence.isEmpty(); 1291 } 1292 1293 /** 1294 * @param value {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1295 */ 1296 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1297 this.sequence = value; 1298 return this; 1299 } 1300 1301 /** 1302 * @return A number to uniquely identify supporting information entries. 1303 */ 1304 public int getSequence() { 1305 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1306 } 1307 1308 /** 1309 * @param value A number to uniquely identify supporting information entries. 1310 */ 1311 public SupportingInformationComponent setSequence(int value) { 1312 if (this.sequence == null) 1313 this.sequence = new PositiveIntType(); 1314 this.sequence.setValue(value); 1315 return this; 1316 } 1317 1318 /** 1319 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1320 */ 1321 public CodeableConcept getCategory() { 1322 if (this.category == null) 1323 if (Configuration.errorOnAutoCreate()) 1324 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1325 else if (Configuration.doAutoCreate()) 1326 this.category = new CodeableConcept(); // cc 1327 return this.category; 1328 } 1329 1330 public boolean hasCategory() { 1331 return this.category != null && !this.category.isEmpty(); 1332 } 1333 1334 /** 1335 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1336 */ 1337 public SupportingInformationComponent setCategory(CodeableConcept value) { 1338 this.category = value; 1339 return this; 1340 } 1341 1342 /** 1343 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1344 */ 1345 public CodeableConcept getCode() { 1346 if (this.code == null) 1347 if (Configuration.errorOnAutoCreate()) 1348 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1349 else if (Configuration.doAutoCreate()) 1350 this.code = new CodeableConcept(); // cc 1351 return this.code; 1352 } 1353 1354 public boolean hasCode() { 1355 return this.code != null && !this.code.isEmpty(); 1356 } 1357 1358 /** 1359 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1360 */ 1361 public SupportingInformationComponent setCode(CodeableConcept value) { 1362 this.code = value; 1363 return this; 1364 } 1365 1366 /** 1367 * @return {@link #timing} (The date when or period to which this information refers.) 1368 */ 1369 public DataType getTiming() { 1370 return this.timing; 1371 } 1372 1373 /** 1374 * @return {@link #timing} (The date when or period to which this information refers.) 1375 */ 1376 public DateType getTimingDateType() throws FHIRException { 1377 if (this.timing == null) 1378 this.timing = new DateType(); 1379 if (!(this.timing instanceof DateType)) 1380 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 1381 return (DateType) this.timing; 1382 } 1383 1384 public boolean hasTimingDateType() { 1385 return this != null && this.timing instanceof DateType; 1386 } 1387 1388 /** 1389 * @return {@link #timing} (The date when or period to which this information refers.) 1390 */ 1391 public Period getTimingPeriod() throws FHIRException { 1392 if (this.timing == null) 1393 this.timing = new Period(); 1394 if (!(this.timing instanceof Period)) 1395 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 1396 return (Period) this.timing; 1397 } 1398 1399 public boolean hasTimingPeriod() { 1400 return this != null && this.timing instanceof Period; 1401 } 1402 1403 public boolean hasTiming() { 1404 return this.timing != null && !this.timing.isEmpty(); 1405 } 1406 1407 /** 1408 * @param value {@link #timing} (The date when or period to which this information refers.) 1409 */ 1410 public SupportingInformationComponent setTiming(DataType value) { 1411 if (value != null && !(value instanceof DateType || value instanceof Period)) 1412 throw new FHIRException("Not the right type for Claim.supportingInfo.timing[x]: "+value.fhirType()); 1413 this.timing = value; 1414 return this; 1415 } 1416 1417 /** 1418 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1419 */ 1420 public DataType getValue() { 1421 return this.value; 1422 } 1423 1424 /** 1425 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1426 */ 1427 public BooleanType getValueBooleanType() throws FHIRException { 1428 if (this.value == null) 1429 this.value = new BooleanType(); 1430 if (!(this.value instanceof BooleanType)) 1431 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1432 return (BooleanType) this.value; 1433 } 1434 1435 public boolean hasValueBooleanType() { 1436 return this != null && this.value instanceof BooleanType; 1437 } 1438 1439 /** 1440 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1441 */ 1442 public StringType getValueStringType() throws FHIRException { 1443 if (this.value == null) 1444 this.value = new StringType(); 1445 if (!(this.value instanceof StringType)) 1446 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1447 return (StringType) this.value; 1448 } 1449 1450 public boolean hasValueStringType() { 1451 return this != null && this.value instanceof StringType; 1452 } 1453 1454 /** 1455 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1456 */ 1457 public Quantity getValueQuantity() throws FHIRException { 1458 if (this.value == null) 1459 this.value = new Quantity(); 1460 if (!(this.value instanceof Quantity)) 1461 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1462 return (Quantity) this.value; 1463 } 1464 1465 public boolean hasValueQuantity() { 1466 return this != null && this.value instanceof Quantity; 1467 } 1468 1469 /** 1470 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1471 */ 1472 public Attachment getValueAttachment() throws FHIRException { 1473 if (this.value == null) 1474 this.value = new Attachment(); 1475 if (!(this.value instanceof Attachment)) 1476 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1477 return (Attachment) this.value; 1478 } 1479 1480 public boolean hasValueAttachment() { 1481 return this != null && this.value instanceof Attachment; 1482 } 1483 1484 /** 1485 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1486 */ 1487 public Reference getValueReference() throws FHIRException { 1488 if (this.value == null) 1489 this.value = new Reference(); 1490 if (!(this.value instanceof Reference)) 1491 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1492 return (Reference) this.value; 1493 } 1494 1495 public boolean hasValueReference() { 1496 return this != null && this.value instanceof Reference; 1497 } 1498 1499 /** 1500 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1501 */ 1502 public Identifier getValueIdentifier() throws FHIRException { 1503 if (this.value == null) 1504 this.value = new Identifier(); 1505 if (!(this.value instanceof Identifier)) 1506 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 1507 return (Identifier) this.value; 1508 } 1509 1510 public boolean hasValueIdentifier() { 1511 return this != null && this.value instanceof Identifier; 1512 } 1513 1514 public boolean hasValue() { 1515 return this.value != null && !this.value.isEmpty(); 1516 } 1517 1518 /** 1519 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1520 */ 1521 public SupportingInformationComponent setValue(DataType value) { 1522 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference || value instanceof Identifier)) 1523 throw new FHIRException("Not the right type for Claim.supportingInfo.value[x]: "+value.fhirType()); 1524 this.value = value; 1525 return this; 1526 } 1527 1528 /** 1529 * @return {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1530 */ 1531 public CodeableConcept getReason() { 1532 if (this.reason == null) 1533 if (Configuration.errorOnAutoCreate()) 1534 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1535 else if (Configuration.doAutoCreate()) 1536 this.reason = new CodeableConcept(); // cc 1537 return this.reason; 1538 } 1539 1540 public boolean hasReason() { 1541 return this.reason != null && !this.reason.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1546 */ 1547 public SupportingInformationComponent setReason(CodeableConcept value) { 1548 this.reason = value; 1549 return this; 1550 } 1551 1552 protected void listChildren(List<Property> children) { 1553 super.listChildren(children); 1554 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1555 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1556 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code)); 1557 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1558 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1559 children.add(new Property("reason", "CodeableConcept", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason)); 1560 } 1561 1562 @Override 1563 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1564 switch (_hash) { 1565 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence); 1566 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1567 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code); 1568 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1569 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1570 case 807935768: /*timingDate*/ return new Property("timing[x]", "date", "The date when or period to which this information refers.", 0, 1, timing); 1571 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Period", "The date when or period to which this information refers.", 0, 1, timing); 1572 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1573 case 111972721: /*value*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1574 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1575 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1576 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1577 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1578 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1579 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1580 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason); 1581 default: return super.getNamedProperty(_hash, _name, _checkValid); 1582 } 1583 1584 } 1585 1586 @Override 1587 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1588 switch (hash) { 1589 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1590 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1591 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1592 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 1593 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1594 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1595 default: return super.getProperty(hash, name, checkValid); 1596 } 1597 1598 } 1599 1600 @Override 1601 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1602 switch (hash) { 1603 case 1349547969: // sequence 1604 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1605 return value; 1606 case 50511102: // category 1607 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1608 return value; 1609 case 3059181: // code 1610 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1611 return value; 1612 case -873664438: // timing 1613 this.timing = TypeConvertor.castToType(value); // DataType 1614 return value; 1615 case 111972721: // value 1616 this.value = TypeConvertor.castToType(value); // DataType 1617 return value; 1618 case -934964668: // reason 1619 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1620 return value; 1621 default: return super.setProperty(hash, name, value); 1622 } 1623 1624 } 1625 1626 @Override 1627 public Base setProperty(String name, Base value) throws FHIRException { 1628 if (name.equals("sequence")) { 1629 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1630 } else if (name.equals("category")) { 1631 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1632 } else if (name.equals("code")) { 1633 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1634 } else if (name.equals("timing[x]")) { 1635 this.timing = TypeConvertor.castToType(value); // DataType 1636 } else if (name.equals("value[x]")) { 1637 this.value = TypeConvertor.castToType(value); // DataType 1638 } else if (name.equals("reason")) { 1639 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1640 } else 1641 return super.setProperty(name, value); 1642 return value; 1643 } 1644 1645 @Override 1646 public void removeChild(String name, Base value) throws FHIRException { 1647 if (name.equals("sequence")) { 1648 this.sequence = null; 1649 } else if (name.equals("category")) { 1650 this.category = null; 1651 } else if (name.equals("code")) { 1652 this.code = null; 1653 } else if (name.equals("timing[x]")) { 1654 this.timing = null; 1655 } else if (name.equals("value[x]")) { 1656 this.value = null; 1657 } else if (name.equals("reason")) { 1658 this.reason = null; 1659 } else 1660 super.removeChild(name, value); 1661 1662 } 1663 1664 @Override 1665 public Base makeProperty(int hash, String name) throws FHIRException { 1666 switch (hash) { 1667 case 1349547969: return getSequenceElement(); 1668 case 50511102: return getCategory(); 1669 case 3059181: return getCode(); 1670 case 164632566: return getTiming(); 1671 case -873664438: return getTiming(); 1672 case -1410166417: return getValue(); 1673 case 111972721: return getValue(); 1674 case -934964668: return getReason(); 1675 default: return super.makeProperty(hash, name); 1676 } 1677 1678 } 1679 1680 @Override 1681 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1682 switch (hash) { 1683 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1684 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1685 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1686 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1687 case 111972721: /*value*/ return new String[] {"boolean", "string", "Quantity", "Attachment", "Reference", "Identifier"}; 1688 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1689 default: return super.getTypesForProperty(hash, name); 1690 } 1691 1692 } 1693 1694 @Override 1695 public Base addChild(String name) throws FHIRException { 1696 if (name.equals("sequence")) { 1697 throw new FHIRException("Cannot call addChild on a singleton property Claim.supportingInfo.sequence"); 1698 } 1699 else if (name.equals("category")) { 1700 this.category = new CodeableConcept(); 1701 return this.category; 1702 } 1703 else if (name.equals("code")) { 1704 this.code = new CodeableConcept(); 1705 return this.code; 1706 } 1707 else if (name.equals("timingDate")) { 1708 this.timing = new DateType(); 1709 return this.timing; 1710 } 1711 else if (name.equals("timingPeriod")) { 1712 this.timing = new Period(); 1713 return this.timing; 1714 } 1715 else if (name.equals("valueBoolean")) { 1716 this.value = new BooleanType(); 1717 return this.value; 1718 } 1719 else if (name.equals("valueString")) { 1720 this.value = new StringType(); 1721 return this.value; 1722 } 1723 else if (name.equals("valueQuantity")) { 1724 this.value = new Quantity(); 1725 return this.value; 1726 } 1727 else if (name.equals("valueAttachment")) { 1728 this.value = new Attachment(); 1729 return this.value; 1730 } 1731 else if (name.equals("valueReference")) { 1732 this.value = new Reference(); 1733 return this.value; 1734 } 1735 else if (name.equals("valueIdentifier")) { 1736 this.value = new Identifier(); 1737 return this.value; 1738 } 1739 else if (name.equals("reason")) { 1740 this.reason = new CodeableConcept(); 1741 return this.reason; 1742 } 1743 else 1744 return super.addChild(name); 1745 } 1746 1747 public SupportingInformationComponent copy() { 1748 SupportingInformationComponent dst = new SupportingInformationComponent(); 1749 copyValues(dst); 1750 return dst; 1751 } 1752 1753 public void copyValues(SupportingInformationComponent dst) { 1754 super.copyValues(dst); 1755 dst.sequence = sequence == null ? null : sequence.copy(); 1756 dst.category = category == null ? null : category.copy(); 1757 dst.code = code == null ? null : code.copy(); 1758 dst.timing = timing == null ? null : timing.copy(); 1759 dst.value = value == null ? null : value.copy(); 1760 dst.reason = reason == null ? null : reason.copy(); 1761 } 1762 1763 @Override 1764 public boolean equalsDeep(Base other_) { 1765 if (!super.equalsDeep(other_)) 1766 return false; 1767 if (!(other_ instanceof SupportingInformationComponent)) 1768 return false; 1769 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1770 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1771 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1772 ; 1773 } 1774 1775 @Override 1776 public boolean equalsShallow(Base other_) { 1777 if (!super.equalsShallow(other_)) 1778 return false; 1779 if (!(other_ instanceof SupportingInformationComponent)) 1780 return false; 1781 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1782 return compareValues(sequence, o.sequence, true); 1783 } 1784 1785 public boolean isEmpty() { 1786 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1787 , timing, value, reason); 1788 } 1789 1790 public String fhirType() { 1791 return "Claim.supportingInfo"; 1792 1793 } 1794 1795 } 1796 1797 @Block() 1798 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1799 /** 1800 * A number to uniquely identify diagnosis entries. 1801 */ 1802 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1803 @Description(shortDefinition="Diagnosis instance identifier", formalDefinition="A number to uniquely identify diagnosis entries." ) 1804 protected PositiveIntType sequence; 1805 1806 /** 1807 * The nature of illness or problem in a coded form or as a reference to an external defined Condition. 1808 */ 1809 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1810 @Description(shortDefinition="Nature of illness or problem", formalDefinition="The nature of illness or problem in a coded form or as a reference to an external defined Condition." ) 1811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1812 protected DataType diagnosis; 1813 1814 /** 1815 * When the condition was observed or the relative ranking. 1816 */ 1817 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1818 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="When the condition was observed or the relative ranking." ) 1819 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1820 protected List<CodeableConcept> type; 1821 1822 /** 1823 * Indication of whether the diagnosis was present on admission to a facility. 1824 */ 1825 @Child(name = "onAdmission", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1826 @Description(shortDefinition="Present on admission", formalDefinition="Indication of whether the diagnosis was present on admission to a facility." ) 1827 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 1828 protected CodeableConcept onAdmission; 1829 1830 private static final long serialVersionUID = -320261526L; 1831 1832 /** 1833 * Constructor 1834 */ 1835 public DiagnosisComponent() { 1836 super(); 1837 } 1838 1839 /** 1840 * Constructor 1841 */ 1842 public DiagnosisComponent(int sequence, DataType diagnosis) { 1843 super(); 1844 this.setSequence(sequence); 1845 this.setDiagnosis(diagnosis); 1846 } 1847 1848 /** 1849 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1850 */ 1851 public PositiveIntType getSequenceElement() { 1852 if (this.sequence == null) 1853 if (Configuration.errorOnAutoCreate()) 1854 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1855 else if (Configuration.doAutoCreate()) 1856 this.sequence = new PositiveIntType(); // bb 1857 return this.sequence; 1858 } 1859 1860 public boolean hasSequenceElement() { 1861 return this.sequence != null && !this.sequence.isEmpty(); 1862 } 1863 1864 public boolean hasSequence() { 1865 return this.sequence != null && !this.sequence.isEmpty(); 1866 } 1867 1868 /** 1869 * @param value {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1870 */ 1871 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1872 this.sequence = value; 1873 return this; 1874 } 1875 1876 /** 1877 * @return A number to uniquely identify diagnosis entries. 1878 */ 1879 public int getSequence() { 1880 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1881 } 1882 1883 /** 1884 * @param value A number to uniquely identify diagnosis entries. 1885 */ 1886 public DiagnosisComponent setSequence(int value) { 1887 if (this.sequence == null) 1888 this.sequence = new PositiveIntType(); 1889 this.sequence.setValue(value); 1890 return this; 1891 } 1892 1893 /** 1894 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1895 */ 1896 public DataType getDiagnosis() { 1897 return this.diagnosis; 1898 } 1899 1900 /** 1901 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1902 */ 1903 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1904 if (this.diagnosis == null) 1905 this.diagnosis = new CodeableConcept(); 1906 if (!(this.diagnosis instanceof CodeableConcept)) 1907 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1908 return (CodeableConcept) this.diagnosis; 1909 } 1910 1911 public boolean hasDiagnosisCodeableConcept() { 1912 return this != null && this.diagnosis instanceof CodeableConcept; 1913 } 1914 1915 /** 1916 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1917 */ 1918 public Reference getDiagnosisReference() throws FHIRException { 1919 if (this.diagnosis == null) 1920 this.diagnosis = new Reference(); 1921 if (!(this.diagnosis instanceof Reference)) 1922 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1923 return (Reference) this.diagnosis; 1924 } 1925 1926 public boolean hasDiagnosisReference() { 1927 return this != null && this.diagnosis instanceof Reference; 1928 } 1929 1930 public boolean hasDiagnosis() { 1931 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1932 } 1933 1934 /** 1935 * @param value {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1936 */ 1937 public DiagnosisComponent setDiagnosis(DataType value) { 1938 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1939 throw new FHIRException("Not the right type for Claim.diagnosis.diagnosis[x]: "+value.fhirType()); 1940 this.diagnosis = value; 1941 return this; 1942 } 1943 1944 /** 1945 * @return {@link #type} (When the condition was observed or the relative ranking.) 1946 */ 1947 public List<CodeableConcept> getType() { 1948 if (this.type == null) 1949 this.type = new ArrayList<CodeableConcept>(); 1950 return this.type; 1951 } 1952 1953 /** 1954 * @return Returns a reference to <code>this</code> for easy method chaining 1955 */ 1956 public DiagnosisComponent setType(List<CodeableConcept> theType) { 1957 this.type = theType; 1958 return this; 1959 } 1960 1961 public boolean hasType() { 1962 if (this.type == null) 1963 return false; 1964 for (CodeableConcept item : this.type) 1965 if (!item.isEmpty()) 1966 return true; 1967 return false; 1968 } 1969 1970 public CodeableConcept addType() { //3 1971 CodeableConcept t = new CodeableConcept(); 1972 if (this.type == null) 1973 this.type = new ArrayList<CodeableConcept>(); 1974 this.type.add(t); 1975 return t; 1976 } 1977 1978 public DiagnosisComponent addType(CodeableConcept t) { //3 1979 if (t == null) 1980 return this; 1981 if (this.type == null) 1982 this.type = new ArrayList<CodeableConcept>(); 1983 this.type.add(t); 1984 return this; 1985 } 1986 1987 /** 1988 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1989 */ 1990 public CodeableConcept getTypeFirstRep() { 1991 if (getType().isEmpty()) { 1992 addType(); 1993 } 1994 return getType().get(0); 1995 } 1996 1997 /** 1998 * @return {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 1999 */ 2000 public CodeableConcept getOnAdmission() { 2001 if (this.onAdmission == null) 2002 if (Configuration.errorOnAutoCreate()) 2003 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 2004 else if (Configuration.doAutoCreate()) 2005 this.onAdmission = new CodeableConcept(); // cc 2006 return this.onAdmission; 2007 } 2008 2009 public boolean hasOnAdmission() { 2010 return this.onAdmission != null && !this.onAdmission.isEmpty(); 2011 } 2012 2013 /** 2014 * @param value {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 2015 */ 2016 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 2017 this.onAdmission = value; 2018 return this; 2019 } 2020 2021 protected void listChildren(List<Property> children) { 2022 super.listChildren(children); 2023 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 2024 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis)); 2025 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2026 children.add(new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 2027 } 2028 2029 @Override 2030 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2031 switch (_hash) { 2032 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 2033 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2034 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2035 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2036 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2037 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2038 case -3386134: /*onAdmission*/ return new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 2039 default: return super.getNamedProperty(_hash, _name, _checkValid); 2040 } 2041 2042 } 2043 2044 @Override 2045 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2046 switch (hash) { 2047 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2048 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // DataType 2049 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2050 case -3386134: /*onAdmission*/ return this.onAdmission == null ? new Base[0] : new Base[] {this.onAdmission}; // CodeableConcept 2051 default: return super.getProperty(hash, name, checkValid); 2052 } 2053 2054 } 2055 2056 @Override 2057 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2058 switch (hash) { 2059 case 1349547969: // sequence 2060 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2061 return value; 2062 case 1196993265: // diagnosis 2063 this.diagnosis = TypeConvertor.castToType(value); // DataType 2064 return value; 2065 case 3575610: // type 2066 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2067 return value; 2068 case -3386134: // onAdmission 2069 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2070 return value; 2071 default: return super.setProperty(hash, name, value); 2072 } 2073 2074 } 2075 2076 @Override 2077 public Base setProperty(String name, Base value) throws FHIRException { 2078 if (name.equals("sequence")) { 2079 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2080 } else if (name.equals("diagnosis[x]")) { 2081 this.diagnosis = TypeConvertor.castToType(value); // DataType 2082 } else if (name.equals("type")) { 2083 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2084 } else if (name.equals("onAdmission")) { 2085 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2086 } else 2087 return super.setProperty(name, value); 2088 return value; 2089 } 2090 2091 @Override 2092 public void removeChild(String name, Base value) throws FHIRException { 2093 if (name.equals("sequence")) { 2094 this.sequence = null; 2095 } else if (name.equals("diagnosis[x]")) { 2096 this.diagnosis = null; 2097 } else if (name.equals("type")) { 2098 this.getType().remove(value); 2099 } else if (name.equals("onAdmission")) { 2100 this.onAdmission = null; 2101 } else 2102 super.removeChild(name, value); 2103 2104 } 2105 2106 @Override 2107 public Base makeProperty(int hash, String name) throws FHIRException { 2108 switch (hash) { 2109 case 1349547969: return getSequenceElement(); 2110 case -1487009809: return getDiagnosis(); 2111 case 1196993265: return getDiagnosis(); 2112 case 3575610: return addType(); 2113 case -3386134: return getOnAdmission(); 2114 default: return super.makeProperty(hash, name); 2115 } 2116 2117 } 2118 2119 @Override 2120 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2121 switch (hash) { 2122 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2123 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 2124 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2125 case -3386134: /*onAdmission*/ return new String[] {"CodeableConcept"}; 2126 default: return super.getTypesForProperty(hash, name); 2127 } 2128 2129 } 2130 2131 @Override 2132 public Base addChild(String name) throws FHIRException { 2133 if (name.equals("sequence")) { 2134 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosis.sequence"); 2135 } 2136 else if (name.equals("diagnosisCodeableConcept")) { 2137 this.diagnosis = new CodeableConcept(); 2138 return this.diagnosis; 2139 } 2140 else if (name.equals("diagnosisReference")) { 2141 this.diagnosis = new Reference(); 2142 return this.diagnosis; 2143 } 2144 else if (name.equals("type")) { 2145 return addType(); 2146 } 2147 else if (name.equals("onAdmission")) { 2148 this.onAdmission = new CodeableConcept(); 2149 return this.onAdmission; 2150 } 2151 else 2152 return super.addChild(name); 2153 } 2154 2155 public DiagnosisComponent copy() { 2156 DiagnosisComponent dst = new DiagnosisComponent(); 2157 copyValues(dst); 2158 return dst; 2159 } 2160 2161 public void copyValues(DiagnosisComponent dst) { 2162 super.copyValues(dst); 2163 dst.sequence = sequence == null ? null : sequence.copy(); 2164 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2165 if (type != null) { 2166 dst.type = new ArrayList<CodeableConcept>(); 2167 for (CodeableConcept i : type) 2168 dst.type.add(i.copy()); 2169 }; 2170 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2171 } 2172 2173 @Override 2174 public boolean equalsDeep(Base other_) { 2175 if (!super.equalsDeep(other_)) 2176 return false; 2177 if (!(other_ instanceof DiagnosisComponent)) 2178 return false; 2179 DiagnosisComponent o = (DiagnosisComponent) other_; 2180 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2181 && compareDeep(onAdmission, o.onAdmission, true); 2182 } 2183 2184 @Override 2185 public boolean equalsShallow(Base other_) { 2186 if (!super.equalsShallow(other_)) 2187 return false; 2188 if (!(other_ instanceof DiagnosisComponent)) 2189 return false; 2190 DiagnosisComponent o = (DiagnosisComponent) other_; 2191 return compareValues(sequence, o.sequence, true); 2192 } 2193 2194 public boolean isEmpty() { 2195 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2196 , onAdmission); 2197 } 2198 2199 public String fhirType() { 2200 return "Claim.diagnosis"; 2201 2202 } 2203 2204 } 2205 2206 @Block() 2207 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2208 /** 2209 * A number to uniquely identify procedure entries. 2210 */ 2211 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2212 @Description(shortDefinition="Procedure instance identifier", formalDefinition="A number to uniquely identify procedure entries." ) 2213 protected PositiveIntType sequence; 2214 2215 /** 2216 * When the condition was observed or the relative ranking. 2217 */ 2218 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2219 @Description(shortDefinition="Category of Procedure", formalDefinition="When the condition was observed or the relative ranking." ) 2220 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-procedure-type") 2221 protected List<CodeableConcept> type; 2222 2223 /** 2224 * Date and optionally time the procedure was performed. 2225 */ 2226 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2227 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed." ) 2228 protected DateTimeType date; 2229 2230 /** 2231 * The code or reference to a Procedure resource which identifies the clinical intervention performed. 2232 */ 2233 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=4, min=1, max=1, modifier=false, summary=false) 2234 @Description(shortDefinition="Specific clinical procedure", formalDefinition="The code or reference to a Procedure resource which identifies the clinical intervention performed." ) 2235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2236 protected DataType procedure; 2237 2238 /** 2239 * Unique Device Identifiers associated with this line item. 2240 */ 2241 @Child(name = "udi", type = {Device.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2242 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 2243 protected List<Reference> udi; 2244 2245 private static final long serialVersionUID = 1165684715L; 2246 2247 /** 2248 * Constructor 2249 */ 2250 public ProcedureComponent() { 2251 super(); 2252 } 2253 2254 /** 2255 * Constructor 2256 */ 2257 public ProcedureComponent(int sequence, DataType procedure) { 2258 super(); 2259 this.setSequence(sequence); 2260 this.setProcedure(procedure); 2261 } 2262 2263 /** 2264 * @return {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2265 */ 2266 public PositiveIntType getSequenceElement() { 2267 if (this.sequence == null) 2268 if (Configuration.errorOnAutoCreate()) 2269 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2270 else if (Configuration.doAutoCreate()) 2271 this.sequence = new PositiveIntType(); // bb 2272 return this.sequence; 2273 } 2274 2275 public boolean hasSequenceElement() { 2276 return this.sequence != null && !this.sequence.isEmpty(); 2277 } 2278 2279 public boolean hasSequence() { 2280 return this.sequence != null && !this.sequence.isEmpty(); 2281 } 2282 2283 /** 2284 * @param value {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2285 */ 2286 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2287 this.sequence = value; 2288 return this; 2289 } 2290 2291 /** 2292 * @return A number to uniquely identify procedure entries. 2293 */ 2294 public int getSequence() { 2295 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2296 } 2297 2298 /** 2299 * @param value A number to uniquely identify procedure entries. 2300 */ 2301 public ProcedureComponent setSequence(int value) { 2302 if (this.sequence == null) 2303 this.sequence = new PositiveIntType(); 2304 this.sequence.setValue(value); 2305 return this; 2306 } 2307 2308 /** 2309 * @return {@link #type} (When the condition was observed or the relative ranking.) 2310 */ 2311 public List<CodeableConcept> getType() { 2312 if (this.type == null) 2313 this.type = new ArrayList<CodeableConcept>(); 2314 return this.type; 2315 } 2316 2317 /** 2318 * @return Returns a reference to <code>this</code> for easy method chaining 2319 */ 2320 public ProcedureComponent setType(List<CodeableConcept> theType) { 2321 this.type = theType; 2322 return this; 2323 } 2324 2325 public boolean hasType() { 2326 if (this.type == null) 2327 return false; 2328 for (CodeableConcept item : this.type) 2329 if (!item.isEmpty()) 2330 return true; 2331 return false; 2332 } 2333 2334 public CodeableConcept addType() { //3 2335 CodeableConcept t = new CodeableConcept(); 2336 if (this.type == null) 2337 this.type = new ArrayList<CodeableConcept>(); 2338 this.type.add(t); 2339 return t; 2340 } 2341 2342 public ProcedureComponent addType(CodeableConcept t) { //3 2343 if (t == null) 2344 return this; 2345 if (this.type == null) 2346 this.type = new ArrayList<CodeableConcept>(); 2347 this.type.add(t); 2348 return this; 2349 } 2350 2351 /** 2352 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2353 */ 2354 public CodeableConcept getTypeFirstRep() { 2355 if (getType().isEmpty()) { 2356 addType(); 2357 } 2358 return getType().get(0); 2359 } 2360 2361 /** 2362 * @return {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2363 */ 2364 public DateTimeType getDateElement() { 2365 if (this.date == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2368 else if (Configuration.doAutoCreate()) 2369 this.date = new DateTimeType(); // bb 2370 return this.date; 2371 } 2372 2373 public boolean hasDateElement() { 2374 return this.date != null && !this.date.isEmpty(); 2375 } 2376 2377 public boolean hasDate() { 2378 return this.date != null && !this.date.isEmpty(); 2379 } 2380 2381 /** 2382 * @param value {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2383 */ 2384 public ProcedureComponent setDateElement(DateTimeType value) { 2385 this.date = value; 2386 return this; 2387 } 2388 2389 /** 2390 * @return Date and optionally time the procedure was performed. 2391 */ 2392 public Date getDate() { 2393 return this.date == null ? null : this.date.getValue(); 2394 } 2395 2396 /** 2397 * @param value Date and optionally time the procedure was performed. 2398 */ 2399 public ProcedureComponent setDate(Date value) { 2400 if (value == null) 2401 this.date = null; 2402 else { 2403 if (this.date == null) 2404 this.date = new DateTimeType(); 2405 this.date.setValue(value); 2406 } 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2412 */ 2413 public DataType getProcedure() { 2414 return this.procedure; 2415 } 2416 2417 /** 2418 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2419 */ 2420 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2421 if (this.procedure == null) 2422 this.procedure = new CodeableConcept(); 2423 if (!(this.procedure instanceof CodeableConcept)) 2424 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2425 return (CodeableConcept) this.procedure; 2426 } 2427 2428 public boolean hasProcedureCodeableConcept() { 2429 return this != null && this.procedure instanceof CodeableConcept; 2430 } 2431 2432 /** 2433 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2434 */ 2435 public Reference getProcedureReference() throws FHIRException { 2436 if (this.procedure == null) 2437 this.procedure = new Reference(); 2438 if (!(this.procedure instanceof Reference)) 2439 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2440 return (Reference) this.procedure; 2441 } 2442 2443 public boolean hasProcedureReference() { 2444 return this != null && this.procedure instanceof Reference; 2445 } 2446 2447 public boolean hasProcedure() { 2448 return this.procedure != null && !this.procedure.isEmpty(); 2449 } 2450 2451 /** 2452 * @param value {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2453 */ 2454 public ProcedureComponent setProcedure(DataType value) { 2455 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2456 throw new FHIRException("Not the right type for Claim.procedure.procedure[x]: "+value.fhirType()); 2457 this.procedure = value; 2458 return this; 2459 } 2460 2461 /** 2462 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 2463 */ 2464 public List<Reference> getUdi() { 2465 if (this.udi == null) 2466 this.udi = new ArrayList<Reference>(); 2467 return this.udi; 2468 } 2469 2470 /** 2471 * @return Returns a reference to <code>this</code> for easy method chaining 2472 */ 2473 public ProcedureComponent setUdi(List<Reference> theUdi) { 2474 this.udi = theUdi; 2475 return this; 2476 } 2477 2478 public boolean hasUdi() { 2479 if (this.udi == null) 2480 return false; 2481 for (Reference item : this.udi) 2482 if (!item.isEmpty()) 2483 return true; 2484 return false; 2485 } 2486 2487 public Reference addUdi() { //3 2488 Reference t = new Reference(); 2489 if (this.udi == null) 2490 this.udi = new ArrayList<Reference>(); 2491 this.udi.add(t); 2492 return t; 2493 } 2494 2495 public ProcedureComponent addUdi(Reference t) { //3 2496 if (t == null) 2497 return this; 2498 if (this.udi == null) 2499 this.udi = new ArrayList<Reference>(); 2500 this.udi.add(t); 2501 return this; 2502 } 2503 2504 /** 2505 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 2506 */ 2507 public Reference getUdiFirstRep() { 2508 if (getUdi().isEmpty()) { 2509 addUdi(); 2510 } 2511 return getUdi().get(0); 2512 } 2513 2514 protected void listChildren(List<Property> children) { 2515 super.listChildren(children); 2516 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 2517 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2518 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 2519 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure)); 2520 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 2521 } 2522 2523 @Override 2524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2525 switch (_hash) { 2526 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence); 2527 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2528 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date); 2529 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2530 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2531 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2532 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2533 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 2534 default: return super.getNamedProperty(_hash, _name, _checkValid); 2535 } 2536 2537 } 2538 2539 @Override 2540 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2541 switch (hash) { 2542 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2543 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2544 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2545 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // DataType 2546 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 2547 default: return super.getProperty(hash, name, checkValid); 2548 } 2549 2550 } 2551 2552 @Override 2553 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2554 switch (hash) { 2555 case 1349547969: // sequence 2556 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2557 return value; 2558 case 3575610: // type 2559 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2560 return value; 2561 case 3076014: // date 2562 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2563 return value; 2564 case -1095204141: // procedure 2565 this.procedure = TypeConvertor.castToType(value); // DataType 2566 return value; 2567 case 115642: // udi 2568 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 2569 return value; 2570 default: return super.setProperty(hash, name, value); 2571 } 2572 2573 } 2574 2575 @Override 2576 public Base setProperty(String name, Base value) throws FHIRException { 2577 if (name.equals("sequence")) { 2578 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2579 } else if (name.equals("type")) { 2580 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2581 } else if (name.equals("date")) { 2582 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2583 } else if (name.equals("procedure[x]")) { 2584 this.procedure = TypeConvertor.castToType(value); // DataType 2585 } else if (name.equals("udi")) { 2586 this.getUdi().add(TypeConvertor.castToReference(value)); 2587 } else 2588 return super.setProperty(name, value); 2589 return value; 2590 } 2591 2592 @Override 2593 public void removeChild(String name, Base value) throws FHIRException { 2594 if (name.equals("sequence")) { 2595 this.sequence = null; 2596 } else if (name.equals("type")) { 2597 this.getType().remove(value); 2598 } else if (name.equals("date")) { 2599 this.date = null; 2600 } else if (name.equals("procedure[x]")) { 2601 this.procedure = null; 2602 } else if (name.equals("udi")) { 2603 this.getUdi().remove(value); 2604 } else 2605 super.removeChild(name, value); 2606 2607 } 2608 2609 @Override 2610 public Base makeProperty(int hash, String name) throws FHIRException { 2611 switch (hash) { 2612 case 1349547969: return getSequenceElement(); 2613 case 3575610: return addType(); 2614 case 3076014: return getDateElement(); 2615 case 1640074445: return getProcedure(); 2616 case -1095204141: return getProcedure(); 2617 case 115642: return addUdi(); 2618 default: return super.makeProperty(hash, name); 2619 } 2620 2621 } 2622 2623 @Override 2624 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2625 switch (hash) { 2626 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2627 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2628 case 3076014: /*date*/ return new String[] {"dateTime"}; 2629 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2630 case 115642: /*udi*/ return new String[] {"Reference"}; 2631 default: return super.getTypesForProperty(hash, name); 2632 } 2633 2634 } 2635 2636 @Override 2637 public Base addChild(String name) throws FHIRException { 2638 if (name.equals("sequence")) { 2639 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedure.sequence"); 2640 } 2641 else if (name.equals("type")) { 2642 return addType(); 2643 } 2644 else if (name.equals("date")) { 2645 throw new FHIRException("Cannot call addChild on a singleton property Claim.procedure.date"); 2646 } 2647 else if (name.equals("procedureCodeableConcept")) { 2648 this.procedure = new CodeableConcept(); 2649 return this.procedure; 2650 } 2651 else if (name.equals("procedureReference")) { 2652 this.procedure = new Reference(); 2653 return this.procedure; 2654 } 2655 else if (name.equals("udi")) { 2656 return addUdi(); 2657 } 2658 else 2659 return super.addChild(name); 2660 } 2661 2662 public ProcedureComponent copy() { 2663 ProcedureComponent dst = new ProcedureComponent(); 2664 copyValues(dst); 2665 return dst; 2666 } 2667 2668 public void copyValues(ProcedureComponent dst) { 2669 super.copyValues(dst); 2670 dst.sequence = sequence == null ? null : sequence.copy(); 2671 if (type != null) { 2672 dst.type = new ArrayList<CodeableConcept>(); 2673 for (CodeableConcept i : type) 2674 dst.type.add(i.copy()); 2675 }; 2676 dst.date = date == null ? null : date.copy(); 2677 dst.procedure = procedure == null ? null : procedure.copy(); 2678 if (udi != null) { 2679 dst.udi = new ArrayList<Reference>(); 2680 for (Reference i : udi) 2681 dst.udi.add(i.copy()); 2682 }; 2683 } 2684 2685 @Override 2686 public boolean equalsDeep(Base other_) { 2687 if (!super.equalsDeep(other_)) 2688 return false; 2689 if (!(other_ instanceof ProcedureComponent)) 2690 return false; 2691 ProcedureComponent o = (ProcedureComponent) other_; 2692 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(date, o.date, true) 2693 && compareDeep(procedure, o.procedure, true) && compareDeep(udi, o.udi, true); 2694 } 2695 2696 @Override 2697 public boolean equalsShallow(Base other_) { 2698 if (!super.equalsShallow(other_)) 2699 return false; 2700 if (!(other_ instanceof ProcedureComponent)) 2701 return false; 2702 ProcedureComponent o = (ProcedureComponent) other_; 2703 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2704 } 2705 2706 public boolean isEmpty() { 2707 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure 2708 , udi); 2709 } 2710 2711 public String fhirType() { 2712 return "Claim.procedure"; 2713 2714 } 2715 2716 } 2717 2718 @Block() 2719 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2720 /** 2721 * A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 2722 */ 2723 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2724 @Description(shortDefinition="Insurance instance identifier", formalDefinition="A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order." ) 2725 protected PositiveIntType sequence; 2726 2727 /** 2728 * A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2729 */ 2730 @Child(name = "focal", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=true) 2731 @Description(shortDefinition="Coverage to be used for adjudication", formalDefinition="A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true." ) 2732 protected BooleanType focal; 2733 2734 /** 2735 * The business identifier to be used when the claim is sent for adjudication against this insurance policy. 2736 */ 2737 @Child(name = "identifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 2738 @Description(shortDefinition="Pre-assigned Claim number", formalDefinition="The business identifier to be used when the claim is sent for adjudication against this insurance policy." ) 2739 protected Identifier identifier; 2740 2741 /** 2742 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 2743 */ 2744 @Child(name = "coverage", type = {Coverage.class}, order=4, min=1, max=1, modifier=false, summary=true) 2745 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 2746 protected Reference coverage; 2747 2748 /** 2749 * A business agreement number established between the provider and the insurer for special business processing purposes. 2750 */ 2751 @Child(name = "businessArrangement", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2752 @Description(shortDefinition="Additional provider contract number", formalDefinition="A business agreement number established between the provider and the insurer for special business processing purposes." ) 2753 protected StringType businessArrangement; 2754 2755 /** 2756 * Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization. 2757 */ 2758 @Child(name = "preAuthRef", type = {StringType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2759 @Description(shortDefinition="Prior authorization reference number", formalDefinition="Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization." ) 2760 protected List<StringType> preAuthRef; 2761 2762 /** 2763 * The result of the adjudication of the line items for the Coverage specified in this insurance. 2764 */ 2765 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=7, min=0, max=1, modifier=false, summary=false) 2766 @Description(shortDefinition="Adjudication results", formalDefinition="The result of the adjudication of the line items for the Coverage specified in this insurance." ) 2767 protected Reference claimResponse; 2768 2769 private static final long serialVersionUID = 481628099L; 2770 2771 /** 2772 * Constructor 2773 */ 2774 public InsuranceComponent() { 2775 super(); 2776 } 2777 2778 /** 2779 * Constructor 2780 */ 2781 public InsuranceComponent(int sequence, boolean focal, Reference coverage) { 2782 super(); 2783 this.setSequence(sequence); 2784 this.setFocal(focal); 2785 this.setCoverage(coverage); 2786 } 2787 2788 /** 2789 * @return {@link #sequence} (A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2790 */ 2791 public PositiveIntType getSequenceElement() { 2792 if (this.sequence == null) 2793 if (Configuration.errorOnAutoCreate()) 2794 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 2795 else if (Configuration.doAutoCreate()) 2796 this.sequence = new PositiveIntType(); // bb 2797 return this.sequence; 2798 } 2799 2800 public boolean hasSequenceElement() { 2801 return this.sequence != null && !this.sequence.isEmpty(); 2802 } 2803 2804 public boolean hasSequence() { 2805 return this.sequence != null && !this.sequence.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #sequence} (A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2810 */ 2811 public InsuranceComponent setSequenceElement(PositiveIntType value) { 2812 this.sequence = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 2818 */ 2819 public int getSequence() { 2820 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2821 } 2822 2823 /** 2824 * @param value A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 2825 */ 2826 public InsuranceComponent setSequence(int value) { 2827 if (this.sequence == null) 2828 this.sequence = new PositiveIntType(); 2829 this.sequence.setValue(value); 2830 return this; 2831 } 2832 2833 /** 2834 * @return {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2835 */ 2836 public BooleanType getFocalElement() { 2837 if (this.focal == null) 2838 if (Configuration.errorOnAutoCreate()) 2839 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 2840 else if (Configuration.doAutoCreate()) 2841 this.focal = new BooleanType(); // bb 2842 return this.focal; 2843 } 2844 2845 public boolean hasFocalElement() { 2846 return this.focal != null && !this.focal.isEmpty(); 2847 } 2848 2849 public boolean hasFocal() { 2850 return this.focal != null && !this.focal.isEmpty(); 2851 } 2852 2853 /** 2854 * @param value {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2855 */ 2856 public InsuranceComponent setFocalElement(BooleanType value) { 2857 this.focal = value; 2858 return this; 2859 } 2860 2861 /** 2862 * @return A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2863 */ 2864 public boolean getFocal() { 2865 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 2866 } 2867 2868 /** 2869 * @param value A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2870 */ 2871 public InsuranceComponent setFocal(boolean value) { 2872 if (this.focal == null) 2873 this.focal = new BooleanType(); 2874 this.focal.setValue(value); 2875 return this; 2876 } 2877 2878 /** 2879 * @return {@link #identifier} (The business identifier to be used when the claim is sent for adjudication against this insurance policy.) 2880 */ 2881 public Identifier getIdentifier() { 2882 if (this.identifier == null) 2883 if (Configuration.errorOnAutoCreate()) 2884 throw new Error("Attempt to auto-create InsuranceComponent.identifier"); 2885 else if (Configuration.doAutoCreate()) 2886 this.identifier = new Identifier(); // cc 2887 return this.identifier; 2888 } 2889 2890 public boolean hasIdentifier() { 2891 return this.identifier != null && !this.identifier.isEmpty(); 2892 } 2893 2894 /** 2895 * @param value {@link #identifier} (The business identifier to be used when the claim is sent for adjudication against this insurance policy.) 2896 */ 2897 public InsuranceComponent setIdentifier(Identifier value) { 2898 this.identifier = value; 2899 return this; 2900 } 2901 2902 /** 2903 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2904 */ 2905 public Reference getCoverage() { 2906 if (this.coverage == null) 2907 if (Configuration.errorOnAutoCreate()) 2908 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2909 else if (Configuration.doAutoCreate()) 2910 this.coverage = new Reference(); // cc 2911 return this.coverage; 2912 } 2913 2914 public boolean hasCoverage() { 2915 return this.coverage != null && !this.coverage.isEmpty(); 2916 } 2917 2918 /** 2919 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2920 */ 2921 public InsuranceComponent setCoverage(Reference value) { 2922 this.coverage = value; 2923 return this; 2924 } 2925 2926 /** 2927 * @return {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2928 */ 2929 public StringType getBusinessArrangementElement() { 2930 if (this.businessArrangement == null) 2931 if (Configuration.errorOnAutoCreate()) 2932 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 2933 else if (Configuration.doAutoCreate()) 2934 this.businessArrangement = new StringType(); // bb 2935 return this.businessArrangement; 2936 } 2937 2938 public boolean hasBusinessArrangementElement() { 2939 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2940 } 2941 2942 public boolean hasBusinessArrangement() { 2943 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 2944 } 2945 2946 /** 2947 * @param value {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 2948 */ 2949 public InsuranceComponent setBusinessArrangementElement(StringType value) { 2950 this.businessArrangement = value; 2951 return this; 2952 } 2953 2954 /** 2955 * @return A business agreement number established between the provider and the insurer for special business processing purposes. 2956 */ 2957 public String getBusinessArrangement() { 2958 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 2959 } 2960 2961 /** 2962 * @param value A business agreement number established between the provider and the insurer for special business processing purposes. 2963 */ 2964 public InsuranceComponent setBusinessArrangement(String value) { 2965 if (Utilities.noString(value)) 2966 this.businessArrangement = null; 2967 else { 2968 if (this.businessArrangement == null) 2969 this.businessArrangement = new StringType(); 2970 this.businessArrangement.setValue(value); 2971 } 2972 return this; 2973 } 2974 2975 /** 2976 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2977 */ 2978 public List<StringType> getPreAuthRef() { 2979 if (this.preAuthRef == null) 2980 this.preAuthRef = new ArrayList<StringType>(); 2981 return this.preAuthRef; 2982 } 2983 2984 /** 2985 * @return Returns a reference to <code>this</code> for easy method chaining 2986 */ 2987 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2988 this.preAuthRef = thePreAuthRef; 2989 return this; 2990 } 2991 2992 public boolean hasPreAuthRef() { 2993 if (this.preAuthRef == null) 2994 return false; 2995 for (StringType item : this.preAuthRef) 2996 if (!item.isEmpty()) 2997 return true; 2998 return false; 2999 } 3000 3001 /** 3002 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 3003 */ 3004 public StringType addPreAuthRefElement() {//2 3005 StringType t = new StringType(); 3006 if (this.preAuthRef == null) 3007 this.preAuthRef = new ArrayList<StringType>(); 3008 this.preAuthRef.add(t); 3009 return t; 3010 } 3011 3012 /** 3013 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 3014 */ 3015 public InsuranceComponent addPreAuthRef(String value) { //1 3016 StringType t = new StringType(); 3017 t.setValue(value); 3018 if (this.preAuthRef == null) 3019 this.preAuthRef = new ArrayList<StringType>(); 3020 this.preAuthRef.add(t); 3021 return this; 3022 } 3023 3024 /** 3025 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 3026 */ 3027 public boolean hasPreAuthRef(String value) { 3028 if (this.preAuthRef == null) 3029 return false; 3030 for (StringType v : this.preAuthRef) 3031 if (v.getValue().equals(value)) // string 3032 return true; 3033 return false; 3034 } 3035 3036 /** 3037 * @return {@link #claimResponse} (The result of the adjudication of the line items for the Coverage specified in this insurance.) 3038 */ 3039 public Reference getClaimResponse() { 3040 if (this.claimResponse == null) 3041 if (Configuration.errorOnAutoCreate()) 3042 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 3043 else if (Configuration.doAutoCreate()) 3044 this.claimResponse = new Reference(); // cc 3045 return this.claimResponse; 3046 } 3047 3048 public boolean hasClaimResponse() { 3049 return this.claimResponse != null && !this.claimResponse.isEmpty(); 3050 } 3051 3052 /** 3053 * @param value {@link #claimResponse} (The result of the adjudication of the line items for the Coverage specified in this insurance.) 3054 */ 3055 public InsuranceComponent setClaimResponse(Reference value) { 3056 this.claimResponse = value; 3057 return this; 3058 } 3059 3060 protected void listChildren(List<Property> children) { 3061 super.listChildren(children); 3062 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 0, 1, sequence)); 3063 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal)); 3064 children.add(new Property("identifier", "Identifier", "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 0, 1, identifier)); 3065 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 3066 children.add(new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement)); 3067 children.add(new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 3068 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, claimResponse)); 3069 } 3070 3071 @Override 3072 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3073 switch (_hash) { 3074 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 0, 1, sequence); 3075 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal); 3076 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier to be used when the claim is sent for adjudication against this insurance policy.", 0, 1, identifier); 3077 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 3078 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement); 3079 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 3080 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, claimResponse); 3081 default: return super.getNamedProperty(_hash, _name, _checkValid); 3082 } 3083 3084 } 3085 3086 @Override 3087 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3088 switch (hash) { 3089 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 3090 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 3091 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3092 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 3093 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 3094 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 3095 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 3096 default: return super.getProperty(hash, name, checkValid); 3097 } 3098 3099 } 3100 3101 @Override 3102 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3103 switch (hash) { 3104 case 1349547969: // sequence 3105 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 3106 return value; 3107 case 97604197: // focal 3108 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 3109 return value; 3110 case -1618432855: // identifier 3111 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3112 return value; 3113 case -351767064: // coverage 3114 this.coverage = TypeConvertor.castToReference(value); // Reference 3115 return value; 3116 case 259920682: // businessArrangement 3117 this.businessArrangement = TypeConvertor.castToString(value); // StringType 3118 return value; 3119 case 522246568: // preAuthRef 3120 this.getPreAuthRef().add(TypeConvertor.castToString(value)); // StringType 3121 return value; 3122 case 689513629: // claimResponse 3123 this.claimResponse = TypeConvertor.castToReference(value); // Reference 3124 return value; 3125 default: return super.setProperty(hash, name, value); 3126 } 3127 3128 } 3129 3130 @Override 3131 public Base setProperty(String name, Base value) throws FHIRException { 3132 if (name.equals("sequence")) { 3133 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 3134 } else if (name.equals("focal")) { 3135 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 3136 } else if (name.equals("identifier")) { 3137 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3138 } else if (name.equals("coverage")) { 3139 this.coverage = TypeConvertor.castToReference(value); // Reference 3140 } else if (name.equals("businessArrangement")) { 3141 this.businessArrangement = TypeConvertor.castToString(value); // StringType 3142 } else if (name.equals("preAuthRef")) { 3143 this.getPreAuthRef().add(TypeConvertor.castToString(value)); 3144 } else if (name.equals("claimResponse")) { 3145 this.claimResponse = TypeConvertor.castToReference(value); // Reference 3146 } else 3147 return super.setProperty(name, value); 3148 return value; 3149 } 3150 3151 @Override 3152 public void removeChild(String name, Base value) throws FHIRException { 3153 if (name.equals("sequence")) { 3154 this.sequence = null; 3155 } else if (name.equals("focal")) { 3156 this.focal = null; 3157 } else if (name.equals("identifier")) { 3158 this.identifier = null; 3159 } else if (name.equals("coverage")) { 3160 this.coverage = null; 3161 } else if (name.equals("businessArrangement")) { 3162 this.businessArrangement = null; 3163 } else if (name.equals("preAuthRef")) { 3164 this.getPreAuthRef().remove(value); 3165 } else if (name.equals("claimResponse")) { 3166 this.claimResponse = null; 3167 } else 3168 super.removeChild(name, value); 3169 3170 } 3171 3172 @Override 3173 public Base makeProperty(int hash, String name) throws FHIRException { 3174 switch (hash) { 3175 case 1349547969: return getSequenceElement(); 3176 case 97604197: return getFocalElement(); 3177 case -1618432855: return getIdentifier(); 3178 case -351767064: return getCoverage(); 3179 case 259920682: return getBusinessArrangementElement(); 3180 case 522246568: return addPreAuthRefElement(); 3181 case 689513629: return getClaimResponse(); 3182 default: return super.makeProperty(hash, name); 3183 } 3184 3185 } 3186 3187 @Override 3188 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3189 switch (hash) { 3190 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 3191 case 97604197: /*focal*/ return new String[] {"boolean"}; 3192 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3193 case -351767064: /*coverage*/ return new String[] {"Reference"}; 3194 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 3195 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 3196 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 3197 default: return super.getTypesForProperty(hash, name); 3198 } 3199 3200 } 3201 3202 @Override 3203 public Base addChild(String name) throws FHIRException { 3204 if (name.equals("sequence")) { 3205 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.sequence"); 3206 } 3207 else if (name.equals("focal")) { 3208 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.focal"); 3209 } 3210 else if (name.equals("identifier")) { 3211 this.identifier = new Identifier(); 3212 return this.identifier; 3213 } 3214 else if (name.equals("coverage")) { 3215 this.coverage = new Reference(); 3216 return this.coverage; 3217 } 3218 else if (name.equals("businessArrangement")) { 3219 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.businessArrangement"); 3220 } 3221 else if (name.equals("preAuthRef")) { 3222 throw new FHIRException("Cannot call addChild on a singleton property Claim.insurance.preAuthRef"); 3223 } 3224 else if (name.equals("claimResponse")) { 3225 this.claimResponse = new Reference(); 3226 return this.claimResponse; 3227 } 3228 else 3229 return super.addChild(name); 3230 } 3231 3232 public InsuranceComponent copy() { 3233 InsuranceComponent dst = new InsuranceComponent(); 3234 copyValues(dst); 3235 return dst; 3236 } 3237 3238 public void copyValues(InsuranceComponent dst) { 3239 super.copyValues(dst); 3240 dst.sequence = sequence == null ? null : sequence.copy(); 3241 dst.focal = focal == null ? null : focal.copy(); 3242 dst.identifier = identifier == null ? null : identifier.copy(); 3243 dst.coverage = coverage == null ? null : coverage.copy(); 3244 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3245 if (preAuthRef != null) { 3246 dst.preAuthRef = new ArrayList<StringType>(); 3247 for (StringType i : preAuthRef) 3248 dst.preAuthRef.add(i.copy()); 3249 }; 3250 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3251 } 3252 3253 @Override 3254 public boolean equalsDeep(Base other_) { 3255 if (!super.equalsDeep(other_)) 3256 return false; 3257 if (!(other_ instanceof InsuranceComponent)) 3258 return false; 3259 InsuranceComponent o = (InsuranceComponent) other_; 3260 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) && compareDeep(identifier, o.identifier, true) 3261 && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 3262 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(claimResponse, o.claimResponse, true) 3263 ; 3264 } 3265 3266 @Override 3267 public boolean equalsShallow(Base other_) { 3268 if (!super.equalsShallow(other_)) 3269 return false; 3270 if (!(other_ instanceof InsuranceComponent)) 3271 return false; 3272 InsuranceComponent o = (InsuranceComponent) other_; 3273 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 3274 && compareValues(preAuthRef, o.preAuthRef, true); 3275 } 3276 3277 public boolean isEmpty() { 3278 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, identifier 3279 , coverage, businessArrangement, preAuthRef, claimResponse); 3280 } 3281 3282 public String fhirType() { 3283 return "Claim.insurance"; 3284 3285 } 3286 3287 } 3288 3289 @Block() 3290 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3291 /** 3292 * Date of an accident event related to the products and services contained in the claim. 3293 */ 3294 @Child(name = "date", type = {DateType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3295 @Description(shortDefinition="When the incident occurred", formalDefinition="Date of an accident event related to the products and services contained in the claim." ) 3296 protected DateType date; 3297 3298 /** 3299 * The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers. 3300 */ 3301 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3302 @Description(shortDefinition="The nature of the accident", formalDefinition="The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers." ) 3303 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 3304 protected CodeableConcept type; 3305 3306 /** 3307 * The physical location of the accident event. 3308 */ 3309 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 3310 @Description(shortDefinition="Where the event occurred", formalDefinition="The physical location of the accident event." ) 3311 protected DataType location; 3312 3313 private static final long serialVersionUID = 11882722L; 3314 3315 /** 3316 * Constructor 3317 */ 3318 public AccidentComponent() { 3319 super(); 3320 } 3321 3322 /** 3323 * Constructor 3324 */ 3325 public AccidentComponent(Date date) { 3326 super(); 3327 this.setDate(date); 3328 } 3329 3330 /** 3331 * @return {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3332 */ 3333 public DateType getDateElement() { 3334 if (this.date == null) 3335 if (Configuration.errorOnAutoCreate()) 3336 throw new Error("Attempt to auto-create AccidentComponent.date"); 3337 else if (Configuration.doAutoCreate()) 3338 this.date = new DateType(); // bb 3339 return this.date; 3340 } 3341 3342 public boolean hasDateElement() { 3343 return this.date != null && !this.date.isEmpty(); 3344 } 3345 3346 public boolean hasDate() { 3347 return this.date != null && !this.date.isEmpty(); 3348 } 3349 3350 /** 3351 * @param value {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3352 */ 3353 public AccidentComponent setDateElement(DateType value) { 3354 this.date = value; 3355 return this; 3356 } 3357 3358 /** 3359 * @return Date of an accident event related to the products and services contained in the claim. 3360 */ 3361 public Date getDate() { 3362 return this.date == null ? null : this.date.getValue(); 3363 } 3364 3365 /** 3366 * @param value Date of an accident event related to the products and services contained in the claim. 3367 */ 3368 public AccidentComponent setDate(Date value) { 3369 if (this.date == null) 3370 this.date = new DateType(); 3371 this.date.setValue(value); 3372 return this; 3373 } 3374 3375 /** 3376 * @return {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3377 */ 3378 public CodeableConcept getType() { 3379 if (this.type == null) 3380 if (Configuration.errorOnAutoCreate()) 3381 throw new Error("Attempt to auto-create AccidentComponent.type"); 3382 else if (Configuration.doAutoCreate()) 3383 this.type = new CodeableConcept(); // cc 3384 return this.type; 3385 } 3386 3387 public boolean hasType() { 3388 return this.type != null && !this.type.isEmpty(); 3389 } 3390 3391 /** 3392 * @param value {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3393 */ 3394 public AccidentComponent setType(CodeableConcept value) { 3395 this.type = value; 3396 return this; 3397 } 3398 3399 /** 3400 * @return {@link #location} (The physical location of the accident event.) 3401 */ 3402 public DataType getLocation() { 3403 return this.location; 3404 } 3405 3406 /** 3407 * @return {@link #location} (The physical location of the accident event.) 3408 */ 3409 public Address getLocationAddress() throws FHIRException { 3410 if (this.location == null) 3411 this.location = new Address(); 3412 if (!(this.location instanceof Address)) 3413 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3414 return (Address) this.location; 3415 } 3416 3417 public boolean hasLocationAddress() { 3418 return this != null && this.location instanceof Address; 3419 } 3420 3421 /** 3422 * @return {@link #location} (The physical location of the accident event.) 3423 */ 3424 public Reference getLocationReference() throws FHIRException { 3425 if (this.location == null) 3426 this.location = new Reference(); 3427 if (!(this.location instanceof Reference)) 3428 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3429 return (Reference) this.location; 3430 } 3431 3432 public boolean hasLocationReference() { 3433 return this != null && this.location instanceof Reference; 3434 } 3435 3436 public boolean hasLocation() { 3437 return this.location != null && !this.location.isEmpty(); 3438 } 3439 3440 /** 3441 * @param value {@link #location} (The physical location of the accident event.) 3442 */ 3443 public AccidentComponent setLocation(DataType value) { 3444 if (value != null && !(value instanceof Address || value instanceof Reference)) 3445 throw new FHIRException("Not the right type for Claim.accident.location[x]: "+value.fhirType()); 3446 this.location = value; 3447 return this; 3448 } 3449 3450 protected void listChildren(List<Property> children) { 3451 super.listChildren(children); 3452 children.add(new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 3453 children.add(new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type)); 3454 children.add(new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location)); 3455 } 3456 3457 @Override 3458 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3459 switch (_hash) { 3460 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 3461 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type); 3462 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3463 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3464 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "The physical location of the accident event.", 0, 1, location); 3465 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3466 default: return super.getNamedProperty(_hash, _name, _checkValid); 3467 } 3468 3469 } 3470 3471 @Override 3472 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3473 switch (hash) { 3474 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3475 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3476 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 3477 default: return super.getProperty(hash, name, checkValid); 3478 } 3479 3480 } 3481 3482 @Override 3483 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3484 switch (hash) { 3485 case 3076014: // date 3486 this.date = TypeConvertor.castToDate(value); // DateType 3487 return value; 3488 case 3575610: // type 3489 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3490 return value; 3491 case 1901043637: // location 3492 this.location = TypeConvertor.castToType(value); // DataType 3493 return value; 3494 default: return super.setProperty(hash, name, value); 3495 } 3496 3497 } 3498 3499 @Override 3500 public Base setProperty(String name, Base value) throws FHIRException { 3501 if (name.equals("date")) { 3502 this.date = TypeConvertor.castToDate(value); // DateType 3503 } else if (name.equals("type")) { 3504 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3505 } else if (name.equals("location[x]")) { 3506 this.location = TypeConvertor.castToType(value); // DataType 3507 } else 3508 return super.setProperty(name, value); 3509 return value; 3510 } 3511 3512 @Override 3513 public void removeChild(String name, Base value) throws FHIRException { 3514 if (name.equals("date")) { 3515 this.date = null; 3516 } else if (name.equals("type")) { 3517 this.type = null; 3518 } else if (name.equals("location[x]")) { 3519 this.location = null; 3520 } else 3521 super.removeChild(name, value); 3522 3523 } 3524 3525 @Override 3526 public Base makeProperty(int hash, String name) throws FHIRException { 3527 switch (hash) { 3528 case 3076014: return getDateElement(); 3529 case 3575610: return getType(); 3530 case 552316075: return getLocation(); 3531 case 1901043637: return getLocation(); 3532 default: return super.makeProperty(hash, name); 3533 } 3534 3535 } 3536 3537 @Override 3538 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3539 switch (hash) { 3540 case 3076014: /*date*/ return new String[] {"date"}; 3541 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3542 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 3543 default: return super.getTypesForProperty(hash, name); 3544 } 3545 3546 } 3547 3548 @Override 3549 public Base addChild(String name) throws FHIRException { 3550 if (name.equals("date")) { 3551 throw new FHIRException("Cannot call addChild on a singleton property Claim.accident.date"); 3552 } 3553 else if (name.equals("type")) { 3554 this.type = new CodeableConcept(); 3555 return this.type; 3556 } 3557 else if (name.equals("locationAddress")) { 3558 this.location = new Address(); 3559 return this.location; 3560 } 3561 else if (name.equals("locationReference")) { 3562 this.location = new Reference(); 3563 return this.location; 3564 } 3565 else 3566 return super.addChild(name); 3567 } 3568 3569 public AccidentComponent copy() { 3570 AccidentComponent dst = new AccidentComponent(); 3571 copyValues(dst); 3572 return dst; 3573 } 3574 3575 public void copyValues(AccidentComponent dst) { 3576 super.copyValues(dst); 3577 dst.date = date == null ? null : date.copy(); 3578 dst.type = type == null ? null : type.copy(); 3579 dst.location = location == null ? null : location.copy(); 3580 } 3581 3582 @Override 3583 public boolean equalsDeep(Base other_) { 3584 if (!super.equalsDeep(other_)) 3585 return false; 3586 if (!(other_ instanceof AccidentComponent)) 3587 return false; 3588 AccidentComponent o = (AccidentComponent) other_; 3589 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 3590 ; 3591 } 3592 3593 @Override 3594 public boolean equalsShallow(Base other_) { 3595 if (!super.equalsShallow(other_)) 3596 return false; 3597 if (!(other_ instanceof AccidentComponent)) 3598 return false; 3599 AccidentComponent o = (AccidentComponent) other_; 3600 return compareValues(date, o.date, true); 3601 } 3602 3603 public boolean isEmpty() { 3604 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 3605 } 3606 3607 public String fhirType() { 3608 return "Claim.accident"; 3609 3610 } 3611 3612 } 3613 3614 @Block() 3615 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 3616 /** 3617 * A number to uniquely identify item entries. 3618 */ 3619 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3620 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 3621 protected PositiveIntType sequence; 3622 3623 /** 3624 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 3625 */ 3626 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3627 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 3628 protected List<Identifier> traceNumber; 3629 3630 /** 3631 * CareTeam members related to this service or product. 3632 */ 3633 @Child(name = "careTeamSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3634 @Description(shortDefinition="Applicable careTeam members", formalDefinition="CareTeam members related to this service or product." ) 3635 protected List<PositiveIntType> careTeamSequence; 3636 3637 /** 3638 * Diagnosis applicable for this service or product. 3639 */ 3640 @Child(name = "diagnosisSequence", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3641 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnosis applicable for this service or product." ) 3642 protected List<PositiveIntType> diagnosisSequence; 3643 3644 /** 3645 * Procedures applicable for this service or product. 3646 */ 3647 @Child(name = "procedureSequence", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3648 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product." ) 3649 protected List<PositiveIntType> procedureSequence; 3650 3651 /** 3652 * Exceptions, special conditions and supporting information applicable for this service or product. 3653 */ 3654 @Child(name = "informationSequence", type = {PositiveIntType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3655 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information applicable for this service or product." ) 3656 protected List<PositiveIntType> informationSequence; 3657 3658 /** 3659 * The type of revenue or cost center providing the product and/or service. 3660 */ 3661 @Child(name = "revenue", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 3662 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 3663 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 3664 protected CodeableConcept revenue; 3665 3666 /** 3667 * Code to identify the general type of benefits under which products and services are provided. 3668 */ 3669 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3670 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 3671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 3672 protected CodeableConcept category; 3673 3674 /** 3675 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 3676 */ 3677 @Child(name = "productOrService", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 3678 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 3679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3680 protected CodeableConcept productOrService; 3681 3682 /** 3683 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 3684 */ 3685 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 3686 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 3687 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3688 protected CodeableConcept productOrServiceEnd; 3689 3690 /** 3691 * Request or Referral for Goods or Service to be rendered. 3692 */ 3693 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3694 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 3695 protected List<Reference> request; 3696 3697 /** 3698 * Item typification or modifiers codes to convey additional context for the product or service. 3699 */ 3700 @Child(name = "modifier", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3701 @Description(shortDefinition="Product or service billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 3702 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3703 protected List<CodeableConcept> modifier; 3704 3705 /** 3706 * Identifies the program under which this may be recovered. 3707 */ 3708 @Child(name = "programCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3709 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 3710 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3711 protected List<CodeableConcept> programCode; 3712 3713 /** 3714 * The date or dates when the service or product was supplied, performed or completed. 3715 */ 3716 @Child(name = "serviced", type = {DateType.class, Period.class}, order=14, min=0, max=1, modifier=false, summary=false) 3717 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 3718 protected DataType serviced; 3719 3720 /** 3721 * Where the product or service was provided. 3722 */ 3723 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=15, min=0, max=1, modifier=false, summary=false) 3724 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 3725 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3726 protected DataType location; 3727 3728 /** 3729 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 3730 */ 3731 @Child(name = "patientPaid", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3732 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 3733 protected Money patientPaid; 3734 3735 /** 3736 * The number of repetitions of a service or product. 3737 */ 3738 @Child(name = "quantity", type = {Quantity.class}, order=17, min=0, max=1, modifier=false, summary=false) 3739 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 3740 protected Quantity quantity; 3741 3742 /** 3743 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 3744 */ 3745 @Child(name = "unitPrice", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 3746 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 3747 protected Money unitPrice; 3748 3749 /** 3750 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3751 */ 3752 @Child(name = "factor", type = {DecimalType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3753 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3754 protected DecimalType factor; 3755 3756 /** 3757 * The total of taxes applicable for this product or service. 3758 */ 3759 @Child(name = "tax", type = {Money.class}, order=20, min=0, max=1, modifier=false, summary=false) 3760 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 3761 protected Money tax; 3762 3763 /** 3764 * The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor. 3765 */ 3766 @Child(name = "net", type = {Money.class}, order=21, min=0, max=1, modifier=false, summary=false) 3767 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor." ) 3768 protected Money net; 3769 3770 /** 3771 * Unique Device Identifiers associated with this line item. 3772 */ 3773 @Child(name = "udi", type = {Device.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3774 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 3775 protected List<Reference> udi; 3776 3777 /** 3778 * Physical location where the service is performed or applies. 3779 */ 3780 @Child(name = "bodySite", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3781 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 3782 protected List<BodySiteComponent> bodySite; 3783 3784 /** 3785 * Healthcare encounters related to this claim. 3786 */ 3787 @Child(name = "encounter", type = {Encounter.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3788 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 3789 protected List<Reference> encounter; 3790 3791 /** 3792 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 3793 */ 3794 @Child(name = "detail", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3795 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 3796 protected List<DetailComponent> detail; 3797 3798 private static final long serialVersionUID = -1174144448L; 3799 3800 /** 3801 * Constructor 3802 */ 3803 public ItemComponent() { 3804 super(); 3805 } 3806 3807 /** 3808 * Constructor 3809 */ 3810 public ItemComponent(int sequence) { 3811 super(); 3812 this.setSequence(sequence); 3813 } 3814 3815 /** 3816 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3817 */ 3818 public PositiveIntType getSequenceElement() { 3819 if (this.sequence == null) 3820 if (Configuration.errorOnAutoCreate()) 3821 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3822 else if (Configuration.doAutoCreate()) 3823 this.sequence = new PositiveIntType(); // bb 3824 return this.sequence; 3825 } 3826 3827 public boolean hasSequenceElement() { 3828 return this.sequence != null && !this.sequence.isEmpty(); 3829 } 3830 3831 public boolean hasSequence() { 3832 return this.sequence != null && !this.sequence.isEmpty(); 3833 } 3834 3835 /** 3836 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3837 */ 3838 public ItemComponent setSequenceElement(PositiveIntType value) { 3839 this.sequence = value; 3840 return this; 3841 } 3842 3843 /** 3844 * @return A number to uniquely identify item entries. 3845 */ 3846 public int getSequence() { 3847 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3848 } 3849 3850 /** 3851 * @param value A number to uniquely identify item entries. 3852 */ 3853 public ItemComponent setSequence(int value) { 3854 if (this.sequence == null) 3855 this.sequence = new PositiveIntType(); 3856 this.sequence.setValue(value); 3857 return this; 3858 } 3859 3860 /** 3861 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 3862 */ 3863 public List<Identifier> getTraceNumber() { 3864 if (this.traceNumber == null) 3865 this.traceNumber = new ArrayList<Identifier>(); 3866 return this.traceNumber; 3867 } 3868 3869 /** 3870 * @return Returns a reference to <code>this</code> for easy method chaining 3871 */ 3872 public ItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 3873 this.traceNumber = theTraceNumber; 3874 return this; 3875 } 3876 3877 public boolean hasTraceNumber() { 3878 if (this.traceNumber == null) 3879 return false; 3880 for (Identifier item : this.traceNumber) 3881 if (!item.isEmpty()) 3882 return true; 3883 return false; 3884 } 3885 3886 public Identifier addTraceNumber() { //3 3887 Identifier t = new Identifier(); 3888 if (this.traceNumber == null) 3889 this.traceNumber = new ArrayList<Identifier>(); 3890 this.traceNumber.add(t); 3891 return t; 3892 } 3893 3894 public ItemComponent addTraceNumber(Identifier t) { //3 3895 if (t == null) 3896 return this; 3897 if (this.traceNumber == null) 3898 this.traceNumber = new ArrayList<Identifier>(); 3899 this.traceNumber.add(t); 3900 return this; 3901 } 3902 3903 /** 3904 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 3905 */ 3906 public Identifier getTraceNumberFirstRep() { 3907 if (getTraceNumber().isEmpty()) { 3908 addTraceNumber(); 3909 } 3910 return getTraceNumber().get(0); 3911 } 3912 3913 /** 3914 * @return {@link #careTeamSequence} (CareTeam members related to this service or product.) 3915 */ 3916 public List<PositiveIntType> getCareTeamSequence() { 3917 if (this.careTeamSequence == null) 3918 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3919 return this.careTeamSequence; 3920 } 3921 3922 /** 3923 * @return Returns a reference to <code>this</code> for easy method chaining 3924 */ 3925 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 3926 this.careTeamSequence = theCareTeamSequence; 3927 return this; 3928 } 3929 3930 public boolean hasCareTeamSequence() { 3931 if (this.careTeamSequence == null) 3932 return false; 3933 for (PositiveIntType item : this.careTeamSequence) 3934 if (!item.isEmpty()) 3935 return true; 3936 return false; 3937 } 3938 3939 /** 3940 * @return {@link #careTeamSequence} (CareTeam members related to this service or product.) 3941 */ 3942 public PositiveIntType addCareTeamSequenceElement() {//2 3943 PositiveIntType t = new PositiveIntType(); 3944 if (this.careTeamSequence == null) 3945 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3946 this.careTeamSequence.add(t); 3947 return t; 3948 } 3949 3950 /** 3951 * @param value {@link #careTeamSequence} (CareTeam members related to this service or product.) 3952 */ 3953 public ItemComponent addCareTeamSequence(int value) { //1 3954 PositiveIntType t = new PositiveIntType(); 3955 t.setValue(value); 3956 if (this.careTeamSequence == null) 3957 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3958 this.careTeamSequence.add(t); 3959 return this; 3960 } 3961 3962 /** 3963 * @param value {@link #careTeamSequence} (CareTeam members related to this service or product.) 3964 */ 3965 public boolean hasCareTeamSequence(int value) { 3966 if (this.careTeamSequence == null) 3967 return false; 3968 for (PositiveIntType v : this.careTeamSequence) 3969 if (v.getValue().equals(value)) // positiveInt 3970 return true; 3971 return false; 3972 } 3973 3974 /** 3975 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 3976 */ 3977 public List<PositiveIntType> getDiagnosisSequence() { 3978 if (this.diagnosisSequence == null) 3979 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3980 return this.diagnosisSequence; 3981 } 3982 3983 /** 3984 * @return Returns a reference to <code>this</code> for easy method chaining 3985 */ 3986 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 3987 this.diagnosisSequence = theDiagnosisSequence; 3988 return this; 3989 } 3990 3991 public boolean hasDiagnosisSequence() { 3992 if (this.diagnosisSequence == null) 3993 return false; 3994 for (PositiveIntType item : this.diagnosisSequence) 3995 if (!item.isEmpty()) 3996 return true; 3997 return false; 3998 } 3999 4000 /** 4001 * @return {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 4002 */ 4003 public PositiveIntType addDiagnosisSequenceElement() {//2 4004 PositiveIntType t = new PositiveIntType(); 4005 if (this.diagnosisSequence == null) 4006 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4007 this.diagnosisSequence.add(t); 4008 return t; 4009 } 4010 4011 /** 4012 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 4013 */ 4014 public ItemComponent addDiagnosisSequence(int value) { //1 4015 PositiveIntType t = new PositiveIntType(); 4016 t.setValue(value); 4017 if (this.diagnosisSequence == null) 4018 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4019 this.diagnosisSequence.add(t); 4020 return this; 4021 } 4022 4023 /** 4024 * @param value {@link #diagnosisSequence} (Diagnosis applicable for this service or product.) 4025 */ 4026 public boolean hasDiagnosisSequence(int value) { 4027 if (this.diagnosisSequence == null) 4028 return false; 4029 for (PositiveIntType v : this.diagnosisSequence) 4030 if (v.getValue().equals(value)) // positiveInt 4031 return true; 4032 return false; 4033 } 4034 4035 /** 4036 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 4037 */ 4038 public List<PositiveIntType> getProcedureSequence() { 4039 if (this.procedureSequence == null) 4040 this.procedureSequence = new ArrayList<PositiveIntType>(); 4041 return this.procedureSequence; 4042 } 4043 4044 /** 4045 * @return Returns a reference to <code>this</code> for easy method chaining 4046 */ 4047 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 4048 this.procedureSequence = theProcedureSequence; 4049 return this; 4050 } 4051 4052 public boolean hasProcedureSequence() { 4053 if (this.procedureSequence == null) 4054 return false; 4055 for (PositiveIntType item : this.procedureSequence) 4056 if (!item.isEmpty()) 4057 return true; 4058 return false; 4059 } 4060 4061 /** 4062 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 4063 */ 4064 public PositiveIntType addProcedureSequenceElement() {//2 4065 PositiveIntType t = new PositiveIntType(); 4066 if (this.procedureSequence == null) 4067 this.procedureSequence = new ArrayList<PositiveIntType>(); 4068 this.procedureSequence.add(t); 4069 return t; 4070 } 4071 4072 /** 4073 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 4074 */ 4075 public ItemComponent addProcedureSequence(int value) { //1 4076 PositiveIntType t = new PositiveIntType(); 4077 t.setValue(value); 4078 if (this.procedureSequence == null) 4079 this.procedureSequence = new ArrayList<PositiveIntType>(); 4080 this.procedureSequence.add(t); 4081 return this; 4082 } 4083 4084 /** 4085 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 4086 */ 4087 public boolean hasProcedureSequence(int value) { 4088 if (this.procedureSequence == null) 4089 return false; 4090 for (PositiveIntType v : this.procedureSequence) 4091 if (v.getValue().equals(value)) // positiveInt 4092 return true; 4093 return false; 4094 } 4095 4096 /** 4097 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 4098 */ 4099 public List<PositiveIntType> getInformationSequence() { 4100 if (this.informationSequence == null) 4101 this.informationSequence = new ArrayList<PositiveIntType>(); 4102 return this.informationSequence; 4103 } 4104 4105 /** 4106 * @return Returns a reference to <code>this</code> for easy method chaining 4107 */ 4108 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 4109 this.informationSequence = theInformationSequence; 4110 return this; 4111 } 4112 4113 public boolean hasInformationSequence() { 4114 if (this.informationSequence == null) 4115 return false; 4116 for (PositiveIntType item : this.informationSequence) 4117 if (!item.isEmpty()) 4118 return true; 4119 return false; 4120 } 4121 4122 /** 4123 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 4124 */ 4125 public PositiveIntType addInformationSequenceElement() {//2 4126 PositiveIntType t = new PositiveIntType(); 4127 if (this.informationSequence == null) 4128 this.informationSequence = new ArrayList<PositiveIntType>(); 4129 this.informationSequence.add(t); 4130 return t; 4131 } 4132 4133 /** 4134 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 4135 */ 4136 public ItemComponent addInformationSequence(int value) { //1 4137 PositiveIntType t = new PositiveIntType(); 4138 t.setValue(value); 4139 if (this.informationSequence == null) 4140 this.informationSequence = new ArrayList<PositiveIntType>(); 4141 this.informationSequence.add(t); 4142 return this; 4143 } 4144 4145 /** 4146 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 4147 */ 4148 public boolean hasInformationSequence(int value) { 4149 if (this.informationSequence == null) 4150 return false; 4151 for (PositiveIntType v : this.informationSequence) 4152 if (v.getValue().equals(value)) // positiveInt 4153 return true; 4154 return false; 4155 } 4156 4157 /** 4158 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4159 */ 4160 public CodeableConcept getRevenue() { 4161 if (this.revenue == null) 4162 if (Configuration.errorOnAutoCreate()) 4163 throw new Error("Attempt to auto-create ItemComponent.revenue"); 4164 else if (Configuration.doAutoCreate()) 4165 this.revenue = new CodeableConcept(); // cc 4166 return this.revenue; 4167 } 4168 4169 public boolean hasRevenue() { 4170 return this.revenue != null && !this.revenue.isEmpty(); 4171 } 4172 4173 /** 4174 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4175 */ 4176 public ItemComponent setRevenue(CodeableConcept value) { 4177 this.revenue = value; 4178 return this; 4179 } 4180 4181 /** 4182 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 4183 */ 4184 public CodeableConcept getCategory() { 4185 if (this.category == null) 4186 if (Configuration.errorOnAutoCreate()) 4187 throw new Error("Attempt to auto-create ItemComponent.category"); 4188 else if (Configuration.doAutoCreate()) 4189 this.category = new CodeableConcept(); // cc 4190 return this.category; 4191 } 4192 4193 public boolean hasCategory() { 4194 return this.category != null && !this.category.isEmpty(); 4195 } 4196 4197 /** 4198 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 4199 */ 4200 public ItemComponent setCategory(CodeableConcept value) { 4201 this.category = value; 4202 return this; 4203 } 4204 4205 /** 4206 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4207 */ 4208 public CodeableConcept getProductOrService() { 4209 if (this.productOrService == null) 4210 if (Configuration.errorOnAutoCreate()) 4211 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 4212 else if (Configuration.doAutoCreate()) 4213 this.productOrService = new CodeableConcept(); // cc 4214 return this.productOrService; 4215 } 4216 4217 public boolean hasProductOrService() { 4218 return this.productOrService != null && !this.productOrService.isEmpty(); 4219 } 4220 4221 /** 4222 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4223 */ 4224 public ItemComponent setProductOrService(CodeableConcept value) { 4225 this.productOrService = value; 4226 return this; 4227 } 4228 4229 /** 4230 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4231 */ 4232 public CodeableConcept getProductOrServiceEnd() { 4233 if (this.productOrServiceEnd == null) 4234 if (Configuration.errorOnAutoCreate()) 4235 throw new Error("Attempt to auto-create ItemComponent.productOrServiceEnd"); 4236 else if (Configuration.doAutoCreate()) 4237 this.productOrServiceEnd = new CodeableConcept(); // cc 4238 return this.productOrServiceEnd; 4239 } 4240 4241 public boolean hasProductOrServiceEnd() { 4242 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 4243 } 4244 4245 /** 4246 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4247 */ 4248 public ItemComponent setProductOrServiceEnd(CodeableConcept value) { 4249 this.productOrServiceEnd = value; 4250 return this; 4251 } 4252 4253 /** 4254 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 4255 */ 4256 public List<Reference> getRequest() { 4257 if (this.request == null) 4258 this.request = new ArrayList<Reference>(); 4259 return this.request; 4260 } 4261 4262 /** 4263 * @return Returns a reference to <code>this</code> for easy method chaining 4264 */ 4265 public ItemComponent setRequest(List<Reference> theRequest) { 4266 this.request = theRequest; 4267 return this; 4268 } 4269 4270 public boolean hasRequest() { 4271 if (this.request == null) 4272 return false; 4273 for (Reference item : this.request) 4274 if (!item.isEmpty()) 4275 return true; 4276 return false; 4277 } 4278 4279 public Reference addRequest() { //3 4280 Reference t = new Reference(); 4281 if (this.request == null) 4282 this.request = new ArrayList<Reference>(); 4283 this.request.add(t); 4284 return t; 4285 } 4286 4287 public ItemComponent addRequest(Reference t) { //3 4288 if (t == null) 4289 return this; 4290 if (this.request == null) 4291 this.request = new ArrayList<Reference>(); 4292 this.request.add(t); 4293 return this; 4294 } 4295 4296 /** 4297 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 4298 */ 4299 public Reference getRequestFirstRep() { 4300 if (getRequest().isEmpty()) { 4301 addRequest(); 4302 } 4303 return getRequest().get(0); 4304 } 4305 4306 /** 4307 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 4308 */ 4309 public List<CodeableConcept> getModifier() { 4310 if (this.modifier == null) 4311 this.modifier = new ArrayList<CodeableConcept>(); 4312 return this.modifier; 4313 } 4314 4315 /** 4316 * @return Returns a reference to <code>this</code> for easy method chaining 4317 */ 4318 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 4319 this.modifier = theModifier; 4320 return this; 4321 } 4322 4323 public boolean hasModifier() { 4324 if (this.modifier == null) 4325 return false; 4326 for (CodeableConcept item : this.modifier) 4327 if (!item.isEmpty()) 4328 return true; 4329 return false; 4330 } 4331 4332 public CodeableConcept addModifier() { //3 4333 CodeableConcept t = new CodeableConcept(); 4334 if (this.modifier == null) 4335 this.modifier = new ArrayList<CodeableConcept>(); 4336 this.modifier.add(t); 4337 return t; 4338 } 4339 4340 public ItemComponent addModifier(CodeableConcept t) { //3 4341 if (t == null) 4342 return this; 4343 if (this.modifier == null) 4344 this.modifier = new ArrayList<CodeableConcept>(); 4345 this.modifier.add(t); 4346 return this; 4347 } 4348 4349 /** 4350 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 4351 */ 4352 public CodeableConcept getModifierFirstRep() { 4353 if (getModifier().isEmpty()) { 4354 addModifier(); 4355 } 4356 return getModifier().get(0); 4357 } 4358 4359 /** 4360 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 4361 */ 4362 public List<CodeableConcept> getProgramCode() { 4363 if (this.programCode == null) 4364 this.programCode = new ArrayList<CodeableConcept>(); 4365 return this.programCode; 4366 } 4367 4368 /** 4369 * @return Returns a reference to <code>this</code> for easy method chaining 4370 */ 4371 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 4372 this.programCode = theProgramCode; 4373 return this; 4374 } 4375 4376 public boolean hasProgramCode() { 4377 if (this.programCode == null) 4378 return false; 4379 for (CodeableConcept item : this.programCode) 4380 if (!item.isEmpty()) 4381 return true; 4382 return false; 4383 } 4384 4385 public CodeableConcept addProgramCode() { //3 4386 CodeableConcept t = new CodeableConcept(); 4387 if (this.programCode == null) 4388 this.programCode = new ArrayList<CodeableConcept>(); 4389 this.programCode.add(t); 4390 return t; 4391 } 4392 4393 public ItemComponent addProgramCode(CodeableConcept t) { //3 4394 if (t == null) 4395 return this; 4396 if (this.programCode == null) 4397 this.programCode = new ArrayList<CodeableConcept>(); 4398 this.programCode.add(t); 4399 return this; 4400 } 4401 4402 /** 4403 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 4404 */ 4405 public CodeableConcept getProgramCodeFirstRep() { 4406 if (getProgramCode().isEmpty()) { 4407 addProgramCode(); 4408 } 4409 return getProgramCode().get(0); 4410 } 4411 4412 /** 4413 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4414 */ 4415 public DataType getServiced() { 4416 return this.serviced; 4417 } 4418 4419 /** 4420 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4421 */ 4422 public DateType getServicedDateType() throws FHIRException { 4423 if (this.serviced == null) 4424 this.serviced = new DateType(); 4425 if (!(this.serviced instanceof DateType)) 4426 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4427 return (DateType) this.serviced; 4428 } 4429 4430 public boolean hasServicedDateType() { 4431 return this != null && this.serviced instanceof DateType; 4432 } 4433 4434 /** 4435 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4436 */ 4437 public Period getServicedPeriod() throws FHIRException { 4438 if (this.serviced == null) 4439 this.serviced = new Period(); 4440 if (!(this.serviced instanceof Period)) 4441 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4442 return (Period) this.serviced; 4443 } 4444 4445 public boolean hasServicedPeriod() { 4446 return this != null && this.serviced instanceof Period; 4447 } 4448 4449 public boolean hasServiced() { 4450 return this.serviced != null && !this.serviced.isEmpty(); 4451 } 4452 4453 /** 4454 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4455 */ 4456 public ItemComponent setServiced(DataType value) { 4457 if (value != null && !(value instanceof DateType || value instanceof Period)) 4458 throw new FHIRException("Not the right type for Claim.item.serviced[x]: "+value.fhirType()); 4459 this.serviced = value; 4460 return this; 4461 } 4462 4463 /** 4464 * @return {@link #location} (Where the product or service was provided.) 4465 */ 4466 public DataType getLocation() { 4467 return this.location; 4468 } 4469 4470 /** 4471 * @return {@link #location} (Where the product or service was provided.) 4472 */ 4473 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 4474 if (this.location == null) 4475 this.location = new CodeableConcept(); 4476 if (!(this.location instanceof CodeableConcept)) 4477 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 4478 return (CodeableConcept) this.location; 4479 } 4480 4481 public boolean hasLocationCodeableConcept() { 4482 return this != null && this.location instanceof CodeableConcept; 4483 } 4484 4485 /** 4486 * @return {@link #location} (Where the product or service was provided.) 4487 */ 4488 public Address getLocationAddress() throws FHIRException { 4489 if (this.location == null) 4490 this.location = new Address(); 4491 if (!(this.location instanceof Address)) 4492 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 4493 return (Address) this.location; 4494 } 4495 4496 public boolean hasLocationAddress() { 4497 return this != null && this.location instanceof Address; 4498 } 4499 4500 /** 4501 * @return {@link #location} (Where the product or service was provided.) 4502 */ 4503 public Reference getLocationReference() throws FHIRException { 4504 if (this.location == null) 4505 this.location = new Reference(); 4506 if (!(this.location instanceof Reference)) 4507 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 4508 return (Reference) this.location; 4509 } 4510 4511 public boolean hasLocationReference() { 4512 return this != null && this.location instanceof Reference; 4513 } 4514 4515 public boolean hasLocation() { 4516 return this.location != null && !this.location.isEmpty(); 4517 } 4518 4519 /** 4520 * @param value {@link #location} (Where the product or service was provided.) 4521 */ 4522 public ItemComponent setLocation(DataType value) { 4523 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 4524 throw new FHIRException("Not the right type for Claim.item.location[x]: "+value.fhirType()); 4525 this.location = value; 4526 return this; 4527 } 4528 4529 /** 4530 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4531 */ 4532 public Money getPatientPaid() { 4533 if (this.patientPaid == null) 4534 if (Configuration.errorOnAutoCreate()) 4535 throw new Error("Attempt to auto-create ItemComponent.patientPaid"); 4536 else if (Configuration.doAutoCreate()) 4537 this.patientPaid = new Money(); // cc 4538 return this.patientPaid; 4539 } 4540 4541 public boolean hasPatientPaid() { 4542 return this.patientPaid != null && !this.patientPaid.isEmpty(); 4543 } 4544 4545 /** 4546 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4547 */ 4548 public ItemComponent setPatientPaid(Money value) { 4549 this.patientPaid = value; 4550 return this; 4551 } 4552 4553 /** 4554 * @return {@link #quantity} (The number of repetitions of a service or product.) 4555 */ 4556 public Quantity getQuantity() { 4557 if (this.quantity == null) 4558 if (Configuration.errorOnAutoCreate()) 4559 throw new Error("Attempt to auto-create ItemComponent.quantity"); 4560 else if (Configuration.doAutoCreate()) 4561 this.quantity = new Quantity(); // cc 4562 return this.quantity; 4563 } 4564 4565 public boolean hasQuantity() { 4566 return this.quantity != null && !this.quantity.isEmpty(); 4567 } 4568 4569 /** 4570 * @param value {@link #quantity} (The number of repetitions of a service or product.) 4571 */ 4572 public ItemComponent setQuantity(Quantity value) { 4573 this.quantity = value; 4574 return this; 4575 } 4576 4577 /** 4578 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4579 */ 4580 public Money getUnitPrice() { 4581 if (this.unitPrice == null) 4582 if (Configuration.errorOnAutoCreate()) 4583 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 4584 else if (Configuration.doAutoCreate()) 4585 this.unitPrice = new Money(); // cc 4586 return this.unitPrice; 4587 } 4588 4589 public boolean hasUnitPrice() { 4590 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4591 } 4592 4593 /** 4594 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4595 */ 4596 public ItemComponent setUnitPrice(Money value) { 4597 this.unitPrice = value; 4598 return this; 4599 } 4600 4601 /** 4602 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4603 */ 4604 public DecimalType getFactorElement() { 4605 if (this.factor == null) 4606 if (Configuration.errorOnAutoCreate()) 4607 throw new Error("Attempt to auto-create ItemComponent.factor"); 4608 else if (Configuration.doAutoCreate()) 4609 this.factor = new DecimalType(); // bb 4610 return this.factor; 4611 } 4612 4613 public boolean hasFactorElement() { 4614 return this.factor != null && !this.factor.isEmpty(); 4615 } 4616 4617 public boolean hasFactor() { 4618 return this.factor != null && !this.factor.isEmpty(); 4619 } 4620 4621 /** 4622 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4623 */ 4624 public ItemComponent setFactorElement(DecimalType value) { 4625 this.factor = value; 4626 return this; 4627 } 4628 4629 /** 4630 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4631 */ 4632 public BigDecimal getFactor() { 4633 return this.factor == null ? null : this.factor.getValue(); 4634 } 4635 4636 /** 4637 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4638 */ 4639 public ItemComponent setFactor(BigDecimal value) { 4640 if (value == null) 4641 this.factor = null; 4642 else { 4643 if (this.factor == null) 4644 this.factor = new DecimalType(); 4645 this.factor.setValue(value); 4646 } 4647 return this; 4648 } 4649 4650 /** 4651 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4652 */ 4653 public ItemComponent setFactor(long value) { 4654 this.factor = new DecimalType(); 4655 this.factor.setValue(value); 4656 return this; 4657 } 4658 4659 /** 4660 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4661 */ 4662 public ItemComponent setFactor(double value) { 4663 this.factor = new DecimalType(); 4664 this.factor.setValue(value); 4665 return this; 4666 } 4667 4668 /** 4669 * @return {@link #tax} (The total of taxes applicable for this product or service.) 4670 */ 4671 public Money getTax() { 4672 if (this.tax == null) 4673 if (Configuration.errorOnAutoCreate()) 4674 throw new Error("Attempt to auto-create ItemComponent.tax"); 4675 else if (Configuration.doAutoCreate()) 4676 this.tax = new Money(); // cc 4677 return this.tax; 4678 } 4679 4680 public boolean hasTax() { 4681 return this.tax != null && !this.tax.isEmpty(); 4682 } 4683 4684 /** 4685 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 4686 */ 4687 public ItemComponent setTax(Money value) { 4688 this.tax = value; 4689 return this; 4690 } 4691 4692 /** 4693 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4694 */ 4695 public Money getNet() { 4696 if (this.net == null) 4697 if (Configuration.errorOnAutoCreate()) 4698 throw new Error("Attempt to auto-create ItemComponent.net"); 4699 else if (Configuration.doAutoCreate()) 4700 this.net = new Money(); // cc 4701 return this.net; 4702 } 4703 4704 public boolean hasNet() { 4705 return this.net != null && !this.net.isEmpty(); 4706 } 4707 4708 /** 4709 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4710 */ 4711 public ItemComponent setNet(Money value) { 4712 this.net = value; 4713 return this; 4714 } 4715 4716 /** 4717 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 4718 */ 4719 public List<Reference> getUdi() { 4720 if (this.udi == null) 4721 this.udi = new ArrayList<Reference>(); 4722 return this.udi; 4723 } 4724 4725 /** 4726 * @return Returns a reference to <code>this</code> for easy method chaining 4727 */ 4728 public ItemComponent setUdi(List<Reference> theUdi) { 4729 this.udi = theUdi; 4730 return this; 4731 } 4732 4733 public boolean hasUdi() { 4734 if (this.udi == null) 4735 return false; 4736 for (Reference item : this.udi) 4737 if (!item.isEmpty()) 4738 return true; 4739 return false; 4740 } 4741 4742 public Reference addUdi() { //3 4743 Reference t = new Reference(); 4744 if (this.udi == null) 4745 this.udi = new ArrayList<Reference>(); 4746 this.udi.add(t); 4747 return t; 4748 } 4749 4750 public ItemComponent addUdi(Reference t) { //3 4751 if (t == null) 4752 return this; 4753 if (this.udi == null) 4754 this.udi = new ArrayList<Reference>(); 4755 this.udi.add(t); 4756 return this; 4757 } 4758 4759 /** 4760 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 4761 */ 4762 public Reference getUdiFirstRep() { 4763 if (getUdi().isEmpty()) { 4764 addUdi(); 4765 } 4766 return getUdi().get(0); 4767 } 4768 4769 /** 4770 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 4771 */ 4772 public List<BodySiteComponent> getBodySite() { 4773 if (this.bodySite == null) 4774 this.bodySite = new ArrayList<BodySiteComponent>(); 4775 return this.bodySite; 4776 } 4777 4778 /** 4779 * @return Returns a reference to <code>this</code> for easy method chaining 4780 */ 4781 public ItemComponent setBodySite(List<BodySiteComponent> theBodySite) { 4782 this.bodySite = theBodySite; 4783 return this; 4784 } 4785 4786 public boolean hasBodySite() { 4787 if (this.bodySite == null) 4788 return false; 4789 for (BodySiteComponent item : this.bodySite) 4790 if (!item.isEmpty()) 4791 return true; 4792 return false; 4793 } 4794 4795 public BodySiteComponent addBodySite() { //3 4796 BodySiteComponent t = new BodySiteComponent(); 4797 if (this.bodySite == null) 4798 this.bodySite = new ArrayList<BodySiteComponent>(); 4799 this.bodySite.add(t); 4800 return t; 4801 } 4802 4803 public ItemComponent addBodySite(BodySiteComponent t) { //3 4804 if (t == null) 4805 return this; 4806 if (this.bodySite == null) 4807 this.bodySite = new ArrayList<BodySiteComponent>(); 4808 this.bodySite.add(t); 4809 return this; 4810 } 4811 4812 /** 4813 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 4814 */ 4815 public BodySiteComponent getBodySiteFirstRep() { 4816 if (getBodySite().isEmpty()) { 4817 addBodySite(); 4818 } 4819 return getBodySite().get(0); 4820 } 4821 4822 /** 4823 * @return {@link #encounter} (Healthcare encounters related to this claim.) 4824 */ 4825 public List<Reference> getEncounter() { 4826 if (this.encounter == null) 4827 this.encounter = new ArrayList<Reference>(); 4828 return this.encounter; 4829 } 4830 4831 /** 4832 * @return Returns a reference to <code>this</code> for easy method chaining 4833 */ 4834 public ItemComponent setEncounter(List<Reference> theEncounter) { 4835 this.encounter = theEncounter; 4836 return this; 4837 } 4838 4839 public boolean hasEncounter() { 4840 if (this.encounter == null) 4841 return false; 4842 for (Reference item : this.encounter) 4843 if (!item.isEmpty()) 4844 return true; 4845 return false; 4846 } 4847 4848 public Reference addEncounter() { //3 4849 Reference t = new Reference(); 4850 if (this.encounter == null) 4851 this.encounter = new ArrayList<Reference>(); 4852 this.encounter.add(t); 4853 return t; 4854 } 4855 4856 public ItemComponent addEncounter(Reference t) { //3 4857 if (t == null) 4858 return this; 4859 if (this.encounter == null) 4860 this.encounter = new ArrayList<Reference>(); 4861 this.encounter.add(t); 4862 return this; 4863 } 4864 4865 /** 4866 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 4867 */ 4868 public Reference getEncounterFirstRep() { 4869 if (getEncounter().isEmpty()) { 4870 addEncounter(); 4871 } 4872 return getEncounter().get(0); 4873 } 4874 4875 /** 4876 * @return {@link #detail} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.) 4877 */ 4878 public List<DetailComponent> getDetail() { 4879 if (this.detail == null) 4880 this.detail = new ArrayList<DetailComponent>(); 4881 return this.detail; 4882 } 4883 4884 /** 4885 * @return Returns a reference to <code>this</code> for easy method chaining 4886 */ 4887 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4888 this.detail = theDetail; 4889 return this; 4890 } 4891 4892 public boolean hasDetail() { 4893 if (this.detail == null) 4894 return false; 4895 for (DetailComponent item : this.detail) 4896 if (!item.isEmpty()) 4897 return true; 4898 return false; 4899 } 4900 4901 public DetailComponent addDetail() { //3 4902 DetailComponent t = new DetailComponent(); 4903 if (this.detail == null) 4904 this.detail = new ArrayList<DetailComponent>(); 4905 this.detail.add(t); 4906 return t; 4907 } 4908 4909 public ItemComponent addDetail(DetailComponent t) { //3 4910 if (t == null) 4911 return this; 4912 if (this.detail == null) 4913 this.detail = new ArrayList<DetailComponent>(); 4914 this.detail.add(t); 4915 return this; 4916 } 4917 4918 /** 4919 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 4920 */ 4921 public DetailComponent getDetailFirstRep() { 4922 if (getDetail().isEmpty()) { 4923 addDetail(); 4924 } 4925 return getDetail().get(0); 4926 } 4927 4928 protected void listChildren(List<Property> children) { 4929 super.listChildren(children); 4930 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 4931 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 4932 children.add(new Property("careTeamSequence", "positiveInt", "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 4933 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnosis applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 4934 children.add(new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 4935 children.add(new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence)); 4936 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 4937 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 4938 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 4939 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 4940 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 4941 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4942 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4943 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 4944 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 4945 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 4946 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4947 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 4948 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4949 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 4950 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net)); 4951 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4952 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 4953 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4954 children.add(new Property("detail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, detail)); 4955 } 4956 4957 @Override 4958 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4959 switch (_hash) { 4960 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 4961 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 4962 case 1070083823: /*careTeamSequence*/ return new Property("careTeamSequence", "positiveInt", "CareTeam members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 4963 case -909769262: /*diagnosisSequence*/ return new Property("diagnosisSequence", "positiveInt", "Diagnosis applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 4964 case -808920140: /*procedureSequence*/ return new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 4965 case -702585587: /*informationSequence*/ return new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence); 4966 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 4967 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 4968 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 4969 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 4970 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 4971 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 4972 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 4973 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4974 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4975 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4976 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4977 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4978 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4979 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 4980 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 4981 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4982 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 4983 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4984 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 4985 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4986 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 4987 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net); 4988 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4989 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 4990 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 4991 case -1335224239: /*detail*/ return new Property("detail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, detail); 4992 default: return super.getNamedProperty(_hash, _name, _checkValid); 4993 } 4994 4995 } 4996 4997 @Override 4998 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4999 switch (hash) { 5000 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 5001 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 5002 case 1070083823: /*careTeamSequence*/ return this.careTeamSequence == null ? new Base[0] : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 5003 case -909769262: /*diagnosisSequence*/ return this.diagnosisSequence == null ? new Base[0] : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 5004 case -808920140: /*procedureSequence*/ return this.procedureSequence == null ? new Base[0] : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 5005 case -702585587: /*informationSequence*/ return this.informationSequence == null ? new Base[0] : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 5006 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5007 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 5008 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 5009 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 5010 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 5011 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5012 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5013 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 5014 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 5015 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 5016 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 5017 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5018 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5019 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 5020 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5021 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5022 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // BodySiteComponent 5023 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 5024 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 5025 default: return super.getProperty(hash, name, checkValid); 5026 } 5027 5028 } 5029 5030 @Override 5031 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5032 switch (hash) { 5033 case 1349547969: // sequence 5034 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 5035 return value; 5036 case 82505966: // traceNumber 5037 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 5038 return value; 5039 case 1070083823: // careTeamSequence 5040 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5041 return value; 5042 case -909769262: // diagnosisSequence 5043 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5044 return value; 5045 case -808920140: // procedureSequence 5046 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5047 return value; 5048 case -702585587: // informationSequence 5049 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5050 return value; 5051 case 1099842588: // revenue 5052 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5053 return value; 5054 case 50511102: // category 5055 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5056 return value; 5057 case 1957227299: // productOrService 5058 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5059 return value; 5060 case -717476168: // productOrServiceEnd 5061 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5062 return value; 5063 case 1095692943: // request 5064 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 5065 return value; 5066 case -615513385: // modifier 5067 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5068 return value; 5069 case 1010065041: // programCode 5070 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5071 return value; 5072 case 1379209295: // serviced 5073 this.serviced = TypeConvertor.castToType(value); // DataType 5074 return value; 5075 case 1901043637: // location 5076 this.location = TypeConvertor.castToType(value); // DataType 5077 return value; 5078 case 525514609: // patientPaid 5079 this.patientPaid = TypeConvertor.castToMoney(value); // Money 5080 return value; 5081 case -1285004149: // quantity 5082 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5083 return value; 5084 case -486196699: // unitPrice 5085 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5086 return value; 5087 case -1282148017: // factor 5088 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5089 return value; 5090 case 114603: // tax 5091 this.tax = TypeConvertor.castToMoney(value); // Money 5092 return value; 5093 case 108957: // net 5094 this.net = TypeConvertor.castToMoney(value); // Money 5095 return value; 5096 case 115642: // udi 5097 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 5098 return value; 5099 case 1702620169: // bodySite 5100 this.getBodySite().add((BodySiteComponent) value); // BodySiteComponent 5101 return value; 5102 case 1524132147: // encounter 5103 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 5104 return value; 5105 case -1335224239: // detail 5106 this.getDetail().add((DetailComponent) value); // DetailComponent 5107 return value; 5108 default: return super.setProperty(hash, name, value); 5109 } 5110 5111 } 5112 5113 @Override 5114 public Base setProperty(String name, Base value) throws FHIRException { 5115 if (name.equals("sequence")) { 5116 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 5117 } else if (name.equals("traceNumber")) { 5118 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 5119 } else if (name.equals("careTeamSequence")) { 5120 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); 5121 } else if (name.equals("diagnosisSequence")) { 5122 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); 5123 } else if (name.equals("procedureSequence")) { 5124 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); 5125 } else if (name.equals("informationSequence")) { 5126 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); 5127 } else if (name.equals("revenue")) { 5128 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5129 } else if (name.equals("category")) { 5130 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5131 } else if (name.equals("productOrService")) { 5132 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5133 } else if (name.equals("productOrServiceEnd")) { 5134 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5135 } else if (name.equals("request")) { 5136 this.getRequest().add(TypeConvertor.castToReference(value)); 5137 } else if (name.equals("modifier")) { 5138 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 5139 } else if (name.equals("programCode")) { 5140 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 5141 } else if (name.equals("serviced[x]")) { 5142 this.serviced = TypeConvertor.castToType(value); // DataType 5143 } else if (name.equals("location[x]")) { 5144 this.location = TypeConvertor.castToType(value); // DataType 5145 } else if (name.equals("patientPaid")) { 5146 this.patientPaid = TypeConvertor.castToMoney(value); // Money 5147 } else if (name.equals("quantity")) { 5148 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5149 } else if (name.equals("unitPrice")) { 5150 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5151 } else if (name.equals("factor")) { 5152 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5153 } else if (name.equals("tax")) { 5154 this.tax = TypeConvertor.castToMoney(value); // Money 5155 } else if (name.equals("net")) { 5156 this.net = TypeConvertor.castToMoney(value); // Money 5157 } else if (name.equals("udi")) { 5158 this.getUdi().add(TypeConvertor.castToReference(value)); 5159 } else if (name.equals("bodySite")) { 5160 this.getBodySite().add((BodySiteComponent) value); 5161 } else if (name.equals("encounter")) { 5162 this.getEncounter().add(TypeConvertor.castToReference(value)); 5163 } else if (name.equals("detail")) { 5164 this.getDetail().add((DetailComponent) value); 5165 } else 5166 return super.setProperty(name, value); 5167 return value; 5168 } 5169 5170 @Override 5171 public void removeChild(String name, Base value) throws FHIRException { 5172 if (name.equals("sequence")) { 5173 this.sequence = null; 5174 } else if (name.equals("traceNumber")) { 5175 this.getTraceNumber().remove(value); 5176 } else if (name.equals("careTeamSequence")) { 5177 this.getCareTeamSequence().remove(value); 5178 } else if (name.equals("diagnosisSequence")) { 5179 this.getDiagnosisSequence().remove(value); 5180 } else if (name.equals("procedureSequence")) { 5181 this.getProcedureSequence().remove(value); 5182 } else if (name.equals("informationSequence")) { 5183 this.getInformationSequence().remove(value); 5184 } else if (name.equals("revenue")) { 5185 this.revenue = null; 5186 } else if (name.equals("category")) { 5187 this.category = null; 5188 } else if (name.equals("productOrService")) { 5189 this.productOrService = null; 5190 } else if (name.equals("productOrServiceEnd")) { 5191 this.productOrServiceEnd = null; 5192 } else if (name.equals("request")) { 5193 this.getRequest().remove(value); 5194 } else if (name.equals("modifier")) { 5195 this.getModifier().remove(value); 5196 } else if (name.equals("programCode")) { 5197 this.getProgramCode().remove(value); 5198 } else if (name.equals("serviced[x]")) { 5199 this.serviced = null; 5200 } else if (name.equals("location[x]")) { 5201 this.location = null; 5202 } else if (name.equals("patientPaid")) { 5203 this.patientPaid = null; 5204 } else if (name.equals("quantity")) { 5205 this.quantity = null; 5206 } else if (name.equals("unitPrice")) { 5207 this.unitPrice = null; 5208 } else if (name.equals("factor")) { 5209 this.factor = null; 5210 } else if (name.equals("tax")) { 5211 this.tax = null; 5212 } else if (name.equals("net")) { 5213 this.net = null; 5214 } else if (name.equals("udi")) { 5215 this.getUdi().remove(value); 5216 } else if (name.equals("bodySite")) { 5217 this.getBodySite().remove((BodySiteComponent) value); 5218 } else if (name.equals("encounter")) { 5219 this.getEncounter().remove(value); 5220 } else if (name.equals("detail")) { 5221 this.getDetail().remove((DetailComponent) value); 5222 } else 5223 super.removeChild(name, value); 5224 5225 } 5226 5227 @Override 5228 public Base makeProperty(int hash, String name) throws FHIRException { 5229 switch (hash) { 5230 case 1349547969: return getSequenceElement(); 5231 case 82505966: return addTraceNumber(); 5232 case 1070083823: return addCareTeamSequenceElement(); 5233 case -909769262: return addDiagnosisSequenceElement(); 5234 case -808920140: return addProcedureSequenceElement(); 5235 case -702585587: return addInformationSequenceElement(); 5236 case 1099842588: return getRevenue(); 5237 case 50511102: return getCategory(); 5238 case 1957227299: return getProductOrService(); 5239 case -717476168: return getProductOrServiceEnd(); 5240 case 1095692943: return addRequest(); 5241 case -615513385: return addModifier(); 5242 case 1010065041: return addProgramCode(); 5243 case -1927922223: return getServiced(); 5244 case 1379209295: return getServiced(); 5245 case 552316075: return getLocation(); 5246 case 1901043637: return getLocation(); 5247 case 525514609: return getPatientPaid(); 5248 case -1285004149: return getQuantity(); 5249 case -486196699: return getUnitPrice(); 5250 case -1282148017: return getFactorElement(); 5251 case 114603: return getTax(); 5252 case 108957: return getNet(); 5253 case 115642: return addUdi(); 5254 case 1702620169: return addBodySite(); 5255 case 1524132147: return addEncounter(); 5256 case -1335224239: return addDetail(); 5257 default: return super.makeProperty(hash, name); 5258 } 5259 5260 } 5261 5262 @Override 5263 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5264 switch (hash) { 5265 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 5266 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 5267 case 1070083823: /*careTeamSequence*/ return new String[] {"positiveInt"}; 5268 case -909769262: /*diagnosisSequence*/ return new String[] {"positiveInt"}; 5269 case -808920140: /*procedureSequence*/ return new String[] {"positiveInt"}; 5270 case -702585587: /*informationSequence*/ return new String[] {"positiveInt"}; 5271 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5272 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5273 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 5274 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 5275 case 1095692943: /*request*/ return new String[] {"Reference"}; 5276 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5277 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 5278 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 5279 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 5280 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 5281 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 5282 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5283 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5284 case 114603: /*tax*/ return new String[] {"Money"}; 5285 case 108957: /*net*/ return new String[] {"Money"}; 5286 case 115642: /*udi*/ return new String[] {"Reference"}; 5287 case 1702620169: /*bodySite*/ return new String[] {}; 5288 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5289 case -1335224239: /*detail*/ return new String[] {}; 5290 default: return super.getTypesForProperty(hash, name); 5291 } 5292 5293 } 5294 5295 @Override 5296 public Base addChild(String name) throws FHIRException { 5297 if (name.equals("sequence")) { 5298 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.sequence"); 5299 } 5300 else if (name.equals("traceNumber")) { 5301 return addTraceNumber(); 5302 } 5303 else if (name.equals("careTeamSequence")) { 5304 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.careTeamSequence"); 5305 } 5306 else if (name.equals("diagnosisSequence")) { 5307 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.diagnosisSequence"); 5308 } 5309 else if (name.equals("procedureSequence")) { 5310 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.procedureSequence"); 5311 } 5312 else if (name.equals("informationSequence")) { 5313 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.informationSequence"); 5314 } 5315 else if (name.equals("revenue")) { 5316 this.revenue = new CodeableConcept(); 5317 return this.revenue; 5318 } 5319 else if (name.equals("category")) { 5320 this.category = new CodeableConcept(); 5321 return this.category; 5322 } 5323 else if (name.equals("productOrService")) { 5324 this.productOrService = new CodeableConcept(); 5325 return this.productOrService; 5326 } 5327 else if (name.equals("productOrServiceEnd")) { 5328 this.productOrServiceEnd = new CodeableConcept(); 5329 return this.productOrServiceEnd; 5330 } 5331 else if (name.equals("request")) { 5332 return addRequest(); 5333 } 5334 else if (name.equals("modifier")) { 5335 return addModifier(); 5336 } 5337 else if (name.equals("programCode")) { 5338 return addProgramCode(); 5339 } 5340 else if (name.equals("servicedDate")) { 5341 this.serviced = new DateType(); 5342 return this.serviced; 5343 } 5344 else if (name.equals("servicedPeriod")) { 5345 this.serviced = new Period(); 5346 return this.serviced; 5347 } 5348 else if (name.equals("locationCodeableConcept")) { 5349 this.location = new CodeableConcept(); 5350 return this.location; 5351 } 5352 else if (name.equals("locationAddress")) { 5353 this.location = new Address(); 5354 return this.location; 5355 } 5356 else if (name.equals("locationReference")) { 5357 this.location = new Reference(); 5358 return this.location; 5359 } 5360 else if (name.equals("patientPaid")) { 5361 this.patientPaid = new Money(); 5362 return this.patientPaid; 5363 } 5364 else if (name.equals("quantity")) { 5365 this.quantity = new Quantity(); 5366 return this.quantity; 5367 } 5368 else if (name.equals("unitPrice")) { 5369 this.unitPrice = new Money(); 5370 return this.unitPrice; 5371 } 5372 else if (name.equals("factor")) { 5373 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.factor"); 5374 } 5375 else if (name.equals("tax")) { 5376 this.tax = new Money(); 5377 return this.tax; 5378 } 5379 else if (name.equals("net")) { 5380 this.net = new Money(); 5381 return this.net; 5382 } 5383 else if (name.equals("udi")) { 5384 return addUdi(); 5385 } 5386 else if (name.equals("bodySite")) { 5387 return addBodySite(); 5388 } 5389 else if (name.equals("encounter")) { 5390 return addEncounter(); 5391 } 5392 else if (name.equals("detail")) { 5393 return addDetail(); 5394 } 5395 else 5396 return super.addChild(name); 5397 } 5398 5399 public ItemComponent copy() { 5400 ItemComponent dst = new ItemComponent(); 5401 copyValues(dst); 5402 return dst; 5403 } 5404 5405 public void copyValues(ItemComponent dst) { 5406 super.copyValues(dst); 5407 dst.sequence = sequence == null ? null : sequence.copy(); 5408 if (traceNumber != null) { 5409 dst.traceNumber = new ArrayList<Identifier>(); 5410 for (Identifier i : traceNumber) 5411 dst.traceNumber.add(i.copy()); 5412 }; 5413 if (careTeamSequence != null) { 5414 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 5415 for (PositiveIntType i : careTeamSequence) 5416 dst.careTeamSequence.add(i.copy()); 5417 }; 5418 if (diagnosisSequence != null) { 5419 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 5420 for (PositiveIntType i : diagnosisSequence) 5421 dst.diagnosisSequence.add(i.copy()); 5422 }; 5423 if (procedureSequence != null) { 5424 dst.procedureSequence = new ArrayList<PositiveIntType>(); 5425 for (PositiveIntType i : procedureSequence) 5426 dst.procedureSequence.add(i.copy()); 5427 }; 5428 if (informationSequence != null) { 5429 dst.informationSequence = new ArrayList<PositiveIntType>(); 5430 for (PositiveIntType i : informationSequence) 5431 dst.informationSequence.add(i.copy()); 5432 }; 5433 dst.revenue = revenue == null ? null : revenue.copy(); 5434 dst.category = category == null ? null : category.copy(); 5435 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5436 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 5437 if (request != null) { 5438 dst.request = new ArrayList<Reference>(); 5439 for (Reference i : request) 5440 dst.request.add(i.copy()); 5441 }; 5442 if (modifier != null) { 5443 dst.modifier = new ArrayList<CodeableConcept>(); 5444 for (CodeableConcept i : modifier) 5445 dst.modifier.add(i.copy()); 5446 }; 5447 if (programCode != null) { 5448 dst.programCode = new ArrayList<CodeableConcept>(); 5449 for (CodeableConcept i : programCode) 5450 dst.programCode.add(i.copy()); 5451 }; 5452 dst.serviced = serviced == null ? null : serviced.copy(); 5453 dst.location = location == null ? null : location.copy(); 5454 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 5455 dst.quantity = quantity == null ? null : quantity.copy(); 5456 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5457 dst.factor = factor == null ? null : factor.copy(); 5458 dst.tax = tax == null ? null : tax.copy(); 5459 dst.net = net == null ? null : net.copy(); 5460 if (udi != null) { 5461 dst.udi = new ArrayList<Reference>(); 5462 for (Reference i : udi) 5463 dst.udi.add(i.copy()); 5464 }; 5465 if (bodySite != null) { 5466 dst.bodySite = new ArrayList<BodySiteComponent>(); 5467 for (BodySiteComponent i : bodySite) 5468 dst.bodySite.add(i.copy()); 5469 }; 5470 if (encounter != null) { 5471 dst.encounter = new ArrayList<Reference>(); 5472 for (Reference i : encounter) 5473 dst.encounter.add(i.copy()); 5474 }; 5475 if (detail != null) { 5476 dst.detail = new ArrayList<DetailComponent>(); 5477 for (DetailComponent i : detail) 5478 dst.detail.add(i.copy()); 5479 }; 5480 } 5481 5482 @Override 5483 public boolean equalsDeep(Base other_) { 5484 if (!super.equalsDeep(other_)) 5485 return false; 5486 if (!(other_ instanceof ItemComponent)) 5487 return false; 5488 ItemComponent o = (ItemComponent) other_; 5489 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 5490 && compareDeep(careTeamSequence, o.careTeamSequence, true) && compareDeep(diagnosisSequence, o.diagnosisSequence, true) 5491 && compareDeep(procedureSequence, o.procedureSequence, true) && compareDeep(informationSequence, o.informationSequence, true) 5492 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 5493 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 5494 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 5495 && compareDeep(location, o.location, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) 5496 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) 5497 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 5498 && compareDeep(encounter, o.encounter, true) && compareDeep(detail, o.detail, true); 5499 } 5500 5501 @Override 5502 public boolean equalsShallow(Base other_) { 5503 if (!super.equalsShallow(other_)) 5504 return false; 5505 if (!(other_ instanceof ItemComponent)) 5506 return false; 5507 ItemComponent o = (ItemComponent) other_; 5508 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 5509 && compareValues(diagnosisSequence, o.diagnosisSequence, true) && compareValues(procedureSequence, o.procedureSequence, true) 5510 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true) 5511 ; 5512 } 5513 5514 public boolean isEmpty() { 5515 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, careTeamSequence 5516 , diagnosisSequence, procedureSequence, informationSequence, revenue, category, productOrService 5517 , productOrServiceEnd, request, modifier, programCode, serviced, location, patientPaid 5518 , quantity, unitPrice, factor, tax, net, udi, bodySite, encounter, detail 5519 ); 5520 } 5521 5522 public String fhirType() { 5523 return "Claim.item"; 5524 5525 } 5526 5527 } 5528 5529 @Block() 5530 public static class BodySiteComponent extends BackboneElement implements IBaseBackboneElement { 5531 /** 5532 * Physical service site on the patient (limb, tooth, etc.). 5533 */ 5534 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5535 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 5536 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 5537 protected List<CodeableReference> site; 5538 5539 /** 5540 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 5541 */ 5542 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5543 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 5544 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 5545 protected List<CodeableConcept> subSite; 5546 5547 private static final long serialVersionUID = 1190632415L; 5548 5549 /** 5550 * Constructor 5551 */ 5552 public BodySiteComponent() { 5553 super(); 5554 } 5555 5556 /** 5557 * Constructor 5558 */ 5559 public BodySiteComponent(CodeableReference site) { 5560 super(); 5561 this.addSite(site); 5562 } 5563 5564 /** 5565 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 5566 */ 5567 public List<CodeableReference> getSite() { 5568 if (this.site == null) 5569 this.site = new ArrayList<CodeableReference>(); 5570 return this.site; 5571 } 5572 5573 /** 5574 * @return Returns a reference to <code>this</code> for easy method chaining 5575 */ 5576 public BodySiteComponent setSite(List<CodeableReference> theSite) { 5577 this.site = theSite; 5578 return this; 5579 } 5580 5581 public boolean hasSite() { 5582 if (this.site == null) 5583 return false; 5584 for (CodeableReference item : this.site) 5585 if (!item.isEmpty()) 5586 return true; 5587 return false; 5588 } 5589 5590 public CodeableReference addSite() { //3 5591 CodeableReference t = new CodeableReference(); 5592 if (this.site == null) 5593 this.site = new ArrayList<CodeableReference>(); 5594 this.site.add(t); 5595 return t; 5596 } 5597 5598 public BodySiteComponent addSite(CodeableReference t) { //3 5599 if (t == null) 5600 return this; 5601 if (this.site == null) 5602 this.site = new ArrayList<CodeableReference>(); 5603 this.site.add(t); 5604 return this; 5605 } 5606 5607 /** 5608 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 5609 */ 5610 public CodeableReference getSiteFirstRep() { 5611 if (getSite().isEmpty()) { 5612 addSite(); 5613 } 5614 return getSite().get(0); 5615 } 5616 5617 /** 5618 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 5619 */ 5620 public List<CodeableConcept> getSubSite() { 5621 if (this.subSite == null) 5622 this.subSite = new ArrayList<CodeableConcept>(); 5623 return this.subSite; 5624 } 5625 5626 /** 5627 * @return Returns a reference to <code>this</code> for easy method chaining 5628 */ 5629 public BodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 5630 this.subSite = theSubSite; 5631 return this; 5632 } 5633 5634 public boolean hasSubSite() { 5635 if (this.subSite == null) 5636 return false; 5637 for (CodeableConcept item : this.subSite) 5638 if (!item.isEmpty()) 5639 return true; 5640 return false; 5641 } 5642 5643 public CodeableConcept addSubSite() { //3 5644 CodeableConcept t = new CodeableConcept(); 5645 if (this.subSite == null) 5646 this.subSite = new ArrayList<CodeableConcept>(); 5647 this.subSite.add(t); 5648 return t; 5649 } 5650 5651 public BodySiteComponent addSubSite(CodeableConcept t) { //3 5652 if (t == null) 5653 return this; 5654 if (this.subSite == null) 5655 this.subSite = new ArrayList<CodeableConcept>(); 5656 this.subSite.add(t); 5657 return this; 5658 } 5659 5660 /** 5661 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 5662 */ 5663 public CodeableConcept getSubSiteFirstRep() { 5664 if (getSubSite().isEmpty()) { 5665 addSubSite(); 5666 } 5667 return getSubSite().get(0); 5668 } 5669 5670 protected void listChildren(List<Property> children) { 5671 super.listChildren(children); 5672 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 5673 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 5674 } 5675 5676 @Override 5677 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5678 switch (_hash) { 5679 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 5680 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 5681 default: return super.getNamedProperty(_hash, _name, _checkValid); 5682 } 5683 5684 } 5685 5686 @Override 5687 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5688 switch (hash) { 5689 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 5690 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5691 default: return super.getProperty(hash, name, checkValid); 5692 } 5693 5694 } 5695 5696 @Override 5697 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5698 switch (hash) { 5699 case 3530567: // site 5700 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5701 return value; 5702 case -1868566105: // subSite 5703 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5704 return value; 5705 default: return super.setProperty(hash, name, value); 5706 } 5707 5708 } 5709 5710 @Override 5711 public Base setProperty(String name, Base value) throws FHIRException { 5712 if (name.equals("site")) { 5713 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 5714 } else if (name.equals("subSite")) { 5715 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 5716 } else 5717 return super.setProperty(name, value); 5718 return value; 5719 } 5720 5721 @Override 5722 public void removeChild(String name, Base value) throws FHIRException { 5723 if (name.equals("site")) { 5724 this.getSite().remove(value); 5725 } else if (name.equals("subSite")) { 5726 this.getSubSite().remove(value); 5727 } else 5728 super.removeChild(name, value); 5729 5730 } 5731 5732 @Override 5733 public Base makeProperty(int hash, String name) throws FHIRException { 5734 switch (hash) { 5735 case 3530567: return addSite(); 5736 case -1868566105: return addSubSite(); 5737 default: return super.makeProperty(hash, name); 5738 } 5739 5740 } 5741 5742 @Override 5743 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5744 switch (hash) { 5745 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 5746 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 5747 default: return super.getTypesForProperty(hash, name); 5748 } 5749 5750 } 5751 5752 @Override 5753 public Base addChild(String name) throws FHIRException { 5754 if (name.equals("site")) { 5755 return addSite(); 5756 } 5757 else if (name.equals("subSite")) { 5758 return addSubSite(); 5759 } 5760 else 5761 return super.addChild(name); 5762 } 5763 5764 public BodySiteComponent copy() { 5765 BodySiteComponent dst = new BodySiteComponent(); 5766 copyValues(dst); 5767 return dst; 5768 } 5769 5770 public void copyValues(BodySiteComponent dst) { 5771 super.copyValues(dst); 5772 if (site != null) { 5773 dst.site = new ArrayList<CodeableReference>(); 5774 for (CodeableReference i : site) 5775 dst.site.add(i.copy()); 5776 }; 5777 if (subSite != null) { 5778 dst.subSite = new ArrayList<CodeableConcept>(); 5779 for (CodeableConcept i : subSite) 5780 dst.subSite.add(i.copy()); 5781 }; 5782 } 5783 5784 @Override 5785 public boolean equalsDeep(Base other_) { 5786 if (!super.equalsDeep(other_)) 5787 return false; 5788 if (!(other_ instanceof BodySiteComponent)) 5789 return false; 5790 BodySiteComponent o = (BodySiteComponent) other_; 5791 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 5792 } 5793 5794 @Override 5795 public boolean equalsShallow(Base other_) { 5796 if (!super.equalsShallow(other_)) 5797 return false; 5798 if (!(other_ instanceof BodySiteComponent)) 5799 return false; 5800 BodySiteComponent o = (BodySiteComponent) other_; 5801 return true; 5802 } 5803 5804 public boolean isEmpty() { 5805 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 5806 } 5807 5808 public String fhirType() { 5809 return "Claim.item.bodySite"; 5810 5811 } 5812 5813 } 5814 5815 @Block() 5816 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 5817 /** 5818 * A number to uniquely identify item entries. 5819 */ 5820 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5821 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 5822 protected PositiveIntType sequence; 5823 5824 /** 5825 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 5826 */ 5827 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5828 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 5829 protected List<Identifier> traceNumber; 5830 5831 /** 5832 * The type of revenue or cost center providing the product and/or service. 5833 */ 5834 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5835 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 5836 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5837 protected CodeableConcept revenue; 5838 5839 /** 5840 * Code to identify the general type of benefits under which products and services are provided. 5841 */ 5842 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5843 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 5844 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 5845 protected CodeableConcept category; 5846 5847 /** 5848 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 5849 */ 5850 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 5851 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 5852 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5853 protected CodeableConcept productOrService; 5854 5855 /** 5856 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 5857 */ 5858 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 5859 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 5860 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5861 protected CodeableConcept productOrServiceEnd; 5862 5863 /** 5864 * Item typification or modifiers codes to convey additional context for the product or service. 5865 */ 5866 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5867 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 5868 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5869 protected List<CodeableConcept> modifier; 5870 5871 /** 5872 * Identifies the program under which this may be recovered. 5873 */ 5874 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5875 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 5876 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5877 protected List<CodeableConcept> programCode; 5878 5879 /** 5880 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 5881 */ 5882 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 5883 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 5884 protected Money patientPaid; 5885 5886 /** 5887 * The number of repetitions of a service or product. 5888 */ 5889 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 5890 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 5891 protected Quantity quantity; 5892 5893 /** 5894 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 5895 */ 5896 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 5897 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 5898 protected Money unitPrice; 5899 5900 /** 5901 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5902 */ 5903 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 5904 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5905 protected DecimalType factor; 5906 5907 /** 5908 * The total of taxes applicable for this product or service. 5909 */ 5910 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 5911 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 5912 protected Money tax; 5913 5914 /** 5915 * The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor. 5916 */ 5917 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 5918 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor." ) 5919 protected Money net; 5920 5921 /** 5922 * Unique Device Identifiers associated with this line item. 5923 */ 5924 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5925 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 5926 protected List<Reference> udi; 5927 5928 /** 5929 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 5930 */ 5931 @Child(name = "subDetail", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5932 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 5933 protected List<SubDetailComponent> subDetail; 5934 5935 private static final long serialVersionUID = -733030497L; 5936 5937 /** 5938 * Constructor 5939 */ 5940 public DetailComponent() { 5941 super(); 5942 } 5943 5944 /** 5945 * Constructor 5946 */ 5947 public DetailComponent(int sequence) { 5948 super(); 5949 this.setSequence(sequence); 5950 } 5951 5952 /** 5953 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5954 */ 5955 public PositiveIntType getSequenceElement() { 5956 if (this.sequence == null) 5957 if (Configuration.errorOnAutoCreate()) 5958 throw new Error("Attempt to auto-create DetailComponent.sequence"); 5959 else if (Configuration.doAutoCreate()) 5960 this.sequence = new PositiveIntType(); // bb 5961 return this.sequence; 5962 } 5963 5964 public boolean hasSequenceElement() { 5965 return this.sequence != null && !this.sequence.isEmpty(); 5966 } 5967 5968 public boolean hasSequence() { 5969 return this.sequence != null && !this.sequence.isEmpty(); 5970 } 5971 5972 /** 5973 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5974 */ 5975 public DetailComponent setSequenceElement(PositiveIntType value) { 5976 this.sequence = value; 5977 return this; 5978 } 5979 5980 /** 5981 * @return A number to uniquely identify item entries. 5982 */ 5983 public int getSequence() { 5984 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 5985 } 5986 5987 /** 5988 * @param value A number to uniquely identify item entries. 5989 */ 5990 public DetailComponent setSequence(int value) { 5991 if (this.sequence == null) 5992 this.sequence = new PositiveIntType(); 5993 this.sequence.setValue(value); 5994 return this; 5995 } 5996 5997 /** 5998 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 5999 */ 6000 public List<Identifier> getTraceNumber() { 6001 if (this.traceNumber == null) 6002 this.traceNumber = new ArrayList<Identifier>(); 6003 return this.traceNumber; 6004 } 6005 6006 /** 6007 * @return Returns a reference to <code>this</code> for easy method chaining 6008 */ 6009 public DetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 6010 this.traceNumber = theTraceNumber; 6011 return this; 6012 } 6013 6014 public boolean hasTraceNumber() { 6015 if (this.traceNumber == null) 6016 return false; 6017 for (Identifier item : this.traceNumber) 6018 if (!item.isEmpty()) 6019 return true; 6020 return false; 6021 } 6022 6023 public Identifier addTraceNumber() { //3 6024 Identifier t = new Identifier(); 6025 if (this.traceNumber == null) 6026 this.traceNumber = new ArrayList<Identifier>(); 6027 this.traceNumber.add(t); 6028 return t; 6029 } 6030 6031 public DetailComponent addTraceNumber(Identifier t) { //3 6032 if (t == null) 6033 return this; 6034 if (this.traceNumber == null) 6035 this.traceNumber = new ArrayList<Identifier>(); 6036 this.traceNumber.add(t); 6037 return this; 6038 } 6039 6040 /** 6041 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 6042 */ 6043 public Identifier getTraceNumberFirstRep() { 6044 if (getTraceNumber().isEmpty()) { 6045 addTraceNumber(); 6046 } 6047 return getTraceNumber().get(0); 6048 } 6049 6050 /** 6051 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6052 */ 6053 public CodeableConcept getRevenue() { 6054 if (this.revenue == null) 6055 if (Configuration.errorOnAutoCreate()) 6056 throw new Error("Attempt to auto-create DetailComponent.revenue"); 6057 else if (Configuration.doAutoCreate()) 6058 this.revenue = new CodeableConcept(); // cc 6059 return this.revenue; 6060 } 6061 6062 public boolean hasRevenue() { 6063 return this.revenue != null && !this.revenue.isEmpty(); 6064 } 6065 6066 /** 6067 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6068 */ 6069 public DetailComponent setRevenue(CodeableConcept value) { 6070 this.revenue = value; 6071 return this; 6072 } 6073 6074 /** 6075 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6076 */ 6077 public CodeableConcept getCategory() { 6078 if (this.category == null) 6079 if (Configuration.errorOnAutoCreate()) 6080 throw new Error("Attempt to auto-create DetailComponent.category"); 6081 else if (Configuration.doAutoCreate()) 6082 this.category = new CodeableConcept(); // cc 6083 return this.category; 6084 } 6085 6086 public boolean hasCategory() { 6087 return this.category != null && !this.category.isEmpty(); 6088 } 6089 6090 /** 6091 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6092 */ 6093 public DetailComponent setCategory(CodeableConcept value) { 6094 this.category = value; 6095 return this; 6096 } 6097 6098 /** 6099 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6100 */ 6101 public CodeableConcept getProductOrService() { 6102 if (this.productOrService == null) 6103 if (Configuration.errorOnAutoCreate()) 6104 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 6105 else if (Configuration.doAutoCreate()) 6106 this.productOrService = new CodeableConcept(); // cc 6107 return this.productOrService; 6108 } 6109 6110 public boolean hasProductOrService() { 6111 return this.productOrService != null && !this.productOrService.isEmpty(); 6112 } 6113 6114 /** 6115 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6116 */ 6117 public DetailComponent setProductOrService(CodeableConcept value) { 6118 this.productOrService = value; 6119 return this; 6120 } 6121 6122 /** 6123 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 6124 */ 6125 public CodeableConcept getProductOrServiceEnd() { 6126 if (this.productOrServiceEnd == null) 6127 if (Configuration.errorOnAutoCreate()) 6128 throw new Error("Attempt to auto-create DetailComponent.productOrServiceEnd"); 6129 else if (Configuration.doAutoCreate()) 6130 this.productOrServiceEnd = new CodeableConcept(); // cc 6131 return this.productOrServiceEnd; 6132 } 6133 6134 public boolean hasProductOrServiceEnd() { 6135 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 6136 } 6137 6138 /** 6139 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 6140 */ 6141 public DetailComponent setProductOrServiceEnd(CodeableConcept value) { 6142 this.productOrServiceEnd = value; 6143 return this; 6144 } 6145 6146 /** 6147 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 6148 */ 6149 public List<CodeableConcept> getModifier() { 6150 if (this.modifier == null) 6151 this.modifier = new ArrayList<CodeableConcept>(); 6152 return this.modifier; 6153 } 6154 6155 /** 6156 * @return Returns a reference to <code>this</code> for easy method chaining 6157 */ 6158 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 6159 this.modifier = theModifier; 6160 return this; 6161 } 6162 6163 public boolean hasModifier() { 6164 if (this.modifier == null) 6165 return false; 6166 for (CodeableConcept item : this.modifier) 6167 if (!item.isEmpty()) 6168 return true; 6169 return false; 6170 } 6171 6172 public CodeableConcept addModifier() { //3 6173 CodeableConcept t = new CodeableConcept(); 6174 if (this.modifier == null) 6175 this.modifier = new ArrayList<CodeableConcept>(); 6176 this.modifier.add(t); 6177 return t; 6178 } 6179 6180 public DetailComponent addModifier(CodeableConcept t) { //3 6181 if (t == null) 6182 return this; 6183 if (this.modifier == null) 6184 this.modifier = new ArrayList<CodeableConcept>(); 6185 this.modifier.add(t); 6186 return this; 6187 } 6188 6189 /** 6190 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 6191 */ 6192 public CodeableConcept getModifierFirstRep() { 6193 if (getModifier().isEmpty()) { 6194 addModifier(); 6195 } 6196 return getModifier().get(0); 6197 } 6198 6199 /** 6200 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 6201 */ 6202 public List<CodeableConcept> getProgramCode() { 6203 if (this.programCode == null) 6204 this.programCode = new ArrayList<CodeableConcept>(); 6205 return this.programCode; 6206 } 6207 6208 /** 6209 * @return Returns a reference to <code>this</code> for easy method chaining 6210 */ 6211 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6212 this.programCode = theProgramCode; 6213 return this; 6214 } 6215 6216 public boolean hasProgramCode() { 6217 if (this.programCode == null) 6218 return false; 6219 for (CodeableConcept item : this.programCode) 6220 if (!item.isEmpty()) 6221 return true; 6222 return false; 6223 } 6224 6225 public CodeableConcept addProgramCode() { //3 6226 CodeableConcept t = new CodeableConcept(); 6227 if (this.programCode == null) 6228 this.programCode = new ArrayList<CodeableConcept>(); 6229 this.programCode.add(t); 6230 return t; 6231 } 6232 6233 public DetailComponent addProgramCode(CodeableConcept t) { //3 6234 if (t == null) 6235 return this; 6236 if (this.programCode == null) 6237 this.programCode = new ArrayList<CodeableConcept>(); 6238 this.programCode.add(t); 6239 return this; 6240 } 6241 6242 /** 6243 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 6244 */ 6245 public CodeableConcept getProgramCodeFirstRep() { 6246 if (getProgramCode().isEmpty()) { 6247 addProgramCode(); 6248 } 6249 return getProgramCode().get(0); 6250 } 6251 6252 /** 6253 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 6254 */ 6255 public Money getPatientPaid() { 6256 if (this.patientPaid == null) 6257 if (Configuration.errorOnAutoCreate()) 6258 throw new Error("Attempt to auto-create DetailComponent.patientPaid"); 6259 else if (Configuration.doAutoCreate()) 6260 this.patientPaid = new Money(); // cc 6261 return this.patientPaid; 6262 } 6263 6264 public boolean hasPatientPaid() { 6265 return this.patientPaid != null && !this.patientPaid.isEmpty(); 6266 } 6267 6268 /** 6269 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 6270 */ 6271 public DetailComponent setPatientPaid(Money value) { 6272 this.patientPaid = value; 6273 return this; 6274 } 6275 6276 /** 6277 * @return {@link #quantity} (The number of repetitions of a service or product.) 6278 */ 6279 public Quantity getQuantity() { 6280 if (this.quantity == null) 6281 if (Configuration.errorOnAutoCreate()) 6282 throw new Error("Attempt to auto-create DetailComponent.quantity"); 6283 else if (Configuration.doAutoCreate()) 6284 this.quantity = new Quantity(); // cc 6285 return this.quantity; 6286 } 6287 6288 public boolean hasQuantity() { 6289 return this.quantity != null && !this.quantity.isEmpty(); 6290 } 6291 6292 /** 6293 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6294 */ 6295 public DetailComponent setQuantity(Quantity value) { 6296 this.quantity = value; 6297 return this; 6298 } 6299 6300 /** 6301 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6302 */ 6303 public Money getUnitPrice() { 6304 if (this.unitPrice == null) 6305 if (Configuration.errorOnAutoCreate()) 6306 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 6307 else if (Configuration.doAutoCreate()) 6308 this.unitPrice = new Money(); // cc 6309 return this.unitPrice; 6310 } 6311 6312 public boolean hasUnitPrice() { 6313 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6314 } 6315 6316 /** 6317 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6318 */ 6319 public DetailComponent setUnitPrice(Money value) { 6320 this.unitPrice = value; 6321 return this; 6322 } 6323 6324 /** 6325 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6326 */ 6327 public DecimalType getFactorElement() { 6328 if (this.factor == null) 6329 if (Configuration.errorOnAutoCreate()) 6330 throw new Error("Attempt to auto-create DetailComponent.factor"); 6331 else if (Configuration.doAutoCreate()) 6332 this.factor = new DecimalType(); // bb 6333 return this.factor; 6334 } 6335 6336 public boolean hasFactorElement() { 6337 return this.factor != null && !this.factor.isEmpty(); 6338 } 6339 6340 public boolean hasFactor() { 6341 return this.factor != null && !this.factor.isEmpty(); 6342 } 6343 6344 /** 6345 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6346 */ 6347 public DetailComponent setFactorElement(DecimalType value) { 6348 this.factor = value; 6349 return this; 6350 } 6351 6352 /** 6353 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6354 */ 6355 public BigDecimal getFactor() { 6356 return this.factor == null ? null : this.factor.getValue(); 6357 } 6358 6359 /** 6360 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6361 */ 6362 public DetailComponent setFactor(BigDecimal value) { 6363 if (value == null) 6364 this.factor = null; 6365 else { 6366 if (this.factor == null) 6367 this.factor = new DecimalType(); 6368 this.factor.setValue(value); 6369 } 6370 return this; 6371 } 6372 6373 /** 6374 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6375 */ 6376 public DetailComponent setFactor(long value) { 6377 this.factor = new DecimalType(); 6378 this.factor.setValue(value); 6379 return this; 6380 } 6381 6382 /** 6383 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6384 */ 6385 public DetailComponent setFactor(double value) { 6386 this.factor = new DecimalType(); 6387 this.factor.setValue(value); 6388 return this; 6389 } 6390 6391 /** 6392 * @return {@link #tax} (The total of taxes applicable for this product or service.) 6393 */ 6394 public Money getTax() { 6395 if (this.tax == null) 6396 if (Configuration.errorOnAutoCreate()) 6397 throw new Error("Attempt to auto-create DetailComponent.tax"); 6398 else if (Configuration.doAutoCreate()) 6399 this.tax = new Money(); // cc 6400 return this.tax; 6401 } 6402 6403 public boolean hasTax() { 6404 return this.tax != null && !this.tax.isEmpty(); 6405 } 6406 6407 /** 6408 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 6409 */ 6410 public DetailComponent setTax(Money value) { 6411 this.tax = value; 6412 return this; 6413 } 6414 6415 /** 6416 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 6417 */ 6418 public Money getNet() { 6419 if (this.net == null) 6420 if (Configuration.errorOnAutoCreate()) 6421 throw new Error("Attempt to auto-create DetailComponent.net"); 6422 else if (Configuration.doAutoCreate()) 6423 this.net = new Money(); // cc 6424 return this.net; 6425 } 6426 6427 public boolean hasNet() { 6428 return this.net != null && !this.net.isEmpty(); 6429 } 6430 6431 /** 6432 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 6433 */ 6434 public DetailComponent setNet(Money value) { 6435 this.net = value; 6436 return this; 6437 } 6438 6439 /** 6440 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 6441 */ 6442 public List<Reference> getUdi() { 6443 if (this.udi == null) 6444 this.udi = new ArrayList<Reference>(); 6445 return this.udi; 6446 } 6447 6448 /** 6449 * @return Returns a reference to <code>this</code> for easy method chaining 6450 */ 6451 public DetailComponent setUdi(List<Reference> theUdi) { 6452 this.udi = theUdi; 6453 return this; 6454 } 6455 6456 public boolean hasUdi() { 6457 if (this.udi == null) 6458 return false; 6459 for (Reference item : this.udi) 6460 if (!item.isEmpty()) 6461 return true; 6462 return false; 6463 } 6464 6465 public Reference addUdi() { //3 6466 Reference t = new Reference(); 6467 if (this.udi == null) 6468 this.udi = new ArrayList<Reference>(); 6469 this.udi.add(t); 6470 return t; 6471 } 6472 6473 public DetailComponent addUdi(Reference t) { //3 6474 if (t == null) 6475 return this; 6476 if (this.udi == null) 6477 this.udi = new ArrayList<Reference>(); 6478 this.udi.add(t); 6479 return this; 6480 } 6481 6482 /** 6483 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 6484 */ 6485 public Reference getUdiFirstRep() { 6486 if (getUdi().isEmpty()) { 6487 addUdi(); 6488 } 6489 return getUdi().get(0); 6490 } 6491 6492 /** 6493 * @return {@link #subDetail} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.) 6494 */ 6495 public List<SubDetailComponent> getSubDetail() { 6496 if (this.subDetail == null) 6497 this.subDetail = new ArrayList<SubDetailComponent>(); 6498 return this.subDetail; 6499 } 6500 6501 /** 6502 * @return Returns a reference to <code>this</code> for easy method chaining 6503 */ 6504 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 6505 this.subDetail = theSubDetail; 6506 return this; 6507 } 6508 6509 public boolean hasSubDetail() { 6510 if (this.subDetail == null) 6511 return false; 6512 for (SubDetailComponent item : this.subDetail) 6513 if (!item.isEmpty()) 6514 return true; 6515 return false; 6516 } 6517 6518 public SubDetailComponent addSubDetail() { //3 6519 SubDetailComponent t = new SubDetailComponent(); 6520 if (this.subDetail == null) 6521 this.subDetail = new ArrayList<SubDetailComponent>(); 6522 this.subDetail.add(t); 6523 return t; 6524 } 6525 6526 public DetailComponent addSubDetail(SubDetailComponent t) { //3 6527 if (t == null) 6528 return this; 6529 if (this.subDetail == null) 6530 this.subDetail = new ArrayList<SubDetailComponent>(); 6531 this.subDetail.add(t); 6532 return this; 6533 } 6534 6535 /** 6536 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 6537 */ 6538 public SubDetailComponent getSubDetailFirstRep() { 6539 if (getSubDetail().isEmpty()) { 6540 addSubDetail(); 6541 } 6542 return getSubDetail().get(0); 6543 } 6544 6545 protected void listChildren(List<Property> children) { 6546 super.listChildren(children); 6547 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 6548 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 6549 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 6550 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 6551 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 6552 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 6553 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6554 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 6555 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 6556 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6557 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 6558 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6559 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 6560 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net)); 6561 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 6562 children.add(new Property("subDetail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 6563 } 6564 6565 @Override 6566 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6567 switch (_hash) { 6568 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 6569 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 6570 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 6571 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 6572 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 6573 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 6574 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 6575 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 6576 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 6577 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6578 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 6579 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6580 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 6581 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net); 6582 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 6583 case -828829007: /*subDetail*/ return new Property("subDetail", "", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, subDetail); 6584 default: return super.getNamedProperty(_hash, _name, _checkValid); 6585 } 6586 6587 } 6588 6589 @Override 6590 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6591 switch (hash) { 6592 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 6593 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 6594 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6595 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6596 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 6597 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 6598 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6599 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 6600 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 6601 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 6602 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6603 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6604 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 6605 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6606 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 6607 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 6608 default: return super.getProperty(hash, name, checkValid); 6609 } 6610 6611 } 6612 6613 @Override 6614 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6615 switch (hash) { 6616 case 1349547969: // sequence 6617 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 6618 return value; 6619 case 82505966: // traceNumber 6620 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 6621 return value; 6622 case 1099842588: // revenue 6623 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6624 return value; 6625 case 50511102: // category 6626 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6627 return value; 6628 case 1957227299: // productOrService 6629 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6630 return value; 6631 case -717476168: // productOrServiceEnd 6632 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6633 return value; 6634 case -615513385: // modifier 6635 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6636 return value; 6637 case 1010065041: // programCode 6638 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6639 return value; 6640 case 525514609: // patientPaid 6641 this.patientPaid = TypeConvertor.castToMoney(value); // Money 6642 return value; 6643 case -1285004149: // quantity 6644 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6645 return value; 6646 case -486196699: // unitPrice 6647 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6648 return value; 6649 case -1282148017: // factor 6650 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6651 return value; 6652 case 114603: // tax 6653 this.tax = TypeConvertor.castToMoney(value); // Money 6654 return value; 6655 case 108957: // net 6656 this.net = TypeConvertor.castToMoney(value); // Money 6657 return value; 6658 case 115642: // udi 6659 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 6660 return value; 6661 case -828829007: // subDetail 6662 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 6663 return value; 6664 default: return super.setProperty(hash, name, value); 6665 } 6666 6667 } 6668 6669 @Override 6670 public Base setProperty(String name, Base value) throws FHIRException { 6671 if (name.equals("sequence")) { 6672 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 6673 } else if (name.equals("traceNumber")) { 6674 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 6675 } else if (name.equals("revenue")) { 6676 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6677 } else if (name.equals("category")) { 6678 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6679 } else if (name.equals("productOrService")) { 6680 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6681 } else if (name.equals("productOrServiceEnd")) { 6682 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6683 } else if (name.equals("modifier")) { 6684 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 6685 } else if (name.equals("programCode")) { 6686 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 6687 } else if (name.equals("patientPaid")) { 6688 this.patientPaid = TypeConvertor.castToMoney(value); // Money 6689 } else if (name.equals("quantity")) { 6690 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6691 } else if (name.equals("unitPrice")) { 6692 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6693 } else if (name.equals("factor")) { 6694 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6695 } else if (name.equals("tax")) { 6696 this.tax = TypeConvertor.castToMoney(value); // Money 6697 } else if (name.equals("net")) { 6698 this.net = TypeConvertor.castToMoney(value); // Money 6699 } else if (name.equals("udi")) { 6700 this.getUdi().add(TypeConvertor.castToReference(value)); 6701 } else if (name.equals("subDetail")) { 6702 this.getSubDetail().add((SubDetailComponent) value); 6703 } else 6704 return super.setProperty(name, value); 6705 return value; 6706 } 6707 6708 @Override 6709 public void removeChild(String name, Base value) throws FHIRException { 6710 if (name.equals("sequence")) { 6711 this.sequence = null; 6712 } else if (name.equals("traceNumber")) { 6713 this.getTraceNumber().remove(value); 6714 } else if (name.equals("revenue")) { 6715 this.revenue = null; 6716 } else if (name.equals("category")) { 6717 this.category = null; 6718 } else if (name.equals("productOrService")) { 6719 this.productOrService = null; 6720 } else if (name.equals("productOrServiceEnd")) { 6721 this.productOrServiceEnd = null; 6722 } else if (name.equals("modifier")) { 6723 this.getModifier().remove(value); 6724 } else if (name.equals("programCode")) { 6725 this.getProgramCode().remove(value); 6726 } else if (name.equals("patientPaid")) { 6727 this.patientPaid = null; 6728 } else if (name.equals("quantity")) { 6729 this.quantity = null; 6730 } else if (name.equals("unitPrice")) { 6731 this.unitPrice = null; 6732 } else if (name.equals("factor")) { 6733 this.factor = null; 6734 } else if (name.equals("tax")) { 6735 this.tax = null; 6736 } else if (name.equals("net")) { 6737 this.net = null; 6738 } else if (name.equals("udi")) { 6739 this.getUdi().remove(value); 6740 } else if (name.equals("subDetail")) { 6741 this.getSubDetail().remove((SubDetailComponent) value); 6742 } else 6743 super.removeChild(name, value); 6744 6745 } 6746 6747 @Override 6748 public Base makeProperty(int hash, String name) throws FHIRException { 6749 switch (hash) { 6750 case 1349547969: return getSequenceElement(); 6751 case 82505966: return addTraceNumber(); 6752 case 1099842588: return getRevenue(); 6753 case 50511102: return getCategory(); 6754 case 1957227299: return getProductOrService(); 6755 case -717476168: return getProductOrServiceEnd(); 6756 case -615513385: return addModifier(); 6757 case 1010065041: return addProgramCode(); 6758 case 525514609: return getPatientPaid(); 6759 case -1285004149: return getQuantity(); 6760 case -486196699: return getUnitPrice(); 6761 case -1282148017: return getFactorElement(); 6762 case 114603: return getTax(); 6763 case 108957: return getNet(); 6764 case 115642: return addUdi(); 6765 case -828829007: return addSubDetail(); 6766 default: return super.makeProperty(hash, name); 6767 } 6768 6769 } 6770 6771 @Override 6772 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6773 switch (hash) { 6774 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 6775 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 6776 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6777 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6778 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 6779 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 6780 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6781 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 6782 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 6783 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 6784 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6785 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6786 case 114603: /*tax*/ return new String[] {"Money"}; 6787 case 108957: /*net*/ return new String[] {"Money"}; 6788 case 115642: /*udi*/ return new String[] {"Reference"}; 6789 case -828829007: /*subDetail*/ return new String[] {}; 6790 default: return super.getTypesForProperty(hash, name); 6791 } 6792 6793 } 6794 6795 @Override 6796 public Base addChild(String name) throws FHIRException { 6797 if (name.equals("sequence")) { 6798 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.sequence"); 6799 } 6800 else if (name.equals("traceNumber")) { 6801 return addTraceNumber(); 6802 } 6803 else if (name.equals("revenue")) { 6804 this.revenue = new CodeableConcept(); 6805 return this.revenue; 6806 } 6807 else if (name.equals("category")) { 6808 this.category = new CodeableConcept(); 6809 return this.category; 6810 } 6811 else if (name.equals("productOrService")) { 6812 this.productOrService = new CodeableConcept(); 6813 return this.productOrService; 6814 } 6815 else if (name.equals("productOrServiceEnd")) { 6816 this.productOrServiceEnd = new CodeableConcept(); 6817 return this.productOrServiceEnd; 6818 } 6819 else if (name.equals("modifier")) { 6820 return addModifier(); 6821 } 6822 else if (name.equals("programCode")) { 6823 return addProgramCode(); 6824 } 6825 else if (name.equals("patientPaid")) { 6826 this.patientPaid = new Money(); 6827 return this.patientPaid; 6828 } 6829 else if (name.equals("quantity")) { 6830 this.quantity = new Quantity(); 6831 return this.quantity; 6832 } 6833 else if (name.equals("unitPrice")) { 6834 this.unitPrice = new Money(); 6835 return this.unitPrice; 6836 } 6837 else if (name.equals("factor")) { 6838 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.factor"); 6839 } 6840 else if (name.equals("tax")) { 6841 this.tax = new Money(); 6842 return this.tax; 6843 } 6844 else if (name.equals("net")) { 6845 this.net = new Money(); 6846 return this.net; 6847 } 6848 else if (name.equals("udi")) { 6849 return addUdi(); 6850 } 6851 else if (name.equals("subDetail")) { 6852 return addSubDetail(); 6853 } 6854 else 6855 return super.addChild(name); 6856 } 6857 6858 public DetailComponent copy() { 6859 DetailComponent dst = new DetailComponent(); 6860 copyValues(dst); 6861 return dst; 6862 } 6863 6864 public void copyValues(DetailComponent dst) { 6865 super.copyValues(dst); 6866 dst.sequence = sequence == null ? null : sequence.copy(); 6867 if (traceNumber != null) { 6868 dst.traceNumber = new ArrayList<Identifier>(); 6869 for (Identifier i : traceNumber) 6870 dst.traceNumber.add(i.copy()); 6871 }; 6872 dst.revenue = revenue == null ? null : revenue.copy(); 6873 dst.category = category == null ? null : category.copy(); 6874 dst.productOrService = productOrService == null ? null : productOrService.copy(); 6875 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 6876 if (modifier != null) { 6877 dst.modifier = new ArrayList<CodeableConcept>(); 6878 for (CodeableConcept i : modifier) 6879 dst.modifier.add(i.copy()); 6880 }; 6881 if (programCode != null) { 6882 dst.programCode = new ArrayList<CodeableConcept>(); 6883 for (CodeableConcept i : programCode) 6884 dst.programCode.add(i.copy()); 6885 }; 6886 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 6887 dst.quantity = quantity == null ? null : quantity.copy(); 6888 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6889 dst.factor = factor == null ? null : factor.copy(); 6890 dst.tax = tax == null ? null : tax.copy(); 6891 dst.net = net == null ? null : net.copy(); 6892 if (udi != null) { 6893 dst.udi = new ArrayList<Reference>(); 6894 for (Reference i : udi) 6895 dst.udi.add(i.copy()); 6896 }; 6897 if (subDetail != null) { 6898 dst.subDetail = new ArrayList<SubDetailComponent>(); 6899 for (SubDetailComponent i : subDetail) 6900 dst.subDetail.add(i.copy()); 6901 }; 6902 } 6903 6904 @Override 6905 public boolean equalsDeep(Base other_) { 6906 if (!super.equalsDeep(other_)) 6907 return false; 6908 if (!(other_ instanceof DetailComponent)) 6909 return false; 6910 DetailComponent o = (DetailComponent) other_; 6911 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 6912 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 6913 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 6914 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 6915 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 6916 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6917 && compareDeep(subDetail, o.subDetail, true); 6918 } 6919 6920 @Override 6921 public boolean equalsShallow(Base other_) { 6922 if (!super.equalsShallow(other_)) 6923 return false; 6924 if (!(other_ instanceof DetailComponent)) 6925 return false; 6926 DetailComponent o = (DetailComponent) other_; 6927 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 6928 } 6929 6930 public boolean isEmpty() { 6931 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 6932 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 6933 , quantity, unitPrice, factor, tax, net, udi, subDetail); 6934 } 6935 6936 public String fhirType() { 6937 return "Claim.item.detail"; 6938 6939 } 6940 6941 } 6942 6943 @Block() 6944 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 6945 /** 6946 * A number to uniquely identify item entries. 6947 */ 6948 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6949 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 6950 protected PositiveIntType sequence; 6951 6952 /** 6953 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 6954 */ 6955 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6956 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 6957 protected List<Identifier> traceNumber; 6958 6959 /** 6960 * The type of revenue or cost center providing the product and/or service. 6961 */ 6962 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6963 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 6964 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 6965 protected CodeableConcept revenue; 6966 6967 /** 6968 * Code to identify the general type of benefits under which products and services are provided. 6969 */ 6970 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 6971 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 6972 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6973 protected CodeableConcept category; 6974 6975 /** 6976 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 6977 */ 6978 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 6979 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 6980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6981 protected CodeableConcept productOrService; 6982 6983 /** 6984 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 6985 */ 6986 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 6987 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 6988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6989 protected CodeableConcept productOrServiceEnd; 6990 6991 /** 6992 * Item typification or modifiers codes to convey additional context for the product or service. 6993 */ 6994 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6995 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 6996 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 6997 protected List<CodeableConcept> modifier; 6998 6999 /** 7000 * Identifies the program under which this may be recovered. 7001 */ 7002 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7003 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 7004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 7005 protected List<CodeableConcept> programCode; 7006 7007 /** 7008 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 7009 */ 7010 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 7011 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 7012 protected Money patientPaid; 7013 7014 /** 7015 * The number of repetitions of a service or product. 7016 */ 7017 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 7018 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 7019 protected Quantity quantity; 7020 7021 /** 7022 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 7023 */ 7024 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 7025 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 7026 protected Money unitPrice; 7027 7028 /** 7029 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7030 */ 7031 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 7032 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 7033 protected DecimalType factor; 7034 7035 /** 7036 * The total of taxes applicable for this product or service. 7037 */ 7038 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 7039 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 7040 protected Money tax; 7041 7042 /** 7043 * The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor. 7044 */ 7045 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 7046 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor." ) 7047 protected Money net; 7048 7049 /** 7050 * Unique Device Identifiers associated with this line item. 7051 */ 7052 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7053 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 7054 protected List<Reference> udi; 7055 7056 private static final long serialVersionUID = -2049468862L; 7057 7058 /** 7059 * Constructor 7060 */ 7061 public SubDetailComponent() { 7062 super(); 7063 } 7064 7065 /** 7066 * Constructor 7067 */ 7068 public SubDetailComponent(int sequence) { 7069 super(); 7070 this.setSequence(sequence); 7071 } 7072 7073 /** 7074 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 7075 */ 7076 public PositiveIntType getSequenceElement() { 7077 if (this.sequence == null) 7078 if (Configuration.errorOnAutoCreate()) 7079 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 7080 else if (Configuration.doAutoCreate()) 7081 this.sequence = new PositiveIntType(); // bb 7082 return this.sequence; 7083 } 7084 7085 public boolean hasSequenceElement() { 7086 return this.sequence != null && !this.sequence.isEmpty(); 7087 } 7088 7089 public boolean hasSequence() { 7090 return this.sequence != null && !this.sequence.isEmpty(); 7091 } 7092 7093 /** 7094 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 7095 */ 7096 public SubDetailComponent setSequenceElement(PositiveIntType value) { 7097 this.sequence = value; 7098 return this; 7099 } 7100 7101 /** 7102 * @return A number to uniquely identify item entries. 7103 */ 7104 public int getSequence() { 7105 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7106 } 7107 7108 /** 7109 * @param value A number to uniquely identify item entries. 7110 */ 7111 public SubDetailComponent setSequence(int value) { 7112 if (this.sequence == null) 7113 this.sequence = new PositiveIntType(); 7114 this.sequence.setValue(value); 7115 return this; 7116 } 7117 7118 /** 7119 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 7120 */ 7121 public List<Identifier> getTraceNumber() { 7122 if (this.traceNumber == null) 7123 this.traceNumber = new ArrayList<Identifier>(); 7124 return this.traceNumber; 7125 } 7126 7127 /** 7128 * @return Returns a reference to <code>this</code> for easy method chaining 7129 */ 7130 public SubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 7131 this.traceNumber = theTraceNumber; 7132 return this; 7133 } 7134 7135 public boolean hasTraceNumber() { 7136 if (this.traceNumber == null) 7137 return false; 7138 for (Identifier item : this.traceNumber) 7139 if (!item.isEmpty()) 7140 return true; 7141 return false; 7142 } 7143 7144 public Identifier addTraceNumber() { //3 7145 Identifier t = new Identifier(); 7146 if (this.traceNumber == null) 7147 this.traceNumber = new ArrayList<Identifier>(); 7148 this.traceNumber.add(t); 7149 return t; 7150 } 7151 7152 public SubDetailComponent addTraceNumber(Identifier t) { //3 7153 if (t == null) 7154 return this; 7155 if (this.traceNumber == null) 7156 this.traceNumber = new ArrayList<Identifier>(); 7157 this.traceNumber.add(t); 7158 return this; 7159 } 7160 7161 /** 7162 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 7163 */ 7164 public Identifier getTraceNumberFirstRep() { 7165 if (getTraceNumber().isEmpty()) { 7166 addTraceNumber(); 7167 } 7168 return getTraceNumber().get(0); 7169 } 7170 7171 /** 7172 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 7173 */ 7174 public CodeableConcept getRevenue() { 7175 if (this.revenue == null) 7176 if (Configuration.errorOnAutoCreate()) 7177 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 7178 else if (Configuration.doAutoCreate()) 7179 this.revenue = new CodeableConcept(); // cc 7180 return this.revenue; 7181 } 7182 7183 public boolean hasRevenue() { 7184 return this.revenue != null && !this.revenue.isEmpty(); 7185 } 7186 7187 /** 7188 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 7189 */ 7190 public SubDetailComponent setRevenue(CodeableConcept value) { 7191 this.revenue = value; 7192 return this; 7193 } 7194 7195 /** 7196 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 7197 */ 7198 public CodeableConcept getCategory() { 7199 if (this.category == null) 7200 if (Configuration.errorOnAutoCreate()) 7201 throw new Error("Attempt to auto-create SubDetailComponent.category"); 7202 else if (Configuration.doAutoCreate()) 7203 this.category = new CodeableConcept(); // cc 7204 return this.category; 7205 } 7206 7207 public boolean hasCategory() { 7208 return this.category != null && !this.category.isEmpty(); 7209 } 7210 7211 /** 7212 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 7213 */ 7214 public SubDetailComponent setCategory(CodeableConcept value) { 7215 this.category = value; 7216 return this; 7217 } 7218 7219 /** 7220 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 7221 */ 7222 public CodeableConcept getProductOrService() { 7223 if (this.productOrService == null) 7224 if (Configuration.errorOnAutoCreate()) 7225 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 7226 else if (Configuration.doAutoCreate()) 7227 this.productOrService = new CodeableConcept(); // cc 7228 return this.productOrService; 7229 } 7230 7231 public boolean hasProductOrService() { 7232 return this.productOrService != null && !this.productOrService.isEmpty(); 7233 } 7234 7235 /** 7236 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 7237 */ 7238 public SubDetailComponent setProductOrService(CodeableConcept value) { 7239 this.productOrService = value; 7240 return this; 7241 } 7242 7243 /** 7244 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 7245 */ 7246 public CodeableConcept getProductOrServiceEnd() { 7247 if (this.productOrServiceEnd == null) 7248 if (Configuration.errorOnAutoCreate()) 7249 throw new Error("Attempt to auto-create SubDetailComponent.productOrServiceEnd"); 7250 else if (Configuration.doAutoCreate()) 7251 this.productOrServiceEnd = new CodeableConcept(); // cc 7252 return this.productOrServiceEnd; 7253 } 7254 7255 public boolean hasProductOrServiceEnd() { 7256 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 7257 } 7258 7259 /** 7260 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 7261 */ 7262 public SubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 7263 this.productOrServiceEnd = value; 7264 return this; 7265 } 7266 7267 /** 7268 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 7269 */ 7270 public List<CodeableConcept> getModifier() { 7271 if (this.modifier == null) 7272 this.modifier = new ArrayList<CodeableConcept>(); 7273 return this.modifier; 7274 } 7275 7276 /** 7277 * @return Returns a reference to <code>this</code> for easy method chaining 7278 */ 7279 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 7280 this.modifier = theModifier; 7281 return this; 7282 } 7283 7284 public boolean hasModifier() { 7285 if (this.modifier == null) 7286 return false; 7287 for (CodeableConcept item : this.modifier) 7288 if (!item.isEmpty()) 7289 return true; 7290 return false; 7291 } 7292 7293 public CodeableConcept addModifier() { //3 7294 CodeableConcept t = new CodeableConcept(); 7295 if (this.modifier == null) 7296 this.modifier = new ArrayList<CodeableConcept>(); 7297 this.modifier.add(t); 7298 return t; 7299 } 7300 7301 public SubDetailComponent addModifier(CodeableConcept t) { //3 7302 if (t == null) 7303 return this; 7304 if (this.modifier == null) 7305 this.modifier = new ArrayList<CodeableConcept>(); 7306 this.modifier.add(t); 7307 return this; 7308 } 7309 7310 /** 7311 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 7312 */ 7313 public CodeableConcept getModifierFirstRep() { 7314 if (getModifier().isEmpty()) { 7315 addModifier(); 7316 } 7317 return getModifier().get(0); 7318 } 7319 7320 /** 7321 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 7322 */ 7323 public List<CodeableConcept> getProgramCode() { 7324 if (this.programCode == null) 7325 this.programCode = new ArrayList<CodeableConcept>(); 7326 return this.programCode; 7327 } 7328 7329 /** 7330 * @return Returns a reference to <code>this</code> for easy method chaining 7331 */ 7332 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 7333 this.programCode = theProgramCode; 7334 return this; 7335 } 7336 7337 public boolean hasProgramCode() { 7338 if (this.programCode == null) 7339 return false; 7340 for (CodeableConcept item : this.programCode) 7341 if (!item.isEmpty()) 7342 return true; 7343 return false; 7344 } 7345 7346 public CodeableConcept addProgramCode() { //3 7347 CodeableConcept t = new CodeableConcept(); 7348 if (this.programCode == null) 7349 this.programCode = new ArrayList<CodeableConcept>(); 7350 this.programCode.add(t); 7351 return t; 7352 } 7353 7354 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 7355 if (t == null) 7356 return this; 7357 if (this.programCode == null) 7358 this.programCode = new ArrayList<CodeableConcept>(); 7359 this.programCode.add(t); 7360 return this; 7361 } 7362 7363 /** 7364 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 7365 */ 7366 public CodeableConcept getProgramCodeFirstRep() { 7367 if (getProgramCode().isEmpty()) { 7368 addProgramCode(); 7369 } 7370 return getProgramCode().get(0); 7371 } 7372 7373 /** 7374 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 7375 */ 7376 public Money getPatientPaid() { 7377 if (this.patientPaid == null) 7378 if (Configuration.errorOnAutoCreate()) 7379 throw new Error("Attempt to auto-create SubDetailComponent.patientPaid"); 7380 else if (Configuration.doAutoCreate()) 7381 this.patientPaid = new Money(); // cc 7382 return this.patientPaid; 7383 } 7384 7385 public boolean hasPatientPaid() { 7386 return this.patientPaid != null && !this.patientPaid.isEmpty(); 7387 } 7388 7389 /** 7390 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 7391 */ 7392 public SubDetailComponent setPatientPaid(Money value) { 7393 this.patientPaid = value; 7394 return this; 7395 } 7396 7397 /** 7398 * @return {@link #quantity} (The number of repetitions of a service or product.) 7399 */ 7400 public Quantity getQuantity() { 7401 if (this.quantity == null) 7402 if (Configuration.errorOnAutoCreate()) 7403 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 7404 else if (Configuration.doAutoCreate()) 7405 this.quantity = new Quantity(); // cc 7406 return this.quantity; 7407 } 7408 7409 public boolean hasQuantity() { 7410 return this.quantity != null && !this.quantity.isEmpty(); 7411 } 7412 7413 /** 7414 * @param value {@link #quantity} (The number of repetitions of a service or product.) 7415 */ 7416 public SubDetailComponent setQuantity(Quantity value) { 7417 this.quantity = value; 7418 return this; 7419 } 7420 7421 /** 7422 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 7423 */ 7424 public Money getUnitPrice() { 7425 if (this.unitPrice == null) 7426 if (Configuration.errorOnAutoCreate()) 7427 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 7428 else if (Configuration.doAutoCreate()) 7429 this.unitPrice = new Money(); // cc 7430 return this.unitPrice; 7431 } 7432 7433 public boolean hasUnitPrice() { 7434 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7435 } 7436 7437 /** 7438 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 7439 */ 7440 public SubDetailComponent setUnitPrice(Money value) { 7441 this.unitPrice = value; 7442 return this; 7443 } 7444 7445 /** 7446 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 7447 */ 7448 public DecimalType getFactorElement() { 7449 if (this.factor == null) 7450 if (Configuration.errorOnAutoCreate()) 7451 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 7452 else if (Configuration.doAutoCreate()) 7453 this.factor = new DecimalType(); // bb 7454 return this.factor; 7455 } 7456 7457 public boolean hasFactorElement() { 7458 return this.factor != null && !this.factor.isEmpty(); 7459 } 7460 7461 public boolean hasFactor() { 7462 return this.factor != null && !this.factor.isEmpty(); 7463 } 7464 7465 /** 7466 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 7467 */ 7468 public SubDetailComponent setFactorElement(DecimalType value) { 7469 this.factor = value; 7470 return this; 7471 } 7472 7473 /** 7474 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7475 */ 7476 public BigDecimal getFactor() { 7477 return this.factor == null ? null : this.factor.getValue(); 7478 } 7479 7480 /** 7481 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7482 */ 7483 public SubDetailComponent setFactor(BigDecimal value) { 7484 if (value == null) 7485 this.factor = null; 7486 else { 7487 if (this.factor == null) 7488 this.factor = new DecimalType(); 7489 this.factor.setValue(value); 7490 } 7491 return this; 7492 } 7493 7494 /** 7495 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7496 */ 7497 public SubDetailComponent setFactor(long value) { 7498 this.factor = new DecimalType(); 7499 this.factor.setValue(value); 7500 return this; 7501 } 7502 7503 /** 7504 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7505 */ 7506 public SubDetailComponent setFactor(double value) { 7507 this.factor = new DecimalType(); 7508 this.factor.setValue(value); 7509 return this; 7510 } 7511 7512 /** 7513 * @return {@link #tax} (The total of taxes applicable for this product or service.) 7514 */ 7515 public Money getTax() { 7516 if (this.tax == null) 7517 if (Configuration.errorOnAutoCreate()) 7518 throw new Error("Attempt to auto-create SubDetailComponent.tax"); 7519 else if (Configuration.doAutoCreate()) 7520 this.tax = new Money(); // cc 7521 return this.tax; 7522 } 7523 7524 public boolean hasTax() { 7525 return this.tax != null && !this.tax.isEmpty(); 7526 } 7527 7528 /** 7529 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 7530 */ 7531 public SubDetailComponent setTax(Money value) { 7532 this.tax = value; 7533 return this; 7534 } 7535 7536 /** 7537 * @return {@link #net} (The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.) 7538 */ 7539 public Money getNet() { 7540 if (this.net == null) 7541 if (Configuration.errorOnAutoCreate()) 7542 throw new Error("Attempt to auto-create SubDetailComponent.net"); 7543 else if (Configuration.doAutoCreate()) 7544 this.net = new Money(); // cc 7545 return this.net; 7546 } 7547 7548 public boolean hasNet() { 7549 return this.net != null && !this.net.isEmpty(); 7550 } 7551 7552 /** 7553 * @param value {@link #net} (The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.) 7554 */ 7555 public SubDetailComponent setNet(Money value) { 7556 this.net = value; 7557 return this; 7558 } 7559 7560 /** 7561 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 7562 */ 7563 public List<Reference> getUdi() { 7564 if (this.udi == null) 7565 this.udi = new ArrayList<Reference>(); 7566 return this.udi; 7567 } 7568 7569 /** 7570 * @return Returns a reference to <code>this</code> for easy method chaining 7571 */ 7572 public SubDetailComponent setUdi(List<Reference> theUdi) { 7573 this.udi = theUdi; 7574 return this; 7575 } 7576 7577 public boolean hasUdi() { 7578 if (this.udi == null) 7579 return false; 7580 for (Reference item : this.udi) 7581 if (!item.isEmpty()) 7582 return true; 7583 return false; 7584 } 7585 7586 public Reference addUdi() { //3 7587 Reference t = new Reference(); 7588 if (this.udi == null) 7589 this.udi = new ArrayList<Reference>(); 7590 this.udi.add(t); 7591 return t; 7592 } 7593 7594 public SubDetailComponent addUdi(Reference t) { //3 7595 if (t == null) 7596 return this; 7597 if (this.udi == null) 7598 this.udi = new ArrayList<Reference>(); 7599 this.udi.add(t); 7600 return this; 7601 } 7602 7603 /** 7604 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 7605 */ 7606 public Reference getUdiFirstRep() { 7607 if (getUdi().isEmpty()) { 7608 addUdi(); 7609 } 7610 return getUdi().get(0); 7611 } 7612 7613 protected void listChildren(List<Property> children) { 7614 super.listChildren(children); 7615 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 7616 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 7617 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7618 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 7619 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 7620 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 7621 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 7622 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7623 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 7624 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 7625 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 7626 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 7627 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 7628 children.add(new Property("net", "Money", "The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 7629 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 7630 } 7631 7632 @Override 7633 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7634 switch (_hash) { 7635 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 7636 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 7637 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7638 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 7639 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 7640 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 7641 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 7642 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7643 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 7644 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 7645 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 7646 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 7647 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 7648 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 7649 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7650 default: return super.getNamedProperty(_hash, _name, _checkValid); 7651 } 7652 7653 } 7654 7655 @Override 7656 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7657 switch (hash) { 7658 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 7659 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 7660 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 7661 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 7662 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 7663 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 7664 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7665 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7666 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 7667 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 7668 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 7669 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 7670 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 7671 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 7672 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7673 default: return super.getProperty(hash, name, checkValid); 7674 } 7675 7676 } 7677 7678 @Override 7679 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7680 switch (hash) { 7681 case 1349547969: // sequence 7682 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7683 return value; 7684 case 82505966: // traceNumber 7685 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 7686 return value; 7687 case 1099842588: // revenue 7688 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7689 return value; 7690 case 50511102: // category 7691 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7692 return value; 7693 case 1957227299: // productOrService 7694 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7695 return value; 7696 case -717476168: // productOrServiceEnd 7697 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7698 return value; 7699 case -615513385: // modifier 7700 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7701 return value; 7702 case 1010065041: // programCode 7703 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7704 return value; 7705 case 525514609: // patientPaid 7706 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7707 return value; 7708 case -1285004149: // quantity 7709 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7710 return value; 7711 case -486196699: // unitPrice 7712 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7713 return value; 7714 case -1282148017: // factor 7715 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7716 return value; 7717 case 114603: // tax 7718 this.tax = TypeConvertor.castToMoney(value); // Money 7719 return value; 7720 case 108957: // net 7721 this.net = TypeConvertor.castToMoney(value); // Money 7722 return value; 7723 case 115642: // udi 7724 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 7725 return value; 7726 default: return super.setProperty(hash, name, value); 7727 } 7728 7729 } 7730 7731 @Override 7732 public Base setProperty(String name, Base value) throws FHIRException { 7733 if (name.equals("sequence")) { 7734 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7735 } else if (name.equals("traceNumber")) { 7736 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 7737 } else if (name.equals("revenue")) { 7738 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7739 } else if (name.equals("category")) { 7740 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7741 } else if (name.equals("productOrService")) { 7742 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7743 } else if (name.equals("productOrServiceEnd")) { 7744 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7745 } else if (name.equals("modifier")) { 7746 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 7747 } else if (name.equals("programCode")) { 7748 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 7749 } else if (name.equals("patientPaid")) { 7750 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7751 } else if (name.equals("quantity")) { 7752 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7753 } else if (name.equals("unitPrice")) { 7754 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7755 } else if (name.equals("factor")) { 7756 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7757 } else if (name.equals("tax")) { 7758 this.tax = TypeConvertor.castToMoney(value); // Money 7759 } else if (name.equals("net")) { 7760 this.net = TypeConvertor.castToMoney(value); // Money 7761 } else if (name.equals("udi")) { 7762 this.getUdi().add(TypeConvertor.castToReference(value)); 7763 } else 7764 return super.setProperty(name, value); 7765 return value; 7766 } 7767 7768 @Override 7769 public void removeChild(String name, Base value) throws FHIRException { 7770 if (name.equals("sequence")) { 7771 this.sequence = null; 7772 } else if (name.equals("traceNumber")) { 7773 this.getTraceNumber().remove(value); 7774 } else if (name.equals("revenue")) { 7775 this.revenue = null; 7776 } else if (name.equals("category")) { 7777 this.category = null; 7778 } else if (name.equals("productOrService")) { 7779 this.productOrService = null; 7780 } else if (name.equals("productOrServiceEnd")) { 7781 this.productOrServiceEnd = null; 7782 } else if (name.equals("modifier")) { 7783 this.getModifier().remove(value); 7784 } else if (name.equals("programCode")) { 7785 this.getProgramCode().remove(value); 7786 } else if (name.equals("patientPaid")) { 7787 this.patientPaid = null; 7788 } else if (name.equals("quantity")) { 7789 this.quantity = null; 7790 } else if (name.equals("unitPrice")) { 7791 this.unitPrice = null; 7792 } else if (name.equals("factor")) { 7793 this.factor = null; 7794 } else if (name.equals("tax")) { 7795 this.tax = null; 7796 } else if (name.equals("net")) { 7797 this.net = null; 7798 } else if (name.equals("udi")) { 7799 this.getUdi().remove(value); 7800 } else 7801 super.removeChild(name, value); 7802 7803 } 7804 7805 @Override 7806 public Base makeProperty(int hash, String name) throws FHIRException { 7807 switch (hash) { 7808 case 1349547969: return getSequenceElement(); 7809 case 82505966: return addTraceNumber(); 7810 case 1099842588: return getRevenue(); 7811 case 50511102: return getCategory(); 7812 case 1957227299: return getProductOrService(); 7813 case -717476168: return getProductOrServiceEnd(); 7814 case -615513385: return addModifier(); 7815 case 1010065041: return addProgramCode(); 7816 case 525514609: return getPatientPaid(); 7817 case -1285004149: return getQuantity(); 7818 case -486196699: return getUnitPrice(); 7819 case -1282148017: return getFactorElement(); 7820 case 114603: return getTax(); 7821 case 108957: return getNet(); 7822 case 115642: return addUdi(); 7823 default: return super.makeProperty(hash, name); 7824 } 7825 7826 } 7827 7828 @Override 7829 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7830 switch (hash) { 7831 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 7832 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 7833 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7834 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7835 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 7836 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 7837 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7838 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 7839 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 7840 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 7841 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 7842 case -1282148017: /*factor*/ return new String[] {"decimal"}; 7843 case 114603: /*tax*/ return new String[] {"Money"}; 7844 case 108957: /*net*/ return new String[] {"Money"}; 7845 case 115642: /*udi*/ return new String[] {"Reference"}; 7846 default: return super.getTypesForProperty(hash, name); 7847 } 7848 7849 } 7850 7851 @Override 7852 public Base addChild(String name) throws FHIRException { 7853 if (name.equals("sequence")) { 7854 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.subDetail.sequence"); 7855 } 7856 else if (name.equals("traceNumber")) { 7857 return addTraceNumber(); 7858 } 7859 else if (name.equals("revenue")) { 7860 this.revenue = new CodeableConcept(); 7861 return this.revenue; 7862 } 7863 else if (name.equals("category")) { 7864 this.category = new CodeableConcept(); 7865 return this.category; 7866 } 7867 else if (name.equals("productOrService")) { 7868 this.productOrService = new CodeableConcept(); 7869 return this.productOrService; 7870 } 7871 else if (name.equals("productOrServiceEnd")) { 7872 this.productOrServiceEnd = new CodeableConcept(); 7873 return this.productOrServiceEnd; 7874 } 7875 else if (name.equals("modifier")) { 7876 return addModifier(); 7877 } 7878 else if (name.equals("programCode")) { 7879 return addProgramCode(); 7880 } 7881 else if (name.equals("patientPaid")) { 7882 this.patientPaid = new Money(); 7883 return this.patientPaid; 7884 } 7885 else if (name.equals("quantity")) { 7886 this.quantity = new Quantity(); 7887 return this.quantity; 7888 } 7889 else if (name.equals("unitPrice")) { 7890 this.unitPrice = new Money(); 7891 return this.unitPrice; 7892 } 7893 else if (name.equals("factor")) { 7894 throw new FHIRException("Cannot call addChild on a singleton property Claim.item.detail.subDetail.factor"); 7895 } 7896 else if (name.equals("tax")) { 7897 this.tax = new Money(); 7898 return this.tax; 7899 } 7900 else if (name.equals("net")) { 7901 this.net = new Money(); 7902 return this.net; 7903 } 7904 else if (name.equals("udi")) { 7905 return addUdi(); 7906 } 7907 else 7908 return super.addChild(name); 7909 } 7910 7911 public SubDetailComponent copy() { 7912 SubDetailComponent dst = new SubDetailComponent(); 7913 copyValues(dst); 7914 return dst; 7915 } 7916 7917 public void copyValues(SubDetailComponent dst) { 7918 super.copyValues(dst); 7919 dst.sequence = sequence == null ? null : sequence.copy(); 7920 if (traceNumber != null) { 7921 dst.traceNumber = new ArrayList<Identifier>(); 7922 for (Identifier i : traceNumber) 7923 dst.traceNumber.add(i.copy()); 7924 }; 7925 dst.revenue = revenue == null ? null : revenue.copy(); 7926 dst.category = category == null ? null : category.copy(); 7927 dst.productOrService = productOrService == null ? null : productOrService.copy(); 7928 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 7929 if (modifier != null) { 7930 dst.modifier = new ArrayList<CodeableConcept>(); 7931 for (CodeableConcept i : modifier) 7932 dst.modifier.add(i.copy()); 7933 }; 7934 if (programCode != null) { 7935 dst.programCode = new ArrayList<CodeableConcept>(); 7936 for (CodeableConcept i : programCode) 7937 dst.programCode.add(i.copy()); 7938 }; 7939 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 7940 dst.quantity = quantity == null ? null : quantity.copy(); 7941 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7942 dst.factor = factor == null ? null : factor.copy(); 7943 dst.tax = tax == null ? null : tax.copy(); 7944 dst.net = net == null ? null : net.copy(); 7945 if (udi != null) { 7946 dst.udi = new ArrayList<Reference>(); 7947 for (Reference i : udi) 7948 dst.udi.add(i.copy()); 7949 }; 7950 } 7951 7952 @Override 7953 public boolean equalsDeep(Base other_) { 7954 if (!super.equalsDeep(other_)) 7955 return false; 7956 if (!(other_ instanceof SubDetailComponent)) 7957 return false; 7958 SubDetailComponent o = (SubDetailComponent) other_; 7959 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 7960 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 7961 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 7962 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 7963 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 7964 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7965 ; 7966 } 7967 7968 @Override 7969 public boolean equalsShallow(Base other_) { 7970 if (!super.equalsShallow(other_)) 7971 return false; 7972 if (!(other_ instanceof SubDetailComponent)) 7973 return false; 7974 SubDetailComponent o = (SubDetailComponent) other_; 7975 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true); 7976 } 7977 7978 public boolean isEmpty() { 7979 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 7980 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 7981 , quantity, unitPrice, factor, tax, net, udi); 7982 } 7983 7984 public String fhirType() { 7985 return "Claim.item.detail.subDetail"; 7986 7987 } 7988 7989 } 7990 7991 /** 7992 * A unique identifier assigned to this claim. 7993 */ 7994 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7995 @Description(shortDefinition="Business Identifier for claim", formalDefinition="A unique identifier assigned to this claim." ) 7996 protected List<Identifier> identifier; 7997 7998 /** 7999 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 8000 */ 8001 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8002 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 8003 protected List<Identifier> traceNumber; 8004 8005 /** 8006 * The status of the resource instance. 8007 */ 8008 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 8009 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 8010 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 8011 protected Enumeration<FinancialResourceStatusCodes> status; 8012 8013 /** 8014 * The category of claim, e.g. oral, pharmacy, vision, institutional, professional. 8015 */ 8016 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 8017 @Description(shortDefinition="Category or discipline", formalDefinition="The category of claim, e.g. oral, pharmacy, vision, institutional, professional." ) 8018 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 8019 protected CodeableConcept type; 8020 8021 /** 8022 * A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service. 8023 */ 8024 @Child(name = "subType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 8025 @Description(shortDefinition="More granular claim type", formalDefinition="A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service." ) 8026 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 8027 protected CodeableConcept subType; 8028 8029 /** 8030 * A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 8031 */ 8032 @Child(name = "use", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 8033 @Description(shortDefinition="claim | preauthorization | predetermination", formalDefinition="A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided." ) 8034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 8035 protected Enumeration<Use> use; 8036 8037 /** 8038 * The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought. 8039 */ 8040 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 8041 @Description(shortDefinition="The recipient of the products and services", formalDefinition="The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought." ) 8042 protected Reference patient; 8043 8044 /** 8045 * The period for which charges are being submitted. 8046 */ 8047 @Child(name = "billablePeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 8048 @Description(shortDefinition="Relevant time frame for the claim", formalDefinition="The period for which charges are being submitted." ) 8049 protected Period billablePeriod; 8050 8051 /** 8052 * The date this resource was created. 8053 */ 8054 @Child(name = "created", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 8055 @Description(shortDefinition="Resource creation date", formalDefinition="The date this resource was created." ) 8056 protected DateTimeType created; 8057 8058 /** 8059 * Individual who created the claim, predetermination or preauthorization. 8060 */ 8061 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=false) 8062 @Description(shortDefinition="Author of the claim", formalDefinition="Individual who created the claim, predetermination or preauthorization." ) 8063 protected Reference enterer; 8064 8065 /** 8066 * The Insurer who is target of the request. 8067 */ 8068 @Child(name = "insurer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=true) 8069 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 8070 protected Reference insurer; 8071 8072 /** 8073 * The provider which is responsible for the claim, predetermination or preauthorization. 8074 */ 8075 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 8076 @Description(shortDefinition="Party responsible for the claim", formalDefinition="The provider which is responsible for the claim, predetermination or preauthorization." ) 8077 protected Reference provider; 8078 8079 /** 8080 * The provider-required urgency of processing the request. Typical values include: stat, normal, deferred. 8081 */ 8082 @Child(name = "priority", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 8083 @Description(shortDefinition="Desired processing urgency", formalDefinition="The provider-required urgency of processing the request. Typical values include: stat, normal, deferred." ) 8084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 8085 protected CodeableConcept priority; 8086 8087 /** 8088 * A code to indicate whether and for whom funds are to be reserved for future claims. 8089 */ 8090 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 8091 @Description(shortDefinition="For whom to reserve funds", formalDefinition="A code to indicate whether and for whom funds are to be reserved for future claims." ) 8092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 8093 protected CodeableConcept fundsReserve; 8094 8095 /** 8096 * Other claims which are related to this claim such as prior submissions or claims for related services or for the same event. 8097 */ 8098 @Child(name = "related", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8099 @Description(shortDefinition="Prior or corollary claims", formalDefinition="Other claims which are related to this claim such as prior submissions or claims for related services or for the same event." ) 8100 protected List<RelatedClaimComponent> related; 8101 8102 /** 8103 * Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments. 8104 */ 8105 @Child(name = "prescription", type = {DeviceRequest.class, MedicationRequest.class, VisionPrescription.class}, order=15, min=0, max=1, modifier=false, summary=false) 8106 @Description(shortDefinition="Prescription authorizing services and products", formalDefinition="Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments." ) 8107 protected Reference prescription; 8108 8109 /** 8110 * Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products. 8111 */ 8112 @Child(name = "originalPrescription", type = {DeviceRequest.class, MedicationRequest.class, VisionPrescription.class}, order=16, min=0, max=1, modifier=false, summary=false) 8113 @Description(shortDefinition="Original prescription if superseded by fulfiller", formalDefinition="Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products." ) 8114 protected Reference originalPrescription; 8115 8116 /** 8117 * The party to be reimbursed for cost of the products and services according to the terms of the policy. 8118 */ 8119 @Child(name = "payee", type = {}, order=17, min=0, max=1, modifier=false, summary=false) 8120 @Description(shortDefinition="Recipient of benefits payable", formalDefinition="The party to be reimbursed for cost of the products and services according to the terms of the policy." ) 8121 protected PayeeComponent payee; 8122 8123 /** 8124 * The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services. 8125 */ 8126 @Child(name = "referral", type = {ServiceRequest.class}, order=18, min=0, max=1, modifier=false, summary=false) 8127 @Description(shortDefinition="Treatment referral", formalDefinition="The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services." ) 8128 protected Reference referral; 8129 8130 /** 8131 * Healthcare encounters related to this claim. 8132 */ 8133 @Child(name = "encounter", type = {Encounter.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8134 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 8135 protected List<Reference> encounter; 8136 8137 /** 8138 * Facility where the services were provided. 8139 */ 8140 @Child(name = "facility", type = {Location.class, Organization.class}, order=20, min=0, max=1, modifier=false, summary=false) 8141 @Description(shortDefinition="Servicing facility", formalDefinition="Facility where the services were provided." ) 8142 protected Reference facility; 8143 8144 /** 8145 * A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system. 8146 */ 8147 @Child(name = "diagnosisRelatedGroup", type = {CodeableConcept.class}, order=21, min=0, max=1, modifier=false, summary=false) 8148 @Description(shortDefinition="Package billing code", formalDefinition="A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system." ) 8149 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 8150 protected CodeableConcept diagnosisRelatedGroup; 8151 8152 /** 8153 * Information code for an event with a corresponding date or period. 8154 */ 8155 @Child(name = "event", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8156 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 8157 protected List<ClaimEventComponent> event; 8158 8159 /** 8160 * The members of the team who provided the products and services. 8161 */ 8162 @Child(name = "careTeam", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8163 @Description(shortDefinition="Members of the care team", formalDefinition="The members of the team who provided the products and services." ) 8164 protected List<CareTeamComponent> careTeam; 8165 8166 /** 8167 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. 8168 */ 8169 @Child(name = "supportingInfo", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8170 @Description(shortDefinition="Supporting information", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues." ) 8171 protected List<SupportingInformationComponent> supportingInfo; 8172 8173 /** 8174 * Information about diagnoses relevant to the claim items. 8175 */ 8176 @Child(name = "diagnosis", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8177 @Description(shortDefinition="Pertinent diagnosis information", formalDefinition="Information about diagnoses relevant to the claim items." ) 8178 protected List<DiagnosisComponent> diagnosis; 8179 8180 /** 8181 * Procedures performed on the patient relevant to the billing items with the claim. 8182 */ 8183 @Child(name = "procedure", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8184 @Description(shortDefinition="Clinical procedures performed", formalDefinition="Procedures performed on the patient relevant to the billing items with the claim." ) 8185 protected List<ProcedureComponent> procedure; 8186 8187 /** 8188 * Financial instruments for reimbursement for the health care products and services specified on the claim. 8189 */ 8190 @Child(name = "insurance", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8191 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services specified on the claim." ) 8192 protected List<InsuranceComponent> insurance; 8193 8194 /** 8195 * Details of an accident which resulted in injuries which required the products and services listed in the claim. 8196 */ 8197 @Child(name = "accident", type = {}, order=28, min=0, max=1, modifier=false, summary=false) 8198 @Description(shortDefinition="Details of the event", formalDefinition="Details of an accident which resulted in injuries which required the products and services listed in the claim." ) 8199 protected AccidentComponent accident; 8200 8201 /** 8202 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 8203 */ 8204 @Child(name = "patientPaid", type = {Money.class}, order=29, min=0, max=1, modifier=false, summary=false) 8205 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 8206 protected Money patientPaid; 8207 8208 /** 8209 * A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details. 8210 */ 8211 @Child(name = "item", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8212 @Description(shortDefinition="Product or service provided", formalDefinition="A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details." ) 8213 protected List<ItemComponent> item; 8214 8215 /** 8216 * The total value of the all the items in the claim. 8217 */ 8218 @Child(name = "total", type = {Money.class}, order=31, min=0, max=1, modifier=false, summary=false) 8219 @Description(shortDefinition="Total claim cost", formalDefinition="The total value of the all the items in the claim." ) 8220 protected Money total; 8221 8222 private static final long serialVersionUID = -64601265L; 8223 8224 /** 8225 * Constructor 8226 */ 8227 public Claim() { 8228 super(); 8229 } 8230 8231 /** 8232 * Constructor 8233 */ 8234 public Claim(FinancialResourceStatusCodes status, CodeableConcept type, Use use, Reference patient, Date created) { 8235 super(); 8236 this.setStatus(status); 8237 this.setType(type); 8238 this.setUse(use); 8239 this.setPatient(patient); 8240 this.setCreated(created); 8241 } 8242 8243 /** 8244 * @return {@link #identifier} (A unique identifier assigned to this claim.) 8245 */ 8246 public List<Identifier> getIdentifier() { 8247 if (this.identifier == null) 8248 this.identifier = new ArrayList<Identifier>(); 8249 return this.identifier; 8250 } 8251 8252 /** 8253 * @return Returns a reference to <code>this</code> for easy method chaining 8254 */ 8255 public Claim setIdentifier(List<Identifier> theIdentifier) { 8256 this.identifier = theIdentifier; 8257 return this; 8258 } 8259 8260 public boolean hasIdentifier() { 8261 if (this.identifier == null) 8262 return false; 8263 for (Identifier item : this.identifier) 8264 if (!item.isEmpty()) 8265 return true; 8266 return false; 8267 } 8268 8269 public Identifier addIdentifier() { //3 8270 Identifier t = new Identifier(); 8271 if (this.identifier == null) 8272 this.identifier = new ArrayList<Identifier>(); 8273 this.identifier.add(t); 8274 return t; 8275 } 8276 8277 public Claim addIdentifier(Identifier t) { //3 8278 if (t == null) 8279 return this; 8280 if (this.identifier == null) 8281 this.identifier = new ArrayList<Identifier>(); 8282 this.identifier.add(t); 8283 return this; 8284 } 8285 8286 /** 8287 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 8288 */ 8289 public Identifier getIdentifierFirstRep() { 8290 if (getIdentifier().isEmpty()) { 8291 addIdentifier(); 8292 } 8293 return getIdentifier().get(0); 8294 } 8295 8296 /** 8297 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 8298 */ 8299 public List<Identifier> getTraceNumber() { 8300 if (this.traceNumber == null) 8301 this.traceNumber = new ArrayList<Identifier>(); 8302 return this.traceNumber; 8303 } 8304 8305 /** 8306 * @return Returns a reference to <code>this</code> for easy method chaining 8307 */ 8308 public Claim setTraceNumber(List<Identifier> theTraceNumber) { 8309 this.traceNumber = theTraceNumber; 8310 return this; 8311 } 8312 8313 public boolean hasTraceNumber() { 8314 if (this.traceNumber == null) 8315 return false; 8316 for (Identifier item : this.traceNumber) 8317 if (!item.isEmpty()) 8318 return true; 8319 return false; 8320 } 8321 8322 public Identifier addTraceNumber() { //3 8323 Identifier t = new Identifier(); 8324 if (this.traceNumber == null) 8325 this.traceNumber = new ArrayList<Identifier>(); 8326 this.traceNumber.add(t); 8327 return t; 8328 } 8329 8330 public Claim addTraceNumber(Identifier t) { //3 8331 if (t == null) 8332 return this; 8333 if (this.traceNumber == null) 8334 this.traceNumber = new ArrayList<Identifier>(); 8335 this.traceNumber.add(t); 8336 return this; 8337 } 8338 8339 /** 8340 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 8341 */ 8342 public Identifier getTraceNumberFirstRep() { 8343 if (getTraceNumber().isEmpty()) { 8344 addTraceNumber(); 8345 } 8346 return getTraceNumber().get(0); 8347 } 8348 8349 /** 8350 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8351 */ 8352 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 8353 if (this.status == null) 8354 if (Configuration.errorOnAutoCreate()) 8355 throw new Error("Attempt to auto-create Claim.status"); 8356 else if (Configuration.doAutoCreate()) 8357 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 8358 return this.status; 8359 } 8360 8361 public boolean hasStatusElement() { 8362 return this.status != null && !this.status.isEmpty(); 8363 } 8364 8365 public boolean hasStatus() { 8366 return this.status != null && !this.status.isEmpty(); 8367 } 8368 8369 /** 8370 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8371 */ 8372 public Claim setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 8373 this.status = value; 8374 return this; 8375 } 8376 8377 /** 8378 * @return The status of the resource instance. 8379 */ 8380 public FinancialResourceStatusCodes getStatus() { 8381 return this.status == null ? null : this.status.getValue(); 8382 } 8383 8384 /** 8385 * @param value The status of the resource instance. 8386 */ 8387 public Claim setStatus(FinancialResourceStatusCodes value) { 8388 if (this.status == null) 8389 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 8390 this.status.setValue(value); 8391 return this; 8392 } 8393 8394 /** 8395 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 8396 */ 8397 public CodeableConcept getType() { 8398 if (this.type == null) 8399 if (Configuration.errorOnAutoCreate()) 8400 throw new Error("Attempt to auto-create Claim.type"); 8401 else if (Configuration.doAutoCreate()) 8402 this.type = new CodeableConcept(); // cc 8403 return this.type; 8404 } 8405 8406 public boolean hasType() { 8407 return this.type != null && !this.type.isEmpty(); 8408 } 8409 8410 /** 8411 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 8412 */ 8413 public Claim setType(CodeableConcept value) { 8414 this.type = value; 8415 return this; 8416 } 8417 8418 /** 8419 * @return {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 8420 */ 8421 public CodeableConcept getSubType() { 8422 if (this.subType == null) 8423 if (Configuration.errorOnAutoCreate()) 8424 throw new Error("Attempt to auto-create Claim.subType"); 8425 else if (Configuration.doAutoCreate()) 8426 this.subType = new CodeableConcept(); // cc 8427 return this.subType; 8428 } 8429 8430 public boolean hasSubType() { 8431 return this.subType != null && !this.subType.isEmpty(); 8432 } 8433 8434 /** 8435 * @param value {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 8436 */ 8437 public Claim setSubType(CodeableConcept value) { 8438 this.subType = value; 8439 return this; 8440 } 8441 8442 /** 8443 * @return {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 8444 */ 8445 public Enumeration<Use> getUseElement() { 8446 if (this.use == null) 8447 if (Configuration.errorOnAutoCreate()) 8448 throw new Error("Attempt to auto-create Claim.use"); 8449 else if (Configuration.doAutoCreate()) 8450 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 8451 return this.use; 8452 } 8453 8454 public boolean hasUseElement() { 8455 return this.use != null && !this.use.isEmpty(); 8456 } 8457 8458 public boolean hasUse() { 8459 return this.use != null && !this.use.isEmpty(); 8460 } 8461 8462 /** 8463 * @param value {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 8464 */ 8465 public Claim setUseElement(Enumeration<Use> value) { 8466 this.use = value; 8467 return this; 8468 } 8469 8470 /** 8471 * @return A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 8472 */ 8473 public Use getUse() { 8474 return this.use == null ? null : this.use.getValue(); 8475 } 8476 8477 /** 8478 * @param value A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 8479 */ 8480 public Claim setUse(Use value) { 8481 if (this.use == null) 8482 this.use = new Enumeration<Use>(new UseEnumFactory()); 8483 this.use.setValue(value); 8484 return this; 8485 } 8486 8487 /** 8488 * @return {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.) 8489 */ 8490 public Reference getPatient() { 8491 if (this.patient == null) 8492 if (Configuration.errorOnAutoCreate()) 8493 throw new Error("Attempt to auto-create Claim.patient"); 8494 else if (Configuration.doAutoCreate()) 8495 this.patient = new Reference(); // cc 8496 return this.patient; 8497 } 8498 8499 public boolean hasPatient() { 8500 return this.patient != null && !this.patient.isEmpty(); 8501 } 8502 8503 /** 8504 * @param value {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.) 8505 */ 8506 public Claim setPatient(Reference value) { 8507 this.patient = value; 8508 return this; 8509 } 8510 8511 /** 8512 * @return {@link #billablePeriod} (The period for which charges are being submitted.) 8513 */ 8514 public Period getBillablePeriod() { 8515 if (this.billablePeriod == null) 8516 if (Configuration.errorOnAutoCreate()) 8517 throw new Error("Attempt to auto-create Claim.billablePeriod"); 8518 else if (Configuration.doAutoCreate()) 8519 this.billablePeriod = new Period(); // cc 8520 return this.billablePeriod; 8521 } 8522 8523 public boolean hasBillablePeriod() { 8524 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 8525 } 8526 8527 /** 8528 * @param value {@link #billablePeriod} (The period for which charges are being submitted.) 8529 */ 8530 public Claim setBillablePeriod(Period value) { 8531 this.billablePeriod = value; 8532 return this; 8533 } 8534 8535 /** 8536 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 8537 */ 8538 public DateTimeType getCreatedElement() { 8539 if (this.created == null) 8540 if (Configuration.errorOnAutoCreate()) 8541 throw new Error("Attempt to auto-create Claim.created"); 8542 else if (Configuration.doAutoCreate()) 8543 this.created = new DateTimeType(); // bb 8544 return this.created; 8545 } 8546 8547 public boolean hasCreatedElement() { 8548 return this.created != null && !this.created.isEmpty(); 8549 } 8550 8551 public boolean hasCreated() { 8552 return this.created != null && !this.created.isEmpty(); 8553 } 8554 8555 /** 8556 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 8557 */ 8558 public Claim setCreatedElement(DateTimeType value) { 8559 this.created = value; 8560 return this; 8561 } 8562 8563 /** 8564 * @return The date this resource was created. 8565 */ 8566 public Date getCreated() { 8567 return this.created == null ? null : this.created.getValue(); 8568 } 8569 8570 /** 8571 * @param value The date this resource was created. 8572 */ 8573 public Claim setCreated(Date value) { 8574 if (this.created == null) 8575 this.created = new DateTimeType(); 8576 this.created.setValue(value); 8577 return this; 8578 } 8579 8580 /** 8581 * @return {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 8582 */ 8583 public Reference getEnterer() { 8584 if (this.enterer == null) 8585 if (Configuration.errorOnAutoCreate()) 8586 throw new Error("Attempt to auto-create Claim.enterer"); 8587 else if (Configuration.doAutoCreate()) 8588 this.enterer = new Reference(); // cc 8589 return this.enterer; 8590 } 8591 8592 public boolean hasEnterer() { 8593 return this.enterer != null && !this.enterer.isEmpty(); 8594 } 8595 8596 /** 8597 * @param value {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 8598 */ 8599 public Claim setEnterer(Reference value) { 8600 this.enterer = value; 8601 return this; 8602 } 8603 8604 /** 8605 * @return {@link #insurer} (The Insurer who is target of the request.) 8606 */ 8607 public Reference getInsurer() { 8608 if (this.insurer == null) 8609 if (Configuration.errorOnAutoCreate()) 8610 throw new Error("Attempt to auto-create Claim.insurer"); 8611 else if (Configuration.doAutoCreate()) 8612 this.insurer = new Reference(); // cc 8613 return this.insurer; 8614 } 8615 8616 public boolean hasInsurer() { 8617 return this.insurer != null && !this.insurer.isEmpty(); 8618 } 8619 8620 /** 8621 * @param value {@link #insurer} (The Insurer who is target of the request.) 8622 */ 8623 public Claim setInsurer(Reference value) { 8624 this.insurer = value; 8625 return this; 8626 } 8627 8628 /** 8629 * @return {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 8630 */ 8631 public Reference getProvider() { 8632 if (this.provider == null) 8633 if (Configuration.errorOnAutoCreate()) 8634 throw new Error("Attempt to auto-create Claim.provider"); 8635 else if (Configuration.doAutoCreate()) 8636 this.provider = new Reference(); // cc 8637 return this.provider; 8638 } 8639 8640 public boolean hasProvider() { 8641 return this.provider != null && !this.provider.isEmpty(); 8642 } 8643 8644 /** 8645 * @param value {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 8646 */ 8647 public Claim setProvider(Reference value) { 8648 this.provider = value; 8649 return this; 8650 } 8651 8652 /** 8653 * @return {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.) 8654 */ 8655 public CodeableConcept getPriority() { 8656 if (this.priority == null) 8657 if (Configuration.errorOnAutoCreate()) 8658 throw new Error("Attempt to auto-create Claim.priority"); 8659 else if (Configuration.doAutoCreate()) 8660 this.priority = new CodeableConcept(); // cc 8661 return this.priority; 8662 } 8663 8664 public boolean hasPriority() { 8665 return this.priority != null && !this.priority.isEmpty(); 8666 } 8667 8668 /** 8669 * @param value {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.) 8670 */ 8671 public Claim setPriority(CodeableConcept value) { 8672 this.priority = value; 8673 return this; 8674 } 8675 8676 /** 8677 * @return {@link #fundsReserve} (A code to indicate whether and for whom funds are to be reserved for future claims.) 8678 */ 8679 public CodeableConcept getFundsReserve() { 8680 if (this.fundsReserve == null) 8681 if (Configuration.errorOnAutoCreate()) 8682 throw new Error("Attempt to auto-create Claim.fundsReserve"); 8683 else if (Configuration.doAutoCreate()) 8684 this.fundsReserve = new CodeableConcept(); // cc 8685 return this.fundsReserve; 8686 } 8687 8688 public boolean hasFundsReserve() { 8689 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 8690 } 8691 8692 /** 8693 * @param value {@link #fundsReserve} (A code to indicate whether and for whom funds are to be reserved for future claims.) 8694 */ 8695 public Claim setFundsReserve(CodeableConcept value) { 8696 this.fundsReserve = value; 8697 return this; 8698 } 8699 8700 /** 8701 * @return {@link #related} (Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.) 8702 */ 8703 public List<RelatedClaimComponent> getRelated() { 8704 if (this.related == null) 8705 this.related = new ArrayList<RelatedClaimComponent>(); 8706 return this.related; 8707 } 8708 8709 /** 8710 * @return Returns a reference to <code>this</code> for easy method chaining 8711 */ 8712 public Claim setRelated(List<RelatedClaimComponent> theRelated) { 8713 this.related = theRelated; 8714 return this; 8715 } 8716 8717 public boolean hasRelated() { 8718 if (this.related == null) 8719 return false; 8720 for (RelatedClaimComponent item : this.related) 8721 if (!item.isEmpty()) 8722 return true; 8723 return false; 8724 } 8725 8726 public RelatedClaimComponent addRelated() { //3 8727 RelatedClaimComponent t = new RelatedClaimComponent(); 8728 if (this.related == null) 8729 this.related = new ArrayList<RelatedClaimComponent>(); 8730 this.related.add(t); 8731 return t; 8732 } 8733 8734 public Claim addRelated(RelatedClaimComponent t) { //3 8735 if (t == null) 8736 return this; 8737 if (this.related == null) 8738 this.related = new ArrayList<RelatedClaimComponent>(); 8739 this.related.add(t); 8740 return this; 8741 } 8742 8743 /** 8744 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist {3} 8745 */ 8746 public RelatedClaimComponent getRelatedFirstRep() { 8747 if (getRelated().isEmpty()) { 8748 addRelated(); 8749 } 8750 return getRelated().get(0); 8751 } 8752 8753 /** 8754 * @return {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 8755 */ 8756 public Reference getPrescription() { 8757 if (this.prescription == null) 8758 if (Configuration.errorOnAutoCreate()) 8759 throw new Error("Attempt to auto-create Claim.prescription"); 8760 else if (Configuration.doAutoCreate()) 8761 this.prescription = new Reference(); // cc 8762 return this.prescription; 8763 } 8764 8765 public boolean hasPrescription() { 8766 return this.prescription != null && !this.prescription.isEmpty(); 8767 } 8768 8769 /** 8770 * @param value {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 8771 */ 8772 public Claim setPrescription(Reference value) { 8773 this.prescription = value; 8774 return this; 8775 } 8776 8777 /** 8778 * @return {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 8779 */ 8780 public Reference getOriginalPrescription() { 8781 if (this.originalPrescription == null) 8782 if (Configuration.errorOnAutoCreate()) 8783 throw new Error("Attempt to auto-create Claim.originalPrescription"); 8784 else if (Configuration.doAutoCreate()) 8785 this.originalPrescription = new Reference(); // cc 8786 return this.originalPrescription; 8787 } 8788 8789 public boolean hasOriginalPrescription() { 8790 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 8791 } 8792 8793 /** 8794 * @param value {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 8795 */ 8796 public Claim setOriginalPrescription(Reference value) { 8797 this.originalPrescription = value; 8798 return this; 8799 } 8800 8801 /** 8802 * @return {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 8803 */ 8804 public PayeeComponent getPayee() { 8805 if (this.payee == null) 8806 if (Configuration.errorOnAutoCreate()) 8807 throw new Error("Attempt to auto-create Claim.payee"); 8808 else if (Configuration.doAutoCreate()) 8809 this.payee = new PayeeComponent(); // cc 8810 return this.payee; 8811 } 8812 8813 public boolean hasPayee() { 8814 return this.payee != null && !this.payee.isEmpty(); 8815 } 8816 8817 /** 8818 * @param value {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 8819 */ 8820 public Claim setPayee(PayeeComponent value) { 8821 this.payee = value; 8822 return this; 8823 } 8824 8825 /** 8826 * @return {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 8827 */ 8828 public Reference getReferral() { 8829 if (this.referral == null) 8830 if (Configuration.errorOnAutoCreate()) 8831 throw new Error("Attempt to auto-create Claim.referral"); 8832 else if (Configuration.doAutoCreate()) 8833 this.referral = new Reference(); // cc 8834 return this.referral; 8835 } 8836 8837 public boolean hasReferral() { 8838 return this.referral != null && !this.referral.isEmpty(); 8839 } 8840 8841 /** 8842 * @param value {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 8843 */ 8844 public Claim setReferral(Reference value) { 8845 this.referral = value; 8846 return this; 8847 } 8848 8849 /** 8850 * @return {@link #encounter} (Healthcare encounters related to this claim.) 8851 */ 8852 public List<Reference> getEncounter() { 8853 if (this.encounter == null) 8854 this.encounter = new ArrayList<Reference>(); 8855 return this.encounter; 8856 } 8857 8858 /** 8859 * @return Returns a reference to <code>this</code> for easy method chaining 8860 */ 8861 public Claim setEncounter(List<Reference> theEncounter) { 8862 this.encounter = theEncounter; 8863 return this; 8864 } 8865 8866 public boolean hasEncounter() { 8867 if (this.encounter == null) 8868 return false; 8869 for (Reference item : this.encounter) 8870 if (!item.isEmpty()) 8871 return true; 8872 return false; 8873 } 8874 8875 public Reference addEncounter() { //3 8876 Reference t = new Reference(); 8877 if (this.encounter == null) 8878 this.encounter = new ArrayList<Reference>(); 8879 this.encounter.add(t); 8880 return t; 8881 } 8882 8883 public Claim addEncounter(Reference t) { //3 8884 if (t == null) 8885 return this; 8886 if (this.encounter == null) 8887 this.encounter = new ArrayList<Reference>(); 8888 this.encounter.add(t); 8889 return this; 8890 } 8891 8892 /** 8893 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 8894 */ 8895 public Reference getEncounterFirstRep() { 8896 if (getEncounter().isEmpty()) { 8897 addEncounter(); 8898 } 8899 return getEncounter().get(0); 8900 } 8901 8902 /** 8903 * @return {@link #facility} (Facility where the services were provided.) 8904 */ 8905 public Reference getFacility() { 8906 if (this.facility == null) 8907 if (Configuration.errorOnAutoCreate()) 8908 throw new Error("Attempt to auto-create Claim.facility"); 8909 else if (Configuration.doAutoCreate()) 8910 this.facility = new Reference(); // cc 8911 return this.facility; 8912 } 8913 8914 public boolean hasFacility() { 8915 return this.facility != null && !this.facility.isEmpty(); 8916 } 8917 8918 /** 8919 * @param value {@link #facility} (Facility where the services were provided.) 8920 */ 8921 public Claim setFacility(Reference value) { 8922 this.facility = value; 8923 return this; 8924 } 8925 8926 /** 8927 * @return {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 8928 */ 8929 public CodeableConcept getDiagnosisRelatedGroup() { 8930 if (this.diagnosisRelatedGroup == null) 8931 if (Configuration.errorOnAutoCreate()) 8932 throw new Error("Attempt to auto-create Claim.diagnosisRelatedGroup"); 8933 else if (Configuration.doAutoCreate()) 8934 this.diagnosisRelatedGroup = new CodeableConcept(); // cc 8935 return this.diagnosisRelatedGroup; 8936 } 8937 8938 public boolean hasDiagnosisRelatedGroup() { 8939 return this.diagnosisRelatedGroup != null && !this.diagnosisRelatedGroup.isEmpty(); 8940 } 8941 8942 /** 8943 * @param value {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 8944 */ 8945 public Claim setDiagnosisRelatedGroup(CodeableConcept value) { 8946 this.diagnosisRelatedGroup = value; 8947 return this; 8948 } 8949 8950 /** 8951 * @return {@link #event} (Information code for an event with a corresponding date or period.) 8952 */ 8953 public List<ClaimEventComponent> getEvent() { 8954 if (this.event == null) 8955 this.event = new ArrayList<ClaimEventComponent>(); 8956 return this.event; 8957 } 8958 8959 /** 8960 * @return Returns a reference to <code>this</code> for easy method chaining 8961 */ 8962 public Claim setEvent(List<ClaimEventComponent> theEvent) { 8963 this.event = theEvent; 8964 return this; 8965 } 8966 8967 public boolean hasEvent() { 8968 if (this.event == null) 8969 return false; 8970 for (ClaimEventComponent item : this.event) 8971 if (!item.isEmpty()) 8972 return true; 8973 return false; 8974 } 8975 8976 public ClaimEventComponent addEvent() { //3 8977 ClaimEventComponent t = new ClaimEventComponent(); 8978 if (this.event == null) 8979 this.event = new ArrayList<ClaimEventComponent>(); 8980 this.event.add(t); 8981 return t; 8982 } 8983 8984 public Claim addEvent(ClaimEventComponent t) { //3 8985 if (t == null) 8986 return this; 8987 if (this.event == null) 8988 this.event = new ArrayList<ClaimEventComponent>(); 8989 this.event.add(t); 8990 return this; 8991 } 8992 8993 /** 8994 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 8995 */ 8996 public ClaimEventComponent getEventFirstRep() { 8997 if (getEvent().isEmpty()) { 8998 addEvent(); 8999 } 9000 return getEvent().get(0); 9001 } 9002 9003 /** 9004 * @return {@link #careTeam} (The members of the team who provided the products and services.) 9005 */ 9006 public List<CareTeamComponent> getCareTeam() { 9007 if (this.careTeam == null) 9008 this.careTeam = new ArrayList<CareTeamComponent>(); 9009 return this.careTeam; 9010 } 9011 9012 /** 9013 * @return Returns a reference to <code>this</code> for easy method chaining 9014 */ 9015 public Claim setCareTeam(List<CareTeamComponent> theCareTeam) { 9016 this.careTeam = theCareTeam; 9017 return this; 9018 } 9019 9020 public boolean hasCareTeam() { 9021 if (this.careTeam == null) 9022 return false; 9023 for (CareTeamComponent item : this.careTeam) 9024 if (!item.isEmpty()) 9025 return true; 9026 return false; 9027 } 9028 9029 public CareTeamComponent addCareTeam() { //3 9030 CareTeamComponent t = new CareTeamComponent(); 9031 if (this.careTeam == null) 9032 this.careTeam = new ArrayList<CareTeamComponent>(); 9033 this.careTeam.add(t); 9034 return t; 9035 } 9036 9037 public Claim addCareTeam(CareTeamComponent t) { //3 9038 if (t == null) 9039 return this; 9040 if (this.careTeam == null) 9041 this.careTeam = new ArrayList<CareTeamComponent>(); 9042 this.careTeam.add(t); 9043 return this; 9044 } 9045 9046 /** 9047 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 9048 */ 9049 public CareTeamComponent getCareTeamFirstRep() { 9050 if (getCareTeam().isEmpty()) { 9051 addCareTeam(); 9052 } 9053 return getCareTeam().get(0); 9054 } 9055 9056 /** 9057 * @return {@link #supportingInfo} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.) 9058 */ 9059 public List<SupportingInformationComponent> getSupportingInfo() { 9060 if (this.supportingInfo == null) 9061 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9062 return this.supportingInfo; 9063 } 9064 9065 /** 9066 * @return Returns a reference to <code>this</code> for easy method chaining 9067 */ 9068 public Claim setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 9069 this.supportingInfo = theSupportingInfo; 9070 return this; 9071 } 9072 9073 public boolean hasSupportingInfo() { 9074 if (this.supportingInfo == null) 9075 return false; 9076 for (SupportingInformationComponent item : this.supportingInfo) 9077 if (!item.isEmpty()) 9078 return true; 9079 return false; 9080 } 9081 9082 public SupportingInformationComponent addSupportingInfo() { //3 9083 SupportingInformationComponent t = new SupportingInformationComponent(); 9084 if (this.supportingInfo == null) 9085 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9086 this.supportingInfo.add(t); 9087 return t; 9088 } 9089 9090 public Claim addSupportingInfo(SupportingInformationComponent t) { //3 9091 if (t == null) 9092 return this; 9093 if (this.supportingInfo == null) 9094 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 9095 this.supportingInfo.add(t); 9096 return this; 9097 } 9098 9099 /** 9100 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 9101 */ 9102 public SupportingInformationComponent getSupportingInfoFirstRep() { 9103 if (getSupportingInfo().isEmpty()) { 9104 addSupportingInfo(); 9105 } 9106 return getSupportingInfo().get(0); 9107 } 9108 9109 /** 9110 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim items.) 9111 */ 9112 public List<DiagnosisComponent> getDiagnosis() { 9113 if (this.diagnosis == null) 9114 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9115 return this.diagnosis; 9116 } 9117 9118 /** 9119 * @return Returns a reference to <code>this</code> for easy method chaining 9120 */ 9121 public Claim setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 9122 this.diagnosis = theDiagnosis; 9123 return this; 9124 } 9125 9126 public boolean hasDiagnosis() { 9127 if (this.diagnosis == null) 9128 return false; 9129 for (DiagnosisComponent item : this.diagnosis) 9130 if (!item.isEmpty()) 9131 return true; 9132 return false; 9133 } 9134 9135 public DiagnosisComponent addDiagnosis() { //3 9136 DiagnosisComponent t = new DiagnosisComponent(); 9137 if (this.diagnosis == null) 9138 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9139 this.diagnosis.add(t); 9140 return t; 9141 } 9142 9143 public Claim addDiagnosis(DiagnosisComponent t) { //3 9144 if (t == null) 9145 return this; 9146 if (this.diagnosis == null) 9147 this.diagnosis = new ArrayList<DiagnosisComponent>(); 9148 this.diagnosis.add(t); 9149 return this; 9150 } 9151 9152 /** 9153 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 9154 */ 9155 public DiagnosisComponent getDiagnosisFirstRep() { 9156 if (getDiagnosis().isEmpty()) { 9157 addDiagnosis(); 9158 } 9159 return getDiagnosis().get(0); 9160 } 9161 9162 /** 9163 * @return {@link #procedure} (Procedures performed on the patient relevant to the billing items with the claim.) 9164 */ 9165 public List<ProcedureComponent> getProcedure() { 9166 if (this.procedure == null) 9167 this.procedure = new ArrayList<ProcedureComponent>(); 9168 return this.procedure; 9169 } 9170 9171 /** 9172 * @return Returns a reference to <code>this</code> for easy method chaining 9173 */ 9174 public Claim setProcedure(List<ProcedureComponent> theProcedure) { 9175 this.procedure = theProcedure; 9176 return this; 9177 } 9178 9179 public boolean hasProcedure() { 9180 if (this.procedure == null) 9181 return false; 9182 for (ProcedureComponent item : this.procedure) 9183 if (!item.isEmpty()) 9184 return true; 9185 return false; 9186 } 9187 9188 public ProcedureComponent addProcedure() { //3 9189 ProcedureComponent t = new ProcedureComponent(); 9190 if (this.procedure == null) 9191 this.procedure = new ArrayList<ProcedureComponent>(); 9192 this.procedure.add(t); 9193 return t; 9194 } 9195 9196 public Claim addProcedure(ProcedureComponent t) { //3 9197 if (t == null) 9198 return this; 9199 if (this.procedure == null) 9200 this.procedure = new ArrayList<ProcedureComponent>(); 9201 this.procedure.add(t); 9202 return this; 9203 } 9204 9205 /** 9206 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 9207 */ 9208 public ProcedureComponent getProcedureFirstRep() { 9209 if (getProcedure().isEmpty()) { 9210 addProcedure(); 9211 } 9212 return getProcedure().get(0); 9213 } 9214 9215 /** 9216 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services specified on the claim.) 9217 */ 9218 public List<InsuranceComponent> getInsurance() { 9219 if (this.insurance == null) 9220 this.insurance = new ArrayList<InsuranceComponent>(); 9221 return this.insurance; 9222 } 9223 9224 /** 9225 * @return Returns a reference to <code>this</code> for easy method chaining 9226 */ 9227 public Claim setInsurance(List<InsuranceComponent> theInsurance) { 9228 this.insurance = theInsurance; 9229 return this; 9230 } 9231 9232 public boolean hasInsurance() { 9233 if (this.insurance == null) 9234 return false; 9235 for (InsuranceComponent item : this.insurance) 9236 if (!item.isEmpty()) 9237 return true; 9238 return false; 9239 } 9240 9241 public InsuranceComponent addInsurance() { //3 9242 InsuranceComponent t = new InsuranceComponent(); 9243 if (this.insurance == null) 9244 this.insurance = new ArrayList<InsuranceComponent>(); 9245 this.insurance.add(t); 9246 return t; 9247 } 9248 9249 public Claim addInsurance(InsuranceComponent t) { //3 9250 if (t == null) 9251 return this; 9252 if (this.insurance == null) 9253 this.insurance = new ArrayList<InsuranceComponent>(); 9254 this.insurance.add(t); 9255 return this; 9256 } 9257 9258 /** 9259 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 9260 */ 9261 public InsuranceComponent getInsuranceFirstRep() { 9262 if (getInsurance().isEmpty()) { 9263 addInsurance(); 9264 } 9265 return getInsurance().get(0); 9266 } 9267 9268 /** 9269 * @return {@link #accident} (Details of an accident which resulted in injuries which required the products and services listed in the claim.) 9270 */ 9271 public AccidentComponent getAccident() { 9272 if (this.accident == null) 9273 if (Configuration.errorOnAutoCreate()) 9274 throw new Error("Attempt to auto-create Claim.accident"); 9275 else if (Configuration.doAutoCreate()) 9276 this.accident = new AccidentComponent(); // cc 9277 return this.accident; 9278 } 9279 9280 public boolean hasAccident() { 9281 return this.accident != null && !this.accident.isEmpty(); 9282 } 9283 9284 /** 9285 * @param value {@link #accident} (Details of an accident which resulted in injuries which required the products and services listed in the claim.) 9286 */ 9287 public Claim setAccident(AccidentComponent value) { 9288 this.accident = value; 9289 return this; 9290 } 9291 9292 /** 9293 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 9294 */ 9295 public Money getPatientPaid() { 9296 if (this.patientPaid == null) 9297 if (Configuration.errorOnAutoCreate()) 9298 throw new Error("Attempt to auto-create Claim.patientPaid"); 9299 else if (Configuration.doAutoCreate()) 9300 this.patientPaid = new Money(); // cc 9301 return this.patientPaid; 9302 } 9303 9304 public boolean hasPatientPaid() { 9305 return this.patientPaid != null && !this.patientPaid.isEmpty(); 9306 } 9307 9308 /** 9309 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 9310 */ 9311 public Claim setPatientPaid(Money value) { 9312 this.patientPaid = value; 9313 return this; 9314 } 9315 9316 /** 9317 * @return {@link #item} (A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.) 9318 */ 9319 public List<ItemComponent> getItem() { 9320 if (this.item == null) 9321 this.item = new ArrayList<ItemComponent>(); 9322 return this.item; 9323 } 9324 9325 /** 9326 * @return Returns a reference to <code>this</code> for easy method chaining 9327 */ 9328 public Claim setItem(List<ItemComponent> theItem) { 9329 this.item = theItem; 9330 return this; 9331 } 9332 9333 public boolean hasItem() { 9334 if (this.item == null) 9335 return false; 9336 for (ItemComponent item : this.item) 9337 if (!item.isEmpty()) 9338 return true; 9339 return false; 9340 } 9341 9342 public ItemComponent addItem() { //3 9343 ItemComponent t = new ItemComponent(); 9344 if (this.item == null) 9345 this.item = new ArrayList<ItemComponent>(); 9346 this.item.add(t); 9347 return t; 9348 } 9349 9350 public Claim addItem(ItemComponent t) { //3 9351 if (t == null) 9352 return this; 9353 if (this.item == null) 9354 this.item = new ArrayList<ItemComponent>(); 9355 this.item.add(t); 9356 return this; 9357 } 9358 9359 /** 9360 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 9361 */ 9362 public ItemComponent getItemFirstRep() { 9363 if (getItem().isEmpty()) { 9364 addItem(); 9365 } 9366 return getItem().get(0); 9367 } 9368 9369 /** 9370 * @return {@link #total} (The total value of the all the items in the claim.) 9371 */ 9372 public Money getTotal() { 9373 if (this.total == null) 9374 if (Configuration.errorOnAutoCreate()) 9375 throw new Error("Attempt to auto-create Claim.total"); 9376 else if (Configuration.doAutoCreate()) 9377 this.total = new Money(); // cc 9378 return this.total; 9379 } 9380 9381 public boolean hasTotal() { 9382 return this.total != null && !this.total.isEmpty(); 9383 } 9384 9385 /** 9386 * @param value {@link #total} (The total value of the all the items in the claim.) 9387 */ 9388 public Claim setTotal(Money value) { 9389 this.total = value; 9390 return this; 9391 } 9392 9393 protected void listChildren(List<Property> children) { 9394 super.listChildren(children); 9395 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, java.lang.Integer.MAX_VALUE, identifier)); 9396 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 9397 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 9398 children.add(new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 9399 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType)); 9400 children.add(new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use)); 9401 children.add(new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 0, 1, patient)); 9402 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod)); 9403 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 9404 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 9405 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 9406 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 9407 children.add(new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.", 0, 1, priority)); 9408 children.add(new Property("fundsReserve", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve)); 9409 children.add(new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related)); 9410 children.add(new Property("prescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription)); 9411 children.add(new Property("originalPrescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription)); 9412 children.add(new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee)); 9413 children.add(new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral)); 9414 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 9415 children.add(new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility)); 9416 children.add(new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup)); 9417 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 9418 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 9419 children.add(new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 9420 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 9421 children.add(new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure)); 9422 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance)); 9423 children.add(new Property("accident", "", "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident)); 9424 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 9425 children.add(new Property("item", "", "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item)); 9426 children.add(new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, total)); 9427 } 9428 9429 @Override 9430 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9431 switch (_hash) { 9432 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this claim.", 0, java.lang.Integer.MAX_VALUE, identifier); 9433 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 9434 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 9435 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 9436 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType); 9437 case 116103: /*use*/ return new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use); 9438 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual or forecast reimbursement is sought.", 0, 1, patient); 9439 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod); 9440 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 9441 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 9442 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 9443 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 9444 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal, deferred.", 0, 1, priority); 9445 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserve); 9446 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related); 9447 case 460301338: /*prescription*/ return new Property("prescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription); 9448 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(DeviceRequest|MedicationRequest|VisionPrescription)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription); 9449 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee); 9450 case -722568291: /*referral*/ return new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral); 9451 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 9452 case 501116579: /*facility*/ return new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility); 9453 case -1599182171: /*diagnosisRelatedGroup*/ return new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup); 9454 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 9455 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 9456 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 9457 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 9458 case -1095204141: /*procedure*/ return new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure); 9459 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance); 9460 case -2143202801: /*accident*/ return new Property("accident", "", "Details of an accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident); 9461 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 9462 case 3242771: /*item*/ return new Property("item", "", "A claim line. Either a simple product or service or a 'group' of details which can each be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item); 9463 case 110549828: /*total*/ return new Property("total", "Money", "The total value of the all the items in the claim.", 0, 1, total); 9464 default: return super.getNamedProperty(_hash, _name, _checkValid); 9465 } 9466 9467 } 9468 9469 @Override 9470 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9471 switch (hash) { 9472 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 9473 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 9474 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 9475 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 9476 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 9477 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 9478 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 9479 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 9480 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 9481 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 9482 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 9483 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 9484 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 9485 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 9486 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 9487 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 9488 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 9489 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 9490 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 9491 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 9492 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 9493 case -1599182171: /*diagnosisRelatedGroup*/ return this.diagnosisRelatedGroup == null ? new Base[0] : new Base[] {this.diagnosisRelatedGroup}; // CodeableConcept 9494 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // ClaimEventComponent 9495 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 9496 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 9497 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 9498 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 9499 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 9500 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 9501 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 9502 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 9503 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // Money 9504 default: return super.getProperty(hash, name, checkValid); 9505 } 9506 9507 } 9508 9509 @Override 9510 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9511 switch (hash) { 9512 case -1618432855: // identifier 9513 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 9514 return value; 9515 case 82505966: // traceNumber 9516 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 9517 return value; 9518 case -892481550: // status 9519 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 9520 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 9521 return value; 9522 case 3575610: // type 9523 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9524 return value; 9525 case -1868521062: // subType 9526 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9527 return value; 9528 case 116103: // use 9529 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 9530 this.use = (Enumeration) value; // Enumeration<Use> 9531 return value; 9532 case -791418107: // patient 9533 this.patient = TypeConvertor.castToReference(value); // Reference 9534 return value; 9535 case -332066046: // billablePeriod 9536 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 9537 return value; 9538 case 1028554472: // created 9539 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 9540 return value; 9541 case -1591951995: // enterer 9542 this.enterer = TypeConvertor.castToReference(value); // Reference 9543 return value; 9544 case 1957615864: // insurer 9545 this.insurer = TypeConvertor.castToReference(value); // Reference 9546 return value; 9547 case -987494927: // provider 9548 this.provider = TypeConvertor.castToReference(value); // Reference 9549 return value; 9550 case -1165461084: // priority 9551 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9552 return value; 9553 case 1314609806: // fundsReserve 9554 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9555 return value; 9556 case 1090493483: // related 9557 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 9558 return value; 9559 case 460301338: // prescription 9560 this.prescription = TypeConvertor.castToReference(value); // Reference 9561 return value; 9562 case -1814015861: // originalPrescription 9563 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 9564 return value; 9565 case 106443592: // payee 9566 this.payee = (PayeeComponent) value; // PayeeComponent 9567 return value; 9568 case -722568291: // referral 9569 this.referral = TypeConvertor.castToReference(value); // Reference 9570 return value; 9571 case 1524132147: // encounter 9572 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 9573 return value; 9574 case 501116579: // facility 9575 this.facility = TypeConvertor.castToReference(value); // Reference 9576 return value; 9577 case -1599182171: // diagnosisRelatedGroup 9578 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9579 return value; 9580 case 96891546: // event 9581 this.getEvent().add((ClaimEventComponent) value); // ClaimEventComponent 9582 return value; 9583 case -7323378: // careTeam 9584 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 9585 return value; 9586 case 1922406657: // supportingInfo 9587 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 9588 return value; 9589 case 1196993265: // diagnosis 9590 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 9591 return value; 9592 case -1095204141: // procedure 9593 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 9594 return value; 9595 case 73049818: // insurance 9596 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 9597 return value; 9598 case -2143202801: // accident 9599 this.accident = (AccidentComponent) value; // AccidentComponent 9600 return value; 9601 case 525514609: // patientPaid 9602 this.patientPaid = TypeConvertor.castToMoney(value); // Money 9603 return value; 9604 case 3242771: // item 9605 this.getItem().add((ItemComponent) value); // ItemComponent 9606 return value; 9607 case 110549828: // total 9608 this.total = TypeConvertor.castToMoney(value); // Money 9609 return value; 9610 default: return super.setProperty(hash, name, value); 9611 } 9612 9613 } 9614 9615 @Override 9616 public Base setProperty(String name, Base value) throws FHIRException { 9617 if (name.equals("identifier")) { 9618 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 9619 } else if (name.equals("traceNumber")) { 9620 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 9621 } else if (name.equals("status")) { 9622 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 9623 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 9624 } else if (name.equals("type")) { 9625 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9626 } else if (name.equals("subType")) { 9627 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9628 } else if (name.equals("use")) { 9629 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 9630 this.use = (Enumeration) value; // Enumeration<Use> 9631 } else if (name.equals("patient")) { 9632 this.patient = TypeConvertor.castToReference(value); // Reference 9633 } else if (name.equals("billablePeriod")) { 9634 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 9635 } else if (name.equals("created")) { 9636 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 9637 } else if (name.equals("enterer")) { 9638 this.enterer = TypeConvertor.castToReference(value); // Reference 9639 } else if (name.equals("insurer")) { 9640 this.insurer = TypeConvertor.castToReference(value); // Reference 9641 } else if (name.equals("provider")) { 9642 this.provider = TypeConvertor.castToReference(value); // Reference 9643 } else if (name.equals("priority")) { 9644 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9645 } else if (name.equals("fundsReserve")) { 9646 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9647 } else if (name.equals("related")) { 9648 this.getRelated().add((RelatedClaimComponent) value); 9649 } else if (name.equals("prescription")) { 9650 this.prescription = TypeConvertor.castToReference(value); // Reference 9651 } else if (name.equals("originalPrescription")) { 9652 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 9653 } else if (name.equals("payee")) { 9654 this.payee = (PayeeComponent) value; // PayeeComponent 9655 } else if (name.equals("referral")) { 9656 this.referral = TypeConvertor.castToReference(value); // Reference 9657 } else if (name.equals("encounter")) { 9658 this.getEncounter().add(TypeConvertor.castToReference(value)); 9659 } else if (name.equals("facility")) { 9660 this.facility = TypeConvertor.castToReference(value); // Reference 9661 } else if (name.equals("diagnosisRelatedGroup")) { 9662 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 9663 } else if (name.equals("event")) { 9664 this.getEvent().add((ClaimEventComponent) value); 9665 } else if (name.equals("careTeam")) { 9666 this.getCareTeam().add((CareTeamComponent) value); 9667 } else if (name.equals("supportingInfo")) { 9668 this.getSupportingInfo().add((SupportingInformationComponent) value); 9669 } else if (name.equals("diagnosis")) { 9670 this.getDiagnosis().add((DiagnosisComponent) value); 9671 } else if (name.equals("procedure")) { 9672 this.getProcedure().add((ProcedureComponent) value); 9673 } else if (name.equals("insurance")) { 9674 this.getInsurance().add((InsuranceComponent) value); 9675 } else if (name.equals("accident")) { 9676 this.accident = (AccidentComponent) value; // AccidentComponent 9677 } else if (name.equals("patientPaid")) { 9678 this.patientPaid = TypeConvertor.castToMoney(value); // Money 9679 } else if (name.equals("item")) { 9680 this.getItem().add((ItemComponent) value); 9681 } else if (name.equals("total")) { 9682 this.total = TypeConvertor.castToMoney(value); // Money 9683 } else 9684 return super.setProperty(name, value); 9685 return value; 9686 } 9687 9688 @Override 9689 public void removeChild(String name, Base value) throws FHIRException { 9690 if (name.equals("identifier")) { 9691 this.getIdentifier().remove(value); 9692 } else if (name.equals("traceNumber")) { 9693 this.getTraceNumber().remove(value); 9694 } else if (name.equals("status")) { 9695 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 9696 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 9697 } else if (name.equals("type")) { 9698 this.type = null; 9699 } else if (name.equals("subType")) { 9700 this.subType = null; 9701 } else if (name.equals("use")) { 9702 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 9703 this.use = (Enumeration) value; // Enumeration<Use> 9704 } else if (name.equals("patient")) { 9705 this.patient = null; 9706 } else if (name.equals("billablePeriod")) { 9707 this.billablePeriod = null; 9708 } else if (name.equals("created")) { 9709 this.created = null; 9710 } else if (name.equals("enterer")) { 9711 this.enterer = null; 9712 } else if (name.equals("insurer")) { 9713 this.insurer = null; 9714 } else if (name.equals("provider")) { 9715 this.provider = null; 9716 } else if (name.equals("priority")) { 9717 this.priority = null; 9718 } else if (name.equals("fundsReserve")) { 9719 this.fundsReserve = null; 9720 } else if (name.equals("related")) { 9721 this.getRelated().remove((RelatedClaimComponent) value); 9722 } else if (name.equals("prescription")) { 9723 this.prescription = null; 9724 } else if (name.equals("originalPrescription")) { 9725 this.originalPrescription = null; 9726 } else if (name.equals("payee")) { 9727 this.payee = (PayeeComponent) value; // PayeeComponent 9728 } else if (name.equals("referral")) { 9729 this.referral = null; 9730 } else if (name.equals("encounter")) { 9731 this.getEncounter().remove(value); 9732 } else if (name.equals("facility")) { 9733 this.facility = null; 9734 } else if (name.equals("diagnosisRelatedGroup")) { 9735 this.diagnosisRelatedGroup = null; 9736 } else if (name.equals("event")) { 9737 this.getEvent().remove((ClaimEventComponent) value); 9738 } else if (name.equals("careTeam")) { 9739 this.getCareTeam().remove((CareTeamComponent) value); 9740 } else if (name.equals("supportingInfo")) { 9741 this.getSupportingInfo().remove((SupportingInformationComponent) value); 9742 } else if (name.equals("diagnosis")) { 9743 this.getDiagnosis().remove((DiagnosisComponent) value); 9744 } else if (name.equals("procedure")) { 9745 this.getProcedure().remove((ProcedureComponent) value); 9746 } else if (name.equals("insurance")) { 9747 this.getInsurance().remove((InsuranceComponent) value); 9748 } else if (name.equals("accident")) { 9749 this.accident = (AccidentComponent) value; // AccidentComponent 9750 } else if (name.equals("patientPaid")) { 9751 this.patientPaid = null; 9752 } else if (name.equals("item")) { 9753 this.getItem().remove((ItemComponent) value); 9754 } else if (name.equals("total")) { 9755 this.total = null; 9756 } else 9757 super.removeChild(name, value); 9758 9759 } 9760 9761 @Override 9762 public Base makeProperty(int hash, String name) throws FHIRException { 9763 switch (hash) { 9764 case -1618432855: return addIdentifier(); 9765 case 82505966: return addTraceNumber(); 9766 case -892481550: return getStatusElement(); 9767 case 3575610: return getType(); 9768 case -1868521062: return getSubType(); 9769 case 116103: return getUseElement(); 9770 case -791418107: return getPatient(); 9771 case -332066046: return getBillablePeriod(); 9772 case 1028554472: return getCreatedElement(); 9773 case -1591951995: return getEnterer(); 9774 case 1957615864: return getInsurer(); 9775 case -987494927: return getProvider(); 9776 case -1165461084: return getPriority(); 9777 case 1314609806: return getFundsReserve(); 9778 case 1090493483: return addRelated(); 9779 case 460301338: return getPrescription(); 9780 case -1814015861: return getOriginalPrescription(); 9781 case 106443592: return getPayee(); 9782 case -722568291: return getReferral(); 9783 case 1524132147: return addEncounter(); 9784 case 501116579: return getFacility(); 9785 case -1599182171: return getDiagnosisRelatedGroup(); 9786 case 96891546: return addEvent(); 9787 case -7323378: return addCareTeam(); 9788 case 1922406657: return addSupportingInfo(); 9789 case 1196993265: return addDiagnosis(); 9790 case -1095204141: return addProcedure(); 9791 case 73049818: return addInsurance(); 9792 case -2143202801: return getAccident(); 9793 case 525514609: return getPatientPaid(); 9794 case 3242771: return addItem(); 9795 case 110549828: return getTotal(); 9796 default: return super.makeProperty(hash, name); 9797 } 9798 9799 } 9800 9801 @Override 9802 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9803 switch (hash) { 9804 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 9805 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 9806 case -892481550: /*status*/ return new String[] {"code"}; 9807 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 9808 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 9809 case 116103: /*use*/ return new String[] {"code"}; 9810 case -791418107: /*patient*/ return new String[] {"Reference"}; 9811 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 9812 case 1028554472: /*created*/ return new String[] {"dateTime"}; 9813 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 9814 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 9815 case -987494927: /*provider*/ return new String[] {"Reference"}; 9816 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 9817 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 9818 case 1090493483: /*related*/ return new String[] {}; 9819 case 460301338: /*prescription*/ return new String[] {"Reference"}; 9820 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 9821 case 106443592: /*payee*/ return new String[] {}; 9822 case -722568291: /*referral*/ return new String[] {"Reference"}; 9823 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 9824 case 501116579: /*facility*/ return new String[] {"Reference"}; 9825 case -1599182171: /*diagnosisRelatedGroup*/ return new String[] {"CodeableConcept"}; 9826 case 96891546: /*event*/ return new String[] {}; 9827 case -7323378: /*careTeam*/ return new String[] {}; 9828 case 1922406657: /*supportingInfo*/ return new String[] {}; 9829 case 1196993265: /*diagnosis*/ return new String[] {}; 9830 case -1095204141: /*procedure*/ return new String[] {}; 9831 case 73049818: /*insurance*/ return new String[] {}; 9832 case -2143202801: /*accident*/ return new String[] {}; 9833 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 9834 case 3242771: /*item*/ return new String[] {}; 9835 case 110549828: /*total*/ return new String[] {"Money"}; 9836 default: return super.getTypesForProperty(hash, name); 9837 } 9838 9839 } 9840 9841 @Override 9842 public Base addChild(String name) throws FHIRException { 9843 if (name.equals("identifier")) { 9844 return addIdentifier(); 9845 } 9846 else if (name.equals("traceNumber")) { 9847 return addTraceNumber(); 9848 } 9849 else if (name.equals("status")) { 9850 throw new FHIRException("Cannot call addChild on a singleton property Claim.status"); 9851 } 9852 else if (name.equals("type")) { 9853 this.type = new CodeableConcept(); 9854 return this.type; 9855 } 9856 else if (name.equals("subType")) { 9857 this.subType = new CodeableConcept(); 9858 return this.subType; 9859 } 9860 else if (name.equals("use")) { 9861 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 9862 } 9863 else if (name.equals("patient")) { 9864 this.patient = new Reference(); 9865 return this.patient; 9866 } 9867 else if (name.equals("billablePeriod")) { 9868 this.billablePeriod = new Period(); 9869 return this.billablePeriod; 9870 } 9871 else if (name.equals("created")) { 9872 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 9873 } 9874 else if (name.equals("enterer")) { 9875 this.enterer = new Reference(); 9876 return this.enterer; 9877 } 9878 else if (name.equals("insurer")) { 9879 this.insurer = new Reference(); 9880 return this.insurer; 9881 } 9882 else if (name.equals("provider")) { 9883 this.provider = new Reference(); 9884 return this.provider; 9885 } 9886 else if (name.equals("priority")) { 9887 this.priority = new CodeableConcept(); 9888 return this.priority; 9889 } 9890 else if (name.equals("fundsReserve")) { 9891 this.fundsReserve = new CodeableConcept(); 9892 return this.fundsReserve; 9893 } 9894 else if (name.equals("related")) { 9895 return addRelated(); 9896 } 9897 else if (name.equals("prescription")) { 9898 this.prescription = new Reference(); 9899 return this.prescription; 9900 } 9901 else if (name.equals("originalPrescription")) { 9902 this.originalPrescription = new Reference(); 9903 return this.originalPrescription; 9904 } 9905 else if (name.equals("payee")) { 9906 this.payee = new PayeeComponent(); 9907 return this.payee; 9908 } 9909 else if (name.equals("referral")) { 9910 this.referral = new Reference(); 9911 return this.referral; 9912 } 9913 else if (name.equals("encounter")) { 9914 return addEncounter(); 9915 } 9916 else if (name.equals("facility")) { 9917 this.facility = new Reference(); 9918 return this.facility; 9919 } 9920 else if (name.equals("diagnosisRelatedGroup")) { 9921 this.diagnosisRelatedGroup = new CodeableConcept(); 9922 return this.diagnosisRelatedGroup; 9923 } 9924 else if (name.equals("event")) { 9925 return addEvent(); 9926 } 9927 else if (name.equals("careTeam")) { 9928 return addCareTeam(); 9929 } 9930 else if (name.equals("supportingInfo")) { 9931 return addSupportingInfo(); 9932 } 9933 else if (name.equals("diagnosis")) { 9934 return addDiagnosis(); 9935 } 9936 else if (name.equals("procedure")) { 9937 return addProcedure(); 9938 } 9939 else if (name.equals("insurance")) { 9940 return addInsurance(); 9941 } 9942 else if (name.equals("accident")) { 9943 this.accident = new AccidentComponent(); 9944 return this.accident; 9945 } 9946 else if (name.equals("patientPaid")) { 9947 this.patientPaid = new Money(); 9948 return this.patientPaid; 9949 } 9950 else if (name.equals("item")) { 9951 return addItem(); 9952 } 9953 else if (name.equals("total")) { 9954 this.total = new Money(); 9955 return this.total; 9956 } 9957 else 9958 return super.addChild(name); 9959 } 9960 9961 public String fhirType() { 9962 return "Claim"; 9963 9964 } 9965 9966 public Claim copy() { 9967 Claim dst = new Claim(); 9968 copyValues(dst); 9969 return dst; 9970 } 9971 9972 public void copyValues(Claim dst) { 9973 super.copyValues(dst); 9974 if (identifier != null) { 9975 dst.identifier = new ArrayList<Identifier>(); 9976 for (Identifier i : identifier) 9977 dst.identifier.add(i.copy()); 9978 }; 9979 if (traceNumber != null) { 9980 dst.traceNumber = new ArrayList<Identifier>(); 9981 for (Identifier i : traceNumber) 9982 dst.traceNumber.add(i.copy()); 9983 }; 9984 dst.status = status == null ? null : status.copy(); 9985 dst.type = type == null ? null : type.copy(); 9986 dst.subType = subType == null ? null : subType.copy(); 9987 dst.use = use == null ? null : use.copy(); 9988 dst.patient = patient == null ? null : patient.copy(); 9989 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 9990 dst.created = created == null ? null : created.copy(); 9991 dst.enterer = enterer == null ? null : enterer.copy(); 9992 dst.insurer = insurer == null ? null : insurer.copy(); 9993 dst.provider = provider == null ? null : provider.copy(); 9994 dst.priority = priority == null ? null : priority.copy(); 9995 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 9996 if (related != null) { 9997 dst.related = new ArrayList<RelatedClaimComponent>(); 9998 for (RelatedClaimComponent i : related) 9999 dst.related.add(i.copy()); 10000 }; 10001 dst.prescription = prescription == null ? null : prescription.copy(); 10002 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 10003 dst.payee = payee == null ? null : payee.copy(); 10004 dst.referral = referral == null ? null : referral.copy(); 10005 if (encounter != null) { 10006 dst.encounter = new ArrayList<Reference>(); 10007 for (Reference i : encounter) 10008 dst.encounter.add(i.copy()); 10009 }; 10010 dst.facility = facility == null ? null : facility.copy(); 10011 dst.diagnosisRelatedGroup = diagnosisRelatedGroup == null ? null : diagnosisRelatedGroup.copy(); 10012 if (event != null) { 10013 dst.event = new ArrayList<ClaimEventComponent>(); 10014 for (ClaimEventComponent i : event) 10015 dst.event.add(i.copy()); 10016 }; 10017 if (careTeam != null) { 10018 dst.careTeam = new ArrayList<CareTeamComponent>(); 10019 for (CareTeamComponent i : careTeam) 10020 dst.careTeam.add(i.copy()); 10021 }; 10022 if (supportingInfo != null) { 10023 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 10024 for (SupportingInformationComponent i : supportingInfo) 10025 dst.supportingInfo.add(i.copy()); 10026 }; 10027 if (diagnosis != null) { 10028 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 10029 for (DiagnosisComponent i : diagnosis) 10030 dst.diagnosis.add(i.copy()); 10031 }; 10032 if (procedure != null) { 10033 dst.procedure = new ArrayList<ProcedureComponent>(); 10034 for (ProcedureComponent i : procedure) 10035 dst.procedure.add(i.copy()); 10036 }; 10037 if (insurance != null) { 10038 dst.insurance = new ArrayList<InsuranceComponent>(); 10039 for (InsuranceComponent i : insurance) 10040 dst.insurance.add(i.copy()); 10041 }; 10042 dst.accident = accident == null ? null : accident.copy(); 10043 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 10044 if (item != null) { 10045 dst.item = new ArrayList<ItemComponent>(); 10046 for (ItemComponent i : item) 10047 dst.item.add(i.copy()); 10048 }; 10049 dst.total = total == null ? null : total.copy(); 10050 } 10051 10052 protected Claim typedCopy() { 10053 return copy(); 10054 } 10055 10056 @Override 10057 public boolean equalsDeep(Base other_) { 10058 if (!super.equalsDeep(other_)) 10059 return false; 10060 if (!(other_ instanceof Claim)) 10061 return false; 10062 Claim o = (Claim) other_; 10063 return compareDeep(identifier, o.identifier, true) && compareDeep(traceNumber, o.traceNumber, true) 10064 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 10065 && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 10066 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) 10067 && compareDeep(provider, o.provider, true) && compareDeep(priority, o.priority, true) && compareDeep(fundsReserve, o.fundsReserve, true) 10068 && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) && compareDeep(originalPrescription, o.originalPrescription, true) 10069 && compareDeep(payee, o.payee, true) && compareDeep(referral, o.referral, true) && compareDeep(encounter, o.encounter, true) 10070 && compareDeep(facility, o.facility, true) && compareDeep(diagnosisRelatedGroup, o.diagnosisRelatedGroup, true) 10071 && compareDeep(event, o.event, true) && compareDeep(careTeam, o.careTeam, true) && compareDeep(supportingInfo, o.supportingInfo, true) 10072 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) && compareDeep(insurance, o.insurance, true) 10073 && compareDeep(accident, o.accident, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(item, o.item, true) 10074 && compareDeep(total, o.total, true); 10075 } 10076 10077 @Override 10078 public boolean equalsShallow(Base other_) { 10079 if (!super.equalsShallow(other_)) 10080 return false; 10081 if (!(other_ instanceof Claim)) 10082 return false; 10083 Claim o = (Claim) other_; 10084 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 10085 ; 10086 } 10087 10088 public boolean isEmpty() { 10089 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, traceNumber, status 10090 , type, subType, use, patient, billablePeriod, created, enterer, insurer, provider 10091 , priority, fundsReserve, related, prescription, originalPrescription, payee, referral 10092 , encounter, facility, diagnosisRelatedGroup, event, careTeam, supportingInfo, diagnosis 10093 , procedure, insurance, accident, patientPaid, item, total); 10094 } 10095 10096 @Override 10097 public ResourceType getResourceType() { 10098 return ResourceType.Claim; 10099 } 10100 10101 /** 10102 * Search parameter: <b>care-team</b> 10103 * <p> 10104 * Description: <b>Member of the CareTeam</b><br> 10105 * Type: <b>reference</b><br> 10106 * Path: <b>Claim.careTeam.provider</b><br> 10107 * </p> 10108 */ 10109 @SearchParamDefinition(name="care-team", path="Claim.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 10110 public static final String SP_CARE_TEAM = "care-team"; 10111 /** 10112 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 10113 * <p> 10114 * Description: <b>Member of the CareTeam</b><br> 10115 * Type: <b>reference</b><br> 10116 * Path: <b>Claim.careTeam.provider</b><br> 10117 * </p> 10118 */ 10119 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 10120 10121/** 10122 * Constant for fluent queries to be used to add include statements. Specifies 10123 * the path value of "<b>Claim:care-team</b>". 10124 */ 10125 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("Claim:care-team").toLocked(); 10126 10127 /** 10128 * Search parameter: <b>created</b> 10129 * <p> 10130 * Description: <b>The creation date for the Claim</b><br> 10131 * Type: <b>date</b><br> 10132 * Path: <b>Claim.created</b><br> 10133 * </p> 10134 */ 10135 @SearchParamDefinition(name="created", path="Claim.created", description="The creation date for the Claim", type="date" ) 10136 public static final String SP_CREATED = "created"; 10137 /** 10138 * <b>Fluent Client</b> search parameter constant for <b>created</b> 10139 * <p> 10140 * Description: <b>The creation date for the Claim</b><br> 10141 * Type: <b>date</b><br> 10142 * Path: <b>Claim.created</b><br> 10143 * </p> 10144 */ 10145 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 10146 10147 /** 10148 * Search parameter: <b>detail-udi</b> 10149 * <p> 10150 * Description: <b>UDI associated with a line item, detail product or service</b><br> 10151 * Type: <b>reference</b><br> 10152 * Path: <b>Claim.item.detail.udi</b><br> 10153 * </p> 10154 */ 10155 @SearchParamDefinition(name="detail-udi", path="Claim.item.detail.udi", description="UDI associated with a line item, detail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 10156 public static final String SP_DETAIL_UDI = "detail-udi"; 10157 /** 10158 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 10159 * <p> 10160 * Description: <b>UDI associated with a line item, detail product or service</b><br> 10161 * Type: <b>reference</b><br> 10162 * Path: <b>Claim.item.detail.udi</b><br> 10163 * </p> 10164 */ 10165 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DETAIL_UDI); 10166 10167/** 10168 * Constant for fluent queries to be used to add include statements. Specifies 10169 * the path value of "<b>Claim:detail-udi</b>". 10170 */ 10171 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include("Claim:detail-udi").toLocked(); 10172 10173 /** 10174 * Search parameter: <b>enterer</b> 10175 * <p> 10176 * Description: <b>The party responsible for the entry of the Claim</b><br> 10177 * Type: <b>reference</b><br> 10178 * Path: <b>Claim.enterer</b><br> 10179 * </p> 10180 */ 10181 @SearchParamDefinition(name="enterer", path="Claim.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 10182 public static final String SP_ENTERER = "enterer"; 10183 /** 10184 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 10185 * <p> 10186 * Description: <b>The party responsible for the entry of the Claim</b><br> 10187 * Type: <b>reference</b><br> 10188 * Path: <b>Claim.enterer</b><br> 10189 * </p> 10190 */ 10191 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 10192 10193/** 10194 * Constant for fluent queries to be used to add include statements. Specifies 10195 * the path value of "<b>Claim:enterer</b>". 10196 */ 10197 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("Claim:enterer").toLocked(); 10198 10199 /** 10200 * Search parameter: <b>facility</b> 10201 * <p> 10202 * Description: <b>Facility where the products or services have been or will be provided</b><br> 10203 * Type: <b>reference</b><br> 10204 * Path: <b>Claim.facility</b><br> 10205 * </p> 10206 */ 10207 @SearchParamDefinition(name="facility", path="Claim.facility", description="Facility where the products or services have been or will be provided", type="reference", target={Location.class, Organization.class } ) 10208 public static final String SP_FACILITY = "facility"; 10209 /** 10210 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 10211 * <p> 10212 * Description: <b>Facility where the products or services have been or will be provided</b><br> 10213 * Type: <b>reference</b><br> 10214 * Path: <b>Claim.facility</b><br> 10215 * </p> 10216 */ 10217 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 10218 10219/** 10220 * Constant for fluent queries to be used to add include statements. Specifies 10221 * the path value of "<b>Claim:facility</b>". 10222 */ 10223 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("Claim:facility").toLocked(); 10224 10225 /** 10226 * Search parameter: <b>insurer</b> 10227 * <p> 10228 * Description: <b>The target payor/insurer for the Claim</b><br> 10229 * Type: <b>reference</b><br> 10230 * Path: <b>Claim.insurer</b><br> 10231 * </p> 10232 */ 10233 @SearchParamDefinition(name="insurer", path="Claim.insurer", description="The target payor/insurer for the Claim", type="reference", target={Organization.class } ) 10234 public static final String SP_INSURER = "insurer"; 10235 /** 10236 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 10237 * <p> 10238 * Description: <b>The target payor/insurer for the Claim</b><br> 10239 * Type: <b>reference</b><br> 10240 * Path: <b>Claim.insurer</b><br> 10241 * </p> 10242 */ 10243 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 10244 10245/** 10246 * Constant for fluent queries to be used to add include statements. Specifies 10247 * the path value of "<b>Claim:insurer</b>". 10248 */ 10249 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Claim:insurer").toLocked(); 10250 10251 /** 10252 * Search parameter: <b>item-udi</b> 10253 * <p> 10254 * Description: <b>UDI associated with a line item product or service</b><br> 10255 * Type: <b>reference</b><br> 10256 * Path: <b>Claim.item.udi</b><br> 10257 * </p> 10258 */ 10259 @SearchParamDefinition(name="item-udi", path="Claim.item.udi", description="UDI associated with a line item product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 10260 public static final String SP_ITEM_UDI = "item-udi"; 10261 /** 10262 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 10263 * <p> 10264 * Description: <b>UDI associated with a line item product or service</b><br> 10265 * Type: <b>reference</b><br> 10266 * Path: <b>Claim.item.udi</b><br> 10267 * </p> 10268 */ 10269 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_UDI); 10270 10271/** 10272 * Constant for fluent queries to be used to add include statements. Specifies 10273 * the path value of "<b>Claim:item-udi</b>". 10274 */ 10275 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include("Claim:item-udi").toLocked(); 10276 10277 /** 10278 * Search parameter: <b>payee</b> 10279 * <p> 10280 * Description: <b>The party receiving any payment for the Claim</b><br> 10281 * Type: <b>reference</b><br> 10282 * Path: <b>Claim.payee.party</b><br> 10283 * </p> 10284 */ 10285 @SearchParamDefinition(name="payee", path="Claim.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 10286 public static final String SP_PAYEE = "payee"; 10287 /** 10288 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 10289 * <p> 10290 * Description: <b>The party receiving any payment for the Claim</b><br> 10291 * Type: <b>reference</b><br> 10292 * Path: <b>Claim.payee.party</b><br> 10293 * </p> 10294 */ 10295 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 10296 10297/** 10298 * Constant for fluent queries to be used to add include statements. Specifies 10299 * the path value of "<b>Claim:payee</b>". 10300 */ 10301 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("Claim:payee").toLocked(); 10302 10303 /** 10304 * Search parameter: <b>priority</b> 10305 * <p> 10306 * Description: <b>Processing priority requested</b><br> 10307 * Type: <b>token</b><br> 10308 * Path: <b>Claim.priority</b><br> 10309 * </p> 10310 */ 10311 @SearchParamDefinition(name="priority", path="Claim.priority", description="Processing priority requested", type="token" ) 10312 public static final String SP_PRIORITY = "priority"; 10313 /** 10314 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 10315 * <p> 10316 * Description: <b>Processing priority requested</b><br> 10317 * Type: <b>token</b><br> 10318 * Path: <b>Claim.priority</b><br> 10319 * </p> 10320 */ 10321 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 10322 10323 /** 10324 * Search parameter: <b>procedure-udi</b> 10325 * <p> 10326 * Description: <b>UDI associated with a procedure</b><br> 10327 * Type: <b>reference</b><br> 10328 * Path: <b>Claim.procedure.udi</b><br> 10329 * </p> 10330 */ 10331 @SearchParamDefinition(name="procedure-udi", path="Claim.procedure.udi", description="UDI associated with a procedure", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 10332 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 10333 /** 10334 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 10335 * <p> 10336 * Description: <b>UDI associated with a procedure</b><br> 10337 * Type: <b>reference</b><br> 10338 * Path: <b>Claim.procedure.udi</b><br> 10339 * </p> 10340 */ 10341 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROCEDURE_UDI); 10342 10343/** 10344 * Constant for fluent queries to be used to add include statements. Specifies 10345 * the path value of "<b>Claim:procedure-udi</b>". 10346 */ 10347 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include("Claim:procedure-udi").toLocked(); 10348 10349 /** 10350 * Search parameter: <b>provider</b> 10351 * <p> 10352 * Description: <b>Provider responsible for the Claim</b><br> 10353 * Type: <b>reference</b><br> 10354 * Path: <b>Claim.provider</b><br> 10355 * </p> 10356 */ 10357 @SearchParamDefinition(name="provider", path="Claim.provider", description="Provider responsible for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 10358 public static final String SP_PROVIDER = "provider"; 10359 /** 10360 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 10361 * <p> 10362 * Description: <b>Provider responsible for the Claim</b><br> 10363 * Type: <b>reference</b><br> 10364 * Path: <b>Claim.provider</b><br> 10365 * </p> 10366 */ 10367 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 10368 10369/** 10370 * Constant for fluent queries to be used to add include statements. Specifies 10371 * the path value of "<b>Claim:provider</b>". 10372 */ 10373 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("Claim:provider").toLocked(); 10374 10375 /** 10376 * Search parameter: <b>status</b> 10377 * <p> 10378 * Description: <b>The status of the Claim instance.</b><br> 10379 * Type: <b>token</b><br> 10380 * Path: <b>Claim.status</b><br> 10381 * </p> 10382 */ 10383 @SearchParamDefinition(name="status", path="Claim.status", description="The status of the Claim instance.", type="token" ) 10384 public static final String SP_STATUS = "status"; 10385 /** 10386 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10387 * <p> 10388 * Description: <b>The status of the Claim instance.</b><br> 10389 * Type: <b>token</b><br> 10390 * Path: <b>Claim.status</b><br> 10391 * </p> 10392 */ 10393 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 10394 10395 /** 10396 * Search parameter: <b>subdetail-udi</b> 10397 * <p> 10398 * Description: <b>UDI associated with a line item, detail, subdetail product or service</b><br> 10399 * Type: <b>reference</b><br> 10400 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 10401 * </p> 10402 */ 10403 @SearchParamDefinition(name="subdetail-udi", path="Claim.item.detail.subDetail.udi", description="UDI associated with a line item, detail, subdetail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 10404 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 10405 /** 10406 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 10407 * <p> 10408 * Description: <b>UDI associated with a line item, detail, subdetail product or service</b><br> 10409 * Type: <b>reference</b><br> 10410 * Path: <b>Claim.item.detail.subDetail.udi</b><br> 10411 * </p> 10412 */ 10413 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBDETAIL_UDI); 10414 10415/** 10416 * Constant for fluent queries to be used to add include statements. Specifies 10417 * the path value of "<b>Claim:subdetail-udi</b>". 10418 */ 10419 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include("Claim:subdetail-udi").toLocked(); 10420 10421 /** 10422 * Search parameter: <b>use</b> 10423 * <p> 10424 * Description: <b>The kind of financial resource</b><br> 10425 * Type: <b>token</b><br> 10426 * Path: <b>Claim.use</b><br> 10427 * </p> 10428 */ 10429 @SearchParamDefinition(name="use", path="Claim.use", description="The kind of financial resource", type="token" ) 10430 public static final String SP_USE = "use"; 10431 /** 10432 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10433 * <p> 10434 * Description: <b>The kind of financial resource</b><br> 10435 * Type: <b>token</b><br> 10436 * Path: <b>Claim.use</b><br> 10437 * </p> 10438 */ 10439 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_USE); 10440 10441 /** 10442 * Search parameter: <b>encounter</b> 10443 * <p> 10444 * Description: <b>Multiple Resources: 10445 10446* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 10447* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 10448* [ChargeItem](chargeitem.html): Encounter associated with event 10449* [Claim](claim.html): Encounters associated with a billed line item 10450* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 10451* [Communication](communication.html): The Encounter during which this Communication was created 10452* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 10453* [Composition](composition.html): Context of the Composition 10454* [Condition](condition.html): The Encounter during which this Condition was created 10455* [DeviceRequest](devicerequest.html): Encounter during which request was created 10456* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 10457* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 10458* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 10459* [Flag](flag.html): Alert relevant during encounter 10460* [ImagingStudy](imagingstudy.html): The context of the study 10461* [List](list.html): Context in which list created 10462* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 10463* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 10464* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 10465* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 10466* [Observation](observation.html): Encounter related to the observation 10467* [Procedure](procedure.html): The Encounter during which this Procedure was created 10468* [Provenance](provenance.html): Encounter related to the Provenance 10469* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 10470* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 10471* [RiskAssessment](riskassessment.html): Where was assessment performed? 10472* [ServiceRequest](servicerequest.html): An encounter in which this request is made 10473* [Task](task.html): Search by encounter 10474* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 10475</b><br> 10476 * Type: <b>reference</b><br> 10477 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 10478 * </p> 10479 */ 10480 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 10481 public static final String SP_ENCOUNTER = "encounter"; 10482 /** 10483 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 10484 * <p> 10485 * Description: <b>Multiple Resources: 10486 10487* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 10488* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 10489* [ChargeItem](chargeitem.html): Encounter associated with event 10490* [Claim](claim.html): Encounters associated with a billed line item 10491* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 10492* [Communication](communication.html): The Encounter during which this Communication was created 10493* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 10494* [Composition](composition.html): Context of the Composition 10495* [Condition](condition.html): The Encounter during which this Condition was created 10496* [DeviceRequest](devicerequest.html): Encounter during which request was created 10497* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 10498* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 10499* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 10500* [Flag](flag.html): Alert relevant during encounter 10501* [ImagingStudy](imagingstudy.html): The context of the study 10502* [List](list.html): Context in which list created 10503* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 10504* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 10505* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 10506* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 10507* [Observation](observation.html): Encounter related to the observation 10508* [Procedure](procedure.html): The Encounter during which this Procedure was created 10509* [Provenance](provenance.html): Encounter related to the Provenance 10510* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 10511* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 10512* [RiskAssessment](riskassessment.html): Where was assessment performed? 10513* [ServiceRequest](servicerequest.html): An encounter in which this request is made 10514* [Task](task.html): Search by encounter 10515* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 10516</b><br> 10517 * Type: <b>reference</b><br> 10518 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 10519 * </p> 10520 */ 10521 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 10522 10523/** 10524 * Constant for fluent queries to be used to add include statements. Specifies 10525 * the path value of "<b>Claim:encounter</b>". 10526 */ 10527 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Claim:encounter").toLocked(); 10528 10529 /** 10530 * Search parameter: <b>identifier</b> 10531 * <p> 10532 * Description: <b>Multiple Resources: 10533 10534* [Account](account.html): Account number 10535* [AdverseEvent](adverseevent.html): Business identifier for the event 10536* [AllergyIntolerance](allergyintolerance.html): External ids for this item 10537* [Appointment](appointment.html): An Identifier of the Appointment 10538* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 10539* [Basic](basic.html): Business identifier 10540* [BodyStructure](bodystructure.html): Bodystructure identifier 10541* [CarePlan](careplan.html): External Ids for this plan 10542* [CareTeam](careteam.html): External Ids for this team 10543* [ChargeItem](chargeitem.html): Business Identifier for item 10544* [Claim](claim.html): The primary identifier of the financial resource 10545* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 10546* [ClinicalImpression](clinicalimpression.html): Business identifier 10547* [Communication](communication.html): Unique identifier 10548* [CommunicationRequest](communicationrequest.html): Unique identifier 10549* [Composition](composition.html): Version-independent identifier for the Composition 10550* [Condition](condition.html): A unique identifier of the condition record 10551* [Consent](consent.html): Identifier for this record (external references) 10552* [Contract](contract.html): The identity of the contract 10553* [Coverage](coverage.html): The primary identifier of the insured and the coverage 10554* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 10555* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 10556* [DetectedIssue](detectedissue.html): Unique id for the detected issue 10557* [DeviceRequest](devicerequest.html): Business identifier for request/order 10558* [DeviceUsage](deviceusage.html): Search by identifier 10559* [DiagnosticReport](diagnosticreport.html): An identifier for the report 10560* [DocumentReference](documentreference.html): Identifier of the attachment binary 10561* [Encounter](encounter.html): Identifier(s) by which this encounter is known 10562* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 10563* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 10564* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 10565* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 10566* [Flag](flag.html): Business identifier 10567* [Goal](goal.html): External Ids for this goal 10568* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 10569* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 10570* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 10571* [Immunization](immunization.html): Business identifier 10572* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 10573* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 10574* [Invoice](invoice.html): Business Identifier for item 10575* [List](list.html): Business identifier 10576* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 10577* [Medication](medication.html): Returns medications with this external identifier 10578* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 10579* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 10580* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 10581* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 10582* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 10583* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 10584* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 10585* [Observation](observation.html): The unique id for a particular observation 10586* [Person](person.html): A person Identifier 10587* [Procedure](procedure.html): A unique identifier for a procedure 10588* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 10589* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 10590* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 10591* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 10592* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 10593* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 10594* [Specimen](specimen.html): The unique identifier associated with the specimen 10595* [SupplyDelivery](supplydelivery.html): External identifier 10596* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 10597* [Task](task.html): Search for a task instance by its business identifier 10598* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 10599</b><br> 10600 * Type: <b>token</b><br> 10601 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 10602 * </p> 10603 */ 10604 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 10605 public static final String SP_IDENTIFIER = "identifier"; 10606 /** 10607 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 10608 * <p> 10609 * Description: <b>Multiple Resources: 10610 10611* [Account](account.html): Account number 10612* [AdverseEvent](adverseevent.html): Business identifier for the event 10613* [AllergyIntolerance](allergyintolerance.html): External ids for this item 10614* [Appointment](appointment.html): An Identifier of the Appointment 10615* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 10616* [Basic](basic.html): Business identifier 10617* [BodyStructure](bodystructure.html): Bodystructure identifier 10618* [CarePlan](careplan.html): External Ids for this plan 10619* [CareTeam](careteam.html): External Ids for this team 10620* [ChargeItem](chargeitem.html): Business Identifier for item 10621* [Claim](claim.html): The primary identifier of the financial resource 10622* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 10623* [ClinicalImpression](clinicalimpression.html): Business identifier 10624* [Communication](communication.html): Unique identifier 10625* [CommunicationRequest](communicationrequest.html): Unique identifier 10626* [Composition](composition.html): Version-independent identifier for the Composition 10627* [Condition](condition.html): A unique identifier of the condition record 10628* [Consent](consent.html): Identifier for this record (external references) 10629* [Contract](contract.html): The identity of the contract 10630* [Coverage](coverage.html): The primary identifier of the insured and the coverage 10631* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 10632* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 10633* [DetectedIssue](detectedissue.html): Unique id for the detected issue 10634* [DeviceRequest](devicerequest.html): Business identifier for request/order 10635* [DeviceUsage](deviceusage.html): Search by identifier 10636* [DiagnosticReport](diagnosticreport.html): An identifier for the report 10637* [DocumentReference](documentreference.html): Identifier of the attachment binary 10638* [Encounter](encounter.html): Identifier(s) by which this encounter is known 10639* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 10640* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 10641* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 10642* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 10643* [Flag](flag.html): Business identifier 10644* [Goal](goal.html): External Ids for this goal 10645* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 10646* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 10647* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 10648* [Immunization](immunization.html): Business identifier 10649* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 10650* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 10651* [Invoice](invoice.html): Business Identifier for item 10652* [List](list.html): Business identifier 10653* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 10654* [Medication](medication.html): Returns medications with this external identifier 10655* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 10656* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 10657* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 10658* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 10659* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 10660* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 10661* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 10662* [Observation](observation.html): The unique id for a particular observation 10663* [Person](person.html): A person Identifier 10664* [Procedure](procedure.html): A unique identifier for a procedure 10665* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 10666* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 10667* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 10668* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 10669* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 10670* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 10671* [Specimen](specimen.html): The unique identifier associated with the specimen 10672* [SupplyDelivery](supplydelivery.html): External identifier 10673* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 10674* [Task](task.html): Search for a task instance by its business identifier 10675* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 10676</b><br> 10677 * Type: <b>token</b><br> 10678 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 10679 * </p> 10680 */ 10681 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 10682 10683 /** 10684 * Search parameter: <b>patient</b> 10685 * <p> 10686 * Description: <b>Multiple Resources: 10687 10688* [Account](account.html): The entity that caused the expenses 10689* [AdverseEvent](adverseevent.html): Subject impacted by event 10690* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 10691* [Appointment](appointment.html): One of the individuals of the appointment is this patient 10692* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 10693* [AuditEvent](auditevent.html): Where the activity involved patient data 10694* [Basic](basic.html): Identifies the focus of this resource 10695* [BodyStructure](bodystructure.html): Who this is about 10696* [CarePlan](careplan.html): Who the care plan is for 10697* [CareTeam](careteam.html): Who care team is for 10698* [ChargeItem](chargeitem.html): Individual service was done for/to 10699* [Claim](claim.html): Patient receiving the products or services 10700* [ClaimResponse](claimresponse.html): The subject of care 10701* [ClinicalImpression](clinicalimpression.html): Patient assessed 10702* [Communication](communication.html): Focus of message 10703* [CommunicationRequest](communicationrequest.html): Focus of message 10704* [Composition](composition.html): Who and/or what the composition is about 10705* [Condition](condition.html): Who has the condition? 10706* [Consent](consent.html): Who the consent applies to 10707* [Contract](contract.html): The identity of the subject of the contract (if a patient) 10708* [Coverage](coverage.html): Retrieve coverages for a patient 10709* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 10710* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 10711* [DetectedIssue](detectedissue.html): Associated patient 10712* [DeviceRequest](devicerequest.html): Individual the service is ordered for 10713* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 10714* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 10715* [DocumentReference](documentreference.html): Who/what is the subject of the document 10716* [Encounter](encounter.html): The patient present at the encounter 10717* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 10718* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 10719* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 10720* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 10721* [Flag](flag.html): The identity of a subject to list flags for 10722* [Goal](goal.html): Who this goal is intended for 10723* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 10724* [ImagingSelection](imagingselection.html): Who the study is about 10725* [ImagingStudy](imagingstudy.html): Who the study is about 10726* [Immunization](immunization.html): The patient for the vaccination record 10727* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 10728* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 10729* [Invoice](invoice.html): Recipient(s) of goods and services 10730* [List](list.html): If all resources have the same subject 10731* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 10732* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 10733* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 10734* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 10735* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 10736* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 10737* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 10738* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 10739* [Observation](observation.html): The subject that the observation is about (if patient) 10740* [Person](person.html): The Person links to this Patient 10741* [Procedure](procedure.html): Search by subject - a patient 10742* [Provenance](provenance.html): Where the activity involved patient data 10743* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 10744* [RelatedPerson](relatedperson.html): The patient this related person is related to 10745* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 10746* [ResearchSubject](researchsubject.html): Who or what is part of study 10747* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 10748* [ServiceRequest](servicerequest.html): Search by subject - a patient 10749* [Specimen](specimen.html): The patient the specimen comes from 10750* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 10751* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 10752* [Task](task.html): Search by patient 10753* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 10754</b><br> 10755 * Type: <b>reference</b><br> 10756 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 10757 * </p> 10758 */ 10759 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 10760 public static final String SP_PATIENT = "patient"; 10761 /** 10762 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 10763 * <p> 10764 * Description: <b>Multiple Resources: 10765 10766* [Account](account.html): The entity that caused the expenses 10767* [AdverseEvent](adverseevent.html): Subject impacted by event 10768* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 10769* [Appointment](appointment.html): One of the individuals of the appointment is this patient 10770* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 10771* [AuditEvent](auditevent.html): Where the activity involved patient data 10772* [Basic](basic.html): Identifies the focus of this resource 10773* [BodyStructure](bodystructure.html): Who this is about 10774* [CarePlan](careplan.html): Who the care plan is for 10775* [CareTeam](careteam.html): Who care team is for 10776* [ChargeItem](chargeitem.html): Individual service was done for/to 10777* [Claim](claim.html): Patient receiving the products or services 10778* [ClaimResponse](claimresponse.html): The subject of care 10779* [ClinicalImpression](clinicalimpression.html): Patient assessed 10780* [Communication](communication.html): Focus of message 10781* [CommunicationRequest](communicationrequest.html): Focus of message 10782* [Composition](composition.html): Who and/or what the composition is about 10783* [Condition](condition.html): Who has the condition? 10784* [Consent](consent.html): Who the consent applies to 10785* [Contract](contract.html): The identity of the subject of the contract (if a patient) 10786* [Coverage](coverage.html): Retrieve coverages for a patient 10787* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 10788* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 10789* [DetectedIssue](detectedissue.html): Associated patient 10790* [DeviceRequest](devicerequest.html): Individual the service is ordered for 10791* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 10792* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 10793* [DocumentReference](documentreference.html): Who/what is the subject of the document 10794* [Encounter](encounter.html): The patient present at the encounter 10795* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 10796* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 10797* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 10798* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 10799* [Flag](flag.html): The identity of a subject to list flags for 10800* [Goal](goal.html): Who this goal is intended for 10801* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 10802* [ImagingSelection](imagingselection.html): Who the study is about 10803* [ImagingStudy](imagingstudy.html): Who the study is about 10804* [Immunization](immunization.html): The patient for the vaccination record 10805* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 10806* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 10807* [Invoice](invoice.html): Recipient(s) of goods and services 10808* [List](list.html): If all resources have the same subject 10809* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 10810* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 10811* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 10812* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 10813* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 10814* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 10815* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 10816* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 10817* [Observation](observation.html): The subject that the observation is about (if patient) 10818* [Person](person.html): The Person links to this Patient 10819* [Procedure](procedure.html): Search by subject - a patient 10820* [Provenance](provenance.html): Where the activity involved patient data 10821* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 10822* [RelatedPerson](relatedperson.html): The patient this related person is related to 10823* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 10824* [ResearchSubject](researchsubject.html): Who or what is part of study 10825* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 10826* [ServiceRequest](servicerequest.html): Search by subject - a patient 10827* [Specimen](specimen.html): The patient the specimen comes from 10828* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 10829* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 10830* [Task](task.html): Search by patient 10831* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 10832</b><br> 10833 * Type: <b>reference</b><br> 10834 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 10835 * </p> 10836 */ 10837 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 10838 10839/** 10840 * Constant for fluent queries to be used to add include statements. Specifies 10841 * the path value of "<b>Claim:patient</b>". 10842 */ 10843 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Claim:patient").toLocked(); 10844 10845 10846} 10847