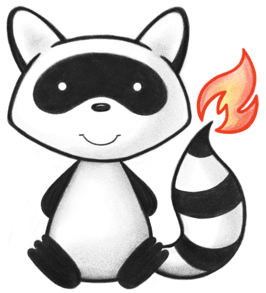
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * This resource provides the adjudication details from the processing of a Claim resource. 053 */ 054@ResourceDef(name="ClaimResponse", profile="http://hl7.org/fhir/StructureDefinition/ClaimResponse") 055public class ClaimResponse extends DomainResource { 056 057 @Block() 058 public static class ClaimResponseEventComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * A coded event such as when a service is expected or a card printed. 061 */ 062 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 063 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 064 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 065 protected CodeableConcept type; 066 067 /** 068 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 069 */ 070 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 071 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 072 protected DataType when; 073 074 private static final long serialVersionUID = -634897375L; 075 076 /** 077 * Constructor 078 */ 079 public ClaimResponseEventComponent() { 080 super(); 081 } 082 083 /** 084 * Constructor 085 */ 086 public ClaimResponseEventComponent(CodeableConcept type, DataType when) { 087 super(); 088 this.setType(type); 089 this.setWhen(when); 090 } 091 092 /** 093 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 094 */ 095 public CodeableConcept getType() { 096 if (this.type == null) 097 if (Configuration.errorOnAutoCreate()) 098 throw new Error("Attempt to auto-create ClaimResponseEventComponent.type"); 099 else if (Configuration.doAutoCreate()) 100 this.type = new CodeableConcept(); // cc 101 return this.type; 102 } 103 104 public boolean hasType() { 105 return this.type != null && !this.type.isEmpty(); 106 } 107 108 /** 109 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 110 */ 111 public ClaimResponseEventComponent setType(CodeableConcept value) { 112 this.type = value; 113 return this; 114 } 115 116 /** 117 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 118 */ 119 public DataType getWhen() { 120 return this.when; 121 } 122 123 /** 124 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 125 */ 126 public DateTimeType getWhenDateTimeType() throws FHIRException { 127 if (this.when == null) 128 this.when = new DateTimeType(); 129 if (!(this.when instanceof DateTimeType)) 130 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 131 return (DateTimeType) this.when; 132 } 133 134 public boolean hasWhenDateTimeType() { 135 return this != null && this.when instanceof DateTimeType; 136 } 137 138 /** 139 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 140 */ 141 public Period getWhenPeriod() throws FHIRException { 142 if (this.when == null) 143 this.when = new Period(); 144 if (!(this.when instanceof Period)) 145 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 146 return (Period) this.when; 147 } 148 149 public boolean hasWhenPeriod() { 150 return this != null && this.when instanceof Period; 151 } 152 153 public boolean hasWhen() { 154 return this.when != null && !this.when.isEmpty(); 155 } 156 157 /** 158 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 159 */ 160 public ClaimResponseEventComponent setWhen(DataType value) { 161 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 162 throw new FHIRException("Not the right type for ClaimResponse.event.when[x]: "+value.fhirType()); 163 this.when = value; 164 return this; 165 } 166 167 protected void listChildren(List<Property> children) { 168 super.listChildren(children); 169 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 170 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 171 } 172 173 @Override 174 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 175 switch (_hash) { 176 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 177 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 178 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 179 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 180 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 181 default: return super.getNamedProperty(_hash, _name, _checkValid); 182 } 183 184 } 185 186 @Override 187 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 188 switch (hash) { 189 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 190 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 191 default: return super.getProperty(hash, name, checkValid); 192 } 193 194 } 195 196 @Override 197 public Base setProperty(int hash, String name, Base value) throws FHIRException { 198 switch (hash) { 199 case 3575610: // type 200 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 201 return value; 202 case 3648314: // when 203 this.when = TypeConvertor.castToType(value); // DataType 204 return value; 205 default: return super.setProperty(hash, name, value); 206 } 207 208 } 209 210 @Override 211 public Base setProperty(String name, Base value) throws FHIRException { 212 if (name.equals("type")) { 213 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 214 } else if (name.equals("when[x]")) { 215 this.when = TypeConvertor.castToType(value); // DataType 216 } else 217 return super.setProperty(name, value); 218 return value; 219 } 220 221 @Override 222 public void removeChild(String name, Base value) throws FHIRException { 223 if (name.equals("type")) { 224 this.type = null; 225 } else if (name.equals("when[x]")) { 226 this.when = null; 227 } else 228 super.removeChild(name, value); 229 230 } 231 232 @Override 233 public Base makeProperty(int hash, String name) throws FHIRException { 234 switch (hash) { 235 case 3575610: return getType(); 236 case 1312831238: return getWhen(); 237 case 3648314: return getWhen(); 238 default: return super.makeProperty(hash, name); 239 } 240 241 } 242 243 @Override 244 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 245 switch (hash) { 246 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 247 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 248 default: return super.getTypesForProperty(hash, name); 249 } 250 251 } 252 253 @Override 254 public Base addChild(String name) throws FHIRException { 255 if (name.equals("type")) { 256 this.type = new CodeableConcept(); 257 return this.type; 258 } 259 else if (name.equals("whenDateTime")) { 260 this.when = new DateTimeType(); 261 return this.when; 262 } 263 else if (name.equals("whenPeriod")) { 264 this.when = new Period(); 265 return this.when; 266 } 267 else 268 return super.addChild(name); 269 } 270 271 public ClaimResponseEventComponent copy() { 272 ClaimResponseEventComponent dst = new ClaimResponseEventComponent(); 273 copyValues(dst); 274 return dst; 275 } 276 277 public void copyValues(ClaimResponseEventComponent dst) { 278 super.copyValues(dst); 279 dst.type = type == null ? null : type.copy(); 280 dst.when = when == null ? null : when.copy(); 281 } 282 283 @Override 284 public boolean equalsDeep(Base other_) { 285 if (!super.equalsDeep(other_)) 286 return false; 287 if (!(other_ instanceof ClaimResponseEventComponent)) 288 return false; 289 ClaimResponseEventComponent o = (ClaimResponseEventComponent) other_; 290 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 291 } 292 293 @Override 294 public boolean equalsShallow(Base other_) { 295 if (!super.equalsShallow(other_)) 296 return false; 297 if (!(other_ instanceof ClaimResponseEventComponent)) 298 return false; 299 ClaimResponseEventComponent o = (ClaimResponseEventComponent) other_; 300 return true; 301 } 302 303 public boolean isEmpty() { 304 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 305 } 306 307 public String fhirType() { 308 return "ClaimResponse.event"; 309 310 } 311 312 } 313 314 @Block() 315 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 316 /** 317 * A number to uniquely reference the claim item entries. 318 */ 319 @Child(name = "itemSequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 320 @Description(shortDefinition="Claim item instance identifier", formalDefinition="A number to uniquely reference the claim item entries." ) 321 protected PositiveIntType itemSequence; 322 323 /** 324 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 325 */ 326 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 327 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 328 protected List<Identifier> traceNumber; 329 330 /** 331 * The numbers associated with notes below which apply to the adjudication of this item. 332 */ 333 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 334 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 335 protected List<PositiveIntType> noteNumber; 336 337 /** 338 * The high-level results of the adjudication if adjudication has been performed. 339 */ 340 @Child(name = "reviewOutcome", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 341 @Description(shortDefinition="Adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 342 protected ReviewOutcomeComponent reviewOutcome; 343 344 /** 345 * If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item. 346 */ 347 @Child(name = "adjudication", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 348 @Description(shortDefinition="Adjudication details", formalDefinition="If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item." ) 349 protected List<AdjudicationComponent> adjudication; 350 351 /** 352 * A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 353 */ 354 @Child(name = "detail", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 355 @Description(shortDefinition="Adjudication for claim details", formalDefinition="A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 356 protected List<ItemDetailComponent> detail; 357 358 private static final long serialVersionUID = -1834500828L; 359 360 /** 361 * Constructor 362 */ 363 public ItemComponent() { 364 super(); 365 } 366 367 /** 368 * Constructor 369 */ 370 public ItemComponent(int itemSequence) { 371 super(); 372 this.setItemSequence(itemSequence); 373 } 374 375 /** 376 * @return {@link #itemSequence} (A number to uniquely reference the claim item entries.). This is the underlying object with id, value and extensions. The accessor "getItemSequence" gives direct access to the value 377 */ 378 public PositiveIntType getItemSequenceElement() { 379 if (this.itemSequence == null) 380 if (Configuration.errorOnAutoCreate()) 381 throw new Error("Attempt to auto-create ItemComponent.itemSequence"); 382 else if (Configuration.doAutoCreate()) 383 this.itemSequence = new PositiveIntType(); // bb 384 return this.itemSequence; 385 } 386 387 public boolean hasItemSequenceElement() { 388 return this.itemSequence != null && !this.itemSequence.isEmpty(); 389 } 390 391 public boolean hasItemSequence() { 392 return this.itemSequence != null && !this.itemSequence.isEmpty(); 393 } 394 395 /** 396 * @param value {@link #itemSequence} (A number to uniquely reference the claim item entries.). This is the underlying object with id, value and extensions. The accessor "getItemSequence" gives direct access to the value 397 */ 398 public ItemComponent setItemSequenceElement(PositiveIntType value) { 399 this.itemSequence = value; 400 return this; 401 } 402 403 /** 404 * @return A number to uniquely reference the claim item entries. 405 */ 406 public int getItemSequence() { 407 return this.itemSequence == null || this.itemSequence.isEmpty() ? 0 : this.itemSequence.getValue(); 408 } 409 410 /** 411 * @param value A number to uniquely reference the claim item entries. 412 */ 413 public ItemComponent setItemSequence(int value) { 414 if (this.itemSequence == null) 415 this.itemSequence = new PositiveIntType(); 416 this.itemSequence.setValue(value); 417 return this; 418 } 419 420 /** 421 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 422 */ 423 public List<Identifier> getTraceNumber() { 424 if (this.traceNumber == null) 425 this.traceNumber = new ArrayList<Identifier>(); 426 return this.traceNumber; 427 } 428 429 /** 430 * @return Returns a reference to <code>this</code> for easy method chaining 431 */ 432 public ItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 433 this.traceNumber = theTraceNumber; 434 return this; 435 } 436 437 public boolean hasTraceNumber() { 438 if (this.traceNumber == null) 439 return false; 440 for (Identifier item : this.traceNumber) 441 if (!item.isEmpty()) 442 return true; 443 return false; 444 } 445 446 public Identifier addTraceNumber() { //3 447 Identifier t = new Identifier(); 448 if (this.traceNumber == null) 449 this.traceNumber = new ArrayList<Identifier>(); 450 this.traceNumber.add(t); 451 return t; 452 } 453 454 public ItemComponent addTraceNumber(Identifier t) { //3 455 if (t == null) 456 return this; 457 if (this.traceNumber == null) 458 this.traceNumber = new ArrayList<Identifier>(); 459 this.traceNumber.add(t); 460 return this; 461 } 462 463 /** 464 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 465 */ 466 public Identifier getTraceNumberFirstRep() { 467 if (getTraceNumber().isEmpty()) { 468 addTraceNumber(); 469 } 470 return getTraceNumber().get(0); 471 } 472 473 /** 474 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 475 */ 476 public List<PositiveIntType> getNoteNumber() { 477 if (this.noteNumber == null) 478 this.noteNumber = new ArrayList<PositiveIntType>(); 479 return this.noteNumber; 480 } 481 482 /** 483 * @return Returns a reference to <code>this</code> for easy method chaining 484 */ 485 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 486 this.noteNumber = theNoteNumber; 487 return this; 488 } 489 490 public boolean hasNoteNumber() { 491 if (this.noteNumber == null) 492 return false; 493 for (PositiveIntType item : this.noteNumber) 494 if (!item.isEmpty()) 495 return true; 496 return false; 497 } 498 499 /** 500 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 501 */ 502 public PositiveIntType addNoteNumberElement() {//2 503 PositiveIntType t = new PositiveIntType(); 504 if (this.noteNumber == null) 505 this.noteNumber = new ArrayList<PositiveIntType>(); 506 this.noteNumber.add(t); 507 return t; 508 } 509 510 /** 511 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 512 */ 513 public ItemComponent addNoteNumber(int value) { //1 514 PositiveIntType t = new PositiveIntType(); 515 t.setValue(value); 516 if (this.noteNumber == null) 517 this.noteNumber = new ArrayList<PositiveIntType>(); 518 this.noteNumber.add(t); 519 return this; 520 } 521 522 /** 523 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 524 */ 525 public boolean hasNoteNumber(int value) { 526 if (this.noteNumber == null) 527 return false; 528 for (PositiveIntType v : this.noteNumber) 529 if (v.getValue().equals(value)) // positiveInt 530 return true; 531 return false; 532 } 533 534 /** 535 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 536 */ 537 public ReviewOutcomeComponent getReviewOutcome() { 538 if (this.reviewOutcome == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create ItemComponent.reviewOutcome"); 541 else if (Configuration.doAutoCreate()) 542 this.reviewOutcome = new ReviewOutcomeComponent(); // cc 543 return this.reviewOutcome; 544 } 545 546 public boolean hasReviewOutcome() { 547 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 552 */ 553 public ItemComponent setReviewOutcome(ReviewOutcomeComponent value) { 554 this.reviewOutcome = value; 555 return this; 556 } 557 558 /** 559 * @return {@link #adjudication} (If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.) 560 */ 561 public List<AdjudicationComponent> getAdjudication() { 562 if (this.adjudication == null) 563 this.adjudication = new ArrayList<AdjudicationComponent>(); 564 return this.adjudication; 565 } 566 567 /** 568 * @return Returns a reference to <code>this</code> for easy method chaining 569 */ 570 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 571 this.adjudication = theAdjudication; 572 return this; 573 } 574 575 public boolean hasAdjudication() { 576 if (this.adjudication == null) 577 return false; 578 for (AdjudicationComponent item : this.adjudication) 579 if (!item.isEmpty()) 580 return true; 581 return false; 582 } 583 584 public AdjudicationComponent addAdjudication() { //3 585 AdjudicationComponent t = new AdjudicationComponent(); 586 if (this.adjudication == null) 587 this.adjudication = new ArrayList<AdjudicationComponent>(); 588 this.adjudication.add(t); 589 return t; 590 } 591 592 public ItemComponent addAdjudication(AdjudicationComponent t) { //3 593 if (t == null) 594 return this; 595 if (this.adjudication == null) 596 this.adjudication = new ArrayList<AdjudicationComponent>(); 597 this.adjudication.add(t); 598 return this; 599 } 600 601 /** 602 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 603 */ 604 public AdjudicationComponent getAdjudicationFirstRep() { 605 if (getAdjudication().isEmpty()) { 606 addAdjudication(); 607 } 608 return getAdjudication().get(0); 609 } 610 611 /** 612 * @return {@link #detail} (A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.) 613 */ 614 public List<ItemDetailComponent> getDetail() { 615 if (this.detail == null) 616 this.detail = new ArrayList<ItemDetailComponent>(); 617 return this.detail; 618 } 619 620 /** 621 * @return Returns a reference to <code>this</code> for easy method chaining 622 */ 623 public ItemComponent setDetail(List<ItemDetailComponent> theDetail) { 624 this.detail = theDetail; 625 return this; 626 } 627 628 public boolean hasDetail() { 629 if (this.detail == null) 630 return false; 631 for (ItemDetailComponent item : this.detail) 632 if (!item.isEmpty()) 633 return true; 634 return false; 635 } 636 637 public ItemDetailComponent addDetail() { //3 638 ItemDetailComponent t = new ItemDetailComponent(); 639 if (this.detail == null) 640 this.detail = new ArrayList<ItemDetailComponent>(); 641 this.detail.add(t); 642 return t; 643 } 644 645 public ItemComponent addDetail(ItemDetailComponent t) { //3 646 if (t == null) 647 return this; 648 if (this.detail == null) 649 this.detail = new ArrayList<ItemDetailComponent>(); 650 this.detail.add(t); 651 return this; 652 } 653 654 /** 655 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 656 */ 657 public ItemDetailComponent getDetailFirstRep() { 658 if (getDetail().isEmpty()) { 659 addDetail(); 660 } 661 return getDetail().get(0); 662 } 663 664 protected void listChildren(List<Property> children) { 665 super.listChildren(children); 666 children.add(new Property("itemSequence", "positiveInt", "A number to uniquely reference the claim item entries.", 0, 1, itemSequence)); 667 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 668 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 669 children.add(new Property("reviewOutcome", "", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 670 children.add(new Property("adjudication", "", "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 671 children.add(new Property("detail", "", "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, detail)); 672 } 673 674 @Override 675 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 676 switch (_hash) { 677 case 1977979892: /*itemSequence*/ return new Property("itemSequence", "positiveInt", "A number to uniquely reference the claim item entries.", 0, 1, itemSequence); 678 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 679 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 680 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 681 case -231349275: /*adjudication*/ return new Property("adjudication", "", "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, adjudication); 682 case -1335224239: /*detail*/ return new Property("detail", "", "A claim detail. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, java.lang.Integer.MAX_VALUE, detail); 683 default: return super.getNamedProperty(_hash, _name, _checkValid); 684 } 685 686 } 687 688 @Override 689 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 690 switch (hash) { 691 case 1977979892: /*itemSequence*/ return this.itemSequence == null ? new Base[0] : new Base[] {this.itemSequence}; // PositiveIntType 692 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 693 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 694 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ReviewOutcomeComponent 695 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 696 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // ItemDetailComponent 697 default: return super.getProperty(hash, name, checkValid); 698 } 699 700 } 701 702 @Override 703 public Base setProperty(int hash, String name, Base value) throws FHIRException { 704 switch (hash) { 705 case 1977979892: // itemSequence 706 this.itemSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 707 return value; 708 case 82505966: // traceNumber 709 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 710 return value; 711 case -1110033957: // noteNumber 712 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 713 return value; 714 case -51825446: // reviewOutcome 715 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 716 return value; 717 case -231349275: // adjudication 718 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 719 return value; 720 case -1335224239: // detail 721 this.getDetail().add((ItemDetailComponent) value); // ItemDetailComponent 722 return value; 723 default: return super.setProperty(hash, name, value); 724 } 725 726 } 727 728 @Override 729 public Base setProperty(String name, Base value) throws FHIRException { 730 if (name.equals("itemSequence")) { 731 this.itemSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 732 } else if (name.equals("traceNumber")) { 733 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 734 } else if (name.equals("noteNumber")) { 735 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 736 } else if (name.equals("reviewOutcome")) { 737 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 738 } else if (name.equals("adjudication")) { 739 this.getAdjudication().add((AdjudicationComponent) value); 740 } else if (name.equals("detail")) { 741 this.getDetail().add((ItemDetailComponent) value); 742 } else 743 return super.setProperty(name, value); 744 return value; 745 } 746 747 @Override 748 public void removeChild(String name, Base value) throws FHIRException { 749 if (name.equals("itemSequence")) { 750 this.itemSequence = null; 751 } else if (name.equals("traceNumber")) { 752 this.getTraceNumber().remove(value); 753 } else if (name.equals("noteNumber")) { 754 this.getNoteNumber().remove(value); 755 } else if (name.equals("reviewOutcome")) { 756 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 757 } else if (name.equals("adjudication")) { 758 this.getAdjudication().remove((AdjudicationComponent) value); 759 } else if (name.equals("detail")) { 760 this.getDetail().remove((ItemDetailComponent) value); 761 } else 762 super.removeChild(name, value); 763 764 } 765 766 @Override 767 public Base makeProperty(int hash, String name) throws FHIRException { 768 switch (hash) { 769 case 1977979892: return getItemSequenceElement(); 770 case 82505966: return addTraceNumber(); 771 case -1110033957: return addNoteNumberElement(); 772 case -51825446: return getReviewOutcome(); 773 case -231349275: return addAdjudication(); 774 case -1335224239: return addDetail(); 775 default: return super.makeProperty(hash, name); 776 } 777 778 } 779 780 @Override 781 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 782 switch (hash) { 783 case 1977979892: /*itemSequence*/ return new String[] {"positiveInt"}; 784 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 785 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 786 case -51825446: /*reviewOutcome*/ return new String[] {}; 787 case -231349275: /*adjudication*/ return new String[] {}; 788 case -1335224239: /*detail*/ return new String[] {}; 789 default: return super.getTypesForProperty(hash, name); 790 } 791 792 } 793 794 @Override 795 public Base addChild(String name) throws FHIRException { 796 if (name.equals("itemSequence")) { 797 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.itemSequence"); 798 } 799 else if (name.equals("traceNumber")) { 800 return addTraceNumber(); 801 } 802 else if (name.equals("noteNumber")) { 803 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.noteNumber"); 804 } 805 else if (name.equals("reviewOutcome")) { 806 this.reviewOutcome = new ReviewOutcomeComponent(); 807 return this.reviewOutcome; 808 } 809 else if (name.equals("adjudication")) { 810 return addAdjudication(); 811 } 812 else if (name.equals("detail")) { 813 return addDetail(); 814 } 815 else 816 return super.addChild(name); 817 } 818 819 public ItemComponent copy() { 820 ItemComponent dst = new ItemComponent(); 821 copyValues(dst); 822 return dst; 823 } 824 825 public void copyValues(ItemComponent dst) { 826 super.copyValues(dst); 827 dst.itemSequence = itemSequence == null ? null : itemSequence.copy(); 828 if (traceNumber != null) { 829 dst.traceNumber = new ArrayList<Identifier>(); 830 for (Identifier i : traceNumber) 831 dst.traceNumber.add(i.copy()); 832 }; 833 if (noteNumber != null) { 834 dst.noteNumber = new ArrayList<PositiveIntType>(); 835 for (PositiveIntType i : noteNumber) 836 dst.noteNumber.add(i.copy()); 837 }; 838 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 839 if (adjudication != null) { 840 dst.adjudication = new ArrayList<AdjudicationComponent>(); 841 for (AdjudicationComponent i : adjudication) 842 dst.adjudication.add(i.copy()); 843 }; 844 if (detail != null) { 845 dst.detail = new ArrayList<ItemDetailComponent>(); 846 for (ItemDetailComponent i : detail) 847 dst.detail.add(i.copy()); 848 }; 849 } 850 851 @Override 852 public boolean equalsDeep(Base other_) { 853 if (!super.equalsDeep(other_)) 854 return false; 855 if (!(other_ instanceof ItemComponent)) 856 return false; 857 ItemComponent o = (ItemComponent) other_; 858 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 859 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 860 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 861 } 862 863 @Override 864 public boolean equalsShallow(Base other_) { 865 if (!super.equalsShallow(other_)) 866 return false; 867 if (!(other_ instanceof ItemComponent)) 868 return false; 869 ItemComponent o = (ItemComponent) other_; 870 return compareValues(itemSequence, o.itemSequence, true) && compareValues(noteNumber, o.noteNumber, true) 871 ; 872 } 873 874 public boolean isEmpty() { 875 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, traceNumber 876 , noteNumber, reviewOutcome, adjudication, detail); 877 } 878 879 public String fhirType() { 880 return "ClaimResponse.item"; 881 882 } 883 884 } 885 886 @Block() 887 public static class ReviewOutcomeComponent extends BackboneElement implements IBaseBackboneElement { 888 /** 889 * The result of the claim, predetermination, or preauthorization adjudication. 890 */ 891 @Child(name = "decision", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 892 @Description(shortDefinition="Result of the adjudication", formalDefinition="The result of the claim, predetermination, or preauthorization adjudication." ) 893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision") 894 protected CodeableConcept decision; 895 896 /** 897 * The reasons for the result of the claim, predetermination, or preauthorization adjudication. 898 */ 899 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 900 @Description(shortDefinition="Reason for result of the adjudication", formalDefinition="The reasons for the result of the claim, predetermination, or preauthorization adjudication." ) 901 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision-reason") 902 protected List<CodeableConcept> reason; 903 904 /** 905 * Reference from the Insurer which is used in later communications which refers to this adjudication. 906 */ 907 @Child(name = "preAuthRef", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 908 @Description(shortDefinition="Preauthorization reference", formalDefinition="Reference from the Insurer which is used in later communications which refers to this adjudication." ) 909 protected StringType preAuthRef; 910 911 /** 912 * The time frame during which this authorization is effective. 913 */ 914 @Child(name = "preAuthPeriod", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 915 @Description(shortDefinition="Preauthorization reference effective period", formalDefinition="The time frame during which this authorization is effective." ) 916 protected Period preAuthPeriod; 917 918 private static final long serialVersionUID = 2126097594L; 919 920 /** 921 * Constructor 922 */ 923 public ReviewOutcomeComponent() { 924 super(); 925 } 926 927 /** 928 * @return {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 929 */ 930 public CodeableConcept getDecision() { 931 if (this.decision == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create ReviewOutcomeComponent.decision"); 934 else if (Configuration.doAutoCreate()) 935 this.decision = new CodeableConcept(); // cc 936 return this.decision; 937 } 938 939 public boolean hasDecision() { 940 return this.decision != null && !this.decision.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 945 */ 946 public ReviewOutcomeComponent setDecision(CodeableConcept value) { 947 this.decision = value; 948 return this; 949 } 950 951 /** 952 * @return {@link #reason} (The reasons for the result of the claim, predetermination, or preauthorization adjudication.) 953 */ 954 public List<CodeableConcept> getReason() { 955 if (this.reason == null) 956 this.reason = new ArrayList<CodeableConcept>(); 957 return this.reason; 958 } 959 960 /** 961 * @return Returns a reference to <code>this</code> for easy method chaining 962 */ 963 public ReviewOutcomeComponent setReason(List<CodeableConcept> theReason) { 964 this.reason = theReason; 965 return this; 966 } 967 968 public boolean hasReason() { 969 if (this.reason == null) 970 return false; 971 for (CodeableConcept item : this.reason) 972 if (!item.isEmpty()) 973 return true; 974 return false; 975 } 976 977 public CodeableConcept addReason() { //3 978 CodeableConcept t = new CodeableConcept(); 979 if (this.reason == null) 980 this.reason = new ArrayList<CodeableConcept>(); 981 this.reason.add(t); 982 return t; 983 } 984 985 public ReviewOutcomeComponent addReason(CodeableConcept t) { //3 986 if (t == null) 987 return this; 988 if (this.reason == null) 989 this.reason = new ArrayList<CodeableConcept>(); 990 this.reason.add(t); 991 return this; 992 } 993 994 /** 995 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 996 */ 997 public CodeableConcept getReasonFirstRep() { 998 if (getReason().isEmpty()) { 999 addReason(); 1000 } 1001 return getReason().get(0); 1002 } 1003 1004 /** 1005 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 1006 */ 1007 public StringType getPreAuthRefElement() { 1008 if (this.preAuthRef == null) 1009 if (Configuration.errorOnAutoCreate()) 1010 throw new Error("Attempt to auto-create ReviewOutcomeComponent.preAuthRef"); 1011 else if (Configuration.doAutoCreate()) 1012 this.preAuthRef = new StringType(); // bb 1013 return this.preAuthRef; 1014 } 1015 1016 public boolean hasPreAuthRefElement() { 1017 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 1018 } 1019 1020 public boolean hasPreAuthRef() { 1021 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 1022 } 1023 1024 /** 1025 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 1026 */ 1027 public ReviewOutcomeComponent setPreAuthRefElement(StringType value) { 1028 this.preAuthRef = value; 1029 return this; 1030 } 1031 1032 /** 1033 * @return Reference from the Insurer which is used in later communications which refers to this adjudication. 1034 */ 1035 public String getPreAuthRef() { 1036 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 1037 } 1038 1039 /** 1040 * @param value Reference from the Insurer which is used in later communications which refers to this adjudication. 1041 */ 1042 public ReviewOutcomeComponent setPreAuthRef(String value) { 1043 if (Utilities.noString(value)) 1044 this.preAuthRef = null; 1045 else { 1046 if (this.preAuthRef == null) 1047 this.preAuthRef = new StringType(); 1048 this.preAuthRef.setValue(value); 1049 } 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 1055 */ 1056 public Period getPreAuthPeriod() { 1057 if (this.preAuthPeriod == null) 1058 if (Configuration.errorOnAutoCreate()) 1059 throw new Error("Attempt to auto-create ReviewOutcomeComponent.preAuthPeriod"); 1060 else if (Configuration.doAutoCreate()) 1061 this.preAuthPeriod = new Period(); // cc 1062 return this.preAuthPeriod; 1063 } 1064 1065 public boolean hasPreAuthPeriod() { 1066 return this.preAuthPeriod != null && !this.preAuthPeriod.isEmpty(); 1067 } 1068 1069 /** 1070 * @param value {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 1071 */ 1072 public ReviewOutcomeComponent setPreAuthPeriod(Period value) { 1073 this.preAuthPeriod = value; 1074 return this; 1075 } 1076 1077 protected void listChildren(List<Property> children) { 1078 super.listChildren(children); 1079 children.add(new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision)); 1080 children.add(new Property("reason", "CodeableConcept", "The reasons for the result of the claim, predetermination, or preauthorization adjudication.", 0, java.lang.Integer.MAX_VALUE, reason)); 1081 children.add(new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef)); 1082 children.add(new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod)); 1083 } 1084 1085 @Override 1086 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1087 switch (_hash) { 1088 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision); 1089 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "The reasons for the result of the claim, predetermination, or preauthorization adjudication.", 0, java.lang.Integer.MAX_VALUE, reason); 1090 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef); 1091 case 1819164812: /*preAuthPeriod*/ return new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod); 1092 default: return super.getNamedProperty(_hash, _name, _checkValid); 1093 } 1094 1095 } 1096 1097 @Override 1098 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1099 switch (hash) { 1100 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 1101 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 1102 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : new Base[] {this.preAuthRef}; // StringType 1103 case 1819164812: /*preAuthPeriod*/ return this.preAuthPeriod == null ? new Base[0] : new Base[] {this.preAuthPeriod}; // Period 1104 default: return super.getProperty(hash, name, checkValid); 1105 } 1106 1107 } 1108 1109 @Override 1110 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1111 switch (hash) { 1112 case 565719004: // decision 1113 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1114 return value; 1115 case -934964668: // reason 1116 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1117 return value; 1118 case 522246568: // preAuthRef 1119 this.preAuthRef = TypeConvertor.castToString(value); // StringType 1120 return value; 1121 case 1819164812: // preAuthPeriod 1122 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 1123 return value; 1124 default: return super.setProperty(hash, name, value); 1125 } 1126 1127 } 1128 1129 @Override 1130 public Base setProperty(String name, Base value) throws FHIRException { 1131 if (name.equals("decision")) { 1132 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1133 } else if (name.equals("reason")) { 1134 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 1135 } else if (name.equals("preAuthRef")) { 1136 this.preAuthRef = TypeConvertor.castToString(value); // StringType 1137 } else if (name.equals("preAuthPeriod")) { 1138 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 1139 } else 1140 return super.setProperty(name, value); 1141 return value; 1142 } 1143 1144 @Override 1145 public void removeChild(String name, Base value) throws FHIRException { 1146 if (name.equals("decision")) { 1147 this.decision = null; 1148 } else if (name.equals("reason")) { 1149 this.getReason().remove(value); 1150 } else if (name.equals("preAuthRef")) { 1151 this.preAuthRef = null; 1152 } else if (name.equals("preAuthPeriod")) { 1153 this.preAuthPeriod = null; 1154 } else 1155 super.removeChild(name, value); 1156 1157 } 1158 1159 @Override 1160 public Base makeProperty(int hash, String name) throws FHIRException { 1161 switch (hash) { 1162 case 565719004: return getDecision(); 1163 case -934964668: return addReason(); 1164 case 522246568: return getPreAuthRefElement(); 1165 case 1819164812: return getPreAuthPeriod(); 1166 default: return super.makeProperty(hash, name); 1167 } 1168 1169 } 1170 1171 @Override 1172 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1173 switch (hash) { 1174 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 1175 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1176 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 1177 case 1819164812: /*preAuthPeriod*/ return new String[] {"Period"}; 1178 default: return super.getTypesForProperty(hash, name); 1179 } 1180 1181 } 1182 1183 @Override 1184 public Base addChild(String name) throws FHIRException { 1185 if (name.equals("decision")) { 1186 this.decision = new CodeableConcept(); 1187 return this.decision; 1188 } 1189 else if (name.equals("reason")) { 1190 return addReason(); 1191 } 1192 else if (name.equals("preAuthRef")) { 1193 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.reviewOutcome.preAuthRef"); 1194 } 1195 else if (name.equals("preAuthPeriod")) { 1196 this.preAuthPeriod = new Period(); 1197 return this.preAuthPeriod; 1198 } 1199 else 1200 return super.addChild(name); 1201 } 1202 1203 public ReviewOutcomeComponent copy() { 1204 ReviewOutcomeComponent dst = new ReviewOutcomeComponent(); 1205 copyValues(dst); 1206 return dst; 1207 } 1208 1209 public void copyValues(ReviewOutcomeComponent dst) { 1210 super.copyValues(dst); 1211 dst.decision = decision == null ? null : decision.copy(); 1212 if (reason != null) { 1213 dst.reason = new ArrayList<CodeableConcept>(); 1214 for (CodeableConcept i : reason) 1215 dst.reason.add(i.copy()); 1216 }; 1217 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 1218 dst.preAuthPeriod = preAuthPeriod == null ? null : preAuthPeriod.copy(); 1219 } 1220 1221 @Override 1222 public boolean equalsDeep(Base other_) { 1223 if (!super.equalsDeep(other_)) 1224 return false; 1225 if (!(other_ instanceof ReviewOutcomeComponent)) 1226 return false; 1227 ReviewOutcomeComponent o = (ReviewOutcomeComponent) other_; 1228 return compareDeep(decision, o.decision, true) && compareDeep(reason, o.reason, true) && compareDeep(preAuthRef, o.preAuthRef, true) 1229 && compareDeep(preAuthPeriod, o.preAuthPeriod, true); 1230 } 1231 1232 @Override 1233 public boolean equalsShallow(Base other_) { 1234 if (!super.equalsShallow(other_)) 1235 return false; 1236 if (!(other_ instanceof ReviewOutcomeComponent)) 1237 return false; 1238 ReviewOutcomeComponent o = (ReviewOutcomeComponent) other_; 1239 return compareValues(preAuthRef, o.preAuthRef, true); 1240 } 1241 1242 public boolean isEmpty() { 1243 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(decision, reason, preAuthRef 1244 , preAuthPeriod); 1245 } 1246 1247 public String fhirType() { 1248 return "ClaimResponse.item.reviewOutcome"; 1249 1250 } 1251 1252 } 1253 1254 @Block() 1255 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 1256 /** 1257 * A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item. 1258 */ 1259 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1260 @Description(shortDefinition="Type of adjudication information", formalDefinition="A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item." ) 1261 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 1262 protected CodeableConcept category; 1263 1264 /** 1265 * A code supporting the understanding of the adjudication result and explaining variance from expected amount. 1266 */ 1267 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1268 @Description(shortDefinition="Explanation of adjudication outcome", formalDefinition="A code supporting the understanding of the adjudication result and explaining variance from expected amount." ) 1269 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-reason") 1270 protected CodeableConcept reason; 1271 1272 /** 1273 * Monetary amount associated with the category. 1274 */ 1275 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 1276 @Description(shortDefinition="Monetary amount", formalDefinition="Monetary amount associated with the category." ) 1277 protected Money amount; 1278 1279 /** 1280 * A non-monetary value associated with the category. Mutually exclusive to the amount element above. 1281 */ 1282 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 1283 @Description(shortDefinition="Non-monetary value", formalDefinition="A non-monetary value associated with the category. Mutually exclusive to the amount element above." ) 1284 protected Quantity quantity; 1285 1286 private static final long serialVersionUID = 29312734L; 1287 1288 /** 1289 * Constructor 1290 */ 1291 public AdjudicationComponent() { 1292 super(); 1293 } 1294 1295 /** 1296 * Constructor 1297 */ 1298 public AdjudicationComponent(CodeableConcept category) { 1299 super(); 1300 this.setCategory(category); 1301 } 1302 1303 /** 1304 * @return {@link #category} (A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.) 1305 */ 1306 public CodeableConcept getCategory() { 1307 if (this.category == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 1310 else if (Configuration.doAutoCreate()) 1311 this.category = new CodeableConcept(); // cc 1312 return this.category; 1313 } 1314 1315 public boolean hasCategory() { 1316 return this.category != null && !this.category.isEmpty(); 1317 } 1318 1319 /** 1320 * @param value {@link #category} (A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.) 1321 */ 1322 public AdjudicationComponent setCategory(CodeableConcept value) { 1323 this.category = value; 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #reason} (A code supporting the understanding of the adjudication result and explaining variance from expected amount.) 1329 */ 1330 public CodeableConcept getReason() { 1331 if (this.reason == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 1334 else if (Configuration.doAutoCreate()) 1335 this.reason = new CodeableConcept(); // cc 1336 return this.reason; 1337 } 1338 1339 public boolean hasReason() { 1340 return this.reason != null && !this.reason.isEmpty(); 1341 } 1342 1343 /** 1344 * @param value {@link #reason} (A code supporting the understanding of the adjudication result and explaining variance from expected amount.) 1345 */ 1346 public AdjudicationComponent setReason(CodeableConcept value) { 1347 this.reason = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return {@link #amount} (Monetary amount associated with the category.) 1353 */ 1354 public Money getAmount() { 1355 if (this.amount == null) 1356 if (Configuration.errorOnAutoCreate()) 1357 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 1358 else if (Configuration.doAutoCreate()) 1359 this.amount = new Money(); // cc 1360 return this.amount; 1361 } 1362 1363 public boolean hasAmount() { 1364 return this.amount != null && !this.amount.isEmpty(); 1365 } 1366 1367 /** 1368 * @param value {@link #amount} (Monetary amount associated with the category.) 1369 */ 1370 public AdjudicationComponent setAmount(Money value) { 1371 this.amount = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #quantity} (A non-monetary value associated with the category. Mutually exclusive to the amount element above.) 1377 */ 1378 public Quantity getQuantity() { 1379 if (this.quantity == null) 1380 if (Configuration.errorOnAutoCreate()) 1381 throw new Error("Attempt to auto-create AdjudicationComponent.quantity"); 1382 else if (Configuration.doAutoCreate()) 1383 this.quantity = new Quantity(); // cc 1384 return this.quantity; 1385 } 1386 1387 public boolean hasQuantity() { 1388 return this.quantity != null && !this.quantity.isEmpty(); 1389 } 1390 1391 /** 1392 * @param value {@link #quantity} (A non-monetary value associated with the category. Mutually exclusive to the amount element above.) 1393 */ 1394 public AdjudicationComponent setQuantity(Quantity value) { 1395 this.quantity = value; 1396 return this; 1397 } 1398 1399 protected void listChildren(List<Property> children) { 1400 super.listChildren(children); 1401 children.add(new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.", 0, 1, category)); 1402 children.add(new Property("reason", "CodeableConcept", "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 0, 1, reason)); 1403 children.add(new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount)); 1404 children.add(new Property("quantity", "Quantity", "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, quantity)); 1405 } 1406 1407 @Override 1408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1409 switch (_hash) { 1410 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include the value submitted, maximum values or percentages allowed or payable under the plan, amounts that: the patient is responsible for in aggregate or pertaining to this item; amounts paid by other coverages; and, the benefit payable for this item.", 0, 1, category); 1411 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 0, 1, reason); 1412 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount); 1413 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, quantity); 1414 default: return super.getNamedProperty(_hash, _name, _checkValid); 1415 } 1416 1417 } 1418 1419 @Override 1420 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1421 switch (hash) { 1422 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1423 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1424 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 1425 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1426 default: return super.getProperty(hash, name, checkValid); 1427 } 1428 1429 } 1430 1431 @Override 1432 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1433 switch (hash) { 1434 case 50511102: // category 1435 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1436 return value; 1437 case -934964668: // reason 1438 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1439 return value; 1440 case -1413853096: // amount 1441 this.amount = TypeConvertor.castToMoney(value); // Money 1442 return value; 1443 case -1285004149: // quantity 1444 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1445 return value; 1446 default: return super.setProperty(hash, name, value); 1447 } 1448 1449 } 1450 1451 @Override 1452 public Base setProperty(String name, Base value) throws FHIRException { 1453 if (name.equals("category")) { 1454 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1455 } else if (name.equals("reason")) { 1456 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1457 } else if (name.equals("amount")) { 1458 this.amount = TypeConvertor.castToMoney(value); // Money 1459 } else if (name.equals("quantity")) { 1460 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1461 } else 1462 return super.setProperty(name, value); 1463 return value; 1464 } 1465 1466 @Override 1467 public void removeChild(String name, Base value) throws FHIRException { 1468 if (name.equals("category")) { 1469 this.category = null; 1470 } else if (name.equals("reason")) { 1471 this.reason = null; 1472 } else if (name.equals("amount")) { 1473 this.amount = null; 1474 } else if (name.equals("quantity")) { 1475 this.quantity = null; 1476 } else 1477 super.removeChild(name, value); 1478 1479 } 1480 1481 @Override 1482 public Base makeProperty(int hash, String name) throws FHIRException { 1483 switch (hash) { 1484 case 50511102: return getCategory(); 1485 case -934964668: return getReason(); 1486 case -1413853096: return getAmount(); 1487 case -1285004149: return getQuantity(); 1488 default: return super.makeProperty(hash, name); 1489 } 1490 1491 } 1492 1493 @Override 1494 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1495 switch (hash) { 1496 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1497 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1498 case -1413853096: /*amount*/ return new String[] {"Money"}; 1499 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1500 default: return super.getTypesForProperty(hash, name); 1501 } 1502 1503 } 1504 1505 @Override 1506 public Base addChild(String name) throws FHIRException { 1507 if (name.equals("category")) { 1508 this.category = new CodeableConcept(); 1509 return this.category; 1510 } 1511 else if (name.equals("reason")) { 1512 this.reason = new CodeableConcept(); 1513 return this.reason; 1514 } 1515 else if (name.equals("amount")) { 1516 this.amount = new Money(); 1517 return this.amount; 1518 } 1519 else if (name.equals("quantity")) { 1520 this.quantity = new Quantity(); 1521 return this.quantity; 1522 } 1523 else 1524 return super.addChild(name); 1525 } 1526 1527 public AdjudicationComponent copy() { 1528 AdjudicationComponent dst = new AdjudicationComponent(); 1529 copyValues(dst); 1530 return dst; 1531 } 1532 1533 public void copyValues(AdjudicationComponent dst) { 1534 super.copyValues(dst); 1535 dst.category = category == null ? null : category.copy(); 1536 dst.reason = reason == null ? null : reason.copy(); 1537 dst.amount = amount == null ? null : amount.copy(); 1538 dst.quantity = quantity == null ? null : quantity.copy(); 1539 } 1540 1541 @Override 1542 public boolean equalsDeep(Base other_) { 1543 if (!super.equalsDeep(other_)) 1544 return false; 1545 if (!(other_ instanceof AdjudicationComponent)) 1546 return false; 1547 AdjudicationComponent o = (AdjudicationComponent) other_; 1548 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) && compareDeep(amount, o.amount, true) 1549 && compareDeep(quantity, o.quantity, true); 1550 } 1551 1552 @Override 1553 public boolean equalsShallow(Base other_) { 1554 if (!super.equalsShallow(other_)) 1555 return false; 1556 if (!(other_ instanceof AdjudicationComponent)) 1557 return false; 1558 AdjudicationComponent o = (AdjudicationComponent) other_; 1559 return true; 1560 } 1561 1562 public boolean isEmpty() { 1563 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount 1564 , quantity); 1565 } 1566 1567 public String fhirType() { 1568 return "ClaimResponse.item.adjudication"; 1569 1570 } 1571 1572 } 1573 1574 @Block() 1575 public static class ItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 1576 /** 1577 * A number to uniquely reference the claim detail entry. 1578 */ 1579 @Child(name = "detailSequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1580 @Description(shortDefinition="Claim detail instance identifier", formalDefinition="A number to uniquely reference the claim detail entry." ) 1581 protected PositiveIntType detailSequence; 1582 1583 /** 1584 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 1585 */ 1586 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1587 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 1588 protected List<Identifier> traceNumber; 1589 1590 /** 1591 * The numbers associated with notes below which apply to the adjudication of this item. 1592 */ 1593 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1594 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 1595 protected List<PositiveIntType> noteNumber; 1596 1597 /** 1598 * The high-level results of the adjudication if adjudication has been performed. 1599 */ 1600 @Child(name = "reviewOutcome", type = {ReviewOutcomeComponent.class}, order=4, min=0, max=1, modifier=false, summary=false) 1601 @Description(shortDefinition="Detail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 1602 protected ReviewOutcomeComponent reviewOutcome; 1603 1604 /** 1605 * The adjudication results. 1606 */ 1607 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1608 @Description(shortDefinition="Detail level adjudication details", formalDefinition="The adjudication results." ) 1609 protected List<AdjudicationComponent> adjudication; 1610 1611 /** 1612 * A sub-detail adjudication of a simple product or service. 1613 */ 1614 @Child(name = "subDetail", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1615 @Description(shortDefinition="Adjudication for claim sub-details", formalDefinition="A sub-detail adjudication of a simple product or service." ) 1616 protected List<SubDetailComponent> subDetail; 1617 1618 private static final long serialVersionUID = -1263137525L; 1619 1620 /** 1621 * Constructor 1622 */ 1623 public ItemDetailComponent() { 1624 super(); 1625 } 1626 1627 /** 1628 * Constructor 1629 */ 1630 public ItemDetailComponent(int detailSequence) { 1631 super(); 1632 this.setDetailSequence(detailSequence); 1633 } 1634 1635 /** 1636 * @return {@link #detailSequence} (A number to uniquely reference the claim detail entry.). This is the underlying object with id, value and extensions. The accessor "getDetailSequence" gives direct access to the value 1637 */ 1638 public PositiveIntType getDetailSequenceElement() { 1639 if (this.detailSequence == null) 1640 if (Configuration.errorOnAutoCreate()) 1641 throw new Error("Attempt to auto-create ItemDetailComponent.detailSequence"); 1642 else if (Configuration.doAutoCreate()) 1643 this.detailSequence = new PositiveIntType(); // bb 1644 return this.detailSequence; 1645 } 1646 1647 public boolean hasDetailSequenceElement() { 1648 return this.detailSequence != null && !this.detailSequence.isEmpty(); 1649 } 1650 1651 public boolean hasDetailSequence() { 1652 return this.detailSequence != null && !this.detailSequence.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #detailSequence} (A number to uniquely reference the claim detail entry.). This is the underlying object with id, value and extensions. The accessor "getDetailSequence" gives direct access to the value 1657 */ 1658 public ItemDetailComponent setDetailSequenceElement(PositiveIntType value) { 1659 this.detailSequence = value; 1660 return this; 1661 } 1662 1663 /** 1664 * @return A number to uniquely reference the claim detail entry. 1665 */ 1666 public int getDetailSequence() { 1667 return this.detailSequence == null || this.detailSequence.isEmpty() ? 0 : this.detailSequence.getValue(); 1668 } 1669 1670 /** 1671 * @param value A number to uniquely reference the claim detail entry. 1672 */ 1673 public ItemDetailComponent setDetailSequence(int value) { 1674 if (this.detailSequence == null) 1675 this.detailSequence = new PositiveIntType(); 1676 this.detailSequence.setValue(value); 1677 return this; 1678 } 1679 1680 /** 1681 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 1682 */ 1683 public List<Identifier> getTraceNumber() { 1684 if (this.traceNumber == null) 1685 this.traceNumber = new ArrayList<Identifier>(); 1686 return this.traceNumber; 1687 } 1688 1689 /** 1690 * @return Returns a reference to <code>this</code> for easy method chaining 1691 */ 1692 public ItemDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 1693 this.traceNumber = theTraceNumber; 1694 return this; 1695 } 1696 1697 public boolean hasTraceNumber() { 1698 if (this.traceNumber == null) 1699 return false; 1700 for (Identifier item : this.traceNumber) 1701 if (!item.isEmpty()) 1702 return true; 1703 return false; 1704 } 1705 1706 public Identifier addTraceNumber() { //3 1707 Identifier t = new Identifier(); 1708 if (this.traceNumber == null) 1709 this.traceNumber = new ArrayList<Identifier>(); 1710 this.traceNumber.add(t); 1711 return t; 1712 } 1713 1714 public ItemDetailComponent addTraceNumber(Identifier t) { //3 1715 if (t == null) 1716 return this; 1717 if (this.traceNumber == null) 1718 this.traceNumber = new ArrayList<Identifier>(); 1719 this.traceNumber.add(t); 1720 return this; 1721 } 1722 1723 /** 1724 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 1725 */ 1726 public Identifier getTraceNumberFirstRep() { 1727 if (getTraceNumber().isEmpty()) { 1728 addTraceNumber(); 1729 } 1730 return getTraceNumber().get(0); 1731 } 1732 1733 /** 1734 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 1735 */ 1736 public List<PositiveIntType> getNoteNumber() { 1737 if (this.noteNumber == null) 1738 this.noteNumber = new ArrayList<PositiveIntType>(); 1739 return this.noteNumber; 1740 } 1741 1742 /** 1743 * @return Returns a reference to <code>this</code> for easy method chaining 1744 */ 1745 public ItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 1746 this.noteNumber = theNoteNumber; 1747 return this; 1748 } 1749 1750 public boolean hasNoteNumber() { 1751 if (this.noteNumber == null) 1752 return false; 1753 for (PositiveIntType item : this.noteNumber) 1754 if (!item.isEmpty()) 1755 return true; 1756 return false; 1757 } 1758 1759 /** 1760 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 1761 */ 1762 public PositiveIntType addNoteNumberElement() {//2 1763 PositiveIntType t = new PositiveIntType(); 1764 if (this.noteNumber == null) 1765 this.noteNumber = new ArrayList<PositiveIntType>(); 1766 this.noteNumber.add(t); 1767 return t; 1768 } 1769 1770 /** 1771 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 1772 */ 1773 public ItemDetailComponent addNoteNumber(int value) { //1 1774 PositiveIntType t = new PositiveIntType(); 1775 t.setValue(value); 1776 if (this.noteNumber == null) 1777 this.noteNumber = new ArrayList<PositiveIntType>(); 1778 this.noteNumber.add(t); 1779 return this; 1780 } 1781 1782 /** 1783 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 1784 */ 1785 public boolean hasNoteNumber(int value) { 1786 if (this.noteNumber == null) 1787 return false; 1788 for (PositiveIntType v : this.noteNumber) 1789 if (v.getValue().equals(value)) // positiveInt 1790 return true; 1791 return false; 1792 } 1793 1794 /** 1795 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 1796 */ 1797 public ReviewOutcomeComponent getReviewOutcome() { 1798 if (this.reviewOutcome == null) 1799 if (Configuration.errorOnAutoCreate()) 1800 throw new Error("Attempt to auto-create ItemDetailComponent.reviewOutcome"); 1801 else if (Configuration.doAutoCreate()) 1802 this.reviewOutcome = new ReviewOutcomeComponent(); // cc 1803 return this.reviewOutcome; 1804 } 1805 1806 public boolean hasReviewOutcome() { 1807 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 1808 } 1809 1810 /** 1811 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 1812 */ 1813 public ItemDetailComponent setReviewOutcome(ReviewOutcomeComponent value) { 1814 this.reviewOutcome = value; 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #adjudication} (The adjudication results.) 1820 */ 1821 public List<AdjudicationComponent> getAdjudication() { 1822 if (this.adjudication == null) 1823 this.adjudication = new ArrayList<AdjudicationComponent>(); 1824 return this.adjudication; 1825 } 1826 1827 /** 1828 * @return Returns a reference to <code>this</code> for easy method chaining 1829 */ 1830 public ItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 1831 this.adjudication = theAdjudication; 1832 return this; 1833 } 1834 1835 public boolean hasAdjudication() { 1836 if (this.adjudication == null) 1837 return false; 1838 for (AdjudicationComponent item : this.adjudication) 1839 if (!item.isEmpty()) 1840 return true; 1841 return false; 1842 } 1843 1844 public AdjudicationComponent addAdjudication() { //3 1845 AdjudicationComponent t = new AdjudicationComponent(); 1846 if (this.adjudication == null) 1847 this.adjudication = new ArrayList<AdjudicationComponent>(); 1848 this.adjudication.add(t); 1849 return t; 1850 } 1851 1852 public ItemDetailComponent addAdjudication(AdjudicationComponent t) { //3 1853 if (t == null) 1854 return this; 1855 if (this.adjudication == null) 1856 this.adjudication = new ArrayList<AdjudicationComponent>(); 1857 this.adjudication.add(t); 1858 return this; 1859 } 1860 1861 /** 1862 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 1863 */ 1864 public AdjudicationComponent getAdjudicationFirstRep() { 1865 if (getAdjudication().isEmpty()) { 1866 addAdjudication(); 1867 } 1868 return getAdjudication().get(0); 1869 } 1870 1871 /** 1872 * @return {@link #subDetail} (A sub-detail adjudication of a simple product or service.) 1873 */ 1874 public List<SubDetailComponent> getSubDetail() { 1875 if (this.subDetail == null) 1876 this.subDetail = new ArrayList<SubDetailComponent>(); 1877 return this.subDetail; 1878 } 1879 1880 /** 1881 * @return Returns a reference to <code>this</code> for easy method chaining 1882 */ 1883 public ItemDetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 1884 this.subDetail = theSubDetail; 1885 return this; 1886 } 1887 1888 public boolean hasSubDetail() { 1889 if (this.subDetail == null) 1890 return false; 1891 for (SubDetailComponent item : this.subDetail) 1892 if (!item.isEmpty()) 1893 return true; 1894 return false; 1895 } 1896 1897 public SubDetailComponent addSubDetail() { //3 1898 SubDetailComponent t = new SubDetailComponent(); 1899 if (this.subDetail == null) 1900 this.subDetail = new ArrayList<SubDetailComponent>(); 1901 this.subDetail.add(t); 1902 return t; 1903 } 1904 1905 public ItemDetailComponent addSubDetail(SubDetailComponent t) { //3 1906 if (t == null) 1907 return this; 1908 if (this.subDetail == null) 1909 this.subDetail = new ArrayList<SubDetailComponent>(); 1910 this.subDetail.add(t); 1911 return this; 1912 } 1913 1914 /** 1915 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 1916 */ 1917 public SubDetailComponent getSubDetailFirstRep() { 1918 if (getSubDetail().isEmpty()) { 1919 addSubDetail(); 1920 } 1921 return getSubDetail().get(0); 1922 } 1923 1924 protected void listChildren(List<Property> children) { 1925 super.listChildren(children); 1926 children.add(new Property("detailSequence", "positiveInt", "A number to uniquely reference the claim detail entry.", 0, 1, detailSequence)); 1927 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 1928 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 1929 children.add(new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 1930 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 1931 children.add(new Property("subDetail", "", "A sub-detail adjudication of a simple product or service.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 1932 } 1933 1934 @Override 1935 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1936 switch (_hash) { 1937 case 1321472818: /*detailSequence*/ return new Property("detailSequence", "positiveInt", "A number to uniquely reference the claim detail entry.", 0, 1, detailSequence); 1938 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 1939 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 1940 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 1941 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 1942 case -828829007: /*subDetail*/ return new Property("subDetail", "", "A sub-detail adjudication of a simple product or service.", 0, java.lang.Integer.MAX_VALUE, subDetail); 1943 default: return super.getNamedProperty(_hash, _name, _checkValid); 1944 } 1945 1946 } 1947 1948 @Override 1949 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1950 switch (hash) { 1951 case 1321472818: /*detailSequence*/ return this.detailSequence == null ? new Base[0] : new Base[] {this.detailSequence}; // PositiveIntType 1952 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 1953 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 1954 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ReviewOutcomeComponent 1955 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 1956 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 1957 default: return super.getProperty(hash, name, checkValid); 1958 } 1959 1960 } 1961 1962 @Override 1963 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1964 switch (hash) { 1965 case 1321472818: // detailSequence 1966 this.detailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1967 return value; 1968 case 82505966: // traceNumber 1969 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 1970 return value; 1971 case -1110033957: // noteNumber 1972 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 1973 return value; 1974 case -51825446: // reviewOutcome 1975 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 1976 return value; 1977 case -231349275: // adjudication 1978 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 1979 return value; 1980 case -828829007: // subDetail 1981 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 1982 return value; 1983 default: return super.setProperty(hash, name, value); 1984 } 1985 1986 } 1987 1988 @Override 1989 public Base setProperty(String name, Base value) throws FHIRException { 1990 if (name.equals("detailSequence")) { 1991 this.detailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1992 } else if (name.equals("traceNumber")) { 1993 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 1994 } else if (name.equals("noteNumber")) { 1995 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 1996 } else if (name.equals("reviewOutcome")) { 1997 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 1998 } else if (name.equals("adjudication")) { 1999 this.getAdjudication().add((AdjudicationComponent) value); 2000 } else if (name.equals("subDetail")) { 2001 this.getSubDetail().add((SubDetailComponent) value); 2002 } else 2003 return super.setProperty(name, value); 2004 return value; 2005 } 2006 2007 @Override 2008 public void removeChild(String name, Base value) throws FHIRException { 2009 if (name.equals("detailSequence")) { 2010 this.detailSequence = null; 2011 } else if (name.equals("traceNumber")) { 2012 this.getTraceNumber().remove(value); 2013 } else if (name.equals("noteNumber")) { 2014 this.getNoteNumber().remove(value); 2015 } else if (name.equals("reviewOutcome")) { 2016 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 2017 } else if (name.equals("adjudication")) { 2018 this.getAdjudication().remove((AdjudicationComponent) value); 2019 } else if (name.equals("subDetail")) { 2020 this.getSubDetail().remove((SubDetailComponent) value); 2021 } else 2022 super.removeChild(name, value); 2023 2024 } 2025 2026 @Override 2027 public Base makeProperty(int hash, String name) throws FHIRException { 2028 switch (hash) { 2029 case 1321472818: return getDetailSequenceElement(); 2030 case 82505966: return addTraceNumber(); 2031 case -1110033957: return addNoteNumberElement(); 2032 case -51825446: return getReviewOutcome(); 2033 case -231349275: return addAdjudication(); 2034 case -828829007: return addSubDetail(); 2035 default: return super.makeProperty(hash, name); 2036 } 2037 2038 } 2039 2040 @Override 2041 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2042 switch (hash) { 2043 case 1321472818: /*detailSequence*/ return new String[] {"positiveInt"}; 2044 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 2045 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 2046 case -51825446: /*reviewOutcome*/ return new String[] {"@ClaimResponse.item.reviewOutcome"}; 2047 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 2048 case -828829007: /*subDetail*/ return new String[] {}; 2049 default: return super.getTypesForProperty(hash, name); 2050 } 2051 2052 } 2053 2054 @Override 2055 public Base addChild(String name) throws FHIRException { 2056 if (name.equals("detailSequence")) { 2057 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.detail.detailSequence"); 2058 } 2059 else if (name.equals("traceNumber")) { 2060 return addTraceNumber(); 2061 } 2062 else if (name.equals("noteNumber")) { 2063 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.detail.noteNumber"); 2064 } 2065 else if (name.equals("reviewOutcome")) { 2066 this.reviewOutcome = new ReviewOutcomeComponent(); 2067 return this.reviewOutcome; 2068 } 2069 else if (name.equals("adjudication")) { 2070 return addAdjudication(); 2071 } 2072 else if (name.equals("subDetail")) { 2073 return addSubDetail(); 2074 } 2075 else 2076 return super.addChild(name); 2077 } 2078 2079 public ItemDetailComponent copy() { 2080 ItemDetailComponent dst = new ItemDetailComponent(); 2081 copyValues(dst); 2082 return dst; 2083 } 2084 2085 public void copyValues(ItemDetailComponent dst) { 2086 super.copyValues(dst); 2087 dst.detailSequence = detailSequence == null ? null : detailSequence.copy(); 2088 if (traceNumber != null) { 2089 dst.traceNumber = new ArrayList<Identifier>(); 2090 for (Identifier i : traceNumber) 2091 dst.traceNumber.add(i.copy()); 2092 }; 2093 if (noteNumber != null) { 2094 dst.noteNumber = new ArrayList<PositiveIntType>(); 2095 for (PositiveIntType i : noteNumber) 2096 dst.noteNumber.add(i.copy()); 2097 }; 2098 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 2099 if (adjudication != null) { 2100 dst.adjudication = new ArrayList<AdjudicationComponent>(); 2101 for (AdjudicationComponent i : adjudication) 2102 dst.adjudication.add(i.copy()); 2103 }; 2104 if (subDetail != null) { 2105 dst.subDetail = new ArrayList<SubDetailComponent>(); 2106 for (SubDetailComponent i : subDetail) 2107 dst.subDetail.add(i.copy()); 2108 }; 2109 } 2110 2111 @Override 2112 public boolean equalsDeep(Base other_) { 2113 if (!super.equalsDeep(other_)) 2114 return false; 2115 if (!(other_ instanceof ItemDetailComponent)) 2116 return false; 2117 ItemDetailComponent o = (ItemDetailComponent) other_; 2118 return compareDeep(detailSequence, o.detailSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 2119 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 2120 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true) 2121 ; 2122 } 2123 2124 @Override 2125 public boolean equalsShallow(Base other_) { 2126 if (!super.equalsShallow(other_)) 2127 return false; 2128 if (!(other_ instanceof ItemDetailComponent)) 2129 return false; 2130 ItemDetailComponent o = (ItemDetailComponent) other_; 2131 return compareValues(detailSequence, o.detailSequence, true) && compareValues(noteNumber, o.noteNumber, true) 2132 ; 2133 } 2134 2135 public boolean isEmpty() { 2136 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(detailSequence, traceNumber 2137 , noteNumber, reviewOutcome, adjudication, subDetail); 2138 } 2139 2140 public String fhirType() { 2141 return "ClaimResponse.item.detail"; 2142 2143 } 2144 2145 } 2146 2147 @Block() 2148 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 2149 /** 2150 * A number to uniquely reference the claim sub-detail entry. 2151 */ 2152 @Child(name = "subDetailSequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2153 @Description(shortDefinition="Claim sub-detail instance identifier", formalDefinition="A number to uniquely reference the claim sub-detail entry." ) 2154 protected PositiveIntType subDetailSequence; 2155 2156 /** 2157 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 2158 */ 2159 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2160 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 2161 protected List<Identifier> traceNumber; 2162 2163 /** 2164 * The numbers associated with notes below which apply to the adjudication of this item. 2165 */ 2166 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2167 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 2168 protected List<PositiveIntType> noteNumber; 2169 2170 /** 2171 * The high-level results of the adjudication if adjudication has been performed. 2172 */ 2173 @Child(name = "reviewOutcome", type = {ReviewOutcomeComponent.class}, order=4, min=0, max=1, modifier=false, summary=false) 2174 @Description(shortDefinition="Subdetail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 2175 protected ReviewOutcomeComponent reviewOutcome; 2176 2177 /** 2178 * The adjudication results. 2179 */ 2180 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2181 @Description(shortDefinition="Subdetail level adjudication details", formalDefinition="The adjudication results." ) 2182 protected List<AdjudicationComponent> adjudication; 2183 2184 private static final long serialVersionUID = 1370798714L; 2185 2186 /** 2187 * Constructor 2188 */ 2189 public SubDetailComponent() { 2190 super(); 2191 } 2192 2193 /** 2194 * Constructor 2195 */ 2196 public SubDetailComponent(int subDetailSequence) { 2197 super(); 2198 this.setSubDetailSequence(subDetailSequence); 2199 } 2200 2201 /** 2202 * @return {@link #subDetailSequence} (A number to uniquely reference the claim sub-detail entry.). This is the underlying object with id, value and extensions. The accessor "getSubDetailSequence" gives direct access to the value 2203 */ 2204 public PositiveIntType getSubDetailSequenceElement() { 2205 if (this.subDetailSequence == null) 2206 if (Configuration.errorOnAutoCreate()) 2207 throw new Error("Attempt to auto-create SubDetailComponent.subDetailSequence"); 2208 else if (Configuration.doAutoCreate()) 2209 this.subDetailSequence = new PositiveIntType(); // bb 2210 return this.subDetailSequence; 2211 } 2212 2213 public boolean hasSubDetailSequenceElement() { 2214 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 2215 } 2216 2217 public boolean hasSubDetailSequence() { 2218 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 2219 } 2220 2221 /** 2222 * @param value {@link #subDetailSequence} (A number to uniquely reference the claim sub-detail entry.). This is the underlying object with id, value and extensions. The accessor "getSubDetailSequence" gives direct access to the value 2223 */ 2224 public SubDetailComponent setSubDetailSequenceElement(PositiveIntType value) { 2225 this.subDetailSequence = value; 2226 return this; 2227 } 2228 2229 /** 2230 * @return A number to uniquely reference the claim sub-detail entry. 2231 */ 2232 public int getSubDetailSequence() { 2233 return this.subDetailSequence == null || this.subDetailSequence.isEmpty() ? 0 : this.subDetailSequence.getValue(); 2234 } 2235 2236 /** 2237 * @param value A number to uniquely reference the claim sub-detail entry. 2238 */ 2239 public SubDetailComponent setSubDetailSequence(int value) { 2240 if (this.subDetailSequence == null) 2241 this.subDetailSequence = new PositiveIntType(); 2242 this.subDetailSequence.setValue(value); 2243 return this; 2244 } 2245 2246 /** 2247 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 2248 */ 2249 public List<Identifier> getTraceNumber() { 2250 if (this.traceNumber == null) 2251 this.traceNumber = new ArrayList<Identifier>(); 2252 return this.traceNumber; 2253 } 2254 2255 /** 2256 * @return Returns a reference to <code>this</code> for easy method chaining 2257 */ 2258 public SubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 2259 this.traceNumber = theTraceNumber; 2260 return this; 2261 } 2262 2263 public boolean hasTraceNumber() { 2264 if (this.traceNumber == null) 2265 return false; 2266 for (Identifier item : this.traceNumber) 2267 if (!item.isEmpty()) 2268 return true; 2269 return false; 2270 } 2271 2272 public Identifier addTraceNumber() { //3 2273 Identifier t = new Identifier(); 2274 if (this.traceNumber == null) 2275 this.traceNumber = new ArrayList<Identifier>(); 2276 this.traceNumber.add(t); 2277 return t; 2278 } 2279 2280 public SubDetailComponent addTraceNumber(Identifier t) { //3 2281 if (t == null) 2282 return this; 2283 if (this.traceNumber == null) 2284 this.traceNumber = new ArrayList<Identifier>(); 2285 this.traceNumber.add(t); 2286 return this; 2287 } 2288 2289 /** 2290 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 2291 */ 2292 public Identifier getTraceNumberFirstRep() { 2293 if (getTraceNumber().isEmpty()) { 2294 addTraceNumber(); 2295 } 2296 return getTraceNumber().get(0); 2297 } 2298 2299 /** 2300 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 2301 */ 2302 public List<PositiveIntType> getNoteNumber() { 2303 if (this.noteNumber == null) 2304 this.noteNumber = new ArrayList<PositiveIntType>(); 2305 return this.noteNumber; 2306 } 2307 2308 /** 2309 * @return Returns a reference to <code>this</code> for easy method chaining 2310 */ 2311 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 2312 this.noteNumber = theNoteNumber; 2313 return this; 2314 } 2315 2316 public boolean hasNoteNumber() { 2317 if (this.noteNumber == null) 2318 return false; 2319 for (PositiveIntType item : this.noteNumber) 2320 if (!item.isEmpty()) 2321 return true; 2322 return false; 2323 } 2324 2325 /** 2326 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 2327 */ 2328 public PositiveIntType addNoteNumberElement() {//2 2329 PositiveIntType t = new PositiveIntType(); 2330 if (this.noteNumber == null) 2331 this.noteNumber = new ArrayList<PositiveIntType>(); 2332 this.noteNumber.add(t); 2333 return t; 2334 } 2335 2336 /** 2337 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 2338 */ 2339 public SubDetailComponent addNoteNumber(int value) { //1 2340 PositiveIntType t = new PositiveIntType(); 2341 t.setValue(value); 2342 if (this.noteNumber == null) 2343 this.noteNumber = new ArrayList<PositiveIntType>(); 2344 this.noteNumber.add(t); 2345 return this; 2346 } 2347 2348 /** 2349 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 2350 */ 2351 public boolean hasNoteNumber(int value) { 2352 if (this.noteNumber == null) 2353 return false; 2354 for (PositiveIntType v : this.noteNumber) 2355 if (v.getValue().equals(value)) // positiveInt 2356 return true; 2357 return false; 2358 } 2359 2360 /** 2361 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 2362 */ 2363 public ReviewOutcomeComponent getReviewOutcome() { 2364 if (this.reviewOutcome == null) 2365 if (Configuration.errorOnAutoCreate()) 2366 throw new Error("Attempt to auto-create SubDetailComponent.reviewOutcome"); 2367 else if (Configuration.doAutoCreate()) 2368 this.reviewOutcome = new ReviewOutcomeComponent(); // cc 2369 return this.reviewOutcome; 2370 } 2371 2372 public boolean hasReviewOutcome() { 2373 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 2374 } 2375 2376 /** 2377 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 2378 */ 2379 public SubDetailComponent setReviewOutcome(ReviewOutcomeComponent value) { 2380 this.reviewOutcome = value; 2381 return this; 2382 } 2383 2384 /** 2385 * @return {@link #adjudication} (The adjudication results.) 2386 */ 2387 public List<AdjudicationComponent> getAdjudication() { 2388 if (this.adjudication == null) 2389 this.adjudication = new ArrayList<AdjudicationComponent>(); 2390 return this.adjudication; 2391 } 2392 2393 /** 2394 * @return Returns a reference to <code>this</code> for easy method chaining 2395 */ 2396 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 2397 this.adjudication = theAdjudication; 2398 return this; 2399 } 2400 2401 public boolean hasAdjudication() { 2402 if (this.adjudication == null) 2403 return false; 2404 for (AdjudicationComponent item : this.adjudication) 2405 if (!item.isEmpty()) 2406 return true; 2407 return false; 2408 } 2409 2410 public AdjudicationComponent addAdjudication() { //3 2411 AdjudicationComponent t = new AdjudicationComponent(); 2412 if (this.adjudication == null) 2413 this.adjudication = new ArrayList<AdjudicationComponent>(); 2414 this.adjudication.add(t); 2415 return t; 2416 } 2417 2418 public SubDetailComponent addAdjudication(AdjudicationComponent t) { //3 2419 if (t == null) 2420 return this; 2421 if (this.adjudication == null) 2422 this.adjudication = new ArrayList<AdjudicationComponent>(); 2423 this.adjudication.add(t); 2424 return this; 2425 } 2426 2427 /** 2428 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 2429 */ 2430 public AdjudicationComponent getAdjudicationFirstRep() { 2431 if (getAdjudication().isEmpty()) { 2432 addAdjudication(); 2433 } 2434 return getAdjudication().get(0); 2435 } 2436 2437 protected void listChildren(List<Property> children) { 2438 super.listChildren(children); 2439 children.add(new Property("subDetailSequence", "positiveInt", "A number to uniquely reference the claim sub-detail entry.", 0, 1, subDetailSequence)); 2440 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 2441 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 2442 children.add(new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 2443 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 2444 } 2445 2446 @Override 2447 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2448 switch (_hash) { 2449 case -855462510: /*subDetailSequence*/ return new Property("subDetailSequence", "positiveInt", "A number to uniquely reference the claim sub-detail entry.", 0, 1, subDetailSequence); 2450 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 2451 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 2452 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 2453 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 2454 default: return super.getNamedProperty(_hash, _name, _checkValid); 2455 } 2456 2457 } 2458 2459 @Override 2460 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2461 switch (hash) { 2462 case -855462510: /*subDetailSequence*/ return this.subDetailSequence == null ? new Base[0] : new Base[] {this.subDetailSequence}; // PositiveIntType 2463 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 2464 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 2465 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ReviewOutcomeComponent 2466 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 2467 default: return super.getProperty(hash, name, checkValid); 2468 } 2469 2470 } 2471 2472 @Override 2473 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2474 switch (hash) { 2475 case -855462510: // subDetailSequence 2476 this.subDetailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2477 return value; 2478 case 82505966: // traceNumber 2479 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 2480 return value; 2481 case -1110033957: // noteNumber 2482 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 2483 return value; 2484 case -51825446: // reviewOutcome 2485 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 2486 return value; 2487 case -231349275: // adjudication 2488 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 2489 return value; 2490 default: return super.setProperty(hash, name, value); 2491 } 2492 2493 } 2494 2495 @Override 2496 public Base setProperty(String name, Base value) throws FHIRException { 2497 if (name.equals("subDetailSequence")) { 2498 this.subDetailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2499 } else if (name.equals("traceNumber")) { 2500 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 2501 } else if (name.equals("noteNumber")) { 2502 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 2503 } else if (name.equals("reviewOutcome")) { 2504 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 2505 } else if (name.equals("adjudication")) { 2506 this.getAdjudication().add((AdjudicationComponent) value); 2507 } else 2508 return super.setProperty(name, value); 2509 return value; 2510 } 2511 2512 @Override 2513 public void removeChild(String name, Base value) throws FHIRException { 2514 if (name.equals("subDetailSequence")) { 2515 this.subDetailSequence = null; 2516 } else if (name.equals("traceNumber")) { 2517 this.getTraceNumber().remove(value); 2518 } else if (name.equals("noteNumber")) { 2519 this.getNoteNumber().remove(value); 2520 } else if (name.equals("reviewOutcome")) { 2521 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 2522 } else if (name.equals("adjudication")) { 2523 this.getAdjudication().remove((AdjudicationComponent) value); 2524 } else 2525 super.removeChild(name, value); 2526 2527 } 2528 2529 @Override 2530 public Base makeProperty(int hash, String name) throws FHIRException { 2531 switch (hash) { 2532 case -855462510: return getSubDetailSequenceElement(); 2533 case 82505966: return addTraceNumber(); 2534 case -1110033957: return addNoteNumberElement(); 2535 case -51825446: return getReviewOutcome(); 2536 case -231349275: return addAdjudication(); 2537 default: return super.makeProperty(hash, name); 2538 } 2539 2540 } 2541 2542 @Override 2543 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2544 switch (hash) { 2545 case -855462510: /*subDetailSequence*/ return new String[] {"positiveInt"}; 2546 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 2547 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 2548 case -51825446: /*reviewOutcome*/ return new String[] {"@ClaimResponse.item.reviewOutcome"}; 2549 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 2550 default: return super.getTypesForProperty(hash, name); 2551 } 2552 2553 } 2554 2555 @Override 2556 public Base addChild(String name) throws FHIRException { 2557 if (name.equals("subDetailSequence")) { 2558 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.detail.subDetail.subDetailSequence"); 2559 } 2560 else if (name.equals("traceNumber")) { 2561 return addTraceNumber(); 2562 } 2563 else if (name.equals("noteNumber")) { 2564 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.item.detail.subDetail.noteNumber"); 2565 } 2566 else if (name.equals("reviewOutcome")) { 2567 this.reviewOutcome = new ReviewOutcomeComponent(); 2568 return this.reviewOutcome; 2569 } 2570 else if (name.equals("adjudication")) { 2571 return addAdjudication(); 2572 } 2573 else 2574 return super.addChild(name); 2575 } 2576 2577 public SubDetailComponent copy() { 2578 SubDetailComponent dst = new SubDetailComponent(); 2579 copyValues(dst); 2580 return dst; 2581 } 2582 2583 public void copyValues(SubDetailComponent dst) { 2584 super.copyValues(dst); 2585 dst.subDetailSequence = subDetailSequence == null ? null : subDetailSequence.copy(); 2586 if (traceNumber != null) { 2587 dst.traceNumber = new ArrayList<Identifier>(); 2588 for (Identifier i : traceNumber) 2589 dst.traceNumber.add(i.copy()); 2590 }; 2591 if (noteNumber != null) { 2592 dst.noteNumber = new ArrayList<PositiveIntType>(); 2593 for (PositiveIntType i : noteNumber) 2594 dst.noteNumber.add(i.copy()); 2595 }; 2596 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 2597 if (adjudication != null) { 2598 dst.adjudication = new ArrayList<AdjudicationComponent>(); 2599 for (AdjudicationComponent i : adjudication) 2600 dst.adjudication.add(i.copy()); 2601 }; 2602 } 2603 2604 @Override 2605 public boolean equalsDeep(Base other_) { 2606 if (!super.equalsDeep(other_)) 2607 return false; 2608 if (!(other_ instanceof SubDetailComponent)) 2609 return false; 2610 SubDetailComponent o = (SubDetailComponent) other_; 2611 return compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 2612 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 2613 && compareDeep(adjudication, o.adjudication, true); 2614 } 2615 2616 @Override 2617 public boolean equalsShallow(Base other_) { 2618 if (!super.equalsShallow(other_)) 2619 return false; 2620 if (!(other_ instanceof SubDetailComponent)) 2621 return false; 2622 SubDetailComponent o = (SubDetailComponent) other_; 2623 return compareValues(subDetailSequence, o.subDetailSequence, true) && compareValues(noteNumber, o.noteNumber, true) 2624 ; 2625 } 2626 2627 public boolean isEmpty() { 2628 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subDetailSequence, traceNumber 2629 , noteNumber, reviewOutcome, adjudication); 2630 } 2631 2632 public String fhirType() { 2633 return "ClaimResponse.item.detail.subDetail"; 2634 2635 } 2636 2637 } 2638 2639 @Block() 2640 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 2641 /** 2642 * Claim items which this service line is intended to replace. 2643 */ 2644 @Child(name = "itemSequence", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2645 @Description(shortDefinition="Item sequence number", formalDefinition="Claim items which this service line is intended to replace." ) 2646 protected List<PositiveIntType> itemSequence; 2647 2648 /** 2649 * The sequence number of the details within the claim item which this line is intended to replace. 2650 */ 2651 @Child(name = "detailSequence", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2652 @Description(shortDefinition="Detail sequence number", formalDefinition="The sequence number of the details within the claim item which this line is intended to replace." ) 2653 protected List<PositiveIntType> detailSequence; 2654 2655 /** 2656 * The sequence number of the sub-details within the details within the claim item which this line is intended to replace. 2657 */ 2658 @Child(name = "subdetailSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2659 @Description(shortDefinition="Subdetail sequence number", formalDefinition="The sequence number of the sub-details within the details within the claim item which this line is intended to replace." ) 2660 protected List<PositiveIntType> subdetailSequence; 2661 2662 /** 2663 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 2664 */ 2665 @Child(name = "traceNumber", type = {Identifier.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2666 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 2667 protected List<Identifier> traceNumber; 2668 2669 /** 2670 * The providers who are authorized for the services rendered to the patient. 2671 */ 2672 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2673 @Description(shortDefinition="Authorized providers", formalDefinition="The providers who are authorized for the services rendered to the patient." ) 2674 protected List<Reference> provider; 2675 2676 /** 2677 * The type of revenue or cost center providing the product and/or service. 2678 */ 2679 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2680 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 2681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 2682 protected CodeableConcept revenue; 2683 2684 /** 2685 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 2686 */ 2687 @Child(name = "productOrService", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 2688 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 2689 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 2690 protected CodeableConcept productOrService; 2691 2692 /** 2693 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 2694 */ 2695 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 2696 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 2697 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 2698 protected CodeableConcept productOrServiceEnd; 2699 2700 /** 2701 * Request or Referral for Goods or Service to be rendered. 2702 */ 2703 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2704 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 2705 protected List<Reference> request; 2706 2707 /** 2708 * Item typification or modifiers codes to convey additional context for the product or service. 2709 */ 2710 @Child(name = "modifier", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2711 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 2712 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 2713 protected List<CodeableConcept> modifier; 2714 2715 /** 2716 * Identifies the program under which this may be recovered. 2717 */ 2718 @Child(name = "programCode", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2719 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 2720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 2721 protected List<CodeableConcept> programCode; 2722 2723 /** 2724 * The date or dates when the service or product was supplied, performed or completed. 2725 */ 2726 @Child(name = "serviced", type = {DateType.class, Period.class}, order=12, min=0, max=1, modifier=false, summary=false) 2727 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 2728 protected DataType serviced; 2729 2730 /** 2731 * Where the product or service was provided. 2732 */ 2733 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=13, min=0, max=1, modifier=false, summary=false) 2734 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 2735 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 2736 protected DataType location; 2737 2738 /** 2739 * The number of repetitions of a service or product. 2740 */ 2741 @Child(name = "quantity", type = {Quantity.class}, order=14, min=0, max=1, modifier=false, summary=false) 2742 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 2743 protected Quantity quantity; 2744 2745 /** 2746 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 2747 */ 2748 @Child(name = "unitPrice", type = {Money.class}, order=15, min=0, max=1, modifier=false, summary=false) 2749 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 2750 protected Money unitPrice; 2751 2752 /** 2753 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 2754 */ 2755 @Child(name = "factor", type = {DecimalType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2756 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 2757 protected DecimalType factor; 2758 2759 /** 2760 * The total of taxes applicable for this product or service. 2761 */ 2762 @Child(name = "tax", type = {Money.class}, order=17, min=0, max=1, modifier=false, summary=false) 2763 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 2764 protected Money tax; 2765 2766 /** 2767 * The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor. 2768 */ 2769 @Child(name = "net", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 2770 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor." ) 2771 protected Money net; 2772 2773 /** 2774 * Physical location where the service is performed or applies. 2775 */ 2776 @Child(name = "bodySite", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2777 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 2778 protected List<BodySiteComponent> bodySite; 2779 2780 /** 2781 * The numbers associated with notes below which apply to the adjudication of this item. 2782 */ 2783 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2784 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 2785 protected List<PositiveIntType> noteNumber; 2786 2787 /** 2788 * The high-level results of the adjudication if adjudication has been performed. 2789 */ 2790 @Child(name = "reviewOutcome", type = {ReviewOutcomeComponent.class}, order=21, min=0, max=1, modifier=false, summary=false) 2791 @Description(shortDefinition="Added items adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 2792 protected ReviewOutcomeComponent reviewOutcome; 2793 2794 /** 2795 * The adjudication results. 2796 */ 2797 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2798 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 2799 protected List<AdjudicationComponent> adjudication; 2800 2801 /** 2802 * The second-tier service adjudications for payor added services. 2803 */ 2804 @Child(name = "detail", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2805 @Description(shortDefinition="Insurer added line details", formalDefinition="The second-tier service adjudications for payor added services." ) 2806 protected List<AddedItemDetailComponent> detail; 2807 2808 private static final long serialVersionUID = 520179532L; 2809 2810 /** 2811 * Constructor 2812 */ 2813 public AddedItemComponent() { 2814 super(); 2815 } 2816 2817 /** 2818 * @return {@link #itemSequence} (Claim items which this service line is intended to replace.) 2819 */ 2820 public List<PositiveIntType> getItemSequence() { 2821 if (this.itemSequence == null) 2822 this.itemSequence = new ArrayList<PositiveIntType>(); 2823 return this.itemSequence; 2824 } 2825 2826 /** 2827 * @return Returns a reference to <code>this</code> for easy method chaining 2828 */ 2829 public AddedItemComponent setItemSequence(List<PositiveIntType> theItemSequence) { 2830 this.itemSequence = theItemSequence; 2831 return this; 2832 } 2833 2834 public boolean hasItemSequence() { 2835 if (this.itemSequence == null) 2836 return false; 2837 for (PositiveIntType item : this.itemSequence) 2838 if (!item.isEmpty()) 2839 return true; 2840 return false; 2841 } 2842 2843 /** 2844 * @return {@link #itemSequence} (Claim items which this service line is intended to replace.) 2845 */ 2846 public PositiveIntType addItemSequenceElement() {//2 2847 PositiveIntType t = new PositiveIntType(); 2848 if (this.itemSequence == null) 2849 this.itemSequence = new ArrayList<PositiveIntType>(); 2850 this.itemSequence.add(t); 2851 return t; 2852 } 2853 2854 /** 2855 * @param value {@link #itemSequence} (Claim items which this service line is intended to replace.) 2856 */ 2857 public AddedItemComponent addItemSequence(int value) { //1 2858 PositiveIntType t = new PositiveIntType(); 2859 t.setValue(value); 2860 if (this.itemSequence == null) 2861 this.itemSequence = new ArrayList<PositiveIntType>(); 2862 this.itemSequence.add(t); 2863 return this; 2864 } 2865 2866 /** 2867 * @param value {@link #itemSequence} (Claim items which this service line is intended to replace.) 2868 */ 2869 public boolean hasItemSequence(int value) { 2870 if (this.itemSequence == null) 2871 return false; 2872 for (PositiveIntType v : this.itemSequence) 2873 if (v.getValue().equals(value)) // positiveInt 2874 return true; 2875 return false; 2876 } 2877 2878 /** 2879 * @return {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 2880 */ 2881 public List<PositiveIntType> getDetailSequence() { 2882 if (this.detailSequence == null) 2883 this.detailSequence = new ArrayList<PositiveIntType>(); 2884 return this.detailSequence; 2885 } 2886 2887 /** 2888 * @return Returns a reference to <code>this</code> for easy method chaining 2889 */ 2890 public AddedItemComponent setDetailSequence(List<PositiveIntType> theDetailSequence) { 2891 this.detailSequence = theDetailSequence; 2892 return this; 2893 } 2894 2895 public boolean hasDetailSequence() { 2896 if (this.detailSequence == null) 2897 return false; 2898 for (PositiveIntType item : this.detailSequence) 2899 if (!item.isEmpty()) 2900 return true; 2901 return false; 2902 } 2903 2904 /** 2905 * @return {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 2906 */ 2907 public PositiveIntType addDetailSequenceElement() {//2 2908 PositiveIntType t = new PositiveIntType(); 2909 if (this.detailSequence == null) 2910 this.detailSequence = new ArrayList<PositiveIntType>(); 2911 this.detailSequence.add(t); 2912 return t; 2913 } 2914 2915 /** 2916 * @param value {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 2917 */ 2918 public AddedItemComponent addDetailSequence(int value) { //1 2919 PositiveIntType t = new PositiveIntType(); 2920 t.setValue(value); 2921 if (this.detailSequence == null) 2922 this.detailSequence = new ArrayList<PositiveIntType>(); 2923 this.detailSequence.add(t); 2924 return this; 2925 } 2926 2927 /** 2928 * @param value {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 2929 */ 2930 public boolean hasDetailSequence(int value) { 2931 if (this.detailSequence == null) 2932 return false; 2933 for (PositiveIntType v : this.detailSequence) 2934 if (v.getValue().equals(value)) // positiveInt 2935 return true; 2936 return false; 2937 } 2938 2939 /** 2940 * @return {@link #subdetailSequence} (The sequence number of the sub-details within the details within the claim item which this line is intended to replace.) 2941 */ 2942 public List<PositiveIntType> getSubdetailSequence() { 2943 if (this.subdetailSequence == null) 2944 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2945 return this.subdetailSequence; 2946 } 2947 2948 /** 2949 * @return Returns a reference to <code>this</code> for easy method chaining 2950 */ 2951 public AddedItemComponent setSubdetailSequence(List<PositiveIntType> theSubdetailSequence) { 2952 this.subdetailSequence = theSubdetailSequence; 2953 return this; 2954 } 2955 2956 public boolean hasSubdetailSequence() { 2957 if (this.subdetailSequence == null) 2958 return false; 2959 for (PositiveIntType item : this.subdetailSequence) 2960 if (!item.isEmpty()) 2961 return true; 2962 return false; 2963 } 2964 2965 /** 2966 * @return {@link #subdetailSequence} (The sequence number of the sub-details within the details within the claim item which this line is intended to replace.) 2967 */ 2968 public PositiveIntType addSubdetailSequenceElement() {//2 2969 PositiveIntType t = new PositiveIntType(); 2970 if (this.subdetailSequence == null) 2971 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2972 this.subdetailSequence.add(t); 2973 return t; 2974 } 2975 2976 /** 2977 * @param value {@link #subdetailSequence} (The sequence number of the sub-details within the details within the claim item which this line is intended to replace.) 2978 */ 2979 public AddedItemComponent addSubdetailSequence(int value) { //1 2980 PositiveIntType t = new PositiveIntType(); 2981 t.setValue(value); 2982 if (this.subdetailSequence == null) 2983 this.subdetailSequence = new ArrayList<PositiveIntType>(); 2984 this.subdetailSequence.add(t); 2985 return this; 2986 } 2987 2988 /** 2989 * @param value {@link #subdetailSequence} (The sequence number of the sub-details within the details within the claim item which this line is intended to replace.) 2990 */ 2991 public boolean hasSubdetailSequence(int value) { 2992 if (this.subdetailSequence == null) 2993 return false; 2994 for (PositiveIntType v : this.subdetailSequence) 2995 if (v.getValue().equals(value)) // positiveInt 2996 return true; 2997 return false; 2998 } 2999 3000 /** 3001 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 3002 */ 3003 public List<Identifier> getTraceNumber() { 3004 if (this.traceNumber == null) 3005 this.traceNumber = new ArrayList<Identifier>(); 3006 return this.traceNumber; 3007 } 3008 3009 /** 3010 * @return Returns a reference to <code>this</code> for easy method chaining 3011 */ 3012 public AddedItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 3013 this.traceNumber = theTraceNumber; 3014 return this; 3015 } 3016 3017 public boolean hasTraceNumber() { 3018 if (this.traceNumber == null) 3019 return false; 3020 for (Identifier item : this.traceNumber) 3021 if (!item.isEmpty()) 3022 return true; 3023 return false; 3024 } 3025 3026 public Identifier addTraceNumber() { //3 3027 Identifier t = new Identifier(); 3028 if (this.traceNumber == null) 3029 this.traceNumber = new ArrayList<Identifier>(); 3030 this.traceNumber.add(t); 3031 return t; 3032 } 3033 3034 public AddedItemComponent addTraceNumber(Identifier t) { //3 3035 if (t == null) 3036 return this; 3037 if (this.traceNumber == null) 3038 this.traceNumber = new ArrayList<Identifier>(); 3039 this.traceNumber.add(t); 3040 return this; 3041 } 3042 3043 /** 3044 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 3045 */ 3046 public Identifier getTraceNumberFirstRep() { 3047 if (getTraceNumber().isEmpty()) { 3048 addTraceNumber(); 3049 } 3050 return getTraceNumber().get(0); 3051 } 3052 3053 /** 3054 * @return {@link #provider} (The providers who are authorized for the services rendered to the patient.) 3055 */ 3056 public List<Reference> getProvider() { 3057 if (this.provider == null) 3058 this.provider = new ArrayList<Reference>(); 3059 return this.provider; 3060 } 3061 3062 /** 3063 * @return Returns a reference to <code>this</code> for easy method chaining 3064 */ 3065 public AddedItemComponent setProvider(List<Reference> theProvider) { 3066 this.provider = theProvider; 3067 return this; 3068 } 3069 3070 public boolean hasProvider() { 3071 if (this.provider == null) 3072 return false; 3073 for (Reference item : this.provider) 3074 if (!item.isEmpty()) 3075 return true; 3076 return false; 3077 } 3078 3079 public Reference addProvider() { //3 3080 Reference t = new Reference(); 3081 if (this.provider == null) 3082 this.provider = new ArrayList<Reference>(); 3083 this.provider.add(t); 3084 return t; 3085 } 3086 3087 public AddedItemComponent addProvider(Reference t) { //3 3088 if (t == null) 3089 return this; 3090 if (this.provider == null) 3091 this.provider = new ArrayList<Reference>(); 3092 this.provider.add(t); 3093 return this; 3094 } 3095 3096 /** 3097 * @return The first repetition of repeating field {@link #provider}, creating it if it does not already exist {3} 3098 */ 3099 public Reference getProviderFirstRep() { 3100 if (getProvider().isEmpty()) { 3101 addProvider(); 3102 } 3103 return getProvider().get(0); 3104 } 3105 3106 /** 3107 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 3108 */ 3109 public CodeableConcept getRevenue() { 3110 if (this.revenue == null) 3111 if (Configuration.errorOnAutoCreate()) 3112 throw new Error("Attempt to auto-create AddedItemComponent.revenue"); 3113 else if (Configuration.doAutoCreate()) 3114 this.revenue = new CodeableConcept(); // cc 3115 return this.revenue; 3116 } 3117 3118 public boolean hasRevenue() { 3119 return this.revenue != null && !this.revenue.isEmpty(); 3120 } 3121 3122 /** 3123 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 3124 */ 3125 public AddedItemComponent setRevenue(CodeableConcept value) { 3126 this.revenue = value; 3127 return this; 3128 } 3129 3130 /** 3131 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 3132 */ 3133 public CodeableConcept getProductOrService() { 3134 if (this.productOrService == null) 3135 if (Configuration.errorOnAutoCreate()) 3136 throw new Error("Attempt to auto-create AddedItemComponent.productOrService"); 3137 else if (Configuration.doAutoCreate()) 3138 this.productOrService = new CodeableConcept(); // cc 3139 return this.productOrService; 3140 } 3141 3142 public boolean hasProductOrService() { 3143 return this.productOrService != null && !this.productOrService.isEmpty(); 3144 } 3145 3146 /** 3147 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 3148 */ 3149 public AddedItemComponent setProductOrService(CodeableConcept value) { 3150 this.productOrService = value; 3151 return this; 3152 } 3153 3154 /** 3155 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 3156 */ 3157 public CodeableConcept getProductOrServiceEnd() { 3158 if (this.productOrServiceEnd == null) 3159 if (Configuration.errorOnAutoCreate()) 3160 throw new Error("Attempt to auto-create AddedItemComponent.productOrServiceEnd"); 3161 else if (Configuration.doAutoCreate()) 3162 this.productOrServiceEnd = new CodeableConcept(); // cc 3163 return this.productOrServiceEnd; 3164 } 3165 3166 public boolean hasProductOrServiceEnd() { 3167 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 3168 } 3169 3170 /** 3171 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 3172 */ 3173 public AddedItemComponent setProductOrServiceEnd(CodeableConcept value) { 3174 this.productOrServiceEnd = value; 3175 return this; 3176 } 3177 3178 /** 3179 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 3180 */ 3181 public List<Reference> getRequest() { 3182 if (this.request == null) 3183 this.request = new ArrayList<Reference>(); 3184 return this.request; 3185 } 3186 3187 /** 3188 * @return Returns a reference to <code>this</code> for easy method chaining 3189 */ 3190 public AddedItemComponent setRequest(List<Reference> theRequest) { 3191 this.request = theRequest; 3192 return this; 3193 } 3194 3195 public boolean hasRequest() { 3196 if (this.request == null) 3197 return false; 3198 for (Reference item : this.request) 3199 if (!item.isEmpty()) 3200 return true; 3201 return false; 3202 } 3203 3204 public Reference addRequest() { //3 3205 Reference t = new Reference(); 3206 if (this.request == null) 3207 this.request = new ArrayList<Reference>(); 3208 this.request.add(t); 3209 return t; 3210 } 3211 3212 public AddedItemComponent addRequest(Reference t) { //3 3213 if (t == null) 3214 return this; 3215 if (this.request == null) 3216 this.request = new ArrayList<Reference>(); 3217 this.request.add(t); 3218 return this; 3219 } 3220 3221 /** 3222 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 3223 */ 3224 public Reference getRequestFirstRep() { 3225 if (getRequest().isEmpty()) { 3226 addRequest(); 3227 } 3228 return getRequest().get(0); 3229 } 3230 3231 /** 3232 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 3233 */ 3234 public List<CodeableConcept> getModifier() { 3235 if (this.modifier == null) 3236 this.modifier = new ArrayList<CodeableConcept>(); 3237 return this.modifier; 3238 } 3239 3240 /** 3241 * @return Returns a reference to <code>this</code> for easy method chaining 3242 */ 3243 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 3244 this.modifier = theModifier; 3245 return this; 3246 } 3247 3248 public boolean hasModifier() { 3249 if (this.modifier == null) 3250 return false; 3251 for (CodeableConcept item : this.modifier) 3252 if (!item.isEmpty()) 3253 return true; 3254 return false; 3255 } 3256 3257 public CodeableConcept addModifier() { //3 3258 CodeableConcept t = new CodeableConcept(); 3259 if (this.modifier == null) 3260 this.modifier = new ArrayList<CodeableConcept>(); 3261 this.modifier.add(t); 3262 return t; 3263 } 3264 3265 public AddedItemComponent addModifier(CodeableConcept t) { //3 3266 if (t == null) 3267 return this; 3268 if (this.modifier == null) 3269 this.modifier = new ArrayList<CodeableConcept>(); 3270 this.modifier.add(t); 3271 return this; 3272 } 3273 3274 /** 3275 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 3276 */ 3277 public CodeableConcept getModifierFirstRep() { 3278 if (getModifier().isEmpty()) { 3279 addModifier(); 3280 } 3281 return getModifier().get(0); 3282 } 3283 3284 /** 3285 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 3286 */ 3287 public List<CodeableConcept> getProgramCode() { 3288 if (this.programCode == null) 3289 this.programCode = new ArrayList<CodeableConcept>(); 3290 return this.programCode; 3291 } 3292 3293 /** 3294 * @return Returns a reference to <code>this</code> for easy method chaining 3295 */ 3296 public AddedItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 3297 this.programCode = theProgramCode; 3298 return this; 3299 } 3300 3301 public boolean hasProgramCode() { 3302 if (this.programCode == null) 3303 return false; 3304 for (CodeableConcept item : this.programCode) 3305 if (!item.isEmpty()) 3306 return true; 3307 return false; 3308 } 3309 3310 public CodeableConcept addProgramCode() { //3 3311 CodeableConcept t = new CodeableConcept(); 3312 if (this.programCode == null) 3313 this.programCode = new ArrayList<CodeableConcept>(); 3314 this.programCode.add(t); 3315 return t; 3316 } 3317 3318 public AddedItemComponent addProgramCode(CodeableConcept t) { //3 3319 if (t == null) 3320 return this; 3321 if (this.programCode == null) 3322 this.programCode = new ArrayList<CodeableConcept>(); 3323 this.programCode.add(t); 3324 return this; 3325 } 3326 3327 /** 3328 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 3329 */ 3330 public CodeableConcept getProgramCodeFirstRep() { 3331 if (getProgramCode().isEmpty()) { 3332 addProgramCode(); 3333 } 3334 return getProgramCode().get(0); 3335 } 3336 3337 /** 3338 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 3339 */ 3340 public DataType getServiced() { 3341 return this.serviced; 3342 } 3343 3344 /** 3345 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 3346 */ 3347 public DateType getServicedDateType() throws FHIRException { 3348 if (this.serviced == null) 3349 this.serviced = new DateType(); 3350 if (!(this.serviced instanceof DateType)) 3351 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3352 return (DateType) this.serviced; 3353 } 3354 3355 public boolean hasServicedDateType() { 3356 return this != null && this.serviced instanceof DateType; 3357 } 3358 3359 /** 3360 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 3361 */ 3362 public Period getServicedPeriod() throws FHIRException { 3363 if (this.serviced == null) 3364 this.serviced = new Period(); 3365 if (!(this.serviced instanceof Period)) 3366 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3367 return (Period) this.serviced; 3368 } 3369 3370 public boolean hasServicedPeriod() { 3371 return this != null && this.serviced instanceof Period; 3372 } 3373 3374 public boolean hasServiced() { 3375 return this.serviced != null && !this.serviced.isEmpty(); 3376 } 3377 3378 /** 3379 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 3380 */ 3381 public AddedItemComponent setServiced(DataType value) { 3382 if (value != null && !(value instanceof DateType || value instanceof Period)) 3383 throw new FHIRException("Not the right type for ClaimResponse.addItem.serviced[x]: "+value.fhirType()); 3384 this.serviced = value; 3385 return this; 3386 } 3387 3388 /** 3389 * @return {@link #location} (Where the product or service was provided.) 3390 */ 3391 public DataType getLocation() { 3392 return this.location; 3393 } 3394 3395 /** 3396 * @return {@link #location} (Where the product or service was provided.) 3397 */ 3398 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 3399 if (this.location == null) 3400 this.location = new CodeableConcept(); 3401 if (!(this.location instanceof CodeableConcept)) 3402 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 3403 return (CodeableConcept) this.location; 3404 } 3405 3406 public boolean hasLocationCodeableConcept() { 3407 return this != null && this.location instanceof CodeableConcept; 3408 } 3409 3410 /** 3411 * @return {@link #location} (Where the product or service was provided.) 3412 */ 3413 public Address getLocationAddress() throws FHIRException { 3414 if (this.location == null) 3415 this.location = new Address(); 3416 if (!(this.location instanceof Address)) 3417 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3418 return (Address) this.location; 3419 } 3420 3421 public boolean hasLocationAddress() { 3422 return this != null && this.location instanceof Address; 3423 } 3424 3425 /** 3426 * @return {@link #location} (Where the product or service was provided.) 3427 */ 3428 public Reference getLocationReference() throws FHIRException { 3429 if (this.location == null) 3430 this.location = new Reference(); 3431 if (!(this.location instanceof Reference)) 3432 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3433 return (Reference) this.location; 3434 } 3435 3436 public boolean hasLocationReference() { 3437 return this != null && this.location instanceof Reference; 3438 } 3439 3440 public boolean hasLocation() { 3441 return this.location != null && !this.location.isEmpty(); 3442 } 3443 3444 /** 3445 * @param value {@link #location} (Where the product or service was provided.) 3446 */ 3447 public AddedItemComponent setLocation(DataType value) { 3448 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 3449 throw new FHIRException("Not the right type for ClaimResponse.addItem.location[x]: "+value.fhirType()); 3450 this.location = value; 3451 return this; 3452 } 3453 3454 /** 3455 * @return {@link #quantity} (The number of repetitions of a service or product.) 3456 */ 3457 public Quantity getQuantity() { 3458 if (this.quantity == null) 3459 if (Configuration.errorOnAutoCreate()) 3460 throw new Error("Attempt to auto-create AddedItemComponent.quantity"); 3461 else if (Configuration.doAutoCreate()) 3462 this.quantity = new Quantity(); // cc 3463 return this.quantity; 3464 } 3465 3466 public boolean hasQuantity() { 3467 return this.quantity != null && !this.quantity.isEmpty(); 3468 } 3469 3470 /** 3471 * @param value {@link #quantity} (The number of repetitions of a service or product.) 3472 */ 3473 public AddedItemComponent setQuantity(Quantity value) { 3474 this.quantity = value; 3475 return this; 3476 } 3477 3478 /** 3479 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 3480 */ 3481 public Money getUnitPrice() { 3482 if (this.unitPrice == null) 3483 if (Configuration.errorOnAutoCreate()) 3484 throw new Error("Attempt to auto-create AddedItemComponent.unitPrice"); 3485 else if (Configuration.doAutoCreate()) 3486 this.unitPrice = new Money(); // cc 3487 return this.unitPrice; 3488 } 3489 3490 public boolean hasUnitPrice() { 3491 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3492 } 3493 3494 /** 3495 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 3496 */ 3497 public AddedItemComponent setUnitPrice(Money value) { 3498 this.unitPrice = value; 3499 return this; 3500 } 3501 3502 /** 3503 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3504 */ 3505 public DecimalType getFactorElement() { 3506 if (this.factor == null) 3507 if (Configuration.errorOnAutoCreate()) 3508 throw new Error("Attempt to auto-create AddedItemComponent.factor"); 3509 else if (Configuration.doAutoCreate()) 3510 this.factor = new DecimalType(); // bb 3511 return this.factor; 3512 } 3513 3514 public boolean hasFactorElement() { 3515 return this.factor != null && !this.factor.isEmpty(); 3516 } 3517 3518 public boolean hasFactor() { 3519 return this.factor != null && !this.factor.isEmpty(); 3520 } 3521 3522 /** 3523 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3524 */ 3525 public AddedItemComponent setFactorElement(DecimalType value) { 3526 this.factor = value; 3527 return this; 3528 } 3529 3530 /** 3531 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3532 */ 3533 public BigDecimal getFactor() { 3534 return this.factor == null ? null : this.factor.getValue(); 3535 } 3536 3537 /** 3538 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3539 */ 3540 public AddedItemComponent setFactor(BigDecimal value) { 3541 if (value == null) 3542 this.factor = null; 3543 else { 3544 if (this.factor == null) 3545 this.factor = new DecimalType(); 3546 this.factor.setValue(value); 3547 } 3548 return this; 3549 } 3550 3551 /** 3552 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3553 */ 3554 public AddedItemComponent setFactor(long value) { 3555 this.factor = new DecimalType(); 3556 this.factor.setValue(value); 3557 return this; 3558 } 3559 3560 /** 3561 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3562 */ 3563 public AddedItemComponent setFactor(double value) { 3564 this.factor = new DecimalType(); 3565 this.factor.setValue(value); 3566 return this; 3567 } 3568 3569 /** 3570 * @return {@link #tax} (The total of taxes applicable for this product or service.) 3571 */ 3572 public Money getTax() { 3573 if (this.tax == null) 3574 if (Configuration.errorOnAutoCreate()) 3575 throw new Error("Attempt to auto-create AddedItemComponent.tax"); 3576 else if (Configuration.doAutoCreate()) 3577 this.tax = new Money(); // cc 3578 return this.tax; 3579 } 3580 3581 public boolean hasTax() { 3582 return this.tax != null && !this.tax.isEmpty(); 3583 } 3584 3585 /** 3586 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 3587 */ 3588 public AddedItemComponent setTax(Money value) { 3589 this.tax = value; 3590 return this; 3591 } 3592 3593 /** 3594 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.) 3595 */ 3596 public Money getNet() { 3597 if (this.net == null) 3598 if (Configuration.errorOnAutoCreate()) 3599 throw new Error("Attempt to auto-create AddedItemComponent.net"); 3600 else if (Configuration.doAutoCreate()) 3601 this.net = new Money(); // cc 3602 return this.net; 3603 } 3604 3605 public boolean hasNet() { 3606 return this.net != null && !this.net.isEmpty(); 3607 } 3608 3609 /** 3610 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.) 3611 */ 3612 public AddedItemComponent setNet(Money value) { 3613 this.net = value; 3614 return this; 3615 } 3616 3617 /** 3618 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 3619 */ 3620 public List<BodySiteComponent> getBodySite() { 3621 if (this.bodySite == null) 3622 this.bodySite = new ArrayList<BodySiteComponent>(); 3623 return this.bodySite; 3624 } 3625 3626 /** 3627 * @return Returns a reference to <code>this</code> for easy method chaining 3628 */ 3629 public AddedItemComponent setBodySite(List<BodySiteComponent> theBodySite) { 3630 this.bodySite = theBodySite; 3631 return this; 3632 } 3633 3634 public boolean hasBodySite() { 3635 if (this.bodySite == null) 3636 return false; 3637 for (BodySiteComponent item : this.bodySite) 3638 if (!item.isEmpty()) 3639 return true; 3640 return false; 3641 } 3642 3643 public BodySiteComponent addBodySite() { //3 3644 BodySiteComponent t = new BodySiteComponent(); 3645 if (this.bodySite == null) 3646 this.bodySite = new ArrayList<BodySiteComponent>(); 3647 this.bodySite.add(t); 3648 return t; 3649 } 3650 3651 public AddedItemComponent addBodySite(BodySiteComponent t) { //3 3652 if (t == null) 3653 return this; 3654 if (this.bodySite == null) 3655 this.bodySite = new ArrayList<BodySiteComponent>(); 3656 this.bodySite.add(t); 3657 return this; 3658 } 3659 3660 /** 3661 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 3662 */ 3663 public BodySiteComponent getBodySiteFirstRep() { 3664 if (getBodySite().isEmpty()) { 3665 addBodySite(); 3666 } 3667 return getBodySite().get(0); 3668 } 3669 3670 /** 3671 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 3672 */ 3673 public List<PositiveIntType> getNoteNumber() { 3674 if (this.noteNumber == null) 3675 this.noteNumber = new ArrayList<PositiveIntType>(); 3676 return this.noteNumber; 3677 } 3678 3679 /** 3680 * @return Returns a reference to <code>this</code> for easy method chaining 3681 */ 3682 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 3683 this.noteNumber = theNoteNumber; 3684 return this; 3685 } 3686 3687 public boolean hasNoteNumber() { 3688 if (this.noteNumber == null) 3689 return false; 3690 for (PositiveIntType item : this.noteNumber) 3691 if (!item.isEmpty()) 3692 return true; 3693 return false; 3694 } 3695 3696 /** 3697 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 3698 */ 3699 public PositiveIntType addNoteNumberElement() {//2 3700 PositiveIntType t = new PositiveIntType(); 3701 if (this.noteNumber == null) 3702 this.noteNumber = new ArrayList<PositiveIntType>(); 3703 this.noteNumber.add(t); 3704 return t; 3705 } 3706 3707 /** 3708 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 3709 */ 3710 public AddedItemComponent addNoteNumber(int value) { //1 3711 PositiveIntType t = new PositiveIntType(); 3712 t.setValue(value); 3713 if (this.noteNumber == null) 3714 this.noteNumber = new ArrayList<PositiveIntType>(); 3715 this.noteNumber.add(t); 3716 return this; 3717 } 3718 3719 /** 3720 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 3721 */ 3722 public boolean hasNoteNumber(int value) { 3723 if (this.noteNumber == null) 3724 return false; 3725 for (PositiveIntType v : this.noteNumber) 3726 if (v.getValue().equals(value)) // positiveInt 3727 return true; 3728 return false; 3729 } 3730 3731 /** 3732 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 3733 */ 3734 public ReviewOutcomeComponent getReviewOutcome() { 3735 if (this.reviewOutcome == null) 3736 if (Configuration.errorOnAutoCreate()) 3737 throw new Error("Attempt to auto-create AddedItemComponent.reviewOutcome"); 3738 else if (Configuration.doAutoCreate()) 3739 this.reviewOutcome = new ReviewOutcomeComponent(); // cc 3740 return this.reviewOutcome; 3741 } 3742 3743 public boolean hasReviewOutcome() { 3744 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 3745 } 3746 3747 /** 3748 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 3749 */ 3750 public AddedItemComponent setReviewOutcome(ReviewOutcomeComponent value) { 3751 this.reviewOutcome = value; 3752 return this; 3753 } 3754 3755 /** 3756 * @return {@link #adjudication} (The adjudication results.) 3757 */ 3758 public List<AdjudicationComponent> getAdjudication() { 3759 if (this.adjudication == null) 3760 this.adjudication = new ArrayList<AdjudicationComponent>(); 3761 return this.adjudication; 3762 } 3763 3764 /** 3765 * @return Returns a reference to <code>this</code> for easy method chaining 3766 */ 3767 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 3768 this.adjudication = theAdjudication; 3769 return this; 3770 } 3771 3772 public boolean hasAdjudication() { 3773 if (this.adjudication == null) 3774 return false; 3775 for (AdjudicationComponent item : this.adjudication) 3776 if (!item.isEmpty()) 3777 return true; 3778 return false; 3779 } 3780 3781 public AdjudicationComponent addAdjudication() { //3 3782 AdjudicationComponent t = new AdjudicationComponent(); 3783 if (this.adjudication == null) 3784 this.adjudication = new ArrayList<AdjudicationComponent>(); 3785 this.adjudication.add(t); 3786 return t; 3787 } 3788 3789 public AddedItemComponent addAdjudication(AdjudicationComponent t) { //3 3790 if (t == null) 3791 return this; 3792 if (this.adjudication == null) 3793 this.adjudication = new ArrayList<AdjudicationComponent>(); 3794 this.adjudication.add(t); 3795 return this; 3796 } 3797 3798 /** 3799 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 3800 */ 3801 public AdjudicationComponent getAdjudicationFirstRep() { 3802 if (getAdjudication().isEmpty()) { 3803 addAdjudication(); 3804 } 3805 return getAdjudication().get(0); 3806 } 3807 3808 /** 3809 * @return {@link #detail} (The second-tier service adjudications for payor added services.) 3810 */ 3811 public List<AddedItemDetailComponent> getDetail() { 3812 if (this.detail == null) 3813 this.detail = new ArrayList<AddedItemDetailComponent>(); 3814 return this.detail; 3815 } 3816 3817 /** 3818 * @return Returns a reference to <code>this</code> for easy method chaining 3819 */ 3820 public AddedItemComponent setDetail(List<AddedItemDetailComponent> theDetail) { 3821 this.detail = theDetail; 3822 return this; 3823 } 3824 3825 public boolean hasDetail() { 3826 if (this.detail == null) 3827 return false; 3828 for (AddedItemDetailComponent item : this.detail) 3829 if (!item.isEmpty()) 3830 return true; 3831 return false; 3832 } 3833 3834 public AddedItemDetailComponent addDetail() { //3 3835 AddedItemDetailComponent t = new AddedItemDetailComponent(); 3836 if (this.detail == null) 3837 this.detail = new ArrayList<AddedItemDetailComponent>(); 3838 this.detail.add(t); 3839 return t; 3840 } 3841 3842 public AddedItemComponent addDetail(AddedItemDetailComponent t) { //3 3843 if (t == null) 3844 return this; 3845 if (this.detail == null) 3846 this.detail = new ArrayList<AddedItemDetailComponent>(); 3847 this.detail.add(t); 3848 return this; 3849 } 3850 3851 /** 3852 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 3853 */ 3854 public AddedItemDetailComponent getDetailFirstRep() { 3855 if (getDetail().isEmpty()) { 3856 addDetail(); 3857 } 3858 return getDetail().get(0); 3859 } 3860 3861 protected void listChildren(List<Property> children) { 3862 super.listChildren(children); 3863 children.add(new Property("itemSequence", "positiveInt", "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence)); 3864 children.add(new Property("detailSequence", "positiveInt", "The sequence number of the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, detailSequence)); 3865 children.add(new Property("subdetailSequence", "positiveInt", "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, subdetailSequence)); 3866 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 3867 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, provider)); 3868 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 3869 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 3870 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 3871 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 3872 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 3873 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 3874 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 3875 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 3876 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 3877 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 3878 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 3879 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 3880 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.", 0, 1, net)); 3881 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 3882 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 3883 children.add(new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 3884 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 3885 children.add(new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail)); 3886 } 3887 3888 @Override 3889 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3890 switch (_hash) { 3891 case 1977979892: /*itemSequence*/ return new Property("itemSequence", "positiveInt", "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence); 3892 case 1321472818: /*detailSequence*/ return new Property("detailSequence", "positiveInt", "The sequence number of the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, detailSequence); 3893 case 146530674: /*subdetailSequence*/ return new Property("subdetailSequence", "positiveInt", "The sequence number of the sub-details within the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, subdetailSequence); 3894 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 3895 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, provider); 3896 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 3897 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 3898 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 3899 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 3900 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 3901 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 3902 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3903 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3904 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3905 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 3906 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 3907 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 3908 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 3909 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 3910 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 3911 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 3912 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 3913 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 3914 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 3915 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.", 0, 1, net); 3916 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 3917 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 3918 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 3919 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 3920 case -1335224239: /*detail*/ return new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 3921 default: return super.getNamedProperty(_hash, _name, _checkValid); 3922 } 3923 3924 } 3925 3926 @Override 3927 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3928 switch (hash) { 3929 case 1977979892: /*itemSequence*/ return this.itemSequence == null ? new Base[0] : this.itemSequence.toArray(new Base[this.itemSequence.size()]); // PositiveIntType 3930 case 1321472818: /*detailSequence*/ return this.detailSequence == null ? new Base[0] : this.detailSequence.toArray(new Base[this.detailSequence.size()]); // PositiveIntType 3931 case 146530674: /*subdetailSequence*/ return this.subdetailSequence == null ? new Base[0] : this.subdetailSequence.toArray(new Base[this.subdetailSequence.size()]); // PositiveIntType 3932 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 3933 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : this.provider.toArray(new Base[this.provider.size()]); // Reference 3934 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 3935 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 3936 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 3937 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 3938 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 3939 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 3940 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 3941 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 3942 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 3943 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 3944 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 3945 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 3946 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 3947 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // BodySiteComponent 3948 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 3949 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ReviewOutcomeComponent 3950 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 3951 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemDetailComponent 3952 default: return super.getProperty(hash, name, checkValid); 3953 } 3954 3955 } 3956 3957 @Override 3958 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3959 switch (hash) { 3960 case 1977979892: // itemSequence 3961 this.getItemSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 3962 return value; 3963 case 1321472818: // detailSequence 3964 this.getDetailSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 3965 return value; 3966 case 146530674: // subdetailSequence 3967 this.getSubdetailSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 3968 return value; 3969 case 82505966: // traceNumber 3970 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 3971 return value; 3972 case -987494927: // provider 3973 this.getProvider().add(TypeConvertor.castToReference(value)); // Reference 3974 return value; 3975 case 1099842588: // revenue 3976 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3977 return value; 3978 case 1957227299: // productOrService 3979 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3980 return value; 3981 case -717476168: // productOrServiceEnd 3982 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3983 return value; 3984 case 1095692943: // request 3985 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 3986 return value; 3987 case -615513385: // modifier 3988 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3989 return value; 3990 case 1010065041: // programCode 3991 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3992 return value; 3993 case 1379209295: // serviced 3994 this.serviced = TypeConvertor.castToType(value); // DataType 3995 return value; 3996 case 1901043637: // location 3997 this.location = TypeConvertor.castToType(value); // DataType 3998 return value; 3999 case -1285004149: // quantity 4000 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4001 return value; 4002 case -486196699: // unitPrice 4003 this.unitPrice = TypeConvertor.castToMoney(value); // Money 4004 return value; 4005 case -1282148017: // factor 4006 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 4007 return value; 4008 case 114603: // tax 4009 this.tax = TypeConvertor.castToMoney(value); // Money 4010 return value; 4011 case 108957: // net 4012 this.net = TypeConvertor.castToMoney(value); // Money 4013 return value; 4014 case 1702620169: // bodySite 4015 this.getBodySite().add((BodySiteComponent) value); // BodySiteComponent 4016 return value; 4017 case -1110033957: // noteNumber 4018 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4019 return value; 4020 case -51825446: // reviewOutcome 4021 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 4022 return value; 4023 case -231349275: // adjudication 4024 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 4025 return value; 4026 case -1335224239: // detail 4027 this.getDetail().add((AddedItemDetailComponent) value); // AddedItemDetailComponent 4028 return value; 4029 default: return super.setProperty(hash, name, value); 4030 } 4031 4032 } 4033 4034 @Override 4035 public Base setProperty(String name, Base value) throws FHIRException { 4036 if (name.equals("itemSequence")) { 4037 this.getItemSequence().add(TypeConvertor.castToPositiveInt(value)); 4038 } else if (name.equals("detailSequence")) { 4039 this.getDetailSequence().add(TypeConvertor.castToPositiveInt(value)); 4040 } else if (name.equals("subdetailSequence")) { 4041 this.getSubdetailSequence().add(TypeConvertor.castToPositiveInt(value)); 4042 } else if (name.equals("traceNumber")) { 4043 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 4044 } else if (name.equals("provider")) { 4045 this.getProvider().add(TypeConvertor.castToReference(value)); 4046 } else if (name.equals("revenue")) { 4047 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4048 } else if (name.equals("productOrService")) { 4049 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4050 } else if (name.equals("productOrServiceEnd")) { 4051 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4052 } else if (name.equals("request")) { 4053 this.getRequest().add(TypeConvertor.castToReference(value)); 4054 } else if (name.equals("modifier")) { 4055 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 4056 } else if (name.equals("programCode")) { 4057 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 4058 } else if (name.equals("serviced[x]")) { 4059 this.serviced = TypeConvertor.castToType(value); // DataType 4060 } else if (name.equals("location[x]")) { 4061 this.location = TypeConvertor.castToType(value); // DataType 4062 } else if (name.equals("quantity")) { 4063 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4064 } else if (name.equals("unitPrice")) { 4065 this.unitPrice = TypeConvertor.castToMoney(value); // Money 4066 } else if (name.equals("factor")) { 4067 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 4068 } else if (name.equals("tax")) { 4069 this.tax = TypeConvertor.castToMoney(value); // Money 4070 } else if (name.equals("net")) { 4071 this.net = TypeConvertor.castToMoney(value); // Money 4072 } else if (name.equals("bodySite")) { 4073 this.getBodySite().add((BodySiteComponent) value); 4074 } else if (name.equals("noteNumber")) { 4075 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 4076 } else if (name.equals("reviewOutcome")) { 4077 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 4078 } else if (name.equals("adjudication")) { 4079 this.getAdjudication().add((AdjudicationComponent) value); 4080 } else if (name.equals("detail")) { 4081 this.getDetail().add((AddedItemDetailComponent) value); 4082 } else 4083 return super.setProperty(name, value); 4084 return value; 4085 } 4086 4087 @Override 4088 public void removeChild(String name, Base value) throws FHIRException { 4089 if (name.equals("itemSequence")) { 4090 this.getItemSequence().remove(value); 4091 } else if (name.equals("detailSequence")) { 4092 this.getDetailSequence().remove(value); 4093 } else if (name.equals("subdetailSequence")) { 4094 this.getSubdetailSequence().remove(value); 4095 } else if (name.equals("traceNumber")) { 4096 this.getTraceNumber().remove(value); 4097 } else if (name.equals("provider")) { 4098 this.getProvider().remove(value); 4099 } else if (name.equals("revenue")) { 4100 this.revenue = null; 4101 } else if (name.equals("productOrService")) { 4102 this.productOrService = null; 4103 } else if (name.equals("productOrServiceEnd")) { 4104 this.productOrServiceEnd = null; 4105 } else if (name.equals("request")) { 4106 this.getRequest().remove(value); 4107 } else if (name.equals("modifier")) { 4108 this.getModifier().remove(value); 4109 } else if (name.equals("programCode")) { 4110 this.getProgramCode().remove(value); 4111 } else if (name.equals("serviced[x]")) { 4112 this.serviced = null; 4113 } else if (name.equals("location[x]")) { 4114 this.location = null; 4115 } else if (name.equals("quantity")) { 4116 this.quantity = null; 4117 } else if (name.equals("unitPrice")) { 4118 this.unitPrice = null; 4119 } else if (name.equals("factor")) { 4120 this.factor = null; 4121 } else if (name.equals("tax")) { 4122 this.tax = null; 4123 } else if (name.equals("net")) { 4124 this.net = null; 4125 } else if (name.equals("bodySite")) { 4126 this.getBodySite().remove((BodySiteComponent) value); 4127 } else if (name.equals("noteNumber")) { 4128 this.getNoteNumber().remove(value); 4129 } else if (name.equals("reviewOutcome")) { 4130 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 4131 } else if (name.equals("adjudication")) { 4132 this.getAdjudication().remove((AdjudicationComponent) value); 4133 } else if (name.equals("detail")) { 4134 this.getDetail().remove((AddedItemDetailComponent) value); 4135 } else 4136 super.removeChild(name, value); 4137 4138 } 4139 4140 @Override 4141 public Base makeProperty(int hash, String name) throws FHIRException { 4142 switch (hash) { 4143 case 1977979892: return addItemSequenceElement(); 4144 case 1321472818: return addDetailSequenceElement(); 4145 case 146530674: return addSubdetailSequenceElement(); 4146 case 82505966: return addTraceNumber(); 4147 case -987494927: return addProvider(); 4148 case 1099842588: return getRevenue(); 4149 case 1957227299: return getProductOrService(); 4150 case -717476168: return getProductOrServiceEnd(); 4151 case 1095692943: return addRequest(); 4152 case -615513385: return addModifier(); 4153 case 1010065041: return addProgramCode(); 4154 case -1927922223: return getServiced(); 4155 case 1379209295: return getServiced(); 4156 case 552316075: return getLocation(); 4157 case 1901043637: return getLocation(); 4158 case -1285004149: return getQuantity(); 4159 case -486196699: return getUnitPrice(); 4160 case -1282148017: return getFactorElement(); 4161 case 114603: return getTax(); 4162 case 108957: return getNet(); 4163 case 1702620169: return addBodySite(); 4164 case -1110033957: return addNoteNumberElement(); 4165 case -51825446: return getReviewOutcome(); 4166 case -231349275: return addAdjudication(); 4167 case -1335224239: return addDetail(); 4168 default: return super.makeProperty(hash, name); 4169 } 4170 4171 } 4172 4173 @Override 4174 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4175 switch (hash) { 4176 case 1977979892: /*itemSequence*/ return new String[] {"positiveInt"}; 4177 case 1321472818: /*detailSequence*/ return new String[] {"positiveInt"}; 4178 case 146530674: /*subdetailSequence*/ return new String[] {"positiveInt"}; 4179 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 4180 case -987494927: /*provider*/ return new String[] {"Reference"}; 4181 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 4182 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 4183 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 4184 case 1095692943: /*request*/ return new String[] {"Reference"}; 4185 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 4186 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 4187 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 4188 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 4189 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 4190 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 4191 case -1282148017: /*factor*/ return new String[] {"decimal"}; 4192 case 114603: /*tax*/ return new String[] {"Money"}; 4193 case 108957: /*net*/ return new String[] {"Money"}; 4194 case 1702620169: /*bodySite*/ return new String[] {}; 4195 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 4196 case -51825446: /*reviewOutcome*/ return new String[] {"@ClaimResponse.item.reviewOutcome"}; 4197 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 4198 case -1335224239: /*detail*/ return new String[] {}; 4199 default: return super.getTypesForProperty(hash, name); 4200 } 4201 4202 } 4203 4204 @Override 4205 public Base addChild(String name) throws FHIRException { 4206 if (name.equals("itemSequence")) { 4207 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.itemSequence"); 4208 } 4209 else if (name.equals("detailSequence")) { 4210 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.detailSequence"); 4211 } 4212 else if (name.equals("subdetailSequence")) { 4213 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.subdetailSequence"); 4214 } 4215 else if (name.equals("traceNumber")) { 4216 return addTraceNumber(); 4217 } 4218 else if (name.equals("provider")) { 4219 return addProvider(); 4220 } 4221 else if (name.equals("revenue")) { 4222 this.revenue = new CodeableConcept(); 4223 return this.revenue; 4224 } 4225 else if (name.equals("productOrService")) { 4226 this.productOrService = new CodeableConcept(); 4227 return this.productOrService; 4228 } 4229 else if (name.equals("productOrServiceEnd")) { 4230 this.productOrServiceEnd = new CodeableConcept(); 4231 return this.productOrServiceEnd; 4232 } 4233 else if (name.equals("request")) { 4234 return addRequest(); 4235 } 4236 else if (name.equals("modifier")) { 4237 return addModifier(); 4238 } 4239 else if (name.equals("programCode")) { 4240 return addProgramCode(); 4241 } 4242 else if (name.equals("servicedDate")) { 4243 this.serviced = new DateType(); 4244 return this.serviced; 4245 } 4246 else if (name.equals("servicedPeriod")) { 4247 this.serviced = new Period(); 4248 return this.serviced; 4249 } 4250 else if (name.equals("locationCodeableConcept")) { 4251 this.location = new CodeableConcept(); 4252 return this.location; 4253 } 4254 else if (name.equals("locationAddress")) { 4255 this.location = new Address(); 4256 return this.location; 4257 } 4258 else if (name.equals("locationReference")) { 4259 this.location = new Reference(); 4260 return this.location; 4261 } 4262 else if (name.equals("quantity")) { 4263 this.quantity = new Quantity(); 4264 return this.quantity; 4265 } 4266 else if (name.equals("unitPrice")) { 4267 this.unitPrice = new Money(); 4268 return this.unitPrice; 4269 } 4270 else if (name.equals("factor")) { 4271 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.factor"); 4272 } 4273 else if (name.equals("tax")) { 4274 this.tax = new Money(); 4275 return this.tax; 4276 } 4277 else if (name.equals("net")) { 4278 this.net = new Money(); 4279 return this.net; 4280 } 4281 else if (name.equals("bodySite")) { 4282 return addBodySite(); 4283 } 4284 else if (name.equals("noteNumber")) { 4285 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.noteNumber"); 4286 } 4287 else if (name.equals("reviewOutcome")) { 4288 this.reviewOutcome = new ReviewOutcomeComponent(); 4289 return this.reviewOutcome; 4290 } 4291 else if (name.equals("adjudication")) { 4292 return addAdjudication(); 4293 } 4294 else if (name.equals("detail")) { 4295 return addDetail(); 4296 } 4297 else 4298 return super.addChild(name); 4299 } 4300 4301 public AddedItemComponent copy() { 4302 AddedItemComponent dst = new AddedItemComponent(); 4303 copyValues(dst); 4304 return dst; 4305 } 4306 4307 public void copyValues(AddedItemComponent dst) { 4308 super.copyValues(dst); 4309 if (itemSequence != null) { 4310 dst.itemSequence = new ArrayList<PositiveIntType>(); 4311 for (PositiveIntType i : itemSequence) 4312 dst.itemSequence.add(i.copy()); 4313 }; 4314 if (detailSequence != null) { 4315 dst.detailSequence = new ArrayList<PositiveIntType>(); 4316 for (PositiveIntType i : detailSequence) 4317 dst.detailSequence.add(i.copy()); 4318 }; 4319 if (subdetailSequence != null) { 4320 dst.subdetailSequence = new ArrayList<PositiveIntType>(); 4321 for (PositiveIntType i : subdetailSequence) 4322 dst.subdetailSequence.add(i.copy()); 4323 }; 4324 if (traceNumber != null) { 4325 dst.traceNumber = new ArrayList<Identifier>(); 4326 for (Identifier i : traceNumber) 4327 dst.traceNumber.add(i.copy()); 4328 }; 4329 if (provider != null) { 4330 dst.provider = new ArrayList<Reference>(); 4331 for (Reference i : provider) 4332 dst.provider.add(i.copy()); 4333 }; 4334 dst.revenue = revenue == null ? null : revenue.copy(); 4335 dst.productOrService = productOrService == null ? null : productOrService.copy(); 4336 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 4337 if (request != null) { 4338 dst.request = new ArrayList<Reference>(); 4339 for (Reference i : request) 4340 dst.request.add(i.copy()); 4341 }; 4342 if (modifier != null) { 4343 dst.modifier = new ArrayList<CodeableConcept>(); 4344 for (CodeableConcept i : modifier) 4345 dst.modifier.add(i.copy()); 4346 }; 4347 if (programCode != null) { 4348 dst.programCode = new ArrayList<CodeableConcept>(); 4349 for (CodeableConcept i : programCode) 4350 dst.programCode.add(i.copy()); 4351 }; 4352 dst.serviced = serviced == null ? null : serviced.copy(); 4353 dst.location = location == null ? null : location.copy(); 4354 dst.quantity = quantity == null ? null : quantity.copy(); 4355 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4356 dst.factor = factor == null ? null : factor.copy(); 4357 dst.tax = tax == null ? null : tax.copy(); 4358 dst.net = net == null ? null : net.copy(); 4359 if (bodySite != null) { 4360 dst.bodySite = new ArrayList<BodySiteComponent>(); 4361 for (BodySiteComponent i : bodySite) 4362 dst.bodySite.add(i.copy()); 4363 }; 4364 if (noteNumber != null) { 4365 dst.noteNumber = new ArrayList<PositiveIntType>(); 4366 for (PositiveIntType i : noteNumber) 4367 dst.noteNumber.add(i.copy()); 4368 }; 4369 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 4370 if (adjudication != null) { 4371 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4372 for (AdjudicationComponent i : adjudication) 4373 dst.adjudication.add(i.copy()); 4374 }; 4375 if (detail != null) { 4376 dst.detail = new ArrayList<AddedItemDetailComponent>(); 4377 for (AddedItemDetailComponent i : detail) 4378 dst.detail.add(i.copy()); 4379 }; 4380 } 4381 4382 @Override 4383 public boolean equalsDeep(Base other_) { 4384 if (!super.equalsDeep(other_)) 4385 return false; 4386 if (!(other_ instanceof AddedItemComponent)) 4387 return false; 4388 AddedItemComponent o = (AddedItemComponent) other_; 4389 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 4390 && compareDeep(subdetailSequence, o.subdetailSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 4391 && compareDeep(provider, o.provider, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 4392 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 4393 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 4394 && compareDeep(location, o.location, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 4395 && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) 4396 && compareDeep(bodySite, o.bodySite, true) && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 4397 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 4398 } 4399 4400 @Override 4401 public boolean equalsShallow(Base other_) { 4402 if (!super.equalsShallow(other_)) 4403 return false; 4404 if (!(other_ instanceof AddedItemComponent)) 4405 return false; 4406 AddedItemComponent o = (AddedItemComponent) other_; 4407 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 4408 && compareValues(subdetailSequence, o.subdetailSequence, true) && compareValues(factor, o.factor, true) 4409 && compareValues(noteNumber, o.noteNumber, true); 4410 } 4411 4412 public boolean isEmpty() { 4413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence 4414 , subdetailSequence, traceNumber, provider, revenue, productOrService, productOrServiceEnd 4415 , request, modifier, programCode, serviced, location, quantity, unitPrice, factor 4416 , tax, net, bodySite, noteNumber, reviewOutcome, adjudication, detail); 4417 } 4418 4419 public String fhirType() { 4420 return "ClaimResponse.addItem"; 4421 4422 } 4423 4424 } 4425 4426 @Block() 4427 public static class BodySiteComponent extends BackboneElement implements IBaseBackboneElement { 4428 /** 4429 * Physical service site on the patient (limb, tooth, etc.). 4430 */ 4431 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4432 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 4433 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 4434 protected List<CodeableReference> site; 4435 4436 /** 4437 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 4438 */ 4439 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4440 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 4441 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 4442 protected List<CodeableConcept> subSite; 4443 4444 private static final long serialVersionUID = 1190632415L; 4445 4446 /** 4447 * Constructor 4448 */ 4449 public BodySiteComponent() { 4450 super(); 4451 } 4452 4453 /** 4454 * Constructor 4455 */ 4456 public BodySiteComponent(CodeableReference site) { 4457 super(); 4458 this.addSite(site); 4459 } 4460 4461 /** 4462 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 4463 */ 4464 public List<CodeableReference> getSite() { 4465 if (this.site == null) 4466 this.site = new ArrayList<CodeableReference>(); 4467 return this.site; 4468 } 4469 4470 /** 4471 * @return Returns a reference to <code>this</code> for easy method chaining 4472 */ 4473 public BodySiteComponent setSite(List<CodeableReference> theSite) { 4474 this.site = theSite; 4475 return this; 4476 } 4477 4478 public boolean hasSite() { 4479 if (this.site == null) 4480 return false; 4481 for (CodeableReference item : this.site) 4482 if (!item.isEmpty()) 4483 return true; 4484 return false; 4485 } 4486 4487 public CodeableReference addSite() { //3 4488 CodeableReference t = new CodeableReference(); 4489 if (this.site == null) 4490 this.site = new ArrayList<CodeableReference>(); 4491 this.site.add(t); 4492 return t; 4493 } 4494 4495 public BodySiteComponent addSite(CodeableReference t) { //3 4496 if (t == null) 4497 return this; 4498 if (this.site == null) 4499 this.site = new ArrayList<CodeableReference>(); 4500 this.site.add(t); 4501 return this; 4502 } 4503 4504 /** 4505 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 4506 */ 4507 public CodeableReference getSiteFirstRep() { 4508 if (getSite().isEmpty()) { 4509 addSite(); 4510 } 4511 return getSite().get(0); 4512 } 4513 4514 /** 4515 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 4516 */ 4517 public List<CodeableConcept> getSubSite() { 4518 if (this.subSite == null) 4519 this.subSite = new ArrayList<CodeableConcept>(); 4520 return this.subSite; 4521 } 4522 4523 /** 4524 * @return Returns a reference to <code>this</code> for easy method chaining 4525 */ 4526 public BodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 4527 this.subSite = theSubSite; 4528 return this; 4529 } 4530 4531 public boolean hasSubSite() { 4532 if (this.subSite == null) 4533 return false; 4534 for (CodeableConcept item : this.subSite) 4535 if (!item.isEmpty()) 4536 return true; 4537 return false; 4538 } 4539 4540 public CodeableConcept addSubSite() { //3 4541 CodeableConcept t = new CodeableConcept(); 4542 if (this.subSite == null) 4543 this.subSite = new ArrayList<CodeableConcept>(); 4544 this.subSite.add(t); 4545 return t; 4546 } 4547 4548 public BodySiteComponent addSubSite(CodeableConcept t) { //3 4549 if (t == null) 4550 return this; 4551 if (this.subSite == null) 4552 this.subSite = new ArrayList<CodeableConcept>(); 4553 this.subSite.add(t); 4554 return this; 4555 } 4556 4557 /** 4558 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 4559 */ 4560 public CodeableConcept getSubSiteFirstRep() { 4561 if (getSubSite().isEmpty()) { 4562 addSubSite(); 4563 } 4564 return getSubSite().get(0); 4565 } 4566 4567 protected void listChildren(List<Property> children) { 4568 super.listChildren(children); 4569 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 4570 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 4571 } 4572 4573 @Override 4574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4575 switch (_hash) { 4576 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 4577 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 4578 default: return super.getNamedProperty(_hash, _name, _checkValid); 4579 } 4580 4581 } 4582 4583 @Override 4584 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4585 switch (hash) { 4586 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 4587 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 4588 default: return super.getProperty(hash, name, checkValid); 4589 } 4590 4591 } 4592 4593 @Override 4594 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4595 switch (hash) { 4596 case 3530567: // site 4597 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4598 return value; 4599 case -1868566105: // subSite 4600 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4601 return value; 4602 default: return super.setProperty(hash, name, value); 4603 } 4604 4605 } 4606 4607 @Override 4608 public Base setProperty(String name, Base value) throws FHIRException { 4609 if (name.equals("site")) { 4610 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 4611 } else if (name.equals("subSite")) { 4612 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 4613 } else 4614 return super.setProperty(name, value); 4615 return value; 4616 } 4617 4618 @Override 4619 public void removeChild(String name, Base value) throws FHIRException { 4620 if (name.equals("site")) { 4621 this.getSite().remove(value); 4622 } else if (name.equals("subSite")) { 4623 this.getSubSite().remove(value); 4624 } else 4625 super.removeChild(name, value); 4626 4627 } 4628 4629 @Override 4630 public Base makeProperty(int hash, String name) throws FHIRException { 4631 switch (hash) { 4632 case 3530567: return addSite(); 4633 case -1868566105: return addSubSite(); 4634 default: return super.makeProperty(hash, name); 4635 } 4636 4637 } 4638 4639 @Override 4640 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4641 switch (hash) { 4642 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 4643 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 4644 default: return super.getTypesForProperty(hash, name); 4645 } 4646 4647 } 4648 4649 @Override 4650 public Base addChild(String name) throws FHIRException { 4651 if (name.equals("site")) { 4652 return addSite(); 4653 } 4654 else if (name.equals("subSite")) { 4655 return addSubSite(); 4656 } 4657 else 4658 return super.addChild(name); 4659 } 4660 4661 public BodySiteComponent copy() { 4662 BodySiteComponent dst = new BodySiteComponent(); 4663 copyValues(dst); 4664 return dst; 4665 } 4666 4667 public void copyValues(BodySiteComponent dst) { 4668 super.copyValues(dst); 4669 if (site != null) { 4670 dst.site = new ArrayList<CodeableReference>(); 4671 for (CodeableReference i : site) 4672 dst.site.add(i.copy()); 4673 }; 4674 if (subSite != null) { 4675 dst.subSite = new ArrayList<CodeableConcept>(); 4676 for (CodeableConcept i : subSite) 4677 dst.subSite.add(i.copy()); 4678 }; 4679 } 4680 4681 @Override 4682 public boolean equalsDeep(Base other_) { 4683 if (!super.equalsDeep(other_)) 4684 return false; 4685 if (!(other_ instanceof BodySiteComponent)) 4686 return false; 4687 BodySiteComponent o = (BodySiteComponent) other_; 4688 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 4689 } 4690 4691 @Override 4692 public boolean equalsShallow(Base other_) { 4693 if (!super.equalsShallow(other_)) 4694 return false; 4695 if (!(other_ instanceof BodySiteComponent)) 4696 return false; 4697 BodySiteComponent o = (BodySiteComponent) other_; 4698 return true; 4699 } 4700 4701 public boolean isEmpty() { 4702 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 4703 } 4704 4705 public String fhirType() { 4706 return "ClaimResponse.addItem.bodySite"; 4707 4708 } 4709 4710 } 4711 4712 @Block() 4713 public static class AddedItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 4714 /** 4715 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 4716 */ 4717 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4718 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 4719 protected List<Identifier> traceNumber; 4720 4721 /** 4722 * The type of revenue or cost center providing the product and/or service. 4723 */ 4724 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4725 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 4726 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 4727 protected CodeableConcept revenue; 4728 4729 /** 4730 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 4731 */ 4732 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 4733 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 4734 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 4735 protected CodeableConcept productOrService; 4736 4737 /** 4738 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 4739 */ 4740 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 4741 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 4742 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 4743 protected CodeableConcept productOrServiceEnd; 4744 4745 /** 4746 * Item typification or modifiers codes to convey additional context for the product or service. 4747 */ 4748 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4749 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 4750 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 4751 protected List<CodeableConcept> modifier; 4752 4753 /** 4754 * The number of repetitions of a service or product. 4755 */ 4756 @Child(name = "quantity", type = {Quantity.class}, order=6, min=0, max=1, modifier=false, summary=false) 4757 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 4758 protected Quantity quantity; 4759 4760 /** 4761 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 4762 */ 4763 @Child(name = "unitPrice", type = {Money.class}, order=7, min=0, max=1, modifier=false, summary=false) 4764 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 4765 protected Money unitPrice; 4766 4767 /** 4768 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4769 */ 4770 @Child(name = "factor", type = {DecimalType.class}, order=8, min=0, max=1, modifier=false, summary=false) 4771 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 4772 protected DecimalType factor; 4773 4774 /** 4775 * The total of taxes applicable for this product or service. 4776 */ 4777 @Child(name = "tax", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 4778 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 4779 protected Money tax; 4780 4781 /** 4782 * The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor. 4783 */ 4784 @Child(name = "net", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 4785 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor." ) 4786 protected Money net; 4787 4788 /** 4789 * The numbers associated with notes below which apply to the adjudication of this item. 4790 */ 4791 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4792 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 4793 protected List<PositiveIntType> noteNumber; 4794 4795 /** 4796 * The high-level results of the adjudication if adjudication has been performed. 4797 */ 4798 @Child(name = "reviewOutcome", type = {ReviewOutcomeComponent.class}, order=12, min=0, max=1, modifier=false, summary=false) 4799 @Description(shortDefinition="Added items detail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 4800 protected ReviewOutcomeComponent reviewOutcome; 4801 4802 /** 4803 * The adjudication results. 4804 */ 4805 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4806 @Description(shortDefinition="Added items detail adjudication", formalDefinition="The adjudication results." ) 4807 protected List<AdjudicationComponent> adjudication; 4808 4809 /** 4810 * The third-tier service adjudications for payor added services. 4811 */ 4812 @Child(name = "subDetail", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4813 @Description(shortDefinition="Insurer added line items", formalDefinition="The third-tier service adjudications for payor added services." ) 4814 protected List<AddedItemSubDetailComponent> subDetail; 4815 4816 private static final long serialVersionUID = 1574685043L; 4817 4818 /** 4819 * Constructor 4820 */ 4821 public AddedItemDetailComponent() { 4822 super(); 4823 } 4824 4825 /** 4826 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 4827 */ 4828 public List<Identifier> getTraceNumber() { 4829 if (this.traceNumber == null) 4830 this.traceNumber = new ArrayList<Identifier>(); 4831 return this.traceNumber; 4832 } 4833 4834 /** 4835 * @return Returns a reference to <code>this</code> for easy method chaining 4836 */ 4837 public AddedItemDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 4838 this.traceNumber = theTraceNumber; 4839 return this; 4840 } 4841 4842 public boolean hasTraceNumber() { 4843 if (this.traceNumber == null) 4844 return false; 4845 for (Identifier item : this.traceNumber) 4846 if (!item.isEmpty()) 4847 return true; 4848 return false; 4849 } 4850 4851 public Identifier addTraceNumber() { //3 4852 Identifier t = new Identifier(); 4853 if (this.traceNumber == null) 4854 this.traceNumber = new ArrayList<Identifier>(); 4855 this.traceNumber.add(t); 4856 return t; 4857 } 4858 4859 public AddedItemDetailComponent addTraceNumber(Identifier t) { //3 4860 if (t == null) 4861 return this; 4862 if (this.traceNumber == null) 4863 this.traceNumber = new ArrayList<Identifier>(); 4864 this.traceNumber.add(t); 4865 return this; 4866 } 4867 4868 /** 4869 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 4870 */ 4871 public Identifier getTraceNumberFirstRep() { 4872 if (getTraceNumber().isEmpty()) { 4873 addTraceNumber(); 4874 } 4875 return getTraceNumber().get(0); 4876 } 4877 4878 /** 4879 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4880 */ 4881 public CodeableConcept getRevenue() { 4882 if (this.revenue == null) 4883 if (Configuration.errorOnAutoCreate()) 4884 throw new Error("Attempt to auto-create AddedItemDetailComponent.revenue"); 4885 else if (Configuration.doAutoCreate()) 4886 this.revenue = new CodeableConcept(); // cc 4887 return this.revenue; 4888 } 4889 4890 public boolean hasRevenue() { 4891 return this.revenue != null && !this.revenue.isEmpty(); 4892 } 4893 4894 /** 4895 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4896 */ 4897 public AddedItemDetailComponent setRevenue(CodeableConcept value) { 4898 this.revenue = value; 4899 return this; 4900 } 4901 4902 /** 4903 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4904 */ 4905 public CodeableConcept getProductOrService() { 4906 if (this.productOrService == null) 4907 if (Configuration.errorOnAutoCreate()) 4908 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrService"); 4909 else if (Configuration.doAutoCreate()) 4910 this.productOrService = new CodeableConcept(); // cc 4911 return this.productOrService; 4912 } 4913 4914 public boolean hasProductOrService() { 4915 return this.productOrService != null && !this.productOrService.isEmpty(); 4916 } 4917 4918 /** 4919 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4920 */ 4921 public AddedItemDetailComponent setProductOrService(CodeableConcept value) { 4922 this.productOrService = value; 4923 return this; 4924 } 4925 4926 /** 4927 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4928 */ 4929 public CodeableConcept getProductOrServiceEnd() { 4930 if (this.productOrServiceEnd == null) 4931 if (Configuration.errorOnAutoCreate()) 4932 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrServiceEnd"); 4933 else if (Configuration.doAutoCreate()) 4934 this.productOrServiceEnd = new CodeableConcept(); // cc 4935 return this.productOrServiceEnd; 4936 } 4937 4938 public boolean hasProductOrServiceEnd() { 4939 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 4940 } 4941 4942 /** 4943 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4944 */ 4945 public AddedItemDetailComponent setProductOrServiceEnd(CodeableConcept value) { 4946 this.productOrServiceEnd = value; 4947 return this; 4948 } 4949 4950 /** 4951 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 4952 */ 4953 public List<CodeableConcept> getModifier() { 4954 if (this.modifier == null) 4955 this.modifier = new ArrayList<CodeableConcept>(); 4956 return this.modifier; 4957 } 4958 4959 /** 4960 * @return Returns a reference to <code>this</code> for easy method chaining 4961 */ 4962 public AddedItemDetailComponent setModifier(List<CodeableConcept> theModifier) { 4963 this.modifier = theModifier; 4964 return this; 4965 } 4966 4967 public boolean hasModifier() { 4968 if (this.modifier == null) 4969 return false; 4970 for (CodeableConcept item : this.modifier) 4971 if (!item.isEmpty()) 4972 return true; 4973 return false; 4974 } 4975 4976 public CodeableConcept addModifier() { //3 4977 CodeableConcept t = new CodeableConcept(); 4978 if (this.modifier == null) 4979 this.modifier = new ArrayList<CodeableConcept>(); 4980 this.modifier.add(t); 4981 return t; 4982 } 4983 4984 public AddedItemDetailComponent addModifier(CodeableConcept t) { //3 4985 if (t == null) 4986 return this; 4987 if (this.modifier == null) 4988 this.modifier = new ArrayList<CodeableConcept>(); 4989 this.modifier.add(t); 4990 return this; 4991 } 4992 4993 /** 4994 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 4995 */ 4996 public CodeableConcept getModifierFirstRep() { 4997 if (getModifier().isEmpty()) { 4998 addModifier(); 4999 } 5000 return getModifier().get(0); 5001 } 5002 5003 /** 5004 * @return {@link #quantity} (The number of repetitions of a service or product.) 5005 */ 5006 public Quantity getQuantity() { 5007 if (this.quantity == null) 5008 if (Configuration.errorOnAutoCreate()) 5009 throw new Error("Attempt to auto-create AddedItemDetailComponent.quantity"); 5010 else if (Configuration.doAutoCreate()) 5011 this.quantity = new Quantity(); // cc 5012 return this.quantity; 5013 } 5014 5015 public boolean hasQuantity() { 5016 return this.quantity != null && !this.quantity.isEmpty(); 5017 } 5018 5019 /** 5020 * @param value {@link #quantity} (The number of repetitions of a service or product.) 5021 */ 5022 public AddedItemDetailComponent setQuantity(Quantity value) { 5023 this.quantity = value; 5024 return this; 5025 } 5026 5027 /** 5028 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 5029 */ 5030 public Money getUnitPrice() { 5031 if (this.unitPrice == null) 5032 if (Configuration.errorOnAutoCreate()) 5033 throw new Error("Attempt to auto-create AddedItemDetailComponent.unitPrice"); 5034 else if (Configuration.doAutoCreate()) 5035 this.unitPrice = new Money(); // cc 5036 return this.unitPrice; 5037 } 5038 5039 public boolean hasUnitPrice() { 5040 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5041 } 5042 5043 /** 5044 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 5045 */ 5046 public AddedItemDetailComponent setUnitPrice(Money value) { 5047 this.unitPrice = value; 5048 return this; 5049 } 5050 5051 /** 5052 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5053 */ 5054 public DecimalType getFactorElement() { 5055 if (this.factor == null) 5056 if (Configuration.errorOnAutoCreate()) 5057 throw new Error("Attempt to auto-create AddedItemDetailComponent.factor"); 5058 else if (Configuration.doAutoCreate()) 5059 this.factor = new DecimalType(); // bb 5060 return this.factor; 5061 } 5062 5063 public boolean hasFactorElement() { 5064 return this.factor != null && !this.factor.isEmpty(); 5065 } 5066 5067 public boolean hasFactor() { 5068 return this.factor != null && !this.factor.isEmpty(); 5069 } 5070 5071 /** 5072 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5073 */ 5074 public AddedItemDetailComponent setFactorElement(DecimalType value) { 5075 this.factor = value; 5076 return this; 5077 } 5078 5079 /** 5080 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5081 */ 5082 public BigDecimal getFactor() { 5083 return this.factor == null ? null : this.factor.getValue(); 5084 } 5085 5086 /** 5087 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5088 */ 5089 public AddedItemDetailComponent setFactor(BigDecimal value) { 5090 if (value == null) 5091 this.factor = null; 5092 else { 5093 if (this.factor == null) 5094 this.factor = new DecimalType(); 5095 this.factor.setValue(value); 5096 } 5097 return this; 5098 } 5099 5100 /** 5101 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5102 */ 5103 public AddedItemDetailComponent setFactor(long value) { 5104 this.factor = new DecimalType(); 5105 this.factor.setValue(value); 5106 return this; 5107 } 5108 5109 /** 5110 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5111 */ 5112 public AddedItemDetailComponent setFactor(double value) { 5113 this.factor = new DecimalType(); 5114 this.factor.setValue(value); 5115 return this; 5116 } 5117 5118 /** 5119 * @return {@link #tax} (The total of taxes applicable for this product or service.) 5120 */ 5121 public Money getTax() { 5122 if (this.tax == null) 5123 if (Configuration.errorOnAutoCreate()) 5124 throw new Error("Attempt to auto-create AddedItemDetailComponent.tax"); 5125 else if (Configuration.doAutoCreate()) 5126 this.tax = new Money(); // cc 5127 return this.tax; 5128 } 5129 5130 public boolean hasTax() { 5131 return this.tax != null && !this.tax.isEmpty(); 5132 } 5133 5134 /** 5135 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 5136 */ 5137 public AddedItemDetailComponent setTax(Money value) { 5138 this.tax = value; 5139 return this; 5140 } 5141 5142 /** 5143 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.) 5144 */ 5145 public Money getNet() { 5146 if (this.net == null) 5147 if (Configuration.errorOnAutoCreate()) 5148 throw new Error("Attempt to auto-create AddedItemDetailComponent.net"); 5149 else if (Configuration.doAutoCreate()) 5150 this.net = new Money(); // cc 5151 return this.net; 5152 } 5153 5154 public boolean hasNet() { 5155 return this.net != null && !this.net.isEmpty(); 5156 } 5157 5158 /** 5159 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.) 5160 */ 5161 public AddedItemDetailComponent setNet(Money value) { 5162 this.net = value; 5163 return this; 5164 } 5165 5166 /** 5167 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 5168 */ 5169 public List<PositiveIntType> getNoteNumber() { 5170 if (this.noteNumber == null) 5171 this.noteNumber = new ArrayList<PositiveIntType>(); 5172 return this.noteNumber; 5173 } 5174 5175 /** 5176 * @return Returns a reference to <code>this</code> for easy method chaining 5177 */ 5178 public AddedItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 5179 this.noteNumber = theNoteNumber; 5180 return this; 5181 } 5182 5183 public boolean hasNoteNumber() { 5184 if (this.noteNumber == null) 5185 return false; 5186 for (PositiveIntType item : this.noteNumber) 5187 if (!item.isEmpty()) 5188 return true; 5189 return false; 5190 } 5191 5192 /** 5193 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 5194 */ 5195 public PositiveIntType addNoteNumberElement() {//2 5196 PositiveIntType t = new PositiveIntType(); 5197 if (this.noteNumber == null) 5198 this.noteNumber = new ArrayList<PositiveIntType>(); 5199 this.noteNumber.add(t); 5200 return t; 5201 } 5202 5203 /** 5204 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 5205 */ 5206 public AddedItemDetailComponent addNoteNumber(int value) { //1 5207 PositiveIntType t = new PositiveIntType(); 5208 t.setValue(value); 5209 if (this.noteNumber == null) 5210 this.noteNumber = new ArrayList<PositiveIntType>(); 5211 this.noteNumber.add(t); 5212 return this; 5213 } 5214 5215 /** 5216 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 5217 */ 5218 public boolean hasNoteNumber(int value) { 5219 if (this.noteNumber == null) 5220 return false; 5221 for (PositiveIntType v : this.noteNumber) 5222 if (v.getValue().equals(value)) // positiveInt 5223 return true; 5224 return false; 5225 } 5226 5227 /** 5228 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 5229 */ 5230 public ReviewOutcomeComponent getReviewOutcome() { 5231 if (this.reviewOutcome == null) 5232 if (Configuration.errorOnAutoCreate()) 5233 throw new Error("Attempt to auto-create AddedItemDetailComponent.reviewOutcome"); 5234 else if (Configuration.doAutoCreate()) 5235 this.reviewOutcome = new ReviewOutcomeComponent(); // cc 5236 return this.reviewOutcome; 5237 } 5238 5239 public boolean hasReviewOutcome() { 5240 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 5241 } 5242 5243 /** 5244 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 5245 */ 5246 public AddedItemDetailComponent setReviewOutcome(ReviewOutcomeComponent value) { 5247 this.reviewOutcome = value; 5248 return this; 5249 } 5250 5251 /** 5252 * @return {@link #adjudication} (The adjudication results.) 5253 */ 5254 public List<AdjudicationComponent> getAdjudication() { 5255 if (this.adjudication == null) 5256 this.adjudication = new ArrayList<AdjudicationComponent>(); 5257 return this.adjudication; 5258 } 5259 5260 /** 5261 * @return Returns a reference to <code>this</code> for easy method chaining 5262 */ 5263 public AddedItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 5264 this.adjudication = theAdjudication; 5265 return this; 5266 } 5267 5268 public boolean hasAdjudication() { 5269 if (this.adjudication == null) 5270 return false; 5271 for (AdjudicationComponent item : this.adjudication) 5272 if (!item.isEmpty()) 5273 return true; 5274 return false; 5275 } 5276 5277 public AdjudicationComponent addAdjudication() { //3 5278 AdjudicationComponent t = new AdjudicationComponent(); 5279 if (this.adjudication == null) 5280 this.adjudication = new ArrayList<AdjudicationComponent>(); 5281 this.adjudication.add(t); 5282 return t; 5283 } 5284 5285 public AddedItemDetailComponent addAdjudication(AdjudicationComponent t) { //3 5286 if (t == null) 5287 return this; 5288 if (this.adjudication == null) 5289 this.adjudication = new ArrayList<AdjudicationComponent>(); 5290 this.adjudication.add(t); 5291 return this; 5292 } 5293 5294 /** 5295 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 5296 */ 5297 public AdjudicationComponent getAdjudicationFirstRep() { 5298 if (getAdjudication().isEmpty()) { 5299 addAdjudication(); 5300 } 5301 return getAdjudication().get(0); 5302 } 5303 5304 /** 5305 * @return {@link #subDetail} (The third-tier service adjudications for payor added services.) 5306 */ 5307 public List<AddedItemSubDetailComponent> getSubDetail() { 5308 if (this.subDetail == null) 5309 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 5310 return this.subDetail; 5311 } 5312 5313 /** 5314 * @return Returns a reference to <code>this</code> for easy method chaining 5315 */ 5316 public AddedItemDetailComponent setSubDetail(List<AddedItemSubDetailComponent> theSubDetail) { 5317 this.subDetail = theSubDetail; 5318 return this; 5319 } 5320 5321 public boolean hasSubDetail() { 5322 if (this.subDetail == null) 5323 return false; 5324 for (AddedItemSubDetailComponent item : this.subDetail) 5325 if (!item.isEmpty()) 5326 return true; 5327 return false; 5328 } 5329 5330 public AddedItemSubDetailComponent addSubDetail() { //3 5331 AddedItemSubDetailComponent t = new AddedItemSubDetailComponent(); 5332 if (this.subDetail == null) 5333 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 5334 this.subDetail.add(t); 5335 return t; 5336 } 5337 5338 public AddedItemDetailComponent addSubDetail(AddedItemSubDetailComponent t) { //3 5339 if (t == null) 5340 return this; 5341 if (this.subDetail == null) 5342 this.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 5343 this.subDetail.add(t); 5344 return this; 5345 } 5346 5347 /** 5348 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 5349 */ 5350 public AddedItemSubDetailComponent getSubDetailFirstRep() { 5351 if (getSubDetail().isEmpty()) { 5352 addSubDetail(); 5353 } 5354 return getSubDetail().get(0); 5355 } 5356 5357 protected void listChildren(List<Property> children) { 5358 super.listChildren(children); 5359 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 5360 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 5361 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 5362 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 5363 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 5364 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 5365 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 5366 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 5367 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 5368 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.", 0, 1, net)); 5369 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 5370 children.add(new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 5371 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 5372 children.add(new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 5373 } 5374 5375 @Override 5376 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5377 switch (_hash) { 5378 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 5379 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 5380 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 5381 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 5382 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 5383 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 5384 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 5385 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 5386 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 5387 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.", 0, 1, net); 5388 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 5389 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 5390 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 5391 case -828829007: /*subDetail*/ return new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 5392 default: return super.getNamedProperty(_hash, _name, _checkValid); 5393 } 5394 5395 } 5396 5397 @Override 5398 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5399 switch (hash) { 5400 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 5401 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5402 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 5403 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 5404 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5405 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 5406 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5407 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5408 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 5409 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5410 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5411 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ReviewOutcomeComponent 5412 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5413 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // AddedItemSubDetailComponent 5414 default: return super.getProperty(hash, name, checkValid); 5415 } 5416 5417 } 5418 5419 @Override 5420 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5421 switch (hash) { 5422 case 82505966: // traceNumber 5423 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 5424 return value; 5425 case 1099842588: // revenue 5426 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5427 return value; 5428 case 1957227299: // productOrService 5429 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5430 return value; 5431 case -717476168: // productOrServiceEnd 5432 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5433 return value; 5434 case -615513385: // modifier 5435 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5436 return value; 5437 case -1285004149: // quantity 5438 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5439 return value; 5440 case -486196699: // unitPrice 5441 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5442 return value; 5443 case -1282148017: // factor 5444 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5445 return value; 5446 case 114603: // tax 5447 this.tax = TypeConvertor.castToMoney(value); // Money 5448 return value; 5449 case 108957: // net 5450 this.net = TypeConvertor.castToMoney(value); // Money 5451 return value; 5452 case -1110033957: // noteNumber 5453 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5454 return value; 5455 case -51825446: // reviewOutcome 5456 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 5457 return value; 5458 case -231349275: // adjudication 5459 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5460 return value; 5461 case -828829007: // subDetail 5462 this.getSubDetail().add((AddedItemSubDetailComponent) value); // AddedItemSubDetailComponent 5463 return value; 5464 default: return super.setProperty(hash, name, value); 5465 } 5466 5467 } 5468 5469 @Override 5470 public Base setProperty(String name, Base value) throws FHIRException { 5471 if (name.equals("traceNumber")) { 5472 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 5473 } else if (name.equals("revenue")) { 5474 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5475 } else if (name.equals("productOrService")) { 5476 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5477 } else if (name.equals("productOrServiceEnd")) { 5478 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5479 } else if (name.equals("modifier")) { 5480 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 5481 } else if (name.equals("quantity")) { 5482 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5483 } else if (name.equals("unitPrice")) { 5484 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5485 } else if (name.equals("factor")) { 5486 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5487 } else if (name.equals("tax")) { 5488 this.tax = TypeConvertor.castToMoney(value); // Money 5489 } else if (name.equals("net")) { 5490 this.net = TypeConvertor.castToMoney(value); // Money 5491 } else if (name.equals("noteNumber")) { 5492 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 5493 } else if (name.equals("reviewOutcome")) { 5494 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 5495 } else if (name.equals("adjudication")) { 5496 this.getAdjudication().add((AdjudicationComponent) value); 5497 } else if (name.equals("subDetail")) { 5498 this.getSubDetail().add((AddedItemSubDetailComponent) value); 5499 } else 5500 return super.setProperty(name, value); 5501 return value; 5502 } 5503 5504 @Override 5505 public void removeChild(String name, Base value) throws FHIRException { 5506 if (name.equals("traceNumber")) { 5507 this.getTraceNumber().remove(value); 5508 } else if (name.equals("revenue")) { 5509 this.revenue = null; 5510 } else if (name.equals("productOrService")) { 5511 this.productOrService = null; 5512 } else if (name.equals("productOrServiceEnd")) { 5513 this.productOrServiceEnd = null; 5514 } else if (name.equals("modifier")) { 5515 this.getModifier().remove(value); 5516 } else if (name.equals("quantity")) { 5517 this.quantity = null; 5518 } else if (name.equals("unitPrice")) { 5519 this.unitPrice = null; 5520 } else if (name.equals("factor")) { 5521 this.factor = null; 5522 } else if (name.equals("tax")) { 5523 this.tax = null; 5524 } else if (name.equals("net")) { 5525 this.net = null; 5526 } else if (name.equals("noteNumber")) { 5527 this.getNoteNumber().remove(value); 5528 } else if (name.equals("reviewOutcome")) { 5529 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 5530 } else if (name.equals("adjudication")) { 5531 this.getAdjudication().remove((AdjudicationComponent) value); 5532 } else if (name.equals("subDetail")) { 5533 this.getSubDetail().remove((AddedItemSubDetailComponent) value); 5534 } else 5535 super.removeChild(name, value); 5536 5537 } 5538 5539 @Override 5540 public Base makeProperty(int hash, String name) throws FHIRException { 5541 switch (hash) { 5542 case 82505966: return addTraceNumber(); 5543 case 1099842588: return getRevenue(); 5544 case 1957227299: return getProductOrService(); 5545 case -717476168: return getProductOrServiceEnd(); 5546 case -615513385: return addModifier(); 5547 case -1285004149: return getQuantity(); 5548 case -486196699: return getUnitPrice(); 5549 case -1282148017: return getFactorElement(); 5550 case 114603: return getTax(); 5551 case 108957: return getNet(); 5552 case -1110033957: return addNoteNumberElement(); 5553 case -51825446: return getReviewOutcome(); 5554 case -231349275: return addAdjudication(); 5555 case -828829007: return addSubDetail(); 5556 default: return super.makeProperty(hash, name); 5557 } 5558 5559 } 5560 5561 @Override 5562 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5563 switch (hash) { 5564 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 5565 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5566 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 5567 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 5568 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5569 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 5570 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5571 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5572 case 114603: /*tax*/ return new String[] {"Money"}; 5573 case 108957: /*net*/ return new String[] {"Money"}; 5574 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 5575 case -51825446: /*reviewOutcome*/ return new String[] {"@ClaimResponse.item.reviewOutcome"}; 5576 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 5577 case -828829007: /*subDetail*/ return new String[] {}; 5578 default: return super.getTypesForProperty(hash, name); 5579 } 5580 5581 } 5582 5583 @Override 5584 public Base addChild(String name) throws FHIRException { 5585 if (name.equals("traceNumber")) { 5586 return addTraceNumber(); 5587 } 5588 else if (name.equals("revenue")) { 5589 this.revenue = new CodeableConcept(); 5590 return this.revenue; 5591 } 5592 else if (name.equals("productOrService")) { 5593 this.productOrService = new CodeableConcept(); 5594 return this.productOrService; 5595 } 5596 else if (name.equals("productOrServiceEnd")) { 5597 this.productOrServiceEnd = new CodeableConcept(); 5598 return this.productOrServiceEnd; 5599 } 5600 else if (name.equals("modifier")) { 5601 return addModifier(); 5602 } 5603 else if (name.equals("quantity")) { 5604 this.quantity = new Quantity(); 5605 return this.quantity; 5606 } 5607 else if (name.equals("unitPrice")) { 5608 this.unitPrice = new Money(); 5609 return this.unitPrice; 5610 } 5611 else if (name.equals("factor")) { 5612 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.detail.factor"); 5613 } 5614 else if (name.equals("tax")) { 5615 this.tax = new Money(); 5616 return this.tax; 5617 } 5618 else if (name.equals("net")) { 5619 this.net = new Money(); 5620 return this.net; 5621 } 5622 else if (name.equals("noteNumber")) { 5623 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.detail.noteNumber"); 5624 } 5625 else if (name.equals("reviewOutcome")) { 5626 this.reviewOutcome = new ReviewOutcomeComponent(); 5627 return this.reviewOutcome; 5628 } 5629 else if (name.equals("adjudication")) { 5630 return addAdjudication(); 5631 } 5632 else if (name.equals("subDetail")) { 5633 return addSubDetail(); 5634 } 5635 else 5636 return super.addChild(name); 5637 } 5638 5639 public AddedItemDetailComponent copy() { 5640 AddedItemDetailComponent dst = new AddedItemDetailComponent(); 5641 copyValues(dst); 5642 return dst; 5643 } 5644 5645 public void copyValues(AddedItemDetailComponent dst) { 5646 super.copyValues(dst); 5647 if (traceNumber != null) { 5648 dst.traceNumber = new ArrayList<Identifier>(); 5649 for (Identifier i : traceNumber) 5650 dst.traceNumber.add(i.copy()); 5651 }; 5652 dst.revenue = revenue == null ? null : revenue.copy(); 5653 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5654 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 5655 if (modifier != null) { 5656 dst.modifier = new ArrayList<CodeableConcept>(); 5657 for (CodeableConcept i : modifier) 5658 dst.modifier.add(i.copy()); 5659 }; 5660 dst.quantity = quantity == null ? null : quantity.copy(); 5661 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5662 dst.factor = factor == null ? null : factor.copy(); 5663 dst.tax = tax == null ? null : tax.copy(); 5664 dst.net = net == null ? null : net.copy(); 5665 if (noteNumber != null) { 5666 dst.noteNumber = new ArrayList<PositiveIntType>(); 5667 for (PositiveIntType i : noteNumber) 5668 dst.noteNumber.add(i.copy()); 5669 }; 5670 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 5671 if (adjudication != null) { 5672 dst.adjudication = new ArrayList<AdjudicationComponent>(); 5673 for (AdjudicationComponent i : adjudication) 5674 dst.adjudication.add(i.copy()); 5675 }; 5676 if (subDetail != null) { 5677 dst.subDetail = new ArrayList<AddedItemSubDetailComponent>(); 5678 for (AddedItemSubDetailComponent i : subDetail) 5679 dst.subDetail.add(i.copy()); 5680 }; 5681 } 5682 5683 @Override 5684 public boolean equalsDeep(Base other_) { 5685 if (!super.equalsDeep(other_)) 5686 return false; 5687 if (!(other_ instanceof AddedItemDetailComponent)) 5688 return false; 5689 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 5690 return compareDeep(traceNumber, o.traceNumber, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 5691 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 5692 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 5693 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(noteNumber, o.noteNumber, true) 5694 && compareDeep(reviewOutcome, o.reviewOutcome, true) && compareDeep(adjudication, o.adjudication, true) 5695 && compareDeep(subDetail, o.subDetail, true); 5696 } 5697 5698 @Override 5699 public boolean equalsShallow(Base other_) { 5700 if (!super.equalsShallow(other_)) 5701 return false; 5702 if (!(other_ instanceof AddedItemDetailComponent)) 5703 return false; 5704 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 5705 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 5706 } 5707 5708 public boolean isEmpty() { 5709 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(traceNumber, revenue, productOrService 5710 , productOrServiceEnd, modifier, quantity, unitPrice, factor, tax, net, noteNumber 5711 , reviewOutcome, adjudication, subDetail); 5712 } 5713 5714 public String fhirType() { 5715 return "ClaimResponse.addItem.detail"; 5716 5717 } 5718 5719 } 5720 5721 @Block() 5722 public static class AddedItemSubDetailComponent extends BackboneElement implements IBaseBackboneElement { 5723 /** 5724 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 5725 */ 5726 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5727 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 5728 protected List<Identifier> traceNumber; 5729 5730 /** 5731 * The type of revenue or cost center providing the product and/or service. 5732 */ 5733 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5734 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 5735 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5736 protected CodeableConcept revenue; 5737 5738 /** 5739 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 5740 */ 5741 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5742 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 5743 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5744 protected CodeableConcept productOrService; 5745 5746 /** 5747 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 5748 */ 5749 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5750 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 5751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5752 protected CodeableConcept productOrServiceEnd; 5753 5754 /** 5755 * Item typification or modifiers codes to convey additional context for the product or service. 5756 */ 5757 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5758 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 5759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5760 protected List<CodeableConcept> modifier; 5761 5762 /** 5763 * The number of repetitions of a service or product. 5764 */ 5765 @Child(name = "quantity", type = {Quantity.class}, order=6, min=0, max=1, modifier=false, summary=false) 5766 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 5767 protected Quantity quantity; 5768 5769 /** 5770 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 5771 */ 5772 @Child(name = "unitPrice", type = {Money.class}, order=7, min=0, max=1, modifier=false, summary=false) 5773 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 5774 protected Money unitPrice; 5775 5776 /** 5777 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5778 */ 5779 @Child(name = "factor", type = {DecimalType.class}, order=8, min=0, max=1, modifier=false, summary=false) 5780 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5781 protected DecimalType factor; 5782 5783 /** 5784 * The total of taxes applicable for this product or service. 5785 */ 5786 @Child(name = "tax", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 5787 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 5788 protected Money tax; 5789 5790 /** 5791 * The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor. 5792 */ 5793 @Child(name = "net", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 5794 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor." ) 5795 protected Money net; 5796 5797 /** 5798 * The numbers associated with notes below which apply to the adjudication of this item. 5799 */ 5800 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5801 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 5802 protected List<PositiveIntType> noteNumber; 5803 5804 /** 5805 * The high-level results of the adjudication if adjudication has been performed. 5806 */ 5807 @Child(name = "reviewOutcome", type = {ReviewOutcomeComponent.class}, order=12, min=0, max=1, modifier=false, summary=false) 5808 @Description(shortDefinition="Added items subdetail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 5809 protected ReviewOutcomeComponent reviewOutcome; 5810 5811 /** 5812 * The adjudication results. 5813 */ 5814 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5815 @Description(shortDefinition="Added items subdetail adjudication", formalDefinition="The adjudication results." ) 5816 protected List<AdjudicationComponent> adjudication; 5817 5818 private static final long serialVersionUID = -1130518441L; 5819 5820 /** 5821 * Constructor 5822 */ 5823 public AddedItemSubDetailComponent() { 5824 super(); 5825 } 5826 5827 /** 5828 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 5829 */ 5830 public List<Identifier> getTraceNumber() { 5831 if (this.traceNumber == null) 5832 this.traceNumber = new ArrayList<Identifier>(); 5833 return this.traceNumber; 5834 } 5835 5836 /** 5837 * @return Returns a reference to <code>this</code> for easy method chaining 5838 */ 5839 public AddedItemSubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 5840 this.traceNumber = theTraceNumber; 5841 return this; 5842 } 5843 5844 public boolean hasTraceNumber() { 5845 if (this.traceNumber == null) 5846 return false; 5847 for (Identifier item : this.traceNumber) 5848 if (!item.isEmpty()) 5849 return true; 5850 return false; 5851 } 5852 5853 public Identifier addTraceNumber() { //3 5854 Identifier t = new Identifier(); 5855 if (this.traceNumber == null) 5856 this.traceNumber = new ArrayList<Identifier>(); 5857 this.traceNumber.add(t); 5858 return t; 5859 } 5860 5861 public AddedItemSubDetailComponent addTraceNumber(Identifier t) { //3 5862 if (t == null) 5863 return this; 5864 if (this.traceNumber == null) 5865 this.traceNumber = new ArrayList<Identifier>(); 5866 this.traceNumber.add(t); 5867 return this; 5868 } 5869 5870 /** 5871 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 5872 */ 5873 public Identifier getTraceNumberFirstRep() { 5874 if (getTraceNumber().isEmpty()) { 5875 addTraceNumber(); 5876 } 5877 return getTraceNumber().get(0); 5878 } 5879 5880 /** 5881 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 5882 */ 5883 public CodeableConcept getRevenue() { 5884 if (this.revenue == null) 5885 if (Configuration.errorOnAutoCreate()) 5886 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.revenue"); 5887 else if (Configuration.doAutoCreate()) 5888 this.revenue = new CodeableConcept(); // cc 5889 return this.revenue; 5890 } 5891 5892 public boolean hasRevenue() { 5893 return this.revenue != null && !this.revenue.isEmpty(); 5894 } 5895 5896 /** 5897 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 5898 */ 5899 public AddedItemSubDetailComponent setRevenue(CodeableConcept value) { 5900 this.revenue = value; 5901 return this; 5902 } 5903 5904 /** 5905 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 5906 */ 5907 public CodeableConcept getProductOrService() { 5908 if (this.productOrService == null) 5909 if (Configuration.errorOnAutoCreate()) 5910 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.productOrService"); 5911 else if (Configuration.doAutoCreate()) 5912 this.productOrService = new CodeableConcept(); // cc 5913 return this.productOrService; 5914 } 5915 5916 public boolean hasProductOrService() { 5917 return this.productOrService != null && !this.productOrService.isEmpty(); 5918 } 5919 5920 /** 5921 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 5922 */ 5923 public AddedItemSubDetailComponent setProductOrService(CodeableConcept value) { 5924 this.productOrService = value; 5925 return this; 5926 } 5927 5928 /** 5929 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 5930 */ 5931 public CodeableConcept getProductOrServiceEnd() { 5932 if (this.productOrServiceEnd == null) 5933 if (Configuration.errorOnAutoCreate()) 5934 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.productOrServiceEnd"); 5935 else if (Configuration.doAutoCreate()) 5936 this.productOrServiceEnd = new CodeableConcept(); // cc 5937 return this.productOrServiceEnd; 5938 } 5939 5940 public boolean hasProductOrServiceEnd() { 5941 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 5942 } 5943 5944 /** 5945 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 5946 */ 5947 public AddedItemSubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 5948 this.productOrServiceEnd = value; 5949 return this; 5950 } 5951 5952 /** 5953 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 5954 */ 5955 public List<CodeableConcept> getModifier() { 5956 if (this.modifier == null) 5957 this.modifier = new ArrayList<CodeableConcept>(); 5958 return this.modifier; 5959 } 5960 5961 /** 5962 * @return Returns a reference to <code>this</code> for easy method chaining 5963 */ 5964 public AddedItemSubDetailComponent setModifier(List<CodeableConcept> theModifier) { 5965 this.modifier = theModifier; 5966 return this; 5967 } 5968 5969 public boolean hasModifier() { 5970 if (this.modifier == null) 5971 return false; 5972 for (CodeableConcept item : this.modifier) 5973 if (!item.isEmpty()) 5974 return true; 5975 return false; 5976 } 5977 5978 public CodeableConcept addModifier() { //3 5979 CodeableConcept t = new CodeableConcept(); 5980 if (this.modifier == null) 5981 this.modifier = new ArrayList<CodeableConcept>(); 5982 this.modifier.add(t); 5983 return t; 5984 } 5985 5986 public AddedItemSubDetailComponent addModifier(CodeableConcept t) { //3 5987 if (t == null) 5988 return this; 5989 if (this.modifier == null) 5990 this.modifier = new ArrayList<CodeableConcept>(); 5991 this.modifier.add(t); 5992 return this; 5993 } 5994 5995 /** 5996 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 5997 */ 5998 public CodeableConcept getModifierFirstRep() { 5999 if (getModifier().isEmpty()) { 6000 addModifier(); 6001 } 6002 return getModifier().get(0); 6003 } 6004 6005 /** 6006 * @return {@link #quantity} (The number of repetitions of a service or product.) 6007 */ 6008 public Quantity getQuantity() { 6009 if (this.quantity == null) 6010 if (Configuration.errorOnAutoCreate()) 6011 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.quantity"); 6012 else if (Configuration.doAutoCreate()) 6013 this.quantity = new Quantity(); // cc 6014 return this.quantity; 6015 } 6016 6017 public boolean hasQuantity() { 6018 return this.quantity != null && !this.quantity.isEmpty(); 6019 } 6020 6021 /** 6022 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6023 */ 6024 public AddedItemSubDetailComponent setQuantity(Quantity value) { 6025 this.quantity = value; 6026 return this; 6027 } 6028 6029 /** 6030 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6031 */ 6032 public Money getUnitPrice() { 6033 if (this.unitPrice == null) 6034 if (Configuration.errorOnAutoCreate()) 6035 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.unitPrice"); 6036 else if (Configuration.doAutoCreate()) 6037 this.unitPrice = new Money(); // cc 6038 return this.unitPrice; 6039 } 6040 6041 public boolean hasUnitPrice() { 6042 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6043 } 6044 6045 /** 6046 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6047 */ 6048 public AddedItemSubDetailComponent setUnitPrice(Money value) { 6049 this.unitPrice = value; 6050 return this; 6051 } 6052 6053 /** 6054 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6055 */ 6056 public DecimalType getFactorElement() { 6057 if (this.factor == null) 6058 if (Configuration.errorOnAutoCreate()) 6059 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.factor"); 6060 else if (Configuration.doAutoCreate()) 6061 this.factor = new DecimalType(); // bb 6062 return this.factor; 6063 } 6064 6065 public boolean hasFactorElement() { 6066 return this.factor != null && !this.factor.isEmpty(); 6067 } 6068 6069 public boolean hasFactor() { 6070 return this.factor != null && !this.factor.isEmpty(); 6071 } 6072 6073 /** 6074 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6075 */ 6076 public AddedItemSubDetailComponent setFactorElement(DecimalType value) { 6077 this.factor = value; 6078 return this; 6079 } 6080 6081 /** 6082 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6083 */ 6084 public BigDecimal getFactor() { 6085 return this.factor == null ? null : this.factor.getValue(); 6086 } 6087 6088 /** 6089 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6090 */ 6091 public AddedItemSubDetailComponent setFactor(BigDecimal value) { 6092 if (value == null) 6093 this.factor = null; 6094 else { 6095 if (this.factor == null) 6096 this.factor = new DecimalType(); 6097 this.factor.setValue(value); 6098 } 6099 return this; 6100 } 6101 6102 /** 6103 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6104 */ 6105 public AddedItemSubDetailComponent setFactor(long value) { 6106 this.factor = new DecimalType(); 6107 this.factor.setValue(value); 6108 return this; 6109 } 6110 6111 /** 6112 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6113 */ 6114 public AddedItemSubDetailComponent setFactor(double value) { 6115 this.factor = new DecimalType(); 6116 this.factor.setValue(value); 6117 return this; 6118 } 6119 6120 /** 6121 * @return {@link #tax} (The total of taxes applicable for this product or service.) 6122 */ 6123 public Money getTax() { 6124 if (this.tax == null) 6125 if (Configuration.errorOnAutoCreate()) 6126 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.tax"); 6127 else if (Configuration.doAutoCreate()) 6128 this.tax = new Money(); // cc 6129 return this.tax; 6130 } 6131 6132 public boolean hasTax() { 6133 return this.tax != null && !this.tax.isEmpty(); 6134 } 6135 6136 /** 6137 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 6138 */ 6139 public AddedItemSubDetailComponent setTax(Money value) { 6140 this.tax = value; 6141 return this; 6142 } 6143 6144 /** 6145 * @return {@link #net} (The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.) 6146 */ 6147 public Money getNet() { 6148 if (this.net == null) 6149 if (Configuration.errorOnAutoCreate()) 6150 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.net"); 6151 else if (Configuration.doAutoCreate()) 6152 this.net = new Money(); // cc 6153 return this.net; 6154 } 6155 6156 public boolean hasNet() { 6157 return this.net != null && !this.net.isEmpty(); 6158 } 6159 6160 /** 6161 * @param value {@link #net} (The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.) 6162 */ 6163 public AddedItemSubDetailComponent setNet(Money value) { 6164 this.net = value; 6165 return this; 6166 } 6167 6168 /** 6169 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 6170 */ 6171 public List<PositiveIntType> getNoteNumber() { 6172 if (this.noteNumber == null) 6173 this.noteNumber = new ArrayList<PositiveIntType>(); 6174 return this.noteNumber; 6175 } 6176 6177 /** 6178 * @return Returns a reference to <code>this</code> for easy method chaining 6179 */ 6180 public AddedItemSubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 6181 this.noteNumber = theNoteNumber; 6182 return this; 6183 } 6184 6185 public boolean hasNoteNumber() { 6186 if (this.noteNumber == null) 6187 return false; 6188 for (PositiveIntType item : this.noteNumber) 6189 if (!item.isEmpty()) 6190 return true; 6191 return false; 6192 } 6193 6194 /** 6195 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 6196 */ 6197 public PositiveIntType addNoteNumberElement() {//2 6198 PositiveIntType t = new PositiveIntType(); 6199 if (this.noteNumber == null) 6200 this.noteNumber = new ArrayList<PositiveIntType>(); 6201 this.noteNumber.add(t); 6202 return t; 6203 } 6204 6205 /** 6206 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 6207 */ 6208 public AddedItemSubDetailComponent addNoteNumber(int value) { //1 6209 PositiveIntType t = new PositiveIntType(); 6210 t.setValue(value); 6211 if (this.noteNumber == null) 6212 this.noteNumber = new ArrayList<PositiveIntType>(); 6213 this.noteNumber.add(t); 6214 return this; 6215 } 6216 6217 /** 6218 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 6219 */ 6220 public boolean hasNoteNumber(int value) { 6221 if (this.noteNumber == null) 6222 return false; 6223 for (PositiveIntType v : this.noteNumber) 6224 if (v.getValue().equals(value)) // positiveInt 6225 return true; 6226 return false; 6227 } 6228 6229 /** 6230 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 6231 */ 6232 public ReviewOutcomeComponent getReviewOutcome() { 6233 if (this.reviewOutcome == null) 6234 if (Configuration.errorOnAutoCreate()) 6235 throw new Error("Attempt to auto-create AddedItemSubDetailComponent.reviewOutcome"); 6236 else if (Configuration.doAutoCreate()) 6237 this.reviewOutcome = new ReviewOutcomeComponent(); // cc 6238 return this.reviewOutcome; 6239 } 6240 6241 public boolean hasReviewOutcome() { 6242 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 6243 } 6244 6245 /** 6246 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 6247 */ 6248 public AddedItemSubDetailComponent setReviewOutcome(ReviewOutcomeComponent value) { 6249 this.reviewOutcome = value; 6250 return this; 6251 } 6252 6253 /** 6254 * @return {@link #adjudication} (The adjudication results.) 6255 */ 6256 public List<AdjudicationComponent> getAdjudication() { 6257 if (this.adjudication == null) 6258 this.adjudication = new ArrayList<AdjudicationComponent>(); 6259 return this.adjudication; 6260 } 6261 6262 /** 6263 * @return Returns a reference to <code>this</code> for easy method chaining 6264 */ 6265 public AddedItemSubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 6266 this.adjudication = theAdjudication; 6267 return this; 6268 } 6269 6270 public boolean hasAdjudication() { 6271 if (this.adjudication == null) 6272 return false; 6273 for (AdjudicationComponent item : this.adjudication) 6274 if (!item.isEmpty()) 6275 return true; 6276 return false; 6277 } 6278 6279 public AdjudicationComponent addAdjudication() { //3 6280 AdjudicationComponent t = new AdjudicationComponent(); 6281 if (this.adjudication == null) 6282 this.adjudication = new ArrayList<AdjudicationComponent>(); 6283 this.adjudication.add(t); 6284 return t; 6285 } 6286 6287 public AddedItemSubDetailComponent addAdjudication(AdjudicationComponent t) { //3 6288 if (t == null) 6289 return this; 6290 if (this.adjudication == null) 6291 this.adjudication = new ArrayList<AdjudicationComponent>(); 6292 this.adjudication.add(t); 6293 return this; 6294 } 6295 6296 /** 6297 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 6298 */ 6299 public AdjudicationComponent getAdjudicationFirstRep() { 6300 if (getAdjudication().isEmpty()) { 6301 addAdjudication(); 6302 } 6303 return getAdjudication().get(0); 6304 } 6305 6306 protected void listChildren(List<Property> children) { 6307 super.listChildren(children); 6308 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 6309 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 6310 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 6311 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 6312 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6313 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6314 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 6315 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6316 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 6317 children.add(new Property("net", "Money", "The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 6318 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 6319 children.add(new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 6320 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 6321 } 6322 6323 @Override 6324 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6325 switch (_hash) { 6326 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 6327 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 6328 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 6329 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 6330 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 6331 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6332 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 6333 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6334 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 6335 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 6336 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 6337 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ClaimResponse.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 6338 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 6339 default: return super.getNamedProperty(_hash, _name, _checkValid); 6340 } 6341 6342 } 6343 6344 @Override 6345 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6346 switch (hash) { 6347 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 6348 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6349 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 6350 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 6351 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6352 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 6353 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6354 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6355 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 6356 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6357 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 6358 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ReviewOutcomeComponent 6359 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 6360 default: return super.getProperty(hash, name, checkValid); 6361 } 6362 6363 } 6364 6365 @Override 6366 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6367 switch (hash) { 6368 case 82505966: // traceNumber 6369 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 6370 return value; 6371 case 1099842588: // revenue 6372 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6373 return value; 6374 case 1957227299: // productOrService 6375 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6376 return value; 6377 case -717476168: // productOrServiceEnd 6378 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6379 return value; 6380 case -615513385: // modifier 6381 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6382 return value; 6383 case -1285004149: // quantity 6384 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6385 return value; 6386 case -486196699: // unitPrice 6387 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6388 return value; 6389 case -1282148017: // factor 6390 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6391 return value; 6392 case 114603: // tax 6393 this.tax = TypeConvertor.castToMoney(value); // Money 6394 return value; 6395 case 108957: // net 6396 this.net = TypeConvertor.castToMoney(value); // Money 6397 return value; 6398 case -1110033957: // noteNumber 6399 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 6400 return value; 6401 case -51825446: // reviewOutcome 6402 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 6403 return value; 6404 case -231349275: // adjudication 6405 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 6406 return value; 6407 default: return super.setProperty(hash, name, value); 6408 } 6409 6410 } 6411 6412 @Override 6413 public Base setProperty(String name, Base value) throws FHIRException { 6414 if (name.equals("traceNumber")) { 6415 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 6416 } else if (name.equals("revenue")) { 6417 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6418 } else if (name.equals("productOrService")) { 6419 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6420 } else if (name.equals("productOrServiceEnd")) { 6421 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6422 } else if (name.equals("modifier")) { 6423 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 6424 } else if (name.equals("quantity")) { 6425 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6426 } else if (name.equals("unitPrice")) { 6427 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6428 } else if (name.equals("factor")) { 6429 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6430 } else if (name.equals("tax")) { 6431 this.tax = TypeConvertor.castToMoney(value); // Money 6432 } else if (name.equals("net")) { 6433 this.net = TypeConvertor.castToMoney(value); // Money 6434 } else if (name.equals("noteNumber")) { 6435 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 6436 } else if (name.equals("reviewOutcome")) { 6437 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 6438 } else if (name.equals("adjudication")) { 6439 this.getAdjudication().add((AdjudicationComponent) value); 6440 } else 6441 return super.setProperty(name, value); 6442 return value; 6443 } 6444 6445 @Override 6446 public void removeChild(String name, Base value) throws FHIRException { 6447 if (name.equals("traceNumber")) { 6448 this.getTraceNumber().remove(value); 6449 } else if (name.equals("revenue")) { 6450 this.revenue = null; 6451 } else if (name.equals("productOrService")) { 6452 this.productOrService = null; 6453 } else if (name.equals("productOrServiceEnd")) { 6454 this.productOrServiceEnd = null; 6455 } else if (name.equals("modifier")) { 6456 this.getModifier().remove(value); 6457 } else if (name.equals("quantity")) { 6458 this.quantity = null; 6459 } else if (name.equals("unitPrice")) { 6460 this.unitPrice = null; 6461 } else if (name.equals("factor")) { 6462 this.factor = null; 6463 } else if (name.equals("tax")) { 6464 this.tax = null; 6465 } else if (name.equals("net")) { 6466 this.net = null; 6467 } else if (name.equals("noteNumber")) { 6468 this.getNoteNumber().remove(value); 6469 } else if (name.equals("reviewOutcome")) { 6470 this.reviewOutcome = (ReviewOutcomeComponent) value; // ReviewOutcomeComponent 6471 } else if (name.equals("adjudication")) { 6472 this.getAdjudication().remove((AdjudicationComponent) value); 6473 } else 6474 super.removeChild(name, value); 6475 6476 } 6477 6478 @Override 6479 public Base makeProperty(int hash, String name) throws FHIRException { 6480 switch (hash) { 6481 case 82505966: return addTraceNumber(); 6482 case 1099842588: return getRevenue(); 6483 case 1957227299: return getProductOrService(); 6484 case -717476168: return getProductOrServiceEnd(); 6485 case -615513385: return addModifier(); 6486 case -1285004149: return getQuantity(); 6487 case -486196699: return getUnitPrice(); 6488 case -1282148017: return getFactorElement(); 6489 case 114603: return getTax(); 6490 case 108957: return getNet(); 6491 case -1110033957: return addNoteNumberElement(); 6492 case -51825446: return getReviewOutcome(); 6493 case -231349275: return addAdjudication(); 6494 default: return super.makeProperty(hash, name); 6495 } 6496 6497 } 6498 6499 @Override 6500 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6501 switch (hash) { 6502 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 6503 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6504 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 6505 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 6506 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6507 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 6508 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6509 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6510 case 114603: /*tax*/ return new String[] {"Money"}; 6511 case 108957: /*net*/ return new String[] {"Money"}; 6512 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 6513 case -51825446: /*reviewOutcome*/ return new String[] {"@ClaimResponse.item.reviewOutcome"}; 6514 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 6515 default: return super.getTypesForProperty(hash, name); 6516 } 6517 6518 } 6519 6520 @Override 6521 public Base addChild(String name) throws FHIRException { 6522 if (name.equals("traceNumber")) { 6523 return addTraceNumber(); 6524 } 6525 else if (name.equals("revenue")) { 6526 this.revenue = new CodeableConcept(); 6527 return this.revenue; 6528 } 6529 else if (name.equals("productOrService")) { 6530 this.productOrService = new CodeableConcept(); 6531 return this.productOrService; 6532 } 6533 else if (name.equals("productOrServiceEnd")) { 6534 this.productOrServiceEnd = new CodeableConcept(); 6535 return this.productOrServiceEnd; 6536 } 6537 else if (name.equals("modifier")) { 6538 return addModifier(); 6539 } 6540 else if (name.equals("quantity")) { 6541 this.quantity = new Quantity(); 6542 return this.quantity; 6543 } 6544 else if (name.equals("unitPrice")) { 6545 this.unitPrice = new Money(); 6546 return this.unitPrice; 6547 } 6548 else if (name.equals("factor")) { 6549 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.detail.subDetail.factor"); 6550 } 6551 else if (name.equals("tax")) { 6552 this.tax = new Money(); 6553 return this.tax; 6554 } 6555 else if (name.equals("net")) { 6556 this.net = new Money(); 6557 return this.net; 6558 } 6559 else if (name.equals("noteNumber")) { 6560 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.addItem.detail.subDetail.noteNumber"); 6561 } 6562 else if (name.equals("reviewOutcome")) { 6563 this.reviewOutcome = new ReviewOutcomeComponent(); 6564 return this.reviewOutcome; 6565 } 6566 else if (name.equals("adjudication")) { 6567 return addAdjudication(); 6568 } 6569 else 6570 return super.addChild(name); 6571 } 6572 6573 public AddedItemSubDetailComponent copy() { 6574 AddedItemSubDetailComponent dst = new AddedItemSubDetailComponent(); 6575 copyValues(dst); 6576 return dst; 6577 } 6578 6579 public void copyValues(AddedItemSubDetailComponent dst) { 6580 super.copyValues(dst); 6581 if (traceNumber != null) { 6582 dst.traceNumber = new ArrayList<Identifier>(); 6583 for (Identifier i : traceNumber) 6584 dst.traceNumber.add(i.copy()); 6585 }; 6586 dst.revenue = revenue == null ? null : revenue.copy(); 6587 dst.productOrService = productOrService == null ? null : productOrService.copy(); 6588 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 6589 if (modifier != null) { 6590 dst.modifier = new ArrayList<CodeableConcept>(); 6591 for (CodeableConcept i : modifier) 6592 dst.modifier.add(i.copy()); 6593 }; 6594 dst.quantity = quantity == null ? null : quantity.copy(); 6595 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6596 dst.factor = factor == null ? null : factor.copy(); 6597 dst.tax = tax == null ? null : tax.copy(); 6598 dst.net = net == null ? null : net.copy(); 6599 if (noteNumber != null) { 6600 dst.noteNumber = new ArrayList<PositiveIntType>(); 6601 for (PositiveIntType i : noteNumber) 6602 dst.noteNumber.add(i.copy()); 6603 }; 6604 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 6605 if (adjudication != null) { 6606 dst.adjudication = new ArrayList<AdjudicationComponent>(); 6607 for (AdjudicationComponent i : adjudication) 6608 dst.adjudication.add(i.copy()); 6609 }; 6610 } 6611 6612 @Override 6613 public boolean equalsDeep(Base other_) { 6614 if (!super.equalsDeep(other_)) 6615 return false; 6616 if (!(other_ instanceof AddedItemSubDetailComponent)) 6617 return false; 6618 AddedItemSubDetailComponent o = (AddedItemSubDetailComponent) other_; 6619 return compareDeep(traceNumber, o.traceNumber, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 6620 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 6621 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 6622 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(noteNumber, o.noteNumber, true) 6623 && compareDeep(reviewOutcome, o.reviewOutcome, true) && compareDeep(adjudication, o.adjudication, true) 6624 ; 6625 } 6626 6627 @Override 6628 public boolean equalsShallow(Base other_) { 6629 if (!super.equalsShallow(other_)) 6630 return false; 6631 if (!(other_ instanceof AddedItemSubDetailComponent)) 6632 return false; 6633 AddedItemSubDetailComponent o = (AddedItemSubDetailComponent) other_; 6634 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 6635 } 6636 6637 public boolean isEmpty() { 6638 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(traceNumber, revenue, productOrService 6639 , productOrServiceEnd, modifier, quantity, unitPrice, factor, tax, net, noteNumber 6640 , reviewOutcome, adjudication); 6641 } 6642 6643 public String fhirType() { 6644 return "ClaimResponse.addItem.detail.subDetail"; 6645 6646 } 6647 6648 } 6649 6650 @Block() 6651 public static class TotalComponent extends BackboneElement implements IBaseBackboneElement { 6652 /** 6653 * A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item. 6654 */ 6655 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 6656 @Description(shortDefinition="Type of adjudication information", formalDefinition="A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item." ) 6657 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 6658 protected CodeableConcept category; 6659 6660 /** 6661 * Monetary total amount associated with the category. 6662 */ 6663 @Child(name = "amount", type = {Money.class}, order=2, min=1, max=1, modifier=false, summary=true) 6664 @Description(shortDefinition="Financial total for the category", formalDefinition="Monetary total amount associated with the category." ) 6665 protected Money amount; 6666 6667 private static final long serialVersionUID = 2012310309L; 6668 6669 /** 6670 * Constructor 6671 */ 6672 public TotalComponent() { 6673 super(); 6674 } 6675 6676 /** 6677 * Constructor 6678 */ 6679 public TotalComponent(CodeableConcept category, Money amount) { 6680 super(); 6681 this.setCategory(category); 6682 this.setAmount(amount); 6683 } 6684 6685 /** 6686 * @return {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 6687 */ 6688 public CodeableConcept getCategory() { 6689 if (this.category == null) 6690 if (Configuration.errorOnAutoCreate()) 6691 throw new Error("Attempt to auto-create TotalComponent.category"); 6692 else if (Configuration.doAutoCreate()) 6693 this.category = new CodeableConcept(); // cc 6694 return this.category; 6695 } 6696 6697 public boolean hasCategory() { 6698 return this.category != null && !this.category.isEmpty(); 6699 } 6700 6701 /** 6702 * @param value {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 6703 */ 6704 public TotalComponent setCategory(CodeableConcept value) { 6705 this.category = value; 6706 return this; 6707 } 6708 6709 /** 6710 * @return {@link #amount} (Monetary total amount associated with the category.) 6711 */ 6712 public Money getAmount() { 6713 if (this.amount == null) 6714 if (Configuration.errorOnAutoCreate()) 6715 throw new Error("Attempt to auto-create TotalComponent.amount"); 6716 else if (Configuration.doAutoCreate()) 6717 this.amount = new Money(); // cc 6718 return this.amount; 6719 } 6720 6721 public boolean hasAmount() { 6722 return this.amount != null && !this.amount.isEmpty(); 6723 } 6724 6725 /** 6726 * @param value {@link #amount} (Monetary total amount associated with the category.) 6727 */ 6728 public TotalComponent setAmount(Money value) { 6729 this.amount = value; 6730 return this; 6731 } 6732 6733 protected void listChildren(List<Property> children) { 6734 super.listChildren(children); 6735 children.add(new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category)); 6736 children.add(new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount)); 6737 } 6738 6739 @Override 6740 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6741 switch (_hash) { 6742 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category); 6743 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount); 6744 default: return super.getNamedProperty(_hash, _name, _checkValid); 6745 } 6746 6747 } 6748 6749 @Override 6750 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6751 switch (hash) { 6752 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6753 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 6754 default: return super.getProperty(hash, name, checkValid); 6755 } 6756 6757 } 6758 6759 @Override 6760 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6761 switch (hash) { 6762 case 50511102: // category 6763 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6764 return value; 6765 case -1413853096: // amount 6766 this.amount = TypeConvertor.castToMoney(value); // Money 6767 return value; 6768 default: return super.setProperty(hash, name, value); 6769 } 6770 6771 } 6772 6773 @Override 6774 public Base setProperty(String name, Base value) throws FHIRException { 6775 if (name.equals("category")) { 6776 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6777 } else if (name.equals("amount")) { 6778 this.amount = TypeConvertor.castToMoney(value); // Money 6779 } else 6780 return super.setProperty(name, value); 6781 return value; 6782 } 6783 6784 @Override 6785 public void removeChild(String name, Base value) throws FHIRException { 6786 if (name.equals("category")) { 6787 this.category = null; 6788 } else if (name.equals("amount")) { 6789 this.amount = null; 6790 } else 6791 super.removeChild(name, value); 6792 6793 } 6794 6795 @Override 6796 public Base makeProperty(int hash, String name) throws FHIRException { 6797 switch (hash) { 6798 case 50511102: return getCategory(); 6799 case -1413853096: return getAmount(); 6800 default: return super.makeProperty(hash, name); 6801 } 6802 6803 } 6804 6805 @Override 6806 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6807 switch (hash) { 6808 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6809 case -1413853096: /*amount*/ return new String[] {"Money"}; 6810 default: return super.getTypesForProperty(hash, name); 6811 } 6812 6813 } 6814 6815 @Override 6816 public Base addChild(String name) throws FHIRException { 6817 if (name.equals("category")) { 6818 this.category = new CodeableConcept(); 6819 return this.category; 6820 } 6821 else if (name.equals("amount")) { 6822 this.amount = new Money(); 6823 return this.amount; 6824 } 6825 else 6826 return super.addChild(name); 6827 } 6828 6829 public TotalComponent copy() { 6830 TotalComponent dst = new TotalComponent(); 6831 copyValues(dst); 6832 return dst; 6833 } 6834 6835 public void copyValues(TotalComponent dst) { 6836 super.copyValues(dst); 6837 dst.category = category == null ? null : category.copy(); 6838 dst.amount = amount == null ? null : amount.copy(); 6839 } 6840 6841 @Override 6842 public boolean equalsDeep(Base other_) { 6843 if (!super.equalsDeep(other_)) 6844 return false; 6845 if (!(other_ instanceof TotalComponent)) 6846 return false; 6847 TotalComponent o = (TotalComponent) other_; 6848 return compareDeep(category, o.category, true) && compareDeep(amount, o.amount, true); 6849 } 6850 6851 @Override 6852 public boolean equalsShallow(Base other_) { 6853 if (!super.equalsShallow(other_)) 6854 return false; 6855 if (!(other_ instanceof TotalComponent)) 6856 return false; 6857 TotalComponent o = (TotalComponent) other_; 6858 return true; 6859 } 6860 6861 public boolean isEmpty() { 6862 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, amount); 6863 } 6864 6865 public String fhirType() { 6866 return "ClaimResponse.total"; 6867 6868 } 6869 6870 } 6871 6872 @Block() 6873 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 6874 /** 6875 * Whether this represents partial or complete payment of the benefits payable. 6876 */ 6877 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 6878 @Description(shortDefinition="Partial or complete payment", formalDefinition="Whether this represents partial or complete payment of the benefits payable." ) 6879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-paymenttype") 6880 protected CodeableConcept type; 6881 6882 /** 6883 * Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication. 6884 */ 6885 @Child(name = "adjustment", type = {Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 6886 @Description(shortDefinition="Payment adjustment for non-claim issues", formalDefinition="Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication." ) 6887 protected Money adjustment; 6888 6889 /** 6890 * Reason for the payment adjustment. 6891 */ 6892 @Child(name = "adjustmentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6893 @Description(shortDefinition="Explanation for the adjustment", formalDefinition="Reason for the payment adjustment." ) 6894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 6895 protected CodeableConcept adjustmentReason; 6896 6897 /** 6898 * Estimated date the payment will be issued or the actual issue date of payment. 6899 */ 6900 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 6901 @Description(shortDefinition="Expected date of payment", formalDefinition="Estimated date the payment will be issued or the actual issue date of payment." ) 6902 protected DateType date; 6903 6904 /** 6905 * Benefits payable less any payment adjustment. 6906 */ 6907 @Child(name = "amount", type = {Money.class}, order=5, min=1, max=1, modifier=false, summary=false) 6908 @Description(shortDefinition="Payable amount after adjustment", formalDefinition="Benefits payable less any payment adjustment." ) 6909 protected Money amount; 6910 6911 /** 6912 * Issuer's unique identifier for the payment instrument. 6913 */ 6914 @Child(name = "identifier", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 6915 @Description(shortDefinition="Business identifier for the payment", formalDefinition="Issuer's unique identifier for the payment instrument." ) 6916 protected Identifier identifier; 6917 6918 private static final long serialVersionUID = 1539906026L; 6919 6920 /** 6921 * Constructor 6922 */ 6923 public PaymentComponent() { 6924 super(); 6925 } 6926 6927 /** 6928 * Constructor 6929 */ 6930 public PaymentComponent(CodeableConcept type, Money amount) { 6931 super(); 6932 this.setType(type); 6933 this.setAmount(amount); 6934 } 6935 6936 /** 6937 * @return {@link #type} (Whether this represents partial or complete payment of the benefits payable.) 6938 */ 6939 public CodeableConcept getType() { 6940 if (this.type == null) 6941 if (Configuration.errorOnAutoCreate()) 6942 throw new Error("Attempt to auto-create PaymentComponent.type"); 6943 else if (Configuration.doAutoCreate()) 6944 this.type = new CodeableConcept(); // cc 6945 return this.type; 6946 } 6947 6948 public boolean hasType() { 6949 return this.type != null && !this.type.isEmpty(); 6950 } 6951 6952 /** 6953 * @param value {@link #type} (Whether this represents partial or complete payment of the benefits payable.) 6954 */ 6955 public PaymentComponent setType(CodeableConcept value) { 6956 this.type = value; 6957 return this; 6958 } 6959 6960 /** 6961 * @return {@link #adjustment} (Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.) 6962 */ 6963 public Money getAdjustment() { 6964 if (this.adjustment == null) 6965 if (Configuration.errorOnAutoCreate()) 6966 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 6967 else if (Configuration.doAutoCreate()) 6968 this.adjustment = new Money(); // cc 6969 return this.adjustment; 6970 } 6971 6972 public boolean hasAdjustment() { 6973 return this.adjustment != null && !this.adjustment.isEmpty(); 6974 } 6975 6976 /** 6977 * @param value {@link #adjustment} (Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.) 6978 */ 6979 public PaymentComponent setAdjustment(Money value) { 6980 this.adjustment = value; 6981 return this; 6982 } 6983 6984 /** 6985 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 6986 */ 6987 public CodeableConcept getAdjustmentReason() { 6988 if (this.adjustmentReason == null) 6989 if (Configuration.errorOnAutoCreate()) 6990 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 6991 else if (Configuration.doAutoCreate()) 6992 this.adjustmentReason = new CodeableConcept(); // cc 6993 return this.adjustmentReason; 6994 } 6995 6996 public boolean hasAdjustmentReason() { 6997 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 6998 } 6999 7000 /** 7001 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 7002 */ 7003 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 7004 this.adjustmentReason = value; 7005 return this; 7006 } 7007 7008 /** 7009 * @return {@link #date} (Estimated date the payment will be issued or the actual issue date of payment.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 7010 */ 7011 public DateType getDateElement() { 7012 if (this.date == null) 7013 if (Configuration.errorOnAutoCreate()) 7014 throw new Error("Attempt to auto-create PaymentComponent.date"); 7015 else if (Configuration.doAutoCreate()) 7016 this.date = new DateType(); // bb 7017 return this.date; 7018 } 7019 7020 public boolean hasDateElement() { 7021 return this.date != null && !this.date.isEmpty(); 7022 } 7023 7024 public boolean hasDate() { 7025 return this.date != null && !this.date.isEmpty(); 7026 } 7027 7028 /** 7029 * @param value {@link #date} (Estimated date the payment will be issued or the actual issue date of payment.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 7030 */ 7031 public PaymentComponent setDateElement(DateType value) { 7032 this.date = value; 7033 return this; 7034 } 7035 7036 /** 7037 * @return Estimated date the payment will be issued or the actual issue date of payment. 7038 */ 7039 public Date getDate() { 7040 return this.date == null ? null : this.date.getValue(); 7041 } 7042 7043 /** 7044 * @param value Estimated date the payment will be issued or the actual issue date of payment. 7045 */ 7046 public PaymentComponent setDate(Date value) { 7047 if (value == null) 7048 this.date = null; 7049 else { 7050 if (this.date == null) 7051 this.date = new DateType(); 7052 this.date.setValue(value); 7053 } 7054 return this; 7055 } 7056 7057 /** 7058 * @return {@link #amount} (Benefits payable less any payment adjustment.) 7059 */ 7060 public Money getAmount() { 7061 if (this.amount == null) 7062 if (Configuration.errorOnAutoCreate()) 7063 throw new Error("Attempt to auto-create PaymentComponent.amount"); 7064 else if (Configuration.doAutoCreate()) 7065 this.amount = new Money(); // cc 7066 return this.amount; 7067 } 7068 7069 public boolean hasAmount() { 7070 return this.amount != null && !this.amount.isEmpty(); 7071 } 7072 7073 /** 7074 * @param value {@link #amount} (Benefits payable less any payment adjustment.) 7075 */ 7076 public PaymentComponent setAmount(Money value) { 7077 this.amount = value; 7078 return this; 7079 } 7080 7081 /** 7082 * @return {@link #identifier} (Issuer's unique identifier for the payment instrument.) 7083 */ 7084 public Identifier getIdentifier() { 7085 if (this.identifier == null) 7086 if (Configuration.errorOnAutoCreate()) 7087 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 7088 else if (Configuration.doAutoCreate()) 7089 this.identifier = new Identifier(); // cc 7090 return this.identifier; 7091 } 7092 7093 public boolean hasIdentifier() { 7094 return this.identifier != null && !this.identifier.isEmpty(); 7095 } 7096 7097 /** 7098 * @param value {@link #identifier} (Issuer's unique identifier for the payment instrument.) 7099 */ 7100 public PaymentComponent setIdentifier(Identifier value) { 7101 this.identifier = value; 7102 return this; 7103 } 7104 7105 protected void listChildren(List<Property> children) { 7106 super.listChildren(children); 7107 children.add(new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type)); 7108 children.add(new Property("adjustment", "Money", "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 0, 1, adjustment)); 7109 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason)); 7110 children.add(new Property("date", "date", "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date)); 7111 children.add(new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount)); 7112 children.add(new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, identifier)); 7113 } 7114 7115 @Override 7116 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7117 switch (_hash) { 7118 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type); 7119 case 1977085293: /*adjustment*/ return new Property("adjustment", "Money", "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 0, 1, adjustment); 7120 case -1255938543: /*adjustmentReason*/ return new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason); 7121 case 3076014: /*date*/ return new Property("date", "date", "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date); 7122 case -1413853096: /*amount*/ return new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount); 7123 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, identifier); 7124 default: return super.getNamedProperty(_hash, _name, _checkValid); 7125 } 7126 7127 } 7128 7129 @Override 7130 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7131 switch (hash) { 7132 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 7133 case 1977085293: /*adjustment*/ return this.adjustment == null ? new Base[0] : new Base[] {this.adjustment}; // Money 7134 case -1255938543: /*adjustmentReason*/ return this.adjustmentReason == null ? new Base[0] : new Base[] {this.adjustmentReason}; // CodeableConcept 7135 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 7136 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 7137 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 7138 default: return super.getProperty(hash, name, checkValid); 7139 } 7140 7141 } 7142 7143 @Override 7144 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7145 switch (hash) { 7146 case 3575610: // type 7147 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7148 return value; 7149 case 1977085293: // adjustment 7150 this.adjustment = TypeConvertor.castToMoney(value); // Money 7151 return value; 7152 case -1255938543: // adjustmentReason 7153 this.adjustmentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7154 return value; 7155 case 3076014: // date 7156 this.date = TypeConvertor.castToDate(value); // DateType 7157 return value; 7158 case -1413853096: // amount 7159 this.amount = TypeConvertor.castToMoney(value); // Money 7160 return value; 7161 case -1618432855: // identifier 7162 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 7163 return value; 7164 default: return super.setProperty(hash, name, value); 7165 } 7166 7167 } 7168 7169 @Override 7170 public Base setProperty(String name, Base value) throws FHIRException { 7171 if (name.equals("type")) { 7172 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7173 } else if (name.equals("adjustment")) { 7174 this.adjustment = TypeConvertor.castToMoney(value); // Money 7175 } else if (name.equals("adjustmentReason")) { 7176 this.adjustmentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7177 } else if (name.equals("date")) { 7178 this.date = TypeConvertor.castToDate(value); // DateType 7179 } else if (name.equals("amount")) { 7180 this.amount = TypeConvertor.castToMoney(value); // Money 7181 } else if (name.equals("identifier")) { 7182 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 7183 } else 7184 return super.setProperty(name, value); 7185 return value; 7186 } 7187 7188 @Override 7189 public void removeChild(String name, Base value) throws FHIRException { 7190 if (name.equals("type")) { 7191 this.type = null; 7192 } else if (name.equals("adjustment")) { 7193 this.adjustment = null; 7194 } else if (name.equals("adjustmentReason")) { 7195 this.adjustmentReason = null; 7196 } else if (name.equals("date")) { 7197 this.date = null; 7198 } else if (name.equals("amount")) { 7199 this.amount = null; 7200 } else if (name.equals("identifier")) { 7201 this.identifier = null; 7202 } else 7203 super.removeChild(name, value); 7204 7205 } 7206 7207 @Override 7208 public Base makeProperty(int hash, String name) throws FHIRException { 7209 switch (hash) { 7210 case 3575610: return getType(); 7211 case 1977085293: return getAdjustment(); 7212 case -1255938543: return getAdjustmentReason(); 7213 case 3076014: return getDateElement(); 7214 case -1413853096: return getAmount(); 7215 case -1618432855: return getIdentifier(); 7216 default: return super.makeProperty(hash, name); 7217 } 7218 7219 } 7220 7221 @Override 7222 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7223 switch (hash) { 7224 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 7225 case 1977085293: /*adjustment*/ return new String[] {"Money"}; 7226 case -1255938543: /*adjustmentReason*/ return new String[] {"CodeableConcept"}; 7227 case 3076014: /*date*/ return new String[] {"date"}; 7228 case -1413853096: /*amount*/ return new String[] {"Money"}; 7229 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 7230 default: return super.getTypesForProperty(hash, name); 7231 } 7232 7233 } 7234 7235 @Override 7236 public Base addChild(String name) throws FHIRException { 7237 if (name.equals("type")) { 7238 this.type = new CodeableConcept(); 7239 return this.type; 7240 } 7241 else if (name.equals("adjustment")) { 7242 this.adjustment = new Money(); 7243 return this.adjustment; 7244 } 7245 else if (name.equals("adjustmentReason")) { 7246 this.adjustmentReason = new CodeableConcept(); 7247 return this.adjustmentReason; 7248 } 7249 else if (name.equals("date")) { 7250 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.payment.date"); 7251 } 7252 else if (name.equals("amount")) { 7253 this.amount = new Money(); 7254 return this.amount; 7255 } 7256 else if (name.equals("identifier")) { 7257 this.identifier = new Identifier(); 7258 return this.identifier; 7259 } 7260 else 7261 return super.addChild(name); 7262 } 7263 7264 public PaymentComponent copy() { 7265 PaymentComponent dst = new PaymentComponent(); 7266 copyValues(dst); 7267 return dst; 7268 } 7269 7270 public void copyValues(PaymentComponent dst) { 7271 super.copyValues(dst); 7272 dst.type = type == null ? null : type.copy(); 7273 dst.adjustment = adjustment == null ? null : adjustment.copy(); 7274 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 7275 dst.date = date == null ? null : date.copy(); 7276 dst.amount = amount == null ? null : amount.copy(); 7277 dst.identifier = identifier == null ? null : identifier.copy(); 7278 } 7279 7280 @Override 7281 public boolean equalsDeep(Base other_) { 7282 if (!super.equalsDeep(other_)) 7283 return false; 7284 if (!(other_ instanceof PaymentComponent)) 7285 return false; 7286 PaymentComponent o = (PaymentComponent) other_; 7287 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) && compareDeep(adjustmentReason, o.adjustmentReason, true) 7288 && compareDeep(date, o.date, true) && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true) 7289 ; 7290 } 7291 7292 @Override 7293 public boolean equalsShallow(Base other_) { 7294 if (!super.equalsShallow(other_)) 7295 return false; 7296 if (!(other_ instanceof PaymentComponent)) 7297 return false; 7298 PaymentComponent o = (PaymentComponent) other_; 7299 return compareValues(date, o.date, true); 7300 } 7301 7302 public boolean isEmpty() { 7303 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason 7304 , date, amount, identifier); 7305 } 7306 7307 public String fhirType() { 7308 return "ClaimResponse.payment"; 7309 7310 } 7311 7312 } 7313 7314 @Block() 7315 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 7316 /** 7317 * A number to uniquely identify a note entry. 7318 */ 7319 @Child(name = "number", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 7320 @Description(shortDefinition="Note instance identifier", formalDefinition="A number to uniquely identify a note entry." ) 7321 protected PositiveIntType number; 7322 7323 /** 7324 * The business purpose of the note text. 7325 */ 7326 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 7327 @Description(shortDefinition="Note purpose", formalDefinition="The business purpose of the note text." ) 7328 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 7329 protected CodeableConcept type; 7330 7331 /** 7332 * The explanation or description associated with the processing. 7333 */ 7334 @Child(name = "text", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 7335 @Description(shortDefinition="Note explanatory text", formalDefinition="The explanation or description associated with the processing." ) 7336 protected StringType text; 7337 7338 /** 7339 * A code to define the language used in the text of the note. 7340 */ 7341 @Child(name = "language", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 7342 @Description(shortDefinition="Language of the text", formalDefinition="A code to define the language used in the text of the note." ) 7343 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 7344 protected CodeableConcept language; 7345 7346 private static final long serialVersionUID = -944255449L; 7347 7348 /** 7349 * Constructor 7350 */ 7351 public NoteComponent() { 7352 super(); 7353 } 7354 7355 /** 7356 * Constructor 7357 */ 7358 public NoteComponent(String text) { 7359 super(); 7360 this.setText(text); 7361 } 7362 7363 /** 7364 * @return {@link #number} (A number to uniquely identify a note entry.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 7365 */ 7366 public PositiveIntType getNumberElement() { 7367 if (this.number == null) 7368 if (Configuration.errorOnAutoCreate()) 7369 throw new Error("Attempt to auto-create NoteComponent.number"); 7370 else if (Configuration.doAutoCreate()) 7371 this.number = new PositiveIntType(); // bb 7372 return this.number; 7373 } 7374 7375 public boolean hasNumberElement() { 7376 return this.number != null && !this.number.isEmpty(); 7377 } 7378 7379 public boolean hasNumber() { 7380 return this.number != null && !this.number.isEmpty(); 7381 } 7382 7383 /** 7384 * @param value {@link #number} (A number to uniquely identify a note entry.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 7385 */ 7386 public NoteComponent setNumberElement(PositiveIntType value) { 7387 this.number = value; 7388 return this; 7389 } 7390 7391 /** 7392 * @return A number to uniquely identify a note entry. 7393 */ 7394 public int getNumber() { 7395 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 7396 } 7397 7398 /** 7399 * @param value A number to uniquely identify a note entry. 7400 */ 7401 public NoteComponent setNumber(int value) { 7402 if (this.number == null) 7403 this.number = new PositiveIntType(); 7404 this.number.setValue(value); 7405 return this; 7406 } 7407 7408 /** 7409 * @return {@link #type} (The business purpose of the note text.) 7410 */ 7411 public CodeableConcept getType() { 7412 if (this.type == null) 7413 if (Configuration.errorOnAutoCreate()) 7414 throw new Error("Attempt to auto-create NoteComponent.type"); 7415 else if (Configuration.doAutoCreate()) 7416 this.type = new CodeableConcept(); // cc 7417 return this.type; 7418 } 7419 7420 public boolean hasType() { 7421 return this.type != null && !this.type.isEmpty(); 7422 } 7423 7424 /** 7425 * @param value {@link #type} (The business purpose of the note text.) 7426 */ 7427 public NoteComponent setType(CodeableConcept value) { 7428 this.type = value; 7429 return this; 7430 } 7431 7432 /** 7433 * @return {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 7434 */ 7435 public StringType getTextElement() { 7436 if (this.text == null) 7437 if (Configuration.errorOnAutoCreate()) 7438 throw new Error("Attempt to auto-create NoteComponent.text"); 7439 else if (Configuration.doAutoCreate()) 7440 this.text = new StringType(); // bb 7441 return this.text; 7442 } 7443 7444 public boolean hasTextElement() { 7445 return this.text != null && !this.text.isEmpty(); 7446 } 7447 7448 public boolean hasText() { 7449 return this.text != null && !this.text.isEmpty(); 7450 } 7451 7452 /** 7453 * @param value {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 7454 */ 7455 public NoteComponent setTextElement(StringType value) { 7456 this.text = value; 7457 return this; 7458 } 7459 7460 /** 7461 * @return The explanation or description associated with the processing. 7462 */ 7463 public String getText() { 7464 return this.text == null ? null : this.text.getValue(); 7465 } 7466 7467 /** 7468 * @param value The explanation or description associated with the processing. 7469 */ 7470 public NoteComponent setText(String value) { 7471 if (this.text == null) 7472 this.text = new StringType(); 7473 this.text.setValue(value); 7474 return this; 7475 } 7476 7477 /** 7478 * @return {@link #language} (A code to define the language used in the text of the note.) 7479 */ 7480 public CodeableConcept getLanguage() { 7481 if (this.language == null) 7482 if (Configuration.errorOnAutoCreate()) 7483 throw new Error("Attempt to auto-create NoteComponent.language"); 7484 else if (Configuration.doAutoCreate()) 7485 this.language = new CodeableConcept(); // cc 7486 return this.language; 7487 } 7488 7489 public boolean hasLanguage() { 7490 return this.language != null && !this.language.isEmpty(); 7491 } 7492 7493 /** 7494 * @param value {@link #language} (A code to define the language used in the text of the note.) 7495 */ 7496 public NoteComponent setLanguage(CodeableConcept value) { 7497 this.language = value; 7498 return this; 7499 } 7500 7501 protected void listChildren(List<Property> children) { 7502 super.listChildren(children); 7503 children.add(new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number)); 7504 children.add(new Property("type", "CodeableConcept", "The business purpose of the note text.", 0, 1, type)); 7505 children.add(new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 7506 children.add(new Property("language", "CodeableConcept", "A code to define the language used in the text of the note.", 0, 1, language)); 7507 } 7508 7509 @Override 7510 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7511 switch (_hash) { 7512 case -1034364087: /*number*/ return new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number); 7513 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The business purpose of the note text.", 0, 1, type); 7514 case 3556653: /*text*/ return new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text); 7515 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "A code to define the language used in the text of the note.", 0, 1, language); 7516 default: return super.getNamedProperty(_hash, _name, _checkValid); 7517 } 7518 7519 } 7520 7521 @Override 7522 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7523 switch (hash) { 7524 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // PositiveIntType 7525 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 7526 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 7527 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 7528 default: return super.getProperty(hash, name, checkValid); 7529 } 7530 7531 } 7532 7533 @Override 7534 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7535 switch (hash) { 7536 case -1034364087: // number 7537 this.number = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7538 return value; 7539 case 3575610: // type 7540 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7541 return value; 7542 case 3556653: // text 7543 this.text = TypeConvertor.castToString(value); // StringType 7544 return value; 7545 case -1613589672: // language 7546 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7547 return value; 7548 default: return super.setProperty(hash, name, value); 7549 } 7550 7551 } 7552 7553 @Override 7554 public Base setProperty(String name, Base value) throws FHIRException { 7555 if (name.equals("number")) { 7556 this.number = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7557 } else if (name.equals("type")) { 7558 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7559 } else if (name.equals("text")) { 7560 this.text = TypeConvertor.castToString(value); // StringType 7561 } else if (name.equals("language")) { 7562 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7563 } else 7564 return super.setProperty(name, value); 7565 return value; 7566 } 7567 7568 @Override 7569 public void removeChild(String name, Base value) throws FHIRException { 7570 if (name.equals("number")) { 7571 this.number = null; 7572 } else if (name.equals("type")) { 7573 this.type = null; 7574 } else if (name.equals("text")) { 7575 this.text = null; 7576 } else if (name.equals("language")) { 7577 this.language = null; 7578 } else 7579 super.removeChild(name, value); 7580 7581 } 7582 7583 @Override 7584 public Base makeProperty(int hash, String name) throws FHIRException { 7585 switch (hash) { 7586 case -1034364087: return getNumberElement(); 7587 case 3575610: return getType(); 7588 case 3556653: return getTextElement(); 7589 case -1613589672: return getLanguage(); 7590 default: return super.makeProperty(hash, name); 7591 } 7592 7593 } 7594 7595 @Override 7596 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7597 switch (hash) { 7598 case -1034364087: /*number*/ return new String[] {"positiveInt"}; 7599 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 7600 case 3556653: /*text*/ return new String[] {"string"}; 7601 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 7602 default: return super.getTypesForProperty(hash, name); 7603 } 7604 7605 } 7606 7607 @Override 7608 public Base addChild(String name) throws FHIRException { 7609 if (name.equals("number")) { 7610 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.processNote.number"); 7611 } 7612 else if (name.equals("type")) { 7613 this.type = new CodeableConcept(); 7614 return this.type; 7615 } 7616 else if (name.equals("text")) { 7617 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.processNote.text"); 7618 } 7619 else if (name.equals("language")) { 7620 this.language = new CodeableConcept(); 7621 return this.language; 7622 } 7623 else 7624 return super.addChild(name); 7625 } 7626 7627 public NoteComponent copy() { 7628 NoteComponent dst = new NoteComponent(); 7629 copyValues(dst); 7630 return dst; 7631 } 7632 7633 public void copyValues(NoteComponent dst) { 7634 super.copyValues(dst); 7635 dst.number = number == null ? null : number.copy(); 7636 dst.type = type == null ? null : type.copy(); 7637 dst.text = text == null ? null : text.copy(); 7638 dst.language = language == null ? null : language.copy(); 7639 } 7640 7641 @Override 7642 public boolean equalsDeep(Base other_) { 7643 if (!super.equalsDeep(other_)) 7644 return false; 7645 if (!(other_ instanceof NoteComponent)) 7646 return false; 7647 NoteComponent o = (NoteComponent) other_; 7648 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 7649 && compareDeep(language, o.language, true); 7650 } 7651 7652 @Override 7653 public boolean equalsShallow(Base other_) { 7654 if (!super.equalsShallow(other_)) 7655 return false; 7656 if (!(other_ instanceof NoteComponent)) 7657 return false; 7658 NoteComponent o = (NoteComponent) other_; 7659 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 7660 } 7661 7662 public boolean isEmpty() { 7663 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language 7664 ); 7665 } 7666 7667 public String fhirType() { 7668 return "ClaimResponse.processNote"; 7669 7670 } 7671 7672 } 7673 7674 @Block() 7675 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 7676 /** 7677 * A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 7678 */ 7679 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 7680 @Description(shortDefinition="Insurance instance identifier", formalDefinition="A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order." ) 7681 protected PositiveIntType sequence; 7682 7683 /** 7684 * A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 7685 */ 7686 @Child(name = "focal", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 7687 @Description(shortDefinition="Coverage to be used for adjudication", formalDefinition="A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true." ) 7688 protected BooleanType focal; 7689 7690 /** 7691 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 7692 */ 7693 @Child(name = "coverage", type = {Coverage.class}, order=3, min=1, max=1, modifier=false, summary=false) 7694 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 7695 protected Reference coverage; 7696 7697 /** 7698 * A business agreement number established between the provider and the insurer for special business processing purposes. 7699 */ 7700 @Child(name = "businessArrangement", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 7701 @Description(shortDefinition="Additional provider contract number", formalDefinition="A business agreement number established between the provider and the insurer for special business processing purposes." ) 7702 protected StringType businessArrangement; 7703 7704 /** 7705 * The result of the adjudication of the line items for the Coverage specified in this insurance. 7706 */ 7707 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=5, min=0, max=1, modifier=false, summary=false) 7708 @Description(shortDefinition="Adjudication results", formalDefinition="The result of the adjudication of the line items for the Coverage specified in this insurance." ) 7709 protected Reference claimResponse; 7710 7711 private static final long serialVersionUID = -218152446L; 7712 7713 /** 7714 * Constructor 7715 */ 7716 public InsuranceComponent() { 7717 super(); 7718 } 7719 7720 /** 7721 * Constructor 7722 */ 7723 public InsuranceComponent(int sequence, boolean focal, Reference coverage) { 7724 super(); 7725 this.setSequence(sequence); 7726 this.setFocal(focal); 7727 this.setCoverage(coverage); 7728 } 7729 7730 /** 7731 * @return {@link #sequence} (A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 7732 */ 7733 public PositiveIntType getSequenceElement() { 7734 if (this.sequence == null) 7735 if (Configuration.errorOnAutoCreate()) 7736 throw new Error("Attempt to auto-create InsuranceComponent.sequence"); 7737 else if (Configuration.doAutoCreate()) 7738 this.sequence = new PositiveIntType(); // bb 7739 return this.sequence; 7740 } 7741 7742 public boolean hasSequenceElement() { 7743 return this.sequence != null && !this.sequence.isEmpty(); 7744 } 7745 7746 public boolean hasSequence() { 7747 return this.sequence != null && !this.sequence.isEmpty(); 7748 } 7749 7750 /** 7751 * @param value {@link #sequence} (A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 7752 */ 7753 public InsuranceComponent setSequenceElement(PositiveIntType value) { 7754 this.sequence = value; 7755 return this; 7756 } 7757 7758 /** 7759 * @return A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 7760 */ 7761 public int getSequence() { 7762 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7763 } 7764 7765 /** 7766 * @param value A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order. 7767 */ 7768 public InsuranceComponent setSequence(int value) { 7769 if (this.sequence == null) 7770 this.sequence = new PositiveIntType(); 7771 this.sequence.setValue(value); 7772 return this; 7773 } 7774 7775 /** 7776 * @return {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 7777 */ 7778 public BooleanType getFocalElement() { 7779 if (this.focal == null) 7780 if (Configuration.errorOnAutoCreate()) 7781 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 7782 else if (Configuration.doAutoCreate()) 7783 this.focal = new BooleanType(); // bb 7784 return this.focal; 7785 } 7786 7787 public boolean hasFocalElement() { 7788 return this.focal != null && !this.focal.isEmpty(); 7789 } 7790 7791 public boolean hasFocal() { 7792 return this.focal != null && !this.focal.isEmpty(); 7793 } 7794 7795 /** 7796 * @param value {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 7797 */ 7798 public InsuranceComponent setFocalElement(BooleanType value) { 7799 this.focal = value; 7800 return this; 7801 } 7802 7803 /** 7804 * @return A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 7805 */ 7806 public boolean getFocal() { 7807 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 7808 } 7809 7810 /** 7811 * @param value A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 7812 */ 7813 public InsuranceComponent setFocal(boolean value) { 7814 if (this.focal == null) 7815 this.focal = new BooleanType(); 7816 this.focal.setValue(value); 7817 return this; 7818 } 7819 7820 /** 7821 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 7822 */ 7823 public Reference getCoverage() { 7824 if (this.coverage == null) 7825 if (Configuration.errorOnAutoCreate()) 7826 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 7827 else if (Configuration.doAutoCreate()) 7828 this.coverage = new Reference(); // cc 7829 return this.coverage; 7830 } 7831 7832 public boolean hasCoverage() { 7833 return this.coverage != null && !this.coverage.isEmpty(); 7834 } 7835 7836 /** 7837 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 7838 */ 7839 public InsuranceComponent setCoverage(Reference value) { 7840 this.coverage = value; 7841 return this; 7842 } 7843 7844 /** 7845 * @return {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 7846 */ 7847 public StringType getBusinessArrangementElement() { 7848 if (this.businessArrangement == null) 7849 if (Configuration.errorOnAutoCreate()) 7850 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 7851 else if (Configuration.doAutoCreate()) 7852 this.businessArrangement = new StringType(); // bb 7853 return this.businessArrangement; 7854 } 7855 7856 public boolean hasBusinessArrangementElement() { 7857 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 7858 } 7859 7860 public boolean hasBusinessArrangement() { 7861 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 7862 } 7863 7864 /** 7865 * @param value {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 7866 */ 7867 public InsuranceComponent setBusinessArrangementElement(StringType value) { 7868 this.businessArrangement = value; 7869 return this; 7870 } 7871 7872 /** 7873 * @return A business agreement number established between the provider and the insurer for special business processing purposes. 7874 */ 7875 public String getBusinessArrangement() { 7876 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 7877 } 7878 7879 /** 7880 * @param value A business agreement number established between the provider and the insurer for special business processing purposes. 7881 */ 7882 public InsuranceComponent setBusinessArrangement(String value) { 7883 if (Utilities.noString(value)) 7884 this.businessArrangement = null; 7885 else { 7886 if (this.businessArrangement == null) 7887 this.businessArrangement = new StringType(); 7888 this.businessArrangement.setValue(value); 7889 } 7890 return this; 7891 } 7892 7893 /** 7894 * @return {@link #claimResponse} (The result of the adjudication of the line items for the Coverage specified in this insurance.) 7895 */ 7896 public Reference getClaimResponse() { 7897 if (this.claimResponse == null) 7898 if (Configuration.errorOnAutoCreate()) 7899 throw new Error("Attempt to auto-create InsuranceComponent.claimResponse"); 7900 else if (Configuration.doAutoCreate()) 7901 this.claimResponse = new Reference(); // cc 7902 return this.claimResponse; 7903 } 7904 7905 public boolean hasClaimResponse() { 7906 return this.claimResponse != null && !this.claimResponse.isEmpty(); 7907 } 7908 7909 /** 7910 * @param value {@link #claimResponse} (The result of the adjudication of the line items for the Coverage specified in this insurance.) 7911 */ 7912 public InsuranceComponent setClaimResponse(Reference value) { 7913 this.claimResponse = value; 7914 return this; 7915 } 7916 7917 protected void listChildren(List<Property> children) { 7918 super.listChildren(children); 7919 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 0, 1, sequence)); 7920 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal)); 7921 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 7922 children.add(new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement)); 7923 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, claimResponse)); 7924 } 7925 7926 @Override 7927 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7928 switch (_hash) { 7929 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify insurance entries and provide a sequence of coverages to convey coordination of benefit order.", 0, 1, sequence); 7930 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal); 7931 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 7932 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement); 7933 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The result of the adjudication of the line items for the Coverage specified in this insurance.", 0, 1, claimResponse); 7934 default: return super.getNamedProperty(_hash, _name, _checkValid); 7935 } 7936 7937 } 7938 7939 @Override 7940 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7941 switch (hash) { 7942 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 7943 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 7944 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 7945 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 7946 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 7947 default: return super.getProperty(hash, name, checkValid); 7948 } 7949 7950 } 7951 7952 @Override 7953 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7954 switch (hash) { 7955 case 1349547969: // sequence 7956 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7957 return value; 7958 case 97604197: // focal 7959 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 7960 return value; 7961 case -351767064: // coverage 7962 this.coverage = TypeConvertor.castToReference(value); // Reference 7963 return value; 7964 case 259920682: // businessArrangement 7965 this.businessArrangement = TypeConvertor.castToString(value); // StringType 7966 return value; 7967 case 689513629: // claimResponse 7968 this.claimResponse = TypeConvertor.castToReference(value); // Reference 7969 return value; 7970 default: return super.setProperty(hash, name, value); 7971 } 7972 7973 } 7974 7975 @Override 7976 public Base setProperty(String name, Base value) throws FHIRException { 7977 if (name.equals("sequence")) { 7978 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7979 } else if (name.equals("focal")) { 7980 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 7981 } else if (name.equals("coverage")) { 7982 this.coverage = TypeConvertor.castToReference(value); // Reference 7983 } else if (name.equals("businessArrangement")) { 7984 this.businessArrangement = TypeConvertor.castToString(value); // StringType 7985 } else if (name.equals("claimResponse")) { 7986 this.claimResponse = TypeConvertor.castToReference(value); // Reference 7987 } else 7988 return super.setProperty(name, value); 7989 return value; 7990 } 7991 7992 @Override 7993 public void removeChild(String name, Base value) throws FHIRException { 7994 if (name.equals("sequence")) { 7995 this.sequence = null; 7996 } else if (name.equals("focal")) { 7997 this.focal = null; 7998 } else if (name.equals("coverage")) { 7999 this.coverage = null; 8000 } else if (name.equals("businessArrangement")) { 8001 this.businessArrangement = null; 8002 } else if (name.equals("claimResponse")) { 8003 this.claimResponse = null; 8004 } else 8005 super.removeChild(name, value); 8006 8007 } 8008 8009 @Override 8010 public Base makeProperty(int hash, String name) throws FHIRException { 8011 switch (hash) { 8012 case 1349547969: return getSequenceElement(); 8013 case 97604197: return getFocalElement(); 8014 case -351767064: return getCoverage(); 8015 case 259920682: return getBusinessArrangementElement(); 8016 case 689513629: return getClaimResponse(); 8017 default: return super.makeProperty(hash, name); 8018 } 8019 8020 } 8021 8022 @Override 8023 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8024 switch (hash) { 8025 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 8026 case 97604197: /*focal*/ return new String[] {"boolean"}; 8027 case -351767064: /*coverage*/ return new String[] {"Reference"}; 8028 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 8029 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 8030 default: return super.getTypesForProperty(hash, name); 8031 } 8032 8033 } 8034 8035 @Override 8036 public Base addChild(String name) throws FHIRException { 8037 if (name.equals("sequence")) { 8038 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.insurance.sequence"); 8039 } 8040 else if (name.equals("focal")) { 8041 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.insurance.focal"); 8042 } 8043 else if (name.equals("coverage")) { 8044 this.coverage = new Reference(); 8045 return this.coverage; 8046 } 8047 else if (name.equals("businessArrangement")) { 8048 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.insurance.businessArrangement"); 8049 } 8050 else if (name.equals("claimResponse")) { 8051 this.claimResponse = new Reference(); 8052 return this.claimResponse; 8053 } 8054 else 8055 return super.addChild(name); 8056 } 8057 8058 public InsuranceComponent copy() { 8059 InsuranceComponent dst = new InsuranceComponent(); 8060 copyValues(dst); 8061 return dst; 8062 } 8063 8064 public void copyValues(InsuranceComponent dst) { 8065 super.copyValues(dst); 8066 dst.sequence = sequence == null ? null : sequence.copy(); 8067 dst.focal = focal == null ? null : focal.copy(); 8068 dst.coverage = coverage == null ? null : coverage.copy(); 8069 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 8070 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 8071 } 8072 8073 @Override 8074 public boolean equalsDeep(Base other_) { 8075 if (!super.equalsDeep(other_)) 8076 return false; 8077 if (!(other_ instanceof InsuranceComponent)) 8078 return false; 8079 InsuranceComponent o = (InsuranceComponent) other_; 8080 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) 8081 && compareDeep(businessArrangement, o.businessArrangement, true) && compareDeep(claimResponse, o.claimResponse, true) 8082 ; 8083 } 8084 8085 @Override 8086 public boolean equalsShallow(Base other_) { 8087 if (!super.equalsShallow(other_)) 8088 return false; 8089 if (!(other_ instanceof InsuranceComponent)) 8090 return false; 8091 InsuranceComponent o = (InsuranceComponent) other_; 8092 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 8093 ; 8094 } 8095 8096 public boolean isEmpty() { 8097 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, focal, coverage 8098 , businessArrangement, claimResponse); 8099 } 8100 8101 public String fhirType() { 8102 return "ClaimResponse.insurance"; 8103 8104 } 8105 8106 } 8107 8108 @Block() 8109 public static class ErrorComponent extends BackboneElement implements IBaseBackboneElement { 8110 /** 8111 * The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8112 */ 8113 @Child(name = "itemSequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 8114 @Description(shortDefinition="Item sequence number", formalDefinition="The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure." ) 8115 protected PositiveIntType itemSequence; 8116 8117 /** 8118 * The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8119 */ 8120 @Child(name = "detailSequence", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 8121 @Description(shortDefinition="Detail sequence number", formalDefinition="The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure." ) 8122 protected PositiveIntType detailSequence; 8123 8124 /** 8125 * The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8126 */ 8127 @Child(name = "subDetailSequence", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=false) 8128 @Description(shortDefinition="Subdetail sequence number", formalDefinition="The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure." ) 8129 protected PositiveIntType subDetailSequence; 8130 8131 /** 8132 * An error code, from a specified code system, which details why the claim could not be adjudicated. 8133 */ 8134 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 8135 @Description(shortDefinition="Error code detailing processing issues", formalDefinition="An error code, from a specified code system, which details why the claim could not be adjudicated." ) 8136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-error") 8137 protected CodeableConcept code; 8138 8139 /** 8140 * A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. 8141 */ 8142 @Child(name = "expression", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8143 @Description(shortDefinition="FHIRPath of element(s) related to issue", formalDefinition="A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised." ) 8144 protected List<StringType> expression; 8145 8146 private static final long serialVersionUID = -514754249L; 8147 8148 /** 8149 * Constructor 8150 */ 8151 public ErrorComponent() { 8152 super(); 8153 } 8154 8155 /** 8156 * Constructor 8157 */ 8158 public ErrorComponent(CodeableConcept code) { 8159 super(); 8160 this.setCode(code); 8161 } 8162 8163 /** 8164 * @return {@link #itemSequence} (The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.). This is the underlying object with id, value and extensions. The accessor "getItemSequence" gives direct access to the value 8165 */ 8166 public PositiveIntType getItemSequenceElement() { 8167 if (this.itemSequence == null) 8168 if (Configuration.errorOnAutoCreate()) 8169 throw new Error("Attempt to auto-create ErrorComponent.itemSequence"); 8170 else if (Configuration.doAutoCreate()) 8171 this.itemSequence = new PositiveIntType(); // bb 8172 return this.itemSequence; 8173 } 8174 8175 public boolean hasItemSequenceElement() { 8176 return this.itemSequence != null && !this.itemSequence.isEmpty(); 8177 } 8178 8179 public boolean hasItemSequence() { 8180 return this.itemSequence != null && !this.itemSequence.isEmpty(); 8181 } 8182 8183 /** 8184 * @param value {@link #itemSequence} (The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.). This is the underlying object with id, value and extensions. The accessor "getItemSequence" gives direct access to the value 8185 */ 8186 public ErrorComponent setItemSequenceElement(PositiveIntType value) { 8187 this.itemSequence = value; 8188 return this; 8189 } 8190 8191 /** 8192 * @return The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8193 */ 8194 public int getItemSequence() { 8195 return this.itemSequence == null || this.itemSequence.isEmpty() ? 0 : this.itemSequence.getValue(); 8196 } 8197 8198 /** 8199 * @param value The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8200 */ 8201 public ErrorComponent setItemSequence(int value) { 8202 if (this.itemSequence == null) 8203 this.itemSequence = new PositiveIntType(); 8204 this.itemSequence.setValue(value); 8205 return this; 8206 } 8207 8208 /** 8209 * @return {@link #detailSequence} (The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.). This is the underlying object with id, value and extensions. The accessor "getDetailSequence" gives direct access to the value 8210 */ 8211 public PositiveIntType getDetailSequenceElement() { 8212 if (this.detailSequence == null) 8213 if (Configuration.errorOnAutoCreate()) 8214 throw new Error("Attempt to auto-create ErrorComponent.detailSequence"); 8215 else if (Configuration.doAutoCreate()) 8216 this.detailSequence = new PositiveIntType(); // bb 8217 return this.detailSequence; 8218 } 8219 8220 public boolean hasDetailSequenceElement() { 8221 return this.detailSequence != null && !this.detailSequence.isEmpty(); 8222 } 8223 8224 public boolean hasDetailSequence() { 8225 return this.detailSequence != null && !this.detailSequence.isEmpty(); 8226 } 8227 8228 /** 8229 * @param value {@link #detailSequence} (The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.). This is the underlying object with id, value and extensions. The accessor "getDetailSequence" gives direct access to the value 8230 */ 8231 public ErrorComponent setDetailSequenceElement(PositiveIntType value) { 8232 this.detailSequence = value; 8233 return this; 8234 } 8235 8236 /** 8237 * @return The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8238 */ 8239 public int getDetailSequence() { 8240 return this.detailSequence == null || this.detailSequence.isEmpty() ? 0 : this.detailSequence.getValue(); 8241 } 8242 8243 /** 8244 * @param value The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8245 */ 8246 public ErrorComponent setDetailSequence(int value) { 8247 if (this.detailSequence == null) 8248 this.detailSequence = new PositiveIntType(); 8249 this.detailSequence.setValue(value); 8250 return this; 8251 } 8252 8253 /** 8254 * @return {@link #subDetailSequence} (The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.). This is the underlying object with id, value and extensions. The accessor "getSubDetailSequence" gives direct access to the value 8255 */ 8256 public PositiveIntType getSubDetailSequenceElement() { 8257 if (this.subDetailSequence == null) 8258 if (Configuration.errorOnAutoCreate()) 8259 throw new Error("Attempt to auto-create ErrorComponent.subDetailSequence"); 8260 else if (Configuration.doAutoCreate()) 8261 this.subDetailSequence = new PositiveIntType(); // bb 8262 return this.subDetailSequence; 8263 } 8264 8265 public boolean hasSubDetailSequenceElement() { 8266 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 8267 } 8268 8269 public boolean hasSubDetailSequence() { 8270 return this.subDetailSequence != null && !this.subDetailSequence.isEmpty(); 8271 } 8272 8273 /** 8274 * @param value {@link #subDetailSequence} (The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.). This is the underlying object with id, value and extensions. The accessor "getSubDetailSequence" gives direct access to the value 8275 */ 8276 public ErrorComponent setSubDetailSequenceElement(PositiveIntType value) { 8277 this.subDetailSequence = value; 8278 return this; 8279 } 8280 8281 /** 8282 * @return The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8283 */ 8284 public int getSubDetailSequence() { 8285 return this.subDetailSequence == null || this.subDetailSequence.isEmpty() ? 0 : this.subDetailSequence.getValue(); 8286 } 8287 8288 /** 8289 * @param value The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure. 8290 */ 8291 public ErrorComponent setSubDetailSequence(int value) { 8292 if (this.subDetailSequence == null) 8293 this.subDetailSequence = new PositiveIntType(); 8294 this.subDetailSequence.setValue(value); 8295 return this; 8296 } 8297 8298 /** 8299 * @return {@link #code} (An error code, from a specified code system, which details why the claim could not be adjudicated.) 8300 */ 8301 public CodeableConcept getCode() { 8302 if (this.code == null) 8303 if (Configuration.errorOnAutoCreate()) 8304 throw new Error("Attempt to auto-create ErrorComponent.code"); 8305 else if (Configuration.doAutoCreate()) 8306 this.code = new CodeableConcept(); // cc 8307 return this.code; 8308 } 8309 8310 public boolean hasCode() { 8311 return this.code != null && !this.code.isEmpty(); 8312 } 8313 8314 /** 8315 * @param value {@link #code} (An error code, from a specified code system, which details why the claim could not be adjudicated.) 8316 */ 8317 public ErrorComponent setCode(CodeableConcept value) { 8318 this.code = value; 8319 return this; 8320 } 8321 8322 /** 8323 * @return {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 8324 */ 8325 public List<StringType> getExpression() { 8326 if (this.expression == null) 8327 this.expression = new ArrayList<StringType>(); 8328 return this.expression; 8329 } 8330 8331 /** 8332 * @return Returns a reference to <code>this</code> for easy method chaining 8333 */ 8334 public ErrorComponent setExpression(List<StringType> theExpression) { 8335 this.expression = theExpression; 8336 return this; 8337 } 8338 8339 public boolean hasExpression() { 8340 if (this.expression == null) 8341 return false; 8342 for (StringType item : this.expression) 8343 if (!item.isEmpty()) 8344 return true; 8345 return false; 8346 } 8347 8348 /** 8349 * @return {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 8350 */ 8351 public StringType addExpressionElement() {//2 8352 StringType t = new StringType(); 8353 if (this.expression == null) 8354 this.expression = new ArrayList<StringType>(); 8355 this.expression.add(t); 8356 return t; 8357 } 8358 8359 /** 8360 * @param value {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 8361 */ 8362 public ErrorComponent addExpression(String value) { //1 8363 StringType t = new StringType(); 8364 t.setValue(value); 8365 if (this.expression == null) 8366 this.expression = new ArrayList<StringType>(); 8367 this.expression.add(t); 8368 return this; 8369 } 8370 8371 /** 8372 * @param value {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 8373 */ 8374 public boolean hasExpression(String value) { 8375 if (this.expression == null) 8376 return false; 8377 for (StringType v : this.expression) 8378 if (v.getValue().equals(value)) // string 8379 return true; 8380 return false; 8381 } 8382 8383 protected void listChildren(List<Property> children) { 8384 super.listChildren(children); 8385 children.add(new Property("itemSequence", "positiveInt", "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 0, 1, itemSequence)); 8386 children.add(new Property("detailSequence", "positiveInt", "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 0, 1, detailSequence)); 8387 children.add(new Property("subDetailSequence", "positiveInt", "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 0, 1, subDetailSequence)); 8388 children.add(new Property("code", "CodeableConcept", "An error code, from a specified code system, which details why the claim could not be adjudicated.", 0, 1, code)); 8389 children.add(new Property("expression", "string", "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression)); 8390 } 8391 8392 @Override 8393 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8394 switch (_hash) { 8395 case 1977979892: /*itemSequence*/ return new Property("itemSequence", "positiveInt", "The sequence number of the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 0, 1, itemSequence); 8396 case 1321472818: /*detailSequence*/ return new Property("detailSequence", "positiveInt", "The sequence number of the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 0, 1, detailSequence); 8397 case -855462510: /*subDetailSequence*/ return new Property("subDetailSequence", "positiveInt", "The sequence number of the sub-detail within the detail within the line item submitted which contains the error. This value is omitted when the error occurs outside of the item structure.", 0, 1, subDetailSequence); 8398 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "An error code, from a specified code system, which details why the claim could not be adjudicated.", 0, 1, code); 8399 case -1795452264: /*expression*/ return new Property("expression", "string", "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression); 8400 default: return super.getNamedProperty(_hash, _name, _checkValid); 8401 } 8402 8403 } 8404 8405 @Override 8406 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8407 switch (hash) { 8408 case 1977979892: /*itemSequence*/ return this.itemSequence == null ? new Base[0] : new Base[] {this.itemSequence}; // PositiveIntType 8409 case 1321472818: /*detailSequence*/ return this.detailSequence == null ? new Base[0] : new Base[] {this.detailSequence}; // PositiveIntType 8410 case -855462510: /*subDetailSequence*/ return this.subDetailSequence == null ? new Base[0] : new Base[] {this.subDetailSequence}; // PositiveIntType 8411 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 8412 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : this.expression.toArray(new Base[this.expression.size()]); // StringType 8413 default: return super.getProperty(hash, name, checkValid); 8414 } 8415 8416 } 8417 8418 @Override 8419 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8420 switch (hash) { 8421 case 1977979892: // itemSequence 8422 this.itemSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8423 return value; 8424 case 1321472818: // detailSequence 8425 this.detailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8426 return value; 8427 case -855462510: // subDetailSequence 8428 this.subDetailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8429 return value; 8430 case 3059181: // code 8431 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8432 return value; 8433 case -1795452264: // expression 8434 this.getExpression().add(TypeConvertor.castToString(value)); // StringType 8435 return value; 8436 default: return super.setProperty(hash, name, value); 8437 } 8438 8439 } 8440 8441 @Override 8442 public Base setProperty(String name, Base value) throws FHIRException { 8443 if (name.equals("itemSequence")) { 8444 this.itemSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8445 } else if (name.equals("detailSequence")) { 8446 this.detailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8447 } else if (name.equals("subDetailSequence")) { 8448 this.subDetailSequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8449 } else if (name.equals("code")) { 8450 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8451 } else if (name.equals("expression")) { 8452 this.getExpression().add(TypeConvertor.castToString(value)); 8453 } else 8454 return super.setProperty(name, value); 8455 return value; 8456 } 8457 8458 @Override 8459 public void removeChild(String name, Base value) throws FHIRException { 8460 if (name.equals("itemSequence")) { 8461 this.itemSequence = null; 8462 } else if (name.equals("detailSequence")) { 8463 this.detailSequence = null; 8464 } else if (name.equals("subDetailSequence")) { 8465 this.subDetailSequence = null; 8466 } else if (name.equals("code")) { 8467 this.code = null; 8468 } else if (name.equals("expression")) { 8469 this.getExpression().remove(value); 8470 } else 8471 super.removeChild(name, value); 8472 8473 } 8474 8475 @Override 8476 public Base makeProperty(int hash, String name) throws FHIRException { 8477 switch (hash) { 8478 case 1977979892: return getItemSequenceElement(); 8479 case 1321472818: return getDetailSequenceElement(); 8480 case -855462510: return getSubDetailSequenceElement(); 8481 case 3059181: return getCode(); 8482 case -1795452264: return addExpressionElement(); 8483 default: return super.makeProperty(hash, name); 8484 } 8485 8486 } 8487 8488 @Override 8489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8490 switch (hash) { 8491 case 1977979892: /*itemSequence*/ return new String[] {"positiveInt"}; 8492 case 1321472818: /*detailSequence*/ return new String[] {"positiveInt"}; 8493 case -855462510: /*subDetailSequence*/ return new String[] {"positiveInt"}; 8494 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 8495 case -1795452264: /*expression*/ return new String[] {"string"}; 8496 default: return super.getTypesForProperty(hash, name); 8497 } 8498 8499 } 8500 8501 @Override 8502 public Base addChild(String name) throws FHIRException { 8503 if (name.equals("itemSequence")) { 8504 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.error.itemSequence"); 8505 } 8506 else if (name.equals("detailSequence")) { 8507 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.error.detailSequence"); 8508 } 8509 else if (name.equals("subDetailSequence")) { 8510 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.error.subDetailSequence"); 8511 } 8512 else if (name.equals("code")) { 8513 this.code = new CodeableConcept(); 8514 return this.code; 8515 } 8516 else if (name.equals("expression")) { 8517 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.error.expression"); 8518 } 8519 else 8520 return super.addChild(name); 8521 } 8522 8523 public ErrorComponent copy() { 8524 ErrorComponent dst = new ErrorComponent(); 8525 copyValues(dst); 8526 return dst; 8527 } 8528 8529 public void copyValues(ErrorComponent dst) { 8530 super.copyValues(dst); 8531 dst.itemSequence = itemSequence == null ? null : itemSequence.copy(); 8532 dst.detailSequence = detailSequence == null ? null : detailSequence.copy(); 8533 dst.subDetailSequence = subDetailSequence == null ? null : subDetailSequence.copy(); 8534 dst.code = code == null ? null : code.copy(); 8535 if (expression != null) { 8536 dst.expression = new ArrayList<StringType>(); 8537 for (StringType i : expression) 8538 dst.expression.add(i.copy()); 8539 }; 8540 } 8541 8542 @Override 8543 public boolean equalsDeep(Base other_) { 8544 if (!super.equalsDeep(other_)) 8545 return false; 8546 if (!(other_ instanceof ErrorComponent)) 8547 return false; 8548 ErrorComponent o = (ErrorComponent) other_; 8549 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 8550 && compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(code, o.code, true) 8551 && compareDeep(expression, o.expression, true); 8552 } 8553 8554 @Override 8555 public boolean equalsShallow(Base other_) { 8556 if (!super.equalsShallow(other_)) 8557 return false; 8558 if (!(other_ instanceof ErrorComponent)) 8559 return false; 8560 ErrorComponent o = (ErrorComponent) other_; 8561 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 8562 && compareValues(subDetailSequence, o.subDetailSequence, true) && compareValues(expression, o.expression, true) 8563 ; 8564 } 8565 8566 public boolean isEmpty() { 8567 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence 8568 , subDetailSequence, code, expression); 8569 } 8570 8571 public String fhirType() { 8572 return "ClaimResponse.error"; 8573 8574 } 8575 8576 } 8577 8578 /** 8579 * A unique identifier assigned to this claim response. 8580 */ 8581 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8582 @Description(shortDefinition="Business Identifier for a claim response", formalDefinition="A unique identifier assigned to this claim response." ) 8583 protected List<Identifier> identifier; 8584 8585 /** 8586 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 8587 */ 8588 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8589 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 8590 protected List<Identifier> traceNumber; 8591 8592 /** 8593 * The status of the resource instance. 8594 */ 8595 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 8596 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 8597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 8598 protected Enumeration<FinancialResourceStatusCodes> status; 8599 8600 /** 8601 * A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service. 8602 */ 8603 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 8604 @Description(shortDefinition="More granular claim type", formalDefinition="A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service." ) 8605 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 8606 protected CodeableConcept type; 8607 8608 /** 8609 * A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service. 8610 */ 8611 @Child(name = "subType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 8612 @Description(shortDefinition="More granular claim type", formalDefinition="A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service." ) 8613 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 8614 protected CodeableConcept subType; 8615 8616 /** 8617 * A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 8618 */ 8619 @Child(name = "use", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 8620 @Description(shortDefinition="claim | preauthorization | predetermination", formalDefinition="A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided." ) 8621 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 8622 protected Enumeration<Use> use; 8623 8624 /** 8625 * The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought. 8626 */ 8627 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 8628 @Description(shortDefinition="The recipient of the products and services", formalDefinition="The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought." ) 8629 protected Reference patient; 8630 8631 /** 8632 * The date this resource was created. 8633 */ 8634 @Child(name = "created", type = {DateTimeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 8635 @Description(shortDefinition="Response creation date", formalDefinition="The date this resource was created." ) 8636 protected DateTimeType created; 8637 8638 /** 8639 * The party responsible for authorization, adjudication and reimbursement. 8640 */ 8641 @Child(name = "insurer", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=true) 8642 @Description(shortDefinition="Party responsible for reimbursement", formalDefinition="The party responsible for authorization, adjudication and reimbursement." ) 8643 protected Reference insurer; 8644 8645 /** 8646 * The provider which is responsible for the claim, predetermination or preauthorization. 8647 */ 8648 @Child(name = "requestor", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 8649 @Description(shortDefinition="Party responsible for the claim", formalDefinition="The provider which is responsible for the claim, predetermination or preauthorization." ) 8650 protected Reference requestor; 8651 8652 /** 8653 * Original request resource reference. 8654 */ 8655 @Child(name = "request", type = {Claim.class}, order=10, min=0, max=1, modifier=false, summary=true) 8656 @Description(shortDefinition="Id of resource triggering adjudication", formalDefinition="Original request resource reference." ) 8657 protected Reference request; 8658 8659 /** 8660 * The outcome of the claim, predetermination, or preauthorization processing. 8661 */ 8662 @Child(name = "outcome", type = {CodeType.class}, order=11, min=1, max=1, modifier=false, summary=true) 8663 @Description(shortDefinition="queued | complete | error | partial", formalDefinition="The outcome of the claim, predetermination, or preauthorization processing." ) 8664 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-outcome") 8665 protected Enumeration<ClaimProcessingCodes> outcome; 8666 8667 /** 8668 * The result of the claim, predetermination, or preauthorization adjudication. 8669 */ 8670 @Child(name = "decision", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 8671 @Description(shortDefinition="Result of the adjudication", formalDefinition="The result of the claim, predetermination, or preauthorization adjudication." ) 8672 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision") 8673 protected CodeableConcept decision; 8674 8675 /** 8676 * A human readable description of the status of the adjudication. 8677 */ 8678 @Child(name = "disposition", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 8679 @Description(shortDefinition="Disposition Message", formalDefinition="A human readable description of the status of the adjudication." ) 8680 protected StringType disposition; 8681 8682 /** 8683 * Reference from the Insurer which is used in later communications which refers to this adjudication. 8684 */ 8685 @Child(name = "preAuthRef", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 8686 @Description(shortDefinition="Preauthorization reference", formalDefinition="Reference from the Insurer which is used in later communications which refers to this adjudication." ) 8687 protected StringType preAuthRef; 8688 8689 /** 8690 * The time frame during which this authorization is effective. 8691 */ 8692 @Child(name = "preAuthPeriod", type = {Period.class}, order=15, min=0, max=1, modifier=false, summary=false) 8693 @Description(shortDefinition="Preauthorization reference effective period", formalDefinition="The time frame during which this authorization is effective." ) 8694 protected Period preAuthPeriod; 8695 8696 /** 8697 * Information code for an event with a corresponding date or period. 8698 */ 8699 @Child(name = "event", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8700 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 8701 protected List<ClaimResponseEventComponent> event; 8702 8703 /** 8704 * Type of Party to be reimbursed: subscriber, provider, other. 8705 */ 8706 @Child(name = "payeeType", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=false) 8707 @Description(shortDefinition="Party to be paid any benefits payable", formalDefinition="Type of Party to be reimbursed: subscriber, provider, other." ) 8708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 8709 protected CodeableConcept payeeType; 8710 8711 /** 8712 * Healthcare encounters related to this claim. 8713 */ 8714 @Child(name = "encounter", type = {Encounter.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8715 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 8716 protected List<Reference> encounter; 8717 8718 /** 8719 * A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system. 8720 */ 8721 @Child(name = "diagnosisRelatedGroup", type = {CodeableConcept.class}, order=19, min=0, max=1, modifier=false, summary=false) 8722 @Description(shortDefinition="Package billing code", formalDefinition="A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system." ) 8723 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 8724 protected CodeableConcept diagnosisRelatedGroup; 8725 8726 /** 8727 * A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details. 8728 */ 8729 @Child(name = "item", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8730 @Description(shortDefinition="Adjudication for claim line items", formalDefinition="A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details." ) 8731 protected List<ItemComponent> item; 8732 8733 /** 8734 * The first-tier service adjudications for payor added product or service lines. 8735 */ 8736 @Child(name = "addItem", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8737 @Description(shortDefinition="Insurer added line items", formalDefinition="The first-tier service adjudications for payor added product or service lines." ) 8738 protected List<AddedItemComponent> addItem; 8739 8740 /** 8741 * The adjudication results which are presented at the header level rather than at the line-item or add-item levels. 8742 */ 8743 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8744 @Description(shortDefinition="Header-level adjudication", formalDefinition="The adjudication results which are presented at the header level rather than at the line-item or add-item levels." ) 8745 protected List<AdjudicationComponent> adjudication; 8746 8747 /** 8748 * Categorized monetary totals for the adjudication. 8749 */ 8750 @Child(name = "total", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 8751 @Description(shortDefinition="Adjudication totals", formalDefinition="Categorized monetary totals for the adjudication." ) 8752 protected List<TotalComponent> total; 8753 8754 /** 8755 * Payment details for the adjudication of the claim. 8756 */ 8757 @Child(name = "payment", type = {}, order=24, min=0, max=1, modifier=false, summary=false) 8758 @Description(shortDefinition="Payment Details", formalDefinition="Payment details for the adjudication of the claim." ) 8759 protected PaymentComponent payment; 8760 8761 /** 8762 * A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom. 8763 */ 8764 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=25, min=0, max=1, modifier=false, summary=false) 8765 @Description(shortDefinition="Funds reserved status", formalDefinition="A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom." ) 8766 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 8767 protected CodeableConcept fundsReserve; 8768 8769 /** 8770 * A code for the form to be used for printing the content. 8771 */ 8772 @Child(name = "formCode", type = {CodeableConcept.class}, order=26, min=0, max=1, modifier=false, summary=false) 8773 @Description(shortDefinition="Printed form identifier", formalDefinition="A code for the form to be used for printing the content." ) 8774 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 8775 protected CodeableConcept formCode; 8776 8777 /** 8778 * The actual form, by reference or inclusion, for printing the content or an EOB. 8779 */ 8780 @Child(name = "form", type = {Attachment.class}, order=27, min=0, max=1, modifier=false, summary=false) 8781 @Description(shortDefinition="Printed reference or actual form", formalDefinition="The actual form, by reference or inclusion, for printing the content or an EOB." ) 8782 protected Attachment form; 8783 8784 /** 8785 * A note that describes or explains adjudication results in a human readable form. 8786 */ 8787 @Child(name = "processNote", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8788 @Description(shortDefinition="Note concerning adjudication", formalDefinition="A note that describes or explains adjudication results in a human readable form." ) 8789 protected List<NoteComponent> processNote; 8790 8791 /** 8792 * Request for additional supporting or authorizing information. 8793 */ 8794 @Child(name = "communicationRequest", type = {CommunicationRequest.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8795 @Description(shortDefinition="Request for additional information", formalDefinition="Request for additional supporting or authorizing information." ) 8796 protected List<Reference> communicationRequest; 8797 8798 /** 8799 * Financial instruments for reimbursement for the health care products and services specified on the claim. 8800 */ 8801 @Child(name = "insurance", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8802 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services specified on the claim." ) 8803 protected List<InsuranceComponent> insurance; 8804 8805 /** 8806 * Errors encountered during the processing of the adjudication. 8807 */ 8808 @Child(name = "error", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8809 @Description(shortDefinition="Processing errors", formalDefinition="Errors encountered during the processing of the adjudication." ) 8810 protected List<ErrorComponent> error; 8811 8812 private static final long serialVersionUID = -1099970187L; 8813 8814 /** 8815 * Constructor 8816 */ 8817 public ClaimResponse() { 8818 super(); 8819 } 8820 8821 /** 8822 * Constructor 8823 */ 8824 public ClaimResponse(FinancialResourceStatusCodes status, CodeableConcept type, Use use, Reference patient, Date created, ClaimProcessingCodes outcome) { 8825 super(); 8826 this.setStatus(status); 8827 this.setType(type); 8828 this.setUse(use); 8829 this.setPatient(patient); 8830 this.setCreated(created); 8831 this.setOutcome(outcome); 8832 } 8833 8834 /** 8835 * @return {@link #identifier} (A unique identifier assigned to this claim response.) 8836 */ 8837 public List<Identifier> getIdentifier() { 8838 if (this.identifier == null) 8839 this.identifier = new ArrayList<Identifier>(); 8840 return this.identifier; 8841 } 8842 8843 /** 8844 * @return Returns a reference to <code>this</code> for easy method chaining 8845 */ 8846 public ClaimResponse setIdentifier(List<Identifier> theIdentifier) { 8847 this.identifier = theIdentifier; 8848 return this; 8849 } 8850 8851 public boolean hasIdentifier() { 8852 if (this.identifier == null) 8853 return false; 8854 for (Identifier item : this.identifier) 8855 if (!item.isEmpty()) 8856 return true; 8857 return false; 8858 } 8859 8860 public Identifier addIdentifier() { //3 8861 Identifier t = new Identifier(); 8862 if (this.identifier == null) 8863 this.identifier = new ArrayList<Identifier>(); 8864 this.identifier.add(t); 8865 return t; 8866 } 8867 8868 public ClaimResponse addIdentifier(Identifier t) { //3 8869 if (t == null) 8870 return this; 8871 if (this.identifier == null) 8872 this.identifier = new ArrayList<Identifier>(); 8873 this.identifier.add(t); 8874 return this; 8875 } 8876 8877 /** 8878 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 8879 */ 8880 public Identifier getIdentifierFirstRep() { 8881 if (getIdentifier().isEmpty()) { 8882 addIdentifier(); 8883 } 8884 return getIdentifier().get(0); 8885 } 8886 8887 /** 8888 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 8889 */ 8890 public List<Identifier> getTraceNumber() { 8891 if (this.traceNumber == null) 8892 this.traceNumber = new ArrayList<Identifier>(); 8893 return this.traceNumber; 8894 } 8895 8896 /** 8897 * @return Returns a reference to <code>this</code> for easy method chaining 8898 */ 8899 public ClaimResponse setTraceNumber(List<Identifier> theTraceNumber) { 8900 this.traceNumber = theTraceNumber; 8901 return this; 8902 } 8903 8904 public boolean hasTraceNumber() { 8905 if (this.traceNumber == null) 8906 return false; 8907 for (Identifier item : this.traceNumber) 8908 if (!item.isEmpty()) 8909 return true; 8910 return false; 8911 } 8912 8913 public Identifier addTraceNumber() { //3 8914 Identifier t = new Identifier(); 8915 if (this.traceNumber == null) 8916 this.traceNumber = new ArrayList<Identifier>(); 8917 this.traceNumber.add(t); 8918 return t; 8919 } 8920 8921 public ClaimResponse addTraceNumber(Identifier t) { //3 8922 if (t == null) 8923 return this; 8924 if (this.traceNumber == null) 8925 this.traceNumber = new ArrayList<Identifier>(); 8926 this.traceNumber.add(t); 8927 return this; 8928 } 8929 8930 /** 8931 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 8932 */ 8933 public Identifier getTraceNumberFirstRep() { 8934 if (getTraceNumber().isEmpty()) { 8935 addTraceNumber(); 8936 } 8937 return getTraceNumber().get(0); 8938 } 8939 8940 /** 8941 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8942 */ 8943 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 8944 if (this.status == null) 8945 if (Configuration.errorOnAutoCreate()) 8946 throw new Error("Attempt to auto-create ClaimResponse.status"); 8947 else if (Configuration.doAutoCreate()) 8948 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 8949 return this.status; 8950 } 8951 8952 public boolean hasStatusElement() { 8953 return this.status != null && !this.status.isEmpty(); 8954 } 8955 8956 public boolean hasStatus() { 8957 return this.status != null && !this.status.isEmpty(); 8958 } 8959 8960 /** 8961 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 8962 */ 8963 public ClaimResponse setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 8964 this.status = value; 8965 return this; 8966 } 8967 8968 /** 8969 * @return The status of the resource instance. 8970 */ 8971 public FinancialResourceStatusCodes getStatus() { 8972 return this.status == null ? null : this.status.getValue(); 8973 } 8974 8975 /** 8976 * @param value The status of the resource instance. 8977 */ 8978 public ClaimResponse setStatus(FinancialResourceStatusCodes value) { 8979 if (this.status == null) 8980 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 8981 this.status.setValue(value); 8982 return this; 8983 } 8984 8985 /** 8986 * @return {@link #type} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 8987 */ 8988 public CodeableConcept getType() { 8989 if (this.type == null) 8990 if (Configuration.errorOnAutoCreate()) 8991 throw new Error("Attempt to auto-create ClaimResponse.type"); 8992 else if (Configuration.doAutoCreate()) 8993 this.type = new CodeableConcept(); // cc 8994 return this.type; 8995 } 8996 8997 public boolean hasType() { 8998 return this.type != null && !this.type.isEmpty(); 8999 } 9000 9001 /** 9002 * @param value {@link #type} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 9003 */ 9004 public ClaimResponse setType(CodeableConcept value) { 9005 this.type = value; 9006 return this; 9007 } 9008 9009 /** 9010 * @return {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 9011 */ 9012 public CodeableConcept getSubType() { 9013 if (this.subType == null) 9014 if (Configuration.errorOnAutoCreate()) 9015 throw new Error("Attempt to auto-create ClaimResponse.subType"); 9016 else if (Configuration.doAutoCreate()) 9017 this.subType = new CodeableConcept(); // cc 9018 return this.subType; 9019 } 9020 9021 public boolean hasSubType() { 9022 return this.subType != null && !this.subType.isEmpty(); 9023 } 9024 9025 /** 9026 * @param value {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 9027 */ 9028 public ClaimResponse setSubType(CodeableConcept value) { 9029 this.subType = value; 9030 return this; 9031 } 9032 9033 /** 9034 * @return {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 9035 */ 9036 public Enumeration<Use> getUseElement() { 9037 if (this.use == null) 9038 if (Configuration.errorOnAutoCreate()) 9039 throw new Error("Attempt to auto-create ClaimResponse.use"); 9040 else if (Configuration.doAutoCreate()) 9041 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 9042 return this.use; 9043 } 9044 9045 public boolean hasUseElement() { 9046 return this.use != null && !this.use.isEmpty(); 9047 } 9048 9049 public boolean hasUse() { 9050 return this.use != null && !this.use.isEmpty(); 9051 } 9052 9053 /** 9054 * @param value {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 9055 */ 9056 public ClaimResponse setUseElement(Enumeration<Use> value) { 9057 this.use = value; 9058 return this; 9059 } 9060 9061 /** 9062 * @return A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 9063 */ 9064 public Use getUse() { 9065 return this.use == null ? null : this.use.getValue(); 9066 } 9067 9068 /** 9069 * @param value A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 9070 */ 9071 public ClaimResponse setUse(Use value) { 9072 if (this.use == null) 9073 this.use = new Enumeration<Use>(new UseEnumFactory()); 9074 this.use.setValue(value); 9075 return this; 9076 } 9077 9078 /** 9079 * @return {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.) 9080 */ 9081 public Reference getPatient() { 9082 if (this.patient == null) 9083 if (Configuration.errorOnAutoCreate()) 9084 throw new Error("Attempt to auto-create ClaimResponse.patient"); 9085 else if (Configuration.doAutoCreate()) 9086 this.patient = new Reference(); // cc 9087 return this.patient; 9088 } 9089 9090 public boolean hasPatient() { 9091 return this.patient != null && !this.patient.isEmpty(); 9092 } 9093 9094 /** 9095 * @param value {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.) 9096 */ 9097 public ClaimResponse setPatient(Reference value) { 9098 this.patient = value; 9099 return this; 9100 } 9101 9102 /** 9103 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 9104 */ 9105 public DateTimeType getCreatedElement() { 9106 if (this.created == null) 9107 if (Configuration.errorOnAutoCreate()) 9108 throw new Error("Attempt to auto-create ClaimResponse.created"); 9109 else if (Configuration.doAutoCreate()) 9110 this.created = new DateTimeType(); // bb 9111 return this.created; 9112 } 9113 9114 public boolean hasCreatedElement() { 9115 return this.created != null && !this.created.isEmpty(); 9116 } 9117 9118 public boolean hasCreated() { 9119 return this.created != null && !this.created.isEmpty(); 9120 } 9121 9122 /** 9123 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 9124 */ 9125 public ClaimResponse setCreatedElement(DateTimeType value) { 9126 this.created = value; 9127 return this; 9128 } 9129 9130 /** 9131 * @return The date this resource was created. 9132 */ 9133 public Date getCreated() { 9134 return this.created == null ? null : this.created.getValue(); 9135 } 9136 9137 /** 9138 * @param value The date this resource was created. 9139 */ 9140 public ClaimResponse setCreated(Date value) { 9141 if (this.created == null) 9142 this.created = new DateTimeType(); 9143 this.created.setValue(value); 9144 return this; 9145 } 9146 9147 /** 9148 * @return {@link #insurer} (The party responsible for authorization, adjudication and reimbursement.) 9149 */ 9150 public Reference getInsurer() { 9151 if (this.insurer == null) 9152 if (Configuration.errorOnAutoCreate()) 9153 throw new Error("Attempt to auto-create ClaimResponse.insurer"); 9154 else if (Configuration.doAutoCreate()) 9155 this.insurer = new Reference(); // cc 9156 return this.insurer; 9157 } 9158 9159 public boolean hasInsurer() { 9160 return this.insurer != null && !this.insurer.isEmpty(); 9161 } 9162 9163 /** 9164 * @param value {@link #insurer} (The party responsible for authorization, adjudication and reimbursement.) 9165 */ 9166 public ClaimResponse setInsurer(Reference value) { 9167 this.insurer = value; 9168 return this; 9169 } 9170 9171 /** 9172 * @return {@link #requestor} (The provider which is responsible for the claim, predetermination or preauthorization.) 9173 */ 9174 public Reference getRequestor() { 9175 if (this.requestor == null) 9176 if (Configuration.errorOnAutoCreate()) 9177 throw new Error("Attempt to auto-create ClaimResponse.requestor"); 9178 else if (Configuration.doAutoCreate()) 9179 this.requestor = new Reference(); // cc 9180 return this.requestor; 9181 } 9182 9183 public boolean hasRequestor() { 9184 return this.requestor != null && !this.requestor.isEmpty(); 9185 } 9186 9187 /** 9188 * @param value {@link #requestor} (The provider which is responsible for the claim, predetermination or preauthorization.) 9189 */ 9190 public ClaimResponse setRequestor(Reference value) { 9191 this.requestor = value; 9192 return this; 9193 } 9194 9195 /** 9196 * @return {@link #request} (Original request resource reference.) 9197 */ 9198 public Reference getRequest() { 9199 if (this.request == null) 9200 if (Configuration.errorOnAutoCreate()) 9201 throw new Error("Attempt to auto-create ClaimResponse.request"); 9202 else if (Configuration.doAutoCreate()) 9203 this.request = new Reference(); // cc 9204 return this.request; 9205 } 9206 9207 public boolean hasRequest() { 9208 return this.request != null && !this.request.isEmpty(); 9209 } 9210 9211 /** 9212 * @param value {@link #request} (Original request resource reference.) 9213 */ 9214 public ClaimResponse setRequest(Reference value) { 9215 this.request = value; 9216 return this; 9217 } 9218 9219 /** 9220 * @return {@link #outcome} (The outcome of the claim, predetermination, or preauthorization processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 9221 */ 9222 public Enumeration<ClaimProcessingCodes> getOutcomeElement() { 9223 if (this.outcome == null) 9224 if (Configuration.errorOnAutoCreate()) 9225 throw new Error("Attempt to auto-create ClaimResponse.outcome"); 9226 else if (Configuration.doAutoCreate()) 9227 this.outcome = new Enumeration<ClaimProcessingCodes>(new ClaimProcessingCodesEnumFactory()); // bb 9228 return this.outcome; 9229 } 9230 9231 public boolean hasOutcomeElement() { 9232 return this.outcome != null && !this.outcome.isEmpty(); 9233 } 9234 9235 public boolean hasOutcome() { 9236 return this.outcome != null && !this.outcome.isEmpty(); 9237 } 9238 9239 /** 9240 * @param value {@link #outcome} (The outcome of the claim, predetermination, or preauthorization processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 9241 */ 9242 public ClaimResponse setOutcomeElement(Enumeration<ClaimProcessingCodes> value) { 9243 this.outcome = value; 9244 return this; 9245 } 9246 9247 /** 9248 * @return The outcome of the claim, predetermination, or preauthorization processing. 9249 */ 9250 public ClaimProcessingCodes getOutcome() { 9251 return this.outcome == null ? null : this.outcome.getValue(); 9252 } 9253 9254 /** 9255 * @param value The outcome of the claim, predetermination, or preauthorization processing. 9256 */ 9257 public ClaimResponse setOutcome(ClaimProcessingCodes value) { 9258 if (this.outcome == null) 9259 this.outcome = new Enumeration<ClaimProcessingCodes>(new ClaimProcessingCodesEnumFactory()); 9260 this.outcome.setValue(value); 9261 return this; 9262 } 9263 9264 /** 9265 * @return {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 9266 */ 9267 public CodeableConcept getDecision() { 9268 if (this.decision == null) 9269 if (Configuration.errorOnAutoCreate()) 9270 throw new Error("Attempt to auto-create ClaimResponse.decision"); 9271 else if (Configuration.doAutoCreate()) 9272 this.decision = new CodeableConcept(); // cc 9273 return this.decision; 9274 } 9275 9276 public boolean hasDecision() { 9277 return this.decision != null && !this.decision.isEmpty(); 9278 } 9279 9280 /** 9281 * @param value {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 9282 */ 9283 public ClaimResponse setDecision(CodeableConcept value) { 9284 this.decision = value; 9285 return this; 9286 } 9287 9288 /** 9289 * @return {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 9290 */ 9291 public StringType getDispositionElement() { 9292 if (this.disposition == null) 9293 if (Configuration.errorOnAutoCreate()) 9294 throw new Error("Attempt to auto-create ClaimResponse.disposition"); 9295 else if (Configuration.doAutoCreate()) 9296 this.disposition = new StringType(); // bb 9297 return this.disposition; 9298 } 9299 9300 public boolean hasDispositionElement() { 9301 return this.disposition != null && !this.disposition.isEmpty(); 9302 } 9303 9304 public boolean hasDisposition() { 9305 return this.disposition != null && !this.disposition.isEmpty(); 9306 } 9307 9308 /** 9309 * @param value {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 9310 */ 9311 public ClaimResponse setDispositionElement(StringType value) { 9312 this.disposition = value; 9313 return this; 9314 } 9315 9316 /** 9317 * @return A human readable description of the status of the adjudication. 9318 */ 9319 public String getDisposition() { 9320 return this.disposition == null ? null : this.disposition.getValue(); 9321 } 9322 9323 /** 9324 * @param value A human readable description of the status of the adjudication. 9325 */ 9326 public ClaimResponse setDisposition(String value) { 9327 if (Utilities.noString(value)) 9328 this.disposition = null; 9329 else { 9330 if (this.disposition == null) 9331 this.disposition = new StringType(); 9332 this.disposition.setValue(value); 9333 } 9334 return this; 9335 } 9336 9337 /** 9338 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 9339 */ 9340 public StringType getPreAuthRefElement() { 9341 if (this.preAuthRef == null) 9342 if (Configuration.errorOnAutoCreate()) 9343 throw new Error("Attempt to auto-create ClaimResponse.preAuthRef"); 9344 else if (Configuration.doAutoCreate()) 9345 this.preAuthRef = new StringType(); // bb 9346 return this.preAuthRef; 9347 } 9348 9349 public boolean hasPreAuthRefElement() { 9350 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 9351 } 9352 9353 public boolean hasPreAuthRef() { 9354 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 9355 } 9356 9357 /** 9358 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 9359 */ 9360 public ClaimResponse setPreAuthRefElement(StringType value) { 9361 this.preAuthRef = value; 9362 return this; 9363 } 9364 9365 /** 9366 * @return Reference from the Insurer which is used in later communications which refers to this adjudication. 9367 */ 9368 public String getPreAuthRef() { 9369 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 9370 } 9371 9372 /** 9373 * @param value Reference from the Insurer which is used in later communications which refers to this adjudication. 9374 */ 9375 public ClaimResponse setPreAuthRef(String value) { 9376 if (Utilities.noString(value)) 9377 this.preAuthRef = null; 9378 else { 9379 if (this.preAuthRef == null) 9380 this.preAuthRef = new StringType(); 9381 this.preAuthRef.setValue(value); 9382 } 9383 return this; 9384 } 9385 9386 /** 9387 * @return {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 9388 */ 9389 public Period getPreAuthPeriod() { 9390 if (this.preAuthPeriod == null) 9391 if (Configuration.errorOnAutoCreate()) 9392 throw new Error("Attempt to auto-create ClaimResponse.preAuthPeriod"); 9393 else if (Configuration.doAutoCreate()) 9394 this.preAuthPeriod = new Period(); // cc 9395 return this.preAuthPeriod; 9396 } 9397 9398 public boolean hasPreAuthPeriod() { 9399 return this.preAuthPeriod != null && !this.preAuthPeriod.isEmpty(); 9400 } 9401 9402 /** 9403 * @param value {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 9404 */ 9405 public ClaimResponse setPreAuthPeriod(Period value) { 9406 this.preAuthPeriod = value; 9407 return this; 9408 } 9409 9410 /** 9411 * @return {@link #event} (Information code for an event with a corresponding date or period.) 9412 */ 9413 public List<ClaimResponseEventComponent> getEvent() { 9414 if (this.event == null) 9415 this.event = new ArrayList<ClaimResponseEventComponent>(); 9416 return this.event; 9417 } 9418 9419 /** 9420 * @return Returns a reference to <code>this</code> for easy method chaining 9421 */ 9422 public ClaimResponse setEvent(List<ClaimResponseEventComponent> theEvent) { 9423 this.event = theEvent; 9424 return this; 9425 } 9426 9427 public boolean hasEvent() { 9428 if (this.event == null) 9429 return false; 9430 for (ClaimResponseEventComponent item : this.event) 9431 if (!item.isEmpty()) 9432 return true; 9433 return false; 9434 } 9435 9436 public ClaimResponseEventComponent addEvent() { //3 9437 ClaimResponseEventComponent t = new ClaimResponseEventComponent(); 9438 if (this.event == null) 9439 this.event = new ArrayList<ClaimResponseEventComponent>(); 9440 this.event.add(t); 9441 return t; 9442 } 9443 9444 public ClaimResponse addEvent(ClaimResponseEventComponent t) { //3 9445 if (t == null) 9446 return this; 9447 if (this.event == null) 9448 this.event = new ArrayList<ClaimResponseEventComponent>(); 9449 this.event.add(t); 9450 return this; 9451 } 9452 9453 /** 9454 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 9455 */ 9456 public ClaimResponseEventComponent getEventFirstRep() { 9457 if (getEvent().isEmpty()) { 9458 addEvent(); 9459 } 9460 return getEvent().get(0); 9461 } 9462 9463 /** 9464 * @return {@link #payeeType} (Type of Party to be reimbursed: subscriber, provider, other.) 9465 */ 9466 public CodeableConcept getPayeeType() { 9467 if (this.payeeType == null) 9468 if (Configuration.errorOnAutoCreate()) 9469 throw new Error("Attempt to auto-create ClaimResponse.payeeType"); 9470 else if (Configuration.doAutoCreate()) 9471 this.payeeType = new CodeableConcept(); // cc 9472 return this.payeeType; 9473 } 9474 9475 public boolean hasPayeeType() { 9476 return this.payeeType != null && !this.payeeType.isEmpty(); 9477 } 9478 9479 /** 9480 * @param value {@link #payeeType} (Type of Party to be reimbursed: subscriber, provider, other.) 9481 */ 9482 public ClaimResponse setPayeeType(CodeableConcept value) { 9483 this.payeeType = value; 9484 return this; 9485 } 9486 9487 /** 9488 * @return {@link #encounter} (Healthcare encounters related to this claim.) 9489 */ 9490 public List<Reference> getEncounter() { 9491 if (this.encounter == null) 9492 this.encounter = new ArrayList<Reference>(); 9493 return this.encounter; 9494 } 9495 9496 /** 9497 * @return Returns a reference to <code>this</code> for easy method chaining 9498 */ 9499 public ClaimResponse setEncounter(List<Reference> theEncounter) { 9500 this.encounter = theEncounter; 9501 return this; 9502 } 9503 9504 public boolean hasEncounter() { 9505 if (this.encounter == null) 9506 return false; 9507 for (Reference item : this.encounter) 9508 if (!item.isEmpty()) 9509 return true; 9510 return false; 9511 } 9512 9513 public Reference addEncounter() { //3 9514 Reference t = new Reference(); 9515 if (this.encounter == null) 9516 this.encounter = new ArrayList<Reference>(); 9517 this.encounter.add(t); 9518 return t; 9519 } 9520 9521 public ClaimResponse addEncounter(Reference t) { //3 9522 if (t == null) 9523 return this; 9524 if (this.encounter == null) 9525 this.encounter = new ArrayList<Reference>(); 9526 this.encounter.add(t); 9527 return this; 9528 } 9529 9530 /** 9531 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 9532 */ 9533 public Reference getEncounterFirstRep() { 9534 if (getEncounter().isEmpty()) { 9535 addEncounter(); 9536 } 9537 return getEncounter().get(0); 9538 } 9539 9540 /** 9541 * @return {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 9542 */ 9543 public CodeableConcept getDiagnosisRelatedGroup() { 9544 if (this.diagnosisRelatedGroup == null) 9545 if (Configuration.errorOnAutoCreate()) 9546 throw new Error("Attempt to auto-create ClaimResponse.diagnosisRelatedGroup"); 9547 else if (Configuration.doAutoCreate()) 9548 this.diagnosisRelatedGroup = new CodeableConcept(); // cc 9549 return this.diagnosisRelatedGroup; 9550 } 9551 9552 public boolean hasDiagnosisRelatedGroup() { 9553 return this.diagnosisRelatedGroup != null && !this.diagnosisRelatedGroup.isEmpty(); 9554 } 9555 9556 /** 9557 * @param value {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 9558 */ 9559 public ClaimResponse setDiagnosisRelatedGroup(CodeableConcept value) { 9560 this.diagnosisRelatedGroup = value; 9561 return this; 9562 } 9563 9564 /** 9565 * @return {@link #item} (A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.) 9566 */ 9567 public List<ItemComponent> getItem() { 9568 if (this.item == null) 9569 this.item = new ArrayList<ItemComponent>(); 9570 return this.item; 9571 } 9572 9573 /** 9574 * @return Returns a reference to <code>this</code> for easy method chaining 9575 */ 9576 public ClaimResponse setItem(List<ItemComponent> theItem) { 9577 this.item = theItem; 9578 return this; 9579 } 9580 9581 public boolean hasItem() { 9582 if (this.item == null) 9583 return false; 9584 for (ItemComponent item : this.item) 9585 if (!item.isEmpty()) 9586 return true; 9587 return false; 9588 } 9589 9590 public ItemComponent addItem() { //3 9591 ItemComponent t = new ItemComponent(); 9592 if (this.item == null) 9593 this.item = new ArrayList<ItemComponent>(); 9594 this.item.add(t); 9595 return t; 9596 } 9597 9598 public ClaimResponse addItem(ItemComponent t) { //3 9599 if (t == null) 9600 return this; 9601 if (this.item == null) 9602 this.item = new ArrayList<ItemComponent>(); 9603 this.item.add(t); 9604 return this; 9605 } 9606 9607 /** 9608 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 9609 */ 9610 public ItemComponent getItemFirstRep() { 9611 if (getItem().isEmpty()) { 9612 addItem(); 9613 } 9614 return getItem().get(0); 9615 } 9616 9617 /** 9618 * @return {@link #addItem} (The first-tier service adjudications for payor added product or service lines.) 9619 */ 9620 public List<AddedItemComponent> getAddItem() { 9621 if (this.addItem == null) 9622 this.addItem = new ArrayList<AddedItemComponent>(); 9623 return this.addItem; 9624 } 9625 9626 /** 9627 * @return Returns a reference to <code>this</code> for easy method chaining 9628 */ 9629 public ClaimResponse setAddItem(List<AddedItemComponent> theAddItem) { 9630 this.addItem = theAddItem; 9631 return this; 9632 } 9633 9634 public boolean hasAddItem() { 9635 if (this.addItem == null) 9636 return false; 9637 for (AddedItemComponent item : this.addItem) 9638 if (!item.isEmpty()) 9639 return true; 9640 return false; 9641 } 9642 9643 public AddedItemComponent addAddItem() { //3 9644 AddedItemComponent t = new AddedItemComponent(); 9645 if (this.addItem == null) 9646 this.addItem = new ArrayList<AddedItemComponent>(); 9647 this.addItem.add(t); 9648 return t; 9649 } 9650 9651 public ClaimResponse addAddItem(AddedItemComponent t) { //3 9652 if (t == null) 9653 return this; 9654 if (this.addItem == null) 9655 this.addItem = new ArrayList<AddedItemComponent>(); 9656 this.addItem.add(t); 9657 return this; 9658 } 9659 9660 /** 9661 * @return The first repetition of repeating field {@link #addItem}, creating it if it does not already exist {3} 9662 */ 9663 public AddedItemComponent getAddItemFirstRep() { 9664 if (getAddItem().isEmpty()) { 9665 addAddItem(); 9666 } 9667 return getAddItem().get(0); 9668 } 9669 9670 /** 9671 * @return {@link #adjudication} (The adjudication results which are presented at the header level rather than at the line-item or add-item levels.) 9672 */ 9673 public List<AdjudicationComponent> getAdjudication() { 9674 if (this.adjudication == null) 9675 this.adjudication = new ArrayList<AdjudicationComponent>(); 9676 return this.adjudication; 9677 } 9678 9679 /** 9680 * @return Returns a reference to <code>this</code> for easy method chaining 9681 */ 9682 public ClaimResponse setAdjudication(List<AdjudicationComponent> theAdjudication) { 9683 this.adjudication = theAdjudication; 9684 return this; 9685 } 9686 9687 public boolean hasAdjudication() { 9688 if (this.adjudication == null) 9689 return false; 9690 for (AdjudicationComponent item : this.adjudication) 9691 if (!item.isEmpty()) 9692 return true; 9693 return false; 9694 } 9695 9696 public AdjudicationComponent addAdjudication() { //3 9697 AdjudicationComponent t = new AdjudicationComponent(); 9698 if (this.adjudication == null) 9699 this.adjudication = new ArrayList<AdjudicationComponent>(); 9700 this.adjudication.add(t); 9701 return t; 9702 } 9703 9704 public ClaimResponse addAdjudication(AdjudicationComponent t) { //3 9705 if (t == null) 9706 return this; 9707 if (this.adjudication == null) 9708 this.adjudication = new ArrayList<AdjudicationComponent>(); 9709 this.adjudication.add(t); 9710 return this; 9711 } 9712 9713 /** 9714 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 9715 */ 9716 public AdjudicationComponent getAdjudicationFirstRep() { 9717 if (getAdjudication().isEmpty()) { 9718 addAdjudication(); 9719 } 9720 return getAdjudication().get(0); 9721 } 9722 9723 /** 9724 * @return {@link #total} (Categorized monetary totals for the adjudication.) 9725 */ 9726 public List<TotalComponent> getTotal() { 9727 if (this.total == null) 9728 this.total = new ArrayList<TotalComponent>(); 9729 return this.total; 9730 } 9731 9732 /** 9733 * @return Returns a reference to <code>this</code> for easy method chaining 9734 */ 9735 public ClaimResponse setTotal(List<TotalComponent> theTotal) { 9736 this.total = theTotal; 9737 return this; 9738 } 9739 9740 public boolean hasTotal() { 9741 if (this.total == null) 9742 return false; 9743 for (TotalComponent item : this.total) 9744 if (!item.isEmpty()) 9745 return true; 9746 return false; 9747 } 9748 9749 public TotalComponent addTotal() { //3 9750 TotalComponent t = new TotalComponent(); 9751 if (this.total == null) 9752 this.total = new ArrayList<TotalComponent>(); 9753 this.total.add(t); 9754 return t; 9755 } 9756 9757 public ClaimResponse addTotal(TotalComponent t) { //3 9758 if (t == null) 9759 return this; 9760 if (this.total == null) 9761 this.total = new ArrayList<TotalComponent>(); 9762 this.total.add(t); 9763 return this; 9764 } 9765 9766 /** 9767 * @return The first repetition of repeating field {@link #total}, creating it if it does not already exist {3} 9768 */ 9769 public TotalComponent getTotalFirstRep() { 9770 if (getTotal().isEmpty()) { 9771 addTotal(); 9772 } 9773 return getTotal().get(0); 9774 } 9775 9776 /** 9777 * @return {@link #payment} (Payment details for the adjudication of the claim.) 9778 */ 9779 public PaymentComponent getPayment() { 9780 if (this.payment == null) 9781 if (Configuration.errorOnAutoCreate()) 9782 throw new Error("Attempt to auto-create ClaimResponse.payment"); 9783 else if (Configuration.doAutoCreate()) 9784 this.payment = new PaymentComponent(); // cc 9785 return this.payment; 9786 } 9787 9788 public boolean hasPayment() { 9789 return this.payment != null && !this.payment.isEmpty(); 9790 } 9791 9792 /** 9793 * @param value {@link #payment} (Payment details for the adjudication of the claim.) 9794 */ 9795 public ClaimResponse setPayment(PaymentComponent value) { 9796 this.payment = value; 9797 return this; 9798 } 9799 9800 /** 9801 * @return {@link #fundsReserve} (A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.) 9802 */ 9803 public CodeableConcept getFundsReserve() { 9804 if (this.fundsReserve == null) 9805 if (Configuration.errorOnAutoCreate()) 9806 throw new Error("Attempt to auto-create ClaimResponse.fundsReserve"); 9807 else if (Configuration.doAutoCreate()) 9808 this.fundsReserve = new CodeableConcept(); // cc 9809 return this.fundsReserve; 9810 } 9811 9812 public boolean hasFundsReserve() { 9813 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 9814 } 9815 9816 /** 9817 * @param value {@link #fundsReserve} (A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.) 9818 */ 9819 public ClaimResponse setFundsReserve(CodeableConcept value) { 9820 this.fundsReserve = value; 9821 return this; 9822 } 9823 9824 /** 9825 * @return {@link #formCode} (A code for the form to be used for printing the content.) 9826 */ 9827 public CodeableConcept getFormCode() { 9828 if (this.formCode == null) 9829 if (Configuration.errorOnAutoCreate()) 9830 throw new Error("Attempt to auto-create ClaimResponse.formCode"); 9831 else if (Configuration.doAutoCreate()) 9832 this.formCode = new CodeableConcept(); // cc 9833 return this.formCode; 9834 } 9835 9836 public boolean hasFormCode() { 9837 return this.formCode != null && !this.formCode.isEmpty(); 9838 } 9839 9840 /** 9841 * @param value {@link #formCode} (A code for the form to be used for printing the content.) 9842 */ 9843 public ClaimResponse setFormCode(CodeableConcept value) { 9844 this.formCode = value; 9845 return this; 9846 } 9847 9848 /** 9849 * @return {@link #form} (The actual form, by reference or inclusion, for printing the content or an EOB.) 9850 */ 9851 public Attachment getForm() { 9852 if (this.form == null) 9853 if (Configuration.errorOnAutoCreate()) 9854 throw new Error("Attempt to auto-create ClaimResponse.form"); 9855 else if (Configuration.doAutoCreate()) 9856 this.form = new Attachment(); // cc 9857 return this.form; 9858 } 9859 9860 public boolean hasForm() { 9861 return this.form != null && !this.form.isEmpty(); 9862 } 9863 9864 /** 9865 * @param value {@link #form} (The actual form, by reference or inclusion, for printing the content or an EOB.) 9866 */ 9867 public ClaimResponse setForm(Attachment value) { 9868 this.form = value; 9869 return this; 9870 } 9871 9872 /** 9873 * @return {@link #processNote} (A note that describes or explains adjudication results in a human readable form.) 9874 */ 9875 public List<NoteComponent> getProcessNote() { 9876 if (this.processNote == null) 9877 this.processNote = new ArrayList<NoteComponent>(); 9878 return this.processNote; 9879 } 9880 9881 /** 9882 * @return Returns a reference to <code>this</code> for easy method chaining 9883 */ 9884 public ClaimResponse setProcessNote(List<NoteComponent> theProcessNote) { 9885 this.processNote = theProcessNote; 9886 return this; 9887 } 9888 9889 public boolean hasProcessNote() { 9890 if (this.processNote == null) 9891 return false; 9892 for (NoteComponent item : this.processNote) 9893 if (!item.isEmpty()) 9894 return true; 9895 return false; 9896 } 9897 9898 public NoteComponent addProcessNote() { //3 9899 NoteComponent t = new NoteComponent(); 9900 if (this.processNote == null) 9901 this.processNote = new ArrayList<NoteComponent>(); 9902 this.processNote.add(t); 9903 return t; 9904 } 9905 9906 public ClaimResponse addProcessNote(NoteComponent t) { //3 9907 if (t == null) 9908 return this; 9909 if (this.processNote == null) 9910 this.processNote = new ArrayList<NoteComponent>(); 9911 this.processNote.add(t); 9912 return this; 9913 } 9914 9915 /** 9916 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist {3} 9917 */ 9918 public NoteComponent getProcessNoteFirstRep() { 9919 if (getProcessNote().isEmpty()) { 9920 addProcessNote(); 9921 } 9922 return getProcessNote().get(0); 9923 } 9924 9925 /** 9926 * @return {@link #communicationRequest} (Request for additional supporting or authorizing information.) 9927 */ 9928 public List<Reference> getCommunicationRequest() { 9929 if (this.communicationRequest == null) 9930 this.communicationRequest = new ArrayList<Reference>(); 9931 return this.communicationRequest; 9932 } 9933 9934 /** 9935 * @return Returns a reference to <code>this</code> for easy method chaining 9936 */ 9937 public ClaimResponse setCommunicationRequest(List<Reference> theCommunicationRequest) { 9938 this.communicationRequest = theCommunicationRequest; 9939 return this; 9940 } 9941 9942 public boolean hasCommunicationRequest() { 9943 if (this.communicationRequest == null) 9944 return false; 9945 for (Reference item : this.communicationRequest) 9946 if (!item.isEmpty()) 9947 return true; 9948 return false; 9949 } 9950 9951 public Reference addCommunicationRequest() { //3 9952 Reference t = new Reference(); 9953 if (this.communicationRequest == null) 9954 this.communicationRequest = new ArrayList<Reference>(); 9955 this.communicationRequest.add(t); 9956 return t; 9957 } 9958 9959 public ClaimResponse addCommunicationRequest(Reference t) { //3 9960 if (t == null) 9961 return this; 9962 if (this.communicationRequest == null) 9963 this.communicationRequest = new ArrayList<Reference>(); 9964 this.communicationRequest.add(t); 9965 return this; 9966 } 9967 9968 /** 9969 * @return The first repetition of repeating field {@link #communicationRequest}, creating it if it does not already exist {3} 9970 */ 9971 public Reference getCommunicationRequestFirstRep() { 9972 if (getCommunicationRequest().isEmpty()) { 9973 addCommunicationRequest(); 9974 } 9975 return getCommunicationRequest().get(0); 9976 } 9977 9978 /** 9979 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services specified on the claim.) 9980 */ 9981 public List<InsuranceComponent> getInsurance() { 9982 if (this.insurance == null) 9983 this.insurance = new ArrayList<InsuranceComponent>(); 9984 return this.insurance; 9985 } 9986 9987 /** 9988 * @return Returns a reference to <code>this</code> for easy method chaining 9989 */ 9990 public ClaimResponse setInsurance(List<InsuranceComponent> theInsurance) { 9991 this.insurance = theInsurance; 9992 return this; 9993 } 9994 9995 public boolean hasInsurance() { 9996 if (this.insurance == null) 9997 return false; 9998 for (InsuranceComponent item : this.insurance) 9999 if (!item.isEmpty()) 10000 return true; 10001 return false; 10002 } 10003 10004 public InsuranceComponent addInsurance() { //3 10005 InsuranceComponent t = new InsuranceComponent(); 10006 if (this.insurance == null) 10007 this.insurance = new ArrayList<InsuranceComponent>(); 10008 this.insurance.add(t); 10009 return t; 10010 } 10011 10012 public ClaimResponse addInsurance(InsuranceComponent t) { //3 10013 if (t == null) 10014 return this; 10015 if (this.insurance == null) 10016 this.insurance = new ArrayList<InsuranceComponent>(); 10017 this.insurance.add(t); 10018 return this; 10019 } 10020 10021 /** 10022 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 10023 */ 10024 public InsuranceComponent getInsuranceFirstRep() { 10025 if (getInsurance().isEmpty()) { 10026 addInsurance(); 10027 } 10028 return getInsurance().get(0); 10029 } 10030 10031 /** 10032 * @return {@link #error} (Errors encountered during the processing of the adjudication.) 10033 */ 10034 public List<ErrorComponent> getError() { 10035 if (this.error == null) 10036 this.error = new ArrayList<ErrorComponent>(); 10037 return this.error; 10038 } 10039 10040 /** 10041 * @return Returns a reference to <code>this</code> for easy method chaining 10042 */ 10043 public ClaimResponse setError(List<ErrorComponent> theError) { 10044 this.error = theError; 10045 return this; 10046 } 10047 10048 public boolean hasError() { 10049 if (this.error == null) 10050 return false; 10051 for (ErrorComponent item : this.error) 10052 if (!item.isEmpty()) 10053 return true; 10054 return false; 10055 } 10056 10057 public ErrorComponent addError() { //3 10058 ErrorComponent t = new ErrorComponent(); 10059 if (this.error == null) 10060 this.error = new ArrayList<ErrorComponent>(); 10061 this.error.add(t); 10062 return t; 10063 } 10064 10065 public ClaimResponse addError(ErrorComponent t) { //3 10066 if (t == null) 10067 return this; 10068 if (this.error == null) 10069 this.error = new ArrayList<ErrorComponent>(); 10070 this.error.add(t); 10071 return this; 10072 } 10073 10074 /** 10075 * @return The first repetition of repeating field {@link #error}, creating it if it does not already exist {3} 10076 */ 10077 public ErrorComponent getErrorFirstRep() { 10078 if (getError().isEmpty()) { 10079 addError(); 10080 } 10081 return getError().get(0); 10082 } 10083 10084 protected void listChildren(List<Property> children) { 10085 super.listChildren(children); 10086 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this claim response.", 0, java.lang.Integer.MAX_VALUE, identifier)); 10087 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 10088 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 10089 children.add(new Property("type", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, type)); 10090 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType)); 10091 children.add(new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use)); 10092 children.add(new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.", 0, 1, patient)); 10093 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 10094 children.add(new Property("insurer", "Reference(Organization)", "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer)); 10095 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, requestor)); 10096 children.add(new Property("request", "Reference(Claim)", "Original request resource reference.", 0, 1, request)); 10097 children.add(new Property("outcome", "code", "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome)); 10098 children.add(new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision)); 10099 children.add(new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition)); 10100 children.add(new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef)); 10101 children.add(new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod)); 10102 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 10103 children.add(new Property("payeeType", "CodeableConcept", "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, payeeType)); 10104 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 10105 children.add(new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup)); 10106 children.add(new Property("item", "", "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item)); 10107 children.add(new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, java.lang.Integer.MAX_VALUE, addItem)); 10108 children.add(new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 10109 children.add(new Property("total", "", "Categorized monetary totals for the adjudication.", 0, java.lang.Integer.MAX_VALUE, total)); 10110 children.add(new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment)); 10111 children.add(new Property("fundsReserve", "CodeableConcept", "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 0, 1, fundsReserve)); 10112 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode)); 10113 children.add(new Property("form", "Attachment", "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form)); 10114 children.add(new Property("processNote", "", "A note that describes or explains adjudication results in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote)); 10115 children.add(new Property("communicationRequest", "Reference(CommunicationRequest)", "Request for additional supporting or authorizing information.", 0, java.lang.Integer.MAX_VALUE, communicationRequest)); 10116 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance)); 10117 children.add(new Property("error", "", "Errors encountered during the processing of the adjudication.", 0, java.lang.Integer.MAX_VALUE, error)); 10118 } 10119 10120 @Override 10121 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10122 switch (_hash) { 10123 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this claim response.", 0, java.lang.Integer.MAX_VALUE, identifier); 10124 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 10125 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 10126 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, type); 10127 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType); 10128 case 116103: /*use*/ return new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use); 10129 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for facast reimbursement is sought.", 0, 1, patient); 10130 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 10131 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer); 10132 case 693934258: /*requestor*/ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, requestor); 10133 case 1095692943: /*request*/ return new Property("request", "Reference(Claim)", "Original request resource reference.", 0, 1, request); 10134 case -1106507950: /*outcome*/ return new Property("outcome", "code", "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome); 10135 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision); 10136 case 583380919: /*disposition*/ return new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition); 10137 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef); 10138 case 1819164812: /*preAuthPeriod*/ return new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod); 10139 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 10140 case -316321118: /*payeeType*/ return new Property("payeeType", "CodeableConcept", "Type of Party to be reimbursed: subscriber, provider, other.", 0, 1, payeeType); 10141 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 10142 case -1599182171: /*diagnosisRelatedGroup*/ return new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup); 10143 case 3242771: /*item*/ return new Property("item", "", "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item); 10144 case -1148899500: /*addItem*/ return new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, java.lang.Integer.MAX_VALUE, addItem); 10145 case -231349275: /*adjudication*/ return new Property("adjudication", "@ClaimResponse.item.adjudication", "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 0, java.lang.Integer.MAX_VALUE, adjudication); 10146 case 110549828: /*total*/ return new Property("total", "", "Categorized monetary totals for the adjudication.", 0, java.lang.Integer.MAX_VALUE, total); 10147 case -786681338: /*payment*/ return new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment); 10148 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 0, 1, fundsReserve); 10149 case 473181393: /*formCode*/ return new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode); 10150 case 3148996: /*form*/ return new Property("form", "Attachment", "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form); 10151 case 202339073: /*processNote*/ return new Property("processNote", "", "A note that describes or explains adjudication results in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote); 10152 case -2071896615: /*communicationRequest*/ return new Property("communicationRequest", "Reference(CommunicationRequest)", "Request for additional supporting or authorizing information.", 0, java.lang.Integer.MAX_VALUE, communicationRequest); 10153 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance); 10154 case 96784904: /*error*/ return new Property("error", "", "Errors encountered during the processing of the adjudication.", 0, java.lang.Integer.MAX_VALUE, error); 10155 default: return super.getNamedProperty(_hash, _name, _checkValid); 10156 } 10157 10158 } 10159 10160 @Override 10161 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10162 switch (hash) { 10163 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 10164 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 10165 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 10166 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 10167 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 10168 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 10169 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 10170 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 10171 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 10172 case 693934258: /*requestor*/ return this.requestor == null ? new Base[0] : new Base[] {this.requestor}; // Reference 10173 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 10174 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<ClaimProcessingCodes> 10175 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 10176 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 10177 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : new Base[] {this.preAuthRef}; // StringType 10178 case 1819164812: /*preAuthPeriod*/ return this.preAuthPeriod == null ? new Base[0] : new Base[] {this.preAuthPeriod}; // Period 10179 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // ClaimResponseEventComponent 10180 case -316321118: /*payeeType*/ return this.payeeType == null ? new Base[0] : new Base[] {this.payeeType}; // CodeableConcept 10181 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 10182 case -1599182171: /*diagnosisRelatedGroup*/ return this.diagnosisRelatedGroup == null ? new Base[0] : new Base[] {this.diagnosisRelatedGroup}; // CodeableConcept 10183 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 10184 case -1148899500: /*addItem*/ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 10185 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 10186 case 110549828: /*total*/ return this.total == null ? new Base[0] : this.total.toArray(new Base[this.total.size()]); // TotalComponent 10187 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // PaymentComponent 10188 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 10189 case 473181393: /*formCode*/ return this.formCode == null ? new Base[0] : new Base[] {this.formCode}; // CodeableConcept 10190 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // Attachment 10191 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 10192 case -2071896615: /*communicationRequest*/ return this.communicationRequest == null ? new Base[0] : this.communicationRequest.toArray(new Base[this.communicationRequest.size()]); // Reference 10193 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 10194 case 96784904: /*error*/ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorComponent 10195 default: return super.getProperty(hash, name, checkValid); 10196 } 10197 10198 } 10199 10200 @Override 10201 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10202 switch (hash) { 10203 case -1618432855: // identifier 10204 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 10205 return value; 10206 case 82505966: // traceNumber 10207 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 10208 return value; 10209 case -892481550: // status 10210 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 10211 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 10212 return value; 10213 case 3575610: // type 10214 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10215 return value; 10216 case -1868521062: // subType 10217 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10218 return value; 10219 case 116103: // use 10220 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 10221 this.use = (Enumeration) value; // Enumeration<Use> 10222 return value; 10223 case -791418107: // patient 10224 this.patient = TypeConvertor.castToReference(value); // Reference 10225 return value; 10226 case 1028554472: // created 10227 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 10228 return value; 10229 case 1957615864: // insurer 10230 this.insurer = TypeConvertor.castToReference(value); // Reference 10231 return value; 10232 case 693934258: // requestor 10233 this.requestor = TypeConvertor.castToReference(value); // Reference 10234 return value; 10235 case 1095692943: // request 10236 this.request = TypeConvertor.castToReference(value); // Reference 10237 return value; 10238 case -1106507950: // outcome 10239 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 10240 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 10241 return value; 10242 case 565719004: // decision 10243 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10244 return value; 10245 case 583380919: // disposition 10246 this.disposition = TypeConvertor.castToString(value); // StringType 10247 return value; 10248 case 522246568: // preAuthRef 10249 this.preAuthRef = TypeConvertor.castToString(value); // StringType 10250 return value; 10251 case 1819164812: // preAuthPeriod 10252 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 10253 return value; 10254 case 96891546: // event 10255 this.getEvent().add((ClaimResponseEventComponent) value); // ClaimResponseEventComponent 10256 return value; 10257 case -316321118: // payeeType 10258 this.payeeType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10259 return value; 10260 case 1524132147: // encounter 10261 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 10262 return value; 10263 case -1599182171: // diagnosisRelatedGroup 10264 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10265 return value; 10266 case 3242771: // item 10267 this.getItem().add((ItemComponent) value); // ItemComponent 10268 return value; 10269 case -1148899500: // addItem 10270 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 10271 return value; 10272 case -231349275: // adjudication 10273 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 10274 return value; 10275 case 110549828: // total 10276 this.getTotal().add((TotalComponent) value); // TotalComponent 10277 return value; 10278 case -786681338: // payment 10279 this.payment = (PaymentComponent) value; // PaymentComponent 10280 return value; 10281 case 1314609806: // fundsReserve 10282 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10283 return value; 10284 case 473181393: // formCode 10285 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10286 return value; 10287 case 3148996: // form 10288 this.form = TypeConvertor.castToAttachment(value); // Attachment 10289 return value; 10290 case 202339073: // processNote 10291 this.getProcessNote().add((NoteComponent) value); // NoteComponent 10292 return value; 10293 case -2071896615: // communicationRequest 10294 this.getCommunicationRequest().add(TypeConvertor.castToReference(value)); // Reference 10295 return value; 10296 case 73049818: // insurance 10297 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 10298 return value; 10299 case 96784904: // error 10300 this.getError().add((ErrorComponent) value); // ErrorComponent 10301 return value; 10302 default: return super.setProperty(hash, name, value); 10303 } 10304 10305 } 10306 10307 @Override 10308 public Base setProperty(String name, Base value) throws FHIRException { 10309 if (name.equals("identifier")) { 10310 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 10311 } else if (name.equals("traceNumber")) { 10312 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 10313 } else if (name.equals("status")) { 10314 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 10315 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 10316 } else if (name.equals("type")) { 10317 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10318 } else if (name.equals("subType")) { 10319 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10320 } else if (name.equals("use")) { 10321 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 10322 this.use = (Enumeration) value; // Enumeration<Use> 10323 } else if (name.equals("patient")) { 10324 this.patient = TypeConvertor.castToReference(value); // Reference 10325 } else if (name.equals("created")) { 10326 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 10327 } else if (name.equals("insurer")) { 10328 this.insurer = TypeConvertor.castToReference(value); // Reference 10329 } else if (name.equals("requestor")) { 10330 this.requestor = TypeConvertor.castToReference(value); // Reference 10331 } else if (name.equals("request")) { 10332 this.request = TypeConvertor.castToReference(value); // Reference 10333 } else if (name.equals("outcome")) { 10334 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 10335 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 10336 } else if (name.equals("decision")) { 10337 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10338 } else if (name.equals("disposition")) { 10339 this.disposition = TypeConvertor.castToString(value); // StringType 10340 } else if (name.equals("preAuthRef")) { 10341 this.preAuthRef = TypeConvertor.castToString(value); // StringType 10342 } else if (name.equals("preAuthPeriod")) { 10343 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 10344 } else if (name.equals("event")) { 10345 this.getEvent().add((ClaimResponseEventComponent) value); 10346 } else if (name.equals("payeeType")) { 10347 this.payeeType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10348 } else if (name.equals("encounter")) { 10349 this.getEncounter().add(TypeConvertor.castToReference(value)); 10350 } else if (name.equals("diagnosisRelatedGroup")) { 10351 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10352 } else if (name.equals("item")) { 10353 this.getItem().add((ItemComponent) value); 10354 } else if (name.equals("addItem")) { 10355 this.getAddItem().add((AddedItemComponent) value); 10356 } else if (name.equals("adjudication")) { 10357 this.getAdjudication().add((AdjudicationComponent) value); 10358 } else if (name.equals("total")) { 10359 this.getTotal().add((TotalComponent) value); 10360 } else if (name.equals("payment")) { 10361 this.payment = (PaymentComponent) value; // PaymentComponent 10362 } else if (name.equals("fundsReserve")) { 10363 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10364 } else if (name.equals("formCode")) { 10365 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10366 } else if (name.equals("form")) { 10367 this.form = TypeConvertor.castToAttachment(value); // Attachment 10368 } else if (name.equals("processNote")) { 10369 this.getProcessNote().add((NoteComponent) value); 10370 } else if (name.equals("communicationRequest")) { 10371 this.getCommunicationRequest().add(TypeConvertor.castToReference(value)); 10372 } else if (name.equals("insurance")) { 10373 this.getInsurance().add((InsuranceComponent) value); 10374 } else if (name.equals("error")) { 10375 this.getError().add((ErrorComponent) value); 10376 } else 10377 return super.setProperty(name, value); 10378 return value; 10379 } 10380 10381 @Override 10382 public void removeChild(String name, Base value) throws FHIRException { 10383 if (name.equals("identifier")) { 10384 this.getIdentifier().remove(value); 10385 } else if (name.equals("traceNumber")) { 10386 this.getTraceNumber().remove(value); 10387 } else if (name.equals("status")) { 10388 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 10389 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 10390 } else if (name.equals("type")) { 10391 this.type = null; 10392 } else if (name.equals("subType")) { 10393 this.subType = null; 10394 } else if (name.equals("use")) { 10395 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 10396 this.use = (Enumeration) value; // Enumeration<Use> 10397 } else if (name.equals("patient")) { 10398 this.patient = null; 10399 } else if (name.equals("created")) { 10400 this.created = null; 10401 } else if (name.equals("insurer")) { 10402 this.insurer = null; 10403 } else if (name.equals("requestor")) { 10404 this.requestor = null; 10405 } else if (name.equals("request")) { 10406 this.request = null; 10407 } else if (name.equals("outcome")) { 10408 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 10409 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 10410 } else if (name.equals("decision")) { 10411 this.decision = null; 10412 } else if (name.equals("disposition")) { 10413 this.disposition = null; 10414 } else if (name.equals("preAuthRef")) { 10415 this.preAuthRef = null; 10416 } else if (name.equals("preAuthPeriod")) { 10417 this.preAuthPeriod = null; 10418 } else if (name.equals("event")) { 10419 this.getEvent().remove((ClaimResponseEventComponent) value); 10420 } else if (name.equals("payeeType")) { 10421 this.payeeType = null; 10422 } else if (name.equals("encounter")) { 10423 this.getEncounter().remove(value); 10424 } else if (name.equals("diagnosisRelatedGroup")) { 10425 this.diagnosisRelatedGroup = null; 10426 } else if (name.equals("item")) { 10427 this.getItem().remove((ItemComponent) value); 10428 } else if (name.equals("addItem")) { 10429 this.getAddItem().remove((AddedItemComponent) value); 10430 } else if (name.equals("adjudication")) { 10431 this.getAdjudication().remove((AdjudicationComponent) value); 10432 } else if (name.equals("total")) { 10433 this.getTotal().remove((TotalComponent) value); 10434 } else if (name.equals("payment")) { 10435 this.payment = (PaymentComponent) value; // PaymentComponent 10436 } else if (name.equals("fundsReserve")) { 10437 this.fundsReserve = null; 10438 } else if (name.equals("formCode")) { 10439 this.formCode = null; 10440 } else if (name.equals("form")) { 10441 this.form = null; 10442 } else if (name.equals("processNote")) { 10443 this.getProcessNote().remove((NoteComponent) value); 10444 } else if (name.equals("communicationRequest")) { 10445 this.getCommunicationRequest().remove(value); 10446 } else if (name.equals("insurance")) { 10447 this.getInsurance().remove((InsuranceComponent) value); 10448 } else if (name.equals("error")) { 10449 this.getError().remove((ErrorComponent) value); 10450 } else 10451 super.removeChild(name, value); 10452 10453 } 10454 10455 @Override 10456 public Base makeProperty(int hash, String name) throws FHIRException { 10457 switch (hash) { 10458 case -1618432855: return addIdentifier(); 10459 case 82505966: return addTraceNumber(); 10460 case -892481550: return getStatusElement(); 10461 case 3575610: return getType(); 10462 case -1868521062: return getSubType(); 10463 case 116103: return getUseElement(); 10464 case -791418107: return getPatient(); 10465 case 1028554472: return getCreatedElement(); 10466 case 1957615864: return getInsurer(); 10467 case 693934258: return getRequestor(); 10468 case 1095692943: return getRequest(); 10469 case -1106507950: return getOutcomeElement(); 10470 case 565719004: return getDecision(); 10471 case 583380919: return getDispositionElement(); 10472 case 522246568: return getPreAuthRefElement(); 10473 case 1819164812: return getPreAuthPeriod(); 10474 case 96891546: return addEvent(); 10475 case -316321118: return getPayeeType(); 10476 case 1524132147: return addEncounter(); 10477 case -1599182171: return getDiagnosisRelatedGroup(); 10478 case 3242771: return addItem(); 10479 case -1148899500: return addAddItem(); 10480 case -231349275: return addAdjudication(); 10481 case 110549828: return addTotal(); 10482 case -786681338: return getPayment(); 10483 case 1314609806: return getFundsReserve(); 10484 case 473181393: return getFormCode(); 10485 case 3148996: return getForm(); 10486 case 202339073: return addProcessNote(); 10487 case -2071896615: return addCommunicationRequest(); 10488 case 73049818: return addInsurance(); 10489 case 96784904: return addError(); 10490 default: return super.makeProperty(hash, name); 10491 } 10492 10493 } 10494 10495 @Override 10496 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10497 switch (hash) { 10498 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 10499 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 10500 case -892481550: /*status*/ return new String[] {"code"}; 10501 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 10502 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 10503 case 116103: /*use*/ return new String[] {"code"}; 10504 case -791418107: /*patient*/ return new String[] {"Reference"}; 10505 case 1028554472: /*created*/ return new String[] {"dateTime"}; 10506 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 10507 case 693934258: /*requestor*/ return new String[] {"Reference"}; 10508 case 1095692943: /*request*/ return new String[] {"Reference"}; 10509 case -1106507950: /*outcome*/ return new String[] {"code"}; 10510 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 10511 case 583380919: /*disposition*/ return new String[] {"string"}; 10512 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 10513 case 1819164812: /*preAuthPeriod*/ return new String[] {"Period"}; 10514 case 96891546: /*event*/ return new String[] {}; 10515 case -316321118: /*payeeType*/ return new String[] {"CodeableConcept"}; 10516 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 10517 case -1599182171: /*diagnosisRelatedGroup*/ return new String[] {"CodeableConcept"}; 10518 case 3242771: /*item*/ return new String[] {}; 10519 case -1148899500: /*addItem*/ return new String[] {}; 10520 case -231349275: /*adjudication*/ return new String[] {"@ClaimResponse.item.adjudication"}; 10521 case 110549828: /*total*/ return new String[] {}; 10522 case -786681338: /*payment*/ return new String[] {}; 10523 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 10524 case 473181393: /*formCode*/ return new String[] {"CodeableConcept"}; 10525 case 3148996: /*form*/ return new String[] {"Attachment"}; 10526 case 202339073: /*processNote*/ return new String[] {}; 10527 case -2071896615: /*communicationRequest*/ return new String[] {"Reference"}; 10528 case 73049818: /*insurance*/ return new String[] {}; 10529 case 96784904: /*error*/ return new String[] {}; 10530 default: return super.getTypesForProperty(hash, name); 10531 } 10532 10533 } 10534 10535 @Override 10536 public Base addChild(String name) throws FHIRException { 10537 if (name.equals("identifier")) { 10538 return addIdentifier(); 10539 } 10540 else if (name.equals("traceNumber")) { 10541 return addTraceNumber(); 10542 } 10543 else if (name.equals("status")) { 10544 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.status"); 10545 } 10546 else if (name.equals("type")) { 10547 this.type = new CodeableConcept(); 10548 return this.type; 10549 } 10550 else if (name.equals("subType")) { 10551 this.subType = new CodeableConcept(); 10552 return this.subType; 10553 } 10554 else if (name.equals("use")) { 10555 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.use"); 10556 } 10557 else if (name.equals("patient")) { 10558 this.patient = new Reference(); 10559 return this.patient; 10560 } 10561 else if (name.equals("created")) { 10562 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.created"); 10563 } 10564 else if (name.equals("insurer")) { 10565 this.insurer = new Reference(); 10566 return this.insurer; 10567 } 10568 else if (name.equals("requestor")) { 10569 this.requestor = new Reference(); 10570 return this.requestor; 10571 } 10572 else if (name.equals("request")) { 10573 this.request = new Reference(); 10574 return this.request; 10575 } 10576 else if (name.equals("outcome")) { 10577 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.outcome"); 10578 } 10579 else if (name.equals("decision")) { 10580 this.decision = new CodeableConcept(); 10581 return this.decision; 10582 } 10583 else if (name.equals("disposition")) { 10584 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.disposition"); 10585 } 10586 else if (name.equals("preAuthRef")) { 10587 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.preAuthRef"); 10588 } 10589 else if (name.equals("preAuthPeriod")) { 10590 this.preAuthPeriod = new Period(); 10591 return this.preAuthPeriod; 10592 } 10593 else if (name.equals("event")) { 10594 return addEvent(); 10595 } 10596 else if (name.equals("payeeType")) { 10597 this.payeeType = new CodeableConcept(); 10598 return this.payeeType; 10599 } 10600 else if (name.equals("encounter")) { 10601 return addEncounter(); 10602 } 10603 else if (name.equals("diagnosisRelatedGroup")) { 10604 this.diagnosisRelatedGroup = new CodeableConcept(); 10605 return this.diagnosisRelatedGroup; 10606 } 10607 else if (name.equals("item")) { 10608 return addItem(); 10609 } 10610 else if (name.equals("addItem")) { 10611 return addAddItem(); 10612 } 10613 else if (name.equals("adjudication")) { 10614 return addAdjudication(); 10615 } 10616 else if (name.equals("total")) { 10617 return addTotal(); 10618 } 10619 else if (name.equals("payment")) { 10620 this.payment = new PaymentComponent(); 10621 return this.payment; 10622 } 10623 else if (name.equals("fundsReserve")) { 10624 this.fundsReserve = new CodeableConcept(); 10625 return this.fundsReserve; 10626 } 10627 else if (name.equals("formCode")) { 10628 this.formCode = new CodeableConcept(); 10629 return this.formCode; 10630 } 10631 else if (name.equals("form")) { 10632 this.form = new Attachment(); 10633 return this.form; 10634 } 10635 else if (name.equals("processNote")) { 10636 return addProcessNote(); 10637 } 10638 else if (name.equals("communicationRequest")) { 10639 return addCommunicationRequest(); 10640 } 10641 else if (name.equals("insurance")) { 10642 return addInsurance(); 10643 } 10644 else if (name.equals("error")) { 10645 return addError(); 10646 } 10647 else 10648 return super.addChild(name); 10649 } 10650 10651 public String fhirType() { 10652 return "ClaimResponse"; 10653 10654 } 10655 10656 public ClaimResponse copy() { 10657 ClaimResponse dst = new ClaimResponse(); 10658 copyValues(dst); 10659 return dst; 10660 } 10661 10662 public void copyValues(ClaimResponse dst) { 10663 super.copyValues(dst); 10664 if (identifier != null) { 10665 dst.identifier = new ArrayList<Identifier>(); 10666 for (Identifier i : identifier) 10667 dst.identifier.add(i.copy()); 10668 }; 10669 if (traceNumber != null) { 10670 dst.traceNumber = new ArrayList<Identifier>(); 10671 for (Identifier i : traceNumber) 10672 dst.traceNumber.add(i.copy()); 10673 }; 10674 dst.status = status == null ? null : status.copy(); 10675 dst.type = type == null ? null : type.copy(); 10676 dst.subType = subType == null ? null : subType.copy(); 10677 dst.use = use == null ? null : use.copy(); 10678 dst.patient = patient == null ? null : patient.copy(); 10679 dst.created = created == null ? null : created.copy(); 10680 dst.insurer = insurer == null ? null : insurer.copy(); 10681 dst.requestor = requestor == null ? null : requestor.copy(); 10682 dst.request = request == null ? null : request.copy(); 10683 dst.outcome = outcome == null ? null : outcome.copy(); 10684 dst.decision = decision == null ? null : decision.copy(); 10685 dst.disposition = disposition == null ? null : disposition.copy(); 10686 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 10687 dst.preAuthPeriod = preAuthPeriod == null ? null : preAuthPeriod.copy(); 10688 if (event != null) { 10689 dst.event = new ArrayList<ClaimResponseEventComponent>(); 10690 for (ClaimResponseEventComponent i : event) 10691 dst.event.add(i.copy()); 10692 }; 10693 dst.payeeType = payeeType == null ? null : payeeType.copy(); 10694 if (encounter != null) { 10695 dst.encounter = new ArrayList<Reference>(); 10696 for (Reference i : encounter) 10697 dst.encounter.add(i.copy()); 10698 }; 10699 dst.diagnosisRelatedGroup = diagnosisRelatedGroup == null ? null : diagnosisRelatedGroup.copy(); 10700 if (item != null) { 10701 dst.item = new ArrayList<ItemComponent>(); 10702 for (ItemComponent i : item) 10703 dst.item.add(i.copy()); 10704 }; 10705 if (addItem != null) { 10706 dst.addItem = new ArrayList<AddedItemComponent>(); 10707 for (AddedItemComponent i : addItem) 10708 dst.addItem.add(i.copy()); 10709 }; 10710 if (adjudication != null) { 10711 dst.adjudication = new ArrayList<AdjudicationComponent>(); 10712 for (AdjudicationComponent i : adjudication) 10713 dst.adjudication.add(i.copy()); 10714 }; 10715 if (total != null) { 10716 dst.total = new ArrayList<TotalComponent>(); 10717 for (TotalComponent i : total) 10718 dst.total.add(i.copy()); 10719 }; 10720 dst.payment = payment == null ? null : payment.copy(); 10721 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 10722 dst.formCode = formCode == null ? null : formCode.copy(); 10723 dst.form = form == null ? null : form.copy(); 10724 if (processNote != null) { 10725 dst.processNote = new ArrayList<NoteComponent>(); 10726 for (NoteComponent i : processNote) 10727 dst.processNote.add(i.copy()); 10728 }; 10729 if (communicationRequest != null) { 10730 dst.communicationRequest = new ArrayList<Reference>(); 10731 for (Reference i : communicationRequest) 10732 dst.communicationRequest.add(i.copy()); 10733 }; 10734 if (insurance != null) { 10735 dst.insurance = new ArrayList<InsuranceComponent>(); 10736 for (InsuranceComponent i : insurance) 10737 dst.insurance.add(i.copy()); 10738 }; 10739 if (error != null) { 10740 dst.error = new ArrayList<ErrorComponent>(); 10741 for (ErrorComponent i : error) 10742 dst.error.add(i.copy()); 10743 }; 10744 } 10745 10746 protected ClaimResponse typedCopy() { 10747 return copy(); 10748 } 10749 10750 @Override 10751 public boolean equalsDeep(Base other_) { 10752 if (!super.equalsDeep(other_)) 10753 return false; 10754 if (!(other_ instanceof ClaimResponse)) 10755 return false; 10756 ClaimResponse o = (ClaimResponse) other_; 10757 return compareDeep(identifier, o.identifier, true) && compareDeep(traceNumber, o.traceNumber, true) 10758 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 10759 && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) && compareDeep(created, o.created, true) 10760 && compareDeep(insurer, o.insurer, true) && compareDeep(requestor, o.requestor, true) && compareDeep(request, o.request, true) 10761 && compareDeep(outcome, o.outcome, true) && compareDeep(decision, o.decision, true) && compareDeep(disposition, o.disposition, true) 10762 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(preAuthPeriod, o.preAuthPeriod, true) 10763 && compareDeep(event, o.event, true) && compareDeep(payeeType, o.payeeType, true) && compareDeep(encounter, o.encounter, true) 10764 && compareDeep(diagnosisRelatedGroup, o.diagnosisRelatedGroup, true) && compareDeep(item, o.item, true) 10765 && compareDeep(addItem, o.addItem, true) && compareDeep(adjudication, o.adjudication, true) && compareDeep(total, o.total, true) 10766 && compareDeep(payment, o.payment, true) && compareDeep(fundsReserve, o.fundsReserve, true) && compareDeep(formCode, o.formCode, true) 10767 && compareDeep(form, o.form, true) && compareDeep(processNote, o.processNote, true) && compareDeep(communicationRequest, o.communicationRequest, true) 10768 && compareDeep(insurance, o.insurance, true) && compareDeep(error, o.error, true); 10769 } 10770 10771 @Override 10772 public boolean equalsShallow(Base other_) { 10773 if (!super.equalsShallow(other_)) 10774 return false; 10775 if (!(other_ instanceof ClaimResponse)) 10776 return false; 10777 ClaimResponse o = (ClaimResponse) other_; 10778 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 10779 && compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true) 10780 ; 10781 } 10782 10783 public boolean isEmpty() { 10784 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, traceNumber, status 10785 , type, subType, use, patient, created, insurer, requestor, request, outcome 10786 , decision, disposition, preAuthRef, preAuthPeriod, event, payeeType, encounter 10787 , diagnosisRelatedGroup, item, addItem, adjudication, total, payment, fundsReserve 10788 , formCode, form, processNote, communicationRequest, insurance, error); 10789 } 10790 10791 @Override 10792 public ResourceType getResourceType() { 10793 return ResourceType.ClaimResponse; 10794 } 10795 10796 /** 10797 * Search parameter: <b>created</b> 10798 * <p> 10799 * Description: <b>The creation date</b><br> 10800 * Type: <b>date</b><br> 10801 * Path: <b>ClaimResponse.created</b><br> 10802 * </p> 10803 */ 10804 @SearchParamDefinition(name="created", path="ClaimResponse.created", description="The creation date", type="date" ) 10805 public static final String SP_CREATED = "created"; 10806 /** 10807 * <b>Fluent Client</b> search parameter constant for <b>created</b> 10808 * <p> 10809 * Description: <b>The creation date</b><br> 10810 * Type: <b>date</b><br> 10811 * Path: <b>ClaimResponse.created</b><br> 10812 * </p> 10813 */ 10814 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 10815 10816 /** 10817 * Search parameter: <b>disposition</b> 10818 * <p> 10819 * Description: <b>The contents of the disposition message</b><br> 10820 * Type: <b>string</b><br> 10821 * Path: <b>ClaimResponse.disposition</b><br> 10822 * </p> 10823 */ 10824 @SearchParamDefinition(name="disposition", path="ClaimResponse.disposition", description="The contents of the disposition message", type="string" ) 10825 public static final String SP_DISPOSITION = "disposition"; 10826 /** 10827 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 10828 * <p> 10829 * Description: <b>The contents of the disposition message</b><br> 10830 * Type: <b>string</b><br> 10831 * Path: <b>ClaimResponse.disposition</b><br> 10832 * </p> 10833 */ 10834 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 10835 10836 /** 10837 * Search parameter: <b>insurer</b> 10838 * <p> 10839 * Description: <b>The organization which generated this resource</b><br> 10840 * Type: <b>reference</b><br> 10841 * Path: <b>ClaimResponse.insurer</b><br> 10842 * </p> 10843 */ 10844 @SearchParamDefinition(name="insurer", path="ClaimResponse.insurer", description="The organization which generated this resource", type="reference", target={Organization.class } ) 10845 public static final String SP_INSURER = "insurer"; 10846 /** 10847 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 10848 * <p> 10849 * Description: <b>The organization which generated this resource</b><br> 10850 * Type: <b>reference</b><br> 10851 * Path: <b>ClaimResponse.insurer</b><br> 10852 * </p> 10853 */ 10854 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 10855 10856/** 10857 * Constant for fluent queries to be used to add include statements. Specifies 10858 * the path value of "<b>ClaimResponse:insurer</b>". 10859 */ 10860 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("ClaimResponse:insurer").toLocked(); 10861 10862 /** 10863 * Search parameter: <b>outcome</b> 10864 * <p> 10865 * Description: <b>The processing outcome</b><br> 10866 * Type: <b>token</b><br> 10867 * Path: <b>ClaimResponse.outcome</b><br> 10868 * </p> 10869 */ 10870 @SearchParamDefinition(name="outcome", path="ClaimResponse.outcome", description="The processing outcome", type="token" ) 10871 public static final String SP_OUTCOME = "outcome"; 10872 /** 10873 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 10874 * <p> 10875 * Description: <b>The processing outcome</b><br> 10876 * Type: <b>token</b><br> 10877 * Path: <b>ClaimResponse.outcome</b><br> 10878 * </p> 10879 */ 10880 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 10881 10882 /** 10883 * Search parameter: <b>payment-date</b> 10884 * <p> 10885 * Description: <b>The expected payment date</b><br> 10886 * Type: <b>date</b><br> 10887 * Path: <b>ClaimResponse.payment.date</b><br> 10888 * </p> 10889 */ 10890 @SearchParamDefinition(name="payment-date", path="ClaimResponse.payment.date", description="The expected payment date", type="date" ) 10891 public static final String SP_PAYMENT_DATE = "payment-date"; 10892 /** 10893 * <b>Fluent Client</b> search parameter constant for <b>payment-date</b> 10894 * <p> 10895 * Description: <b>The expected payment date</b><br> 10896 * Type: <b>date</b><br> 10897 * Path: <b>ClaimResponse.payment.date</b><br> 10898 * </p> 10899 */ 10900 public static final ca.uhn.fhir.rest.gclient.DateClientParam PAYMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PAYMENT_DATE); 10901 10902 /** 10903 * Search parameter: <b>request</b> 10904 * <p> 10905 * Description: <b>The claim reference</b><br> 10906 * Type: <b>reference</b><br> 10907 * Path: <b>ClaimResponse.request</b><br> 10908 * </p> 10909 */ 10910 @SearchParamDefinition(name="request", path="ClaimResponse.request", description="The claim reference", type="reference", target={Claim.class } ) 10911 public static final String SP_REQUEST = "request"; 10912 /** 10913 * <b>Fluent Client</b> search parameter constant for <b>request</b> 10914 * <p> 10915 * Description: <b>The claim reference</b><br> 10916 * Type: <b>reference</b><br> 10917 * Path: <b>ClaimResponse.request</b><br> 10918 * </p> 10919 */ 10920 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 10921 10922/** 10923 * Constant for fluent queries to be used to add include statements. Specifies 10924 * the path value of "<b>ClaimResponse:request</b>". 10925 */ 10926 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("ClaimResponse:request").toLocked(); 10927 10928 /** 10929 * Search parameter: <b>requestor</b> 10930 * <p> 10931 * Description: <b>The Provider of the claim</b><br> 10932 * Type: <b>reference</b><br> 10933 * Path: <b>ClaimResponse.requestor</b><br> 10934 * </p> 10935 */ 10936 @SearchParamDefinition(name="requestor", path="ClaimResponse.requestor", description="The Provider of the claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 10937 public static final String SP_REQUESTOR = "requestor"; 10938 /** 10939 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 10940 * <p> 10941 * Description: <b>The Provider of the claim</b><br> 10942 * Type: <b>reference</b><br> 10943 * Path: <b>ClaimResponse.requestor</b><br> 10944 * </p> 10945 */ 10946 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTOR); 10947 10948/** 10949 * Constant for fluent queries to be used to add include statements. Specifies 10950 * the path value of "<b>ClaimResponse:requestor</b>". 10951 */ 10952 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include("ClaimResponse:requestor").toLocked(); 10953 10954 /** 10955 * Search parameter: <b>status</b> 10956 * <p> 10957 * Description: <b>The status of the ClaimResponse</b><br> 10958 * Type: <b>token</b><br> 10959 * Path: <b>ClaimResponse.status</b><br> 10960 * </p> 10961 */ 10962 @SearchParamDefinition(name="status", path="ClaimResponse.status", description="The status of the ClaimResponse", type="token" ) 10963 public static final String SP_STATUS = "status"; 10964 /** 10965 * <b>Fluent Client</b> search parameter constant for <b>status</b> 10966 * <p> 10967 * Description: <b>The status of the ClaimResponse</b><br> 10968 * Type: <b>token</b><br> 10969 * Path: <b>ClaimResponse.status</b><br> 10970 * </p> 10971 */ 10972 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 10973 10974 /** 10975 * Search parameter: <b>use</b> 10976 * <p> 10977 * Description: <b>The type of claim</b><br> 10978 * Type: <b>token</b><br> 10979 * Path: <b>ClaimResponse.use</b><br> 10980 * </p> 10981 */ 10982 @SearchParamDefinition(name="use", path="ClaimResponse.use", description="The type of claim", type="token" ) 10983 public static final String SP_USE = "use"; 10984 /** 10985 * <b>Fluent Client</b> search parameter constant for <b>use</b> 10986 * <p> 10987 * Description: <b>The type of claim</b><br> 10988 * Type: <b>token</b><br> 10989 * Path: <b>ClaimResponse.use</b><br> 10990 * </p> 10991 */ 10992 public static final ca.uhn.fhir.rest.gclient.TokenClientParam USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_USE); 10993 10994 /** 10995 * Search parameter: <b>identifier</b> 10996 * <p> 10997 * Description: <b>Multiple Resources: 10998 10999* [Account](account.html): Account number 11000* [AdverseEvent](adverseevent.html): Business identifier for the event 11001* [AllergyIntolerance](allergyintolerance.html): External ids for this item 11002* [Appointment](appointment.html): An Identifier of the Appointment 11003* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 11004* [Basic](basic.html): Business identifier 11005* [BodyStructure](bodystructure.html): Bodystructure identifier 11006* [CarePlan](careplan.html): External Ids for this plan 11007* [CareTeam](careteam.html): External Ids for this team 11008* [ChargeItem](chargeitem.html): Business Identifier for item 11009* [Claim](claim.html): The primary identifier of the financial resource 11010* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 11011* [ClinicalImpression](clinicalimpression.html): Business identifier 11012* [Communication](communication.html): Unique identifier 11013* [CommunicationRequest](communicationrequest.html): Unique identifier 11014* [Composition](composition.html): Version-independent identifier for the Composition 11015* [Condition](condition.html): A unique identifier of the condition record 11016* [Consent](consent.html): Identifier for this record (external references) 11017* [Contract](contract.html): The identity of the contract 11018* [Coverage](coverage.html): The primary identifier of the insured and the coverage 11019* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 11020* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 11021* [DetectedIssue](detectedissue.html): Unique id for the detected issue 11022* [DeviceRequest](devicerequest.html): Business identifier for request/order 11023* [DeviceUsage](deviceusage.html): Search by identifier 11024* [DiagnosticReport](diagnosticreport.html): An identifier for the report 11025* [DocumentReference](documentreference.html): Identifier of the attachment binary 11026* [Encounter](encounter.html): Identifier(s) by which this encounter is known 11027* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 11028* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 11029* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 11030* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 11031* [Flag](flag.html): Business identifier 11032* [Goal](goal.html): External Ids for this goal 11033* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 11034* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 11035* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 11036* [Immunization](immunization.html): Business identifier 11037* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 11038* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 11039* [Invoice](invoice.html): Business Identifier for item 11040* [List](list.html): Business identifier 11041* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 11042* [Medication](medication.html): Returns medications with this external identifier 11043* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 11044* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 11045* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 11046* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 11047* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 11048* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 11049* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 11050* [Observation](observation.html): The unique id for a particular observation 11051* [Person](person.html): A person Identifier 11052* [Procedure](procedure.html): A unique identifier for a procedure 11053* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 11054* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 11055* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 11056* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 11057* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 11058* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 11059* [Specimen](specimen.html): The unique identifier associated with the specimen 11060* [SupplyDelivery](supplydelivery.html): External identifier 11061* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 11062* [Task](task.html): Search for a task instance by its business identifier 11063* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 11064</b><br> 11065 * Type: <b>token</b><br> 11066 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 11067 * </p> 11068 */ 11069 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 11070 public static final String SP_IDENTIFIER = "identifier"; 11071 /** 11072 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 11073 * <p> 11074 * Description: <b>Multiple Resources: 11075 11076* [Account](account.html): Account number 11077* [AdverseEvent](adverseevent.html): Business identifier for the event 11078* [AllergyIntolerance](allergyintolerance.html): External ids for this item 11079* [Appointment](appointment.html): An Identifier of the Appointment 11080* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 11081* [Basic](basic.html): Business identifier 11082* [BodyStructure](bodystructure.html): Bodystructure identifier 11083* [CarePlan](careplan.html): External Ids for this plan 11084* [CareTeam](careteam.html): External Ids for this team 11085* [ChargeItem](chargeitem.html): Business Identifier for item 11086* [Claim](claim.html): The primary identifier of the financial resource 11087* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 11088* [ClinicalImpression](clinicalimpression.html): Business identifier 11089* [Communication](communication.html): Unique identifier 11090* [CommunicationRequest](communicationrequest.html): Unique identifier 11091* [Composition](composition.html): Version-independent identifier for the Composition 11092* [Condition](condition.html): A unique identifier of the condition record 11093* [Consent](consent.html): Identifier for this record (external references) 11094* [Contract](contract.html): The identity of the contract 11095* [Coverage](coverage.html): The primary identifier of the insured and the coverage 11096* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 11097* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 11098* [DetectedIssue](detectedissue.html): Unique id for the detected issue 11099* [DeviceRequest](devicerequest.html): Business identifier for request/order 11100* [DeviceUsage](deviceusage.html): Search by identifier 11101* [DiagnosticReport](diagnosticreport.html): An identifier for the report 11102* [DocumentReference](documentreference.html): Identifier of the attachment binary 11103* [Encounter](encounter.html): Identifier(s) by which this encounter is known 11104* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 11105* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 11106* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 11107* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 11108* [Flag](flag.html): Business identifier 11109* [Goal](goal.html): External Ids for this goal 11110* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 11111* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 11112* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 11113* [Immunization](immunization.html): Business identifier 11114* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 11115* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 11116* [Invoice](invoice.html): Business Identifier for item 11117* [List](list.html): Business identifier 11118* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 11119* [Medication](medication.html): Returns medications with this external identifier 11120* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 11121* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 11122* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 11123* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 11124* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 11125* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 11126* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 11127* [Observation](observation.html): The unique id for a particular observation 11128* [Person](person.html): A person Identifier 11129* [Procedure](procedure.html): A unique identifier for a procedure 11130* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 11131* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 11132* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 11133* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 11134* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 11135* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 11136* [Specimen](specimen.html): The unique identifier associated with the specimen 11137* [SupplyDelivery](supplydelivery.html): External identifier 11138* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 11139* [Task](task.html): Search for a task instance by its business identifier 11140* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 11141</b><br> 11142 * Type: <b>token</b><br> 11143 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 11144 * </p> 11145 */ 11146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 11147 11148 /** 11149 * Search parameter: <b>patient</b> 11150 * <p> 11151 * Description: <b>Multiple Resources: 11152 11153* [Account](account.html): The entity that caused the expenses 11154* [AdverseEvent](adverseevent.html): Subject impacted by event 11155* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 11156* [Appointment](appointment.html): One of the individuals of the appointment is this patient 11157* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 11158* [AuditEvent](auditevent.html): Where the activity involved patient data 11159* [Basic](basic.html): Identifies the focus of this resource 11160* [BodyStructure](bodystructure.html): Who this is about 11161* [CarePlan](careplan.html): Who the care plan is for 11162* [CareTeam](careteam.html): Who care team is for 11163* [ChargeItem](chargeitem.html): Individual service was done for/to 11164* [Claim](claim.html): Patient receiving the products or services 11165* [ClaimResponse](claimresponse.html): The subject of care 11166* [ClinicalImpression](clinicalimpression.html): Patient assessed 11167* [Communication](communication.html): Focus of message 11168* [CommunicationRequest](communicationrequest.html): Focus of message 11169* [Composition](composition.html): Who and/or what the composition is about 11170* [Condition](condition.html): Who has the condition? 11171* [Consent](consent.html): Who the consent applies to 11172* [Contract](contract.html): The identity of the subject of the contract (if a patient) 11173* [Coverage](coverage.html): Retrieve coverages for a patient 11174* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 11175* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 11176* [DetectedIssue](detectedissue.html): Associated patient 11177* [DeviceRequest](devicerequest.html): Individual the service is ordered for 11178* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 11179* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 11180* [DocumentReference](documentreference.html): Who/what is the subject of the document 11181* [Encounter](encounter.html): The patient present at the encounter 11182* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 11183* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 11184* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 11185* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 11186* [Flag](flag.html): The identity of a subject to list flags for 11187* [Goal](goal.html): Who this goal is intended for 11188* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 11189* [ImagingSelection](imagingselection.html): Who the study is about 11190* [ImagingStudy](imagingstudy.html): Who the study is about 11191* [Immunization](immunization.html): The patient for the vaccination record 11192* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 11193* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 11194* [Invoice](invoice.html): Recipient(s) of goods and services 11195* [List](list.html): If all resources have the same subject 11196* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 11197* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 11198* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 11199* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 11200* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 11201* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 11202* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 11203* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 11204* [Observation](observation.html): The subject that the observation is about (if patient) 11205* [Person](person.html): The Person links to this Patient 11206* [Procedure](procedure.html): Search by subject - a patient 11207* [Provenance](provenance.html): Where the activity involved patient data 11208* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 11209* [RelatedPerson](relatedperson.html): The patient this related person is related to 11210* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 11211* [ResearchSubject](researchsubject.html): Who or what is part of study 11212* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 11213* [ServiceRequest](servicerequest.html): Search by subject - a patient 11214* [Specimen](specimen.html): The patient the specimen comes from 11215* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 11216* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 11217* [Task](task.html): Search by patient 11218* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 11219</b><br> 11220 * Type: <b>reference</b><br> 11221 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 11222 * </p> 11223 */ 11224 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 11225 public static final String SP_PATIENT = "patient"; 11226 /** 11227 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 11228 * <p> 11229 * Description: <b>Multiple Resources: 11230 11231* [Account](account.html): The entity that caused the expenses 11232* [AdverseEvent](adverseevent.html): Subject impacted by event 11233* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 11234* [Appointment](appointment.html): One of the individuals of the appointment is this patient 11235* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 11236* [AuditEvent](auditevent.html): Where the activity involved patient data 11237* [Basic](basic.html): Identifies the focus of this resource 11238* [BodyStructure](bodystructure.html): Who this is about 11239* [CarePlan](careplan.html): Who the care plan is for 11240* [CareTeam](careteam.html): Who care team is for 11241* [ChargeItem](chargeitem.html): Individual service was done for/to 11242* [Claim](claim.html): Patient receiving the products or services 11243* [ClaimResponse](claimresponse.html): The subject of care 11244* [ClinicalImpression](clinicalimpression.html): Patient assessed 11245* [Communication](communication.html): Focus of message 11246* [CommunicationRequest](communicationrequest.html): Focus of message 11247* [Composition](composition.html): Who and/or what the composition is about 11248* [Condition](condition.html): Who has the condition? 11249* [Consent](consent.html): Who the consent applies to 11250* [Contract](contract.html): The identity of the subject of the contract (if a patient) 11251* [Coverage](coverage.html): Retrieve coverages for a patient 11252* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 11253* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 11254* [DetectedIssue](detectedissue.html): Associated patient 11255* [DeviceRequest](devicerequest.html): Individual the service is ordered for 11256* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 11257* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 11258* [DocumentReference](documentreference.html): Who/what is the subject of the document 11259* [Encounter](encounter.html): The patient present at the encounter 11260* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 11261* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 11262* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 11263* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 11264* [Flag](flag.html): The identity of a subject to list flags for 11265* [Goal](goal.html): Who this goal is intended for 11266* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 11267* [ImagingSelection](imagingselection.html): Who the study is about 11268* [ImagingStudy](imagingstudy.html): Who the study is about 11269* [Immunization](immunization.html): The patient for the vaccination record 11270* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 11271* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 11272* [Invoice](invoice.html): Recipient(s) of goods and services 11273* [List](list.html): If all resources have the same subject 11274* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 11275* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 11276* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 11277* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 11278* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 11279* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 11280* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 11281* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 11282* [Observation](observation.html): The subject that the observation is about (if patient) 11283* [Person](person.html): The Person links to this Patient 11284* [Procedure](procedure.html): Search by subject - a patient 11285* [Provenance](provenance.html): Where the activity involved patient data 11286* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 11287* [RelatedPerson](relatedperson.html): The patient this related person is related to 11288* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 11289* [ResearchSubject](researchsubject.html): Who or what is part of study 11290* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 11291* [ServiceRequest](servicerequest.html): Search by subject - a patient 11292* [Specimen](specimen.html): The patient the specimen comes from 11293* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 11294* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 11295* [Task](task.html): Search by patient 11296* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 11297</b><br> 11298 * Type: <b>reference</b><br> 11299 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 11300 * </p> 11301 */ 11302 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 11303 11304/** 11305 * Constant for fluent queries to be used to add include statements. Specifies 11306 * the path value of "<b>ClaimResponse:patient</b>". 11307 */ 11308 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ClaimResponse:patient").toLocked(); 11309 11310 11311} 11312