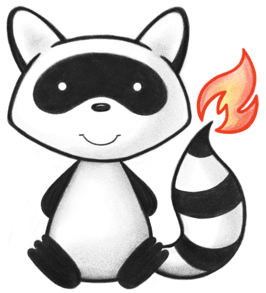
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 052 */ 053@ResourceDef(name="ClinicalImpression", profile="http://hl7.org/fhir/StructureDefinition/ClinicalImpression") 054public class ClinicalImpression extends DomainResource { 055 056 @Block() 057 public static class ClinicalImpressionFindingComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions. 060 */ 061 @Child(name = "item", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="What was found", formalDefinition="Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 064 protected CodeableReference item; 065 066 /** 067 * Which investigations support finding or diagnosis. 068 */ 069 @Child(name = "basis", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Which investigations support finding", formalDefinition="Which investigations support finding or diagnosis." ) 071 protected StringType basis; 072 073 private static final long serialVersionUID = -1363589306L; 074 075 /** 076 * Constructor 077 */ 078 public ClinicalImpressionFindingComponent() { 079 super(); 080 } 081 082 /** 083 * @return {@link #item} (Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.) 084 */ 085 public CodeableReference getItem() { 086 if (this.item == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.item"); 089 else if (Configuration.doAutoCreate()) 090 this.item = new CodeableReference(); // cc 091 return this.item; 092 } 093 094 public boolean hasItem() { 095 return this.item != null && !this.item.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #item} (Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.) 100 */ 101 public ClinicalImpressionFindingComponent setItem(CodeableReference value) { 102 this.item = value; 103 return this; 104 } 105 106 /** 107 * @return {@link #basis} (Which investigations support finding or diagnosis.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 108 */ 109 public StringType getBasisElement() { 110 if (this.basis == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.basis"); 113 else if (Configuration.doAutoCreate()) 114 this.basis = new StringType(); // bb 115 return this.basis; 116 } 117 118 public boolean hasBasisElement() { 119 return this.basis != null && !this.basis.isEmpty(); 120 } 121 122 public boolean hasBasis() { 123 return this.basis != null && !this.basis.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #basis} (Which investigations support finding or diagnosis.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 128 */ 129 public ClinicalImpressionFindingComponent setBasisElement(StringType value) { 130 this.basis = value; 131 return this; 132 } 133 134 /** 135 * @return Which investigations support finding or diagnosis. 136 */ 137 public String getBasis() { 138 return this.basis == null ? null : this.basis.getValue(); 139 } 140 141 /** 142 * @param value Which investigations support finding or diagnosis. 143 */ 144 public ClinicalImpressionFindingComponent setBasis(String value) { 145 if (Utilities.noString(value)) 146 this.basis = null; 147 else { 148 if (this.basis == null) 149 this.basis = new StringType(); 150 this.basis.setValue(value); 151 } 152 return this; 153 } 154 155 protected void listChildren(List<Property> children) { 156 super.listChildren(children); 157 children.add(new Property("item", "CodeableReference(Condition|Observation|DocumentReference)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item)); 158 children.add(new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, basis)); 159 } 160 161 @Override 162 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 163 switch (_hash) { 164 case 3242771: /*item*/ return new Property("item", "CodeableReference(Condition|Observation|DocumentReference)", "Specific text, code or reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, item); 165 case 93508670: /*basis*/ return new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, basis); 166 default: return super.getNamedProperty(_hash, _name, _checkValid); 167 } 168 169 } 170 171 @Override 172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 173 switch (hash) { 174 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 175 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : new Base[] {this.basis}; // StringType 176 default: return super.getProperty(hash, name, checkValid); 177 } 178 179 } 180 181 @Override 182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 183 switch (hash) { 184 case 3242771: // item 185 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 186 return value; 187 case 93508670: // basis 188 this.basis = TypeConvertor.castToString(value); // StringType 189 return value; 190 default: return super.setProperty(hash, name, value); 191 } 192 193 } 194 195 @Override 196 public Base setProperty(String name, Base value) throws FHIRException { 197 if (name.equals("item")) { 198 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 199 } else if (name.equals("basis")) { 200 this.basis = TypeConvertor.castToString(value); // StringType 201 } else 202 return super.setProperty(name, value); 203 return value; 204 } 205 206 @Override 207 public void removeChild(String name, Base value) throws FHIRException { 208 if (name.equals("item")) { 209 this.item = null; 210 } else if (name.equals("basis")) { 211 this.basis = null; 212 } else 213 super.removeChild(name, value); 214 215 } 216 217 @Override 218 public Base makeProperty(int hash, String name) throws FHIRException { 219 switch (hash) { 220 case 3242771: return getItem(); 221 case 93508670: return getBasisElement(); 222 default: return super.makeProperty(hash, name); 223 } 224 225 } 226 227 @Override 228 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 229 switch (hash) { 230 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 231 case 93508670: /*basis*/ return new String[] {"string"}; 232 default: return super.getTypesForProperty(hash, name); 233 } 234 235 } 236 237 @Override 238 public Base addChild(String name) throws FHIRException { 239 if (name.equals("item")) { 240 this.item = new CodeableReference(); 241 return this.item; 242 } 243 else if (name.equals("basis")) { 244 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.finding.basis"); 245 } 246 else 247 return super.addChild(name); 248 } 249 250 public ClinicalImpressionFindingComponent copy() { 251 ClinicalImpressionFindingComponent dst = new ClinicalImpressionFindingComponent(); 252 copyValues(dst); 253 return dst; 254 } 255 256 public void copyValues(ClinicalImpressionFindingComponent dst) { 257 super.copyValues(dst); 258 dst.item = item == null ? null : item.copy(); 259 dst.basis = basis == null ? null : basis.copy(); 260 } 261 262 @Override 263 public boolean equalsDeep(Base other_) { 264 if (!super.equalsDeep(other_)) 265 return false; 266 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 267 return false; 268 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 269 return compareDeep(item, o.item, true) && compareDeep(basis, o.basis, true); 270 } 271 272 @Override 273 public boolean equalsShallow(Base other_) { 274 if (!super.equalsShallow(other_)) 275 return false; 276 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 277 return false; 278 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 279 return compareValues(basis, o.basis, true); 280 } 281 282 public boolean isEmpty() { 283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, basis); 284 } 285 286 public String fhirType() { 287 return "ClinicalImpression.finding"; 288 289 } 290 291 } 292 293 /** 294 * Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 295 */ 296 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 297 @Description(shortDefinition="Business identifier", formalDefinition="Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 298 protected List<Identifier> identifier; 299 300 /** 301 * Identifies the workflow status of the assessment. 302 */ 303 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 304 @Description(shortDefinition="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition="Identifies the workflow status of the assessment." ) 305 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 306 protected Enumeration<EventStatus> status; 307 308 /** 309 * Captures the reason for the current state of the ClinicalImpression. 310 */ 311 @Child(name = "statusReason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 312 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the ClinicalImpression." ) 313 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinicalimpression-status-reason") 314 protected CodeableConcept statusReason; 315 316 /** 317 * A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it. 318 */ 319 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 320 @Description(shortDefinition="Why/how the assessment was performed", formalDefinition="A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it." ) 321 protected StringType description; 322 323 /** 324 * The patient or group of individuals assessed as part of this record. 325 */ 326 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=1, max=1, modifier=false, summary=true) 327 @Description(shortDefinition="Patient or group assessed", formalDefinition="The patient or group of individuals assessed as part of this record." ) 328 protected Reference subject; 329 330 /** 331 * The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated. 332 */ 333 @Child(name = "encounter", type = {Encounter.class}, order=5, min=0, max=1, modifier=false, summary=true) 334 @Description(shortDefinition="The Encounter during which this ClinicalImpression was created", formalDefinition="The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated." ) 335 protected Reference encounter; 336 337 /** 338 * The point in time or period over which the subject was assessed. 339 */ 340 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 341 @Description(shortDefinition="Time of assessment", formalDefinition="The point in time or period over which the subject was assessed." ) 342 protected DataType effective; 343 344 /** 345 * Indicates when the documentation of the assessment was complete. 346 */ 347 @Child(name = "date", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 348 @Description(shortDefinition="When the assessment was documented", formalDefinition="Indicates when the documentation of the assessment was complete." ) 349 protected DateTimeType date; 350 351 /** 352 * The clinician performing the assessment. 353 */ 354 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class}, order=8, min=0, max=1, modifier=false, summary=true) 355 @Description(shortDefinition="The clinician performing the assessment", formalDefinition="The clinician performing the assessment." ) 356 protected Reference performer; 357 358 /** 359 * A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes. 360 */ 361 @Child(name = "previous", type = {ClinicalImpression.class}, order=9, min=0, max=1, modifier=false, summary=false) 362 @Description(shortDefinition="Reference to last assessment", formalDefinition="A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes." ) 363 protected Reference previous; 364 365 /** 366 * A list of the relevant problems/conditions for a patient. 367 */ 368 @Child(name = "problem", type = {Condition.class, AllergyIntolerance.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 369 @Description(shortDefinition="Relevant impressions of patient state", formalDefinition="A list of the relevant problems/conditions for a patient." ) 370 protected List<Reference> problem; 371 372 /** 373 * Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change. It is a subjective assessment of the direction of the change. 374 */ 375 @Child(name = "changePattern", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 376 @Description(shortDefinition="Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change", formalDefinition="Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change. It is a subjective assessment of the direction of the change." ) 377 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinicalimpression-change-pattern") 378 protected CodeableConcept changePattern; 379 380 /** 381 * Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis. 382 */ 383 @Child(name = "protocol", type = {UriType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 384 @Description(shortDefinition="Clinical Protocol followed", formalDefinition="Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis." ) 385 protected List<UriType> protocol; 386 387 /** 388 * A text summary of the investigations and the diagnosis. 389 */ 390 @Child(name = "summary", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 391 @Description(shortDefinition="Summary of the assessment", formalDefinition="A text summary of the investigations and the diagnosis." ) 392 protected StringType summary; 393 394 /** 395 * Specific findings or diagnoses that were considered likely or relevant to ongoing treatment. 396 */ 397 @Child(name = "finding", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 398 @Description(shortDefinition="Possible or likely findings and diagnoses", formalDefinition="Specific findings or diagnoses that were considered likely or relevant to ongoing treatment." ) 399 protected List<ClinicalImpressionFindingComponent> finding; 400 401 /** 402 * Estimate of likely outcome. 403 */ 404 @Child(name = "prognosisCodeableConcept", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 405 @Description(shortDefinition="Estimate of likely outcome", formalDefinition="Estimate of likely outcome." ) 406 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinicalimpression-prognosis") 407 protected List<CodeableConcept> prognosisCodeableConcept; 408 409 /** 410 * RiskAssessment expressing likely outcome. 411 */ 412 @Child(name = "prognosisReference", type = {RiskAssessment.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 413 @Description(shortDefinition="RiskAssessment expressing likely outcome", formalDefinition="RiskAssessment expressing likely outcome." ) 414 protected List<Reference> prognosisReference; 415 416 /** 417 * Information supporting the clinical impression, which can contain investigation results. 418 */ 419 @Child(name = "supportingInfo", type = {Reference.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 420 @Description(shortDefinition="Information supporting the clinical impression", formalDefinition="Information supporting the clinical impression, which can contain investigation results." ) 421 protected List<Reference> supportingInfo; 422 423 /** 424 * Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear. 425 */ 426 @Child(name = "note", type = {Annotation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 427 @Description(shortDefinition="Comments made about the ClinicalImpression", formalDefinition="Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear." ) 428 protected List<Annotation> note; 429 430 private static final long serialVersionUID = -1360437701L; 431 432 /** 433 * Constructor 434 */ 435 public ClinicalImpression() { 436 super(); 437 } 438 439 /** 440 * Constructor 441 */ 442 public ClinicalImpression(EventStatus status, Reference subject) { 443 super(); 444 this.setStatus(status); 445 this.setSubject(subject); 446 } 447 448 /** 449 * @return {@link #identifier} (Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 450 */ 451 public List<Identifier> getIdentifier() { 452 if (this.identifier == null) 453 this.identifier = new ArrayList<Identifier>(); 454 return this.identifier; 455 } 456 457 /** 458 * @return Returns a reference to <code>this</code> for easy method chaining 459 */ 460 public ClinicalImpression setIdentifier(List<Identifier> theIdentifier) { 461 this.identifier = theIdentifier; 462 return this; 463 } 464 465 public boolean hasIdentifier() { 466 if (this.identifier == null) 467 return false; 468 for (Identifier item : this.identifier) 469 if (!item.isEmpty()) 470 return true; 471 return false; 472 } 473 474 public Identifier addIdentifier() { //3 475 Identifier t = new Identifier(); 476 if (this.identifier == null) 477 this.identifier = new ArrayList<Identifier>(); 478 this.identifier.add(t); 479 return t; 480 } 481 482 public ClinicalImpression addIdentifier(Identifier t) { //3 483 if (t == null) 484 return this; 485 if (this.identifier == null) 486 this.identifier = new ArrayList<Identifier>(); 487 this.identifier.add(t); 488 return this; 489 } 490 491 /** 492 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 493 */ 494 public Identifier getIdentifierFirstRep() { 495 if (getIdentifier().isEmpty()) { 496 addIdentifier(); 497 } 498 return getIdentifier().get(0); 499 } 500 501 /** 502 * @return {@link #status} (Identifies the workflow status of the assessment.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 503 */ 504 public Enumeration<EventStatus> getStatusElement() { 505 if (this.status == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create ClinicalImpression.status"); 508 else if (Configuration.doAutoCreate()) 509 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); // bb 510 return this.status; 511 } 512 513 public boolean hasStatusElement() { 514 return this.status != null && !this.status.isEmpty(); 515 } 516 517 public boolean hasStatus() { 518 return this.status != null && !this.status.isEmpty(); 519 } 520 521 /** 522 * @param value {@link #status} (Identifies the workflow status of the assessment.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 523 */ 524 public ClinicalImpression setStatusElement(Enumeration<EventStatus> value) { 525 this.status = value; 526 return this; 527 } 528 529 /** 530 * @return Identifies the workflow status of the assessment. 531 */ 532 public EventStatus getStatus() { 533 return this.status == null ? null : this.status.getValue(); 534 } 535 536 /** 537 * @param value Identifies the workflow status of the assessment. 538 */ 539 public ClinicalImpression setStatus(EventStatus value) { 540 if (this.status == null) 541 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); 542 this.status.setValue(value); 543 return this; 544 } 545 546 /** 547 * @return {@link #statusReason} (Captures the reason for the current state of the ClinicalImpression.) 548 */ 549 public CodeableConcept getStatusReason() { 550 if (this.statusReason == null) 551 if (Configuration.errorOnAutoCreate()) 552 throw new Error("Attempt to auto-create ClinicalImpression.statusReason"); 553 else if (Configuration.doAutoCreate()) 554 this.statusReason = new CodeableConcept(); // cc 555 return this.statusReason; 556 } 557 558 public boolean hasStatusReason() { 559 return this.statusReason != null && !this.statusReason.isEmpty(); 560 } 561 562 /** 563 * @param value {@link #statusReason} (Captures the reason for the current state of the ClinicalImpression.) 564 */ 565 public ClinicalImpression setStatusReason(CodeableConcept value) { 566 this.statusReason = value; 567 return this; 568 } 569 570 /** 571 * @return {@link #description} (A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 572 */ 573 public StringType getDescriptionElement() { 574 if (this.description == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create ClinicalImpression.description"); 577 else if (Configuration.doAutoCreate()) 578 this.description = new StringType(); // bb 579 return this.description; 580 } 581 582 public boolean hasDescriptionElement() { 583 return this.description != null && !this.description.isEmpty(); 584 } 585 586 public boolean hasDescription() { 587 return this.description != null && !this.description.isEmpty(); 588 } 589 590 /** 591 * @param value {@link #description} (A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 592 */ 593 public ClinicalImpression setDescriptionElement(StringType value) { 594 this.description = value; 595 return this; 596 } 597 598 /** 599 * @return A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it. 600 */ 601 public String getDescription() { 602 return this.description == null ? null : this.description.getValue(); 603 } 604 605 /** 606 * @param value A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it. 607 */ 608 public ClinicalImpression setDescription(String value) { 609 if (Utilities.noString(value)) 610 this.description = null; 611 else { 612 if (this.description == null) 613 this.description = new StringType(); 614 this.description.setValue(value); 615 } 616 return this; 617 } 618 619 /** 620 * @return {@link #subject} (The patient or group of individuals assessed as part of this record.) 621 */ 622 public Reference getSubject() { 623 if (this.subject == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create ClinicalImpression.subject"); 626 else if (Configuration.doAutoCreate()) 627 this.subject = new Reference(); // cc 628 return this.subject; 629 } 630 631 public boolean hasSubject() { 632 return this.subject != null && !this.subject.isEmpty(); 633 } 634 635 /** 636 * @param value {@link #subject} (The patient or group of individuals assessed as part of this record.) 637 */ 638 public ClinicalImpression setSubject(Reference value) { 639 this.subject = value; 640 return this; 641 } 642 643 /** 644 * @return {@link #encounter} (The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.) 645 */ 646 public Reference getEncounter() { 647 if (this.encounter == null) 648 if (Configuration.errorOnAutoCreate()) 649 throw new Error("Attempt to auto-create ClinicalImpression.encounter"); 650 else if (Configuration.doAutoCreate()) 651 this.encounter = new Reference(); // cc 652 return this.encounter; 653 } 654 655 public boolean hasEncounter() { 656 return this.encounter != null && !this.encounter.isEmpty(); 657 } 658 659 /** 660 * @param value {@link #encounter} (The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.) 661 */ 662 public ClinicalImpression setEncounter(Reference value) { 663 this.encounter = value; 664 return this; 665 } 666 667 /** 668 * @return {@link #effective} (The point in time or period over which the subject was assessed.) 669 */ 670 public DataType getEffective() { 671 return this.effective; 672 } 673 674 /** 675 * @return {@link #effective} (The point in time or period over which the subject was assessed.) 676 */ 677 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 678 if (this.effective == null) 679 this.effective = new DateTimeType(); 680 if (!(this.effective instanceof DateTimeType)) 681 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 682 return (DateTimeType) this.effective; 683 } 684 685 public boolean hasEffectiveDateTimeType() { 686 return this.effective instanceof DateTimeType; 687 } 688 689 /** 690 * @return {@link #effective} (The point in time or period over which the subject was assessed.) 691 */ 692 public Period getEffectivePeriod() throws FHIRException { 693 if (this.effective == null) 694 this.effective = new Period(); 695 if (!(this.effective instanceof Period)) 696 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 697 return (Period) this.effective; 698 } 699 700 public boolean hasEffectivePeriod() { 701 return this.effective instanceof Period; 702 } 703 704 public boolean hasEffective() { 705 return this.effective != null && !this.effective.isEmpty(); 706 } 707 708 /** 709 * @param value {@link #effective} (The point in time or period over which the subject was assessed.) 710 */ 711 public ClinicalImpression setEffective(DataType value) { 712 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 713 throw new FHIRException("Not the right type for ClinicalImpression.effective[x]: "+value.fhirType()); 714 this.effective = value; 715 return this; 716 } 717 718 /** 719 * @return {@link #date} (Indicates when the documentation of the assessment was complete.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 720 */ 721 public DateTimeType getDateElement() { 722 if (this.date == null) 723 if (Configuration.errorOnAutoCreate()) 724 throw new Error("Attempt to auto-create ClinicalImpression.date"); 725 else if (Configuration.doAutoCreate()) 726 this.date = new DateTimeType(); // bb 727 return this.date; 728 } 729 730 public boolean hasDateElement() { 731 return this.date != null && !this.date.isEmpty(); 732 } 733 734 public boolean hasDate() { 735 return this.date != null && !this.date.isEmpty(); 736 } 737 738 /** 739 * @param value {@link #date} (Indicates when the documentation of the assessment was complete.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 740 */ 741 public ClinicalImpression setDateElement(DateTimeType value) { 742 this.date = value; 743 return this; 744 } 745 746 /** 747 * @return Indicates when the documentation of the assessment was complete. 748 */ 749 public Date getDate() { 750 return this.date == null ? null : this.date.getValue(); 751 } 752 753 /** 754 * @param value Indicates when the documentation of the assessment was complete. 755 */ 756 public ClinicalImpression setDate(Date value) { 757 if (value == null) 758 this.date = null; 759 else { 760 if (this.date == null) 761 this.date = new DateTimeType(); 762 this.date.setValue(value); 763 } 764 return this; 765 } 766 767 /** 768 * @return {@link #performer} (The clinician performing the assessment.) 769 */ 770 public Reference getPerformer() { 771 if (this.performer == null) 772 if (Configuration.errorOnAutoCreate()) 773 throw new Error("Attempt to auto-create ClinicalImpression.performer"); 774 else if (Configuration.doAutoCreate()) 775 this.performer = new Reference(); // cc 776 return this.performer; 777 } 778 779 public boolean hasPerformer() { 780 return this.performer != null && !this.performer.isEmpty(); 781 } 782 783 /** 784 * @param value {@link #performer} (The clinician performing the assessment.) 785 */ 786 public ClinicalImpression setPerformer(Reference value) { 787 this.performer = value; 788 return this; 789 } 790 791 /** 792 * @return {@link #previous} (A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 793 */ 794 public Reference getPrevious() { 795 if (this.previous == null) 796 if (Configuration.errorOnAutoCreate()) 797 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 798 else if (Configuration.doAutoCreate()) 799 this.previous = new Reference(); // cc 800 return this.previous; 801 } 802 803 public boolean hasPrevious() { 804 return this.previous != null && !this.previous.isEmpty(); 805 } 806 807 /** 808 * @param value {@link #previous} (A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.) 809 */ 810 public ClinicalImpression setPrevious(Reference value) { 811 this.previous = value; 812 return this; 813 } 814 815 /** 816 * @return {@link #problem} (A list of the relevant problems/conditions for a patient.) 817 */ 818 public List<Reference> getProblem() { 819 if (this.problem == null) 820 this.problem = new ArrayList<Reference>(); 821 return this.problem; 822 } 823 824 /** 825 * @return Returns a reference to <code>this</code> for easy method chaining 826 */ 827 public ClinicalImpression setProblem(List<Reference> theProblem) { 828 this.problem = theProblem; 829 return this; 830 } 831 832 public boolean hasProblem() { 833 if (this.problem == null) 834 return false; 835 for (Reference item : this.problem) 836 if (!item.isEmpty()) 837 return true; 838 return false; 839 } 840 841 public Reference addProblem() { //3 842 Reference t = new Reference(); 843 if (this.problem == null) 844 this.problem = new ArrayList<Reference>(); 845 this.problem.add(t); 846 return t; 847 } 848 849 public ClinicalImpression addProblem(Reference t) { //3 850 if (t == null) 851 return this; 852 if (this.problem == null) 853 this.problem = new ArrayList<Reference>(); 854 this.problem.add(t); 855 return this; 856 } 857 858 /** 859 * @return The first repetition of repeating field {@link #problem}, creating it if it does not already exist {3} 860 */ 861 public Reference getProblemFirstRep() { 862 if (getProblem().isEmpty()) { 863 addProblem(); 864 } 865 return getProblem().get(0); 866 } 867 868 /** 869 * @return {@link #changePattern} (Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change. It is a subjective assessment of the direction of the change.) 870 */ 871 public CodeableConcept getChangePattern() { 872 if (this.changePattern == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create ClinicalImpression.changePattern"); 875 else if (Configuration.doAutoCreate()) 876 this.changePattern = new CodeableConcept(); // cc 877 return this.changePattern; 878 } 879 880 public boolean hasChangePattern() { 881 return this.changePattern != null && !this.changePattern.isEmpty(); 882 } 883 884 /** 885 * @param value {@link #changePattern} (Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change. It is a subjective assessment of the direction of the change.) 886 */ 887 public ClinicalImpression setChangePattern(CodeableConcept value) { 888 this.changePattern = value; 889 return this; 890 } 891 892 /** 893 * @return {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 894 */ 895 public List<UriType> getProtocol() { 896 if (this.protocol == null) 897 this.protocol = new ArrayList<UriType>(); 898 return this.protocol; 899 } 900 901 /** 902 * @return Returns a reference to <code>this</code> for easy method chaining 903 */ 904 public ClinicalImpression setProtocol(List<UriType> theProtocol) { 905 this.protocol = theProtocol; 906 return this; 907 } 908 909 public boolean hasProtocol() { 910 if (this.protocol == null) 911 return false; 912 for (UriType item : this.protocol) 913 if (!item.isEmpty()) 914 return true; 915 return false; 916 } 917 918 /** 919 * @return {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 920 */ 921 public UriType addProtocolElement() {//2 922 UriType t = new UriType(); 923 if (this.protocol == null) 924 this.protocol = new ArrayList<UriType>(); 925 this.protocol.add(t); 926 return t; 927 } 928 929 /** 930 * @param value {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 931 */ 932 public ClinicalImpression addProtocol(String value) { //1 933 UriType t = new UriType(); 934 t.setValue(value); 935 if (this.protocol == null) 936 this.protocol = new ArrayList<UriType>(); 937 this.protocol.add(t); 938 return this; 939 } 940 941 /** 942 * @param value {@link #protocol} (Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.) 943 */ 944 public boolean hasProtocol(String value) { 945 if (this.protocol == null) 946 return false; 947 for (UriType v : this.protocol) 948 if (v.getValue().equals(value)) // uri 949 return true; 950 return false; 951 } 952 953 /** 954 * @return {@link #summary} (A text summary of the investigations and the diagnosis.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 955 */ 956 public StringType getSummaryElement() { 957 if (this.summary == null) 958 if (Configuration.errorOnAutoCreate()) 959 throw new Error("Attempt to auto-create ClinicalImpression.summary"); 960 else if (Configuration.doAutoCreate()) 961 this.summary = new StringType(); // bb 962 return this.summary; 963 } 964 965 public boolean hasSummaryElement() { 966 return this.summary != null && !this.summary.isEmpty(); 967 } 968 969 public boolean hasSummary() { 970 return this.summary != null && !this.summary.isEmpty(); 971 } 972 973 /** 974 * @param value {@link #summary} (A text summary of the investigations and the diagnosis.). This is the underlying object with id, value and extensions. The accessor "getSummary" gives direct access to the value 975 */ 976 public ClinicalImpression setSummaryElement(StringType value) { 977 this.summary = value; 978 return this; 979 } 980 981 /** 982 * @return A text summary of the investigations and the diagnosis. 983 */ 984 public String getSummary() { 985 return this.summary == null ? null : this.summary.getValue(); 986 } 987 988 /** 989 * @param value A text summary of the investigations and the diagnosis. 990 */ 991 public ClinicalImpression setSummary(String value) { 992 if (Utilities.noString(value)) 993 this.summary = null; 994 else { 995 if (this.summary == null) 996 this.summary = new StringType(); 997 this.summary.setValue(value); 998 } 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #finding} (Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.) 1004 */ 1005 public List<ClinicalImpressionFindingComponent> getFinding() { 1006 if (this.finding == null) 1007 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1008 return this.finding; 1009 } 1010 1011 /** 1012 * @return Returns a reference to <code>this</code> for easy method chaining 1013 */ 1014 public ClinicalImpression setFinding(List<ClinicalImpressionFindingComponent> theFinding) { 1015 this.finding = theFinding; 1016 return this; 1017 } 1018 1019 public boolean hasFinding() { 1020 if (this.finding == null) 1021 return false; 1022 for (ClinicalImpressionFindingComponent item : this.finding) 1023 if (!item.isEmpty()) 1024 return true; 1025 return false; 1026 } 1027 1028 public ClinicalImpressionFindingComponent addFinding() { //3 1029 ClinicalImpressionFindingComponent t = new ClinicalImpressionFindingComponent(); 1030 if (this.finding == null) 1031 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1032 this.finding.add(t); 1033 return t; 1034 } 1035 1036 public ClinicalImpression addFinding(ClinicalImpressionFindingComponent t) { //3 1037 if (t == null) 1038 return this; 1039 if (this.finding == null) 1040 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1041 this.finding.add(t); 1042 return this; 1043 } 1044 1045 /** 1046 * @return The first repetition of repeating field {@link #finding}, creating it if it does not already exist {3} 1047 */ 1048 public ClinicalImpressionFindingComponent getFindingFirstRep() { 1049 if (getFinding().isEmpty()) { 1050 addFinding(); 1051 } 1052 return getFinding().get(0); 1053 } 1054 1055 /** 1056 * @return {@link #prognosisCodeableConcept} (Estimate of likely outcome.) 1057 */ 1058 public List<CodeableConcept> getPrognosisCodeableConcept() { 1059 if (this.prognosisCodeableConcept == null) 1060 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1061 return this.prognosisCodeableConcept; 1062 } 1063 1064 /** 1065 * @return Returns a reference to <code>this</code> for easy method chaining 1066 */ 1067 public ClinicalImpression setPrognosisCodeableConcept(List<CodeableConcept> thePrognosisCodeableConcept) { 1068 this.prognosisCodeableConcept = thePrognosisCodeableConcept; 1069 return this; 1070 } 1071 1072 public boolean hasPrognosisCodeableConcept() { 1073 if (this.prognosisCodeableConcept == null) 1074 return false; 1075 for (CodeableConcept item : this.prognosisCodeableConcept) 1076 if (!item.isEmpty()) 1077 return true; 1078 return false; 1079 } 1080 1081 public CodeableConcept addPrognosisCodeableConcept() { //3 1082 CodeableConcept t = new CodeableConcept(); 1083 if (this.prognosisCodeableConcept == null) 1084 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1085 this.prognosisCodeableConcept.add(t); 1086 return t; 1087 } 1088 1089 public ClinicalImpression addPrognosisCodeableConcept(CodeableConcept t) { //3 1090 if (t == null) 1091 return this; 1092 if (this.prognosisCodeableConcept == null) 1093 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1094 this.prognosisCodeableConcept.add(t); 1095 return this; 1096 } 1097 1098 /** 1099 * @return The first repetition of repeating field {@link #prognosisCodeableConcept}, creating it if it does not already exist {3} 1100 */ 1101 public CodeableConcept getPrognosisCodeableConceptFirstRep() { 1102 if (getPrognosisCodeableConcept().isEmpty()) { 1103 addPrognosisCodeableConcept(); 1104 } 1105 return getPrognosisCodeableConcept().get(0); 1106 } 1107 1108 /** 1109 * @return {@link #prognosisReference} (RiskAssessment expressing likely outcome.) 1110 */ 1111 public List<Reference> getPrognosisReference() { 1112 if (this.prognosisReference == null) 1113 this.prognosisReference = new ArrayList<Reference>(); 1114 return this.prognosisReference; 1115 } 1116 1117 /** 1118 * @return Returns a reference to <code>this</code> for easy method chaining 1119 */ 1120 public ClinicalImpression setPrognosisReference(List<Reference> thePrognosisReference) { 1121 this.prognosisReference = thePrognosisReference; 1122 return this; 1123 } 1124 1125 public boolean hasPrognosisReference() { 1126 if (this.prognosisReference == null) 1127 return false; 1128 for (Reference item : this.prognosisReference) 1129 if (!item.isEmpty()) 1130 return true; 1131 return false; 1132 } 1133 1134 public Reference addPrognosisReference() { //3 1135 Reference t = new Reference(); 1136 if (this.prognosisReference == null) 1137 this.prognosisReference = new ArrayList<Reference>(); 1138 this.prognosisReference.add(t); 1139 return t; 1140 } 1141 1142 public ClinicalImpression addPrognosisReference(Reference t) { //3 1143 if (t == null) 1144 return this; 1145 if (this.prognosisReference == null) 1146 this.prognosisReference = new ArrayList<Reference>(); 1147 this.prognosisReference.add(t); 1148 return this; 1149 } 1150 1151 /** 1152 * @return The first repetition of repeating field {@link #prognosisReference}, creating it if it does not already exist {3} 1153 */ 1154 public Reference getPrognosisReferenceFirstRep() { 1155 if (getPrognosisReference().isEmpty()) { 1156 addPrognosisReference(); 1157 } 1158 return getPrognosisReference().get(0); 1159 } 1160 1161 /** 1162 * @return {@link #supportingInfo} (Information supporting the clinical impression, which can contain investigation results.) 1163 */ 1164 public List<Reference> getSupportingInfo() { 1165 if (this.supportingInfo == null) 1166 this.supportingInfo = new ArrayList<Reference>(); 1167 return this.supportingInfo; 1168 } 1169 1170 /** 1171 * @return Returns a reference to <code>this</code> for easy method chaining 1172 */ 1173 public ClinicalImpression setSupportingInfo(List<Reference> theSupportingInfo) { 1174 this.supportingInfo = theSupportingInfo; 1175 return this; 1176 } 1177 1178 public boolean hasSupportingInfo() { 1179 if (this.supportingInfo == null) 1180 return false; 1181 for (Reference item : this.supportingInfo) 1182 if (!item.isEmpty()) 1183 return true; 1184 return false; 1185 } 1186 1187 public Reference addSupportingInfo() { //3 1188 Reference t = new Reference(); 1189 if (this.supportingInfo == null) 1190 this.supportingInfo = new ArrayList<Reference>(); 1191 this.supportingInfo.add(t); 1192 return t; 1193 } 1194 1195 public ClinicalImpression addSupportingInfo(Reference t) { //3 1196 if (t == null) 1197 return this; 1198 if (this.supportingInfo == null) 1199 this.supportingInfo = new ArrayList<Reference>(); 1200 this.supportingInfo.add(t); 1201 return this; 1202 } 1203 1204 /** 1205 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 1206 */ 1207 public Reference getSupportingInfoFirstRep() { 1208 if (getSupportingInfo().isEmpty()) { 1209 addSupportingInfo(); 1210 } 1211 return getSupportingInfo().get(0); 1212 } 1213 1214 /** 1215 * @return {@link #note} (Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.) 1216 */ 1217 public List<Annotation> getNote() { 1218 if (this.note == null) 1219 this.note = new ArrayList<Annotation>(); 1220 return this.note; 1221 } 1222 1223 /** 1224 * @return Returns a reference to <code>this</code> for easy method chaining 1225 */ 1226 public ClinicalImpression setNote(List<Annotation> theNote) { 1227 this.note = theNote; 1228 return this; 1229 } 1230 1231 public boolean hasNote() { 1232 if (this.note == null) 1233 return false; 1234 for (Annotation item : this.note) 1235 if (!item.isEmpty()) 1236 return true; 1237 return false; 1238 } 1239 1240 public Annotation addNote() { //3 1241 Annotation t = new Annotation(); 1242 if (this.note == null) 1243 this.note = new ArrayList<Annotation>(); 1244 this.note.add(t); 1245 return t; 1246 } 1247 1248 public ClinicalImpression addNote(Annotation t) { //3 1249 if (t == null) 1250 return this; 1251 if (this.note == null) 1252 this.note = new ArrayList<Annotation>(); 1253 this.note.add(t); 1254 return this; 1255 } 1256 1257 /** 1258 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1259 */ 1260 public Annotation getNoteFirstRep() { 1261 if (getNote().isEmpty()) { 1262 addNote(); 1263 } 1264 return getNote().get(0); 1265 } 1266 1267 protected void listChildren(List<Property> children) { 1268 super.listChildren(children); 1269 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1270 children.add(new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, status)); 1271 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the ClinicalImpression.", 0, 1, statusReason)); 1272 children.add(new Property("description", "string", "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.", 0, 1, description)); 1273 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group of individuals assessed as part of this record.", 0, 1, subject)); 1274 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1275 children.add(new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective)); 1276 children.add(new Property("date", "dateTime", "Indicates when the documentation of the assessment was complete.", 0, 1, date)); 1277 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole)", "The clinician performing the assessment.", 0, 1, performer)); 1278 children.add(new Property("previous", "Reference(ClinicalImpression)", "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 0, 1, previous)); 1279 children.add(new Property("problem", "Reference(Condition|AllergyIntolerance)", "A list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem)); 1280 children.add(new Property("changePattern", "CodeableConcept", "Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change. It is a subjective assessment of the direction of the change.", 0, 1, changePattern)); 1281 children.add(new Property("protocol", "uri", "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 0, java.lang.Integer.MAX_VALUE, protocol)); 1282 children.add(new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1, summary)); 1283 children.add(new Property("finding", "", "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.", 0, java.lang.Integer.MAX_VALUE, finding)); 1284 children.add(new Property("prognosisCodeableConcept", "CodeableConcept", "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisCodeableConcept)); 1285 children.add(new Property("prognosisReference", "Reference(RiskAssessment)", "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference)); 1286 children.add(new Property("supportingInfo", "Reference(Any)", "Information supporting the clinical impression, which can contain investigation results.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 1287 children.add(new Property("note", "Annotation", "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 0, java.lang.Integer.MAX_VALUE, note)); 1288 } 1289 1290 @Override 1291 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1292 switch (_hash) { 1293 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1294 case -892481550: /*status*/ return new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, status); 1295 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the ClinicalImpression.", 0, 1, statusReason); 1296 case -1724546052: /*description*/ return new Property("description", "string", "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.", 0, 1, description); 1297 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group of individuals assessed as part of this record.", 0, 1, subject); 1298 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1299 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1300 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1301 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1302 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "Period", "The point in time or period over which the subject was assessed.", 0, 1, effective); 1303 case 3076014: /*date*/ return new Property("date", "dateTime", "Indicates when the documentation of the assessment was complete.", 0, 1, date); 1304 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole)", "The clinician performing the assessment.", 0, 1, performer); 1305 case -1273775369: /*previous*/ return new Property("previous", "Reference(ClinicalImpression)", "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 0, 1, previous); 1306 case -309542241: /*problem*/ return new Property("problem", "Reference(Condition|AllergyIntolerance)", "A list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem); 1307 case -1770133056: /*changePattern*/ return new Property("changePattern", "CodeableConcept", "Change in the status/pattern of a subject's condition since previously assessed, such as worsening, improving, or no change. It is a subjective assessment of the direction of the change.", 0, 1, changePattern); 1308 case -989163880: /*protocol*/ return new Property("protocol", "uri", "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 0, java.lang.Integer.MAX_VALUE, protocol); 1309 case -1857640538: /*summary*/ return new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1, summary); 1310 case -853173367: /*finding*/ return new Property("finding", "", "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.", 0, java.lang.Integer.MAX_VALUE, finding); 1311 case -676337953: /*prognosisCodeableConcept*/ return new Property("prognosisCodeableConcept", "CodeableConcept", "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisCodeableConcept); 1312 case -587137783: /*prognosisReference*/ return new Property("prognosisReference", "Reference(RiskAssessment)", "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference); 1313 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Information supporting the clinical impression, which can contain investigation results.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 1314 case 3387378: /*note*/ return new Property("note", "Annotation", "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 0, java.lang.Integer.MAX_VALUE, note); 1315 default: return super.getNamedProperty(_hash, _name, _checkValid); 1316 } 1317 1318 } 1319 1320 @Override 1321 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1322 switch (hash) { 1323 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1324 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EventStatus> 1325 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 1326 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1327 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1328 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1329 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // DataType 1330 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1331 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1332 case -1273775369: /*previous*/ return this.previous == null ? new Base[0] : new Base[] {this.previous}; // Reference 1333 case -309542241: /*problem*/ return this.problem == null ? new Base[0] : this.problem.toArray(new Base[this.problem.size()]); // Reference 1334 case -1770133056: /*changePattern*/ return this.changePattern == null ? new Base[0] : new Base[] {this.changePattern}; // CodeableConcept 1335 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // UriType 1336 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : new Base[] {this.summary}; // StringType 1337 case -853173367: /*finding*/ return this.finding == null ? new Base[0] : this.finding.toArray(new Base[this.finding.size()]); // ClinicalImpressionFindingComponent 1338 case -676337953: /*prognosisCodeableConcept*/ return this.prognosisCodeableConcept == null ? new Base[0] : this.prognosisCodeableConcept.toArray(new Base[this.prognosisCodeableConcept.size()]); // CodeableConcept 1339 case -587137783: /*prognosisReference*/ return this.prognosisReference == null ? new Base[0] : this.prognosisReference.toArray(new Base[this.prognosisReference.size()]); // Reference 1340 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 1341 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1342 default: return super.getProperty(hash, name, checkValid); 1343 } 1344 1345 } 1346 1347 @Override 1348 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1349 switch (hash) { 1350 case -1618432855: // identifier 1351 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1352 return value; 1353 case -892481550: // status 1354 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1355 this.status = (Enumeration) value; // Enumeration<EventStatus> 1356 return value; 1357 case 2051346646: // statusReason 1358 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1359 return value; 1360 case -1724546052: // description 1361 this.description = TypeConvertor.castToString(value); // StringType 1362 return value; 1363 case -1867885268: // subject 1364 this.subject = TypeConvertor.castToReference(value); // Reference 1365 return value; 1366 case 1524132147: // encounter 1367 this.encounter = TypeConvertor.castToReference(value); // Reference 1368 return value; 1369 case -1468651097: // effective 1370 this.effective = TypeConvertor.castToType(value); // DataType 1371 return value; 1372 case 3076014: // date 1373 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1374 return value; 1375 case 481140686: // performer 1376 this.performer = TypeConvertor.castToReference(value); // Reference 1377 return value; 1378 case -1273775369: // previous 1379 this.previous = TypeConvertor.castToReference(value); // Reference 1380 return value; 1381 case -309542241: // problem 1382 this.getProblem().add(TypeConvertor.castToReference(value)); // Reference 1383 return value; 1384 case -1770133056: // changePattern 1385 this.changePattern = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1386 return value; 1387 case -989163880: // protocol 1388 this.getProtocol().add(TypeConvertor.castToUri(value)); // UriType 1389 return value; 1390 case -1857640538: // summary 1391 this.summary = TypeConvertor.castToString(value); // StringType 1392 return value; 1393 case -853173367: // finding 1394 this.getFinding().add((ClinicalImpressionFindingComponent) value); // ClinicalImpressionFindingComponent 1395 return value; 1396 case -676337953: // prognosisCodeableConcept 1397 this.getPrognosisCodeableConcept().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1398 return value; 1399 case -587137783: // prognosisReference 1400 this.getPrognosisReference().add(TypeConvertor.castToReference(value)); // Reference 1401 return value; 1402 case 1922406657: // supportingInfo 1403 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); // Reference 1404 return value; 1405 case 3387378: // note 1406 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1407 return value; 1408 default: return super.setProperty(hash, name, value); 1409 } 1410 1411 } 1412 1413 @Override 1414 public Base setProperty(String name, Base value) throws FHIRException { 1415 if (name.equals("identifier")) { 1416 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1417 } else if (name.equals("status")) { 1418 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1419 this.status = (Enumeration) value; // Enumeration<EventStatus> 1420 } else if (name.equals("statusReason")) { 1421 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1422 } else if (name.equals("description")) { 1423 this.description = TypeConvertor.castToString(value); // StringType 1424 } else if (name.equals("subject")) { 1425 this.subject = TypeConvertor.castToReference(value); // Reference 1426 } else if (name.equals("encounter")) { 1427 this.encounter = TypeConvertor.castToReference(value); // Reference 1428 } else if (name.equals("effective[x]")) { 1429 this.effective = TypeConvertor.castToType(value); // DataType 1430 } else if (name.equals("date")) { 1431 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1432 } else if (name.equals("performer")) { 1433 this.performer = TypeConvertor.castToReference(value); // Reference 1434 } else if (name.equals("previous")) { 1435 this.previous = TypeConvertor.castToReference(value); // Reference 1436 } else if (name.equals("problem")) { 1437 this.getProblem().add(TypeConvertor.castToReference(value)); 1438 } else if (name.equals("changePattern")) { 1439 this.changePattern = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1440 } else if (name.equals("protocol")) { 1441 this.getProtocol().add(TypeConvertor.castToUri(value)); 1442 } else if (name.equals("summary")) { 1443 this.summary = TypeConvertor.castToString(value); // StringType 1444 } else if (name.equals("finding")) { 1445 this.getFinding().add((ClinicalImpressionFindingComponent) value); 1446 } else if (name.equals("prognosisCodeableConcept")) { 1447 this.getPrognosisCodeableConcept().add(TypeConvertor.castToCodeableConcept(value)); 1448 } else if (name.equals("prognosisReference")) { 1449 this.getPrognosisReference().add(TypeConvertor.castToReference(value)); 1450 } else if (name.equals("supportingInfo")) { 1451 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); 1452 } else if (name.equals("note")) { 1453 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1454 } else 1455 return super.setProperty(name, value); 1456 return value; 1457 } 1458 1459 @Override 1460 public void removeChild(String name, Base value) throws FHIRException { 1461 if (name.equals("identifier")) { 1462 this.getIdentifier().remove(value); 1463 } else if (name.equals("status")) { 1464 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1465 this.status = (Enumeration) value; // Enumeration<EventStatus> 1466 } else if (name.equals("statusReason")) { 1467 this.statusReason = null; 1468 } else if (name.equals("description")) { 1469 this.description = null; 1470 } else if (name.equals("subject")) { 1471 this.subject = null; 1472 } else if (name.equals("encounter")) { 1473 this.encounter = null; 1474 } else if (name.equals("effective[x]")) { 1475 this.effective = null; 1476 } else if (name.equals("date")) { 1477 this.date = null; 1478 } else if (name.equals("performer")) { 1479 this.performer = null; 1480 } else if (name.equals("previous")) { 1481 this.previous = null; 1482 } else if (name.equals("problem")) { 1483 this.getProblem().remove(value); 1484 } else if (name.equals("changePattern")) { 1485 this.changePattern = null; 1486 } else if (name.equals("protocol")) { 1487 this.getProtocol().remove(value); 1488 } else if (name.equals("summary")) { 1489 this.summary = null; 1490 } else if (name.equals("finding")) { 1491 this.getFinding().remove((ClinicalImpressionFindingComponent) value); 1492 } else if (name.equals("prognosisCodeableConcept")) { 1493 this.getPrognosisCodeableConcept().remove(value); 1494 } else if (name.equals("prognosisReference")) { 1495 this.getPrognosisReference().remove(value); 1496 } else if (name.equals("supportingInfo")) { 1497 this.getSupportingInfo().remove(value); 1498 } else if (name.equals("note")) { 1499 this.getNote().remove(value); 1500 } else 1501 super.removeChild(name, value); 1502 1503 } 1504 1505 @Override 1506 public Base makeProperty(int hash, String name) throws FHIRException { 1507 switch (hash) { 1508 case -1618432855: return addIdentifier(); 1509 case -892481550: return getStatusElement(); 1510 case 2051346646: return getStatusReason(); 1511 case -1724546052: return getDescriptionElement(); 1512 case -1867885268: return getSubject(); 1513 case 1524132147: return getEncounter(); 1514 case 247104889: return getEffective(); 1515 case -1468651097: return getEffective(); 1516 case 3076014: return getDateElement(); 1517 case 481140686: return getPerformer(); 1518 case -1273775369: return getPrevious(); 1519 case -309542241: return addProblem(); 1520 case -1770133056: return getChangePattern(); 1521 case -989163880: return addProtocolElement(); 1522 case -1857640538: return getSummaryElement(); 1523 case -853173367: return addFinding(); 1524 case -676337953: return addPrognosisCodeableConcept(); 1525 case -587137783: return addPrognosisReference(); 1526 case 1922406657: return addSupportingInfo(); 1527 case 3387378: return addNote(); 1528 default: return super.makeProperty(hash, name); 1529 } 1530 1531 } 1532 1533 @Override 1534 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1535 switch (hash) { 1536 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1537 case -892481550: /*status*/ return new String[] {"code"}; 1538 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 1539 case -1724546052: /*description*/ return new String[] {"string"}; 1540 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1541 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1542 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 1543 case 3076014: /*date*/ return new String[] {"dateTime"}; 1544 case 481140686: /*performer*/ return new String[] {"Reference"}; 1545 case -1273775369: /*previous*/ return new String[] {"Reference"}; 1546 case -309542241: /*problem*/ return new String[] {"Reference"}; 1547 case -1770133056: /*changePattern*/ return new String[] {"CodeableConcept"}; 1548 case -989163880: /*protocol*/ return new String[] {"uri"}; 1549 case -1857640538: /*summary*/ return new String[] {"string"}; 1550 case -853173367: /*finding*/ return new String[] {}; 1551 case -676337953: /*prognosisCodeableConcept*/ return new String[] {"CodeableConcept"}; 1552 case -587137783: /*prognosisReference*/ return new String[] {"Reference"}; 1553 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 1554 case 3387378: /*note*/ return new String[] {"Annotation"}; 1555 default: return super.getTypesForProperty(hash, name); 1556 } 1557 1558 } 1559 1560 @Override 1561 public Base addChild(String name) throws FHIRException { 1562 if (name.equals("identifier")) { 1563 return addIdentifier(); 1564 } 1565 else if (name.equals("status")) { 1566 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.status"); 1567 } 1568 else if (name.equals("statusReason")) { 1569 this.statusReason = new CodeableConcept(); 1570 return this.statusReason; 1571 } 1572 else if (name.equals("description")) { 1573 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.description"); 1574 } 1575 else if (name.equals("subject")) { 1576 this.subject = new Reference(); 1577 return this.subject; 1578 } 1579 else if (name.equals("encounter")) { 1580 this.encounter = new Reference(); 1581 return this.encounter; 1582 } 1583 else if (name.equals("effectiveDateTime")) { 1584 this.effective = new DateTimeType(); 1585 return this.effective; 1586 } 1587 else if (name.equals("effectivePeriod")) { 1588 this.effective = new Period(); 1589 return this.effective; 1590 } 1591 else if (name.equals("date")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.date"); 1593 } 1594 else if (name.equals("performer")) { 1595 this.performer = new Reference(); 1596 return this.performer; 1597 } 1598 else if (name.equals("previous")) { 1599 this.previous = new Reference(); 1600 return this.previous; 1601 } 1602 else if (name.equals("problem")) { 1603 return addProblem(); 1604 } 1605 else if (name.equals("changePattern")) { 1606 this.changePattern = new CodeableConcept(); 1607 return this.changePattern; 1608 } 1609 else if (name.equals("protocol")) { 1610 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.protocol"); 1611 } 1612 else if (name.equals("summary")) { 1613 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.summary"); 1614 } 1615 else if (name.equals("finding")) { 1616 return addFinding(); 1617 } 1618 else if (name.equals("prognosisCodeableConcept")) { 1619 return addPrognosisCodeableConcept(); 1620 } 1621 else if (name.equals("prognosisReference")) { 1622 return addPrognosisReference(); 1623 } 1624 else if (name.equals("supportingInfo")) { 1625 return addSupportingInfo(); 1626 } 1627 else if (name.equals("note")) { 1628 return addNote(); 1629 } 1630 else 1631 return super.addChild(name); 1632 } 1633 1634 public String fhirType() { 1635 return "ClinicalImpression"; 1636 1637 } 1638 1639 public ClinicalImpression copy() { 1640 ClinicalImpression dst = new ClinicalImpression(); 1641 copyValues(dst); 1642 return dst; 1643 } 1644 1645 public void copyValues(ClinicalImpression dst) { 1646 super.copyValues(dst); 1647 if (identifier != null) { 1648 dst.identifier = new ArrayList<Identifier>(); 1649 for (Identifier i : identifier) 1650 dst.identifier.add(i.copy()); 1651 }; 1652 dst.status = status == null ? null : status.copy(); 1653 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1654 dst.description = description == null ? null : description.copy(); 1655 dst.subject = subject == null ? null : subject.copy(); 1656 dst.encounter = encounter == null ? null : encounter.copy(); 1657 dst.effective = effective == null ? null : effective.copy(); 1658 dst.date = date == null ? null : date.copy(); 1659 dst.performer = performer == null ? null : performer.copy(); 1660 dst.previous = previous == null ? null : previous.copy(); 1661 if (problem != null) { 1662 dst.problem = new ArrayList<Reference>(); 1663 for (Reference i : problem) 1664 dst.problem.add(i.copy()); 1665 }; 1666 dst.changePattern = changePattern == null ? null : changePattern.copy(); 1667 if (protocol != null) { 1668 dst.protocol = new ArrayList<UriType>(); 1669 for (UriType i : protocol) 1670 dst.protocol.add(i.copy()); 1671 }; 1672 dst.summary = summary == null ? null : summary.copy(); 1673 if (finding != null) { 1674 dst.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1675 for (ClinicalImpressionFindingComponent i : finding) 1676 dst.finding.add(i.copy()); 1677 }; 1678 if (prognosisCodeableConcept != null) { 1679 dst.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1680 for (CodeableConcept i : prognosisCodeableConcept) 1681 dst.prognosisCodeableConcept.add(i.copy()); 1682 }; 1683 if (prognosisReference != null) { 1684 dst.prognosisReference = new ArrayList<Reference>(); 1685 for (Reference i : prognosisReference) 1686 dst.prognosisReference.add(i.copy()); 1687 }; 1688 if (supportingInfo != null) { 1689 dst.supportingInfo = new ArrayList<Reference>(); 1690 for (Reference i : supportingInfo) 1691 dst.supportingInfo.add(i.copy()); 1692 }; 1693 if (note != null) { 1694 dst.note = new ArrayList<Annotation>(); 1695 for (Annotation i : note) 1696 dst.note.add(i.copy()); 1697 }; 1698 } 1699 1700 protected ClinicalImpression typedCopy() { 1701 return copy(); 1702 } 1703 1704 @Override 1705 public boolean equalsDeep(Base other_) { 1706 if (!super.equalsDeep(other_)) 1707 return false; 1708 if (!(other_ instanceof ClinicalImpression)) 1709 return false; 1710 ClinicalImpression o = (ClinicalImpression) other_; 1711 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 1712 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1713 && compareDeep(effective, o.effective, true) && compareDeep(date, o.date, true) && compareDeep(performer, o.performer, true) 1714 && compareDeep(previous, o.previous, true) && compareDeep(problem, o.problem, true) && compareDeep(changePattern, o.changePattern, true) 1715 && compareDeep(protocol, o.protocol, true) && compareDeep(summary, o.summary, true) && compareDeep(finding, o.finding, true) 1716 && compareDeep(prognosisCodeableConcept, o.prognosisCodeableConcept, true) && compareDeep(prognosisReference, o.prognosisReference, true) 1717 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(note, o.note, true); 1718 } 1719 1720 @Override 1721 public boolean equalsShallow(Base other_) { 1722 if (!super.equalsShallow(other_)) 1723 return false; 1724 if (!(other_ instanceof ClinicalImpression)) 1725 return false; 1726 ClinicalImpression o = (ClinicalImpression) other_; 1727 return compareValues(status, o.status, true) && compareValues(description, o.description, true) && compareValues(date, o.date, true) 1728 && compareValues(protocol, o.protocol, true) && compareValues(summary, o.summary, true); 1729 } 1730 1731 public boolean isEmpty() { 1732 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusReason 1733 , description, subject, encounter, effective, date, performer, previous, problem 1734 , changePattern, protocol, summary, finding, prognosisCodeableConcept, prognosisReference 1735 , supportingInfo, note); 1736 } 1737 1738 @Override 1739 public ResourceType getResourceType() { 1740 return ResourceType.ClinicalImpression; 1741 } 1742 1743 /** 1744 * Search parameter: <b>finding-code</b> 1745 * <p> 1746 * Description: <b>Reference to a concept (by class)</b><br> 1747 * Type: <b>token</b><br> 1748 * Path: <b>ClinicalImpression.finding.item.concept</b><br> 1749 * </p> 1750 */ 1751 @SearchParamDefinition(name="finding-code", path="ClinicalImpression.finding.item.concept", description="Reference to a concept (by class)", type="token" ) 1752 public static final String SP_FINDING_CODE = "finding-code"; 1753 /** 1754 * <b>Fluent Client</b> search parameter constant for <b>finding-code</b> 1755 * <p> 1756 * Description: <b>Reference to a concept (by class)</b><br> 1757 * Type: <b>token</b><br> 1758 * Path: <b>ClinicalImpression.finding.item.concept</b><br> 1759 * </p> 1760 */ 1761 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FINDING_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FINDING_CODE); 1762 1763 /** 1764 * Search parameter: <b>finding-ref</b> 1765 * <p> 1766 * Description: <b>Reference to a resource (by instance)</b><br> 1767 * Type: <b>reference</b><br> 1768 * Path: <b>ClinicalImpression.finding.item.reference</b><br> 1769 * </p> 1770 */ 1771 @SearchParamDefinition(name="finding-ref", path="ClinicalImpression.finding.item.reference", description="Reference to a resource (by instance)", type="reference", target={Condition.class, DocumentReference.class, Observation.class } ) 1772 public static final String SP_FINDING_REF = "finding-ref"; 1773 /** 1774 * <b>Fluent Client</b> search parameter constant for <b>finding-ref</b> 1775 * <p> 1776 * Description: <b>Reference to a resource (by instance)</b><br> 1777 * Type: <b>reference</b><br> 1778 * Path: <b>ClinicalImpression.finding.item.reference</b><br> 1779 * </p> 1780 */ 1781 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FINDING_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FINDING_REF); 1782 1783/** 1784 * Constant for fluent queries to be used to add include statements. Specifies 1785 * the path value of "<b>ClinicalImpression:finding-ref</b>". 1786 */ 1787 public static final ca.uhn.fhir.model.api.Include INCLUDE_FINDING_REF = new ca.uhn.fhir.model.api.Include("ClinicalImpression:finding-ref").toLocked(); 1788 1789 /** 1790 * Search parameter: <b>performer</b> 1791 * <p> 1792 * Description: <b>The clinician performing the assessment</b><br> 1793 * Type: <b>reference</b><br> 1794 * Path: <b>ClinicalImpression.performer</b><br> 1795 * </p> 1796 */ 1797 @SearchParamDefinition(name="performer", path="ClinicalImpression.performer", description="The clinician performing the assessment", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class, PractitionerRole.class } ) 1798 public static final String SP_PERFORMER = "performer"; 1799 /** 1800 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 1801 * <p> 1802 * Description: <b>The clinician performing the assessment</b><br> 1803 * Type: <b>reference</b><br> 1804 * Path: <b>ClinicalImpression.performer</b><br> 1805 * </p> 1806 */ 1807 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 1808 1809/** 1810 * Constant for fluent queries to be used to add include statements. Specifies 1811 * the path value of "<b>ClinicalImpression:performer</b>". 1812 */ 1813 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("ClinicalImpression:performer").toLocked(); 1814 1815 /** 1816 * Search parameter: <b>previous</b> 1817 * <p> 1818 * Description: <b>Reference to last assessment</b><br> 1819 * Type: <b>reference</b><br> 1820 * Path: <b>ClinicalImpression.previous</b><br> 1821 * </p> 1822 */ 1823 @SearchParamDefinition(name="previous", path="ClinicalImpression.previous", description="Reference to last assessment", type="reference", target={ClinicalImpression.class } ) 1824 public static final String SP_PREVIOUS = "previous"; 1825 /** 1826 * <b>Fluent Client</b> search parameter constant for <b>previous</b> 1827 * <p> 1828 * Description: <b>Reference to last assessment</b><br> 1829 * Type: <b>reference</b><br> 1830 * Path: <b>ClinicalImpression.previous</b><br> 1831 * </p> 1832 */ 1833 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREVIOUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREVIOUS); 1834 1835/** 1836 * Constant for fluent queries to be used to add include statements. Specifies 1837 * the path value of "<b>ClinicalImpression:previous</b>". 1838 */ 1839 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREVIOUS = new ca.uhn.fhir.model.api.Include("ClinicalImpression:previous").toLocked(); 1840 1841 /** 1842 * Search parameter: <b>problem</b> 1843 * <p> 1844 * Description: <b>Relevant impressions of patient state</b><br> 1845 * Type: <b>reference</b><br> 1846 * Path: <b>ClinicalImpression.problem</b><br> 1847 * </p> 1848 */ 1849 @SearchParamDefinition(name="problem", path="ClinicalImpression.problem", description="Relevant impressions of patient state", type="reference", target={AllergyIntolerance.class, Condition.class } ) 1850 public static final String SP_PROBLEM = "problem"; 1851 /** 1852 * <b>Fluent Client</b> search parameter constant for <b>problem</b> 1853 * <p> 1854 * Description: <b>Relevant impressions of patient state</b><br> 1855 * Type: <b>reference</b><br> 1856 * Path: <b>ClinicalImpression.problem</b><br> 1857 * </p> 1858 */ 1859 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROBLEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROBLEM); 1860 1861/** 1862 * Constant for fluent queries to be used to add include statements. Specifies 1863 * the path value of "<b>ClinicalImpression:problem</b>". 1864 */ 1865 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROBLEM = new ca.uhn.fhir.model.api.Include("ClinicalImpression:problem").toLocked(); 1866 1867 /** 1868 * Search parameter: <b>status</b> 1869 * <p> 1870 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown</b><br> 1871 * Type: <b>token</b><br> 1872 * Path: <b>ClinicalImpression.status</b><br> 1873 * </p> 1874 */ 1875 @SearchParamDefinition(name="status", path="ClinicalImpression.status", description="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type="token" ) 1876 public static final String SP_STATUS = "status"; 1877 /** 1878 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1879 * <p> 1880 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown</b><br> 1881 * Type: <b>token</b><br> 1882 * Path: <b>ClinicalImpression.status</b><br> 1883 * </p> 1884 */ 1885 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1886 1887 /** 1888 * Search parameter: <b>subject</b> 1889 * <p> 1890 * Description: <b>Patient or group assessed</b><br> 1891 * Type: <b>reference</b><br> 1892 * Path: <b>ClinicalImpression.subject</b><br> 1893 * </p> 1894 */ 1895 @SearchParamDefinition(name="subject", path="ClinicalImpression.subject", description="Patient or group assessed", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 1896 public static final String SP_SUBJECT = "subject"; 1897 /** 1898 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1899 * <p> 1900 * Description: <b>Patient or group assessed</b><br> 1901 * Type: <b>reference</b><br> 1902 * Path: <b>ClinicalImpression.subject</b><br> 1903 * </p> 1904 */ 1905 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1906 1907/** 1908 * Constant for fluent queries to be used to add include statements. Specifies 1909 * the path value of "<b>ClinicalImpression:subject</b>". 1910 */ 1911 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ClinicalImpression:subject").toLocked(); 1912 1913 /** 1914 * Search parameter: <b>supporting-info</b> 1915 * <p> 1916 * Description: <b>Information supporting the clinical impression</b><br> 1917 * Type: <b>reference</b><br> 1918 * Path: <b>ClinicalImpression.supportingInfo</b><br> 1919 * </p> 1920 */ 1921 @SearchParamDefinition(name="supporting-info", path="ClinicalImpression.supportingInfo", description="Information supporting the clinical impression", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 1922 public static final String SP_SUPPORTING_INFO = "supporting-info"; 1923 /** 1924 * <b>Fluent Client</b> search parameter constant for <b>supporting-info</b> 1925 * <p> 1926 * Description: <b>Information supporting the clinical impression</b><br> 1927 * Type: <b>reference</b><br> 1928 * Path: <b>ClinicalImpression.supportingInfo</b><br> 1929 * </p> 1930 */ 1931 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTING_INFO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPORTING_INFO); 1932 1933/** 1934 * Constant for fluent queries to be used to add include statements. Specifies 1935 * the path value of "<b>ClinicalImpression:supporting-info</b>". 1936 */ 1937 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTING_INFO = new ca.uhn.fhir.model.api.Include("ClinicalImpression:supporting-info").toLocked(); 1938 1939 /** 1940 * Search parameter: <b>date</b> 1941 * <p> 1942 * Description: <b>Multiple Resources: 1943 1944* [AdverseEvent](adverseevent.html): When the event occurred 1945* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1946* [Appointment](appointment.html): Appointment date/time. 1947* [AuditEvent](auditevent.html): Time when the event was recorded 1948* [CarePlan](careplan.html): Time period plan covers 1949* [CareTeam](careteam.html): A date within the coverage time period. 1950* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1951* [Composition](composition.html): Composition editing time 1952* [Consent](consent.html): When consent was agreed to 1953* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1954* [DocumentReference](documentreference.html): When this document reference was created 1955* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1956* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1957* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1958* [Flag](flag.html): Time period when flag is active 1959* [Immunization](immunization.html): Vaccination (non)-Administration Date 1960* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1961* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1962* [Invoice](invoice.html): Invoice date / posting date 1963* [List](list.html): When the list was prepared 1964* [MeasureReport](measurereport.html): The date of the measure report 1965* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1966* [Observation](observation.html): Clinically relevant time/time-period for observation 1967* [Procedure](procedure.html): When the procedure occurred or is occurring 1968* [ResearchSubject](researchsubject.html): Start and end of participation 1969* [RiskAssessment](riskassessment.html): When was assessment made? 1970* [SupplyRequest](supplyrequest.html): When the request was made 1971</b><br> 1972 * Type: <b>date</b><br> 1973 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1974 * </p> 1975 */ 1976 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1977 public static final String SP_DATE = "date"; 1978 /** 1979 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1980 * <p> 1981 * Description: <b>Multiple Resources: 1982 1983* [AdverseEvent](adverseevent.html): When the event occurred 1984* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1985* [Appointment](appointment.html): Appointment date/time. 1986* [AuditEvent](auditevent.html): Time when the event was recorded 1987* [CarePlan](careplan.html): Time period plan covers 1988* [CareTeam](careteam.html): A date within the coverage time period. 1989* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1990* [Composition](composition.html): Composition editing time 1991* [Consent](consent.html): When consent was agreed to 1992* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1993* [DocumentReference](documentreference.html): When this document reference was created 1994* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1995* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1996* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1997* [Flag](flag.html): Time period when flag is active 1998* [Immunization](immunization.html): Vaccination (non)-Administration Date 1999* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2000* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2001* [Invoice](invoice.html): Invoice date / posting date 2002* [List](list.html): When the list was prepared 2003* [MeasureReport](measurereport.html): The date of the measure report 2004* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2005* [Observation](observation.html): Clinically relevant time/time-period for observation 2006* [Procedure](procedure.html): When the procedure occurred or is occurring 2007* [ResearchSubject](researchsubject.html): Start and end of participation 2008* [RiskAssessment](riskassessment.html): When was assessment made? 2009* [SupplyRequest](supplyrequest.html): When the request was made 2010</b><br> 2011 * Type: <b>date</b><br> 2012 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2013 * </p> 2014 */ 2015 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2016 2017 /** 2018 * Search parameter: <b>encounter</b> 2019 * <p> 2020 * Description: <b>Multiple Resources: 2021 2022* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2023* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2024* [ChargeItem](chargeitem.html): Encounter associated with event 2025* [Claim](claim.html): Encounters associated with a billed line item 2026* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2027* [Communication](communication.html): The Encounter during which this Communication was created 2028* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2029* [Composition](composition.html): Context of the Composition 2030* [Condition](condition.html): The Encounter during which this Condition was created 2031* [DeviceRequest](devicerequest.html): Encounter during which request was created 2032* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2033* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2034* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2035* [Flag](flag.html): Alert relevant during encounter 2036* [ImagingStudy](imagingstudy.html): The context of the study 2037* [List](list.html): Context in which list created 2038* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2039* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2040* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2041* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2042* [Observation](observation.html): Encounter related to the observation 2043* [Procedure](procedure.html): The Encounter during which this Procedure was created 2044* [Provenance](provenance.html): Encounter related to the Provenance 2045* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2046* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2047* [RiskAssessment](riskassessment.html): Where was assessment performed? 2048* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2049* [Task](task.html): Search by encounter 2050* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2051</b><br> 2052 * Type: <b>reference</b><br> 2053 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2054 * </p> 2055 */ 2056 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2057 public static final String SP_ENCOUNTER = "encounter"; 2058 /** 2059 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2060 * <p> 2061 * Description: <b>Multiple Resources: 2062 2063* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2064* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2065* [ChargeItem](chargeitem.html): Encounter associated with event 2066* [Claim](claim.html): Encounters associated with a billed line item 2067* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2068* [Communication](communication.html): The Encounter during which this Communication was created 2069* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2070* [Composition](composition.html): Context of the Composition 2071* [Condition](condition.html): The Encounter during which this Condition was created 2072* [DeviceRequest](devicerequest.html): Encounter during which request was created 2073* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2074* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2075* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2076* [Flag](flag.html): Alert relevant during encounter 2077* [ImagingStudy](imagingstudy.html): The context of the study 2078* [List](list.html): Context in which list created 2079* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2080* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2081* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2082* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2083* [Observation](observation.html): Encounter related to the observation 2084* [Procedure](procedure.html): The Encounter during which this Procedure was created 2085* [Provenance](provenance.html): Encounter related to the Provenance 2086* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2087* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2088* [RiskAssessment](riskassessment.html): Where was assessment performed? 2089* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2090* [Task](task.html): Search by encounter 2091* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2092</b><br> 2093 * Type: <b>reference</b><br> 2094 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2095 * </p> 2096 */ 2097 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2098 2099/** 2100 * Constant for fluent queries to be used to add include statements. Specifies 2101 * the path value of "<b>ClinicalImpression:encounter</b>". 2102 */ 2103 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ClinicalImpression:encounter").toLocked(); 2104 2105 /** 2106 * Search parameter: <b>identifier</b> 2107 * <p> 2108 * Description: <b>Multiple Resources: 2109 2110* [Account](account.html): Account number 2111* [AdverseEvent](adverseevent.html): Business identifier for the event 2112* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2113* [Appointment](appointment.html): An Identifier of the Appointment 2114* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2115* [Basic](basic.html): Business identifier 2116* [BodyStructure](bodystructure.html): Bodystructure identifier 2117* [CarePlan](careplan.html): External Ids for this plan 2118* [CareTeam](careteam.html): External Ids for this team 2119* [ChargeItem](chargeitem.html): Business Identifier for item 2120* [Claim](claim.html): The primary identifier of the financial resource 2121* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2122* [ClinicalImpression](clinicalimpression.html): Business identifier 2123* [Communication](communication.html): Unique identifier 2124* [CommunicationRequest](communicationrequest.html): Unique identifier 2125* [Composition](composition.html): Version-independent identifier for the Composition 2126* [Condition](condition.html): A unique identifier of the condition record 2127* [Consent](consent.html): Identifier for this record (external references) 2128* [Contract](contract.html): The identity of the contract 2129* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2130* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2131* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2132* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2133* [DeviceRequest](devicerequest.html): Business identifier for request/order 2134* [DeviceUsage](deviceusage.html): Search by identifier 2135* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2136* [DocumentReference](documentreference.html): Identifier of the attachment binary 2137* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2138* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2139* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2140* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2141* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2142* [Flag](flag.html): Business identifier 2143* [Goal](goal.html): External Ids for this goal 2144* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2145* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2146* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2147* [Immunization](immunization.html): Business identifier 2148* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2149* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2150* [Invoice](invoice.html): Business Identifier for item 2151* [List](list.html): Business identifier 2152* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2153* [Medication](medication.html): Returns medications with this external identifier 2154* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2155* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2156* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2157* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2158* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2159* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2160* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2161* [Observation](observation.html): The unique id for a particular observation 2162* [Person](person.html): A person Identifier 2163* [Procedure](procedure.html): A unique identifier for a procedure 2164* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2165* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2166* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2167* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2168* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2169* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2170* [Specimen](specimen.html): The unique identifier associated with the specimen 2171* [SupplyDelivery](supplydelivery.html): External identifier 2172* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2173* [Task](task.html): Search for a task instance by its business identifier 2174* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2175</b><br> 2176 * Type: <b>token</b><br> 2177 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2178 * </p> 2179 */ 2180 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2181 public static final String SP_IDENTIFIER = "identifier"; 2182 /** 2183 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2184 * <p> 2185 * Description: <b>Multiple Resources: 2186 2187* [Account](account.html): Account number 2188* [AdverseEvent](adverseevent.html): Business identifier for the event 2189* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2190* [Appointment](appointment.html): An Identifier of the Appointment 2191* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2192* [Basic](basic.html): Business identifier 2193* [BodyStructure](bodystructure.html): Bodystructure identifier 2194* [CarePlan](careplan.html): External Ids for this plan 2195* [CareTeam](careteam.html): External Ids for this team 2196* [ChargeItem](chargeitem.html): Business Identifier for item 2197* [Claim](claim.html): The primary identifier of the financial resource 2198* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2199* [ClinicalImpression](clinicalimpression.html): Business identifier 2200* [Communication](communication.html): Unique identifier 2201* [CommunicationRequest](communicationrequest.html): Unique identifier 2202* [Composition](composition.html): Version-independent identifier for the Composition 2203* [Condition](condition.html): A unique identifier of the condition record 2204* [Consent](consent.html): Identifier for this record (external references) 2205* [Contract](contract.html): The identity of the contract 2206* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2207* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2208* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2209* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2210* [DeviceRequest](devicerequest.html): Business identifier for request/order 2211* [DeviceUsage](deviceusage.html): Search by identifier 2212* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2213* [DocumentReference](documentreference.html): Identifier of the attachment binary 2214* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2215* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2216* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2217* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2218* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2219* [Flag](flag.html): Business identifier 2220* [Goal](goal.html): External Ids for this goal 2221* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2222* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2223* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2224* [Immunization](immunization.html): Business identifier 2225* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2226* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2227* [Invoice](invoice.html): Business Identifier for item 2228* [List](list.html): Business identifier 2229* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2230* [Medication](medication.html): Returns medications with this external identifier 2231* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2232* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2233* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2234* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2235* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2236* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2237* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2238* [Observation](observation.html): The unique id for a particular observation 2239* [Person](person.html): A person Identifier 2240* [Procedure](procedure.html): A unique identifier for a procedure 2241* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2242* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2243* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2244* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2245* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2246* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2247* [Specimen](specimen.html): The unique identifier associated with the specimen 2248* [SupplyDelivery](supplydelivery.html): External identifier 2249* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2250* [Task](task.html): Search for a task instance by its business identifier 2251* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2252</b><br> 2253 * Type: <b>token</b><br> 2254 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2255 * </p> 2256 */ 2257 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2258 2259 /** 2260 * Search parameter: <b>patient</b> 2261 * <p> 2262 * Description: <b>Multiple Resources: 2263 2264* [Account](account.html): The entity that caused the expenses 2265* [AdverseEvent](adverseevent.html): Subject impacted by event 2266* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2267* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2268* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2269* [AuditEvent](auditevent.html): Where the activity involved patient data 2270* [Basic](basic.html): Identifies the focus of this resource 2271* [BodyStructure](bodystructure.html): Who this is about 2272* [CarePlan](careplan.html): Who the care plan is for 2273* [CareTeam](careteam.html): Who care team is for 2274* [ChargeItem](chargeitem.html): Individual service was done for/to 2275* [Claim](claim.html): Patient receiving the products or services 2276* [ClaimResponse](claimresponse.html): The subject of care 2277* [ClinicalImpression](clinicalimpression.html): Patient assessed 2278* [Communication](communication.html): Focus of message 2279* [CommunicationRequest](communicationrequest.html): Focus of message 2280* [Composition](composition.html): Who and/or what the composition is about 2281* [Condition](condition.html): Who has the condition? 2282* [Consent](consent.html): Who the consent applies to 2283* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2284* [Coverage](coverage.html): Retrieve coverages for a patient 2285* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2286* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2287* [DetectedIssue](detectedissue.html): Associated patient 2288* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2289* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2290* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2291* [DocumentReference](documentreference.html): Who/what is the subject of the document 2292* [Encounter](encounter.html): The patient present at the encounter 2293* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2294* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2295* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2296* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2297* [Flag](flag.html): The identity of a subject to list flags for 2298* [Goal](goal.html): Who this goal is intended for 2299* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2300* [ImagingSelection](imagingselection.html): Who the study is about 2301* [ImagingStudy](imagingstudy.html): Who the study is about 2302* [Immunization](immunization.html): The patient for the vaccination record 2303* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2304* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2305* [Invoice](invoice.html): Recipient(s) of goods and services 2306* [List](list.html): If all resources have the same subject 2307* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2308* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2309* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2310* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2311* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2312* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2313* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2314* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2315* [Observation](observation.html): The subject that the observation is about (if patient) 2316* [Person](person.html): The Person links to this Patient 2317* [Procedure](procedure.html): Search by subject - a patient 2318* [Provenance](provenance.html): Where the activity involved patient data 2319* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2320* [RelatedPerson](relatedperson.html): The patient this related person is related to 2321* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2322* [ResearchSubject](researchsubject.html): Who or what is part of study 2323* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2324* [ServiceRequest](servicerequest.html): Search by subject - a patient 2325* [Specimen](specimen.html): The patient the specimen comes from 2326* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2327* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2328* [Task](task.html): Search by patient 2329* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2330</b><br> 2331 * Type: <b>reference</b><br> 2332 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2333 * </p> 2334 */ 2335 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2336 public static final String SP_PATIENT = "patient"; 2337 /** 2338 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2339 * <p> 2340 * Description: <b>Multiple Resources: 2341 2342* [Account](account.html): The entity that caused the expenses 2343* [AdverseEvent](adverseevent.html): Subject impacted by event 2344* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2345* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2346* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2347* [AuditEvent](auditevent.html): Where the activity involved patient data 2348* [Basic](basic.html): Identifies the focus of this resource 2349* [BodyStructure](bodystructure.html): Who this is about 2350* [CarePlan](careplan.html): Who the care plan is for 2351* [CareTeam](careteam.html): Who care team is for 2352* [ChargeItem](chargeitem.html): Individual service was done for/to 2353* [Claim](claim.html): Patient receiving the products or services 2354* [ClaimResponse](claimresponse.html): The subject of care 2355* [ClinicalImpression](clinicalimpression.html): Patient assessed 2356* [Communication](communication.html): Focus of message 2357* [CommunicationRequest](communicationrequest.html): Focus of message 2358* [Composition](composition.html): Who and/or what the composition is about 2359* [Condition](condition.html): Who has the condition? 2360* [Consent](consent.html): Who the consent applies to 2361* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2362* [Coverage](coverage.html): Retrieve coverages for a patient 2363* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2364* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2365* [DetectedIssue](detectedissue.html): Associated patient 2366* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2367* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2368* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2369* [DocumentReference](documentreference.html): Who/what is the subject of the document 2370* [Encounter](encounter.html): The patient present at the encounter 2371* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2372* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2373* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2374* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2375* [Flag](flag.html): The identity of a subject to list flags for 2376* [Goal](goal.html): Who this goal is intended for 2377* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2378* [ImagingSelection](imagingselection.html): Who the study is about 2379* [ImagingStudy](imagingstudy.html): Who the study is about 2380* [Immunization](immunization.html): The patient for the vaccination record 2381* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2382* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2383* [Invoice](invoice.html): Recipient(s) of goods and services 2384* [List](list.html): If all resources have the same subject 2385* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2386* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2387* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2388* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2389* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2390* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2391* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2392* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2393* [Observation](observation.html): The subject that the observation is about (if patient) 2394* [Person](person.html): The Person links to this Patient 2395* [Procedure](procedure.html): Search by subject - a patient 2396* [Provenance](provenance.html): Where the activity involved patient data 2397* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2398* [RelatedPerson](relatedperson.html): The patient this related person is related to 2399* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2400* [ResearchSubject](researchsubject.html): Who or what is part of study 2401* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2402* [ServiceRequest](servicerequest.html): Search by subject - a patient 2403* [Specimen](specimen.html): The patient the specimen comes from 2404* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2405* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2406* [Task](task.html): Search by patient 2407* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2408</b><br> 2409 * Type: <b>reference</b><br> 2410 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2411 * </p> 2412 */ 2413 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2414 2415/** 2416 * Constant for fluent queries to be used to add include statements. Specifies 2417 * the path value of "<b>ClinicalImpression:patient</b>". 2418 */ 2419 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ClinicalImpression:patient").toLocked(); 2420 2421 2422} 2423