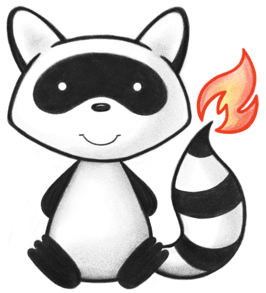
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A single issue - either an indication, contraindication, interaction or an undesirable effect for a medicinal product, medication, device or procedure. 052 */ 053@ResourceDef(name="ClinicalUseDefinition", profile="http://hl7.org/fhir/StructureDefinition/ClinicalUseDefinition") 054public class ClinicalUseDefinition extends DomainResource { 055 056 public enum ClinicalUseDefinitionType { 057 /** 058 * A reason for giving the medication. 059 */ 060 INDICATION, 061 /** 062 * A reason for not giving the medication. 063 */ 064 CONTRAINDICATION, 065 /** 066 * Interactions between the medication and other substances. 067 */ 068 INTERACTION, 069 /** 070 * Side effects or adverse effects associated with the medication. 071 */ 072 UNDESIRABLEEFFECT, 073 /** 074 * A general warning or issue that is not specifically one of the other types. 075 */ 076 WARNING, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static ClinicalUseDefinitionType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("indication".equals(codeString)) 085 return INDICATION; 086 if ("contraindication".equals(codeString)) 087 return CONTRAINDICATION; 088 if ("interaction".equals(codeString)) 089 return INTERACTION; 090 if ("undesirable-effect".equals(codeString)) 091 return UNDESIRABLEEFFECT; 092 if ("warning".equals(codeString)) 093 return WARNING; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown ClinicalUseDefinitionType code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case INDICATION: return "indication"; 102 case CONTRAINDICATION: return "contraindication"; 103 case INTERACTION: return "interaction"; 104 case UNDESIRABLEEFFECT: return "undesirable-effect"; 105 case WARNING: return "warning"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case INDICATION: return "http://hl7.org/fhir/clinical-use-definition-type"; 113 case CONTRAINDICATION: return "http://hl7.org/fhir/clinical-use-definition-type"; 114 case INTERACTION: return "http://hl7.org/fhir/clinical-use-definition-type"; 115 case UNDESIRABLEEFFECT: return "http://hl7.org/fhir/clinical-use-definition-type"; 116 case WARNING: return "http://hl7.org/fhir/clinical-use-definition-type"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case INDICATION: return "A reason for giving the medication."; 124 case CONTRAINDICATION: return "A reason for not giving the medication."; 125 case INTERACTION: return "Interactions between the medication and other substances."; 126 case UNDESIRABLEEFFECT: return "Side effects or adverse effects associated with the medication."; 127 case WARNING: return "A general warning or issue that is not specifically one of the other types."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case INDICATION: return "Indication"; 135 case CONTRAINDICATION: return "Contraindication"; 136 case INTERACTION: return "Interaction"; 137 case UNDESIRABLEEFFECT: return "Undesirable Effect"; 138 case WARNING: return "Warning"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class ClinicalUseDefinitionTypeEnumFactory implements EnumFactory<ClinicalUseDefinitionType> { 146 public ClinicalUseDefinitionType fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("indication".equals(codeString)) 151 return ClinicalUseDefinitionType.INDICATION; 152 if ("contraindication".equals(codeString)) 153 return ClinicalUseDefinitionType.CONTRAINDICATION; 154 if ("interaction".equals(codeString)) 155 return ClinicalUseDefinitionType.INTERACTION; 156 if ("undesirable-effect".equals(codeString)) 157 return ClinicalUseDefinitionType.UNDESIRABLEEFFECT; 158 if ("warning".equals(codeString)) 159 return ClinicalUseDefinitionType.WARNING; 160 throw new IllegalArgumentException("Unknown ClinicalUseDefinitionType code '"+codeString+"'"); 161 } 162 public Enumeration<ClinicalUseDefinitionType> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.NULL, code); 170 if ("indication".equals(codeString)) 171 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.INDICATION, code); 172 if ("contraindication".equals(codeString)) 173 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.CONTRAINDICATION, code); 174 if ("interaction".equals(codeString)) 175 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.INTERACTION, code); 176 if ("undesirable-effect".equals(codeString)) 177 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.UNDESIRABLEEFFECT, code); 178 if ("warning".equals(codeString)) 179 return new Enumeration<ClinicalUseDefinitionType>(this, ClinicalUseDefinitionType.WARNING, code); 180 throw new FHIRException("Unknown ClinicalUseDefinitionType code '"+codeString+"'"); 181 } 182 public String toCode(ClinicalUseDefinitionType code) { 183 if (code == ClinicalUseDefinitionType.NULL) 184 return null; 185 if (code == ClinicalUseDefinitionType.INDICATION) 186 return "indication"; 187 if (code == ClinicalUseDefinitionType.CONTRAINDICATION) 188 return "contraindication"; 189 if (code == ClinicalUseDefinitionType.INTERACTION) 190 return "interaction"; 191 if (code == ClinicalUseDefinitionType.UNDESIRABLEEFFECT) 192 return "undesirable-effect"; 193 if (code == ClinicalUseDefinitionType.WARNING) 194 return "warning"; 195 return "?"; 196 } 197 public String toSystem(ClinicalUseDefinitionType code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class ClinicalUseDefinitionContraindicationComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The situation that is being documented as contraindicating against this item. 206 */ 207 @Child(name = "diseaseSymptomProcedure", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 208 @Description(shortDefinition="The situation that is being documented as contraindicating against this item", formalDefinition="The situation that is being documented as contraindicating against this item." ) 209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/disease-symptom-procedure") 210 protected CodeableReference diseaseSymptomProcedure; 211 212 /** 213 * The status of the disease or symptom for the contraindication, for example "chronic" or "metastatic". 214 */ 215 @Child(name = "diseaseStatus", type = {CodeableReference.class}, order=2, min=0, max=1, modifier=false, summary=true) 216 @Description(shortDefinition="The status of the disease or symptom for the contraindication", formalDefinition="The status of the disease or symptom for the contraindication, for example \"chronic\" or \"metastatic\"." ) 217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/disease-status") 218 protected CodeableReference diseaseStatus; 219 220 /** 221 * A comorbidity (concurrent condition) or coinfection. 222 */ 223 @Child(name = "comorbidity", type = {CodeableReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 224 @Description(shortDefinition="A comorbidity (concurrent condition) or coinfection", formalDefinition="A comorbidity (concurrent condition) or coinfection." ) 225 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/disease-symptom-procedure") 226 protected List<CodeableReference> comorbidity; 227 228 /** 229 * The indication which this is a contraidication for. 230 */ 231 @Child(name = "indication", type = {ClinicalUseDefinition.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 232 @Description(shortDefinition="The indication which this is a contraidication for", formalDefinition="The indication which this is a contraidication for." ) 233 protected List<Reference> indication; 234 235 /** 236 * An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements. 237 */ 238 @Child(name = "applicability", type = {Expression.class}, order=5, min=0, max=1, modifier=false, summary=false) 239 @Description(shortDefinition="An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements", formalDefinition="An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements." ) 240 protected Expression applicability; 241 242 /** 243 * Information about the use of the medicinal product in relation to other therapies described as part of the contraindication. 244 */ 245 @Child(name = "otherTherapy", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 246 @Description(shortDefinition="Information about use of the product in relation to other therapies described as part of the contraindication", formalDefinition="Information about the use of the medicinal product in relation to other therapies described as part of the contraindication." ) 247 protected List<ClinicalUseDefinitionContraindicationOtherTherapyComponent> otherTherapy; 248 249 private static final long serialVersionUID = 1942194420L; 250 251 /** 252 * Constructor 253 */ 254 public ClinicalUseDefinitionContraindicationComponent() { 255 super(); 256 } 257 258 /** 259 * @return {@link #diseaseSymptomProcedure} (The situation that is being documented as contraindicating against this item.) 260 */ 261 public CodeableReference getDiseaseSymptomProcedure() { 262 if (this.diseaseSymptomProcedure == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create ClinicalUseDefinitionContraindicationComponent.diseaseSymptomProcedure"); 265 else if (Configuration.doAutoCreate()) 266 this.diseaseSymptomProcedure = new CodeableReference(); // cc 267 return this.diseaseSymptomProcedure; 268 } 269 270 public boolean hasDiseaseSymptomProcedure() { 271 return this.diseaseSymptomProcedure != null && !this.diseaseSymptomProcedure.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #diseaseSymptomProcedure} (The situation that is being documented as contraindicating against this item.) 276 */ 277 public ClinicalUseDefinitionContraindicationComponent setDiseaseSymptomProcedure(CodeableReference value) { 278 this.diseaseSymptomProcedure = value; 279 return this; 280 } 281 282 /** 283 * @return {@link #diseaseStatus} (The status of the disease or symptom for the contraindication, for example "chronic" or "metastatic".) 284 */ 285 public CodeableReference getDiseaseStatus() { 286 if (this.diseaseStatus == null) 287 if (Configuration.errorOnAutoCreate()) 288 throw new Error("Attempt to auto-create ClinicalUseDefinitionContraindicationComponent.diseaseStatus"); 289 else if (Configuration.doAutoCreate()) 290 this.diseaseStatus = new CodeableReference(); // cc 291 return this.diseaseStatus; 292 } 293 294 public boolean hasDiseaseStatus() { 295 return this.diseaseStatus != null && !this.diseaseStatus.isEmpty(); 296 } 297 298 /** 299 * @param value {@link #diseaseStatus} (The status of the disease or symptom for the contraindication, for example "chronic" or "metastatic".) 300 */ 301 public ClinicalUseDefinitionContraindicationComponent setDiseaseStatus(CodeableReference value) { 302 this.diseaseStatus = value; 303 return this; 304 } 305 306 /** 307 * @return {@link #comorbidity} (A comorbidity (concurrent condition) or coinfection.) 308 */ 309 public List<CodeableReference> getComorbidity() { 310 if (this.comorbidity == null) 311 this.comorbidity = new ArrayList<CodeableReference>(); 312 return this.comorbidity; 313 } 314 315 /** 316 * @return Returns a reference to <code>this</code> for easy method chaining 317 */ 318 public ClinicalUseDefinitionContraindicationComponent setComorbidity(List<CodeableReference> theComorbidity) { 319 this.comorbidity = theComorbidity; 320 return this; 321 } 322 323 public boolean hasComorbidity() { 324 if (this.comorbidity == null) 325 return false; 326 for (CodeableReference item : this.comorbidity) 327 if (!item.isEmpty()) 328 return true; 329 return false; 330 } 331 332 public CodeableReference addComorbidity() { //3 333 CodeableReference t = new CodeableReference(); 334 if (this.comorbidity == null) 335 this.comorbidity = new ArrayList<CodeableReference>(); 336 this.comorbidity.add(t); 337 return t; 338 } 339 340 public ClinicalUseDefinitionContraindicationComponent addComorbidity(CodeableReference t) { //3 341 if (t == null) 342 return this; 343 if (this.comorbidity == null) 344 this.comorbidity = new ArrayList<CodeableReference>(); 345 this.comorbidity.add(t); 346 return this; 347 } 348 349 /** 350 * @return The first repetition of repeating field {@link #comorbidity}, creating it if it does not already exist {3} 351 */ 352 public CodeableReference getComorbidityFirstRep() { 353 if (getComorbidity().isEmpty()) { 354 addComorbidity(); 355 } 356 return getComorbidity().get(0); 357 } 358 359 /** 360 * @return {@link #indication} (The indication which this is a contraidication for.) 361 */ 362 public List<Reference> getIndication() { 363 if (this.indication == null) 364 this.indication = new ArrayList<Reference>(); 365 return this.indication; 366 } 367 368 /** 369 * @return Returns a reference to <code>this</code> for easy method chaining 370 */ 371 public ClinicalUseDefinitionContraindicationComponent setIndication(List<Reference> theIndication) { 372 this.indication = theIndication; 373 return this; 374 } 375 376 public boolean hasIndication() { 377 if (this.indication == null) 378 return false; 379 for (Reference item : this.indication) 380 if (!item.isEmpty()) 381 return true; 382 return false; 383 } 384 385 public Reference addIndication() { //3 386 Reference t = new Reference(); 387 if (this.indication == null) 388 this.indication = new ArrayList<Reference>(); 389 this.indication.add(t); 390 return t; 391 } 392 393 public ClinicalUseDefinitionContraindicationComponent addIndication(Reference t) { //3 394 if (t == null) 395 return this; 396 if (this.indication == null) 397 this.indication = new ArrayList<Reference>(); 398 this.indication.add(t); 399 return this; 400 } 401 402 /** 403 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist {3} 404 */ 405 public Reference getIndicationFirstRep() { 406 if (getIndication().isEmpty()) { 407 addIndication(); 408 } 409 return getIndication().get(0); 410 } 411 412 /** 413 * @return {@link #applicability} (An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.) 414 */ 415 public Expression getApplicability() { 416 if (this.applicability == null) 417 if (Configuration.errorOnAutoCreate()) 418 throw new Error("Attempt to auto-create ClinicalUseDefinitionContraindicationComponent.applicability"); 419 else if (Configuration.doAutoCreate()) 420 this.applicability = new Expression(); // cc 421 return this.applicability; 422 } 423 424 public boolean hasApplicability() { 425 return this.applicability != null && !this.applicability.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #applicability} (An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.) 430 */ 431 public ClinicalUseDefinitionContraindicationComponent setApplicability(Expression value) { 432 this.applicability = value; 433 return this; 434 } 435 436 /** 437 * @return {@link #otherTherapy} (Information about the use of the medicinal product in relation to other therapies described as part of the contraindication.) 438 */ 439 public List<ClinicalUseDefinitionContraindicationOtherTherapyComponent> getOtherTherapy() { 440 if (this.otherTherapy == null) 441 this.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 442 return this.otherTherapy; 443 } 444 445 /** 446 * @return Returns a reference to <code>this</code> for easy method chaining 447 */ 448 public ClinicalUseDefinitionContraindicationComponent setOtherTherapy(List<ClinicalUseDefinitionContraindicationOtherTherapyComponent> theOtherTherapy) { 449 this.otherTherapy = theOtherTherapy; 450 return this; 451 } 452 453 public boolean hasOtherTherapy() { 454 if (this.otherTherapy == null) 455 return false; 456 for (ClinicalUseDefinitionContraindicationOtherTherapyComponent item : this.otherTherapy) 457 if (!item.isEmpty()) 458 return true; 459 return false; 460 } 461 462 public ClinicalUseDefinitionContraindicationOtherTherapyComponent addOtherTherapy() { //3 463 ClinicalUseDefinitionContraindicationOtherTherapyComponent t = new ClinicalUseDefinitionContraindicationOtherTherapyComponent(); 464 if (this.otherTherapy == null) 465 this.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 466 this.otherTherapy.add(t); 467 return t; 468 } 469 470 public ClinicalUseDefinitionContraindicationComponent addOtherTherapy(ClinicalUseDefinitionContraindicationOtherTherapyComponent t) { //3 471 if (t == null) 472 return this; 473 if (this.otherTherapy == null) 474 this.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 475 this.otherTherapy.add(t); 476 return this; 477 } 478 479 /** 480 * @return The first repetition of repeating field {@link #otherTherapy}, creating it if it does not already exist {3} 481 */ 482 public ClinicalUseDefinitionContraindicationOtherTherapyComponent getOtherTherapyFirstRep() { 483 if (getOtherTherapy().isEmpty()) { 484 addOtherTherapy(); 485 } 486 return getOtherTherapy().get(0); 487 } 488 489 protected void listChildren(List<Property> children) { 490 super.listChildren(children); 491 children.add(new Property("diseaseSymptomProcedure", "CodeableReference(ObservationDefinition)", "The situation that is being documented as contraindicating against this item.", 0, 1, diseaseSymptomProcedure)); 492 children.add(new Property("diseaseStatus", "CodeableReference(ObservationDefinition)", "The status of the disease or symptom for the contraindication, for example \"chronic\" or \"metastatic\".", 0, 1, diseaseStatus)); 493 children.add(new Property("comorbidity", "CodeableReference(ObservationDefinition)", "A comorbidity (concurrent condition) or coinfection.", 0, java.lang.Integer.MAX_VALUE, comorbidity)); 494 children.add(new Property("indication", "Reference(ClinicalUseDefinition)", "The indication which this is a contraidication for.", 0, java.lang.Integer.MAX_VALUE, indication)); 495 children.add(new Property("applicability", "Expression", "An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.", 0, 1, applicability)); 496 children.add(new Property("otherTherapy", "", "Information about the use of the medicinal product in relation to other therapies described as part of the contraindication.", 0, java.lang.Integer.MAX_VALUE, otherTherapy)); 497 } 498 499 @Override 500 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 501 switch (_hash) { 502 case -1497395130: /*diseaseSymptomProcedure*/ return new Property("diseaseSymptomProcedure", "CodeableReference(ObservationDefinition)", "The situation that is being documented as contraindicating against this item.", 0, 1, diseaseSymptomProcedure); 503 case -505503602: /*diseaseStatus*/ return new Property("diseaseStatus", "CodeableReference(ObservationDefinition)", "The status of the disease or symptom for the contraindication, for example \"chronic\" or \"metastatic\".", 0, 1, diseaseStatus); 504 case -406395211: /*comorbidity*/ return new Property("comorbidity", "CodeableReference(ObservationDefinition)", "A comorbidity (concurrent condition) or coinfection.", 0, java.lang.Integer.MAX_VALUE, comorbidity); 505 case -597168804: /*indication*/ return new Property("indication", "Reference(ClinicalUseDefinition)", "The indication which this is a contraidication for.", 0, java.lang.Integer.MAX_VALUE, indication); 506 case -1526770491: /*applicability*/ return new Property("applicability", "Expression", "An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.", 0, 1, applicability); 507 case -544509127: /*otherTherapy*/ return new Property("otherTherapy", "", "Information about the use of the medicinal product in relation to other therapies described as part of the contraindication.", 0, java.lang.Integer.MAX_VALUE, otherTherapy); 508 default: return super.getNamedProperty(_hash, _name, _checkValid); 509 } 510 511 } 512 513 @Override 514 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 515 switch (hash) { 516 case -1497395130: /*diseaseSymptomProcedure*/ return this.diseaseSymptomProcedure == null ? new Base[0] : new Base[] {this.diseaseSymptomProcedure}; // CodeableReference 517 case -505503602: /*diseaseStatus*/ return this.diseaseStatus == null ? new Base[0] : new Base[] {this.diseaseStatus}; // CodeableReference 518 case -406395211: /*comorbidity*/ return this.comorbidity == null ? new Base[0] : this.comorbidity.toArray(new Base[this.comorbidity.size()]); // CodeableReference 519 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // Reference 520 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : new Base[] {this.applicability}; // Expression 521 case -544509127: /*otherTherapy*/ return this.otherTherapy == null ? new Base[0] : this.otherTherapy.toArray(new Base[this.otherTherapy.size()]); // ClinicalUseDefinitionContraindicationOtherTherapyComponent 522 default: return super.getProperty(hash, name, checkValid); 523 } 524 525 } 526 527 @Override 528 public Base setProperty(int hash, String name, Base value) throws FHIRException { 529 switch (hash) { 530 case -1497395130: // diseaseSymptomProcedure 531 this.diseaseSymptomProcedure = TypeConvertor.castToCodeableReference(value); // CodeableReference 532 return value; 533 case -505503602: // diseaseStatus 534 this.diseaseStatus = TypeConvertor.castToCodeableReference(value); // CodeableReference 535 return value; 536 case -406395211: // comorbidity 537 this.getComorbidity().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 538 return value; 539 case -597168804: // indication 540 this.getIndication().add(TypeConvertor.castToReference(value)); // Reference 541 return value; 542 case -1526770491: // applicability 543 this.applicability = TypeConvertor.castToExpression(value); // Expression 544 return value; 545 case -544509127: // otherTherapy 546 this.getOtherTherapy().add((ClinicalUseDefinitionContraindicationOtherTherapyComponent) value); // ClinicalUseDefinitionContraindicationOtherTherapyComponent 547 return value; 548 default: return super.setProperty(hash, name, value); 549 } 550 551 } 552 553 @Override 554 public Base setProperty(String name, Base value) throws FHIRException { 555 if (name.equals("diseaseSymptomProcedure")) { 556 this.diseaseSymptomProcedure = TypeConvertor.castToCodeableReference(value); // CodeableReference 557 } else if (name.equals("diseaseStatus")) { 558 this.diseaseStatus = TypeConvertor.castToCodeableReference(value); // CodeableReference 559 } else if (name.equals("comorbidity")) { 560 this.getComorbidity().add(TypeConvertor.castToCodeableReference(value)); 561 } else if (name.equals("indication")) { 562 this.getIndication().add(TypeConvertor.castToReference(value)); 563 } else if (name.equals("applicability")) { 564 this.applicability = TypeConvertor.castToExpression(value); // Expression 565 } else if (name.equals("otherTherapy")) { 566 this.getOtherTherapy().add((ClinicalUseDefinitionContraindicationOtherTherapyComponent) value); 567 } else 568 return super.setProperty(name, value); 569 return value; 570 } 571 572 @Override 573 public void removeChild(String name, Base value) throws FHIRException { 574 if (name.equals("diseaseSymptomProcedure")) { 575 this.diseaseSymptomProcedure = null; 576 } else if (name.equals("diseaseStatus")) { 577 this.diseaseStatus = null; 578 } else if (name.equals("comorbidity")) { 579 this.getComorbidity().remove(value); 580 } else if (name.equals("indication")) { 581 this.getIndication().remove(value); 582 } else if (name.equals("applicability")) { 583 this.applicability = null; 584 } else if (name.equals("otherTherapy")) { 585 this.getOtherTherapy().remove((ClinicalUseDefinitionContraindicationOtherTherapyComponent) value); 586 } else 587 super.removeChild(name, value); 588 589 } 590 591 @Override 592 public Base makeProperty(int hash, String name) throws FHIRException { 593 switch (hash) { 594 case -1497395130: return getDiseaseSymptomProcedure(); 595 case -505503602: return getDiseaseStatus(); 596 case -406395211: return addComorbidity(); 597 case -597168804: return addIndication(); 598 case -1526770491: return getApplicability(); 599 case -544509127: return addOtherTherapy(); 600 default: return super.makeProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 607 switch (hash) { 608 case -1497395130: /*diseaseSymptomProcedure*/ return new String[] {"CodeableReference"}; 609 case -505503602: /*diseaseStatus*/ return new String[] {"CodeableReference"}; 610 case -406395211: /*comorbidity*/ return new String[] {"CodeableReference"}; 611 case -597168804: /*indication*/ return new String[] {"Reference"}; 612 case -1526770491: /*applicability*/ return new String[] {"Expression"}; 613 case -544509127: /*otherTherapy*/ return new String[] {}; 614 default: return super.getTypesForProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public Base addChild(String name) throws FHIRException { 621 if (name.equals("diseaseSymptomProcedure")) { 622 this.diseaseSymptomProcedure = new CodeableReference(); 623 return this.diseaseSymptomProcedure; 624 } 625 else if (name.equals("diseaseStatus")) { 626 this.diseaseStatus = new CodeableReference(); 627 return this.diseaseStatus; 628 } 629 else if (name.equals("comorbidity")) { 630 return addComorbidity(); 631 } 632 else if (name.equals("indication")) { 633 return addIndication(); 634 } 635 else if (name.equals("applicability")) { 636 this.applicability = new Expression(); 637 return this.applicability; 638 } 639 else if (name.equals("otherTherapy")) { 640 return addOtherTherapy(); 641 } 642 else 643 return super.addChild(name); 644 } 645 646 public ClinicalUseDefinitionContraindicationComponent copy() { 647 ClinicalUseDefinitionContraindicationComponent dst = new ClinicalUseDefinitionContraindicationComponent(); 648 copyValues(dst); 649 return dst; 650 } 651 652 public void copyValues(ClinicalUseDefinitionContraindicationComponent dst) { 653 super.copyValues(dst); 654 dst.diseaseSymptomProcedure = diseaseSymptomProcedure == null ? null : diseaseSymptomProcedure.copy(); 655 dst.diseaseStatus = diseaseStatus == null ? null : diseaseStatus.copy(); 656 if (comorbidity != null) { 657 dst.comorbidity = new ArrayList<CodeableReference>(); 658 for (CodeableReference i : comorbidity) 659 dst.comorbidity.add(i.copy()); 660 }; 661 if (indication != null) { 662 dst.indication = new ArrayList<Reference>(); 663 for (Reference i : indication) 664 dst.indication.add(i.copy()); 665 }; 666 dst.applicability = applicability == null ? null : applicability.copy(); 667 if (otherTherapy != null) { 668 dst.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 669 for (ClinicalUseDefinitionContraindicationOtherTherapyComponent i : otherTherapy) 670 dst.otherTherapy.add(i.copy()); 671 }; 672 } 673 674 @Override 675 public boolean equalsDeep(Base other_) { 676 if (!super.equalsDeep(other_)) 677 return false; 678 if (!(other_ instanceof ClinicalUseDefinitionContraindicationComponent)) 679 return false; 680 ClinicalUseDefinitionContraindicationComponent o = (ClinicalUseDefinitionContraindicationComponent) other_; 681 return compareDeep(diseaseSymptomProcedure, o.diseaseSymptomProcedure, true) && compareDeep(diseaseStatus, o.diseaseStatus, true) 682 && compareDeep(comorbidity, o.comorbidity, true) && compareDeep(indication, o.indication, true) 683 && compareDeep(applicability, o.applicability, true) && compareDeep(otherTherapy, o.otherTherapy, true) 684 ; 685 } 686 687 @Override 688 public boolean equalsShallow(Base other_) { 689 if (!super.equalsShallow(other_)) 690 return false; 691 if (!(other_ instanceof ClinicalUseDefinitionContraindicationComponent)) 692 return false; 693 ClinicalUseDefinitionContraindicationComponent o = (ClinicalUseDefinitionContraindicationComponent) other_; 694 return true; 695 } 696 697 public boolean isEmpty() { 698 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(diseaseSymptomProcedure, diseaseStatus 699 , comorbidity, indication, applicability, otherTherapy); 700 } 701 702 public String fhirType() { 703 return "ClinicalUseDefinition.contraindication"; 704 705 } 706 707 } 708 709 @Block() 710 public static class ClinicalUseDefinitionContraindicationOtherTherapyComponent extends BackboneElement implements IBaseBackboneElement { 711 /** 712 * The type of relationship between the medicinal product indication or contraindication and another therapy. 713 */ 714 @Child(name = "relationshipType", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 715 @Description(shortDefinition="The type of relationship between the product indication/contraindication and another therapy", formalDefinition="The type of relationship between the medicinal product indication or contraindication and another therapy." ) 716 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/therapy-relationship-type") 717 protected CodeableConcept relationshipType; 718 719 /** 720 * Reference to a specific medication (active substance, medicinal product or class of products, biological, food etc.) as part of an indication or contraindication. 721 */ 722 @Child(name = "treatment", type = {CodeableReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 723 @Description(shortDefinition="Reference to a specific medication, substance etc. as part of an indication or contraindication", formalDefinition="Reference to a specific medication (active substance, medicinal product or class of products, biological, food etc.) as part of an indication or contraindication." ) 724 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/therapy") 725 protected CodeableReference treatment; 726 727 private static final long serialVersionUID = -1638121853L; 728 729 /** 730 * Constructor 731 */ 732 public ClinicalUseDefinitionContraindicationOtherTherapyComponent() { 733 super(); 734 } 735 736 /** 737 * Constructor 738 */ 739 public ClinicalUseDefinitionContraindicationOtherTherapyComponent(CodeableConcept relationshipType, CodeableReference treatment) { 740 super(); 741 this.setRelationshipType(relationshipType); 742 this.setTreatment(treatment); 743 } 744 745 /** 746 * @return {@link #relationshipType} (The type of relationship between the medicinal product indication or contraindication and another therapy.) 747 */ 748 public CodeableConcept getRelationshipType() { 749 if (this.relationshipType == null) 750 if (Configuration.errorOnAutoCreate()) 751 throw new Error("Attempt to auto-create ClinicalUseDefinitionContraindicationOtherTherapyComponent.relationshipType"); 752 else if (Configuration.doAutoCreate()) 753 this.relationshipType = new CodeableConcept(); // cc 754 return this.relationshipType; 755 } 756 757 public boolean hasRelationshipType() { 758 return this.relationshipType != null && !this.relationshipType.isEmpty(); 759 } 760 761 /** 762 * @param value {@link #relationshipType} (The type of relationship between the medicinal product indication or contraindication and another therapy.) 763 */ 764 public ClinicalUseDefinitionContraindicationOtherTherapyComponent setRelationshipType(CodeableConcept value) { 765 this.relationshipType = value; 766 return this; 767 } 768 769 /** 770 * @return {@link #treatment} (Reference to a specific medication (active substance, medicinal product or class of products, biological, food etc.) as part of an indication or contraindication.) 771 */ 772 public CodeableReference getTreatment() { 773 if (this.treatment == null) 774 if (Configuration.errorOnAutoCreate()) 775 throw new Error("Attempt to auto-create ClinicalUseDefinitionContraindicationOtherTherapyComponent.treatment"); 776 else if (Configuration.doAutoCreate()) 777 this.treatment = new CodeableReference(); // cc 778 return this.treatment; 779 } 780 781 public boolean hasTreatment() { 782 return this.treatment != null && !this.treatment.isEmpty(); 783 } 784 785 /** 786 * @param value {@link #treatment} (Reference to a specific medication (active substance, medicinal product or class of products, biological, food etc.) as part of an indication or contraindication.) 787 */ 788 public ClinicalUseDefinitionContraindicationOtherTherapyComponent setTreatment(CodeableReference value) { 789 this.treatment = value; 790 return this; 791 } 792 793 protected void listChildren(List<Property> children) { 794 super.listChildren(children); 795 children.add(new Property("relationshipType", "CodeableConcept", "The type of relationship between the medicinal product indication or contraindication and another therapy.", 0, 1, relationshipType)); 796 children.add(new Property("treatment", "CodeableReference(MedicinalProductDefinition|Medication|Substance|SubstanceDefinition|NutritionProduct|BiologicallyDerivedProduct|ActivityDefinition)", "Reference to a specific medication (active substance, medicinal product or class of products, biological, food etc.) as part of an indication or contraindication.", 0, 1, treatment)); 797 } 798 799 @Override 800 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 801 switch (_hash) { 802 case -1602839150: /*relationshipType*/ return new Property("relationshipType", "CodeableConcept", "The type of relationship between the medicinal product indication or contraindication and another therapy.", 0, 1, relationshipType); 803 case -63342472: /*treatment*/ return new Property("treatment", "CodeableReference(MedicinalProductDefinition|Medication|Substance|SubstanceDefinition|NutritionProduct|BiologicallyDerivedProduct|ActivityDefinition)", "Reference to a specific medication (active substance, medicinal product or class of products, biological, food etc.) as part of an indication or contraindication.", 0, 1, treatment); 804 default: return super.getNamedProperty(_hash, _name, _checkValid); 805 } 806 807 } 808 809 @Override 810 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 811 switch (hash) { 812 case -1602839150: /*relationshipType*/ return this.relationshipType == null ? new Base[0] : new Base[] {this.relationshipType}; // CodeableConcept 813 case -63342472: /*treatment*/ return this.treatment == null ? new Base[0] : new Base[] {this.treatment}; // CodeableReference 814 default: return super.getProperty(hash, name, checkValid); 815 } 816 817 } 818 819 @Override 820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 821 switch (hash) { 822 case -1602839150: // relationshipType 823 this.relationshipType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 824 return value; 825 case -63342472: // treatment 826 this.treatment = TypeConvertor.castToCodeableReference(value); // CodeableReference 827 return value; 828 default: return super.setProperty(hash, name, value); 829 } 830 831 } 832 833 @Override 834 public Base setProperty(String name, Base value) throws FHIRException { 835 if (name.equals("relationshipType")) { 836 this.relationshipType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 837 } else if (name.equals("treatment")) { 838 this.treatment = TypeConvertor.castToCodeableReference(value); // CodeableReference 839 } else 840 return super.setProperty(name, value); 841 return value; 842 } 843 844 @Override 845 public void removeChild(String name, Base value) throws FHIRException { 846 if (name.equals("relationshipType")) { 847 this.relationshipType = null; 848 } else if (name.equals("treatment")) { 849 this.treatment = null; 850 } else 851 super.removeChild(name, value); 852 853 } 854 855 @Override 856 public Base makeProperty(int hash, String name) throws FHIRException { 857 switch (hash) { 858 case -1602839150: return getRelationshipType(); 859 case -63342472: return getTreatment(); 860 default: return super.makeProperty(hash, name); 861 } 862 863 } 864 865 @Override 866 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 867 switch (hash) { 868 case -1602839150: /*relationshipType*/ return new String[] {"CodeableConcept"}; 869 case -63342472: /*treatment*/ return new String[] {"CodeableReference"}; 870 default: return super.getTypesForProperty(hash, name); 871 } 872 873 } 874 875 @Override 876 public Base addChild(String name) throws FHIRException { 877 if (name.equals("relationshipType")) { 878 this.relationshipType = new CodeableConcept(); 879 return this.relationshipType; 880 } 881 else if (name.equals("treatment")) { 882 this.treatment = new CodeableReference(); 883 return this.treatment; 884 } 885 else 886 return super.addChild(name); 887 } 888 889 public ClinicalUseDefinitionContraindicationOtherTherapyComponent copy() { 890 ClinicalUseDefinitionContraindicationOtherTherapyComponent dst = new ClinicalUseDefinitionContraindicationOtherTherapyComponent(); 891 copyValues(dst); 892 return dst; 893 } 894 895 public void copyValues(ClinicalUseDefinitionContraindicationOtherTherapyComponent dst) { 896 super.copyValues(dst); 897 dst.relationshipType = relationshipType == null ? null : relationshipType.copy(); 898 dst.treatment = treatment == null ? null : treatment.copy(); 899 } 900 901 @Override 902 public boolean equalsDeep(Base other_) { 903 if (!super.equalsDeep(other_)) 904 return false; 905 if (!(other_ instanceof ClinicalUseDefinitionContraindicationOtherTherapyComponent)) 906 return false; 907 ClinicalUseDefinitionContraindicationOtherTherapyComponent o = (ClinicalUseDefinitionContraindicationOtherTherapyComponent) other_; 908 return compareDeep(relationshipType, o.relationshipType, true) && compareDeep(treatment, o.treatment, true) 909 ; 910 } 911 912 @Override 913 public boolean equalsShallow(Base other_) { 914 if (!super.equalsShallow(other_)) 915 return false; 916 if (!(other_ instanceof ClinicalUseDefinitionContraindicationOtherTherapyComponent)) 917 return false; 918 ClinicalUseDefinitionContraindicationOtherTherapyComponent o = (ClinicalUseDefinitionContraindicationOtherTherapyComponent) other_; 919 return true; 920 } 921 922 public boolean isEmpty() { 923 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relationshipType, treatment 924 ); 925 } 926 927 public String fhirType() { 928 return "ClinicalUseDefinition.contraindication.otherTherapy"; 929 930 } 931 932 } 933 934 @Block() 935 public static class ClinicalUseDefinitionIndicationComponent extends BackboneElement implements IBaseBackboneElement { 936 /** 937 * The situation that is being documented as an indicaton for this item. 938 */ 939 @Child(name = "diseaseSymptomProcedure", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 940 @Description(shortDefinition="The situation that is being documented as an indicaton for this item", formalDefinition="The situation that is being documented as an indicaton for this item." ) 941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/disease-symptom-procedure") 942 protected CodeableReference diseaseSymptomProcedure; 943 944 /** 945 * The status of the disease or symptom for the indication, for example "chronic" or "metastatic". 946 */ 947 @Child(name = "diseaseStatus", type = {CodeableReference.class}, order=2, min=0, max=1, modifier=false, summary=true) 948 @Description(shortDefinition="The status of the disease or symptom for the indication", formalDefinition="The status of the disease or symptom for the indication, for example \"chronic\" or \"metastatic\"." ) 949 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/disease-status") 950 protected CodeableReference diseaseStatus; 951 952 /** 953 * A comorbidity (concurrent condition) or coinfection as part of the indication. 954 */ 955 @Child(name = "comorbidity", type = {CodeableReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 956 @Description(shortDefinition="A comorbidity or coinfection as part of the indication", formalDefinition="A comorbidity (concurrent condition) or coinfection as part of the indication." ) 957 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/disease-symptom-procedure") 958 protected List<CodeableReference> comorbidity; 959 960 /** 961 * The intended effect, aim or strategy to be achieved. 962 */ 963 @Child(name = "intendedEffect", type = {CodeableReference.class}, order=4, min=0, max=1, modifier=false, summary=true) 964 @Description(shortDefinition="The intended effect, aim or strategy to be achieved", formalDefinition="The intended effect, aim or strategy to be achieved." ) 965 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-intended-use") 966 protected CodeableReference intendedEffect; 967 968 /** 969 * Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months). 970 */ 971 @Child(name = "duration", type = {Range.class, StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 972 @Description(shortDefinition="Timing or duration information", formalDefinition="Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months)." ) 973 protected DataType duration; 974 975 /** 976 * An unwanted side effect or negative outcome that may happen if you use the drug (or other subject of this resource) for this indication. 977 */ 978 @Child(name = "undesirableEffect", type = {ClinicalUseDefinition.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 979 @Description(shortDefinition="An unwanted side effect or negative outcome of the subject of this resource when being used for this indication", formalDefinition="An unwanted side effect or negative outcome that may happen if you use the drug (or other subject of this resource) for this indication." ) 980 protected List<Reference> undesirableEffect; 981 982 /** 983 * An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements. 984 */ 985 @Child(name = "applicability", type = {Expression.class}, order=7, min=0, max=1, modifier=false, summary=false) 986 @Description(shortDefinition="An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements", formalDefinition="An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements." ) 987 protected Expression applicability; 988 989 /** 990 * Information about the use of the medicinal product in relation to other therapies described as part of the indication. 991 */ 992 @Child(name = "otherTherapy", type = {ClinicalUseDefinitionContraindicationOtherTherapyComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 993 @Description(shortDefinition="The use of the medicinal product in relation to other therapies described as part of the indication", formalDefinition="Information about the use of the medicinal product in relation to other therapies described as part of the indication." ) 994 protected List<ClinicalUseDefinitionContraindicationOtherTherapyComponent> otherTherapy; 995 996 private static final long serialVersionUID = 809598459L; 997 998 /** 999 * Constructor 1000 */ 1001 public ClinicalUseDefinitionIndicationComponent() { 1002 super(); 1003 } 1004 1005 /** 1006 * @return {@link #diseaseSymptomProcedure} (The situation that is being documented as an indicaton for this item.) 1007 */ 1008 public CodeableReference getDiseaseSymptomProcedure() { 1009 if (this.diseaseSymptomProcedure == null) 1010 if (Configuration.errorOnAutoCreate()) 1011 throw new Error("Attempt to auto-create ClinicalUseDefinitionIndicationComponent.diseaseSymptomProcedure"); 1012 else if (Configuration.doAutoCreate()) 1013 this.diseaseSymptomProcedure = new CodeableReference(); // cc 1014 return this.diseaseSymptomProcedure; 1015 } 1016 1017 public boolean hasDiseaseSymptomProcedure() { 1018 return this.diseaseSymptomProcedure != null && !this.diseaseSymptomProcedure.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #diseaseSymptomProcedure} (The situation that is being documented as an indicaton for this item.) 1023 */ 1024 public ClinicalUseDefinitionIndicationComponent setDiseaseSymptomProcedure(CodeableReference value) { 1025 this.diseaseSymptomProcedure = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return {@link #diseaseStatus} (The status of the disease or symptom for the indication, for example "chronic" or "metastatic".) 1031 */ 1032 public CodeableReference getDiseaseStatus() { 1033 if (this.diseaseStatus == null) 1034 if (Configuration.errorOnAutoCreate()) 1035 throw new Error("Attempt to auto-create ClinicalUseDefinitionIndicationComponent.diseaseStatus"); 1036 else if (Configuration.doAutoCreate()) 1037 this.diseaseStatus = new CodeableReference(); // cc 1038 return this.diseaseStatus; 1039 } 1040 1041 public boolean hasDiseaseStatus() { 1042 return this.diseaseStatus != null && !this.diseaseStatus.isEmpty(); 1043 } 1044 1045 /** 1046 * @param value {@link #diseaseStatus} (The status of the disease or symptom for the indication, for example "chronic" or "metastatic".) 1047 */ 1048 public ClinicalUseDefinitionIndicationComponent setDiseaseStatus(CodeableReference value) { 1049 this.diseaseStatus = value; 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #comorbidity} (A comorbidity (concurrent condition) or coinfection as part of the indication.) 1055 */ 1056 public List<CodeableReference> getComorbidity() { 1057 if (this.comorbidity == null) 1058 this.comorbidity = new ArrayList<CodeableReference>(); 1059 return this.comorbidity; 1060 } 1061 1062 /** 1063 * @return Returns a reference to <code>this</code> for easy method chaining 1064 */ 1065 public ClinicalUseDefinitionIndicationComponent setComorbidity(List<CodeableReference> theComorbidity) { 1066 this.comorbidity = theComorbidity; 1067 return this; 1068 } 1069 1070 public boolean hasComorbidity() { 1071 if (this.comorbidity == null) 1072 return false; 1073 for (CodeableReference item : this.comorbidity) 1074 if (!item.isEmpty()) 1075 return true; 1076 return false; 1077 } 1078 1079 public CodeableReference addComorbidity() { //3 1080 CodeableReference t = new CodeableReference(); 1081 if (this.comorbidity == null) 1082 this.comorbidity = new ArrayList<CodeableReference>(); 1083 this.comorbidity.add(t); 1084 return t; 1085 } 1086 1087 public ClinicalUseDefinitionIndicationComponent addComorbidity(CodeableReference t) { //3 1088 if (t == null) 1089 return this; 1090 if (this.comorbidity == null) 1091 this.comorbidity = new ArrayList<CodeableReference>(); 1092 this.comorbidity.add(t); 1093 return this; 1094 } 1095 1096 /** 1097 * @return The first repetition of repeating field {@link #comorbidity}, creating it if it does not already exist {3} 1098 */ 1099 public CodeableReference getComorbidityFirstRep() { 1100 if (getComorbidity().isEmpty()) { 1101 addComorbidity(); 1102 } 1103 return getComorbidity().get(0); 1104 } 1105 1106 /** 1107 * @return {@link #intendedEffect} (The intended effect, aim or strategy to be achieved.) 1108 */ 1109 public CodeableReference getIntendedEffect() { 1110 if (this.intendedEffect == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create ClinicalUseDefinitionIndicationComponent.intendedEffect"); 1113 else if (Configuration.doAutoCreate()) 1114 this.intendedEffect = new CodeableReference(); // cc 1115 return this.intendedEffect; 1116 } 1117 1118 public boolean hasIntendedEffect() { 1119 return this.intendedEffect != null && !this.intendedEffect.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #intendedEffect} (The intended effect, aim or strategy to be achieved.) 1124 */ 1125 public ClinicalUseDefinitionIndicationComponent setIntendedEffect(CodeableReference value) { 1126 this.intendedEffect = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #duration} (Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).) 1132 */ 1133 public DataType getDuration() { 1134 return this.duration; 1135 } 1136 1137 /** 1138 * @return {@link #duration} (Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).) 1139 */ 1140 public Range getDurationRange() throws FHIRException { 1141 if (this.duration == null) 1142 this.duration = new Range(); 1143 if (!(this.duration instanceof Range)) 1144 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.duration.getClass().getName()+" was encountered"); 1145 return (Range) this.duration; 1146 } 1147 1148 public boolean hasDurationRange() { 1149 return this.duration instanceof Range; 1150 } 1151 1152 /** 1153 * @return {@link #duration} (Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).) 1154 */ 1155 public StringType getDurationStringType() throws FHIRException { 1156 if (this.duration == null) 1157 this.duration = new StringType(); 1158 if (!(this.duration instanceof StringType)) 1159 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.duration.getClass().getName()+" was encountered"); 1160 return (StringType) this.duration; 1161 } 1162 1163 public boolean hasDurationStringType() { 1164 return this.duration instanceof StringType; 1165 } 1166 1167 public boolean hasDuration() { 1168 return this.duration != null && !this.duration.isEmpty(); 1169 } 1170 1171 /** 1172 * @param value {@link #duration} (Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).) 1173 */ 1174 public ClinicalUseDefinitionIndicationComponent setDuration(DataType value) { 1175 if (value != null && !(value instanceof Range || value instanceof StringType)) 1176 throw new FHIRException("Not the right type for ClinicalUseDefinition.indication.duration[x]: "+value.fhirType()); 1177 this.duration = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return {@link #undesirableEffect} (An unwanted side effect or negative outcome that may happen if you use the drug (or other subject of this resource) for this indication.) 1183 */ 1184 public List<Reference> getUndesirableEffect() { 1185 if (this.undesirableEffect == null) 1186 this.undesirableEffect = new ArrayList<Reference>(); 1187 return this.undesirableEffect; 1188 } 1189 1190 /** 1191 * @return Returns a reference to <code>this</code> for easy method chaining 1192 */ 1193 public ClinicalUseDefinitionIndicationComponent setUndesirableEffect(List<Reference> theUndesirableEffect) { 1194 this.undesirableEffect = theUndesirableEffect; 1195 return this; 1196 } 1197 1198 public boolean hasUndesirableEffect() { 1199 if (this.undesirableEffect == null) 1200 return false; 1201 for (Reference item : this.undesirableEffect) 1202 if (!item.isEmpty()) 1203 return true; 1204 return false; 1205 } 1206 1207 public Reference addUndesirableEffect() { //3 1208 Reference t = new Reference(); 1209 if (this.undesirableEffect == null) 1210 this.undesirableEffect = new ArrayList<Reference>(); 1211 this.undesirableEffect.add(t); 1212 return t; 1213 } 1214 1215 public ClinicalUseDefinitionIndicationComponent addUndesirableEffect(Reference t) { //3 1216 if (t == null) 1217 return this; 1218 if (this.undesirableEffect == null) 1219 this.undesirableEffect = new ArrayList<Reference>(); 1220 this.undesirableEffect.add(t); 1221 return this; 1222 } 1223 1224 /** 1225 * @return The first repetition of repeating field {@link #undesirableEffect}, creating it if it does not already exist {3} 1226 */ 1227 public Reference getUndesirableEffectFirstRep() { 1228 if (getUndesirableEffect().isEmpty()) { 1229 addUndesirableEffect(); 1230 } 1231 return getUndesirableEffect().get(0); 1232 } 1233 1234 /** 1235 * @return {@link #applicability} (An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.) 1236 */ 1237 public Expression getApplicability() { 1238 if (this.applicability == null) 1239 if (Configuration.errorOnAutoCreate()) 1240 throw new Error("Attempt to auto-create ClinicalUseDefinitionIndicationComponent.applicability"); 1241 else if (Configuration.doAutoCreate()) 1242 this.applicability = new Expression(); // cc 1243 return this.applicability; 1244 } 1245 1246 public boolean hasApplicability() { 1247 return this.applicability != null && !this.applicability.isEmpty(); 1248 } 1249 1250 /** 1251 * @param value {@link #applicability} (An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.) 1252 */ 1253 public ClinicalUseDefinitionIndicationComponent setApplicability(Expression value) { 1254 this.applicability = value; 1255 return this; 1256 } 1257 1258 /** 1259 * @return {@link #otherTherapy} (Information about the use of the medicinal product in relation to other therapies described as part of the indication.) 1260 */ 1261 public List<ClinicalUseDefinitionContraindicationOtherTherapyComponent> getOtherTherapy() { 1262 if (this.otherTherapy == null) 1263 this.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 1264 return this.otherTherapy; 1265 } 1266 1267 /** 1268 * @return Returns a reference to <code>this</code> for easy method chaining 1269 */ 1270 public ClinicalUseDefinitionIndicationComponent setOtherTherapy(List<ClinicalUseDefinitionContraindicationOtherTherapyComponent> theOtherTherapy) { 1271 this.otherTherapy = theOtherTherapy; 1272 return this; 1273 } 1274 1275 public boolean hasOtherTherapy() { 1276 if (this.otherTherapy == null) 1277 return false; 1278 for (ClinicalUseDefinitionContraindicationOtherTherapyComponent item : this.otherTherapy) 1279 if (!item.isEmpty()) 1280 return true; 1281 return false; 1282 } 1283 1284 public ClinicalUseDefinitionContraindicationOtherTherapyComponent addOtherTherapy() { //3 1285 ClinicalUseDefinitionContraindicationOtherTherapyComponent t = new ClinicalUseDefinitionContraindicationOtherTherapyComponent(); 1286 if (this.otherTherapy == null) 1287 this.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 1288 this.otherTherapy.add(t); 1289 return t; 1290 } 1291 1292 public ClinicalUseDefinitionIndicationComponent addOtherTherapy(ClinicalUseDefinitionContraindicationOtherTherapyComponent t) { //3 1293 if (t == null) 1294 return this; 1295 if (this.otherTherapy == null) 1296 this.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 1297 this.otherTherapy.add(t); 1298 return this; 1299 } 1300 1301 /** 1302 * @return The first repetition of repeating field {@link #otherTherapy}, creating it if it does not already exist {3} 1303 */ 1304 public ClinicalUseDefinitionContraindicationOtherTherapyComponent getOtherTherapyFirstRep() { 1305 if (getOtherTherapy().isEmpty()) { 1306 addOtherTherapy(); 1307 } 1308 return getOtherTherapy().get(0); 1309 } 1310 1311 protected void listChildren(List<Property> children) { 1312 super.listChildren(children); 1313 children.add(new Property("diseaseSymptomProcedure", "CodeableReference(ObservationDefinition)", "The situation that is being documented as an indicaton for this item.", 0, 1, diseaseSymptomProcedure)); 1314 children.add(new Property("diseaseStatus", "CodeableReference(ObservationDefinition)", "The status of the disease or symptom for the indication, for example \"chronic\" or \"metastatic\".", 0, 1, diseaseStatus)); 1315 children.add(new Property("comorbidity", "CodeableReference(ObservationDefinition)", "A comorbidity (concurrent condition) or coinfection as part of the indication.", 0, java.lang.Integer.MAX_VALUE, comorbidity)); 1316 children.add(new Property("intendedEffect", "CodeableReference(ObservationDefinition)", "The intended effect, aim or strategy to be achieved.", 0, 1, intendedEffect)); 1317 children.add(new Property("duration[x]", "Range|string", "Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).", 0, 1, duration)); 1318 children.add(new Property("undesirableEffect", "Reference(ClinicalUseDefinition)", "An unwanted side effect or negative outcome that may happen if you use the drug (or other subject of this resource) for this indication.", 0, java.lang.Integer.MAX_VALUE, undesirableEffect)); 1319 children.add(new Property("applicability", "Expression", "An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.", 0, 1, applicability)); 1320 children.add(new Property("otherTherapy", "@ClinicalUseDefinition.contraindication.otherTherapy", "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 0, java.lang.Integer.MAX_VALUE, otherTherapy)); 1321 } 1322 1323 @Override 1324 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1325 switch (_hash) { 1326 case -1497395130: /*diseaseSymptomProcedure*/ return new Property("diseaseSymptomProcedure", "CodeableReference(ObservationDefinition)", "The situation that is being documented as an indicaton for this item.", 0, 1, diseaseSymptomProcedure); 1327 case -505503602: /*diseaseStatus*/ return new Property("diseaseStatus", "CodeableReference(ObservationDefinition)", "The status of the disease or symptom for the indication, for example \"chronic\" or \"metastatic\".", 0, 1, diseaseStatus); 1328 case -406395211: /*comorbidity*/ return new Property("comorbidity", "CodeableReference(ObservationDefinition)", "A comorbidity (concurrent condition) or coinfection as part of the indication.", 0, java.lang.Integer.MAX_VALUE, comorbidity); 1329 case 1587112348: /*intendedEffect*/ return new Property("intendedEffect", "CodeableReference(ObservationDefinition)", "The intended effect, aim or strategy to be achieved.", 0, 1, intendedEffect); 1330 case -478069140: /*duration[x]*/ return new Property("duration[x]", "Range|string", "Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).", 0, 1, duration); 1331 case -1992012396: /*duration*/ return new Property("duration[x]", "Range|string", "Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).", 0, 1, duration); 1332 case 128079881: /*durationRange*/ return new Property("duration[x]", "Range", "Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).", 0, 1, duration); 1333 case -278193467: /*durationString*/ return new Property("duration[x]", "string", "Timing or duration information, that may be associated with use with the indicated condition e.g. Adult patients suffering from myocardial infarction (from a few days until less than 35 days), ischaemic stroke (from 7 days until less than 6 months).", 0, 1, duration); 1334 case 444367565: /*undesirableEffect*/ return new Property("undesirableEffect", "Reference(ClinicalUseDefinition)", "An unwanted side effect or negative outcome that may happen if you use the drug (or other subject of this resource) for this indication.", 0, java.lang.Integer.MAX_VALUE, undesirableEffect); 1335 case -1526770491: /*applicability*/ return new Property("applicability", "Expression", "An expression that returns true or false, indicating whether the indication is applicable or not, after having applied its other elements.", 0, 1, applicability); 1336 case -544509127: /*otherTherapy*/ return new Property("otherTherapy", "@ClinicalUseDefinition.contraindication.otherTherapy", "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 0, java.lang.Integer.MAX_VALUE, otherTherapy); 1337 default: return super.getNamedProperty(_hash, _name, _checkValid); 1338 } 1339 1340 } 1341 1342 @Override 1343 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1344 switch (hash) { 1345 case -1497395130: /*diseaseSymptomProcedure*/ return this.diseaseSymptomProcedure == null ? new Base[0] : new Base[] {this.diseaseSymptomProcedure}; // CodeableReference 1346 case -505503602: /*diseaseStatus*/ return this.diseaseStatus == null ? new Base[0] : new Base[] {this.diseaseStatus}; // CodeableReference 1347 case -406395211: /*comorbidity*/ return this.comorbidity == null ? new Base[0] : this.comorbidity.toArray(new Base[this.comorbidity.size()]); // CodeableReference 1348 case 1587112348: /*intendedEffect*/ return this.intendedEffect == null ? new Base[0] : new Base[] {this.intendedEffect}; // CodeableReference 1349 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // DataType 1350 case 444367565: /*undesirableEffect*/ return this.undesirableEffect == null ? new Base[0] : this.undesirableEffect.toArray(new Base[this.undesirableEffect.size()]); // Reference 1351 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : new Base[] {this.applicability}; // Expression 1352 case -544509127: /*otherTherapy*/ return this.otherTherapy == null ? new Base[0] : this.otherTherapy.toArray(new Base[this.otherTherapy.size()]); // ClinicalUseDefinitionContraindicationOtherTherapyComponent 1353 default: return super.getProperty(hash, name, checkValid); 1354 } 1355 1356 } 1357 1358 @Override 1359 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1360 switch (hash) { 1361 case -1497395130: // diseaseSymptomProcedure 1362 this.diseaseSymptomProcedure = TypeConvertor.castToCodeableReference(value); // CodeableReference 1363 return value; 1364 case -505503602: // diseaseStatus 1365 this.diseaseStatus = TypeConvertor.castToCodeableReference(value); // CodeableReference 1366 return value; 1367 case -406395211: // comorbidity 1368 this.getComorbidity().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1369 return value; 1370 case 1587112348: // intendedEffect 1371 this.intendedEffect = TypeConvertor.castToCodeableReference(value); // CodeableReference 1372 return value; 1373 case -1992012396: // duration 1374 this.duration = TypeConvertor.castToType(value); // DataType 1375 return value; 1376 case 444367565: // undesirableEffect 1377 this.getUndesirableEffect().add(TypeConvertor.castToReference(value)); // Reference 1378 return value; 1379 case -1526770491: // applicability 1380 this.applicability = TypeConvertor.castToExpression(value); // Expression 1381 return value; 1382 case -544509127: // otherTherapy 1383 this.getOtherTherapy().add((ClinicalUseDefinitionContraindicationOtherTherapyComponent) value); // ClinicalUseDefinitionContraindicationOtherTherapyComponent 1384 return value; 1385 default: return super.setProperty(hash, name, value); 1386 } 1387 1388 } 1389 1390 @Override 1391 public Base setProperty(String name, Base value) throws FHIRException { 1392 if (name.equals("diseaseSymptomProcedure")) { 1393 this.diseaseSymptomProcedure = TypeConvertor.castToCodeableReference(value); // CodeableReference 1394 } else if (name.equals("diseaseStatus")) { 1395 this.diseaseStatus = TypeConvertor.castToCodeableReference(value); // CodeableReference 1396 } else if (name.equals("comorbidity")) { 1397 this.getComorbidity().add(TypeConvertor.castToCodeableReference(value)); 1398 } else if (name.equals("intendedEffect")) { 1399 this.intendedEffect = TypeConvertor.castToCodeableReference(value); // CodeableReference 1400 } else if (name.equals("duration[x]")) { 1401 this.duration = TypeConvertor.castToType(value); // DataType 1402 } else if (name.equals("undesirableEffect")) { 1403 this.getUndesirableEffect().add(TypeConvertor.castToReference(value)); 1404 } else if (name.equals("applicability")) { 1405 this.applicability = TypeConvertor.castToExpression(value); // Expression 1406 } else if (name.equals("otherTherapy")) { 1407 this.getOtherTherapy().add((ClinicalUseDefinitionContraindicationOtherTherapyComponent) value); 1408 } else 1409 return super.setProperty(name, value); 1410 return value; 1411 } 1412 1413 @Override 1414 public void removeChild(String name, Base value) throws FHIRException { 1415 if (name.equals("diseaseSymptomProcedure")) { 1416 this.diseaseSymptomProcedure = null; 1417 } else if (name.equals("diseaseStatus")) { 1418 this.diseaseStatus = null; 1419 } else if (name.equals("comorbidity")) { 1420 this.getComorbidity().remove(value); 1421 } else if (name.equals("intendedEffect")) { 1422 this.intendedEffect = null; 1423 } else if (name.equals("duration[x]")) { 1424 this.duration = null; 1425 } else if (name.equals("undesirableEffect")) { 1426 this.getUndesirableEffect().remove(value); 1427 } else if (name.equals("applicability")) { 1428 this.applicability = null; 1429 } else if (name.equals("otherTherapy")) { 1430 this.getOtherTherapy().remove((ClinicalUseDefinitionContraindicationOtherTherapyComponent) value); 1431 } else 1432 super.removeChild(name, value); 1433 1434 } 1435 1436 @Override 1437 public Base makeProperty(int hash, String name) throws FHIRException { 1438 switch (hash) { 1439 case -1497395130: return getDiseaseSymptomProcedure(); 1440 case -505503602: return getDiseaseStatus(); 1441 case -406395211: return addComorbidity(); 1442 case 1587112348: return getIntendedEffect(); 1443 case -478069140: return getDuration(); 1444 case -1992012396: return getDuration(); 1445 case 444367565: return addUndesirableEffect(); 1446 case -1526770491: return getApplicability(); 1447 case -544509127: return addOtherTherapy(); 1448 default: return super.makeProperty(hash, name); 1449 } 1450 1451 } 1452 1453 @Override 1454 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1455 switch (hash) { 1456 case -1497395130: /*diseaseSymptomProcedure*/ return new String[] {"CodeableReference"}; 1457 case -505503602: /*diseaseStatus*/ return new String[] {"CodeableReference"}; 1458 case -406395211: /*comorbidity*/ return new String[] {"CodeableReference"}; 1459 case 1587112348: /*intendedEffect*/ return new String[] {"CodeableReference"}; 1460 case -1992012396: /*duration*/ return new String[] {"Range", "string"}; 1461 case 444367565: /*undesirableEffect*/ return new String[] {"Reference"}; 1462 case -1526770491: /*applicability*/ return new String[] {"Expression"}; 1463 case -544509127: /*otherTherapy*/ return new String[] {"@ClinicalUseDefinition.contraindication.otherTherapy"}; 1464 default: return super.getTypesForProperty(hash, name); 1465 } 1466 1467 } 1468 1469 @Override 1470 public Base addChild(String name) throws FHIRException { 1471 if (name.equals("diseaseSymptomProcedure")) { 1472 this.diseaseSymptomProcedure = new CodeableReference(); 1473 return this.diseaseSymptomProcedure; 1474 } 1475 else if (name.equals("diseaseStatus")) { 1476 this.diseaseStatus = new CodeableReference(); 1477 return this.diseaseStatus; 1478 } 1479 else if (name.equals("comorbidity")) { 1480 return addComorbidity(); 1481 } 1482 else if (name.equals("intendedEffect")) { 1483 this.intendedEffect = new CodeableReference(); 1484 return this.intendedEffect; 1485 } 1486 else if (name.equals("durationRange")) { 1487 this.duration = new Range(); 1488 return this.duration; 1489 } 1490 else if (name.equals("durationString")) { 1491 this.duration = new StringType(); 1492 return this.duration; 1493 } 1494 else if (name.equals("undesirableEffect")) { 1495 return addUndesirableEffect(); 1496 } 1497 else if (name.equals("applicability")) { 1498 this.applicability = new Expression(); 1499 return this.applicability; 1500 } 1501 else if (name.equals("otherTherapy")) { 1502 return addOtherTherapy(); 1503 } 1504 else 1505 return super.addChild(name); 1506 } 1507 1508 public ClinicalUseDefinitionIndicationComponent copy() { 1509 ClinicalUseDefinitionIndicationComponent dst = new ClinicalUseDefinitionIndicationComponent(); 1510 copyValues(dst); 1511 return dst; 1512 } 1513 1514 public void copyValues(ClinicalUseDefinitionIndicationComponent dst) { 1515 super.copyValues(dst); 1516 dst.diseaseSymptomProcedure = diseaseSymptomProcedure == null ? null : diseaseSymptomProcedure.copy(); 1517 dst.diseaseStatus = diseaseStatus == null ? null : diseaseStatus.copy(); 1518 if (comorbidity != null) { 1519 dst.comorbidity = new ArrayList<CodeableReference>(); 1520 for (CodeableReference i : comorbidity) 1521 dst.comorbidity.add(i.copy()); 1522 }; 1523 dst.intendedEffect = intendedEffect == null ? null : intendedEffect.copy(); 1524 dst.duration = duration == null ? null : duration.copy(); 1525 if (undesirableEffect != null) { 1526 dst.undesirableEffect = new ArrayList<Reference>(); 1527 for (Reference i : undesirableEffect) 1528 dst.undesirableEffect.add(i.copy()); 1529 }; 1530 dst.applicability = applicability == null ? null : applicability.copy(); 1531 if (otherTherapy != null) { 1532 dst.otherTherapy = new ArrayList<ClinicalUseDefinitionContraindicationOtherTherapyComponent>(); 1533 for (ClinicalUseDefinitionContraindicationOtherTherapyComponent i : otherTherapy) 1534 dst.otherTherapy.add(i.copy()); 1535 }; 1536 } 1537 1538 @Override 1539 public boolean equalsDeep(Base other_) { 1540 if (!super.equalsDeep(other_)) 1541 return false; 1542 if (!(other_ instanceof ClinicalUseDefinitionIndicationComponent)) 1543 return false; 1544 ClinicalUseDefinitionIndicationComponent o = (ClinicalUseDefinitionIndicationComponent) other_; 1545 return compareDeep(diseaseSymptomProcedure, o.diseaseSymptomProcedure, true) && compareDeep(diseaseStatus, o.diseaseStatus, true) 1546 && compareDeep(comorbidity, o.comorbidity, true) && compareDeep(intendedEffect, o.intendedEffect, true) 1547 && compareDeep(duration, o.duration, true) && compareDeep(undesirableEffect, o.undesirableEffect, true) 1548 && compareDeep(applicability, o.applicability, true) && compareDeep(otherTherapy, o.otherTherapy, true) 1549 ; 1550 } 1551 1552 @Override 1553 public boolean equalsShallow(Base other_) { 1554 if (!super.equalsShallow(other_)) 1555 return false; 1556 if (!(other_ instanceof ClinicalUseDefinitionIndicationComponent)) 1557 return false; 1558 ClinicalUseDefinitionIndicationComponent o = (ClinicalUseDefinitionIndicationComponent) other_; 1559 return true; 1560 } 1561 1562 public boolean isEmpty() { 1563 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(diseaseSymptomProcedure, diseaseStatus 1564 , comorbidity, intendedEffect, duration, undesirableEffect, applicability, otherTherapy 1565 ); 1566 } 1567 1568 public String fhirType() { 1569 return "ClinicalUseDefinition.indication"; 1570 1571 } 1572 1573 } 1574 1575 @Block() 1576 public static class ClinicalUseDefinitionInteractionComponent extends BackboneElement implements IBaseBackboneElement { 1577 /** 1578 * The specific medication, product, food, substance etc. or laboratory test that interacts. 1579 */ 1580 @Child(name = "interactant", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1581 @Description(shortDefinition="The specific medication, product, food etc. or laboratory test that interacts", formalDefinition="The specific medication, product, food, substance etc. or laboratory test that interacts." ) 1582 protected List<ClinicalUseDefinitionInteractionInteractantComponent> interactant; 1583 1584 /** 1585 * The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction. 1586 */ 1587 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1588 @Description(shortDefinition="The type of the interaction e.g. drug-drug interaction, drug-lab test interaction", formalDefinition="The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction." ) 1589 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interaction-type") 1590 protected CodeableConcept type; 1591 1592 /** 1593 * The effect of the interaction, for example "reduced gastric absorption of primary medication". 1594 */ 1595 @Child(name = "effect", type = {CodeableReference.class}, order=3, min=0, max=1, modifier=false, summary=true) 1596 @Description(shortDefinition="The effect of the interaction, for example \"reduced gastric absorption of primary medication\"", formalDefinition="The effect of the interaction, for example \"reduced gastric absorption of primary medication\"." ) 1597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interaction-effect") 1598 protected CodeableReference effect; 1599 1600 /** 1601 * The incidence of the interaction, e.g. theoretical, observed. 1602 */ 1603 @Child(name = "incidence", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1604 @Description(shortDefinition="The incidence of the interaction, e.g. theoretical, observed", formalDefinition="The incidence of the interaction, e.g. theoretical, observed." ) 1605 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interaction-incidence") 1606 protected CodeableConcept incidence; 1607 1608 /** 1609 * Actions for managing the interaction. 1610 */ 1611 @Child(name = "management", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1612 @Description(shortDefinition="Actions for managing the interaction", formalDefinition="Actions for managing the interaction." ) 1613 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interaction-management") 1614 protected List<CodeableConcept> management; 1615 1616 private static final long serialVersionUID = 2072955553L; 1617 1618 /** 1619 * Constructor 1620 */ 1621 public ClinicalUseDefinitionInteractionComponent() { 1622 super(); 1623 } 1624 1625 /** 1626 * @return {@link #interactant} (The specific medication, product, food, substance etc. or laboratory test that interacts.) 1627 */ 1628 public List<ClinicalUseDefinitionInteractionInteractantComponent> getInteractant() { 1629 if (this.interactant == null) 1630 this.interactant = new ArrayList<ClinicalUseDefinitionInteractionInteractantComponent>(); 1631 return this.interactant; 1632 } 1633 1634 /** 1635 * @return Returns a reference to <code>this</code> for easy method chaining 1636 */ 1637 public ClinicalUseDefinitionInteractionComponent setInteractant(List<ClinicalUseDefinitionInteractionInteractantComponent> theInteractant) { 1638 this.interactant = theInteractant; 1639 return this; 1640 } 1641 1642 public boolean hasInteractant() { 1643 if (this.interactant == null) 1644 return false; 1645 for (ClinicalUseDefinitionInteractionInteractantComponent item : this.interactant) 1646 if (!item.isEmpty()) 1647 return true; 1648 return false; 1649 } 1650 1651 public ClinicalUseDefinitionInteractionInteractantComponent addInteractant() { //3 1652 ClinicalUseDefinitionInteractionInteractantComponent t = new ClinicalUseDefinitionInteractionInteractantComponent(); 1653 if (this.interactant == null) 1654 this.interactant = new ArrayList<ClinicalUseDefinitionInteractionInteractantComponent>(); 1655 this.interactant.add(t); 1656 return t; 1657 } 1658 1659 public ClinicalUseDefinitionInteractionComponent addInteractant(ClinicalUseDefinitionInteractionInteractantComponent t) { //3 1660 if (t == null) 1661 return this; 1662 if (this.interactant == null) 1663 this.interactant = new ArrayList<ClinicalUseDefinitionInteractionInteractantComponent>(); 1664 this.interactant.add(t); 1665 return this; 1666 } 1667 1668 /** 1669 * @return The first repetition of repeating field {@link #interactant}, creating it if it does not already exist {3} 1670 */ 1671 public ClinicalUseDefinitionInteractionInteractantComponent getInteractantFirstRep() { 1672 if (getInteractant().isEmpty()) { 1673 addInteractant(); 1674 } 1675 return getInteractant().get(0); 1676 } 1677 1678 /** 1679 * @return {@link #type} (The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.) 1680 */ 1681 public CodeableConcept getType() { 1682 if (this.type == null) 1683 if (Configuration.errorOnAutoCreate()) 1684 throw new Error("Attempt to auto-create ClinicalUseDefinitionInteractionComponent.type"); 1685 else if (Configuration.doAutoCreate()) 1686 this.type = new CodeableConcept(); // cc 1687 return this.type; 1688 } 1689 1690 public boolean hasType() { 1691 return this.type != null && !this.type.isEmpty(); 1692 } 1693 1694 /** 1695 * @param value {@link #type} (The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.) 1696 */ 1697 public ClinicalUseDefinitionInteractionComponent setType(CodeableConcept value) { 1698 this.type = value; 1699 return this; 1700 } 1701 1702 /** 1703 * @return {@link #effect} (The effect of the interaction, for example "reduced gastric absorption of primary medication".) 1704 */ 1705 public CodeableReference getEffect() { 1706 if (this.effect == null) 1707 if (Configuration.errorOnAutoCreate()) 1708 throw new Error("Attempt to auto-create ClinicalUseDefinitionInteractionComponent.effect"); 1709 else if (Configuration.doAutoCreate()) 1710 this.effect = new CodeableReference(); // cc 1711 return this.effect; 1712 } 1713 1714 public boolean hasEffect() { 1715 return this.effect != null && !this.effect.isEmpty(); 1716 } 1717 1718 /** 1719 * @param value {@link #effect} (The effect of the interaction, for example "reduced gastric absorption of primary medication".) 1720 */ 1721 public ClinicalUseDefinitionInteractionComponent setEffect(CodeableReference value) { 1722 this.effect = value; 1723 return this; 1724 } 1725 1726 /** 1727 * @return {@link #incidence} (The incidence of the interaction, e.g. theoretical, observed.) 1728 */ 1729 public CodeableConcept getIncidence() { 1730 if (this.incidence == null) 1731 if (Configuration.errorOnAutoCreate()) 1732 throw new Error("Attempt to auto-create ClinicalUseDefinitionInteractionComponent.incidence"); 1733 else if (Configuration.doAutoCreate()) 1734 this.incidence = new CodeableConcept(); // cc 1735 return this.incidence; 1736 } 1737 1738 public boolean hasIncidence() { 1739 return this.incidence != null && !this.incidence.isEmpty(); 1740 } 1741 1742 /** 1743 * @param value {@link #incidence} (The incidence of the interaction, e.g. theoretical, observed.) 1744 */ 1745 public ClinicalUseDefinitionInteractionComponent setIncidence(CodeableConcept value) { 1746 this.incidence = value; 1747 return this; 1748 } 1749 1750 /** 1751 * @return {@link #management} (Actions for managing the interaction.) 1752 */ 1753 public List<CodeableConcept> getManagement() { 1754 if (this.management == null) 1755 this.management = new ArrayList<CodeableConcept>(); 1756 return this.management; 1757 } 1758 1759 /** 1760 * @return Returns a reference to <code>this</code> for easy method chaining 1761 */ 1762 public ClinicalUseDefinitionInteractionComponent setManagement(List<CodeableConcept> theManagement) { 1763 this.management = theManagement; 1764 return this; 1765 } 1766 1767 public boolean hasManagement() { 1768 if (this.management == null) 1769 return false; 1770 for (CodeableConcept item : this.management) 1771 if (!item.isEmpty()) 1772 return true; 1773 return false; 1774 } 1775 1776 public CodeableConcept addManagement() { //3 1777 CodeableConcept t = new CodeableConcept(); 1778 if (this.management == null) 1779 this.management = new ArrayList<CodeableConcept>(); 1780 this.management.add(t); 1781 return t; 1782 } 1783 1784 public ClinicalUseDefinitionInteractionComponent addManagement(CodeableConcept t) { //3 1785 if (t == null) 1786 return this; 1787 if (this.management == null) 1788 this.management = new ArrayList<CodeableConcept>(); 1789 this.management.add(t); 1790 return this; 1791 } 1792 1793 /** 1794 * @return The first repetition of repeating field {@link #management}, creating it if it does not already exist {3} 1795 */ 1796 public CodeableConcept getManagementFirstRep() { 1797 if (getManagement().isEmpty()) { 1798 addManagement(); 1799 } 1800 return getManagement().get(0); 1801 } 1802 1803 protected void listChildren(List<Property> children) { 1804 super.listChildren(children); 1805 children.add(new Property("interactant", "", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, java.lang.Integer.MAX_VALUE, interactant)); 1806 children.add(new Property("type", "CodeableConcept", "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.", 0, 1, type)); 1807 children.add(new Property("effect", "CodeableReference(ObservationDefinition)", "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".", 0, 1, effect)); 1808 children.add(new Property("incidence", "CodeableConcept", "The incidence of the interaction, e.g. theoretical, observed.", 0, 1, incidence)); 1809 children.add(new Property("management", "CodeableConcept", "Actions for managing the interaction.", 0, java.lang.Integer.MAX_VALUE, management)); 1810 } 1811 1812 @Override 1813 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1814 switch (_hash) { 1815 case 1844097009: /*interactant*/ return new Property("interactant", "", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, java.lang.Integer.MAX_VALUE, interactant); 1816 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.", 0, 1, type); 1817 case -1306084975: /*effect*/ return new Property("effect", "CodeableReference(ObservationDefinition)", "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".", 0, 1, effect); 1818 case -1598467132: /*incidence*/ return new Property("incidence", "CodeableConcept", "The incidence of the interaction, e.g. theoretical, observed.", 0, 1, incidence); 1819 case -1799980989: /*management*/ return new Property("management", "CodeableConcept", "Actions for managing the interaction.", 0, java.lang.Integer.MAX_VALUE, management); 1820 default: return super.getNamedProperty(_hash, _name, _checkValid); 1821 } 1822 1823 } 1824 1825 @Override 1826 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1827 switch (hash) { 1828 case 1844097009: /*interactant*/ return this.interactant == null ? new Base[0] : this.interactant.toArray(new Base[this.interactant.size()]); // ClinicalUseDefinitionInteractionInteractantComponent 1829 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1830 case -1306084975: /*effect*/ return this.effect == null ? new Base[0] : new Base[] {this.effect}; // CodeableReference 1831 case -1598467132: /*incidence*/ return this.incidence == null ? new Base[0] : new Base[] {this.incidence}; // CodeableConcept 1832 case -1799980989: /*management*/ return this.management == null ? new Base[0] : this.management.toArray(new Base[this.management.size()]); // CodeableConcept 1833 default: return super.getProperty(hash, name, checkValid); 1834 } 1835 1836 } 1837 1838 @Override 1839 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1840 switch (hash) { 1841 case 1844097009: // interactant 1842 this.getInteractant().add((ClinicalUseDefinitionInteractionInteractantComponent) value); // ClinicalUseDefinitionInteractionInteractantComponent 1843 return value; 1844 case 3575610: // type 1845 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1846 return value; 1847 case -1306084975: // effect 1848 this.effect = TypeConvertor.castToCodeableReference(value); // CodeableReference 1849 return value; 1850 case -1598467132: // incidence 1851 this.incidence = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1852 return value; 1853 case -1799980989: // management 1854 this.getManagement().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1855 return value; 1856 default: return super.setProperty(hash, name, value); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base setProperty(String name, Base value) throws FHIRException { 1863 if (name.equals("interactant")) { 1864 this.getInteractant().add((ClinicalUseDefinitionInteractionInteractantComponent) value); 1865 } else if (name.equals("type")) { 1866 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1867 } else if (name.equals("effect")) { 1868 this.effect = TypeConvertor.castToCodeableReference(value); // CodeableReference 1869 } else if (name.equals("incidence")) { 1870 this.incidence = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1871 } else if (name.equals("management")) { 1872 this.getManagement().add(TypeConvertor.castToCodeableConcept(value)); 1873 } else 1874 return super.setProperty(name, value); 1875 return value; 1876 } 1877 1878 @Override 1879 public void removeChild(String name, Base value) throws FHIRException { 1880 if (name.equals("interactant")) { 1881 this.getInteractant().remove((ClinicalUseDefinitionInteractionInteractantComponent) value); 1882 } else if (name.equals("type")) { 1883 this.type = null; 1884 } else if (name.equals("effect")) { 1885 this.effect = null; 1886 } else if (name.equals("incidence")) { 1887 this.incidence = null; 1888 } else if (name.equals("management")) { 1889 this.getManagement().remove(value); 1890 } else 1891 super.removeChild(name, value); 1892 1893 } 1894 1895 @Override 1896 public Base makeProperty(int hash, String name) throws FHIRException { 1897 switch (hash) { 1898 case 1844097009: return addInteractant(); 1899 case 3575610: return getType(); 1900 case -1306084975: return getEffect(); 1901 case -1598467132: return getIncidence(); 1902 case -1799980989: return addManagement(); 1903 default: return super.makeProperty(hash, name); 1904 } 1905 1906 } 1907 1908 @Override 1909 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1910 switch (hash) { 1911 case 1844097009: /*interactant*/ return new String[] {}; 1912 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1913 case -1306084975: /*effect*/ return new String[] {"CodeableReference"}; 1914 case -1598467132: /*incidence*/ return new String[] {"CodeableConcept"}; 1915 case -1799980989: /*management*/ return new String[] {"CodeableConcept"}; 1916 default: return super.getTypesForProperty(hash, name); 1917 } 1918 1919 } 1920 1921 @Override 1922 public Base addChild(String name) throws FHIRException { 1923 if (name.equals("interactant")) { 1924 return addInteractant(); 1925 } 1926 else if (name.equals("type")) { 1927 this.type = new CodeableConcept(); 1928 return this.type; 1929 } 1930 else if (name.equals("effect")) { 1931 this.effect = new CodeableReference(); 1932 return this.effect; 1933 } 1934 else if (name.equals("incidence")) { 1935 this.incidence = new CodeableConcept(); 1936 return this.incidence; 1937 } 1938 else if (name.equals("management")) { 1939 return addManagement(); 1940 } 1941 else 1942 return super.addChild(name); 1943 } 1944 1945 public ClinicalUseDefinitionInteractionComponent copy() { 1946 ClinicalUseDefinitionInteractionComponent dst = new ClinicalUseDefinitionInteractionComponent(); 1947 copyValues(dst); 1948 return dst; 1949 } 1950 1951 public void copyValues(ClinicalUseDefinitionInteractionComponent dst) { 1952 super.copyValues(dst); 1953 if (interactant != null) { 1954 dst.interactant = new ArrayList<ClinicalUseDefinitionInteractionInteractantComponent>(); 1955 for (ClinicalUseDefinitionInteractionInteractantComponent i : interactant) 1956 dst.interactant.add(i.copy()); 1957 }; 1958 dst.type = type == null ? null : type.copy(); 1959 dst.effect = effect == null ? null : effect.copy(); 1960 dst.incidence = incidence == null ? null : incidence.copy(); 1961 if (management != null) { 1962 dst.management = new ArrayList<CodeableConcept>(); 1963 for (CodeableConcept i : management) 1964 dst.management.add(i.copy()); 1965 }; 1966 } 1967 1968 @Override 1969 public boolean equalsDeep(Base other_) { 1970 if (!super.equalsDeep(other_)) 1971 return false; 1972 if (!(other_ instanceof ClinicalUseDefinitionInteractionComponent)) 1973 return false; 1974 ClinicalUseDefinitionInteractionComponent o = (ClinicalUseDefinitionInteractionComponent) other_; 1975 return compareDeep(interactant, o.interactant, true) && compareDeep(type, o.type, true) && compareDeep(effect, o.effect, true) 1976 && compareDeep(incidence, o.incidence, true) && compareDeep(management, o.management, true); 1977 } 1978 1979 @Override 1980 public boolean equalsShallow(Base other_) { 1981 if (!super.equalsShallow(other_)) 1982 return false; 1983 if (!(other_ instanceof ClinicalUseDefinitionInteractionComponent)) 1984 return false; 1985 ClinicalUseDefinitionInteractionComponent o = (ClinicalUseDefinitionInteractionComponent) other_; 1986 return true; 1987 } 1988 1989 public boolean isEmpty() { 1990 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(interactant, type, effect 1991 , incidence, management); 1992 } 1993 1994 public String fhirType() { 1995 return "ClinicalUseDefinition.interaction"; 1996 1997 } 1998 1999 } 2000 2001 @Block() 2002 public static class ClinicalUseDefinitionInteractionInteractantComponent extends BackboneElement implements IBaseBackboneElement { 2003 /** 2004 * The specific medication, product, food, substance etc. or laboratory test that interacts. 2005 */ 2006 @Child(name = "item", type = {MedicinalProductDefinition.class, Medication.class, Substance.class, NutritionProduct.class, BiologicallyDerivedProduct.class, ObservationDefinition.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 2007 @Description(shortDefinition="The specific medication, product, food etc. or laboratory test that interacts", formalDefinition="The specific medication, product, food, substance etc. or laboratory test that interacts." ) 2008 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interactant") 2009 protected DataType item; 2010 2011 private static final long serialVersionUID = 1847936859L; 2012 2013 /** 2014 * Constructor 2015 */ 2016 public ClinicalUseDefinitionInteractionInteractantComponent() { 2017 super(); 2018 } 2019 2020 /** 2021 * Constructor 2022 */ 2023 public ClinicalUseDefinitionInteractionInteractantComponent(DataType item) { 2024 super(); 2025 this.setItem(item); 2026 } 2027 2028 /** 2029 * @return {@link #item} (The specific medication, product, food, substance etc. or laboratory test that interacts.) 2030 */ 2031 public DataType getItem() { 2032 return this.item; 2033 } 2034 2035 /** 2036 * @return {@link #item} (The specific medication, product, food, substance etc. or laboratory test that interacts.) 2037 */ 2038 public Reference getItemReference() throws FHIRException { 2039 if (this.item == null) 2040 this.item = new Reference(); 2041 if (!(this.item instanceof Reference)) 2042 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 2043 return (Reference) this.item; 2044 } 2045 2046 public boolean hasItemReference() { 2047 return this.item instanceof Reference; 2048 } 2049 2050 /** 2051 * @return {@link #item} (The specific medication, product, food, substance etc. or laboratory test that interacts.) 2052 */ 2053 public CodeableConcept getItemCodeableConcept() throws FHIRException { 2054 if (this.item == null) 2055 this.item = new CodeableConcept(); 2056 if (!(this.item instanceof CodeableConcept)) 2057 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 2058 return (CodeableConcept) this.item; 2059 } 2060 2061 public boolean hasItemCodeableConcept() { 2062 return this.item instanceof CodeableConcept; 2063 } 2064 2065 public boolean hasItem() { 2066 return this.item != null && !this.item.isEmpty(); 2067 } 2068 2069 /** 2070 * @param value {@link #item} (The specific medication, product, food, substance etc. or laboratory test that interacts.) 2071 */ 2072 public ClinicalUseDefinitionInteractionInteractantComponent setItem(DataType value) { 2073 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 2074 throw new FHIRException("Not the right type for ClinicalUseDefinition.interaction.interactant.item[x]: "+value.fhirType()); 2075 this.item = value; 2076 return this; 2077 } 2078 2079 protected void listChildren(List<Property> children) { 2080 super.listChildren(children); 2081 children.add(new Property("item[x]", "Reference(MedicinalProductDefinition|Medication|Substance|NutritionProduct|BiologicallyDerivedProduct|ObservationDefinition)|CodeableConcept", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, 1, item)); 2082 } 2083 2084 @Override 2085 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2086 switch (_hash) { 2087 case 2116201613: /*item[x]*/ return new Property("item[x]", "Reference(MedicinalProductDefinition|Medication|Substance|NutritionProduct|BiologicallyDerivedProduct|ObservationDefinition)|CodeableConcept", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, 1, item); 2088 case 3242771: /*item*/ return new Property("item[x]", "Reference(MedicinalProductDefinition|Medication|Substance|NutritionProduct|BiologicallyDerivedProduct|ObservationDefinition)|CodeableConcept", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, 1, item); 2089 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(MedicinalProductDefinition|Medication|Substance|NutritionProduct|BiologicallyDerivedProduct|ObservationDefinition)", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, 1, item); 2090 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "The specific medication, product, food, substance etc. or laboratory test that interacts.", 0, 1, item); 2091 default: return super.getNamedProperty(_hash, _name, _checkValid); 2092 } 2093 2094 } 2095 2096 @Override 2097 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2098 switch (hash) { 2099 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 2100 default: return super.getProperty(hash, name, checkValid); 2101 } 2102 2103 } 2104 2105 @Override 2106 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2107 switch (hash) { 2108 case 3242771: // item 2109 this.item = TypeConvertor.castToType(value); // DataType 2110 return value; 2111 default: return super.setProperty(hash, name, value); 2112 } 2113 2114 } 2115 2116 @Override 2117 public Base setProperty(String name, Base value) throws FHIRException { 2118 if (name.equals("item[x]")) { 2119 this.item = TypeConvertor.castToType(value); // DataType 2120 } else 2121 return super.setProperty(name, value); 2122 return value; 2123 } 2124 2125 @Override 2126 public void removeChild(String name, Base value) throws FHIRException { 2127 if (name.equals("item[x]")) { 2128 this.item = null; 2129 } else 2130 super.removeChild(name, value); 2131 2132 } 2133 2134 @Override 2135 public Base makeProperty(int hash, String name) throws FHIRException { 2136 switch (hash) { 2137 case 2116201613: return getItem(); 2138 case 3242771: return getItem(); 2139 default: return super.makeProperty(hash, name); 2140 } 2141 2142 } 2143 2144 @Override 2145 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2146 switch (hash) { 2147 case 3242771: /*item*/ return new String[] {"Reference", "CodeableConcept"}; 2148 default: return super.getTypesForProperty(hash, name); 2149 } 2150 2151 } 2152 2153 @Override 2154 public Base addChild(String name) throws FHIRException { 2155 if (name.equals("itemReference")) { 2156 this.item = new Reference(); 2157 return this.item; 2158 } 2159 else if (name.equals("itemCodeableConcept")) { 2160 this.item = new CodeableConcept(); 2161 return this.item; 2162 } 2163 else 2164 return super.addChild(name); 2165 } 2166 2167 public ClinicalUseDefinitionInteractionInteractantComponent copy() { 2168 ClinicalUseDefinitionInteractionInteractantComponent dst = new ClinicalUseDefinitionInteractionInteractantComponent(); 2169 copyValues(dst); 2170 return dst; 2171 } 2172 2173 public void copyValues(ClinicalUseDefinitionInteractionInteractantComponent dst) { 2174 super.copyValues(dst); 2175 dst.item = item == null ? null : item.copy(); 2176 } 2177 2178 @Override 2179 public boolean equalsDeep(Base other_) { 2180 if (!super.equalsDeep(other_)) 2181 return false; 2182 if (!(other_ instanceof ClinicalUseDefinitionInteractionInteractantComponent)) 2183 return false; 2184 ClinicalUseDefinitionInteractionInteractantComponent o = (ClinicalUseDefinitionInteractionInteractantComponent) other_; 2185 return compareDeep(item, o.item, true); 2186 } 2187 2188 @Override 2189 public boolean equalsShallow(Base other_) { 2190 if (!super.equalsShallow(other_)) 2191 return false; 2192 if (!(other_ instanceof ClinicalUseDefinitionInteractionInteractantComponent)) 2193 return false; 2194 ClinicalUseDefinitionInteractionInteractantComponent o = (ClinicalUseDefinitionInteractionInteractantComponent) other_; 2195 return true; 2196 } 2197 2198 public boolean isEmpty() { 2199 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 2200 } 2201 2202 public String fhirType() { 2203 return "ClinicalUseDefinition.interaction.interactant"; 2204 2205 } 2206 2207 } 2208 2209 @Block() 2210 public static class ClinicalUseDefinitionUndesirableEffectComponent extends BackboneElement implements IBaseBackboneElement { 2211 /** 2212 * The situation in which the undesirable effect may manifest. 2213 */ 2214 @Child(name = "symptomConditionEffect", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 2215 @Description(shortDefinition="The situation in which the undesirable effect may manifest", formalDefinition="The situation in which the undesirable effect may manifest." ) 2216 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/undesirable-effect-symptom") 2217 protected CodeableReference symptomConditionEffect; 2218 2219 /** 2220 * High level classification of the effect. 2221 */ 2222 @Child(name = "classification", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2223 @Description(shortDefinition="High level classification of the effect", formalDefinition="High level classification of the effect." ) 2224 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/undesirable-effect-classification") 2225 protected CodeableConcept classification; 2226 2227 /** 2228 * How often the effect is seen. 2229 */ 2230 @Child(name = "frequencyOfOccurrence", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 2231 @Description(shortDefinition="How often the effect is seen", formalDefinition="How often the effect is seen." ) 2232 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/undesirable-effect-frequency") 2233 protected CodeableConcept frequencyOfOccurrence; 2234 2235 private static final long serialVersionUID = -55472609L; 2236 2237 /** 2238 * Constructor 2239 */ 2240 public ClinicalUseDefinitionUndesirableEffectComponent() { 2241 super(); 2242 } 2243 2244 /** 2245 * @return {@link #symptomConditionEffect} (The situation in which the undesirable effect may manifest.) 2246 */ 2247 public CodeableReference getSymptomConditionEffect() { 2248 if (this.symptomConditionEffect == null) 2249 if (Configuration.errorOnAutoCreate()) 2250 throw new Error("Attempt to auto-create ClinicalUseDefinitionUndesirableEffectComponent.symptomConditionEffect"); 2251 else if (Configuration.doAutoCreate()) 2252 this.symptomConditionEffect = new CodeableReference(); // cc 2253 return this.symptomConditionEffect; 2254 } 2255 2256 public boolean hasSymptomConditionEffect() { 2257 return this.symptomConditionEffect != null && !this.symptomConditionEffect.isEmpty(); 2258 } 2259 2260 /** 2261 * @param value {@link #symptomConditionEffect} (The situation in which the undesirable effect may manifest.) 2262 */ 2263 public ClinicalUseDefinitionUndesirableEffectComponent setSymptomConditionEffect(CodeableReference value) { 2264 this.symptomConditionEffect = value; 2265 return this; 2266 } 2267 2268 /** 2269 * @return {@link #classification} (High level classification of the effect.) 2270 */ 2271 public CodeableConcept getClassification() { 2272 if (this.classification == null) 2273 if (Configuration.errorOnAutoCreate()) 2274 throw new Error("Attempt to auto-create ClinicalUseDefinitionUndesirableEffectComponent.classification"); 2275 else if (Configuration.doAutoCreate()) 2276 this.classification = new CodeableConcept(); // cc 2277 return this.classification; 2278 } 2279 2280 public boolean hasClassification() { 2281 return this.classification != null && !this.classification.isEmpty(); 2282 } 2283 2284 /** 2285 * @param value {@link #classification} (High level classification of the effect.) 2286 */ 2287 public ClinicalUseDefinitionUndesirableEffectComponent setClassification(CodeableConcept value) { 2288 this.classification = value; 2289 return this; 2290 } 2291 2292 /** 2293 * @return {@link #frequencyOfOccurrence} (How often the effect is seen.) 2294 */ 2295 public CodeableConcept getFrequencyOfOccurrence() { 2296 if (this.frequencyOfOccurrence == null) 2297 if (Configuration.errorOnAutoCreate()) 2298 throw new Error("Attempt to auto-create ClinicalUseDefinitionUndesirableEffectComponent.frequencyOfOccurrence"); 2299 else if (Configuration.doAutoCreate()) 2300 this.frequencyOfOccurrence = new CodeableConcept(); // cc 2301 return this.frequencyOfOccurrence; 2302 } 2303 2304 public boolean hasFrequencyOfOccurrence() { 2305 return this.frequencyOfOccurrence != null && !this.frequencyOfOccurrence.isEmpty(); 2306 } 2307 2308 /** 2309 * @param value {@link #frequencyOfOccurrence} (How often the effect is seen.) 2310 */ 2311 public ClinicalUseDefinitionUndesirableEffectComponent setFrequencyOfOccurrence(CodeableConcept value) { 2312 this.frequencyOfOccurrence = value; 2313 return this; 2314 } 2315 2316 protected void listChildren(List<Property> children) { 2317 super.listChildren(children); 2318 children.add(new Property("symptomConditionEffect", "CodeableReference(ObservationDefinition)", "The situation in which the undesirable effect may manifest.", 0, 1, symptomConditionEffect)); 2319 children.add(new Property("classification", "CodeableConcept", "High level classification of the effect.", 0, 1, classification)); 2320 children.add(new Property("frequencyOfOccurrence", "CodeableConcept", "How often the effect is seen.", 0, 1, frequencyOfOccurrence)); 2321 } 2322 2323 @Override 2324 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2325 switch (_hash) { 2326 case -650549981: /*symptomConditionEffect*/ return new Property("symptomConditionEffect", "CodeableReference(ObservationDefinition)", "The situation in which the undesirable effect may manifest.", 0, 1, symptomConditionEffect); 2327 case 382350310: /*classification*/ return new Property("classification", "CodeableConcept", "High level classification of the effect.", 0, 1, classification); 2328 case 791175812: /*frequencyOfOccurrence*/ return new Property("frequencyOfOccurrence", "CodeableConcept", "How often the effect is seen.", 0, 1, frequencyOfOccurrence); 2329 default: return super.getNamedProperty(_hash, _name, _checkValid); 2330 } 2331 2332 } 2333 2334 @Override 2335 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2336 switch (hash) { 2337 case -650549981: /*symptomConditionEffect*/ return this.symptomConditionEffect == null ? new Base[0] : new Base[] {this.symptomConditionEffect}; // CodeableReference 2338 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : new Base[] {this.classification}; // CodeableConcept 2339 case 791175812: /*frequencyOfOccurrence*/ return this.frequencyOfOccurrence == null ? new Base[0] : new Base[] {this.frequencyOfOccurrence}; // CodeableConcept 2340 default: return super.getProperty(hash, name, checkValid); 2341 } 2342 2343 } 2344 2345 @Override 2346 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2347 switch (hash) { 2348 case -650549981: // symptomConditionEffect 2349 this.symptomConditionEffect = TypeConvertor.castToCodeableReference(value); // CodeableReference 2350 return value; 2351 case 382350310: // classification 2352 this.classification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2353 return value; 2354 case 791175812: // frequencyOfOccurrence 2355 this.frequencyOfOccurrence = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2356 return value; 2357 default: return super.setProperty(hash, name, value); 2358 } 2359 2360 } 2361 2362 @Override 2363 public Base setProperty(String name, Base value) throws FHIRException { 2364 if (name.equals("symptomConditionEffect")) { 2365 this.symptomConditionEffect = TypeConvertor.castToCodeableReference(value); // CodeableReference 2366 } else if (name.equals("classification")) { 2367 this.classification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2368 } else if (name.equals("frequencyOfOccurrence")) { 2369 this.frequencyOfOccurrence = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2370 } else 2371 return super.setProperty(name, value); 2372 return value; 2373 } 2374 2375 @Override 2376 public void removeChild(String name, Base value) throws FHIRException { 2377 if (name.equals("symptomConditionEffect")) { 2378 this.symptomConditionEffect = null; 2379 } else if (name.equals("classification")) { 2380 this.classification = null; 2381 } else if (name.equals("frequencyOfOccurrence")) { 2382 this.frequencyOfOccurrence = null; 2383 } else 2384 super.removeChild(name, value); 2385 2386 } 2387 2388 @Override 2389 public Base makeProperty(int hash, String name) throws FHIRException { 2390 switch (hash) { 2391 case -650549981: return getSymptomConditionEffect(); 2392 case 382350310: return getClassification(); 2393 case 791175812: return getFrequencyOfOccurrence(); 2394 default: return super.makeProperty(hash, name); 2395 } 2396 2397 } 2398 2399 @Override 2400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2401 switch (hash) { 2402 case -650549981: /*symptomConditionEffect*/ return new String[] {"CodeableReference"}; 2403 case 382350310: /*classification*/ return new String[] {"CodeableConcept"}; 2404 case 791175812: /*frequencyOfOccurrence*/ return new String[] {"CodeableConcept"}; 2405 default: return super.getTypesForProperty(hash, name); 2406 } 2407 2408 } 2409 2410 @Override 2411 public Base addChild(String name) throws FHIRException { 2412 if (name.equals("symptomConditionEffect")) { 2413 this.symptomConditionEffect = new CodeableReference(); 2414 return this.symptomConditionEffect; 2415 } 2416 else if (name.equals("classification")) { 2417 this.classification = new CodeableConcept(); 2418 return this.classification; 2419 } 2420 else if (name.equals("frequencyOfOccurrence")) { 2421 this.frequencyOfOccurrence = new CodeableConcept(); 2422 return this.frequencyOfOccurrence; 2423 } 2424 else 2425 return super.addChild(name); 2426 } 2427 2428 public ClinicalUseDefinitionUndesirableEffectComponent copy() { 2429 ClinicalUseDefinitionUndesirableEffectComponent dst = new ClinicalUseDefinitionUndesirableEffectComponent(); 2430 copyValues(dst); 2431 return dst; 2432 } 2433 2434 public void copyValues(ClinicalUseDefinitionUndesirableEffectComponent dst) { 2435 super.copyValues(dst); 2436 dst.symptomConditionEffect = symptomConditionEffect == null ? null : symptomConditionEffect.copy(); 2437 dst.classification = classification == null ? null : classification.copy(); 2438 dst.frequencyOfOccurrence = frequencyOfOccurrence == null ? null : frequencyOfOccurrence.copy(); 2439 } 2440 2441 @Override 2442 public boolean equalsDeep(Base other_) { 2443 if (!super.equalsDeep(other_)) 2444 return false; 2445 if (!(other_ instanceof ClinicalUseDefinitionUndesirableEffectComponent)) 2446 return false; 2447 ClinicalUseDefinitionUndesirableEffectComponent o = (ClinicalUseDefinitionUndesirableEffectComponent) other_; 2448 return compareDeep(symptomConditionEffect, o.symptomConditionEffect, true) && compareDeep(classification, o.classification, true) 2449 && compareDeep(frequencyOfOccurrence, o.frequencyOfOccurrence, true); 2450 } 2451 2452 @Override 2453 public boolean equalsShallow(Base other_) { 2454 if (!super.equalsShallow(other_)) 2455 return false; 2456 if (!(other_ instanceof ClinicalUseDefinitionUndesirableEffectComponent)) 2457 return false; 2458 ClinicalUseDefinitionUndesirableEffectComponent o = (ClinicalUseDefinitionUndesirableEffectComponent) other_; 2459 return true; 2460 } 2461 2462 public boolean isEmpty() { 2463 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(symptomConditionEffect, classification 2464 , frequencyOfOccurrence); 2465 } 2466 2467 public String fhirType() { 2468 return "ClinicalUseDefinition.undesirableEffect"; 2469 2470 } 2471 2472 } 2473 2474 @Block() 2475 public static class ClinicalUseDefinitionWarningComponent extends BackboneElement implements IBaseBackboneElement { 2476 /** 2477 * A textual definition of this warning, with formatting. 2478 */ 2479 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2480 @Description(shortDefinition="A textual definition of this warning, with formatting", formalDefinition="A textual definition of this warning, with formatting." ) 2481 protected MarkdownType description; 2482 2483 /** 2484 * A coded or unformatted textual definition of this warning. 2485 */ 2486 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2487 @Description(shortDefinition="A coded or unformatted textual definition of this warning", formalDefinition="A coded or unformatted textual definition of this warning." ) 2488 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/warning-type") 2489 protected CodeableConcept code; 2490 2491 private static final long serialVersionUID = 213710553L; 2492 2493 /** 2494 * Constructor 2495 */ 2496 public ClinicalUseDefinitionWarningComponent() { 2497 super(); 2498 } 2499 2500 /** 2501 * @return {@link #description} (A textual definition of this warning, with formatting.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2502 */ 2503 public MarkdownType getDescriptionElement() { 2504 if (this.description == null) 2505 if (Configuration.errorOnAutoCreate()) 2506 throw new Error("Attempt to auto-create ClinicalUseDefinitionWarningComponent.description"); 2507 else if (Configuration.doAutoCreate()) 2508 this.description = new MarkdownType(); // bb 2509 return this.description; 2510 } 2511 2512 public boolean hasDescriptionElement() { 2513 return this.description != null && !this.description.isEmpty(); 2514 } 2515 2516 public boolean hasDescription() { 2517 return this.description != null && !this.description.isEmpty(); 2518 } 2519 2520 /** 2521 * @param value {@link #description} (A textual definition of this warning, with formatting.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2522 */ 2523 public ClinicalUseDefinitionWarningComponent setDescriptionElement(MarkdownType value) { 2524 this.description = value; 2525 return this; 2526 } 2527 2528 /** 2529 * @return A textual definition of this warning, with formatting. 2530 */ 2531 public String getDescription() { 2532 return this.description == null ? null : this.description.getValue(); 2533 } 2534 2535 /** 2536 * @param value A textual definition of this warning, with formatting. 2537 */ 2538 public ClinicalUseDefinitionWarningComponent setDescription(String value) { 2539 if (Utilities.noString(value)) 2540 this.description = null; 2541 else { 2542 if (this.description == null) 2543 this.description = new MarkdownType(); 2544 this.description.setValue(value); 2545 } 2546 return this; 2547 } 2548 2549 /** 2550 * @return {@link #code} (A coded or unformatted textual definition of this warning.) 2551 */ 2552 public CodeableConcept getCode() { 2553 if (this.code == null) 2554 if (Configuration.errorOnAutoCreate()) 2555 throw new Error("Attempt to auto-create ClinicalUseDefinitionWarningComponent.code"); 2556 else if (Configuration.doAutoCreate()) 2557 this.code = new CodeableConcept(); // cc 2558 return this.code; 2559 } 2560 2561 public boolean hasCode() { 2562 return this.code != null && !this.code.isEmpty(); 2563 } 2564 2565 /** 2566 * @param value {@link #code} (A coded or unformatted textual definition of this warning.) 2567 */ 2568 public ClinicalUseDefinitionWarningComponent setCode(CodeableConcept value) { 2569 this.code = value; 2570 return this; 2571 } 2572 2573 protected void listChildren(List<Property> children) { 2574 super.listChildren(children); 2575 children.add(new Property("description", "markdown", "A textual definition of this warning, with formatting.", 0, 1, description)); 2576 children.add(new Property("code", "CodeableConcept", "A coded or unformatted textual definition of this warning.", 0, 1, code)); 2577 } 2578 2579 @Override 2580 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2581 switch (_hash) { 2582 case -1724546052: /*description*/ return new Property("description", "markdown", "A textual definition of this warning, with formatting.", 0, 1, description); 2583 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A coded or unformatted textual definition of this warning.", 0, 1, code); 2584 default: return super.getNamedProperty(_hash, _name, _checkValid); 2585 } 2586 2587 } 2588 2589 @Override 2590 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2591 switch (hash) { 2592 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2593 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2594 default: return super.getProperty(hash, name, checkValid); 2595 } 2596 2597 } 2598 2599 @Override 2600 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2601 switch (hash) { 2602 case -1724546052: // description 2603 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2604 return value; 2605 case 3059181: // code 2606 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2607 return value; 2608 default: return super.setProperty(hash, name, value); 2609 } 2610 2611 } 2612 2613 @Override 2614 public Base setProperty(String name, Base value) throws FHIRException { 2615 if (name.equals("description")) { 2616 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2617 } else if (name.equals("code")) { 2618 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2619 } else 2620 return super.setProperty(name, value); 2621 return value; 2622 } 2623 2624 @Override 2625 public void removeChild(String name, Base value) throws FHIRException { 2626 if (name.equals("description")) { 2627 this.description = null; 2628 } else if (name.equals("code")) { 2629 this.code = null; 2630 } else 2631 super.removeChild(name, value); 2632 2633 } 2634 2635 @Override 2636 public Base makeProperty(int hash, String name) throws FHIRException { 2637 switch (hash) { 2638 case -1724546052: return getDescriptionElement(); 2639 case 3059181: return getCode(); 2640 default: return super.makeProperty(hash, name); 2641 } 2642 2643 } 2644 2645 @Override 2646 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2647 switch (hash) { 2648 case -1724546052: /*description*/ return new String[] {"markdown"}; 2649 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2650 default: return super.getTypesForProperty(hash, name); 2651 } 2652 2653 } 2654 2655 @Override 2656 public Base addChild(String name) throws FHIRException { 2657 if (name.equals("description")) { 2658 throw new FHIRException("Cannot call addChild on a singleton property ClinicalUseDefinition.warning.description"); 2659 } 2660 else if (name.equals("code")) { 2661 this.code = new CodeableConcept(); 2662 return this.code; 2663 } 2664 else 2665 return super.addChild(name); 2666 } 2667 2668 public ClinicalUseDefinitionWarningComponent copy() { 2669 ClinicalUseDefinitionWarningComponent dst = new ClinicalUseDefinitionWarningComponent(); 2670 copyValues(dst); 2671 return dst; 2672 } 2673 2674 public void copyValues(ClinicalUseDefinitionWarningComponent dst) { 2675 super.copyValues(dst); 2676 dst.description = description == null ? null : description.copy(); 2677 dst.code = code == null ? null : code.copy(); 2678 } 2679 2680 @Override 2681 public boolean equalsDeep(Base other_) { 2682 if (!super.equalsDeep(other_)) 2683 return false; 2684 if (!(other_ instanceof ClinicalUseDefinitionWarningComponent)) 2685 return false; 2686 ClinicalUseDefinitionWarningComponent o = (ClinicalUseDefinitionWarningComponent) other_; 2687 return compareDeep(description, o.description, true) && compareDeep(code, o.code, true); 2688 } 2689 2690 @Override 2691 public boolean equalsShallow(Base other_) { 2692 if (!super.equalsShallow(other_)) 2693 return false; 2694 if (!(other_ instanceof ClinicalUseDefinitionWarningComponent)) 2695 return false; 2696 ClinicalUseDefinitionWarningComponent o = (ClinicalUseDefinitionWarningComponent) other_; 2697 return compareValues(description, o.description, true); 2698 } 2699 2700 public boolean isEmpty() { 2701 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, code); 2702 } 2703 2704 public String fhirType() { 2705 return "ClinicalUseDefinition.warning"; 2706 2707 } 2708 2709 } 2710 2711 /** 2712 * Business identifier for this issue. 2713 */ 2714 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2715 @Description(shortDefinition="Business identifier for this issue", formalDefinition="Business identifier for this issue." ) 2716 protected List<Identifier> identifier; 2717 2718 /** 2719 * indication | contraindication | interaction | undesirable-effect | warning. 2720 */ 2721 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2722 @Description(shortDefinition="indication | contraindication | interaction | undesirable-effect | warning", formalDefinition="indication | contraindication | interaction | undesirable-effect | warning." ) 2723 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-use-definition-type") 2724 protected Enumeration<ClinicalUseDefinitionType> type; 2725 2726 /** 2727 * A categorisation of the issue, primarily for dividing warnings into subject heading areas such as "Pregnancy and Lactation", "Overdose", "Effects on Ability to Drive and Use Machines". 2728 */ 2729 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2730 @Description(shortDefinition="A categorisation of the issue, primarily for dividing warnings into subject heading areas such as \"Pregnancy\", \"Overdose\"", formalDefinition="A categorisation of the issue, primarily for dividing warnings into subject heading areas such as \"Pregnancy and Lactation\", \"Overdose\", \"Effects on Ability to Drive and Use Machines\"." ) 2731 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-use-definition-category") 2732 protected List<CodeableConcept> category; 2733 2734 /** 2735 * The medication, product, substance, device, procedure etc. for which this is an indication. 2736 */ 2737 @Child(name = "subject", type = {MedicinalProductDefinition.class, Medication.class, ActivityDefinition.class, PlanDefinition.class, Device.class, DeviceDefinition.class, Substance.class, NutritionProduct.class, BiologicallyDerivedProduct.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2738 @Description(shortDefinition="The medication, product, substance, device, procedure etc. for which this is an indication", formalDefinition="The medication, product, substance, device, procedure etc. for which this is an indication." ) 2739 protected List<Reference> subject; 2740 2741 /** 2742 * Whether this is a current issue or one that has been retired etc. 2743 */ 2744 @Child(name = "status", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 2745 @Description(shortDefinition="Whether this is a current issue or one that has been retired etc", formalDefinition="Whether this is a current issue or one that has been retired etc." ) 2746 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2747 protected CodeableConcept status; 2748 2749 /** 2750 * Specifics for when this is a contraindication. 2751 */ 2752 @Child(name = "contraindication", type = {}, order=5, min=0, max=1, modifier=false, summary=true) 2753 @Description(shortDefinition="Specifics for when this is a contraindication", formalDefinition="Specifics for when this is a contraindication." ) 2754 protected ClinicalUseDefinitionContraindicationComponent contraindication; 2755 2756 /** 2757 * Specifics for when this is an indication. 2758 */ 2759 @Child(name = "indication", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 2760 @Description(shortDefinition="Specifics for when this is an indication", formalDefinition="Specifics for when this is an indication." ) 2761 protected ClinicalUseDefinitionIndicationComponent indication; 2762 2763 /** 2764 * Specifics for when this is an interaction. 2765 */ 2766 @Child(name = "interaction", type = {}, order=7, min=0, max=1, modifier=false, summary=true) 2767 @Description(shortDefinition="Specifics for when this is an interaction", formalDefinition="Specifics for when this is an interaction." ) 2768 protected ClinicalUseDefinitionInteractionComponent interaction; 2769 2770 /** 2771 * The population group to which this applies. 2772 */ 2773 @Child(name = "population", type = {Group.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2774 @Description(shortDefinition="The population group to which this applies", formalDefinition="The population group to which this applies." ) 2775 protected List<Reference> population; 2776 2777 /** 2778 * Logic used by the clinical use definition. 2779 */ 2780 @Child(name = "library", type = {CanonicalType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2781 @Description(shortDefinition="Logic used by the clinical use definition", formalDefinition="Logic used by the clinical use definition." ) 2782 protected List<CanonicalType> library; 2783 2784 /** 2785 * Describe the possible undesirable effects (negative outcomes) from the use of the medicinal product as treatment. 2786 */ 2787 @Child(name = "undesirableEffect", type = {}, order=10, min=0, max=1, modifier=false, summary=true) 2788 @Description(shortDefinition="A possible negative outcome from the use of this treatment", formalDefinition="Describe the possible undesirable effects (negative outcomes) from the use of the medicinal product as treatment." ) 2789 protected ClinicalUseDefinitionUndesirableEffectComponent undesirableEffect; 2790 2791 /** 2792 * A critical piece of information about environmental, health or physical risks or hazards that serve as caution to the user. For example 'Do not operate heavy machinery', 'May cause drowsiness', or 'Get medical advice/attention if you feel unwell'. 2793 */ 2794 @Child(name = "warning", type = {}, order=11, min=0, max=1, modifier=false, summary=true) 2795 @Description(shortDefinition="Critical environmental, health or physical risks or hazards. For example 'Do not operate heavy machinery', 'May cause drowsiness'", formalDefinition="A critical piece of information about environmental, health or physical risks or hazards that serve as caution to the user. For example 'Do not operate heavy machinery', 'May cause drowsiness', or 'Get medical advice/attention if you feel unwell'." ) 2796 protected ClinicalUseDefinitionWarningComponent warning; 2797 2798 private static final long serialVersionUID = -539149948L; 2799 2800 /** 2801 * Constructor 2802 */ 2803 public ClinicalUseDefinition() { 2804 super(); 2805 } 2806 2807 /** 2808 * Constructor 2809 */ 2810 public ClinicalUseDefinition(ClinicalUseDefinitionType type) { 2811 super(); 2812 this.setType(type); 2813 } 2814 2815 /** 2816 * @return {@link #identifier} (Business identifier for this issue.) 2817 */ 2818 public List<Identifier> getIdentifier() { 2819 if (this.identifier == null) 2820 this.identifier = new ArrayList<Identifier>(); 2821 return this.identifier; 2822 } 2823 2824 /** 2825 * @return Returns a reference to <code>this</code> for easy method chaining 2826 */ 2827 public ClinicalUseDefinition setIdentifier(List<Identifier> theIdentifier) { 2828 this.identifier = theIdentifier; 2829 return this; 2830 } 2831 2832 public boolean hasIdentifier() { 2833 if (this.identifier == null) 2834 return false; 2835 for (Identifier item : this.identifier) 2836 if (!item.isEmpty()) 2837 return true; 2838 return false; 2839 } 2840 2841 public Identifier addIdentifier() { //3 2842 Identifier t = new Identifier(); 2843 if (this.identifier == null) 2844 this.identifier = new ArrayList<Identifier>(); 2845 this.identifier.add(t); 2846 return t; 2847 } 2848 2849 public ClinicalUseDefinition addIdentifier(Identifier t) { //3 2850 if (t == null) 2851 return this; 2852 if (this.identifier == null) 2853 this.identifier = new ArrayList<Identifier>(); 2854 this.identifier.add(t); 2855 return this; 2856 } 2857 2858 /** 2859 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2860 */ 2861 public Identifier getIdentifierFirstRep() { 2862 if (getIdentifier().isEmpty()) { 2863 addIdentifier(); 2864 } 2865 return getIdentifier().get(0); 2866 } 2867 2868 /** 2869 * @return {@link #type} (indication | contraindication | interaction | undesirable-effect | warning.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2870 */ 2871 public Enumeration<ClinicalUseDefinitionType> getTypeElement() { 2872 if (this.type == null) 2873 if (Configuration.errorOnAutoCreate()) 2874 throw new Error("Attempt to auto-create ClinicalUseDefinition.type"); 2875 else if (Configuration.doAutoCreate()) 2876 this.type = new Enumeration<ClinicalUseDefinitionType>(new ClinicalUseDefinitionTypeEnumFactory()); // bb 2877 return this.type; 2878 } 2879 2880 public boolean hasTypeElement() { 2881 return this.type != null && !this.type.isEmpty(); 2882 } 2883 2884 public boolean hasType() { 2885 return this.type != null && !this.type.isEmpty(); 2886 } 2887 2888 /** 2889 * @param value {@link #type} (indication | contraindication | interaction | undesirable-effect | warning.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2890 */ 2891 public ClinicalUseDefinition setTypeElement(Enumeration<ClinicalUseDefinitionType> value) { 2892 this.type = value; 2893 return this; 2894 } 2895 2896 /** 2897 * @return indication | contraindication | interaction | undesirable-effect | warning. 2898 */ 2899 public ClinicalUseDefinitionType getType() { 2900 return this.type == null ? null : this.type.getValue(); 2901 } 2902 2903 /** 2904 * @param value indication | contraindication | interaction | undesirable-effect | warning. 2905 */ 2906 public ClinicalUseDefinition setType(ClinicalUseDefinitionType value) { 2907 if (this.type == null) 2908 this.type = new Enumeration<ClinicalUseDefinitionType>(new ClinicalUseDefinitionTypeEnumFactory()); 2909 this.type.setValue(value); 2910 return this; 2911 } 2912 2913 /** 2914 * @return {@link #category} (A categorisation of the issue, primarily for dividing warnings into subject heading areas such as "Pregnancy and Lactation", "Overdose", "Effects on Ability to Drive and Use Machines".) 2915 */ 2916 public List<CodeableConcept> getCategory() { 2917 if (this.category == null) 2918 this.category = new ArrayList<CodeableConcept>(); 2919 return this.category; 2920 } 2921 2922 /** 2923 * @return Returns a reference to <code>this</code> for easy method chaining 2924 */ 2925 public ClinicalUseDefinition setCategory(List<CodeableConcept> theCategory) { 2926 this.category = theCategory; 2927 return this; 2928 } 2929 2930 public boolean hasCategory() { 2931 if (this.category == null) 2932 return false; 2933 for (CodeableConcept item : this.category) 2934 if (!item.isEmpty()) 2935 return true; 2936 return false; 2937 } 2938 2939 public CodeableConcept addCategory() { //3 2940 CodeableConcept t = new CodeableConcept(); 2941 if (this.category == null) 2942 this.category = new ArrayList<CodeableConcept>(); 2943 this.category.add(t); 2944 return t; 2945 } 2946 2947 public ClinicalUseDefinition addCategory(CodeableConcept t) { //3 2948 if (t == null) 2949 return this; 2950 if (this.category == null) 2951 this.category = new ArrayList<CodeableConcept>(); 2952 this.category.add(t); 2953 return this; 2954 } 2955 2956 /** 2957 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2958 */ 2959 public CodeableConcept getCategoryFirstRep() { 2960 if (getCategory().isEmpty()) { 2961 addCategory(); 2962 } 2963 return getCategory().get(0); 2964 } 2965 2966 /** 2967 * @return {@link #subject} (The medication, product, substance, device, procedure etc. for which this is an indication.) 2968 */ 2969 public List<Reference> getSubject() { 2970 if (this.subject == null) 2971 this.subject = new ArrayList<Reference>(); 2972 return this.subject; 2973 } 2974 2975 /** 2976 * @return Returns a reference to <code>this</code> for easy method chaining 2977 */ 2978 public ClinicalUseDefinition setSubject(List<Reference> theSubject) { 2979 this.subject = theSubject; 2980 return this; 2981 } 2982 2983 public boolean hasSubject() { 2984 if (this.subject == null) 2985 return false; 2986 for (Reference item : this.subject) 2987 if (!item.isEmpty()) 2988 return true; 2989 return false; 2990 } 2991 2992 public Reference addSubject() { //3 2993 Reference t = new Reference(); 2994 if (this.subject == null) 2995 this.subject = new ArrayList<Reference>(); 2996 this.subject.add(t); 2997 return t; 2998 } 2999 3000 public ClinicalUseDefinition addSubject(Reference t) { //3 3001 if (t == null) 3002 return this; 3003 if (this.subject == null) 3004 this.subject = new ArrayList<Reference>(); 3005 this.subject.add(t); 3006 return this; 3007 } 3008 3009 /** 3010 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 3011 */ 3012 public Reference getSubjectFirstRep() { 3013 if (getSubject().isEmpty()) { 3014 addSubject(); 3015 } 3016 return getSubject().get(0); 3017 } 3018 3019 /** 3020 * @return {@link #status} (Whether this is a current issue or one that has been retired etc.) 3021 */ 3022 public CodeableConcept getStatus() { 3023 if (this.status == null) 3024 if (Configuration.errorOnAutoCreate()) 3025 throw new Error("Attempt to auto-create ClinicalUseDefinition.status"); 3026 else if (Configuration.doAutoCreate()) 3027 this.status = new CodeableConcept(); // cc 3028 return this.status; 3029 } 3030 3031 public boolean hasStatus() { 3032 return this.status != null && !this.status.isEmpty(); 3033 } 3034 3035 /** 3036 * @param value {@link #status} (Whether this is a current issue or one that has been retired etc.) 3037 */ 3038 public ClinicalUseDefinition setStatus(CodeableConcept value) { 3039 this.status = value; 3040 return this; 3041 } 3042 3043 /** 3044 * @return {@link #contraindication} (Specifics for when this is a contraindication.) 3045 */ 3046 public ClinicalUseDefinitionContraindicationComponent getContraindication() { 3047 if (this.contraindication == null) 3048 if (Configuration.errorOnAutoCreate()) 3049 throw new Error("Attempt to auto-create ClinicalUseDefinition.contraindication"); 3050 else if (Configuration.doAutoCreate()) 3051 this.contraindication = new ClinicalUseDefinitionContraindicationComponent(); // cc 3052 return this.contraindication; 3053 } 3054 3055 public boolean hasContraindication() { 3056 return this.contraindication != null && !this.contraindication.isEmpty(); 3057 } 3058 3059 /** 3060 * @param value {@link #contraindication} (Specifics for when this is a contraindication.) 3061 */ 3062 public ClinicalUseDefinition setContraindication(ClinicalUseDefinitionContraindicationComponent value) { 3063 this.contraindication = value; 3064 return this; 3065 } 3066 3067 /** 3068 * @return {@link #indication} (Specifics for when this is an indication.) 3069 */ 3070 public ClinicalUseDefinitionIndicationComponent getIndication() { 3071 if (this.indication == null) 3072 if (Configuration.errorOnAutoCreate()) 3073 throw new Error("Attempt to auto-create ClinicalUseDefinition.indication"); 3074 else if (Configuration.doAutoCreate()) 3075 this.indication = new ClinicalUseDefinitionIndicationComponent(); // cc 3076 return this.indication; 3077 } 3078 3079 public boolean hasIndication() { 3080 return this.indication != null && !this.indication.isEmpty(); 3081 } 3082 3083 /** 3084 * @param value {@link #indication} (Specifics for when this is an indication.) 3085 */ 3086 public ClinicalUseDefinition setIndication(ClinicalUseDefinitionIndicationComponent value) { 3087 this.indication = value; 3088 return this; 3089 } 3090 3091 /** 3092 * @return {@link #interaction} (Specifics for when this is an interaction.) 3093 */ 3094 public ClinicalUseDefinitionInteractionComponent getInteraction() { 3095 if (this.interaction == null) 3096 if (Configuration.errorOnAutoCreate()) 3097 throw new Error("Attempt to auto-create ClinicalUseDefinition.interaction"); 3098 else if (Configuration.doAutoCreate()) 3099 this.interaction = new ClinicalUseDefinitionInteractionComponent(); // cc 3100 return this.interaction; 3101 } 3102 3103 public boolean hasInteraction() { 3104 return this.interaction != null && !this.interaction.isEmpty(); 3105 } 3106 3107 /** 3108 * @param value {@link #interaction} (Specifics for when this is an interaction.) 3109 */ 3110 public ClinicalUseDefinition setInteraction(ClinicalUseDefinitionInteractionComponent value) { 3111 this.interaction = value; 3112 return this; 3113 } 3114 3115 /** 3116 * @return {@link #population} (The population group to which this applies.) 3117 */ 3118 public List<Reference> getPopulation() { 3119 if (this.population == null) 3120 this.population = new ArrayList<Reference>(); 3121 return this.population; 3122 } 3123 3124 /** 3125 * @return Returns a reference to <code>this</code> for easy method chaining 3126 */ 3127 public ClinicalUseDefinition setPopulation(List<Reference> thePopulation) { 3128 this.population = thePopulation; 3129 return this; 3130 } 3131 3132 public boolean hasPopulation() { 3133 if (this.population == null) 3134 return false; 3135 for (Reference item : this.population) 3136 if (!item.isEmpty()) 3137 return true; 3138 return false; 3139 } 3140 3141 public Reference addPopulation() { //3 3142 Reference t = new Reference(); 3143 if (this.population == null) 3144 this.population = new ArrayList<Reference>(); 3145 this.population.add(t); 3146 return t; 3147 } 3148 3149 public ClinicalUseDefinition addPopulation(Reference t) { //3 3150 if (t == null) 3151 return this; 3152 if (this.population == null) 3153 this.population = new ArrayList<Reference>(); 3154 this.population.add(t); 3155 return this; 3156 } 3157 3158 /** 3159 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist {3} 3160 */ 3161 public Reference getPopulationFirstRep() { 3162 if (getPopulation().isEmpty()) { 3163 addPopulation(); 3164 } 3165 return getPopulation().get(0); 3166 } 3167 3168 /** 3169 * @return {@link #library} (Logic used by the clinical use definition.) 3170 */ 3171 public List<CanonicalType> getLibrary() { 3172 if (this.library == null) 3173 this.library = new ArrayList<CanonicalType>(); 3174 return this.library; 3175 } 3176 3177 /** 3178 * @return Returns a reference to <code>this</code> for easy method chaining 3179 */ 3180 public ClinicalUseDefinition setLibrary(List<CanonicalType> theLibrary) { 3181 this.library = theLibrary; 3182 return this; 3183 } 3184 3185 public boolean hasLibrary() { 3186 if (this.library == null) 3187 return false; 3188 for (CanonicalType item : this.library) 3189 if (!item.isEmpty()) 3190 return true; 3191 return false; 3192 } 3193 3194 /** 3195 * @return {@link #library} (Logic used by the clinical use definition.) 3196 */ 3197 public CanonicalType addLibraryElement() {//2 3198 CanonicalType t = new CanonicalType(); 3199 if (this.library == null) 3200 this.library = new ArrayList<CanonicalType>(); 3201 this.library.add(t); 3202 return t; 3203 } 3204 3205 /** 3206 * @param value {@link #library} (Logic used by the clinical use definition.) 3207 */ 3208 public ClinicalUseDefinition addLibrary(String value) { //1 3209 CanonicalType t = new CanonicalType(); 3210 t.setValue(value); 3211 if (this.library == null) 3212 this.library = new ArrayList<CanonicalType>(); 3213 this.library.add(t); 3214 return this; 3215 } 3216 3217 /** 3218 * @param value {@link #library} (Logic used by the clinical use definition.) 3219 */ 3220 public boolean hasLibrary(String value) { 3221 if (this.library == null) 3222 return false; 3223 for (CanonicalType v : this.library) 3224 if (v.getValue().equals(value)) // canonical 3225 return true; 3226 return false; 3227 } 3228 3229 /** 3230 * @return {@link #undesirableEffect} (Describe the possible undesirable effects (negative outcomes) from the use of the medicinal product as treatment.) 3231 */ 3232 public ClinicalUseDefinitionUndesirableEffectComponent getUndesirableEffect() { 3233 if (this.undesirableEffect == null) 3234 if (Configuration.errorOnAutoCreate()) 3235 throw new Error("Attempt to auto-create ClinicalUseDefinition.undesirableEffect"); 3236 else if (Configuration.doAutoCreate()) 3237 this.undesirableEffect = new ClinicalUseDefinitionUndesirableEffectComponent(); // cc 3238 return this.undesirableEffect; 3239 } 3240 3241 public boolean hasUndesirableEffect() { 3242 return this.undesirableEffect != null && !this.undesirableEffect.isEmpty(); 3243 } 3244 3245 /** 3246 * @param value {@link #undesirableEffect} (Describe the possible undesirable effects (negative outcomes) from the use of the medicinal product as treatment.) 3247 */ 3248 public ClinicalUseDefinition setUndesirableEffect(ClinicalUseDefinitionUndesirableEffectComponent value) { 3249 this.undesirableEffect = value; 3250 return this; 3251 } 3252 3253 /** 3254 * @return {@link #warning} (A critical piece of information about environmental, health or physical risks or hazards that serve as caution to the user. For example 'Do not operate heavy machinery', 'May cause drowsiness', or 'Get medical advice/attention if you feel unwell'.) 3255 */ 3256 public ClinicalUseDefinitionWarningComponent getWarning() { 3257 if (this.warning == null) 3258 if (Configuration.errorOnAutoCreate()) 3259 throw new Error("Attempt to auto-create ClinicalUseDefinition.warning"); 3260 else if (Configuration.doAutoCreate()) 3261 this.warning = new ClinicalUseDefinitionWarningComponent(); // cc 3262 return this.warning; 3263 } 3264 3265 public boolean hasWarning() { 3266 return this.warning != null && !this.warning.isEmpty(); 3267 } 3268 3269 /** 3270 * @param value {@link #warning} (A critical piece of information about environmental, health or physical risks or hazards that serve as caution to the user. For example 'Do not operate heavy machinery', 'May cause drowsiness', or 'Get medical advice/attention if you feel unwell'.) 3271 */ 3272 public ClinicalUseDefinition setWarning(ClinicalUseDefinitionWarningComponent value) { 3273 this.warning = value; 3274 return this; 3275 } 3276 3277 protected void listChildren(List<Property> children) { 3278 super.listChildren(children); 3279 children.add(new Property("identifier", "Identifier", "Business identifier for this issue.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3280 children.add(new Property("type", "code", "indication | contraindication | interaction | undesirable-effect | warning.", 0, 1, type)); 3281 children.add(new Property("category", "CodeableConcept", "A categorisation of the issue, primarily for dividing warnings into subject heading areas such as \"Pregnancy and Lactation\", \"Overdose\", \"Effects on Ability to Drive and Use Machines\".", 0, java.lang.Integer.MAX_VALUE, category)); 3282 children.add(new Property("subject", "Reference(MedicinalProductDefinition|Medication|ActivityDefinition|PlanDefinition|Device|DeviceDefinition|Substance|NutritionProduct|BiologicallyDerivedProduct)", "The medication, product, substance, device, procedure etc. for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject)); 3283 children.add(new Property("status", "CodeableConcept", "Whether this is a current issue or one that has been retired etc.", 0, 1, status)); 3284 children.add(new Property("contraindication", "", "Specifics for when this is a contraindication.", 0, 1, contraindication)); 3285 children.add(new Property("indication", "", "Specifics for when this is an indication.", 0, 1, indication)); 3286 children.add(new Property("interaction", "", "Specifics for when this is an interaction.", 0, 1, interaction)); 3287 children.add(new Property("population", "Reference(Group)", "The population group to which this applies.", 0, java.lang.Integer.MAX_VALUE, population)); 3288 children.add(new Property("library", "canonical(Library)", "Logic used by the clinical use definition.", 0, java.lang.Integer.MAX_VALUE, library)); 3289 children.add(new Property("undesirableEffect", "", "Describe the possible undesirable effects (negative outcomes) from the use of the medicinal product as treatment.", 0, 1, undesirableEffect)); 3290 children.add(new Property("warning", "", "A critical piece of information about environmental, health or physical risks or hazards that serve as caution to the user. For example 'Do not operate heavy machinery', 'May cause drowsiness', or 'Get medical advice/attention if you feel unwell'.", 0, 1, warning)); 3291 } 3292 3293 @Override 3294 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3295 switch (_hash) { 3296 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this issue.", 0, java.lang.Integer.MAX_VALUE, identifier); 3297 case 3575610: /*type*/ return new Property("type", "code", "indication | contraindication | interaction | undesirable-effect | warning.", 0, 1, type); 3298 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A categorisation of the issue, primarily for dividing warnings into subject heading areas such as \"Pregnancy and Lactation\", \"Overdose\", \"Effects on Ability to Drive and Use Machines\".", 0, java.lang.Integer.MAX_VALUE, category); 3299 case -1867885268: /*subject*/ return new Property("subject", "Reference(MedicinalProductDefinition|Medication|ActivityDefinition|PlanDefinition|Device|DeviceDefinition|Substance|NutritionProduct|BiologicallyDerivedProduct)", "The medication, product, substance, device, procedure etc. for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject); 3300 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Whether this is a current issue or one that has been retired etc.", 0, 1, status); 3301 case 107135229: /*contraindication*/ return new Property("contraindication", "", "Specifics for when this is a contraindication.", 0, 1, contraindication); 3302 case -597168804: /*indication*/ return new Property("indication", "", "Specifics for when this is an indication.", 0, 1, indication); 3303 case 1844104722: /*interaction*/ return new Property("interaction", "", "Specifics for when this is an interaction.", 0, 1, interaction); 3304 case -2023558323: /*population*/ return new Property("population", "Reference(Group)", "The population group to which this applies.", 0, java.lang.Integer.MAX_VALUE, population); 3305 case 166208699: /*library*/ return new Property("library", "canonical(Library)", "Logic used by the clinical use definition.", 0, java.lang.Integer.MAX_VALUE, library); 3306 case 444367565: /*undesirableEffect*/ return new Property("undesirableEffect", "", "Describe the possible undesirable effects (negative outcomes) from the use of the medicinal product as treatment.", 0, 1, undesirableEffect); 3307 case 1124446108: /*warning*/ return new Property("warning", "", "A critical piece of information about environmental, health or physical risks or hazards that serve as caution to the user. For example 'Do not operate heavy machinery', 'May cause drowsiness', or 'Get medical advice/attention if you feel unwell'.", 0, 1, warning); 3308 default: return super.getNamedProperty(_hash, _name, _checkValid); 3309 } 3310 3311 } 3312 3313 @Override 3314 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3315 switch (hash) { 3316 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3317 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ClinicalUseDefinitionType> 3318 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3319 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 3320 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 3321 case 107135229: /*contraindication*/ return this.contraindication == null ? new Base[0] : new Base[] {this.contraindication}; // ClinicalUseDefinitionContraindicationComponent 3322 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : new Base[] {this.indication}; // ClinicalUseDefinitionIndicationComponent 3323 case 1844104722: /*interaction*/ return this.interaction == null ? new Base[0] : new Base[] {this.interaction}; // ClinicalUseDefinitionInteractionComponent 3324 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // Reference 3325 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 3326 case 444367565: /*undesirableEffect*/ return this.undesirableEffect == null ? new Base[0] : new Base[] {this.undesirableEffect}; // ClinicalUseDefinitionUndesirableEffectComponent 3327 case 1124446108: /*warning*/ return this.warning == null ? new Base[0] : new Base[] {this.warning}; // ClinicalUseDefinitionWarningComponent 3328 default: return super.getProperty(hash, name, checkValid); 3329 } 3330 3331 } 3332 3333 @Override 3334 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3335 switch (hash) { 3336 case -1618432855: // identifier 3337 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3338 return value; 3339 case 3575610: // type 3340 value = new ClinicalUseDefinitionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3341 this.type = (Enumeration) value; // Enumeration<ClinicalUseDefinitionType> 3342 return value; 3343 case 50511102: // category 3344 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3345 return value; 3346 case -1867885268: // subject 3347 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 3348 return value; 3349 case -892481550: // status 3350 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3351 return value; 3352 case 107135229: // contraindication 3353 this.contraindication = (ClinicalUseDefinitionContraindicationComponent) value; // ClinicalUseDefinitionContraindicationComponent 3354 return value; 3355 case -597168804: // indication 3356 this.indication = (ClinicalUseDefinitionIndicationComponent) value; // ClinicalUseDefinitionIndicationComponent 3357 return value; 3358 case 1844104722: // interaction 3359 this.interaction = (ClinicalUseDefinitionInteractionComponent) value; // ClinicalUseDefinitionInteractionComponent 3360 return value; 3361 case -2023558323: // population 3362 this.getPopulation().add(TypeConvertor.castToReference(value)); // Reference 3363 return value; 3364 case 166208699: // library 3365 this.getLibrary().add(TypeConvertor.castToCanonical(value)); // CanonicalType 3366 return value; 3367 case 444367565: // undesirableEffect 3368 this.undesirableEffect = (ClinicalUseDefinitionUndesirableEffectComponent) value; // ClinicalUseDefinitionUndesirableEffectComponent 3369 return value; 3370 case 1124446108: // warning 3371 this.warning = (ClinicalUseDefinitionWarningComponent) value; // ClinicalUseDefinitionWarningComponent 3372 return value; 3373 default: return super.setProperty(hash, name, value); 3374 } 3375 3376 } 3377 3378 @Override 3379 public Base setProperty(String name, Base value) throws FHIRException { 3380 if (name.equals("identifier")) { 3381 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3382 } else if (name.equals("type")) { 3383 value = new ClinicalUseDefinitionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3384 this.type = (Enumeration) value; // Enumeration<ClinicalUseDefinitionType> 3385 } else if (name.equals("category")) { 3386 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3387 } else if (name.equals("subject")) { 3388 this.getSubject().add(TypeConvertor.castToReference(value)); 3389 } else if (name.equals("status")) { 3390 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3391 } else if (name.equals("contraindication")) { 3392 this.contraindication = (ClinicalUseDefinitionContraindicationComponent) value; // ClinicalUseDefinitionContraindicationComponent 3393 } else if (name.equals("indication")) { 3394 this.indication = (ClinicalUseDefinitionIndicationComponent) value; // ClinicalUseDefinitionIndicationComponent 3395 } else if (name.equals("interaction")) { 3396 this.interaction = (ClinicalUseDefinitionInteractionComponent) value; // ClinicalUseDefinitionInteractionComponent 3397 } else if (name.equals("population")) { 3398 this.getPopulation().add(TypeConvertor.castToReference(value)); 3399 } else if (name.equals("library")) { 3400 this.getLibrary().add(TypeConvertor.castToCanonical(value)); 3401 } else if (name.equals("undesirableEffect")) { 3402 this.undesirableEffect = (ClinicalUseDefinitionUndesirableEffectComponent) value; // ClinicalUseDefinitionUndesirableEffectComponent 3403 } else if (name.equals("warning")) { 3404 this.warning = (ClinicalUseDefinitionWarningComponent) value; // ClinicalUseDefinitionWarningComponent 3405 } else 3406 return super.setProperty(name, value); 3407 return value; 3408 } 3409 3410 @Override 3411 public void removeChild(String name, Base value) throws FHIRException { 3412 if (name.equals("identifier")) { 3413 this.getIdentifier().remove(value); 3414 } else if (name.equals("type")) { 3415 value = new ClinicalUseDefinitionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3416 this.type = (Enumeration) value; // Enumeration<ClinicalUseDefinitionType> 3417 } else if (name.equals("category")) { 3418 this.getCategory().remove(value); 3419 } else if (name.equals("subject")) { 3420 this.getSubject().remove(value); 3421 } else if (name.equals("status")) { 3422 this.status = null; 3423 } else if (name.equals("contraindication")) { 3424 this.contraindication = (ClinicalUseDefinitionContraindicationComponent) value; // ClinicalUseDefinitionContraindicationComponent 3425 } else if (name.equals("indication")) { 3426 this.indication = (ClinicalUseDefinitionIndicationComponent) value; // ClinicalUseDefinitionIndicationComponent 3427 } else if (name.equals("interaction")) { 3428 this.interaction = (ClinicalUseDefinitionInteractionComponent) value; // ClinicalUseDefinitionInteractionComponent 3429 } else if (name.equals("population")) { 3430 this.getPopulation().remove(value); 3431 } else if (name.equals("library")) { 3432 this.getLibrary().remove(value); 3433 } else if (name.equals("undesirableEffect")) { 3434 this.undesirableEffect = (ClinicalUseDefinitionUndesirableEffectComponent) value; // ClinicalUseDefinitionUndesirableEffectComponent 3435 } else if (name.equals("warning")) { 3436 this.warning = (ClinicalUseDefinitionWarningComponent) value; // ClinicalUseDefinitionWarningComponent 3437 } else 3438 super.removeChild(name, value); 3439 3440 } 3441 3442 @Override 3443 public Base makeProperty(int hash, String name) throws FHIRException { 3444 switch (hash) { 3445 case -1618432855: return addIdentifier(); 3446 case 3575610: return getTypeElement(); 3447 case 50511102: return addCategory(); 3448 case -1867885268: return addSubject(); 3449 case -892481550: return getStatus(); 3450 case 107135229: return getContraindication(); 3451 case -597168804: return getIndication(); 3452 case 1844104722: return getInteraction(); 3453 case -2023558323: return addPopulation(); 3454 case 166208699: return addLibraryElement(); 3455 case 444367565: return getUndesirableEffect(); 3456 case 1124446108: return getWarning(); 3457 default: return super.makeProperty(hash, name); 3458 } 3459 3460 } 3461 3462 @Override 3463 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3464 switch (hash) { 3465 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3466 case 3575610: /*type*/ return new String[] {"code"}; 3467 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3468 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3469 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 3470 case 107135229: /*contraindication*/ return new String[] {}; 3471 case -597168804: /*indication*/ return new String[] {}; 3472 case 1844104722: /*interaction*/ return new String[] {}; 3473 case -2023558323: /*population*/ return new String[] {"Reference"}; 3474 case 166208699: /*library*/ return new String[] {"canonical"}; 3475 case 444367565: /*undesirableEffect*/ return new String[] {}; 3476 case 1124446108: /*warning*/ return new String[] {}; 3477 default: return super.getTypesForProperty(hash, name); 3478 } 3479 3480 } 3481 3482 @Override 3483 public Base addChild(String name) throws FHIRException { 3484 if (name.equals("identifier")) { 3485 return addIdentifier(); 3486 } 3487 else if (name.equals("type")) { 3488 throw new FHIRException("Cannot call addChild on a singleton property ClinicalUseDefinition.type"); 3489 } 3490 else if (name.equals("category")) { 3491 return addCategory(); 3492 } 3493 else if (name.equals("subject")) { 3494 return addSubject(); 3495 } 3496 else if (name.equals("status")) { 3497 this.status = new CodeableConcept(); 3498 return this.status; 3499 } 3500 else if (name.equals("contraindication")) { 3501 this.contraindication = new ClinicalUseDefinitionContraindicationComponent(); 3502 return this.contraindication; 3503 } 3504 else if (name.equals("indication")) { 3505 this.indication = new ClinicalUseDefinitionIndicationComponent(); 3506 return this.indication; 3507 } 3508 else if (name.equals("interaction")) { 3509 this.interaction = new ClinicalUseDefinitionInteractionComponent(); 3510 return this.interaction; 3511 } 3512 else if (name.equals("population")) { 3513 return addPopulation(); 3514 } 3515 else if (name.equals("library")) { 3516 throw new FHIRException("Cannot call addChild on a singleton property ClinicalUseDefinition.library"); 3517 } 3518 else if (name.equals("undesirableEffect")) { 3519 this.undesirableEffect = new ClinicalUseDefinitionUndesirableEffectComponent(); 3520 return this.undesirableEffect; 3521 } 3522 else if (name.equals("warning")) { 3523 this.warning = new ClinicalUseDefinitionWarningComponent(); 3524 return this.warning; 3525 } 3526 else 3527 return super.addChild(name); 3528 } 3529 3530 public String fhirType() { 3531 return "ClinicalUseDefinition"; 3532 3533 } 3534 3535 public ClinicalUseDefinition copy() { 3536 ClinicalUseDefinition dst = new ClinicalUseDefinition(); 3537 copyValues(dst); 3538 return dst; 3539 } 3540 3541 public void copyValues(ClinicalUseDefinition dst) { 3542 super.copyValues(dst); 3543 if (identifier != null) { 3544 dst.identifier = new ArrayList<Identifier>(); 3545 for (Identifier i : identifier) 3546 dst.identifier.add(i.copy()); 3547 }; 3548 dst.type = type == null ? null : type.copy(); 3549 if (category != null) { 3550 dst.category = new ArrayList<CodeableConcept>(); 3551 for (CodeableConcept i : category) 3552 dst.category.add(i.copy()); 3553 }; 3554 if (subject != null) { 3555 dst.subject = new ArrayList<Reference>(); 3556 for (Reference i : subject) 3557 dst.subject.add(i.copy()); 3558 }; 3559 dst.status = status == null ? null : status.copy(); 3560 dst.contraindication = contraindication == null ? null : contraindication.copy(); 3561 dst.indication = indication == null ? null : indication.copy(); 3562 dst.interaction = interaction == null ? null : interaction.copy(); 3563 if (population != null) { 3564 dst.population = new ArrayList<Reference>(); 3565 for (Reference i : population) 3566 dst.population.add(i.copy()); 3567 }; 3568 if (library != null) { 3569 dst.library = new ArrayList<CanonicalType>(); 3570 for (CanonicalType i : library) 3571 dst.library.add(i.copy()); 3572 }; 3573 dst.undesirableEffect = undesirableEffect == null ? null : undesirableEffect.copy(); 3574 dst.warning = warning == null ? null : warning.copy(); 3575 } 3576 3577 protected ClinicalUseDefinition typedCopy() { 3578 return copy(); 3579 } 3580 3581 @Override 3582 public boolean equalsDeep(Base other_) { 3583 if (!super.equalsDeep(other_)) 3584 return false; 3585 if (!(other_ instanceof ClinicalUseDefinition)) 3586 return false; 3587 ClinicalUseDefinition o = (ClinicalUseDefinition) other_; 3588 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 3589 && compareDeep(subject, o.subject, true) && compareDeep(status, o.status, true) && compareDeep(contraindication, o.contraindication, true) 3590 && compareDeep(indication, o.indication, true) && compareDeep(interaction, o.interaction, true) 3591 && compareDeep(population, o.population, true) && compareDeep(library, o.library, true) && compareDeep(undesirableEffect, o.undesirableEffect, true) 3592 && compareDeep(warning, o.warning, true); 3593 } 3594 3595 @Override 3596 public boolean equalsShallow(Base other_) { 3597 if (!super.equalsShallow(other_)) 3598 return false; 3599 if (!(other_ instanceof ClinicalUseDefinition)) 3600 return false; 3601 ClinicalUseDefinition o = (ClinicalUseDefinition) other_; 3602 return compareValues(type, o.type, true) && compareValues(library, o.library, true); 3603 } 3604 3605 public boolean isEmpty() { 3606 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, category 3607 , subject, status, contraindication, indication, interaction, population, library 3608 , undesirableEffect, warning); 3609 } 3610 3611 @Override 3612 public ResourceType getResourceType() { 3613 return ResourceType.ClinicalUseDefinition; 3614 } 3615 3616 /** 3617 * Search parameter: <b>contraindication-reference</b> 3618 * <p> 3619 * Description: <b>The situation that is being documented as contraindicating against this item, as a reference</b><br> 3620 * Type: <b>reference</b><br> 3621 * Path: <b>ClinicalUseDefinition.contraindication.diseaseSymptomProcedure.reference</b><br> 3622 * </p> 3623 */ 3624 @SearchParamDefinition(name="contraindication-reference", path="ClinicalUseDefinition.contraindication.diseaseSymptomProcedure.reference", description="The situation that is being documented as contraindicating against this item, as a reference", type="reference", target={ObservationDefinition.class } ) 3625 public static final String SP_CONTRAINDICATION_REFERENCE = "contraindication-reference"; 3626 /** 3627 * <b>Fluent Client</b> search parameter constant for <b>contraindication-reference</b> 3628 * <p> 3629 * Description: <b>The situation that is being documented as contraindicating against this item, as a reference</b><br> 3630 * Type: <b>reference</b><br> 3631 * Path: <b>ClinicalUseDefinition.contraindication.diseaseSymptomProcedure.reference</b><br> 3632 * </p> 3633 */ 3634 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTRAINDICATION_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTRAINDICATION_REFERENCE); 3635 3636/** 3637 * Constant for fluent queries to be used to add include statements. Specifies 3638 * the path value of "<b>ClinicalUseDefinition:contraindication-reference</b>". 3639 */ 3640 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTRAINDICATION_REFERENCE = new ca.uhn.fhir.model.api.Include("ClinicalUseDefinition:contraindication-reference").toLocked(); 3641 3642 /** 3643 * Search parameter: <b>contraindication</b> 3644 * <p> 3645 * Description: <b>The situation that is being documented as contraindicating against this item, as a code</b><br> 3646 * Type: <b>token</b><br> 3647 * Path: <b>ClinicalUseDefinition.contraindication.diseaseSymptomProcedure.concept</b><br> 3648 * </p> 3649 */ 3650 @SearchParamDefinition(name="contraindication", path="ClinicalUseDefinition.contraindication.diseaseSymptomProcedure.concept", description="The situation that is being documented as contraindicating against this item, as a code", type="token" ) 3651 public static final String SP_CONTRAINDICATION = "contraindication"; 3652 /** 3653 * <b>Fluent Client</b> search parameter constant for <b>contraindication</b> 3654 * <p> 3655 * Description: <b>The situation that is being documented as contraindicating against this item, as a code</b><br> 3656 * Type: <b>token</b><br> 3657 * Path: <b>ClinicalUseDefinition.contraindication.diseaseSymptomProcedure.concept</b><br> 3658 * </p> 3659 */ 3660 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTRAINDICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTRAINDICATION); 3661 3662 /** 3663 * Search parameter: <b>effect-reference</b> 3664 * <p> 3665 * Description: <b>The situation in which the undesirable effect may manifest, as a reference</b><br> 3666 * Type: <b>reference</b><br> 3667 * Path: <b>ClinicalUseDefinition.undesirableEffect.symptomConditionEffect.reference</b><br> 3668 * </p> 3669 */ 3670 @SearchParamDefinition(name="effect-reference", path="ClinicalUseDefinition.undesirableEffect.symptomConditionEffect.reference", description="The situation in which the undesirable effect may manifest, as a reference", type="reference", target={ObservationDefinition.class } ) 3671 public static final String SP_EFFECT_REFERENCE = "effect-reference"; 3672 /** 3673 * <b>Fluent Client</b> search parameter constant for <b>effect-reference</b> 3674 * <p> 3675 * Description: <b>The situation in which the undesirable effect may manifest, as a reference</b><br> 3676 * Type: <b>reference</b><br> 3677 * Path: <b>ClinicalUseDefinition.undesirableEffect.symptomConditionEffect.reference</b><br> 3678 * </p> 3679 */ 3680 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EFFECT_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EFFECT_REFERENCE); 3681 3682/** 3683 * Constant for fluent queries to be used to add include statements. Specifies 3684 * the path value of "<b>ClinicalUseDefinition:effect-reference</b>". 3685 */ 3686 public static final ca.uhn.fhir.model.api.Include INCLUDE_EFFECT_REFERENCE = new ca.uhn.fhir.model.api.Include("ClinicalUseDefinition:effect-reference").toLocked(); 3687 3688 /** 3689 * Search parameter: <b>effect</b> 3690 * <p> 3691 * Description: <b>The situation in which the undesirable effect may manifest, as a code</b><br> 3692 * Type: <b>token</b><br> 3693 * Path: <b>ClinicalUseDefinition.undesirableEffect.symptomConditionEffect.concept</b><br> 3694 * </p> 3695 */ 3696 @SearchParamDefinition(name="effect", path="ClinicalUseDefinition.undesirableEffect.symptomConditionEffect.concept", description="The situation in which the undesirable effect may manifest, as a code", type="token" ) 3697 public static final String SP_EFFECT = "effect"; 3698 /** 3699 * <b>Fluent Client</b> search parameter constant for <b>effect</b> 3700 * <p> 3701 * Description: <b>The situation in which the undesirable effect may manifest, as a code</b><br> 3702 * Type: <b>token</b><br> 3703 * Path: <b>ClinicalUseDefinition.undesirableEffect.symptomConditionEffect.concept</b><br> 3704 * </p> 3705 */ 3706 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EFFECT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EFFECT); 3707 3708 /** 3709 * Search parameter: <b>identifier</b> 3710 * <p> 3711 * Description: <b>Business identifier for this issue</b><br> 3712 * Type: <b>token</b><br> 3713 * Path: <b>ClinicalUseDefinition.identifier</b><br> 3714 * </p> 3715 */ 3716 @SearchParamDefinition(name="identifier", path="ClinicalUseDefinition.identifier", description="Business identifier for this issue", type="token" ) 3717 public static final String SP_IDENTIFIER = "identifier"; 3718 /** 3719 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3720 * <p> 3721 * Description: <b>Business identifier for this issue</b><br> 3722 * Type: <b>token</b><br> 3723 * Path: <b>ClinicalUseDefinition.identifier</b><br> 3724 * </p> 3725 */ 3726 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3727 3728 /** 3729 * Search parameter: <b>indication-reference</b> 3730 * <p> 3731 * Description: <b>The situation that is being documented as an indicaton for this item, as a reference</b><br> 3732 * Type: <b>reference</b><br> 3733 * Path: <b>ClinicalUseDefinition.indication.diseaseSymptomProcedure.reference</b><br> 3734 * </p> 3735 */ 3736 @SearchParamDefinition(name="indication-reference", path="ClinicalUseDefinition.indication.diseaseSymptomProcedure.reference", description="The situation that is being documented as an indicaton for this item, as a reference", type="reference", target={ObservationDefinition.class } ) 3737 public static final String SP_INDICATION_REFERENCE = "indication-reference"; 3738 /** 3739 * <b>Fluent Client</b> search parameter constant for <b>indication-reference</b> 3740 * <p> 3741 * Description: <b>The situation that is being documented as an indicaton for this item, as a reference</b><br> 3742 * Type: <b>reference</b><br> 3743 * Path: <b>ClinicalUseDefinition.indication.diseaseSymptomProcedure.reference</b><br> 3744 * </p> 3745 */ 3746 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INDICATION_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INDICATION_REFERENCE); 3747 3748/** 3749 * Constant for fluent queries to be used to add include statements. Specifies 3750 * the path value of "<b>ClinicalUseDefinition:indication-reference</b>". 3751 */ 3752 public static final ca.uhn.fhir.model.api.Include INCLUDE_INDICATION_REFERENCE = new ca.uhn.fhir.model.api.Include("ClinicalUseDefinition:indication-reference").toLocked(); 3753 3754 /** 3755 * Search parameter: <b>indication</b> 3756 * <p> 3757 * Description: <b>The situation that is being documented as an indicaton for this item, as a code</b><br> 3758 * Type: <b>token</b><br> 3759 * Path: <b>ClinicalUseDefinition.indication.diseaseSymptomProcedure.concept</b><br> 3760 * </p> 3761 */ 3762 @SearchParamDefinition(name="indication", path="ClinicalUseDefinition.indication.diseaseSymptomProcedure.concept", description="The situation that is being documented as an indicaton for this item, as a code", type="token" ) 3763 public static final String SP_INDICATION = "indication"; 3764 /** 3765 * <b>Fluent Client</b> search parameter constant for <b>indication</b> 3766 * <p> 3767 * Description: <b>The situation that is being documented as an indicaton for this item, as a code</b><br> 3768 * Type: <b>token</b><br> 3769 * Path: <b>ClinicalUseDefinition.indication.diseaseSymptomProcedure.concept</b><br> 3770 * </p> 3771 */ 3772 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INDICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INDICATION); 3773 3774 /** 3775 * Search parameter: <b>interaction</b> 3776 * <p> 3777 * Description: <b>The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction</b><br> 3778 * Type: <b>token</b><br> 3779 * Path: <b>ClinicalUseDefinition.interaction.type</b><br> 3780 * </p> 3781 */ 3782 @SearchParamDefinition(name="interaction", path="ClinicalUseDefinition.interaction.type", description="The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction", type="token" ) 3783 public static final String SP_INTERACTION = "interaction"; 3784 /** 3785 * <b>Fluent Client</b> search parameter constant for <b>interaction</b> 3786 * <p> 3787 * Description: <b>The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction</b><br> 3788 * Type: <b>token</b><br> 3789 * Path: <b>ClinicalUseDefinition.interaction.type</b><br> 3790 * </p> 3791 */ 3792 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTERACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTERACTION); 3793 3794 /** 3795 * Search parameter: <b>product</b> 3796 * <p> 3797 * Description: <b>The medicinal product for which this is a clinical usage issue</b><br> 3798 * Type: <b>reference</b><br> 3799 * Path: <b>ClinicalUseDefinition.subject.where(resolve() is MedicinalProductDefinition)</b><br> 3800 * </p> 3801 */ 3802 @SearchParamDefinition(name="product", path="ClinicalUseDefinition.subject.where(resolve() is MedicinalProductDefinition)", description="The medicinal product for which this is a clinical usage issue", type="reference", target={MedicinalProductDefinition.class } ) 3803 public static final String SP_PRODUCT = "product"; 3804 /** 3805 * <b>Fluent Client</b> search parameter constant for <b>product</b> 3806 * <p> 3807 * Description: <b>The medicinal product for which this is a clinical usage issue</b><br> 3808 * Type: <b>reference</b><br> 3809 * Path: <b>ClinicalUseDefinition.subject.where(resolve() is MedicinalProductDefinition)</b><br> 3810 * </p> 3811 */ 3812 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRODUCT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRODUCT); 3813 3814/** 3815 * Constant for fluent queries to be used to add include statements. Specifies 3816 * the path value of "<b>ClinicalUseDefinition:product</b>". 3817 */ 3818 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRODUCT = new ca.uhn.fhir.model.api.Include("ClinicalUseDefinition:product").toLocked(); 3819 3820 /** 3821 * Search parameter: <b>status</b> 3822 * <p> 3823 * Description: <b>Whether this is a current issue or one that has been retired etc.</b><br> 3824 * Type: <b>token</b><br> 3825 * Path: <b>ClinicalUseDefinition.status</b><br> 3826 * </p> 3827 */ 3828 @SearchParamDefinition(name="status", path="ClinicalUseDefinition.status", description="Whether this is a current issue or one that has been retired etc.", type="token" ) 3829 public static final String SP_STATUS = "status"; 3830 /** 3831 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3832 * <p> 3833 * Description: <b>Whether this is a current issue or one that has been retired etc.</b><br> 3834 * Type: <b>token</b><br> 3835 * Path: <b>ClinicalUseDefinition.status</b><br> 3836 * </p> 3837 */ 3838 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3839 3840 /** 3841 * Search parameter: <b>subject</b> 3842 * <p> 3843 * Description: <b>The resource for which this is a clinical usage issue</b><br> 3844 * Type: <b>reference</b><br> 3845 * Path: <b>ClinicalUseDefinition.subject</b><br> 3846 * </p> 3847 */ 3848 @SearchParamDefinition(name="subject", path="ClinicalUseDefinition.subject", description="The resource for which this is a clinical usage issue", type="reference", target={ActivityDefinition.class, BiologicallyDerivedProduct.class, Device.class, DeviceDefinition.class, Medication.class, MedicinalProductDefinition.class, NutritionProduct.class, PlanDefinition.class, Substance.class } ) 3849 public static final String SP_SUBJECT = "subject"; 3850 /** 3851 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3852 * <p> 3853 * Description: <b>The resource for which this is a clinical usage issue</b><br> 3854 * Type: <b>reference</b><br> 3855 * Path: <b>ClinicalUseDefinition.subject</b><br> 3856 * </p> 3857 */ 3858 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3859 3860/** 3861 * Constant for fluent queries to be used to add include statements. Specifies 3862 * the path value of "<b>ClinicalUseDefinition:subject</b>". 3863 */ 3864 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ClinicalUseDefinition:subject").toLocked(); 3865 3866 /** 3867 * Search parameter: <b>type</b> 3868 * <p> 3869 * Description: <b>indication | contraindication | interaction | undesirable-effect | warning</b><br> 3870 * Type: <b>token</b><br> 3871 * Path: <b>ClinicalUseDefinition.type</b><br> 3872 * </p> 3873 */ 3874 @SearchParamDefinition(name="type", path="ClinicalUseDefinition.type", description="indication | contraindication | interaction | undesirable-effect | warning", type="token" ) 3875 public static final String SP_TYPE = "type"; 3876 /** 3877 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3878 * <p> 3879 * Description: <b>indication | contraindication | interaction | undesirable-effect | warning</b><br> 3880 * Type: <b>token</b><br> 3881 * Path: <b>ClinicalUseDefinition.type</b><br> 3882 * </p> 3883 */ 3884 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3885 3886 3887} 3888