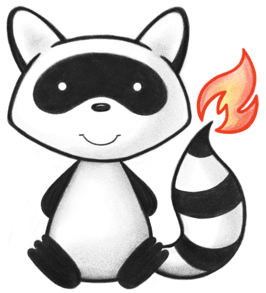
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content. 052 */ 053@ResourceDef(name="CodeSystem", profile="http://hl7.org/fhir/StructureDefinition/CodeSystem") 054public class CodeSystem extends MetadataResource { 055 056 public enum CodeSystemHierarchyMeaning { 057 /** 058 * No particular relationship between the concepts can be assumed, except what can be determined by inspection of the definitions of the elements (possible reasons to use this: importing from a source where this is not defined, or where various parts of the hierarchy have different meanings). 059 */ 060 GROUPEDBY, 061 /** 062 * A hierarchy where the child concepts have an IS-A relationship with the parents - that is, all the properties of the parent are also true for its child concepts. Not that is-a is a property of the concepts, so additional subsumption relationships may be defined using properties. 063 */ 064 ISA, 065 /** 066 * Child elements list the individual parts of a composite whole (e.g. body site). 067 */ 068 PARTOF, 069 /** 070 * Child concepts in the hierarchy may have only one parent, and there is a presumption that the code system is a \"closed world\" meaning all things must be in the hierarchy. This results in concepts such as \"not otherwise classified.\". 071 */ 072 CLASSIFIEDWITH, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static CodeSystemHierarchyMeaning fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("grouped-by".equals(codeString)) 081 return GROUPEDBY; 082 if ("is-a".equals(codeString)) 083 return ISA; 084 if ("part-of".equals(codeString)) 085 return PARTOF; 086 if ("classified-with".equals(codeString)) 087 return CLASSIFIEDWITH; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown CodeSystemHierarchyMeaning code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case GROUPEDBY: return "grouped-by"; 096 case ISA: return "is-a"; 097 case PARTOF: return "part-of"; 098 case CLASSIFIEDWITH: return "classified-with"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case GROUPEDBY: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 106 case ISA: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 107 case PARTOF: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 108 case CLASSIFIEDWITH: return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case GROUPEDBY: return "No particular relationship between the concepts can be assumed, except what can be determined by inspection of the definitions of the elements (possible reasons to use this: importing from a source where this is not defined, or where various parts of the hierarchy have different meanings)."; 116 case ISA: return "A hierarchy where the child concepts have an IS-A relationship with the parents - that is, all the properties of the parent are also true for its child concepts. Not that is-a is a property of the concepts, so additional subsumption relationships may be defined using properties."; 117 case PARTOF: return "Child elements list the individual parts of a composite whole (e.g. body site)."; 118 case CLASSIFIEDWITH: return "Child concepts in the hierarchy may have only one parent, and there is a presumption that the code system is a \"closed world\" meaning all things must be in the hierarchy. This results in concepts such as \"not otherwise classified.\"."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case GROUPEDBY: return "Grouped By"; 126 case ISA: return "Is-A"; 127 case PARTOF: return "Part Of"; 128 case CLASSIFIEDWITH: return "Classified With"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class CodeSystemHierarchyMeaningEnumFactory implements EnumFactory<CodeSystemHierarchyMeaning> { 136 public CodeSystemHierarchyMeaning fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("grouped-by".equals(codeString)) 141 return CodeSystemHierarchyMeaning.GROUPEDBY; 142 if ("is-a".equals(codeString)) 143 return CodeSystemHierarchyMeaning.ISA; 144 if ("part-of".equals(codeString)) 145 return CodeSystemHierarchyMeaning.PARTOF; 146 if ("classified-with".equals(codeString)) 147 return CodeSystemHierarchyMeaning.CLASSIFIEDWITH; 148 throw new IllegalArgumentException("Unknown CodeSystemHierarchyMeaning code '"+codeString+"'"); 149 } 150 public Enumeration<CodeSystemHierarchyMeaning> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.NULL, code); 158 if ("grouped-by".equals(codeString)) 159 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.GROUPEDBY, code); 160 if ("is-a".equals(codeString)) 161 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.ISA, code); 162 if ("part-of".equals(codeString)) 163 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.PARTOF, code); 164 if ("classified-with".equals(codeString)) 165 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.CLASSIFIEDWITH, code); 166 throw new FHIRException("Unknown CodeSystemHierarchyMeaning code '"+codeString+"'"); 167 } 168 public String toCode(CodeSystemHierarchyMeaning code) { 169 if (code == CodeSystemHierarchyMeaning.NULL) 170 return null; 171 if (code == CodeSystemHierarchyMeaning.GROUPEDBY) 172 return "grouped-by"; 173 if (code == CodeSystemHierarchyMeaning.ISA) 174 return "is-a"; 175 if (code == CodeSystemHierarchyMeaning.PARTOF) 176 return "part-of"; 177 if (code == CodeSystemHierarchyMeaning.CLASSIFIEDWITH) 178 return "classified-with"; 179 return "?"; 180 } 181 public String toSystem(CodeSystemHierarchyMeaning code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum PropertyType { 187 /** 188 * The property value is a code that identifies a concept defined in the code system. 189 */ 190 CODE, 191 /** 192 * The property value is a code defined in an external code system. This may be used for translations, but is not the intent. 193 */ 194 CODING, 195 /** 196 * The property value is a string. 197 */ 198 STRING, 199 /** 200 * The property value is an integer (often used to assign ranking values to concepts for supporting score assessments). 201 */ 202 INTEGER, 203 /** 204 * The property value is a boolean true | false. 205 */ 206 BOOLEAN, 207 /** 208 * The property is a date or a date + time. 209 */ 210 DATETIME, 211 /** 212 * The property value is a decimal number. 213 */ 214 DECIMAL, 215 /** 216 * added to help the parsers with the generic types 217 */ 218 NULL; 219 public static PropertyType fromCode(String codeString) throws FHIRException { 220 if (codeString == null || "".equals(codeString)) 221 return null; 222 if ("code".equals(codeString)) 223 return CODE; 224 if ("Coding".equals(codeString)) 225 return CODING; 226 if ("string".equals(codeString)) 227 return STRING; 228 if ("integer".equals(codeString)) 229 return INTEGER; 230 if ("boolean".equals(codeString)) 231 return BOOLEAN; 232 if ("dateTime".equals(codeString)) 233 return DATETIME; 234 if ("decimal".equals(codeString)) 235 return DECIMAL; 236 if (Configuration.isAcceptInvalidEnums()) 237 return null; 238 else 239 throw new FHIRException("Unknown PropertyType code '"+codeString+"'"); 240 } 241 public String toCode() { 242 switch (this) { 243 case CODE: return "code"; 244 case CODING: return "Coding"; 245 case STRING: return "string"; 246 case INTEGER: return "integer"; 247 case BOOLEAN: return "boolean"; 248 case DATETIME: return "dateTime"; 249 case DECIMAL: return "decimal"; 250 case NULL: return null; 251 default: return "?"; 252 } 253 } 254 public String getSystem() { 255 switch (this) { 256 case CODE: return "http://hl7.org/fhir/concept-property-type"; 257 case CODING: return "http://hl7.org/fhir/concept-property-type"; 258 case STRING: return "http://hl7.org/fhir/concept-property-type"; 259 case INTEGER: return "http://hl7.org/fhir/concept-property-type"; 260 case BOOLEAN: return "http://hl7.org/fhir/concept-property-type"; 261 case DATETIME: return "http://hl7.org/fhir/concept-property-type"; 262 case DECIMAL: return "http://hl7.org/fhir/concept-property-type"; 263 case NULL: return null; 264 default: return "?"; 265 } 266 } 267 public String getDefinition() { 268 switch (this) { 269 case CODE: return "The property value is a code that identifies a concept defined in the code system."; 270 case CODING: return "The property value is a code defined in an external code system. This may be used for translations, but is not the intent."; 271 case STRING: return "The property value is a string."; 272 case INTEGER: return "The property value is an integer (often used to assign ranking values to concepts for supporting score assessments)."; 273 case BOOLEAN: return "The property value is a boolean true | false."; 274 case DATETIME: return "The property is a date or a date + time."; 275 case DECIMAL: return "The property value is a decimal number."; 276 case NULL: return null; 277 default: return "?"; 278 } 279 } 280 public String getDisplay() { 281 switch (this) { 282 case CODE: return "code (internal reference)"; 283 case CODING: return "Coding (external reference)"; 284 case STRING: return "string"; 285 case INTEGER: return "integer"; 286 case BOOLEAN: return "boolean"; 287 case DATETIME: return "dateTime"; 288 case DECIMAL: return "decimal"; 289 case NULL: return null; 290 default: return "?"; 291 } 292 } 293 } 294 295 public static class PropertyTypeEnumFactory implements EnumFactory<PropertyType> { 296 public PropertyType fromCode(String codeString) throws IllegalArgumentException { 297 if (codeString == null || "".equals(codeString)) 298 if (codeString == null || "".equals(codeString)) 299 return null; 300 if ("code".equals(codeString)) 301 return PropertyType.CODE; 302 if ("Coding".equals(codeString)) 303 return PropertyType.CODING; 304 if ("string".equals(codeString)) 305 return PropertyType.STRING; 306 if ("integer".equals(codeString)) 307 return PropertyType.INTEGER; 308 if ("boolean".equals(codeString)) 309 return PropertyType.BOOLEAN; 310 if ("dateTime".equals(codeString)) 311 return PropertyType.DATETIME; 312 if ("decimal".equals(codeString)) 313 return PropertyType.DECIMAL; 314 throw new IllegalArgumentException("Unknown PropertyType code '"+codeString+"'"); 315 } 316 public Enumeration<PropertyType> fromType(PrimitiveType<?> code) throws FHIRException { 317 if (code == null) 318 return null; 319 if (code.isEmpty()) 320 return new Enumeration<PropertyType>(this, PropertyType.NULL, code); 321 String codeString = ((PrimitiveType) code).asStringValue(); 322 if (codeString == null || "".equals(codeString)) 323 return new Enumeration<PropertyType>(this, PropertyType.NULL, code); 324 if ("code".equals(codeString)) 325 return new Enumeration<PropertyType>(this, PropertyType.CODE, code); 326 if ("Coding".equals(codeString)) 327 return new Enumeration<PropertyType>(this, PropertyType.CODING, code); 328 if ("string".equals(codeString)) 329 return new Enumeration<PropertyType>(this, PropertyType.STRING, code); 330 if ("integer".equals(codeString)) 331 return new Enumeration<PropertyType>(this, PropertyType.INTEGER, code); 332 if ("boolean".equals(codeString)) 333 return new Enumeration<PropertyType>(this, PropertyType.BOOLEAN, code); 334 if ("dateTime".equals(codeString)) 335 return new Enumeration<PropertyType>(this, PropertyType.DATETIME, code); 336 if ("decimal".equals(codeString)) 337 return new Enumeration<PropertyType>(this, PropertyType.DECIMAL, code); 338 throw new FHIRException("Unknown PropertyType code '"+codeString+"'"); 339 } 340 public String toCode(PropertyType code) { 341 if (code == PropertyType.NULL) 342 return null; 343 if (code == PropertyType.CODE) 344 return "code"; 345 if (code == PropertyType.CODING) 346 return "Coding"; 347 if (code == PropertyType.STRING) 348 return "string"; 349 if (code == PropertyType.INTEGER) 350 return "integer"; 351 if (code == PropertyType.BOOLEAN) 352 return "boolean"; 353 if (code == PropertyType.DATETIME) 354 return "dateTime"; 355 if (code == PropertyType.DECIMAL) 356 return "decimal"; 357 return "?"; 358 } 359 public String toSystem(PropertyType code) { 360 return code.getSystem(); 361 } 362 } 363 364 @Block() 365 public static class CodeSystemFilterComponent extends BackboneElement implements IBaseBackboneElement { 366 /** 367 * The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter. 368 */ 369 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 370 @Description(shortDefinition="Code that identifies the filter", formalDefinition="The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter." ) 371 protected CodeType code; 372 373 /** 374 * A description of how or why the filter is used. 375 */ 376 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 377 @Description(shortDefinition="How or why the filter is used", formalDefinition="A description of how or why the filter is used." ) 378 protected StringType description; 379 380 /** 381 * A list of operators that can be used with the filter. 382 */ 383 @Child(name = "operator", type = {CodeType.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 384 @Description(shortDefinition="= | is-a | descendent-of | is-not-a | regex | in | not-in | generalizes | child-of | descendent-leaf | exists", formalDefinition="A list of operators that can be used with the filter." ) 385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/filter-operator") 386 protected List<Enumeration<FilterOperator>> operator; 387 388 /** 389 * A description of what the value for the filter should be. 390 */ 391 @Child(name = "value", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 392 @Description(shortDefinition="What to use for the value", formalDefinition="A description of what the value for the filter should be." ) 393 protected StringType value; 394 395 private static final long serialVersionUID = -1087409836L; 396 397 /** 398 * Constructor 399 */ 400 public CodeSystemFilterComponent() { 401 super(); 402 } 403 404 /** 405 * Constructor 406 */ 407 public CodeSystemFilterComponent(String code, FilterOperator operator, String value) { 408 super(); 409 this.setCode(code); 410 this.addOperator(operator); 411 this.setValue(value); 412 } 413 414 /** 415 * @return {@link #code} (The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 416 */ 417 public CodeType getCodeElement() { 418 if (this.code == null) 419 if (Configuration.errorOnAutoCreate()) 420 throw new Error("Attempt to auto-create CodeSystemFilterComponent.code"); 421 else if (Configuration.doAutoCreate()) 422 this.code = new CodeType(); // bb 423 return this.code; 424 } 425 426 public boolean hasCodeElement() { 427 return this.code != null && !this.code.isEmpty(); 428 } 429 430 public boolean hasCode() { 431 return this.code != null && !this.code.isEmpty(); 432 } 433 434 /** 435 * @param value {@link #code} (The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 436 */ 437 public CodeSystemFilterComponent setCodeElement(CodeType value) { 438 this.code = value; 439 return this; 440 } 441 442 /** 443 * @return The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter. 444 */ 445 public String getCode() { 446 return this.code == null ? null : this.code.getValue(); 447 } 448 449 /** 450 * @param value The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter. 451 */ 452 public CodeSystemFilterComponent setCode(String value) { 453 if (this.code == null) 454 this.code = new CodeType(); 455 this.code.setValue(value); 456 return this; 457 } 458 459 /** 460 * @return {@link #description} (A description of how or why the filter is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 461 */ 462 public StringType getDescriptionElement() { 463 if (this.description == null) 464 if (Configuration.errorOnAutoCreate()) 465 throw new Error("Attempt to auto-create CodeSystemFilterComponent.description"); 466 else if (Configuration.doAutoCreate()) 467 this.description = new StringType(); // bb 468 return this.description; 469 } 470 471 public boolean hasDescriptionElement() { 472 return this.description != null && !this.description.isEmpty(); 473 } 474 475 public boolean hasDescription() { 476 return this.description != null && !this.description.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #description} (A description of how or why the filter is used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 481 */ 482 public CodeSystemFilterComponent setDescriptionElement(StringType value) { 483 this.description = value; 484 return this; 485 } 486 487 /** 488 * @return A description of how or why the filter is used. 489 */ 490 public String getDescription() { 491 return this.description == null ? null : this.description.getValue(); 492 } 493 494 /** 495 * @param value A description of how or why the filter is used. 496 */ 497 public CodeSystemFilterComponent setDescription(String value) { 498 if (Utilities.noString(value)) 499 this.description = null; 500 else { 501 if (this.description == null) 502 this.description = new StringType(); 503 this.description.setValue(value); 504 } 505 return this; 506 } 507 508 /** 509 * @return {@link #operator} (A list of operators that can be used with the filter.) 510 */ 511 public List<Enumeration<FilterOperator>> getOperator() { 512 if (this.operator == null) 513 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 514 return this.operator; 515 } 516 517 /** 518 * @return Returns a reference to <code>this</code> for easy method chaining 519 */ 520 public CodeSystemFilterComponent setOperator(List<Enumeration<FilterOperator>> theOperator) { 521 this.operator = theOperator; 522 return this; 523 } 524 525 public boolean hasOperator() { 526 if (this.operator == null) 527 return false; 528 for (Enumeration<FilterOperator> item : this.operator) 529 if (!item.isEmpty()) 530 return true; 531 return false; 532 } 533 534 /** 535 * @return {@link #operator} (A list of operators that can be used with the filter.) 536 */ 537 public Enumeration<FilterOperator> addOperatorElement() {//2 538 Enumeration<FilterOperator> t = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 539 if (this.operator == null) 540 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 541 this.operator.add(t); 542 return t; 543 } 544 545 /** 546 * @param value {@link #operator} (A list of operators that can be used with the filter.) 547 */ 548 public CodeSystemFilterComponent addOperator(FilterOperator value) { //1 549 Enumeration<FilterOperator> t = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 550 t.setValue(value); 551 if (this.operator == null) 552 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 553 this.operator.add(t); 554 return this; 555 } 556 557 /** 558 * @param value {@link #operator} (A list of operators that can be used with the filter.) 559 */ 560 public boolean hasOperator(FilterOperator value) { 561 if (this.operator == null) 562 return false; 563 for (Enumeration<FilterOperator> v : this.operator) 564 if (v.getValue().equals(value)) // code 565 return true; 566 return false; 567 } 568 569 /** 570 * @return {@link #value} (A description of what the value for the filter should be.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 571 */ 572 public StringType getValueElement() { 573 if (this.value == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create CodeSystemFilterComponent.value"); 576 else if (Configuration.doAutoCreate()) 577 this.value = new StringType(); // bb 578 return this.value; 579 } 580 581 public boolean hasValueElement() { 582 return this.value != null && !this.value.isEmpty(); 583 } 584 585 public boolean hasValue() { 586 return this.value != null && !this.value.isEmpty(); 587 } 588 589 /** 590 * @param value {@link #value} (A description of what the value for the filter should be.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 591 */ 592 public CodeSystemFilterComponent setValueElement(StringType value) { 593 this.value = value; 594 return this; 595 } 596 597 /** 598 * @return A description of what the value for the filter should be. 599 */ 600 public String getValue() { 601 return this.value == null ? null : this.value.getValue(); 602 } 603 604 /** 605 * @param value A description of what the value for the filter should be. 606 */ 607 public CodeSystemFilterComponent setValue(String value) { 608 if (this.value == null) 609 this.value = new StringType(); 610 this.value.setValue(value); 611 return this; 612 } 613 614 protected void listChildren(List<Property> children) { 615 super.listChildren(children); 616 children.add(new Property("code", "code", "The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter.", 0, 1, code)); 617 children.add(new Property("description", "string", "A description of how or why the filter is used.", 0, 1, description)); 618 children.add(new Property("operator", "code", "A list of operators that can be used with the filter.", 0, java.lang.Integer.MAX_VALUE, operator)); 619 children.add(new Property("value", "string", "A description of what the value for the filter should be.", 0, 1, value)); 620 } 621 622 @Override 623 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 624 switch (_hash) { 625 case 3059181: /*code*/ return new Property("code", "code", "The code that identifies this filter when it is used as a filter in [ValueSet](valueset.html#).compose.include.filter.", 0, 1, code); 626 case -1724546052: /*description*/ return new Property("description", "string", "A description of how or why the filter is used.", 0, 1, description); 627 case -500553564: /*operator*/ return new Property("operator", "code", "A list of operators that can be used with the filter.", 0, java.lang.Integer.MAX_VALUE, operator); 628 case 111972721: /*value*/ return new Property("value", "string", "A description of what the value for the filter should be.", 0, 1, value); 629 default: return super.getNamedProperty(_hash, _name, _checkValid); 630 } 631 632 } 633 634 @Override 635 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 636 switch (hash) { 637 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 638 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 639 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : this.operator.toArray(new Base[this.operator.size()]); // Enumeration<FilterOperator> 640 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 641 default: return super.getProperty(hash, name, checkValid); 642 } 643 644 } 645 646 @Override 647 public Base setProperty(int hash, String name, Base value) throws FHIRException { 648 switch (hash) { 649 case 3059181: // code 650 this.code = TypeConvertor.castToCode(value); // CodeType 651 return value; 652 case -1724546052: // description 653 this.description = TypeConvertor.castToString(value); // StringType 654 return value; 655 case -500553564: // operator 656 value = new FilterOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 657 this.getOperator().add((Enumeration) value); // Enumeration<FilterOperator> 658 return value; 659 case 111972721: // value 660 this.value = TypeConvertor.castToString(value); // StringType 661 return value; 662 default: return super.setProperty(hash, name, value); 663 } 664 665 } 666 667 @Override 668 public Base setProperty(String name, Base value) throws FHIRException { 669 if (name.equals("code")) { 670 this.code = TypeConvertor.castToCode(value); // CodeType 671 } else if (name.equals("description")) { 672 this.description = TypeConvertor.castToString(value); // StringType 673 } else if (name.equals("operator")) { 674 value = new FilterOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 675 this.getOperator().add((Enumeration) value); 676 } else if (name.equals("value")) { 677 this.value = TypeConvertor.castToString(value); // StringType 678 } else 679 return super.setProperty(name, value); 680 return value; 681 } 682 683 @Override 684 public void removeChild(String name, Base value) throws FHIRException { 685 if (name.equals("code")) { 686 this.code = null; 687 } else if (name.equals("description")) { 688 this.description = null; 689 } else if (name.equals("operator")) { 690 value = new FilterOperatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 691 this.getOperator().remove((Enumeration) value); 692 } else if (name.equals("value")) { 693 this.value = null; 694 } else 695 super.removeChild(name, value); 696 697 } 698 699 @Override 700 public Base makeProperty(int hash, String name) throws FHIRException { 701 switch (hash) { 702 case 3059181: return getCodeElement(); 703 case -1724546052: return getDescriptionElement(); 704 case -500553564: return addOperatorElement(); 705 case 111972721: return getValueElement(); 706 default: return super.makeProperty(hash, name); 707 } 708 709 } 710 711 @Override 712 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 713 switch (hash) { 714 case 3059181: /*code*/ return new String[] {"code"}; 715 case -1724546052: /*description*/ return new String[] {"string"}; 716 case -500553564: /*operator*/ return new String[] {"code"}; 717 case 111972721: /*value*/ return new String[] {"string"}; 718 default: return super.getTypesForProperty(hash, name); 719 } 720 721 } 722 723 @Override 724 public Base addChild(String name) throws FHIRException { 725 if (name.equals("code")) { 726 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.filter.code"); 727 } 728 else if (name.equals("description")) { 729 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.filter.description"); 730 } 731 else if (name.equals("operator")) { 732 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.filter.operator"); 733 } 734 else if (name.equals("value")) { 735 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.filter.value"); 736 } 737 else 738 return super.addChild(name); 739 } 740 741 public CodeSystemFilterComponent copy() { 742 CodeSystemFilterComponent dst = new CodeSystemFilterComponent(); 743 copyValues(dst); 744 return dst; 745 } 746 747 public void copyValues(CodeSystemFilterComponent dst) { 748 super.copyValues(dst); 749 dst.code = code == null ? null : code.copy(); 750 dst.description = description == null ? null : description.copy(); 751 if (operator != null) { 752 dst.operator = new ArrayList<Enumeration<FilterOperator>>(); 753 for (Enumeration<FilterOperator> i : operator) 754 dst.operator.add(i.copy()); 755 }; 756 dst.value = value == null ? null : value.copy(); 757 } 758 759 @Override 760 public boolean equalsDeep(Base other_) { 761 if (!super.equalsDeep(other_)) 762 return false; 763 if (!(other_ instanceof CodeSystemFilterComponent)) 764 return false; 765 CodeSystemFilterComponent o = (CodeSystemFilterComponent) other_; 766 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) && compareDeep(operator, o.operator, true) 767 && compareDeep(value, o.value, true); 768 } 769 770 @Override 771 public boolean equalsShallow(Base other_) { 772 if (!super.equalsShallow(other_)) 773 return false; 774 if (!(other_ instanceof CodeSystemFilterComponent)) 775 return false; 776 CodeSystemFilterComponent o = (CodeSystemFilterComponent) other_; 777 return compareValues(code, o.code, true) && compareValues(description, o.description, true) && compareValues(operator, o.operator, true) 778 && compareValues(value, o.value, true); 779 } 780 781 public boolean isEmpty() { 782 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, operator 783 , value); 784 } 785 786 public String fhirType() { 787 return "CodeSystem.filter"; 788 789 } 790 791 } 792 793 @Block() 794 public static class PropertyComponent extends BackboneElement implements IBaseBackboneElement { 795 /** 796 * A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters. 797 */ 798 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 799 @Description(shortDefinition="Identifies the property on the concepts, and when referred to in operations", formalDefinition="A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters." ) 800 protected CodeType code; 801 802 /** 803 * Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 804 */ 805 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 806 @Description(shortDefinition="Formal identifier for the property", formalDefinition="Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system." ) 807 protected UriType uri; 808 809 /** 810 * A description of the property- why it is defined, and how its value might be used. 811 */ 812 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 813 @Description(shortDefinition="Why the property is defined, and/or what it conveys", formalDefinition="A description of the property- why it is defined, and how its value might be used." ) 814 protected StringType description; 815 816 /** 817 * The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to another defined concept). 818 */ 819 @Child(name = "type", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 820 @Description(shortDefinition="code | Coding | string | integer | boolean | dateTime | decimal", formalDefinition="The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to another defined concept)." ) 821 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concept-property-type") 822 protected Enumeration<PropertyType> type; 823 824 private static final long serialVersionUID = -1810713373L; 825 826 /** 827 * Constructor 828 */ 829 public PropertyComponent() { 830 super(); 831 } 832 833 /** 834 * Constructor 835 */ 836 public PropertyComponent(String code, PropertyType type) { 837 super(); 838 this.setCode(code); 839 this.setType(type); 840 } 841 842 /** 843 * @return {@link #code} (A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 844 */ 845 public CodeType getCodeElement() { 846 if (this.code == null) 847 if (Configuration.errorOnAutoCreate()) 848 throw new Error("Attempt to auto-create PropertyComponent.code"); 849 else if (Configuration.doAutoCreate()) 850 this.code = new CodeType(); // bb 851 return this.code; 852 } 853 854 public boolean hasCodeElement() { 855 return this.code != null && !this.code.isEmpty(); 856 } 857 858 public boolean hasCode() { 859 return this.code != null && !this.code.isEmpty(); 860 } 861 862 /** 863 * @param value {@link #code} (A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 864 */ 865 public PropertyComponent setCodeElement(CodeType value) { 866 this.code = value; 867 return this; 868 } 869 870 /** 871 * @return A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters. 872 */ 873 public String getCode() { 874 return this.code == null ? null : this.code.getValue(); 875 } 876 877 /** 878 * @param value A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters. 879 */ 880 public PropertyComponent setCode(String value) { 881 if (this.code == null) 882 this.code = new CodeType(); 883 this.code.setValue(value); 884 return this; 885 } 886 887 /** 888 * @return {@link #uri} (Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 889 */ 890 public UriType getUriElement() { 891 if (this.uri == null) 892 if (Configuration.errorOnAutoCreate()) 893 throw new Error("Attempt to auto-create PropertyComponent.uri"); 894 else if (Configuration.doAutoCreate()) 895 this.uri = new UriType(); // bb 896 return this.uri; 897 } 898 899 public boolean hasUriElement() { 900 return this.uri != null && !this.uri.isEmpty(); 901 } 902 903 public boolean hasUri() { 904 return this.uri != null && !this.uri.isEmpty(); 905 } 906 907 /** 908 * @param value {@link #uri} (Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 909 */ 910 public PropertyComponent setUriElement(UriType value) { 911 this.uri = value; 912 return this; 913 } 914 915 /** 916 * @return Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 917 */ 918 public String getUri() { 919 return this.uri == null ? null : this.uri.getValue(); 920 } 921 922 /** 923 * @param value Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system. 924 */ 925 public PropertyComponent setUri(String value) { 926 if (Utilities.noString(value)) 927 this.uri = null; 928 else { 929 if (this.uri == null) 930 this.uri = new UriType(); 931 this.uri.setValue(value); 932 } 933 return this; 934 } 935 936 /** 937 * @return {@link #description} (A description of the property- why it is defined, and how its value might be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 938 */ 939 public StringType getDescriptionElement() { 940 if (this.description == null) 941 if (Configuration.errorOnAutoCreate()) 942 throw new Error("Attempt to auto-create PropertyComponent.description"); 943 else if (Configuration.doAutoCreate()) 944 this.description = new StringType(); // bb 945 return this.description; 946 } 947 948 public boolean hasDescriptionElement() { 949 return this.description != null && !this.description.isEmpty(); 950 } 951 952 public boolean hasDescription() { 953 return this.description != null && !this.description.isEmpty(); 954 } 955 956 /** 957 * @param value {@link #description} (A description of the property- why it is defined, and how its value might be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 958 */ 959 public PropertyComponent setDescriptionElement(StringType value) { 960 this.description = value; 961 return this; 962 } 963 964 /** 965 * @return A description of the property- why it is defined, and how its value might be used. 966 */ 967 public String getDescription() { 968 return this.description == null ? null : this.description.getValue(); 969 } 970 971 /** 972 * @param value A description of the property- why it is defined, and how its value might be used. 973 */ 974 public PropertyComponent setDescription(String value) { 975 if (Utilities.noString(value)) 976 this.description = null; 977 else { 978 if (this.description == null) 979 this.description = new StringType(); 980 this.description.setValue(value); 981 } 982 return this; 983 } 984 985 /** 986 * @return {@link #type} (The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to another defined concept).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 987 */ 988 public Enumeration<PropertyType> getTypeElement() { 989 if (this.type == null) 990 if (Configuration.errorOnAutoCreate()) 991 throw new Error("Attempt to auto-create PropertyComponent.type"); 992 else if (Configuration.doAutoCreate()) 993 this.type = new Enumeration<PropertyType>(new PropertyTypeEnumFactory()); // bb 994 return this.type; 995 } 996 997 public boolean hasTypeElement() { 998 return this.type != null && !this.type.isEmpty(); 999 } 1000 1001 public boolean hasType() { 1002 return this.type != null && !this.type.isEmpty(); 1003 } 1004 1005 /** 1006 * @param value {@link #type} (The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to another defined concept).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1007 */ 1008 public PropertyComponent setTypeElement(Enumeration<PropertyType> value) { 1009 this.type = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to another defined concept). 1015 */ 1016 public PropertyType getType() { 1017 return this.type == null ? null : this.type.getValue(); 1018 } 1019 1020 /** 1021 * @param value The type of the property value. Properties of type "code" contain a code defined by the code system (e.g. a reference to another defined concept). 1022 */ 1023 public PropertyComponent setType(PropertyType value) { 1024 if (this.type == null) 1025 this.type = new Enumeration<PropertyType>(new PropertyTypeEnumFactory()); 1026 this.type.setValue(value); 1027 return this; 1028 } 1029 1030 protected void listChildren(List<Property> children) { 1031 super.listChildren(children); 1032 children.add(new Property("code", "code", "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.", 0, 1, code)); 1033 children.add(new Property("uri", "uri", "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 0, 1, uri)); 1034 children.add(new Property("description", "string", "A description of the property- why it is defined, and how its value might be used.", 0, 1, description)); 1035 children.add(new Property("type", "code", "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to another defined concept).", 0, 1, type)); 1036 } 1037 1038 @Override 1039 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1040 switch (_hash) { 1041 case 3059181: /*code*/ return new Property("code", "code", "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.", 0, 1, code); 1042 case 116076: /*uri*/ return new Property("uri", "uri", "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 0, 1, uri); 1043 case -1724546052: /*description*/ return new Property("description", "string", "A description of the property- why it is defined, and how its value might be used.", 0, 1, description); 1044 case 3575610: /*type*/ return new Property("type", "code", "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to another defined concept).", 0, 1, type); 1045 default: return super.getNamedProperty(_hash, _name, _checkValid); 1046 } 1047 1048 } 1049 1050 @Override 1051 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1052 switch (hash) { 1053 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1054 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 1055 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1056 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<PropertyType> 1057 default: return super.getProperty(hash, name, checkValid); 1058 } 1059 1060 } 1061 1062 @Override 1063 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1064 switch (hash) { 1065 case 3059181: // code 1066 this.code = TypeConvertor.castToCode(value); // CodeType 1067 return value; 1068 case 116076: // uri 1069 this.uri = TypeConvertor.castToUri(value); // UriType 1070 return value; 1071 case -1724546052: // description 1072 this.description = TypeConvertor.castToString(value); // StringType 1073 return value; 1074 case 3575610: // type 1075 value = new PropertyTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1076 this.type = (Enumeration) value; // Enumeration<PropertyType> 1077 return value; 1078 default: return super.setProperty(hash, name, value); 1079 } 1080 1081 } 1082 1083 @Override 1084 public Base setProperty(String name, Base value) throws FHIRException { 1085 if (name.equals("code")) { 1086 this.code = TypeConvertor.castToCode(value); // CodeType 1087 } else if (name.equals("uri")) { 1088 this.uri = TypeConvertor.castToUri(value); // UriType 1089 } else if (name.equals("description")) { 1090 this.description = TypeConvertor.castToString(value); // StringType 1091 } else if (name.equals("type")) { 1092 value = new PropertyTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1093 this.type = (Enumeration) value; // Enumeration<PropertyType> 1094 } else 1095 return super.setProperty(name, value); 1096 return value; 1097 } 1098 1099 @Override 1100 public void removeChild(String name, Base value) throws FHIRException { 1101 if (name.equals("code")) { 1102 this.code = null; 1103 } else if (name.equals("uri")) { 1104 this.uri = null; 1105 } else if (name.equals("description")) { 1106 this.description = null; 1107 } else if (name.equals("type")) { 1108 value = new PropertyTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1109 this.type = (Enumeration) value; // Enumeration<PropertyType> 1110 } else 1111 super.removeChild(name, value); 1112 1113 } 1114 1115 @Override 1116 public Base makeProperty(int hash, String name) throws FHIRException { 1117 switch (hash) { 1118 case 3059181: return getCodeElement(); 1119 case 116076: return getUriElement(); 1120 case -1724546052: return getDescriptionElement(); 1121 case 3575610: return getTypeElement(); 1122 default: return super.makeProperty(hash, name); 1123 } 1124 1125 } 1126 1127 @Override 1128 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1129 switch (hash) { 1130 case 3059181: /*code*/ return new String[] {"code"}; 1131 case 116076: /*uri*/ return new String[] {"uri"}; 1132 case -1724546052: /*description*/ return new String[] {"string"}; 1133 case 3575610: /*type*/ return new String[] {"code"}; 1134 default: return super.getTypesForProperty(hash, name); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base addChild(String name) throws FHIRException { 1141 if (name.equals("code")) { 1142 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.property.code"); 1143 } 1144 else if (name.equals("uri")) { 1145 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.property.uri"); 1146 } 1147 else if (name.equals("description")) { 1148 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.property.description"); 1149 } 1150 else if (name.equals("type")) { 1151 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.property.type"); 1152 } 1153 else 1154 return super.addChild(name); 1155 } 1156 1157 public PropertyComponent copy() { 1158 PropertyComponent dst = new PropertyComponent(); 1159 copyValues(dst); 1160 return dst; 1161 } 1162 1163 public void copyValues(PropertyComponent dst) { 1164 super.copyValues(dst); 1165 dst.code = code == null ? null : code.copy(); 1166 dst.uri = uri == null ? null : uri.copy(); 1167 dst.description = description == null ? null : description.copy(); 1168 dst.type = type == null ? null : type.copy(); 1169 } 1170 1171 @Override 1172 public boolean equalsDeep(Base other_) { 1173 if (!super.equalsDeep(other_)) 1174 return false; 1175 if (!(other_ instanceof PropertyComponent)) 1176 return false; 1177 PropertyComponent o = (PropertyComponent) other_; 1178 return compareDeep(code, o.code, true) && compareDeep(uri, o.uri, true) && compareDeep(description, o.description, true) 1179 && compareDeep(type, o.type, true); 1180 } 1181 1182 @Override 1183 public boolean equalsShallow(Base other_) { 1184 if (!super.equalsShallow(other_)) 1185 return false; 1186 if (!(other_ instanceof PropertyComponent)) 1187 return false; 1188 PropertyComponent o = (PropertyComponent) other_; 1189 return compareValues(code, o.code, true) && compareValues(uri, o.uri, true) && compareValues(description, o.description, true) 1190 && compareValues(type, o.type, true); 1191 } 1192 1193 public boolean isEmpty() { 1194 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, uri, description, type 1195 ); 1196 } 1197 1198 public String fhirType() { 1199 return "CodeSystem.property"; 1200 1201 } 1202 1203 } 1204 1205 @Block() 1206 public static class ConceptDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 1207 /** 1208 * A code - a text symbol - that uniquely identifies the concept within the code system. 1209 */ 1210 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1211 @Description(shortDefinition="Code that identifies concept", formalDefinition="A code - a text symbol - that uniquely identifies the concept within the code system." ) 1212 protected CodeType code; 1213 1214 /** 1215 * A human readable string that is the recommended default way to present this concept to a user. 1216 */ 1217 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1218 @Description(shortDefinition="Text to display to the user", formalDefinition="A human readable string that is the recommended default way to present this concept to a user." ) 1219 protected StringType display; 1220 1221 /** 1222 * The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept. 1223 */ 1224 @Child(name = "definition", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1225 @Description(shortDefinition="Formal definition", formalDefinition="The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept." ) 1226 protected StringType definition; 1227 1228 /** 1229 * Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc. 1230 */ 1231 @Child(name = "designation", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1232 @Description(shortDefinition="Additional representations for the concept", formalDefinition="Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc." ) 1233 protected List<ConceptDefinitionDesignationComponent> designation; 1234 1235 /** 1236 * A property value for this concept. 1237 */ 1238 @Child(name = "property", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1239 @Description(shortDefinition="Property value for the concept", formalDefinition="A property value for this concept." ) 1240 protected List<ConceptPropertyComponent> property; 1241 1242 /** 1243 * Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning. 1244 */ 1245 @Child(name = "concept", type = {ConceptDefinitionComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1246 @Description(shortDefinition="Child Concepts (is-a/contains/categorizes)", formalDefinition="Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning." ) 1247 protected List<ConceptDefinitionComponent> concept; 1248 1249 private static final long serialVersionUID = 878320988L; 1250 1251 /** 1252 * Constructor 1253 */ 1254 public ConceptDefinitionComponent() { 1255 super(); 1256 } 1257 1258 /** 1259 * Constructor 1260 */ 1261 public ConceptDefinitionComponent(String code) { 1262 super(); 1263 this.setCode(code); 1264 } 1265 1266 /** 1267 * @return {@link #code} (A code - a text symbol - that uniquely identifies the concept within the code system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1268 */ 1269 public CodeType getCodeElement() { 1270 if (this.code == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create ConceptDefinitionComponent.code"); 1273 else if (Configuration.doAutoCreate()) 1274 this.code = new CodeType(); // bb 1275 return this.code; 1276 } 1277 1278 public boolean hasCodeElement() { 1279 return this.code != null && !this.code.isEmpty(); 1280 } 1281 1282 public boolean hasCode() { 1283 return this.code != null && !this.code.isEmpty(); 1284 } 1285 1286 /** 1287 * @param value {@link #code} (A code - a text symbol - that uniquely identifies the concept within the code system.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1288 */ 1289 public ConceptDefinitionComponent setCodeElement(CodeType value) { 1290 this.code = value; 1291 return this; 1292 } 1293 1294 /** 1295 * @return A code - a text symbol - that uniquely identifies the concept within the code system. 1296 */ 1297 public String getCode() { 1298 return this.code == null ? null : this.code.getValue(); 1299 } 1300 1301 /** 1302 * @param value A code - a text symbol - that uniquely identifies the concept within the code system. 1303 */ 1304 public ConceptDefinitionComponent setCode(String value) { 1305 if (this.code == null) 1306 this.code = new CodeType(); 1307 this.code.setValue(value); 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #display} (A human readable string that is the recommended default way to present this concept to a user.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1313 */ 1314 public StringType getDisplayElement() { 1315 if (this.display == null) 1316 if (Configuration.errorOnAutoCreate()) 1317 throw new Error("Attempt to auto-create ConceptDefinitionComponent.display"); 1318 else if (Configuration.doAutoCreate()) 1319 this.display = new StringType(); // bb 1320 return this.display; 1321 } 1322 1323 public boolean hasDisplayElement() { 1324 return this.display != null && !this.display.isEmpty(); 1325 } 1326 1327 public boolean hasDisplay() { 1328 return this.display != null && !this.display.isEmpty(); 1329 } 1330 1331 /** 1332 * @param value {@link #display} (A human readable string that is the recommended default way to present this concept to a user.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1333 */ 1334 public ConceptDefinitionComponent setDisplayElement(StringType value) { 1335 this.display = value; 1336 return this; 1337 } 1338 1339 /** 1340 * @return A human readable string that is the recommended default way to present this concept to a user. 1341 */ 1342 public String getDisplay() { 1343 return this.display == null ? null : this.display.getValue(); 1344 } 1345 1346 /** 1347 * @param value A human readable string that is the recommended default way to present this concept to a user. 1348 */ 1349 public ConceptDefinitionComponent setDisplay(String value) { 1350 if (Utilities.noString(value)) 1351 this.display = null; 1352 else { 1353 if (this.display == null) 1354 this.display = new StringType(); 1355 this.display.setValue(value); 1356 } 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #definition} (The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1362 */ 1363 public StringType getDefinitionElement() { 1364 if (this.definition == null) 1365 if (Configuration.errorOnAutoCreate()) 1366 throw new Error("Attempt to auto-create ConceptDefinitionComponent.definition"); 1367 else if (Configuration.doAutoCreate()) 1368 this.definition = new StringType(); // bb 1369 return this.definition; 1370 } 1371 1372 public boolean hasDefinitionElement() { 1373 return this.definition != null && !this.definition.isEmpty(); 1374 } 1375 1376 public boolean hasDefinition() { 1377 return this.definition != null && !this.definition.isEmpty(); 1378 } 1379 1380 /** 1381 * @param value {@link #definition} (The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 1382 */ 1383 public ConceptDefinitionComponent setDefinitionElement(StringType value) { 1384 this.definition = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept. 1390 */ 1391 public String getDefinition() { 1392 return this.definition == null ? null : this.definition.getValue(); 1393 } 1394 1395 /** 1396 * @param value The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept. 1397 */ 1398 public ConceptDefinitionComponent setDefinition(String value) { 1399 if (Utilities.noString(value)) 1400 this.definition = null; 1401 else { 1402 if (this.definition == null) 1403 this.definition = new StringType(); 1404 this.definition.setValue(value); 1405 } 1406 return this; 1407 } 1408 1409 /** 1410 * @return {@link #designation} (Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.) 1411 */ 1412 public List<ConceptDefinitionDesignationComponent> getDesignation() { 1413 if (this.designation == null) 1414 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1415 return this.designation; 1416 } 1417 1418 /** 1419 * @return Returns a reference to <code>this</code> for easy method chaining 1420 */ 1421 public ConceptDefinitionComponent setDesignation(List<ConceptDefinitionDesignationComponent> theDesignation) { 1422 this.designation = theDesignation; 1423 return this; 1424 } 1425 1426 public boolean hasDesignation() { 1427 if (this.designation == null) 1428 return false; 1429 for (ConceptDefinitionDesignationComponent item : this.designation) 1430 if (!item.isEmpty()) 1431 return true; 1432 return false; 1433 } 1434 1435 public ConceptDefinitionDesignationComponent addDesignation() { //3 1436 ConceptDefinitionDesignationComponent t = new ConceptDefinitionDesignationComponent(); 1437 if (this.designation == null) 1438 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1439 this.designation.add(t); 1440 return t; 1441 } 1442 1443 public ConceptDefinitionComponent addDesignation(ConceptDefinitionDesignationComponent t) { //3 1444 if (t == null) 1445 return this; 1446 if (this.designation == null) 1447 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1448 this.designation.add(t); 1449 return this; 1450 } 1451 1452 /** 1453 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist {3} 1454 */ 1455 public ConceptDefinitionDesignationComponent getDesignationFirstRep() { 1456 if (getDesignation().isEmpty()) { 1457 addDesignation(); 1458 } 1459 return getDesignation().get(0); 1460 } 1461 1462 /** 1463 * @return {@link #property} (A property value for this concept.) 1464 */ 1465 public List<ConceptPropertyComponent> getProperty() { 1466 if (this.property == null) 1467 this.property = new ArrayList<ConceptPropertyComponent>(); 1468 return this.property; 1469 } 1470 1471 /** 1472 * @return Returns a reference to <code>this</code> for easy method chaining 1473 */ 1474 public ConceptDefinitionComponent setProperty(List<ConceptPropertyComponent> theProperty) { 1475 this.property = theProperty; 1476 return this; 1477 } 1478 1479 public boolean hasProperty() { 1480 if (this.property == null) 1481 return false; 1482 for (ConceptPropertyComponent item : this.property) 1483 if (!item.isEmpty()) 1484 return true; 1485 return false; 1486 } 1487 1488 public ConceptPropertyComponent addProperty() { //3 1489 ConceptPropertyComponent t = new ConceptPropertyComponent(); 1490 if (this.property == null) 1491 this.property = new ArrayList<ConceptPropertyComponent>(); 1492 this.property.add(t); 1493 return t; 1494 } 1495 1496 public ConceptDefinitionComponent addProperty(ConceptPropertyComponent t) { //3 1497 if (t == null) 1498 return this; 1499 if (this.property == null) 1500 this.property = new ArrayList<ConceptPropertyComponent>(); 1501 this.property.add(t); 1502 return this; 1503 } 1504 1505 /** 1506 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 1507 */ 1508 public ConceptPropertyComponent getPropertyFirstRep() { 1509 if (getProperty().isEmpty()) { 1510 addProperty(); 1511 } 1512 return getProperty().get(0); 1513 } 1514 1515 /** 1516 * @return {@link #concept} (Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.) 1517 */ 1518 public List<ConceptDefinitionComponent> getConcept() { 1519 if (this.concept == null) 1520 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1521 return this.concept; 1522 } 1523 1524 /** 1525 * @return Returns a reference to <code>this</code> for easy method chaining 1526 */ 1527 public ConceptDefinitionComponent setConcept(List<ConceptDefinitionComponent> theConcept) { 1528 this.concept = theConcept; 1529 return this; 1530 } 1531 1532 public boolean hasConcept() { 1533 if (this.concept == null) 1534 return false; 1535 for (ConceptDefinitionComponent item : this.concept) 1536 if (!item.isEmpty()) 1537 return true; 1538 return false; 1539 } 1540 1541 public ConceptDefinitionComponent addConcept() { //3 1542 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 1543 if (this.concept == null) 1544 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1545 this.concept.add(t); 1546 return t; 1547 } 1548 1549 public ConceptDefinitionComponent addConcept(ConceptDefinitionComponent t) { //3 1550 if (t == null) 1551 return this; 1552 if (this.concept == null) 1553 this.concept = new ArrayList<ConceptDefinitionComponent>(); 1554 this.concept.add(t); 1555 return this; 1556 } 1557 1558 /** 1559 * @return The first repetition of repeating field {@link #concept}, creating it if it does not already exist {3} 1560 */ 1561 public ConceptDefinitionComponent getConceptFirstRep() { 1562 if (getConcept().isEmpty()) { 1563 addConcept(); 1564 } 1565 return getConcept().get(0); 1566 } 1567 1568 protected void listChildren(List<Property> children) { 1569 super.listChildren(children); 1570 children.add(new Property("code", "code", "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1, code)); 1571 children.add(new Property("display", "string", "A human readable string that is the recommended default way to present this concept to a user.", 0, 1, display)); 1572 children.add(new Property("definition", "string", "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 0, 1, definition)); 1573 children.add(new Property("designation", "", "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation)); 1574 children.add(new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property)); 1575 children.add(new Property("concept", "@CodeSystem.concept", "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.", 0, java.lang.Integer.MAX_VALUE, concept)); 1576 } 1577 1578 @Override 1579 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1580 switch (_hash) { 1581 case 3059181: /*code*/ return new Property("code", "code", "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1, code); 1582 case 1671764162: /*display*/ return new Property("display", "string", "A human readable string that is the recommended default way to present this concept to a user.", 0, 1, display); 1583 case -1014418093: /*definition*/ return new Property("definition", "string", "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 0, 1, definition); 1584 case -900931593: /*designation*/ return new Property("designation", "", "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation); 1585 case -993141291: /*property*/ return new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property); 1586 case 951024232: /*concept*/ return new Property("concept", "@CodeSystem.concept", "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.", 0, java.lang.Integer.MAX_VALUE, concept); 1587 default: return super.getNamedProperty(_hash, _name, _checkValid); 1588 } 1589 1590 } 1591 1592 @Override 1593 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1594 switch (hash) { 1595 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1596 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1597 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // StringType 1598 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // ConceptDefinitionDesignationComponent 1599 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // ConceptPropertyComponent 1600 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptDefinitionComponent 1601 default: return super.getProperty(hash, name, checkValid); 1602 } 1603 1604 } 1605 1606 @Override 1607 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1608 switch (hash) { 1609 case 3059181: // code 1610 this.code = TypeConvertor.castToCode(value); // CodeType 1611 return value; 1612 case 1671764162: // display 1613 this.display = TypeConvertor.castToString(value); // StringType 1614 return value; 1615 case -1014418093: // definition 1616 this.definition = TypeConvertor.castToString(value); // StringType 1617 return value; 1618 case -900931593: // designation 1619 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); // ConceptDefinitionDesignationComponent 1620 return value; 1621 case -993141291: // property 1622 this.getProperty().add((ConceptPropertyComponent) value); // ConceptPropertyComponent 1623 return value; 1624 case 951024232: // concept 1625 this.getConcept().add((ConceptDefinitionComponent) value); // ConceptDefinitionComponent 1626 return value; 1627 default: return super.setProperty(hash, name, value); 1628 } 1629 1630 } 1631 1632 @Override 1633 public Base setProperty(String name, Base value) throws FHIRException { 1634 if (name.equals("code")) { 1635 this.code = TypeConvertor.castToCode(value); // CodeType 1636 } else if (name.equals("display")) { 1637 this.display = TypeConvertor.castToString(value); // StringType 1638 } else if (name.equals("definition")) { 1639 this.definition = TypeConvertor.castToString(value); // StringType 1640 } else if (name.equals("designation")) { 1641 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); 1642 } else if (name.equals("property")) { 1643 this.getProperty().add((ConceptPropertyComponent) value); 1644 } else if (name.equals("concept")) { 1645 this.getConcept().add((ConceptDefinitionComponent) value); 1646 } else 1647 return super.setProperty(name, value); 1648 return value; 1649 } 1650 1651 @Override 1652 public void removeChild(String name, Base value) throws FHIRException { 1653 if (name.equals("code")) { 1654 this.code = null; 1655 } else if (name.equals("display")) { 1656 this.display = null; 1657 } else if (name.equals("definition")) { 1658 this.definition = null; 1659 } else if (name.equals("designation")) { 1660 this.getDesignation().remove((ConceptDefinitionDesignationComponent) value); 1661 } else if (name.equals("property")) { 1662 this.getProperty().remove((ConceptPropertyComponent) value); 1663 } else if (name.equals("concept")) { 1664 this.getConcept().remove((ConceptDefinitionComponent) value); 1665 } else 1666 super.removeChild(name, value); 1667 1668 } 1669 1670 @Override 1671 public Base makeProperty(int hash, String name) throws FHIRException { 1672 switch (hash) { 1673 case 3059181: return getCodeElement(); 1674 case 1671764162: return getDisplayElement(); 1675 case -1014418093: return getDefinitionElement(); 1676 case -900931593: return addDesignation(); 1677 case -993141291: return addProperty(); 1678 case 951024232: return addConcept(); 1679 default: return super.makeProperty(hash, name); 1680 } 1681 1682 } 1683 1684 @Override 1685 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1686 switch (hash) { 1687 case 3059181: /*code*/ return new String[] {"code"}; 1688 case 1671764162: /*display*/ return new String[] {"string"}; 1689 case -1014418093: /*definition*/ return new String[] {"string"}; 1690 case -900931593: /*designation*/ return new String[] {}; 1691 case -993141291: /*property*/ return new String[] {}; 1692 case 951024232: /*concept*/ return new String[] {"@CodeSystem.concept"}; 1693 default: return super.getTypesForProperty(hash, name); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base addChild(String name) throws FHIRException { 1700 if (name.equals("code")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.concept.code"); 1702 } 1703 else if (name.equals("display")) { 1704 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.concept.display"); 1705 } 1706 else if (name.equals("definition")) { 1707 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.concept.definition"); 1708 } 1709 else if (name.equals("designation")) { 1710 return addDesignation(); 1711 } 1712 else if (name.equals("property")) { 1713 return addProperty(); 1714 } 1715 else if (name.equals("concept")) { 1716 return addConcept(); 1717 } 1718 else 1719 return super.addChild(name); 1720 } 1721 1722 public ConceptDefinitionComponent copy() { 1723 ConceptDefinitionComponent dst = new ConceptDefinitionComponent(); 1724 copyValues(dst); 1725 return dst; 1726 } 1727 1728 public void copyValues(ConceptDefinitionComponent dst) { 1729 super.copyValues(dst); 1730 dst.code = code == null ? null : code.copy(); 1731 dst.display = display == null ? null : display.copy(); 1732 dst.definition = definition == null ? null : definition.copy(); 1733 if (designation != null) { 1734 dst.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 1735 for (ConceptDefinitionDesignationComponent i : designation) 1736 dst.designation.add(i.copy()); 1737 }; 1738 if (property != null) { 1739 dst.property = new ArrayList<ConceptPropertyComponent>(); 1740 for (ConceptPropertyComponent i : property) 1741 dst.property.add(i.copy()); 1742 }; 1743 if (concept != null) { 1744 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 1745 for (ConceptDefinitionComponent i : concept) 1746 dst.concept.add(i.copy()); 1747 }; 1748 } 1749 1750 @Override 1751 public boolean equalsDeep(Base other_) { 1752 if (!super.equalsDeep(other_)) 1753 return false; 1754 if (!(other_ instanceof ConceptDefinitionComponent)) 1755 return false; 1756 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other_; 1757 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(definition, o.definition, true) 1758 && compareDeep(designation, o.designation, true) && compareDeep(property, o.property, true) && compareDeep(concept, o.concept, true) 1759 ; 1760 } 1761 1762 @Override 1763 public boolean equalsShallow(Base other_) { 1764 if (!super.equalsShallow(other_)) 1765 return false; 1766 if (!(other_ instanceof ConceptDefinitionComponent)) 1767 return false; 1768 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other_; 1769 return compareValues(code, o.code, true) && compareValues(display, o.display, true) && compareValues(definition, o.definition, true) 1770 ; 1771 } 1772 1773 public boolean isEmpty() { 1774 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, definition 1775 , designation, property, concept); 1776 } 1777 1778 public String fhirType() { 1779 return "CodeSystem.concept"; 1780 1781 } 1782 1783// added from java-adornments.txt: 1784@Override 1785 public String toString() { 1786 return getCode()+": "+getDisplay(); 1787 } 1788// end addition 1789 } 1790 1791 @Block() 1792 public static class ConceptDefinitionDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1793 /** 1794 * The language this designation is defined for. 1795 */ 1796 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1797 @Description(shortDefinition="Human language of the designation", formalDefinition="The language this designation is defined for." ) 1798 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 1799 protected CodeType language; 1800 1801 /** 1802 * A code that details how this designation would be used. 1803 */ 1804 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 1805 @Description(shortDefinition="Details how this designation would be used", formalDefinition="A code that details how this designation would be used." ) 1806 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1807 protected Coding use; 1808 1809 /** 1810 * Additional codes that detail how this designation would be used, if there is more than one use. 1811 */ 1812 @Child(name = "additionalUse", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1813 @Description(shortDefinition="Additional ways how this designation would be used", formalDefinition="Additional codes that detail how this designation would be used, if there is more than one use." ) 1814 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1815 protected List<Coding> additionalUse; 1816 1817 /** 1818 * The text value for this designation. 1819 */ 1820 @Child(name = "value", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 1821 @Description(shortDefinition="The text value for this designation", formalDefinition="The text value for this designation." ) 1822 protected StringType value; 1823 1824 private static final long serialVersionUID = -141147882L; 1825 1826 /** 1827 * Constructor 1828 */ 1829 public ConceptDefinitionDesignationComponent() { 1830 super(); 1831 } 1832 1833 /** 1834 * Constructor 1835 */ 1836 public ConceptDefinitionDesignationComponent(String value) { 1837 super(); 1838 this.setValue(value); 1839 } 1840 1841 /** 1842 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1843 */ 1844 public CodeType getLanguageElement() { 1845 if (this.language == null) 1846 if (Configuration.errorOnAutoCreate()) 1847 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.language"); 1848 else if (Configuration.doAutoCreate()) 1849 this.language = new CodeType(); // bb 1850 return this.language; 1851 } 1852 1853 public boolean hasLanguageElement() { 1854 return this.language != null && !this.language.isEmpty(); 1855 } 1856 1857 public boolean hasLanguage() { 1858 return this.language != null && !this.language.isEmpty(); 1859 } 1860 1861 /** 1862 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1863 */ 1864 public ConceptDefinitionDesignationComponent setLanguageElement(CodeType value) { 1865 this.language = value; 1866 return this; 1867 } 1868 1869 /** 1870 * @return The language this designation is defined for. 1871 */ 1872 public String getLanguage() { 1873 return this.language == null ? null : this.language.getValue(); 1874 } 1875 1876 /** 1877 * @param value The language this designation is defined for. 1878 */ 1879 public ConceptDefinitionDesignationComponent setLanguage(String value) { 1880 if (Utilities.noString(value)) 1881 this.language = null; 1882 else { 1883 if (this.language == null) 1884 this.language = new CodeType(); 1885 this.language.setValue(value); 1886 } 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #use} (A code that details how this designation would be used.) 1892 */ 1893 public Coding getUse() { 1894 if (this.use == null) 1895 if (Configuration.errorOnAutoCreate()) 1896 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.use"); 1897 else if (Configuration.doAutoCreate()) 1898 this.use = new Coding(); // cc 1899 return this.use; 1900 } 1901 1902 public boolean hasUse() { 1903 return this.use != null && !this.use.isEmpty(); 1904 } 1905 1906 /** 1907 * @param value {@link #use} (A code that details how this designation would be used.) 1908 */ 1909 public ConceptDefinitionDesignationComponent setUse(Coding value) { 1910 this.use = value; 1911 return this; 1912 } 1913 1914 /** 1915 * @return {@link #additionalUse} (Additional codes that detail how this designation would be used, if there is more than one use.) 1916 */ 1917 public List<Coding> getAdditionalUse() { 1918 if (this.additionalUse == null) 1919 this.additionalUse = new ArrayList<Coding>(); 1920 return this.additionalUse; 1921 } 1922 1923 /** 1924 * @return Returns a reference to <code>this</code> for easy method chaining 1925 */ 1926 public ConceptDefinitionDesignationComponent setAdditionalUse(List<Coding> theAdditionalUse) { 1927 this.additionalUse = theAdditionalUse; 1928 return this; 1929 } 1930 1931 public boolean hasAdditionalUse() { 1932 if (this.additionalUse == null) 1933 return false; 1934 for (Coding item : this.additionalUse) 1935 if (!item.isEmpty()) 1936 return true; 1937 return false; 1938 } 1939 1940 public Coding addAdditionalUse() { //3 1941 Coding t = new Coding(); 1942 if (this.additionalUse == null) 1943 this.additionalUse = new ArrayList<Coding>(); 1944 this.additionalUse.add(t); 1945 return t; 1946 } 1947 1948 public ConceptDefinitionDesignationComponent addAdditionalUse(Coding t) { //3 1949 if (t == null) 1950 return this; 1951 if (this.additionalUse == null) 1952 this.additionalUse = new ArrayList<Coding>(); 1953 this.additionalUse.add(t); 1954 return this; 1955 } 1956 1957 /** 1958 * @return The first repetition of repeating field {@link #additionalUse}, creating it if it does not already exist {3} 1959 */ 1960 public Coding getAdditionalUseFirstRep() { 1961 if (getAdditionalUse().isEmpty()) { 1962 addAdditionalUse(); 1963 } 1964 return getAdditionalUse().get(0); 1965 } 1966 1967 /** 1968 * @return {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1969 */ 1970 public StringType getValueElement() { 1971 if (this.value == null) 1972 if (Configuration.errorOnAutoCreate()) 1973 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.value"); 1974 else if (Configuration.doAutoCreate()) 1975 this.value = new StringType(); // bb 1976 return this.value; 1977 } 1978 1979 public boolean hasValueElement() { 1980 return this.value != null && !this.value.isEmpty(); 1981 } 1982 1983 public boolean hasValue() { 1984 return this.value != null && !this.value.isEmpty(); 1985 } 1986 1987 /** 1988 * @param value {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1989 */ 1990 public ConceptDefinitionDesignationComponent setValueElement(StringType value) { 1991 this.value = value; 1992 return this; 1993 } 1994 1995 /** 1996 * @return The text value for this designation. 1997 */ 1998 public String getValue() { 1999 return this.value == null ? null : this.value.getValue(); 2000 } 2001 2002 /** 2003 * @param value The text value for this designation. 2004 */ 2005 public ConceptDefinitionDesignationComponent setValue(String value) { 2006 if (this.value == null) 2007 this.value = new StringType(); 2008 this.value.setValue(value); 2009 return this; 2010 } 2011 2012 protected void listChildren(List<Property> children) { 2013 super.listChildren(children); 2014 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 2015 children.add(new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use)); 2016 children.add(new Property("additionalUse", "Coding", "Additional codes that detail how this designation would be used, if there is more than one use.", 0, java.lang.Integer.MAX_VALUE, additionalUse)); 2017 children.add(new Property("value", "string", "The text value for this designation.", 0, 1, value)); 2018 } 2019 2020 @Override 2021 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2022 switch (_hash) { 2023 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 2024 case 116103: /*use*/ return new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use); 2025 case 938414048: /*additionalUse*/ return new Property("additionalUse", "Coding", "Additional codes that detail how this designation would be used, if there is more than one use.", 0, java.lang.Integer.MAX_VALUE, additionalUse); 2026 case 111972721: /*value*/ return new Property("value", "string", "The text value for this designation.", 0, 1, value); 2027 default: return super.getNamedProperty(_hash, _name, _checkValid); 2028 } 2029 2030 } 2031 2032 @Override 2033 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2034 switch (hash) { 2035 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 2036 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 2037 case 938414048: /*additionalUse*/ return this.additionalUse == null ? new Base[0] : this.additionalUse.toArray(new Base[this.additionalUse.size()]); // Coding 2038 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 2039 default: return super.getProperty(hash, name, checkValid); 2040 } 2041 2042 } 2043 2044 @Override 2045 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2046 switch (hash) { 2047 case -1613589672: // language 2048 this.language = TypeConvertor.castToCode(value); // CodeType 2049 return value; 2050 case 116103: // use 2051 this.use = TypeConvertor.castToCoding(value); // Coding 2052 return value; 2053 case 938414048: // additionalUse 2054 this.getAdditionalUse().add(TypeConvertor.castToCoding(value)); // Coding 2055 return value; 2056 case 111972721: // value 2057 this.value = TypeConvertor.castToString(value); // StringType 2058 return value; 2059 default: return super.setProperty(hash, name, value); 2060 } 2061 2062 } 2063 2064 @Override 2065 public Base setProperty(String name, Base value) throws FHIRException { 2066 if (name.equals("language")) { 2067 this.language = TypeConvertor.castToCode(value); // CodeType 2068 } else if (name.equals("use")) { 2069 this.use = TypeConvertor.castToCoding(value); // Coding 2070 } else if (name.equals("additionalUse")) { 2071 this.getAdditionalUse().add(TypeConvertor.castToCoding(value)); 2072 } else if (name.equals("value")) { 2073 this.value = TypeConvertor.castToString(value); // StringType 2074 } else 2075 return super.setProperty(name, value); 2076 return value; 2077 } 2078 2079 @Override 2080 public void removeChild(String name, Base value) throws FHIRException { 2081 if (name.equals("language")) { 2082 this.language = null; 2083 } else if (name.equals("use")) { 2084 this.use = null; 2085 } else if (name.equals("additionalUse")) { 2086 this.getAdditionalUse().remove(value); 2087 } else if (name.equals("value")) { 2088 this.value = null; 2089 } else 2090 super.removeChild(name, value); 2091 2092 } 2093 2094 @Override 2095 public Base makeProperty(int hash, String name) throws FHIRException { 2096 switch (hash) { 2097 case -1613589672: return getLanguageElement(); 2098 case 116103: return getUse(); 2099 case 938414048: return addAdditionalUse(); 2100 case 111972721: return getValueElement(); 2101 default: return super.makeProperty(hash, name); 2102 } 2103 2104 } 2105 2106 @Override 2107 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2108 switch (hash) { 2109 case -1613589672: /*language*/ return new String[] {"code"}; 2110 case 116103: /*use*/ return new String[] {"Coding"}; 2111 case 938414048: /*additionalUse*/ return new String[] {"Coding"}; 2112 case 111972721: /*value*/ return new String[] {"string"}; 2113 default: return super.getTypesForProperty(hash, name); 2114 } 2115 2116 } 2117 2118 @Override 2119 public Base addChild(String name) throws FHIRException { 2120 if (name.equals("language")) { 2121 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.concept.designation.language"); 2122 } 2123 else if (name.equals("use")) { 2124 this.use = new Coding(); 2125 return this.use; 2126 } 2127 else if (name.equals("additionalUse")) { 2128 return addAdditionalUse(); 2129 } 2130 else if (name.equals("value")) { 2131 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.concept.designation.value"); 2132 } 2133 else 2134 return super.addChild(name); 2135 } 2136 2137 public ConceptDefinitionDesignationComponent copy() { 2138 ConceptDefinitionDesignationComponent dst = new ConceptDefinitionDesignationComponent(); 2139 copyValues(dst); 2140 return dst; 2141 } 2142 2143 public void copyValues(ConceptDefinitionDesignationComponent dst) { 2144 super.copyValues(dst); 2145 dst.language = language == null ? null : language.copy(); 2146 dst.use = use == null ? null : use.copy(); 2147 if (additionalUse != null) { 2148 dst.additionalUse = new ArrayList<Coding>(); 2149 for (Coding i : additionalUse) 2150 dst.additionalUse.add(i.copy()); 2151 }; 2152 dst.value = value == null ? null : value.copy(); 2153 } 2154 2155 @Override 2156 public boolean equalsDeep(Base other_) { 2157 if (!super.equalsDeep(other_)) 2158 return false; 2159 if (!(other_ instanceof ConceptDefinitionDesignationComponent)) 2160 return false; 2161 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other_; 2162 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) && compareDeep(additionalUse, o.additionalUse, true) 2163 && compareDeep(value, o.value, true); 2164 } 2165 2166 @Override 2167 public boolean equalsShallow(Base other_) { 2168 if (!super.equalsShallow(other_)) 2169 return false; 2170 if (!(other_ instanceof ConceptDefinitionDesignationComponent)) 2171 return false; 2172 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other_; 2173 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 2174 } 2175 2176 public boolean isEmpty() { 2177 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use, additionalUse 2178 , value); 2179 } 2180 2181 public String fhirType() { 2182 return "CodeSystem.concept.designation"; 2183 2184 } 2185 2186 } 2187 2188 @Block() 2189 public static class ConceptPropertyComponent extends BackboneElement implements IBaseBackboneElement { 2190 /** 2191 * A code that is a reference to CodeSystem.property.code. 2192 */ 2193 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2194 @Description(shortDefinition="Reference to CodeSystem.property.code", formalDefinition="A code that is a reference to CodeSystem.property.code." ) 2195 protected CodeType code; 2196 2197 /** 2198 * The value of this property. 2199 */ 2200 @Child(name = "value", type = {CodeType.class, Coding.class, StringType.class, IntegerType.class, BooleanType.class, DateTimeType.class, DecimalType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2201 @Description(shortDefinition="Value of the property for this concept", formalDefinition="The value of this property." ) 2202 protected DataType value; 2203 2204 private static final long serialVersionUID = -422546419L; 2205 2206 /** 2207 * Constructor 2208 */ 2209 public ConceptPropertyComponent() { 2210 super(); 2211 } 2212 2213 /** 2214 * Constructor 2215 */ 2216 public ConceptPropertyComponent(String code, DataType value) { 2217 super(); 2218 this.setCode(code); 2219 this.setValue(value); 2220 } 2221 2222 /** 2223 * @return {@link #code} (A code that is a reference to CodeSystem.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2224 */ 2225 public CodeType getCodeElement() { 2226 if (this.code == null) 2227 if (Configuration.errorOnAutoCreate()) 2228 throw new Error("Attempt to auto-create ConceptPropertyComponent.code"); 2229 else if (Configuration.doAutoCreate()) 2230 this.code = new CodeType(); // bb 2231 return this.code; 2232 } 2233 2234 public boolean hasCodeElement() { 2235 return this.code != null && !this.code.isEmpty(); 2236 } 2237 2238 public boolean hasCode() { 2239 return this.code != null && !this.code.isEmpty(); 2240 } 2241 2242 /** 2243 * @param value {@link #code} (A code that is a reference to CodeSystem.property.code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2244 */ 2245 public ConceptPropertyComponent setCodeElement(CodeType value) { 2246 this.code = value; 2247 return this; 2248 } 2249 2250 /** 2251 * @return A code that is a reference to CodeSystem.property.code. 2252 */ 2253 public String getCode() { 2254 return this.code == null ? null : this.code.getValue(); 2255 } 2256 2257 /** 2258 * @param value A code that is a reference to CodeSystem.property.code. 2259 */ 2260 public ConceptPropertyComponent setCode(String value) { 2261 if (this.code == null) 2262 this.code = new CodeType(); 2263 this.code.setValue(value); 2264 return this; 2265 } 2266 2267 /** 2268 * @return {@link #value} (The value of this property.) 2269 */ 2270 public DataType getValue() { 2271 return this.value; 2272 } 2273 2274 /** 2275 * @return {@link #value} (The value of this property.) 2276 */ 2277 public CodeType getValueCodeType() throws FHIRException { 2278 if (this.value == null) 2279 this.value = new CodeType(); 2280 if (!(this.value instanceof CodeType)) 2281 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2282 return (CodeType) this.value; 2283 } 2284 2285 public boolean hasValueCodeType() { 2286 return this != null && this.value instanceof CodeType; 2287 } 2288 2289 /** 2290 * @return {@link #value} (The value of this property.) 2291 */ 2292 public Coding getValueCoding() throws FHIRException { 2293 if (this.value == null) 2294 this.value = new Coding(); 2295 if (!(this.value instanceof Coding)) 2296 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 2297 return (Coding) this.value; 2298 } 2299 2300 public boolean hasValueCoding() { 2301 return this != null && this.value instanceof Coding; 2302 } 2303 2304 /** 2305 * @return {@link #value} (The value of this property.) 2306 */ 2307 public StringType getValueStringType() throws FHIRException { 2308 if (this.value == null) 2309 this.value = new StringType(); 2310 if (!(this.value instanceof StringType)) 2311 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2312 return (StringType) this.value; 2313 } 2314 2315 public boolean hasValueStringType() { 2316 return this != null && this.value instanceof StringType; 2317 } 2318 2319 /** 2320 * @return {@link #value} (The value of this property.) 2321 */ 2322 public IntegerType getValueIntegerType() throws FHIRException { 2323 if (this.value == null) 2324 this.value = new IntegerType(); 2325 if (!(this.value instanceof IntegerType)) 2326 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2327 return (IntegerType) this.value; 2328 } 2329 2330 public boolean hasValueIntegerType() { 2331 return this != null && this.value instanceof IntegerType; 2332 } 2333 2334 /** 2335 * @return {@link #value} (The value of this property.) 2336 */ 2337 public BooleanType getValueBooleanType() throws FHIRException { 2338 if (this.value == null) 2339 this.value = new BooleanType(); 2340 if (!(this.value instanceof BooleanType)) 2341 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2342 return (BooleanType) this.value; 2343 } 2344 2345 public boolean hasValueBooleanType() { 2346 return this != null && this.value instanceof BooleanType; 2347 } 2348 2349 /** 2350 * @return {@link #value} (The value of this property.) 2351 */ 2352 public DateTimeType getValueDateTimeType() throws FHIRException { 2353 if (this.value == null) 2354 this.value = new DateTimeType(); 2355 if (!(this.value instanceof DateTimeType)) 2356 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2357 return (DateTimeType) this.value; 2358 } 2359 2360 public boolean hasValueDateTimeType() { 2361 return this != null && this.value instanceof DateTimeType; 2362 } 2363 2364 /** 2365 * @return {@link #value} (The value of this property.) 2366 */ 2367 public DecimalType getValueDecimalType() throws FHIRException { 2368 if (this.value == null) 2369 this.value = new DecimalType(); 2370 if (!(this.value instanceof DecimalType)) 2371 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2372 return (DecimalType) this.value; 2373 } 2374 2375 public boolean hasValueDecimalType() { 2376 return this != null && this.value instanceof DecimalType; 2377 } 2378 2379 public boolean hasValue() { 2380 return this.value != null && !this.value.isEmpty(); 2381 } 2382 2383 /** 2384 * @param value {@link #value} (The value of this property.) 2385 */ 2386 public ConceptPropertyComponent setValue(DataType value) { 2387 if (value != null && !(value instanceof CodeType || value instanceof Coding || value instanceof StringType || value instanceof IntegerType || value instanceof BooleanType || value instanceof DateTimeType || value instanceof DecimalType)) 2388 throw new FHIRException("Not the right type for CodeSystem.concept.property.value[x]: "+value.fhirType()); 2389 this.value = value; 2390 return this; 2391 } 2392 2393 protected void listChildren(List<Property> children) { 2394 super.listChildren(children); 2395 children.add(new Property("code", "code", "A code that is a reference to CodeSystem.property.code.", 0, 1, code)); 2396 children.add(new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this property.", 0, 1, value)); 2397 } 2398 2399 @Override 2400 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2401 switch (_hash) { 2402 case 3059181: /*code*/ return new Property("code", "code", "A code that is a reference to CodeSystem.property.code.", 0, 1, code); 2403 case -1410166417: /*value[x]*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this property.", 0, 1, value); 2404 case 111972721: /*value*/ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", "The value of this property.", 0, 1, value); 2405 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of this property.", 0, 1, value); 2406 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of this property.", 0, 1, value); 2407 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of this property.", 0, 1, value); 2408 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of this property.", 0, 1, value); 2409 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of this property.", 0, 1, value); 2410 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of this property.", 0, 1, value); 2411 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of this property.", 0, 1, value); 2412 default: return super.getNamedProperty(_hash, _name, _checkValid); 2413 } 2414 2415 } 2416 2417 @Override 2418 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2419 switch (hash) { 2420 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2421 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2422 default: return super.getProperty(hash, name, checkValid); 2423 } 2424 2425 } 2426 2427 @Override 2428 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2429 switch (hash) { 2430 case 3059181: // code 2431 this.code = TypeConvertor.castToCode(value); // CodeType 2432 return value; 2433 case 111972721: // value 2434 this.value = TypeConvertor.castToType(value); // DataType 2435 return value; 2436 default: return super.setProperty(hash, name, value); 2437 } 2438 2439 } 2440 2441 @Override 2442 public Base setProperty(String name, Base value) throws FHIRException { 2443 if (name.equals("code")) { 2444 this.code = TypeConvertor.castToCode(value); // CodeType 2445 } else if (name.equals("value[x]")) { 2446 this.value = TypeConvertor.castToType(value); // DataType 2447 } else 2448 return super.setProperty(name, value); 2449 return value; 2450 } 2451 2452 @Override 2453 public void removeChild(String name, Base value) throws FHIRException { 2454 if (name.equals("code")) { 2455 this.code = null; 2456 } else if (name.equals("value[x]")) { 2457 this.value = null; 2458 } else 2459 super.removeChild(name, value); 2460 2461 } 2462 2463 @Override 2464 public Base makeProperty(int hash, String name) throws FHIRException { 2465 switch (hash) { 2466 case 3059181: return getCodeElement(); 2467 case -1410166417: return getValue(); 2468 case 111972721: return getValue(); 2469 default: return super.makeProperty(hash, name); 2470 } 2471 2472 } 2473 2474 @Override 2475 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2476 switch (hash) { 2477 case 3059181: /*code*/ return new String[] {"code"}; 2478 case 111972721: /*value*/ return new String[] {"code", "Coding", "string", "integer", "boolean", "dateTime", "decimal"}; 2479 default: return super.getTypesForProperty(hash, name); 2480 } 2481 2482 } 2483 2484 @Override 2485 public Base addChild(String name) throws FHIRException { 2486 if (name.equals("code")) { 2487 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.concept.property.code"); 2488 } 2489 else if (name.equals("valueCode")) { 2490 this.value = new CodeType(); 2491 return this.value; 2492 } 2493 else if (name.equals("valueCoding")) { 2494 this.value = new Coding(); 2495 return this.value; 2496 } 2497 else if (name.equals("valueString")) { 2498 this.value = new StringType(); 2499 return this.value; 2500 } 2501 else if (name.equals("valueInteger")) { 2502 this.value = new IntegerType(); 2503 return this.value; 2504 } 2505 else if (name.equals("valueBoolean")) { 2506 this.value = new BooleanType(); 2507 return this.value; 2508 } 2509 else if (name.equals("valueDateTime")) { 2510 this.value = new DateTimeType(); 2511 return this.value; 2512 } 2513 else if (name.equals("valueDecimal")) { 2514 this.value = new DecimalType(); 2515 return this.value; 2516 } 2517 else 2518 return super.addChild(name); 2519 } 2520 2521 public ConceptPropertyComponent copy() { 2522 ConceptPropertyComponent dst = new ConceptPropertyComponent(); 2523 copyValues(dst); 2524 return dst; 2525 } 2526 2527 public void copyValues(ConceptPropertyComponent dst) { 2528 super.copyValues(dst); 2529 dst.code = code == null ? null : code.copy(); 2530 dst.value = value == null ? null : value.copy(); 2531 } 2532 2533 @Override 2534 public boolean equalsDeep(Base other_) { 2535 if (!super.equalsDeep(other_)) 2536 return false; 2537 if (!(other_ instanceof ConceptPropertyComponent)) 2538 return false; 2539 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 2540 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 2541 } 2542 2543 @Override 2544 public boolean equalsShallow(Base other_) { 2545 if (!super.equalsShallow(other_)) 2546 return false; 2547 if (!(other_ instanceof ConceptPropertyComponent)) 2548 return false; 2549 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 2550 return compareValues(code, o.code, true); 2551 } 2552 2553 public boolean isEmpty() { 2554 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 2555 } 2556 2557 public String fhirType() { 2558 return "CodeSystem.concept.property"; 2559 2560 } 2561 2562 } 2563 2564 /** 2565 * An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system. 2566 */ 2567 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2568 @Description(shortDefinition="Canonical identifier for this code system, represented as a URI (globally unique) (Coding.system)", formalDefinition="An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system." ) 2569 protected UriType url; 2570 2571 /** 2572 * A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance. 2573 */ 2574 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2575 @Description(shortDefinition="Additional identifier for the code system (business identifier)", formalDefinition="A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2576 protected List<Identifier> identifier; 2577 2578 /** 2579 * The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version. 2580 */ 2581 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2582 @Description(shortDefinition="Business version of the code system (Coding.version)", formalDefinition="The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version." ) 2583 protected StringType version; 2584 2585 /** 2586 * Indicates the mechanism used to compare versions to determine which CodeSystem is more current. 2587 */ 2588 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2589 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which CodeSystem is more current." ) 2590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2591 protected DataType versionAlgorithm; 2592 2593 /** 2594 * A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2595 */ 2596 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2597 @Description(shortDefinition="Name for this code system (computer friendly)", formalDefinition="A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2598 protected StringType name; 2599 2600 /** 2601 * A short, descriptive, user-friendly title for the code system. 2602 */ 2603 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2604 @Description(shortDefinition="Name for this code system (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the code system." ) 2605 protected StringType title; 2606 2607 /** 2608 * The status of this code system. Enables tracking the life-cycle of the content. 2609 */ 2610 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 2611 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this code system. Enables tracking the life-cycle of the content." ) 2612 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2613 protected Enumeration<PublicationStatus> status; 2614 2615 /** 2616 * A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2617 */ 2618 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 2619 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 2620 protected BooleanType experimental; 2621 2622 /** 2623 * The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes. 2624 */ 2625 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2626 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes." ) 2627 protected DateTimeType date; 2628 2629 /** 2630 * The name of the organization or individual responsible for the release and ongoing maintenance of the code system. 2631 */ 2632 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2633 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the code system." ) 2634 protected StringType publisher; 2635 2636 /** 2637 * Contact details to assist a user in finding and communicating with the publisher. 2638 */ 2639 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2640 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2641 protected List<ContactDetail> contact; 2642 2643 /** 2644 * A free text natural language description of the code system from a consumer's perspective. 2645 */ 2646 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2647 @Description(shortDefinition="Natural language description of the code system", formalDefinition="A free text natural language description of the code system from a consumer's perspective." ) 2648 protected MarkdownType description; 2649 2650 /** 2651 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances. 2652 */ 2653 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2654 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances." ) 2655 protected List<UsageContext> useContext; 2656 2657 /** 2658 * A legal or geographic region in which the code system is intended to be used. 2659 */ 2660 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2661 @Description(shortDefinition="Intended jurisdiction for code system (if applicable)", formalDefinition="A legal or geographic region in which the code system is intended to be used." ) 2662 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2663 protected List<CodeableConcept> jurisdiction; 2664 2665 /** 2666 * Explanation of why this code system is needed and why it has been designed as it has. 2667 */ 2668 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 2669 @Description(shortDefinition="Why this code system is defined", formalDefinition="Explanation of why this code system is needed and why it has been designed as it has." ) 2670 protected MarkdownType purpose; 2671 2672 /** 2673 * A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system. 2674 */ 2675 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 2676 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system." ) 2677 protected MarkdownType copyright; 2678 2679 /** 2680 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2681 */ 2682 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2683 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2684 protected StringType copyrightLabel; 2685 2686 /** 2687 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2688 */ 2689 @Child(name = "approvalDate", type = {DateType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2690 @Description(shortDefinition="When the CodeSystem was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 2691 protected DateType approvalDate; 2692 2693 /** 2694 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 2695 */ 2696 @Child(name = "lastReviewDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2697 @Description(shortDefinition="When the CodeSystem was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 2698 protected DateType lastReviewDate; 2699 2700 /** 2701 * The period during which the CodeSystem content was or is planned to be in active use. 2702 */ 2703 @Child(name = "effectivePeriod", type = {Period.class}, order=19, min=0, max=1, modifier=false, summary=true) 2704 @Description(shortDefinition="When the CodeSystem is expected to be used", formalDefinition="The period during which the CodeSystem content was or is planned to be in active use." ) 2705 protected Period effectivePeriod; 2706 2707 /** 2708 * Descriptions related to the content of the CodeSystem. Topics provide a high-level categorization as well as keywords for the CodeSystem that can be useful for filtering and searching. 2709 */ 2710 @Child(name = "topic", type = {CodeableConcept.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2711 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptions related to the content of the CodeSystem. Topics provide a high-level categorization as well as keywords for the CodeSystem that can be useful for filtering and searching." ) 2712 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 2713 protected List<CodeableConcept> topic; 2714 2715 /** 2716 * An individiual or organization primarily involved in the creation and maintenance of the CodeSystem. 2717 */ 2718 @Child(name = "author", type = {ContactDetail.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2719 @Description(shortDefinition="Who authored the CodeSystem", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the CodeSystem." ) 2720 protected List<ContactDetail> author; 2721 2722 /** 2723 * An individual or organization primarily responsible for internal coherence of the CodeSystem. 2724 */ 2725 @Child(name = "editor", type = {ContactDetail.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2726 @Description(shortDefinition="Who edited the CodeSystem", formalDefinition="An individual or organization primarily responsible for internal coherence of the CodeSystem." ) 2727 protected List<ContactDetail> editor; 2728 2729 /** 2730 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the CodeSystem. 2731 */ 2732 @Child(name = "reviewer", type = {ContactDetail.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2733 @Description(shortDefinition="Who reviewed the CodeSystem", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the CodeSystem." ) 2734 protected List<ContactDetail> reviewer; 2735 2736 /** 2737 * An individual or organization asserted by the publisher to be responsible for officially endorsing the CodeSystem for use in some setting. 2738 */ 2739 @Child(name = "endorser", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2740 @Description(shortDefinition="Who endorsed the CodeSystem", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the CodeSystem for use in some setting." ) 2741 protected List<ContactDetail> endorser; 2742 2743 /** 2744 * Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts. 2745 */ 2746 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2747 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts." ) 2748 protected List<RelatedArtifact> relatedArtifact; 2749 2750 /** 2751 * If code comparison is case sensitive when codes within this system are compared to each other. 2752 */ 2753 @Child(name = "caseSensitive", type = {BooleanType.class}, order=26, min=0, max=1, modifier=false, summary=true) 2754 @Description(shortDefinition="If code comparison is case sensitive", formalDefinition="If code comparison is case sensitive when codes within this system are compared to each other." ) 2755 protected BooleanType caseSensitive; 2756 2757 /** 2758 * Canonical reference to the value set that contains all codes in the code system independent of code status. 2759 */ 2760 @Child(name = "valueSet", type = {CanonicalType.class}, order=27, min=0, max=1, modifier=false, summary=true) 2761 @Description(shortDefinition="Canonical reference to the value set with entire code system", formalDefinition="Canonical reference to the value set that contains all codes in the code system independent of code status." ) 2762 protected CanonicalType valueSet; 2763 2764 /** 2765 * The meaning of the hierarchy of concepts as represented in this resource. 2766 */ 2767 @Child(name = "hierarchyMeaning", type = {CodeType.class}, order=28, min=0, max=1, modifier=false, summary=true) 2768 @Description(shortDefinition="grouped-by | is-a | part-of | classified-with", formalDefinition="The meaning of the hierarchy of concepts as represented in this resource." ) 2769 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/codesystem-hierarchy-meaning") 2770 protected Enumeration<CodeSystemHierarchyMeaning> hierarchyMeaning; 2771 2772 /** 2773 * The code system defines a compositional (post-coordination) grammar. 2774 */ 2775 @Child(name = "compositional", type = {BooleanType.class}, order=29, min=0, max=1, modifier=false, summary=true) 2776 @Description(shortDefinition="If code system defines a compositional grammar", formalDefinition="The code system defines a compositional (post-coordination) grammar." ) 2777 protected BooleanType compositional; 2778 2779 /** 2780 * This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system. 2781 */ 2782 @Child(name = "versionNeeded", type = {BooleanType.class}, order=30, min=0, max=1, modifier=false, summary=true) 2783 @Description(shortDefinition="If definitions are not stable", formalDefinition="This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system." ) 2784 protected BooleanType versionNeeded; 2785 2786 /** 2787 * The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance. 2788 */ 2789 @Child(name = "content", type = {CodeType.class}, order=31, min=1, max=1, modifier=false, summary=true) 2790 @Description(shortDefinition="not-present | example | fragment | complete | supplement", formalDefinition="The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance." ) 2791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/codesystem-content-mode") 2792 protected Enumeration<CodeSystemContentMode> content; 2793 2794 /** 2795 * The canonical URL of the code system that this code system supplement is adding designations and properties to. 2796 */ 2797 @Child(name = "supplements", type = {CanonicalType.class}, order=32, min=0, max=1, modifier=false, summary=true) 2798 @Description(shortDefinition="Canonical URL of Code System this adds designations and properties to", formalDefinition="The canonical URL of the code system that this code system supplement is adding designations and properties to." ) 2799 protected CanonicalType supplements; 2800 2801 /** 2802 * The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward. 2803 */ 2804 @Child(name = "count", type = {UnsignedIntType.class}, order=33, min=0, max=1, modifier=false, summary=true) 2805 @Description(shortDefinition="Total concepts in the code system", formalDefinition="The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward." ) 2806 protected UnsignedIntType count; 2807 2808 /** 2809 * A filter that can be used in a value set compose statement when selecting concepts using a filter. 2810 */ 2811 @Child(name = "filter", type = {}, order=34, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2812 @Description(shortDefinition="Filter that can be used in a value set", formalDefinition="A filter that can be used in a value set compose statement when selecting concepts using a filter." ) 2813 protected List<CodeSystemFilterComponent> filter; 2814 2815 /** 2816 * A property defines an additional slot through which additional information can be provided about a concept. 2817 */ 2818 @Child(name = "property", type = {}, order=35, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2819 @Description(shortDefinition="Additional information supplied about each concept", formalDefinition="A property defines an additional slot through which additional information can be provided about a concept." ) 2820 protected List<PropertyComponent> property; 2821 2822 /** 2823 * Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are. 2824 */ 2825 @Child(name = "concept", type = {}, order=36, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2826 @Description(shortDefinition="Concepts in the code system", formalDefinition="Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are." ) 2827 protected List<ConceptDefinitionComponent> concept; 2828 2829 private static final long serialVersionUID = -937961842L; 2830 2831 /** 2832 * Constructor 2833 */ 2834 public CodeSystem() { 2835 super(); 2836 } 2837 2838 /** 2839 * Constructor 2840 */ 2841 public CodeSystem(PublicationStatus status, CodeSystemContentMode content) { 2842 super(); 2843 this.setStatus(status); 2844 this.setContent(content); 2845 } 2846 2847 /** 2848 * @return {@link #url} (An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2849 */ 2850 public UriType getUrlElement() { 2851 if (this.url == null) 2852 if (Configuration.errorOnAutoCreate()) 2853 throw new Error("Attempt to auto-create CodeSystem.url"); 2854 else if (Configuration.doAutoCreate()) 2855 this.url = new UriType(); // bb 2856 return this.url; 2857 } 2858 2859 public boolean hasUrlElement() { 2860 return this.url != null && !this.url.isEmpty(); 2861 } 2862 2863 public boolean hasUrl() { 2864 return this.url != null && !this.url.isEmpty(); 2865 } 2866 2867 /** 2868 * @param value {@link #url} (An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2869 */ 2870 public CodeSystem setUrlElement(UriType value) { 2871 this.url = value; 2872 return this; 2873 } 2874 2875 /** 2876 * @return An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system. 2877 */ 2878 public String getUrl() { 2879 return this.url == null ? null : this.url.getValue(); 2880 } 2881 2882 /** 2883 * @param value An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system. 2884 */ 2885 public CodeSystem setUrl(String value) { 2886 if (Utilities.noString(value)) 2887 this.url = null; 2888 else { 2889 if (this.url == null) 2890 this.url = new UriType(); 2891 this.url.setValue(value); 2892 } 2893 return this; 2894 } 2895 2896 /** 2897 * @return {@link #identifier} (A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2898 */ 2899 public List<Identifier> getIdentifier() { 2900 if (this.identifier == null) 2901 this.identifier = new ArrayList<Identifier>(); 2902 return this.identifier; 2903 } 2904 2905 /** 2906 * @return Returns a reference to <code>this</code> for easy method chaining 2907 */ 2908 public CodeSystem setIdentifier(List<Identifier> theIdentifier) { 2909 this.identifier = theIdentifier; 2910 return this; 2911 } 2912 2913 public boolean hasIdentifier() { 2914 if (this.identifier == null) 2915 return false; 2916 for (Identifier item : this.identifier) 2917 if (!item.isEmpty()) 2918 return true; 2919 return false; 2920 } 2921 2922 public Identifier addIdentifier() { //3 2923 Identifier t = new Identifier(); 2924 if (this.identifier == null) 2925 this.identifier = new ArrayList<Identifier>(); 2926 this.identifier.add(t); 2927 return t; 2928 } 2929 2930 public CodeSystem addIdentifier(Identifier t) { //3 2931 if (t == null) 2932 return this; 2933 if (this.identifier == null) 2934 this.identifier = new ArrayList<Identifier>(); 2935 this.identifier.add(t); 2936 return this; 2937 } 2938 2939 /** 2940 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2941 */ 2942 public Identifier getIdentifierFirstRep() { 2943 if (getIdentifier().isEmpty()) { 2944 addIdentifier(); 2945 } 2946 return getIdentifier().get(0); 2947 } 2948 2949 /** 2950 * @return {@link #version} (The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2951 */ 2952 public StringType getVersionElement() { 2953 if (this.version == null) 2954 if (Configuration.errorOnAutoCreate()) 2955 throw new Error("Attempt to auto-create CodeSystem.version"); 2956 else if (Configuration.doAutoCreate()) 2957 this.version = new StringType(); // bb 2958 return this.version; 2959 } 2960 2961 public boolean hasVersionElement() { 2962 return this.version != null && !this.version.isEmpty(); 2963 } 2964 2965 public boolean hasVersion() { 2966 return this.version != null && !this.version.isEmpty(); 2967 } 2968 2969 /** 2970 * @param value {@link #version} (The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2971 */ 2972 public CodeSystem setVersionElement(StringType value) { 2973 this.version = value; 2974 return this; 2975 } 2976 2977 /** 2978 * @return The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version. 2979 */ 2980 public String getVersion() { 2981 return this.version == null ? null : this.version.getValue(); 2982 } 2983 2984 /** 2985 * @param value The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version. 2986 */ 2987 public CodeSystem setVersion(String value) { 2988 if (Utilities.noString(value)) 2989 this.version = null; 2990 else { 2991 if (this.version == null) 2992 this.version = new StringType(); 2993 this.version.setValue(value); 2994 } 2995 return this; 2996 } 2997 2998 /** 2999 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which CodeSystem is more current.) 3000 */ 3001 public DataType getVersionAlgorithm() { 3002 return this.versionAlgorithm; 3003 } 3004 3005 /** 3006 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which CodeSystem is more current.) 3007 */ 3008 public StringType getVersionAlgorithmStringType() throws FHIRException { 3009 if (this.versionAlgorithm == null) 3010 this.versionAlgorithm = new StringType(); 3011 if (!(this.versionAlgorithm instanceof StringType)) 3012 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3013 return (StringType) this.versionAlgorithm; 3014 } 3015 3016 public boolean hasVersionAlgorithmStringType() { 3017 return this != null && this.versionAlgorithm instanceof StringType; 3018 } 3019 3020 /** 3021 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which CodeSystem is more current.) 3022 */ 3023 public Coding getVersionAlgorithmCoding() throws FHIRException { 3024 if (this.versionAlgorithm == null) 3025 this.versionAlgorithm = new Coding(); 3026 if (!(this.versionAlgorithm instanceof Coding)) 3027 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3028 return (Coding) this.versionAlgorithm; 3029 } 3030 3031 public boolean hasVersionAlgorithmCoding() { 3032 return this != null && this.versionAlgorithm instanceof Coding; 3033 } 3034 3035 public boolean hasVersionAlgorithm() { 3036 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 3037 } 3038 3039 /** 3040 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which CodeSystem is more current.) 3041 */ 3042 public CodeSystem setVersionAlgorithm(DataType value) { 3043 if (value != null && !(value instanceof StringType || value instanceof Coding)) 3044 throw new FHIRException("Not the right type for CodeSystem.versionAlgorithm[x]: "+value.fhirType()); 3045 this.versionAlgorithm = value; 3046 return this; 3047 } 3048 3049 /** 3050 * @return {@link #name} (A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3051 */ 3052 public StringType getNameElement() { 3053 if (this.name == null) 3054 if (Configuration.errorOnAutoCreate()) 3055 throw new Error("Attempt to auto-create CodeSystem.name"); 3056 else if (Configuration.doAutoCreate()) 3057 this.name = new StringType(); // bb 3058 return this.name; 3059 } 3060 3061 public boolean hasNameElement() { 3062 return this.name != null && !this.name.isEmpty(); 3063 } 3064 3065 public boolean hasName() { 3066 return this.name != null && !this.name.isEmpty(); 3067 } 3068 3069 /** 3070 * @param value {@link #name} (A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3071 */ 3072 public CodeSystem setNameElement(StringType value) { 3073 this.name = value; 3074 return this; 3075 } 3076 3077 /** 3078 * @return A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3079 */ 3080 public String getName() { 3081 return this.name == null ? null : this.name.getValue(); 3082 } 3083 3084 /** 3085 * @param value A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3086 */ 3087 public CodeSystem setName(String value) { 3088 if (Utilities.noString(value)) 3089 this.name = null; 3090 else { 3091 if (this.name == null) 3092 this.name = new StringType(); 3093 this.name.setValue(value); 3094 } 3095 return this; 3096 } 3097 3098 /** 3099 * @return {@link #title} (A short, descriptive, user-friendly title for the code system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3100 */ 3101 public StringType getTitleElement() { 3102 if (this.title == null) 3103 if (Configuration.errorOnAutoCreate()) 3104 throw new Error("Attempt to auto-create CodeSystem.title"); 3105 else if (Configuration.doAutoCreate()) 3106 this.title = new StringType(); // bb 3107 return this.title; 3108 } 3109 3110 public boolean hasTitleElement() { 3111 return this.title != null && !this.title.isEmpty(); 3112 } 3113 3114 public boolean hasTitle() { 3115 return this.title != null && !this.title.isEmpty(); 3116 } 3117 3118 /** 3119 * @param value {@link #title} (A short, descriptive, user-friendly title for the code system.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3120 */ 3121 public CodeSystem setTitleElement(StringType value) { 3122 this.title = value; 3123 return this; 3124 } 3125 3126 /** 3127 * @return A short, descriptive, user-friendly title for the code system. 3128 */ 3129 public String getTitle() { 3130 return this.title == null ? null : this.title.getValue(); 3131 } 3132 3133 /** 3134 * @param value A short, descriptive, user-friendly title for the code system. 3135 */ 3136 public CodeSystem setTitle(String value) { 3137 if (Utilities.noString(value)) 3138 this.title = null; 3139 else { 3140 if (this.title == null) 3141 this.title = new StringType(); 3142 this.title.setValue(value); 3143 } 3144 return this; 3145 } 3146 3147 /** 3148 * @return {@link #status} (The status of this code system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3149 */ 3150 public Enumeration<PublicationStatus> getStatusElement() { 3151 if (this.status == null) 3152 if (Configuration.errorOnAutoCreate()) 3153 throw new Error("Attempt to auto-create CodeSystem.status"); 3154 else if (Configuration.doAutoCreate()) 3155 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3156 return this.status; 3157 } 3158 3159 public boolean hasStatusElement() { 3160 return this.status != null && !this.status.isEmpty(); 3161 } 3162 3163 public boolean hasStatus() { 3164 return this.status != null && !this.status.isEmpty(); 3165 } 3166 3167 /** 3168 * @param value {@link #status} (The status of this code system. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3169 */ 3170 public CodeSystem setStatusElement(Enumeration<PublicationStatus> value) { 3171 this.status = value; 3172 return this; 3173 } 3174 3175 /** 3176 * @return The status of this code system. Enables tracking the life-cycle of the content. 3177 */ 3178 public PublicationStatus getStatus() { 3179 return this.status == null ? null : this.status.getValue(); 3180 } 3181 3182 /** 3183 * @param value The status of this code system. Enables tracking the life-cycle of the content. 3184 */ 3185 public CodeSystem setStatus(PublicationStatus value) { 3186 if (this.status == null) 3187 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3188 this.status.setValue(value); 3189 return this; 3190 } 3191 3192 /** 3193 * @return {@link #experimental} (A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3194 */ 3195 public BooleanType getExperimentalElement() { 3196 if (this.experimental == null) 3197 if (Configuration.errorOnAutoCreate()) 3198 throw new Error("Attempt to auto-create CodeSystem.experimental"); 3199 else if (Configuration.doAutoCreate()) 3200 this.experimental = new BooleanType(); // bb 3201 return this.experimental; 3202 } 3203 3204 public boolean hasExperimentalElement() { 3205 return this.experimental != null && !this.experimental.isEmpty(); 3206 } 3207 3208 public boolean hasExperimental() { 3209 return this.experimental != null && !this.experimental.isEmpty(); 3210 } 3211 3212 /** 3213 * @param value {@link #experimental} (A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3214 */ 3215 public CodeSystem setExperimentalElement(BooleanType value) { 3216 this.experimental = value; 3217 return this; 3218 } 3219 3220 /** 3221 * @return A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3222 */ 3223 public boolean getExperimental() { 3224 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3225 } 3226 3227 /** 3228 * @param value A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3229 */ 3230 public CodeSystem setExperimental(boolean value) { 3231 if (this.experimental == null) 3232 this.experimental = new BooleanType(); 3233 this.experimental.setValue(value); 3234 return this; 3235 } 3236 3237 /** 3238 * @return {@link #date} (The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3239 */ 3240 public DateTimeType getDateElement() { 3241 if (this.date == null) 3242 if (Configuration.errorOnAutoCreate()) 3243 throw new Error("Attempt to auto-create CodeSystem.date"); 3244 else if (Configuration.doAutoCreate()) 3245 this.date = new DateTimeType(); // bb 3246 return this.date; 3247 } 3248 3249 public boolean hasDateElement() { 3250 return this.date != null && !this.date.isEmpty(); 3251 } 3252 3253 public boolean hasDate() { 3254 return this.date != null && !this.date.isEmpty(); 3255 } 3256 3257 /** 3258 * @param value {@link #date} (The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3259 */ 3260 public CodeSystem setDateElement(DateTimeType value) { 3261 this.date = value; 3262 return this; 3263 } 3264 3265 /** 3266 * @return The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes. 3267 */ 3268 public Date getDate() { 3269 return this.date == null ? null : this.date.getValue(); 3270 } 3271 3272 /** 3273 * @param value The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes. 3274 */ 3275 public CodeSystem setDate(Date value) { 3276 if (value == null) 3277 this.date = null; 3278 else { 3279 if (this.date == null) 3280 this.date = new DateTimeType(); 3281 this.date.setValue(value); 3282 } 3283 return this; 3284 } 3285 3286 /** 3287 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the code system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3288 */ 3289 public StringType getPublisherElement() { 3290 if (this.publisher == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create CodeSystem.publisher"); 3293 else if (Configuration.doAutoCreate()) 3294 this.publisher = new StringType(); // bb 3295 return this.publisher; 3296 } 3297 3298 public boolean hasPublisherElement() { 3299 return this.publisher != null && !this.publisher.isEmpty(); 3300 } 3301 3302 public boolean hasPublisher() { 3303 return this.publisher != null && !this.publisher.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the code system.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3308 */ 3309 public CodeSystem setPublisherElement(StringType value) { 3310 this.publisher = value; 3311 return this; 3312 } 3313 3314 /** 3315 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the code system. 3316 */ 3317 public String getPublisher() { 3318 return this.publisher == null ? null : this.publisher.getValue(); 3319 } 3320 3321 /** 3322 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the code system. 3323 */ 3324 public CodeSystem setPublisher(String value) { 3325 if (Utilities.noString(value)) 3326 this.publisher = null; 3327 else { 3328 if (this.publisher == null) 3329 this.publisher = new StringType(); 3330 this.publisher.setValue(value); 3331 } 3332 return this; 3333 } 3334 3335 /** 3336 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3337 */ 3338 public List<ContactDetail> getContact() { 3339 if (this.contact == null) 3340 this.contact = new ArrayList<ContactDetail>(); 3341 return this.contact; 3342 } 3343 3344 /** 3345 * @return Returns a reference to <code>this</code> for easy method chaining 3346 */ 3347 public CodeSystem setContact(List<ContactDetail> theContact) { 3348 this.contact = theContact; 3349 return this; 3350 } 3351 3352 public boolean hasContact() { 3353 if (this.contact == null) 3354 return false; 3355 for (ContactDetail item : this.contact) 3356 if (!item.isEmpty()) 3357 return true; 3358 return false; 3359 } 3360 3361 public ContactDetail addContact() { //3 3362 ContactDetail t = new ContactDetail(); 3363 if (this.contact == null) 3364 this.contact = new ArrayList<ContactDetail>(); 3365 this.contact.add(t); 3366 return t; 3367 } 3368 3369 public CodeSystem addContact(ContactDetail t) { //3 3370 if (t == null) 3371 return this; 3372 if (this.contact == null) 3373 this.contact = new ArrayList<ContactDetail>(); 3374 this.contact.add(t); 3375 return this; 3376 } 3377 3378 /** 3379 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3380 */ 3381 public ContactDetail getContactFirstRep() { 3382 if (getContact().isEmpty()) { 3383 addContact(); 3384 } 3385 return getContact().get(0); 3386 } 3387 3388 /** 3389 * @return {@link #description} (A free text natural language description of the code system from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3390 */ 3391 public MarkdownType getDescriptionElement() { 3392 if (this.description == null) 3393 if (Configuration.errorOnAutoCreate()) 3394 throw new Error("Attempt to auto-create CodeSystem.description"); 3395 else if (Configuration.doAutoCreate()) 3396 this.description = new MarkdownType(); // bb 3397 return this.description; 3398 } 3399 3400 public boolean hasDescriptionElement() { 3401 return this.description != null && !this.description.isEmpty(); 3402 } 3403 3404 public boolean hasDescription() { 3405 return this.description != null && !this.description.isEmpty(); 3406 } 3407 3408 /** 3409 * @param value {@link #description} (A free text natural language description of the code system from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3410 */ 3411 public CodeSystem setDescriptionElement(MarkdownType value) { 3412 this.description = value; 3413 return this; 3414 } 3415 3416 /** 3417 * @return A free text natural language description of the code system from a consumer's perspective. 3418 */ 3419 public String getDescription() { 3420 return this.description == null ? null : this.description.getValue(); 3421 } 3422 3423 /** 3424 * @param value A free text natural language description of the code system from a consumer's perspective. 3425 */ 3426 public CodeSystem setDescription(String value) { 3427 if (Utilities.noString(value)) 3428 this.description = null; 3429 else { 3430 if (this.description == null) 3431 this.description = new MarkdownType(); 3432 this.description.setValue(value); 3433 } 3434 return this; 3435 } 3436 3437 /** 3438 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances.) 3439 */ 3440 public List<UsageContext> getUseContext() { 3441 if (this.useContext == null) 3442 this.useContext = new ArrayList<UsageContext>(); 3443 return this.useContext; 3444 } 3445 3446 /** 3447 * @return Returns a reference to <code>this</code> for easy method chaining 3448 */ 3449 public CodeSystem setUseContext(List<UsageContext> theUseContext) { 3450 this.useContext = theUseContext; 3451 return this; 3452 } 3453 3454 public boolean hasUseContext() { 3455 if (this.useContext == null) 3456 return false; 3457 for (UsageContext item : this.useContext) 3458 if (!item.isEmpty()) 3459 return true; 3460 return false; 3461 } 3462 3463 public UsageContext addUseContext() { //3 3464 UsageContext t = new UsageContext(); 3465 if (this.useContext == null) 3466 this.useContext = new ArrayList<UsageContext>(); 3467 this.useContext.add(t); 3468 return t; 3469 } 3470 3471 public CodeSystem addUseContext(UsageContext t) { //3 3472 if (t == null) 3473 return this; 3474 if (this.useContext == null) 3475 this.useContext = new ArrayList<UsageContext>(); 3476 this.useContext.add(t); 3477 return this; 3478 } 3479 3480 /** 3481 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3482 */ 3483 public UsageContext getUseContextFirstRep() { 3484 if (getUseContext().isEmpty()) { 3485 addUseContext(); 3486 } 3487 return getUseContext().get(0); 3488 } 3489 3490 /** 3491 * @return {@link #jurisdiction} (A legal or geographic region in which the code system is intended to be used.) 3492 */ 3493 public List<CodeableConcept> getJurisdiction() { 3494 if (this.jurisdiction == null) 3495 this.jurisdiction = new ArrayList<CodeableConcept>(); 3496 return this.jurisdiction; 3497 } 3498 3499 /** 3500 * @return Returns a reference to <code>this</code> for easy method chaining 3501 */ 3502 public CodeSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 3503 this.jurisdiction = theJurisdiction; 3504 return this; 3505 } 3506 3507 public boolean hasJurisdiction() { 3508 if (this.jurisdiction == null) 3509 return false; 3510 for (CodeableConcept item : this.jurisdiction) 3511 if (!item.isEmpty()) 3512 return true; 3513 return false; 3514 } 3515 3516 public CodeableConcept addJurisdiction() { //3 3517 CodeableConcept t = new CodeableConcept(); 3518 if (this.jurisdiction == null) 3519 this.jurisdiction = new ArrayList<CodeableConcept>(); 3520 this.jurisdiction.add(t); 3521 return t; 3522 } 3523 3524 public CodeSystem addJurisdiction(CodeableConcept t) { //3 3525 if (t == null) 3526 return this; 3527 if (this.jurisdiction == null) 3528 this.jurisdiction = new ArrayList<CodeableConcept>(); 3529 this.jurisdiction.add(t); 3530 return this; 3531 } 3532 3533 /** 3534 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3535 */ 3536 public CodeableConcept getJurisdictionFirstRep() { 3537 if (getJurisdiction().isEmpty()) { 3538 addJurisdiction(); 3539 } 3540 return getJurisdiction().get(0); 3541 } 3542 3543 /** 3544 * @return {@link #purpose} (Explanation of why this code system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3545 */ 3546 public MarkdownType getPurposeElement() { 3547 if (this.purpose == null) 3548 if (Configuration.errorOnAutoCreate()) 3549 throw new Error("Attempt to auto-create CodeSystem.purpose"); 3550 else if (Configuration.doAutoCreate()) 3551 this.purpose = new MarkdownType(); // bb 3552 return this.purpose; 3553 } 3554 3555 public boolean hasPurposeElement() { 3556 return this.purpose != null && !this.purpose.isEmpty(); 3557 } 3558 3559 public boolean hasPurpose() { 3560 return this.purpose != null && !this.purpose.isEmpty(); 3561 } 3562 3563 /** 3564 * @param value {@link #purpose} (Explanation of why this code system is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3565 */ 3566 public CodeSystem setPurposeElement(MarkdownType value) { 3567 this.purpose = value; 3568 return this; 3569 } 3570 3571 /** 3572 * @return Explanation of why this code system is needed and why it has been designed as it has. 3573 */ 3574 public String getPurpose() { 3575 return this.purpose == null ? null : this.purpose.getValue(); 3576 } 3577 3578 /** 3579 * @param value Explanation of why this code system is needed and why it has been designed as it has. 3580 */ 3581 public CodeSystem setPurpose(String value) { 3582 if (Utilities.noString(value)) 3583 this.purpose = null; 3584 else { 3585 if (this.purpose == null) 3586 this.purpose = new MarkdownType(); 3587 this.purpose.setValue(value); 3588 } 3589 return this; 3590 } 3591 3592 /** 3593 * @return {@link #copyright} (A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3594 */ 3595 public MarkdownType getCopyrightElement() { 3596 if (this.copyright == null) 3597 if (Configuration.errorOnAutoCreate()) 3598 throw new Error("Attempt to auto-create CodeSystem.copyright"); 3599 else if (Configuration.doAutoCreate()) 3600 this.copyright = new MarkdownType(); // bb 3601 return this.copyright; 3602 } 3603 3604 public boolean hasCopyrightElement() { 3605 return this.copyright != null && !this.copyright.isEmpty(); 3606 } 3607 3608 public boolean hasCopyright() { 3609 return this.copyright != null && !this.copyright.isEmpty(); 3610 } 3611 3612 /** 3613 * @param value {@link #copyright} (A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3614 */ 3615 public CodeSystem setCopyrightElement(MarkdownType value) { 3616 this.copyright = value; 3617 return this; 3618 } 3619 3620 /** 3621 * @return A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system. 3622 */ 3623 public String getCopyright() { 3624 return this.copyright == null ? null : this.copyright.getValue(); 3625 } 3626 3627 /** 3628 * @param value A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system. 3629 */ 3630 public CodeSystem setCopyright(String value) { 3631 if (Utilities.noString(value)) 3632 this.copyright = null; 3633 else { 3634 if (this.copyright == null) 3635 this.copyright = new MarkdownType(); 3636 this.copyright.setValue(value); 3637 } 3638 return this; 3639 } 3640 3641 /** 3642 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3643 */ 3644 public StringType getCopyrightLabelElement() { 3645 if (this.copyrightLabel == null) 3646 if (Configuration.errorOnAutoCreate()) 3647 throw new Error("Attempt to auto-create CodeSystem.copyrightLabel"); 3648 else if (Configuration.doAutoCreate()) 3649 this.copyrightLabel = new StringType(); // bb 3650 return this.copyrightLabel; 3651 } 3652 3653 public boolean hasCopyrightLabelElement() { 3654 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3655 } 3656 3657 public boolean hasCopyrightLabel() { 3658 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3659 } 3660 3661 /** 3662 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3663 */ 3664 public CodeSystem setCopyrightLabelElement(StringType value) { 3665 this.copyrightLabel = value; 3666 return this; 3667 } 3668 3669 /** 3670 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3671 */ 3672 public String getCopyrightLabel() { 3673 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 3674 } 3675 3676 /** 3677 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3678 */ 3679 public CodeSystem setCopyrightLabel(String value) { 3680 if (Utilities.noString(value)) 3681 this.copyrightLabel = null; 3682 else { 3683 if (this.copyrightLabel == null) 3684 this.copyrightLabel = new StringType(); 3685 this.copyrightLabel.setValue(value); 3686 } 3687 return this; 3688 } 3689 3690 /** 3691 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3692 */ 3693 public DateType getApprovalDateElement() { 3694 if (this.approvalDate == null) 3695 if (Configuration.errorOnAutoCreate()) 3696 throw new Error("Attempt to auto-create CodeSystem.approvalDate"); 3697 else if (Configuration.doAutoCreate()) 3698 this.approvalDate = new DateType(); // bb 3699 return this.approvalDate; 3700 } 3701 3702 public boolean hasApprovalDateElement() { 3703 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3704 } 3705 3706 public boolean hasApprovalDate() { 3707 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3708 } 3709 3710 /** 3711 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3712 */ 3713 public CodeSystem setApprovalDateElement(DateType value) { 3714 this.approvalDate = value; 3715 return this; 3716 } 3717 3718 /** 3719 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3720 */ 3721 public Date getApprovalDate() { 3722 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3723 } 3724 3725 /** 3726 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3727 */ 3728 public CodeSystem setApprovalDate(Date value) { 3729 if (value == null) 3730 this.approvalDate = null; 3731 else { 3732 if (this.approvalDate == null) 3733 this.approvalDate = new DateType(); 3734 this.approvalDate.setValue(value); 3735 } 3736 return this; 3737 } 3738 3739 /** 3740 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3741 */ 3742 public DateType getLastReviewDateElement() { 3743 if (this.lastReviewDate == null) 3744 if (Configuration.errorOnAutoCreate()) 3745 throw new Error("Attempt to auto-create CodeSystem.lastReviewDate"); 3746 else if (Configuration.doAutoCreate()) 3747 this.lastReviewDate = new DateType(); // bb 3748 return this.lastReviewDate; 3749 } 3750 3751 public boolean hasLastReviewDateElement() { 3752 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3753 } 3754 3755 public boolean hasLastReviewDate() { 3756 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3757 } 3758 3759 /** 3760 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3761 */ 3762 public CodeSystem setLastReviewDateElement(DateType value) { 3763 this.lastReviewDate = value; 3764 return this; 3765 } 3766 3767 /** 3768 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3769 */ 3770 public Date getLastReviewDate() { 3771 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3772 } 3773 3774 /** 3775 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3776 */ 3777 public CodeSystem setLastReviewDate(Date value) { 3778 if (value == null) 3779 this.lastReviewDate = null; 3780 else { 3781 if (this.lastReviewDate == null) 3782 this.lastReviewDate = new DateType(); 3783 this.lastReviewDate.setValue(value); 3784 } 3785 return this; 3786 } 3787 3788 /** 3789 * @return {@link #effectivePeriod} (The period during which the CodeSystem content was or is planned to be in active use.) 3790 */ 3791 public Period getEffectivePeriod() { 3792 if (this.effectivePeriod == null) 3793 if (Configuration.errorOnAutoCreate()) 3794 throw new Error("Attempt to auto-create CodeSystem.effectivePeriod"); 3795 else if (Configuration.doAutoCreate()) 3796 this.effectivePeriod = new Period(); // cc 3797 return this.effectivePeriod; 3798 } 3799 3800 public boolean hasEffectivePeriod() { 3801 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3802 } 3803 3804 /** 3805 * @param value {@link #effectivePeriod} (The period during which the CodeSystem content was or is planned to be in active use.) 3806 */ 3807 public CodeSystem setEffectivePeriod(Period value) { 3808 this.effectivePeriod = value; 3809 return this; 3810 } 3811 3812 /** 3813 * @return {@link #topic} (Descriptions related to the content of the CodeSystem. Topics provide a high-level categorization as well as keywords for the CodeSystem that can be useful for filtering and searching.) 3814 */ 3815 public List<CodeableConcept> getTopic() { 3816 if (this.topic == null) 3817 this.topic = new ArrayList<CodeableConcept>(); 3818 return this.topic; 3819 } 3820 3821 /** 3822 * @return Returns a reference to <code>this</code> for easy method chaining 3823 */ 3824 public CodeSystem setTopic(List<CodeableConcept> theTopic) { 3825 this.topic = theTopic; 3826 return this; 3827 } 3828 3829 public boolean hasTopic() { 3830 if (this.topic == null) 3831 return false; 3832 for (CodeableConcept item : this.topic) 3833 if (!item.isEmpty()) 3834 return true; 3835 return false; 3836 } 3837 3838 public CodeableConcept addTopic() { //3 3839 CodeableConcept t = new CodeableConcept(); 3840 if (this.topic == null) 3841 this.topic = new ArrayList<CodeableConcept>(); 3842 this.topic.add(t); 3843 return t; 3844 } 3845 3846 public CodeSystem addTopic(CodeableConcept t) { //3 3847 if (t == null) 3848 return this; 3849 if (this.topic == null) 3850 this.topic = new ArrayList<CodeableConcept>(); 3851 this.topic.add(t); 3852 return this; 3853 } 3854 3855 /** 3856 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 3857 */ 3858 public CodeableConcept getTopicFirstRep() { 3859 if (getTopic().isEmpty()) { 3860 addTopic(); 3861 } 3862 return getTopic().get(0); 3863 } 3864 3865 /** 3866 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the CodeSystem.) 3867 */ 3868 public List<ContactDetail> getAuthor() { 3869 if (this.author == null) 3870 this.author = new ArrayList<ContactDetail>(); 3871 return this.author; 3872 } 3873 3874 /** 3875 * @return Returns a reference to <code>this</code> for easy method chaining 3876 */ 3877 public CodeSystem setAuthor(List<ContactDetail> theAuthor) { 3878 this.author = theAuthor; 3879 return this; 3880 } 3881 3882 public boolean hasAuthor() { 3883 if (this.author == null) 3884 return false; 3885 for (ContactDetail item : this.author) 3886 if (!item.isEmpty()) 3887 return true; 3888 return false; 3889 } 3890 3891 public ContactDetail addAuthor() { //3 3892 ContactDetail t = new ContactDetail(); 3893 if (this.author == null) 3894 this.author = new ArrayList<ContactDetail>(); 3895 this.author.add(t); 3896 return t; 3897 } 3898 3899 public CodeSystem addAuthor(ContactDetail t) { //3 3900 if (t == null) 3901 return this; 3902 if (this.author == null) 3903 this.author = new ArrayList<ContactDetail>(); 3904 this.author.add(t); 3905 return this; 3906 } 3907 3908 /** 3909 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 3910 */ 3911 public ContactDetail getAuthorFirstRep() { 3912 if (getAuthor().isEmpty()) { 3913 addAuthor(); 3914 } 3915 return getAuthor().get(0); 3916 } 3917 3918 /** 3919 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the CodeSystem.) 3920 */ 3921 public List<ContactDetail> getEditor() { 3922 if (this.editor == null) 3923 this.editor = new ArrayList<ContactDetail>(); 3924 return this.editor; 3925 } 3926 3927 /** 3928 * @return Returns a reference to <code>this</code> for easy method chaining 3929 */ 3930 public CodeSystem setEditor(List<ContactDetail> theEditor) { 3931 this.editor = theEditor; 3932 return this; 3933 } 3934 3935 public boolean hasEditor() { 3936 if (this.editor == null) 3937 return false; 3938 for (ContactDetail item : this.editor) 3939 if (!item.isEmpty()) 3940 return true; 3941 return false; 3942 } 3943 3944 public ContactDetail addEditor() { //3 3945 ContactDetail t = new ContactDetail(); 3946 if (this.editor == null) 3947 this.editor = new ArrayList<ContactDetail>(); 3948 this.editor.add(t); 3949 return t; 3950 } 3951 3952 public CodeSystem addEditor(ContactDetail t) { //3 3953 if (t == null) 3954 return this; 3955 if (this.editor == null) 3956 this.editor = new ArrayList<ContactDetail>(); 3957 this.editor.add(t); 3958 return this; 3959 } 3960 3961 /** 3962 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 3963 */ 3964 public ContactDetail getEditorFirstRep() { 3965 if (getEditor().isEmpty()) { 3966 addEditor(); 3967 } 3968 return getEditor().get(0); 3969 } 3970 3971 /** 3972 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the CodeSystem.) 3973 */ 3974 public List<ContactDetail> getReviewer() { 3975 if (this.reviewer == null) 3976 this.reviewer = new ArrayList<ContactDetail>(); 3977 return this.reviewer; 3978 } 3979 3980 /** 3981 * @return Returns a reference to <code>this</code> for easy method chaining 3982 */ 3983 public CodeSystem setReviewer(List<ContactDetail> theReviewer) { 3984 this.reviewer = theReviewer; 3985 return this; 3986 } 3987 3988 public boolean hasReviewer() { 3989 if (this.reviewer == null) 3990 return false; 3991 for (ContactDetail item : this.reviewer) 3992 if (!item.isEmpty()) 3993 return true; 3994 return false; 3995 } 3996 3997 public ContactDetail addReviewer() { //3 3998 ContactDetail t = new ContactDetail(); 3999 if (this.reviewer == null) 4000 this.reviewer = new ArrayList<ContactDetail>(); 4001 this.reviewer.add(t); 4002 return t; 4003 } 4004 4005 public CodeSystem addReviewer(ContactDetail t) { //3 4006 if (t == null) 4007 return this; 4008 if (this.reviewer == null) 4009 this.reviewer = new ArrayList<ContactDetail>(); 4010 this.reviewer.add(t); 4011 return this; 4012 } 4013 4014 /** 4015 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 4016 */ 4017 public ContactDetail getReviewerFirstRep() { 4018 if (getReviewer().isEmpty()) { 4019 addReviewer(); 4020 } 4021 return getReviewer().get(0); 4022 } 4023 4024 /** 4025 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the CodeSystem for use in some setting.) 4026 */ 4027 public List<ContactDetail> getEndorser() { 4028 if (this.endorser == null) 4029 this.endorser = new ArrayList<ContactDetail>(); 4030 return this.endorser; 4031 } 4032 4033 /** 4034 * @return Returns a reference to <code>this</code> for easy method chaining 4035 */ 4036 public CodeSystem setEndorser(List<ContactDetail> theEndorser) { 4037 this.endorser = theEndorser; 4038 return this; 4039 } 4040 4041 public boolean hasEndorser() { 4042 if (this.endorser == null) 4043 return false; 4044 for (ContactDetail item : this.endorser) 4045 if (!item.isEmpty()) 4046 return true; 4047 return false; 4048 } 4049 4050 public ContactDetail addEndorser() { //3 4051 ContactDetail t = new ContactDetail(); 4052 if (this.endorser == null) 4053 this.endorser = new ArrayList<ContactDetail>(); 4054 this.endorser.add(t); 4055 return t; 4056 } 4057 4058 public CodeSystem addEndorser(ContactDetail t) { //3 4059 if (t == null) 4060 return this; 4061 if (this.endorser == null) 4062 this.endorser = new ArrayList<ContactDetail>(); 4063 this.endorser.add(t); 4064 return this; 4065 } 4066 4067 /** 4068 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 4069 */ 4070 public ContactDetail getEndorserFirstRep() { 4071 if (getEndorser().isEmpty()) { 4072 addEndorser(); 4073 } 4074 return getEndorser().get(0); 4075 } 4076 4077 /** 4078 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 4079 */ 4080 public List<RelatedArtifact> getRelatedArtifact() { 4081 if (this.relatedArtifact == null) 4082 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4083 return this.relatedArtifact; 4084 } 4085 4086 /** 4087 * @return Returns a reference to <code>this</code> for easy method chaining 4088 */ 4089 public CodeSystem setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4090 this.relatedArtifact = theRelatedArtifact; 4091 return this; 4092 } 4093 4094 public boolean hasRelatedArtifact() { 4095 if (this.relatedArtifact == null) 4096 return false; 4097 for (RelatedArtifact item : this.relatedArtifact) 4098 if (!item.isEmpty()) 4099 return true; 4100 return false; 4101 } 4102 4103 public RelatedArtifact addRelatedArtifact() { //3 4104 RelatedArtifact t = new RelatedArtifact(); 4105 if (this.relatedArtifact == null) 4106 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4107 this.relatedArtifact.add(t); 4108 return t; 4109 } 4110 4111 public CodeSystem addRelatedArtifact(RelatedArtifact t) { //3 4112 if (t == null) 4113 return this; 4114 if (this.relatedArtifact == null) 4115 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4116 this.relatedArtifact.add(t); 4117 return this; 4118 } 4119 4120 /** 4121 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 4122 */ 4123 public RelatedArtifact getRelatedArtifactFirstRep() { 4124 if (getRelatedArtifact().isEmpty()) { 4125 addRelatedArtifact(); 4126 } 4127 return getRelatedArtifact().get(0); 4128 } 4129 4130 /** 4131 * @return {@link #caseSensitive} (If code comparison is case sensitive when codes within this system are compared to each other.). This is the underlying object with id, value and extensions. The accessor "getCaseSensitive" gives direct access to the value 4132 */ 4133 public BooleanType getCaseSensitiveElement() { 4134 if (this.caseSensitive == null) 4135 if (Configuration.errorOnAutoCreate()) 4136 throw new Error("Attempt to auto-create CodeSystem.caseSensitive"); 4137 else if (Configuration.doAutoCreate()) 4138 this.caseSensitive = new BooleanType(); // bb 4139 return this.caseSensitive; 4140 } 4141 4142 public boolean hasCaseSensitiveElement() { 4143 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 4144 } 4145 4146 public boolean hasCaseSensitive() { 4147 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 4148 } 4149 4150 /** 4151 * @param value {@link #caseSensitive} (If code comparison is case sensitive when codes within this system are compared to each other.). This is the underlying object with id, value and extensions. The accessor "getCaseSensitive" gives direct access to the value 4152 */ 4153 public CodeSystem setCaseSensitiveElement(BooleanType value) { 4154 this.caseSensitive = value; 4155 return this; 4156 } 4157 4158 /** 4159 * @return If code comparison is case sensitive when codes within this system are compared to each other. 4160 */ 4161 public boolean getCaseSensitive() { 4162 return this.caseSensitive == null || this.caseSensitive.isEmpty() ? false : this.caseSensitive.getValue(); 4163 } 4164 4165 /** 4166 * @param value If code comparison is case sensitive when codes within this system are compared to each other. 4167 */ 4168 public CodeSystem setCaseSensitive(boolean value) { 4169 if (this.caseSensitive == null) 4170 this.caseSensitive = new BooleanType(); 4171 this.caseSensitive.setValue(value); 4172 return this; 4173 } 4174 4175 /** 4176 * @return {@link #valueSet} (Canonical reference to the value set that contains all codes in the code system independent of code status.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4177 */ 4178 public CanonicalType getValueSetElement() { 4179 if (this.valueSet == null) 4180 if (Configuration.errorOnAutoCreate()) 4181 throw new Error("Attempt to auto-create CodeSystem.valueSet"); 4182 else if (Configuration.doAutoCreate()) 4183 this.valueSet = new CanonicalType(); // bb 4184 return this.valueSet; 4185 } 4186 4187 public boolean hasValueSetElement() { 4188 return this.valueSet != null && !this.valueSet.isEmpty(); 4189 } 4190 4191 public boolean hasValueSet() { 4192 return this.valueSet != null && !this.valueSet.isEmpty(); 4193 } 4194 4195 /** 4196 * @param value {@link #valueSet} (Canonical reference to the value set that contains all codes in the code system independent of code status.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4197 */ 4198 public CodeSystem setValueSetElement(CanonicalType value) { 4199 this.valueSet = value; 4200 return this; 4201 } 4202 4203 /** 4204 * @return Canonical reference to the value set that contains all codes in the code system independent of code status. 4205 */ 4206 public String getValueSet() { 4207 return this.valueSet == null ? null : this.valueSet.getValue(); 4208 } 4209 4210 /** 4211 * @param value Canonical reference to the value set that contains all codes in the code system independent of code status. 4212 */ 4213 public CodeSystem setValueSet(String value) { 4214 if (Utilities.noString(value)) 4215 this.valueSet = null; 4216 else { 4217 if (this.valueSet == null) 4218 this.valueSet = new CanonicalType(); 4219 this.valueSet.setValue(value); 4220 } 4221 return this; 4222 } 4223 4224 /** 4225 * @return {@link #hierarchyMeaning} (The meaning of the hierarchy of concepts as represented in this resource.). This is the underlying object with id, value and extensions. The accessor "getHierarchyMeaning" gives direct access to the value 4226 */ 4227 public Enumeration<CodeSystemHierarchyMeaning> getHierarchyMeaningElement() { 4228 if (this.hierarchyMeaning == null) 4229 if (Configuration.errorOnAutoCreate()) 4230 throw new Error("Attempt to auto-create CodeSystem.hierarchyMeaning"); 4231 else if (Configuration.doAutoCreate()) 4232 this.hierarchyMeaning = new Enumeration<CodeSystemHierarchyMeaning>(new CodeSystemHierarchyMeaningEnumFactory()); // bb 4233 return this.hierarchyMeaning; 4234 } 4235 4236 public boolean hasHierarchyMeaningElement() { 4237 return this.hierarchyMeaning != null && !this.hierarchyMeaning.isEmpty(); 4238 } 4239 4240 public boolean hasHierarchyMeaning() { 4241 return this.hierarchyMeaning != null && !this.hierarchyMeaning.isEmpty(); 4242 } 4243 4244 /** 4245 * @param value {@link #hierarchyMeaning} (The meaning of the hierarchy of concepts as represented in this resource.). This is the underlying object with id, value and extensions. The accessor "getHierarchyMeaning" gives direct access to the value 4246 */ 4247 public CodeSystem setHierarchyMeaningElement(Enumeration<CodeSystemHierarchyMeaning> value) { 4248 this.hierarchyMeaning = value; 4249 return this; 4250 } 4251 4252 /** 4253 * @return The meaning of the hierarchy of concepts as represented in this resource. 4254 */ 4255 public CodeSystemHierarchyMeaning getHierarchyMeaning() { 4256 return this.hierarchyMeaning == null ? null : this.hierarchyMeaning.getValue(); 4257 } 4258 4259 /** 4260 * @param value The meaning of the hierarchy of concepts as represented in this resource. 4261 */ 4262 public CodeSystem setHierarchyMeaning(CodeSystemHierarchyMeaning value) { 4263 if (value == null) 4264 this.hierarchyMeaning = null; 4265 else { 4266 if (this.hierarchyMeaning == null) 4267 this.hierarchyMeaning = new Enumeration<CodeSystemHierarchyMeaning>(new CodeSystemHierarchyMeaningEnumFactory()); 4268 this.hierarchyMeaning.setValue(value); 4269 } 4270 return this; 4271 } 4272 4273 /** 4274 * @return {@link #compositional} (The code system defines a compositional (post-coordination) grammar.). This is the underlying object with id, value and extensions. The accessor "getCompositional" gives direct access to the value 4275 */ 4276 public BooleanType getCompositionalElement() { 4277 if (this.compositional == null) 4278 if (Configuration.errorOnAutoCreate()) 4279 throw new Error("Attempt to auto-create CodeSystem.compositional"); 4280 else if (Configuration.doAutoCreate()) 4281 this.compositional = new BooleanType(); // bb 4282 return this.compositional; 4283 } 4284 4285 public boolean hasCompositionalElement() { 4286 return this.compositional != null && !this.compositional.isEmpty(); 4287 } 4288 4289 public boolean hasCompositional() { 4290 return this.compositional != null && !this.compositional.isEmpty(); 4291 } 4292 4293 /** 4294 * @param value {@link #compositional} (The code system defines a compositional (post-coordination) grammar.). This is the underlying object with id, value and extensions. The accessor "getCompositional" gives direct access to the value 4295 */ 4296 public CodeSystem setCompositionalElement(BooleanType value) { 4297 this.compositional = value; 4298 return this; 4299 } 4300 4301 /** 4302 * @return The code system defines a compositional (post-coordination) grammar. 4303 */ 4304 public boolean getCompositional() { 4305 return this.compositional == null || this.compositional.isEmpty() ? false : this.compositional.getValue(); 4306 } 4307 4308 /** 4309 * @param value The code system defines a compositional (post-coordination) grammar. 4310 */ 4311 public CodeSystem setCompositional(boolean value) { 4312 if (this.compositional == null) 4313 this.compositional = new BooleanType(); 4314 this.compositional.setValue(value); 4315 return this; 4316 } 4317 4318 /** 4319 * @return {@link #versionNeeded} (This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.). This is the underlying object with id, value and extensions. The accessor "getVersionNeeded" gives direct access to the value 4320 */ 4321 public BooleanType getVersionNeededElement() { 4322 if (this.versionNeeded == null) 4323 if (Configuration.errorOnAutoCreate()) 4324 throw new Error("Attempt to auto-create CodeSystem.versionNeeded"); 4325 else if (Configuration.doAutoCreate()) 4326 this.versionNeeded = new BooleanType(); // bb 4327 return this.versionNeeded; 4328 } 4329 4330 public boolean hasVersionNeededElement() { 4331 return this.versionNeeded != null && !this.versionNeeded.isEmpty(); 4332 } 4333 4334 public boolean hasVersionNeeded() { 4335 return this.versionNeeded != null && !this.versionNeeded.isEmpty(); 4336 } 4337 4338 /** 4339 * @param value {@link #versionNeeded} (This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.). This is the underlying object with id, value and extensions. The accessor "getVersionNeeded" gives direct access to the value 4340 */ 4341 public CodeSystem setVersionNeededElement(BooleanType value) { 4342 this.versionNeeded = value; 4343 return this; 4344 } 4345 4346 /** 4347 * @return This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system. 4348 */ 4349 public boolean getVersionNeeded() { 4350 return this.versionNeeded == null || this.versionNeeded.isEmpty() ? false : this.versionNeeded.getValue(); 4351 } 4352 4353 /** 4354 * @param value This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system. 4355 */ 4356 public CodeSystem setVersionNeeded(boolean value) { 4357 if (this.versionNeeded == null) 4358 this.versionNeeded = new BooleanType(); 4359 this.versionNeeded.setValue(value); 4360 return this; 4361 } 4362 4363 /** 4364 * @return {@link #content} (The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 4365 */ 4366 public Enumeration<CodeSystemContentMode> getContentElement() { 4367 if (this.content == null) 4368 if (Configuration.errorOnAutoCreate()) 4369 throw new Error("Attempt to auto-create CodeSystem.content"); 4370 else if (Configuration.doAutoCreate()) 4371 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); // bb 4372 return this.content; 4373 } 4374 4375 public boolean hasContentElement() { 4376 return this.content != null && !this.content.isEmpty(); 4377 } 4378 4379 public boolean hasContent() { 4380 return this.content != null && !this.content.isEmpty(); 4381 } 4382 4383 /** 4384 * @param value {@link #content} (The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 4385 */ 4386 public CodeSystem setContentElement(Enumeration<CodeSystemContentMode> value) { 4387 this.content = value; 4388 return this; 4389 } 4390 4391 /** 4392 * @return The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance. 4393 */ 4394 public CodeSystemContentMode getContent() { 4395 return this.content == null ? null : this.content.getValue(); 4396 } 4397 4398 /** 4399 * @param value The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance. 4400 */ 4401 public CodeSystem setContent(CodeSystemContentMode value) { 4402 if (this.content == null) 4403 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); 4404 this.content.setValue(value); 4405 return this; 4406 } 4407 4408 /** 4409 * @return {@link #supplements} (The canonical URL of the code system that this code system supplement is adding designations and properties to.). This is the underlying object with id, value and extensions. The accessor "getSupplements" gives direct access to the value 4410 */ 4411 public CanonicalType getSupplementsElement() { 4412 if (this.supplements == null) 4413 if (Configuration.errorOnAutoCreate()) 4414 throw new Error("Attempt to auto-create CodeSystem.supplements"); 4415 else if (Configuration.doAutoCreate()) 4416 this.supplements = new CanonicalType(); // bb 4417 return this.supplements; 4418 } 4419 4420 public boolean hasSupplementsElement() { 4421 return this.supplements != null && !this.supplements.isEmpty(); 4422 } 4423 4424 public boolean hasSupplements() { 4425 return this.supplements != null && !this.supplements.isEmpty(); 4426 } 4427 4428 /** 4429 * @param value {@link #supplements} (The canonical URL of the code system that this code system supplement is adding designations and properties to.). This is the underlying object with id, value and extensions. The accessor "getSupplements" gives direct access to the value 4430 */ 4431 public CodeSystem setSupplementsElement(CanonicalType value) { 4432 this.supplements = value; 4433 return this; 4434 } 4435 4436 /** 4437 * @return The canonical URL of the code system that this code system supplement is adding designations and properties to. 4438 */ 4439 public String getSupplements() { 4440 return this.supplements == null ? null : this.supplements.getValue(); 4441 } 4442 4443 /** 4444 * @param value The canonical URL of the code system that this code system supplement is adding designations and properties to. 4445 */ 4446 public CodeSystem setSupplements(String value) { 4447 if (Utilities.noString(value)) 4448 this.supplements = null; 4449 else { 4450 if (this.supplements == null) 4451 this.supplements = new CanonicalType(); 4452 this.supplements.setValue(value); 4453 } 4454 return this; 4455 } 4456 4457 /** 4458 * @return {@link #count} (The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 4459 */ 4460 public UnsignedIntType getCountElement() { 4461 if (this.count == null) 4462 if (Configuration.errorOnAutoCreate()) 4463 throw new Error("Attempt to auto-create CodeSystem.count"); 4464 else if (Configuration.doAutoCreate()) 4465 this.count = new UnsignedIntType(); // bb 4466 return this.count; 4467 } 4468 4469 public boolean hasCountElement() { 4470 return this.count != null && !this.count.isEmpty(); 4471 } 4472 4473 public boolean hasCount() { 4474 return this.count != null && !this.count.isEmpty(); 4475 } 4476 4477 /** 4478 * @param value {@link #count} (The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 4479 */ 4480 public CodeSystem setCountElement(UnsignedIntType value) { 4481 this.count = value; 4482 return this; 4483 } 4484 4485 /** 4486 * @return The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward. 4487 */ 4488 public int getCount() { 4489 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 4490 } 4491 4492 /** 4493 * @param value The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward. 4494 */ 4495 public CodeSystem setCount(int value) { 4496 if (this.count == null) 4497 this.count = new UnsignedIntType(); 4498 this.count.setValue(value); 4499 return this; 4500 } 4501 4502 /** 4503 * @return {@link #filter} (A filter that can be used in a value set compose statement when selecting concepts using a filter.) 4504 */ 4505 public List<CodeSystemFilterComponent> getFilter() { 4506 if (this.filter == null) 4507 this.filter = new ArrayList<CodeSystemFilterComponent>(); 4508 return this.filter; 4509 } 4510 4511 /** 4512 * @return Returns a reference to <code>this</code> for easy method chaining 4513 */ 4514 public CodeSystem setFilter(List<CodeSystemFilterComponent> theFilter) { 4515 this.filter = theFilter; 4516 return this; 4517 } 4518 4519 public boolean hasFilter() { 4520 if (this.filter == null) 4521 return false; 4522 for (CodeSystemFilterComponent item : this.filter) 4523 if (!item.isEmpty()) 4524 return true; 4525 return false; 4526 } 4527 4528 public CodeSystemFilterComponent addFilter() { //3 4529 CodeSystemFilterComponent t = new CodeSystemFilterComponent(); 4530 if (this.filter == null) 4531 this.filter = new ArrayList<CodeSystemFilterComponent>(); 4532 this.filter.add(t); 4533 return t; 4534 } 4535 4536 public CodeSystem addFilter(CodeSystemFilterComponent t) { //3 4537 if (t == null) 4538 return this; 4539 if (this.filter == null) 4540 this.filter = new ArrayList<CodeSystemFilterComponent>(); 4541 this.filter.add(t); 4542 return this; 4543 } 4544 4545 /** 4546 * @return The first repetition of repeating field {@link #filter}, creating it if it does not already exist {3} 4547 */ 4548 public CodeSystemFilterComponent getFilterFirstRep() { 4549 if (getFilter().isEmpty()) { 4550 addFilter(); 4551 } 4552 return getFilter().get(0); 4553 } 4554 4555 /** 4556 * @return {@link #property} (A property defines an additional slot through which additional information can be provided about a concept.) 4557 */ 4558 public List<PropertyComponent> getProperty() { 4559 if (this.property == null) 4560 this.property = new ArrayList<PropertyComponent>(); 4561 return this.property; 4562 } 4563 4564 /** 4565 * @return Returns a reference to <code>this</code> for easy method chaining 4566 */ 4567 public CodeSystem setProperty(List<PropertyComponent> theProperty) { 4568 this.property = theProperty; 4569 return this; 4570 } 4571 4572 public boolean hasProperty() { 4573 if (this.property == null) 4574 return false; 4575 for (PropertyComponent item : this.property) 4576 if (!item.isEmpty()) 4577 return true; 4578 return false; 4579 } 4580 4581 public PropertyComponent addProperty() { //3 4582 PropertyComponent t = new PropertyComponent(); 4583 if (this.property == null) 4584 this.property = new ArrayList<PropertyComponent>(); 4585 this.property.add(t); 4586 return t; 4587 } 4588 4589 public CodeSystem addProperty(PropertyComponent t) { //3 4590 if (t == null) 4591 return this; 4592 if (this.property == null) 4593 this.property = new ArrayList<PropertyComponent>(); 4594 this.property.add(t); 4595 return this; 4596 } 4597 4598 /** 4599 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 4600 */ 4601 public PropertyComponent getPropertyFirstRep() { 4602 if (getProperty().isEmpty()) { 4603 addProperty(); 4604 } 4605 return getProperty().get(0); 4606 } 4607 4608 /** 4609 * @return {@link #concept} (Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are.) 4610 */ 4611 public List<ConceptDefinitionComponent> getConcept() { 4612 if (this.concept == null) 4613 this.concept = new ArrayList<ConceptDefinitionComponent>(); 4614 return this.concept; 4615 } 4616 4617 /** 4618 * @return Returns a reference to <code>this</code> for easy method chaining 4619 */ 4620 public CodeSystem setConcept(List<ConceptDefinitionComponent> theConcept) { 4621 this.concept = theConcept; 4622 return this; 4623 } 4624 4625 public boolean hasConcept() { 4626 if (this.concept == null) 4627 return false; 4628 for (ConceptDefinitionComponent item : this.concept) 4629 if (!item.isEmpty()) 4630 return true; 4631 return false; 4632 } 4633 4634 public ConceptDefinitionComponent addConcept() { //3 4635 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 4636 if (this.concept == null) 4637 this.concept = new ArrayList<ConceptDefinitionComponent>(); 4638 this.concept.add(t); 4639 return t; 4640 } 4641 4642 public CodeSystem addConcept(ConceptDefinitionComponent t) { //3 4643 if (t == null) 4644 return this; 4645 if (this.concept == null) 4646 this.concept = new ArrayList<ConceptDefinitionComponent>(); 4647 this.concept.add(t); 4648 return this; 4649 } 4650 4651 /** 4652 * @return The first repetition of repeating field {@link #concept}, creating it if it does not already exist {3} 4653 */ 4654 public ConceptDefinitionComponent getConceptFirstRep() { 4655 if (getConcept().isEmpty()) { 4656 addConcept(); 4657 } 4658 return getConcept().get(0); 4659 } 4660 4661 protected void listChildren(List<Property> children) { 4662 super.listChildren(children); 4663 children.add(new Property("url", "uri", "An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system.", 0, 1, url)); 4664 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4665 children.add(new Property("version", "string", "The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version.", 0, 1, version)); 4666 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which CodeSystem is more current.", 0, 1, versionAlgorithm)); 4667 children.add(new Property("name", "string", "A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4668 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the code system.", 0, 1, title)); 4669 children.add(new Property("status", "code", "The status of this code system. Enables tracking the life-cycle of the content.", 0, 1, status)); 4670 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 4671 children.add(new Property("date", "dateTime", "The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.", 0, 1, date)); 4672 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the code system.", 0, 1, publisher)); 4673 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4674 children.add(new Property("description", "markdown", "A free text natural language description of the code system from a consumer's perspective.", 0, 1, description)); 4675 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4676 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the code system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 4677 children.add(new Property("purpose", "markdown", "Explanation of why this code system is needed and why it has been designed as it has.", 0, 1, purpose)); 4678 children.add(new Property("copyright", "markdown", "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.", 0, 1, copyright)); 4679 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 4680 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 4681 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 4682 children.add(new Property("effectivePeriod", "Period", "The period during which the CodeSystem content was or is planned to be in active use.", 0, 1, effectivePeriod)); 4683 children.add(new Property("topic", "CodeableConcept", "Descriptions related to the content of the CodeSystem. Topics provide a high-level categorization as well as keywords for the CodeSystem that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 4684 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the CodeSystem.", 0, java.lang.Integer.MAX_VALUE, author)); 4685 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the CodeSystem.", 0, java.lang.Integer.MAX_VALUE, editor)); 4686 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the CodeSystem.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 4687 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the CodeSystem for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 4688 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4689 children.add(new Property("caseSensitive", "boolean", "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 1, caseSensitive)); 4690 children.add(new Property("valueSet", "canonical(ValueSet)", "Canonical reference to the value set that contains all codes in the code system independent of code status.", 0, 1, valueSet)); 4691 children.add(new Property("hierarchyMeaning", "code", "The meaning of the hierarchy of concepts as represented in this resource.", 0, 1, hierarchyMeaning)); 4692 children.add(new Property("compositional", "boolean", "The code system defines a compositional (post-coordination) grammar.", 0, 1, compositional)); 4693 children.add(new Property("versionNeeded", "boolean", "This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.", 0, 1, versionNeeded)); 4694 children.add(new Property("content", "code", "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.", 0, 1, content)); 4695 children.add(new Property("supplements", "canonical(CodeSystem)", "The canonical URL of the code system that this code system supplement is adding designations and properties to.", 0, 1, supplements)); 4696 children.add(new Property("count", "unsignedInt", "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.", 0, 1, count)); 4697 children.add(new Property("filter", "", "A filter that can be used in a value set compose statement when selecting concepts using a filter.", 0, java.lang.Integer.MAX_VALUE, filter)); 4698 children.add(new Property("property", "", "A property defines an additional slot through which additional information can be provided about a concept.", 0, java.lang.Integer.MAX_VALUE, property)); 4699 children.add(new Property("concept", "", "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are.", 0, java.lang.Integer.MAX_VALUE, concept)); 4700 } 4701 4702 @Override 4703 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4704 switch (_hash) { 4705 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system.", 0, 1, url); 4706 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4707 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version.", 0, 1, version); 4708 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which CodeSystem is more current.", 0, 1, versionAlgorithm); 4709 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which CodeSystem is more current.", 0, 1, versionAlgorithm); 4710 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which CodeSystem is more current.", 0, 1, versionAlgorithm); 4711 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which CodeSystem is more current.", 0, 1, versionAlgorithm); 4712 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4713 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the code system.", 0, 1, title); 4714 case -892481550: /*status*/ return new Property("status", "code", "The status of this code system. Enables tracking the life-cycle of the content.", 0, 1, status); 4715 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 4716 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the code system was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.", 0, 1, date); 4717 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the code system.", 0, 1, publisher); 4718 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4719 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the code system from a consumer's perspective.", 0, 1, description); 4720 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4721 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the code system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4722 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this code system is needed and why it has been designed as it has.", 0, 1, purpose); 4723 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.", 0, 1, copyright); 4724 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 4725 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 4726 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 4727 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the CodeSystem content was or is planned to be in active use.", 0, 1, effectivePeriod); 4728 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptions related to the content of the CodeSystem. Topics provide a high-level categorization as well as keywords for the CodeSystem that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 4729 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the CodeSystem.", 0, java.lang.Integer.MAX_VALUE, author); 4730 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the CodeSystem.", 0, java.lang.Integer.MAX_VALUE, editor); 4731 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the CodeSystem.", 0, java.lang.Integer.MAX_VALUE, reviewer); 4732 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the CodeSystem for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 4733 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4734 case -35616442: /*caseSensitive*/ return new Property("caseSensitive", "boolean", "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 1, caseSensitive); 4735 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "Canonical reference to the value set that contains all codes in the code system independent of code status.", 0, 1, valueSet); 4736 case 1913078280: /*hierarchyMeaning*/ return new Property("hierarchyMeaning", "code", "The meaning of the hierarchy of concepts as represented in this resource.", 0, 1, hierarchyMeaning); 4737 case 1248023381: /*compositional*/ return new Property("compositional", "boolean", "The code system defines a compositional (post-coordination) grammar.", 0, 1, compositional); 4738 case 617270957: /*versionNeeded*/ return new Property("versionNeeded", "boolean", "This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.", 0, 1, versionNeeded); 4739 case 951530617: /*content*/ return new Property("content", "code", "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.", 0, 1, content); 4740 case -596951334: /*supplements*/ return new Property("supplements", "canonical(CodeSystem)", "The canonical URL of the code system that this code system supplement is adding designations and properties to.", 0, 1, supplements); 4741 case 94851343: /*count*/ return new Property("count", "unsignedInt", "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.", 0, 1, count); 4742 case -1274492040: /*filter*/ return new Property("filter", "", "A filter that can be used in a value set compose statement when selecting concepts using a filter.", 0, java.lang.Integer.MAX_VALUE, filter); 4743 case -993141291: /*property*/ return new Property("property", "", "A property defines an additional slot through which additional information can be provided about a concept.", 0, java.lang.Integer.MAX_VALUE, property); 4744 case 951024232: /*concept*/ return new Property("concept", "", "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are.", 0, java.lang.Integer.MAX_VALUE, concept); 4745 default: return super.getNamedProperty(_hash, _name, _checkValid); 4746 } 4747 4748 } 4749 4750 @Override 4751 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4752 switch (hash) { 4753 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4754 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4755 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4756 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4757 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4758 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4759 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4760 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4761 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4762 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4763 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4764 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4765 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4766 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4767 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4768 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4769 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4770 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4771 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4772 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4773 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 4774 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4775 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4776 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4777 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4778 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4779 case -35616442: /*caseSensitive*/ return this.caseSensitive == null ? new Base[0] : new Base[] {this.caseSensitive}; // BooleanType 4780 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 4781 case 1913078280: /*hierarchyMeaning*/ return this.hierarchyMeaning == null ? new Base[0] : new Base[] {this.hierarchyMeaning}; // Enumeration<CodeSystemHierarchyMeaning> 4782 case 1248023381: /*compositional*/ return this.compositional == null ? new Base[0] : new Base[] {this.compositional}; // BooleanType 4783 case 617270957: /*versionNeeded*/ return this.versionNeeded == null ? new Base[0] : new Base[] {this.versionNeeded}; // BooleanType 4784 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Enumeration<CodeSystemContentMode> 4785 case -596951334: /*supplements*/ return this.supplements == null ? new Base[0] : new Base[] {this.supplements}; // CanonicalType 4786 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // UnsignedIntType 4787 case -1274492040: /*filter*/ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // CodeSystemFilterComponent 4788 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // PropertyComponent 4789 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptDefinitionComponent 4790 default: return super.getProperty(hash, name, checkValid); 4791 } 4792 4793 } 4794 4795 @Override 4796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4797 switch (hash) { 4798 case 116079: // url 4799 this.url = TypeConvertor.castToUri(value); // UriType 4800 return value; 4801 case -1618432855: // identifier 4802 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4803 return value; 4804 case 351608024: // version 4805 this.version = TypeConvertor.castToString(value); // StringType 4806 return value; 4807 case 1508158071: // versionAlgorithm 4808 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4809 return value; 4810 case 3373707: // name 4811 this.name = TypeConvertor.castToString(value); // StringType 4812 return value; 4813 case 110371416: // title 4814 this.title = TypeConvertor.castToString(value); // StringType 4815 return value; 4816 case -892481550: // status 4817 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4818 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4819 return value; 4820 case -404562712: // experimental 4821 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4822 return value; 4823 case 3076014: // date 4824 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4825 return value; 4826 case 1447404028: // publisher 4827 this.publisher = TypeConvertor.castToString(value); // StringType 4828 return value; 4829 case 951526432: // contact 4830 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4831 return value; 4832 case -1724546052: // description 4833 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4834 return value; 4835 case -669707736: // useContext 4836 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4837 return value; 4838 case -507075711: // jurisdiction 4839 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4840 return value; 4841 case -220463842: // purpose 4842 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4843 return value; 4844 case 1522889671: // copyright 4845 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4846 return value; 4847 case 765157229: // copyrightLabel 4848 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4849 return value; 4850 case 223539345: // approvalDate 4851 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4852 return value; 4853 case -1687512484: // lastReviewDate 4854 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4855 return value; 4856 case -403934648: // effectivePeriod 4857 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4858 return value; 4859 case 110546223: // topic 4860 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4861 return value; 4862 case -1406328437: // author 4863 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4864 return value; 4865 case -1307827859: // editor 4866 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4867 return value; 4868 case -261190139: // reviewer 4869 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4870 return value; 4871 case 1740277666: // endorser 4872 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4873 return value; 4874 case 666807069: // relatedArtifact 4875 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4876 return value; 4877 case -35616442: // caseSensitive 4878 this.caseSensitive = TypeConvertor.castToBoolean(value); // BooleanType 4879 return value; 4880 case -1410174671: // valueSet 4881 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4882 return value; 4883 case 1913078280: // hierarchyMeaning 4884 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 4885 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 4886 return value; 4887 case 1248023381: // compositional 4888 this.compositional = TypeConvertor.castToBoolean(value); // BooleanType 4889 return value; 4890 case 617270957: // versionNeeded 4891 this.versionNeeded = TypeConvertor.castToBoolean(value); // BooleanType 4892 return value; 4893 case 951530617: // content 4894 value = new CodeSystemContentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4895 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 4896 return value; 4897 case -596951334: // supplements 4898 this.supplements = TypeConvertor.castToCanonical(value); // CanonicalType 4899 return value; 4900 case 94851343: // count 4901 this.count = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 4902 return value; 4903 case -1274492040: // filter 4904 this.getFilter().add((CodeSystemFilterComponent) value); // CodeSystemFilterComponent 4905 return value; 4906 case -993141291: // property 4907 this.getProperty().add((PropertyComponent) value); // PropertyComponent 4908 return value; 4909 case 951024232: // concept 4910 this.getConcept().add((ConceptDefinitionComponent) value); // ConceptDefinitionComponent 4911 return value; 4912 default: return super.setProperty(hash, name, value); 4913 } 4914 4915 } 4916 4917 @Override 4918 public Base setProperty(String name, Base value) throws FHIRException { 4919 if (name.equals("url")) { 4920 this.url = TypeConvertor.castToUri(value); // UriType 4921 } else if (name.equals("identifier")) { 4922 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4923 } else if (name.equals("version")) { 4924 this.version = TypeConvertor.castToString(value); // StringType 4925 } else if (name.equals("versionAlgorithm[x]")) { 4926 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4927 } else if (name.equals("name")) { 4928 this.name = TypeConvertor.castToString(value); // StringType 4929 } else if (name.equals("title")) { 4930 this.title = TypeConvertor.castToString(value); // StringType 4931 } else if (name.equals("status")) { 4932 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4933 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4934 } else if (name.equals("experimental")) { 4935 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4936 } else if (name.equals("date")) { 4937 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4938 } else if (name.equals("publisher")) { 4939 this.publisher = TypeConvertor.castToString(value); // StringType 4940 } else if (name.equals("contact")) { 4941 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4942 } else if (name.equals("description")) { 4943 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4944 } else if (name.equals("useContext")) { 4945 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4946 } else if (name.equals("jurisdiction")) { 4947 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4948 } else if (name.equals("purpose")) { 4949 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4950 } else if (name.equals("copyright")) { 4951 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4952 } else if (name.equals("copyrightLabel")) { 4953 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4954 } else if (name.equals("approvalDate")) { 4955 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4956 } else if (name.equals("lastReviewDate")) { 4957 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4958 } else if (name.equals("effectivePeriod")) { 4959 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4960 } else if (name.equals("topic")) { 4961 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 4962 } else if (name.equals("author")) { 4963 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 4964 } else if (name.equals("editor")) { 4965 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 4966 } else if (name.equals("reviewer")) { 4967 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 4968 } else if (name.equals("endorser")) { 4969 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 4970 } else if (name.equals("relatedArtifact")) { 4971 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4972 } else if (name.equals("caseSensitive")) { 4973 this.caseSensitive = TypeConvertor.castToBoolean(value); // BooleanType 4974 } else if (name.equals("valueSet")) { 4975 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4976 } else if (name.equals("hierarchyMeaning")) { 4977 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 4978 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 4979 } else if (name.equals("compositional")) { 4980 this.compositional = TypeConvertor.castToBoolean(value); // BooleanType 4981 } else if (name.equals("versionNeeded")) { 4982 this.versionNeeded = TypeConvertor.castToBoolean(value); // BooleanType 4983 } else if (name.equals("content")) { 4984 value = new CodeSystemContentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4985 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 4986 } else if (name.equals("supplements")) { 4987 this.supplements = TypeConvertor.castToCanonical(value); // CanonicalType 4988 } else if (name.equals("count")) { 4989 this.count = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 4990 } else if (name.equals("filter")) { 4991 this.getFilter().add((CodeSystemFilterComponent) value); 4992 } else if (name.equals("property")) { 4993 this.getProperty().add((PropertyComponent) value); 4994 } else if (name.equals("concept")) { 4995 this.getConcept().add((ConceptDefinitionComponent) value); 4996 } else 4997 return super.setProperty(name, value); 4998 return value; 4999 } 5000 5001 @Override 5002 public void removeChild(String name, Base value) throws FHIRException { 5003 if (name.equals("url")) { 5004 this.url = null; 5005 } else if (name.equals("identifier")) { 5006 this.getIdentifier().remove(value); 5007 } else if (name.equals("version")) { 5008 this.version = null; 5009 } else if (name.equals("versionAlgorithm[x]")) { 5010 this.versionAlgorithm = null; 5011 } else if (name.equals("name")) { 5012 this.name = null; 5013 } else if (name.equals("title")) { 5014 this.title = null; 5015 } else if (name.equals("status")) { 5016 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5017 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5018 } else if (name.equals("experimental")) { 5019 this.experimental = null; 5020 } else if (name.equals("date")) { 5021 this.date = null; 5022 } else if (name.equals("publisher")) { 5023 this.publisher = null; 5024 } else if (name.equals("contact")) { 5025 this.getContact().remove(value); 5026 } else if (name.equals("description")) { 5027 this.description = null; 5028 } else if (name.equals("useContext")) { 5029 this.getUseContext().remove(value); 5030 } else if (name.equals("jurisdiction")) { 5031 this.getJurisdiction().remove(value); 5032 } else if (name.equals("purpose")) { 5033 this.purpose = null; 5034 } else if (name.equals("copyright")) { 5035 this.copyright = null; 5036 } else if (name.equals("copyrightLabel")) { 5037 this.copyrightLabel = null; 5038 } else if (name.equals("approvalDate")) { 5039 this.approvalDate = null; 5040 } else if (name.equals("lastReviewDate")) { 5041 this.lastReviewDate = null; 5042 } else if (name.equals("effectivePeriod")) { 5043 this.effectivePeriod = null; 5044 } else if (name.equals("topic")) { 5045 this.getTopic().remove(value); 5046 } else if (name.equals("author")) { 5047 this.getAuthor().remove(value); 5048 } else if (name.equals("editor")) { 5049 this.getEditor().remove(value); 5050 } else if (name.equals("reviewer")) { 5051 this.getReviewer().remove(value); 5052 } else if (name.equals("endorser")) { 5053 this.getEndorser().remove(value); 5054 } else if (name.equals("relatedArtifact")) { 5055 this.getRelatedArtifact().remove(value); 5056 } else if (name.equals("caseSensitive")) { 5057 this.caseSensitive = null; 5058 } else if (name.equals("valueSet")) { 5059 this.valueSet = null; 5060 } else if (name.equals("hierarchyMeaning")) { 5061 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 5062 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 5063 } else if (name.equals("compositional")) { 5064 this.compositional = null; 5065 } else if (name.equals("versionNeeded")) { 5066 this.versionNeeded = null; 5067 } else if (name.equals("content")) { 5068 value = new CodeSystemContentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5069 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 5070 } else if (name.equals("supplements")) { 5071 this.supplements = null; 5072 } else if (name.equals("count")) { 5073 this.count = null; 5074 } else if (name.equals("filter")) { 5075 this.getFilter().remove((CodeSystemFilterComponent) value); 5076 } else if (name.equals("property")) { 5077 this.getProperty().remove((PropertyComponent) value); 5078 } else if (name.equals("concept")) { 5079 this.getConcept().remove((ConceptDefinitionComponent) value); 5080 } else 5081 super.removeChild(name, value); 5082 5083 } 5084 5085 @Override 5086 public Base makeProperty(int hash, String name) throws FHIRException { 5087 switch (hash) { 5088 case 116079: return getUrlElement(); 5089 case -1618432855: return addIdentifier(); 5090 case 351608024: return getVersionElement(); 5091 case -115699031: return getVersionAlgorithm(); 5092 case 1508158071: return getVersionAlgorithm(); 5093 case 3373707: return getNameElement(); 5094 case 110371416: return getTitleElement(); 5095 case -892481550: return getStatusElement(); 5096 case -404562712: return getExperimentalElement(); 5097 case 3076014: return getDateElement(); 5098 case 1447404028: return getPublisherElement(); 5099 case 951526432: return addContact(); 5100 case -1724546052: return getDescriptionElement(); 5101 case -669707736: return addUseContext(); 5102 case -507075711: return addJurisdiction(); 5103 case -220463842: return getPurposeElement(); 5104 case 1522889671: return getCopyrightElement(); 5105 case 765157229: return getCopyrightLabelElement(); 5106 case 223539345: return getApprovalDateElement(); 5107 case -1687512484: return getLastReviewDateElement(); 5108 case -403934648: return getEffectivePeriod(); 5109 case 110546223: return addTopic(); 5110 case -1406328437: return addAuthor(); 5111 case -1307827859: return addEditor(); 5112 case -261190139: return addReviewer(); 5113 case 1740277666: return addEndorser(); 5114 case 666807069: return addRelatedArtifact(); 5115 case -35616442: return getCaseSensitiveElement(); 5116 case -1410174671: return getValueSetElement(); 5117 case 1913078280: return getHierarchyMeaningElement(); 5118 case 1248023381: return getCompositionalElement(); 5119 case 617270957: return getVersionNeededElement(); 5120 case 951530617: return getContentElement(); 5121 case -596951334: return getSupplementsElement(); 5122 case 94851343: return getCountElement(); 5123 case -1274492040: return addFilter(); 5124 case -993141291: return addProperty(); 5125 case 951024232: return addConcept(); 5126 default: return super.makeProperty(hash, name); 5127 } 5128 5129 } 5130 5131 @Override 5132 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5133 switch (hash) { 5134 case 116079: /*url*/ return new String[] {"uri"}; 5135 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5136 case 351608024: /*version*/ return new String[] {"string"}; 5137 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 5138 case 3373707: /*name*/ return new String[] {"string"}; 5139 case 110371416: /*title*/ return new String[] {"string"}; 5140 case -892481550: /*status*/ return new String[] {"code"}; 5141 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5142 case 3076014: /*date*/ return new String[] {"dateTime"}; 5143 case 1447404028: /*publisher*/ return new String[] {"string"}; 5144 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5145 case -1724546052: /*description*/ return new String[] {"markdown"}; 5146 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5147 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 5148 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5149 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5150 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 5151 case 223539345: /*approvalDate*/ return new String[] {"date"}; 5152 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 5153 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 5154 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 5155 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 5156 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 5157 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 5158 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 5159 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 5160 case -35616442: /*caseSensitive*/ return new String[] {"boolean"}; 5161 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 5162 case 1913078280: /*hierarchyMeaning*/ return new String[] {"code"}; 5163 case 1248023381: /*compositional*/ return new String[] {"boolean"}; 5164 case 617270957: /*versionNeeded*/ return new String[] {"boolean"}; 5165 case 951530617: /*content*/ return new String[] {"code"}; 5166 case -596951334: /*supplements*/ return new String[] {"canonical"}; 5167 case 94851343: /*count*/ return new String[] {"unsignedInt"}; 5168 case -1274492040: /*filter*/ return new String[] {}; 5169 case -993141291: /*property*/ return new String[] {}; 5170 case 951024232: /*concept*/ return new String[] {}; 5171 default: return super.getTypesForProperty(hash, name); 5172 } 5173 5174 } 5175 5176 @Override 5177 public Base addChild(String name) throws FHIRException { 5178 if (name.equals("url")) { 5179 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.url"); 5180 } 5181 else if (name.equals("identifier")) { 5182 return addIdentifier(); 5183 } 5184 else if (name.equals("version")) { 5185 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.version"); 5186 } 5187 else if (name.equals("versionAlgorithmString")) { 5188 this.versionAlgorithm = new StringType(); 5189 return this.versionAlgorithm; 5190 } 5191 else if (name.equals("versionAlgorithmCoding")) { 5192 this.versionAlgorithm = new Coding(); 5193 return this.versionAlgorithm; 5194 } 5195 else if (name.equals("name")) { 5196 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.name"); 5197 } 5198 else if (name.equals("title")) { 5199 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.title"); 5200 } 5201 else if (name.equals("status")) { 5202 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.status"); 5203 } 5204 else if (name.equals("experimental")) { 5205 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.experimental"); 5206 } 5207 else if (name.equals("date")) { 5208 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.date"); 5209 } 5210 else if (name.equals("publisher")) { 5211 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.publisher"); 5212 } 5213 else if (name.equals("contact")) { 5214 return addContact(); 5215 } 5216 else if (name.equals("description")) { 5217 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 5218 } 5219 else if (name.equals("useContext")) { 5220 return addUseContext(); 5221 } 5222 else if (name.equals("jurisdiction")) { 5223 return addJurisdiction(); 5224 } 5225 else if (name.equals("purpose")) { 5226 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.purpose"); 5227 } 5228 else if (name.equals("copyright")) { 5229 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.copyright"); 5230 } 5231 else if (name.equals("copyrightLabel")) { 5232 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.copyrightLabel"); 5233 } 5234 else if (name.equals("approvalDate")) { 5235 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.approvalDate"); 5236 } 5237 else if (name.equals("lastReviewDate")) { 5238 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.lastReviewDate"); 5239 } 5240 else if (name.equals("effectivePeriod")) { 5241 this.effectivePeriod = new Period(); 5242 return this.effectivePeriod; 5243 } 5244 else if (name.equals("topic")) { 5245 return addTopic(); 5246 } 5247 else if (name.equals("author")) { 5248 return addAuthor(); 5249 } 5250 else if (name.equals("editor")) { 5251 return addEditor(); 5252 } 5253 else if (name.equals("reviewer")) { 5254 return addReviewer(); 5255 } 5256 else if (name.equals("endorser")) { 5257 return addEndorser(); 5258 } 5259 else if (name.equals("relatedArtifact")) { 5260 return addRelatedArtifact(); 5261 } 5262 else if (name.equals("caseSensitive")) { 5263 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.caseSensitive"); 5264 } 5265 else if (name.equals("valueSet")) { 5266 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.valueSet"); 5267 } 5268 else if (name.equals("hierarchyMeaning")) { 5269 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.hierarchyMeaning"); 5270 } 5271 else if (name.equals("compositional")) { 5272 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.compositional"); 5273 } 5274 else if (name.equals("versionNeeded")) { 5275 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.versionNeeded"); 5276 } 5277 else if (name.equals("content")) { 5278 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.content"); 5279 } 5280 else if (name.equals("supplements")) { 5281 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.supplements"); 5282 } 5283 else if (name.equals("count")) { 5284 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.count"); 5285 } 5286 else if (name.equals("filter")) { 5287 return addFilter(); 5288 } 5289 else if (name.equals("property")) { 5290 return addProperty(); 5291 } 5292 else if (name.equals("concept")) { 5293 return addConcept(); 5294 } 5295 else 5296 return super.addChild(name); 5297 } 5298 5299 public String fhirType() { 5300 return "CodeSystem"; 5301 5302 } 5303 5304 public CodeSystem copy() { 5305 CodeSystem dst = new CodeSystem(); 5306 copyValues(dst); 5307 return dst; 5308 } 5309 5310 public void copyValues(CodeSystem dst) { 5311 super.copyValues(dst); 5312 dst.url = url == null ? null : url.copy(); 5313 if (identifier != null) { 5314 dst.identifier = new ArrayList<Identifier>(); 5315 for (Identifier i : identifier) 5316 dst.identifier.add(i.copy()); 5317 }; 5318 dst.version = version == null ? null : version.copy(); 5319 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 5320 dst.name = name == null ? null : name.copy(); 5321 dst.title = title == null ? null : title.copy(); 5322 dst.status = status == null ? null : status.copy(); 5323 dst.experimental = experimental == null ? null : experimental.copy(); 5324 dst.date = date == null ? null : date.copy(); 5325 dst.publisher = publisher == null ? null : publisher.copy(); 5326 if (contact != null) { 5327 dst.contact = new ArrayList<ContactDetail>(); 5328 for (ContactDetail i : contact) 5329 dst.contact.add(i.copy()); 5330 }; 5331 dst.description = description == null ? null : description.copy(); 5332 if (useContext != null) { 5333 dst.useContext = new ArrayList<UsageContext>(); 5334 for (UsageContext i : useContext) 5335 dst.useContext.add(i.copy()); 5336 }; 5337 if (jurisdiction != null) { 5338 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5339 for (CodeableConcept i : jurisdiction) 5340 dst.jurisdiction.add(i.copy()); 5341 }; 5342 dst.purpose = purpose == null ? null : purpose.copy(); 5343 dst.copyright = copyright == null ? null : copyright.copy(); 5344 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 5345 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5346 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5347 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5348 if (topic != null) { 5349 dst.topic = new ArrayList<CodeableConcept>(); 5350 for (CodeableConcept i : topic) 5351 dst.topic.add(i.copy()); 5352 }; 5353 if (author != null) { 5354 dst.author = new ArrayList<ContactDetail>(); 5355 for (ContactDetail i : author) 5356 dst.author.add(i.copy()); 5357 }; 5358 if (editor != null) { 5359 dst.editor = new ArrayList<ContactDetail>(); 5360 for (ContactDetail i : editor) 5361 dst.editor.add(i.copy()); 5362 }; 5363 if (reviewer != null) { 5364 dst.reviewer = new ArrayList<ContactDetail>(); 5365 for (ContactDetail i : reviewer) 5366 dst.reviewer.add(i.copy()); 5367 }; 5368 if (endorser != null) { 5369 dst.endorser = new ArrayList<ContactDetail>(); 5370 for (ContactDetail i : endorser) 5371 dst.endorser.add(i.copy()); 5372 }; 5373 if (relatedArtifact != null) { 5374 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5375 for (RelatedArtifact i : relatedArtifact) 5376 dst.relatedArtifact.add(i.copy()); 5377 }; 5378 dst.caseSensitive = caseSensitive == null ? null : caseSensitive.copy(); 5379 dst.valueSet = valueSet == null ? null : valueSet.copy(); 5380 dst.hierarchyMeaning = hierarchyMeaning == null ? null : hierarchyMeaning.copy(); 5381 dst.compositional = compositional == null ? null : compositional.copy(); 5382 dst.versionNeeded = versionNeeded == null ? null : versionNeeded.copy(); 5383 dst.content = content == null ? null : content.copy(); 5384 dst.supplements = supplements == null ? null : supplements.copy(); 5385 dst.count = count == null ? null : count.copy(); 5386 if (filter != null) { 5387 dst.filter = new ArrayList<CodeSystemFilterComponent>(); 5388 for (CodeSystemFilterComponent i : filter) 5389 dst.filter.add(i.copy()); 5390 }; 5391 if (property != null) { 5392 dst.property = new ArrayList<PropertyComponent>(); 5393 for (PropertyComponent i : property) 5394 dst.property.add(i.copy()); 5395 }; 5396 if (concept != null) { 5397 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 5398 for (ConceptDefinitionComponent i : concept) 5399 dst.concept.add(i.copy()); 5400 }; 5401 } 5402 5403 protected CodeSystem typedCopy() { 5404 return copy(); 5405 } 5406 5407 @Override 5408 public boolean equalsDeep(Base other_) { 5409 if (!super.equalsDeep(other_)) 5410 return false; 5411 if (!(other_ instanceof CodeSystem)) 5412 return false; 5413 CodeSystem o = (CodeSystem) other_; 5414 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5415 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 5416 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 5417 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 5418 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 5419 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 5420 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5421 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 5422 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5423 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(caseSensitive, o.caseSensitive, true) 5424 && compareDeep(valueSet, o.valueSet, true) && compareDeep(hierarchyMeaning, o.hierarchyMeaning, true) 5425 && compareDeep(compositional, o.compositional, true) && compareDeep(versionNeeded, o.versionNeeded, true) 5426 && compareDeep(content, o.content, true) && compareDeep(supplements, o.supplements, true) && compareDeep(count, o.count, true) 5427 && compareDeep(filter, o.filter, true) && compareDeep(property, o.property, true) && compareDeep(concept, o.concept, true) 5428 ; 5429 } 5430 5431 @Override 5432 public boolean equalsShallow(Base other_) { 5433 if (!super.equalsShallow(other_)) 5434 return false; 5435 if (!(other_ instanceof CodeSystem)) 5436 return false; 5437 CodeSystem o = (CodeSystem) other_; 5438 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5439 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 5440 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 5441 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 5442 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 5443 && compareValues(caseSensitive, o.caseSensitive, true) && compareValues(valueSet, o.valueSet, true) 5444 && compareValues(hierarchyMeaning, o.hierarchyMeaning, true) && compareValues(compositional, o.compositional, true) 5445 && compareValues(versionNeeded, o.versionNeeded, true) && compareValues(content, o.content, true) && compareValues(supplements, o.supplements, true) 5446 && compareValues(count, o.count, true); 5447 } 5448 5449 public boolean isEmpty() { 5450 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5451 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 5452 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, approvalDate 5453 , lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact 5454 , caseSensitive, valueSet, hierarchyMeaning, compositional, versionNeeded, content 5455 , supplements, count, filter, property, concept); 5456 } 5457 5458 @Override 5459 public ResourceType getResourceType() { 5460 return ResourceType.CodeSystem; 5461 } 5462 5463 /** 5464 * Search parameter: <b>context-quantity</b> 5465 * <p> 5466 * Description: <b>Multiple Resources: 5467 5468* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5469* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5470* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5471* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5472* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5473* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5474* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5475* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5476* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5477* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5478* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5479* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5480* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5481* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5482* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5483* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5484* [Library](library.html): A quantity- or range-valued use context assigned to the library 5485* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5486* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5487* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5488* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5489* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5490* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5491* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5492* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5493* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5494* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5495* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5496* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5497* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5498</b><br> 5499 * Type: <b>quantity</b><br> 5500 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5501 * </p> 5502 */ 5503 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5504 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5505 /** 5506 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5507 * <p> 5508 * Description: <b>Multiple Resources: 5509 5510* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5511* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5512* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5513* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5514* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5515* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5516* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5517* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5518* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5519* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5520* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5521* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5522* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5523* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5524* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5525* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5526* [Library](library.html): A quantity- or range-valued use context assigned to the library 5527* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5528* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5529* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5530* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5531* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5532* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5533* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5534* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5535* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5536* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5537* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5538* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5539* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5540</b><br> 5541 * Type: <b>quantity</b><br> 5542 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5543 * </p> 5544 */ 5545 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5546 5547 /** 5548 * Search parameter: <b>context-type-quantity</b> 5549 * <p> 5550 * Description: <b>Multiple Resources: 5551 5552* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5553* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5554* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5555* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5556* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5557* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5558* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5559* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5560* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5561* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5562* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5563* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5564* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5565* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5566* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5567* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5568* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5569* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5570* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5571* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5572* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5573* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5574* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5575* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5576* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5577* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5578* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5579* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5580* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5581* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5582</b><br> 5583 * Type: <b>composite</b><br> 5584 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5585 * </p> 5586 */ 5587 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5588 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5589 /** 5590 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5591 * <p> 5592 * Description: <b>Multiple Resources: 5593 5594* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5595* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5596* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5597* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5598* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5599* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5600* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5601* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5602* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5603* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5604* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5605* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5606* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5607* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5608* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5609* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5610* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5611* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5612* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5613* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5614* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5615* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5616* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5617* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5618* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5619* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5620* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5621* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5622* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5623* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5624</b><br> 5625 * Type: <b>composite</b><br> 5626 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5627 * </p> 5628 */ 5629 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5630 5631 /** 5632 * Search parameter: <b>context-type-value</b> 5633 * <p> 5634 * Description: <b>Multiple Resources: 5635 5636* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5637* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5638* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5639* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5640* [Citation](citation.html): A use context type and value assigned to the citation 5641* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5642* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5643* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5644* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5645* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5646* [Evidence](evidence.html): A use context type and value assigned to the evidence 5647* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5648* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5649* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5650* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5651* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5652* [Library](library.html): A use context type and value assigned to the library 5653* [Measure](measure.html): A use context type and value assigned to the measure 5654* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5655* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5656* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5657* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5658* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5659* [Requirements](requirements.html): A use context type and value assigned to the requirements 5660* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5661* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5662* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5663* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5664* [TestScript](testscript.html): A use context type and value assigned to the test script 5665* [ValueSet](valueset.html): A use context type and value assigned to the value set 5666</b><br> 5667 * Type: <b>composite</b><br> 5668 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5669 * </p> 5670 */ 5671 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 5672 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5673 /** 5674 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5675 * <p> 5676 * Description: <b>Multiple Resources: 5677 5678* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5679* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5680* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5681* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5682* [Citation](citation.html): A use context type and value assigned to the citation 5683* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5684* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5685* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5686* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5687* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5688* [Evidence](evidence.html): A use context type and value assigned to the evidence 5689* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5690* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5691* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5692* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5693* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5694* [Library](library.html): A use context type and value assigned to the library 5695* [Measure](measure.html): A use context type and value assigned to the measure 5696* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5697* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5698* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5699* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5700* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5701* [Requirements](requirements.html): A use context type and value assigned to the requirements 5702* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5703* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5704* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5705* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5706* [TestScript](testscript.html): A use context type and value assigned to the test script 5707* [ValueSet](valueset.html): A use context type and value assigned to the value set 5708</b><br> 5709 * Type: <b>composite</b><br> 5710 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5711 * </p> 5712 */ 5713 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 5714 5715 /** 5716 * Search parameter: <b>context-type</b> 5717 * <p> 5718 * Description: <b>Multiple Resources: 5719 5720* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5721* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5722* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5723* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5724* [Citation](citation.html): A type of use context assigned to the citation 5725* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5726* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5727* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5728* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5729* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5730* [Evidence](evidence.html): A type of use context assigned to the evidence 5731* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5732* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5733* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5734* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5735* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5736* [Library](library.html): A type of use context assigned to the library 5737* [Measure](measure.html): A type of use context assigned to the measure 5738* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5739* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5740* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5741* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5742* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5743* [Requirements](requirements.html): A type of use context assigned to the requirements 5744* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5745* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5746* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5747* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5748* [TestScript](testscript.html): A type of use context assigned to the test script 5749* [ValueSet](valueset.html): A type of use context assigned to the value set 5750</b><br> 5751 * Type: <b>token</b><br> 5752 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5753 * </p> 5754 */ 5755 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 5756 public static final String SP_CONTEXT_TYPE = "context-type"; 5757 /** 5758 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5759 * <p> 5760 * Description: <b>Multiple Resources: 5761 5762* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5763* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5764* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5765* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5766* [Citation](citation.html): A type of use context assigned to the citation 5767* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5768* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5769* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5770* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5771* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5772* [Evidence](evidence.html): A type of use context assigned to the evidence 5773* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5774* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5775* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5776* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5777* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5778* [Library](library.html): A type of use context assigned to the library 5779* [Measure](measure.html): A type of use context assigned to the measure 5780* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5781* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5782* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5783* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5784* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5785* [Requirements](requirements.html): A type of use context assigned to the requirements 5786* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5787* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5788* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5789* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5790* [TestScript](testscript.html): A type of use context assigned to the test script 5791* [ValueSet](valueset.html): A type of use context assigned to the value set 5792</b><br> 5793 * Type: <b>token</b><br> 5794 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5795 * </p> 5796 */ 5797 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 5798 5799 /** 5800 * Search parameter: <b>context</b> 5801 * <p> 5802 * Description: <b>Multiple Resources: 5803 5804* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5805* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5806* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5807* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5808* [Citation](citation.html): A use context assigned to the citation 5809* [CodeSystem](codesystem.html): A use context assigned to the code system 5810* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5811* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5812* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5813* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5814* [Evidence](evidence.html): A use context assigned to the evidence 5815* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5816* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5817* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5818* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5819* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5820* [Library](library.html): A use context assigned to the library 5821* [Measure](measure.html): A use context assigned to the measure 5822* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5823* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5824* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5825* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5826* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5827* [Requirements](requirements.html): A use context assigned to the requirements 5828* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5829* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5830* [StructureMap](structuremap.html): A use context assigned to the structure map 5831* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5832* [TestScript](testscript.html): A use context assigned to the test script 5833* [ValueSet](valueset.html): A use context assigned to the value set 5834</b><br> 5835 * Type: <b>token</b><br> 5836 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5837 * </p> 5838 */ 5839 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 5840 public static final String SP_CONTEXT = "context"; 5841 /** 5842 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5843 * <p> 5844 * Description: <b>Multiple Resources: 5845 5846* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5847* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5848* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5849* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5850* [Citation](citation.html): A use context assigned to the citation 5851* [CodeSystem](codesystem.html): A use context assigned to the code system 5852* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5853* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5854* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5855* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5856* [Evidence](evidence.html): A use context assigned to the evidence 5857* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5858* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5859* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5860* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5861* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5862* [Library](library.html): A use context assigned to the library 5863* [Measure](measure.html): A use context assigned to the measure 5864* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5865* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5866* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5867* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5868* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5869* [Requirements](requirements.html): A use context assigned to the requirements 5870* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5871* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5872* [StructureMap](structuremap.html): A use context assigned to the structure map 5873* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5874* [TestScript](testscript.html): A use context assigned to the test script 5875* [ValueSet](valueset.html): A use context assigned to the value set 5876</b><br> 5877 * Type: <b>token</b><br> 5878 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5879 * </p> 5880 */ 5881 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 5882 5883 /** 5884 * Search parameter: <b>date</b> 5885 * <p> 5886 * Description: <b>Multiple Resources: 5887 5888* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5889* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5890* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5891* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5892* [Citation](citation.html): The citation publication date 5893* [CodeSystem](codesystem.html): The code system publication date 5894* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5895* [ConceptMap](conceptmap.html): The concept map publication date 5896* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5897* [EventDefinition](eventdefinition.html): The event definition publication date 5898* [Evidence](evidence.html): The evidence publication date 5899* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5900* [ExampleScenario](examplescenario.html): The example scenario publication date 5901* [GraphDefinition](graphdefinition.html): The graph definition publication date 5902* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5903* [Library](library.html): The library publication date 5904* [Measure](measure.html): The measure publication date 5905* [MessageDefinition](messagedefinition.html): The message definition publication date 5906* [NamingSystem](namingsystem.html): The naming system publication date 5907* [OperationDefinition](operationdefinition.html): The operation definition publication date 5908* [PlanDefinition](plandefinition.html): The plan definition publication date 5909* [Questionnaire](questionnaire.html): The questionnaire publication date 5910* [Requirements](requirements.html): The requirements publication date 5911* [SearchParameter](searchparameter.html): The search parameter publication date 5912* [StructureDefinition](structuredefinition.html): The structure definition publication date 5913* [StructureMap](structuremap.html): The structure map publication date 5914* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5915* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5916* [TestScript](testscript.html): The test script publication date 5917* [ValueSet](valueset.html): The value set publication date 5918</b><br> 5919 * Type: <b>date</b><br> 5920 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5921 * </p> 5922 */ 5923 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 5924 public static final String SP_DATE = "date"; 5925 /** 5926 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5927 * <p> 5928 * Description: <b>Multiple Resources: 5929 5930* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5931* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5932* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5933* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5934* [Citation](citation.html): The citation publication date 5935* [CodeSystem](codesystem.html): The code system publication date 5936* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5937* [ConceptMap](conceptmap.html): The concept map publication date 5938* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5939* [EventDefinition](eventdefinition.html): The event definition publication date 5940* [Evidence](evidence.html): The evidence publication date 5941* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5942* [ExampleScenario](examplescenario.html): The example scenario publication date 5943* [GraphDefinition](graphdefinition.html): The graph definition publication date 5944* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5945* [Library](library.html): The library publication date 5946* [Measure](measure.html): The measure publication date 5947* [MessageDefinition](messagedefinition.html): The message definition publication date 5948* [NamingSystem](namingsystem.html): The naming system publication date 5949* [OperationDefinition](operationdefinition.html): The operation definition publication date 5950* [PlanDefinition](plandefinition.html): The plan definition publication date 5951* [Questionnaire](questionnaire.html): The questionnaire publication date 5952* [Requirements](requirements.html): The requirements publication date 5953* [SearchParameter](searchparameter.html): The search parameter publication date 5954* [StructureDefinition](structuredefinition.html): The structure definition publication date 5955* [StructureMap](structuremap.html): The structure map publication date 5956* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5957* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5958* [TestScript](testscript.html): The test script publication date 5959* [ValueSet](valueset.html): The value set publication date 5960</b><br> 5961 * Type: <b>date</b><br> 5962 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5963 * </p> 5964 */ 5965 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5966 5967 /** 5968 * Search parameter: <b>description</b> 5969 * <p> 5970 * Description: <b>Multiple Resources: 5971 5972* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5973* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5974* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5975* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5976* [Citation](citation.html): The description of the citation 5977* [CodeSystem](codesystem.html): The description of the code system 5978* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5979* [ConceptMap](conceptmap.html): The description of the concept map 5980* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5981* [EventDefinition](eventdefinition.html): The description of the event definition 5982* [Evidence](evidence.html): The description of the evidence 5983* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5984* [GraphDefinition](graphdefinition.html): The description of the graph definition 5985* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5986* [Library](library.html): The description of the library 5987* [Measure](measure.html): The description of the measure 5988* [MessageDefinition](messagedefinition.html): The description of the message definition 5989* [NamingSystem](namingsystem.html): The description of the naming system 5990* [OperationDefinition](operationdefinition.html): The description of the operation definition 5991* [PlanDefinition](plandefinition.html): The description of the plan definition 5992* [Questionnaire](questionnaire.html): The description of the questionnaire 5993* [Requirements](requirements.html): The description of the requirements 5994* [SearchParameter](searchparameter.html): The description of the search parameter 5995* [StructureDefinition](structuredefinition.html): The description of the structure definition 5996* [StructureMap](structuremap.html): The description of the structure map 5997* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5998* [TestScript](testscript.html): The description of the test script 5999* [ValueSet](valueset.html): The description of the value set 6000</b><br> 6001 * Type: <b>string</b><br> 6002 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 6003 * </p> 6004 */ 6005 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 6006 public static final String SP_DESCRIPTION = "description"; 6007 /** 6008 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6009 * <p> 6010 * Description: <b>Multiple Resources: 6011 6012* [ActivityDefinition](activitydefinition.html): The description of the activity definition 6013* [ActorDefinition](actordefinition.html): The description of the Actor Definition 6014* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 6015* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 6016* [Citation](citation.html): The description of the citation 6017* [CodeSystem](codesystem.html): The description of the code system 6018* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 6019* [ConceptMap](conceptmap.html): The description of the concept map 6020* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 6021* [EventDefinition](eventdefinition.html): The description of the event definition 6022* [Evidence](evidence.html): The description of the evidence 6023* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 6024* [GraphDefinition](graphdefinition.html): The description of the graph definition 6025* [ImplementationGuide](implementationguide.html): The description of the implementation guide 6026* [Library](library.html): The description of the library 6027* [Measure](measure.html): The description of the measure 6028* [MessageDefinition](messagedefinition.html): The description of the message definition 6029* [NamingSystem](namingsystem.html): The description of the naming system 6030* [OperationDefinition](operationdefinition.html): The description of the operation definition 6031* [PlanDefinition](plandefinition.html): The description of the plan definition 6032* [Questionnaire](questionnaire.html): The description of the questionnaire 6033* [Requirements](requirements.html): The description of the requirements 6034* [SearchParameter](searchparameter.html): The description of the search parameter 6035* [StructureDefinition](structuredefinition.html): The description of the structure definition 6036* [StructureMap](structuremap.html): The description of the structure map 6037* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 6038* [TestScript](testscript.html): The description of the test script 6039* [ValueSet](valueset.html): The description of the value set 6040</b><br> 6041 * Type: <b>string</b><br> 6042 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 6043 * </p> 6044 */ 6045 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 6046 6047 /** 6048 * Search parameter: <b>identifier</b> 6049 * <p> 6050 * Description: <b>Multiple Resources: 6051 6052* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6053* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6054* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6055* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6056* [Citation](citation.html): External identifier for the citation 6057* [CodeSystem](codesystem.html): External identifier for the code system 6058* [ConceptMap](conceptmap.html): External identifier for the concept map 6059* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6060* [EventDefinition](eventdefinition.html): External identifier for the event definition 6061* [Evidence](evidence.html): External identifier for the evidence 6062* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6063* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6064* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6065* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6066* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6067* [Library](library.html): External identifier for the library 6068* [Measure](measure.html): External identifier for the measure 6069* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6070* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6071* [NamingSystem](namingsystem.html): External identifier for the naming system 6072* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6073* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6074* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6075* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6076* [Requirements](requirements.html): External identifier for the requirements 6077* [SearchParameter](searchparameter.html): External identifier for the search parameter 6078* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6079* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6080* [StructureMap](structuremap.html): External identifier for the structure map 6081* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6082* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6083* [TestPlan](testplan.html): An identifier for the test plan 6084* [TestScript](testscript.html): External identifier for the test script 6085* [ValueSet](valueset.html): External identifier for the value set 6086</b><br> 6087 * Type: <b>token</b><br> 6088 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6089 * </p> 6090 */ 6091 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 6092 public static final String SP_IDENTIFIER = "identifier"; 6093 /** 6094 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6095 * <p> 6096 * Description: <b>Multiple Resources: 6097 6098* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6099* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6100* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6101* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6102* [Citation](citation.html): External identifier for the citation 6103* [CodeSystem](codesystem.html): External identifier for the code system 6104* [ConceptMap](conceptmap.html): External identifier for the concept map 6105* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6106* [EventDefinition](eventdefinition.html): External identifier for the event definition 6107* [Evidence](evidence.html): External identifier for the evidence 6108* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6109* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6110* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6111* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6112* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6113* [Library](library.html): External identifier for the library 6114* [Measure](measure.html): External identifier for the measure 6115* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6116* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6117* [NamingSystem](namingsystem.html): External identifier for the naming system 6118* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6119* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6120* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6121* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6122* [Requirements](requirements.html): External identifier for the requirements 6123* [SearchParameter](searchparameter.html): External identifier for the search parameter 6124* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6125* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6126* [StructureMap](structuremap.html): External identifier for the structure map 6127* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6128* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6129* [TestPlan](testplan.html): An identifier for the test plan 6130* [TestScript](testscript.html): External identifier for the test script 6131* [ValueSet](valueset.html): External identifier for the value set 6132</b><br> 6133 * Type: <b>token</b><br> 6134 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6135 * </p> 6136 */ 6137 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6138 6139 /** 6140 * Search parameter: <b>jurisdiction</b> 6141 * <p> 6142 * Description: <b>Multiple Resources: 6143 6144* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6145* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6146* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6147* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6148* [Citation](citation.html): Intended jurisdiction for the citation 6149* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6150* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6151* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6152* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6153* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6154* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6155* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6156* [Library](library.html): Intended jurisdiction for the library 6157* [Measure](measure.html): Intended jurisdiction for the measure 6158* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6159* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6160* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6161* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6162* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6163* [Requirements](requirements.html): Intended jurisdiction for the requirements 6164* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6165* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6166* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6167* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6168* [TestScript](testscript.html): Intended jurisdiction for the test script 6169* [ValueSet](valueset.html): Intended jurisdiction for the value set 6170</b><br> 6171 * Type: <b>token</b><br> 6172 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6173 * </p> 6174 */ 6175 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 6176 public static final String SP_JURISDICTION = "jurisdiction"; 6177 /** 6178 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6179 * <p> 6180 * Description: <b>Multiple Resources: 6181 6182* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6183* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6184* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6185* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6186* [Citation](citation.html): Intended jurisdiction for the citation 6187* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6188* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6189* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6190* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6191* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6192* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6193* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6194* [Library](library.html): Intended jurisdiction for the library 6195* [Measure](measure.html): Intended jurisdiction for the measure 6196* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6197* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6198* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6199* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6200* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6201* [Requirements](requirements.html): Intended jurisdiction for the requirements 6202* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6203* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6204* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6205* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6206* [TestScript](testscript.html): Intended jurisdiction for the test script 6207* [ValueSet](valueset.html): Intended jurisdiction for the value set 6208</b><br> 6209 * Type: <b>token</b><br> 6210 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6211 * </p> 6212 */ 6213 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 6214 6215 /** 6216 * Search parameter: <b>name</b> 6217 * <p> 6218 * Description: <b>Multiple Resources: 6219 6220* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6221* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6222* [Citation](citation.html): Computationally friendly name of the citation 6223* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6224* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6225* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6226* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6227* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6228* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6229* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6230* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6231* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6232* [Library](library.html): Computationally friendly name of the library 6233* [Measure](measure.html): Computationally friendly name of the measure 6234* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6235* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6236* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6237* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6238* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6239* [Requirements](requirements.html): Computationally friendly name of the requirements 6240* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6241* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6242* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6243* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6244* [TestScript](testscript.html): Computationally friendly name of the test script 6245* [ValueSet](valueset.html): Computationally friendly name of the value set 6246</b><br> 6247 * Type: <b>string</b><br> 6248 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6249 * </p> 6250 */ 6251 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 6252 public static final String SP_NAME = "name"; 6253 /** 6254 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6255 * <p> 6256 * Description: <b>Multiple Resources: 6257 6258* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6259* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6260* [Citation](citation.html): Computationally friendly name of the citation 6261* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6262* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6263* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6264* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6265* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6266* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6267* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6268* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6269* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6270* [Library](library.html): Computationally friendly name of the library 6271* [Measure](measure.html): Computationally friendly name of the measure 6272* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6273* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6274* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6275* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6276* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6277* [Requirements](requirements.html): Computationally friendly name of the requirements 6278* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6279* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6280* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6281* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6282* [TestScript](testscript.html): Computationally friendly name of the test script 6283* [ValueSet](valueset.html): Computationally friendly name of the value set 6284</b><br> 6285 * Type: <b>string</b><br> 6286 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6287 * </p> 6288 */ 6289 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6290 6291 /** 6292 * Search parameter: <b>publisher</b> 6293 * <p> 6294 * Description: <b>Multiple Resources: 6295 6296* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6297* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6298* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6299* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6300* [Citation](citation.html): Name of the publisher of the citation 6301* [CodeSystem](codesystem.html): Name of the publisher of the code system 6302* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6303* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6304* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6305* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6306* [Evidence](evidence.html): Name of the publisher of the evidence 6307* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6308* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6309* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6310* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6311* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6312* [Library](library.html): Name of the publisher of the library 6313* [Measure](measure.html): Name of the publisher of the measure 6314* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6315* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6316* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6317* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6318* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6319* [Requirements](requirements.html): Name of the publisher of the requirements 6320* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6321* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6322* [StructureMap](structuremap.html): Name of the publisher of the structure map 6323* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6324* [TestScript](testscript.html): Name of the publisher of the test script 6325* [ValueSet](valueset.html): Name of the publisher of the value set 6326</b><br> 6327 * Type: <b>string</b><br> 6328 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6329 * </p> 6330 */ 6331 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 6332 public static final String SP_PUBLISHER = "publisher"; 6333 /** 6334 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6335 * <p> 6336 * Description: <b>Multiple Resources: 6337 6338* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6339* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6340* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6341* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6342* [Citation](citation.html): Name of the publisher of the citation 6343* [CodeSystem](codesystem.html): Name of the publisher of the code system 6344* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6345* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6346* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6347* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6348* [Evidence](evidence.html): Name of the publisher of the evidence 6349* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6350* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6351* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6352* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6353* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6354* [Library](library.html): Name of the publisher of the library 6355* [Measure](measure.html): Name of the publisher of the measure 6356* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6357* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6358* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6359* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6360* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6361* [Requirements](requirements.html): Name of the publisher of the requirements 6362* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6363* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6364* [StructureMap](structuremap.html): Name of the publisher of the structure map 6365* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6366* [TestScript](testscript.html): Name of the publisher of the test script 6367* [ValueSet](valueset.html): Name of the publisher of the value set 6368</b><br> 6369 * Type: <b>string</b><br> 6370 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6371 * </p> 6372 */ 6373 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6374 6375 /** 6376 * Search parameter: <b>status</b> 6377 * <p> 6378 * Description: <b>Multiple Resources: 6379 6380* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6381* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6382* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6383* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6384* [Citation](citation.html): The current status of the citation 6385* [CodeSystem](codesystem.html): The current status of the code system 6386* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6387* [ConceptMap](conceptmap.html): The current status of the concept map 6388* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6389* [EventDefinition](eventdefinition.html): The current status of the event definition 6390* [Evidence](evidence.html): The current status of the evidence 6391* [EvidenceReport](evidencereport.html): The current status of the evidence report 6392* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6393* [ExampleScenario](examplescenario.html): The current status of the example scenario 6394* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6395* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6396* [Library](library.html): The current status of the library 6397* [Measure](measure.html): The current status of the measure 6398* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6399* [MessageDefinition](messagedefinition.html): The current status of the message definition 6400* [NamingSystem](namingsystem.html): The current status of the naming system 6401* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6402* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6403* [PlanDefinition](plandefinition.html): The current status of the plan definition 6404* [Questionnaire](questionnaire.html): The current status of the questionnaire 6405* [Requirements](requirements.html): The current status of the requirements 6406* [SearchParameter](searchparameter.html): The current status of the search parameter 6407* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6408* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6409* [StructureMap](structuremap.html): The current status of the structure map 6410* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6411* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6412* [TestPlan](testplan.html): The current status of the test plan 6413* [TestScript](testscript.html): The current status of the test script 6414* [ValueSet](valueset.html): The current status of the value set 6415</b><br> 6416 * Type: <b>token</b><br> 6417 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6418 * </p> 6419 */ 6420 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 6421 public static final String SP_STATUS = "status"; 6422 /** 6423 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6424 * <p> 6425 * Description: <b>Multiple Resources: 6426 6427* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6428* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6429* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6430* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6431* [Citation](citation.html): The current status of the citation 6432* [CodeSystem](codesystem.html): The current status of the code system 6433* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6434* [ConceptMap](conceptmap.html): The current status of the concept map 6435* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6436* [EventDefinition](eventdefinition.html): The current status of the event definition 6437* [Evidence](evidence.html): The current status of the evidence 6438* [EvidenceReport](evidencereport.html): The current status of the evidence report 6439* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6440* [ExampleScenario](examplescenario.html): The current status of the example scenario 6441* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6442* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6443* [Library](library.html): The current status of the library 6444* [Measure](measure.html): The current status of the measure 6445* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6446* [MessageDefinition](messagedefinition.html): The current status of the message definition 6447* [NamingSystem](namingsystem.html): The current status of the naming system 6448* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6449* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6450* [PlanDefinition](plandefinition.html): The current status of the plan definition 6451* [Questionnaire](questionnaire.html): The current status of the questionnaire 6452* [Requirements](requirements.html): The current status of the requirements 6453* [SearchParameter](searchparameter.html): The current status of the search parameter 6454* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6455* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6456* [StructureMap](structuremap.html): The current status of the structure map 6457* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6458* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6459* [TestPlan](testplan.html): The current status of the test plan 6460* [TestScript](testscript.html): The current status of the test script 6461* [ValueSet](valueset.html): The current status of the value set 6462</b><br> 6463 * Type: <b>token</b><br> 6464 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6465 * </p> 6466 */ 6467 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6468 6469 /** 6470 * Search parameter: <b>title</b> 6471 * <p> 6472 * Description: <b>Multiple Resources: 6473 6474* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6475* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6476* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6477* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6478* [Citation](citation.html): The human-friendly name of the citation 6479* [CodeSystem](codesystem.html): The human-friendly name of the code system 6480* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6481* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6482* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6483* [Evidence](evidence.html): The human-friendly name of the evidence 6484* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6485* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6486* [Library](library.html): The human-friendly name of the library 6487* [Measure](measure.html): The human-friendly name of the measure 6488* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6489* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6490* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6491* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6492* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6493* [Requirements](requirements.html): The human-friendly name of the requirements 6494* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6495* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6496* [StructureMap](structuremap.html): The human-friendly name of the structure map 6497* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6498* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6499* [TestScript](testscript.html): The human-friendly name of the test script 6500* [ValueSet](valueset.html): The human-friendly name of the value set 6501</b><br> 6502 * Type: <b>string</b><br> 6503 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6504 * </p> 6505 */ 6506 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 6507 public static final String SP_TITLE = "title"; 6508 /** 6509 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6510 * <p> 6511 * Description: <b>Multiple Resources: 6512 6513* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6514* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6515* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6516* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6517* [Citation](citation.html): The human-friendly name of the citation 6518* [CodeSystem](codesystem.html): The human-friendly name of the code system 6519* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6520* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6521* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6522* [Evidence](evidence.html): The human-friendly name of the evidence 6523* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6524* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6525* [Library](library.html): The human-friendly name of the library 6526* [Measure](measure.html): The human-friendly name of the measure 6527* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6528* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6529* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6530* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6531* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6532* [Requirements](requirements.html): The human-friendly name of the requirements 6533* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6534* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6535* [StructureMap](structuremap.html): The human-friendly name of the structure map 6536* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6537* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6538* [TestScript](testscript.html): The human-friendly name of the test script 6539* [ValueSet](valueset.html): The human-friendly name of the value set 6540</b><br> 6541 * Type: <b>string</b><br> 6542 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6543 * </p> 6544 */ 6545 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6546 6547 /** 6548 * Search parameter: <b>url</b> 6549 * <p> 6550 * Description: <b>Multiple Resources: 6551 6552* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6553* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6554* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6555* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6556* [Citation](citation.html): The uri that identifies the citation 6557* [CodeSystem](codesystem.html): The uri that identifies the code system 6558* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6559* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6560* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6561* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6562* [Evidence](evidence.html): The uri that identifies the evidence 6563* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6564* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6565* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6566* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6567* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6568* [Library](library.html): The uri that identifies the library 6569* [Measure](measure.html): The uri that identifies the measure 6570* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6571* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6572* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6573* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6574* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6575* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6576* [Requirements](requirements.html): The uri that identifies the requirements 6577* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6578* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6579* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6580* [StructureMap](structuremap.html): The uri that identifies the structure map 6581* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6582* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6583* [TestPlan](testplan.html): The uri that identifies the test plan 6584* [TestScript](testscript.html): The uri that identifies the test script 6585* [ValueSet](valueset.html): The uri that identifies the value set 6586</b><br> 6587 * Type: <b>uri</b><br> 6588 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6589 * </p> 6590 */ 6591 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6592 public static final String SP_URL = "url"; 6593 /** 6594 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6595 * <p> 6596 * Description: <b>Multiple Resources: 6597 6598* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6599* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6600* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6601* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6602* [Citation](citation.html): The uri that identifies the citation 6603* [CodeSystem](codesystem.html): The uri that identifies the code system 6604* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6605* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6606* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6607* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6608* [Evidence](evidence.html): The uri that identifies the evidence 6609* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6610* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6611* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6612* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6613* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6614* [Library](library.html): The uri that identifies the library 6615* [Measure](measure.html): The uri that identifies the measure 6616* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6617* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6618* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6619* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6620* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6621* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6622* [Requirements](requirements.html): The uri that identifies the requirements 6623* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6624* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6625* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6626* [StructureMap](structuremap.html): The uri that identifies the structure map 6627* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6628* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6629* [TestPlan](testplan.html): The uri that identifies the test plan 6630* [TestScript](testscript.html): The uri that identifies the test script 6631* [ValueSet](valueset.html): The uri that identifies the value set 6632</b><br> 6633 * Type: <b>uri</b><br> 6634 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6635 * </p> 6636 */ 6637 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6638 6639 /** 6640 * Search parameter: <b>version</b> 6641 * <p> 6642 * Description: <b>Multiple Resources: 6643 6644* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6645* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6646* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6647* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6648* [Citation](citation.html): The business version of the citation 6649* [CodeSystem](codesystem.html): The business version of the code system 6650* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6651* [ConceptMap](conceptmap.html): The business version of the concept map 6652* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6653* [EventDefinition](eventdefinition.html): The business version of the event definition 6654* [Evidence](evidence.html): The business version of the evidence 6655* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6656* [ExampleScenario](examplescenario.html): The business version of the example scenario 6657* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6658* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6659* [Library](library.html): The business version of the library 6660* [Measure](measure.html): The business version of the measure 6661* [MessageDefinition](messagedefinition.html): The business version of the message definition 6662* [NamingSystem](namingsystem.html): The business version of the naming system 6663* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6664* [PlanDefinition](plandefinition.html): The business version of the plan definition 6665* [Questionnaire](questionnaire.html): The business version of the questionnaire 6666* [Requirements](requirements.html): The business version of the requirements 6667* [SearchParameter](searchparameter.html): The business version of the search parameter 6668* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6669* [StructureMap](structuremap.html): The business version of the structure map 6670* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6671* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6672* [TestScript](testscript.html): The business version of the test script 6673* [ValueSet](valueset.html): The business version of the value set 6674</b><br> 6675 * Type: <b>token</b><br> 6676 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6677 * </p> 6678 */ 6679 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 6680 public static final String SP_VERSION = "version"; 6681 /** 6682 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6683 * <p> 6684 * Description: <b>Multiple Resources: 6685 6686* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6687* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6688* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6689* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6690* [Citation](citation.html): The business version of the citation 6691* [CodeSystem](codesystem.html): The business version of the code system 6692* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6693* [ConceptMap](conceptmap.html): The business version of the concept map 6694* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6695* [EventDefinition](eventdefinition.html): The business version of the event definition 6696* [Evidence](evidence.html): The business version of the evidence 6697* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6698* [ExampleScenario](examplescenario.html): The business version of the example scenario 6699* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6700* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6701* [Library](library.html): The business version of the library 6702* [Measure](measure.html): The business version of the measure 6703* [MessageDefinition](messagedefinition.html): The business version of the message definition 6704* [NamingSystem](namingsystem.html): The business version of the naming system 6705* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6706* [PlanDefinition](plandefinition.html): The business version of the plan definition 6707* [Questionnaire](questionnaire.html): The business version of the questionnaire 6708* [Requirements](requirements.html): The business version of the requirements 6709* [SearchParameter](searchparameter.html): The business version of the search parameter 6710* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6711* [StructureMap](structuremap.html): The business version of the structure map 6712* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6713* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6714* [TestScript](testscript.html): The business version of the test script 6715* [ValueSet](valueset.html): The business version of the value set 6716</b><br> 6717 * Type: <b>token</b><br> 6718 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6719 * </p> 6720 */ 6721 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6722 6723 /** 6724 * Search parameter: <b>code</b> 6725 * <p> 6726 * Description: <b>A code defined in the code system</b><br> 6727 * Type: <b>token</b><br> 6728 * Path: <b>CodeSystem.concept.code</b><br> 6729 * </p> 6730 */ 6731 @SearchParamDefinition(name="code", path="CodeSystem.concept.code", description="A code defined in the code system", type="token" ) 6732 public static final String SP_CODE = "code"; 6733 /** 6734 * <b>Fluent Client</b> search parameter constant for <b>code</b> 6735 * <p> 6736 * Description: <b>A code defined in the code system</b><br> 6737 * Type: <b>token</b><br> 6738 * Path: <b>CodeSystem.concept.code</b><br> 6739 * </p> 6740 */ 6741 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 6742 6743 /** 6744 * Search parameter: <b>content-mode</b> 6745 * <p> 6746 * Description: <b>not-present | example | fragment | complete | supplement</b><br> 6747 * Type: <b>token</b><br> 6748 * Path: <b>CodeSystem.content</b><br> 6749 * </p> 6750 */ 6751 @SearchParamDefinition(name="content-mode", path="CodeSystem.content", description="not-present | example | fragment | complete | supplement", type="token" ) 6752 public static final String SP_CONTENT_MODE = "content-mode"; 6753 /** 6754 * <b>Fluent Client</b> search parameter constant for <b>content-mode</b> 6755 * <p> 6756 * Description: <b>not-present | example | fragment | complete | supplement</b><br> 6757 * Type: <b>token</b><br> 6758 * Path: <b>CodeSystem.content</b><br> 6759 * </p> 6760 */ 6761 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENT_MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTENT_MODE); 6762 6763 /** 6764 * Search parameter: <b>language</b> 6765 * <p> 6766 * Description: <b>A language in which a designation is provided</b><br> 6767 * Type: <b>token</b><br> 6768 * Path: <b>CodeSystem.concept.designation.language</b><br> 6769 * </p> 6770 */ 6771 @SearchParamDefinition(name="language", path="CodeSystem.concept.designation.language", description="A language in which a designation is provided", type="token" ) 6772 public static final String SP_LANGUAGE = "language"; 6773 /** 6774 * <b>Fluent Client</b> search parameter constant for <b>language</b> 6775 * <p> 6776 * Description: <b>A language in which a designation is provided</b><br> 6777 * Type: <b>token</b><br> 6778 * Path: <b>CodeSystem.concept.designation.language</b><br> 6779 * </p> 6780 */ 6781 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LANGUAGE); 6782 6783 /** 6784 * Search parameter: <b>supplements</b> 6785 * <p> 6786 * Description: <b>Find code system supplements for the referenced code system</b><br> 6787 * Type: <b>reference</b><br> 6788 * Path: <b>CodeSystem.supplements</b><br> 6789 * </p> 6790 */ 6791 @SearchParamDefinition(name="supplements", path="CodeSystem.supplements", description="Find code system supplements for the referenced code system", type="reference", target={CodeSystem.class } ) 6792 public static final String SP_SUPPLEMENTS = "supplements"; 6793 /** 6794 * <b>Fluent Client</b> search parameter constant for <b>supplements</b> 6795 * <p> 6796 * Description: <b>Find code system supplements for the referenced code system</b><br> 6797 * Type: <b>reference</b><br> 6798 * Path: <b>CodeSystem.supplements</b><br> 6799 * </p> 6800 */ 6801 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLEMENTS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPLEMENTS); 6802 6803/** 6804 * Constant for fluent queries to be used to add include statements. Specifies 6805 * the path value of "<b>CodeSystem:supplements</b>". 6806 */ 6807 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLEMENTS = new ca.uhn.fhir.model.api.Include("CodeSystem:supplements").toLocked(); 6808 6809 /** 6810 * Search parameter: <b>system</b> 6811 * <p> 6812 * Description: <b>The system for any codes defined by this code system (same as 'url')</b><br> 6813 * Type: <b>uri</b><br> 6814 * Path: <b>CodeSystem.url</b><br> 6815 * </p> 6816 */ 6817 @SearchParamDefinition(name="system", path="CodeSystem.url", description="The system for any codes defined by this code system (same as 'url')", type="uri" ) 6818 public static final String SP_SYSTEM = "system"; 6819 /** 6820 * <b>Fluent Client</b> search parameter constant for <b>system</b> 6821 * <p> 6822 * Description: <b>The system for any codes defined by this code system (same as 'url')</b><br> 6823 * Type: <b>uri</b><br> 6824 * Path: <b>CodeSystem.url</b><br> 6825 * </p> 6826 */ 6827 public static final ca.uhn.fhir.rest.gclient.UriClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SYSTEM); 6828 6829 /** 6830 * Search parameter: <b>derived-from</b> 6831 * <p> 6832 * Description: <b>Multiple Resources: 6833 6834* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6835* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6836* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6837* [EventDefinition](eventdefinition.html): What resource is being referenced 6838* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6839* [Library](library.html): What resource is being referenced 6840* [Measure](measure.html): What resource is being referenced 6841* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6842* [PlanDefinition](plandefinition.html): What resource is being referenced 6843* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6844</b><br> 6845 * Type: <b>reference</b><br> 6846 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6847 * </p> 6848 */ 6849 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6850 public static final String SP_DERIVED_FROM = "derived-from"; 6851 /** 6852 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 6853 * <p> 6854 * Description: <b>Multiple Resources: 6855 6856* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6857* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6858* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6859* [EventDefinition](eventdefinition.html): What resource is being referenced 6860* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6861* [Library](library.html): What resource is being referenced 6862* [Measure](measure.html): What resource is being referenced 6863* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6864* [PlanDefinition](plandefinition.html): What resource is being referenced 6865* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6866</b><br> 6867 * Type: <b>reference</b><br> 6868 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6869 * </p> 6870 */ 6871 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 6872 6873/** 6874 * Constant for fluent queries to be used to add include statements. Specifies 6875 * the path value of "<b>CodeSystem:derived-from</b>". 6876 */ 6877 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("CodeSystem:derived-from").toLocked(); 6878 6879 /** 6880 * Search parameter: <b>effective</b> 6881 * <p> 6882 * Description: <b>Multiple Resources: 6883 6884* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 6885* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 6886* [Citation](citation.html): The time during which the citation is intended to be in use 6887* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 6888* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 6889* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 6890* [Library](library.html): The time during which the library is intended to be in use 6891* [Measure](measure.html): The time during which the measure is intended to be in use 6892* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 6893* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 6894* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 6895* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 6896</b><br> 6897 * Type: <b>date</b><br> 6898 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 6899 * </p> 6900 */ 6901 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 6902 public static final String SP_EFFECTIVE = "effective"; 6903 /** 6904 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6905 * <p> 6906 * Description: <b>Multiple Resources: 6907 6908* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 6909* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 6910* [Citation](citation.html): The time during which the citation is intended to be in use 6911* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 6912* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 6913* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 6914* [Library](library.html): The time during which the library is intended to be in use 6915* [Measure](measure.html): The time during which the measure is intended to be in use 6916* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 6917* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 6918* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 6919* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 6920</b><br> 6921 * Type: <b>date</b><br> 6922 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 6923 * </p> 6924 */ 6925 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 6926 6927 /** 6928 * Search parameter: <b>predecessor</b> 6929 * <p> 6930 * Description: <b>Multiple Resources: 6931 6932* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6933* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6934* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6935* [EventDefinition](eventdefinition.html): What resource is being referenced 6936* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6937* [Library](library.html): What resource is being referenced 6938* [Measure](measure.html): What resource is being referenced 6939* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6940* [PlanDefinition](plandefinition.html): What resource is being referenced 6941* [ValueSet](valueset.html): The predecessor of the ValueSet 6942</b><br> 6943 * Type: <b>reference</b><br> 6944 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6945 * </p> 6946 */ 6947 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6948 public static final String SP_PREDECESSOR = "predecessor"; 6949 /** 6950 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6951 * <p> 6952 * Description: <b>Multiple Resources: 6953 6954* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6955* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6956* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6957* [EventDefinition](eventdefinition.html): What resource is being referenced 6958* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6959* [Library](library.html): What resource is being referenced 6960* [Measure](measure.html): What resource is being referenced 6961* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6962* [PlanDefinition](plandefinition.html): What resource is being referenced 6963* [ValueSet](valueset.html): The predecessor of the ValueSet 6964</b><br> 6965 * Type: <b>reference</b><br> 6966 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6967 * </p> 6968 */ 6969 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 6970 6971/** 6972 * Constant for fluent queries to be used to add include statements. Specifies 6973 * the path value of "<b>CodeSystem:predecessor</b>". 6974 */ 6975 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("CodeSystem:predecessor").toLocked(); 6976 6977 /** 6978 * Search parameter: <b>topic</b> 6979 * <p> 6980 * Description: <b>Multiple Resources: 6981 6982* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6983* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6984* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6985* [EventDefinition](eventdefinition.html): Topics associated with the module 6986* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6987* [Library](library.html): Topics associated with the module 6988* [Measure](measure.html): Topics associated with the measure 6989* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6990* [PlanDefinition](plandefinition.html): Topics associated with the module 6991* [ValueSet](valueset.html): Topics associated with the ValueSet 6992</b><br> 6993 * Type: <b>token</b><br> 6994 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6995 * </p> 6996 */ 6997 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 6998 public static final String SP_TOPIC = "topic"; 6999 /** 7000 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 7001 * <p> 7002 * Description: <b>Multiple Resources: 7003 7004* [ActivityDefinition](activitydefinition.html): Topics associated with the module 7005* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 7006* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 7007* [EventDefinition](eventdefinition.html): Topics associated with the module 7008* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 7009* [Library](library.html): Topics associated with the module 7010* [Measure](measure.html): Topics associated with the measure 7011* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 7012* [PlanDefinition](plandefinition.html): Topics associated with the module 7013* [ValueSet](valueset.html): Topics associated with the ValueSet 7014</b><br> 7015 * Type: <b>token</b><br> 7016 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 7017 * </p> 7018 */ 7019 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 7020 7021// Manual code (from Configuration.txt): 7022public PropertyComponent getProperty(String code) { 7023 for (PropertyComponent pd : getProperty()) { 7024 if (pd.getCode().equalsIgnoreCase(code)) 7025 return pd; 7026 } 7027 return null; 7028 } 7029 7030 public ConceptDefinitionComponent getDefinitionByCode(String code) { 7031 return getDefinitionByCode(getConcept(), code); 7032 } 7033 7034 private ConceptDefinitionComponent getDefinitionByCode(List<ConceptDefinitionComponent> list, String code) { 7035 for (ConceptDefinitionComponent t : list) { 7036 if (code.equals(t.getCode())) { 7037 return t; 7038 } 7039 ConceptDefinitionComponent cc = getDefinitionByCode(t.getConcept(), code); 7040 if (cc != null) { 7041 return cc; 7042 } 7043 } 7044 return null; 7045 } 7046 7047 7048 public boolean supplements(CodeSystem cs) { 7049 if (!hasSupplements()) { 7050 return false; 7051 } else if (getSupplements().contains("|")) { 7052 return getSupplements().equals(cs.getVersionedUrl()); 7053 7054 } else { 7055 return getSupplements().equals(cs.getUrl()); 7056 } 7057 } 7058 7059// end addition 7060 7061 7062} 7063