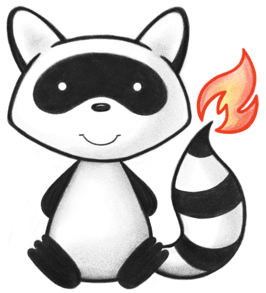
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * CodeableConcept Type: A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 050 */ 051@DatatypeDef(name="CodeableConcept") 052public class CodeableConcept extends DataType implements ICompositeType { 053 054 /** 055 * A reference to a code defined by a terminology system. 056 */ 057 @Child(name = "coding", type = {Coding.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 058 @Description(shortDefinition="Code defined by a terminology system", formalDefinition="A reference to a code defined by a terminology system." ) 059 protected List<Coding> coding; 060 061 /** 062 * A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user. 063 */ 064 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="Plain text representation of the concept", formalDefinition="A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user." ) 066 protected StringType text; 067 068 private static final long serialVersionUID = 760353246L; 069 070 /** 071 * Constructor 072 */ 073 public CodeableConcept() { 074 super(); 075 } 076 077 /** 078 * @return {@link #coding} (A reference to a code defined by a terminology system.) 079 */ 080 public List<Coding> getCoding() { 081 if (this.coding == null) 082 this.coding = new ArrayList<Coding>(); 083 return this.coding; 084 } 085 086 /** 087 * @return Returns a reference to <code>this</code> for easy method chaining 088 */ 089 public CodeableConcept setCoding(List<Coding> theCoding) { 090 this.coding = theCoding; 091 return this; 092 } 093 094 public boolean hasCoding() { 095 if (this.coding == null) 096 return false; 097 for (Coding item : this.coding) 098 if (!item.isEmpty()) 099 return true; 100 return false; 101 } 102 103 public Coding addCoding() { //3 104 Coding t = new Coding(); 105 if (this.coding == null) 106 this.coding = new ArrayList<Coding>(); 107 this.coding.add(t); 108 return t; 109 } 110 111 public CodeableConcept addCoding(Coding t) { //3 112 if (t == null) 113 return this; 114 if (this.coding == null) 115 this.coding = new ArrayList<Coding>(); 116 this.coding.add(t); 117 return this; 118 } 119 120 /** 121 * @return The first repetition of repeating field {@link #coding}, creating it if it does not already exist {3} 122 */ 123 public Coding getCodingFirstRep() { 124 if (getCoding().isEmpty()) { 125 addCoding(); 126 } 127 return getCoding().get(0); 128 } 129 130 /** 131 * @return {@link #text} (A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 132 */ 133 public StringType getTextElement() { 134 if (this.text == null) 135 if (Configuration.errorOnAutoCreate()) 136 throw new Error("Attempt to auto-create CodeableConcept.text"); 137 else if (Configuration.doAutoCreate()) 138 this.text = new StringType(); // bb 139 return this.text; 140 } 141 142 public boolean hasTextElement() { 143 return this.text != null && !this.text.isEmpty(); 144 } 145 146 public boolean hasText() { 147 return this.text != null && !this.text.isEmpty(); 148 } 149 150 /** 151 * @param value {@link #text} (A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 152 */ 153 public CodeableConcept setTextElement(StringType value) { 154 this.text = value; 155 return this; 156 } 157 158 /** 159 * @return A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user. 160 */ 161 public String getText() { 162 return this.text == null ? null : this.text.getValue(); 163 } 164 165 /** 166 * @param value A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user. 167 */ 168 public CodeableConcept setText(String value) { 169 if (Utilities.noString(value)) 170 this.text = null; 171 else { 172 if (this.text == null) 173 this.text = new StringType(); 174 this.text.setValue(value); 175 } 176 return this; 177 } 178 179 protected void listChildren(List<Property> children) { 180 super.listChildren(children); 181 children.add(new Property("coding", "Coding", "A reference to a code defined by a terminology system.", 0, java.lang.Integer.MAX_VALUE, coding)); 182 children.add(new Property("text", "string", "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.", 0, 1, text)); 183 } 184 185 @Override 186 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 187 switch (_hash) { 188 case -1355086998: /*coding*/ return new Property("coding", "Coding", "A reference to a code defined by a terminology system.", 0, java.lang.Integer.MAX_VALUE, coding); 189 case 3556653: /*text*/ return new Property("text", "string", "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.", 0, 1, text); 190 default: return super.getNamedProperty(_hash, _name, _checkValid); 191 } 192 193 } 194 195 @Override 196 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 197 switch (hash) { 198 case -1355086998: /*coding*/ return this.coding == null ? new Base[0] : this.coding.toArray(new Base[this.coding.size()]); // Coding 199 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 200 default: return super.getProperty(hash, name, checkValid); 201 } 202 203 } 204 205 @Override 206 public Base setProperty(int hash, String name, Base value) throws FHIRException { 207 switch (hash) { 208 case -1355086998: // coding 209 this.getCoding().add(TypeConvertor.castToCoding(value)); // Coding 210 return value; 211 case 3556653: // text 212 this.text = TypeConvertor.castToString(value); // StringType 213 return value; 214 default: return super.setProperty(hash, name, value); 215 } 216 217 } 218 219 @Override 220 public Base setProperty(String name, Base value) throws FHIRException { 221 if (name.equals("coding")) { 222 this.getCoding().add(TypeConvertor.castToCoding(value)); 223 } else if (name.equals("text")) { 224 this.text = TypeConvertor.castToString(value); // StringType 225 } else 226 return super.setProperty(name, value); 227 return value; 228 } 229 230 @Override 231 public void removeChild(String name, Base value) throws FHIRException { 232 if (name.equals("coding")) { 233 this.getCoding().remove(value); 234 } else if (name.equals("text")) { 235 this.text = null; 236 } else 237 super.removeChild(name, value); 238 239 } 240 241 @Override 242 public Base makeProperty(int hash, String name) throws FHIRException { 243 switch (hash) { 244 case -1355086998: return addCoding(); 245 case 3556653: return getTextElement(); 246 default: return super.makeProperty(hash, name); 247 } 248 249 } 250 251 @Override 252 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 253 switch (hash) { 254 case -1355086998: /*coding*/ return new String[] {"Coding"}; 255 case 3556653: /*text*/ return new String[] {"string"}; 256 default: return super.getTypesForProperty(hash, name); 257 } 258 259 } 260 261 @Override 262 public Base addChild(String name) throws FHIRException { 263 if (name.equals("coding")) { 264 return addCoding(); 265 } 266 else if (name.equals("text")) { 267 throw new FHIRException("Cannot call addChild on a singleton property CodeableConcept.text"); 268 } 269 else 270 return super.addChild(name); 271 } 272 273 public String fhirType() { 274 return "CodeableConcept"; 275 276 } 277 278 public CodeableConcept copy() { 279 CodeableConcept dst = new CodeableConcept(); 280 copyValues(dst); 281 return dst; 282 } 283 284 public void copyValues(CodeableConcept dst) { 285 super.copyValues(dst); 286 if (coding != null) { 287 dst.coding = new ArrayList<Coding>(); 288 for (Coding i : coding) 289 dst.coding.add(i.copy()); 290 }; 291 dst.text = text == null ? null : text.copy(); 292 } 293 294 protected CodeableConcept typedCopy() { 295 return copy(); 296 } 297 298 @Override 299 public boolean equalsDeep(Base other_) { 300 if (!super.equalsDeep(other_)) 301 return false; 302 if (!(other_ instanceof CodeableConcept)) 303 return false; 304 CodeableConcept o = (CodeableConcept) other_; 305 return compareDeep(coding, o.coding, true) && compareDeep(text, o.text, true); 306 } 307 308 @Override 309 public boolean equalsShallow(Base other_) { 310 if (!super.equalsShallow(other_)) 311 return false; 312 if (!(other_ instanceof CodeableConcept)) 313 return false; 314 CodeableConcept o = (CodeableConcept) other_; 315 return compareValues(text, o.text, true); 316 } 317 318 public boolean isEmpty() { 319 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coding, text); 320 } 321 322// Manual code (from Configuration.txt): 323public boolean hasCoding(String system, String code) { 324 for (Coding c : getCoding()) { 325 if (system.equals(c.getSystem()) && code.equals(c.getCode())) 326 return true; 327 } 328 return false; 329 } 330 331 public CodeableConcept(Coding code) { 332 super(); 333 addCoding(code); 334 } 335 336 337 public boolean matches(CodeableConcept other) { 338 for (Coding c : other.getCoding()) { 339 if (hasCoding(c.getSystem(), c.getCode())) { 340 return true; 341 } 342 } 343 return false; 344 } 345 346 public boolean hasCoding(Coding coding) { 347 return hasCoding(coding.getSystem(), coding.getCode()); 348 } 349 350 public boolean hasCoding(String system) { 351 for (Coding c : getCoding()) { 352 if (system.equals(c.getSystem())) { 353 return true; 354 } 355 } 356 return false; 357 } 358 359 public String getCode(String system) { 360 for (Coding c : getCoding()) { 361 if (system.equals(c.getSystem())) { 362 return c.getCode(); 363 } 364 } 365 return null; 366 } 367 368 public static CodeableConcept merge(CodeableConcept l, CodeableConcept r) { 369 CodeableConcept res = new CodeableConcept(); 370 List<Coding> handled = new ArrayList<>(); 371 for (Coding c : l.getCoding()) { 372 boolean done = false; 373 for (Coding t : r.getCoding()) { 374 if (t.matches(c)) { 375 handled.add(t); 376 res.getCoding().add(Coding.merge(c, t)); 377 done = true; 378 break; 379 } 380 } 381 if (!done) { 382 res.getCoding().add(c.copy()); 383 } 384 } 385 for (Coding c : r.getCoding()) { 386 if (!handled.contains(c)) { 387 res.getCoding().add(c); 388 } 389 } 390 if (l.hasText()) { 391 res.setText(l.getText()); 392 } else { 393 res.setText(r.getText()); 394 } 395 return res; 396 } 397 398 public static CodeableConcept intersect(CodeableConcept l, CodeableConcept r) { 399 CodeableConcept res = new CodeableConcept(); 400 for (Coding c : l.getCoding()) { 401 for (Coding t : r.getCoding()) { 402 if (t.matches(c)) { 403 res.getCoding().add(Coding.intersect(c, t)); 404 break; 405 } 406 } 407 } 408 if (l.hasText() && r.hasText() && l.getText().equals(r.getText())) { 409 res.setText(l.getText()); 410 } 411 return res; 412 } 413 414 415 public void addCoding(String system, String code, String display) { 416 getCoding().add(new Coding(system, code, display)); 417 } 418 419 @Override 420 public String toString() { 421 return hasCoding() ? getCoding().toString() : "["+getText()+"]"; 422 } 423 424 public void removeCoding(String system, String version, String code) { 425 getCoding().removeIf(c -> 426 (system == null || system.equals(c.getSystem())) && 427 (version == null || version.equals(c.getVersion())) && 428 (code == null || code.equals(c.getCode()))); 429 } 430 431// end addition 432 433} 434