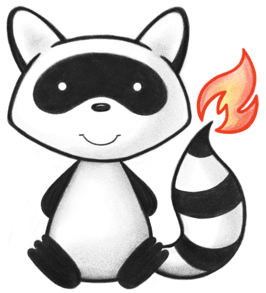
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * CodeableReference Type: A reference to a resource (by instance), or instead, a reference to a concept defined in a terminology or ontology (by class). 049 */ 050@DatatypeDef(name="CodeableReference") 051public class CodeableReference extends DataType implements ICompositeType { 052 053 /** 054 * A reference to a concept - e.g. the information is identified by its general class to the degree of precision found in the terminology. 055 */ 056 @Child(name = "concept", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="Reference to a concept (by class)", formalDefinition="A reference to a concept - e.g. the information is identified by its general class to the degree of precision found in the terminology." ) 058 protected CodeableConcept concept; 059 060 /** 061 * A reference to a resource the provides exact details about the information being referenced. 062 */ 063 @Child(name = "reference", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="Reference to a resource (by instance)", formalDefinition="A reference to a resource the provides exact details about the information being referenced." ) 065 protected Reference reference; 066 067 private static final long serialVersionUID = 2070287445L; 068 069 /** 070 * Constructor 071 */ 072 public CodeableReference() { 073 super(); 074 } 075 076 /** 077 * @return {@link #concept} (A reference to a concept - e.g. the information is identified by its general class to the degree of precision found in the terminology.) 078 */ 079 public CodeableConcept getConcept() { 080 if (this.concept == null) 081 if (Configuration.errorOnAutoCreate()) 082 throw new Error("Attempt to auto-create CodeableReference.concept"); 083 else if (Configuration.doAutoCreate()) 084 this.concept = new CodeableConcept(); // cc 085 return this.concept; 086 } 087 088 public boolean hasConcept() { 089 return this.concept != null && !this.concept.isEmpty(); 090 } 091 092 /** 093 * @param value {@link #concept} (A reference to a concept - e.g. the information is identified by its general class to the degree of precision found in the terminology.) 094 */ 095 public CodeableReference setConcept(CodeableConcept value) { 096 this.concept = value; 097 return this; 098 } 099 100 /** 101 * @return {@link #reference} (A reference to a resource the provides exact details about the information being referenced.) 102 */ 103 public Reference getReference() { 104 if (this.reference == null) 105 if (Configuration.errorOnAutoCreate()) 106 throw new Error("Attempt to auto-create CodeableReference.reference"); 107 else if (Configuration.doAutoCreate()) 108 this.reference = new Reference(); // cc 109 return this.reference; 110 } 111 112 public boolean hasReference() { 113 return this.reference != null && !this.reference.isEmpty(); 114 } 115 116 /** 117 * @param value {@link #reference} (A reference to a resource the provides exact details about the information being referenced.) 118 */ 119 public CodeableReference setReference(Reference value) { 120 this.reference = value; 121 return this; 122 } 123 124 protected void listChildren(List<Property> children) { 125 super.listChildren(children); 126 children.add(new Property("concept", "CodeableConcept", "A reference to a concept - e.g. the information is identified by its general class to the degree of precision found in the terminology.", 0, 1, concept)); 127 children.add(new Property("reference", "Reference", "A reference to a resource the provides exact details about the information being referenced.", 0, 1, reference)); 128 } 129 130 @Override 131 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 132 switch (_hash) { 133 case 951024232: /*concept*/ return new Property("concept", "CodeableConcept", "A reference to a concept - e.g. the information is identified by its general class to the degree of precision found in the terminology.", 0, 1, concept); 134 case -925155509: /*reference*/ return new Property("reference", "Reference", "A reference to a resource the provides exact details about the information being referenced.", 0, 1, reference); 135 default: return super.getNamedProperty(_hash, _name, _checkValid); 136 } 137 138 } 139 140 @Override 141 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 142 switch (hash) { 143 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : new Base[] {this.concept}; // CodeableConcept 144 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 145 default: return super.getProperty(hash, name, checkValid); 146 } 147 148 } 149 150 @Override 151 public Base setProperty(int hash, String name, Base value) throws FHIRException { 152 switch (hash) { 153 case 951024232: // concept 154 this.concept = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 155 return value; 156 case -925155509: // reference 157 this.reference = TypeConvertor.castToReference(value); // Reference 158 return value; 159 default: return super.setProperty(hash, name, value); 160 } 161 162 } 163 164 @Override 165 public Base setProperty(String name, Base value) throws FHIRException { 166 if (name.equals("concept")) { 167 this.concept = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 168 } else if (name.equals("reference")) { 169 this.reference = TypeConvertor.castToReference(value); // Reference 170 } else 171 return super.setProperty(name, value); 172 return value; 173 } 174 175 @Override 176 public void removeChild(String name, Base value) throws FHIRException { 177 if (name.equals("concept")) { 178 this.concept = null; 179 } else if (name.equals("reference")) { 180 this.reference = null; 181 } else 182 super.removeChild(name, value); 183 184 } 185 186 @Override 187 public Base makeProperty(int hash, String name) throws FHIRException { 188 switch (hash) { 189 case 951024232: return getConcept(); 190 case -925155509: return getReference(); 191 default: return super.makeProperty(hash, name); 192 } 193 194 } 195 196 @Override 197 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 198 switch (hash) { 199 case 951024232: /*concept*/ return new String[] {"CodeableConcept"}; 200 case -925155509: /*reference*/ return new String[] {"Reference"}; 201 default: return super.getTypesForProperty(hash, name); 202 } 203 204 } 205 206 @Override 207 public Base addChild(String name) throws FHIRException { 208 if (name.equals("concept")) { 209 this.concept = new CodeableConcept(); 210 return this.concept; 211 } 212 else if (name.equals("reference")) { 213 this.reference = new Reference(); 214 return this.reference; 215 } 216 else 217 return super.addChild(name); 218 } 219 220 public String fhirType() { 221 return "CodeableReference"; 222 223 } 224 225 public CodeableReference copy() { 226 CodeableReference dst = new CodeableReference(); 227 copyValues(dst); 228 return dst; 229 } 230 231 public void copyValues(CodeableReference dst) { 232 super.copyValues(dst); 233 dst.concept = concept == null ? null : concept.copy(); 234 dst.reference = reference == null ? null : reference.copy(); 235 } 236 237 protected CodeableReference typedCopy() { 238 return copy(); 239 } 240 241 @Override 242 public boolean equalsDeep(Base other_) { 243 if (!super.equalsDeep(other_)) 244 return false; 245 if (!(other_ instanceof CodeableReference)) 246 return false; 247 CodeableReference o = (CodeableReference) other_; 248 return compareDeep(concept, o.concept, true) && compareDeep(reference, o.reference, true); 249 } 250 251 @Override 252 public boolean equalsShallow(Base other_) { 253 if (!super.equalsShallow(other_)) 254 return false; 255 if (!(other_ instanceof CodeableReference)) 256 return false; 257 CodeableReference o = (CodeableReference) other_; 258 return true; 259 } 260 261 public boolean isEmpty() { 262 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(concept, reference); 263 } 264 265// Manual code (from Configuration.txt): 266 267 public CodeableReference(CodeableConcept cc) { 268 super(); 269 setConcept(cc); 270 } 271 272 public CodeableReference(Reference ref) { 273 super(); 274 setReference(ref); 275 } 276 277// end addition 278 279} 280