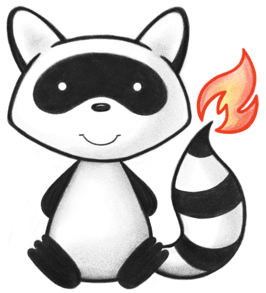
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.instance.model.api.IBaseCoding; 049/** 050 * Coding Type: A reference to a code defined by a terminology system. 051 */ 052@DatatypeDef(name="Coding") 053public class Coding extends DataType implements IBaseCoding, ICompositeType, ICoding { 054 055 /** 056 * The identification of the code system that defines the meaning of the symbol in the code. 057 */ 058 @Child(name = "system", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Identity of the terminology system", formalDefinition="The identification of the code system that defines the meaning of the symbol in the code." ) 060 protected UriType system; 061 062 /** 063 * The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 064 */ 065 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="Version of the system - if relevant", formalDefinition="The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged." ) 067 protected StringType version; 068 069 /** 070 * A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination). 071 */ 072 @Child(name = "code", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="Symbol in syntax defined by the system", formalDefinition="A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination)." ) 074 protected CodeType code; 075 076 /** 077 * A representation of the meaning of the code in the system, following the rules of the system. 078 */ 079 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="Representation defined by the system", formalDefinition="A representation of the meaning of the code in the system, following the rules of the system." ) 081 protected StringType display; 082 083 /** 084 * Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays). 085 */ 086 @Child(name = "userSelected", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 087 @Description(shortDefinition="If this coding was chosen directly by the user", formalDefinition="Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays)." ) 088 protected BooleanType userSelected; 089 090 private static final long serialVersionUID = -1417514061L; 091 092 /** 093 * Constructor 094 */ 095 public Coding() { 096 super(); 097 } 098 099 /** 100 * Convenience constructor 101 * 102 * @param theSystem The {@link #setSystem(String) code system} 103 * @param theCode The {@link #setCode(String) code} 104 * @param theDisplay The {@link #setDisplay(String) human readable display} 105 */ 106 public Coding(String theSystem, String theCode, String theDisplay) { 107 setSystem(theSystem); 108 setCode(theCode); 109 setDisplay(theDisplay); 110 } 111 /** 112 * @return {@link #system} (The identification of the code system that defines the meaning of the symbol in the code.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 113 */ 114 public UriType getSystemElement() { 115 if (this.system == null) 116 if (Configuration.errorOnAutoCreate()) 117 throw new Error("Attempt to auto-create Coding.system"); 118 else if (Configuration.doAutoCreate()) 119 this.system = new UriType(); // bb 120 return this.system; 121 } 122 123 public boolean hasSystemElement() { 124 return this.system != null && !this.system.isEmpty(); 125 } 126 127 public boolean hasSystem() { 128 return this.system != null && !this.system.isEmpty(); 129 } 130 131 /** 132 * @param value {@link #system} (The identification of the code system that defines the meaning of the symbol in the code.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 133 */ 134 public Coding setSystemElement(UriType value) { 135 this.system = value; 136 return this; 137 } 138 139 /** 140 * @return The identification of the code system that defines the meaning of the symbol in the code. 141 */ 142 public String getSystem() { 143 return this.system == null ? null : this.system.getValue(); 144 } 145 146 /** 147 * @param value The identification of the code system that defines the meaning of the symbol in the code. 148 */ 149 public Coding setSystem(String value) { 150 if (Utilities.noString(value)) 151 this.system = null; 152 else { 153 if (this.system == null) 154 this.system = new UriType(); 155 this.system.setValue(value); 156 } 157 return this; 158 } 159 160 /** 161 * @return {@link #version} (The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 162 */ 163 public StringType getVersionElement() { 164 if (this.version == null) 165 if (Configuration.errorOnAutoCreate()) 166 throw new Error("Attempt to auto-create Coding.version"); 167 else if (Configuration.doAutoCreate()) 168 this.version = new StringType(); // bb 169 return this.version; 170 } 171 172 public boolean hasVersionElement() { 173 return this.version != null && !this.version.isEmpty(); 174 } 175 176 public boolean hasVersion() { 177 return this.version != null && !this.version.isEmpty(); 178 } 179 180 /** 181 * @param value {@link #version} (The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 182 */ 183 public Coding setVersionElement(StringType value) { 184 this.version = value; 185 return this; 186 } 187 188 /** 189 * @return The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 190 */ 191 public String getVersion() { 192 return this.version == null ? null : this.version.getValue(); 193 } 194 195 /** 196 * @param value The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged. 197 */ 198 public Coding setVersion(String value) { 199 if (Utilities.noString(value)) 200 this.version = null; 201 else { 202 if (this.version == null) 203 this.version = new StringType(); 204 this.version.setValue(value); 205 } 206 return this; 207 } 208 209 /** 210 * @return {@link #code} (A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 211 */ 212 public CodeType getCodeElement() { 213 if (this.code == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create Coding.code"); 216 else if (Configuration.doAutoCreate()) 217 this.code = new CodeType(); // bb 218 return this.code; 219 } 220 221 public boolean hasCodeElement() { 222 return this.code != null && !this.code.isEmpty(); 223 } 224 225 public boolean hasCode() { 226 return this.code != null && !this.code.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #code} (A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 231 */ 232 public Coding setCodeElement(CodeType value) { 233 this.code = value; 234 return this; 235 } 236 237 /** 238 * @return A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination). 239 */ 240 public String getCode() { 241 return this.code == null ? null : this.code.getValue(); 242 } 243 244 /** 245 * @param value A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination). 246 */ 247 public Coding setCode(String value) { 248 if (Utilities.noString(value)) 249 this.code = null; 250 else { 251 if (this.code == null) 252 this.code = new CodeType(); 253 this.code.setValue(value); 254 } 255 return this; 256 } 257 258 /** 259 * @return {@link #display} (A representation of the meaning of the code in the system, following the rules of the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 260 */ 261 public StringType getDisplayElement() { 262 if (this.display == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create Coding.display"); 265 else if (Configuration.doAutoCreate()) 266 this.display = new StringType(); // bb 267 return this.display; 268 } 269 270 public boolean hasDisplayElement() { 271 return this.display != null && !this.display.isEmpty(); 272 } 273 274 public boolean hasDisplay() { 275 return this.display != null && !this.display.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #display} (A representation of the meaning of the code in the system, following the rules of the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 280 */ 281 public Coding setDisplayElement(StringType value) { 282 this.display = value; 283 return this; 284 } 285 286 /** 287 * @return A representation of the meaning of the code in the system, following the rules of the system. 288 */ 289 public String getDisplay() { 290 return this.display == null ? null : this.display.getValue(); 291 } 292 293 /** 294 * @param value A representation of the meaning of the code in the system, following the rules of the system. 295 */ 296 public Coding setDisplay(String value) { 297 if (Utilities.noString(value)) 298 this.display = null; 299 else { 300 if (this.display == null) 301 this.display = new StringType(); 302 this.display.setValue(value); 303 } 304 return this; 305 } 306 307 /** 308 * @return {@link #userSelected} (Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).). This is the underlying object with id, value and extensions. The accessor "getUserSelected" gives direct access to the value 309 */ 310 public BooleanType getUserSelectedElement() { 311 if (this.userSelected == null) 312 if (Configuration.errorOnAutoCreate()) 313 throw new Error("Attempt to auto-create Coding.userSelected"); 314 else if (Configuration.doAutoCreate()) 315 this.userSelected = new BooleanType(); // bb 316 return this.userSelected; 317 } 318 319 public boolean hasUserSelectedElement() { 320 return this.userSelected != null && !this.userSelected.isEmpty(); 321 } 322 323 public boolean hasUserSelected() { 324 return this.userSelected != null && !this.userSelected.isEmpty(); 325 } 326 327 /** 328 * @param value {@link #userSelected} (Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).). This is the underlying object with id, value and extensions. The accessor "getUserSelected" gives direct access to the value 329 */ 330 public Coding setUserSelectedElement(BooleanType value) { 331 this.userSelected = value; 332 return this; 333 } 334 335 /** 336 * @return Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays). 337 */ 338 public boolean getUserSelected() { 339 return this.userSelected == null || this.userSelected.isEmpty() ? false : this.userSelected.getValue(); 340 } 341 342 /** 343 * @param value Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays). 344 */ 345 public Coding setUserSelected(boolean value) { 346 if (this.userSelected == null) 347 this.userSelected = new BooleanType(); 348 this.userSelected.setValue(value); 349 return this; 350 } 351 352 protected void listChildren(List<Property> children) { 353 super.listChildren(children); 354 children.add(new Property("system", "uri", "The identification of the code system that defines the meaning of the symbol in the code.", 0, 1, system)); 355 children.add(new Property("version", "string", "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 0, 1, version)); 356 children.add(new Property("code", "code", "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 0, 1, code)); 357 children.add(new Property("display", "string", "A representation of the meaning of the code in the system, following the rules of the system.", 0, 1, display)); 358 children.add(new Property("userSelected", "boolean", "Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).", 0, 1, userSelected)); 359 } 360 361 @Override 362 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 363 switch (_hash) { 364 case -887328209: /*system*/ return new Property("system", "uri", "The identification of the code system that defines the meaning of the symbol in the code.", 0, 1, system); 365 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 0, 1, version); 366 case 3059181: /*code*/ return new Property("code", "code", "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 0, 1, code); 367 case 1671764162: /*display*/ return new Property("display", "string", "A representation of the meaning of the code in the system, following the rules of the system.", 0, 1, display); 368 case 423643014: /*userSelected*/ return new Property("userSelected", "boolean", "Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).", 0, 1, userSelected); 369 default: return super.getNamedProperty(_hash, _name, _checkValid); 370 } 371 372 } 373 374 @Override 375 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 376 switch (hash) { 377 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 378 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 379 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 380 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 381 case 423643014: /*userSelected*/ return this.userSelected == null ? new Base[0] : new Base[] {this.userSelected}; // BooleanType 382 default: return super.getProperty(hash, name, checkValid); 383 } 384 385 } 386 387 @Override 388 public Base setProperty(int hash, String name, Base value) throws FHIRException { 389 switch (hash) { 390 case -887328209: // system 391 this.system = TypeConvertor.castToUri(value); // UriType 392 return value; 393 case 351608024: // version 394 this.version = TypeConvertor.castToString(value); // StringType 395 return value; 396 case 3059181: // code 397 this.code = TypeConvertor.castToCode(value); // CodeType 398 return value; 399 case 1671764162: // display 400 this.display = TypeConvertor.castToString(value); // StringType 401 return value; 402 case 423643014: // userSelected 403 this.userSelected = TypeConvertor.castToBoolean(value); // BooleanType 404 return value; 405 default: return super.setProperty(hash, name, value); 406 } 407 408 } 409 410 @Override 411 public Base setProperty(String name, Base value) throws FHIRException { 412 if (name.equals("system")) { 413 this.system = TypeConvertor.castToUri(value); // UriType 414 } else if (name.equals("version")) { 415 this.version = TypeConvertor.castToString(value); // StringType 416 } else if (name.equals("code")) { 417 this.code = TypeConvertor.castToCode(value); // CodeType 418 } else if (name.equals("display")) { 419 this.display = TypeConvertor.castToString(value); // StringType 420 } else if (name.equals("userSelected")) { 421 this.userSelected = TypeConvertor.castToBoolean(value); // BooleanType 422 } else 423 return super.setProperty(name, value); 424 return value; 425 } 426 427 @Override 428 public void removeChild(String name, Base value) throws FHIRException { 429 if (name.equals("system")) { 430 this.system = null; 431 } else if (name.equals("version")) { 432 this.version = null; 433 } else if (name.equals("code")) { 434 this.code = null; 435 } else if (name.equals("display")) { 436 this.display = null; 437 } else if (name.equals("userSelected")) { 438 this.userSelected = null; 439 } else 440 super.removeChild(name, value); 441 442 } 443 444 @Override 445 public Base makeProperty(int hash, String name) throws FHIRException { 446 switch (hash) { 447 case -887328209: return getSystemElement(); 448 case 351608024: return getVersionElement(); 449 case 3059181: return getCodeElement(); 450 case 1671764162: return getDisplayElement(); 451 case 423643014: return getUserSelectedElement(); 452 default: return super.makeProperty(hash, name); 453 } 454 455 } 456 457 @Override 458 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 459 switch (hash) { 460 case -887328209: /*system*/ return new String[] {"uri"}; 461 case 351608024: /*version*/ return new String[] {"string"}; 462 case 3059181: /*code*/ return new String[] {"code"}; 463 case 1671764162: /*display*/ return new String[] {"string"}; 464 case 423643014: /*userSelected*/ return new String[] {"boolean"}; 465 default: return super.getTypesForProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public Base addChild(String name) throws FHIRException { 472 if (name.equals("system")) { 473 throw new FHIRException("Cannot call addChild on a singleton property Coding.system"); 474 } 475 else if (name.equals("version")) { 476 throw new FHIRException("Cannot call addChild on a singleton property Coding.version"); 477 } 478 else if (name.equals("code")) { 479 throw new FHIRException("Cannot call addChild on a singleton property Coding.code"); 480 } 481 else if (name.equals("display")) { 482 throw new FHIRException("Cannot call addChild on a singleton property Coding.display"); 483 } 484 else if (name.equals("userSelected")) { 485 throw new FHIRException("Cannot call addChild on a singleton property Coding.userSelected"); 486 } 487 else 488 return super.addChild(name); 489 } 490 491 public String fhirType() { 492 return "Coding"; 493 494 } 495 496 public Coding copy() { 497 Coding dst = new Coding(); 498 copyValues(dst); 499 return dst; 500 } 501 502 public void copyValues(Coding dst) { 503 super.copyValues(dst); 504 dst.system = system == null ? null : system.copy(); 505 dst.version = version == null ? null : version.copy(); 506 dst.code = code == null ? null : code.copy(); 507 dst.display = display == null ? null : display.copy(); 508 dst.userSelected = userSelected == null ? null : userSelected.copy(); 509 } 510 511 protected Coding typedCopy() { 512 return copy(); 513 } 514 515 @Override 516 public boolean equalsDeep(Base other_) { 517 if (!super.equalsDeep(other_)) 518 return false; 519 if (!(other_ instanceof Coding)) 520 return false; 521 Coding o = (Coding) other_; 522 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) && compareDeep(code, o.code, true) 523 && compareDeep(display, o.display, true) && compareDeep(userSelected, o.userSelected, true); 524 } 525 526 @Override 527 public boolean equalsShallow(Base other_) { 528 if (!super.equalsShallow(other_)) 529 return false; 530 if (!(other_ instanceof Coding)) 531 return false; 532 Coding o = (Coding) other_; 533 return compareValues(system, o.system, true) && compareValues(version, o.version, true) && compareValues(code, o.code, true) 534 && compareValues(display, o.display, true) && compareValues(userSelected, o.userSelected, true); 535 } 536 537 public boolean isEmpty() { 538 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, code, display 539 , userSelected); 540 } 541 542// Manual code (from Configuration.txt): 543@Override 544 public boolean supportsVersion() { 545 return true; 546 } 547 548 @Override 549 public boolean supportsDisplay() { 550 return true; 551 } 552 553 554 public boolean is(String system, String code) { 555 return hasSystem() && hasCode() && this.getSystem().equals(system) && this.getCode().equals(code); 556 } 557 558 public String toString() { 559 String base = hasSystem() ? getSystem() : ""; 560 if (hasVersion()) 561 base = base+"|"+getVersion(); 562 base = base + "#"+getCode(); 563 if (hasDisplay()) 564 base = base+": '"+getDisplay()+"'"; 565 return base; 566 } 567 568 public static Coding fromLiteral(String value) { 569 String sv = value.contains("#") ? value.substring(0, value.indexOf("#")) : value; 570 String cp = value.contains("#") ? value.substring(value.indexOf("#")+1) : null; 571 572 String system = sv.contains("|") ? sv.substring(0, sv.indexOf("|")) : sv; 573 String version = sv.contains("|") ? sv.substring(sv.indexOf("|")+1) : null; 574 575 String code = cp != null && cp.contains("'") ? cp.substring(0, cp.indexOf("'")) : cp; 576 String display = cp != null && cp.contains("'") ? cp.substring(cp.indexOf("'")+1) : null; 577 if (display != null) { 578 display = display.trim(); 579 display = display.substring(0, display.length() -1); 580 } 581 if ((system == null || !Utilities.isAbsoluteUrl(system)) && code == null) { 582 return null; 583 } else { 584 return new Coding(system, version, code, display); 585 } 586 } 587 588 public boolean matches(Coding other) { 589 return other.hasCode() && this.hasCode() && other.hasSystem() && this.hasSystem() && this.getCode().equals(other.getCode()) && this.getSystem().equals(other.getSystem()) ; 590 } 591 592 593 public static Coding merge(Coding l, Coding r) { 594 Coding res = new Coding(); 595 if (l.hasSystem()) { 596 res.setSystem(l.getSystem()); 597 } else { 598 res.setSystem(r.getSystem()); 599 } 600 if (l.hasVersion()) { 601 res.setVersion(l.getVersion()); 602 } else { 603 res.setVersion(r.getVersion()); 604 } 605 if (l.hasCode()) { 606 res.setCode(l.getCode()); 607 } else { 608 res.setCode(r.getCode()); 609 } 610 if (l.hasDisplay()) { 611 res.setDisplay(l.getDisplay()); 612 } else { 613 res.setDisplay(r.getDisplay()); 614 } 615 if (l.hasUserSelected()) { 616 res.setUserSelected(l.getUserSelected()); 617 } else { 618 res.setUserSelected(r.getUserSelected()); 619 } 620 return res; 621 } 622 623 public static Coding intersect(Coding l, Coding r) { 624 Coding res = new Coding(); 625 if (l.hasSystem() && l.getSystem().equals(r.getSystem())) { 626 res.setSystem(l.getSystem()); 627 } 628 if (l.hasVersion() && l.getVersion().equals(r.getVersion())) { 629 res.setVersion(l.getVersion()); 630 } 631 if (l.hasCode() && l.getCode().equals(r.getCode())) { 632 res.setCode(l.getCode()); 633 } 634 if (l.hasDisplay() && l.getDisplay().equals(r.getDisplay())) { 635 res.setDisplay(l.getDisplay()); 636 } 637 if (l.hasUserSelected() && l.getUserSelected() == r.getUserSelected()) { 638 res.setUserSelected(l.getUserSelected()); 639 } 640 return res; 641 } 642 643 public Coding(String theSystem, String theVersion, String theCode, String theDisplay) { 644 setSystem(theSystem); 645 setVersion(theVersion); 646 setCode(theCode); 647 setDisplay(theDisplay); 648 } 649// end addition 650 651 public String getVersionedSystem() { 652 return hasVersion() ? getSystem()+"|"+getVersion() : getSystem(); 653 } 654 655} 656