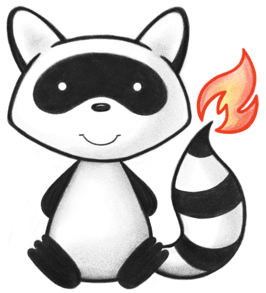
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A clinical or business level record of information being transmitted or shared; e.g. an alert that was sent to a responsible provider, a public health agency communication to a provider/reporter in response to a case report for a reportable condition. 052 */ 053@ResourceDef(name="Communication", profile="http://hl7.org/fhir/StructureDefinition/Communication") 054public class Communication extends DomainResource { 055 056 @Block() 057 public static class CommunicationPayloadComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A communicated content (or for multi-part communications, one portion of the communication). 060 */ 061 @Child(name = "content", type = {Attachment.class, Reference.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Message part content", formalDefinition="A communicated content (or for multi-part communications, one portion of the communication)." ) 063 protected DataType content; 064 065 private static final long serialVersionUID = -1954179063L; 066 067 /** 068 * Constructor 069 */ 070 public CommunicationPayloadComponent() { 071 super(); 072 } 073 074 /** 075 * Constructor 076 */ 077 public CommunicationPayloadComponent(DataType content) { 078 super(); 079 this.setContent(content); 080 } 081 082 /** 083 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 084 */ 085 public DataType getContent() { 086 return this.content; 087 } 088 089 /** 090 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 091 */ 092 public Attachment getContentAttachment() throws FHIRException { 093 if (this.content == null) 094 this.content = new Attachment(); 095 if (!(this.content instanceof Attachment)) 096 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 097 return (Attachment) this.content; 098 } 099 100 public boolean hasContentAttachment() { 101 return this.content instanceof Attachment; 102 } 103 104 /** 105 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 106 */ 107 public Reference getContentReference() throws FHIRException { 108 if (this.content == null) 109 this.content = new Reference(); 110 if (!(this.content instanceof Reference)) 111 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 112 return (Reference) this.content; 113 } 114 115 public boolean hasContentReference() { 116 return this.content instanceof Reference; 117 } 118 119 /** 120 * @return {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 121 */ 122 public CodeableConcept getContentCodeableConcept() throws FHIRException { 123 if (this.content == null) 124 this.content = new CodeableConcept(); 125 if (!(this.content instanceof CodeableConcept)) 126 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.content.getClass().getName()+" was encountered"); 127 return (CodeableConcept) this.content; 128 } 129 130 public boolean hasContentCodeableConcept() { 131 return this.content instanceof CodeableConcept; 132 } 133 134 public boolean hasContent() { 135 return this.content != null && !this.content.isEmpty(); 136 } 137 138 /** 139 * @param value {@link #content} (A communicated content (or for multi-part communications, one portion of the communication).) 140 */ 141 public CommunicationPayloadComponent setContent(DataType value) { 142 if (value != null && !(value instanceof Attachment || value instanceof Reference || value instanceof CodeableConcept)) 143 throw new FHIRException("Not the right type for Communication.payload.content[x]: "+value.fhirType()); 144 this.content = value; 145 return this; 146 } 147 148 protected void listChildren(List<Property> children) { 149 super.listChildren(children); 150 children.add(new Property("content[x]", "Attachment|Reference(Any)|CodeableConcept", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content)); 151 } 152 153 @Override 154 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 155 switch (_hash) { 156 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(Any)|CodeableConcept", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 157 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(Any)|CodeableConcept", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 158 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 159 case 1193747154: /*contentReference*/ return new Property("content[x]", "Reference(Any)", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 160 case 1554698728: /*contentCodeableConcept*/ return new Property("content[x]", "CodeableConcept", "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 161 default: return super.getNamedProperty(_hash, _name, _checkValid); 162 } 163 164 } 165 166 @Override 167 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 168 switch (hash) { 169 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // DataType 170 default: return super.getProperty(hash, name, checkValid); 171 } 172 173 } 174 175 @Override 176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 177 switch (hash) { 178 case 951530617: // content 179 this.content = TypeConvertor.castToType(value); // DataType 180 return value; 181 default: return super.setProperty(hash, name, value); 182 } 183 184 } 185 186 @Override 187 public Base setProperty(String name, Base value) throws FHIRException { 188 if (name.equals("content[x]")) { 189 this.content = TypeConvertor.castToType(value); // DataType 190 } else 191 return super.setProperty(name, value); 192 return value; 193 } 194 195 @Override 196 public void removeChild(String name, Base value) throws FHIRException { 197 if (name.equals("content[x]")) { 198 this.content = null; 199 } else 200 super.removeChild(name, value); 201 202 } 203 204 @Override 205 public Base makeProperty(int hash, String name) throws FHIRException { 206 switch (hash) { 207 case 264548711: return getContent(); 208 case 951530617: return getContent(); 209 default: return super.makeProperty(hash, name); 210 } 211 212 } 213 214 @Override 215 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 216 switch (hash) { 217 case 951530617: /*content*/ return new String[] {"Attachment", "Reference", "CodeableConcept"}; 218 default: return super.getTypesForProperty(hash, name); 219 } 220 221 } 222 223 @Override 224 public Base addChild(String name) throws FHIRException { 225 if (name.equals("contentAttachment")) { 226 this.content = new Attachment(); 227 return this.content; 228 } 229 else if (name.equals("contentReference")) { 230 this.content = new Reference(); 231 return this.content; 232 } 233 else if (name.equals("contentCodeableConcept")) { 234 this.content = new CodeableConcept(); 235 return this.content; 236 } 237 else 238 return super.addChild(name); 239 } 240 241 public CommunicationPayloadComponent copy() { 242 CommunicationPayloadComponent dst = new CommunicationPayloadComponent(); 243 copyValues(dst); 244 return dst; 245 } 246 247 public void copyValues(CommunicationPayloadComponent dst) { 248 super.copyValues(dst); 249 dst.content = content == null ? null : content.copy(); 250 } 251 252 @Override 253 public boolean equalsDeep(Base other_) { 254 if (!super.equalsDeep(other_)) 255 return false; 256 if (!(other_ instanceof CommunicationPayloadComponent)) 257 return false; 258 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 259 return compareDeep(content, o.content, true); 260 } 261 262 @Override 263 public boolean equalsShallow(Base other_) { 264 if (!super.equalsShallow(other_)) 265 return false; 266 if (!(other_ instanceof CommunicationPayloadComponent)) 267 return false; 268 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 269 return true; 270 } 271 272 public boolean isEmpty() { 273 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 274 } 275 276 public String fhirType() { 277 return "Communication.payload"; 278 279 } 280 281 } 282 283 /** 284 * Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 285 */ 286 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 287 @Description(shortDefinition="Unique identifier", formalDefinition="Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 288 protected List<Identifier> identifier; 289 290 /** 291 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication. 292 */ 293 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 294 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication." ) 295 protected List<CanonicalType> instantiatesCanonical; 296 297 /** 298 * The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication. 299 */ 300 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 301 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication." ) 302 protected List<UriType> instantiatesUri; 303 304 /** 305 * An order, proposal or plan fulfilled in whole or in part by this Communication. 306 */ 307 @Child(name = "basedOn", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 308 @Description(shortDefinition="Request fulfilled by this communication", formalDefinition="An order, proposal or plan fulfilled in whole or in part by this Communication." ) 309 protected List<Reference> basedOn; 310 311 /** 312 * A larger event (e.g. Communication, Procedure) of which this particular communication is a component or step. 313 */ 314 @Child(name = "partOf", type = {Reference.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 315 @Description(shortDefinition="Part of referenced event (e.g. Communication, Procedure)", formalDefinition="A larger event (e.g. Communication, Procedure) of which this particular communication is a component or step." ) 316 protected List<Reference> partOf; 317 318 /** 319 * Prior communication that this communication is in response to. 320 */ 321 @Child(name = "inResponseTo", type = {Communication.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 322 @Description(shortDefinition="Reply to", formalDefinition="Prior communication that this communication is in response to." ) 323 protected List<Reference> inResponseTo; 324 325 /** 326 * The status of the transmission. 327 */ 328 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 329 @Description(shortDefinition="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition="The status of the transmission." ) 330 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-status") 331 protected Enumeration<EventStatus> status; 332 333 /** 334 * Captures the reason for the current state of the Communication. 335 */ 336 @Child(name = "statusReason", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 337 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the Communication." ) 338 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-not-done-reason") 339 protected CodeableConcept statusReason; 340 341 /** 342 * The type of message conveyed such as alert, notification, reminder, instruction, etc. 343 */ 344 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 345 @Description(shortDefinition="Message category", formalDefinition="The type of message conveyed such as alert, notification, reminder, instruction, etc." ) 346 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-category") 347 protected List<CodeableConcept> category; 348 349 /** 350 * Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine. 351 */ 352 @Child(name = "priority", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 353 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine." ) 354 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 355 protected Enumeration<RequestPriority> priority; 356 357 /** 358 * A channel that was used for this communication (e.g. email, fax). 359 */ 360 @Child(name = "medium", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 361 @Description(shortDefinition="A channel of communication", formalDefinition="A channel that was used for this communication (e.g. email, fax)." ) 362 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ParticipationMode") 363 protected List<CodeableConcept> medium; 364 365 /** 366 * The patient or group that was the focus of this communication. 367 */ 368 @Child(name = "subject", type = {Patient.class, Group.class}, order=11, min=0, max=1, modifier=false, summary=true) 369 @Description(shortDefinition="Focus of message", formalDefinition="The patient or group that was the focus of this communication." ) 370 protected Reference subject; 371 372 /** 373 * Description of the purpose/content, similar to a subject line in an email. 374 */ 375 @Child(name = "topic", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 376 @Description(shortDefinition="Description of the purpose/content", formalDefinition="Description of the purpose/content, similar to a subject line in an email." ) 377 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-topic") 378 protected CodeableConcept topic; 379 380 /** 381 * Other resources that pertain to this communication and to which this communication should be associated. 382 */ 383 @Child(name = "about", type = {Reference.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 384 @Description(shortDefinition="Resources that pertain to this communication", formalDefinition="Other resources that pertain to this communication and to which this communication should be associated." ) 385 protected List<Reference> about; 386 387 /** 388 * The Encounter during which this Communication was created or to which the creation of this record is tightly associated. 389 */ 390 @Child(name = "encounter", type = {Encounter.class}, order=14, min=0, max=1, modifier=false, summary=true) 391 @Description(shortDefinition="The Encounter during which this Communication was created", formalDefinition="The Encounter during which this Communication was created or to which the creation of this record is tightly associated." ) 392 protected Reference encounter; 393 394 /** 395 * The time when this communication was sent. 396 */ 397 @Child(name = "sent", type = {DateTimeType.class}, order=15, min=0, max=1, modifier=false, summary=false) 398 @Description(shortDefinition="When sent", formalDefinition="The time when this communication was sent." ) 399 protected DateTimeType sent; 400 401 /** 402 * The time when this communication arrived at the destination. 403 */ 404 @Child(name = "received", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 405 @Description(shortDefinition="When received", formalDefinition="The time when this communication arrived at the destination." ) 406 protected DateTimeType received; 407 408 /** 409 * The entity (e.g. person, organization, clinical information system, care team or device) which is the target of the communication. 410 */ 411 @Child(name = "recipient", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Endpoint.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 412 @Description(shortDefinition="Who the information is shared with", formalDefinition="The entity (e.g. person, organization, clinical information system, care team or device) which is the target of the communication." ) 413 protected List<Reference> recipient; 414 415 /** 416 * The entity (e.g. person, organization, clinical information system, or device) which is the source of the communication. 417 */ 418 @Child(name = "sender", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, HealthcareService.class, Endpoint.class, CareTeam.class}, order=18, min=0, max=1, modifier=false, summary=false) 419 @Description(shortDefinition="Who shares the information", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which is the source of the communication." ) 420 protected Reference sender; 421 422 /** 423 * The reason or justification for the communication. 424 */ 425 @Child(name = "reason", type = {CodeableReference.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 426 @Description(shortDefinition="Indication for message", formalDefinition="The reason or justification for the communication." ) 427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 428 protected List<CodeableReference> reason; 429 430 /** 431 * Text, attachment(s), or resource(s) that was communicated to the recipient. 432 */ 433 @Child(name = "payload", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 434 @Description(shortDefinition="Message payload", formalDefinition="Text, attachment(s), or resource(s) that was communicated to the recipient." ) 435 protected List<CommunicationPayloadComponent> payload; 436 437 /** 438 * Additional notes or commentary about the communication by the sender, receiver or other interested parties. 439 */ 440 @Child(name = "note", type = {Annotation.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 441 @Description(shortDefinition="Comments made about the communication", formalDefinition="Additional notes or commentary about the communication by the sender, receiver or other interested parties." ) 442 protected List<Annotation> note; 443 444 private static final long serialVersionUID = 1848181185L; 445 446 /** 447 * Constructor 448 */ 449 public Communication() { 450 super(); 451 } 452 453 /** 454 * Constructor 455 */ 456 public Communication(EventStatus status) { 457 super(); 458 this.setStatus(status); 459 } 460 461 /** 462 * @return {@link #identifier} (Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 463 */ 464 public List<Identifier> getIdentifier() { 465 if (this.identifier == null) 466 this.identifier = new ArrayList<Identifier>(); 467 return this.identifier; 468 } 469 470 /** 471 * @return Returns a reference to <code>this</code> for easy method chaining 472 */ 473 public Communication setIdentifier(List<Identifier> theIdentifier) { 474 this.identifier = theIdentifier; 475 return this; 476 } 477 478 public boolean hasIdentifier() { 479 if (this.identifier == null) 480 return false; 481 for (Identifier item : this.identifier) 482 if (!item.isEmpty()) 483 return true; 484 return false; 485 } 486 487 public Identifier addIdentifier() { //3 488 Identifier t = new Identifier(); 489 if (this.identifier == null) 490 this.identifier = new ArrayList<Identifier>(); 491 this.identifier.add(t); 492 return t; 493 } 494 495 public Communication addIdentifier(Identifier t) { //3 496 if (t == null) 497 return this; 498 if (this.identifier == null) 499 this.identifier = new ArrayList<Identifier>(); 500 this.identifier.add(t); 501 return this; 502 } 503 504 /** 505 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 506 */ 507 public Identifier getIdentifierFirstRep() { 508 if (getIdentifier().isEmpty()) { 509 addIdentifier(); 510 } 511 return getIdentifier().get(0); 512 } 513 514 /** 515 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 516 */ 517 public List<CanonicalType> getInstantiatesCanonical() { 518 if (this.instantiatesCanonical == null) 519 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 520 return this.instantiatesCanonical; 521 } 522 523 /** 524 * @return Returns a reference to <code>this</code> for easy method chaining 525 */ 526 public Communication setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 527 this.instantiatesCanonical = theInstantiatesCanonical; 528 return this; 529 } 530 531 public boolean hasInstantiatesCanonical() { 532 if (this.instantiatesCanonical == null) 533 return false; 534 for (CanonicalType item : this.instantiatesCanonical) 535 if (!item.isEmpty()) 536 return true; 537 return false; 538 } 539 540 /** 541 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 542 */ 543 public CanonicalType addInstantiatesCanonicalElement() {//2 544 CanonicalType t = new CanonicalType(); 545 if (this.instantiatesCanonical == null) 546 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 547 this.instantiatesCanonical.add(t); 548 return t; 549 } 550 551 /** 552 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 553 */ 554 public Communication addInstantiatesCanonical(String value) { //1 555 CanonicalType t = new CanonicalType(); 556 t.setValue(value); 557 if (this.instantiatesCanonical == null) 558 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 559 this.instantiatesCanonical.add(t); 560 return this; 561 } 562 563 /** 564 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 565 */ 566 public boolean hasInstantiatesCanonical(String value) { 567 if (this.instantiatesCanonical == null) 568 return false; 569 for (CanonicalType v : this.instantiatesCanonical) 570 if (v.getValue().equals(value)) // canonical 571 return true; 572 return false; 573 } 574 575 /** 576 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 577 */ 578 public List<UriType> getInstantiatesUri() { 579 if (this.instantiatesUri == null) 580 this.instantiatesUri = new ArrayList<UriType>(); 581 return this.instantiatesUri; 582 } 583 584 /** 585 * @return Returns a reference to <code>this</code> for easy method chaining 586 */ 587 public Communication setInstantiatesUri(List<UriType> theInstantiatesUri) { 588 this.instantiatesUri = theInstantiatesUri; 589 return this; 590 } 591 592 public boolean hasInstantiatesUri() { 593 if (this.instantiatesUri == null) 594 return false; 595 for (UriType item : this.instantiatesUri) 596 if (!item.isEmpty()) 597 return true; 598 return false; 599 } 600 601 /** 602 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 603 */ 604 public UriType addInstantiatesUriElement() {//2 605 UriType t = new UriType(); 606 if (this.instantiatesUri == null) 607 this.instantiatesUri = new ArrayList<UriType>(); 608 this.instantiatesUri.add(t); 609 return t; 610 } 611 612 /** 613 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 614 */ 615 public Communication addInstantiatesUri(String value) { //1 616 UriType t = new UriType(); 617 t.setValue(value); 618 if (this.instantiatesUri == null) 619 this.instantiatesUri = new ArrayList<UriType>(); 620 this.instantiatesUri.add(t); 621 return this; 622 } 623 624 /** 625 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.) 626 */ 627 public boolean hasInstantiatesUri(String value) { 628 if (this.instantiatesUri == null) 629 return false; 630 for (UriType v : this.instantiatesUri) 631 if (v.getValue().equals(value)) // uri 632 return true; 633 return false; 634 } 635 636 /** 637 * @return {@link #basedOn} (An order, proposal or plan fulfilled in whole or in part by this Communication.) 638 */ 639 public List<Reference> getBasedOn() { 640 if (this.basedOn == null) 641 this.basedOn = new ArrayList<Reference>(); 642 return this.basedOn; 643 } 644 645 /** 646 * @return Returns a reference to <code>this</code> for easy method chaining 647 */ 648 public Communication setBasedOn(List<Reference> theBasedOn) { 649 this.basedOn = theBasedOn; 650 return this; 651 } 652 653 public boolean hasBasedOn() { 654 if (this.basedOn == null) 655 return false; 656 for (Reference item : this.basedOn) 657 if (!item.isEmpty()) 658 return true; 659 return false; 660 } 661 662 public Reference addBasedOn() { //3 663 Reference t = new Reference(); 664 if (this.basedOn == null) 665 this.basedOn = new ArrayList<Reference>(); 666 this.basedOn.add(t); 667 return t; 668 } 669 670 public Communication addBasedOn(Reference t) { //3 671 if (t == null) 672 return this; 673 if (this.basedOn == null) 674 this.basedOn = new ArrayList<Reference>(); 675 this.basedOn.add(t); 676 return this; 677 } 678 679 /** 680 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 681 */ 682 public Reference getBasedOnFirstRep() { 683 if (getBasedOn().isEmpty()) { 684 addBasedOn(); 685 } 686 return getBasedOn().get(0); 687 } 688 689 /** 690 * @return {@link #partOf} (A larger event (e.g. Communication, Procedure) of which this particular communication is a component or step.) 691 */ 692 public List<Reference> getPartOf() { 693 if (this.partOf == null) 694 this.partOf = new ArrayList<Reference>(); 695 return this.partOf; 696 } 697 698 /** 699 * @return Returns a reference to <code>this</code> for easy method chaining 700 */ 701 public Communication setPartOf(List<Reference> thePartOf) { 702 this.partOf = thePartOf; 703 return this; 704 } 705 706 public boolean hasPartOf() { 707 if (this.partOf == null) 708 return false; 709 for (Reference item : this.partOf) 710 if (!item.isEmpty()) 711 return true; 712 return false; 713 } 714 715 public Reference addPartOf() { //3 716 Reference t = new Reference(); 717 if (this.partOf == null) 718 this.partOf = new ArrayList<Reference>(); 719 this.partOf.add(t); 720 return t; 721 } 722 723 public Communication addPartOf(Reference t) { //3 724 if (t == null) 725 return this; 726 if (this.partOf == null) 727 this.partOf = new ArrayList<Reference>(); 728 this.partOf.add(t); 729 return this; 730 } 731 732 /** 733 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 734 */ 735 public Reference getPartOfFirstRep() { 736 if (getPartOf().isEmpty()) { 737 addPartOf(); 738 } 739 return getPartOf().get(0); 740 } 741 742 /** 743 * @return {@link #inResponseTo} (Prior communication that this communication is in response to.) 744 */ 745 public List<Reference> getInResponseTo() { 746 if (this.inResponseTo == null) 747 this.inResponseTo = new ArrayList<Reference>(); 748 return this.inResponseTo; 749 } 750 751 /** 752 * @return Returns a reference to <code>this</code> for easy method chaining 753 */ 754 public Communication setInResponseTo(List<Reference> theInResponseTo) { 755 this.inResponseTo = theInResponseTo; 756 return this; 757 } 758 759 public boolean hasInResponseTo() { 760 if (this.inResponseTo == null) 761 return false; 762 for (Reference item : this.inResponseTo) 763 if (!item.isEmpty()) 764 return true; 765 return false; 766 } 767 768 public Reference addInResponseTo() { //3 769 Reference t = new Reference(); 770 if (this.inResponseTo == null) 771 this.inResponseTo = new ArrayList<Reference>(); 772 this.inResponseTo.add(t); 773 return t; 774 } 775 776 public Communication addInResponseTo(Reference t) { //3 777 if (t == null) 778 return this; 779 if (this.inResponseTo == null) 780 this.inResponseTo = new ArrayList<Reference>(); 781 this.inResponseTo.add(t); 782 return this; 783 } 784 785 /** 786 * @return The first repetition of repeating field {@link #inResponseTo}, creating it if it does not already exist {3} 787 */ 788 public Reference getInResponseToFirstRep() { 789 if (getInResponseTo().isEmpty()) { 790 addInResponseTo(); 791 } 792 return getInResponseTo().get(0); 793 } 794 795 /** 796 * @return {@link #status} (The status of the transmission.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 797 */ 798 public Enumeration<EventStatus> getStatusElement() { 799 if (this.status == null) 800 if (Configuration.errorOnAutoCreate()) 801 throw new Error("Attempt to auto-create Communication.status"); 802 else if (Configuration.doAutoCreate()) 803 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); // bb 804 return this.status; 805 } 806 807 public boolean hasStatusElement() { 808 return this.status != null && !this.status.isEmpty(); 809 } 810 811 public boolean hasStatus() { 812 return this.status != null && !this.status.isEmpty(); 813 } 814 815 /** 816 * @param value {@link #status} (The status of the transmission.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 817 */ 818 public Communication setStatusElement(Enumeration<EventStatus> value) { 819 this.status = value; 820 return this; 821 } 822 823 /** 824 * @return The status of the transmission. 825 */ 826 public EventStatus getStatus() { 827 return this.status == null ? null : this.status.getValue(); 828 } 829 830 /** 831 * @param value The status of the transmission. 832 */ 833 public Communication setStatus(EventStatus value) { 834 if (this.status == null) 835 this.status = new Enumeration<EventStatus>(new EventStatusEnumFactory()); 836 this.status.setValue(value); 837 return this; 838 } 839 840 /** 841 * @return {@link #statusReason} (Captures the reason for the current state of the Communication.) 842 */ 843 public CodeableConcept getStatusReason() { 844 if (this.statusReason == null) 845 if (Configuration.errorOnAutoCreate()) 846 throw new Error("Attempt to auto-create Communication.statusReason"); 847 else if (Configuration.doAutoCreate()) 848 this.statusReason = new CodeableConcept(); // cc 849 return this.statusReason; 850 } 851 852 public boolean hasStatusReason() { 853 return this.statusReason != null && !this.statusReason.isEmpty(); 854 } 855 856 /** 857 * @param value {@link #statusReason} (Captures the reason for the current state of the Communication.) 858 */ 859 public Communication setStatusReason(CodeableConcept value) { 860 this.statusReason = value; 861 return this; 862 } 863 864 /** 865 * @return {@link #category} (The type of message conveyed such as alert, notification, reminder, instruction, etc.) 866 */ 867 public List<CodeableConcept> getCategory() { 868 if (this.category == null) 869 this.category = new ArrayList<CodeableConcept>(); 870 return this.category; 871 } 872 873 /** 874 * @return Returns a reference to <code>this</code> for easy method chaining 875 */ 876 public Communication setCategory(List<CodeableConcept> theCategory) { 877 this.category = theCategory; 878 return this; 879 } 880 881 public boolean hasCategory() { 882 if (this.category == null) 883 return false; 884 for (CodeableConcept item : this.category) 885 if (!item.isEmpty()) 886 return true; 887 return false; 888 } 889 890 public CodeableConcept addCategory() { //3 891 CodeableConcept t = new CodeableConcept(); 892 if (this.category == null) 893 this.category = new ArrayList<CodeableConcept>(); 894 this.category.add(t); 895 return t; 896 } 897 898 public Communication addCategory(CodeableConcept t) { //3 899 if (t == null) 900 return this; 901 if (this.category == null) 902 this.category = new ArrayList<CodeableConcept>(); 903 this.category.add(t); 904 return this; 905 } 906 907 /** 908 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 909 */ 910 public CodeableConcept getCategoryFirstRep() { 911 if (getCategory().isEmpty()) { 912 addCategory(); 913 } 914 return getCategory().get(0); 915 } 916 917 /** 918 * @return {@link #priority} (Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 919 */ 920 public Enumeration<RequestPriority> getPriorityElement() { 921 if (this.priority == null) 922 if (Configuration.errorOnAutoCreate()) 923 throw new Error("Attempt to auto-create Communication.priority"); 924 else if (Configuration.doAutoCreate()) 925 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 926 return this.priority; 927 } 928 929 public boolean hasPriorityElement() { 930 return this.priority != null && !this.priority.isEmpty(); 931 } 932 933 public boolean hasPriority() { 934 return this.priority != null && !this.priority.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #priority} (Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 939 */ 940 public Communication setPriorityElement(Enumeration<RequestPriority> value) { 941 this.priority = value; 942 return this; 943 } 944 945 /** 946 * @return Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine. 947 */ 948 public RequestPriority getPriority() { 949 return this.priority == null ? null : this.priority.getValue(); 950 } 951 952 /** 953 * @param value Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine. 954 */ 955 public Communication setPriority(RequestPriority value) { 956 if (value == null) 957 this.priority = null; 958 else { 959 if (this.priority == null) 960 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 961 this.priority.setValue(value); 962 } 963 return this; 964 } 965 966 /** 967 * @return {@link #medium} (A channel that was used for this communication (e.g. email, fax).) 968 */ 969 public List<CodeableConcept> getMedium() { 970 if (this.medium == null) 971 this.medium = new ArrayList<CodeableConcept>(); 972 return this.medium; 973 } 974 975 /** 976 * @return Returns a reference to <code>this</code> for easy method chaining 977 */ 978 public Communication setMedium(List<CodeableConcept> theMedium) { 979 this.medium = theMedium; 980 return this; 981 } 982 983 public boolean hasMedium() { 984 if (this.medium == null) 985 return false; 986 for (CodeableConcept item : this.medium) 987 if (!item.isEmpty()) 988 return true; 989 return false; 990 } 991 992 public CodeableConcept addMedium() { //3 993 CodeableConcept t = new CodeableConcept(); 994 if (this.medium == null) 995 this.medium = new ArrayList<CodeableConcept>(); 996 this.medium.add(t); 997 return t; 998 } 999 1000 public Communication addMedium(CodeableConcept t) { //3 1001 if (t == null) 1002 return this; 1003 if (this.medium == null) 1004 this.medium = new ArrayList<CodeableConcept>(); 1005 this.medium.add(t); 1006 return this; 1007 } 1008 1009 /** 1010 * @return The first repetition of repeating field {@link #medium}, creating it if it does not already exist {3} 1011 */ 1012 public CodeableConcept getMediumFirstRep() { 1013 if (getMedium().isEmpty()) { 1014 addMedium(); 1015 } 1016 return getMedium().get(0); 1017 } 1018 1019 /** 1020 * @return {@link #subject} (The patient or group that was the focus of this communication.) 1021 */ 1022 public Reference getSubject() { 1023 if (this.subject == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create Communication.subject"); 1026 else if (Configuration.doAutoCreate()) 1027 this.subject = new Reference(); // cc 1028 return this.subject; 1029 } 1030 1031 public boolean hasSubject() { 1032 return this.subject != null && !this.subject.isEmpty(); 1033 } 1034 1035 /** 1036 * @param value {@link #subject} (The patient or group that was the focus of this communication.) 1037 */ 1038 public Communication setSubject(Reference value) { 1039 this.subject = value; 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #topic} (Description of the purpose/content, similar to a subject line in an email.) 1045 */ 1046 public CodeableConcept getTopic() { 1047 if (this.topic == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create Communication.topic"); 1050 else if (Configuration.doAutoCreate()) 1051 this.topic = new CodeableConcept(); // cc 1052 return this.topic; 1053 } 1054 1055 public boolean hasTopic() { 1056 return this.topic != null && !this.topic.isEmpty(); 1057 } 1058 1059 /** 1060 * @param value {@link #topic} (Description of the purpose/content, similar to a subject line in an email.) 1061 */ 1062 public Communication setTopic(CodeableConcept value) { 1063 this.topic = value; 1064 return this; 1065 } 1066 1067 /** 1068 * @return {@link #about} (Other resources that pertain to this communication and to which this communication should be associated.) 1069 */ 1070 public List<Reference> getAbout() { 1071 if (this.about == null) 1072 this.about = new ArrayList<Reference>(); 1073 return this.about; 1074 } 1075 1076 /** 1077 * @return Returns a reference to <code>this</code> for easy method chaining 1078 */ 1079 public Communication setAbout(List<Reference> theAbout) { 1080 this.about = theAbout; 1081 return this; 1082 } 1083 1084 public boolean hasAbout() { 1085 if (this.about == null) 1086 return false; 1087 for (Reference item : this.about) 1088 if (!item.isEmpty()) 1089 return true; 1090 return false; 1091 } 1092 1093 public Reference addAbout() { //3 1094 Reference t = new Reference(); 1095 if (this.about == null) 1096 this.about = new ArrayList<Reference>(); 1097 this.about.add(t); 1098 return t; 1099 } 1100 1101 public Communication addAbout(Reference t) { //3 1102 if (t == null) 1103 return this; 1104 if (this.about == null) 1105 this.about = new ArrayList<Reference>(); 1106 this.about.add(t); 1107 return this; 1108 } 1109 1110 /** 1111 * @return The first repetition of repeating field {@link #about}, creating it if it does not already exist {3} 1112 */ 1113 public Reference getAboutFirstRep() { 1114 if (getAbout().isEmpty()) { 1115 addAbout(); 1116 } 1117 return getAbout().get(0); 1118 } 1119 1120 /** 1121 * @return {@link #encounter} (The Encounter during which this Communication was created or to which the creation of this record is tightly associated.) 1122 */ 1123 public Reference getEncounter() { 1124 if (this.encounter == null) 1125 if (Configuration.errorOnAutoCreate()) 1126 throw new Error("Attempt to auto-create Communication.encounter"); 1127 else if (Configuration.doAutoCreate()) 1128 this.encounter = new Reference(); // cc 1129 return this.encounter; 1130 } 1131 1132 public boolean hasEncounter() { 1133 return this.encounter != null && !this.encounter.isEmpty(); 1134 } 1135 1136 /** 1137 * @param value {@link #encounter} (The Encounter during which this Communication was created or to which the creation of this record is tightly associated.) 1138 */ 1139 public Communication setEncounter(Reference value) { 1140 this.encounter = value; 1141 return this; 1142 } 1143 1144 /** 1145 * @return {@link #sent} (The time when this communication was sent.). This is the underlying object with id, value and extensions. The accessor "getSent" gives direct access to the value 1146 */ 1147 public DateTimeType getSentElement() { 1148 if (this.sent == null) 1149 if (Configuration.errorOnAutoCreate()) 1150 throw new Error("Attempt to auto-create Communication.sent"); 1151 else if (Configuration.doAutoCreate()) 1152 this.sent = new DateTimeType(); // bb 1153 return this.sent; 1154 } 1155 1156 public boolean hasSentElement() { 1157 return this.sent != null && !this.sent.isEmpty(); 1158 } 1159 1160 public boolean hasSent() { 1161 return this.sent != null && !this.sent.isEmpty(); 1162 } 1163 1164 /** 1165 * @param value {@link #sent} (The time when this communication was sent.). This is the underlying object with id, value and extensions. The accessor "getSent" gives direct access to the value 1166 */ 1167 public Communication setSentElement(DateTimeType value) { 1168 this.sent = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return The time when this communication was sent. 1174 */ 1175 public Date getSent() { 1176 return this.sent == null ? null : this.sent.getValue(); 1177 } 1178 1179 /** 1180 * @param value The time when this communication was sent. 1181 */ 1182 public Communication setSent(Date value) { 1183 if (value == null) 1184 this.sent = null; 1185 else { 1186 if (this.sent == null) 1187 this.sent = new DateTimeType(); 1188 this.sent.setValue(value); 1189 } 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #received} (The time when this communication arrived at the destination.). This is the underlying object with id, value and extensions. The accessor "getReceived" gives direct access to the value 1195 */ 1196 public DateTimeType getReceivedElement() { 1197 if (this.received == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create Communication.received"); 1200 else if (Configuration.doAutoCreate()) 1201 this.received = new DateTimeType(); // bb 1202 return this.received; 1203 } 1204 1205 public boolean hasReceivedElement() { 1206 return this.received != null && !this.received.isEmpty(); 1207 } 1208 1209 public boolean hasReceived() { 1210 return this.received != null && !this.received.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #received} (The time when this communication arrived at the destination.). This is the underlying object with id, value and extensions. The accessor "getReceived" gives direct access to the value 1215 */ 1216 public Communication setReceivedElement(DateTimeType value) { 1217 this.received = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return The time when this communication arrived at the destination. 1223 */ 1224 public Date getReceived() { 1225 return this.received == null ? null : this.received.getValue(); 1226 } 1227 1228 /** 1229 * @param value The time when this communication arrived at the destination. 1230 */ 1231 public Communication setReceived(Date value) { 1232 if (value == null) 1233 this.received = null; 1234 else { 1235 if (this.received == null) 1236 this.received = new DateTimeType(); 1237 this.received.setValue(value); 1238 } 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #recipient} (The entity (e.g. person, organization, clinical information system, care team or device) which is the target of the communication.) 1244 */ 1245 public List<Reference> getRecipient() { 1246 if (this.recipient == null) 1247 this.recipient = new ArrayList<Reference>(); 1248 return this.recipient; 1249 } 1250 1251 /** 1252 * @return Returns a reference to <code>this</code> for easy method chaining 1253 */ 1254 public Communication setRecipient(List<Reference> theRecipient) { 1255 this.recipient = theRecipient; 1256 return this; 1257 } 1258 1259 public boolean hasRecipient() { 1260 if (this.recipient == null) 1261 return false; 1262 for (Reference item : this.recipient) 1263 if (!item.isEmpty()) 1264 return true; 1265 return false; 1266 } 1267 1268 public Reference addRecipient() { //3 1269 Reference t = new Reference(); 1270 if (this.recipient == null) 1271 this.recipient = new ArrayList<Reference>(); 1272 this.recipient.add(t); 1273 return t; 1274 } 1275 1276 public Communication addRecipient(Reference t) { //3 1277 if (t == null) 1278 return this; 1279 if (this.recipient == null) 1280 this.recipient = new ArrayList<Reference>(); 1281 this.recipient.add(t); 1282 return this; 1283 } 1284 1285 /** 1286 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist {3} 1287 */ 1288 public Reference getRecipientFirstRep() { 1289 if (getRecipient().isEmpty()) { 1290 addRecipient(); 1291 } 1292 return getRecipient().get(0); 1293 } 1294 1295 /** 1296 * @return {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which is the source of the communication.) 1297 */ 1298 public Reference getSender() { 1299 if (this.sender == null) 1300 if (Configuration.errorOnAutoCreate()) 1301 throw new Error("Attempt to auto-create Communication.sender"); 1302 else if (Configuration.doAutoCreate()) 1303 this.sender = new Reference(); // cc 1304 return this.sender; 1305 } 1306 1307 public boolean hasSender() { 1308 return this.sender != null && !this.sender.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #sender} (The entity (e.g. person, organization, clinical information system, or device) which is the source of the communication.) 1313 */ 1314 public Communication setSender(Reference value) { 1315 this.sender = value; 1316 return this; 1317 } 1318 1319 /** 1320 * @return {@link #reason} (The reason or justification for the communication.) 1321 */ 1322 public List<CodeableReference> getReason() { 1323 if (this.reason == null) 1324 this.reason = new ArrayList<CodeableReference>(); 1325 return this.reason; 1326 } 1327 1328 /** 1329 * @return Returns a reference to <code>this</code> for easy method chaining 1330 */ 1331 public Communication setReason(List<CodeableReference> theReason) { 1332 this.reason = theReason; 1333 return this; 1334 } 1335 1336 public boolean hasReason() { 1337 if (this.reason == null) 1338 return false; 1339 for (CodeableReference item : this.reason) 1340 if (!item.isEmpty()) 1341 return true; 1342 return false; 1343 } 1344 1345 public CodeableReference addReason() { //3 1346 CodeableReference t = new CodeableReference(); 1347 if (this.reason == null) 1348 this.reason = new ArrayList<CodeableReference>(); 1349 this.reason.add(t); 1350 return t; 1351 } 1352 1353 public Communication addReason(CodeableReference t) { //3 1354 if (t == null) 1355 return this; 1356 if (this.reason == null) 1357 this.reason = new ArrayList<CodeableReference>(); 1358 this.reason.add(t); 1359 return this; 1360 } 1361 1362 /** 1363 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1364 */ 1365 public CodeableReference getReasonFirstRep() { 1366 if (getReason().isEmpty()) { 1367 addReason(); 1368 } 1369 return getReason().get(0); 1370 } 1371 1372 /** 1373 * @return {@link #payload} (Text, attachment(s), or resource(s) that was communicated to the recipient.) 1374 */ 1375 public List<CommunicationPayloadComponent> getPayload() { 1376 if (this.payload == null) 1377 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1378 return this.payload; 1379 } 1380 1381 /** 1382 * @return Returns a reference to <code>this</code> for easy method chaining 1383 */ 1384 public Communication setPayload(List<CommunicationPayloadComponent> thePayload) { 1385 this.payload = thePayload; 1386 return this; 1387 } 1388 1389 public boolean hasPayload() { 1390 if (this.payload == null) 1391 return false; 1392 for (CommunicationPayloadComponent item : this.payload) 1393 if (!item.isEmpty()) 1394 return true; 1395 return false; 1396 } 1397 1398 public CommunicationPayloadComponent addPayload() { //3 1399 CommunicationPayloadComponent t = new CommunicationPayloadComponent(); 1400 if (this.payload == null) 1401 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1402 this.payload.add(t); 1403 return t; 1404 } 1405 1406 public Communication addPayload(CommunicationPayloadComponent t) { //3 1407 if (t == null) 1408 return this; 1409 if (this.payload == null) 1410 this.payload = new ArrayList<CommunicationPayloadComponent>(); 1411 this.payload.add(t); 1412 return this; 1413 } 1414 1415 /** 1416 * @return The first repetition of repeating field {@link #payload}, creating it if it does not already exist {3} 1417 */ 1418 public CommunicationPayloadComponent getPayloadFirstRep() { 1419 if (getPayload().isEmpty()) { 1420 addPayload(); 1421 } 1422 return getPayload().get(0); 1423 } 1424 1425 /** 1426 * @return {@link #note} (Additional notes or commentary about the communication by the sender, receiver or other interested parties.) 1427 */ 1428 public List<Annotation> getNote() { 1429 if (this.note == null) 1430 this.note = new ArrayList<Annotation>(); 1431 return this.note; 1432 } 1433 1434 /** 1435 * @return Returns a reference to <code>this</code> for easy method chaining 1436 */ 1437 public Communication setNote(List<Annotation> theNote) { 1438 this.note = theNote; 1439 return this; 1440 } 1441 1442 public boolean hasNote() { 1443 if (this.note == null) 1444 return false; 1445 for (Annotation item : this.note) 1446 if (!item.isEmpty()) 1447 return true; 1448 return false; 1449 } 1450 1451 public Annotation addNote() { //3 1452 Annotation t = new Annotation(); 1453 if (this.note == null) 1454 this.note = new ArrayList<Annotation>(); 1455 this.note.add(t); 1456 return t; 1457 } 1458 1459 public Communication addNote(Annotation t) { //3 1460 if (t == null) 1461 return this; 1462 if (this.note == null) 1463 this.note = new ArrayList<Annotation>(); 1464 this.note.add(t); 1465 return this; 1466 } 1467 1468 /** 1469 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1470 */ 1471 public Annotation getNoteFirstRep() { 1472 if (getNote().isEmpty()) { 1473 addNote(); 1474 } 1475 return getNote().get(0); 1476 } 1477 1478 protected void listChildren(List<Property> children) { 1479 super.listChildren(children); 1480 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1481 children.add(new Property("instantiatesCanonical", "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 1482 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 1483 children.add(new Property("basedOn", "Reference(Any)", "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1484 children.add(new Property("partOf", "Reference(Any)", "A larger event (e.g. Communication, Procedure) of which this particular communication is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1485 children.add(new Property("inResponseTo", "Reference(Communication)", "Prior communication that this communication is in response to.", 0, java.lang.Integer.MAX_VALUE, inResponseTo)); 1486 children.add(new Property("status", "code", "The status of the transmission.", 0, 1, status)); 1487 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the Communication.", 0, 1, statusReason)); 1488 children.add(new Property("category", "CodeableConcept", "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 1489 children.add(new Property("priority", "code", "Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.", 0, 1, priority)); 1490 children.add(new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 1491 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group that was the focus of this communication.", 0, 1, subject)); 1492 children.add(new Property("topic", "CodeableConcept", "Description of the purpose/content, similar to a subject line in an email.", 0, 1, topic)); 1493 children.add(new Property("about", "Reference(Any)", "Other resources that pertain to this communication and to which this communication should be associated.", 0, java.lang.Integer.MAX_VALUE, about)); 1494 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this Communication was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1495 children.add(new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent)); 1496 children.add(new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 1, received)); 1497 children.add(new Property("recipient", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Endpoint)", "The entity (e.g. person, organization, clinical information system, care team or device) which is the target of the communication.", 0, java.lang.Integer.MAX_VALUE, recipient)); 1498 children.add(new Property("sender", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService|Endpoint|CareTeam)", "The entity (e.g. person, organization, clinical information system, or device) which is the source of the communication.", 0, 1, sender)); 1499 children.add(new Property("reason", "CodeableReference(Any)", "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reason)); 1500 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload)); 1501 children.add(new Property("note", "Annotation", "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 0, java.lang.Integer.MAX_VALUE, note)); 1502 } 1503 1504 @Override 1505 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1506 switch (_hash) { 1507 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1508 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 1509 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 1510 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1511 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Any)", "A larger event (e.g. Communication, Procedure) of which this particular communication is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1512 case 1932956065: /*inResponseTo*/ return new Property("inResponseTo", "Reference(Communication)", "Prior communication that this communication is in response to.", 0, java.lang.Integer.MAX_VALUE, inResponseTo); 1513 case -892481550: /*status*/ return new Property("status", "code", "The status of the transmission.", 0, 1, status); 1514 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the Communication.", 0, 1, statusReason); 1515 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category); 1516 case -1165461084: /*priority*/ return new Property("priority", "code", "Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.", 0, 1, priority); 1517 case -1078030475: /*medium*/ return new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 1518 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group that was the focus of this communication.", 0, 1, subject); 1519 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Description of the purpose/content, similar to a subject line in an email.", 0, 1, topic); 1520 case 92611469: /*about*/ return new Property("about", "Reference(Any)", "Other resources that pertain to this communication and to which this communication should be associated.", 0, java.lang.Integer.MAX_VALUE, about); 1521 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this Communication was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1522 case 3526552: /*sent*/ return new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent); 1523 case -808719903: /*received*/ return new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 1, received); 1524 case 820081177: /*recipient*/ return new Property("recipient", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Endpoint)", "The entity (e.g. person, organization, clinical information system, care team or device) which is the target of the communication.", 0, java.lang.Integer.MAX_VALUE, recipient); 1525 case -905962955: /*sender*/ return new Property("sender", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService|Endpoint|CareTeam)", "The entity (e.g. person, organization, clinical information system, or device) which is the source of the communication.", 0, 1, sender); 1526 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Any)", "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reason); 1527 case -786701938: /*payload*/ return new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload); 1528 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 0, java.lang.Integer.MAX_VALUE, note); 1529 default: return super.getNamedProperty(_hash, _name, _checkValid); 1530 } 1531 1532 } 1533 1534 @Override 1535 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1536 switch (hash) { 1537 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1538 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 1539 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 1540 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1541 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1542 case 1932956065: /*inResponseTo*/ return this.inResponseTo == null ? new Base[0] : this.inResponseTo.toArray(new Base[this.inResponseTo.size()]); // Reference 1543 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EventStatus> 1544 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 1545 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1546 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1547 case -1078030475: /*medium*/ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 1548 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1549 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // CodeableConcept 1550 case 92611469: /*about*/ return this.about == null ? new Base[0] : this.about.toArray(new Base[this.about.size()]); // Reference 1551 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1552 case 3526552: /*sent*/ return this.sent == null ? new Base[0] : new Base[] {this.sent}; // DateTimeType 1553 case -808719903: /*received*/ return this.received == null ? new Base[0] : new Base[] {this.received}; // DateTimeType 1554 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1555 case -905962955: /*sender*/ return this.sender == null ? new Base[0] : new Base[] {this.sender}; // Reference 1556 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1557 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationPayloadComponent 1558 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1559 default: return super.getProperty(hash, name, checkValid); 1560 } 1561 1562 } 1563 1564 @Override 1565 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1566 switch (hash) { 1567 case -1618432855: // identifier 1568 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1569 return value; 1570 case 8911915: // instantiatesCanonical 1571 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1572 return value; 1573 case -1926393373: // instantiatesUri 1574 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 1575 return value; 1576 case -332612366: // basedOn 1577 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1578 return value; 1579 case -995410646: // partOf 1580 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1581 return value; 1582 case 1932956065: // inResponseTo 1583 this.getInResponseTo().add(TypeConvertor.castToReference(value)); // Reference 1584 return value; 1585 case -892481550: // status 1586 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1587 this.status = (Enumeration) value; // Enumeration<EventStatus> 1588 return value; 1589 case 2051346646: // statusReason 1590 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1591 return value; 1592 case 50511102: // category 1593 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1594 return value; 1595 case -1165461084: // priority 1596 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1597 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1598 return value; 1599 case -1078030475: // medium 1600 this.getMedium().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1601 return value; 1602 case -1867885268: // subject 1603 this.subject = TypeConvertor.castToReference(value); // Reference 1604 return value; 1605 case 110546223: // topic 1606 this.topic = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1607 return value; 1608 case 92611469: // about 1609 this.getAbout().add(TypeConvertor.castToReference(value)); // Reference 1610 return value; 1611 case 1524132147: // encounter 1612 this.encounter = TypeConvertor.castToReference(value); // Reference 1613 return value; 1614 case 3526552: // sent 1615 this.sent = TypeConvertor.castToDateTime(value); // DateTimeType 1616 return value; 1617 case -808719903: // received 1618 this.received = TypeConvertor.castToDateTime(value); // DateTimeType 1619 return value; 1620 case 820081177: // recipient 1621 this.getRecipient().add(TypeConvertor.castToReference(value)); // Reference 1622 return value; 1623 case -905962955: // sender 1624 this.sender = TypeConvertor.castToReference(value); // Reference 1625 return value; 1626 case -934964668: // reason 1627 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1628 return value; 1629 case -786701938: // payload 1630 this.getPayload().add((CommunicationPayloadComponent) value); // CommunicationPayloadComponent 1631 return value; 1632 case 3387378: // note 1633 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1634 return value; 1635 default: return super.setProperty(hash, name, value); 1636 } 1637 1638 } 1639 1640 @Override 1641 public Base setProperty(String name, Base value) throws FHIRException { 1642 if (name.equals("identifier")) { 1643 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1644 } else if (name.equals("instantiatesCanonical")) { 1645 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 1646 } else if (name.equals("instantiatesUri")) { 1647 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 1648 } else if (name.equals("basedOn")) { 1649 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1650 } else if (name.equals("partOf")) { 1651 this.getPartOf().add(TypeConvertor.castToReference(value)); 1652 } else if (name.equals("inResponseTo")) { 1653 this.getInResponseTo().add(TypeConvertor.castToReference(value)); 1654 } else if (name.equals("status")) { 1655 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1656 this.status = (Enumeration) value; // Enumeration<EventStatus> 1657 } else if (name.equals("statusReason")) { 1658 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1659 } else if (name.equals("category")) { 1660 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1661 } else if (name.equals("priority")) { 1662 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1663 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1664 } else if (name.equals("medium")) { 1665 this.getMedium().add(TypeConvertor.castToCodeableConcept(value)); 1666 } else if (name.equals("subject")) { 1667 this.subject = TypeConvertor.castToReference(value); // Reference 1668 } else if (name.equals("topic")) { 1669 this.topic = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1670 } else if (name.equals("about")) { 1671 this.getAbout().add(TypeConvertor.castToReference(value)); 1672 } else if (name.equals("encounter")) { 1673 this.encounter = TypeConvertor.castToReference(value); // Reference 1674 } else if (name.equals("sent")) { 1675 this.sent = TypeConvertor.castToDateTime(value); // DateTimeType 1676 } else if (name.equals("received")) { 1677 this.received = TypeConvertor.castToDateTime(value); // DateTimeType 1678 } else if (name.equals("recipient")) { 1679 this.getRecipient().add(TypeConvertor.castToReference(value)); 1680 } else if (name.equals("sender")) { 1681 this.sender = TypeConvertor.castToReference(value); // Reference 1682 } else if (name.equals("reason")) { 1683 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1684 } else if (name.equals("payload")) { 1685 this.getPayload().add((CommunicationPayloadComponent) value); 1686 } else if (name.equals("note")) { 1687 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1688 } else 1689 return super.setProperty(name, value); 1690 return value; 1691 } 1692 1693 @Override 1694 public void removeChild(String name, Base value) throws FHIRException { 1695 if (name.equals("identifier")) { 1696 this.getIdentifier().remove(value); 1697 } else if (name.equals("instantiatesCanonical")) { 1698 this.getInstantiatesCanonical().remove(value); 1699 } else if (name.equals("instantiatesUri")) { 1700 this.getInstantiatesUri().remove(value); 1701 } else if (name.equals("basedOn")) { 1702 this.getBasedOn().remove(value); 1703 } else if (name.equals("partOf")) { 1704 this.getPartOf().remove(value); 1705 } else if (name.equals("inResponseTo")) { 1706 this.getInResponseTo().remove(value); 1707 } else if (name.equals("status")) { 1708 value = new EventStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1709 this.status = (Enumeration) value; // Enumeration<EventStatus> 1710 } else if (name.equals("statusReason")) { 1711 this.statusReason = null; 1712 } else if (name.equals("category")) { 1713 this.getCategory().remove(value); 1714 } else if (name.equals("priority")) { 1715 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1716 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1717 } else if (name.equals("medium")) { 1718 this.getMedium().remove(value); 1719 } else if (name.equals("subject")) { 1720 this.subject = null; 1721 } else if (name.equals("topic")) { 1722 this.topic = null; 1723 } else if (name.equals("about")) { 1724 this.getAbout().remove(value); 1725 } else if (name.equals("encounter")) { 1726 this.encounter = null; 1727 } else if (name.equals("sent")) { 1728 this.sent = null; 1729 } else if (name.equals("received")) { 1730 this.received = null; 1731 } else if (name.equals("recipient")) { 1732 this.getRecipient().remove(value); 1733 } else if (name.equals("sender")) { 1734 this.sender = null; 1735 } else if (name.equals("reason")) { 1736 this.getReason().remove(value); 1737 } else if (name.equals("payload")) { 1738 this.getPayload().remove((CommunicationPayloadComponent) value); 1739 } else if (name.equals("note")) { 1740 this.getNote().remove(value); 1741 } else 1742 super.removeChild(name, value); 1743 1744 } 1745 1746 @Override 1747 public Base makeProperty(int hash, String name) throws FHIRException { 1748 switch (hash) { 1749 case -1618432855: return addIdentifier(); 1750 case 8911915: return addInstantiatesCanonicalElement(); 1751 case -1926393373: return addInstantiatesUriElement(); 1752 case -332612366: return addBasedOn(); 1753 case -995410646: return addPartOf(); 1754 case 1932956065: return addInResponseTo(); 1755 case -892481550: return getStatusElement(); 1756 case 2051346646: return getStatusReason(); 1757 case 50511102: return addCategory(); 1758 case -1165461084: return getPriorityElement(); 1759 case -1078030475: return addMedium(); 1760 case -1867885268: return getSubject(); 1761 case 110546223: return getTopic(); 1762 case 92611469: return addAbout(); 1763 case 1524132147: return getEncounter(); 1764 case 3526552: return getSentElement(); 1765 case -808719903: return getReceivedElement(); 1766 case 820081177: return addRecipient(); 1767 case -905962955: return getSender(); 1768 case -934964668: return addReason(); 1769 case -786701938: return addPayload(); 1770 case 3387378: return addNote(); 1771 default: return super.makeProperty(hash, name); 1772 } 1773 1774 } 1775 1776 @Override 1777 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1778 switch (hash) { 1779 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1780 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 1781 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 1782 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1783 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1784 case 1932956065: /*inResponseTo*/ return new String[] {"Reference"}; 1785 case -892481550: /*status*/ return new String[] {"code"}; 1786 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 1787 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1788 case -1165461084: /*priority*/ return new String[] {"code"}; 1789 case -1078030475: /*medium*/ return new String[] {"CodeableConcept"}; 1790 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1791 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 1792 case 92611469: /*about*/ return new String[] {"Reference"}; 1793 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1794 case 3526552: /*sent*/ return new String[] {"dateTime"}; 1795 case -808719903: /*received*/ return new String[] {"dateTime"}; 1796 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1797 case -905962955: /*sender*/ return new String[] {"Reference"}; 1798 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1799 case -786701938: /*payload*/ return new String[] {}; 1800 case 3387378: /*note*/ return new String[] {"Annotation"}; 1801 default: return super.getTypesForProperty(hash, name); 1802 } 1803 1804 } 1805 1806 @Override 1807 public Base addChild(String name) throws FHIRException { 1808 if (name.equals("identifier")) { 1809 return addIdentifier(); 1810 } 1811 else if (name.equals("instantiatesCanonical")) { 1812 throw new FHIRException("Cannot call addChild on a singleton property Communication.instantiatesCanonical"); 1813 } 1814 else if (name.equals("instantiatesUri")) { 1815 throw new FHIRException("Cannot call addChild on a singleton property Communication.instantiatesUri"); 1816 } 1817 else if (name.equals("basedOn")) { 1818 return addBasedOn(); 1819 } 1820 else if (name.equals("partOf")) { 1821 return addPartOf(); 1822 } 1823 else if (name.equals("inResponseTo")) { 1824 return addInResponseTo(); 1825 } 1826 else if (name.equals("status")) { 1827 throw new FHIRException("Cannot call addChild on a singleton property Communication.status"); 1828 } 1829 else if (name.equals("statusReason")) { 1830 this.statusReason = new CodeableConcept(); 1831 return this.statusReason; 1832 } 1833 else if (name.equals("category")) { 1834 return addCategory(); 1835 } 1836 else if (name.equals("priority")) { 1837 throw new FHIRException("Cannot call addChild on a singleton property Communication.priority"); 1838 } 1839 else if (name.equals("medium")) { 1840 return addMedium(); 1841 } 1842 else if (name.equals("subject")) { 1843 this.subject = new Reference(); 1844 return this.subject; 1845 } 1846 else if (name.equals("topic")) { 1847 this.topic = new CodeableConcept(); 1848 return this.topic; 1849 } 1850 else if (name.equals("about")) { 1851 return addAbout(); 1852 } 1853 else if (name.equals("encounter")) { 1854 this.encounter = new Reference(); 1855 return this.encounter; 1856 } 1857 else if (name.equals("sent")) { 1858 throw new FHIRException("Cannot call addChild on a singleton property Communication.sent"); 1859 } 1860 else if (name.equals("received")) { 1861 throw new FHIRException("Cannot call addChild on a singleton property Communication.received"); 1862 } 1863 else if (name.equals("recipient")) { 1864 return addRecipient(); 1865 } 1866 else if (name.equals("sender")) { 1867 this.sender = new Reference(); 1868 return this.sender; 1869 } 1870 else if (name.equals("reason")) { 1871 return addReason(); 1872 } 1873 else if (name.equals("payload")) { 1874 return addPayload(); 1875 } 1876 else if (name.equals("note")) { 1877 return addNote(); 1878 } 1879 else 1880 return super.addChild(name); 1881 } 1882 1883 public String fhirType() { 1884 return "Communication"; 1885 1886 } 1887 1888 public Communication copy() { 1889 Communication dst = new Communication(); 1890 copyValues(dst); 1891 return dst; 1892 } 1893 1894 public void copyValues(Communication dst) { 1895 super.copyValues(dst); 1896 if (identifier != null) { 1897 dst.identifier = new ArrayList<Identifier>(); 1898 for (Identifier i : identifier) 1899 dst.identifier.add(i.copy()); 1900 }; 1901 if (instantiatesCanonical != null) { 1902 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 1903 for (CanonicalType i : instantiatesCanonical) 1904 dst.instantiatesCanonical.add(i.copy()); 1905 }; 1906 if (instantiatesUri != null) { 1907 dst.instantiatesUri = new ArrayList<UriType>(); 1908 for (UriType i : instantiatesUri) 1909 dst.instantiatesUri.add(i.copy()); 1910 }; 1911 if (basedOn != null) { 1912 dst.basedOn = new ArrayList<Reference>(); 1913 for (Reference i : basedOn) 1914 dst.basedOn.add(i.copy()); 1915 }; 1916 if (partOf != null) { 1917 dst.partOf = new ArrayList<Reference>(); 1918 for (Reference i : partOf) 1919 dst.partOf.add(i.copy()); 1920 }; 1921 if (inResponseTo != null) { 1922 dst.inResponseTo = new ArrayList<Reference>(); 1923 for (Reference i : inResponseTo) 1924 dst.inResponseTo.add(i.copy()); 1925 }; 1926 dst.status = status == null ? null : status.copy(); 1927 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1928 if (category != null) { 1929 dst.category = new ArrayList<CodeableConcept>(); 1930 for (CodeableConcept i : category) 1931 dst.category.add(i.copy()); 1932 }; 1933 dst.priority = priority == null ? null : priority.copy(); 1934 if (medium != null) { 1935 dst.medium = new ArrayList<CodeableConcept>(); 1936 for (CodeableConcept i : medium) 1937 dst.medium.add(i.copy()); 1938 }; 1939 dst.subject = subject == null ? null : subject.copy(); 1940 dst.topic = topic == null ? null : topic.copy(); 1941 if (about != null) { 1942 dst.about = new ArrayList<Reference>(); 1943 for (Reference i : about) 1944 dst.about.add(i.copy()); 1945 }; 1946 dst.encounter = encounter == null ? null : encounter.copy(); 1947 dst.sent = sent == null ? null : sent.copy(); 1948 dst.received = received == null ? null : received.copy(); 1949 if (recipient != null) { 1950 dst.recipient = new ArrayList<Reference>(); 1951 for (Reference i : recipient) 1952 dst.recipient.add(i.copy()); 1953 }; 1954 dst.sender = sender == null ? null : sender.copy(); 1955 if (reason != null) { 1956 dst.reason = new ArrayList<CodeableReference>(); 1957 for (CodeableReference i : reason) 1958 dst.reason.add(i.copy()); 1959 }; 1960 if (payload != null) { 1961 dst.payload = new ArrayList<CommunicationPayloadComponent>(); 1962 for (CommunicationPayloadComponent i : payload) 1963 dst.payload.add(i.copy()); 1964 }; 1965 if (note != null) { 1966 dst.note = new ArrayList<Annotation>(); 1967 for (Annotation i : note) 1968 dst.note.add(i.copy()); 1969 }; 1970 } 1971 1972 protected Communication typedCopy() { 1973 return copy(); 1974 } 1975 1976 @Override 1977 public boolean equalsDeep(Base other_) { 1978 if (!super.equalsDeep(other_)) 1979 return false; 1980 if (!(other_ instanceof Communication)) 1981 return false; 1982 Communication o = (Communication) other_; 1983 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 1984 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 1985 && compareDeep(partOf, o.partOf, true) && compareDeep(inResponseTo, o.inResponseTo, true) && compareDeep(status, o.status, true) 1986 && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 1987 && compareDeep(medium, o.medium, true) && compareDeep(subject, o.subject, true) && compareDeep(topic, o.topic, true) 1988 && compareDeep(about, o.about, true) && compareDeep(encounter, o.encounter, true) && compareDeep(sent, o.sent, true) 1989 && compareDeep(received, o.received, true) && compareDeep(recipient, o.recipient, true) && compareDeep(sender, o.sender, true) 1990 && compareDeep(reason, o.reason, true) && compareDeep(payload, o.payload, true) && compareDeep(note, o.note, true) 1991 ; 1992 } 1993 1994 @Override 1995 public boolean equalsShallow(Base other_) { 1996 if (!super.equalsShallow(other_)) 1997 return false; 1998 if (!(other_ instanceof Communication)) 1999 return false; 2000 Communication o = (Communication) other_; 2001 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 2002 && compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(sent, o.sent, true) 2003 && compareValues(received, o.received, true); 2004 } 2005 2006 public boolean isEmpty() { 2007 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 2008 , instantiatesUri, basedOn, partOf, inResponseTo, status, statusReason, category 2009 , priority, medium, subject, topic, about, encounter, sent, received, recipient 2010 , sender, reason, payload, note); 2011 } 2012 2013 @Override 2014 public ResourceType getResourceType() { 2015 return ResourceType.Communication; 2016 } 2017 2018 /** 2019 * Search parameter: <b>based-on</b> 2020 * <p> 2021 * Description: <b>Request fulfilled by this communication</b><br> 2022 * Type: <b>reference</b><br> 2023 * Path: <b>Communication.basedOn</b><br> 2024 * </p> 2025 */ 2026 @SearchParamDefinition(name="based-on", path="Communication.basedOn", description="Request fulfilled by this communication", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2027 public static final String SP_BASED_ON = "based-on"; 2028 /** 2029 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2030 * <p> 2031 * Description: <b>Request fulfilled by this communication</b><br> 2032 * Type: <b>reference</b><br> 2033 * Path: <b>Communication.basedOn</b><br> 2034 * </p> 2035 */ 2036 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2037 2038/** 2039 * Constant for fluent queries to be used to add include statements. Specifies 2040 * the path value of "<b>Communication:based-on</b>". 2041 */ 2042 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Communication:based-on").toLocked(); 2043 2044 /** 2045 * Search parameter: <b>category</b> 2046 * <p> 2047 * Description: <b>Message category</b><br> 2048 * Type: <b>token</b><br> 2049 * Path: <b>Communication.category</b><br> 2050 * </p> 2051 */ 2052 @SearchParamDefinition(name="category", path="Communication.category", description="Message category", type="token" ) 2053 public static final String SP_CATEGORY = "category"; 2054 /** 2055 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2056 * <p> 2057 * Description: <b>Message category</b><br> 2058 * Type: <b>token</b><br> 2059 * Path: <b>Communication.category</b><br> 2060 * </p> 2061 */ 2062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2063 2064 /** 2065 * Search parameter: <b>instantiates-canonical</b> 2066 * <p> 2067 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2068 * Type: <b>reference</b><br> 2069 * Path: <b>Communication.instantiatesCanonical</b><br> 2070 * </p> 2071 */ 2072 @SearchParamDefinition(name="instantiates-canonical", path="Communication.instantiatesCanonical", description="Instantiates FHIR protocol or definition", type="reference", target={ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class } ) 2073 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 2074 /** 2075 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 2076 * <p> 2077 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2078 * Type: <b>reference</b><br> 2079 * Path: <b>Communication.instantiatesCanonical</b><br> 2080 * </p> 2081 */ 2082 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 2083 2084/** 2085 * Constant for fluent queries to be used to add include statements. Specifies 2086 * the path value of "<b>Communication:instantiates-canonical</b>". 2087 */ 2088 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("Communication:instantiates-canonical").toLocked(); 2089 2090 /** 2091 * Search parameter: <b>instantiates-uri</b> 2092 * <p> 2093 * Description: <b>Instantiates external protocol or definition</b><br> 2094 * Type: <b>uri</b><br> 2095 * Path: <b>Communication.instantiatesUri</b><br> 2096 * </p> 2097 */ 2098 @SearchParamDefinition(name="instantiates-uri", path="Communication.instantiatesUri", description="Instantiates external protocol or definition", type="uri" ) 2099 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 2100 /** 2101 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 2102 * <p> 2103 * Description: <b>Instantiates external protocol or definition</b><br> 2104 * Type: <b>uri</b><br> 2105 * Path: <b>Communication.instantiatesUri</b><br> 2106 * </p> 2107 */ 2108 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 2109 2110 /** 2111 * Search parameter: <b>medium</b> 2112 * <p> 2113 * Description: <b>A channel of communication</b><br> 2114 * Type: <b>token</b><br> 2115 * Path: <b>Communication.medium</b><br> 2116 * </p> 2117 */ 2118 @SearchParamDefinition(name="medium", path="Communication.medium", description="A channel of communication", type="token" ) 2119 public static final String SP_MEDIUM = "medium"; 2120 /** 2121 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 2122 * <p> 2123 * Description: <b>A channel of communication</b><br> 2124 * Type: <b>token</b><br> 2125 * Path: <b>Communication.medium</b><br> 2126 * </p> 2127 */ 2128 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MEDIUM); 2129 2130 /** 2131 * Search parameter: <b>part-of</b> 2132 * <p> 2133 * Description: <b>Part of referenced event (e.g. Communication, Procedure)</b><br> 2134 * Type: <b>reference</b><br> 2135 * Path: <b>Communication.partOf</b><br> 2136 * </p> 2137 */ 2138 @SearchParamDefinition(name="part-of", path="Communication.partOf", description="Part of referenced event (e.g. Communication, Procedure)", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2139 public static final String SP_PART_OF = "part-of"; 2140 /** 2141 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2142 * <p> 2143 * Description: <b>Part of referenced event (e.g. Communication, Procedure)</b><br> 2144 * Type: <b>reference</b><br> 2145 * Path: <b>Communication.partOf</b><br> 2146 * </p> 2147 */ 2148 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2149 2150/** 2151 * Constant for fluent queries to be used to add include statements. Specifies 2152 * the path value of "<b>Communication:part-of</b>". 2153 */ 2154 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Communication:part-of").toLocked(); 2155 2156 /** 2157 * Search parameter: <b>received</b> 2158 * <p> 2159 * Description: <b>When received</b><br> 2160 * Type: <b>date</b><br> 2161 * Path: <b>Communication.received</b><br> 2162 * </p> 2163 */ 2164 @SearchParamDefinition(name="received", path="Communication.received", description="When received", type="date" ) 2165 public static final String SP_RECEIVED = "received"; 2166 /** 2167 * <b>Fluent Client</b> search parameter constant for <b>received</b> 2168 * <p> 2169 * Description: <b>When received</b><br> 2170 * Type: <b>date</b><br> 2171 * Path: <b>Communication.received</b><br> 2172 * </p> 2173 */ 2174 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECEIVED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECEIVED); 2175 2176 /** 2177 * Search parameter: <b>recipient</b> 2178 * <p> 2179 * Description: <b>Who the information is shared with</b><br> 2180 * Type: <b>reference</b><br> 2181 * Path: <b>Communication.recipient</b><br> 2182 * </p> 2183 */ 2184 @SearchParamDefinition(name="recipient", path="Communication.recipient", description="Who the information is shared with", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson"), @ca.uhn.fhir.model.api.annotation.Compartment(name="EXAMPLE") }, target={CareTeam.class, Device.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2185 public static final String SP_RECIPIENT = "recipient"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2188 * <p> 2189 * Description: <b>Who the information is shared with</b><br> 2190 * Type: <b>reference</b><br> 2191 * Path: <b>Communication.recipient</b><br> 2192 * </p> 2193 */ 2194 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2195 2196/** 2197 * Constant for fluent queries to be used to add include statements. Specifies 2198 * the path value of "<b>Communication:recipient</b>". 2199 */ 2200 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("Communication:recipient").toLocked(); 2201 2202 /** 2203 * Search parameter: <b>sender</b> 2204 * <p> 2205 * Description: <b>Who shares the information</b><br> 2206 * Type: <b>reference</b><br> 2207 * Path: <b>Communication.sender</b><br> 2208 * </p> 2209 */ 2210 @SearchParamDefinition(name="sender", path="Communication.sender", description="Who shares the information", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson"), @ca.uhn.fhir.model.api.annotation.Compartment(name="EXAMPLE") }, target={CareTeam.class, Device.class, Endpoint.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2211 public static final String SP_SENDER = "sender"; 2212 /** 2213 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2214 * <p> 2215 * Description: <b>Who shares the information</b><br> 2216 * Type: <b>reference</b><br> 2217 * Path: <b>Communication.sender</b><br> 2218 * </p> 2219 */ 2220 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SENDER); 2221 2222/** 2223 * Constant for fluent queries to be used to add include statements. Specifies 2224 * the path value of "<b>Communication:sender</b>". 2225 */ 2226 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include("Communication:sender").toLocked(); 2227 2228 /** 2229 * Search parameter: <b>sent</b> 2230 * <p> 2231 * Description: <b>When sent</b><br> 2232 * Type: <b>date</b><br> 2233 * Path: <b>Communication.sent</b><br> 2234 * </p> 2235 */ 2236 @SearchParamDefinition(name="sent", path="Communication.sent", description="When sent", type="date" ) 2237 public static final String SP_SENT = "sent"; 2238 /** 2239 * <b>Fluent Client</b> search parameter constant for <b>sent</b> 2240 * <p> 2241 * Description: <b>When sent</b><br> 2242 * Type: <b>date</b><br> 2243 * Path: <b>Communication.sent</b><br> 2244 * </p> 2245 */ 2246 public static final ca.uhn.fhir.rest.gclient.DateClientParam SENT = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_SENT); 2247 2248 /** 2249 * Search parameter: <b>status</b> 2250 * <p> 2251 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown</b><br> 2252 * Type: <b>token</b><br> 2253 * Path: <b>Communication.status</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name="status", path="Communication.status", description="preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type="token" ) 2257 public static final String SP_STATUS = "status"; 2258 /** 2259 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2260 * <p> 2261 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown</b><br> 2262 * Type: <b>token</b><br> 2263 * Path: <b>Communication.status</b><br> 2264 * </p> 2265 */ 2266 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2267 2268 /** 2269 * Search parameter: <b>subject</b> 2270 * <p> 2271 * Description: <b>Focus of message</b><br> 2272 * Type: <b>reference</b><br> 2273 * Path: <b>Communication.subject</b><br> 2274 * </p> 2275 */ 2276 @SearchParamDefinition(name="subject", path="Communication.subject", description="Focus of message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2277 public static final String SP_SUBJECT = "subject"; 2278 /** 2279 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2280 * <p> 2281 * Description: <b>Focus of message</b><br> 2282 * Type: <b>reference</b><br> 2283 * Path: <b>Communication.subject</b><br> 2284 * </p> 2285 */ 2286 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2287 2288/** 2289 * Constant for fluent queries to be used to add include statements. Specifies 2290 * the path value of "<b>Communication:subject</b>". 2291 */ 2292 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Communication:subject").toLocked(); 2293 2294 /** 2295 * Search parameter: <b>topic</b> 2296 * <p> 2297 * Description: <b>Description of the purpose/content</b><br> 2298 * Type: <b>token</b><br> 2299 * Path: <b>Communication.topic</b><br> 2300 * </p> 2301 */ 2302 @SearchParamDefinition(name="topic", path="Communication.topic", description="Description of the purpose/content", type="token" ) 2303 public static final String SP_TOPIC = "topic"; 2304 /** 2305 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 2306 * <p> 2307 * Description: <b>Description of the purpose/content</b><br> 2308 * Type: <b>token</b><br> 2309 * Path: <b>Communication.topic</b><br> 2310 * </p> 2311 */ 2312 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 2313 2314 /** 2315 * Search parameter: <b>encounter</b> 2316 * <p> 2317 * Description: <b>Multiple Resources: 2318 2319* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2320* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2321* [ChargeItem](chargeitem.html): Encounter associated with event 2322* [Claim](claim.html): Encounters associated with a billed line item 2323* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2324* [Communication](communication.html): The Encounter during which this Communication was created 2325* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2326* [Composition](composition.html): Context of the Composition 2327* [Condition](condition.html): The Encounter during which this Condition was created 2328* [DeviceRequest](devicerequest.html): Encounter during which request was created 2329* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2330* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2331* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2332* [Flag](flag.html): Alert relevant during encounter 2333* [ImagingStudy](imagingstudy.html): The context of the study 2334* [List](list.html): Context in which list created 2335* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2336* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2337* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2338* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2339* [Observation](observation.html): Encounter related to the observation 2340* [Procedure](procedure.html): The Encounter during which this Procedure was created 2341* [Provenance](provenance.html): Encounter related to the Provenance 2342* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2343* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2344* [RiskAssessment](riskassessment.html): Where was assessment performed? 2345* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2346* [Task](task.html): Search by encounter 2347* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2348</b><br> 2349 * Type: <b>reference</b><br> 2350 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2351 * </p> 2352 */ 2353 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2354 public static final String SP_ENCOUNTER = "encounter"; 2355 /** 2356 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2357 * <p> 2358 * Description: <b>Multiple Resources: 2359 2360* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2361* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2362* [ChargeItem](chargeitem.html): Encounter associated with event 2363* [Claim](claim.html): Encounters associated with a billed line item 2364* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2365* [Communication](communication.html): The Encounter during which this Communication was created 2366* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2367* [Composition](composition.html): Context of the Composition 2368* [Condition](condition.html): The Encounter during which this Condition was created 2369* [DeviceRequest](devicerequest.html): Encounter during which request was created 2370* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2371* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2372* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2373* [Flag](flag.html): Alert relevant during encounter 2374* [ImagingStudy](imagingstudy.html): The context of the study 2375* [List](list.html): Context in which list created 2376* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2377* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2378* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2379* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2380* [Observation](observation.html): Encounter related to the observation 2381* [Procedure](procedure.html): The Encounter during which this Procedure was created 2382* [Provenance](provenance.html): Encounter related to the Provenance 2383* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2384* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2385* [RiskAssessment](riskassessment.html): Where was assessment performed? 2386* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2387* [Task](task.html): Search by encounter 2388* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2389</b><br> 2390 * Type: <b>reference</b><br> 2391 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2392 * </p> 2393 */ 2394 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2395 2396/** 2397 * Constant for fluent queries to be used to add include statements. Specifies 2398 * the path value of "<b>Communication:encounter</b>". 2399 */ 2400 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Communication:encounter").toLocked(); 2401 2402 /** 2403 * Search parameter: <b>identifier</b> 2404 * <p> 2405 * Description: <b>Multiple Resources: 2406 2407* [Account](account.html): Account number 2408* [AdverseEvent](adverseevent.html): Business identifier for the event 2409* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2410* [Appointment](appointment.html): An Identifier of the Appointment 2411* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2412* [Basic](basic.html): Business identifier 2413* [BodyStructure](bodystructure.html): Bodystructure identifier 2414* [CarePlan](careplan.html): External Ids for this plan 2415* [CareTeam](careteam.html): External Ids for this team 2416* [ChargeItem](chargeitem.html): Business Identifier for item 2417* [Claim](claim.html): The primary identifier of the financial resource 2418* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2419* [ClinicalImpression](clinicalimpression.html): Business identifier 2420* [Communication](communication.html): Unique identifier 2421* [CommunicationRequest](communicationrequest.html): Unique identifier 2422* [Composition](composition.html): Version-independent identifier for the Composition 2423* [Condition](condition.html): A unique identifier of the condition record 2424* [Consent](consent.html): Identifier for this record (external references) 2425* [Contract](contract.html): The identity of the contract 2426* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2427* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2428* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2429* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2430* [DeviceRequest](devicerequest.html): Business identifier for request/order 2431* [DeviceUsage](deviceusage.html): Search by identifier 2432* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2433* [DocumentReference](documentreference.html): Identifier of the attachment binary 2434* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2435* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2436* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2437* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2438* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2439* [Flag](flag.html): Business identifier 2440* [Goal](goal.html): External Ids for this goal 2441* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2442* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2443* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2444* [Immunization](immunization.html): Business identifier 2445* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2446* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2447* [Invoice](invoice.html): Business Identifier for item 2448* [List](list.html): Business identifier 2449* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2450* [Medication](medication.html): Returns medications with this external identifier 2451* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2452* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2453* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2454* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2455* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2456* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2457* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2458* [Observation](observation.html): The unique id for a particular observation 2459* [Person](person.html): A person Identifier 2460* [Procedure](procedure.html): A unique identifier for a procedure 2461* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2462* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2463* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2464* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2465* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2466* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2467* [Specimen](specimen.html): The unique identifier associated with the specimen 2468* [SupplyDelivery](supplydelivery.html): External identifier 2469* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2470* [Task](task.html): Search for a task instance by its business identifier 2471* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2472</b><br> 2473 * Type: <b>token</b><br> 2474 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2475 * </p> 2476 */ 2477 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2478 public static final String SP_IDENTIFIER = "identifier"; 2479 /** 2480 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2481 * <p> 2482 * Description: <b>Multiple Resources: 2483 2484* [Account](account.html): Account number 2485* [AdverseEvent](adverseevent.html): Business identifier for the event 2486* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2487* [Appointment](appointment.html): An Identifier of the Appointment 2488* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2489* [Basic](basic.html): Business identifier 2490* [BodyStructure](bodystructure.html): Bodystructure identifier 2491* [CarePlan](careplan.html): External Ids for this plan 2492* [CareTeam](careteam.html): External Ids for this team 2493* [ChargeItem](chargeitem.html): Business Identifier for item 2494* [Claim](claim.html): The primary identifier of the financial resource 2495* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2496* [ClinicalImpression](clinicalimpression.html): Business identifier 2497* [Communication](communication.html): Unique identifier 2498* [CommunicationRequest](communicationrequest.html): Unique identifier 2499* [Composition](composition.html): Version-independent identifier for the Composition 2500* [Condition](condition.html): A unique identifier of the condition record 2501* [Consent](consent.html): Identifier for this record (external references) 2502* [Contract](contract.html): The identity of the contract 2503* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2504* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2505* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2506* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2507* [DeviceRequest](devicerequest.html): Business identifier for request/order 2508* [DeviceUsage](deviceusage.html): Search by identifier 2509* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2510* [DocumentReference](documentreference.html): Identifier of the attachment binary 2511* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2512* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2513* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2514* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2515* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2516* [Flag](flag.html): Business identifier 2517* [Goal](goal.html): External Ids for this goal 2518* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2519* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2520* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2521* [Immunization](immunization.html): Business identifier 2522* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2523* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2524* [Invoice](invoice.html): Business Identifier for item 2525* [List](list.html): Business identifier 2526* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2527* [Medication](medication.html): Returns medications with this external identifier 2528* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2529* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2530* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2531* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2532* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2533* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2534* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2535* [Observation](observation.html): The unique id for a particular observation 2536* [Person](person.html): A person Identifier 2537* [Procedure](procedure.html): A unique identifier for a procedure 2538* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2539* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2540* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2541* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2542* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2543* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2544* [Specimen](specimen.html): The unique identifier associated with the specimen 2545* [SupplyDelivery](supplydelivery.html): External identifier 2546* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2547* [Task](task.html): Search for a task instance by its business identifier 2548* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2549</b><br> 2550 * Type: <b>token</b><br> 2551 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2552 * </p> 2553 */ 2554 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2555 2556 /** 2557 * Search parameter: <b>patient</b> 2558 * <p> 2559 * Description: <b>Multiple Resources: 2560 2561* [Account](account.html): The entity that caused the expenses 2562* [AdverseEvent](adverseevent.html): Subject impacted by event 2563* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2564* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2565* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2566* [AuditEvent](auditevent.html): Where the activity involved patient data 2567* [Basic](basic.html): Identifies the focus of this resource 2568* [BodyStructure](bodystructure.html): Who this is about 2569* [CarePlan](careplan.html): Who the care plan is for 2570* [CareTeam](careteam.html): Who care team is for 2571* [ChargeItem](chargeitem.html): Individual service was done for/to 2572* [Claim](claim.html): Patient receiving the products or services 2573* [ClaimResponse](claimresponse.html): The subject of care 2574* [ClinicalImpression](clinicalimpression.html): Patient assessed 2575* [Communication](communication.html): Focus of message 2576* [CommunicationRequest](communicationrequest.html): Focus of message 2577* [Composition](composition.html): Who and/or what the composition is about 2578* [Condition](condition.html): Who has the condition? 2579* [Consent](consent.html): Who the consent applies to 2580* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2581* [Coverage](coverage.html): Retrieve coverages for a patient 2582* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2583* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2584* [DetectedIssue](detectedissue.html): Associated patient 2585* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2586* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2587* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2588* [DocumentReference](documentreference.html): Who/what is the subject of the document 2589* [Encounter](encounter.html): The patient present at the encounter 2590* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2591* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2592* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2593* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2594* [Flag](flag.html): The identity of a subject to list flags for 2595* [Goal](goal.html): Who this goal is intended for 2596* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2597* [ImagingSelection](imagingselection.html): Who the study is about 2598* [ImagingStudy](imagingstudy.html): Who the study is about 2599* [Immunization](immunization.html): The patient for the vaccination record 2600* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2601* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2602* [Invoice](invoice.html): Recipient(s) of goods and services 2603* [List](list.html): If all resources have the same subject 2604* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2605* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2606* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2607* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2608* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2609* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2610* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2611* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2612* [Observation](observation.html): The subject that the observation is about (if patient) 2613* [Person](person.html): The Person links to this Patient 2614* [Procedure](procedure.html): Search by subject - a patient 2615* [Provenance](provenance.html): Where the activity involved patient data 2616* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2617* [RelatedPerson](relatedperson.html): The patient this related person is related to 2618* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2619* [ResearchSubject](researchsubject.html): Who or what is part of study 2620* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2621* [ServiceRequest](servicerequest.html): Search by subject - a patient 2622* [Specimen](specimen.html): The patient the specimen comes from 2623* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2624* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2625* [Task](task.html): Search by patient 2626* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2627</b><br> 2628 * Type: <b>reference</b><br> 2629 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2630 * </p> 2631 */ 2632 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2633 public static final String SP_PATIENT = "patient"; 2634 /** 2635 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2636 * <p> 2637 * Description: <b>Multiple Resources: 2638 2639* [Account](account.html): The entity that caused the expenses 2640* [AdverseEvent](adverseevent.html): Subject impacted by event 2641* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2642* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2643* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2644* [AuditEvent](auditevent.html): Where the activity involved patient data 2645* [Basic](basic.html): Identifies the focus of this resource 2646* [BodyStructure](bodystructure.html): Who this is about 2647* [CarePlan](careplan.html): Who the care plan is for 2648* [CareTeam](careteam.html): Who care team is for 2649* [ChargeItem](chargeitem.html): Individual service was done for/to 2650* [Claim](claim.html): Patient receiving the products or services 2651* [ClaimResponse](claimresponse.html): The subject of care 2652* [ClinicalImpression](clinicalimpression.html): Patient assessed 2653* [Communication](communication.html): Focus of message 2654* [CommunicationRequest](communicationrequest.html): Focus of message 2655* [Composition](composition.html): Who and/or what the composition is about 2656* [Condition](condition.html): Who has the condition? 2657* [Consent](consent.html): Who the consent applies to 2658* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2659* [Coverage](coverage.html): Retrieve coverages for a patient 2660* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2661* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2662* [DetectedIssue](detectedissue.html): Associated patient 2663* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2664* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2665* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2666* [DocumentReference](documentreference.html): Who/what is the subject of the document 2667* [Encounter](encounter.html): The patient present at the encounter 2668* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2669* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2670* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2671* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2672* [Flag](flag.html): The identity of a subject to list flags for 2673* [Goal](goal.html): Who this goal is intended for 2674* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2675* [ImagingSelection](imagingselection.html): Who the study is about 2676* [ImagingStudy](imagingstudy.html): Who the study is about 2677* [Immunization](immunization.html): The patient for the vaccination record 2678* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2679* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2680* [Invoice](invoice.html): Recipient(s) of goods and services 2681* [List](list.html): If all resources have the same subject 2682* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2683* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2684* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2685* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2686* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2687* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2688* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2689* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2690* [Observation](observation.html): The subject that the observation is about (if patient) 2691* [Person](person.html): The Person links to this Patient 2692* [Procedure](procedure.html): Search by subject - a patient 2693* [Provenance](provenance.html): Where the activity involved patient data 2694* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2695* [RelatedPerson](relatedperson.html): The patient this related person is related to 2696* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2697* [ResearchSubject](researchsubject.html): Who or what is part of study 2698* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2699* [ServiceRequest](servicerequest.html): Search by subject - a patient 2700* [Specimen](specimen.html): The patient the specimen comes from 2701* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2702* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2703* [Task](task.html): Search by patient 2704* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2705</b><br> 2706 * Type: <b>reference</b><br> 2707 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2708 * </p> 2709 */ 2710 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2711 2712/** 2713 * Constant for fluent queries to be used to add include statements. Specifies 2714 * the path value of "<b>Communication:patient</b>". 2715 */ 2716 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Communication:patient").toLocked(); 2717 2718 2719} 2720