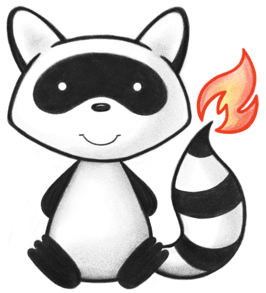
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 052 */ 053@ResourceDef(name="CommunicationRequest", profile="http://hl7.org/fhir/StructureDefinition/CommunicationRequest") 054public class CommunicationRequest extends DomainResource { 055 056 @Block() 057 public static class CommunicationRequestPayloadComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The communicated content (or for multi-part communications, one portion of the communication). 060 */ 061 @Child(name = "content", type = {Attachment.class, Reference.class, CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Message part content", formalDefinition="The communicated content (or for multi-part communications, one portion of the communication)." ) 063 protected DataType content; 064 065 private static final long serialVersionUID = -1954179063L; 066 067 /** 068 * Constructor 069 */ 070 public CommunicationRequestPayloadComponent() { 071 super(); 072 } 073 074 /** 075 * Constructor 076 */ 077 public CommunicationRequestPayloadComponent(DataType content) { 078 super(); 079 this.setContent(content); 080 } 081 082 /** 083 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 084 */ 085 public DataType getContent() { 086 return this.content; 087 } 088 089 /** 090 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 091 */ 092 public Attachment getContentAttachment() throws FHIRException { 093 if (this.content == null) 094 this.content = new Attachment(); 095 if (!(this.content instanceof Attachment)) 096 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 097 return (Attachment) this.content; 098 } 099 100 public boolean hasContentAttachment() { 101 return this.content instanceof Attachment; 102 } 103 104 /** 105 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 106 */ 107 public Reference getContentReference() throws FHIRException { 108 if (this.content == null) 109 this.content = new Reference(); 110 if (!(this.content instanceof Reference)) 111 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 112 return (Reference) this.content; 113 } 114 115 public boolean hasContentReference() { 116 return this.content instanceof Reference; 117 } 118 119 /** 120 * @return {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 121 */ 122 public CodeableConcept getContentCodeableConcept() throws FHIRException { 123 if (this.content == null) 124 this.content = new CodeableConcept(); 125 if (!(this.content instanceof CodeableConcept)) 126 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.content.getClass().getName()+" was encountered"); 127 return (CodeableConcept) this.content; 128 } 129 130 public boolean hasContentCodeableConcept() { 131 return this.content instanceof CodeableConcept; 132 } 133 134 public boolean hasContent() { 135 return this.content != null && !this.content.isEmpty(); 136 } 137 138 /** 139 * @param value {@link #content} (The communicated content (or for multi-part communications, one portion of the communication).) 140 */ 141 public CommunicationRequestPayloadComponent setContent(DataType value) { 142 if (value != null && !(value instanceof Attachment || value instanceof Reference || value instanceof CodeableConcept)) 143 throw new FHIRException("Not the right type for CommunicationRequest.payload.content[x]: "+value.fhirType()); 144 this.content = value; 145 return this; 146 } 147 148 protected void listChildren(List<Property> children) { 149 super.listChildren(children); 150 children.add(new Property("content[x]", "Attachment|Reference(Any)|CodeableConcept", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content)); 151 } 152 153 @Override 154 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 155 switch (_hash) { 156 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(Any)|CodeableConcept", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 157 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(Any)|CodeableConcept", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 158 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 159 case 1193747154: /*contentReference*/ return new Property("content[x]", "Reference(Any)", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 160 case 1554698728: /*contentCodeableConcept*/ return new Property("content[x]", "CodeableConcept", "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, content); 161 default: return super.getNamedProperty(_hash, _name, _checkValid); 162 } 163 164 } 165 166 @Override 167 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 168 switch (hash) { 169 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // DataType 170 default: return super.getProperty(hash, name, checkValid); 171 } 172 173 } 174 175 @Override 176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 177 switch (hash) { 178 case 951530617: // content 179 this.content = TypeConvertor.castToType(value); // DataType 180 return value; 181 default: return super.setProperty(hash, name, value); 182 } 183 184 } 185 186 @Override 187 public Base setProperty(String name, Base value) throws FHIRException { 188 if (name.equals("content[x]")) { 189 this.content = TypeConvertor.castToType(value); // DataType 190 } else 191 return super.setProperty(name, value); 192 return value; 193 } 194 195 @Override 196 public void removeChild(String name, Base value) throws FHIRException { 197 if (name.equals("content[x]")) { 198 this.content = null; 199 } else 200 super.removeChild(name, value); 201 202 } 203 204 @Override 205 public Base makeProperty(int hash, String name) throws FHIRException { 206 switch (hash) { 207 case 264548711: return getContent(); 208 case 951530617: return getContent(); 209 default: return super.makeProperty(hash, name); 210 } 211 212 } 213 214 @Override 215 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 216 switch (hash) { 217 case 951530617: /*content*/ return new String[] {"Attachment", "Reference", "CodeableConcept"}; 218 default: return super.getTypesForProperty(hash, name); 219 } 220 221 } 222 223 @Override 224 public Base addChild(String name) throws FHIRException { 225 if (name.equals("contentAttachment")) { 226 this.content = new Attachment(); 227 return this.content; 228 } 229 else if (name.equals("contentReference")) { 230 this.content = new Reference(); 231 return this.content; 232 } 233 else if (name.equals("contentCodeableConcept")) { 234 this.content = new CodeableConcept(); 235 return this.content; 236 } 237 else 238 return super.addChild(name); 239 } 240 241 public CommunicationRequestPayloadComponent copy() { 242 CommunicationRequestPayloadComponent dst = new CommunicationRequestPayloadComponent(); 243 copyValues(dst); 244 return dst; 245 } 246 247 public void copyValues(CommunicationRequestPayloadComponent dst) { 248 super.copyValues(dst); 249 dst.content = content == null ? null : content.copy(); 250 } 251 252 @Override 253 public boolean equalsDeep(Base other_) { 254 if (!super.equalsDeep(other_)) 255 return false; 256 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 257 return false; 258 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 259 return compareDeep(content, o.content, true); 260 } 261 262 @Override 263 public boolean equalsShallow(Base other_) { 264 if (!super.equalsShallow(other_)) 265 return false; 266 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 267 return false; 268 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 269 return true; 270 } 271 272 public boolean isEmpty() { 273 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 274 } 275 276 public String fhirType() { 277 return "CommunicationRequest.payload"; 278 279 } 280 281 } 282 283 /** 284 * Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 285 */ 286 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 287 @Description(shortDefinition="Unique identifier", formalDefinition="Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 288 protected List<Identifier> identifier; 289 290 /** 291 * A plan or proposal that is fulfilled in whole or in part by this request. 292 */ 293 @Child(name = "basedOn", type = {Reference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 294 @Description(shortDefinition="Fulfills plan or proposal", formalDefinition="A plan or proposal that is fulfilled in whole or in part by this request." ) 295 protected List<Reference> basedOn; 296 297 /** 298 * Completed or terminated request(s) whose function is taken by this new request. 299 */ 300 @Child(name = "replaces", type = {CommunicationRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 301 @Description(shortDefinition="Request(s) replaced by this request", formalDefinition="Completed or terminated request(s) whose function is taken by this new request." ) 302 protected List<Reference> replaces; 303 304 /** 305 * A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 306 */ 307 @Child(name = "groupIdentifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 308 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 309 protected Identifier groupIdentifier; 310 311 /** 312 * The status of the proposal or order. 313 */ 314 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 315 @Description(shortDefinition="draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition="The status of the proposal or order." ) 316 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 317 protected Enumeration<RequestStatus> status; 318 319 /** 320 * Captures the reason for the current state of the CommunicationRequest. 321 */ 322 @Child(name = "statusReason", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 323 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current state of the CommunicationRequest." ) 324 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-request-status-reason") 325 protected CodeableConcept statusReason; 326 327 /** 328 * Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain. 329 */ 330 @Child(name = "intent", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 331 @Description(shortDefinition="proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain." ) 332 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 333 protected Enumeration<RequestIntent> intent; 334 335 /** 336 * The type of message to be sent such as alert, notification, reminder, instruction, etc. 337 */ 338 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 339 @Description(shortDefinition="Message category", formalDefinition="The type of message to be sent such as alert, notification, reminder, instruction, etc." ) 340 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/communication-category") 341 protected List<CodeableConcept> category; 342 343 /** 344 * Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine. 345 */ 346 @Child(name = "priority", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 347 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine." ) 348 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 349 protected Enumeration<RequestPriority> priority; 350 351 /** 352 * If true indicates that the CommunicationRequest is asking for the specified action to *not* occur. 353 */ 354 @Child(name = "doNotPerform", type = {BooleanType.class}, order=9, min=0, max=1, modifier=true, summary=true) 355 @Description(shortDefinition="True if request is prohibiting action", formalDefinition="If true indicates that the CommunicationRequest is asking for the specified action to *not* occur." ) 356 protected BooleanType doNotPerform; 357 358 /** 359 * A channel that was used for this communication (e.g. email, fax). 360 */ 361 @Child(name = "medium", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 362 @Description(shortDefinition="A channel of communication", formalDefinition="A channel that was used for this communication (e.g. email, fax)." ) 363 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ParticipationMode") 364 protected List<CodeableConcept> medium; 365 366 /** 367 * The patient or group that is the focus of this communication request. 368 */ 369 @Child(name = "subject", type = {Patient.class, Group.class}, order=11, min=0, max=1, modifier=false, summary=false) 370 @Description(shortDefinition="Focus of message", formalDefinition="The patient or group that is the focus of this communication request." ) 371 protected Reference subject; 372 373 /** 374 * Other resources that pertain to this communication request and to which this communication request should be associated. 375 */ 376 @Child(name = "about", type = {Reference.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 377 @Description(shortDefinition="Resources that pertain to this communication request", formalDefinition="Other resources that pertain to this communication request and to which this communication request should be associated." ) 378 protected List<Reference> about; 379 380 /** 381 * The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated. 382 */ 383 @Child(name = "encounter", type = {Encounter.class}, order=13, min=0, max=1, modifier=false, summary=true) 384 @Description(shortDefinition="The Encounter during which this CommunicationRequest was created", formalDefinition="The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated." ) 385 protected Reference encounter; 386 387 /** 388 * Text, attachment(s), or resource(s) to be communicated to the recipient. 389 */ 390 @Child(name = "payload", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 391 @Description(shortDefinition="Message payload", formalDefinition="Text, attachment(s), or resource(s) to be communicated to the recipient." ) 392 protected List<CommunicationRequestPayloadComponent> payload; 393 394 /** 395 * The time when this communication is to occur. 396 */ 397 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=15, min=0, max=1, modifier=false, summary=true) 398 @Description(shortDefinition="When scheduled", formalDefinition="The time when this communication is to occur." ) 399 protected DataType occurrence; 400 401 /** 402 * For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 403 */ 404 @Child(name = "authoredOn", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=true) 405 @Description(shortDefinition="When request transitioned to being actionable", formalDefinition="For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation." ) 406 protected DateTimeType authoredOn; 407 408 /** 409 * The device, individual, or organization who asks for the information to be shared. 410 */ 411 @Child(name = "requester", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=17, min=0, max=1, modifier=false, summary=true) 412 @Description(shortDefinition="Who asks for the information to be shared", formalDefinition="The device, individual, or organization who asks for the information to be shared." ) 413 protected Reference requester; 414 415 /** 416 * The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication. 417 */ 418 @Child(name = "recipient", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Group.class, CareTeam.class, HealthcareService.class, Endpoint.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 419 @Description(shortDefinition="Who to share the information with", formalDefinition="The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication." ) 420 protected List<Reference> recipient; 421 422 /** 423 * The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication. 424 */ 425 @Child(name = "informationProvider", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, HealthcareService.class, Endpoint.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 426 @Description(shortDefinition="Who should share the information", formalDefinition="The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication." ) 427 protected List<Reference> informationProvider; 428 429 /** 430 * Describes why the request is being made in coded or textual form. 431 */ 432 @Child(name = "reason", type = {CodeableReference.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 433 @Description(shortDefinition="Why is communication needed?", formalDefinition="Describes why the request is being made in coded or textual form." ) 434 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActReason") 435 protected List<CodeableReference> reason; 436 437 /** 438 * Comments made about the request by the requester, sender, recipient, subject or other participants. 439 */ 440 @Child(name = "note", type = {Annotation.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 441 @Description(shortDefinition="Comments made about communication request", formalDefinition="Comments made about the request by the requester, sender, recipient, subject or other participants." ) 442 protected List<Annotation> note; 443 444 private static final long serialVersionUID = -442811807L; 445 446 /** 447 * Constructor 448 */ 449 public CommunicationRequest() { 450 super(); 451 } 452 453 /** 454 * Constructor 455 */ 456 public CommunicationRequest(RequestStatus status, RequestIntent intent) { 457 super(); 458 this.setStatus(status); 459 this.setIntent(intent); 460 } 461 462 /** 463 * @return {@link #identifier} (Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 464 */ 465 public List<Identifier> getIdentifier() { 466 if (this.identifier == null) 467 this.identifier = new ArrayList<Identifier>(); 468 return this.identifier; 469 } 470 471 /** 472 * @return Returns a reference to <code>this</code> for easy method chaining 473 */ 474 public CommunicationRequest setIdentifier(List<Identifier> theIdentifier) { 475 this.identifier = theIdentifier; 476 return this; 477 } 478 479 public boolean hasIdentifier() { 480 if (this.identifier == null) 481 return false; 482 for (Identifier item : this.identifier) 483 if (!item.isEmpty()) 484 return true; 485 return false; 486 } 487 488 public Identifier addIdentifier() { //3 489 Identifier t = new Identifier(); 490 if (this.identifier == null) 491 this.identifier = new ArrayList<Identifier>(); 492 this.identifier.add(t); 493 return t; 494 } 495 496 public CommunicationRequest addIdentifier(Identifier t) { //3 497 if (t == null) 498 return this; 499 if (this.identifier == null) 500 this.identifier = new ArrayList<Identifier>(); 501 this.identifier.add(t); 502 return this; 503 } 504 505 /** 506 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 507 */ 508 public Identifier getIdentifierFirstRep() { 509 if (getIdentifier().isEmpty()) { 510 addIdentifier(); 511 } 512 return getIdentifier().get(0); 513 } 514 515 /** 516 * @return {@link #basedOn} (A plan or proposal that is fulfilled in whole or in part by this request.) 517 */ 518 public List<Reference> getBasedOn() { 519 if (this.basedOn == null) 520 this.basedOn = new ArrayList<Reference>(); 521 return this.basedOn; 522 } 523 524 /** 525 * @return Returns a reference to <code>this</code> for easy method chaining 526 */ 527 public CommunicationRequest setBasedOn(List<Reference> theBasedOn) { 528 this.basedOn = theBasedOn; 529 return this; 530 } 531 532 public boolean hasBasedOn() { 533 if (this.basedOn == null) 534 return false; 535 for (Reference item : this.basedOn) 536 if (!item.isEmpty()) 537 return true; 538 return false; 539 } 540 541 public Reference addBasedOn() { //3 542 Reference t = new Reference(); 543 if (this.basedOn == null) 544 this.basedOn = new ArrayList<Reference>(); 545 this.basedOn.add(t); 546 return t; 547 } 548 549 public CommunicationRequest addBasedOn(Reference t) { //3 550 if (t == null) 551 return this; 552 if (this.basedOn == null) 553 this.basedOn = new ArrayList<Reference>(); 554 this.basedOn.add(t); 555 return this; 556 } 557 558 /** 559 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 560 */ 561 public Reference getBasedOnFirstRep() { 562 if (getBasedOn().isEmpty()) { 563 addBasedOn(); 564 } 565 return getBasedOn().get(0); 566 } 567 568 /** 569 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 570 */ 571 public List<Reference> getReplaces() { 572 if (this.replaces == null) 573 this.replaces = new ArrayList<Reference>(); 574 return this.replaces; 575 } 576 577 /** 578 * @return Returns a reference to <code>this</code> for easy method chaining 579 */ 580 public CommunicationRequest setReplaces(List<Reference> theReplaces) { 581 this.replaces = theReplaces; 582 return this; 583 } 584 585 public boolean hasReplaces() { 586 if (this.replaces == null) 587 return false; 588 for (Reference item : this.replaces) 589 if (!item.isEmpty()) 590 return true; 591 return false; 592 } 593 594 public Reference addReplaces() { //3 595 Reference t = new Reference(); 596 if (this.replaces == null) 597 this.replaces = new ArrayList<Reference>(); 598 this.replaces.add(t); 599 return t; 600 } 601 602 public CommunicationRequest addReplaces(Reference t) { //3 603 if (t == null) 604 return this; 605 if (this.replaces == null) 606 this.replaces = new ArrayList<Reference>(); 607 this.replaces.add(t); 608 return this; 609 } 610 611 /** 612 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist {3} 613 */ 614 public Reference getReplacesFirstRep() { 615 if (getReplaces().isEmpty()) { 616 addReplaces(); 617 } 618 return getReplaces().get(0); 619 } 620 621 /** 622 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 623 */ 624 public Identifier getGroupIdentifier() { 625 if (this.groupIdentifier == null) 626 if (Configuration.errorOnAutoCreate()) 627 throw new Error("Attempt to auto-create CommunicationRequest.groupIdentifier"); 628 else if (Configuration.doAutoCreate()) 629 this.groupIdentifier = new Identifier(); // cc 630 return this.groupIdentifier; 631 } 632 633 public boolean hasGroupIdentifier() { 634 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 635 } 636 637 /** 638 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 639 */ 640 public CommunicationRequest setGroupIdentifier(Identifier value) { 641 this.groupIdentifier = value; 642 return this; 643 } 644 645 /** 646 * @return {@link #status} (The status of the proposal or order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 647 */ 648 public Enumeration<RequestStatus> getStatusElement() { 649 if (this.status == null) 650 if (Configuration.errorOnAutoCreate()) 651 throw new Error("Attempt to auto-create CommunicationRequest.status"); 652 else if (Configuration.doAutoCreate()) 653 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 654 return this.status; 655 } 656 657 public boolean hasStatusElement() { 658 return this.status != null && !this.status.isEmpty(); 659 } 660 661 public boolean hasStatus() { 662 return this.status != null && !this.status.isEmpty(); 663 } 664 665 /** 666 * @param value {@link #status} (The status of the proposal or order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 667 */ 668 public CommunicationRequest setStatusElement(Enumeration<RequestStatus> value) { 669 this.status = value; 670 return this; 671 } 672 673 /** 674 * @return The status of the proposal or order. 675 */ 676 public RequestStatus getStatus() { 677 return this.status == null ? null : this.status.getValue(); 678 } 679 680 /** 681 * @param value The status of the proposal or order. 682 */ 683 public CommunicationRequest setStatus(RequestStatus value) { 684 if (this.status == null) 685 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 686 this.status.setValue(value); 687 return this; 688 } 689 690 /** 691 * @return {@link #statusReason} (Captures the reason for the current state of the CommunicationRequest.) 692 */ 693 public CodeableConcept getStatusReason() { 694 if (this.statusReason == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create CommunicationRequest.statusReason"); 697 else if (Configuration.doAutoCreate()) 698 this.statusReason = new CodeableConcept(); // cc 699 return this.statusReason; 700 } 701 702 public boolean hasStatusReason() { 703 return this.statusReason != null && !this.statusReason.isEmpty(); 704 } 705 706 /** 707 * @param value {@link #statusReason} (Captures the reason for the current state of the CommunicationRequest.) 708 */ 709 public CommunicationRequest setStatusReason(CodeableConcept value) { 710 this.statusReason = value; 711 return this; 712 } 713 714 /** 715 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 716 */ 717 public Enumeration<RequestIntent> getIntentElement() { 718 if (this.intent == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create CommunicationRequest.intent"); 721 else if (Configuration.doAutoCreate()) 722 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 723 return this.intent; 724 } 725 726 public boolean hasIntentElement() { 727 return this.intent != null && !this.intent.isEmpty(); 728 } 729 730 public boolean hasIntent() { 731 return this.intent != null && !this.intent.isEmpty(); 732 } 733 734 /** 735 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 736 */ 737 public CommunicationRequest setIntentElement(Enumeration<RequestIntent> value) { 738 this.intent = value; 739 return this; 740 } 741 742 /** 743 * @return Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain. 744 */ 745 public RequestIntent getIntent() { 746 return this.intent == null ? null : this.intent.getValue(); 747 } 748 749 /** 750 * @param value Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain. 751 */ 752 public CommunicationRequest setIntent(RequestIntent value) { 753 if (this.intent == null) 754 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 755 this.intent.setValue(value); 756 return this; 757 } 758 759 /** 760 * @return {@link #category} (The type of message to be sent such as alert, notification, reminder, instruction, etc.) 761 */ 762 public List<CodeableConcept> getCategory() { 763 if (this.category == null) 764 this.category = new ArrayList<CodeableConcept>(); 765 return this.category; 766 } 767 768 /** 769 * @return Returns a reference to <code>this</code> for easy method chaining 770 */ 771 public CommunicationRequest setCategory(List<CodeableConcept> theCategory) { 772 this.category = theCategory; 773 return this; 774 } 775 776 public boolean hasCategory() { 777 if (this.category == null) 778 return false; 779 for (CodeableConcept item : this.category) 780 if (!item.isEmpty()) 781 return true; 782 return false; 783 } 784 785 public CodeableConcept addCategory() { //3 786 CodeableConcept t = new CodeableConcept(); 787 if (this.category == null) 788 this.category = new ArrayList<CodeableConcept>(); 789 this.category.add(t); 790 return t; 791 } 792 793 public CommunicationRequest addCategory(CodeableConcept t) { //3 794 if (t == null) 795 return this; 796 if (this.category == null) 797 this.category = new ArrayList<CodeableConcept>(); 798 this.category.add(t); 799 return this; 800 } 801 802 /** 803 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 804 */ 805 public CodeableConcept getCategoryFirstRep() { 806 if (getCategory().isEmpty()) { 807 addCategory(); 808 } 809 return getCategory().get(0); 810 } 811 812 /** 813 * @return {@link #priority} (Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 814 */ 815 public Enumeration<RequestPriority> getPriorityElement() { 816 if (this.priority == null) 817 if (Configuration.errorOnAutoCreate()) 818 throw new Error("Attempt to auto-create CommunicationRequest.priority"); 819 else if (Configuration.doAutoCreate()) 820 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 821 return this.priority; 822 } 823 824 public boolean hasPriorityElement() { 825 return this.priority != null && !this.priority.isEmpty(); 826 } 827 828 public boolean hasPriority() { 829 return this.priority != null && !this.priority.isEmpty(); 830 } 831 832 /** 833 * @param value {@link #priority} (Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 834 */ 835 public CommunicationRequest setPriorityElement(Enumeration<RequestPriority> value) { 836 this.priority = value; 837 return this; 838 } 839 840 /** 841 * @return Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine. 842 */ 843 public RequestPriority getPriority() { 844 return this.priority == null ? null : this.priority.getValue(); 845 } 846 847 /** 848 * @param value Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine. 849 */ 850 public CommunicationRequest setPriority(RequestPriority value) { 851 if (value == null) 852 this.priority = null; 853 else { 854 if (this.priority == null) 855 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 856 this.priority.setValue(value); 857 } 858 return this; 859 } 860 861 /** 862 * @return {@link #doNotPerform} (If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 863 */ 864 public BooleanType getDoNotPerformElement() { 865 if (this.doNotPerform == null) 866 if (Configuration.errorOnAutoCreate()) 867 throw new Error("Attempt to auto-create CommunicationRequest.doNotPerform"); 868 else if (Configuration.doAutoCreate()) 869 this.doNotPerform = new BooleanType(); // bb 870 return this.doNotPerform; 871 } 872 873 public boolean hasDoNotPerformElement() { 874 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 875 } 876 877 public boolean hasDoNotPerform() { 878 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 879 } 880 881 /** 882 * @param value {@link #doNotPerform} (If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 883 */ 884 public CommunicationRequest setDoNotPerformElement(BooleanType value) { 885 this.doNotPerform = value; 886 return this; 887 } 888 889 /** 890 * @return If true indicates that the CommunicationRequest is asking for the specified action to *not* occur. 891 */ 892 public boolean getDoNotPerform() { 893 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 894 } 895 896 /** 897 * @param value If true indicates that the CommunicationRequest is asking for the specified action to *not* occur. 898 */ 899 public CommunicationRequest setDoNotPerform(boolean value) { 900 if (this.doNotPerform == null) 901 this.doNotPerform = new BooleanType(); 902 this.doNotPerform.setValue(value); 903 return this; 904 } 905 906 /** 907 * @return {@link #medium} (A channel that was used for this communication (e.g. email, fax).) 908 */ 909 public List<CodeableConcept> getMedium() { 910 if (this.medium == null) 911 this.medium = new ArrayList<CodeableConcept>(); 912 return this.medium; 913 } 914 915 /** 916 * @return Returns a reference to <code>this</code> for easy method chaining 917 */ 918 public CommunicationRequest setMedium(List<CodeableConcept> theMedium) { 919 this.medium = theMedium; 920 return this; 921 } 922 923 public boolean hasMedium() { 924 if (this.medium == null) 925 return false; 926 for (CodeableConcept item : this.medium) 927 if (!item.isEmpty()) 928 return true; 929 return false; 930 } 931 932 public CodeableConcept addMedium() { //3 933 CodeableConcept t = new CodeableConcept(); 934 if (this.medium == null) 935 this.medium = new ArrayList<CodeableConcept>(); 936 this.medium.add(t); 937 return t; 938 } 939 940 public CommunicationRequest addMedium(CodeableConcept t) { //3 941 if (t == null) 942 return this; 943 if (this.medium == null) 944 this.medium = new ArrayList<CodeableConcept>(); 945 this.medium.add(t); 946 return this; 947 } 948 949 /** 950 * @return The first repetition of repeating field {@link #medium}, creating it if it does not already exist {3} 951 */ 952 public CodeableConcept getMediumFirstRep() { 953 if (getMedium().isEmpty()) { 954 addMedium(); 955 } 956 return getMedium().get(0); 957 } 958 959 /** 960 * @return {@link #subject} (The patient or group that is the focus of this communication request.) 961 */ 962 public Reference getSubject() { 963 if (this.subject == null) 964 if (Configuration.errorOnAutoCreate()) 965 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 966 else if (Configuration.doAutoCreate()) 967 this.subject = new Reference(); // cc 968 return this.subject; 969 } 970 971 public boolean hasSubject() { 972 return this.subject != null && !this.subject.isEmpty(); 973 } 974 975 /** 976 * @param value {@link #subject} (The patient or group that is the focus of this communication request.) 977 */ 978 public CommunicationRequest setSubject(Reference value) { 979 this.subject = value; 980 return this; 981 } 982 983 /** 984 * @return {@link #about} (Other resources that pertain to this communication request and to which this communication request should be associated.) 985 */ 986 public List<Reference> getAbout() { 987 if (this.about == null) 988 this.about = new ArrayList<Reference>(); 989 return this.about; 990 } 991 992 /** 993 * @return Returns a reference to <code>this</code> for easy method chaining 994 */ 995 public CommunicationRequest setAbout(List<Reference> theAbout) { 996 this.about = theAbout; 997 return this; 998 } 999 1000 public boolean hasAbout() { 1001 if (this.about == null) 1002 return false; 1003 for (Reference item : this.about) 1004 if (!item.isEmpty()) 1005 return true; 1006 return false; 1007 } 1008 1009 public Reference addAbout() { //3 1010 Reference t = new Reference(); 1011 if (this.about == null) 1012 this.about = new ArrayList<Reference>(); 1013 this.about.add(t); 1014 return t; 1015 } 1016 1017 public CommunicationRequest addAbout(Reference t) { //3 1018 if (t == null) 1019 return this; 1020 if (this.about == null) 1021 this.about = new ArrayList<Reference>(); 1022 this.about.add(t); 1023 return this; 1024 } 1025 1026 /** 1027 * @return The first repetition of repeating field {@link #about}, creating it if it does not already exist {3} 1028 */ 1029 public Reference getAboutFirstRep() { 1030 if (getAbout().isEmpty()) { 1031 addAbout(); 1032 } 1033 return getAbout().get(0); 1034 } 1035 1036 /** 1037 * @return {@link #encounter} (The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.) 1038 */ 1039 public Reference getEncounter() { 1040 if (this.encounter == null) 1041 if (Configuration.errorOnAutoCreate()) 1042 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1043 else if (Configuration.doAutoCreate()) 1044 this.encounter = new Reference(); // cc 1045 return this.encounter; 1046 } 1047 1048 public boolean hasEncounter() { 1049 return this.encounter != null && !this.encounter.isEmpty(); 1050 } 1051 1052 /** 1053 * @param value {@link #encounter} (The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.) 1054 */ 1055 public CommunicationRequest setEncounter(Reference value) { 1056 this.encounter = value; 1057 return this; 1058 } 1059 1060 /** 1061 * @return {@link #payload} (Text, attachment(s), or resource(s) to be communicated to the recipient.) 1062 */ 1063 public List<CommunicationRequestPayloadComponent> getPayload() { 1064 if (this.payload == null) 1065 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1066 return this.payload; 1067 } 1068 1069 /** 1070 * @return Returns a reference to <code>this</code> for easy method chaining 1071 */ 1072 public CommunicationRequest setPayload(List<CommunicationRequestPayloadComponent> thePayload) { 1073 this.payload = thePayload; 1074 return this; 1075 } 1076 1077 public boolean hasPayload() { 1078 if (this.payload == null) 1079 return false; 1080 for (CommunicationRequestPayloadComponent item : this.payload) 1081 if (!item.isEmpty()) 1082 return true; 1083 return false; 1084 } 1085 1086 public CommunicationRequestPayloadComponent addPayload() { //3 1087 CommunicationRequestPayloadComponent t = new CommunicationRequestPayloadComponent(); 1088 if (this.payload == null) 1089 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1090 this.payload.add(t); 1091 return t; 1092 } 1093 1094 public CommunicationRequest addPayload(CommunicationRequestPayloadComponent t) { //3 1095 if (t == null) 1096 return this; 1097 if (this.payload == null) 1098 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1099 this.payload.add(t); 1100 return this; 1101 } 1102 1103 /** 1104 * @return The first repetition of repeating field {@link #payload}, creating it if it does not already exist {3} 1105 */ 1106 public CommunicationRequestPayloadComponent getPayloadFirstRep() { 1107 if (getPayload().isEmpty()) { 1108 addPayload(); 1109 } 1110 return getPayload().get(0); 1111 } 1112 1113 /** 1114 * @return {@link #occurrence} (The time when this communication is to occur.) 1115 */ 1116 public DataType getOccurrence() { 1117 return this.occurrence; 1118 } 1119 1120 /** 1121 * @return {@link #occurrence} (The time when this communication is to occur.) 1122 */ 1123 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1124 if (this.occurrence == null) 1125 this.occurrence = new DateTimeType(); 1126 if (!(this.occurrence instanceof DateTimeType)) 1127 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1128 return (DateTimeType) this.occurrence; 1129 } 1130 1131 public boolean hasOccurrenceDateTimeType() { 1132 return this.occurrence instanceof DateTimeType; 1133 } 1134 1135 /** 1136 * @return {@link #occurrence} (The time when this communication is to occur.) 1137 */ 1138 public Period getOccurrencePeriod() throws FHIRException { 1139 if (this.occurrence == null) 1140 this.occurrence = new Period(); 1141 if (!(this.occurrence instanceof Period)) 1142 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1143 return (Period) this.occurrence; 1144 } 1145 1146 public boolean hasOccurrencePeriod() { 1147 return this.occurrence instanceof Period; 1148 } 1149 1150 public boolean hasOccurrence() { 1151 return this.occurrence != null && !this.occurrence.isEmpty(); 1152 } 1153 1154 /** 1155 * @param value {@link #occurrence} (The time when this communication is to occur.) 1156 */ 1157 public CommunicationRequest setOccurrence(DataType value) { 1158 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1159 throw new FHIRException("Not the right type for CommunicationRequest.occurrence[x]: "+value.fhirType()); 1160 this.occurrence = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return {@link #authoredOn} (For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1166 */ 1167 public DateTimeType getAuthoredOnElement() { 1168 if (this.authoredOn == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create CommunicationRequest.authoredOn"); 1171 else if (Configuration.doAutoCreate()) 1172 this.authoredOn = new DateTimeType(); // bb 1173 return this.authoredOn; 1174 } 1175 1176 public boolean hasAuthoredOnElement() { 1177 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1178 } 1179 1180 public boolean hasAuthoredOn() { 1181 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #authoredOn} (For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1186 */ 1187 public CommunicationRequest setAuthoredOnElement(DateTimeType value) { 1188 this.authoredOn = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1194 */ 1195 public Date getAuthoredOn() { 1196 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1197 } 1198 1199 /** 1200 * @param value For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation. 1201 */ 1202 public CommunicationRequest setAuthoredOn(Date value) { 1203 if (value == null) 1204 this.authoredOn = null; 1205 else { 1206 if (this.authoredOn == null) 1207 this.authoredOn = new DateTimeType(); 1208 this.authoredOn.setValue(value); 1209 } 1210 return this; 1211 } 1212 1213 /** 1214 * @return {@link #requester} (The device, individual, or organization who asks for the information to be shared.) 1215 */ 1216 public Reference getRequester() { 1217 if (this.requester == null) 1218 if (Configuration.errorOnAutoCreate()) 1219 throw new Error("Attempt to auto-create CommunicationRequest.requester"); 1220 else if (Configuration.doAutoCreate()) 1221 this.requester = new Reference(); // cc 1222 return this.requester; 1223 } 1224 1225 public boolean hasRequester() { 1226 return this.requester != null && !this.requester.isEmpty(); 1227 } 1228 1229 /** 1230 * @param value {@link #requester} (The device, individual, or organization who asks for the information to be shared.) 1231 */ 1232 public CommunicationRequest setRequester(Reference value) { 1233 this.requester = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #recipient} (The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.) 1239 */ 1240 public List<Reference> getRecipient() { 1241 if (this.recipient == null) 1242 this.recipient = new ArrayList<Reference>(); 1243 return this.recipient; 1244 } 1245 1246 /** 1247 * @return Returns a reference to <code>this</code> for easy method chaining 1248 */ 1249 public CommunicationRequest setRecipient(List<Reference> theRecipient) { 1250 this.recipient = theRecipient; 1251 return this; 1252 } 1253 1254 public boolean hasRecipient() { 1255 if (this.recipient == null) 1256 return false; 1257 for (Reference item : this.recipient) 1258 if (!item.isEmpty()) 1259 return true; 1260 return false; 1261 } 1262 1263 public Reference addRecipient() { //3 1264 Reference t = new Reference(); 1265 if (this.recipient == null) 1266 this.recipient = new ArrayList<Reference>(); 1267 this.recipient.add(t); 1268 return t; 1269 } 1270 1271 public CommunicationRequest addRecipient(Reference t) { //3 1272 if (t == null) 1273 return this; 1274 if (this.recipient == null) 1275 this.recipient = new ArrayList<Reference>(); 1276 this.recipient.add(t); 1277 return this; 1278 } 1279 1280 /** 1281 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist {3} 1282 */ 1283 public Reference getRecipientFirstRep() { 1284 if (getRecipient().isEmpty()) { 1285 addRecipient(); 1286 } 1287 return getRecipient().get(0); 1288 } 1289 1290 /** 1291 * @return {@link #informationProvider} (The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.) 1292 */ 1293 public List<Reference> getInformationProvider() { 1294 if (this.informationProvider == null) 1295 this.informationProvider = new ArrayList<Reference>(); 1296 return this.informationProvider; 1297 } 1298 1299 /** 1300 * @return Returns a reference to <code>this</code> for easy method chaining 1301 */ 1302 public CommunicationRequest setInformationProvider(List<Reference> theInformationProvider) { 1303 this.informationProvider = theInformationProvider; 1304 return this; 1305 } 1306 1307 public boolean hasInformationProvider() { 1308 if (this.informationProvider == null) 1309 return false; 1310 for (Reference item : this.informationProvider) 1311 if (!item.isEmpty()) 1312 return true; 1313 return false; 1314 } 1315 1316 public Reference addInformationProvider() { //3 1317 Reference t = new Reference(); 1318 if (this.informationProvider == null) 1319 this.informationProvider = new ArrayList<Reference>(); 1320 this.informationProvider.add(t); 1321 return t; 1322 } 1323 1324 public CommunicationRequest addInformationProvider(Reference t) { //3 1325 if (t == null) 1326 return this; 1327 if (this.informationProvider == null) 1328 this.informationProvider = new ArrayList<Reference>(); 1329 this.informationProvider.add(t); 1330 return this; 1331 } 1332 1333 /** 1334 * @return The first repetition of repeating field {@link #informationProvider}, creating it if it does not already exist {3} 1335 */ 1336 public Reference getInformationProviderFirstRep() { 1337 if (getInformationProvider().isEmpty()) { 1338 addInformationProvider(); 1339 } 1340 return getInformationProvider().get(0); 1341 } 1342 1343 /** 1344 * @return {@link #reason} (Describes why the request is being made in coded or textual form.) 1345 */ 1346 public List<CodeableReference> getReason() { 1347 if (this.reason == null) 1348 this.reason = new ArrayList<CodeableReference>(); 1349 return this.reason; 1350 } 1351 1352 /** 1353 * @return Returns a reference to <code>this</code> for easy method chaining 1354 */ 1355 public CommunicationRequest setReason(List<CodeableReference> theReason) { 1356 this.reason = theReason; 1357 return this; 1358 } 1359 1360 public boolean hasReason() { 1361 if (this.reason == null) 1362 return false; 1363 for (CodeableReference item : this.reason) 1364 if (!item.isEmpty()) 1365 return true; 1366 return false; 1367 } 1368 1369 public CodeableReference addReason() { //3 1370 CodeableReference t = new CodeableReference(); 1371 if (this.reason == null) 1372 this.reason = new ArrayList<CodeableReference>(); 1373 this.reason.add(t); 1374 return t; 1375 } 1376 1377 public CommunicationRequest addReason(CodeableReference t) { //3 1378 if (t == null) 1379 return this; 1380 if (this.reason == null) 1381 this.reason = new ArrayList<CodeableReference>(); 1382 this.reason.add(t); 1383 return this; 1384 } 1385 1386 /** 1387 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1388 */ 1389 public CodeableReference getReasonFirstRep() { 1390 if (getReason().isEmpty()) { 1391 addReason(); 1392 } 1393 return getReason().get(0); 1394 } 1395 1396 /** 1397 * @return {@link #note} (Comments made about the request by the requester, sender, recipient, subject or other participants.) 1398 */ 1399 public List<Annotation> getNote() { 1400 if (this.note == null) 1401 this.note = new ArrayList<Annotation>(); 1402 return this.note; 1403 } 1404 1405 /** 1406 * @return Returns a reference to <code>this</code> for easy method chaining 1407 */ 1408 public CommunicationRequest setNote(List<Annotation> theNote) { 1409 this.note = theNote; 1410 return this; 1411 } 1412 1413 public boolean hasNote() { 1414 if (this.note == null) 1415 return false; 1416 for (Annotation item : this.note) 1417 if (!item.isEmpty()) 1418 return true; 1419 return false; 1420 } 1421 1422 public Annotation addNote() { //3 1423 Annotation t = new Annotation(); 1424 if (this.note == null) 1425 this.note = new ArrayList<Annotation>(); 1426 this.note.add(t); 1427 return t; 1428 } 1429 1430 public CommunicationRequest addNote(Annotation t) { //3 1431 if (t == null) 1432 return this; 1433 if (this.note == null) 1434 this.note = new ArrayList<Annotation>(); 1435 this.note.add(t); 1436 return this; 1437 } 1438 1439 /** 1440 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1441 */ 1442 public Annotation getNoteFirstRep() { 1443 if (getNote().isEmpty()) { 1444 addNote(); 1445 } 1446 return getNote().get(0); 1447 } 1448 1449 protected void listChildren(List<Property> children) { 1450 super.listChildren(children); 1451 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1452 children.add(new Property("basedOn", "Reference(Any)", "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1453 children.add(new Property("replaces", "Reference(CommunicationRequest)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces)); 1454 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 1455 children.add(new Property("status", "code", "The status of the proposal or order.", 0, 1, status)); 1456 children.add(new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason)); 1457 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain.", 0, 1, intent)); 1458 children.add(new Property("category", "CodeableConcept", "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 1459 children.add(new Property("priority", "code", "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 0, 1, priority)); 1460 children.add(new Property("doNotPerform", "boolean", "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, doNotPerform)); 1461 children.add(new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 1462 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group that is the focus of this communication request.", 0, 1, subject)); 1463 children.add(new Property("about", "Reference(Any)", "Other resources that pertain to this communication request and to which this communication request should be associated.", 0, java.lang.Integer.MAX_VALUE, about)); 1464 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1465 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload)); 1466 children.add(new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence)); 1467 children.add(new Property("authoredOn", "dateTime", "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 0, 1, authoredOn)); 1468 children.add(new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The device, individual, or organization who asks for the information to be shared.", 0, 1, requester)); 1469 children.add(new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService|Endpoint)", "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 0, java.lang.Integer.MAX_VALUE, recipient)); 1470 children.add(new Property("informationProvider", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService|Endpoint)", "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 0, java.lang.Integer.MAX_VALUE, informationProvider)); 1471 children.add(new Property("reason", "CodeableReference(Any)", "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason)); 1472 children.add(new Property("note", "Annotation", "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1473 } 1474 1475 @Override 1476 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1477 switch (_hash) { 1478 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1479 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1480 case -430332865: /*replaces*/ return new Property("replaces", "Reference(CommunicationRequest)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces); 1481 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 1482 case -892481550: /*status*/ return new Property("status", "code", "The status of the proposal or order.", 0, 1, status); 1483 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason); 1484 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the CommunicationRequest and where the request fits into the workflow chain.", 0, 1, intent); 1485 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, java.lang.Integer.MAX_VALUE, category); 1486 case -1165461084: /*priority*/ return new Property("priority", "code", "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 0, 1, priority); 1487 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, doNotPerform); 1488 case -1078030475: /*medium*/ return new Property("medium", "CodeableConcept", "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 1489 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group that is the focus of this communication request.", 0, 1, subject); 1490 case 92611469: /*about*/ return new Property("about", "Reference(Any)", "Other resources that pertain to this communication request and to which this communication request should be associated.", 0, java.lang.Integer.MAX_VALUE, about); 1491 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1492 case -786701938: /*payload*/ return new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, payload); 1493 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence); 1494 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, occurrence); 1495 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The time when this communication is to occur.", 0, 1, occurrence); 1496 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The time when this communication is to occur.", 0, 1, occurrence); 1497 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 0, 1, authoredOn); 1498 case 693933948: /*requester*/ return new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The device, individual, or organization who asks for the information to be shared.", 0, 1, requester); 1499 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService|Endpoint)", "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 0, java.lang.Integer.MAX_VALUE, recipient); 1500 case 1255338813: /*informationProvider*/ return new Property("informationProvider", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService|Endpoint)", "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 0, java.lang.Integer.MAX_VALUE, informationProvider); 1501 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Any)", "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason); 1502 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1503 default: return super.getNamedProperty(_hash, _name, _checkValid); 1504 } 1505 1506 } 1507 1508 @Override 1509 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1510 switch (hash) { 1511 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1512 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1513 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 1514 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 1515 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 1516 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 1517 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 1518 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1519 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1520 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 1521 case -1078030475: /*medium*/ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 1522 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1523 case 92611469: /*about*/ return this.about == null ? new Base[0] : this.about.toArray(new Base[this.about.size()]); // Reference 1524 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1525 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationRequestPayloadComponent 1526 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1527 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 1528 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 1529 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1530 case 1255338813: /*informationProvider*/ return this.informationProvider == null ? new Base[0] : this.informationProvider.toArray(new Base[this.informationProvider.size()]); // Reference 1531 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1532 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1533 default: return super.getProperty(hash, name, checkValid); 1534 } 1535 1536 } 1537 1538 @Override 1539 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1540 switch (hash) { 1541 case -1618432855: // identifier 1542 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1543 return value; 1544 case -332612366: // basedOn 1545 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1546 return value; 1547 case -430332865: // replaces 1548 this.getReplaces().add(TypeConvertor.castToReference(value)); // Reference 1549 return value; 1550 case -445338488: // groupIdentifier 1551 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1552 return value; 1553 case -892481550: // status 1554 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1555 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1556 return value; 1557 case 2051346646: // statusReason 1558 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1559 return value; 1560 case -1183762788: // intent 1561 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1562 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 1563 return value; 1564 case 50511102: // category 1565 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1566 return value; 1567 case -1165461084: // priority 1568 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1569 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1570 return value; 1571 case -1788508167: // doNotPerform 1572 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 1573 return value; 1574 case -1078030475: // medium 1575 this.getMedium().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1576 return value; 1577 case -1867885268: // subject 1578 this.subject = TypeConvertor.castToReference(value); // Reference 1579 return value; 1580 case 92611469: // about 1581 this.getAbout().add(TypeConvertor.castToReference(value)); // Reference 1582 return value; 1583 case 1524132147: // encounter 1584 this.encounter = TypeConvertor.castToReference(value); // Reference 1585 return value; 1586 case -786701938: // payload 1587 this.getPayload().add((CommunicationRequestPayloadComponent) value); // CommunicationRequestPayloadComponent 1588 return value; 1589 case 1687874001: // occurrence 1590 this.occurrence = TypeConvertor.castToType(value); // DataType 1591 return value; 1592 case -1500852503: // authoredOn 1593 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 1594 return value; 1595 case 693933948: // requester 1596 this.requester = TypeConvertor.castToReference(value); // Reference 1597 return value; 1598 case 820081177: // recipient 1599 this.getRecipient().add(TypeConvertor.castToReference(value)); // Reference 1600 return value; 1601 case 1255338813: // informationProvider 1602 this.getInformationProvider().add(TypeConvertor.castToReference(value)); // Reference 1603 return value; 1604 case -934964668: // reason 1605 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1606 return value; 1607 case 3387378: // note 1608 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1609 return value; 1610 default: return super.setProperty(hash, name, value); 1611 } 1612 1613 } 1614 1615 @Override 1616 public Base setProperty(String name, Base value) throws FHIRException { 1617 if (name.equals("identifier")) { 1618 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1619 } else if (name.equals("basedOn")) { 1620 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1621 } else if (name.equals("replaces")) { 1622 this.getReplaces().add(TypeConvertor.castToReference(value)); 1623 } else if (name.equals("groupIdentifier")) { 1624 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1625 } else if (name.equals("status")) { 1626 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1627 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1628 } else if (name.equals("statusReason")) { 1629 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1630 } else if (name.equals("intent")) { 1631 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1632 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 1633 } else if (name.equals("category")) { 1634 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1635 } else if (name.equals("priority")) { 1636 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1637 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1638 } else if (name.equals("doNotPerform")) { 1639 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 1640 } else if (name.equals("medium")) { 1641 this.getMedium().add(TypeConvertor.castToCodeableConcept(value)); 1642 } else if (name.equals("subject")) { 1643 this.subject = TypeConvertor.castToReference(value); // Reference 1644 } else if (name.equals("about")) { 1645 this.getAbout().add(TypeConvertor.castToReference(value)); 1646 } else if (name.equals("encounter")) { 1647 this.encounter = TypeConvertor.castToReference(value); // Reference 1648 } else if (name.equals("payload")) { 1649 this.getPayload().add((CommunicationRequestPayloadComponent) value); 1650 } else if (name.equals("occurrence[x]")) { 1651 this.occurrence = TypeConvertor.castToType(value); // DataType 1652 } else if (name.equals("authoredOn")) { 1653 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 1654 } else if (name.equals("requester")) { 1655 this.requester = TypeConvertor.castToReference(value); // Reference 1656 } else if (name.equals("recipient")) { 1657 this.getRecipient().add(TypeConvertor.castToReference(value)); 1658 } else if (name.equals("informationProvider")) { 1659 this.getInformationProvider().add(TypeConvertor.castToReference(value)); 1660 } else if (name.equals("reason")) { 1661 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1662 } else if (name.equals("note")) { 1663 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1664 } else 1665 return super.setProperty(name, value); 1666 return value; 1667 } 1668 1669 @Override 1670 public void removeChild(String name, Base value) throws FHIRException { 1671 if (name.equals("identifier")) { 1672 this.getIdentifier().remove(value); 1673 } else if (name.equals("basedOn")) { 1674 this.getBasedOn().remove(value); 1675 } else if (name.equals("replaces")) { 1676 this.getReplaces().remove(value); 1677 } else if (name.equals("groupIdentifier")) { 1678 this.groupIdentifier = null; 1679 } else if (name.equals("status")) { 1680 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1681 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1682 } else if (name.equals("statusReason")) { 1683 this.statusReason = null; 1684 } else if (name.equals("intent")) { 1685 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1686 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 1687 } else if (name.equals("category")) { 1688 this.getCategory().remove(value); 1689 } else if (name.equals("priority")) { 1690 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1691 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1692 } else if (name.equals("doNotPerform")) { 1693 this.doNotPerform = null; 1694 } else if (name.equals("medium")) { 1695 this.getMedium().remove(value); 1696 } else if (name.equals("subject")) { 1697 this.subject = null; 1698 } else if (name.equals("about")) { 1699 this.getAbout().remove(value); 1700 } else if (name.equals("encounter")) { 1701 this.encounter = null; 1702 } else if (name.equals("payload")) { 1703 this.getPayload().remove((CommunicationRequestPayloadComponent) value); 1704 } else if (name.equals("occurrence[x]")) { 1705 this.occurrence = null; 1706 } else if (name.equals("authoredOn")) { 1707 this.authoredOn = null; 1708 } else if (name.equals("requester")) { 1709 this.requester = null; 1710 } else if (name.equals("recipient")) { 1711 this.getRecipient().remove(value); 1712 } else if (name.equals("informationProvider")) { 1713 this.getInformationProvider().remove(value); 1714 } else if (name.equals("reason")) { 1715 this.getReason().remove(value); 1716 } else if (name.equals("note")) { 1717 this.getNote().remove(value); 1718 } else 1719 super.removeChild(name, value); 1720 1721 } 1722 1723 @Override 1724 public Base makeProperty(int hash, String name) throws FHIRException { 1725 switch (hash) { 1726 case -1618432855: return addIdentifier(); 1727 case -332612366: return addBasedOn(); 1728 case -430332865: return addReplaces(); 1729 case -445338488: return getGroupIdentifier(); 1730 case -892481550: return getStatusElement(); 1731 case 2051346646: return getStatusReason(); 1732 case -1183762788: return getIntentElement(); 1733 case 50511102: return addCategory(); 1734 case -1165461084: return getPriorityElement(); 1735 case -1788508167: return getDoNotPerformElement(); 1736 case -1078030475: return addMedium(); 1737 case -1867885268: return getSubject(); 1738 case 92611469: return addAbout(); 1739 case 1524132147: return getEncounter(); 1740 case -786701938: return addPayload(); 1741 case -2022646513: return getOccurrence(); 1742 case 1687874001: return getOccurrence(); 1743 case -1500852503: return getAuthoredOnElement(); 1744 case 693933948: return getRequester(); 1745 case 820081177: return addRecipient(); 1746 case 1255338813: return addInformationProvider(); 1747 case -934964668: return addReason(); 1748 case 3387378: return addNote(); 1749 default: return super.makeProperty(hash, name); 1750 } 1751 1752 } 1753 1754 @Override 1755 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1756 switch (hash) { 1757 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1758 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1759 case -430332865: /*replaces*/ return new String[] {"Reference"}; 1760 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 1761 case -892481550: /*status*/ return new String[] {"code"}; 1762 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 1763 case -1183762788: /*intent*/ return new String[] {"code"}; 1764 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1765 case -1165461084: /*priority*/ return new String[] {"code"}; 1766 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 1767 case -1078030475: /*medium*/ return new String[] {"CodeableConcept"}; 1768 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1769 case 92611469: /*about*/ return new String[] {"Reference"}; 1770 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1771 case -786701938: /*payload*/ return new String[] {}; 1772 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 1773 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 1774 case 693933948: /*requester*/ return new String[] {"Reference"}; 1775 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1776 case 1255338813: /*informationProvider*/ return new String[] {"Reference"}; 1777 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1778 case 3387378: /*note*/ return new String[] {"Annotation"}; 1779 default: return super.getTypesForProperty(hash, name); 1780 } 1781 1782 } 1783 1784 @Override 1785 public Base addChild(String name) throws FHIRException { 1786 if (name.equals("identifier")) { 1787 return addIdentifier(); 1788 } 1789 else if (name.equals("basedOn")) { 1790 return addBasedOn(); 1791 } 1792 else if (name.equals("replaces")) { 1793 return addReplaces(); 1794 } 1795 else if (name.equals("groupIdentifier")) { 1796 this.groupIdentifier = new Identifier(); 1797 return this.groupIdentifier; 1798 } 1799 else if (name.equals("status")) { 1800 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.status"); 1801 } 1802 else if (name.equals("statusReason")) { 1803 this.statusReason = new CodeableConcept(); 1804 return this.statusReason; 1805 } 1806 else if (name.equals("intent")) { 1807 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.intent"); 1808 } 1809 else if (name.equals("category")) { 1810 return addCategory(); 1811 } 1812 else if (name.equals("priority")) { 1813 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.priority"); 1814 } 1815 else if (name.equals("doNotPerform")) { 1816 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.doNotPerform"); 1817 } 1818 else if (name.equals("medium")) { 1819 return addMedium(); 1820 } 1821 else if (name.equals("subject")) { 1822 this.subject = new Reference(); 1823 return this.subject; 1824 } 1825 else if (name.equals("about")) { 1826 return addAbout(); 1827 } 1828 else if (name.equals("encounter")) { 1829 this.encounter = new Reference(); 1830 return this.encounter; 1831 } 1832 else if (name.equals("payload")) { 1833 return addPayload(); 1834 } 1835 else if (name.equals("occurrenceDateTime")) { 1836 this.occurrence = new DateTimeType(); 1837 return this.occurrence; 1838 } 1839 else if (name.equals("occurrencePeriod")) { 1840 this.occurrence = new Period(); 1841 return this.occurrence; 1842 } 1843 else if (name.equals("authoredOn")) { 1844 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.authoredOn"); 1845 } 1846 else if (name.equals("requester")) { 1847 this.requester = new Reference(); 1848 return this.requester; 1849 } 1850 else if (name.equals("recipient")) { 1851 return addRecipient(); 1852 } 1853 else if (name.equals("informationProvider")) { 1854 return addInformationProvider(); 1855 } 1856 else if (name.equals("reason")) { 1857 return addReason(); 1858 } 1859 else if (name.equals("note")) { 1860 return addNote(); 1861 } 1862 else 1863 return super.addChild(name); 1864 } 1865 1866 public String fhirType() { 1867 return "CommunicationRequest"; 1868 1869 } 1870 1871 public CommunicationRequest copy() { 1872 CommunicationRequest dst = new CommunicationRequest(); 1873 copyValues(dst); 1874 return dst; 1875 } 1876 1877 public void copyValues(CommunicationRequest dst) { 1878 super.copyValues(dst); 1879 if (identifier != null) { 1880 dst.identifier = new ArrayList<Identifier>(); 1881 for (Identifier i : identifier) 1882 dst.identifier.add(i.copy()); 1883 }; 1884 if (basedOn != null) { 1885 dst.basedOn = new ArrayList<Reference>(); 1886 for (Reference i : basedOn) 1887 dst.basedOn.add(i.copy()); 1888 }; 1889 if (replaces != null) { 1890 dst.replaces = new ArrayList<Reference>(); 1891 for (Reference i : replaces) 1892 dst.replaces.add(i.copy()); 1893 }; 1894 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 1895 dst.status = status == null ? null : status.copy(); 1896 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1897 dst.intent = intent == null ? null : intent.copy(); 1898 if (category != null) { 1899 dst.category = new ArrayList<CodeableConcept>(); 1900 for (CodeableConcept i : category) 1901 dst.category.add(i.copy()); 1902 }; 1903 dst.priority = priority == null ? null : priority.copy(); 1904 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 1905 if (medium != null) { 1906 dst.medium = new ArrayList<CodeableConcept>(); 1907 for (CodeableConcept i : medium) 1908 dst.medium.add(i.copy()); 1909 }; 1910 dst.subject = subject == null ? null : subject.copy(); 1911 if (about != null) { 1912 dst.about = new ArrayList<Reference>(); 1913 for (Reference i : about) 1914 dst.about.add(i.copy()); 1915 }; 1916 dst.encounter = encounter == null ? null : encounter.copy(); 1917 if (payload != null) { 1918 dst.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1919 for (CommunicationRequestPayloadComponent i : payload) 1920 dst.payload.add(i.copy()); 1921 }; 1922 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1923 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 1924 dst.requester = requester == null ? null : requester.copy(); 1925 if (recipient != null) { 1926 dst.recipient = new ArrayList<Reference>(); 1927 for (Reference i : recipient) 1928 dst.recipient.add(i.copy()); 1929 }; 1930 if (informationProvider != null) { 1931 dst.informationProvider = new ArrayList<Reference>(); 1932 for (Reference i : informationProvider) 1933 dst.informationProvider.add(i.copy()); 1934 }; 1935 if (reason != null) { 1936 dst.reason = new ArrayList<CodeableReference>(); 1937 for (CodeableReference i : reason) 1938 dst.reason.add(i.copy()); 1939 }; 1940 if (note != null) { 1941 dst.note = new ArrayList<Annotation>(); 1942 for (Annotation i : note) 1943 dst.note.add(i.copy()); 1944 }; 1945 } 1946 1947 protected CommunicationRequest typedCopy() { 1948 return copy(); 1949 } 1950 1951 @Override 1952 public boolean equalsDeep(Base other_) { 1953 if (!super.equalsDeep(other_)) 1954 return false; 1955 if (!(other_ instanceof CommunicationRequest)) 1956 return false; 1957 CommunicationRequest o = (CommunicationRequest) other_; 1958 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(replaces, o.replaces, true) 1959 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(status, o.status, true) 1960 && compareDeep(statusReason, o.statusReason, true) && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) 1961 && compareDeep(priority, o.priority, true) && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(medium, o.medium, true) 1962 && compareDeep(subject, o.subject, true) && compareDeep(about, o.about, true) && compareDeep(encounter, o.encounter, true) 1963 && compareDeep(payload, o.payload, true) && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) 1964 && compareDeep(requester, o.requester, true) && compareDeep(recipient, o.recipient, true) && compareDeep(informationProvider, o.informationProvider, true) 1965 && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true); 1966 } 1967 1968 @Override 1969 public boolean equalsShallow(Base other_) { 1970 if (!super.equalsShallow(other_)) 1971 return false; 1972 if (!(other_ instanceof CommunicationRequest)) 1973 return false; 1974 CommunicationRequest o = (CommunicationRequest) other_; 1975 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 1976 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true) 1977 ; 1978 } 1979 1980 public boolean isEmpty() { 1981 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, replaces 1982 , groupIdentifier, status, statusReason, intent, category, priority, doNotPerform 1983 , medium, subject, about, encounter, payload, occurrence, authoredOn, requester 1984 , recipient, informationProvider, reason, note); 1985 } 1986 1987 @Override 1988 public ResourceType getResourceType() { 1989 return ResourceType.CommunicationRequest; 1990 } 1991 1992 /** 1993 * Search parameter: <b>authored</b> 1994 * <p> 1995 * Description: <b>When request transitioned to being actionable</b><br> 1996 * Type: <b>date</b><br> 1997 * Path: <b>CommunicationRequest.authoredOn</b><br> 1998 * </p> 1999 */ 2000 @SearchParamDefinition(name="authored", path="CommunicationRequest.authoredOn", description="When request transitioned to being actionable", type="date" ) 2001 public static final String SP_AUTHORED = "authored"; 2002 /** 2003 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2004 * <p> 2005 * Description: <b>When request transitioned to being actionable</b><br> 2006 * Type: <b>date</b><br> 2007 * Path: <b>CommunicationRequest.authoredOn</b><br> 2008 * </p> 2009 */ 2010 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 2011 2012 /** 2013 * Search parameter: <b>based-on</b> 2014 * <p> 2015 * Description: <b>Fulfills plan or proposal</b><br> 2016 * Type: <b>reference</b><br> 2017 * Path: <b>CommunicationRequest.basedOn</b><br> 2018 * </p> 2019 */ 2020 @SearchParamDefinition(name="based-on", path="CommunicationRequest.basedOn", description="Fulfills plan or proposal", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2021 public static final String SP_BASED_ON = "based-on"; 2022 /** 2023 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2024 * <p> 2025 * Description: <b>Fulfills plan or proposal</b><br> 2026 * Type: <b>reference</b><br> 2027 * Path: <b>CommunicationRequest.basedOn</b><br> 2028 * </p> 2029 */ 2030 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2031 2032/** 2033 * Constant for fluent queries to be used to add include statements. Specifies 2034 * the path value of "<b>CommunicationRequest:based-on</b>". 2035 */ 2036 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("CommunicationRequest:based-on").toLocked(); 2037 2038 /** 2039 * Search parameter: <b>category</b> 2040 * <p> 2041 * Description: <b>Message category</b><br> 2042 * Type: <b>token</b><br> 2043 * Path: <b>CommunicationRequest.category</b><br> 2044 * </p> 2045 */ 2046 @SearchParamDefinition(name="category", path="CommunicationRequest.category", description="Message category", type="token" ) 2047 public static final String SP_CATEGORY = "category"; 2048 /** 2049 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2050 * <p> 2051 * Description: <b>Message category</b><br> 2052 * Type: <b>token</b><br> 2053 * Path: <b>CommunicationRequest.category</b><br> 2054 * </p> 2055 */ 2056 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2057 2058 /** 2059 * Search parameter: <b>group-identifier</b> 2060 * <p> 2061 * Description: <b>Composite request this is part of</b><br> 2062 * Type: <b>token</b><br> 2063 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 2064 * </p> 2065 */ 2066 @SearchParamDefinition(name="group-identifier", path="CommunicationRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 2067 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 2068 /** 2069 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 2070 * <p> 2071 * Description: <b>Composite request this is part of</b><br> 2072 * Type: <b>token</b><br> 2073 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 2074 * </p> 2075 */ 2076 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 2077 2078 /** 2079 * Search parameter: <b>information-provider</b> 2080 * <p> 2081 * Description: <b>Who should share the information</b><br> 2082 * Type: <b>reference</b><br> 2083 * Path: <b>CommunicationRequest.informationProvider</b><br> 2084 * </p> 2085 */ 2086 @SearchParamDefinition(name="information-provider", path="CommunicationRequest.informationProvider", description="Who should share the information", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Endpoint.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2087 public static final String SP_INFORMATION_PROVIDER = "information-provider"; 2088 /** 2089 * <b>Fluent Client</b> search parameter constant for <b>information-provider</b> 2090 * <p> 2091 * Description: <b>Who should share the information</b><br> 2092 * Type: <b>reference</b><br> 2093 * Path: <b>CommunicationRequest.informationProvider</b><br> 2094 * </p> 2095 */ 2096 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INFORMATION_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INFORMATION_PROVIDER); 2097 2098/** 2099 * Constant for fluent queries to be used to add include statements. Specifies 2100 * the path value of "<b>CommunicationRequest:information-provider</b>". 2101 */ 2102 public static final ca.uhn.fhir.model.api.Include INCLUDE_INFORMATION_PROVIDER = new ca.uhn.fhir.model.api.Include("CommunicationRequest:information-provider").toLocked(); 2103 2104 /** 2105 * Search parameter: <b>medium</b> 2106 * <p> 2107 * Description: <b>A channel of communication</b><br> 2108 * Type: <b>token</b><br> 2109 * Path: <b>CommunicationRequest.medium</b><br> 2110 * </p> 2111 */ 2112 @SearchParamDefinition(name="medium", path="CommunicationRequest.medium", description="A channel of communication", type="token" ) 2113 public static final String SP_MEDIUM = "medium"; 2114 /** 2115 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 2116 * <p> 2117 * Description: <b>A channel of communication</b><br> 2118 * Type: <b>token</b><br> 2119 * Path: <b>CommunicationRequest.medium</b><br> 2120 * </p> 2121 */ 2122 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MEDIUM); 2123 2124 /** 2125 * Search parameter: <b>occurrence</b> 2126 * <p> 2127 * Description: <b>When scheduled</b><br> 2128 * Type: <b>date</b><br> 2129 * Path: <b>CommunicationRequest.occurrence.ofType(dateTime) | CommunicationRequest.occurrence.ofType(Period)</b><br> 2130 * </p> 2131 */ 2132 @SearchParamDefinition(name="occurrence", path="CommunicationRequest.occurrence.ofType(dateTime) | CommunicationRequest.occurrence.ofType(Period)", description="When scheduled", type="date" ) 2133 public static final String SP_OCCURRENCE = "occurrence"; 2134 /** 2135 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 2136 * <p> 2137 * Description: <b>When scheduled</b><br> 2138 * Type: <b>date</b><br> 2139 * Path: <b>CommunicationRequest.occurrence.ofType(dateTime) | CommunicationRequest.occurrence.ofType(Period)</b><br> 2140 * </p> 2141 */ 2142 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 2143 2144 /** 2145 * Search parameter: <b>priority</b> 2146 * <p> 2147 * Description: <b>routine | urgent | asap | stat</b><br> 2148 * Type: <b>token</b><br> 2149 * Path: <b>CommunicationRequest.priority</b><br> 2150 * </p> 2151 */ 2152 @SearchParamDefinition(name="priority", path="CommunicationRequest.priority", description="routine | urgent | asap | stat", type="token" ) 2153 public static final String SP_PRIORITY = "priority"; 2154 /** 2155 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 2156 * <p> 2157 * Description: <b>routine | urgent | asap | stat</b><br> 2158 * Type: <b>token</b><br> 2159 * Path: <b>CommunicationRequest.priority</b><br> 2160 * </p> 2161 */ 2162 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 2163 2164 /** 2165 * Search parameter: <b>recipient</b> 2166 * <p> 2167 * Description: <b>Who to share the information with</b><br> 2168 * Type: <b>reference</b><br> 2169 * Path: <b>CommunicationRequest.recipient</b><br> 2170 * </p> 2171 */ 2172 @SearchParamDefinition(name="recipient", path="CommunicationRequest.recipient", description="Who to share the information with", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson"), @ca.uhn.fhir.model.api.annotation.Compartment(name="EXAMPLE") }, target={CareTeam.class, Device.class, Endpoint.class, Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2173 public static final String SP_RECIPIENT = "recipient"; 2174 /** 2175 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2176 * <p> 2177 * Description: <b>Who to share the information with</b><br> 2178 * Type: <b>reference</b><br> 2179 * Path: <b>CommunicationRequest.recipient</b><br> 2180 * </p> 2181 */ 2182 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2183 2184/** 2185 * Constant for fluent queries to be used to add include statements. Specifies 2186 * the path value of "<b>CommunicationRequest:recipient</b>". 2187 */ 2188 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:recipient").toLocked(); 2189 2190 /** 2191 * Search parameter: <b>replaces</b> 2192 * <p> 2193 * Description: <b>Request(s) replaced by this request</b><br> 2194 * Type: <b>reference</b><br> 2195 * Path: <b>CommunicationRequest.replaces</b><br> 2196 * </p> 2197 */ 2198 @SearchParamDefinition(name="replaces", path="CommunicationRequest.replaces", description="Request(s) replaced by this request", type="reference", target={CommunicationRequest.class } ) 2199 public static final String SP_REPLACES = "replaces"; 2200 /** 2201 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 2202 * <p> 2203 * Description: <b>Request(s) replaced by this request</b><br> 2204 * Type: <b>reference</b><br> 2205 * Path: <b>CommunicationRequest.replaces</b><br> 2206 * </p> 2207 */ 2208 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 2209 2210/** 2211 * Constant for fluent queries to be used to add include statements. Specifies 2212 * the path value of "<b>CommunicationRequest:replaces</b>". 2213 */ 2214 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("CommunicationRequest:replaces").toLocked(); 2215 2216 /** 2217 * Search parameter: <b>requester</b> 2218 * <p> 2219 * Description: <b>Who asks for the information to be shared</b><br> 2220 * Type: <b>reference</b><br> 2221 * Path: <b>CommunicationRequest.requester</b><br> 2222 * </p> 2223 */ 2224 @SearchParamDefinition(name="requester", path="CommunicationRequest.requester", description="Who asks for the information to be shared", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2225 public static final String SP_REQUESTER = "requester"; 2226 /** 2227 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2228 * <p> 2229 * Description: <b>Who asks for the information to be shared</b><br> 2230 * Type: <b>reference</b><br> 2231 * Path: <b>CommunicationRequest.requester</b><br> 2232 * </p> 2233 */ 2234 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2235 2236/** 2237 * Constant for fluent queries to be used to add include statements. Specifies 2238 * the path value of "<b>CommunicationRequest:requester</b>". 2239 */ 2240 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("CommunicationRequest:requester").toLocked(); 2241 2242 /** 2243 * Search parameter: <b>status</b> 2244 * <p> 2245 * Description: <b>draft | active | on-hold | revoked | completed | entered-in-error | unknown</b><br> 2246 * Type: <b>token</b><br> 2247 * Path: <b>CommunicationRequest.status</b><br> 2248 * </p> 2249 */ 2250 @SearchParamDefinition(name="status", path="CommunicationRequest.status", description="draft | active | on-hold | revoked | completed | entered-in-error | unknown", type="token" ) 2251 public static final String SP_STATUS = "status"; 2252 /** 2253 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2254 * <p> 2255 * Description: <b>draft | active | on-hold | revoked | completed | entered-in-error | unknown</b><br> 2256 * Type: <b>token</b><br> 2257 * Path: <b>CommunicationRequest.status</b><br> 2258 * </p> 2259 */ 2260 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2261 2262 /** 2263 * Search parameter: <b>subject</b> 2264 * <p> 2265 * Description: <b>Focus of message</b><br> 2266 * Type: <b>reference</b><br> 2267 * Path: <b>CommunicationRequest.subject</b><br> 2268 * </p> 2269 */ 2270 @SearchParamDefinition(name="subject", path="CommunicationRequest.subject", description="Focus of message", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2271 public static final String SP_SUBJECT = "subject"; 2272 /** 2273 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2274 * <p> 2275 * Description: <b>Focus of message</b><br> 2276 * Type: <b>reference</b><br> 2277 * Path: <b>CommunicationRequest.subject</b><br> 2278 * </p> 2279 */ 2280 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2281 2282/** 2283 * Constant for fluent queries to be used to add include statements. Specifies 2284 * the path value of "<b>CommunicationRequest:subject</b>". 2285 */ 2286 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:subject").toLocked(); 2287 2288 /** 2289 * Search parameter: <b>encounter</b> 2290 * <p> 2291 * Description: <b>Multiple Resources: 2292 2293* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2294* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2295* [ChargeItem](chargeitem.html): Encounter associated with event 2296* [Claim](claim.html): Encounters associated with a billed line item 2297* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2298* [Communication](communication.html): The Encounter during which this Communication was created 2299* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2300* [Composition](composition.html): Context of the Composition 2301* [Condition](condition.html): The Encounter during which this Condition was created 2302* [DeviceRequest](devicerequest.html): Encounter during which request was created 2303* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2304* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2305* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2306* [Flag](flag.html): Alert relevant during encounter 2307* [ImagingStudy](imagingstudy.html): The context of the study 2308* [List](list.html): Context in which list created 2309* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2310* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2311* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2312* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2313* [Observation](observation.html): Encounter related to the observation 2314* [Procedure](procedure.html): The Encounter during which this Procedure was created 2315* [Provenance](provenance.html): Encounter related to the Provenance 2316* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2317* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2318* [RiskAssessment](riskassessment.html): Where was assessment performed? 2319* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2320* [Task](task.html): Search by encounter 2321* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2322</b><br> 2323 * Type: <b>reference</b><br> 2324 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2325 * </p> 2326 */ 2327 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2328 public static final String SP_ENCOUNTER = "encounter"; 2329 /** 2330 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2331 * <p> 2332 * Description: <b>Multiple Resources: 2333 2334* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2335* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2336* [ChargeItem](chargeitem.html): Encounter associated with event 2337* [Claim](claim.html): Encounters associated with a billed line item 2338* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2339* [Communication](communication.html): The Encounter during which this Communication was created 2340* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2341* [Composition](composition.html): Context of the Composition 2342* [Condition](condition.html): The Encounter during which this Condition was created 2343* [DeviceRequest](devicerequest.html): Encounter during which request was created 2344* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2345* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2346* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2347* [Flag](flag.html): Alert relevant during encounter 2348* [ImagingStudy](imagingstudy.html): The context of the study 2349* [List](list.html): Context in which list created 2350* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2351* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2352* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2353* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2354* [Observation](observation.html): Encounter related to the observation 2355* [Procedure](procedure.html): The Encounter during which this Procedure was created 2356* [Provenance](provenance.html): Encounter related to the Provenance 2357* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2358* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2359* [RiskAssessment](riskassessment.html): Where was assessment performed? 2360* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2361* [Task](task.html): Search by encounter 2362* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2363</b><br> 2364 * Type: <b>reference</b><br> 2365 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2366 * </p> 2367 */ 2368 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2369 2370/** 2371 * Constant for fluent queries to be used to add include statements. Specifies 2372 * the path value of "<b>CommunicationRequest:encounter</b>". 2373 */ 2374 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("CommunicationRequest:encounter").toLocked(); 2375 2376 /** 2377 * Search parameter: <b>identifier</b> 2378 * <p> 2379 * Description: <b>Multiple Resources: 2380 2381* [Account](account.html): Account number 2382* [AdverseEvent](adverseevent.html): Business identifier for the event 2383* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2384* [Appointment](appointment.html): An Identifier of the Appointment 2385* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2386* [Basic](basic.html): Business identifier 2387* [BodyStructure](bodystructure.html): Bodystructure identifier 2388* [CarePlan](careplan.html): External Ids for this plan 2389* [CareTeam](careteam.html): External Ids for this team 2390* [ChargeItem](chargeitem.html): Business Identifier for item 2391* [Claim](claim.html): The primary identifier of the financial resource 2392* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2393* [ClinicalImpression](clinicalimpression.html): Business identifier 2394* [Communication](communication.html): Unique identifier 2395* [CommunicationRequest](communicationrequest.html): Unique identifier 2396* [Composition](composition.html): Version-independent identifier for the Composition 2397* [Condition](condition.html): A unique identifier of the condition record 2398* [Consent](consent.html): Identifier for this record (external references) 2399* [Contract](contract.html): The identity of the contract 2400* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2401* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2402* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2403* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2404* [DeviceRequest](devicerequest.html): Business identifier for request/order 2405* [DeviceUsage](deviceusage.html): Search by identifier 2406* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2407* [DocumentReference](documentreference.html): Identifier of the attachment binary 2408* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2409* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2410* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2411* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2412* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2413* [Flag](flag.html): Business identifier 2414* [Goal](goal.html): External Ids for this goal 2415* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2416* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2417* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2418* [Immunization](immunization.html): Business identifier 2419* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2420* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2421* [Invoice](invoice.html): Business Identifier for item 2422* [List](list.html): Business identifier 2423* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2424* [Medication](medication.html): Returns medications with this external identifier 2425* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2426* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2427* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2428* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2429* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2430* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2431* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2432* [Observation](observation.html): The unique id for a particular observation 2433* [Person](person.html): A person Identifier 2434* [Procedure](procedure.html): A unique identifier for a procedure 2435* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2436* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2437* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2438* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2439* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2440* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2441* [Specimen](specimen.html): The unique identifier associated with the specimen 2442* [SupplyDelivery](supplydelivery.html): External identifier 2443* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2444* [Task](task.html): Search for a task instance by its business identifier 2445* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2446</b><br> 2447 * Type: <b>token</b><br> 2448 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2449 * </p> 2450 */ 2451 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2452 public static final String SP_IDENTIFIER = "identifier"; 2453 /** 2454 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2455 * <p> 2456 * Description: <b>Multiple Resources: 2457 2458* [Account](account.html): Account number 2459* [AdverseEvent](adverseevent.html): Business identifier for the event 2460* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2461* [Appointment](appointment.html): An Identifier of the Appointment 2462* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2463* [Basic](basic.html): Business identifier 2464* [BodyStructure](bodystructure.html): Bodystructure identifier 2465* [CarePlan](careplan.html): External Ids for this plan 2466* [CareTeam](careteam.html): External Ids for this team 2467* [ChargeItem](chargeitem.html): Business Identifier for item 2468* [Claim](claim.html): The primary identifier of the financial resource 2469* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2470* [ClinicalImpression](clinicalimpression.html): Business identifier 2471* [Communication](communication.html): Unique identifier 2472* [CommunicationRequest](communicationrequest.html): Unique identifier 2473* [Composition](composition.html): Version-independent identifier for the Composition 2474* [Condition](condition.html): A unique identifier of the condition record 2475* [Consent](consent.html): Identifier for this record (external references) 2476* [Contract](contract.html): The identity of the contract 2477* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2478* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2479* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2480* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2481* [DeviceRequest](devicerequest.html): Business identifier for request/order 2482* [DeviceUsage](deviceusage.html): Search by identifier 2483* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2484* [DocumentReference](documentreference.html): Identifier of the attachment binary 2485* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2486* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2487* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2488* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2489* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2490* [Flag](flag.html): Business identifier 2491* [Goal](goal.html): External Ids for this goal 2492* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2493* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2494* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2495* [Immunization](immunization.html): Business identifier 2496* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2497* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2498* [Invoice](invoice.html): Business Identifier for item 2499* [List](list.html): Business identifier 2500* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2501* [Medication](medication.html): Returns medications with this external identifier 2502* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2503* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2504* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2505* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2506* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2507* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2508* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2509* [Observation](observation.html): The unique id for a particular observation 2510* [Person](person.html): A person Identifier 2511* [Procedure](procedure.html): A unique identifier for a procedure 2512* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2513* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2514* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2515* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2516* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2517* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2518* [Specimen](specimen.html): The unique identifier associated with the specimen 2519* [SupplyDelivery](supplydelivery.html): External identifier 2520* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2521* [Task](task.html): Search for a task instance by its business identifier 2522* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2523</b><br> 2524 * Type: <b>token</b><br> 2525 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2526 * </p> 2527 */ 2528 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2529 2530 /** 2531 * Search parameter: <b>patient</b> 2532 * <p> 2533 * Description: <b>Multiple Resources: 2534 2535* [Account](account.html): The entity that caused the expenses 2536* [AdverseEvent](adverseevent.html): Subject impacted by event 2537* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2538* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2539* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2540* [AuditEvent](auditevent.html): Where the activity involved patient data 2541* [Basic](basic.html): Identifies the focus of this resource 2542* [BodyStructure](bodystructure.html): Who this is about 2543* [CarePlan](careplan.html): Who the care plan is for 2544* [CareTeam](careteam.html): Who care team is for 2545* [ChargeItem](chargeitem.html): Individual service was done for/to 2546* [Claim](claim.html): Patient receiving the products or services 2547* [ClaimResponse](claimresponse.html): The subject of care 2548* [ClinicalImpression](clinicalimpression.html): Patient assessed 2549* [Communication](communication.html): Focus of message 2550* [CommunicationRequest](communicationrequest.html): Focus of message 2551* [Composition](composition.html): Who and/or what the composition is about 2552* [Condition](condition.html): Who has the condition? 2553* [Consent](consent.html): Who the consent applies to 2554* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2555* [Coverage](coverage.html): Retrieve coverages for a patient 2556* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2557* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2558* [DetectedIssue](detectedissue.html): Associated patient 2559* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2560* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2561* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2562* [DocumentReference](documentreference.html): Who/what is the subject of the document 2563* [Encounter](encounter.html): The patient present at the encounter 2564* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2565* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2566* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2567* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2568* [Flag](flag.html): The identity of a subject to list flags for 2569* [Goal](goal.html): Who this goal is intended for 2570* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2571* [ImagingSelection](imagingselection.html): Who the study is about 2572* [ImagingStudy](imagingstudy.html): Who the study is about 2573* [Immunization](immunization.html): The patient for the vaccination record 2574* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2575* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2576* [Invoice](invoice.html): Recipient(s) of goods and services 2577* [List](list.html): If all resources have the same subject 2578* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2579* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2580* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2581* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2582* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2583* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2584* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2585* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2586* [Observation](observation.html): The subject that the observation is about (if patient) 2587* [Person](person.html): The Person links to this Patient 2588* [Procedure](procedure.html): Search by subject - a patient 2589* [Provenance](provenance.html): Where the activity involved patient data 2590* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2591* [RelatedPerson](relatedperson.html): The patient this related person is related to 2592* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2593* [ResearchSubject](researchsubject.html): Who or what is part of study 2594* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2595* [ServiceRequest](servicerequest.html): Search by subject - a patient 2596* [Specimen](specimen.html): The patient the specimen comes from 2597* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2598* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2599* [Task](task.html): Search by patient 2600* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2601</b><br> 2602 * Type: <b>reference</b><br> 2603 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2604 * </p> 2605 */ 2606 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2607 public static final String SP_PATIENT = "patient"; 2608 /** 2609 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2610 * <p> 2611 * Description: <b>Multiple Resources: 2612 2613* [Account](account.html): The entity that caused the expenses 2614* [AdverseEvent](adverseevent.html): Subject impacted by event 2615* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2616* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2617* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2618* [AuditEvent](auditevent.html): Where the activity involved patient data 2619* [Basic](basic.html): Identifies the focus of this resource 2620* [BodyStructure](bodystructure.html): Who this is about 2621* [CarePlan](careplan.html): Who the care plan is for 2622* [CareTeam](careteam.html): Who care team is for 2623* [ChargeItem](chargeitem.html): Individual service was done for/to 2624* [Claim](claim.html): Patient receiving the products or services 2625* [ClaimResponse](claimresponse.html): The subject of care 2626* [ClinicalImpression](clinicalimpression.html): Patient assessed 2627* [Communication](communication.html): Focus of message 2628* [CommunicationRequest](communicationrequest.html): Focus of message 2629* [Composition](composition.html): Who and/or what the composition is about 2630* [Condition](condition.html): Who has the condition? 2631* [Consent](consent.html): Who the consent applies to 2632* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2633* [Coverage](coverage.html): Retrieve coverages for a patient 2634* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2635* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2636* [DetectedIssue](detectedissue.html): Associated patient 2637* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2638* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2639* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2640* [DocumentReference](documentreference.html): Who/what is the subject of the document 2641* [Encounter](encounter.html): The patient present at the encounter 2642* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2643* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2644* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2645* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2646* [Flag](flag.html): The identity of a subject to list flags for 2647* [Goal](goal.html): Who this goal is intended for 2648* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2649* [ImagingSelection](imagingselection.html): Who the study is about 2650* [ImagingStudy](imagingstudy.html): Who the study is about 2651* [Immunization](immunization.html): The patient for the vaccination record 2652* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2653* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2654* [Invoice](invoice.html): Recipient(s) of goods and services 2655* [List](list.html): If all resources have the same subject 2656* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2657* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2658* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2659* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2660* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2661* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2662* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2663* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2664* [Observation](observation.html): The subject that the observation is about (if patient) 2665* [Person](person.html): The Person links to this Patient 2666* [Procedure](procedure.html): Search by subject - a patient 2667* [Provenance](provenance.html): Where the activity involved patient data 2668* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2669* [RelatedPerson](relatedperson.html): The patient this related person is related to 2670* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2671* [ResearchSubject](researchsubject.html): Who or what is part of study 2672* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2673* [ServiceRequest](servicerequest.html): Search by subject - a patient 2674* [Specimen](specimen.html): The patient the specimen comes from 2675* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2676* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2677* [Task](task.html): Search by patient 2678* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2679</b><br> 2680 * Type: <b>reference</b><br> 2681 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2682 * </p> 2683 */ 2684 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2685 2686/** 2687 * Constant for fluent queries to be used to add include statements. Specifies 2688 * the path value of "<b>CommunicationRequest:patient</b>". 2689 */ 2690 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CommunicationRequest:patient").toLocked(); 2691 2692 2693} 2694