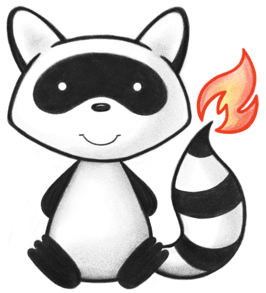
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.List; 035 036import org.apache.commons.lang3.NotImplementedException; 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.Utilities; 039 040/** 041 * See http://www.healthintersections.com.au/?p=1941 042 * 043 * @author Grahame 044 * 045 */ 046public class Comparison { 047 048 public class MatchProfile { 049 050 } 051 052 public static boolean matches(String c1, String c2, MatchProfile profile) { 053 if (Utilities.noString(c1) || Utilities.noString(c2)) 054 return false; 055 c1 = Utilities.normalize(c1); 056 c2 = Utilities.normalize(c2); 057 return c1.equals(c2); 058 } 059 060 public static <T extends Enum<?>> boolean matches(Enumeration<T> e1, Enumeration<T> e2, MatchProfile profile) { 061 if (e1 == null || e2 == null) 062 return false; 063 return e1.getValue().equals(e2.getValue()); 064 } 065 066 public static boolean matches(CodeableConcept c1, CodeableConcept c2, MatchProfile profile) throws FHIRException { 067 if (profile != null) 068 throw new NotImplementedException("Not Implemented Yet"); 069 070 if (c1.getCoding().isEmpty() && c2.getCoding().isEmpty()) { 071 return matches(c1.getText(), c2.getText(), null); 072 } else { 073 // in the absence of specific guidance, we just require that all codes match 074 boolean ok = true; 075 for (Coding c : c1.getCoding()) { 076 ok = ok && inList(c2.getCoding(), c, null); 077 } 078 for (Coding c : c2.getCoding()) { 079 ok = ok && inList(c1.getCoding(), c, null); 080 } 081 return ok; 082 } 083 } 084 085 public static void merge(CodeableConcept dst, CodeableConcept src) { 086 if (dst.getTextElement() == null && src.getTextElement() != null) 087 dst.setTextElement(src.getTextElement()); 088 } 089 090 091 public static boolean inList(List<Coding> list, Coding c, MatchProfile profile) { 092 for (Coding item : list) { 093 if (matches(item, c, profile)) 094 return true; 095 } 096 return false; 097 } 098 099 public static boolean matches(Coding c1, Coding c2, MatchProfile profile) { 100 if (profile != null) 101 throw new NotImplementedException("Not Implemented Yet"); 102 103 // in the absence of a profile, we ignore version 104 return matches(c1.getSystem(), c2.getSystem(), null) && matches(c1.getCode(), c2.getCode(), null); 105 } 106 107 public static boolean matches(Identifier i1, Identifier i2, MatchProfile profile) { 108 if (profile != null) 109 throw new NotImplementedException("Not Implemented Yet"); 110 111 // in the absence of a profile, we ignore version 112 return matches(i1.getSystem(), i2.getSystem(), null) && matches(i1.getValue(), i2.getValue(), null); 113 } 114 115 public static void merge(Identifier dst, Identifier src) { 116 if (dst.getUseElement() == null && src.getUseElement() != null) 117 dst.setUseElement(src.getUseElement()); 118 if (dst.getType() == null && src.getType() != null) 119 dst.setType(src.getType()); 120 if (dst.getPeriod() == null && src.getPeriod() != null) 121 dst.setPeriod(src.getPeriod()); 122 if (dst.getAssigner() == null && src.getAssigner() != null) 123 dst.setAssigner(src.getAssigner()); 124 } 125 126 public static boolean matches(ContactPoint c1, ContactPoint c2, Object profile) { 127 if (profile != null) 128 throw new NotImplementedException("Not Implemented Yet"); 129 130 // in the absence of a profile, we insist on system 131 return matches(c1.getSystemElement(), c2.getSystemElement(), null) && matches(c1.getValue(), c2.getValue(), null); 132 } 133 134 public static void merge(ContactPoint dst, ContactPoint src) { 135 if (dst.getUseElement() == null && src.getUseElement() != null) 136 dst.setUseElement(src.getUseElement()); 137 if (dst.getPeriod() == null && src.getPeriod() != null) 138 dst.setPeriod(src.getPeriod()); 139 } 140 141}