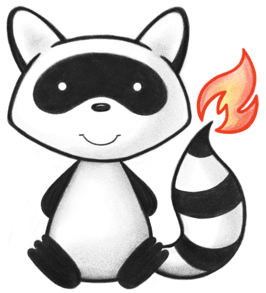
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A compartment definition that defines how resources are accessed on a server. 052 */ 053@ResourceDef(name="CompartmentDefinition", profile="http://hl7.org/fhir/StructureDefinition/CompartmentDefinition") 054public class CompartmentDefinition extends CanonicalResource { 055 056 @Block() 057 public static class CompartmentDefinitionResourceComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The name of a resource supported by the server. 060 */ 061 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Name of resource type", formalDefinition="The name of a resource supported by the server." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 064 protected CodeType code; 065 066 /** 067 * The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,. 068 */ 069 @Child(name = "param", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 070 @Description(shortDefinition="Search Parameter Name, or chained parameters", formalDefinition="The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,." ) 071 protected List<StringType> param; 072 073 /** 074 * Additional documentation about the resource and compartment. 075 */ 076 @Child(name = "documentation", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 077 @Description(shortDefinition="Additional documentation about the resource and compartment", formalDefinition="Additional documentation about the resource and compartment." ) 078 protected StringType documentation; 079 080 /** 081 * Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)). 082 */ 083 @Child(name = "startParam", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=false) 084 @Description(shortDefinition="Search Param for interpreting $everything.start", formalDefinition="Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html))." ) 085 protected UriType startParam; 086 087 /** 088 * Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)). 089 */ 090 @Child(name = "endParam", type = {UriType.class}, order=5, min=0, max=1, modifier=false, summary=false) 091 @Description(shortDefinition="Search Param for interpreting $everything.end", formalDefinition="Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html))." ) 092 protected UriType endParam; 093 094 private static final long serialVersionUID = 1245370010L; 095 096 /** 097 * Constructor 098 */ 099 public CompartmentDefinitionResourceComponent() { 100 super(); 101 } 102 103 /** 104 * Constructor 105 */ 106 public CompartmentDefinitionResourceComponent(String code) { 107 super(); 108 this.setCode(code); 109 } 110 111 /** 112 * @return {@link #code} (The name of a resource supported by the server.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 113 */ 114 public CodeType getCodeElement() { 115 if (this.code == null) 116 if (Configuration.errorOnAutoCreate()) 117 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.code"); 118 else if (Configuration.doAutoCreate()) 119 this.code = new CodeType(); // bb 120 return this.code; 121 } 122 123 public boolean hasCodeElement() { 124 return this.code != null && !this.code.isEmpty(); 125 } 126 127 public boolean hasCode() { 128 return this.code != null && !this.code.isEmpty(); 129 } 130 131 /** 132 * @param value {@link #code} (The name of a resource supported by the server.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 133 */ 134 public CompartmentDefinitionResourceComponent setCodeElement(CodeType value) { 135 this.code = value; 136 return this; 137 } 138 139 /** 140 * @return The name of a resource supported by the server. 141 */ 142 public String getCode() { 143 return this.code == null ? null : this.code.getValue(); 144 } 145 146 /** 147 * @param value The name of a resource supported by the server. 148 */ 149 public CompartmentDefinitionResourceComponent setCode(String value) { 150 if (this.code == null) 151 this.code = new CodeType(); 152 this.code.setValue(value); 153 return this; 154 } 155 156 /** 157 * @return {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 158 */ 159 public List<StringType> getParam() { 160 if (this.param == null) 161 this.param = new ArrayList<StringType>(); 162 return this.param; 163 } 164 165 /** 166 * @return Returns a reference to <code>this</code> for easy method chaining 167 */ 168 public CompartmentDefinitionResourceComponent setParam(List<StringType> theParam) { 169 this.param = theParam; 170 return this; 171 } 172 173 public boolean hasParam() { 174 if (this.param == null) 175 return false; 176 for (StringType item : this.param) 177 if (!item.isEmpty()) 178 return true; 179 return false; 180 } 181 182 /** 183 * @return {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 184 */ 185 public StringType addParamElement() {//2 186 StringType t = new StringType(); 187 if (this.param == null) 188 this.param = new ArrayList<StringType>(); 189 this.param.add(t); 190 return t; 191 } 192 193 /** 194 * @param value {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 195 */ 196 public CompartmentDefinitionResourceComponent addParam(String value) { //1 197 StringType t = new StringType(); 198 t.setValue(value); 199 if (this.param == null) 200 this.param = new ArrayList<StringType>(); 201 this.param.add(t); 202 return this; 203 } 204 205 /** 206 * @param value {@link #param} (The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.) 207 */ 208 public boolean hasParam(String value) { 209 if (this.param == null) 210 return false; 211 for (StringType v : this.param) 212 if (v.getValue().equals(value)) // string 213 return true; 214 return false; 215 } 216 217 /** 218 * @return {@link #documentation} (Additional documentation about the resource and compartment.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 219 */ 220 public StringType getDocumentationElement() { 221 if (this.documentation == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.documentation"); 224 else if (Configuration.doAutoCreate()) 225 this.documentation = new StringType(); // bb 226 return this.documentation; 227 } 228 229 public boolean hasDocumentationElement() { 230 return this.documentation != null && !this.documentation.isEmpty(); 231 } 232 233 public boolean hasDocumentation() { 234 return this.documentation != null && !this.documentation.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #documentation} (Additional documentation about the resource and compartment.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 239 */ 240 public CompartmentDefinitionResourceComponent setDocumentationElement(StringType value) { 241 this.documentation = value; 242 return this; 243 } 244 245 /** 246 * @return Additional documentation about the resource and compartment. 247 */ 248 public String getDocumentation() { 249 return this.documentation == null ? null : this.documentation.getValue(); 250 } 251 252 /** 253 * @param value Additional documentation about the resource and compartment. 254 */ 255 public CompartmentDefinitionResourceComponent setDocumentation(String value) { 256 if (Utilities.noString(value)) 257 this.documentation = null; 258 else { 259 if (this.documentation == null) 260 this.documentation = new StringType(); 261 this.documentation.setValue(value); 262 } 263 return this; 264 } 265 266 /** 267 * @return {@link #startParam} (Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)).). This is the underlying object with id, value and extensions. The accessor "getStartParam" gives direct access to the value 268 */ 269 public UriType getStartParamElement() { 270 if (this.startParam == null) 271 if (Configuration.errorOnAutoCreate()) 272 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.startParam"); 273 else if (Configuration.doAutoCreate()) 274 this.startParam = new UriType(); // bb 275 return this.startParam; 276 } 277 278 public boolean hasStartParamElement() { 279 return this.startParam != null && !this.startParam.isEmpty(); 280 } 281 282 public boolean hasStartParam() { 283 return this.startParam != null && !this.startParam.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #startParam} (Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)).). This is the underlying object with id, value and extensions. The accessor "getStartParam" gives direct access to the value 288 */ 289 public CompartmentDefinitionResourceComponent setStartParamElement(UriType value) { 290 this.startParam = value; 291 return this; 292 } 293 294 /** 295 * @return Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)). 296 */ 297 public String getStartParam() { 298 return this.startParam == null ? null : this.startParam.getValue(); 299 } 300 301 /** 302 * @param value Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)). 303 */ 304 public CompartmentDefinitionResourceComponent setStartParam(String value) { 305 if (Utilities.noString(value)) 306 this.startParam = null; 307 else { 308 if (this.startParam == null) 309 this.startParam = new UriType(); 310 this.startParam.setValue(value); 311 } 312 return this; 313 } 314 315 /** 316 * @return {@link #endParam} (Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)).). This is the underlying object with id, value and extensions. The accessor "getEndParam" gives direct access to the value 317 */ 318 public UriType getEndParamElement() { 319 if (this.endParam == null) 320 if (Configuration.errorOnAutoCreate()) 321 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.endParam"); 322 else if (Configuration.doAutoCreate()) 323 this.endParam = new UriType(); // bb 324 return this.endParam; 325 } 326 327 public boolean hasEndParamElement() { 328 return this.endParam != null && !this.endParam.isEmpty(); 329 } 330 331 public boolean hasEndParam() { 332 return this.endParam != null && !this.endParam.isEmpty(); 333 } 334 335 /** 336 * @param value {@link #endParam} (Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)).). This is the underlying object with id, value and extensions. The accessor "getEndParam" gives direct access to the value 337 */ 338 public CompartmentDefinitionResourceComponent setEndParamElement(UriType value) { 339 this.endParam = value; 340 return this; 341 } 342 343 /** 344 * @return Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)). 345 */ 346 public String getEndParam() { 347 return this.endParam == null ? null : this.endParam.getValue(); 348 } 349 350 /** 351 * @param value Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)). 352 */ 353 public CompartmentDefinitionResourceComponent setEndParam(String value) { 354 if (Utilities.noString(value)) 355 this.endParam = null; 356 else { 357 if (this.endParam == null) 358 this.endParam = new UriType(); 359 this.endParam.setValue(value); 360 } 361 return this; 362 } 363 364 protected void listChildren(List<Property> children) { 365 super.listChildren(children); 366 children.add(new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code)); 367 children.add(new Property("param", "string", "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 0, java.lang.Integer.MAX_VALUE, param)); 368 children.add(new Property("documentation", "string", "Additional documentation about the resource and compartment.", 0, 1, documentation)); 369 children.add(new Property("startParam", "uri", "Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)).", 0, 1, startParam)); 370 children.add(new Property("endParam", "uri", "Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)).", 0, 1, endParam)); 371 } 372 373 @Override 374 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 375 switch (_hash) { 376 case 3059181: /*code*/ return new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code); 377 case 106436749: /*param*/ return new Property("param", "string", "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 0, java.lang.Integer.MAX_VALUE, param); 378 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Additional documentation about the resource and compartment.", 0, 1, documentation); 379 case -1587556021: /*startParam*/ return new Property("startParam", "uri", "Search Parameter for mapping requests made with $everything.start (e.g. on [Patient.$everything](patient-operation-everything.html)).", 0, 1, startParam); 380 case 1711140978: /*endParam*/ return new Property("endParam", "uri", "Search Parameter for mapping requests made with $everything.end (e.g. on [Patient.$everything](patient-operation-everything.html)).", 0, 1, endParam); 381 default: return super.getNamedProperty(_hash, _name, _checkValid); 382 } 383 384 } 385 386 @Override 387 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 388 switch (hash) { 389 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 390 case 106436749: /*param*/ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // StringType 391 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 392 case -1587556021: /*startParam*/ return this.startParam == null ? new Base[0] : new Base[] {this.startParam}; // UriType 393 case 1711140978: /*endParam*/ return this.endParam == null ? new Base[0] : new Base[] {this.endParam}; // UriType 394 default: return super.getProperty(hash, name, checkValid); 395 } 396 397 } 398 399 @Override 400 public Base setProperty(int hash, String name, Base value) throws FHIRException { 401 switch (hash) { 402 case 3059181: // code 403 this.code = TypeConvertor.castToCode(value); // CodeType 404 return value; 405 case 106436749: // param 406 this.getParam().add(TypeConvertor.castToString(value)); // StringType 407 return value; 408 case 1587405498: // documentation 409 this.documentation = TypeConvertor.castToString(value); // StringType 410 return value; 411 case -1587556021: // startParam 412 this.startParam = TypeConvertor.castToUri(value); // UriType 413 return value; 414 case 1711140978: // endParam 415 this.endParam = TypeConvertor.castToUri(value); // UriType 416 return value; 417 default: return super.setProperty(hash, name, value); 418 } 419 420 } 421 422 @Override 423 public Base setProperty(String name, Base value) throws FHIRException { 424 if (name.equals("code")) { 425 this.code = TypeConvertor.castToCode(value); // CodeType 426 } else if (name.equals("param")) { 427 this.getParam().add(TypeConvertor.castToString(value)); 428 } else if (name.equals("documentation")) { 429 this.documentation = TypeConvertor.castToString(value); // StringType 430 } else if (name.equals("startParam")) { 431 this.startParam = TypeConvertor.castToUri(value); // UriType 432 } else if (name.equals("endParam")) { 433 this.endParam = TypeConvertor.castToUri(value); // UriType 434 } else 435 return super.setProperty(name, value); 436 return value; 437 } 438 439 @Override 440 public void removeChild(String name, Base value) throws FHIRException { 441 if (name.equals("code")) { 442 this.code = null; 443 } else if (name.equals("param")) { 444 this.getParam().remove(value); 445 } else if (name.equals("documentation")) { 446 this.documentation = null; 447 } else if (name.equals("startParam")) { 448 this.startParam = null; 449 } else if (name.equals("endParam")) { 450 this.endParam = null; 451 } else 452 super.removeChild(name, value); 453 454 } 455 456 @Override 457 public Base makeProperty(int hash, String name) throws FHIRException { 458 switch (hash) { 459 case 3059181: return getCodeElement(); 460 case 106436749: return addParamElement(); 461 case 1587405498: return getDocumentationElement(); 462 case -1587556021: return getStartParamElement(); 463 case 1711140978: return getEndParamElement(); 464 default: return super.makeProperty(hash, name); 465 } 466 467 } 468 469 @Override 470 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 471 switch (hash) { 472 case 3059181: /*code*/ return new String[] {"code"}; 473 case 106436749: /*param*/ return new String[] {"string"}; 474 case 1587405498: /*documentation*/ return new String[] {"string"}; 475 case -1587556021: /*startParam*/ return new String[] {"uri"}; 476 case 1711140978: /*endParam*/ return new String[] {"uri"}; 477 default: return super.getTypesForProperty(hash, name); 478 } 479 480 } 481 482 @Override 483 public Base addChild(String name) throws FHIRException { 484 if (name.equals("code")) { 485 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.resource.code"); 486 } 487 else if (name.equals("param")) { 488 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.resource.param"); 489 } 490 else if (name.equals("documentation")) { 491 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.resource.documentation"); 492 } 493 else if (name.equals("startParam")) { 494 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.resource.startParam"); 495 } 496 else if (name.equals("endParam")) { 497 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.resource.endParam"); 498 } 499 else 500 return super.addChild(name); 501 } 502 503 public CompartmentDefinitionResourceComponent copy() { 504 CompartmentDefinitionResourceComponent dst = new CompartmentDefinitionResourceComponent(); 505 copyValues(dst); 506 return dst; 507 } 508 509 public void copyValues(CompartmentDefinitionResourceComponent dst) { 510 super.copyValues(dst); 511 dst.code = code == null ? null : code.copy(); 512 if (param != null) { 513 dst.param = new ArrayList<StringType>(); 514 for (StringType i : param) 515 dst.param.add(i.copy()); 516 }; 517 dst.documentation = documentation == null ? null : documentation.copy(); 518 dst.startParam = startParam == null ? null : startParam.copy(); 519 dst.endParam = endParam == null ? null : endParam.copy(); 520 } 521 522 @Override 523 public boolean equalsDeep(Base other_) { 524 if (!super.equalsDeep(other_)) 525 return false; 526 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 527 return false; 528 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 529 return compareDeep(code, o.code, true) && compareDeep(param, o.param, true) && compareDeep(documentation, o.documentation, true) 530 && compareDeep(startParam, o.startParam, true) && compareDeep(endParam, o.endParam, true); 531 } 532 533 @Override 534 public boolean equalsShallow(Base other_) { 535 if (!super.equalsShallow(other_)) 536 return false; 537 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 538 return false; 539 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 540 return compareValues(code, o.code, true) && compareValues(param, o.param, true) && compareValues(documentation, o.documentation, true) 541 && compareValues(startParam, o.startParam, true) && compareValues(endParam, o.endParam, true); 542 } 543 544 public boolean isEmpty() { 545 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, param, documentation 546 , startParam, endParam); 547 } 548 549 public String fhirType() { 550 return "CompartmentDefinition.resource"; 551 552 } 553 554 } 555 556 /** 557 * An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers. 558 */ 559 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 560 @Description(shortDefinition="Canonical identifier for this compartment definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers." ) 561 protected UriType url; 562 563 /** 564 * The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 565 */ 566 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 567 @Description(shortDefinition="Business version of the compartment definition", formalDefinition="The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 568 protected StringType version; 569 570 /** 571 * Indicates the mechanism used to compare versions to determine which is more current. 572 */ 573 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 574 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 575 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 576 protected DataType versionAlgorithm; 577 578 /** 579 * A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 580 */ 581 @Child(name = "name", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 582 @Description(shortDefinition="Name for this compartment definition (computer friendly)", formalDefinition="A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 583 protected StringType name; 584 585 /** 586 * A short, descriptive, user-friendly title for the capability statement. 587 */ 588 @Child(name = "title", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 589 @Description(shortDefinition="Name for this compartment definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the capability statement." ) 590 protected StringType title; 591 592 /** 593 * The status of this compartment definition. Enables tracking the life-cycle of the content. 594 */ 595 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 596 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this compartment definition. Enables tracking the life-cycle of the content." ) 597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 598 protected Enumeration<PublicationStatus> status; 599 600 /** 601 * A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 602 */ 603 @Child(name = "experimental", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 604 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 605 protected BooleanType experimental; 606 607 /** 608 * The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes. 609 */ 610 @Child(name = "date", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 611 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes." ) 612 protected DateTimeType date; 613 614 /** 615 * The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition. 616 */ 617 @Child(name = "publisher", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 618 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition." ) 619 protected StringType publisher; 620 621 /** 622 * Contact details to assist a user in finding and communicating with the publisher. 623 */ 624 @Child(name = "contact", type = {ContactDetail.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 625 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 626 protected List<ContactDetail> contact; 627 628 /** 629 * A free text natural language description of the compartment definition from a consumer's perspective. 630 */ 631 @Child(name = "description", type = {MarkdownType.class}, order=10, min=0, max=1, modifier=false, summary=false) 632 @Description(shortDefinition="Natural language description of the compartment definition", formalDefinition="A free text natural language description of the compartment definition from a consumer's perspective." ) 633 protected MarkdownType description; 634 635 /** 636 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances. 637 */ 638 @Child(name = "useContext", type = {UsageContext.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 639 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances." ) 640 protected List<UsageContext> useContext; 641 642 /** 643 * Explanation of why this compartment definition is needed and why it has been designed as it has. 644 */ 645 @Child(name = "purpose", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=false) 646 @Description(shortDefinition="Why this compartment definition is defined", formalDefinition="Explanation of why this compartment definition is needed and why it has been designed as it has." ) 647 protected MarkdownType purpose; 648 649 /** 650 * Which compartment this definition describes. 651 */ 652 @Child(name = "code", type = {CodeType.class}, order=13, min=1, max=1, modifier=false, summary=true) 653 @Description(shortDefinition="Patient | Encounter | RelatedPerson | Practitioner | Device | EpisodeOfCare", formalDefinition="Which compartment this definition describes." ) 654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/compartment-type") 655 protected Enumeration<CompartmentType> code; 656 657 /** 658 * Whether the search syntax is supported,. 659 */ 660 @Child(name = "search", type = {BooleanType.class}, order=14, min=1, max=1, modifier=false, summary=true) 661 @Description(shortDefinition="Whether the search syntax is supported", formalDefinition="Whether the search syntax is supported,." ) 662 protected BooleanType search; 663 664 /** 665 * Information about how a resource is related to the compartment. 666 */ 667 @Child(name = "resource", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 668 @Description(shortDefinition="How a resource is related to the compartment", formalDefinition="Information about how a resource is related to the compartment." ) 669 protected List<CompartmentDefinitionResourceComponent> resource; 670 671 private static final long serialVersionUID = 140986198L; 672 673 /** 674 * Constructor 675 */ 676 public CompartmentDefinition() { 677 super(); 678 } 679 680 /** 681 * Constructor 682 */ 683 public CompartmentDefinition(String url, String name, PublicationStatus status, CompartmentType code, boolean search) { 684 super(); 685 this.setUrl(url); 686 this.setName(name); 687 this.setStatus(status); 688 this.setCode(code); 689 this.setSearch(search); 690 } 691 692 /** 693 * @return {@link #url} (An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 694 */ 695 public UriType getUrlElement() { 696 if (this.url == null) 697 if (Configuration.errorOnAutoCreate()) 698 throw new Error("Attempt to auto-create CompartmentDefinition.url"); 699 else if (Configuration.doAutoCreate()) 700 this.url = new UriType(); // bb 701 return this.url; 702 } 703 704 public boolean hasUrlElement() { 705 return this.url != null && !this.url.isEmpty(); 706 } 707 708 public boolean hasUrl() { 709 return this.url != null && !this.url.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #url} (An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 714 */ 715 public CompartmentDefinition setUrlElement(UriType value) { 716 this.url = value; 717 return this; 718 } 719 720 /** 721 * @return An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers. 722 */ 723 public String getUrl() { 724 return this.url == null ? null : this.url.getValue(); 725 } 726 727 /** 728 * @param value An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers. 729 */ 730 public CompartmentDefinition setUrl(String value) { 731 if (this.url == null) 732 this.url = new UriType(); 733 this.url.setValue(value); 734 return this; 735 } 736 737 /** 738 * @return {@link #version} (The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 739 */ 740 public StringType getVersionElement() { 741 if (this.version == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create CompartmentDefinition.version"); 744 else if (Configuration.doAutoCreate()) 745 this.version = new StringType(); // bb 746 return this.version; 747 } 748 749 public boolean hasVersionElement() { 750 return this.version != null && !this.version.isEmpty(); 751 } 752 753 public boolean hasVersion() { 754 return this.version != null && !this.version.isEmpty(); 755 } 756 757 /** 758 * @param value {@link #version} (The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 759 */ 760 public CompartmentDefinition setVersionElement(StringType value) { 761 this.version = value; 762 return this; 763 } 764 765 /** 766 * @return The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 767 */ 768 public String getVersion() { 769 return this.version == null ? null : this.version.getValue(); 770 } 771 772 /** 773 * @param value The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 774 */ 775 public CompartmentDefinition setVersion(String value) { 776 if (Utilities.noString(value)) 777 this.version = null; 778 else { 779 if (this.version == null) 780 this.version = new StringType(); 781 this.version.setValue(value); 782 } 783 return this; 784 } 785 786 /** 787 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 788 */ 789 public DataType getVersionAlgorithm() { 790 return this.versionAlgorithm; 791 } 792 793 /** 794 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 795 */ 796 public StringType getVersionAlgorithmStringType() throws FHIRException { 797 if (this.versionAlgorithm == null) 798 this.versionAlgorithm = new StringType(); 799 if (!(this.versionAlgorithm instanceof StringType)) 800 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 801 return (StringType) this.versionAlgorithm; 802 } 803 804 public boolean hasVersionAlgorithmStringType() { 805 return this != null && this.versionAlgorithm instanceof StringType; 806 } 807 808 /** 809 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 810 */ 811 public Coding getVersionAlgorithmCoding() throws FHIRException { 812 if (this.versionAlgorithm == null) 813 this.versionAlgorithm = new Coding(); 814 if (!(this.versionAlgorithm instanceof Coding)) 815 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 816 return (Coding) this.versionAlgorithm; 817 } 818 819 public boolean hasVersionAlgorithmCoding() { 820 return this != null && this.versionAlgorithm instanceof Coding; 821 } 822 823 public boolean hasVersionAlgorithm() { 824 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 829 */ 830 public CompartmentDefinition setVersionAlgorithm(DataType value) { 831 if (value != null && !(value instanceof StringType || value instanceof Coding)) 832 throw new FHIRException("Not the right type for CompartmentDefinition.versionAlgorithm[x]: "+value.fhirType()); 833 this.versionAlgorithm = value; 834 return this; 835 } 836 837 /** 838 * @return {@link #name} (A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 839 */ 840 public StringType getNameElement() { 841 if (this.name == null) 842 if (Configuration.errorOnAutoCreate()) 843 throw new Error("Attempt to auto-create CompartmentDefinition.name"); 844 else if (Configuration.doAutoCreate()) 845 this.name = new StringType(); // bb 846 return this.name; 847 } 848 849 public boolean hasNameElement() { 850 return this.name != null && !this.name.isEmpty(); 851 } 852 853 public boolean hasName() { 854 return this.name != null && !this.name.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #name} (A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 859 */ 860 public CompartmentDefinition setNameElement(StringType value) { 861 this.name = value; 862 return this; 863 } 864 865 /** 866 * @return A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 867 */ 868 public String getName() { 869 return this.name == null ? null : this.name.getValue(); 870 } 871 872 /** 873 * @param value A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 874 */ 875 public CompartmentDefinition setName(String value) { 876 if (this.name == null) 877 this.name = new StringType(); 878 this.name.setValue(value); 879 return this; 880 } 881 882 /** 883 * @return {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 884 */ 885 public StringType getTitleElement() { 886 if (this.title == null) 887 if (Configuration.errorOnAutoCreate()) 888 throw new Error("Attempt to auto-create CompartmentDefinition.title"); 889 else if (Configuration.doAutoCreate()) 890 this.title = new StringType(); // bb 891 return this.title; 892 } 893 894 public boolean hasTitleElement() { 895 return this.title != null && !this.title.isEmpty(); 896 } 897 898 public boolean hasTitle() { 899 return this.title != null && !this.title.isEmpty(); 900 } 901 902 /** 903 * @param value {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 904 */ 905 public CompartmentDefinition setTitleElement(StringType value) { 906 this.title = value; 907 return this; 908 } 909 910 /** 911 * @return A short, descriptive, user-friendly title for the capability statement. 912 */ 913 public String getTitle() { 914 return this.title == null ? null : this.title.getValue(); 915 } 916 917 /** 918 * @param value A short, descriptive, user-friendly title for the capability statement. 919 */ 920 public CompartmentDefinition setTitle(String value) { 921 if (Utilities.noString(value)) 922 this.title = null; 923 else { 924 if (this.title == null) 925 this.title = new StringType(); 926 this.title.setValue(value); 927 } 928 return this; 929 } 930 931 /** 932 * @return {@link #status} (The status of this compartment definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 933 */ 934 public Enumeration<PublicationStatus> getStatusElement() { 935 if (this.status == null) 936 if (Configuration.errorOnAutoCreate()) 937 throw new Error("Attempt to auto-create CompartmentDefinition.status"); 938 else if (Configuration.doAutoCreate()) 939 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 940 return this.status; 941 } 942 943 public boolean hasStatusElement() { 944 return this.status != null && !this.status.isEmpty(); 945 } 946 947 public boolean hasStatus() { 948 return this.status != null && !this.status.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #status} (The status of this compartment definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 953 */ 954 public CompartmentDefinition setStatusElement(Enumeration<PublicationStatus> value) { 955 this.status = value; 956 return this; 957 } 958 959 /** 960 * @return The status of this compartment definition. Enables tracking the life-cycle of the content. 961 */ 962 public PublicationStatus getStatus() { 963 return this.status == null ? null : this.status.getValue(); 964 } 965 966 /** 967 * @param value The status of this compartment definition. Enables tracking the life-cycle of the content. 968 */ 969 public CompartmentDefinition setStatus(PublicationStatus value) { 970 if (this.status == null) 971 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 972 this.status.setValue(value); 973 return this; 974 } 975 976 /** 977 * @return {@link #experimental} (A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 978 */ 979 public BooleanType getExperimentalElement() { 980 if (this.experimental == null) 981 if (Configuration.errorOnAutoCreate()) 982 throw new Error("Attempt to auto-create CompartmentDefinition.experimental"); 983 else if (Configuration.doAutoCreate()) 984 this.experimental = new BooleanType(); // bb 985 return this.experimental; 986 } 987 988 public boolean hasExperimentalElement() { 989 return this.experimental != null && !this.experimental.isEmpty(); 990 } 991 992 public boolean hasExperimental() { 993 return this.experimental != null && !this.experimental.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #experimental} (A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 998 */ 999 public CompartmentDefinition setExperimentalElement(BooleanType value) { 1000 this.experimental = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1006 */ 1007 public boolean getExperimental() { 1008 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1009 } 1010 1011 /** 1012 * @param value A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1013 */ 1014 public CompartmentDefinition setExperimental(boolean value) { 1015 if (this.experimental == null) 1016 this.experimental = new BooleanType(); 1017 this.experimental.setValue(value); 1018 return this; 1019 } 1020 1021 /** 1022 * @return {@link #date} (The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1023 */ 1024 public DateTimeType getDateElement() { 1025 if (this.date == null) 1026 if (Configuration.errorOnAutoCreate()) 1027 throw new Error("Attempt to auto-create CompartmentDefinition.date"); 1028 else if (Configuration.doAutoCreate()) 1029 this.date = new DateTimeType(); // bb 1030 return this.date; 1031 } 1032 1033 public boolean hasDateElement() { 1034 return this.date != null && !this.date.isEmpty(); 1035 } 1036 1037 public boolean hasDate() { 1038 return this.date != null && !this.date.isEmpty(); 1039 } 1040 1041 /** 1042 * @param value {@link #date} (The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1043 */ 1044 public CompartmentDefinition setDateElement(DateTimeType value) { 1045 this.date = value; 1046 return this; 1047 } 1048 1049 /** 1050 * @return The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes. 1051 */ 1052 public Date getDate() { 1053 return this.date == null ? null : this.date.getValue(); 1054 } 1055 1056 /** 1057 * @param value The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes. 1058 */ 1059 public CompartmentDefinition setDate(Date value) { 1060 if (value == null) 1061 this.date = null; 1062 else { 1063 if (this.date == null) 1064 this.date = new DateTimeType(); 1065 this.date.setValue(value); 1066 } 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1072 */ 1073 public StringType getPublisherElement() { 1074 if (this.publisher == null) 1075 if (Configuration.errorOnAutoCreate()) 1076 throw new Error("Attempt to auto-create CompartmentDefinition.publisher"); 1077 else if (Configuration.doAutoCreate()) 1078 this.publisher = new StringType(); // bb 1079 return this.publisher; 1080 } 1081 1082 public boolean hasPublisherElement() { 1083 return this.publisher != null && !this.publisher.isEmpty(); 1084 } 1085 1086 public boolean hasPublisher() { 1087 return this.publisher != null && !this.publisher.isEmpty(); 1088 } 1089 1090 /** 1091 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1092 */ 1093 public CompartmentDefinition setPublisherElement(StringType value) { 1094 this.publisher = value; 1095 return this; 1096 } 1097 1098 /** 1099 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition. 1100 */ 1101 public String getPublisher() { 1102 return this.publisher == null ? null : this.publisher.getValue(); 1103 } 1104 1105 /** 1106 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition. 1107 */ 1108 public CompartmentDefinition setPublisher(String value) { 1109 if (Utilities.noString(value)) 1110 this.publisher = null; 1111 else { 1112 if (this.publisher == null) 1113 this.publisher = new StringType(); 1114 this.publisher.setValue(value); 1115 } 1116 return this; 1117 } 1118 1119 /** 1120 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1121 */ 1122 public List<ContactDetail> getContact() { 1123 if (this.contact == null) 1124 this.contact = new ArrayList<ContactDetail>(); 1125 return this.contact; 1126 } 1127 1128 /** 1129 * @return Returns a reference to <code>this</code> for easy method chaining 1130 */ 1131 public CompartmentDefinition setContact(List<ContactDetail> theContact) { 1132 this.contact = theContact; 1133 return this; 1134 } 1135 1136 public boolean hasContact() { 1137 if (this.contact == null) 1138 return false; 1139 for (ContactDetail item : this.contact) 1140 if (!item.isEmpty()) 1141 return true; 1142 return false; 1143 } 1144 1145 public ContactDetail addContact() { //3 1146 ContactDetail t = new ContactDetail(); 1147 if (this.contact == null) 1148 this.contact = new ArrayList<ContactDetail>(); 1149 this.contact.add(t); 1150 return t; 1151 } 1152 1153 public CompartmentDefinition addContact(ContactDetail t) { //3 1154 if (t == null) 1155 return this; 1156 if (this.contact == null) 1157 this.contact = new ArrayList<ContactDetail>(); 1158 this.contact.add(t); 1159 return this; 1160 } 1161 1162 /** 1163 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1164 */ 1165 public ContactDetail getContactFirstRep() { 1166 if (getContact().isEmpty()) { 1167 addContact(); 1168 } 1169 return getContact().get(0); 1170 } 1171 1172 /** 1173 * @return {@link #description} (A free text natural language description of the compartment definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1174 */ 1175 public MarkdownType getDescriptionElement() { 1176 if (this.description == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create CompartmentDefinition.description"); 1179 else if (Configuration.doAutoCreate()) 1180 this.description = new MarkdownType(); // bb 1181 return this.description; 1182 } 1183 1184 public boolean hasDescriptionElement() { 1185 return this.description != null && !this.description.isEmpty(); 1186 } 1187 1188 public boolean hasDescription() { 1189 return this.description != null && !this.description.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #description} (A free text natural language description of the compartment definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1194 */ 1195 public CompartmentDefinition setDescriptionElement(MarkdownType value) { 1196 this.description = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return A free text natural language description of the compartment definition from a consumer's perspective. 1202 */ 1203 public String getDescription() { 1204 return this.description == null ? null : this.description.getValue(); 1205 } 1206 1207 /** 1208 * @param value A free text natural language description of the compartment definition from a consumer's perspective. 1209 */ 1210 public CompartmentDefinition setDescription(String value) { 1211 if (Utilities.noString(value)) 1212 this.description = null; 1213 else { 1214 if (this.description == null) 1215 this.description = new MarkdownType(); 1216 this.description.setValue(value); 1217 } 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.) 1223 */ 1224 public List<UsageContext> getUseContext() { 1225 if (this.useContext == null) 1226 this.useContext = new ArrayList<UsageContext>(); 1227 return this.useContext; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public CompartmentDefinition setUseContext(List<UsageContext> theUseContext) { 1234 this.useContext = theUseContext; 1235 return this; 1236 } 1237 1238 public boolean hasUseContext() { 1239 if (this.useContext == null) 1240 return false; 1241 for (UsageContext item : this.useContext) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 public UsageContext addUseContext() { //3 1248 UsageContext t = new UsageContext(); 1249 if (this.useContext == null) 1250 this.useContext = new ArrayList<UsageContext>(); 1251 this.useContext.add(t); 1252 return t; 1253 } 1254 1255 public CompartmentDefinition addUseContext(UsageContext t) { //3 1256 if (t == null) 1257 return this; 1258 if (this.useContext == null) 1259 this.useContext = new ArrayList<UsageContext>(); 1260 this.useContext.add(t); 1261 return this; 1262 } 1263 1264 /** 1265 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1266 */ 1267 public UsageContext getUseContextFirstRep() { 1268 if (getUseContext().isEmpty()) { 1269 addUseContext(); 1270 } 1271 return getUseContext().get(0); 1272 } 1273 1274 /** 1275 * @return {@link #purpose} (Explanation of why this compartment definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1276 */ 1277 public MarkdownType getPurposeElement() { 1278 if (this.purpose == null) 1279 if (Configuration.errorOnAutoCreate()) 1280 throw new Error("Attempt to auto-create CompartmentDefinition.purpose"); 1281 else if (Configuration.doAutoCreate()) 1282 this.purpose = new MarkdownType(); // bb 1283 return this.purpose; 1284 } 1285 1286 public boolean hasPurposeElement() { 1287 return this.purpose != null && !this.purpose.isEmpty(); 1288 } 1289 1290 public boolean hasPurpose() { 1291 return this.purpose != null && !this.purpose.isEmpty(); 1292 } 1293 1294 /** 1295 * @param value {@link #purpose} (Explanation of why this compartment definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1296 */ 1297 public CompartmentDefinition setPurposeElement(MarkdownType value) { 1298 this.purpose = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return Explanation of why this compartment definition is needed and why it has been designed as it has. 1304 */ 1305 public String getPurpose() { 1306 return this.purpose == null ? null : this.purpose.getValue(); 1307 } 1308 1309 /** 1310 * @param value Explanation of why this compartment definition is needed and why it has been designed as it has. 1311 */ 1312 public CompartmentDefinition setPurpose(String value) { 1313 if (Utilities.noString(value)) 1314 this.purpose = null; 1315 else { 1316 if (this.purpose == null) 1317 this.purpose = new MarkdownType(); 1318 this.purpose.setValue(value); 1319 } 1320 return this; 1321 } 1322 1323 /** 1324 * @return {@link #code} (Which compartment this definition describes.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1325 */ 1326 public Enumeration<CompartmentType> getCodeElement() { 1327 if (this.code == null) 1328 if (Configuration.errorOnAutoCreate()) 1329 throw new Error("Attempt to auto-create CompartmentDefinition.code"); 1330 else if (Configuration.doAutoCreate()) 1331 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); // bb 1332 return this.code; 1333 } 1334 1335 public boolean hasCodeElement() { 1336 return this.code != null && !this.code.isEmpty(); 1337 } 1338 1339 public boolean hasCode() { 1340 return this.code != null && !this.code.isEmpty(); 1341 } 1342 1343 /** 1344 * @param value {@link #code} (Which compartment this definition describes.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1345 */ 1346 public CompartmentDefinition setCodeElement(Enumeration<CompartmentType> value) { 1347 this.code = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return Which compartment this definition describes. 1353 */ 1354 public CompartmentType getCode() { 1355 return this.code == null ? null : this.code.getValue(); 1356 } 1357 1358 /** 1359 * @param value Which compartment this definition describes. 1360 */ 1361 public CompartmentDefinition setCode(CompartmentType value) { 1362 if (this.code == null) 1363 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); 1364 this.code.setValue(value); 1365 return this; 1366 } 1367 1368 /** 1369 * @return {@link #search} (Whether the search syntax is supported,.). This is the underlying object with id, value and extensions. The accessor "getSearch" gives direct access to the value 1370 */ 1371 public BooleanType getSearchElement() { 1372 if (this.search == null) 1373 if (Configuration.errorOnAutoCreate()) 1374 throw new Error("Attempt to auto-create CompartmentDefinition.search"); 1375 else if (Configuration.doAutoCreate()) 1376 this.search = new BooleanType(); // bb 1377 return this.search; 1378 } 1379 1380 public boolean hasSearchElement() { 1381 return this.search != null && !this.search.isEmpty(); 1382 } 1383 1384 public boolean hasSearch() { 1385 return this.search != null && !this.search.isEmpty(); 1386 } 1387 1388 /** 1389 * @param value {@link #search} (Whether the search syntax is supported,.). This is the underlying object with id, value and extensions. The accessor "getSearch" gives direct access to the value 1390 */ 1391 public CompartmentDefinition setSearchElement(BooleanType value) { 1392 this.search = value; 1393 return this; 1394 } 1395 1396 /** 1397 * @return Whether the search syntax is supported,. 1398 */ 1399 public boolean getSearch() { 1400 return this.search == null || this.search.isEmpty() ? false : this.search.getValue(); 1401 } 1402 1403 /** 1404 * @param value Whether the search syntax is supported,. 1405 */ 1406 public CompartmentDefinition setSearch(boolean value) { 1407 if (this.search == null) 1408 this.search = new BooleanType(); 1409 this.search.setValue(value); 1410 return this; 1411 } 1412 1413 /** 1414 * @return {@link #resource} (Information about how a resource is related to the compartment.) 1415 */ 1416 public List<CompartmentDefinitionResourceComponent> getResource() { 1417 if (this.resource == null) 1418 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1419 return this.resource; 1420 } 1421 1422 /** 1423 * @return Returns a reference to <code>this</code> for easy method chaining 1424 */ 1425 public CompartmentDefinition setResource(List<CompartmentDefinitionResourceComponent> theResource) { 1426 this.resource = theResource; 1427 return this; 1428 } 1429 1430 public boolean hasResource() { 1431 if (this.resource == null) 1432 return false; 1433 for (CompartmentDefinitionResourceComponent item : this.resource) 1434 if (!item.isEmpty()) 1435 return true; 1436 return false; 1437 } 1438 1439 public CompartmentDefinitionResourceComponent addResource() { //3 1440 CompartmentDefinitionResourceComponent t = new CompartmentDefinitionResourceComponent(); 1441 if (this.resource == null) 1442 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1443 this.resource.add(t); 1444 return t; 1445 } 1446 1447 public CompartmentDefinition addResource(CompartmentDefinitionResourceComponent t) { //3 1448 if (t == null) 1449 return this; 1450 if (this.resource == null) 1451 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1452 this.resource.add(t); 1453 return this; 1454 } 1455 1456 /** 1457 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist {3} 1458 */ 1459 public CompartmentDefinitionResourceComponent getResourceFirstRep() { 1460 if (getResource().isEmpty()) { 1461 addResource(); 1462 } 1463 return getResource().get(0); 1464 } 1465 1466 /** 1467 * not supported on this implementation 1468 */ 1469 @Override 1470 public int getIdentifierMax() { 1471 return 0; 1472 } 1473 /** 1474 * @return {@link #identifier} (A formal identifier that is used to identify this compartment definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1475 */ 1476 public List<Identifier> getIdentifier() { 1477 return new ArrayList<>(); 1478 } 1479 /** 1480 * @return Returns a reference to <code>this</code> for easy method chaining 1481 */ 1482 public CompartmentDefinition setIdentifier(List<Identifier> theIdentifier) { 1483 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"identifier\""); 1484 } 1485 public boolean hasIdentifier() { 1486 return false; 1487 } 1488 1489 public Identifier addIdentifier() { //3 1490 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"identifier\""); 1491 } 1492 public CompartmentDefinition addIdentifier(Identifier t) { //3 1493 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"identifier\""); 1494 } 1495 /** 1496 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {2} 1497 */ 1498 public Identifier getIdentifierFirstRep() { 1499 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"identifier\""); 1500 } 1501 /** 1502 * not supported on this implementation 1503 */ 1504 @Override 1505 public int getJurisdictionMax() { 1506 return 0; 1507 } 1508 /** 1509 * @return {@link #jurisdiction} (A legal or geographic region in which the compartment definition is intended to be used.) 1510 */ 1511 public List<CodeableConcept> getJurisdiction() { 1512 return new ArrayList<>(); 1513 } 1514 /** 1515 * @return Returns a reference to <code>this</code> for easy method chaining 1516 */ 1517 public CompartmentDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1518 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"jurisdiction\""); 1519 } 1520 public boolean hasJurisdiction() { 1521 return false; 1522 } 1523 1524 public CodeableConcept addJurisdiction() { //3 1525 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"jurisdiction\""); 1526 } 1527 public CompartmentDefinition addJurisdiction(CodeableConcept t) { //3 1528 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"jurisdiction\""); 1529 } 1530 /** 1531 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {2} 1532 */ 1533 public CodeableConcept getJurisdictionFirstRep() { 1534 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"jurisdiction\""); 1535 } 1536 /** 1537 * not supported on this implementation 1538 */ 1539 @Override 1540 public int getCopyrightMax() { 1541 return 0; 1542 } 1543 /** 1544 * @return {@link #copyright} (A copyright statement relating to the compartment definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1545 */ 1546 public MarkdownType getCopyrightElement() { 1547 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyright\""); 1548 } 1549 1550 public boolean hasCopyrightElement() { 1551 return false; 1552 } 1553 public boolean hasCopyright() { 1554 return false; 1555 } 1556 1557 /** 1558 * @param value {@link #copyright} (A copyright statement relating to the compartment definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the compartment definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1559 */ 1560 public CompartmentDefinition setCopyrightElement(MarkdownType value) { 1561 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyright\""); 1562 } 1563 public String getCopyright() { 1564 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyright\""); 1565 } 1566 /** 1567 * @param value A copyright statement relating to the compartment definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the compartment definition. 1568 */ 1569 public CompartmentDefinition setCopyright(String value) { 1570 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyright\""); 1571 } 1572 /** 1573 * not supported on this implementation 1574 */ 1575 @Override 1576 public int getCopyrightLabelMax() { 1577 return 0; 1578 } 1579 /** 1580 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1581 */ 1582 public StringType getCopyrightLabelElement() { 1583 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyrightLabel\""); 1584 } 1585 1586 public boolean hasCopyrightLabelElement() { 1587 return false; 1588 } 1589 public boolean hasCopyrightLabel() { 1590 return false; 1591 } 1592 1593 /** 1594 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1595 */ 1596 public CompartmentDefinition setCopyrightLabelElement(StringType value) { 1597 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyrightLabel\""); 1598 } 1599 public String getCopyrightLabel() { 1600 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyrightLabel\""); 1601 } 1602 /** 1603 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1604 */ 1605 public CompartmentDefinition setCopyrightLabel(String value) { 1606 throw new Error("The resource type \"CompartmentDefinition\" does not implement the property \"copyrightLabel\""); 1607 } 1608 protected void listChildren(List<Property> children) { 1609 super.listChildren(children); 1610 children.add(new Property("url", "uri", "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.", 0, 1, url)); 1611 children.add(new Property("version", "string", "The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 1612 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 1613 children.add(new Property("name", "string", "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1614 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title)); 1615 children.add(new Property("status", "code", "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1616 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 1617 children.add(new Property("date", "dateTime", "The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 0, 1, date)); 1618 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition.", 0, 1, publisher)); 1619 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1620 children.add(new Property("description", "markdown", "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, description)); 1621 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1622 children.add(new Property("purpose", "markdown", "Explanation of why this compartment definition is needed and why it has been designed as it has.", 0, 1, purpose)); 1623 children.add(new Property("code", "code", "Which compartment this definition describes.", 0, 1, code)); 1624 children.add(new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search)); 1625 children.add(new Property("resource", "", "Information about how a resource is related to the compartment.", 0, java.lang.Integer.MAX_VALUE, resource)); 1626 } 1627 1628 @Override 1629 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1630 switch (_hash) { 1631 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.", 0, 1, url); 1632 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 1633 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1634 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1635 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1636 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1637 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1638 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title); 1639 case -892481550: /*status*/ return new Property("status", "code", "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1640 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 1641 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the compartment definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 0, 1, date); 1642 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the compartment definition.", 0, 1, publisher); 1643 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1644 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, description); 1645 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1646 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this compartment definition is needed and why it has been designed as it has.", 0, 1, purpose); 1647 case 3059181: /*code*/ return new Property("code", "code", "Which compartment this definition describes.", 0, 1, code); 1648 case -906336856: /*search*/ return new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search); 1649 case -341064690: /*resource*/ return new Property("resource", "", "Information about how a resource is related to the compartment.", 0, java.lang.Integer.MAX_VALUE, resource); 1650 default: return super.getNamedProperty(_hash, _name, _checkValid); 1651 } 1652 1653 } 1654 1655 @Override 1656 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1657 switch (hash) { 1658 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1659 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1660 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 1661 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1662 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1663 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1664 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1665 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1666 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1667 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1668 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1669 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1670 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 1671 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<CompartmentType> 1672 case -906336856: /*search*/ return this.search == null ? new Base[0] : new Base[] {this.search}; // BooleanType 1673 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CompartmentDefinitionResourceComponent 1674 default: return super.getProperty(hash, name, checkValid); 1675 } 1676 1677 } 1678 1679 @Override 1680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1681 switch (hash) { 1682 case 116079: // url 1683 this.url = TypeConvertor.castToUri(value); // UriType 1684 return value; 1685 case 351608024: // version 1686 this.version = TypeConvertor.castToString(value); // StringType 1687 return value; 1688 case 1508158071: // versionAlgorithm 1689 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 1690 return value; 1691 case 3373707: // name 1692 this.name = TypeConvertor.castToString(value); // StringType 1693 return value; 1694 case 110371416: // title 1695 this.title = TypeConvertor.castToString(value); // StringType 1696 return value; 1697 case -892481550: // status 1698 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1699 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1700 return value; 1701 case -404562712: // experimental 1702 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 1703 return value; 1704 case 3076014: // date 1705 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1706 return value; 1707 case 1447404028: // publisher 1708 this.publisher = TypeConvertor.castToString(value); // StringType 1709 return value; 1710 case 951526432: // contact 1711 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1712 return value; 1713 case -1724546052: // description 1714 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1715 return value; 1716 case -669707736: // useContext 1717 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 1718 return value; 1719 case -220463842: // purpose 1720 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 1721 return value; 1722 case 3059181: // code 1723 value = new CompartmentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1724 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1725 return value; 1726 case -906336856: // search 1727 this.search = TypeConvertor.castToBoolean(value); // BooleanType 1728 return value; 1729 case -341064690: // resource 1730 this.getResource().add((CompartmentDefinitionResourceComponent) value); // CompartmentDefinitionResourceComponent 1731 return value; 1732 default: return super.setProperty(hash, name, value); 1733 } 1734 1735 } 1736 1737 @Override 1738 public Base setProperty(String name, Base value) throws FHIRException { 1739 if (name.equals("url")) { 1740 this.url = TypeConvertor.castToUri(value); // UriType 1741 } else if (name.equals("version")) { 1742 this.version = TypeConvertor.castToString(value); // StringType 1743 } else if (name.equals("versionAlgorithm[x]")) { 1744 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 1745 } else if (name.equals("name")) { 1746 this.name = TypeConvertor.castToString(value); // StringType 1747 } else if (name.equals("title")) { 1748 this.title = TypeConvertor.castToString(value); // StringType 1749 } else if (name.equals("status")) { 1750 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1751 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1752 } else if (name.equals("experimental")) { 1753 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 1754 } else if (name.equals("date")) { 1755 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1756 } else if (name.equals("publisher")) { 1757 this.publisher = TypeConvertor.castToString(value); // StringType 1758 } else if (name.equals("contact")) { 1759 this.getContact().add(TypeConvertor.castToContactDetail(value)); 1760 } else if (name.equals("description")) { 1761 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1762 } else if (name.equals("useContext")) { 1763 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 1764 } else if (name.equals("purpose")) { 1765 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 1766 } else if (name.equals("code")) { 1767 value = new CompartmentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1768 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1769 } else if (name.equals("search")) { 1770 this.search = TypeConvertor.castToBoolean(value); // BooleanType 1771 } else if (name.equals("resource")) { 1772 this.getResource().add((CompartmentDefinitionResourceComponent) value); 1773 } else 1774 return super.setProperty(name, value); 1775 return value; 1776 } 1777 1778 @Override 1779 public void removeChild(String name, Base value) throws FHIRException { 1780 if (name.equals("url")) { 1781 this.url = null; 1782 } else if (name.equals("version")) { 1783 this.version = null; 1784 } else if (name.equals("versionAlgorithm[x]")) { 1785 this.versionAlgorithm = null; 1786 } else if (name.equals("name")) { 1787 this.name = null; 1788 } else if (name.equals("title")) { 1789 this.title = null; 1790 } else if (name.equals("status")) { 1791 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1792 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1793 } else if (name.equals("experimental")) { 1794 this.experimental = null; 1795 } else if (name.equals("date")) { 1796 this.date = null; 1797 } else if (name.equals("publisher")) { 1798 this.publisher = null; 1799 } else if (name.equals("contact")) { 1800 this.getContact().remove(value); 1801 } else if (name.equals("description")) { 1802 this.description = null; 1803 } else if (name.equals("useContext")) { 1804 this.getUseContext().remove(value); 1805 } else if (name.equals("purpose")) { 1806 this.purpose = null; 1807 } else if (name.equals("code")) { 1808 value = new CompartmentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1809 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1810 } else if (name.equals("search")) { 1811 this.search = null; 1812 } else if (name.equals("resource")) { 1813 this.getResource().remove((CompartmentDefinitionResourceComponent) value); 1814 } else 1815 super.removeChild(name, value); 1816 1817 } 1818 1819 @Override 1820 public Base makeProperty(int hash, String name) throws FHIRException { 1821 switch (hash) { 1822 case 116079: return getUrlElement(); 1823 case 351608024: return getVersionElement(); 1824 case -115699031: return getVersionAlgorithm(); 1825 case 1508158071: return getVersionAlgorithm(); 1826 case 3373707: return getNameElement(); 1827 case 110371416: return getTitleElement(); 1828 case -892481550: return getStatusElement(); 1829 case -404562712: return getExperimentalElement(); 1830 case 3076014: return getDateElement(); 1831 case 1447404028: return getPublisherElement(); 1832 case 951526432: return addContact(); 1833 case -1724546052: return getDescriptionElement(); 1834 case -669707736: return addUseContext(); 1835 case -220463842: return getPurposeElement(); 1836 case 3059181: return getCodeElement(); 1837 case -906336856: return getSearchElement(); 1838 case -341064690: return addResource(); 1839 default: return super.makeProperty(hash, name); 1840 } 1841 1842 } 1843 1844 @Override 1845 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1846 switch (hash) { 1847 case 116079: /*url*/ return new String[] {"uri"}; 1848 case 351608024: /*version*/ return new String[] {"string"}; 1849 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 1850 case 3373707: /*name*/ return new String[] {"string"}; 1851 case 110371416: /*title*/ return new String[] {"string"}; 1852 case -892481550: /*status*/ return new String[] {"code"}; 1853 case -404562712: /*experimental*/ return new String[] {"boolean"}; 1854 case 3076014: /*date*/ return new String[] {"dateTime"}; 1855 case 1447404028: /*publisher*/ return new String[] {"string"}; 1856 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1857 case -1724546052: /*description*/ return new String[] {"markdown"}; 1858 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1859 case -220463842: /*purpose*/ return new String[] {"markdown"}; 1860 case 3059181: /*code*/ return new String[] {"code"}; 1861 case -906336856: /*search*/ return new String[] {"boolean"}; 1862 case -341064690: /*resource*/ return new String[] {}; 1863 default: return super.getTypesForProperty(hash, name); 1864 } 1865 1866 } 1867 1868 @Override 1869 public Base addChild(String name) throws FHIRException { 1870 if (name.equals("url")) { 1871 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.url"); 1872 } 1873 else if (name.equals("version")) { 1874 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.version"); 1875 } 1876 else if (name.equals("versionAlgorithmString")) { 1877 this.versionAlgorithm = new StringType(); 1878 return this.versionAlgorithm; 1879 } 1880 else if (name.equals("versionAlgorithmCoding")) { 1881 this.versionAlgorithm = new Coding(); 1882 return this.versionAlgorithm; 1883 } 1884 else if (name.equals("name")) { 1885 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.name"); 1886 } 1887 else if (name.equals("title")) { 1888 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.title"); 1889 } 1890 else if (name.equals("status")) { 1891 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.status"); 1892 } 1893 else if (name.equals("experimental")) { 1894 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.experimental"); 1895 } 1896 else if (name.equals("date")) { 1897 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.date"); 1898 } 1899 else if (name.equals("publisher")) { 1900 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.publisher"); 1901 } 1902 else if (name.equals("contact")) { 1903 return addContact(); 1904 } 1905 else if (name.equals("description")) { 1906 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.description"); 1907 } 1908 else if (name.equals("useContext")) { 1909 return addUseContext(); 1910 } 1911 else if (name.equals("purpose")) { 1912 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.purpose"); 1913 } 1914 else if (name.equals("code")) { 1915 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 1916 } 1917 else if (name.equals("search")) { 1918 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.search"); 1919 } 1920 else if (name.equals("resource")) { 1921 return addResource(); 1922 } 1923 else 1924 return super.addChild(name); 1925 } 1926 1927 public String fhirType() { 1928 return "CompartmentDefinition"; 1929 1930 } 1931 1932 public CompartmentDefinition copy() { 1933 CompartmentDefinition dst = new CompartmentDefinition(); 1934 copyValues(dst); 1935 return dst; 1936 } 1937 1938 public void copyValues(CompartmentDefinition dst) { 1939 super.copyValues(dst); 1940 dst.url = url == null ? null : url.copy(); 1941 dst.version = version == null ? null : version.copy(); 1942 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 1943 dst.name = name == null ? null : name.copy(); 1944 dst.title = title == null ? null : title.copy(); 1945 dst.status = status == null ? null : status.copy(); 1946 dst.experimental = experimental == null ? null : experimental.copy(); 1947 dst.date = date == null ? null : date.copy(); 1948 dst.publisher = publisher == null ? null : publisher.copy(); 1949 if (contact != null) { 1950 dst.contact = new ArrayList<ContactDetail>(); 1951 for (ContactDetail i : contact) 1952 dst.contact.add(i.copy()); 1953 }; 1954 dst.description = description == null ? null : description.copy(); 1955 if (useContext != null) { 1956 dst.useContext = new ArrayList<UsageContext>(); 1957 for (UsageContext i : useContext) 1958 dst.useContext.add(i.copy()); 1959 }; 1960 dst.purpose = purpose == null ? null : purpose.copy(); 1961 dst.code = code == null ? null : code.copy(); 1962 dst.search = search == null ? null : search.copy(); 1963 if (resource != null) { 1964 dst.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1965 for (CompartmentDefinitionResourceComponent i : resource) 1966 dst.resource.add(i.copy()); 1967 }; 1968 } 1969 1970 protected CompartmentDefinition typedCopy() { 1971 return copy(); 1972 } 1973 1974 @Override 1975 public boolean equalsDeep(Base other_) { 1976 if (!super.equalsDeep(other_)) 1977 return false; 1978 if (!(other_ instanceof CompartmentDefinition)) 1979 return false; 1980 CompartmentDefinition o = (CompartmentDefinition) other_; 1981 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(versionAlgorithm, o.versionAlgorithm, true) 1982 && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) && compareDeep(status, o.status, true) 1983 && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) 1984 && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 1985 && compareDeep(purpose, o.purpose, true) && compareDeep(code, o.code, true) && compareDeep(search, o.search, true) 1986 && compareDeep(resource, o.resource, true); 1987 } 1988 1989 @Override 1990 public boolean equalsShallow(Base other_) { 1991 if (!super.equalsShallow(other_)) 1992 return false; 1993 if (!(other_ instanceof CompartmentDefinition)) 1994 return false; 1995 CompartmentDefinition o = (CompartmentDefinition) other_; 1996 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 1997 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 1998 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 1999 && compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) && compareValues(search, o.search, true) 2000 ; 2001 } 2002 2003 public boolean isEmpty() { 2004 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, version, versionAlgorithm 2005 , name, title, status, experimental, date, publisher, contact, description, useContext 2006 , purpose, code, search, resource); 2007 } 2008 2009 @Override 2010 public ResourceType getResourceType() { 2011 return ResourceType.CompartmentDefinition; 2012 } 2013 2014 /** 2015 * Search parameter: <b>context-quantity</b> 2016 * <p> 2017 * Description: <b>Multiple Resources: 2018 2019* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2020* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2021* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2022* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2023* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2024* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2025* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2026* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2027* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2028* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2029* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2030* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2031* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2032* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2033* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2034* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2035* [Library](library.html): A quantity- or range-valued use context assigned to the library 2036* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2037* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2038* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2039* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2040* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2041* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2042* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2043* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2044* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2045* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2046* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2047* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2048* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2049</b><br> 2050 * Type: <b>quantity</b><br> 2051 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2052 * </p> 2053 */ 2054 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2055 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2056 /** 2057 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2058 * <p> 2059 * Description: <b>Multiple Resources: 2060 2061* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2062* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2063* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2064* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2065* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2066* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2067* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2068* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2069* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2070* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2071* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2072* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2073* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2074* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2075* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2076* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2077* [Library](library.html): A quantity- or range-valued use context assigned to the library 2078* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2079* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2080* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2081* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2082* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2083* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2084* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2085* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2086* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2087* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2088* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2089* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2090* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2091</b><br> 2092 * Type: <b>quantity</b><br> 2093 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2094 * </p> 2095 */ 2096 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2097 2098 /** 2099 * Search parameter: <b>context-type-quantity</b> 2100 * <p> 2101 * Description: <b>Multiple Resources: 2102 2103* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2104* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2105* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2106* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2107* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2108* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2109* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2110* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2111* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2112* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2113* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2114* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2115* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2116* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2117* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2118* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2119* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2120* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2121* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2122* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2123* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2124* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2125* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2126* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2127* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2128* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2129* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2130* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2131* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2132* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2133</b><br> 2134 * Type: <b>composite</b><br> 2135 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2136 * </p> 2137 */ 2138 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2139 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2140 /** 2141 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2142 * <p> 2143 * Description: <b>Multiple Resources: 2144 2145* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2146* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2147* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2148* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2149* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2150* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2151* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2152* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2153* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2154* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2155* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2156* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2157* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2158* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2159* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2160* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2161* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2162* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2163* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2164* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2165* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2166* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2167* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2168* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2169* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2170* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2171* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2172* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2173* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2174* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2175</b><br> 2176 * Type: <b>composite</b><br> 2177 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2178 * </p> 2179 */ 2180 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 2181 2182 /** 2183 * Search parameter: <b>context-type-value</b> 2184 * <p> 2185 * Description: <b>Multiple Resources: 2186 2187* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2188* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2189* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2190* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2191* [Citation](citation.html): A use context type and value assigned to the citation 2192* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2193* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2194* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2195* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2196* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2197* [Evidence](evidence.html): A use context type and value assigned to the evidence 2198* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2199* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2200* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2201* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2202* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2203* [Library](library.html): A use context type and value assigned to the library 2204* [Measure](measure.html): A use context type and value assigned to the measure 2205* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2206* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2207* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2208* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2209* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2210* [Requirements](requirements.html): A use context type and value assigned to the requirements 2211* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2212* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2213* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2214* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2215* [TestScript](testscript.html): A use context type and value assigned to the test script 2216* [ValueSet](valueset.html): A use context type and value assigned to the value set 2217</b><br> 2218 * Type: <b>composite</b><br> 2219 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2220 * </p> 2221 */ 2222 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 2223 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2224 /** 2225 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2226 * <p> 2227 * Description: <b>Multiple Resources: 2228 2229* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2230* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2231* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2232* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2233* [Citation](citation.html): A use context type and value assigned to the citation 2234* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2235* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2236* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2237* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2238* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2239* [Evidence](evidence.html): A use context type and value assigned to the evidence 2240* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2241* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2242* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2243* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2244* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2245* [Library](library.html): A use context type and value assigned to the library 2246* [Measure](measure.html): A use context type and value assigned to the measure 2247* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2248* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2249* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2250* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2251* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2252* [Requirements](requirements.html): A use context type and value assigned to the requirements 2253* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2254* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2255* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2256* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2257* [TestScript](testscript.html): A use context type and value assigned to the test script 2258* [ValueSet](valueset.html): A use context type and value assigned to the value set 2259</b><br> 2260 * Type: <b>composite</b><br> 2261 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2262 * </p> 2263 */ 2264 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 2265 2266 /** 2267 * Search parameter: <b>context-type</b> 2268 * <p> 2269 * Description: <b>Multiple Resources: 2270 2271* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2272* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2273* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2274* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2275* [Citation](citation.html): A type of use context assigned to the citation 2276* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2277* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2278* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2279* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2280* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2281* [Evidence](evidence.html): A type of use context assigned to the evidence 2282* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2283* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2284* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2285* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2286* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2287* [Library](library.html): A type of use context assigned to the library 2288* [Measure](measure.html): A type of use context assigned to the measure 2289* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2290* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2291* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2292* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2293* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2294* [Requirements](requirements.html): A type of use context assigned to the requirements 2295* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2296* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2297* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2298* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2299* [TestScript](testscript.html): A type of use context assigned to the test script 2300* [ValueSet](valueset.html): A type of use context assigned to the value set 2301</b><br> 2302 * Type: <b>token</b><br> 2303 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2304 * </p> 2305 */ 2306 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 2307 public static final String SP_CONTEXT_TYPE = "context-type"; 2308 /** 2309 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2310 * <p> 2311 * Description: <b>Multiple Resources: 2312 2313* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2314* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2315* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2316* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2317* [Citation](citation.html): A type of use context assigned to the citation 2318* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2319* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2320* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2321* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2322* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2323* [Evidence](evidence.html): A type of use context assigned to the evidence 2324* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2325* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2326* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2327* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2328* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2329* [Library](library.html): A type of use context assigned to the library 2330* [Measure](measure.html): A type of use context assigned to the measure 2331* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2332* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2333* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2334* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2335* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2336* [Requirements](requirements.html): A type of use context assigned to the requirements 2337* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2338* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2339* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2340* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2341* [TestScript](testscript.html): A type of use context assigned to the test script 2342* [ValueSet](valueset.html): A type of use context assigned to the value set 2343</b><br> 2344 * Type: <b>token</b><br> 2345 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2346 * </p> 2347 */ 2348 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 2349 2350 /** 2351 * Search parameter: <b>context</b> 2352 * <p> 2353 * Description: <b>Multiple Resources: 2354 2355* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 2356* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 2357* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 2358* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 2359* [Citation](citation.html): A use context assigned to the citation 2360* [CodeSystem](codesystem.html): A use context assigned to the code system 2361* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 2362* [ConceptMap](conceptmap.html): A use context assigned to the concept map 2363* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 2364* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 2365* [Evidence](evidence.html): A use context assigned to the evidence 2366* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 2367* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 2368* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 2369* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 2370* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 2371* [Library](library.html): A use context assigned to the library 2372* [Measure](measure.html): A use context assigned to the measure 2373* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 2374* [NamingSystem](namingsystem.html): A use context assigned to the naming system 2375* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 2376* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 2377* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 2378* [Requirements](requirements.html): A use context assigned to the requirements 2379* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 2380* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 2381* [StructureMap](structuremap.html): A use context assigned to the structure map 2382* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 2383* [TestScript](testscript.html): A use context assigned to the test script 2384* [ValueSet](valueset.html): A use context assigned to the value set 2385</b><br> 2386 * Type: <b>token</b><br> 2387 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 2388 * </p> 2389 */ 2390 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 2391 public static final String SP_CONTEXT = "context"; 2392 /** 2393 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2394 * <p> 2395 * Description: <b>Multiple Resources: 2396 2397* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 2398* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 2399* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 2400* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 2401* [Citation](citation.html): A use context assigned to the citation 2402* [CodeSystem](codesystem.html): A use context assigned to the code system 2403* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 2404* [ConceptMap](conceptmap.html): A use context assigned to the concept map 2405* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 2406* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 2407* [Evidence](evidence.html): A use context assigned to the evidence 2408* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 2409* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 2410* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 2411* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 2412* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 2413* [Library](library.html): A use context assigned to the library 2414* [Measure](measure.html): A use context assigned to the measure 2415* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 2416* [NamingSystem](namingsystem.html): A use context assigned to the naming system 2417* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 2418* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 2419* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 2420* [Requirements](requirements.html): A use context assigned to the requirements 2421* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 2422* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 2423* [StructureMap](structuremap.html): A use context assigned to the structure map 2424* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 2425* [TestScript](testscript.html): A use context assigned to the test script 2426* [ValueSet](valueset.html): A use context assigned to the value set 2427</b><br> 2428 * Type: <b>token</b><br> 2429 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 2430 * </p> 2431 */ 2432 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 2433 2434 /** 2435 * Search parameter: <b>date</b> 2436 * <p> 2437 * Description: <b>Multiple Resources: 2438 2439* [ActivityDefinition](activitydefinition.html): The activity definition publication date 2440* [ActorDefinition](actordefinition.html): The Actor Definition publication date 2441* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 2442* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 2443* [Citation](citation.html): The citation publication date 2444* [CodeSystem](codesystem.html): The code system publication date 2445* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 2446* [ConceptMap](conceptmap.html): The concept map publication date 2447* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 2448* [EventDefinition](eventdefinition.html): The event definition publication date 2449* [Evidence](evidence.html): The evidence publication date 2450* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 2451* [ExampleScenario](examplescenario.html): The example scenario publication date 2452* [GraphDefinition](graphdefinition.html): The graph definition publication date 2453* [ImplementationGuide](implementationguide.html): The implementation guide publication date 2454* [Library](library.html): The library publication date 2455* [Measure](measure.html): The measure publication date 2456* [MessageDefinition](messagedefinition.html): The message definition publication date 2457* [NamingSystem](namingsystem.html): The naming system publication date 2458* [OperationDefinition](operationdefinition.html): The operation definition publication date 2459* [PlanDefinition](plandefinition.html): The plan definition publication date 2460* [Questionnaire](questionnaire.html): The questionnaire publication date 2461* [Requirements](requirements.html): The requirements publication date 2462* [SearchParameter](searchparameter.html): The search parameter publication date 2463* [StructureDefinition](structuredefinition.html): The structure definition publication date 2464* [StructureMap](structuremap.html): The structure map publication date 2465* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 2466* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 2467* [TestScript](testscript.html): The test script publication date 2468* [ValueSet](valueset.html): The value set publication date 2469</b><br> 2470 * Type: <b>date</b><br> 2471 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 2472 * </p> 2473 */ 2474 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 2475 public static final String SP_DATE = "date"; 2476 /** 2477 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2478 * <p> 2479 * Description: <b>Multiple Resources: 2480 2481* [ActivityDefinition](activitydefinition.html): The activity definition publication date 2482* [ActorDefinition](actordefinition.html): The Actor Definition publication date 2483* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 2484* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 2485* [Citation](citation.html): The citation publication date 2486* [CodeSystem](codesystem.html): The code system publication date 2487* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 2488* [ConceptMap](conceptmap.html): The concept map publication date 2489* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 2490* [EventDefinition](eventdefinition.html): The event definition publication date 2491* [Evidence](evidence.html): The evidence publication date 2492* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 2493* [ExampleScenario](examplescenario.html): The example scenario publication date 2494* [GraphDefinition](graphdefinition.html): The graph definition publication date 2495* [ImplementationGuide](implementationguide.html): The implementation guide publication date 2496* [Library](library.html): The library publication date 2497* [Measure](measure.html): The measure publication date 2498* [MessageDefinition](messagedefinition.html): The message definition publication date 2499* [NamingSystem](namingsystem.html): The naming system publication date 2500* [OperationDefinition](operationdefinition.html): The operation definition publication date 2501* [PlanDefinition](plandefinition.html): The plan definition publication date 2502* [Questionnaire](questionnaire.html): The questionnaire publication date 2503* [Requirements](requirements.html): The requirements publication date 2504* [SearchParameter](searchparameter.html): The search parameter publication date 2505* [StructureDefinition](structuredefinition.html): The structure definition publication date 2506* [StructureMap](structuremap.html): The structure map publication date 2507* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 2508* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 2509* [TestScript](testscript.html): The test script publication date 2510* [ValueSet](valueset.html): The value set publication date 2511</b><br> 2512 * Type: <b>date</b><br> 2513 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 2514 * </p> 2515 */ 2516 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2517 2518 /** 2519 * Search parameter: <b>description</b> 2520 * <p> 2521 * Description: <b>Multiple Resources: 2522 2523* [ActivityDefinition](activitydefinition.html): The description of the activity definition 2524* [ActorDefinition](actordefinition.html): The description of the Actor Definition 2525* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 2526* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 2527* [Citation](citation.html): The description of the citation 2528* [CodeSystem](codesystem.html): The description of the code system 2529* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 2530* [ConceptMap](conceptmap.html): The description of the concept map 2531* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 2532* [EventDefinition](eventdefinition.html): The description of the event definition 2533* [Evidence](evidence.html): The description of the evidence 2534* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 2535* [GraphDefinition](graphdefinition.html): The description of the graph definition 2536* [ImplementationGuide](implementationguide.html): The description of the implementation guide 2537* [Library](library.html): The description of the library 2538* [Measure](measure.html): The description of the measure 2539* [MessageDefinition](messagedefinition.html): The description of the message definition 2540* [NamingSystem](namingsystem.html): The description of the naming system 2541* [OperationDefinition](operationdefinition.html): The description of the operation definition 2542* [PlanDefinition](plandefinition.html): The description of the plan definition 2543* [Questionnaire](questionnaire.html): The description of the questionnaire 2544* [Requirements](requirements.html): The description of the requirements 2545* [SearchParameter](searchparameter.html): The description of the search parameter 2546* [StructureDefinition](structuredefinition.html): The description of the structure definition 2547* [StructureMap](structuremap.html): The description of the structure map 2548* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 2549* [TestScript](testscript.html): The description of the test script 2550* [ValueSet](valueset.html): The description of the value set 2551</b><br> 2552 * Type: <b>string</b><br> 2553 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 2554 * </p> 2555 */ 2556 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 2557 public static final String SP_DESCRIPTION = "description"; 2558 /** 2559 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2560 * <p> 2561 * Description: <b>Multiple Resources: 2562 2563* [ActivityDefinition](activitydefinition.html): The description of the activity definition 2564* [ActorDefinition](actordefinition.html): The description of the Actor Definition 2565* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 2566* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 2567* [Citation](citation.html): The description of the citation 2568* [CodeSystem](codesystem.html): The description of the code system 2569* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 2570* [ConceptMap](conceptmap.html): The description of the concept map 2571* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 2572* [EventDefinition](eventdefinition.html): The description of the event definition 2573* [Evidence](evidence.html): The description of the evidence 2574* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 2575* [GraphDefinition](graphdefinition.html): The description of the graph definition 2576* [ImplementationGuide](implementationguide.html): The description of the implementation guide 2577* [Library](library.html): The description of the library 2578* [Measure](measure.html): The description of the measure 2579* [MessageDefinition](messagedefinition.html): The description of the message definition 2580* [NamingSystem](namingsystem.html): The description of the naming system 2581* [OperationDefinition](operationdefinition.html): The description of the operation definition 2582* [PlanDefinition](plandefinition.html): The description of the plan definition 2583* [Questionnaire](questionnaire.html): The description of the questionnaire 2584* [Requirements](requirements.html): The description of the requirements 2585* [SearchParameter](searchparameter.html): The description of the search parameter 2586* [StructureDefinition](structuredefinition.html): The description of the structure definition 2587* [StructureMap](structuremap.html): The description of the structure map 2588* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 2589* [TestScript](testscript.html): The description of the test script 2590* [ValueSet](valueset.html): The description of the value set 2591</b><br> 2592 * Type: <b>string</b><br> 2593 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 2594 * </p> 2595 */ 2596 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2597 2598 /** 2599 * Search parameter: <b>name</b> 2600 * <p> 2601 * Description: <b>Multiple Resources: 2602 2603* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 2604* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 2605* [Citation](citation.html): Computationally friendly name of the citation 2606* [CodeSystem](codesystem.html): Computationally friendly name of the code system 2607* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 2608* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 2609* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 2610* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 2611* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 2612* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 2613* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 2614* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 2615* [Library](library.html): Computationally friendly name of the library 2616* [Measure](measure.html): Computationally friendly name of the measure 2617* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 2618* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 2619* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 2620* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 2621* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 2622* [Requirements](requirements.html): Computationally friendly name of the requirements 2623* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 2624* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 2625* [StructureMap](structuremap.html): Computationally friendly name of the structure map 2626* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 2627* [TestScript](testscript.html): Computationally friendly name of the test script 2628* [ValueSet](valueset.html): Computationally friendly name of the value set 2629</b><br> 2630 * Type: <b>string</b><br> 2631 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 2632 * </p> 2633 */ 2634 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 2635 public static final String SP_NAME = "name"; 2636 /** 2637 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2638 * <p> 2639 * Description: <b>Multiple Resources: 2640 2641* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 2642* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 2643* [Citation](citation.html): Computationally friendly name of the citation 2644* [CodeSystem](codesystem.html): Computationally friendly name of the code system 2645* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 2646* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 2647* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 2648* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 2649* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 2650* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 2651* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 2652* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 2653* [Library](library.html): Computationally friendly name of the library 2654* [Measure](measure.html): Computationally friendly name of the measure 2655* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 2656* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 2657* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 2658* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 2659* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 2660* [Requirements](requirements.html): Computationally friendly name of the requirements 2661* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 2662* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 2663* [StructureMap](structuremap.html): Computationally friendly name of the structure map 2664* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 2665* [TestScript](testscript.html): Computationally friendly name of the test script 2666* [ValueSet](valueset.html): Computationally friendly name of the value set 2667</b><br> 2668 * Type: <b>string</b><br> 2669 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 2670 * </p> 2671 */ 2672 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2673 2674 /** 2675 * Search parameter: <b>publisher</b> 2676 * <p> 2677 * Description: <b>Multiple Resources: 2678 2679* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 2680* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 2681* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 2682* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 2683* [Citation](citation.html): Name of the publisher of the citation 2684* [CodeSystem](codesystem.html): Name of the publisher of the code system 2685* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 2686* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 2687* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 2688* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 2689* [Evidence](evidence.html): Name of the publisher of the evidence 2690* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 2691* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 2692* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 2693* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 2694* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 2695* [Library](library.html): Name of the publisher of the library 2696* [Measure](measure.html): Name of the publisher of the measure 2697* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 2698* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 2699* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 2700* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 2701* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 2702* [Requirements](requirements.html): Name of the publisher of the requirements 2703* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 2704* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 2705* [StructureMap](structuremap.html): Name of the publisher of the structure map 2706* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 2707* [TestScript](testscript.html): Name of the publisher of the test script 2708* [ValueSet](valueset.html): Name of the publisher of the value set 2709</b><br> 2710 * Type: <b>string</b><br> 2711 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 2712 * </p> 2713 */ 2714 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 2715 public static final String SP_PUBLISHER = "publisher"; 2716 /** 2717 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2718 * <p> 2719 * Description: <b>Multiple Resources: 2720 2721* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 2722* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 2723* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 2724* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 2725* [Citation](citation.html): Name of the publisher of the citation 2726* [CodeSystem](codesystem.html): Name of the publisher of the code system 2727* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 2728* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 2729* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 2730* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 2731* [Evidence](evidence.html): Name of the publisher of the evidence 2732* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 2733* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 2734* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 2735* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 2736* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 2737* [Library](library.html): Name of the publisher of the library 2738* [Measure](measure.html): Name of the publisher of the measure 2739* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 2740* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 2741* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 2742* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 2743* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 2744* [Requirements](requirements.html): Name of the publisher of the requirements 2745* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 2746* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 2747* [StructureMap](structuremap.html): Name of the publisher of the structure map 2748* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 2749* [TestScript](testscript.html): Name of the publisher of the test script 2750* [ValueSet](valueset.html): Name of the publisher of the value set 2751</b><br> 2752 * Type: <b>string</b><br> 2753 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 2754 * </p> 2755 */ 2756 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2757 2758 /** 2759 * Search parameter: <b>status</b> 2760 * <p> 2761 * Description: <b>Multiple Resources: 2762 2763* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 2764* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 2765* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 2766* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 2767* [Citation](citation.html): The current status of the citation 2768* [CodeSystem](codesystem.html): The current status of the code system 2769* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 2770* [ConceptMap](conceptmap.html): The current status of the concept map 2771* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 2772* [EventDefinition](eventdefinition.html): The current status of the event definition 2773* [Evidence](evidence.html): The current status of the evidence 2774* [EvidenceReport](evidencereport.html): The current status of the evidence report 2775* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 2776* [ExampleScenario](examplescenario.html): The current status of the example scenario 2777* [GraphDefinition](graphdefinition.html): The current status of the graph definition 2778* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 2779* [Library](library.html): The current status of the library 2780* [Measure](measure.html): The current status of the measure 2781* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 2782* [MessageDefinition](messagedefinition.html): The current status of the message definition 2783* [NamingSystem](namingsystem.html): The current status of the naming system 2784* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 2785* [OperationDefinition](operationdefinition.html): The current status of the operation definition 2786* [PlanDefinition](plandefinition.html): The current status of the plan definition 2787* [Questionnaire](questionnaire.html): The current status of the questionnaire 2788* [Requirements](requirements.html): The current status of the requirements 2789* [SearchParameter](searchparameter.html): The current status of the search parameter 2790* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 2791* [StructureDefinition](structuredefinition.html): The current status of the structure definition 2792* [StructureMap](structuremap.html): The current status of the structure map 2793* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 2794* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 2795* [TestPlan](testplan.html): The current status of the test plan 2796* [TestScript](testscript.html): The current status of the test script 2797* [ValueSet](valueset.html): The current status of the value set 2798</b><br> 2799 * Type: <b>token</b><br> 2800 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 2801 * </p> 2802 */ 2803 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 2804 public static final String SP_STATUS = "status"; 2805 /** 2806 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2807 * <p> 2808 * Description: <b>Multiple Resources: 2809 2810* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 2811* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 2812* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 2813* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 2814* [Citation](citation.html): The current status of the citation 2815* [CodeSystem](codesystem.html): The current status of the code system 2816* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 2817* [ConceptMap](conceptmap.html): The current status of the concept map 2818* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 2819* [EventDefinition](eventdefinition.html): The current status of the event definition 2820* [Evidence](evidence.html): The current status of the evidence 2821* [EvidenceReport](evidencereport.html): The current status of the evidence report 2822* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 2823* [ExampleScenario](examplescenario.html): The current status of the example scenario 2824* [GraphDefinition](graphdefinition.html): The current status of the graph definition 2825* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 2826* [Library](library.html): The current status of the library 2827* [Measure](measure.html): The current status of the measure 2828* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 2829* [MessageDefinition](messagedefinition.html): The current status of the message definition 2830* [NamingSystem](namingsystem.html): The current status of the naming system 2831* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 2832* [OperationDefinition](operationdefinition.html): The current status of the operation definition 2833* [PlanDefinition](plandefinition.html): The current status of the plan definition 2834* [Questionnaire](questionnaire.html): The current status of the questionnaire 2835* [Requirements](requirements.html): The current status of the requirements 2836* [SearchParameter](searchparameter.html): The current status of the search parameter 2837* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 2838* [StructureDefinition](structuredefinition.html): The current status of the structure definition 2839* [StructureMap](structuremap.html): The current status of the structure map 2840* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 2841* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 2842* [TestPlan](testplan.html): The current status of the test plan 2843* [TestScript](testscript.html): The current status of the test script 2844* [ValueSet](valueset.html): The current status of the value set 2845</b><br> 2846 * Type: <b>token</b><br> 2847 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 2848 * </p> 2849 */ 2850 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2851 2852 /** 2853 * Search parameter: <b>url</b> 2854 * <p> 2855 * Description: <b>Multiple Resources: 2856 2857* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 2858* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 2859* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 2860* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 2861* [Citation](citation.html): The uri that identifies the citation 2862* [CodeSystem](codesystem.html): The uri that identifies the code system 2863* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 2864* [ConceptMap](conceptmap.html): The URI that identifies the concept map 2865* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 2866* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 2867* [Evidence](evidence.html): The uri that identifies the evidence 2868* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 2869* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 2870* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 2871* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 2872* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 2873* [Library](library.html): The uri that identifies the library 2874* [Measure](measure.html): The uri that identifies the measure 2875* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 2876* [NamingSystem](namingsystem.html): The uri that identifies the naming system 2877* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 2878* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 2879* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 2880* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 2881* [Requirements](requirements.html): The uri that identifies the requirements 2882* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 2883* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 2884* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 2885* [StructureMap](structuremap.html): The uri that identifies the structure map 2886* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 2887* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 2888* [TestPlan](testplan.html): The uri that identifies the test plan 2889* [TestScript](testscript.html): The uri that identifies the test script 2890* [ValueSet](valueset.html): The uri that identifies the value set 2891</b><br> 2892 * Type: <b>uri</b><br> 2893 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 2894 * </p> 2895 */ 2896 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 2897 public static final String SP_URL = "url"; 2898 /** 2899 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2900 * <p> 2901 * Description: <b>Multiple Resources: 2902 2903* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 2904* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 2905* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 2906* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 2907* [Citation](citation.html): The uri that identifies the citation 2908* [CodeSystem](codesystem.html): The uri that identifies the code system 2909* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 2910* [ConceptMap](conceptmap.html): The URI that identifies the concept map 2911* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 2912* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 2913* [Evidence](evidence.html): The uri that identifies the evidence 2914* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 2915* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 2916* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 2917* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 2918* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 2919* [Library](library.html): The uri that identifies the library 2920* [Measure](measure.html): The uri that identifies the measure 2921* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 2922* [NamingSystem](namingsystem.html): The uri that identifies the naming system 2923* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 2924* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 2925* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 2926* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 2927* [Requirements](requirements.html): The uri that identifies the requirements 2928* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 2929* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 2930* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 2931* [StructureMap](structuremap.html): The uri that identifies the structure map 2932* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 2933* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 2934* [TestPlan](testplan.html): The uri that identifies the test plan 2935* [TestScript](testscript.html): The uri that identifies the test script 2936* [ValueSet](valueset.html): The uri that identifies the value set 2937</b><br> 2938 * Type: <b>uri</b><br> 2939 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 2940 * </p> 2941 */ 2942 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2943 2944 /** 2945 * Search parameter: <b>version</b> 2946 * <p> 2947 * Description: <b>Multiple Resources: 2948 2949* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 2950* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 2951* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 2952* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 2953* [Citation](citation.html): The business version of the citation 2954* [CodeSystem](codesystem.html): The business version of the code system 2955* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 2956* [ConceptMap](conceptmap.html): The business version of the concept map 2957* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 2958* [EventDefinition](eventdefinition.html): The business version of the event definition 2959* [Evidence](evidence.html): The business version of the evidence 2960* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 2961* [ExampleScenario](examplescenario.html): The business version of the example scenario 2962* [GraphDefinition](graphdefinition.html): The business version of the graph definition 2963* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 2964* [Library](library.html): The business version of the library 2965* [Measure](measure.html): The business version of the measure 2966* [MessageDefinition](messagedefinition.html): The business version of the message definition 2967* [NamingSystem](namingsystem.html): The business version of the naming system 2968* [OperationDefinition](operationdefinition.html): The business version of the operation definition 2969* [PlanDefinition](plandefinition.html): The business version of the plan definition 2970* [Questionnaire](questionnaire.html): The business version of the questionnaire 2971* [Requirements](requirements.html): The business version of the requirements 2972* [SearchParameter](searchparameter.html): The business version of the search parameter 2973* [StructureDefinition](structuredefinition.html): The business version of the structure definition 2974* [StructureMap](structuremap.html): The business version of the structure map 2975* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 2976* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 2977* [TestScript](testscript.html): The business version of the test script 2978* [ValueSet](valueset.html): The business version of the value set 2979</b><br> 2980 * Type: <b>token</b><br> 2981 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 2982 * </p> 2983 */ 2984 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 2985 public static final String SP_VERSION = "version"; 2986 /** 2987 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2988 * <p> 2989 * Description: <b>Multiple Resources: 2990 2991* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 2992* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 2993* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 2994* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 2995* [Citation](citation.html): The business version of the citation 2996* [CodeSystem](codesystem.html): The business version of the code system 2997* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 2998* [ConceptMap](conceptmap.html): The business version of the concept map 2999* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3000* [EventDefinition](eventdefinition.html): The business version of the event definition 3001* [Evidence](evidence.html): The business version of the evidence 3002* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3003* [ExampleScenario](examplescenario.html): The business version of the example scenario 3004* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3005* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3006* [Library](library.html): The business version of the library 3007* [Measure](measure.html): The business version of the measure 3008* [MessageDefinition](messagedefinition.html): The business version of the message definition 3009* [NamingSystem](namingsystem.html): The business version of the naming system 3010* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3011* [PlanDefinition](plandefinition.html): The business version of the plan definition 3012* [Questionnaire](questionnaire.html): The business version of the questionnaire 3013* [Requirements](requirements.html): The business version of the requirements 3014* [SearchParameter](searchparameter.html): The business version of the search parameter 3015* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3016* [StructureMap](structuremap.html): The business version of the structure map 3017* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3018* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3019* [TestScript](testscript.html): The business version of the test script 3020* [ValueSet](valueset.html): The business version of the value set 3021</b><br> 3022 * Type: <b>token</b><br> 3023 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3024 * </p> 3025 */ 3026 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3027 3028 /** 3029 * Search parameter: <b>code</b> 3030 * <p> 3031 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | Device</b><br> 3032 * Type: <b>token</b><br> 3033 * Path: <b>CompartmentDefinition.code</b><br> 3034 * </p> 3035 */ 3036 @SearchParamDefinition(name="code", path="CompartmentDefinition.code", description="Patient | Encounter | RelatedPerson | Practitioner | Device", type="token" ) 3037 public static final String SP_CODE = "code"; 3038 /** 3039 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3040 * <p> 3041 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | Device</b><br> 3042 * Type: <b>token</b><br> 3043 * Path: <b>CompartmentDefinition.code</b><br> 3044 * </p> 3045 */ 3046 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3047 3048 /** 3049 * Search parameter: <b>resource</b> 3050 * <p> 3051 * Description: <b>Name of resource type</b><br> 3052 * Type: <b>token</b><br> 3053 * Path: <b>CompartmentDefinition.resource.code</b><br> 3054 * </p> 3055 */ 3056 @SearchParamDefinition(name="resource", path="CompartmentDefinition.resource.code", description="Name of resource type", type="token" ) 3057 public static final String SP_RESOURCE = "resource"; 3058 /** 3059 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 3060 * <p> 3061 * Description: <b>Name of resource type</b><br> 3062 * Type: <b>token</b><br> 3063 * Path: <b>CompartmentDefinition.resource.code</b><br> 3064 * </p> 3065 */ 3066 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESOURCE); 3067 3068// Manual code (from Configuration.txt): 3069 public boolean supportsCopyright() { 3070 return false; 3071 } 3072 3073// end addition 3074 3075} 3076