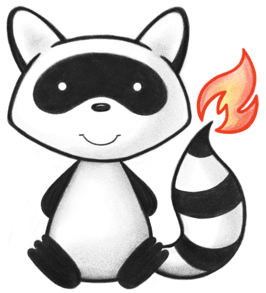
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.). 052 */ 053@ResourceDef(name="Composition", profile="http://hl7.org/fhir/StructureDefinition/Composition") 054public class Composition extends DomainResource { 055 056 @Block() 057 public static class CompositionAttesterComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The type of attestation the authenticator offers. 060 */ 061 @Child(name = "mode", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="personal | professional | legal | official", formalDefinition="The type of attestation the authenticator offers." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-attestation-mode") 064 protected CodeableConcept mode; 065 066 /** 067 * When the composition was attested by the party. 068 */ 069 @Child(name = "time", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="When the composition was attested", formalDefinition="When the composition was attested by the party." ) 071 protected DateTimeType time; 072 073 /** 074 * Who attested the composition in the specified way. 075 */ 076 @Child(name = "party", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 077 @Description(shortDefinition="Who attested the composition", formalDefinition="Who attested the composition in the specified way." ) 078 protected Reference party; 079 080 private static final long serialVersionUID = 545132751L; 081 082 /** 083 * Constructor 084 */ 085 public CompositionAttesterComponent() { 086 super(); 087 } 088 089 /** 090 * Constructor 091 */ 092 public CompositionAttesterComponent(CodeableConcept mode) { 093 super(); 094 this.setMode(mode); 095 } 096 097 /** 098 * @return {@link #mode} (The type of attestation the authenticator offers.) 099 */ 100 public CodeableConcept getMode() { 101 if (this.mode == null) 102 if (Configuration.errorOnAutoCreate()) 103 throw new Error("Attempt to auto-create CompositionAttesterComponent.mode"); 104 else if (Configuration.doAutoCreate()) 105 this.mode = new CodeableConcept(); // cc 106 return this.mode; 107 } 108 109 public boolean hasMode() { 110 return this.mode != null && !this.mode.isEmpty(); 111 } 112 113 /** 114 * @param value {@link #mode} (The type of attestation the authenticator offers.) 115 */ 116 public CompositionAttesterComponent setMode(CodeableConcept value) { 117 this.mode = value; 118 return this; 119 } 120 121 /** 122 * @return {@link #time} (When the composition was attested by the party.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 123 */ 124 public DateTimeType getTimeElement() { 125 if (this.time == null) 126 if (Configuration.errorOnAutoCreate()) 127 throw new Error("Attempt to auto-create CompositionAttesterComponent.time"); 128 else if (Configuration.doAutoCreate()) 129 this.time = new DateTimeType(); // bb 130 return this.time; 131 } 132 133 public boolean hasTimeElement() { 134 return this.time != null && !this.time.isEmpty(); 135 } 136 137 public boolean hasTime() { 138 return this.time != null && !this.time.isEmpty(); 139 } 140 141 /** 142 * @param value {@link #time} (When the composition was attested by the party.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 143 */ 144 public CompositionAttesterComponent setTimeElement(DateTimeType value) { 145 this.time = value; 146 return this; 147 } 148 149 /** 150 * @return When the composition was attested by the party. 151 */ 152 public Date getTime() { 153 return this.time == null ? null : this.time.getValue(); 154 } 155 156 /** 157 * @param value When the composition was attested by the party. 158 */ 159 public CompositionAttesterComponent setTime(Date value) { 160 if (value == null) 161 this.time = null; 162 else { 163 if (this.time == null) 164 this.time = new DateTimeType(); 165 this.time.setValue(value); 166 } 167 return this; 168 } 169 170 /** 171 * @return {@link #party} (Who attested the composition in the specified way.) 172 */ 173 public Reference getParty() { 174 if (this.party == null) 175 if (Configuration.errorOnAutoCreate()) 176 throw new Error("Attempt to auto-create CompositionAttesterComponent.party"); 177 else if (Configuration.doAutoCreate()) 178 this.party = new Reference(); // cc 179 return this.party; 180 } 181 182 public boolean hasParty() { 183 return this.party != null && !this.party.isEmpty(); 184 } 185 186 /** 187 * @param value {@link #party} (Who attested the composition in the specified way.) 188 */ 189 public CompositionAttesterComponent setParty(Reference value) { 190 this.party = value; 191 return this; 192 } 193 194 protected void listChildren(List<Property> children) { 195 super.listChildren(children); 196 children.add(new Property("mode", "CodeableConcept", "The type of attestation the authenticator offers.", 0, 1, mode)); 197 children.add(new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, time)); 198 children.add(new Property("party", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Who attested the composition in the specified way.", 0, 1, party)); 199 } 200 201 @Override 202 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 203 switch (_hash) { 204 case 3357091: /*mode*/ return new Property("mode", "CodeableConcept", "The type of attestation the authenticator offers.", 0, 1, mode); 205 case 3560141: /*time*/ return new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, time); 206 case 106437350: /*party*/ return new Property("party", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Who attested the composition in the specified way.", 0, 1, party); 207 default: return super.getNamedProperty(_hash, _name, _checkValid); 208 } 209 210 } 211 212 @Override 213 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 214 switch (hash) { 215 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeableConcept 216 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DateTimeType 217 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 218 default: return super.getProperty(hash, name, checkValid); 219 } 220 221 } 222 223 @Override 224 public Base setProperty(int hash, String name, Base value) throws FHIRException { 225 switch (hash) { 226 case 3357091: // mode 227 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 228 return value; 229 case 3560141: // time 230 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 231 return value; 232 case 106437350: // party 233 this.party = TypeConvertor.castToReference(value); // Reference 234 return value; 235 default: return super.setProperty(hash, name, value); 236 } 237 238 } 239 240 @Override 241 public Base setProperty(String name, Base value) throws FHIRException { 242 if (name.equals("mode")) { 243 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 244 } else if (name.equals("time")) { 245 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 246 } else if (name.equals("party")) { 247 this.party = TypeConvertor.castToReference(value); // Reference 248 } else 249 return super.setProperty(name, value); 250 return value; 251 } 252 253 @Override 254 public void removeChild(String name, Base value) throws FHIRException { 255 if (name.equals("mode")) { 256 this.mode = null; 257 } else if (name.equals("time")) { 258 this.time = null; 259 } else if (name.equals("party")) { 260 this.party = null; 261 } else 262 super.removeChild(name, value); 263 264 } 265 266 @Override 267 public Base makeProperty(int hash, String name) throws FHIRException { 268 switch (hash) { 269 case 3357091: return getMode(); 270 case 3560141: return getTimeElement(); 271 case 106437350: return getParty(); 272 default: return super.makeProperty(hash, name); 273 } 274 275 } 276 277 @Override 278 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 279 switch (hash) { 280 case 3357091: /*mode*/ return new String[] {"CodeableConcept"}; 281 case 3560141: /*time*/ return new String[] {"dateTime"}; 282 case 106437350: /*party*/ return new String[] {"Reference"}; 283 default: return super.getTypesForProperty(hash, name); 284 } 285 286 } 287 288 @Override 289 public Base addChild(String name) throws FHIRException { 290 if (name.equals("mode")) { 291 this.mode = new CodeableConcept(); 292 return this.mode; 293 } 294 else if (name.equals("time")) { 295 throw new FHIRException("Cannot call addChild on a singleton property Composition.attester.time"); 296 } 297 else if (name.equals("party")) { 298 this.party = new Reference(); 299 return this.party; 300 } 301 else 302 return super.addChild(name); 303 } 304 305 public CompositionAttesterComponent copy() { 306 CompositionAttesterComponent dst = new CompositionAttesterComponent(); 307 copyValues(dst); 308 return dst; 309 } 310 311 public void copyValues(CompositionAttesterComponent dst) { 312 super.copyValues(dst); 313 dst.mode = mode == null ? null : mode.copy(); 314 dst.time = time == null ? null : time.copy(); 315 dst.party = party == null ? null : party.copy(); 316 } 317 318 @Override 319 public boolean equalsDeep(Base other_) { 320 if (!super.equalsDeep(other_)) 321 return false; 322 if (!(other_ instanceof CompositionAttesterComponent)) 323 return false; 324 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 325 return compareDeep(mode, o.mode, true) && compareDeep(time, o.time, true) && compareDeep(party, o.party, true) 326 ; 327 } 328 329 @Override 330 public boolean equalsShallow(Base other_) { 331 if (!super.equalsShallow(other_)) 332 return false; 333 if (!(other_ instanceof CompositionAttesterComponent)) 334 return false; 335 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 336 return compareValues(time, o.time, true); 337 } 338 339 public boolean isEmpty() { 340 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, time, party); 341 } 342 343 public String fhirType() { 344 return "Composition.attester"; 345 346 } 347 348 } 349 350 @Block() 351 public static class CompositionEventComponent extends BackboneElement implements IBaseBackboneElement { 352 /** 353 * The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time. 354 */ 355 @Child(name = "period", type = {Period.class}, order=1, min=0, max=1, modifier=false, summary=true) 356 @Description(shortDefinition="The period covered by the documentation", formalDefinition="The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time." ) 357 protected Period period; 358 359 /** 360 * Represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a "History and Physical Report" in which case the procedure being documented is necessarily a "History and Physical" act. The events may be included as a code or as a reference to an other resource. 361 */ 362 @Child(name = "detail", type = {CodeableReference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 363 @Description(shortDefinition="The event(s) being documented, as code(s), reference(s), or both", formalDefinition="Represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which case the procedure being documented is necessarily a \"History and Physical\" act. The events may be included as a code or as a reference to an other resource." ) 364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActCode") 365 protected List<CodeableReference> detail; 366 367 private static final long serialVersionUID = -2108044093L; 368 369 /** 370 * Constructor 371 */ 372 public CompositionEventComponent() { 373 super(); 374 } 375 376 /** 377 * @return {@link #period} (The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.) 378 */ 379 public Period getPeriod() { 380 if (this.period == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create CompositionEventComponent.period"); 383 else if (Configuration.doAutoCreate()) 384 this.period = new Period(); // cc 385 return this.period; 386 } 387 388 public boolean hasPeriod() { 389 return this.period != null && !this.period.isEmpty(); 390 } 391 392 /** 393 * @param value {@link #period} (The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.) 394 */ 395 public CompositionEventComponent setPeriod(Period value) { 396 this.period = value; 397 return this; 398 } 399 400 /** 401 * @return {@link #detail} (Represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a "History and Physical Report" in which case the procedure being documented is necessarily a "History and Physical" act. The events may be included as a code or as a reference to an other resource.) 402 */ 403 public List<CodeableReference> getDetail() { 404 if (this.detail == null) 405 this.detail = new ArrayList<CodeableReference>(); 406 return this.detail; 407 } 408 409 /** 410 * @return Returns a reference to <code>this</code> for easy method chaining 411 */ 412 public CompositionEventComponent setDetail(List<CodeableReference> theDetail) { 413 this.detail = theDetail; 414 return this; 415 } 416 417 public boolean hasDetail() { 418 if (this.detail == null) 419 return false; 420 for (CodeableReference item : this.detail) 421 if (!item.isEmpty()) 422 return true; 423 return false; 424 } 425 426 public CodeableReference addDetail() { //3 427 CodeableReference t = new CodeableReference(); 428 if (this.detail == null) 429 this.detail = new ArrayList<CodeableReference>(); 430 this.detail.add(t); 431 return t; 432 } 433 434 public CompositionEventComponent addDetail(CodeableReference t) { //3 435 if (t == null) 436 return this; 437 if (this.detail == null) 438 this.detail = new ArrayList<CodeableReference>(); 439 this.detail.add(t); 440 return this; 441 } 442 443 /** 444 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 445 */ 446 public CodeableReference getDetailFirstRep() { 447 if (getDetail().isEmpty()) { 448 addDetail(); 449 } 450 return getDetail().get(0); 451 } 452 453 protected void listChildren(List<Property> children) { 454 super.listChildren(children); 455 children.add(new Property("period", "Period", "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 0, 1, period)); 456 children.add(new Property("detail", "CodeableReference(Any)", "Represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which case the procedure being documented is necessarily a \"History and Physical\" act. The events may be included as a code or as a reference to an other resource.", 0, java.lang.Integer.MAX_VALUE, detail)); 457 } 458 459 @Override 460 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 461 switch (_hash) { 462 case -991726143: /*period*/ return new Property("period", "Period", "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 0, 1, period); 463 case -1335224239: /*detail*/ return new Property("detail", "CodeableReference(Any)", "Represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which case the procedure being documented is necessarily a \"History and Physical\" act. The events may be included as a code or as a reference to an other resource.", 0, java.lang.Integer.MAX_VALUE, detail); 464 default: return super.getNamedProperty(_hash, _name, _checkValid); 465 } 466 467 } 468 469 @Override 470 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 471 switch (hash) { 472 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 473 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // CodeableReference 474 default: return super.getProperty(hash, name, checkValid); 475 } 476 477 } 478 479 @Override 480 public Base setProperty(int hash, String name, Base value) throws FHIRException { 481 switch (hash) { 482 case -991726143: // period 483 this.period = TypeConvertor.castToPeriod(value); // Period 484 return value; 485 case -1335224239: // detail 486 this.getDetail().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 487 return value; 488 default: return super.setProperty(hash, name, value); 489 } 490 491 } 492 493 @Override 494 public Base setProperty(String name, Base value) throws FHIRException { 495 if (name.equals("period")) { 496 this.period = TypeConvertor.castToPeriod(value); // Period 497 } else if (name.equals("detail")) { 498 this.getDetail().add(TypeConvertor.castToCodeableReference(value)); 499 } else 500 return super.setProperty(name, value); 501 return value; 502 } 503 504 @Override 505 public void removeChild(String name, Base value) throws FHIRException { 506 if (name.equals("period")) { 507 this.period = null; 508 } else if (name.equals("detail")) { 509 this.getDetail().remove(value); 510 } else 511 super.removeChild(name, value); 512 513 } 514 515 @Override 516 public Base makeProperty(int hash, String name) throws FHIRException { 517 switch (hash) { 518 case -991726143: return getPeriod(); 519 case -1335224239: return addDetail(); 520 default: return super.makeProperty(hash, name); 521 } 522 523 } 524 525 @Override 526 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 527 switch (hash) { 528 case -991726143: /*period*/ return new String[] {"Period"}; 529 case -1335224239: /*detail*/ return new String[] {"CodeableReference"}; 530 default: return super.getTypesForProperty(hash, name); 531 } 532 533 } 534 535 @Override 536 public Base addChild(String name) throws FHIRException { 537 if (name.equals("period")) { 538 this.period = new Period(); 539 return this.period; 540 } 541 else if (name.equals("detail")) { 542 return addDetail(); 543 } 544 else 545 return super.addChild(name); 546 } 547 548 public CompositionEventComponent copy() { 549 CompositionEventComponent dst = new CompositionEventComponent(); 550 copyValues(dst); 551 return dst; 552 } 553 554 public void copyValues(CompositionEventComponent dst) { 555 super.copyValues(dst); 556 dst.period = period == null ? null : period.copy(); 557 if (detail != null) { 558 dst.detail = new ArrayList<CodeableReference>(); 559 for (CodeableReference i : detail) 560 dst.detail.add(i.copy()); 561 }; 562 } 563 564 @Override 565 public boolean equalsDeep(Base other_) { 566 if (!super.equalsDeep(other_)) 567 return false; 568 if (!(other_ instanceof CompositionEventComponent)) 569 return false; 570 CompositionEventComponent o = (CompositionEventComponent) other_; 571 return compareDeep(period, o.period, true) && compareDeep(detail, o.detail, true); 572 } 573 574 @Override 575 public boolean equalsShallow(Base other_) { 576 if (!super.equalsShallow(other_)) 577 return false; 578 if (!(other_ instanceof CompositionEventComponent)) 579 return false; 580 CompositionEventComponent o = (CompositionEventComponent) other_; 581 return true; 582 } 583 584 public boolean isEmpty() { 585 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(period, detail); 586 } 587 588 public String fhirType() { 589 return "Composition.event"; 590 591 } 592 593 } 594 595 @Block() 596 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 597 /** 598 * The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 599 */ 600 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 601 @Description(shortDefinition="Label for section (e.g. for ToC)", formalDefinition="The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents." ) 602 protected StringType title; 603 604 /** 605 * A code identifying the kind of content contained within the section. This must be consistent with the section title. 606 */ 607 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 608 @Description(shortDefinition="Classification of section (recommended)", formalDefinition="A code identifying the kind of content contained within the section. This must be consistent with the section title." ) 609 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/doc-section-codes") 610 protected CodeableConcept code; 611 612 /** 613 * Identifies who is responsible for the information in this section, not necessarily who typed it in. 614 */ 615 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Device.class, Patient.class, RelatedPerson.class, Organization.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 616 @Description(shortDefinition="Who and/or what authored the section", formalDefinition="Identifies who is responsible for the information in this section, not necessarily who typed it in." ) 617 protected List<Reference> author; 618 619 /** 620 * The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources). 621 */ 622 @Child(name = "focus", type = {Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 623 @Description(shortDefinition="Who/what the section is about, when it is not about the subject of composition", formalDefinition="The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources)." ) 624 protected Reference focus; 625 626 /** 627 * A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. 628 */ 629 @Child(name = "text", type = {Narrative.class}, order=5, min=0, max=1, modifier=false, summary=false) 630 @Description(shortDefinition="Text summary of the section, for human interpretation", formalDefinition="A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative." ) 631 protected Narrative text; 632 633 /** 634 * Specifies the order applied to the items in the section entries. 635 */ 636 @Child(name = "orderedBy", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 637 @Description(shortDefinition="Order of section entries", formalDefinition="Specifies the order applied to the items in the section entries." ) 638 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-order") 639 protected CodeableConcept orderedBy; 640 641 /** 642 * A reference to the actual resource from which the narrative in the section is derived. 643 */ 644 @Child(name = "entry", type = {Reference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 645 @Description(shortDefinition="A reference to data that supports this section", formalDefinition="A reference to the actual resource from which the narrative in the section is derived." ) 646 protected List<Reference> entry; 647 648 /** 649 * If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason. 650 */ 651 @Child(name = "emptyReason", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 652 @Description(shortDefinition="Why the section is empty", formalDefinition="If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason." ) 653 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-empty-reason") 654 protected CodeableConcept emptyReason; 655 656 /** 657 * A nested sub-section within this section. 658 */ 659 @Child(name = "section", type = {SectionComponent.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 660 @Description(shortDefinition="Nested Section", formalDefinition="A nested sub-section within this section." ) 661 protected List<SectionComponent> section; 662 663 private static final long serialVersionUID = 902802086L; 664 665 /** 666 * Constructor 667 */ 668 public SectionComponent() { 669 super(); 670 } 671 672 /** 673 * @return {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 674 */ 675 public StringType getTitleElement() { 676 if (this.title == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create SectionComponent.title"); 679 else if (Configuration.doAutoCreate()) 680 this.title = new StringType(); // bb 681 return this.title; 682 } 683 684 public boolean hasTitleElement() { 685 return this.title != null && !this.title.isEmpty(); 686 } 687 688 public boolean hasTitle() { 689 return this.title != null && !this.title.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 694 */ 695 public SectionComponent setTitleElement(StringType value) { 696 this.title = value; 697 return this; 698 } 699 700 /** 701 * @return The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 702 */ 703 public String getTitle() { 704 return this.title == null ? null : this.title.getValue(); 705 } 706 707 /** 708 * @param value The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 709 */ 710 public SectionComponent setTitle(String value) { 711 if (Utilities.noString(value)) 712 this.title = null; 713 else { 714 if (this.title == null) 715 this.title = new StringType(); 716 this.title.setValue(value); 717 } 718 return this; 719 } 720 721 /** 722 * @return {@link #code} (A code identifying the kind of content contained within the section. This must be consistent with the section title.) 723 */ 724 public CodeableConcept getCode() { 725 if (this.code == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create SectionComponent.code"); 728 else if (Configuration.doAutoCreate()) 729 this.code = new CodeableConcept(); // cc 730 return this.code; 731 } 732 733 public boolean hasCode() { 734 return this.code != null && !this.code.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #code} (A code identifying the kind of content contained within the section. This must be consistent with the section title.) 739 */ 740 public SectionComponent setCode(CodeableConcept value) { 741 this.code = value; 742 return this; 743 } 744 745 /** 746 * @return {@link #author} (Identifies who is responsible for the information in this section, not necessarily who typed it in.) 747 */ 748 public List<Reference> getAuthor() { 749 if (this.author == null) 750 this.author = new ArrayList<Reference>(); 751 return this.author; 752 } 753 754 /** 755 * @return Returns a reference to <code>this</code> for easy method chaining 756 */ 757 public SectionComponent setAuthor(List<Reference> theAuthor) { 758 this.author = theAuthor; 759 return this; 760 } 761 762 public boolean hasAuthor() { 763 if (this.author == null) 764 return false; 765 for (Reference item : this.author) 766 if (!item.isEmpty()) 767 return true; 768 return false; 769 } 770 771 public Reference addAuthor() { //3 772 Reference t = new Reference(); 773 if (this.author == null) 774 this.author = new ArrayList<Reference>(); 775 this.author.add(t); 776 return t; 777 } 778 779 public SectionComponent addAuthor(Reference t) { //3 780 if (t == null) 781 return this; 782 if (this.author == null) 783 this.author = new ArrayList<Reference>(); 784 this.author.add(t); 785 return this; 786 } 787 788 /** 789 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 790 */ 791 public Reference getAuthorFirstRep() { 792 if (getAuthor().isEmpty()) { 793 addAuthor(); 794 } 795 return getAuthor().get(0); 796 } 797 798 /** 799 * @return {@link #focus} (The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).) 800 */ 801 public Reference getFocus() { 802 if (this.focus == null) 803 if (Configuration.errorOnAutoCreate()) 804 throw new Error("Attempt to auto-create SectionComponent.focus"); 805 else if (Configuration.doAutoCreate()) 806 this.focus = new Reference(); // cc 807 return this.focus; 808 } 809 810 public boolean hasFocus() { 811 return this.focus != null && !this.focus.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #focus} (The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).) 816 */ 817 public SectionComponent setFocus(Reference value) { 818 this.focus = value; 819 return this; 820 } 821 822 /** 823 * @return {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative.) 824 */ 825 public Narrative getText() { 826 if (this.text == null) 827 if (Configuration.errorOnAutoCreate()) 828 throw new Error("Attempt to auto-create SectionComponent.text"); 829 else if (Configuration.doAutoCreate()) 830 this.text = new Narrative(); // cc 831 return this.text; 832 } 833 834 public boolean hasText() { 835 return this.text != null && !this.text.isEmpty(); 836 } 837 838 /** 839 * @param value {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative.) 840 */ 841 public SectionComponent setText(Narrative value) { 842 this.text = value; 843 return this; 844 } 845 846 /** 847 * @return {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 848 */ 849 public CodeableConcept getOrderedBy() { 850 if (this.orderedBy == null) 851 if (Configuration.errorOnAutoCreate()) 852 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 853 else if (Configuration.doAutoCreate()) 854 this.orderedBy = new CodeableConcept(); // cc 855 return this.orderedBy; 856 } 857 858 public boolean hasOrderedBy() { 859 return this.orderedBy != null && !this.orderedBy.isEmpty(); 860 } 861 862 /** 863 * @param value {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 864 */ 865 public SectionComponent setOrderedBy(CodeableConcept value) { 866 this.orderedBy = value; 867 return this; 868 } 869 870 /** 871 * @return {@link #entry} (A reference to the actual resource from which the narrative in the section is derived.) 872 */ 873 public List<Reference> getEntry() { 874 if (this.entry == null) 875 this.entry = new ArrayList<Reference>(); 876 return this.entry; 877 } 878 879 /** 880 * @return Returns a reference to <code>this</code> for easy method chaining 881 */ 882 public SectionComponent setEntry(List<Reference> theEntry) { 883 this.entry = theEntry; 884 return this; 885 } 886 887 public boolean hasEntry() { 888 if (this.entry == null) 889 return false; 890 for (Reference item : this.entry) 891 if (!item.isEmpty()) 892 return true; 893 return false; 894 } 895 896 public Reference addEntry() { //3 897 Reference t = new Reference(); 898 if (this.entry == null) 899 this.entry = new ArrayList<Reference>(); 900 this.entry.add(t); 901 return t; 902 } 903 904 public SectionComponent addEntry(Reference t) { //3 905 if (t == null) 906 return this; 907 if (this.entry == null) 908 this.entry = new ArrayList<Reference>(); 909 this.entry.add(t); 910 return this; 911 } 912 913 /** 914 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist {3} 915 */ 916 public Reference getEntryFirstRep() { 917 if (getEntry().isEmpty()) { 918 addEntry(); 919 } 920 return getEntry().get(0); 921 } 922 923 /** 924 * @return {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 925 */ 926 public CodeableConcept getEmptyReason() { 927 if (this.emptyReason == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 930 else if (Configuration.doAutoCreate()) 931 this.emptyReason = new CodeableConcept(); // cc 932 return this.emptyReason; 933 } 934 935 public boolean hasEmptyReason() { 936 return this.emptyReason != null && !this.emptyReason.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 941 */ 942 public SectionComponent setEmptyReason(CodeableConcept value) { 943 this.emptyReason = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #section} (A nested sub-section within this section.) 949 */ 950 public List<SectionComponent> getSection() { 951 if (this.section == null) 952 this.section = new ArrayList<SectionComponent>(); 953 return this.section; 954 } 955 956 /** 957 * @return Returns a reference to <code>this</code> for easy method chaining 958 */ 959 public SectionComponent setSection(List<SectionComponent> theSection) { 960 this.section = theSection; 961 return this; 962 } 963 964 public boolean hasSection() { 965 if (this.section == null) 966 return false; 967 for (SectionComponent item : this.section) 968 if (!item.isEmpty()) 969 return true; 970 return false; 971 } 972 973 public SectionComponent addSection() { //3 974 SectionComponent t = new SectionComponent(); 975 if (this.section == null) 976 this.section = new ArrayList<SectionComponent>(); 977 this.section.add(t); 978 return t; 979 } 980 981 public SectionComponent addSection(SectionComponent t) { //3 982 if (t == null) 983 return this; 984 if (this.section == null) 985 this.section = new ArrayList<SectionComponent>(); 986 this.section.add(t); 987 return this; 988 } 989 990 /** 991 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist {3} 992 */ 993 public SectionComponent getSectionFirstRep() { 994 if (getSection().isEmpty()) { 995 addSection(); 996 } 997 return getSection().get(0); 998 } 999 1000 protected void listChildren(List<Property> children) { 1001 super.listChildren(children); 1002 children.add(new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title)); 1003 children.add(new Property("code", "CodeableConcept", "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 0, 1, code)); 1004 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author)); 1005 children.add(new Property("focus", "Reference(Any)", "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).", 0, 1, focus)); 1006 children.add(new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 0, 1, text)); 1007 children.add(new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy)); 1008 children.add(new Property("entry", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entry)); 1009 children.add(new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason)); 1010 children.add(new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section)); 1011 } 1012 1013 @Override 1014 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1015 switch (_hash) { 1016 case 110371416: /*title*/ return new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title); 1017 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 0, 1, code); 1018 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author); 1019 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).", 0, 1, focus); 1020 case 3556653: /*text*/ return new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 0, 1, text); 1021 case -391079516: /*orderedBy*/ return new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy); 1022 case 96667762: /*entry*/ return new Property("entry", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entry); 1023 case 1140135409: /*emptyReason*/ return new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason); 1024 case 1970241253: /*section*/ return new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section); 1025 default: return super.getNamedProperty(_hash, _name, _checkValid); 1026 } 1027 1028 } 1029 1030 @Override 1031 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1032 switch (hash) { 1033 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1034 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1035 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 1036 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // Reference 1037 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // Narrative 1038 case -391079516: /*orderedBy*/ return this.orderedBy == null ? new Base[0] : new Base[] {this.orderedBy}; // CodeableConcept 1039 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // Reference 1040 case 1140135409: /*emptyReason*/ return this.emptyReason == null ? new Base[0] : new Base[] {this.emptyReason}; // CodeableConcept 1041 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 1042 default: return super.getProperty(hash, name, checkValid); 1043 } 1044 1045 } 1046 1047 @Override 1048 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1049 switch (hash) { 1050 case 110371416: // title 1051 this.title = TypeConvertor.castToString(value); // StringType 1052 return value; 1053 case 3059181: // code 1054 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1055 return value; 1056 case -1406328437: // author 1057 this.getAuthor().add(TypeConvertor.castToReference(value)); // Reference 1058 return value; 1059 case 97604824: // focus 1060 this.focus = TypeConvertor.castToReference(value); // Reference 1061 return value; 1062 case 3556653: // text 1063 this.text = TypeConvertor.castToNarrative(value); // Narrative 1064 return value; 1065 case -391079516: // orderedBy 1066 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1067 return value; 1068 case 96667762: // entry 1069 this.getEntry().add(TypeConvertor.castToReference(value)); // Reference 1070 return value; 1071 case 1140135409: // emptyReason 1072 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1073 return value; 1074 case 1970241253: // section 1075 this.getSection().add((SectionComponent) value); // SectionComponent 1076 return value; 1077 default: return super.setProperty(hash, name, value); 1078 } 1079 1080 } 1081 1082 @Override 1083 public Base setProperty(String name, Base value) throws FHIRException { 1084 if (name.equals("title")) { 1085 this.title = TypeConvertor.castToString(value); // StringType 1086 } else if (name.equals("code")) { 1087 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1088 } else if (name.equals("author")) { 1089 this.getAuthor().add(TypeConvertor.castToReference(value)); 1090 } else if (name.equals("focus")) { 1091 this.focus = TypeConvertor.castToReference(value); // Reference 1092 } else if (name.equals("text")) { 1093 this.text = TypeConvertor.castToNarrative(value); // Narrative 1094 } else if (name.equals("orderedBy")) { 1095 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1096 } else if (name.equals("entry")) { 1097 this.getEntry().add(TypeConvertor.castToReference(value)); 1098 } else if (name.equals("emptyReason")) { 1099 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1100 } else if (name.equals("section")) { 1101 this.getSection().add((SectionComponent) value); 1102 } else 1103 return super.setProperty(name, value); 1104 return value; 1105 } 1106 1107 @Override 1108 public void removeChild(String name, Base value) throws FHIRException { 1109 if (name.equals("title")) { 1110 this.title = null; 1111 } else if (name.equals("code")) { 1112 this.code = null; 1113 } else if (name.equals("author")) { 1114 this.getAuthor().remove(value); 1115 } else if (name.equals("focus")) { 1116 this.focus = null; 1117 } else if (name.equals("text")) { 1118 this.text = null; 1119 } else if (name.equals("orderedBy")) { 1120 this.orderedBy = null; 1121 } else if (name.equals("entry")) { 1122 this.getEntry().remove(value); 1123 } else if (name.equals("emptyReason")) { 1124 this.emptyReason = null; 1125 } else if (name.equals("section")) { 1126 this.getSection().remove((SectionComponent) value); 1127 } else 1128 super.removeChild(name, value); 1129 1130 } 1131 1132 @Override 1133 public Base makeProperty(int hash, String name) throws FHIRException { 1134 switch (hash) { 1135 case 110371416: return getTitleElement(); 1136 case 3059181: return getCode(); 1137 case -1406328437: return addAuthor(); 1138 case 97604824: return getFocus(); 1139 case 3556653: return getText(); 1140 case -391079516: return getOrderedBy(); 1141 case 96667762: return addEntry(); 1142 case 1140135409: return getEmptyReason(); 1143 case 1970241253: return addSection(); 1144 default: return super.makeProperty(hash, name); 1145 } 1146 1147 } 1148 1149 @Override 1150 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1151 switch (hash) { 1152 case 110371416: /*title*/ return new String[] {"string"}; 1153 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1154 case -1406328437: /*author*/ return new String[] {"Reference"}; 1155 case 97604824: /*focus*/ return new String[] {"Reference"}; 1156 case 3556653: /*text*/ return new String[] {"Narrative"}; 1157 case -391079516: /*orderedBy*/ return new String[] {"CodeableConcept"}; 1158 case 96667762: /*entry*/ return new String[] {"Reference"}; 1159 case 1140135409: /*emptyReason*/ return new String[] {"CodeableConcept"}; 1160 case 1970241253: /*section*/ return new String[] {"@Composition.section"}; 1161 default: return super.getTypesForProperty(hash, name); 1162 } 1163 1164 } 1165 1166 @Override 1167 public Base addChild(String name) throws FHIRException { 1168 if (name.equals("title")) { 1169 throw new FHIRException("Cannot call addChild on a singleton property Composition.section.title"); 1170 } 1171 else if (name.equals("code")) { 1172 this.code = new CodeableConcept(); 1173 return this.code; 1174 } 1175 else if (name.equals("author")) { 1176 return addAuthor(); 1177 } 1178 else if (name.equals("focus")) { 1179 this.focus = new Reference(); 1180 return this.focus; 1181 } 1182 else if (name.equals("text")) { 1183 this.text = new Narrative(); 1184 return this.text; 1185 } 1186 else if (name.equals("orderedBy")) { 1187 this.orderedBy = new CodeableConcept(); 1188 return this.orderedBy; 1189 } 1190 else if (name.equals("entry")) { 1191 return addEntry(); 1192 } 1193 else if (name.equals("emptyReason")) { 1194 this.emptyReason = new CodeableConcept(); 1195 return this.emptyReason; 1196 } 1197 else if (name.equals("section")) { 1198 return addSection(); 1199 } 1200 else 1201 return super.addChild(name); 1202 } 1203 1204 public SectionComponent copy() { 1205 SectionComponent dst = new SectionComponent(); 1206 copyValues(dst); 1207 return dst; 1208 } 1209 1210 public void copyValues(SectionComponent dst) { 1211 super.copyValues(dst); 1212 dst.title = title == null ? null : title.copy(); 1213 dst.code = code == null ? null : code.copy(); 1214 if (author != null) { 1215 dst.author = new ArrayList<Reference>(); 1216 for (Reference i : author) 1217 dst.author.add(i.copy()); 1218 }; 1219 dst.focus = focus == null ? null : focus.copy(); 1220 dst.text = text == null ? null : text.copy(); 1221 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1222 if (entry != null) { 1223 dst.entry = new ArrayList<Reference>(); 1224 for (Reference i : entry) 1225 dst.entry.add(i.copy()); 1226 }; 1227 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1228 if (section != null) { 1229 dst.section = new ArrayList<SectionComponent>(); 1230 for (SectionComponent i : section) 1231 dst.section.add(i.copy()); 1232 }; 1233 } 1234 1235 @Override 1236 public boolean equalsDeep(Base other_) { 1237 if (!super.equalsDeep(other_)) 1238 return false; 1239 if (!(other_ instanceof SectionComponent)) 1240 return false; 1241 SectionComponent o = (SectionComponent) other_; 1242 return compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(author, o.author, true) 1243 && compareDeep(focus, o.focus, true) && compareDeep(text, o.text, true) && compareDeep(orderedBy, o.orderedBy, true) 1244 && compareDeep(entry, o.entry, true) && compareDeep(emptyReason, o.emptyReason, true) && compareDeep(section, o.section, true) 1245 ; 1246 } 1247 1248 @Override 1249 public boolean equalsShallow(Base other_) { 1250 if (!super.equalsShallow(other_)) 1251 return false; 1252 if (!(other_ instanceof SectionComponent)) 1253 return false; 1254 SectionComponent o = (SectionComponent) other_; 1255 return compareValues(title, o.title, true); 1256 } 1257 1258 public boolean isEmpty() { 1259 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, code, author, focus 1260 , text, orderedBy, entry, emptyReason, section); 1261 } 1262 1263 public String fhirType() { 1264 return "Composition.section"; 1265 1266 } 1267 1268 } 1269 1270 /** 1271 * An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers. 1272 */ 1273 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1274 @Description(shortDefinition="Canonical identifier for this Composition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers." ) 1275 protected UriType url; 1276 1277 /** 1278 * A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time. 1279 */ 1280 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1281 @Description(shortDefinition="Version-independent identifier for the Composition", formalDefinition="A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time." ) 1282 protected List<Identifier> identifier; 1283 1284 /** 1285 * An explicitly assigned identifer of a variation of the content in the Composition. 1286 */ 1287 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1288 @Description(shortDefinition="An explicitly assigned identifer of a variation of the content in the Composition", formalDefinition="An explicitly assigned identifer of a variation of the content in the Composition." ) 1289 protected StringType version; 1290 1291 /** 1292 * The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document. 1293 */ 1294 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 1295 @Description(shortDefinition="registered | partial | preliminary | final | amended | corrected | appended | cancelled | entered-in-error | deprecated | unknown", formalDefinition="The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document." ) 1296 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-status") 1297 protected Enumeration<CompositionStatus> status; 1298 1299 /** 1300 * Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition. 1301 */ 1302 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 1303 @Description(shortDefinition="Kind of composition (LOINC if possible)", formalDefinition="Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition." ) 1304 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/doc-typecodes") 1305 protected CodeableConcept type; 1306 1307 /** 1308 * A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type. 1309 */ 1310 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1311 @Description(shortDefinition="Categorization of Composition", formalDefinition="A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type." ) 1312 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referenced-item-category") 1313 protected List<CodeableConcept> category; 1314 1315 /** 1316 * Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure). 1317 */ 1318 @Child(name = "subject", type = {Reference.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1319 @Description(shortDefinition="Who and/or what the composition is about", formalDefinition="Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure)." ) 1320 protected List<Reference> subject; 1321 1322 /** 1323 * Describes the clinical encounter or type of care this documentation is associated with. 1324 */ 1325 @Child(name = "encounter", type = {Encounter.class}, order=7, min=0, max=1, modifier=false, summary=true) 1326 @Description(shortDefinition="Context of the Composition", formalDefinition="Describes the clinical encounter or type of care this documentation is associated with." ) 1327 protected Reference encounter; 1328 1329 /** 1330 * The composition editing time, when the composition was last logically changed by the author. 1331 */ 1332 @Child(name = "date", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 1333 @Description(shortDefinition="Composition editing time", formalDefinition="The composition editing time, when the composition was last logically changed by the author." ) 1334 protected DateTimeType date; 1335 1336 /** 1337 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Composition instances. 1338 */ 1339 @Child(name = "useContext", type = {UsageContext.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1340 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Composition instances." ) 1341 protected List<UsageContext> useContext; 1342 1343 /** 1344 * Identifies who is responsible for the information in the composition, not necessarily who typed it in. 1345 */ 1346 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Device.class, Patient.class, RelatedPerson.class, Organization.class}, order=10, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1347 @Description(shortDefinition="Who and/or what authored the composition", formalDefinition="Identifies who is responsible for the information in the composition, not necessarily who typed it in." ) 1348 protected List<Reference> author; 1349 1350 /** 1351 * A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1352 */ 1353 @Child(name = "name", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1354 @Description(shortDefinition="Name for this Composition (computer friendly)", formalDefinition="A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1355 protected StringType name; 1356 1357 /** 1358 * Official human-readable label for the composition. 1359 */ 1360 @Child(name = "title", type = {StringType.class}, order=12, min=1, max=1, modifier=false, summary=true) 1361 @Description(shortDefinition="Human Readable name/title", formalDefinition="Official human-readable label for the composition." ) 1362 protected StringType title; 1363 1364 /** 1365 * For any additional notes. 1366 */ 1367 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1368 @Description(shortDefinition="For any additional notes", formalDefinition="For any additional notes." ) 1369 protected List<Annotation> note; 1370 1371 /** 1372 * A participant who has attested to the accuracy of the composition/document. 1373 */ 1374 @Child(name = "attester", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1375 @Description(shortDefinition="Attests to accuracy of composition", formalDefinition="A participant who has attested to the accuracy of the composition/document." ) 1376 protected List<CompositionAttesterComponent> attester; 1377 1378 /** 1379 * Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information. 1380 */ 1381 @Child(name = "custodian", type = {Organization.class}, order=15, min=0, max=1, modifier=false, summary=true) 1382 @Description(shortDefinition="Organization which maintains the composition", formalDefinition="Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information." ) 1383 protected Reference custodian; 1384 1385 /** 1386 * Relationships that this composition has with other compositions or documents that already exist. 1387 */ 1388 @Child(name = "relatesTo", type = {RelatedArtifact.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1389 @Description(shortDefinition="Relationships to other compositions/documents", formalDefinition="Relationships that this composition has with other compositions or documents that already exist." ) 1390 protected List<RelatedArtifact> relatesTo; 1391 1392 /** 1393 * The clinical service, such as a colonoscopy or an appendectomy, being documented. 1394 */ 1395 @Child(name = "event", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1396 @Description(shortDefinition="The clinical service(s) being documented", formalDefinition="The clinical service, such as a colonoscopy or an appendectomy, being documented." ) 1397 protected List<CompositionEventComponent> event; 1398 1399 /** 1400 * The root of the sections that make up the composition. 1401 */ 1402 @Child(name = "section", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1403 @Description(shortDefinition="Composition is broken into sections", formalDefinition="The root of the sections that make up the composition." ) 1404 protected List<SectionComponent> section; 1405 1406 private static final long serialVersionUID = 2030933035L; 1407 1408 /** 1409 * Constructor 1410 */ 1411 public Composition() { 1412 super(); 1413 } 1414 1415 /** 1416 * Constructor 1417 */ 1418 public Composition(CompositionStatus status, CodeableConcept type, Date date, Reference author, String title) { 1419 super(); 1420 this.setStatus(status); 1421 this.setType(type); 1422 this.setDate(date); 1423 this.addAuthor(author); 1424 this.setTitle(title); 1425 } 1426 1427 /** 1428 * @return {@link #url} (An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1429 */ 1430 public UriType getUrlElement() { 1431 if (this.url == null) 1432 if (Configuration.errorOnAutoCreate()) 1433 throw new Error("Attempt to auto-create Composition.url"); 1434 else if (Configuration.doAutoCreate()) 1435 this.url = new UriType(); // bb 1436 return this.url; 1437 } 1438 1439 public boolean hasUrlElement() { 1440 return this.url != null && !this.url.isEmpty(); 1441 } 1442 1443 public boolean hasUrl() { 1444 return this.url != null && !this.url.isEmpty(); 1445 } 1446 1447 /** 1448 * @param value {@link #url} (An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1449 */ 1450 public Composition setUrlElement(UriType value) { 1451 this.url = value; 1452 return this; 1453 } 1454 1455 /** 1456 * @return An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers. 1457 */ 1458 public String getUrl() { 1459 return this.url == null ? null : this.url.getValue(); 1460 } 1461 1462 /** 1463 * @param value An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers. 1464 */ 1465 public Composition setUrl(String value) { 1466 if (Utilities.noString(value)) 1467 this.url = null; 1468 else { 1469 if (this.url == null) 1470 this.url = new UriType(); 1471 this.url.setValue(value); 1472 } 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #identifier} (A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.) 1478 */ 1479 public List<Identifier> getIdentifier() { 1480 if (this.identifier == null) 1481 this.identifier = new ArrayList<Identifier>(); 1482 return this.identifier; 1483 } 1484 1485 /** 1486 * @return Returns a reference to <code>this</code> for easy method chaining 1487 */ 1488 public Composition setIdentifier(List<Identifier> theIdentifier) { 1489 this.identifier = theIdentifier; 1490 return this; 1491 } 1492 1493 public boolean hasIdentifier() { 1494 if (this.identifier == null) 1495 return false; 1496 for (Identifier item : this.identifier) 1497 if (!item.isEmpty()) 1498 return true; 1499 return false; 1500 } 1501 1502 public Identifier addIdentifier() { //3 1503 Identifier t = new Identifier(); 1504 if (this.identifier == null) 1505 this.identifier = new ArrayList<Identifier>(); 1506 this.identifier.add(t); 1507 return t; 1508 } 1509 1510 public Composition addIdentifier(Identifier t) { //3 1511 if (t == null) 1512 return this; 1513 if (this.identifier == null) 1514 this.identifier = new ArrayList<Identifier>(); 1515 this.identifier.add(t); 1516 return this; 1517 } 1518 1519 /** 1520 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1521 */ 1522 public Identifier getIdentifierFirstRep() { 1523 if (getIdentifier().isEmpty()) { 1524 addIdentifier(); 1525 } 1526 return getIdentifier().get(0); 1527 } 1528 1529 /** 1530 * @return {@link #version} (An explicitly assigned identifer of a variation of the content in the Composition.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1531 */ 1532 public StringType getVersionElement() { 1533 if (this.version == null) 1534 if (Configuration.errorOnAutoCreate()) 1535 throw new Error("Attempt to auto-create Composition.version"); 1536 else if (Configuration.doAutoCreate()) 1537 this.version = new StringType(); // bb 1538 return this.version; 1539 } 1540 1541 public boolean hasVersionElement() { 1542 return this.version != null && !this.version.isEmpty(); 1543 } 1544 1545 public boolean hasVersion() { 1546 return this.version != null && !this.version.isEmpty(); 1547 } 1548 1549 /** 1550 * @param value {@link #version} (An explicitly assigned identifer of a variation of the content in the Composition.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1551 */ 1552 public Composition setVersionElement(StringType value) { 1553 this.version = value; 1554 return this; 1555 } 1556 1557 /** 1558 * @return An explicitly assigned identifer of a variation of the content in the Composition. 1559 */ 1560 public String getVersion() { 1561 return this.version == null ? null : this.version.getValue(); 1562 } 1563 1564 /** 1565 * @param value An explicitly assigned identifer of a variation of the content in the Composition. 1566 */ 1567 public Composition setVersion(String value) { 1568 if (Utilities.noString(value)) 1569 this.version = null; 1570 else { 1571 if (this.version == null) 1572 this.version = new StringType(); 1573 this.version.setValue(value); 1574 } 1575 return this; 1576 } 1577 1578 /** 1579 * @return {@link #status} (The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1580 */ 1581 public Enumeration<CompositionStatus> getStatusElement() { 1582 if (this.status == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create Composition.status"); 1585 else if (Configuration.doAutoCreate()) 1586 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); // bb 1587 return this.status; 1588 } 1589 1590 public boolean hasStatusElement() { 1591 return this.status != null && !this.status.isEmpty(); 1592 } 1593 1594 public boolean hasStatus() { 1595 return this.status != null && !this.status.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #status} (The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1600 */ 1601 public Composition setStatusElement(Enumeration<CompositionStatus> value) { 1602 this.status = value; 1603 return this; 1604 } 1605 1606 /** 1607 * @return The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document. 1608 */ 1609 public CompositionStatus getStatus() { 1610 return this.status == null ? null : this.status.getValue(); 1611 } 1612 1613 /** 1614 * @param value The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document. 1615 */ 1616 public Composition setStatus(CompositionStatus value) { 1617 if (this.status == null) 1618 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); 1619 this.status.setValue(value); 1620 return this; 1621 } 1622 1623 /** 1624 * @return {@link #type} (Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.) 1625 */ 1626 public CodeableConcept getType() { 1627 if (this.type == null) 1628 if (Configuration.errorOnAutoCreate()) 1629 throw new Error("Attempt to auto-create Composition.type"); 1630 else if (Configuration.doAutoCreate()) 1631 this.type = new CodeableConcept(); // cc 1632 return this.type; 1633 } 1634 1635 public boolean hasType() { 1636 return this.type != null && !this.type.isEmpty(); 1637 } 1638 1639 /** 1640 * @param value {@link #type} (Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.) 1641 */ 1642 public Composition setType(CodeableConcept value) { 1643 this.type = value; 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #category} (A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.) 1649 */ 1650 public List<CodeableConcept> getCategory() { 1651 if (this.category == null) 1652 this.category = new ArrayList<CodeableConcept>(); 1653 return this.category; 1654 } 1655 1656 /** 1657 * @return Returns a reference to <code>this</code> for easy method chaining 1658 */ 1659 public Composition setCategory(List<CodeableConcept> theCategory) { 1660 this.category = theCategory; 1661 return this; 1662 } 1663 1664 public boolean hasCategory() { 1665 if (this.category == null) 1666 return false; 1667 for (CodeableConcept item : this.category) 1668 if (!item.isEmpty()) 1669 return true; 1670 return false; 1671 } 1672 1673 public CodeableConcept addCategory() { //3 1674 CodeableConcept t = new CodeableConcept(); 1675 if (this.category == null) 1676 this.category = new ArrayList<CodeableConcept>(); 1677 this.category.add(t); 1678 return t; 1679 } 1680 1681 public Composition addCategory(CodeableConcept t) { //3 1682 if (t == null) 1683 return this; 1684 if (this.category == null) 1685 this.category = new ArrayList<CodeableConcept>(); 1686 this.category.add(t); 1687 return this; 1688 } 1689 1690 /** 1691 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1692 */ 1693 public CodeableConcept getCategoryFirstRep() { 1694 if (getCategory().isEmpty()) { 1695 addCategory(); 1696 } 1697 return getCategory().get(0); 1698 } 1699 1700 /** 1701 * @return {@link #subject} (Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).) 1702 */ 1703 public List<Reference> getSubject() { 1704 if (this.subject == null) 1705 this.subject = new ArrayList<Reference>(); 1706 return this.subject; 1707 } 1708 1709 /** 1710 * @return Returns a reference to <code>this</code> for easy method chaining 1711 */ 1712 public Composition setSubject(List<Reference> theSubject) { 1713 this.subject = theSubject; 1714 return this; 1715 } 1716 1717 public boolean hasSubject() { 1718 if (this.subject == null) 1719 return false; 1720 for (Reference item : this.subject) 1721 if (!item.isEmpty()) 1722 return true; 1723 return false; 1724 } 1725 1726 public Reference addSubject() { //3 1727 Reference t = new Reference(); 1728 if (this.subject == null) 1729 this.subject = new ArrayList<Reference>(); 1730 this.subject.add(t); 1731 return t; 1732 } 1733 1734 public Composition addSubject(Reference t) { //3 1735 if (t == null) 1736 return this; 1737 if (this.subject == null) 1738 this.subject = new ArrayList<Reference>(); 1739 this.subject.add(t); 1740 return this; 1741 } 1742 1743 /** 1744 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 1745 */ 1746 public Reference getSubjectFirstRep() { 1747 if (getSubject().isEmpty()) { 1748 addSubject(); 1749 } 1750 return getSubject().get(0); 1751 } 1752 1753 /** 1754 * @return {@link #encounter} (Describes the clinical encounter or type of care this documentation is associated with.) 1755 */ 1756 public Reference getEncounter() { 1757 if (this.encounter == null) 1758 if (Configuration.errorOnAutoCreate()) 1759 throw new Error("Attempt to auto-create Composition.encounter"); 1760 else if (Configuration.doAutoCreate()) 1761 this.encounter = new Reference(); // cc 1762 return this.encounter; 1763 } 1764 1765 public boolean hasEncounter() { 1766 return this.encounter != null && !this.encounter.isEmpty(); 1767 } 1768 1769 /** 1770 * @param value {@link #encounter} (Describes the clinical encounter or type of care this documentation is associated with.) 1771 */ 1772 public Composition setEncounter(Reference value) { 1773 this.encounter = value; 1774 return this; 1775 } 1776 1777 /** 1778 * @return {@link #date} (The composition editing time, when the composition was last logically changed by the author.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1779 */ 1780 public DateTimeType getDateElement() { 1781 if (this.date == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create Composition.date"); 1784 else if (Configuration.doAutoCreate()) 1785 this.date = new DateTimeType(); // bb 1786 return this.date; 1787 } 1788 1789 public boolean hasDateElement() { 1790 return this.date != null && !this.date.isEmpty(); 1791 } 1792 1793 public boolean hasDate() { 1794 return this.date != null && !this.date.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #date} (The composition editing time, when the composition was last logically changed by the author.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1799 */ 1800 public Composition setDateElement(DateTimeType value) { 1801 this.date = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return The composition editing time, when the composition was last logically changed by the author. 1807 */ 1808 public Date getDate() { 1809 return this.date == null ? null : this.date.getValue(); 1810 } 1811 1812 /** 1813 * @param value The composition editing time, when the composition was last logically changed by the author. 1814 */ 1815 public Composition setDate(Date value) { 1816 if (this.date == null) 1817 this.date = new DateTimeType(); 1818 this.date.setValue(value); 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Composition instances.) 1824 */ 1825 public List<UsageContext> getUseContext() { 1826 if (this.useContext == null) 1827 this.useContext = new ArrayList<UsageContext>(); 1828 return this.useContext; 1829 } 1830 1831 /** 1832 * @return Returns a reference to <code>this</code> for easy method chaining 1833 */ 1834 public Composition setUseContext(List<UsageContext> theUseContext) { 1835 this.useContext = theUseContext; 1836 return this; 1837 } 1838 1839 public boolean hasUseContext() { 1840 if (this.useContext == null) 1841 return false; 1842 for (UsageContext item : this.useContext) 1843 if (!item.isEmpty()) 1844 return true; 1845 return false; 1846 } 1847 1848 public UsageContext addUseContext() { //3 1849 UsageContext t = new UsageContext(); 1850 if (this.useContext == null) 1851 this.useContext = new ArrayList<UsageContext>(); 1852 this.useContext.add(t); 1853 return t; 1854 } 1855 1856 public Composition addUseContext(UsageContext t) { //3 1857 if (t == null) 1858 return this; 1859 if (this.useContext == null) 1860 this.useContext = new ArrayList<UsageContext>(); 1861 this.useContext.add(t); 1862 return this; 1863 } 1864 1865 /** 1866 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1867 */ 1868 public UsageContext getUseContextFirstRep() { 1869 if (getUseContext().isEmpty()) { 1870 addUseContext(); 1871 } 1872 return getUseContext().get(0); 1873 } 1874 1875 /** 1876 * @return {@link #author} (Identifies who is responsible for the information in the composition, not necessarily who typed it in.) 1877 */ 1878 public List<Reference> getAuthor() { 1879 if (this.author == null) 1880 this.author = new ArrayList<Reference>(); 1881 return this.author; 1882 } 1883 1884 /** 1885 * @return Returns a reference to <code>this</code> for easy method chaining 1886 */ 1887 public Composition setAuthor(List<Reference> theAuthor) { 1888 this.author = theAuthor; 1889 return this; 1890 } 1891 1892 public boolean hasAuthor() { 1893 if (this.author == null) 1894 return false; 1895 for (Reference item : this.author) 1896 if (!item.isEmpty()) 1897 return true; 1898 return false; 1899 } 1900 1901 public Reference addAuthor() { //3 1902 Reference t = new Reference(); 1903 if (this.author == null) 1904 this.author = new ArrayList<Reference>(); 1905 this.author.add(t); 1906 return t; 1907 } 1908 1909 public Composition addAuthor(Reference t) { //3 1910 if (t == null) 1911 return this; 1912 if (this.author == null) 1913 this.author = new ArrayList<Reference>(); 1914 this.author.add(t); 1915 return this; 1916 } 1917 1918 /** 1919 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 1920 */ 1921 public Reference getAuthorFirstRep() { 1922 if (getAuthor().isEmpty()) { 1923 addAuthor(); 1924 } 1925 return getAuthor().get(0); 1926 } 1927 1928 /** 1929 * @return {@link #name} (A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1930 */ 1931 public StringType getNameElement() { 1932 if (this.name == null) 1933 if (Configuration.errorOnAutoCreate()) 1934 throw new Error("Attempt to auto-create Composition.name"); 1935 else if (Configuration.doAutoCreate()) 1936 this.name = new StringType(); // bb 1937 return this.name; 1938 } 1939 1940 public boolean hasNameElement() { 1941 return this.name != null && !this.name.isEmpty(); 1942 } 1943 1944 public boolean hasName() { 1945 return this.name != null && !this.name.isEmpty(); 1946 } 1947 1948 /** 1949 * @param value {@link #name} (A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1950 */ 1951 public Composition setNameElement(StringType value) { 1952 this.name = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1958 */ 1959 public String getName() { 1960 return this.name == null ? null : this.name.getValue(); 1961 } 1962 1963 /** 1964 * @param value A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1965 */ 1966 public Composition setName(String value) { 1967 if (Utilities.noString(value)) 1968 this.name = null; 1969 else { 1970 if (this.name == null) 1971 this.name = new StringType(); 1972 this.name.setValue(value); 1973 } 1974 return this; 1975 } 1976 1977 /** 1978 * @return {@link #title} (Official human-readable label for the composition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1979 */ 1980 public StringType getTitleElement() { 1981 if (this.title == null) 1982 if (Configuration.errorOnAutoCreate()) 1983 throw new Error("Attempt to auto-create Composition.title"); 1984 else if (Configuration.doAutoCreate()) 1985 this.title = new StringType(); // bb 1986 return this.title; 1987 } 1988 1989 public boolean hasTitleElement() { 1990 return this.title != null && !this.title.isEmpty(); 1991 } 1992 1993 public boolean hasTitle() { 1994 return this.title != null && !this.title.isEmpty(); 1995 } 1996 1997 /** 1998 * @param value {@link #title} (Official human-readable label for the composition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1999 */ 2000 public Composition setTitleElement(StringType value) { 2001 this.title = value; 2002 return this; 2003 } 2004 2005 /** 2006 * @return Official human-readable label for the composition. 2007 */ 2008 public String getTitle() { 2009 return this.title == null ? null : this.title.getValue(); 2010 } 2011 2012 /** 2013 * @param value Official human-readable label for the composition. 2014 */ 2015 public Composition setTitle(String value) { 2016 if (this.title == null) 2017 this.title = new StringType(); 2018 this.title.setValue(value); 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #note} (For any additional notes.) 2024 */ 2025 public List<Annotation> getNote() { 2026 if (this.note == null) 2027 this.note = new ArrayList<Annotation>(); 2028 return this.note; 2029 } 2030 2031 /** 2032 * @return Returns a reference to <code>this</code> for easy method chaining 2033 */ 2034 public Composition setNote(List<Annotation> theNote) { 2035 this.note = theNote; 2036 return this; 2037 } 2038 2039 public boolean hasNote() { 2040 if (this.note == null) 2041 return false; 2042 for (Annotation item : this.note) 2043 if (!item.isEmpty()) 2044 return true; 2045 return false; 2046 } 2047 2048 public Annotation addNote() { //3 2049 Annotation t = new Annotation(); 2050 if (this.note == null) 2051 this.note = new ArrayList<Annotation>(); 2052 this.note.add(t); 2053 return t; 2054 } 2055 2056 public Composition addNote(Annotation t) { //3 2057 if (t == null) 2058 return this; 2059 if (this.note == null) 2060 this.note = new ArrayList<Annotation>(); 2061 this.note.add(t); 2062 return this; 2063 } 2064 2065 /** 2066 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2067 */ 2068 public Annotation getNoteFirstRep() { 2069 if (getNote().isEmpty()) { 2070 addNote(); 2071 } 2072 return getNote().get(0); 2073 } 2074 2075 /** 2076 * @return {@link #attester} (A participant who has attested to the accuracy of the composition/document.) 2077 */ 2078 public List<CompositionAttesterComponent> getAttester() { 2079 if (this.attester == null) 2080 this.attester = new ArrayList<CompositionAttesterComponent>(); 2081 return this.attester; 2082 } 2083 2084 /** 2085 * @return Returns a reference to <code>this</code> for easy method chaining 2086 */ 2087 public Composition setAttester(List<CompositionAttesterComponent> theAttester) { 2088 this.attester = theAttester; 2089 return this; 2090 } 2091 2092 public boolean hasAttester() { 2093 if (this.attester == null) 2094 return false; 2095 for (CompositionAttesterComponent item : this.attester) 2096 if (!item.isEmpty()) 2097 return true; 2098 return false; 2099 } 2100 2101 public CompositionAttesterComponent addAttester() { //3 2102 CompositionAttesterComponent t = new CompositionAttesterComponent(); 2103 if (this.attester == null) 2104 this.attester = new ArrayList<CompositionAttesterComponent>(); 2105 this.attester.add(t); 2106 return t; 2107 } 2108 2109 public Composition addAttester(CompositionAttesterComponent t) { //3 2110 if (t == null) 2111 return this; 2112 if (this.attester == null) 2113 this.attester = new ArrayList<CompositionAttesterComponent>(); 2114 this.attester.add(t); 2115 return this; 2116 } 2117 2118 /** 2119 * @return The first repetition of repeating field {@link #attester}, creating it if it does not already exist {3} 2120 */ 2121 public CompositionAttesterComponent getAttesterFirstRep() { 2122 if (getAttester().isEmpty()) { 2123 addAttester(); 2124 } 2125 return getAttester().get(0); 2126 } 2127 2128 /** 2129 * @return {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2130 */ 2131 public Reference getCustodian() { 2132 if (this.custodian == null) 2133 if (Configuration.errorOnAutoCreate()) 2134 throw new Error("Attempt to auto-create Composition.custodian"); 2135 else if (Configuration.doAutoCreate()) 2136 this.custodian = new Reference(); // cc 2137 return this.custodian; 2138 } 2139 2140 public boolean hasCustodian() { 2141 return this.custodian != null && !this.custodian.isEmpty(); 2142 } 2143 2144 /** 2145 * @param value {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.) 2146 */ 2147 public Composition setCustodian(Reference value) { 2148 this.custodian = value; 2149 return this; 2150 } 2151 2152 /** 2153 * @return {@link #relatesTo} (Relationships that this composition has with other compositions or documents that already exist.) 2154 */ 2155 public List<RelatedArtifact> getRelatesTo() { 2156 if (this.relatesTo == null) 2157 this.relatesTo = new ArrayList<RelatedArtifact>(); 2158 return this.relatesTo; 2159 } 2160 2161 /** 2162 * @return Returns a reference to <code>this</code> for easy method chaining 2163 */ 2164 public Composition setRelatesTo(List<RelatedArtifact> theRelatesTo) { 2165 this.relatesTo = theRelatesTo; 2166 return this; 2167 } 2168 2169 public boolean hasRelatesTo() { 2170 if (this.relatesTo == null) 2171 return false; 2172 for (RelatedArtifact item : this.relatesTo) 2173 if (!item.isEmpty()) 2174 return true; 2175 return false; 2176 } 2177 2178 public RelatedArtifact addRelatesTo() { //3 2179 RelatedArtifact t = new RelatedArtifact(); 2180 if (this.relatesTo == null) 2181 this.relatesTo = new ArrayList<RelatedArtifact>(); 2182 this.relatesTo.add(t); 2183 return t; 2184 } 2185 2186 public Composition addRelatesTo(RelatedArtifact t) { //3 2187 if (t == null) 2188 return this; 2189 if (this.relatesTo == null) 2190 this.relatesTo = new ArrayList<RelatedArtifact>(); 2191 this.relatesTo.add(t); 2192 return this; 2193 } 2194 2195 /** 2196 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist {3} 2197 */ 2198 public RelatedArtifact getRelatesToFirstRep() { 2199 if (getRelatesTo().isEmpty()) { 2200 addRelatesTo(); 2201 } 2202 return getRelatesTo().get(0); 2203 } 2204 2205 /** 2206 * @return {@link #event} (The clinical service, such as a colonoscopy or an appendectomy, being documented.) 2207 */ 2208 public List<CompositionEventComponent> getEvent() { 2209 if (this.event == null) 2210 this.event = new ArrayList<CompositionEventComponent>(); 2211 return this.event; 2212 } 2213 2214 /** 2215 * @return Returns a reference to <code>this</code> for easy method chaining 2216 */ 2217 public Composition setEvent(List<CompositionEventComponent> theEvent) { 2218 this.event = theEvent; 2219 return this; 2220 } 2221 2222 public boolean hasEvent() { 2223 if (this.event == null) 2224 return false; 2225 for (CompositionEventComponent item : this.event) 2226 if (!item.isEmpty()) 2227 return true; 2228 return false; 2229 } 2230 2231 public CompositionEventComponent addEvent() { //3 2232 CompositionEventComponent t = new CompositionEventComponent(); 2233 if (this.event == null) 2234 this.event = new ArrayList<CompositionEventComponent>(); 2235 this.event.add(t); 2236 return t; 2237 } 2238 2239 public Composition addEvent(CompositionEventComponent t) { //3 2240 if (t == null) 2241 return this; 2242 if (this.event == null) 2243 this.event = new ArrayList<CompositionEventComponent>(); 2244 this.event.add(t); 2245 return this; 2246 } 2247 2248 /** 2249 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 2250 */ 2251 public CompositionEventComponent getEventFirstRep() { 2252 if (getEvent().isEmpty()) { 2253 addEvent(); 2254 } 2255 return getEvent().get(0); 2256 } 2257 2258 /** 2259 * @return {@link #section} (The root of the sections that make up the composition.) 2260 */ 2261 public List<SectionComponent> getSection() { 2262 if (this.section == null) 2263 this.section = new ArrayList<SectionComponent>(); 2264 return this.section; 2265 } 2266 2267 /** 2268 * @return Returns a reference to <code>this</code> for easy method chaining 2269 */ 2270 public Composition setSection(List<SectionComponent> theSection) { 2271 this.section = theSection; 2272 return this; 2273 } 2274 2275 public boolean hasSection() { 2276 if (this.section == null) 2277 return false; 2278 for (SectionComponent item : this.section) 2279 if (!item.isEmpty()) 2280 return true; 2281 return false; 2282 } 2283 2284 public SectionComponent addSection() { //3 2285 SectionComponent t = new SectionComponent(); 2286 if (this.section == null) 2287 this.section = new ArrayList<SectionComponent>(); 2288 this.section.add(t); 2289 return t; 2290 } 2291 2292 public Composition addSection(SectionComponent t) { //3 2293 if (t == null) 2294 return this; 2295 if (this.section == null) 2296 this.section = new ArrayList<SectionComponent>(); 2297 this.section.add(t); 2298 return this; 2299 } 2300 2301 /** 2302 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist {3} 2303 */ 2304 public SectionComponent getSectionFirstRep() { 2305 if (getSection().isEmpty()) { 2306 addSection(); 2307 } 2308 return getSection().get(0); 2309 } 2310 2311 protected void listChildren(List<Property> children) { 2312 super.listChildren(children); 2313 children.add(new Property("url", "uri", "An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers.", 0, 1, url)); 2314 children.add(new Property("identifier", "Identifier", "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2315 children.add(new Property("version", "string", "An explicitly assigned identifer of a variation of the content in the Composition.", 0, 1, version)); 2316 children.add(new Property("status", "code", "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 0, 1, status)); 2317 children.add(new Property("type", "CodeableConcept", "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 0, 1, type)); 2318 children.add(new Property("category", "CodeableConcept", "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 0, java.lang.Integer.MAX_VALUE, category)); 2319 children.add(new Property("subject", "Reference(Any)", "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 0, java.lang.Integer.MAX_VALUE, subject)); 2320 children.add(new Property("encounter", "Reference(Encounter)", "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter)); 2321 children.add(new Property("date", "dateTime", "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date)); 2322 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Composition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2323 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author)); 2324 children.add(new Property("name", "string", "A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2325 children.add(new Property("title", "string", "Official human-readable label for the composition.", 0, 1, title)); 2326 children.add(new Property("note", "Annotation", "For any additional notes.", 0, java.lang.Integer.MAX_VALUE, note)); 2327 children.add(new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 0, java.lang.Integer.MAX_VALUE, attester)); 2328 children.add(new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 0, 1, custodian)); 2329 children.add(new Property("relatesTo", "RelatedArtifact", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 2330 children.add(new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 0, java.lang.Integer.MAX_VALUE, event)); 2331 children.add(new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section)); 2332 } 2333 2334 @Override 2335 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2336 switch (_hash) { 2337 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this Composition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Composition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Composition is stored on different servers.", 0, 1, url); 2338 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.", 0, java.lang.Integer.MAX_VALUE, identifier); 2339 case 351608024: /*version*/ return new Property("version", "string", "An explicitly assigned identifer of a variation of the content in the Composition.", 0, 1, version); 2340 case -892481550: /*status*/ return new Property("status", "code", "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 0, 1, status); 2341 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 0, 1, type); 2342 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 0, java.lang.Integer.MAX_VALUE, category); 2343 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 0, java.lang.Integer.MAX_VALUE, subject); 2344 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter); 2345 case 3076014: /*date*/ return new Property("date", "dateTime", "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date); 2346 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Composition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2347 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author); 2348 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the composition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2349 case 110371416: /*title*/ return new Property("title", "string", "Official human-readable label for the composition.", 0, 1, title); 2350 case 3387378: /*note*/ return new Property("note", "Annotation", "For any additional notes.", 0, java.lang.Integer.MAX_VALUE, note); 2351 case 542920370: /*attester*/ return new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 0, java.lang.Integer.MAX_VALUE, attester); 2352 case 1611297262: /*custodian*/ return new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 0, 1, custodian); 2353 case -7765931: /*relatesTo*/ return new Property("relatesTo", "RelatedArtifact", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 2354 case 96891546: /*event*/ return new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 0, java.lang.Integer.MAX_VALUE, event); 2355 case 1970241253: /*section*/ return new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section); 2356 default: return super.getNamedProperty(_hash, _name, _checkValid); 2357 } 2358 2359 } 2360 2361 @Override 2362 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2363 switch (hash) { 2364 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2365 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2366 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2367 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<CompositionStatus> 2368 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2369 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2370 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 2371 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2372 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2373 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2374 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2375 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2376 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2377 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2378 case 542920370: /*attester*/ return this.attester == null ? new Base[0] : this.attester.toArray(new Base[this.attester.size()]); // CompositionAttesterComponent 2379 case 1611297262: /*custodian*/ return this.custodian == null ? new Base[0] : new Base[] {this.custodian}; // Reference 2380 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // RelatedArtifact 2381 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CompositionEventComponent 2382 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 2383 default: return super.getProperty(hash, name, checkValid); 2384 } 2385 2386 } 2387 2388 @Override 2389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2390 switch (hash) { 2391 case 116079: // url 2392 this.url = TypeConvertor.castToUri(value); // UriType 2393 return value; 2394 case -1618432855: // identifier 2395 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2396 return value; 2397 case 351608024: // version 2398 this.version = TypeConvertor.castToString(value); // StringType 2399 return value; 2400 case -892481550: // status 2401 value = new CompositionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2402 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 2403 return value; 2404 case 3575610: // type 2405 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2406 return value; 2407 case 50511102: // category 2408 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2409 return value; 2410 case -1867885268: // subject 2411 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 2412 return value; 2413 case 1524132147: // encounter 2414 this.encounter = TypeConvertor.castToReference(value); // Reference 2415 return value; 2416 case 3076014: // date 2417 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2418 return value; 2419 case -669707736: // useContext 2420 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2421 return value; 2422 case -1406328437: // author 2423 this.getAuthor().add(TypeConvertor.castToReference(value)); // Reference 2424 return value; 2425 case 3373707: // name 2426 this.name = TypeConvertor.castToString(value); // StringType 2427 return value; 2428 case 110371416: // title 2429 this.title = TypeConvertor.castToString(value); // StringType 2430 return value; 2431 case 3387378: // note 2432 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2433 return value; 2434 case 542920370: // attester 2435 this.getAttester().add((CompositionAttesterComponent) value); // CompositionAttesterComponent 2436 return value; 2437 case 1611297262: // custodian 2438 this.custodian = TypeConvertor.castToReference(value); // Reference 2439 return value; 2440 case -7765931: // relatesTo 2441 this.getRelatesTo().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 2442 return value; 2443 case 96891546: // event 2444 this.getEvent().add((CompositionEventComponent) value); // CompositionEventComponent 2445 return value; 2446 case 1970241253: // section 2447 this.getSection().add((SectionComponent) value); // SectionComponent 2448 return value; 2449 default: return super.setProperty(hash, name, value); 2450 } 2451 2452 } 2453 2454 @Override 2455 public Base setProperty(String name, Base value) throws FHIRException { 2456 if (name.equals("url")) { 2457 this.url = TypeConvertor.castToUri(value); // UriType 2458 } else if (name.equals("identifier")) { 2459 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2460 } else if (name.equals("version")) { 2461 this.version = TypeConvertor.castToString(value); // StringType 2462 } else if (name.equals("status")) { 2463 value = new CompositionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2464 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 2465 } else if (name.equals("type")) { 2466 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2467 } else if (name.equals("category")) { 2468 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2469 } else if (name.equals("subject")) { 2470 this.getSubject().add(TypeConvertor.castToReference(value)); 2471 } else if (name.equals("encounter")) { 2472 this.encounter = TypeConvertor.castToReference(value); // Reference 2473 } else if (name.equals("date")) { 2474 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2475 } else if (name.equals("useContext")) { 2476 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2477 } else if (name.equals("author")) { 2478 this.getAuthor().add(TypeConvertor.castToReference(value)); 2479 } else if (name.equals("name")) { 2480 this.name = TypeConvertor.castToString(value); // StringType 2481 } else if (name.equals("title")) { 2482 this.title = TypeConvertor.castToString(value); // StringType 2483 } else if (name.equals("note")) { 2484 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2485 } else if (name.equals("attester")) { 2486 this.getAttester().add((CompositionAttesterComponent) value); 2487 } else if (name.equals("custodian")) { 2488 this.custodian = TypeConvertor.castToReference(value); // Reference 2489 } else if (name.equals("relatesTo")) { 2490 this.getRelatesTo().add(TypeConvertor.castToRelatedArtifact(value)); 2491 } else if (name.equals("event")) { 2492 this.getEvent().add((CompositionEventComponent) value); 2493 } else if (name.equals("section")) { 2494 this.getSection().add((SectionComponent) value); 2495 } else 2496 return super.setProperty(name, value); 2497 return value; 2498 } 2499 2500 @Override 2501 public void removeChild(String name, Base value) throws FHIRException { 2502 if (name.equals("url")) { 2503 this.url = null; 2504 } else if (name.equals("identifier")) { 2505 this.getIdentifier().remove(value); 2506 } else if (name.equals("version")) { 2507 this.version = null; 2508 } else if (name.equals("status")) { 2509 value = new CompositionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2510 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 2511 } else if (name.equals("type")) { 2512 this.type = null; 2513 } else if (name.equals("category")) { 2514 this.getCategory().remove(value); 2515 } else if (name.equals("subject")) { 2516 this.getSubject().remove(value); 2517 } else if (name.equals("encounter")) { 2518 this.encounter = null; 2519 } else if (name.equals("date")) { 2520 this.date = null; 2521 } else if (name.equals("useContext")) { 2522 this.getUseContext().remove(value); 2523 } else if (name.equals("author")) { 2524 this.getAuthor().remove(value); 2525 } else if (name.equals("name")) { 2526 this.name = null; 2527 } else if (name.equals("title")) { 2528 this.title = null; 2529 } else if (name.equals("note")) { 2530 this.getNote().remove(value); 2531 } else if (name.equals("attester")) { 2532 this.getAttester().remove((CompositionAttesterComponent) value); 2533 } else if (name.equals("custodian")) { 2534 this.custodian = null; 2535 } else if (name.equals("relatesTo")) { 2536 this.getRelatesTo().remove(value); 2537 } else if (name.equals("event")) { 2538 this.getEvent().remove((CompositionEventComponent) value); 2539 } else if (name.equals("section")) { 2540 this.getSection().remove((SectionComponent) value); 2541 } else 2542 super.removeChild(name, value); 2543 2544 } 2545 2546 @Override 2547 public Base makeProperty(int hash, String name) throws FHIRException { 2548 switch (hash) { 2549 case 116079: return getUrlElement(); 2550 case -1618432855: return addIdentifier(); 2551 case 351608024: return getVersionElement(); 2552 case -892481550: return getStatusElement(); 2553 case 3575610: return getType(); 2554 case 50511102: return addCategory(); 2555 case -1867885268: return addSubject(); 2556 case 1524132147: return getEncounter(); 2557 case 3076014: return getDateElement(); 2558 case -669707736: return addUseContext(); 2559 case -1406328437: return addAuthor(); 2560 case 3373707: return getNameElement(); 2561 case 110371416: return getTitleElement(); 2562 case 3387378: return addNote(); 2563 case 542920370: return addAttester(); 2564 case 1611297262: return getCustodian(); 2565 case -7765931: return addRelatesTo(); 2566 case 96891546: return addEvent(); 2567 case 1970241253: return addSection(); 2568 default: return super.makeProperty(hash, name); 2569 } 2570 2571 } 2572 2573 @Override 2574 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2575 switch (hash) { 2576 case 116079: /*url*/ return new String[] {"uri"}; 2577 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2578 case 351608024: /*version*/ return new String[] {"string"}; 2579 case -892481550: /*status*/ return new String[] {"code"}; 2580 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2581 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2582 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2583 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2584 case 3076014: /*date*/ return new String[] {"dateTime"}; 2585 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2586 case -1406328437: /*author*/ return new String[] {"Reference"}; 2587 case 3373707: /*name*/ return new String[] {"string"}; 2588 case 110371416: /*title*/ return new String[] {"string"}; 2589 case 3387378: /*note*/ return new String[] {"Annotation"}; 2590 case 542920370: /*attester*/ return new String[] {}; 2591 case 1611297262: /*custodian*/ return new String[] {"Reference"}; 2592 case -7765931: /*relatesTo*/ return new String[] {"RelatedArtifact"}; 2593 case 96891546: /*event*/ return new String[] {}; 2594 case 1970241253: /*section*/ return new String[] {}; 2595 default: return super.getTypesForProperty(hash, name); 2596 } 2597 2598 } 2599 2600 @Override 2601 public Base addChild(String name) throws FHIRException { 2602 if (name.equals("url")) { 2603 throw new FHIRException("Cannot call addChild on a singleton property Composition.url"); 2604 } 2605 else if (name.equals("identifier")) { 2606 return addIdentifier(); 2607 } 2608 else if (name.equals("version")) { 2609 throw new FHIRException("Cannot call addChild on a singleton property Composition.version"); 2610 } 2611 else if (name.equals("status")) { 2612 throw new FHIRException("Cannot call addChild on a singleton property Composition.status"); 2613 } 2614 else if (name.equals("type")) { 2615 this.type = new CodeableConcept(); 2616 return this.type; 2617 } 2618 else if (name.equals("category")) { 2619 return addCategory(); 2620 } 2621 else if (name.equals("subject")) { 2622 return addSubject(); 2623 } 2624 else if (name.equals("encounter")) { 2625 this.encounter = new Reference(); 2626 return this.encounter; 2627 } 2628 else if (name.equals("date")) { 2629 throw new FHIRException("Cannot call addChild on a singleton property Composition.date"); 2630 } 2631 else if (name.equals("useContext")) { 2632 return addUseContext(); 2633 } 2634 else if (name.equals("author")) { 2635 return addAuthor(); 2636 } 2637 else if (name.equals("name")) { 2638 throw new FHIRException("Cannot call addChild on a singleton property Composition.name"); 2639 } 2640 else if (name.equals("title")) { 2641 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 2642 } 2643 else if (name.equals("note")) { 2644 return addNote(); 2645 } 2646 else if (name.equals("attester")) { 2647 return addAttester(); 2648 } 2649 else if (name.equals("custodian")) { 2650 this.custodian = new Reference(); 2651 return this.custodian; 2652 } 2653 else if (name.equals("relatesTo")) { 2654 return addRelatesTo(); 2655 } 2656 else if (name.equals("event")) { 2657 return addEvent(); 2658 } 2659 else if (name.equals("section")) { 2660 return addSection(); 2661 } 2662 else 2663 return super.addChild(name); 2664 } 2665 2666 public String fhirType() { 2667 return "Composition"; 2668 2669 } 2670 2671 public Composition copy() { 2672 Composition dst = new Composition(); 2673 copyValues(dst); 2674 return dst; 2675 } 2676 2677 public void copyValues(Composition dst) { 2678 super.copyValues(dst); 2679 dst.url = url == null ? null : url.copy(); 2680 if (identifier != null) { 2681 dst.identifier = new ArrayList<Identifier>(); 2682 for (Identifier i : identifier) 2683 dst.identifier.add(i.copy()); 2684 }; 2685 dst.version = version == null ? null : version.copy(); 2686 dst.status = status == null ? null : status.copy(); 2687 dst.type = type == null ? null : type.copy(); 2688 if (category != null) { 2689 dst.category = new ArrayList<CodeableConcept>(); 2690 for (CodeableConcept i : category) 2691 dst.category.add(i.copy()); 2692 }; 2693 if (subject != null) { 2694 dst.subject = new ArrayList<Reference>(); 2695 for (Reference i : subject) 2696 dst.subject.add(i.copy()); 2697 }; 2698 dst.encounter = encounter == null ? null : encounter.copy(); 2699 dst.date = date == null ? null : date.copy(); 2700 if (useContext != null) { 2701 dst.useContext = new ArrayList<UsageContext>(); 2702 for (UsageContext i : useContext) 2703 dst.useContext.add(i.copy()); 2704 }; 2705 if (author != null) { 2706 dst.author = new ArrayList<Reference>(); 2707 for (Reference i : author) 2708 dst.author.add(i.copy()); 2709 }; 2710 dst.name = name == null ? null : name.copy(); 2711 dst.title = title == null ? null : title.copy(); 2712 if (note != null) { 2713 dst.note = new ArrayList<Annotation>(); 2714 for (Annotation i : note) 2715 dst.note.add(i.copy()); 2716 }; 2717 if (attester != null) { 2718 dst.attester = new ArrayList<CompositionAttesterComponent>(); 2719 for (CompositionAttesterComponent i : attester) 2720 dst.attester.add(i.copy()); 2721 }; 2722 dst.custodian = custodian == null ? null : custodian.copy(); 2723 if (relatesTo != null) { 2724 dst.relatesTo = new ArrayList<RelatedArtifact>(); 2725 for (RelatedArtifact i : relatesTo) 2726 dst.relatesTo.add(i.copy()); 2727 }; 2728 if (event != null) { 2729 dst.event = new ArrayList<CompositionEventComponent>(); 2730 for (CompositionEventComponent i : event) 2731 dst.event.add(i.copy()); 2732 }; 2733 if (section != null) { 2734 dst.section = new ArrayList<SectionComponent>(); 2735 for (SectionComponent i : section) 2736 dst.section.add(i.copy()); 2737 }; 2738 } 2739 2740 protected Composition typedCopy() { 2741 return copy(); 2742 } 2743 2744 @Override 2745 public boolean equalsDeep(Base other_) { 2746 if (!super.equalsDeep(other_)) 2747 return false; 2748 if (!(other_ instanceof Composition)) 2749 return false; 2750 Composition o = (Composition) other_; 2751 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2752 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 2753 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) && compareDeep(date, o.date, true) 2754 && compareDeep(useContext, o.useContext, true) && compareDeep(author, o.author, true) && compareDeep(name, o.name, true) 2755 && compareDeep(title, o.title, true) && compareDeep(note, o.note, true) && compareDeep(attester, o.attester, true) 2756 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(event, o.event, true) 2757 && compareDeep(section, o.section, true); 2758 } 2759 2760 @Override 2761 public boolean equalsShallow(Base other_) { 2762 if (!super.equalsShallow(other_)) 2763 return false; 2764 if (!(other_ instanceof Composition)) 2765 return false; 2766 Composition o = (Composition) other_; 2767 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(status, o.status, true) 2768 && compareValues(date, o.date, true) && compareValues(name, o.name, true) && compareValues(title, o.title, true) 2769 ; 2770 } 2771 2772 public boolean isEmpty() { 2773 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2774 , status, type, category, subject, encounter, date, useContext, author, name 2775 , title, note, attester, custodian, relatesTo, event, section); 2776 } 2777 2778 @Override 2779 public ResourceType getResourceType() { 2780 return ResourceType.Composition; 2781 } 2782 2783 /** 2784 * Search parameter: <b>attester</b> 2785 * <p> 2786 * Description: <b>Who attested the composition</b><br> 2787 * Type: <b>reference</b><br> 2788 * Path: <b>Composition.attester.party</b><br> 2789 * </p> 2790 */ 2791 @SearchParamDefinition(name="attester", path="Composition.attester.party", description="Who attested the composition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2792 public static final String SP_ATTESTER = "attester"; 2793 /** 2794 * <b>Fluent Client</b> search parameter constant for <b>attester</b> 2795 * <p> 2796 * Description: <b>Who attested the composition</b><br> 2797 * Type: <b>reference</b><br> 2798 * Path: <b>Composition.attester.party</b><br> 2799 * </p> 2800 */ 2801 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ATTESTER); 2802 2803/** 2804 * Constant for fluent queries to be used to add include statements. Specifies 2805 * the path value of "<b>Composition:attester</b>". 2806 */ 2807 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTER = new ca.uhn.fhir.model.api.Include("Composition:attester").toLocked(); 2808 2809 /** 2810 * Search parameter: <b>author</b> 2811 * <p> 2812 * Description: <b>Who and/or what authored the composition</b><br> 2813 * Type: <b>reference</b><br> 2814 * Path: <b>Composition.author</b><br> 2815 * </p> 2816 */ 2817 @SearchParamDefinition(name="author", path="Composition.author", description="Who and/or what authored the composition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2818 public static final String SP_AUTHOR = "author"; 2819 /** 2820 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2821 * <p> 2822 * Description: <b>Who and/or what authored the composition</b><br> 2823 * Type: <b>reference</b><br> 2824 * Path: <b>Composition.author</b><br> 2825 * </p> 2826 */ 2827 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2828 2829/** 2830 * Constant for fluent queries to be used to add include statements. Specifies 2831 * the path value of "<b>Composition:author</b>". 2832 */ 2833 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Composition:author").toLocked(); 2834 2835 /** 2836 * Search parameter: <b>category</b> 2837 * <p> 2838 * Description: <b>Categorization of Composition</b><br> 2839 * Type: <b>token</b><br> 2840 * Path: <b>Composition.category</b><br> 2841 * </p> 2842 */ 2843 @SearchParamDefinition(name="category", path="Composition.category", description="Categorization of Composition", type="token" ) 2844 public static final String SP_CATEGORY = "category"; 2845 /** 2846 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2847 * <p> 2848 * Description: <b>Categorization of Composition</b><br> 2849 * Type: <b>token</b><br> 2850 * Path: <b>Composition.category</b><br> 2851 * </p> 2852 */ 2853 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2854 2855 /** 2856 * Search parameter: <b>entry</b> 2857 * <p> 2858 * Description: <b>A reference to data that supports this section</b><br> 2859 * Type: <b>reference</b><br> 2860 * Path: <b>Composition.section.entry</b><br> 2861 * </p> 2862 */ 2863 @SearchParamDefinition(name="entry", path="Composition.section.entry", description="A reference to data that supports this section", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2864 public static final String SP_ENTRY = "entry"; 2865 /** 2866 * <b>Fluent Client</b> search parameter constant for <b>entry</b> 2867 * <p> 2868 * Description: <b>A reference to data that supports this section</b><br> 2869 * Type: <b>reference</b><br> 2870 * Path: <b>Composition.section.entry</b><br> 2871 * </p> 2872 */ 2873 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTRY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTRY); 2874 2875/** 2876 * Constant for fluent queries to be used to add include statements. Specifies 2877 * the path value of "<b>Composition:entry</b>". 2878 */ 2879 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTRY = new ca.uhn.fhir.model.api.Include("Composition:entry").toLocked(); 2880 2881 /** 2882 * Search parameter: <b>event-code</b> 2883 * <p> 2884 * Description: <b>Main clinical acts documented as codes</b><br> 2885 * Type: <b>token</b><br> 2886 * Path: <b>Composition.event.detail.concept</b><br> 2887 * </p> 2888 */ 2889 @SearchParamDefinition(name="event-code", path="Composition.event.detail.concept", description="Main clinical acts documented as codes", type="token" ) 2890 public static final String SP_EVENT_CODE = "event-code"; 2891 /** 2892 * <b>Fluent Client</b> search parameter constant for <b>event-code</b> 2893 * <p> 2894 * Description: <b>Main clinical acts documented as codes</b><br> 2895 * Type: <b>token</b><br> 2896 * Path: <b>Composition.event.detail.concept</b><br> 2897 * </p> 2898 */ 2899 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT_CODE); 2900 2901 /** 2902 * Search parameter: <b>event-reference</b> 2903 * <p> 2904 * Description: <b>Main clinical acts documented as references</b><br> 2905 * Type: <b>reference</b><br> 2906 * Path: <b>Composition.event.detail.reference</b><br> 2907 * </p> 2908 */ 2909 @SearchParamDefinition(name="event-reference", path="Composition.event.detail.reference", description="Main clinical acts documented as references", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2910 public static final String SP_EVENT_REFERENCE = "event-reference"; 2911 /** 2912 * <b>Fluent Client</b> search parameter constant for <b>event-reference</b> 2913 * <p> 2914 * Description: <b>Main clinical acts documented as references</b><br> 2915 * Type: <b>reference</b><br> 2916 * Path: <b>Composition.event.detail.reference</b><br> 2917 * </p> 2918 */ 2919 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVENT_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EVENT_REFERENCE); 2920 2921/** 2922 * Constant for fluent queries to be used to add include statements. Specifies 2923 * the path value of "<b>Composition:event-reference</b>". 2924 */ 2925 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVENT_REFERENCE = new ca.uhn.fhir.model.api.Include("Composition:event-reference").toLocked(); 2926 2927 /** 2928 * Search parameter: <b>period</b> 2929 * <p> 2930 * Description: <b>The period covered by the documentation</b><br> 2931 * Type: <b>date</b><br> 2932 * Path: <b>Composition.event.period</b><br> 2933 * </p> 2934 */ 2935 @SearchParamDefinition(name="period", path="Composition.event.period", description="The period covered by the documentation", type="date" ) 2936 public static final String SP_PERIOD = "period"; 2937 /** 2938 * <b>Fluent Client</b> search parameter constant for <b>period</b> 2939 * <p> 2940 * Description: <b>The period covered by the documentation</b><br> 2941 * Type: <b>date</b><br> 2942 * Path: <b>Composition.event.period</b><br> 2943 * </p> 2944 */ 2945 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 2946 2947 /** 2948 * Search parameter: <b>related</b> 2949 * <p> 2950 * Description: <b>Target of the relationship</b><br> 2951 * Type: <b>reference</b><br> 2952 * Path: <b>Composition.relatesTo.resourceReference</b><br> 2953 * </p> 2954 */ 2955 @SearchParamDefinition(name="related", path="Composition.relatesTo.resourceReference", description="Target of the relationship", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2956 public static final String SP_RELATED = "related"; 2957 /** 2958 * <b>Fluent Client</b> search parameter constant for <b>related</b> 2959 * <p> 2960 * Description: <b>Target of the relationship</b><br> 2961 * Type: <b>reference</b><br> 2962 * Path: <b>Composition.relatesTo.resourceReference</b><br> 2963 * </p> 2964 */ 2965 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATED); 2966 2967/** 2968 * Constant for fluent queries to be used to add include statements. Specifies 2969 * the path value of "<b>Composition:related</b>". 2970 */ 2971 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED = new ca.uhn.fhir.model.api.Include("Composition:related").toLocked(); 2972 2973 /** 2974 * Search parameter: <b>section-code-text</b> 2975 * <p> 2976 * Description: <b>Search on the section narrative of the resource</b><br> 2977 * Type: <b>composite</b><br> 2978 * Path: <b>Composition.section</b><br> 2979 * </p> 2980 */ 2981 @SearchParamDefinition(name="section-code-text", path="Composition.section", description="Search on the section narrative of the resource", type="composite", compositeOf={"section", "section-text"} ) 2982 public static final String SP_SECTION_CODE_TEXT = "section-code-text"; 2983 /** 2984 * <b>Fluent Client</b> search parameter constant for <b>section-code-text</b> 2985 * <p> 2986 * Description: <b>Search on the section narrative of the resource</b><br> 2987 * Type: <b>composite</b><br> 2988 * Path: <b>Composition.section</b><br> 2989 * </p> 2990 */ 2991 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.SpecialClientParam> SECTION_CODE_TEXT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.SpecialClientParam>(SP_SECTION_CODE_TEXT); 2992 2993 /** 2994 * Search parameter: <b>section-text</b> 2995 * <p> 2996 * Description: <b>Search on the section narrative of the resource</b><br> 2997 * Type: <b>special</b><br> 2998 * Path: <b>Composition.section.text | Composition.section.section.text</b><br> 2999 * </p> 3000 */ 3001 @SearchParamDefinition(name="section-text", path="Composition.section.text | Composition.section.section.text", description="Search on the section narrative of the resource", type="special" ) 3002 public static final String SP_SECTION_TEXT = "section-text"; 3003 /** 3004 * <b>Fluent Client</b> search parameter constant for <b>section-text</b> 3005 * <p> 3006 * Description: <b>Search on the section narrative of the resource</b><br> 3007 * Type: <b>special</b><br> 3008 * Path: <b>Composition.section.text | Composition.section.section.text</b><br> 3009 * </p> 3010 */ 3011 public static final ca.uhn.fhir.rest.gclient.SpecialClientParam SECTION_TEXT = new ca.uhn.fhir.rest.gclient.SpecialClientParam(SP_SECTION_TEXT); 3012 3013 /** 3014 * Search parameter: <b>section</b> 3015 * <p> 3016 * Description: <b>Classification of section (recommended)</b><br> 3017 * Type: <b>token</b><br> 3018 * Path: <b>Composition.section.code</b><br> 3019 * </p> 3020 */ 3021 @SearchParamDefinition(name="section", path="Composition.section.code", description="Classification of section (recommended)", type="token" ) 3022 public static final String SP_SECTION = "section"; 3023 /** 3024 * <b>Fluent Client</b> search parameter constant for <b>section</b> 3025 * <p> 3026 * Description: <b>Classification of section (recommended)</b><br> 3027 * Type: <b>token</b><br> 3028 * Path: <b>Composition.section.code</b><br> 3029 * </p> 3030 */ 3031 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECTION); 3032 3033 /** 3034 * Search parameter: <b>status</b> 3035 * <p> 3036 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 3037 * Type: <b>token</b><br> 3038 * Path: <b>Composition.status</b><br> 3039 * </p> 3040 */ 3041 @SearchParamDefinition(name="status", path="Composition.status", description="preliminary | final | amended | entered-in-error", type="token" ) 3042 public static final String SP_STATUS = "status"; 3043 /** 3044 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3045 * <p> 3046 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 3047 * Type: <b>token</b><br> 3048 * Path: <b>Composition.status</b><br> 3049 * </p> 3050 */ 3051 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3052 3053 /** 3054 * Search parameter: <b>subject</b> 3055 * <p> 3056 * Description: <b>Who and/or what the composition is about</b><br> 3057 * Type: <b>reference</b><br> 3058 * Path: <b>Composition.subject</b><br> 3059 * </p> 3060 */ 3061 @SearchParamDefinition(name="subject", path="Composition.subject", description="Who and/or what the composition is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3062 public static final String SP_SUBJECT = "subject"; 3063 /** 3064 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3065 * <p> 3066 * Description: <b>Who and/or what the composition is about</b><br> 3067 * Type: <b>reference</b><br> 3068 * Path: <b>Composition.subject</b><br> 3069 * </p> 3070 */ 3071 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3072 3073/** 3074 * Constant for fluent queries to be used to add include statements. Specifies 3075 * the path value of "<b>Composition:subject</b>". 3076 */ 3077 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Composition:subject").toLocked(); 3078 3079 /** 3080 * Search parameter: <b>title</b> 3081 * <p> 3082 * Description: <b>Human Readable name/title</b><br> 3083 * Type: <b>string</b><br> 3084 * Path: <b>Composition.title</b><br> 3085 * </p> 3086 */ 3087 @SearchParamDefinition(name="title", path="Composition.title", description="Human Readable name/title", type="string" ) 3088 public static final String SP_TITLE = "title"; 3089 /** 3090 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3091 * <p> 3092 * Description: <b>Human Readable name/title</b><br> 3093 * Type: <b>string</b><br> 3094 * Path: <b>Composition.title</b><br> 3095 * </p> 3096 */ 3097 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3098 3099 /** 3100 * Search parameter: <b>url</b> 3101 * <p> 3102 * Description: <b>The uri that identifies the activity definition</b><br> 3103 * Type: <b>uri</b><br> 3104 * Path: <b>Composition.url</b><br> 3105 * </p> 3106 */ 3107 @SearchParamDefinition(name="url", path="Composition.url", description="The uri that identifies the activity definition", type="uri" ) 3108 public static final String SP_URL = "url"; 3109 /** 3110 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3111 * <p> 3112 * Description: <b>The uri that identifies the activity definition</b><br> 3113 * Type: <b>uri</b><br> 3114 * Path: <b>Composition.url</b><br> 3115 * </p> 3116 */ 3117 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3118 3119 /** 3120 * Search parameter: <b>version</b> 3121 * <p> 3122 * Description: <b>The business version of the activity definition</b><br> 3123 * Type: <b>token</b><br> 3124 * Path: <b>Composition.version</b><br> 3125 * </p> 3126 */ 3127 @SearchParamDefinition(name="version", path="Composition.version", description="The business version of the activity definition", type="token" ) 3128 public static final String SP_VERSION = "version"; 3129 /** 3130 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3131 * <p> 3132 * Description: <b>The business version of the activity definition</b><br> 3133 * Type: <b>token</b><br> 3134 * Path: <b>Composition.version</b><br> 3135 * </p> 3136 */ 3137 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3138 3139 /** 3140 * Search parameter: <b>date</b> 3141 * <p> 3142 * Description: <b>Multiple Resources: 3143 3144* [AdverseEvent](adverseevent.html): When the event occurred 3145* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3146* [Appointment](appointment.html): Appointment date/time. 3147* [AuditEvent](auditevent.html): Time when the event was recorded 3148* [CarePlan](careplan.html): Time period plan covers 3149* [CareTeam](careteam.html): A date within the coverage time period. 3150* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3151* [Composition](composition.html): Composition editing time 3152* [Consent](consent.html): When consent was agreed to 3153* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3154* [DocumentReference](documentreference.html): When this document reference was created 3155* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3156* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3157* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3158* [Flag](flag.html): Time period when flag is active 3159* [Immunization](immunization.html): Vaccination (non)-Administration Date 3160* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3161* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3162* [Invoice](invoice.html): Invoice date / posting date 3163* [List](list.html): When the list was prepared 3164* [MeasureReport](measurereport.html): The date of the measure report 3165* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3166* [Observation](observation.html): Clinically relevant time/time-period for observation 3167* [Procedure](procedure.html): When the procedure occurred or is occurring 3168* [ResearchSubject](researchsubject.html): Start and end of participation 3169* [RiskAssessment](riskassessment.html): When was assessment made? 3170* [SupplyRequest](supplyrequest.html): When the request was made 3171</b><br> 3172 * Type: <b>date</b><br> 3173 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3174 * </p> 3175 */ 3176 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 3177 public static final String SP_DATE = "date"; 3178 /** 3179 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3180 * <p> 3181 * Description: <b>Multiple Resources: 3182 3183* [AdverseEvent](adverseevent.html): When the event occurred 3184* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3185* [Appointment](appointment.html): Appointment date/time. 3186* [AuditEvent](auditevent.html): Time when the event was recorded 3187* [CarePlan](careplan.html): Time period plan covers 3188* [CareTeam](careteam.html): A date within the coverage time period. 3189* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3190* [Composition](composition.html): Composition editing time 3191* [Consent](consent.html): When consent was agreed to 3192* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3193* [DocumentReference](documentreference.html): When this document reference was created 3194* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3195* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3196* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3197* [Flag](flag.html): Time period when flag is active 3198* [Immunization](immunization.html): Vaccination (non)-Administration Date 3199* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3200* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3201* [Invoice](invoice.html): Invoice date / posting date 3202* [List](list.html): When the list was prepared 3203* [MeasureReport](measurereport.html): The date of the measure report 3204* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3205* [Observation](observation.html): Clinically relevant time/time-period for observation 3206* [Procedure](procedure.html): When the procedure occurred or is occurring 3207* [ResearchSubject](researchsubject.html): Start and end of participation 3208* [RiskAssessment](riskassessment.html): When was assessment made? 3209* [SupplyRequest](supplyrequest.html): When the request was made 3210</b><br> 3211 * Type: <b>date</b><br> 3212 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3213 * </p> 3214 */ 3215 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3216 3217 /** 3218 * Search parameter: <b>encounter</b> 3219 * <p> 3220 * Description: <b>Multiple Resources: 3221 3222* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3223* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3224* [ChargeItem](chargeitem.html): Encounter associated with event 3225* [Claim](claim.html): Encounters associated with a billed line item 3226* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3227* [Communication](communication.html): The Encounter during which this Communication was created 3228* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3229* [Composition](composition.html): Context of the Composition 3230* [Condition](condition.html): The Encounter during which this Condition was created 3231* [DeviceRequest](devicerequest.html): Encounter during which request was created 3232* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3233* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3234* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3235* [Flag](flag.html): Alert relevant during encounter 3236* [ImagingStudy](imagingstudy.html): The context of the study 3237* [List](list.html): Context in which list created 3238* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3239* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3240* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3241* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3242* [Observation](observation.html): Encounter related to the observation 3243* [Procedure](procedure.html): The Encounter during which this Procedure was created 3244* [Provenance](provenance.html): Encounter related to the Provenance 3245* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3246* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3247* [RiskAssessment](riskassessment.html): Where was assessment performed? 3248* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3249* [Task](task.html): Search by encounter 3250* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3251</b><br> 3252 * Type: <b>reference</b><br> 3253 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3254 * </p> 3255 */ 3256 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 3257 public static final String SP_ENCOUNTER = "encounter"; 3258 /** 3259 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3260 * <p> 3261 * Description: <b>Multiple Resources: 3262 3263* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3264* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3265* [ChargeItem](chargeitem.html): Encounter associated with event 3266* [Claim](claim.html): Encounters associated with a billed line item 3267* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3268* [Communication](communication.html): The Encounter during which this Communication was created 3269* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3270* [Composition](composition.html): Context of the Composition 3271* [Condition](condition.html): The Encounter during which this Condition was created 3272* [DeviceRequest](devicerequest.html): Encounter during which request was created 3273* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3274* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3275* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3276* [Flag](flag.html): Alert relevant during encounter 3277* [ImagingStudy](imagingstudy.html): The context of the study 3278* [List](list.html): Context in which list created 3279* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3280* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3281* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3282* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3283* [Observation](observation.html): Encounter related to the observation 3284* [Procedure](procedure.html): The Encounter during which this Procedure was created 3285* [Provenance](provenance.html): Encounter related to the Provenance 3286* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3287* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3288* [RiskAssessment](riskassessment.html): Where was assessment performed? 3289* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3290* [Task](task.html): Search by encounter 3291* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3292</b><br> 3293 * Type: <b>reference</b><br> 3294 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3295 * </p> 3296 */ 3297 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3298 3299/** 3300 * Constant for fluent queries to be used to add include statements. Specifies 3301 * the path value of "<b>Composition:encounter</b>". 3302 */ 3303 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Composition:encounter").toLocked(); 3304 3305 /** 3306 * Search parameter: <b>identifier</b> 3307 * <p> 3308 * Description: <b>Multiple Resources: 3309 3310* [Account](account.html): Account number 3311* [AdverseEvent](adverseevent.html): Business identifier for the event 3312* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3313* [Appointment](appointment.html): An Identifier of the Appointment 3314* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3315* [Basic](basic.html): Business identifier 3316* [BodyStructure](bodystructure.html): Bodystructure identifier 3317* [CarePlan](careplan.html): External Ids for this plan 3318* [CareTeam](careteam.html): External Ids for this team 3319* [ChargeItem](chargeitem.html): Business Identifier for item 3320* [Claim](claim.html): The primary identifier of the financial resource 3321* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3322* [ClinicalImpression](clinicalimpression.html): Business identifier 3323* [Communication](communication.html): Unique identifier 3324* [CommunicationRequest](communicationrequest.html): Unique identifier 3325* [Composition](composition.html): Version-independent identifier for the Composition 3326* [Condition](condition.html): A unique identifier of the condition record 3327* [Consent](consent.html): Identifier for this record (external references) 3328* [Contract](contract.html): The identity of the contract 3329* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3330* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3331* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3332* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3333* [DeviceRequest](devicerequest.html): Business identifier for request/order 3334* [DeviceUsage](deviceusage.html): Search by identifier 3335* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3336* [DocumentReference](documentreference.html): Identifier of the attachment binary 3337* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3338* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3339* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3340* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3341* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3342* [Flag](flag.html): Business identifier 3343* [Goal](goal.html): External Ids for this goal 3344* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3345* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3346* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3347* [Immunization](immunization.html): Business identifier 3348* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3349* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3350* [Invoice](invoice.html): Business Identifier for item 3351* [List](list.html): Business identifier 3352* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3353* [Medication](medication.html): Returns medications with this external identifier 3354* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3355* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3356* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3357* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3358* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3359* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3360* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3361* [Observation](observation.html): The unique id for a particular observation 3362* [Person](person.html): A person Identifier 3363* [Procedure](procedure.html): A unique identifier for a procedure 3364* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3365* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3366* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3367* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3368* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3369* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3370* [Specimen](specimen.html): The unique identifier associated with the specimen 3371* [SupplyDelivery](supplydelivery.html): External identifier 3372* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3373* [Task](task.html): Search for a task instance by its business identifier 3374* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3375</b><br> 3376 * Type: <b>token</b><br> 3377 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3378 * </p> 3379 */ 3380 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3381 public static final String SP_IDENTIFIER = "identifier"; 3382 /** 3383 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3384 * <p> 3385 * Description: <b>Multiple Resources: 3386 3387* [Account](account.html): Account number 3388* [AdverseEvent](adverseevent.html): Business identifier for the event 3389* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3390* [Appointment](appointment.html): An Identifier of the Appointment 3391* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3392* [Basic](basic.html): Business identifier 3393* [BodyStructure](bodystructure.html): Bodystructure identifier 3394* [CarePlan](careplan.html): External Ids for this plan 3395* [CareTeam](careteam.html): External Ids for this team 3396* [ChargeItem](chargeitem.html): Business Identifier for item 3397* [Claim](claim.html): The primary identifier of the financial resource 3398* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3399* [ClinicalImpression](clinicalimpression.html): Business identifier 3400* [Communication](communication.html): Unique identifier 3401* [CommunicationRequest](communicationrequest.html): Unique identifier 3402* [Composition](composition.html): Version-independent identifier for the Composition 3403* [Condition](condition.html): A unique identifier of the condition record 3404* [Consent](consent.html): Identifier for this record (external references) 3405* [Contract](contract.html): The identity of the contract 3406* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3407* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3408* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3409* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3410* [DeviceRequest](devicerequest.html): Business identifier for request/order 3411* [DeviceUsage](deviceusage.html): Search by identifier 3412* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3413* [DocumentReference](documentreference.html): Identifier of the attachment binary 3414* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3415* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3416* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3417* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3418* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3419* [Flag](flag.html): Business identifier 3420* [Goal](goal.html): External Ids for this goal 3421* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3422* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3423* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3424* [Immunization](immunization.html): Business identifier 3425* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3426* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3427* [Invoice](invoice.html): Business Identifier for item 3428* [List](list.html): Business identifier 3429* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3430* [Medication](medication.html): Returns medications with this external identifier 3431* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3432* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3433* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3434* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3435* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3436* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3437* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3438* [Observation](observation.html): The unique id for a particular observation 3439* [Person](person.html): A person Identifier 3440* [Procedure](procedure.html): A unique identifier for a procedure 3441* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3442* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3443* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3444* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3445* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3446* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3447* [Specimen](specimen.html): The unique identifier associated with the specimen 3448* [SupplyDelivery](supplydelivery.html): External identifier 3449* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3450* [Task](task.html): Search for a task instance by its business identifier 3451* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3452</b><br> 3453 * Type: <b>token</b><br> 3454 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3455 * </p> 3456 */ 3457 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3458 3459 /** 3460 * Search parameter: <b>patient</b> 3461 * <p> 3462 * Description: <b>Multiple Resources: 3463 3464* [Account](account.html): The entity that caused the expenses 3465* [AdverseEvent](adverseevent.html): Subject impacted by event 3466* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3467* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3468* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3469* [AuditEvent](auditevent.html): Where the activity involved patient data 3470* [Basic](basic.html): Identifies the focus of this resource 3471* [BodyStructure](bodystructure.html): Who this is about 3472* [CarePlan](careplan.html): Who the care plan is for 3473* [CareTeam](careteam.html): Who care team is for 3474* [ChargeItem](chargeitem.html): Individual service was done for/to 3475* [Claim](claim.html): Patient receiving the products or services 3476* [ClaimResponse](claimresponse.html): The subject of care 3477* [ClinicalImpression](clinicalimpression.html): Patient assessed 3478* [Communication](communication.html): Focus of message 3479* [CommunicationRequest](communicationrequest.html): Focus of message 3480* [Composition](composition.html): Who and/or what the composition is about 3481* [Condition](condition.html): Who has the condition? 3482* [Consent](consent.html): Who the consent applies to 3483* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3484* [Coverage](coverage.html): Retrieve coverages for a patient 3485* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3486* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3487* [DetectedIssue](detectedissue.html): Associated patient 3488* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3489* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3490* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3491* [DocumentReference](documentreference.html): Who/what is the subject of the document 3492* [Encounter](encounter.html): The patient present at the encounter 3493* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3494* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3495* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3496* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3497* [Flag](flag.html): The identity of a subject to list flags for 3498* [Goal](goal.html): Who this goal is intended for 3499* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3500* [ImagingSelection](imagingselection.html): Who the study is about 3501* [ImagingStudy](imagingstudy.html): Who the study is about 3502* [Immunization](immunization.html): The patient for the vaccination record 3503* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3504* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3505* [Invoice](invoice.html): Recipient(s) of goods and services 3506* [List](list.html): If all resources have the same subject 3507* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3508* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3509* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3510* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3511* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3512* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3513* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3514* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3515* [Observation](observation.html): The subject that the observation is about (if patient) 3516* [Person](person.html): The Person links to this Patient 3517* [Procedure](procedure.html): Search by subject - a patient 3518* [Provenance](provenance.html): Where the activity involved patient data 3519* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3520* [RelatedPerson](relatedperson.html): The patient this related person is related to 3521* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3522* [ResearchSubject](researchsubject.html): Who or what is part of study 3523* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3524* [ServiceRequest](servicerequest.html): Search by subject - a patient 3525* [Specimen](specimen.html): The patient the specimen comes from 3526* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3527* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3528* [Task](task.html): Search by patient 3529* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3530</b><br> 3531 * Type: <b>reference</b><br> 3532 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3533 * </p> 3534 */ 3535 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3536 public static final String SP_PATIENT = "patient"; 3537 /** 3538 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3539 * <p> 3540 * Description: <b>Multiple Resources: 3541 3542* [Account](account.html): The entity that caused the expenses 3543* [AdverseEvent](adverseevent.html): Subject impacted by event 3544* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3545* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3546* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3547* [AuditEvent](auditevent.html): Where the activity involved patient data 3548* [Basic](basic.html): Identifies the focus of this resource 3549* [BodyStructure](bodystructure.html): Who this is about 3550* [CarePlan](careplan.html): Who the care plan is for 3551* [CareTeam](careteam.html): Who care team is for 3552* [ChargeItem](chargeitem.html): Individual service was done for/to 3553* [Claim](claim.html): Patient receiving the products or services 3554* [ClaimResponse](claimresponse.html): The subject of care 3555* [ClinicalImpression](clinicalimpression.html): Patient assessed 3556* [Communication](communication.html): Focus of message 3557* [CommunicationRequest](communicationrequest.html): Focus of message 3558* [Composition](composition.html): Who and/or what the composition is about 3559* [Condition](condition.html): Who has the condition? 3560* [Consent](consent.html): Who the consent applies to 3561* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3562* [Coverage](coverage.html): Retrieve coverages for a patient 3563* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3564* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3565* [DetectedIssue](detectedissue.html): Associated patient 3566* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3567* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3568* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3569* [DocumentReference](documentreference.html): Who/what is the subject of the document 3570* [Encounter](encounter.html): The patient present at the encounter 3571* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3572* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3573* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3574* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3575* [Flag](flag.html): The identity of a subject to list flags for 3576* [Goal](goal.html): Who this goal is intended for 3577* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3578* [ImagingSelection](imagingselection.html): Who the study is about 3579* [ImagingStudy](imagingstudy.html): Who the study is about 3580* [Immunization](immunization.html): The patient for the vaccination record 3581* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3582* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3583* [Invoice](invoice.html): Recipient(s) of goods and services 3584* [List](list.html): If all resources have the same subject 3585* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3586* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3587* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3588* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3589* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3590* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3591* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3592* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3593* [Observation](observation.html): The subject that the observation is about (if patient) 3594* [Person](person.html): The Person links to this Patient 3595* [Procedure](procedure.html): Search by subject - a patient 3596* [Provenance](provenance.html): Where the activity involved patient data 3597* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3598* [RelatedPerson](relatedperson.html): The patient this related person is related to 3599* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3600* [ResearchSubject](researchsubject.html): Who or what is part of study 3601* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3602* [ServiceRequest](servicerequest.html): Search by subject - a patient 3603* [Specimen](specimen.html): The patient the specimen comes from 3604* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3605* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3606* [Task](task.html): Search by patient 3607* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3608</b><br> 3609 * Type: <b>reference</b><br> 3610 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3611 * </p> 3612 */ 3613 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3614 3615/** 3616 * Constant for fluent queries to be used to add include statements. Specifies 3617 * the path value of "<b>Composition:patient</b>". 3618 */ 3619 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Composition:patient").toLocked(); 3620 3621 /** 3622 * Search parameter: <b>type</b> 3623 * <p> 3624 * Description: <b>Multiple Resources: 3625 3626* [Account](account.html): E.g. patient, expense, depreciation 3627* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3628* [Composition](composition.html): Kind of composition (LOINC if possible) 3629* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3630* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3631* [Encounter](encounter.html): Specific type of encounter 3632* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3633* [Invoice](invoice.html): Type of Invoice 3634* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3635* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3636* [Specimen](specimen.html): The specimen type 3637</b><br> 3638 * Type: <b>token</b><br> 3639 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3640 * </p> 3641 */ 3642 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 3643 public static final String SP_TYPE = "type"; 3644 /** 3645 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3646 * <p> 3647 * Description: <b>Multiple Resources: 3648 3649* [Account](account.html): E.g. patient, expense, depreciation 3650* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3651* [Composition](composition.html): Kind of composition (LOINC if possible) 3652* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3653* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3654* [Encounter](encounter.html): Specific type of encounter 3655* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3656* [Invoice](invoice.html): Type of Invoice 3657* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3658* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3659* [Specimen](specimen.html): The specimen type 3660</b><br> 3661 * Type: <b>token</b><br> 3662 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3663 * </p> 3664 */ 3665 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3666 3667 3668} 3669