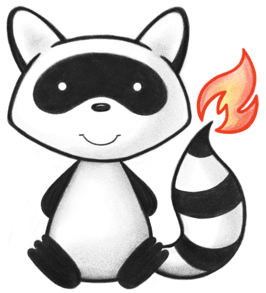
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models. 052 */ 053@ResourceDef(name="ConceptMap", profile="http://hl7.org/fhir/StructureDefinition/ConceptMap") 054public class ConceptMap extends MetadataResource { 055 056 public enum ConceptMapAttributeType { 057 /** 058 * The attribute value is a code defined in the code system in context. 059 */ 060 CODE, 061 /** 062 * The attribute value is a code defined in a code system. 063 */ 064 CODING, 065 /** 066 * The attribute value is a string. 067 */ 068 STRING, 069 /** 070 * The attribute value is a boolean true | false. 071 */ 072 BOOLEAN, 073 /** 074 * The attribute is a Quantity (may represent an integer or a decimal with no units). 075 */ 076 QUANTITY, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static ConceptMapAttributeType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("code".equals(codeString)) 085 return CODE; 086 if ("Coding".equals(codeString)) 087 return CODING; 088 if ("string".equals(codeString)) 089 return STRING; 090 if ("boolean".equals(codeString)) 091 return BOOLEAN; 092 if ("Quantity".equals(codeString)) 093 return QUANTITY; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown ConceptMapAttributeType code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case CODE: return "code"; 102 case CODING: return "Coding"; 103 case STRING: return "string"; 104 case BOOLEAN: return "boolean"; 105 case QUANTITY: return "Quantity"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case CODE: return "http://hl7.org/fhir/conceptmap-attribute-type"; 113 case CODING: return "http://hl7.org/fhir/conceptmap-attribute-type"; 114 case STRING: return "http://hl7.org/fhir/conceptmap-attribute-type"; 115 case BOOLEAN: return "http://hl7.org/fhir/conceptmap-attribute-type"; 116 case QUANTITY: return "http://hl7.org/fhir/conceptmap-attribute-type"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case CODE: return "The attribute value is a code defined in the code system in context."; 124 case CODING: return "The attribute value is a code defined in a code system."; 125 case STRING: return "The attribute value is a string."; 126 case BOOLEAN: return "The attribute value is a boolean true | false."; 127 case QUANTITY: return "The attribute is a Quantity (may represent an integer or a decimal with no units)."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case CODE: return "code"; 135 case CODING: return "Coding"; 136 case STRING: return "string"; 137 case BOOLEAN: return "boolean"; 138 case QUANTITY: return "Quantity"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class ConceptMapAttributeTypeEnumFactory implements EnumFactory<ConceptMapAttributeType> { 146 public ConceptMapAttributeType fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("code".equals(codeString)) 151 return ConceptMapAttributeType.CODE; 152 if ("Coding".equals(codeString)) 153 return ConceptMapAttributeType.CODING; 154 if ("string".equals(codeString)) 155 return ConceptMapAttributeType.STRING; 156 if ("boolean".equals(codeString)) 157 return ConceptMapAttributeType.BOOLEAN; 158 if ("Quantity".equals(codeString)) 159 return ConceptMapAttributeType.QUANTITY; 160 throw new IllegalArgumentException("Unknown ConceptMapAttributeType code '"+codeString+"'"); 161 } 162 public Enumeration<ConceptMapAttributeType> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.NULL, code); 170 if ("code".equals(codeString)) 171 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.CODE, code); 172 if ("Coding".equals(codeString)) 173 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.CODING, code); 174 if ("string".equals(codeString)) 175 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.STRING, code); 176 if ("boolean".equals(codeString)) 177 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.BOOLEAN, code); 178 if ("Quantity".equals(codeString)) 179 return new Enumeration<ConceptMapAttributeType>(this, ConceptMapAttributeType.QUANTITY, code); 180 throw new FHIRException("Unknown ConceptMapAttributeType code '"+codeString+"'"); 181 } 182 public String toCode(ConceptMapAttributeType code) { 183 if (code == ConceptMapAttributeType.NULL) 184 return null; 185 if (code == ConceptMapAttributeType.CODE) 186 return "code"; 187 if (code == ConceptMapAttributeType.CODING) 188 return "Coding"; 189 if (code == ConceptMapAttributeType.STRING) 190 return "string"; 191 if (code == ConceptMapAttributeType.BOOLEAN) 192 return "boolean"; 193 if (code == ConceptMapAttributeType.QUANTITY) 194 return "Quantity"; 195 return "?"; 196 } 197 public String toSystem(ConceptMapAttributeType code) { 198 return code.getSystem(); 199 } 200 } 201 202 public enum ConceptMapGroupUnmappedMode { 203 /** 204 * Use the code as provided in the $translate request in one of the following input parameters: sourceCode, sourceCoding, sourceCodeableConcept. 205 */ 206 USESOURCECODE, 207 /** 208 * Use the code(s) explicitly provided in the group.unmapped 'code' or 'valueSet' element. 209 */ 210 FIXED, 211 /** 212 * Use the map identified by the canonical URL in the url element. 213 */ 214 OTHERMAP, 215 /** 216 * added to help the parsers with the generic types 217 */ 218 NULL; 219 public static ConceptMapGroupUnmappedMode fromCode(String codeString) throws FHIRException { 220 if (codeString == null || "".equals(codeString)) 221 return null; 222 if ("use-source-code".equals(codeString)) 223 return USESOURCECODE; 224 if ("fixed".equals(codeString)) 225 return FIXED; 226 if ("other-map".equals(codeString)) 227 return OTHERMAP; 228 if (Configuration.isAcceptInvalidEnums()) 229 return null; 230 else 231 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '"+codeString+"'"); 232 } 233 public String toCode() { 234 switch (this) { 235 case USESOURCECODE: return "use-source-code"; 236 case FIXED: return "fixed"; 237 case OTHERMAP: return "other-map"; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 public String getSystem() { 243 switch (this) { 244 case USESOURCECODE: return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 245 case FIXED: return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 246 case OTHERMAP: return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 247 case NULL: return null; 248 default: return "?"; 249 } 250 } 251 public String getDefinition() { 252 switch (this) { 253 case USESOURCECODE: return "Use the code as provided in the $translate request in one of the following input parameters: sourceCode, sourceCoding, sourceCodeableConcept."; 254 case FIXED: return "Use the code(s) explicitly provided in the group.unmapped 'code' or 'valueSet' element."; 255 case OTHERMAP: return "Use the map identified by the canonical URL in the url element."; 256 case NULL: return null; 257 default: return "?"; 258 } 259 } 260 public String getDisplay() { 261 switch (this) { 262 case USESOURCECODE: return "Use Provided Source Code"; 263 case FIXED: return "Fixed Code"; 264 case OTHERMAP: return "Other Map"; 265 case NULL: return null; 266 default: return "?"; 267 } 268 } 269 } 270 271 public static class ConceptMapGroupUnmappedModeEnumFactory implements EnumFactory<ConceptMapGroupUnmappedMode> { 272 public ConceptMapGroupUnmappedMode fromCode(String codeString) throws IllegalArgumentException { 273 if (codeString == null || "".equals(codeString)) 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("use-source-code".equals(codeString)) 277 return ConceptMapGroupUnmappedMode.USESOURCECODE; 278 if ("fixed".equals(codeString)) 279 return ConceptMapGroupUnmappedMode.FIXED; 280 if ("other-map".equals(codeString)) 281 return ConceptMapGroupUnmappedMode.OTHERMAP; 282 throw new IllegalArgumentException("Unknown ConceptMapGroupUnmappedMode code '"+codeString+"'"); 283 } 284 public Enumeration<ConceptMapGroupUnmappedMode> fromType(PrimitiveType<?> code) throws FHIRException { 285 if (code == null) 286 return null; 287 if (code.isEmpty()) 288 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.NULL, code); 289 String codeString = ((PrimitiveType) code).asStringValue(); 290 if (codeString == null || "".equals(codeString)) 291 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.NULL, code); 292 if ("use-source-code".equals(codeString)) 293 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.USESOURCECODE, code); 294 if ("fixed".equals(codeString)) 295 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.FIXED, code); 296 if ("other-map".equals(codeString)) 297 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.OTHERMAP, code); 298 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '"+codeString+"'"); 299 } 300 public String toCode(ConceptMapGroupUnmappedMode code) { 301 if (code == ConceptMapGroupUnmappedMode.NULL) 302 return null; 303 if (code == ConceptMapGroupUnmappedMode.USESOURCECODE) 304 return "use-source-code"; 305 if (code == ConceptMapGroupUnmappedMode.FIXED) 306 return "fixed"; 307 if (code == ConceptMapGroupUnmappedMode.OTHERMAP) 308 return "other-map"; 309 return "?"; 310 } 311 public String toSystem(ConceptMapGroupUnmappedMode code) { 312 return code.getSystem(); 313 } 314 } 315 316 public enum ConceptMapPropertyType { 317 /** 318 * The property value is a code defined in an external code system. This may be used for translations, but is not the intent. 319 */ 320 CODING, 321 /** 322 * The property value is a string. 323 */ 324 STRING, 325 /** 326 * The property value is an integer (often used to assign ranking values to concepts for supporting score assessments). 327 */ 328 INTEGER, 329 /** 330 * The property value is a boolean true | false. 331 */ 332 BOOLEAN, 333 /** 334 * The property is a date or a date + time. 335 */ 336 DATETIME, 337 /** 338 * The property value is a decimal number. 339 */ 340 DECIMAL, 341 /** 342 * The property value is a code as defined in the CodeSystem in ConceptMap.property.system. 343 */ 344 CODE, 345 /** 346 * added to help the parsers with the generic types 347 */ 348 NULL; 349 public static ConceptMapPropertyType fromCode(String codeString) throws FHIRException { 350 if (codeString == null || "".equals(codeString)) 351 return null; 352 if ("Coding".equals(codeString)) 353 return CODING; 354 if ("string".equals(codeString)) 355 return STRING; 356 if ("integer".equals(codeString)) 357 return INTEGER; 358 if ("boolean".equals(codeString)) 359 return BOOLEAN; 360 if ("dateTime".equals(codeString)) 361 return DATETIME; 362 if ("decimal".equals(codeString)) 363 return DECIMAL; 364 if ("code".equals(codeString)) 365 return CODE; 366 if (Configuration.isAcceptInvalidEnums()) 367 return null; 368 else 369 throw new FHIRException("Unknown ConceptMapPropertyType code '"+codeString+"'"); 370 } 371 public String toCode() { 372 switch (this) { 373 case CODING: return "Coding"; 374 case STRING: return "string"; 375 case INTEGER: return "integer"; 376 case BOOLEAN: return "boolean"; 377 case DATETIME: return "dateTime"; 378 case DECIMAL: return "decimal"; 379 case CODE: return "code"; 380 case NULL: return null; 381 default: return "?"; 382 } 383 } 384 public String getSystem() { 385 switch (this) { 386 case CODING: return "http://hl7.org/fhir/conceptmap-property-type"; 387 case STRING: return "http://hl7.org/fhir/conceptmap-property-type"; 388 case INTEGER: return "http://hl7.org/fhir/conceptmap-property-type"; 389 case BOOLEAN: return "http://hl7.org/fhir/conceptmap-property-type"; 390 case DATETIME: return "http://hl7.org/fhir/conceptmap-property-type"; 391 case DECIMAL: return "http://hl7.org/fhir/conceptmap-property-type"; 392 case CODE: return "http://hl7.org/fhir/conceptmap-property-type"; 393 case NULL: return null; 394 default: return "?"; 395 } 396 } 397 public String getDefinition() { 398 switch (this) { 399 case CODING: return "The property value is a code defined in an external code system. This may be used for translations, but is not the intent."; 400 case STRING: return "The property value is a string."; 401 case INTEGER: return "The property value is an integer (often used to assign ranking values to concepts for supporting score assessments)."; 402 case BOOLEAN: return "The property value is a boolean true | false."; 403 case DATETIME: return "The property is a date or a date + time."; 404 case DECIMAL: return "The property value is a decimal number."; 405 case CODE: return "The property value is a code as defined in the CodeSystem in ConceptMap.property.system."; 406 case NULL: return null; 407 default: return "?"; 408 } 409 } 410 public String getDisplay() { 411 switch (this) { 412 case CODING: return "Coding (external reference)"; 413 case STRING: return "string"; 414 case INTEGER: return "integer"; 415 case BOOLEAN: return "boolean"; 416 case DATETIME: return "dateTime"; 417 case DECIMAL: return "decimal"; 418 case CODE: return "code"; 419 case NULL: return null; 420 default: return "?"; 421 } 422 } 423 } 424 425 public static class ConceptMapPropertyTypeEnumFactory implements EnumFactory<ConceptMapPropertyType> { 426 public ConceptMapPropertyType fromCode(String codeString) throws IllegalArgumentException { 427 if (codeString == null || "".equals(codeString)) 428 if (codeString == null || "".equals(codeString)) 429 return null; 430 if ("Coding".equals(codeString)) 431 return ConceptMapPropertyType.CODING; 432 if ("string".equals(codeString)) 433 return ConceptMapPropertyType.STRING; 434 if ("integer".equals(codeString)) 435 return ConceptMapPropertyType.INTEGER; 436 if ("boolean".equals(codeString)) 437 return ConceptMapPropertyType.BOOLEAN; 438 if ("dateTime".equals(codeString)) 439 return ConceptMapPropertyType.DATETIME; 440 if ("decimal".equals(codeString)) 441 return ConceptMapPropertyType.DECIMAL; 442 if ("code".equals(codeString)) 443 return ConceptMapPropertyType.CODE; 444 throw new IllegalArgumentException("Unknown ConceptMapPropertyType code '"+codeString+"'"); 445 } 446 public Enumeration<ConceptMapPropertyType> fromType(PrimitiveType<?> code) throws FHIRException { 447 if (code == null) 448 return null; 449 if (code.isEmpty()) 450 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.NULL, code); 451 String codeString = ((PrimitiveType) code).asStringValue(); 452 if (codeString == null || "".equals(codeString)) 453 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.NULL, code); 454 if ("Coding".equals(codeString)) 455 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.CODING, code); 456 if ("string".equals(codeString)) 457 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.STRING, code); 458 if ("integer".equals(codeString)) 459 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.INTEGER, code); 460 if ("boolean".equals(codeString)) 461 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.BOOLEAN, code); 462 if ("dateTime".equals(codeString)) 463 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.DATETIME, code); 464 if ("decimal".equals(codeString)) 465 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.DECIMAL, code); 466 if ("code".equals(codeString)) 467 return new Enumeration<ConceptMapPropertyType>(this, ConceptMapPropertyType.CODE, code); 468 throw new FHIRException("Unknown ConceptMapPropertyType code '"+codeString+"'"); 469 } 470 public String toCode(ConceptMapPropertyType code) { 471 if (code == ConceptMapPropertyType.NULL) 472 return null; 473 if (code == ConceptMapPropertyType.CODING) 474 return "Coding"; 475 if (code == ConceptMapPropertyType.STRING) 476 return "string"; 477 if (code == ConceptMapPropertyType.INTEGER) 478 return "integer"; 479 if (code == ConceptMapPropertyType.BOOLEAN) 480 return "boolean"; 481 if (code == ConceptMapPropertyType.DATETIME) 482 return "dateTime"; 483 if (code == ConceptMapPropertyType.DECIMAL) 484 return "decimal"; 485 if (code == ConceptMapPropertyType.CODE) 486 return "code"; 487 return "?"; 488 } 489 public String toSystem(ConceptMapPropertyType code) { 490 return code.getSystem(); 491 } 492 } 493 494 @Block() 495 public static class PropertyComponent extends BackboneElement implements IBaseBackboneElement { 496 /** 497 * A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation. 498 */ 499 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 500 @Description(shortDefinition="Identifies the property on the mappings, and when referred to in the $translate operation", formalDefinition="A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation." ) 501 protected CodeType code; 502 503 /** 504 * Reference to the formal meaning of the property. 505 */ 506 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 507 @Description(shortDefinition="Formal identifier for the property", formalDefinition="Reference to the formal meaning of the property." ) 508 protected UriType uri; 509 510 /** 511 * A description of the property - why it is defined, and how its value might be used. 512 */ 513 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 514 @Description(shortDefinition="Why the property is defined, and/or what it conveys", formalDefinition="A description of the property - why it is defined, and how its value might be used." ) 515 protected StringType description; 516 517 /** 518 * The type of the property value. 519 */ 520 @Child(name = "type", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 521 @Description(shortDefinition="Coding | string | integer | boolean | dateTime | decimal | code", formalDefinition="The type of the property value." ) 522 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conceptmap-property-type") 523 protected Enumeration<ConceptMapPropertyType> type; 524 525 /** 526 * The CodeSystem that defines the codes from which values of type ```code``` in property values. 527 */ 528 @Child(name = "system", type = {CanonicalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 529 @Description(shortDefinition="The CodeSystem from which code values come", formalDefinition="The CodeSystem that defines the codes from which values of type ```code``` in property values." ) 530 protected CanonicalType system; 531 532 private static final long serialVersionUID = 1693472150L; 533 534 /** 535 * Constructor 536 */ 537 public PropertyComponent() { 538 super(); 539 } 540 541 /** 542 * Constructor 543 */ 544 public PropertyComponent(String code, ConceptMapPropertyType type) { 545 super(); 546 this.setCode(code); 547 this.setType(type); 548 } 549 550 /** 551 * @return {@link #code} (A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 552 */ 553 public CodeType getCodeElement() { 554 if (this.code == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create PropertyComponent.code"); 557 else if (Configuration.doAutoCreate()) 558 this.code = new CodeType(); // bb 559 return this.code; 560 } 561 562 public boolean hasCodeElement() { 563 return this.code != null && !this.code.isEmpty(); 564 } 565 566 public boolean hasCode() { 567 return this.code != null && !this.code.isEmpty(); 568 } 569 570 /** 571 * @param value {@link #code} (A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 572 */ 573 public PropertyComponent setCodeElement(CodeType value) { 574 this.code = value; 575 return this; 576 } 577 578 /** 579 * @return A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation. 580 */ 581 public String getCode() { 582 return this.code == null ? null : this.code.getValue(); 583 } 584 585 /** 586 * @param value A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation. 587 */ 588 public PropertyComponent setCode(String value) { 589 if (this.code == null) 590 this.code = new CodeType(); 591 this.code.setValue(value); 592 return this; 593 } 594 595 /** 596 * @return {@link #uri} (Reference to the formal meaning of the property.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 597 */ 598 public UriType getUriElement() { 599 if (this.uri == null) 600 if (Configuration.errorOnAutoCreate()) 601 throw new Error("Attempt to auto-create PropertyComponent.uri"); 602 else if (Configuration.doAutoCreate()) 603 this.uri = new UriType(); // bb 604 return this.uri; 605 } 606 607 public boolean hasUriElement() { 608 return this.uri != null && !this.uri.isEmpty(); 609 } 610 611 public boolean hasUri() { 612 return this.uri != null && !this.uri.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #uri} (Reference to the formal meaning of the property.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 617 */ 618 public PropertyComponent setUriElement(UriType value) { 619 this.uri = value; 620 return this; 621 } 622 623 /** 624 * @return Reference to the formal meaning of the property. 625 */ 626 public String getUri() { 627 return this.uri == null ? null : this.uri.getValue(); 628 } 629 630 /** 631 * @param value Reference to the formal meaning of the property. 632 */ 633 public PropertyComponent setUri(String value) { 634 if (Utilities.noString(value)) 635 this.uri = null; 636 else { 637 if (this.uri == null) 638 this.uri = new UriType(); 639 this.uri.setValue(value); 640 } 641 return this; 642 } 643 644 /** 645 * @return {@link #description} (A description of the property - why it is defined, and how its value might be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 646 */ 647 public StringType getDescriptionElement() { 648 if (this.description == null) 649 if (Configuration.errorOnAutoCreate()) 650 throw new Error("Attempt to auto-create PropertyComponent.description"); 651 else if (Configuration.doAutoCreate()) 652 this.description = new StringType(); // bb 653 return this.description; 654 } 655 656 public boolean hasDescriptionElement() { 657 return this.description != null && !this.description.isEmpty(); 658 } 659 660 public boolean hasDescription() { 661 return this.description != null && !this.description.isEmpty(); 662 } 663 664 /** 665 * @param value {@link #description} (A description of the property - why it is defined, and how its value might be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 666 */ 667 public PropertyComponent setDescriptionElement(StringType value) { 668 this.description = value; 669 return this; 670 } 671 672 /** 673 * @return A description of the property - why it is defined, and how its value might be used. 674 */ 675 public String getDescription() { 676 return this.description == null ? null : this.description.getValue(); 677 } 678 679 /** 680 * @param value A description of the property - why it is defined, and how its value might be used. 681 */ 682 public PropertyComponent setDescription(String value) { 683 if (Utilities.noString(value)) 684 this.description = null; 685 else { 686 if (this.description == null) 687 this.description = new StringType(); 688 this.description.setValue(value); 689 } 690 return this; 691 } 692 693 /** 694 * @return {@link #type} (The type of the property value.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 695 */ 696 public Enumeration<ConceptMapPropertyType> getTypeElement() { 697 if (this.type == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create PropertyComponent.type"); 700 else if (Configuration.doAutoCreate()) 701 this.type = new Enumeration<ConceptMapPropertyType>(new ConceptMapPropertyTypeEnumFactory()); // bb 702 return this.type; 703 } 704 705 public boolean hasTypeElement() { 706 return this.type != null && !this.type.isEmpty(); 707 } 708 709 public boolean hasType() { 710 return this.type != null && !this.type.isEmpty(); 711 } 712 713 /** 714 * @param value {@link #type} (The type of the property value.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 715 */ 716 public PropertyComponent setTypeElement(Enumeration<ConceptMapPropertyType> value) { 717 this.type = value; 718 return this; 719 } 720 721 /** 722 * @return The type of the property value. 723 */ 724 public ConceptMapPropertyType getType() { 725 return this.type == null ? null : this.type.getValue(); 726 } 727 728 /** 729 * @param value The type of the property value. 730 */ 731 public PropertyComponent setType(ConceptMapPropertyType value) { 732 if (this.type == null) 733 this.type = new Enumeration<ConceptMapPropertyType>(new ConceptMapPropertyTypeEnumFactory()); 734 this.type.setValue(value); 735 return this; 736 } 737 738 /** 739 * @return {@link #system} (The CodeSystem that defines the codes from which values of type ```code``` in property values.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 740 */ 741 public CanonicalType getSystemElement() { 742 if (this.system == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create PropertyComponent.system"); 745 else if (Configuration.doAutoCreate()) 746 this.system = new CanonicalType(); // bb 747 return this.system; 748 } 749 750 public boolean hasSystemElement() { 751 return this.system != null && !this.system.isEmpty(); 752 } 753 754 public boolean hasSystem() { 755 return this.system != null && !this.system.isEmpty(); 756 } 757 758 /** 759 * @param value {@link #system} (The CodeSystem that defines the codes from which values of type ```code``` in property values.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 760 */ 761 public PropertyComponent setSystemElement(CanonicalType value) { 762 this.system = value; 763 return this; 764 } 765 766 /** 767 * @return The CodeSystem that defines the codes from which values of type ```code``` in property values. 768 */ 769 public String getSystem() { 770 return this.system == null ? null : this.system.getValue(); 771 } 772 773 /** 774 * @param value The CodeSystem that defines the codes from which values of type ```code``` in property values. 775 */ 776 public PropertyComponent setSystem(String value) { 777 if (Utilities.noString(value)) 778 this.system = null; 779 else { 780 if (this.system == null) 781 this.system = new CanonicalType(); 782 this.system.setValue(value); 783 } 784 return this; 785 } 786 787 protected void listChildren(List<Property> children) { 788 super.listChildren(children); 789 children.add(new Property("code", "code", "A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation.", 0, 1, code)); 790 children.add(new Property("uri", "uri", "Reference to the formal meaning of the property.", 0, 1, uri)); 791 children.add(new Property("description", "string", "A description of the property - why it is defined, and how its value might be used.", 0, 1, description)); 792 children.add(new Property("type", "code", "The type of the property value.", 0, 1, type)); 793 children.add(new Property("system", "canonical(CodeSystem)", "The CodeSystem that defines the codes from which values of type ```code``` in property values.", 0, 1, system)); 794 } 795 796 @Override 797 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 798 switch (_hash) { 799 case 3059181: /*code*/ return new Property("code", "code", "A code that is used to identify the property. The code is used internally (in ConceptMap.group.element.target.property.code) and also in the $translate operation.", 0, 1, code); 800 case 116076: /*uri*/ return new Property("uri", "uri", "Reference to the formal meaning of the property.", 0, 1, uri); 801 case -1724546052: /*description*/ return new Property("description", "string", "A description of the property - why it is defined, and how its value might be used.", 0, 1, description); 802 case 3575610: /*type*/ return new Property("type", "code", "The type of the property value.", 0, 1, type); 803 case -887328209: /*system*/ return new Property("system", "canonical(CodeSystem)", "The CodeSystem that defines the codes from which values of type ```code``` in property values.", 0, 1, system); 804 default: return super.getNamedProperty(_hash, _name, _checkValid); 805 } 806 807 } 808 809 @Override 810 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 811 switch (hash) { 812 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 813 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 814 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 815 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConceptMapPropertyType> 816 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // CanonicalType 817 default: return super.getProperty(hash, name, checkValid); 818 } 819 820 } 821 822 @Override 823 public Base setProperty(int hash, String name, Base value) throws FHIRException { 824 switch (hash) { 825 case 3059181: // code 826 this.code = TypeConvertor.castToCode(value); // CodeType 827 return value; 828 case 116076: // uri 829 this.uri = TypeConvertor.castToUri(value); // UriType 830 return value; 831 case -1724546052: // description 832 this.description = TypeConvertor.castToString(value); // StringType 833 return value; 834 case 3575610: // type 835 value = new ConceptMapPropertyTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 836 this.type = (Enumeration) value; // Enumeration<ConceptMapPropertyType> 837 return value; 838 case -887328209: // system 839 this.system = TypeConvertor.castToCanonical(value); // CanonicalType 840 return value; 841 default: return super.setProperty(hash, name, value); 842 } 843 844 } 845 846 @Override 847 public Base setProperty(String name, Base value) throws FHIRException { 848 if (name.equals("code")) { 849 this.code = TypeConvertor.castToCode(value); // CodeType 850 } else if (name.equals("uri")) { 851 this.uri = TypeConvertor.castToUri(value); // UriType 852 } else if (name.equals("description")) { 853 this.description = TypeConvertor.castToString(value); // StringType 854 } else if (name.equals("type")) { 855 value = new ConceptMapPropertyTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 856 this.type = (Enumeration) value; // Enumeration<ConceptMapPropertyType> 857 } else if (name.equals("system")) { 858 this.system = TypeConvertor.castToCanonical(value); // CanonicalType 859 } else 860 return super.setProperty(name, value); 861 return value; 862 } 863 864 @Override 865 public void removeChild(String name, Base value) throws FHIRException { 866 if (name.equals("code")) { 867 this.code = null; 868 } else if (name.equals("uri")) { 869 this.uri = null; 870 } else if (name.equals("description")) { 871 this.description = null; 872 } else if (name.equals("type")) { 873 value = new ConceptMapPropertyTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 874 this.type = (Enumeration) value; // Enumeration<ConceptMapPropertyType> 875 } else if (name.equals("system")) { 876 this.system = null; 877 } else 878 super.removeChild(name, value); 879 880 } 881 882 @Override 883 public Base makeProperty(int hash, String name) throws FHIRException { 884 switch (hash) { 885 case 3059181: return getCodeElement(); 886 case 116076: return getUriElement(); 887 case -1724546052: return getDescriptionElement(); 888 case 3575610: return getTypeElement(); 889 case -887328209: return getSystemElement(); 890 default: return super.makeProperty(hash, name); 891 } 892 893 } 894 895 @Override 896 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 897 switch (hash) { 898 case 3059181: /*code*/ return new String[] {"code"}; 899 case 116076: /*uri*/ return new String[] {"uri"}; 900 case -1724546052: /*description*/ return new String[] {"string"}; 901 case 3575610: /*type*/ return new String[] {"code"}; 902 case -887328209: /*system*/ return new String[] {"canonical"}; 903 default: return super.getTypesForProperty(hash, name); 904 } 905 906 } 907 908 @Override 909 public Base addChild(String name) throws FHIRException { 910 if (name.equals("code")) { 911 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property.code"); 912 } 913 else if (name.equals("uri")) { 914 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property.uri"); 915 } 916 else if (name.equals("description")) { 917 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property.description"); 918 } 919 else if (name.equals("type")) { 920 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property.type"); 921 } 922 else if (name.equals("system")) { 923 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property.system"); 924 } 925 else 926 return super.addChild(name); 927 } 928 929 public PropertyComponent copy() { 930 PropertyComponent dst = new PropertyComponent(); 931 copyValues(dst); 932 return dst; 933 } 934 935 public void copyValues(PropertyComponent dst) { 936 super.copyValues(dst); 937 dst.code = code == null ? null : code.copy(); 938 dst.uri = uri == null ? null : uri.copy(); 939 dst.description = description == null ? null : description.copy(); 940 dst.type = type == null ? null : type.copy(); 941 dst.system = system == null ? null : system.copy(); 942 } 943 944 @Override 945 public boolean equalsDeep(Base other_) { 946 if (!super.equalsDeep(other_)) 947 return false; 948 if (!(other_ instanceof PropertyComponent)) 949 return false; 950 PropertyComponent o = (PropertyComponent) other_; 951 return compareDeep(code, o.code, true) && compareDeep(uri, o.uri, true) && compareDeep(description, o.description, true) 952 && compareDeep(type, o.type, true) && compareDeep(system, o.system, true); 953 } 954 955 @Override 956 public boolean equalsShallow(Base other_) { 957 if (!super.equalsShallow(other_)) 958 return false; 959 if (!(other_ instanceof PropertyComponent)) 960 return false; 961 PropertyComponent o = (PropertyComponent) other_; 962 return compareValues(code, o.code, true) && compareValues(uri, o.uri, true) && compareValues(description, o.description, true) 963 && compareValues(type, o.type, true) && compareValues(system, o.system, true); 964 } 965 966 public boolean isEmpty() { 967 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, uri, description, type 968 , system); 969 } 970 971 public String fhirType() { 972 return "ConceptMap.property"; 973 974 } 975 976 } 977 978 @Block() 979 public static class AdditionalAttributeComponent extends BackboneElement implements IBaseBackboneElement { 980 /** 981 * A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute. 982 */ 983 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 984 @Description(shortDefinition="Identifies this additional attribute through this resource", formalDefinition="A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute." ) 985 protected CodeType code; 986 987 /** 988 * Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}. 989 */ 990 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 991 @Description(shortDefinition="Formal identifier for the data element referred to in this attribte", formalDefinition="Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}." ) 992 protected UriType uri; 993 994 /** 995 * A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element. 996 */ 997 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 998 @Description(shortDefinition="Why the additional attribute is defined, and/or what the data element it refers to is", formalDefinition="A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element." ) 999 protected StringType description; 1000 1001 /** 1002 * The type of the source data contained in this concept map for this data element. 1003 */ 1004 @Child(name = "type", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1005 @Description(shortDefinition="code | Coding | string | boolean | Quantity", formalDefinition="The type of the source data contained in this concept map for this data element." ) 1006 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conceptmap-attribute-type") 1007 protected Enumeration<ConceptMapAttributeType> type; 1008 1009 private static final long serialVersionUID = -1930701116L; 1010 1011 /** 1012 * Constructor 1013 */ 1014 public AdditionalAttributeComponent() { 1015 super(); 1016 } 1017 1018 /** 1019 * Constructor 1020 */ 1021 public AdditionalAttributeComponent(String code, ConceptMapAttributeType type) { 1022 super(); 1023 this.setCode(code); 1024 this.setType(type); 1025 } 1026 1027 /** 1028 * @return {@link #code} (A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1029 */ 1030 public CodeType getCodeElement() { 1031 if (this.code == null) 1032 if (Configuration.errorOnAutoCreate()) 1033 throw new Error("Attempt to auto-create AdditionalAttributeComponent.code"); 1034 else if (Configuration.doAutoCreate()) 1035 this.code = new CodeType(); // bb 1036 return this.code; 1037 } 1038 1039 public boolean hasCodeElement() { 1040 return this.code != null && !this.code.isEmpty(); 1041 } 1042 1043 public boolean hasCode() { 1044 return this.code != null && !this.code.isEmpty(); 1045 } 1046 1047 /** 1048 * @param value {@link #code} (A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1049 */ 1050 public AdditionalAttributeComponent setCodeElement(CodeType value) { 1051 this.code = value; 1052 return this; 1053 } 1054 1055 /** 1056 * @return A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute. 1057 */ 1058 public String getCode() { 1059 return this.code == null ? null : this.code.getValue(); 1060 } 1061 1062 /** 1063 * @param value A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute. 1064 */ 1065 public AdditionalAttributeComponent setCode(String value) { 1066 if (this.code == null) 1067 this.code = new CodeType(); 1068 this.code.setValue(value); 1069 return this; 1070 } 1071 1072 /** 1073 * @return {@link #uri} (Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 1074 */ 1075 public UriType getUriElement() { 1076 if (this.uri == null) 1077 if (Configuration.errorOnAutoCreate()) 1078 throw new Error("Attempt to auto-create AdditionalAttributeComponent.uri"); 1079 else if (Configuration.doAutoCreate()) 1080 this.uri = new UriType(); // bb 1081 return this.uri; 1082 } 1083 1084 public boolean hasUriElement() { 1085 return this.uri != null && !this.uri.isEmpty(); 1086 } 1087 1088 public boolean hasUri() { 1089 return this.uri != null && !this.uri.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #uri} (Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 1094 */ 1095 public AdditionalAttributeComponent setUriElement(UriType value) { 1096 this.uri = value; 1097 return this; 1098 } 1099 1100 /** 1101 * @return Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}. 1102 */ 1103 public String getUri() { 1104 return this.uri == null ? null : this.uri.getValue(); 1105 } 1106 1107 /** 1108 * @param value Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}. 1109 */ 1110 public AdditionalAttributeComponent setUri(String value) { 1111 if (Utilities.noString(value)) 1112 this.uri = null; 1113 else { 1114 if (this.uri == null) 1115 this.uri = new UriType(); 1116 this.uri.setValue(value); 1117 } 1118 return this; 1119 } 1120 1121 /** 1122 * @return {@link #description} (A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1123 */ 1124 public StringType getDescriptionElement() { 1125 if (this.description == null) 1126 if (Configuration.errorOnAutoCreate()) 1127 throw new Error("Attempt to auto-create AdditionalAttributeComponent.description"); 1128 else if (Configuration.doAutoCreate()) 1129 this.description = new StringType(); // bb 1130 return this.description; 1131 } 1132 1133 public boolean hasDescriptionElement() { 1134 return this.description != null && !this.description.isEmpty(); 1135 } 1136 1137 public boolean hasDescription() { 1138 return this.description != null && !this.description.isEmpty(); 1139 } 1140 1141 /** 1142 * @param value {@link #description} (A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1143 */ 1144 public AdditionalAttributeComponent setDescriptionElement(StringType value) { 1145 this.description = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element. 1151 */ 1152 public String getDescription() { 1153 return this.description == null ? null : this.description.getValue(); 1154 } 1155 1156 /** 1157 * @param value A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element. 1158 */ 1159 public AdditionalAttributeComponent setDescription(String value) { 1160 if (Utilities.noString(value)) 1161 this.description = null; 1162 else { 1163 if (this.description == null) 1164 this.description = new StringType(); 1165 this.description.setValue(value); 1166 } 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #type} (The type of the source data contained in this concept map for this data element.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1172 */ 1173 public Enumeration<ConceptMapAttributeType> getTypeElement() { 1174 if (this.type == null) 1175 if (Configuration.errorOnAutoCreate()) 1176 throw new Error("Attempt to auto-create AdditionalAttributeComponent.type"); 1177 else if (Configuration.doAutoCreate()) 1178 this.type = new Enumeration<ConceptMapAttributeType>(new ConceptMapAttributeTypeEnumFactory()); // bb 1179 return this.type; 1180 } 1181 1182 public boolean hasTypeElement() { 1183 return this.type != null && !this.type.isEmpty(); 1184 } 1185 1186 public boolean hasType() { 1187 return this.type != null && !this.type.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #type} (The type of the source data contained in this concept map for this data element.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1192 */ 1193 public AdditionalAttributeComponent setTypeElement(Enumeration<ConceptMapAttributeType> value) { 1194 this.type = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return The type of the source data contained in this concept map for this data element. 1200 */ 1201 public ConceptMapAttributeType getType() { 1202 return this.type == null ? null : this.type.getValue(); 1203 } 1204 1205 /** 1206 * @param value The type of the source data contained in this concept map for this data element. 1207 */ 1208 public AdditionalAttributeComponent setType(ConceptMapAttributeType value) { 1209 if (this.type == null) 1210 this.type = new Enumeration<ConceptMapAttributeType>(new ConceptMapAttributeTypeEnumFactory()); 1211 this.type.setValue(value); 1212 return this; 1213 } 1214 1215 protected void listChildren(List<Property> children) { 1216 super.listChildren(children); 1217 children.add(new Property("code", "code", "A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute.", 0, 1, code)); 1218 children.add(new Property("uri", "uri", "Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}.", 0, 1, uri)); 1219 children.add(new Property("description", "string", "A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element.", 0, 1, description)); 1220 children.add(new Property("type", "code", "The type of the source data contained in this concept map for this data element.", 0, 1, type)); 1221 } 1222 1223 @Override 1224 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1225 switch (_hash) { 1226 case 3059181: /*code*/ return new Property("code", "code", "A code that is used to identify this additional data attribute. The code is used internally in ConceptMap.group.element.target.dependsOn.attribute and ConceptMap.group.element.target.product.attribute.", 0, 1, code); 1227 case 116076: /*uri*/ return new Property("uri", "uri", "Reference to the formal definition of the source/target data element. For elements defined by the FHIR specification, or using a FHIR logical model, the correct format is {canonical-url}#{element-id}.", 0, 1, uri); 1228 case -1724546052: /*description*/ return new Property("description", "string", "A description of the additional attribute and/or the data element it refers to - why it is defined, and how the value might be used in mappings, and a discussion of issues associated with the use of the data element.", 0, 1, description); 1229 case 3575610: /*type*/ return new Property("type", "code", "The type of the source data contained in this concept map for this data element.", 0, 1, type); 1230 default: return super.getNamedProperty(_hash, _name, _checkValid); 1231 } 1232 1233 } 1234 1235 @Override 1236 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1237 switch (hash) { 1238 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1239 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 1240 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1241 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConceptMapAttributeType> 1242 default: return super.getProperty(hash, name, checkValid); 1243 } 1244 1245 } 1246 1247 @Override 1248 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1249 switch (hash) { 1250 case 3059181: // code 1251 this.code = TypeConvertor.castToCode(value); // CodeType 1252 return value; 1253 case 116076: // uri 1254 this.uri = TypeConvertor.castToUri(value); // UriType 1255 return value; 1256 case -1724546052: // description 1257 this.description = TypeConvertor.castToString(value); // StringType 1258 return value; 1259 case 3575610: // type 1260 value = new ConceptMapAttributeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1261 this.type = (Enumeration) value; // Enumeration<ConceptMapAttributeType> 1262 return value; 1263 default: return super.setProperty(hash, name, value); 1264 } 1265 1266 } 1267 1268 @Override 1269 public Base setProperty(String name, Base value) throws FHIRException { 1270 if (name.equals("code")) { 1271 this.code = TypeConvertor.castToCode(value); // CodeType 1272 } else if (name.equals("uri")) { 1273 this.uri = TypeConvertor.castToUri(value); // UriType 1274 } else if (name.equals("description")) { 1275 this.description = TypeConvertor.castToString(value); // StringType 1276 } else if (name.equals("type")) { 1277 value = new ConceptMapAttributeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1278 this.type = (Enumeration) value; // Enumeration<ConceptMapAttributeType> 1279 } else 1280 return super.setProperty(name, value); 1281 return value; 1282 } 1283 1284 @Override 1285 public void removeChild(String name, Base value) throws FHIRException { 1286 if (name.equals("code")) { 1287 this.code = null; 1288 } else if (name.equals("uri")) { 1289 this.uri = null; 1290 } else if (name.equals("description")) { 1291 this.description = null; 1292 } else if (name.equals("type")) { 1293 value = new ConceptMapAttributeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1294 this.type = (Enumeration) value; // Enumeration<ConceptMapAttributeType> 1295 } else 1296 super.removeChild(name, value); 1297 1298 } 1299 1300 @Override 1301 public Base makeProperty(int hash, String name) throws FHIRException { 1302 switch (hash) { 1303 case 3059181: return getCodeElement(); 1304 case 116076: return getUriElement(); 1305 case -1724546052: return getDescriptionElement(); 1306 case 3575610: return getTypeElement(); 1307 default: return super.makeProperty(hash, name); 1308 } 1309 1310 } 1311 1312 @Override 1313 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1314 switch (hash) { 1315 case 3059181: /*code*/ return new String[] {"code"}; 1316 case 116076: /*uri*/ return new String[] {"uri"}; 1317 case -1724546052: /*description*/ return new String[] {"string"}; 1318 case 3575610: /*type*/ return new String[] {"code"}; 1319 default: return super.getTypesForProperty(hash, name); 1320 } 1321 1322 } 1323 1324 @Override 1325 public Base addChild(String name) throws FHIRException { 1326 if (name.equals("code")) { 1327 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.additionalAttribute.code"); 1328 } 1329 else if (name.equals("uri")) { 1330 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.additionalAttribute.uri"); 1331 } 1332 else if (name.equals("description")) { 1333 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.additionalAttribute.description"); 1334 } 1335 else if (name.equals("type")) { 1336 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.additionalAttribute.type"); 1337 } 1338 else 1339 return super.addChild(name); 1340 } 1341 1342 public AdditionalAttributeComponent copy() { 1343 AdditionalAttributeComponent dst = new AdditionalAttributeComponent(); 1344 copyValues(dst); 1345 return dst; 1346 } 1347 1348 public void copyValues(AdditionalAttributeComponent dst) { 1349 super.copyValues(dst); 1350 dst.code = code == null ? null : code.copy(); 1351 dst.uri = uri == null ? null : uri.copy(); 1352 dst.description = description == null ? null : description.copy(); 1353 dst.type = type == null ? null : type.copy(); 1354 } 1355 1356 @Override 1357 public boolean equalsDeep(Base other_) { 1358 if (!super.equalsDeep(other_)) 1359 return false; 1360 if (!(other_ instanceof AdditionalAttributeComponent)) 1361 return false; 1362 AdditionalAttributeComponent o = (AdditionalAttributeComponent) other_; 1363 return compareDeep(code, o.code, true) && compareDeep(uri, o.uri, true) && compareDeep(description, o.description, true) 1364 && compareDeep(type, o.type, true); 1365 } 1366 1367 @Override 1368 public boolean equalsShallow(Base other_) { 1369 if (!super.equalsShallow(other_)) 1370 return false; 1371 if (!(other_ instanceof AdditionalAttributeComponent)) 1372 return false; 1373 AdditionalAttributeComponent o = (AdditionalAttributeComponent) other_; 1374 return compareValues(code, o.code, true) && compareValues(uri, o.uri, true) && compareValues(description, o.description, true) 1375 && compareValues(type, o.type, true); 1376 } 1377 1378 public boolean isEmpty() { 1379 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, uri, description, type 1380 ); 1381 } 1382 1383 public String fhirType() { 1384 return "ConceptMap.additionalAttribute"; 1385 1386 } 1387 1388 } 1389 1390 @Block() 1391 public static class ConceptMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 1392 /** 1393 * An absolute URI that identifies the source system where the concepts to be mapped are defined. 1394 */ 1395 @Child(name = "source", type = {CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1396 @Description(shortDefinition="Source system where concepts to be mapped are defined", formalDefinition="An absolute URI that identifies the source system where the concepts to be mapped are defined." ) 1397 protected CanonicalType source; 1398 1399 /** 1400 * An absolute URI that identifies the target system that the concepts will be mapped to. 1401 */ 1402 @Child(name = "target", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1403 @Description(shortDefinition="Target system that the concepts are to be mapped to", formalDefinition="An absolute URI that identifies the target system that the concepts will be mapped to." ) 1404 protected CanonicalType target; 1405 1406 /** 1407 * Mappings for an individual concept in the source to one or more concepts in the target. 1408 */ 1409 @Child(name = "element", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1410 @Description(shortDefinition="Mappings for a concept from the source set", formalDefinition="Mappings for an individual concept in the source to one or more concepts in the target." ) 1411 protected List<SourceElementComponent> element; 1412 1413 /** 1414 * What to do when there is no mapping to a target concept from the source concept and ConceptMap.group.element.noMap is not true. This provides the "default" to be applied when there is no target concept mapping specified or the expansion of ConceptMap.group.element.target.valueSet is empty. 1415 */ 1416 @Child(name = "unmapped", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 1417 @Description(shortDefinition="What to do when there is no mapping target for the source concept and ConceptMap.group.element.noMap is not true", formalDefinition="What to do when there is no mapping to a target concept from the source concept and ConceptMap.group.element.noMap is not true. This provides the \"default\" to be applied when there is no target concept mapping specified or the expansion of ConceptMap.group.element.target.valueSet is empty." ) 1418 protected ConceptMapGroupUnmappedComponent unmapped; 1419 1420 private static final long serialVersionUID = 1307192416L; 1421 1422 /** 1423 * Constructor 1424 */ 1425 public ConceptMapGroupComponent() { 1426 super(); 1427 } 1428 1429 /** 1430 * Constructor 1431 */ 1432 public ConceptMapGroupComponent(SourceElementComponent element) { 1433 super(); 1434 this.addElement(element); 1435 } 1436 1437 /** 1438 * @return {@link #source} (An absolute URI that identifies the source system where the concepts to be mapped are defined.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 1439 */ 1440 public CanonicalType getSourceElement() { 1441 if (this.source == null) 1442 if (Configuration.errorOnAutoCreate()) 1443 throw new Error("Attempt to auto-create ConceptMapGroupComponent.source"); 1444 else if (Configuration.doAutoCreate()) 1445 this.source = new CanonicalType(); // bb 1446 return this.source; 1447 } 1448 1449 public boolean hasSourceElement() { 1450 return this.source != null && !this.source.isEmpty(); 1451 } 1452 1453 public boolean hasSource() { 1454 return this.source != null && !this.source.isEmpty(); 1455 } 1456 1457 /** 1458 * @param value {@link #source} (An absolute URI that identifies the source system where the concepts to be mapped are defined.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 1459 */ 1460 public ConceptMapGroupComponent setSourceElement(CanonicalType value) { 1461 this.source = value; 1462 return this; 1463 } 1464 1465 /** 1466 * @return An absolute URI that identifies the source system where the concepts to be mapped are defined. 1467 */ 1468 public String getSource() { 1469 return this.source == null ? null : this.source.getValue(); 1470 } 1471 1472 /** 1473 * @param value An absolute URI that identifies the source system where the concepts to be mapped are defined. 1474 */ 1475 public ConceptMapGroupComponent setSource(String value) { 1476 if (Utilities.noString(value)) 1477 this.source = null; 1478 else { 1479 if (this.source == null) 1480 this.source = new CanonicalType(); 1481 this.source.setValue(value); 1482 } 1483 return this; 1484 } 1485 1486 /** 1487 * @return {@link #target} (An absolute URI that identifies the target system that the concepts will be mapped to.). This is the underlying object with id, value and extensions. The accessor "getTarget" gives direct access to the value 1488 */ 1489 public CanonicalType getTargetElement() { 1490 if (this.target == null) 1491 if (Configuration.errorOnAutoCreate()) 1492 throw new Error("Attempt to auto-create ConceptMapGroupComponent.target"); 1493 else if (Configuration.doAutoCreate()) 1494 this.target = new CanonicalType(); // bb 1495 return this.target; 1496 } 1497 1498 public boolean hasTargetElement() { 1499 return this.target != null && !this.target.isEmpty(); 1500 } 1501 1502 public boolean hasTarget() { 1503 return this.target != null && !this.target.isEmpty(); 1504 } 1505 1506 /** 1507 * @param value {@link #target} (An absolute URI that identifies the target system that the concepts will be mapped to.). This is the underlying object with id, value and extensions. The accessor "getTarget" gives direct access to the value 1508 */ 1509 public ConceptMapGroupComponent setTargetElement(CanonicalType value) { 1510 this.target = value; 1511 return this; 1512 } 1513 1514 /** 1515 * @return An absolute URI that identifies the target system that the concepts will be mapped to. 1516 */ 1517 public String getTarget() { 1518 return this.target == null ? null : this.target.getValue(); 1519 } 1520 1521 /** 1522 * @param value An absolute URI that identifies the target system that the concepts will be mapped to. 1523 */ 1524 public ConceptMapGroupComponent setTarget(String value) { 1525 if (Utilities.noString(value)) 1526 this.target = null; 1527 else { 1528 if (this.target == null) 1529 this.target = new CanonicalType(); 1530 this.target.setValue(value); 1531 } 1532 return this; 1533 } 1534 1535 /** 1536 * @return {@link #element} (Mappings for an individual concept in the source to one or more concepts in the target.) 1537 */ 1538 public List<SourceElementComponent> getElement() { 1539 if (this.element == null) 1540 this.element = new ArrayList<SourceElementComponent>(); 1541 return this.element; 1542 } 1543 1544 /** 1545 * @return Returns a reference to <code>this</code> for easy method chaining 1546 */ 1547 public ConceptMapGroupComponent setElement(List<SourceElementComponent> theElement) { 1548 this.element = theElement; 1549 return this; 1550 } 1551 1552 public boolean hasElement() { 1553 if (this.element == null) 1554 return false; 1555 for (SourceElementComponent item : this.element) 1556 if (!item.isEmpty()) 1557 return true; 1558 return false; 1559 } 1560 1561 public SourceElementComponent addElement() { //3 1562 SourceElementComponent t = new SourceElementComponent(); 1563 if (this.element == null) 1564 this.element = new ArrayList<SourceElementComponent>(); 1565 this.element.add(t); 1566 return t; 1567 } 1568 1569 public ConceptMapGroupComponent addElement(SourceElementComponent t) { //3 1570 if (t == null) 1571 return this; 1572 if (this.element == null) 1573 this.element = new ArrayList<SourceElementComponent>(); 1574 this.element.add(t); 1575 return this; 1576 } 1577 1578 /** 1579 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist {3} 1580 */ 1581 public SourceElementComponent getElementFirstRep() { 1582 if (getElement().isEmpty()) { 1583 addElement(); 1584 } 1585 return getElement().get(0); 1586 } 1587 1588 /** 1589 * @return {@link #unmapped} (What to do when there is no mapping to a target concept from the source concept and ConceptMap.group.element.noMap is not true. This provides the "default" to be applied when there is no target concept mapping specified or the expansion of ConceptMap.group.element.target.valueSet is empty.) 1590 */ 1591 public ConceptMapGroupUnmappedComponent getUnmapped() { 1592 if (this.unmapped == null) 1593 if (Configuration.errorOnAutoCreate()) 1594 throw new Error("Attempt to auto-create ConceptMapGroupComponent.unmapped"); 1595 else if (Configuration.doAutoCreate()) 1596 this.unmapped = new ConceptMapGroupUnmappedComponent(); // cc 1597 return this.unmapped; 1598 } 1599 1600 public boolean hasUnmapped() { 1601 return this.unmapped != null && !this.unmapped.isEmpty(); 1602 } 1603 1604 /** 1605 * @param value {@link #unmapped} (What to do when there is no mapping to a target concept from the source concept and ConceptMap.group.element.noMap is not true. This provides the "default" to be applied when there is no target concept mapping specified or the expansion of ConceptMap.group.element.target.valueSet is empty.) 1606 */ 1607 public ConceptMapGroupComponent setUnmapped(ConceptMapGroupUnmappedComponent value) { 1608 this.unmapped = value; 1609 return this; 1610 } 1611 1612 protected void listChildren(List<Property> children) { 1613 super.listChildren(children); 1614 children.add(new Property("source", "canonical(CodeSystem)", "An absolute URI that identifies the source system where the concepts to be mapped are defined.", 0, 1, source)); 1615 children.add(new Property("target", "canonical(CodeSystem)", "An absolute URI that identifies the target system that the concepts will be mapped to.", 0, 1, target)); 1616 children.add(new Property("element", "", "Mappings for an individual concept in the source to one or more concepts in the target.", 0, java.lang.Integer.MAX_VALUE, element)); 1617 children.add(new Property("unmapped", "", "What to do when there is no mapping to a target concept from the source concept and ConceptMap.group.element.noMap is not true. This provides the \"default\" to be applied when there is no target concept mapping specified or the expansion of ConceptMap.group.element.target.valueSet is empty.", 0, 1, unmapped)); 1618 } 1619 1620 @Override 1621 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1622 switch (_hash) { 1623 case -896505829: /*source*/ return new Property("source", "canonical(CodeSystem)", "An absolute URI that identifies the source system where the concepts to be mapped are defined.", 0, 1, source); 1624 case -880905839: /*target*/ return new Property("target", "canonical(CodeSystem)", "An absolute URI that identifies the target system that the concepts will be mapped to.", 0, 1, target); 1625 case -1662836996: /*element*/ return new Property("element", "", "Mappings for an individual concept in the source to one or more concepts in the target.", 0, java.lang.Integer.MAX_VALUE, element); 1626 case -194857460: /*unmapped*/ return new Property("unmapped", "", "What to do when there is no mapping to a target concept from the source concept and ConceptMap.group.element.noMap is not true. This provides the \"default\" to be applied when there is no target concept mapping specified or the expansion of ConceptMap.group.element.target.valueSet is empty.", 0, 1, unmapped); 1627 default: return super.getNamedProperty(_hash, _name, _checkValid); 1628 } 1629 1630 } 1631 1632 @Override 1633 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1634 switch (hash) { 1635 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // CanonicalType 1636 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // CanonicalType 1637 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // SourceElementComponent 1638 case -194857460: /*unmapped*/ return this.unmapped == null ? new Base[0] : new Base[] {this.unmapped}; // ConceptMapGroupUnmappedComponent 1639 default: return super.getProperty(hash, name, checkValid); 1640 } 1641 1642 } 1643 1644 @Override 1645 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1646 switch (hash) { 1647 case -896505829: // source 1648 this.source = TypeConvertor.castToCanonical(value); // CanonicalType 1649 return value; 1650 case -880905839: // target 1651 this.target = TypeConvertor.castToCanonical(value); // CanonicalType 1652 return value; 1653 case -1662836996: // element 1654 this.getElement().add((SourceElementComponent) value); // SourceElementComponent 1655 return value; 1656 case -194857460: // unmapped 1657 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 1658 return value; 1659 default: return super.setProperty(hash, name, value); 1660 } 1661 1662 } 1663 1664 @Override 1665 public Base setProperty(String name, Base value) throws FHIRException { 1666 if (name.equals("source")) { 1667 this.source = TypeConvertor.castToCanonical(value); // CanonicalType 1668 } else if (name.equals("target")) { 1669 this.target = TypeConvertor.castToCanonical(value); // CanonicalType 1670 } else if (name.equals("element")) { 1671 this.getElement().add((SourceElementComponent) value); 1672 } else if (name.equals("unmapped")) { 1673 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 1674 } else 1675 return super.setProperty(name, value); 1676 return value; 1677 } 1678 1679 @Override 1680 public void removeChild(String name, Base value) throws FHIRException { 1681 if (name.equals("source")) { 1682 this.source = null; 1683 } else if (name.equals("target")) { 1684 this.target = null; 1685 } else if (name.equals("element")) { 1686 this.getElement().remove((SourceElementComponent) value); 1687 } else if (name.equals("unmapped")) { 1688 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 1689 } else 1690 super.removeChild(name, value); 1691 1692 } 1693 1694 @Override 1695 public Base makeProperty(int hash, String name) throws FHIRException { 1696 switch (hash) { 1697 case -896505829: return getSourceElement(); 1698 case -880905839: return getTargetElement(); 1699 case -1662836996: return addElement(); 1700 case -194857460: return getUnmapped(); 1701 default: return super.makeProperty(hash, name); 1702 } 1703 1704 } 1705 1706 @Override 1707 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1708 switch (hash) { 1709 case -896505829: /*source*/ return new String[] {"canonical"}; 1710 case -880905839: /*target*/ return new String[] {"canonical"}; 1711 case -1662836996: /*element*/ return new String[] {}; 1712 case -194857460: /*unmapped*/ return new String[] {}; 1713 default: return super.getTypesForProperty(hash, name); 1714 } 1715 1716 } 1717 1718 @Override 1719 public Base addChild(String name) throws FHIRException { 1720 if (name.equals("source")) { 1721 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.source"); 1722 } 1723 else if (name.equals("target")) { 1724 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.target"); 1725 } 1726 else if (name.equals("element")) { 1727 return addElement(); 1728 } 1729 else if (name.equals("unmapped")) { 1730 this.unmapped = new ConceptMapGroupUnmappedComponent(); 1731 return this.unmapped; 1732 } 1733 else 1734 return super.addChild(name); 1735 } 1736 1737 public ConceptMapGroupComponent copy() { 1738 ConceptMapGroupComponent dst = new ConceptMapGroupComponent(); 1739 copyValues(dst); 1740 return dst; 1741 } 1742 1743 public void copyValues(ConceptMapGroupComponent dst) { 1744 super.copyValues(dst); 1745 dst.source = source == null ? null : source.copy(); 1746 dst.target = target == null ? null : target.copy(); 1747 if (element != null) { 1748 dst.element = new ArrayList<SourceElementComponent>(); 1749 for (SourceElementComponent i : element) 1750 dst.element.add(i.copy()); 1751 }; 1752 dst.unmapped = unmapped == null ? null : unmapped.copy(); 1753 } 1754 1755 @Override 1756 public boolean equalsDeep(Base other_) { 1757 if (!super.equalsDeep(other_)) 1758 return false; 1759 if (!(other_ instanceof ConceptMapGroupComponent)) 1760 return false; 1761 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 1762 return compareDeep(source, o.source, true) && compareDeep(target, o.target, true) && compareDeep(element, o.element, true) 1763 && compareDeep(unmapped, o.unmapped, true); 1764 } 1765 1766 @Override 1767 public boolean equalsShallow(Base other_) { 1768 if (!super.equalsShallow(other_)) 1769 return false; 1770 if (!(other_ instanceof ConceptMapGroupComponent)) 1771 return false; 1772 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 1773 return compareValues(source, o.source, true) && compareValues(target, o.target, true); 1774 } 1775 1776 public boolean isEmpty() { 1777 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, target, element 1778 , unmapped); 1779 } 1780 1781 public String fhirType() { 1782 return "ConceptMap.group"; 1783 1784 } 1785 1786 public SourceElementComponent getOrAddElement(String code) { 1787 for (SourceElementComponent e : getElement()) { 1788 if (code.equals(e.getCode())) { 1789 return e; 1790 } 1791 } 1792 return addElement().setCode(code); 1793 } 1794 1795 } 1796 1797 @Block() 1798 public static class SourceElementComponent extends BackboneElement implements IBaseBackboneElement { 1799 @Override 1800 public String toString() { 1801 return "SourceElementComponent [code=" + code + ", display=" + display + ", noMap=" + noMap + "]"; 1802 } 1803 1804 /** 1805 * Identity (code or path) or the element/item being mapped. 1806 */ 1807 @Child(name = "code", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1808 @Description(shortDefinition="Identifies element being mapped", formalDefinition="Identity (code or path) or the element/item being mapped." ) 1809 protected CodeType code; 1810 1811 /** 1812 * The display for the code. The display is only provided to help editors when editing the concept map. 1813 */ 1814 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1815 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 1816 protected StringType display; 1817 1818 /** 1819 * The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule. 1820 */ 1821 @Child(name = "valueSet", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1822 @Description(shortDefinition="Identifies the set of concepts being mapped", formalDefinition="The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule." ) 1823 protected CanonicalType valueSet; 1824 1825 /** 1826 * If noMap = true this indicates that no mapping to a target concept exists for this source concept. 1827 */ 1828 @Child(name = "noMap", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1829 @Description(shortDefinition="No mapping to a target concept for this source concept", formalDefinition="If noMap = true this indicates that no mapping to a target concept exists for this source concept." ) 1830 protected BooleanType noMap; 1831 1832 /** 1833 * A concept from the target value set that this concept maps to. 1834 */ 1835 @Child(name = "target", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1836 @Description(shortDefinition="Concept in target system for element", formalDefinition="A concept from the target value set that this concept maps to." ) 1837 protected List<TargetElementComponent> target; 1838 1839 private static final long serialVersionUID = 1485743554L; 1840 1841 /** 1842 * Constructor 1843 */ 1844 public SourceElementComponent() { 1845 super(); 1846 } 1847 1848 /** 1849 * @return {@link #code} (Identity (code or path) or the element/item being mapped.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1850 */ 1851 public CodeType getCodeElement() { 1852 if (this.code == null) 1853 if (Configuration.errorOnAutoCreate()) 1854 throw new Error("Attempt to auto-create SourceElementComponent.code"); 1855 else if (Configuration.doAutoCreate()) 1856 this.code = new CodeType(); // bb 1857 return this.code; 1858 } 1859 1860 public boolean hasCodeElement() { 1861 return this.code != null && !this.code.isEmpty(); 1862 } 1863 1864 public boolean hasCode() { 1865 return this.code != null && !this.code.isEmpty(); 1866 } 1867 1868 /** 1869 * @param value {@link #code} (Identity (code or path) or the element/item being mapped.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1870 */ 1871 public SourceElementComponent setCodeElement(CodeType value) { 1872 this.code = value; 1873 return this; 1874 } 1875 1876 /** 1877 * @return Identity (code or path) or the element/item being mapped. 1878 */ 1879 public String getCode() { 1880 return this.code == null ? null : this.code.getValue(); 1881 } 1882 1883 /** 1884 * @param value Identity (code or path) or the element/item being mapped. 1885 */ 1886 public SourceElementComponent setCode(String value) { 1887 if (Utilities.noString(value)) 1888 this.code = null; 1889 else { 1890 if (this.code == null) 1891 this.code = new CodeType(); 1892 this.code.setValue(value); 1893 } 1894 return this; 1895 } 1896 1897 /** 1898 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1899 */ 1900 public StringType getDisplayElement() { 1901 if (this.display == null) 1902 if (Configuration.errorOnAutoCreate()) 1903 throw new Error("Attempt to auto-create SourceElementComponent.display"); 1904 else if (Configuration.doAutoCreate()) 1905 this.display = new StringType(); // bb 1906 return this.display; 1907 } 1908 1909 public boolean hasDisplayElement() { 1910 return this.display != null && !this.display.isEmpty(); 1911 } 1912 1913 public boolean hasDisplay() { 1914 return this.display != null && !this.display.isEmpty(); 1915 } 1916 1917 /** 1918 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1919 */ 1920 public SourceElementComponent setDisplayElement(StringType value) { 1921 this.display = value; 1922 return this; 1923 } 1924 1925 /** 1926 * @return The display for the code. The display is only provided to help editors when editing the concept map. 1927 */ 1928 public String getDisplay() { 1929 return this.display == null ? null : this.display.getValue(); 1930 } 1931 1932 /** 1933 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 1934 */ 1935 public SourceElementComponent setDisplay(String value) { 1936 if (Utilities.noString(value)) 1937 this.display = null; 1938 else { 1939 if (this.display == null) 1940 this.display = new StringType(); 1941 this.display.setValue(value); 1942 } 1943 return this; 1944 } 1945 1946 /** 1947 * @return {@link #valueSet} (The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 1948 */ 1949 public CanonicalType getValueSetElement() { 1950 if (this.valueSet == null) 1951 if (Configuration.errorOnAutoCreate()) 1952 throw new Error("Attempt to auto-create SourceElementComponent.valueSet"); 1953 else if (Configuration.doAutoCreate()) 1954 this.valueSet = new CanonicalType(); // bb 1955 return this.valueSet; 1956 } 1957 1958 public boolean hasValueSetElement() { 1959 return this.valueSet != null && !this.valueSet.isEmpty(); 1960 } 1961 1962 public boolean hasValueSet() { 1963 return this.valueSet != null && !this.valueSet.isEmpty(); 1964 } 1965 1966 /** 1967 * @param value {@link #valueSet} (The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 1968 */ 1969 public SourceElementComponent setValueSetElement(CanonicalType value) { 1970 this.valueSet = value; 1971 return this; 1972 } 1973 1974 /** 1975 * @return The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule. 1976 */ 1977 public String getValueSet() { 1978 return this.valueSet == null ? null : this.valueSet.getValue(); 1979 } 1980 1981 /** 1982 * @param value The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule. 1983 */ 1984 public SourceElementComponent setValueSet(String value) { 1985 if (Utilities.noString(value)) 1986 this.valueSet = null; 1987 else { 1988 if (this.valueSet == null) 1989 this.valueSet = new CanonicalType(); 1990 this.valueSet.setValue(value); 1991 } 1992 return this; 1993 } 1994 1995 /** 1996 * @return {@link #noMap} (If noMap = true this indicates that no mapping to a target concept exists for this source concept.). This is the underlying object with id, value and extensions. The accessor "getNoMap" gives direct access to the value 1997 */ 1998 public BooleanType getNoMapElement() { 1999 if (this.noMap == null) 2000 if (Configuration.errorOnAutoCreate()) 2001 throw new Error("Attempt to auto-create SourceElementComponent.noMap"); 2002 else if (Configuration.doAutoCreate()) 2003 this.noMap = new BooleanType(); // bb 2004 return this.noMap; 2005 } 2006 2007 public boolean hasNoMapElement() { 2008 return this.noMap != null && !this.noMap.isEmpty(); 2009 } 2010 2011 public boolean hasNoMap() { 2012 return this.noMap != null && !this.noMap.isEmpty(); 2013 } 2014 2015 /** 2016 * @param value {@link #noMap} (If noMap = true this indicates that no mapping to a target concept exists for this source concept.). This is the underlying object with id, value and extensions. The accessor "getNoMap" gives direct access to the value 2017 */ 2018 public SourceElementComponent setNoMapElement(BooleanType value) { 2019 this.noMap = value; 2020 return this; 2021 } 2022 2023 /** 2024 * @return If noMap = true this indicates that no mapping to a target concept exists for this source concept. 2025 */ 2026 public boolean getNoMap() { 2027 return this.noMap == null || this.noMap.isEmpty() ? false : this.noMap.getValue(); 2028 } 2029 2030 /** 2031 * @param value If noMap = true this indicates that no mapping to a target concept exists for this source concept. 2032 */ 2033 public SourceElementComponent setNoMap(boolean value) { 2034 if (this.noMap == null) 2035 this.noMap = new BooleanType(); 2036 this.noMap.setValue(value); 2037 return this; 2038 } 2039 2040 /** 2041 * @return {@link #target} (A concept from the target value set that this concept maps to.) 2042 */ 2043 public List<TargetElementComponent> getTarget() { 2044 if (this.target == null) 2045 this.target = new ArrayList<TargetElementComponent>(); 2046 return this.target; 2047 } 2048 2049 /** 2050 * @return Returns a reference to <code>this</code> for easy method chaining 2051 */ 2052 public SourceElementComponent setTarget(List<TargetElementComponent> theTarget) { 2053 this.target = theTarget; 2054 return this; 2055 } 2056 2057 public boolean hasTarget() { 2058 if (this.target == null) 2059 return false; 2060 for (TargetElementComponent item : this.target) 2061 if (!item.isEmpty()) 2062 return true; 2063 return false; 2064 } 2065 2066 public TargetElementComponent addTarget() { //3 2067 TargetElementComponent t = new TargetElementComponent(); 2068 if (this.target == null) 2069 this.target = new ArrayList<TargetElementComponent>(); 2070 this.target.add(t); 2071 return t; 2072 } 2073 2074 public SourceElementComponent addTarget(TargetElementComponent t) { //3 2075 if (t == null) 2076 return this; 2077 if (this.target == null) 2078 this.target = new ArrayList<TargetElementComponent>(); 2079 this.target.add(t); 2080 return this; 2081 } 2082 2083 /** 2084 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 2085 */ 2086 public TargetElementComponent getTargetFirstRep() { 2087 if (getTarget().isEmpty()) { 2088 addTarget(); 2089 } 2090 return getTarget().get(0); 2091 } 2092 2093 protected void listChildren(List<Property> children) { 2094 super.listChildren(children); 2095 children.add(new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1, code)); 2096 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 2097 children.add(new Property("valueSet", "canonical(ValueSet)", "The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule.", 0, 1, valueSet)); 2098 children.add(new Property("noMap", "boolean", "If noMap = true this indicates that no mapping to a target concept exists for this source concept.", 0, 1, noMap)); 2099 children.add(new Property("target", "", "A concept from the target value set that this concept maps to.", 0, java.lang.Integer.MAX_VALUE, target)); 2100 } 2101 2102 @Override 2103 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2104 switch (_hash) { 2105 case 3059181: /*code*/ return new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1, code); 2106 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 2107 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "The set of concepts from the ConceptMap.group.source code system which are all being mapped to the target as part of this mapping rule.", 0, 1, valueSet); 2108 case 104971227: /*noMap*/ return new Property("noMap", "boolean", "If noMap = true this indicates that no mapping to a target concept exists for this source concept.", 0, 1, noMap); 2109 case -880905839: /*target*/ return new Property("target", "", "A concept from the target value set that this concept maps to.", 0, java.lang.Integer.MAX_VALUE, target); 2110 default: return super.getNamedProperty(_hash, _name, _checkValid); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2117 switch (hash) { 2118 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2119 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 2120 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 2121 case 104971227: /*noMap*/ return this.noMap == null ? new Base[0] : new Base[] {this.noMap}; // BooleanType 2122 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // TargetElementComponent 2123 default: return super.getProperty(hash, name, checkValid); 2124 } 2125 2126 } 2127 2128 @Override 2129 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2130 switch (hash) { 2131 case 3059181: // code 2132 this.code = TypeConvertor.castToCode(value); // CodeType 2133 return value; 2134 case 1671764162: // display 2135 this.display = TypeConvertor.castToString(value); // StringType 2136 return value; 2137 case -1410174671: // valueSet 2138 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 2139 return value; 2140 case 104971227: // noMap 2141 this.noMap = TypeConvertor.castToBoolean(value); // BooleanType 2142 return value; 2143 case -880905839: // target 2144 this.getTarget().add((TargetElementComponent) value); // TargetElementComponent 2145 return value; 2146 default: return super.setProperty(hash, name, value); 2147 } 2148 2149 } 2150 2151 @Override 2152 public Base setProperty(String name, Base value) throws FHIRException { 2153 if (name.equals("code")) { 2154 this.code = TypeConvertor.castToCode(value); // CodeType 2155 } else if (name.equals("display")) { 2156 this.display = TypeConvertor.castToString(value); // StringType 2157 } else if (name.equals("valueSet")) { 2158 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 2159 } else if (name.equals("noMap")) { 2160 this.noMap = TypeConvertor.castToBoolean(value); // BooleanType 2161 } else if (name.equals("target")) { 2162 this.getTarget().add((TargetElementComponent) value); 2163 } else 2164 return super.setProperty(name, value); 2165 return value; 2166 } 2167 2168 @Override 2169 public void removeChild(String name, Base value) throws FHIRException { 2170 if (name.equals("code")) { 2171 this.code = null; 2172 } else if (name.equals("display")) { 2173 this.display = null; 2174 } else if (name.equals("valueSet")) { 2175 this.valueSet = null; 2176 } else if (name.equals("noMap")) { 2177 this.noMap = null; 2178 } else if (name.equals("target")) { 2179 this.getTarget().remove((TargetElementComponent) value); 2180 } else 2181 super.removeChild(name, value); 2182 2183 } 2184 2185 @Override 2186 public Base makeProperty(int hash, String name) throws FHIRException { 2187 switch (hash) { 2188 case 3059181: return getCodeElement(); 2189 case 1671764162: return getDisplayElement(); 2190 case -1410174671: return getValueSetElement(); 2191 case 104971227: return getNoMapElement(); 2192 case -880905839: return addTarget(); 2193 default: return super.makeProperty(hash, name); 2194 } 2195 2196 } 2197 2198 @Override 2199 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2200 switch (hash) { 2201 case 3059181: /*code*/ return new String[] {"code"}; 2202 case 1671764162: /*display*/ return new String[] {"string"}; 2203 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 2204 case 104971227: /*noMap*/ return new String[] {"boolean"}; 2205 case -880905839: /*target*/ return new String[] {}; 2206 default: return super.getTypesForProperty(hash, name); 2207 } 2208 2209 } 2210 2211 @Override 2212 public Base addChild(String name) throws FHIRException { 2213 if (name.equals("code")) { 2214 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.code"); 2215 } 2216 else if (name.equals("display")) { 2217 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.display"); 2218 } 2219 else if (name.equals("valueSet")) { 2220 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.valueSet"); 2221 } 2222 else if (name.equals("noMap")) { 2223 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.noMap"); 2224 } 2225 else if (name.equals("target")) { 2226 return addTarget(); 2227 } 2228 else 2229 return super.addChild(name); 2230 } 2231 2232 public SourceElementComponent copy() { 2233 SourceElementComponent dst = new SourceElementComponent(); 2234 copyValues(dst); 2235 return dst; 2236 } 2237 2238 public void copyValues(SourceElementComponent dst) { 2239 super.copyValues(dst); 2240 dst.code = code == null ? null : code.copy(); 2241 dst.display = display == null ? null : display.copy(); 2242 dst.valueSet = valueSet == null ? null : valueSet.copy(); 2243 dst.noMap = noMap == null ? null : noMap.copy(); 2244 if (target != null) { 2245 dst.target = new ArrayList<TargetElementComponent>(); 2246 for (TargetElementComponent i : target) 2247 dst.target.add(i.copy()); 2248 }; 2249 } 2250 2251 @Override 2252 public boolean equalsDeep(Base other_) { 2253 if (!super.equalsDeep(other_)) 2254 return false; 2255 if (!(other_ instanceof SourceElementComponent)) 2256 return false; 2257 SourceElementComponent o = (SourceElementComponent) other_; 2258 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(valueSet, o.valueSet, true) 2259 && compareDeep(noMap, o.noMap, true) && compareDeep(target, o.target, true); 2260 } 2261 2262 @Override 2263 public boolean equalsShallow(Base other_) { 2264 if (!super.equalsShallow(other_)) 2265 return false; 2266 if (!(other_ instanceof SourceElementComponent)) 2267 return false; 2268 SourceElementComponent o = (SourceElementComponent) other_; 2269 return compareValues(code, o.code, true) && compareValues(display, o.display, true) && compareValues(valueSet, o.valueSet, true) 2270 && compareValues(noMap, o.noMap, true); 2271 } 2272 2273 public boolean isEmpty() { 2274 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, valueSet 2275 , noMap, target); 2276 } 2277 2278 public String fhirType() { 2279 return "ConceptMap.group.element"; 2280 2281 } 2282 2283 public boolean hasTargetCode(String code) { 2284 for (TargetElementComponent tgt : getTarget()) { 2285 if (code.equals(tgt.getCode())) { 2286 return true; 2287 } 2288 } 2289 return false; 2290 } 2291 2292 public TargetElementComponent addTarget(String code, ConceptMapRelationship relationship) { 2293 TargetElementComponent tgt = addTarget(); 2294 tgt.setCode(code); 2295 tgt.setRelationship(relationship); 2296 return tgt; 2297 } 2298 } 2299 2300 @Block() 2301 public static class TargetElementComponent extends BackboneElement implements IBaseBackboneElement { 2302 @Override 2303 public String toString() { 2304 return "TargetElementComponent [code=" + code + ", relationship=" + relationship + "]"; 2305 } 2306 2307 /** 2308 * Identity (code or path) or the element/item that the map refers to. 2309 */ 2310 @Child(name = "code", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2311 @Description(shortDefinition="Code that identifies the target element", formalDefinition="Identity (code or path) or the element/item that the map refers to." ) 2312 protected CodeType code; 2313 2314 /** 2315 * The display for the code. The display is only provided to help editors when editing the concept map. 2316 */ 2317 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2318 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 2319 protected StringType display; 2320 2321 /** 2322 * The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set. 2323 */ 2324 @Child(name = "valueSet", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2325 @Description(shortDefinition="Identifies the set of target concepts", formalDefinition="The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set." ) 2326 protected CanonicalType valueSet; 2327 2328 /** 2329 * The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target). 2330 */ 2331 @Child(name = "relationship", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=false) 2332 @Description(shortDefinition="related-to | equivalent | source-is-narrower-than-target | source-is-broader-than-target | not-related-to", formalDefinition="The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target)." ) 2333 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concept-map-relationship") 2334 protected Enumeration<ConceptMapRelationship> relationship; 2335 2336 /** 2337 * A description of status/issues in mapping that conveys additional information not represented in the structured data. 2338 */ 2339 @Child(name = "comment", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2340 @Description(shortDefinition="Description of status/issues in mapping", formalDefinition="A description of status/issues in mapping that conveys additional information not represented in the structured data." ) 2341 protected StringType comment; 2342 2343 /** 2344 * A property value for this source -> target mapping. 2345 */ 2346 @Child(name = "property", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2347 @Description(shortDefinition="Property value for the source -> target mapping", formalDefinition="A property value for this source -> target mapping." ) 2348 protected List<MappingPropertyComponent> property; 2349 2350 /** 2351 * A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified data attribute can be resolved, and it has the specified value. 2352 */ 2353 @Child(name = "dependsOn", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2354 @Description(shortDefinition="Other properties required for this mapping", formalDefinition="A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified data attribute can be resolved, and it has the specified value." ) 2355 protected List<OtherElementComponent> dependsOn; 2356 2357 /** 2358 * Product is the output of a ConceptMap that provides additional values that go in other attributes / data elemnts of the target data. 2359 */ 2360 @Child(name = "product", type = {OtherElementComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2361 @Description(shortDefinition="Other data elements that this mapping also produces", formalDefinition="Product is the output of a ConceptMap that provides additional values that go in other attributes / data elemnts of the target data." ) 2362 protected List<OtherElementComponent> product; 2363 2364 private static final long serialVersionUID = 792559555L; 2365 2366 /** 2367 * Constructor 2368 */ 2369 public TargetElementComponent() { 2370 super(); 2371 } 2372 2373 /** 2374 * Constructor 2375 */ 2376 public TargetElementComponent(ConceptMapRelationship relationship) { 2377 super(); 2378 this.setRelationship(relationship); 2379 } 2380 2381 /** 2382 * @return {@link #code} (Identity (code or path) or the element/item that the map refers to.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2383 */ 2384 public CodeType getCodeElement() { 2385 if (this.code == null) 2386 if (Configuration.errorOnAutoCreate()) 2387 throw new Error("Attempt to auto-create TargetElementComponent.code"); 2388 else if (Configuration.doAutoCreate()) 2389 this.code = new CodeType(); // bb 2390 return this.code; 2391 } 2392 2393 public boolean hasCodeElement() { 2394 return this.code != null && !this.code.isEmpty(); 2395 } 2396 2397 public boolean hasCode() { 2398 return this.code != null && !this.code.isEmpty(); 2399 } 2400 2401 /** 2402 * @param value {@link #code} (Identity (code or path) or the element/item that the map refers to.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2403 */ 2404 public TargetElementComponent setCodeElement(CodeType value) { 2405 this.code = value; 2406 return this; 2407 } 2408 2409 /** 2410 * @return Identity (code or path) or the element/item that the map refers to. 2411 */ 2412 public String getCode() { 2413 return this.code == null ? null : this.code.getValue(); 2414 } 2415 2416 /** 2417 * @param value Identity (code or path) or the element/item that the map refers to. 2418 */ 2419 public TargetElementComponent setCode(String value) { 2420 if (Utilities.noString(value)) 2421 this.code = null; 2422 else { 2423 if (this.code == null) 2424 this.code = new CodeType(); 2425 this.code.setValue(value); 2426 } 2427 return this; 2428 } 2429 2430 /** 2431 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 2432 */ 2433 public StringType getDisplayElement() { 2434 if (this.display == null) 2435 if (Configuration.errorOnAutoCreate()) 2436 throw new Error("Attempt to auto-create TargetElementComponent.display"); 2437 else if (Configuration.doAutoCreate()) 2438 this.display = new StringType(); // bb 2439 return this.display; 2440 } 2441 2442 public boolean hasDisplayElement() { 2443 return this.display != null && !this.display.isEmpty(); 2444 } 2445 2446 public boolean hasDisplay() { 2447 return this.display != null && !this.display.isEmpty(); 2448 } 2449 2450 /** 2451 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 2452 */ 2453 public TargetElementComponent setDisplayElement(StringType value) { 2454 this.display = value; 2455 return this; 2456 } 2457 2458 /** 2459 * @return The display for the code. The display is only provided to help editors when editing the concept map. 2460 */ 2461 public String getDisplay() { 2462 return this.display == null ? null : this.display.getValue(); 2463 } 2464 2465 /** 2466 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 2467 */ 2468 public TargetElementComponent setDisplay(String value) { 2469 if (Utilities.noString(value)) 2470 this.display = null; 2471 else { 2472 if (this.display == null) 2473 this.display = new StringType(); 2474 this.display.setValue(value); 2475 } 2476 return this; 2477 } 2478 2479 /** 2480 * @return {@link #valueSet} (The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 2481 */ 2482 public CanonicalType getValueSetElement() { 2483 if (this.valueSet == null) 2484 if (Configuration.errorOnAutoCreate()) 2485 throw new Error("Attempt to auto-create TargetElementComponent.valueSet"); 2486 else if (Configuration.doAutoCreate()) 2487 this.valueSet = new CanonicalType(); // bb 2488 return this.valueSet; 2489 } 2490 2491 public boolean hasValueSetElement() { 2492 return this.valueSet != null && !this.valueSet.isEmpty(); 2493 } 2494 2495 public boolean hasValueSet() { 2496 return this.valueSet != null && !this.valueSet.isEmpty(); 2497 } 2498 2499 /** 2500 * @param value {@link #valueSet} (The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 2501 */ 2502 public TargetElementComponent setValueSetElement(CanonicalType value) { 2503 this.valueSet = value; 2504 return this; 2505 } 2506 2507 /** 2508 * @return The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set. 2509 */ 2510 public String getValueSet() { 2511 return this.valueSet == null ? null : this.valueSet.getValue(); 2512 } 2513 2514 /** 2515 * @param value The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set. 2516 */ 2517 public TargetElementComponent setValueSet(String value) { 2518 if (Utilities.noString(value)) 2519 this.valueSet = null; 2520 else { 2521 if (this.valueSet == null) 2522 this.valueSet = new CanonicalType(); 2523 this.valueSet.setValue(value); 2524 } 2525 return this; 2526 } 2527 2528 /** 2529 * @return {@link #relationship} (The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target).). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 2530 */ 2531 public Enumeration<ConceptMapRelationship> getRelationshipElement() { 2532 if (this.relationship == null) 2533 if (Configuration.errorOnAutoCreate()) 2534 throw new Error("Attempt to auto-create TargetElementComponent.relationship"); 2535 else if (Configuration.doAutoCreate()) 2536 this.relationship = new Enumeration<ConceptMapRelationship>(new ConceptMapRelationshipEnumFactory()); // bb 2537 return this.relationship; 2538 } 2539 2540 public boolean hasRelationshipElement() { 2541 return this.relationship != null && !this.relationship.isEmpty(); 2542 } 2543 2544 public boolean hasRelationship() { 2545 return this.relationship != null && !this.relationship.isEmpty(); 2546 } 2547 2548 /** 2549 * @param value {@link #relationship} (The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target).). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 2550 */ 2551 public TargetElementComponent setRelationshipElement(Enumeration<ConceptMapRelationship> value) { 2552 this.relationship = value; 2553 return this; 2554 } 2555 2556 /** 2557 * @return The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target). 2558 */ 2559 public ConceptMapRelationship getRelationship() { 2560 return this.relationship == null ? null : this.relationship.getValue(); 2561 } 2562 2563 /** 2564 * @param value The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target). 2565 */ 2566 public TargetElementComponent setRelationship(ConceptMapRelationship value) { 2567 if (this.relationship == null) 2568 this.relationship = new Enumeration<ConceptMapRelationship>(new ConceptMapRelationshipEnumFactory()); 2569 this.relationship.setValue(value); 2570 return this; 2571 } 2572 2573 /** 2574 * @return {@link #comment} (A description of status/issues in mapping that conveys additional information not represented in the structured data.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2575 */ 2576 public StringType getCommentElement() { 2577 if (this.comment == null) 2578 if (Configuration.errorOnAutoCreate()) 2579 throw new Error("Attempt to auto-create TargetElementComponent.comment"); 2580 else if (Configuration.doAutoCreate()) 2581 this.comment = new StringType(); // bb 2582 return this.comment; 2583 } 2584 2585 public boolean hasCommentElement() { 2586 return this.comment != null && !this.comment.isEmpty(); 2587 } 2588 2589 public boolean hasComment() { 2590 return this.comment != null && !this.comment.isEmpty(); 2591 } 2592 2593 /** 2594 * @param value {@link #comment} (A description of status/issues in mapping that conveys additional information not represented in the structured data.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2595 */ 2596 public TargetElementComponent setCommentElement(StringType value) { 2597 this.comment = value; 2598 return this; 2599 } 2600 2601 /** 2602 * @return A description of status/issues in mapping that conveys additional information not represented in the structured data. 2603 */ 2604 public String getComment() { 2605 return this.comment == null ? null : this.comment.getValue(); 2606 } 2607 2608 /** 2609 * @param value A description of status/issues in mapping that conveys additional information not represented in the structured data. 2610 */ 2611 public TargetElementComponent setComment(String value) { 2612 if (Utilities.noString(value)) 2613 this.comment = null; 2614 else { 2615 if (this.comment == null) 2616 this.comment = new StringType(); 2617 this.comment.setValue(value); 2618 } 2619 return this; 2620 } 2621 2622 /** 2623 * @return {@link #property} (A property value for this source -> target mapping.) 2624 */ 2625 public List<MappingPropertyComponent> getProperty() { 2626 if (this.property == null) 2627 this.property = new ArrayList<MappingPropertyComponent>(); 2628 return this.property; 2629 } 2630 2631 /** 2632 * @return Returns a reference to <code>this</code> for easy method chaining 2633 */ 2634 public TargetElementComponent setProperty(List<MappingPropertyComponent> theProperty) { 2635 this.property = theProperty; 2636 return this; 2637 } 2638 2639 public boolean hasProperty() { 2640 if (this.property == null) 2641 return false; 2642 for (MappingPropertyComponent item : this.property) 2643 if (!item.isEmpty()) 2644 return true; 2645 return false; 2646 } 2647 2648 public MappingPropertyComponent addProperty() { //3 2649 MappingPropertyComponent t = new MappingPropertyComponent(); 2650 if (this.property == null) 2651 this.property = new ArrayList<MappingPropertyComponent>(); 2652 this.property.add(t); 2653 return t; 2654 } 2655 2656 public TargetElementComponent addProperty(MappingPropertyComponent t) { //3 2657 if (t == null) 2658 return this; 2659 if (this.property == null) 2660 this.property = new ArrayList<MappingPropertyComponent>(); 2661 this.property.add(t); 2662 return this; 2663 } 2664 2665 /** 2666 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 2667 */ 2668 public MappingPropertyComponent getPropertyFirstRep() { 2669 if (getProperty().isEmpty()) { 2670 addProperty(); 2671 } 2672 return getProperty().get(0); 2673 } 2674 2675 /** 2676 * @return {@link #dependsOn} (A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified data attribute can be resolved, and it has the specified value.) 2677 */ 2678 public List<OtherElementComponent> getDependsOn() { 2679 if (this.dependsOn == null) 2680 this.dependsOn = new ArrayList<OtherElementComponent>(); 2681 return this.dependsOn; 2682 } 2683 2684 /** 2685 * @return Returns a reference to <code>this</code> for easy method chaining 2686 */ 2687 public TargetElementComponent setDependsOn(List<OtherElementComponent> theDependsOn) { 2688 this.dependsOn = theDependsOn; 2689 return this; 2690 } 2691 2692 public boolean hasDependsOn() { 2693 if (this.dependsOn == null) 2694 return false; 2695 for (OtherElementComponent item : this.dependsOn) 2696 if (!item.isEmpty()) 2697 return true; 2698 return false; 2699 } 2700 2701 public OtherElementComponent addDependsOn() { //3 2702 OtherElementComponent t = new OtherElementComponent(); 2703 if (this.dependsOn == null) 2704 this.dependsOn = new ArrayList<OtherElementComponent>(); 2705 this.dependsOn.add(t); 2706 return t; 2707 } 2708 2709 public TargetElementComponent addDependsOn(OtherElementComponent t) { //3 2710 if (t == null) 2711 return this; 2712 if (this.dependsOn == null) 2713 this.dependsOn = new ArrayList<OtherElementComponent>(); 2714 this.dependsOn.add(t); 2715 return this; 2716 } 2717 2718 /** 2719 * @return The first repetition of repeating field {@link #dependsOn}, creating it if it does not already exist {3} 2720 */ 2721 public OtherElementComponent getDependsOnFirstRep() { 2722 if (getDependsOn().isEmpty()) { 2723 addDependsOn(); 2724 } 2725 return getDependsOn().get(0); 2726 } 2727 2728 /** 2729 * @return {@link #product} (Product is the output of a ConceptMap that provides additional values that go in other attributes / data elemnts of the target data.) 2730 */ 2731 public List<OtherElementComponent> getProduct() { 2732 if (this.product == null) 2733 this.product = new ArrayList<OtherElementComponent>(); 2734 return this.product; 2735 } 2736 2737 /** 2738 * @return Returns a reference to <code>this</code> for easy method chaining 2739 */ 2740 public TargetElementComponent setProduct(List<OtherElementComponent> theProduct) { 2741 this.product = theProduct; 2742 return this; 2743 } 2744 2745 public boolean hasProduct() { 2746 if (this.product == null) 2747 return false; 2748 for (OtherElementComponent item : this.product) 2749 if (!item.isEmpty()) 2750 return true; 2751 return false; 2752 } 2753 2754 public OtherElementComponent addProduct() { //3 2755 OtherElementComponent t = new OtherElementComponent(); 2756 if (this.product == null) 2757 this.product = new ArrayList<OtherElementComponent>(); 2758 this.product.add(t); 2759 return t; 2760 } 2761 2762 public TargetElementComponent addProduct(OtherElementComponent t) { //3 2763 if (t == null) 2764 return this; 2765 if (this.product == null) 2766 this.product = new ArrayList<OtherElementComponent>(); 2767 this.product.add(t); 2768 return this; 2769 } 2770 2771 /** 2772 * @return The first repetition of repeating field {@link #product}, creating it if it does not already exist {3} 2773 */ 2774 public OtherElementComponent getProductFirstRep() { 2775 if (getProduct().isEmpty()) { 2776 addProduct(); 2777 } 2778 return getProduct().get(0); 2779 } 2780 2781 protected void listChildren(List<Property> children) { 2782 super.listChildren(children); 2783 children.add(new Property("code", "code", "Identity (code or path) or the element/item that the map refers to.", 0, 1, code)); 2784 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 2785 children.add(new Property("valueSet", "canonical(ValueSet)", "The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set.", 0, 1, valueSet)); 2786 children.add(new Property("relationship", "code", "The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target).", 0, 1, relationship)); 2787 children.add(new Property("comment", "string", "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 0, 1, comment)); 2788 children.add(new Property("property", "", "A property value for this source -> target mapping.", 0, java.lang.Integer.MAX_VALUE, property)); 2789 children.add(new Property("dependsOn", "", "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified data attribute can be resolved, and it has the specified value.", 0, java.lang.Integer.MAX_VALUE, dependsOn)); 2790 children.add(new Property("product", "@ConceptMap.group.element.target.dependsOn", "Product is the output of a ConceptMap that provides additional values that go in other attributes / data elemnts of the target data.", 0, java.lang.Integer.MAX_VALUE, product)); 2791 } 2792 2793 @Override 2794 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2795 switch (_hash) { 2796 case 3059181: /*code*/ return new Property("code", "code", "Identity (code or path) or the element/item that the map refers to.", 0, 1, code); 2797 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 2798 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "The set of concepts from the ConceptMap.group.target code system which are all being mapped to as part of this mapping rule. The effect of using this data element is the same as having multiple ConceptMap.group.element.target elements with one for each concept in the ConceptMap.group.element.target.valueSet value set.", 0, 1, valueSet); 2799 case -261851592: /*relationship*/ return new Property("relationship", "code", "The relationship between the source and target concepts. The relationship is read from source to target (e.g. source-is-narrower-than-target).", 0, 1, relationship); 2800 case 950398559: /*comment*/ return new Property("comment", "string", "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 0, 1, comment); 2801 case -993141291: /*property*/ return new Property("property", "", "A property value for this source -> target mapping.", 0, java.lang.Integer.MAX_VALUE, property); 2802 case -1109214266: /*dependsOn*/ return new Property("dependsOn", "", "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified data attribute can be resolved, and it has the specified value.", 0, java.lang.Integer.MAX_VALUE, dependsOn); 2803 case -309474065: /*product*/ return new Property("product", "@ConceptMap.group.element.target.dependsOn", "Product is the output of a ConceptMap that provides additional values that go in other attributes / data elemnts of the target data.", 0, java.lang.Integer.MAX_VALUE, product); 2804 default: return super.getNamedProperty(_hash, _name, _checkValid); 2805 } 2806 2807 } 2808 2809 @Override 2810 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2811 switch (hash) { 2812 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2813 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 2814 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 2815 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ConceptMapRelationship> 2816 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 2817 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // MappingPropertyComponent 2818 case -1109214266: /*dependsOn*/ return this.dependsOn == null ? new Base[0] : this.dependsOn.toArray(new Base[this.dependsOn.size()]); // OtherElementComponent 2819 case -309474065: /*product*/ return this.product == null ? new Base[0] : this.product.toArray(new Base[this.product.size()]); // OtherElementComponent 2820 default: return super.getProperty(hash, name, checkValid); 2821 } 2822 2823 } 2824 2825 @Override 2826 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2827 switch (hash) { 2828 case 3059181: // code 2829 this.code = TypeConvertor.castToCode(value); // CodeType 2830 return value; 2831 case 1671764162: // display 2832 this.display = TypeConvertor.castToString(value); // StringType 2833 return value; 2834 case -1410174671: // valueSet 2835 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 2836 return value; 2837 case -261851592: // relationship 2838 value = new ConceptMapRelationshipEnumFactory().fromType(TypeConvertor.castToCode(value)); 2839 this.relationship = (Enumeration) value; // Enumeration<ConceptMapRelationship> 2840 return value; 2841 case 950398559: // comment 2842 this.comment = TypeConvertor.castToString(value); // StringType 2843 return value; 2844 case -993141291: // property 2845 this.getProperty().add((MappingPropertyComponent) value); // MappingPropertyComponent 2846 return value; 2847 case -1109214266: // dependsOn 2848 this.getDependsOn().add((OtherElementComponent) value); // OtherElementComponent 2849 return value; 2850 case -309474065: // product 2851 this.getProduct().add((OtherElementComponent) value); // OtherElementComponent 2852 return value; 2853 default: return super.setProperty(hash, name, value); 2854 } 2855 2856 } 2857 2858 @Override 2859 public Base setProperty(String name, Base value) throws FHIRException { 2860 if (name.equals("code")) { 2861 this.code = TypeConvertor.castToCode(value); // CodeType 2862 } else if (name.equals("display")) { 2863 this.display = TypeConvertor.castToString(value); // StringType 2864 } else if (name.equals("valueSet")) { 2865 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 2866 } else if (name.equals("relationship")) { 2867 value = new ConceptMapRelationshipEnumFactory().fromType(TypeConvertor.castToCode(value)); 2868 this.relationship = (Enumeration) value; // Enumeration<ConceptMapRelationship> 2869 } else if (name.equals("comment")) { 2870 this.comment = TypeConvertor.castToString(value); // StringType 2871 } else if (name.equals("property")) { 2872 this.getProperty().add((MappingPropertyComponent) value); 2873 } else if (name.equals("dependsOn")) { 2874 this.getDependsOn().add((OtherElementComponent) value); 2875 } else if (name.equals("product")) { 2876 this.getProduct().add((OtherElementComponent) value); 2877 } else 2878 return super.setProperty(name, value); 2879 return value; 2880 } 2881 2882 @Override 2883 public void removeChild(String name, Base value) throws FHIRException { 2884 if (name.equals("code")) { 2885 this.code = null; 2886 } else if (name.equals("display")) { 2887 this.display = null; 2888 } else if (name.equals("valueSet")) { 2889 this.valueSet = null; 2890 } else if (name.equals("relationship")) { 2891 value = new ConceptMapRelationshipEnumFactory().fromType(TypeConvertor.castToCode(value)); 2892 this.relationship = (Enumeration) value; // Enumeration<ConceptMapRelationship> 2893 } else if (name.equals("comment")) { 2894 this.comment = null; 2895 } else if (name.equals("property")) { 2896 this.getProperty().remove((MappingPropertyComponent) value); 2897 } else if (name.equals("dependsOn")) { 2898 this.getDependsOn().remove((OtherElementComponent) value); 2899 } else if (name.equals("product")) { 2900 this.getProduct().remove((OtherElementComponent) value); 2901 } else 2902 super.removeChild(name, value); 2903 2904 } 2905 2906 @Override 2907 public Base makeProperty(int hash, String name) throws FHIRException { 2908 switch (hash) { 2909 case 3059181: return getCodeElement(); 2910 case 1671764162: return getDisplayElement(); 2911 case -1410174671: return getValueSetElement(); 2912 case -261851592: return getRelationshipElement(); 2913 case 950398559: return getCommentElement(); 2914 case -993141291: return addProperty(); 2915 case -1109214266: return addDependsOn(); 2916 case -309474065: return addProduct(); 2917 default: return super.makeProperty(hash, name); 2918 } 2919 2920 } 2921 2922 @Override 2923 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2924 switch (hash) { 2925 case 3059181: /*code*/ return new String[] {"code"}; 2926 case 1671764162: /*display*/ return new String[] {"string"}; 2927 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 2928 case -261851592: /*relationship*/ return new String[] {"code"}; 2929 case 950398559: /*comment*/ return new String[] {"string"}; 2930 case -993141291: /*property*/ return new String[] {}; 2931 case -1109214266: /*dependsOn*/ return new String[] {}; 2932 case -309474065: /*product*/ return new String[] {"@ConceptMap.group.element.target.dependsOn"}; 2933 default: return super.getTypesForProperty(hash, name); 2934 } 2935 2936 } 2937 2938 @Override 2939 public Base addChild(String name) throws FHIRException { 2940 if (name.equals("code")) { 2941 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.code"); 2942 } 2943 else if (name.equals("display")) { 2944 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.display"); 2945 } 2946 else if (name.equals("valueSet")) { 2947 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.valueSet"); 2948 } 2949 else if (name.equals("relationship")) { 2950 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.relationship"); 2951 } 2952 else if (name.equals("comment")) { 2953 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.comment"); 2954 } 2955 else if (name.equals("property")) { 2956 return addProperty(); 2957 } 2958 else if (name.equals("dependsOn")) { 2959 return addDependsOn(); 2960 } 2961 else if (name.equals("product")) { 2962 return addProduct(); 2963 } 2964 else 2965 return super.addChild(name); 2966 } 2967 2968 public TargetElementComponent copy() { 2969 TargetElementComponent dst = new TargetElementComponent(); 2970 copyValues(dst); 2971 return dst; 2972 } 2973 2974 public void copyValues(TargetElementComponent dst) { 2975 super.copyValues(dst); 2976 dst.code = code == null ? null : code.copy(); 2977 dst.display = display == null ? null : display.copy(); 2978 dst.valueSet = valueSet == null ? null : valueSet.copy(); 2979 dst.relationship = relationship == null ? null : relationship.copy(); 2980 dst.comment = comment == null ? null : comment.copy(); 2981 if (property != null) { 2982 dst.property = new ArrayList<MappingPropertyComponent>(); 2983 for (MappingPropertyComponent i : property) 2984 dst.property.add(i.copy()); 2985 }; 2986 if (dependsOn != null) { 2987 dst.dependsOn = new ArrayList<OtherElementComponent>(); 2988 for (OtherElementComponent i : dependsOn) 2989 dst.dependsOn.add(i.copy()); 2990 }; 2991 if (product != null) { 2992 dst.product = new ArrayList<OtherElementComponent>(); 2993 for (OtherElementComponent i : product) 2994 dst.product.add(i.copy()); 2995 }; 2996 } 2997 2998 @Override 2999 public boolean equalsDeep(Base other_) { 3000 if (!super.equalsDeep(other_)) 3001 return false; 3002 if (!(other_ instanceof TargetElementComponent)) 3003 return false; 3004 TargetElementComponent o = (TargetElementComponent) other_; 3005 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(valueSet, o.valueSet, true) 3006 && compareDeep(relationship, o.relationship, true) && compareDeep(comment, o.comment, true) && compareDeep(property, o.property, true) 3007 && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(product, o.product, true); 3008 } 3009 3010 @Override 3011 public boolean equalsShallow(Base other_) { 3012 if (!super.equalsShallow(other_)) 3013 return false; 3014 if (!(other_ instanceof TargetElementComponent)) 3015 return false; 3016 TargetElementComponent o = (TargetElementComponent) other_; 3017 return compareValues(code, o.code, true) && compareValues(display, o.display, true) && compareValues(valueSet, o.valueSet, true) 3018 && compareValues(relationship, o.relationship, true) && compareValues(comment, o.comment, true); 3019 } 3020 3021 public boolean isEmpty() { 3022 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, valueSet 3023 , relationship, comment, property, dependsOn, product); 3024 } 3025 3026 public String fhirType() { 3027 return "ConceptMap.group.element.target"; 3028 3029 } 3030 3031 } 3032 3033 @Block() 3034 public static class MappingPropertyComponent extends BackboneElement implements IBaseBackboneElement { 3035 /** 3036 * A reference to a mapping property defined in ConceptMap.property. 3037 */ 3038 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3039 @Description(shortDefinition="Reference to ConceptMap.property.code", formalDefinition="A reference to a mapping property defined in ConceptMap.property." ) 3040 protected CodeType code; 3041 3042 /** 3043 * The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element. 3044 */ 3045 @Child(name = "value", type = {Coding.class, StringType.class, IntegerType.class, BooleanType.class, DateTimeType.class, DecimalType.class, CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 3046 @Description(shortDefinition="Value of the property for this concept", formalDefinition="The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element." ) 3047 protected DataType value; 3048 3049 private static final long serialVersionUID = -422546419L; 3050 3051 /** 3052 * Constructor 3053 */ 3054 public MappingPropertyComponent() { 3055 super(); 3056 } 3057 3058 /** 3059 * Constructor 3060 */ 3061 public MappingPropertyComponent(String code, DataType value) { 3062 super(); 3063 this.setCode(code); 3064 this.setValue(value); 3065 } 3066 3067 /** 3068 * @return {@link #code} (A reference to a mapping property defined in ConceptMap.property.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3069 */ 3070 public CodeType getCodeElement() { 3071 if (this.code == null) 3072 if (Configuration.errorOnAutoCreate()) 3073 throw new Error("Attempt to auto-create MappingPropertyComponent.code"); 3074 else if (Configuration.doAutoCreate()) 3075 this.code = new CodeType(); // bb 3076 return this.code; 3077 } 3078 3079 public boolean hasCodeElement() { 3080 return this.code != null && !this.code.isEmpty(); 3081 } 3082 3083 public boolean hasCode() { 3084 return this.code != null && !this.code.isEmpty(); 3085 } 3086 3087 /** 3088 * @param value {@link #code} (A reference to a mapping property defined in ConceptMap.property.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3089 */ 3090 public MappingPropertyComponent setCodeElement(CodeType value) { 3091 this.code = value; 3092 return this; 3093 } 3094 3095 /** 3096 * @return A reference to a mapping property defined in ConceptMap.property. 3097 */ 3098 public String getCode() { 3099 return this.code == null ? null : this.code.getValue(); 3100 } 3101 3102 /** 3103 * @param value A reference to a mapping property defined in ConceptMap.property. 3104 */ 3105 public MappingPropertyComponent setCode(String value) { 3106 if (this.code == null) 3107 this.code = new CodeType(); 3108 this.code.setValue(value); 3109 return this; 3110 } 3111 3112 /** 3113 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3114 */ 3115 public DataType getValue() { 3116 return this.value; 3117 } 3118 3119 /** 3120 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3121 */ 3122 public Coding getValueCoding() throws FHIRException { 3123 if (this.value == null) 3124 this.value = new Coding(); 3125 if (!(this.value instanceof Coding)) 3126 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 3127 return (Coding) this.value; 3128 } 3129 3130 public boolean hasValueCoding() { 3131 return this != null && this.value instanceof Coding; 3132 } 3133 3134 /** 3135 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3136 */ 3137 public StringType getValueStringType() throws FHIRException { 3138 if (this.value == null) 3139 this.value = new StringType(); 3140 if (!(this.value instanceof StringType)) 3141 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3142 return (StringType) this.value; 3143 } 3144 3145 public boolean hasValueStringType() { 3146 return this != null && this.value instanceof StringType; 3147 } 3148 3149 /** 3150 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3151 */ 3152 public IntegerType getValueIntegerType() throws FHIRException { 3153 if (this.value == null) 3154 this.value = new IntegerType(); 3155 if (!(this.value instanceof IntegerType)) 3156 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 3157 return (IntegerType) this.value; 3158 } 3159 3160 public boolean hasValueIntegerType() { 3161 return this != null && this.value instanceof IntegerType; 3162 } 3163 3164 /** 3165 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3166 */ 3167 public BooleanType getValueBooleanType() throws FHIRException { 3168 if (this.value == null) 3169 this.value = new BooleanType(); 3170 if (!(this.value instanceof BooleanType)) 3171 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 3172 return (BooleanType) this.value; 3173 } 3174 3175 public boolean hasValueBooleanType() { 3176 return this != null && this.value instanceof BooleanType; 3177 } 3178 3179 /** 3180 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3181 */ 3182 public DateTimeType getValueDateTimeType() throws FHIRException { 3183 if (this.value == null) 3184 this.value = new DateTimeType(); 3185 if (!(this.value instanceof DateTimeType)) 3186 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3187 return (DateTimeType) this.value; 3188 } 3189 3190 public boolean hasValueDateTimeType() { 3191 return this != null && this.value instanceof DateTimeType; 3192 } 3193 3194 /** 3195 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3196 */ 3197 public DecimalType getValueDecimalType() throws FHIRException { 3198 if (this.value == null) 3199 this.value = new DecimalType(); 3200 if (!(this.value instanceof DecimalType)) 3201 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 3202 return (DecimalType) this.value; 3203 } 3204 3205 public boolean hasValueDecimalType() { 3206 return this != null && this.value instanceof DecimalType; 3207 } 3208 3209 /** 3210 * @return {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3211 */ 3212 public CodeType getValueCodeType() throws FHIRException { 3213 if (this.value == null) 3214 this.value = new CodeType(); 3215 if (!(this.value instanceof CodeType)) 3216 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3217 return (CodeType) this.value; 3218 } 3219 3220 public boolean hasValueCodeType() { 3221 return this != null && this.value instanceof CodeType; 3222 } 3223 3224 public boolean hasValue() { 3225 return this.value != null && !this.value.isEmpty(); 3226 } 3227 3228 /** 3229 * @param value {@link #value} (The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.) 3230 */ 3231 public MappingPropertyComponent setValue(DataType value) { 3232 if (value != null && !(value instanceof Coding || value instanceof StringType || value instanceof IntegerType || value instanceof BooleanType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof CodeType)) 3233 throw new FHIRException("Not the right type for ConceptMap.group.element.target.property.value[x]: "+value.fhirType()); 3234 this.value = value; 3235 return this; 3236 } 3237 3238 protected void listChildren(List<Property> children) { 3239 super.listChildren(children); 3240 children.add(new Property("code", "code", "A reference to a mapping property defined in ConceptMap.property.", 0, 1, code)); 3241 children.add(new Property("value[x]", "Coding|string|integer|boolean|dateTime|decimal|code", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value)); 3242 } 3243 3244 @Override 3245 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3246 switch (_hash) { 3247 case 3059181: /*code*/ return new Property("code", "code", "A reference to a mapping property defined in ConceptMap.property.", 0, 1, code); 3248 case -1410166417: /*value[x]*/ return new Property("value[x]", "Coding|string|integer|boolean|dateTime|decimal|code", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3249 case 111972721: /*value*/ return new Property("value[x]", "Coding|string|integer|boolean|dateTime|decimal|code", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3250 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3251 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3252 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3253 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3254 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3255 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3256 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of this property. If the type chosen for this element is 'code', then the property SHALL be defined in a ConceptMap.property element.", 0, 1, value); 3257 default: return super.getNamedProperty(_hash, _name, _checkValid); 3258 } 3259 3260 } 3261 3262 @Override 3263 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3264 switch (hash) { 3265 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 3266 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3267 default: return super.getProperty(hash, name, checkValid); 3268 } 3269 3270 } 3271 3272 @Override 3273 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3274 switch (hash) { 3275 case 3059181: // code 3276 this.code = TypeConvertor.castToCode(value); // CodeType 3277 return value; 3278 case 111972721: // value 3279 this.value = TypeConvertor.castToType(value); // DataType 3280 return value; 3281 default: return super.setProperty(hash, name, value); 3282 } 3283 3284 } 3285 3286 @Override 3287 public Base setProperty(String name, Base value) throws FHIRException { 3288 if (name.equals("code")) { 3289 this.code = TypeConvertor.castToCode(value); // CodeType 3290 } else if (name.equals("value[x]")) { 3291 this.value = TypeConvertor.castToType(value); // DataType 3292 } else 3293 return super.setProperty(name, value); 3294 return value; 3295 } 3296 3297 @Override 3298 public void removeChild(String name, Base value) throws FHIRException { 3299 if (name.equals("code")) { 3300 this.code = null; 3301 } else if (name.equals("value[x]")) { 3302 this.value = null; 3303 } else 3304 super.removeChild(name, value); 3305 3306 } 3307 3308 @Override 3309 public Base makeProperty(int hash, String name) throws FHIRException { 3310 switch (hash) { 3311 case 3059181: return getCodeElement(); 3312 case -1410166417: return getValue(); 3313 case 111972721: return getValue(); 3314 default: return super.makeProperty(hash, name); 3315 } 3316 3317 } 3318 3319 @Override 3320 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3321 switch (hash) { 3322 case 3059181: /*code*/ return new String[] {"code"}; 3323 case 111972721: /*value*/ return new String[] {"Coding", "string", "integer", "boolean", "dateTime", "decimal", "code"}; 3324 default: return super.getTypesForProperty(hash, name); 3325 } 3326 3327 } 3328 3329 @Override 3330 public Base addChild(String name) throws FHIRException { 3331 if (name.equals("code")) { 3332 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.property.code"); 3333 } 3334 else if (name.equals("valueCoding")) { 3335 this.value = new Coding(); 3336 return this.value; 3337 } 3338 else if (name.equals("valueString")) { 3339 this.value = new StringType(); 3340 return this.value; 3341 } 3342 else if (name.equals("valueInteger")) { 3343 this.value = new IntegerType(); 3344 return this.value; 3345 } 3346 else if (name.equals("valueBoolean")) { 3347 this.value = new BooleanType(); 3348 return this.value; 3349 } 3350 else if (name.equals("valueDateTime")) { 3351 this.value = new DateTimeType(); 3352 return this.value; 3353 } 3354 else if (name.equals("valueDecimal")) { 3355 this.value = new DecimalType(); 3356 return this.value; 3357 } 3358 else if (name.equals("valueCode")) { 3359 this.value = new CodeType(); 3360 return this.value; 3361 } 3362 else 3363 return super.addChild(name); 3364 } 3365 3366 public MappingPropertyComponent copy() { 3367 MappingPropertyComponent dst = new MappingPropertyComponent(); 3368 copyValues(dst); 3369 return dst; 3370 } 3371 3372 public void copyValues(MappingPropertyComponent dst) { 3373 super.copyValues(dst); 3374 dst.code = code == null ? null : code.copy(); 3375 dst.value = value == null ? null : value.copy(); 3376 } 3377 3378 @Override 3379 public boolean equalsDeep(Base other_) { 3380 if (!super.equalsDeep(other_)) 3381 return false; 3382 if (!(other_ instanceof MappingPropertyComponent)) 3383 return false; 3384 MappingPropertyComponent o = (MappingPropertyComponent) other_; 3385 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 3386 } 3387 3388 @Override 3389 public boolean equalsShallow(Base other_) { 3390 if (!super.equalsShallow(other_)) 3391 return false; 3392 if (!(other_ instanceof MappingPropertyComponent)) 3393 return false; 3394 MappingPropertyComponent o = (MappingPropertyComponent) other_; 3395 return compareValues(code, o.code, true); 3396 } 3397 3398 public boolean isEmpty() { 3399 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 3400 } 3401 3402 public String fhirType() { 3403 return "ConceptMap.group.element.target.property"; 3404 3405 } 3406 3407 } 3408 3409 @Block() 3410 public static class OtherElementComponent extends BackboneElement implements IBaseBackboneElement { 3411 /** 3412 * A reference to the additional attribute that holds a value the map depends on. 3413 */ 3414 @Child(name = "attribute", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3415 @Description(shortDefinition="A reference to a mapping attribute defined in ConceptMap.additionalAttribute", formalDefinition="A reference to the additional attribute that holds a value the map depends on." ) 3416 protected CodeType attribute; 3417 3418 /** 3419 * Data element value that the map depends on / produces. 3420 */ 3421 @Child(name = "value", type = {CodeType.class, Coding.class, StringType.class, BooleanType.class, Quantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 3422 @Description(shortDefinition="Value of the referenced data element", formalDefinition="Data element value that the map depends on / produces." ) 3423 protected DataType value; 3424 3425 /** 3426 * This mapping applies if the data element value is a code from this value set. 3427 */ 3428 @Child(name = "valueSet", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3429 @Description(shortDefinition="The mapping depends on a data element with a value from this value set", formalDefinition="This mapping applies if the data element value is a code from this value set." ) 3430 protected CanonicalType valueSet; 3431 3432 private static final long serialVersionUID = 2103818133L; 3433 3434 /** 3435 * Constructor 3436 */ 3437 public OtherElementComponent() { 3438 super(); 3439 } 3440 3441 /** 3442 * Constructor 3443 */ 3444 public OtherElementComponent(String attribute) { 3445 super(); 3446 this.setAttribute(attribute); 3447 } 3448 3449 /** 3450 * @return {@link #attribute} (A reference to the additional attribute that holds a value the map depends on.). This is the underlying object with id, value and extensions. The accessor "getAttribute" gives direct access to the value 3451 */ 3452 public CodeType getAttributeElement() { 3453 if (this.attribute == null) 3454 if (Configuration.errorOnAutoCreate()) 3455 throw new Error("Attempt to auto-create OtherElementComponent.attribute"); 3456 else if (Configuration.doAutoCreate()) 3457 this.attribute = new CodeType(); // bb 3458 return this.attribute; 3459 } 3460 3461 public boolean hasAttributeElement() { 3462 return this.attribute != null && !this.attribute.isEmpty(); 3463 } 3464 3465 public boolean hasAttribute() { 3466 return this.attribute != null && !this.attribute.isEmpty(); 3467 } 3468 3469 /** 3470 * @param value {@link #attribute} (A reference to the additional attribute that holds a value the map depends on.). This is the underlying object with id, value and extensions. The accessor "getAttribute" gives direct access to the value 3471 */ 3472 public OtherElementComponent setAttributeElement(CodeType value) { 3473 this.attribute = value; 3474 return this; 3475 } 3476 3477 /** 3478 * @return A reference to the additional attribute that holds a value the map depends on. 3479 */ 3480 public String getAttribute() { 3481 return this.attribute == null ? null : this.attribute.getValue(); 3482 } 3483 3484 /** 3485 * @param value A reference to the additional attribute that holds a value the map depends on. 3486 */ 3487 public OtherElementComponent setAttribute(String value) { 3488 if (this.attribute == null) 3489 this.attribute = new CodeType(); 3490 this.attribute.setValue(value); 3491 return this; 3492 } 3493 3494 /** 3495 * @return {@link #value} (Data element value that the map depends on / produces.) 3496 */ 3497 public DataType getValue() { 3498 return this.value; 3499 } 3500 3501 /** 3502 * @return {@link #value} (Data element value that the map depends on / produces.) 3503 */ 3504 public CodeType getValueCodeType() throws FHIRException { 3505 if (this.value == null) 3506 this.value = new CodeType(); 3507 if (!(this.value instanceof CodeType)) 3508 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3509 return (CodeType) this.value; 3510 } 3511 3512 public boolean hasValueCodeType() { 3513 return this != null && this.value instanceof CodeType; 3514 } 3515 3516 /** 3517 * @return {@link #value} (Data element value that the map depends on / produces.) 3518 */ 3519 public Coding getValueCoding() throws FHIRException { 3520 if (this.value == null) 3521 this.value = new Coding(); 3522 if (!(this.value instanceof Coding)) 3523 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 3524 return (Coding) this.value; 3525 } 3526 3527 public boolean hasValueCoding() { 3528 return this != null && this.value instanceof Coding; 3529 } 3530 3531 /** 3532 * @return {@link #value} (Data element value that the map depends on / produces.) 3533 */ 3534 public StringType getValueStringType() throws FHIRException { 3535 if (this.value == null) 3536 this.value = new StringType(); 3537 if (!(this.value instanceof StringType)) 3538 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3539 return (StringType) this.value; 3540 } 3541 3542 public boolean hasValueStringType() { 3543 return this != null && this.value instanceof StringType; 3544 } 3545 3546 /** 3547 * @return {@link #value} (Data element value that the map depends on / produces.) 3548 */ 3549 public BooleanType getValueBooleanType() throws FHIRException { 3550 if (this.value == null) 3551 this.value = new BooleanType(); 3552 if (!(this.value instanceof BooleanType)) 3553 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 3554 return (BooleanType) this.value; 3555 } 3556 3557 public boolean hasValueBooleanType() { 3558 return this != null && this.value instanceof BooleanType; 3559 } 3560 3561 /** 3562 * @return {@link #value} (Data element value that the map depends on / produces.) 3563 */ 3564 public Quantity getValueQuantity() throws FHIRException { 3565 if (this.value == null) 3566 this.value = new Quantity(); 3567 if (!(this.value instanceof Quantity)) 3568 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3569 return (Quantity) this.value; 3570 } 3571 3572 public boolean hasValueQuantity() { 3573 return this != null && this.value instanceof Quantity; 3574 } 3575 3576 public boolean hasValue() { 3577 return this.value != null && !this.value.isEmpty(); 3578 } 3579 3580 /** 3581 * @param value {@link #value} (Data element value that the map depends on / produces.) 3582 */ 3583 public OtherElementComponent setValue(DataType value) { 3584 if (value != null && !(value instanceof CodeType || value instanceof Coding || value instanceof StringType || value instanceof BooleanType || value instanceof Quantity)) 3585 throw new FHIRException("Not the right type for ConceptMap.group.element.target.dependsOn.value[x]: "+value.fhirType()); 3586 this.value = value; 3587 return this; 3588 } 3589 3590 /** 3591 * @return {@link #valueSet} (This mapping applies if the data element value is a code from this value set.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 3592 */ 3593 public CanonicalType getValueSetElement() { 3594 if (this.valueSet == null) 3595 if (Configuration.errorOnAutoCreate()) 3596 throw new Error("Attempt to auto-create OtherElementComponent.valueSet"); 3597 else if (Configuration.doAutoCreate()) 3598 this.valueSet = new CanonicalType(); // bb 3599 return this.valueSet; 3600 } 3601 3602 public boolean hasValueSetElement() { 3603 return this.valueSet != null && !this.valueSet.isEmpty(); 3604 } 3605 3606 public boolean hasValueSet() { 3607 return this.valueSet != null && !this.valueSet.isEmpty(); 3608 } 3609 3610 /** 3611 * @param value {@link #valueSet} (This mapping applies if the data element value is a code from this value set.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 3612 */ 3613 public OtherElementComponent setValueSetElement(CanonicalType value) { 3614 this.valueSet = value; 3615 return this; 3616 } 3617 3618 /** 3619 * @return This mapping applies if the data element value is a code from this value set. 3620 */ 3621 public String getValueSet() { 3622 return this.valueSet == null ? null : this.valueSet.getValue(); 3623 } 3624 3625 /** 3626 * @param value This mapping applies if the data element value is a code from this value set. 3627 */ 3628 public OtherElementComponent setValueSet(String value) { 3629 if (Utilities.noString(value)) 3630 this.valueSet = null; 3631 else { 3632 if (this.valueSet == null) 3633 this.valueSet = new CanonicalType(); 3634 this.valueSet.setValue(value); 3635 } 3636 return this; 3637 } 3638 3639 protected void listChildren(List<Property> children) { 3640 super.listChildren(children); 3641 children.add(new Property("attribute", "code", "A reference to the additional attribute that holds a value the map depends on.", 0, 1, attribute)); 3642 children.add(new Property("value[x]", "code|Coding|string|boolean|Quantity", "Data element value that the map depends on / produces.", 0, 1, value)); 3643 children.add(new Property("valueSet", "canonical(ValueSet)", "This mapping applies if the data element value is a code from this value set.", 0, 1, valueSet)); 3644 } 3645 3646 @Override 3647 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3648 switch (_hash) { 3649 case 13085340: /*attribute*/ return new Property("attribute", "code", "A reference to the additional attribute that holds a value the map depends on.", 0, 1, attribute); 3650 case -1410166417: /*value[x]*/ return new Property("value[x]", "code|Coding|string|boolean|Quantity", "Data element value that the map depends on / produces.", 0, 1, value); 3651 case 111972721: /*value*/ return new Property("value[x]", "code|Coding|string|boolean|Quantity", "Data element value that the map depends on / produces.", 0, 1, value); 3652 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "Data element value that the map depends on / produces.", 0, 1, value); 3653 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "Data element value that the map depends on / produces.", 0, 1, value); 3654 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Data element value that the map depends on / produces.", 0, 1, value); 3655 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Data element value that the map depends on / produces.", 0, 1, value); 3656 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Data element value that the map depends on / produces.", 0, 1, value); 3657 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "This mapping applies if the data element value is a code from this value set.", 0, 1, valueSet); 3658 default: return super.getNamedProperty(_hash, _name, _checkValid); 3659 } 3660 3661 } 3662 3663 @Override 3664 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3665 switch (hash) { 3666 case 13085340: /*attribute*/ return this.attribute == null ? new Base[0] : new Base[] {this.attribute}; // CodeType 3667 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3668 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 3669 default: return super.getProperty(hash, name, checkValid); 3670 } 3671 3672 } 3673 3674 @Override 3675 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3676 switch (hash) { 3677 case 13085340: // attribute 3678 this.attribute = TypeConvertor.castToCode(value); // CodeType 3679 return value; 3680 case 111972721: // value 3681 this.value = TypeConvertor.castToType(value); // DataType 3682 return value; 3683 case -1410174671: // valueSet 3684 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 3685 return value; 3686 default: return super.setProperty(hash, name, value); 3687 } 3688 3689 } 3690 3691 @Override 3692 public Base setProperty(String name, Base value) throws FHIRException { 3693 if (name.equals("attribute")) { 3694 this.attribute = TypeConvertor.castToCode(value); // CodeType 3695 } else if (name.equals("value[x]")) { 3696 this.value = TypeConvertor.castToType(value); // DataType 3697 } else if (name.equals("valueSet")) { 3698 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 3699 } else 3700 return super.setProperty(name, value); 3701 return value; 3702 } 3703 3704 @Override 3705 public void removeChild(String name, Base value) throws FHIRException { 3706 if (name.equals("attribute")) { 3707 this.attribute = null; 3708 } else if (name.equals("value[x]")) { 3709 this.value = null; 3710 } else if (name.equals("valueSet")) { 3711 this.valueSet = null; 3712 } else 3713 super.removeChild(name, value); 3714 3715 } 3716 3717 @Override 3718 public Base makeProperty(int hash, String name) throws FHIRException { 3719 switch (hash) { 3720 case 13085340: return getAttributeElement(); 3721 case -1410166417: return getValue(); 3722 case 111972721: return getValue(); 3723 case -1410174671: return getValueSetElement(); 3724 default: return super.makeProperty(hash, name); 3725 } 3726 3727 } 3728 3729 @Override 3730 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3731 switch (hash) { 3732 case 13085340: /*attribute*/ return new String[] {"code"}; 3733 case 111972721: /*value*/ return new String[] {"code", "Coding", "string", "boolean", "Quantity"}; 3734 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 3735 default: return super.getTypesForProperty(hash, name); 3736 } 3737 3738 } 3739 3740 @Override 3741 public Base addChild(String name) throws FHIRException { 3742 if (name.equals("attribute")) { 3743 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.dependsOn.attribute"); 3744 } 3745 else if (name.equals("valueCode")) { 3746 this.value = new CodeType(); 3747 return this.value; 3748 } 3749 else if (name.equals("valueCoding")) { 3750 this.value = new Coding(); 3751 return this.value; 3752 } 3753 else if (name.equals("valueString")) { 3754 this.value = new StringType(); 3755 return this.value; 3756 } 3757 else if (name.equals("valueBoolean")) { 3758 this.value = new BooleanType(); 3759 return this.value; 3760 } 3761 else if (name.equals("valueQuantity")) { 3762 this.value = new Quantity(); 3763 return this.value; 3764 } 3765 else if (name.equals("valueSet")) { 3766 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.element.target.dependsOn.valueSet"); 3767 } 3768 else 3769 return super.addChild(name); 3770 } 3771 3772 public OtherElementComponent copy() { 3773 OtherElementComponent dst = new OtherElementComponent(); 3774 copyValues(dst); 3775 return dst; 3776 } 3777 3778 public void copyValues(OtherElementComponent dst) { 3779 super.copyValues(dst); 3780 dst.attribute = attribute == null ? null : attribute.copy(); 3781 dst.value = value == null ? null : value.copy(); 3782 dst.valueSet = valueSet == null ? null : valueSet.copy(); 3783 } 3784 3785 @Override 3786 public boolean equalsDeep(Base other_) { 3787 if (!super.equalsDeep(other_)) 3788 return false; 3789 if (!(other_ instanceof OtherElementComponent)) 3790 return false; 3791 OtherElementComponent o = (OtherElementComponent) other_; 3792 return compareDeep(attribute, o.attribute, true) && compareDeep(value, o.value, true) && compareDeep(valueSet, o.valueSet, true) 3793 ; 3794 } 3795 3796 @Override 3797 public boolean equalsShallow(Base other_) { 3798 if (!super.equalsShallow(other_)) 3799 return false; 3800 if (!(other_ instanceof OtherElementComponent)) 3801 return false; 3802 OtherElementComponent o = (OtherElementComponent) other_; 3803 return compareValues(attribute, o.attribute, true) && compareValues(valueSet, o.valueSet, true); 3804 } 3805 3806 public boolean isEmpty() { 3807 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(attribute, value, valueSet 3808 ); 3809 } 3810 3811 public String fhirType() { 3812 return "ConceptMap.group.element.target.dependsOn"; 3813 3814 } 3815 3816 } 3817 3818 @Block() 3819 public static class ConceptMapGroupUnmappedComponent extends BackboneElement implements IBaseBackboneElement { 3820 /** 3821 * Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL). 3822 */ 3823 @Child(name = "mode", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3824 @Description(shortDefinition="use-source-code | fixed | other-map", formalDefinition="Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL)." ) 3825 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conceptmap-unmapped-mode") 3826 protected Enumeration<ConceptMapGroupUnmappedMode> mode; 3827 3828 /** 3829 * The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code. 3830 */ 3831 @Child(name = "code", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3832 @Description(shortDefinition="Fixed code when mode = fixed", formalDefinition="The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code." ) 3833 protected CodeType code; 3834 3835 /** 3836 * The display for the code. The display is only provided to help editors when editing the concept map. 3837 */ 3838 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3839 @Description(shortDefinition="Display for the code", formalDefinition="The display for the code. The display is only provided to help editors when editing the concept map." ) 3840 protected StringType display; 3841 3842 /** 3843 * The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes. 3844 */ 3845 @Child(name = "valueSet", type = {CanonicalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3846 @Description(shortDefinition="Fixed code set when mode = fixed", formalDefinition="The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes." ) 3847 protected CanonicalType valueSet; 3848 3849 /** 3850 * The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'. 3851 */ 3852 @Child(name = "relationship", type = {CodeType.class}, order=5, min=0, max=1, modifier=true, summary=false) 3853 @Description(shortDefinition="related-to | equivalent | source-is-narrower-than-target | source-is-broader-than-target | not-related-to", formalDefinition="The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'." ) 3854 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concept-map-relationship") 3855 protected Enumeration<ConceptMapRelationship> relationship; 3856 3857 /** 3858 * The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept. 3859 */ 3860 @Child(name = "otherMap", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3861 @Description(shortDefinition="canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped", formalDefinition="The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept." ) 3862 protected CanonicalType otherMap; 3863 3864 private static final long serialVersionUID = 449945387L; 3865 3866 /** 3867 * Constructor 3868 */ 3869 public ConceptMapGroupUnmappedComponent() { 3870 super(); 3871 } 3872 3873 /** 3874 * Constructor 3875 */ 3876 public ConceptMapGroupUnmappedComponent(ConceptMapGroupUnmappedMode mode) { 3877 super(); 3878 this.setMode(mode); 3879 } 3880 3881 /** 3882 * @return {@link #mode} (Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 3883 */ 3884 public Enumeration<ConceptMapGroupUnmappedMode> getModeElement() { 3885 if (this.mode == null) 3886 if (Configuration.errorOnAutoCreate()) 3887 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.mode"); 3888 else if (Configuration.doAutoCreate()) 3889 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); // bb 3890 return this.mode; 3891 } 3892 3893 public boolean hasModeElement() { 3894 return this.mode != null && !this.mode.isEmpty(); 3895 } 3896 3897 public boolean hasMode() { 3898 return this.mode != null && !this.mode.isEmpty(); 3899 } 3900 3901 /** 3902 * @param value {@link #mode} (Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 3903 */ 3904 public ConceptMapGroupUnmappedComponent setModeElement(Enumeration<ConceptMapGroupUnmappedMode> value) { 3905 this.mode = value; 3906 return this; 3907 } 3908 3909 /** 3910 * @return Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL). 3911 */ 3912 public ConceptMapGroupUnmappedMode getMode() { 3913 return this.mode == null ? null : this.mode.getValue(); 3914 } 3915 3916 /** 3917 * @param value Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL). 3918 */ 3919 public ConceptMapGroupUnmappedComponent setMode(ConceptMapGroupUnmappedMode value) { 3920 if (this.mode == null) 3921 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); 3922 this.mode.setValue(value); 3923 return this; 3924 } 3925 3926 /** 3927 * @return {@link #code} (The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3928 */ 3929 public CodeType getCodeElement() { 3930 if (this.code == null) 3931 if (Configuration.errorOnAutoCreate()) 3932 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.code"); 3933 else if (Configuration.doAutoCreate()) 3934 this.code = new CodeType(); // bb 3935 return this.code; 3936 } 3937 3938 public boolean hasCodeElement() { 3939 return this.code != null && !this.code.isEmpty(); 3940 } 3941 3942 public boolean hasCode() { 3943 return this.code != null && !this.code.isEmpty(); 3944 } 3945 3946 /** 3947 * @param value {@link #code} (The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3948 */ 3949 public ConceptMapGroupUnmappedComponent setCodeElement(CodeType value) { 3950 this.code = value; 3951 return this; 3952 } 3953 3954 /** 3955 * @return The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code. 3956 */ 3957 public String getCode() { 3958 return this.code == null ? null : this.code.getValue(); 3959 } 3960 3961 /** 3962 * @param value The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code. 3963 */ 3964 public ConceptMapGroupUnmappedComponent setCode(String value) { 3965 if (Utilities.noString(value)) 3966 this.code = null; 3967 else { 3968 if (this.code == null) 3969 this.code = new CodeType(); 3970 this.code.setValue(value); 3971 } 3972 return this; 3973 } 3974 3975 /** 3976 * @return {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 3977 */ 3978 public StringType getDisplayElement() { 3979 if (this.display == null) 3980 if (Configuration.errorOnAutoCreate()) 3981 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.display"); 3982 else if (Configuration.doAutoCreate()) 3983 this.display = new StringType(); // bb 3984 return this.display; 3985 } 3986 3987 public boolean hasDisplayElement() { 3988 return this.display != null && !this.display.isEmpty(); 3989 } 3990 3991 public boolean hasDisplay() { 3992 return this.display != null && !this.display.isEmpty(); 3993 } 3994 3995 /** 3996 * @param value {@link #display} (The display for the code. The display is only provided to help editors when editing the concept map.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 3997 */ 3998 public ConceptMapGroupUnmappedComponent setDisplayElement(StringType value) { 3999 this.display = value; 4000 return this; 4001 } 4002 4003 /** 4004 * @return The display for the code. The display is only provided to help editors when editing the concept map. 4005 */ 4006 public String getDisplay() { 4007 return this.display == null ? null : this.display.getValue(); 4008 } 4009 4010 /** 4011 * @param value The display for the code. The display is only provided to help editors when editing the concept map. 4012 */ 4013 public ConceptMapGroupUnmappedComponent setDisplay(String value) { 4014 if (Utilities.noString(value)) 4015 this.display = null; 4016 else { 4017 if (this.display == null) 4018 this.display = new StringType(); 4019 this.display.setValue(value); 4020 } 4021 return this; 4022 } 4023 4024 /** 4025 * @return {@link #valueSet} (The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4026 */ 4027 public CanonicalType getValueSetElement() { 4028 if (this.valueSet == null) 4029 if (Configuration.errorOnAutoCreate()) 4030 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.valueSet"); 4031 else if (Configuration.doAutoCreate()) 4032 this.valueSet = new CanonicalType(); // bb 4033 return this.valueSet; 4034 } 4035 4036 public boolean hasValueSetElement() { 4037 return this.valueSet != null && !this.valueSet.isEmpty(); 4038 } 4039 4040 public boolean hasValueSet() { 4041 return this.valueSet != null && !this.valueSet.isEmpty(); 4042 } 4043 4044 /** 4045 * @param value {@link #valueSet} (The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4046 */ 4047 public ConceptMapGroupUnmappedComponent setValueSetElement(CanonicalType value) { 4048 this.valueSet = value; 4049 return this; 4050 } 4051 4052 /** 4053 * @return The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes. 4054 */ 4055 public String getValueSet() { 4056 return this.valueSet == null ? null : this.valueSet.getValue(); 4057 } 4058 4059 /** 4060 * @param value The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes. 4061 */ 4062 public ConceptMapGroupUnmappedComponent setValueSet(String value) { 4063 if (Utilities.noString(value)) 4064 this.valueSet = null; 4065 else { 4066 if (this.valueSet == null) 4067 this.valueSet = new CanonicalType(); 4068 this.valueSet.setValue(value); 4069 } 4070 return this; 4071 } 4072 4073 /** 4074 * @return {@link #relationship} (The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 4075 */ 4076 public Enumeration<ConceptMapRelationship> getRelationshipElement() { 4077 if (this.relationship == null) 4078 if (Configuration.errorOnAutoCreate()) 4079 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.relationship"); 4080 else if (Configuration.doAutoCreate()) 4081 this.relationship = new Enumeration<ConceptMapRelationship>(new ConceptMapRelationshipEnumFactory()); // bb 4082 return this.relationship; 4083 } 4084 4085 public boolean hasRelationshipElement() { 4086 return this.relationship != null && !this.relationship.isEmpty(); 4087 } 4088 4089 public boolean hasRelationship() { 4090 return this.relationship != null && !this.relationship.isEmpty(); 4091 } 4092 4093 /** 4094 * @param value {@link #relationship} (The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 4095 */ 4096 public ConceptMapGroupUnmappedComponent setRelationshipElement(Enumeration<ConceptMapRelationship> value) { 4097 this.relationship = value; 4098 return this; 4099 } 4100 4101 /** 4102 * @return The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'. 4103 */ 4104 public ConceptMapRelationship getRelationship() { 4105 return this.relationship == null ? null : this.relationship.getValue(); 4106 } 4107 4108 /** 4109 * @param value The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'. 4110 */ 4111 public ConceptMapGroupUnmappedComponent setRelationship(ConceptMapRelationship value) { 4112 if (value == null) 4113 this.relationship = null; 4114 else { 4115 if (this.relationship == null) 4116 this.relationship = new Enumeration<ConceptMapRelationship>(new ConceptMapRelationshipEnumFactory()); 4117 this.relationship.setValue(value); 4118 } 4119 return this; 4120 } 4121 4122 /** 4123 * @return {@link #otherMap} (The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.). This is the underlying object with id, value and extensions. The accessor "getOtherMap" gives direct access to the value 4124 */ 4125 public CanonicalType getOtherMapElement() { 4126 if (this.otherMap == null) 4127 if (Configuration.errorOnAutoCreate()) 4128 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.otherMap"); 4129 else if (Configuration.doAutoCreate()) 4130 this.otherMap = new CanonicalType(); // bb 4131 return this.otherMap; 4132 } 4133 4134 public boolean hasOtherMapElement() { 4135 return this.otherMap != null && !this.otherMap.isEmpty(); 4136 } 4137 4138 public boolean hasOtherMap() { 4139 return this.otherMap != null && !this.otherMap.isEmpty(); 4140 } 4141 4142 /** 4143 * @param value {@link #otherMap} (The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.). This is the underlying object with id, value and extensions. The accessor "getOtherMap" gives direct access to the value 4144 */ 4145 public ConceptMapGroupUnmappedComponent setOtherMapElement(CanonicalType value) { 4146 this.otherMap = value; 4147 return this; 4148 } 4149 4150 /** 4151 * @return The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept. 4152 */ 4153 public String getOtherMap() { 4154 return this.otherMap == null ? null : this.otherMap.getValue(); 4155 } 4156 4157 /** 4158 * @param value The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept. 4159 */ 4160 public ConceptMapGroupUnmappedComponent setOtherMap(String value) { 4161 if (Utilities.noString(value)) 4162 this.otherMap = null; 4163 else { 4164 if (this.otherMap == null) 4165 this.otherMap = new CanonicalType(); 4166 this.otherMap.setValue(value); 4167 } 4168 return this; 4169 } 4170 4171 protected void listChildren(List<Property> children) { 4172 super.listChildren(children); 4173 children.add(new Property("mode", "code", "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 0, 1, mode)); 4174 children.add(new Property("code", "code", "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 1, code)); 4175 children.add(new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display)); 4176 children.add(new Property("valueSet", "canonical(ValueSet)", "The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes.", 0, 1, valueSet)); 4177 children.add(new Property("relationship", "code", "The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'.", 0, 1, relationship)); 4178 children.add(new Property("otherMap", "canonical(ConceptMap)", "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.", 0, 1, otherMap)); 4179 } 4180 4181 @Override 4182 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4183 switch (_hash) { 4184 case 3357091: /*mode*/ return new Property("mode", "code", "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped source code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 0, 1, mode); 4185 case 3059181: /*code*/ return new Property("code", "code", "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 1, code); 4186 case 1671764162: /*display*/ return new Property("display", "string", "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, display); 4187 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "The set of fixed codes to use when the mode = 'fixed' - all unmapped codes are mapped to each of the fixed codes.", 0, 1, valueSet); 4188 case -261851592: /*relationship*/ return new Property("relationship", "code", "The default relationship value to apply between the source and target concepts when the source code is unmapped and the mode is 'fixed' or 'use-source-code'.", 0, 1, relationship); 4189 case -1171155924: /*otherMap*/ return new Property("otherMap", "canonical(ConceptMap)", "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.", 0, 1, otherMap); 4190 default: return super.getNamedProperty(_hash, _name, _checkValid); 4191 } 4192 4193 } 4194 4195 @Override 4196 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4197 switch (hash) { 4198 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<ConceptMapGroupUnmappedMode> 4199 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 4200 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 4201 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 4202 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ConceptMapRelationship> 4203 case -1171155924: /*otherMap*/ return this.otherMap == null ? new Base[0] : new Base[] {this.otherMap}; // CanonicalType 4204 default: return super.getProperty(hash, name, checkValid); 4205 } 4206 4207 } 4208 4209 @Override 4210 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4211 switch (hash) { 4212 case 3357091: // mode 4213 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4214 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 4215 return value; 4216 case 3059181: // code 4217 this.code = TypeConvertor.castToCode(value); // CodeType 4218 return value; 4219 case 1671764162: // display 4220 this.display = TypeConvertor.castToString(value); // StringType 4221 return value; 4222 case -1410174671: // valueSet 4223 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4224 return value; 4225 case -261851592: // relationship 4226 value = new ConceptMapRelationshipEnumFactory().fromType(TypeConvertor.castToCode(value)); 4227 this.relationship = (Enumeration) value; // Enumeration<ConceptMapRelationship> 4228 return value; 4229 case -1171155924: // otherMap 4230 this.otherMap = TypeConvertor.castToCanonical(value); // CanonicalType 4231 return value; 4232 default: return super.setProperty(hash, name, value); 4233 } 4234 4235 } 4236 4237 @Override 4238 public Base setProperty(String name, Base value) throws FHIRException { 4239 if (name.equals("mode")) { 4240 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4241 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 4242 } else if (name.equals("code")) { 4243 this.code = TypeConvertor.castToCode(value); // CodeType 4244 } else if (name.equals("display")) { 4245 this.display = TypeConvertor.castToString(value); // StringType 4246 } else if (name.equals("valueSet")) { 4247 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4248 } else if (name.equals("relationship")) { 4249 value = new ConceptMapRelationshipEnumFactory().fromType(TypeConvertor.castToCode(value)); 4250 this.relationship = (Enumeration) value; // Enumeration<ConceptMapRelationship> 4251 } else if (name.equals("otherMap")) { 4252 this.otherMap = TypeConvertor.castToCanonical(value); // CanonicalType 4253 } else 4254 return super.setProperty(name, value); 4255 return value; 4256 } 4257 4258 @Override 4259 public void removeChild(String name, Base value) throws FHIRException { 4260 if (name.equals("mode")) { 4261 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4262 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 4263 } else if (name.equals("code")) { 4264 this.code = null; 4265 } else if (name.equals("display")) { 4266 this.display = null; 4267 } else if (name.equals("valueSet")) { 4268 this.valueSet = null; 4269 } else if (name.equals("relationship")) { 4270 value = new ConceptMapRelationshipEnumFactory().fromType(TypeConvertor.castToCode(value)); 4271 this.relationship = (Enumeration) value; // Enumeration<ConceptMapRelationship> 4272 } else if (name.equals("otherMap")) { 4273 this.otherMap = null; 4274 } else 4275 super.removeChild(name, value); 4276 4277 } 4278 4279 @Override 4280 public Base makeProperty(int hash, String name) throws FHIRException { 4281 switch (hash) { 4282 case 3357091: return getModeElement(); 4283 case 3059181: return getCodeElement(); 4284 case 1671764162: return getDisplayElement(); 4285 case -1410174671: return getValueSetElement(); 4286 case -261851592: return getRelationshipElement(); 4287 case -1171155924: return getOtherMapElement(); 4288 default: return super.makeProperty(hash, name); 4289 } 4290 4291 } 4292 4293 @Override 4294 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4295 switch (hash) { 4296 case 3357091: /*mode*/ return new String[] {"code"}; 4297 case 3059181: /*code*/ return new String[] {"code"}; 4298 case 1671764162: /*display*/ return new String[] {"string"}; 4299 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 4300 case -261851592: /*relationship*/ return new String[] {"code"}; 4301 case -1171155924: /*otherMap*/ return new String[] {"canonical"}; 4302 default: return super.getTypesForProperty(hash, name); 4303 } 4304 4305 } 4306 4307 @Override 4308 public Base addChild(String name) throws FHIRException { 4309 if (name.equals("mode")) { 4310 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.unmapped.mode"); 4311 } 4312 else if (name.equals("code")) { 4313 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.unmapped.code"); 4314 } 4315 else if (name.equals("display")) { 4316 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.unmapped.display"); 4317 } 4318 else if (name.equals("valueSet")) { 4319 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.unmapped.valueSet"); 4320 } 4321 else if (name.equals("relationship")) { 4322 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.unmapped.relationship"); 4323 } 4324 else if (name.equals("otherMap")) { 4325 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.group.unmapped.otherMap"); 4326 } 4327 else 4328 return super.addChild(name); 4329 } 4330 4331 public ConceptMapGroupUnmappedComponent copy() { 4332 ConceptMapGroupUnmappedComponent dst = new ConceptMapGroupUnmappedComponent(); 4333 copyValues(dst); 4334 return dst; 4335 } 4336 4337 public void copyValues(ConceptMapGroupUnmappedComponent dst) { 4338 super.copyValues(dst); 4339 dst.mode = mode == null ? null : mode.copy(); 4340 dst.code = code == null ? null : code.copy(); 4341 dst.display = display == null ? null : display.copy(); 4342 dst.valueSet = valueSet == null ? null : valueSet.copy(); 4343 dst.relationship = relationship == null ? null : relationship.copy(); 4344 dst.otherMap = otherMap == null ? null : otherMap.copy(); 4345 } 4346 4347 @Override 4348 public boolean equalsDeep(Base other_) { 4349 if (!super.equalsDeep(other_)) 4350 return false; 4351 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 4352 return false; 4353 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 4354 return compareDeep(mode, o.mode, true) && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 4355 && compareDeep(valueSet, o.valueSet, true) && compareDeep(relationship, o.relationship, true) && compareDeep(otherMap, o.otherMap, true) 4356 ; 4357 } 4358 4359 @Override 4360 public boolean equalsShallow(Base other_) { 4361 if (!super.equalsShallow(other_)) 4362 return false; 4363 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 4364 return false; 4365 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 4366 return compareValues(mode, o.mode, true) && compareValues(code, o.code, true) && compareValues(display, o.display, true) 4367 && compareValues(valueSet, o.valueSet, true) && compareValues(relationship, o.relationship, true) && compareValues(otherMap, o.otherMap, true) 4368 ; 4369 } 4370 4371 public boolean isEmpty() { 4372 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, code, display, valueSet 4373 , relationship, otherMap); 4374 } 4375 4376 public String fhirType() { 4377 return "ConceptMap.group.unmapped"; 4378 4379 } 4380 4381 } 4382 4383 /** 4384 * An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers. 4385 */ 4386 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 4387 @Description(shortDefinition="Canonical identifier for this concept map, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers." ) 4388 protected UriType url; 4389 4390 /** 4391 * A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance. 4392 */ 4393 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4394 @Description(shortDefinition="Additional identifier for the concept map", formalDefinition="A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 4395 protected List<Identifier> identifier; 4396 4397 /** 4398 * The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4399 */ 4400 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4401 @Description(shortDefinition="Business version of the concept map", formalDefinition="The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 4402 protected StringType version; 4403 4404 /** 4405 * Indicates the mechanism used to compare versions to determine which ConceptMap is more current. 4406 */ 4407 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 4408 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which ConceptMap is more current." ) 4409 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 4410 protected DataType versionAlgorithm; 4411 4412 /** 4413 * A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4414 */ 4415 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 4416 @Description(shortDefinition="Name for this concept map (computer friendly)", formalDefinition="A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 4417 protected StringType name; 4418 4419 /** 4420 * A short, descriptive, user-friendly title for the concept map. 4421 */ 4422 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 4423 @Description(shortDefinition="Name for this concept map (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the concept map." ) 4424 protected StringType title; 4425 4426 /** 4427 * The status of this concept map. Enables tracking the life-cycle of the content. 4428 */ 4429 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 4430 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this concept map. Enables tracking the life-cycle of the content." ) 4431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 4432 protected Enumeration<PublicationStatus> status; 4433 4434 /** 4435 * A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 4436 */ 4437 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 4438 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 4439 protected BooleanType experimental; 4440 4441 /** 4442 * The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes. 4443 */ 4444 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 4445 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes." ) 4446 protected DateTimeType date; 4447 4448 /** 4449 * The name of the organization or individual responsible for the release and ongoing maintenance of the concept map. 4450 */ 4451 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 4452 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the concept map." ) 4453 protected StringType publisher; 4454 4455 /** 4456 * Contact details to assist a user in finding and communicating with the publisher. 4457 */ 4458 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4459 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 4460 protected List<ContactDetail> contact; 4461 4462 /** 4463 * A free text natural language description of the concept map from a consumer's perspective. 4464 */ 4465 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 4466 @Description(shortDefinition="Natural language description of the concept map", formalDefinition="A free text natural language description of the concept map from a consumer's perspective." ) 4467 protected MarkdownType description; 4468 4469 /** 4470 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances. 4471 */ 4472 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4473 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances." ) 4474 protected List<UsageContext> useContext; 4475 4476 /** 4477 * A legal or geographic region in which the concept map is intended to be used. 4478 */ 4479 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4480 @Description(shortDefinition="Intended jurisdiction for concept map (if applicable)", formalDefinition="A legal or geographic region in which the concept map is intended to be used." ) 4481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 4482 protected List<CodeableConcept> jurisdiction; 4483 4484 /** 4485 * Explanation of why this concept map is needed and why it has been designed as it has. 4486 */ 4487 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 4488 @Description(shortDefinition="Why this concept map is defined", formalDefinition="Explanation of why this concept map is needed and why it has been designed as it has." ) 4489 protected MarkdownType purpose; 4490 4491 /** 4492 * A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map. 4493 */ 4494 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 4495 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map." ) 4496 protected MarkdownType copyright; 4497 4498 /** 4499 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4500 */ 4501 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 4502 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 4503 protected StringType copyrightLabel; 4504 4505 /** 4506 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4507 */ 4508 @Child(name = "approvalDate", type = {DateType.class}, order=17, min=0, max=1, modifier=false, summary=false) 4509 @Description(shortDefinition="When the ConceptMap was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 4510 protected DateType approvalDate; 4511 4512 /** 4513 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4514 */ 4515 @Child(name = "lastReviewDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 4516 @Description(shortDefinition="When the ConceptMap was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 4517 protected DateType lastReviewDate; 4518 4519 /** 4520 * The period during which the ConceptMap content was or is planned to be in active use. 4521 */ 4522 @Child(name = "effectivePeriod", type = {Period.class}, order=19, min=0, max=1, modifier=false, summary=true) 4523 @Description(shortDefinition="When the ConceptMap is expected to be used", formalDefinition="The period during which the ConceptMap content was or is planned to be in active use." ) 4524 protected Period effectivePeriod; 4525 4526 /** 4527 * Descriptions related to the content of the ConceptMap. Topics provide a high-level categorization as well as keywords for the ConceptMap that can be useful for filtering and searching. 4528 */ 4529 @Child(name = "topic", type = {CodeableConcept.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4530 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptions related to the content of the ConceptMap. Topics provide a high-level categorization as well as keywords for the ConceptMap that can be useful for filtering and searching." ) 4531 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 4532 protected List<CodeableConcept> topic; 4533 4534 /** 4535 * An individiual or organization primarily involved in the creation and maintenance of the ConceptMap. 4536 */ 4537 @Child(name = "author", type = {ContactDetail.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4538 @Description(shortDefinition="Who authored the ConceptMap", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the ConceptMap." ) 4539 protected List<ContactDetail> author; 4540 4541 /** 4542 * An individual or organization primarily responsible for internal coherence of the ConceptMap. 4543 */ 4544 @Child(name = "editor", type = {ContactDetail.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4545 @Description(shortDefinition="Who edited the ConceptMap", formalDefinition="An individual or organization primarily responsible for internal coherence of the ConceptMap." ) 4546 protected List<ContactDetail> editor; 4547 4548 /** 4549 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ConceptMap. 4550 */ 4551 @Child(name = "reviewer", type = {ContactDetail.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4552 @Description(shortDefinition="Who reviewed the ConceptMap", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ConceptMap." ) 4553 protected List<ContactDetail> reviewer; 4554 4555 /** 4556 * An individual or organization asserted by the publisher to be responsible for officially endorsing the ConceptMap for use in some setting. 4557 */ 4558 @Child(name = "endorser", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4559 @Description(shortDefinition="Who endorsed the ConceptMap", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the ConceptMap for use in some setting." ) 4560 protected List<ContactDetail> endorser; 4561 4562 /** 4563 * Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts. 4564 */ 4565 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4566 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts." ) 4567 protected List<RelatedArtifact> relatedArtifact; 4568 4569 /** 4570 * A property defines a slot through which additional information can be provided about a map from source -> target. 4571 */ 4572 @Child(name = "property", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4573 @Description(shortDefinition="Additional properties of the mapping", formalDefinition="A property defines a slot through which additional information can be provided about a map from source -> target." ) 4574 protected List<PropertyComponent> property; 4575 4576 /** 4577 * An additionalAttribute defines an additional data element found in the source or target data model where the data will come from or be mapped to. Some mappings are based on data in addition to the source data element, where codes in multiple fields are combined to a single field (or vice versa). 4578 */ 4579 @Child(name = "additionalAttribute", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4580 @Description(shortDefinition="Definition of an additional attribute to act as a data source or target", formalDefinition="An additionalAttribute defines an additional data element found in the source or target data model where the data will come from or be mapped to. Some mappings are based on data in addition to the source data element, where codes in multiple fields are combined to a single field (or vice versa)." ) 4581 protected List<AdditionalAttributeComponent> additionalAttribute; 4582 4583 /** 4584 * Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set. 4585 */ 4586 @Child(name = "sourceScope", type = {UriType.class, CanonicalType.class}, order=28, min=0, max=1, modifier=false, summary=true) 4587 @Description(shortDefinition="The source value set that contains the concepts that are being mapped", formalDefinition="Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set." ) 4588 protected DataType sourceScope; 4589 4590 /** 4591 * Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set. 4592 */ 4593 @Child(name = "targetScope", type = {UriType.class, CanonicalType.class}, order=29, min=0, max=1, modifier=false, summary=true) 4594 @Description(shortDefinition="The target value set which provides context for the mappings", formalDefinition="Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set." ) 4595 protected DataType targetScope; 4596 4597 /** 4598 * A group of mappings that all have the same source and target system. 4599 */ 4600 @Child(name = "group", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4601 @Description(shortDefinition="Same source and target systems", formalDefinition="A group of mappings that all have the same source and target system." ) 4602 protected List<ConceptMapGroupComponent> group; 4603 4604 private static final long serialVersionUID = 1990005194L; 4605 4606 /** 4607 * Constructor 4608 */ 4609 public ConceptMap() { 4610 super(); 4611 } 4612 4613 /** 4614 * Constructor 4615 */ 4616 public ConceptMap(PublicationStatus status) { 4617 super(); 4618 this.setStatus(status); 4619 } 4620 4621 /** 4622 * @return {@link #url} (An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 4623 */ 4624 public UriType getUrlElement() { 4625 if (this.url == null) 4626 if (Configuration.errorOnAutoCreate()) 4627 throw new Error("Attempt to auto-create ConceptMap.url"); 4628 else if (Configuration.doAutoCreate()) 4629 this.url = new UriType(); // bb 4630 return this.url; 4631 } 4632 4633 public boolean hasUrlElement() { 4634 return this.url != null && !this.url.isEmpty(); 4635 } 4636 4637 public boolean hasUrl() { 4638 return this.url != null && !this.url.isEmpty(); 4639 } 4640 4641 /** 4642 * @param value {@link #url} (An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 4643 */ 4644 public ConceptMap setUrlElement(UriType value) { 4645 this.url = value; 4646 return this; 4647 } 4648 4649 /** 4650 * @return An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers. 4651 */ 4652 public String getUrl() { 4653 return this.url == null ? null : this.url.getValue(); 4654 } 4655 4656 /** 4657 * @param value An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers. 4658 */ 4659 public ConceptMap setUrl(String value) { 4660 if (Utilities.noString(value)) 4661 this.url = null; 4662 else { 4663 if (this.url == null) 4664 this.url = new UriType(); 4665 this.url.setValue(value); 4666 } 4667 return this; 4668 } 4669 4670 /** 4671 * @return {@link #identifier} (A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.) 4672 */ 4673 public List<Identifier> getIdentifier() { 4674 if (this.identifier == null) 4675 this.identifier = new ArrayList<Identifier>(); 4676 return this.identifier; 4677 } 4678 4679 /** 4680 * @return Returns a reference to <code>this</code> for easy method chaining 4681 */ 4682 public ConceptMap setIdentifier(List<Identifier> theIdentifier) { 4683 this.identifier = theIdentifier; 4684 return this; 4685 } 4686 4687 public boolean hasIdentifier() { 4688 if (this.identifier == null) 4689 return false; 4690 for (Identifier item : this.identifier) 4691 if (!item.isEmpty()) 4692 return true; 4693 return false; 4694 } 4695 4696 public Identifier addIdentifier() { //3 4697 Identifier t = new Identifier(); 4698 if (this.identifier == null) 4699 this.identifier = new ArrayList<Identifier>(); 4700 this.identifier.add(t); 4701 return t; 4702 } 4703 4704 public ConceptMap addIdentifier(Identifier t) { //3 4705 if (t == null) 4706 return this; 4707 if (this.identifier == null) 4708 this.identifier = new ArrayList<Identifier>(); 4709 this.identifier.add(t); 4710 return this; 4711 } 4712 4713 /** 4714 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 4715 */ 4716 public Identifier getIdentifierFirstRep() { 4717 if (getIdentifier().isEmpty()) { 4718 addIdentifier(); 4719 } 4720 return getIdentifier().get(0); 4721 } 4722 4723 /** 4724 * @return {@link #version} (The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 4725 */ 4726 public StringType getVersionElement() { 4727 if (this.version == null) 4728 if (Configuration.errorOnAutoCreate()) 4729 throw new Error("Attempt to auto-create ConceptMap.version"); 4730 else if (Configuration.doAutoCreate()) 4731 this.version = new StringType(); // bb 4732 return this.version; 4733 } 4734 4735 public boolean hasVersionElement() { 4736 return this.version != null && !this.version.isEmpty(); 4737 } 4738 4739 public boolean hasVersion() { 4740 return this.version != null && !this.version.isEmpty(); 4741 } 4742 4743 /** 4744 * @param value {@link #version} (The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 4745 */ 4746 public ConceptMap setVersionElement(StringType value) { 4747 this.version = value; 4748 return this; 4749 } 4750 4751 /** 4752 * @return The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4753 */ 4754 public String getVersion() { 4755 return this.version == null ? null : this.version.getValue(); 4756 } 4757 4758 /** 4759 * @param value The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4760 */ 4761 public ConceptMap setVersion(String value) { 4762 if (Utilities.noString(value)) 4763 this.version = null; 4764 else { 4765 if (this.version == null) 4766 this.version = new StringType(); 4767 this.version.setValue(value); 4768 } 4769 return this; 4770 } 4771 4772 /** 4773 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ConceptMap is more current.) 4774 */ 4775 public DataType getVersionAlgorithm() { 4776 return this.versionAlgorithm; 4777 } 4778 4779 /** 4780 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ConceptMap is more current.) 4781 */ 4782 public StringType getVersionAlgorithmStringType() throws FHIRException { 4783 if (this.versionAlgorithm == null) 4784 this.versionAlgorithm = new StringType(); 4785 if (!(this.versionAlgorithm instanceof StringType)) 4786 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4787 return (StringType) this.versionAlgorithm; 4788 } 4789 4790 public boolean hasVersionAlgorithmStringType() { 4791 return this != null && this.versionAlgorithm instanceof StringType; 4792 } 4793 4794 /** 4795 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ConceptMap is more current.) 4796 */ 4797 public Coding getVersionAlgorithmCoding() throws FHIRException { 4798 if (this.versionAlgorithm == null) 4799 this.versionAlgorithm = new Coding(); 4800 if (!(this.versionAlgorithm instanceof Coding)) 4801 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4802 return (Coding) this.versionAlgorithm; 4803 } 4804 4805 public boolean hasVersionAlgorithmCoding() { 4806 return this != null && this.versionAlgorithm instanceof Coding; 4807 } 4808 4809 public boolean hasVersionAlgorithm() { 4810 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 4811 } 4812 4813 /** 4814 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which ConceptMap is more current.) 4815 */ 4816 public ConceptMap setVersionAlgorithm(DataType value) { 4817 if (value != null && !(value instanceof StringType || value instanceof Coding)) 4818 throw new FHIRException("Not the right type for ConceptMap.versionAlgorithm[x]: "+value.fhirType()); 4819 this.versionAlgorithm = value; 4820 return this; 4821 } 4822 4823 /** 4824 * @return {@link #name} (A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4825 */ 4826 public StringType getNameElement() { 4827 if (this.name == null) 4828 if (Configuration.errorOnAutoCreate()) 4829 throw new Error("Attempt to auto-create ConceptMap.name"); 4830 else if (Configuration.doAutoCreate()) 4831 this.name = new StringType(); // bb 4832 return this.name; 4833 } 4834 4835 public boolean hasNameElement() { 4836 return this.name != null && !this.name.isEmpty(); 4837 } 4838 4839 public boolean hasName() { 4840 return this.name != null && !this.name.isEmpty(); 4841 } 4842 4843 /** 4844 * @param value {@link #name} (A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4845 */ 4846 public ConceptMap setNameElement(StringType value) { 4847 this.name = value; 4848 return this; 4849 } 4850 4851 /** 4852 * @return A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4853 */ 4854 public String getName() { 4855 return this.name == null ? null : this.name.getValue(); 4856 } 4857 4858 /** 4859 * @param value A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4860 */ 4861 public ConceptMap setName(String value) { 4862 if (Utilities.noString(value)) 4863 this.name = null; 4864 else { 4865 if (this.name == null) 4866 this.name = new StringType(); 4867 this.name.setValue(value); 4868 } 4869 return this; 4870 } 4871 4872 /** 4873 * @return {@link #title} (A short, descriptive, user-friendly title for the concept map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4874 */ 4875 public StringType getTitleElement() { 4876 if (this.title == null) 4877 if (Configuration.errorOnAutoCreate()) 4878 throw new Error("Attempt to auto-create ConceptMap.title"); 4879 else if (Configuration.doAutoCreate()) 4880 this.title = new StringType(); // bb 4881 return this.title; 4882 } 4883 4884 public boolean hasTitleElement() { 4885 return this.title != null && !this.title.isEmpty(); 4886 } 4887 4888 public boolean hasTitle() { 4889 return this.title != null && !this.title.isEmpty(); 4890 } 4891 4892 /** 4893 * @param value {@link #title} (A short, descriptive, user-friendly title for the concept map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4894 */ 4895 public ConceptMap setTitleElement(StringType value) { 4896 this.title = value; 4897 return this; 4898 } 4899 4900 /** 4901 * @return A short, descriptive, user-friendly title for the concept map. 4902 */ 4903 public String getTitle() { 4904 return this.title == null ? null : this.title.getValue(); 4905 } 4906 4907 /** 4908 * @param value A short, descriptive, user-friendly title for the concept map. 4909 */ 4910 public ConceptMap setTitle(String value) { 4911 if (Utilities.noString(value)) 4912 this.title = null; 4913 else { 4914 if (this.title == null) 4915 this.title = new StringType(); 4916 this.title.setValue(value); 4917 } 4918 return this; 4919 } 4920 4921 /** 4922 * @return {@link #status} (The status of this concept map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4923 */ 4924 public Enumeration<PublicationStatus> getStatusElement() { 4925 if (this.status == null) 4926 if (Configuration.errorOnAutoCreate()) 4927 throw new Error("Attempt to auto-create ConceptMap.status"); 4928 else if (Configuration.doAutoCreate()) 4929 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 4930 return this.status; 4931 } 4932 4933 public boolean hasStatusElement() { 4934 return this.status != null && !this.status.isEmpty(); 4935 } 4936 4937 public boolean hasStatus() { 4938 return this.status != null && !this.status.isEmpty(); 4939 } 4940 4941 /** 4942 * @param value {@link #status} (The status of this concept map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4943 */ 4944 public ConceptMap setStatusElement(Enumeration<PublicationStatus> value) { 4945 this.status = value; 4946 return this; 4947 } 4948 4949 /** 4950 * @return The status of this concept map. Enables tracking the life-cycle of the content. 4951 */ 4952 public PublicationStatus getStatus() { 4953 return this.status == null ? null : this.status.getValue(); 4954 } 4955 4956 /** 4957 * @param value The status of this concept map. Enables tracking the life-cycle of the content. 4958 */ 4959 public ConceptMap setStatus(PublicationStatus value) { 4960 if (this.status == null) 4961 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4962 this.status.setValue(value); 4963 return this; 4964 } 4965 4966 /** 4967 * @return {@link #experimental} (A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4968 */ 4969 public BooleanType getExperimentalElement() { 4970 if (this.experimental == null) 4971 if (Configuration.errorOnAutoCreate()) 4972 throw new Error("Attempt to auto-create ConceptMap.experimental"); 4973 else if (Configuration.doAutoCreate()) 4974 this.experimental = new BooleanType(); // bb 4975 return this.experimental; 4976 } 4977 4978 public boolean hasExperimentalElement() { 4979 return this.experimental != null && !this.experimental.isEmpty(); 4980 } 4981 4982 public boolean hasExperimental() { 4983 return this.experimental != null && !this.experimental.isEmpty(); 4984 } 4985 4986 /** 4987 * @param value {@link #experimental} (A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4988 */ 4989 public ConceptMap setExperimentalElement(BooleanType value) { 4990 this.experimental = value; 4991 return this; 4992 } 4993 4994 /** 4995 * @return A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 4996 */ 4997 public boolean getExperimental() { 4998 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4999 } 5000 5001 /** 5002 * @param value A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5003 */ 5004 public ConceptMap setExperimental(boolean value) { 5005 if (this.experimental == null) 5006 this.experimental = new BooleanType(); 5007 this.experimental.setValue(value); 5008 return this; 5009 } 5010 5011 /** 5012 * @return {@link #date} (The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5013 */ 5014 public DateTimeType getDateElement() { 5015 if (this.date == null) 5016 if (Configuration.errorOnAutoCreate()) 5017 throw new Error("Attempt to auto-create ConceptMap.date"); 5018 else if (Configuration.doAutoCreate()) 5019 this.date = new DateTimeType(); // bb 5020 return this.date; 5021 } 5022 5023 public boolean hasDateElement() { 5024 return this.date != null && !this.date.isEmpty(); 5025 } 5026 5027 public boolean hasDate() { 5028 return this.date != null && !this.date.isEmpty(); 5029 } 5030 5031 /** 5032 * @param value {@link #date} (The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5033 */ 5034 public ConceptMap setDateElement(DateTimeType value) { 5035 this.date = value; 5036 return this; 5037 } 5038 5039 /** 5040 * @return The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes. 5041 */ 5042 public Date getDate() { 5043 return this.date == null ? null : this.date.getValue(); 5044 } 5045 5046 /** 5047 * @param value The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes. 5048 */ 5049 public ConceptMap setDate(Date value) { 5050 if (value == null) 5051 this.date = null; 5052 else { 5053 if (this.date == null) 5054 this.date = new DateTimeType(); 5055 this.date.setValue(value); 5056 } 5057 return this; 5058 } 5059 5060 /** 5061 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the concept map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 5062 */ 5063 public StringType getPublisherElement() { 5064 if (this.publisher == null) 5065 if (Configuration.errorOnAutoCreate()) 5066 throw new Error("Attempt to auto-create ConceptMap.publisher"); 5067 else if (Configuration.doAutoCreate()) 5068 this.publisher = new StringType(); // bb 5069 return this.publisher; 5070 } 5071 5072 public boolean hasPublisherElement() { 5073 return this.publisher != null && !this.publisher.isEmpty(); 5074 } 5075 5076 public boolean hasPublisher() { 5077 return this.publisher != null && !this.publisher.isEmpty(); 5078 } 5079 5080 /** 5081 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the concept map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 5082 */ 5083 public ConceptMap setPublisherElement(StringType value) { 5084 this.publisher = value; 5085 return this; 5086 } 5087 5088 /** 5089 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the concept map. 5090 */ 5091 public String getPublisher() { 5092 return this.publisher == null ? null : this.publisher.getValue(); 5093 } 5094 5095 /** 5096 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the concept map. 5097 */ 5098 public ConceptMap setPublisher(String value) { 5099 if (Utilities.noString(value)) 5100 this.publisher = null; 5101 else { 5102 if (this.publisher == null) 5103 this.publisher = new StringType(); 5104 this.publisher.setValue(value); 5105 } 5106 return this; 5107 } 5108 5109 /** 5110 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 5111 */ 5112 public List<ContactDetail> getContact() { 5113 if (this.contact == null) 5114 this.contact = new ArrayList<ContactDetail>(); 5115 return this.contact; 5116 } 5117 5118 /** 5119 * @return Returns a reference to <code>this</code> for easy method chaining 5120 */ 5121 public ConceptMap setContact(List<ContactDetail> theContact) { 5122 this.contact = theContact; 5123 return this; 5124 } 5125 5126 public boolean hasContact() { 5127 if (this.contact == null) 5128 return false; 5129 for (ContactDetail item : this.contact) 5130 if (!item.isEmpty()) 5131 return true; 5132 return false; 5133 } 5134 5135 public ContactDetail addContact() { //3 5136 ContactDetail t = new ContactDetail(); 5137 if (this.contact == null) 5138 this.contact = new ArrayList<ContactDetail>(); 5139 this.contact.add(t); 5140 return t; 5141 } 5142 5143 public ConceptMap addContact(ContactDetail t) { //3 5144 if (t == null) 5145 return this; 5146 if (this.contact == null) 5147 this.contact = new ArrayList<ContactDetail>(); 5148 this.contact.add(t); 5149 return this; 5150 } 5151 5152 /** 5153 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 5154 */ 5155 public ContactDetail getContactFirstRep() { 5156 if (getContact().isEmpty()) { 5157 addContact(); 5158 } 5159 return getContact().get(0); 5160 } 5161 5162 /** 5163 * @return {@link #description} (A free text natural language description of the concept map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5164 */ 5165 public MarkdownType getDescriptionElement() { 5166 if (this.description == null) 5167 if (Configuration.errorOnAutoCreate()) 5168 throw new Error("Attempt to auto-create ConceptMap.description"); 5169 else if (Configuration.doAutoCreate()) 5170 this.description = new MarkdownType(); // bb 5171 return this.description; 5172 } 5173 5174 public boolean hasDescriptionElement() { 5175 return this.description != null && !this.description.isEmpty(); 5176 } 5177 5178 public boolean hasDescription() { 5179 return this.description != null && !this.description.isEmpty(); 5180 } 5181 5182 /** 5183 * @param value {@link #description} (A free text natural language description of the concept map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5184 */ 5185 public ConceptMap setDescriptionElement(MarkdownType value) { 5186 this.description = value; 5187 return this; 5188 } 5189 5190 /** 5191 * @return A free text natural language description of the concept map from a consumer's perspective. 5192 */ 5193 public String getDescription() { 5194 return this.description == null ? null : this.description.getValue(); 5195 } 5196 5197 /** 5198 * @param value A free text natural language description of the concept map from a consumer's perspective. 5199 */ 5200 public ConceptMap setDescription(String value) { 5201 if (Utilities.noString(value)) 5202 this.description = null; 5203 else { 5204 if (this.description == null) 5205 this.description = new MarkdownType(); 5206 this.description.setValue(value); 5207 } 5208 return this; 5209 } 5210 5211 /** 5212 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.) 5213 */ 5214 public List<UsageContext> getUseContext() { 5215 if (this.useContext == null) 5216 this.useContext = new ArrayList<UsageContext>(); 5217 return this.useContext; 5218 } 5219 5220 /** 5221 * @return Returns a reference to <code>this</code> for easy method chaining 5222 */ 5223 public ConceptMap setUseContext(List<UsageContext> theUseContext) { 5224 this.useContext = theUseContext; 5225 return this; 5226 } 5227 5228 public boolean hasUseContext() { 5229 if (this.useContext == null) 5230 return false; 5231 for (UsageContext item : this.useContext) 5232 if (!item.isEmpty()) 5233 return true; 5234 return false; 5235 } 5236 5237 public UsageContext addUseContext() { //3 5238 UsageContext t = new UsageContext(); 5239 if (this.useContext == null) 5240 this.useContext = new ArrayList<UsageContext>(); 5241 this.useContext.add(t); 5242 return t; 5243 } 5244 5245 public ConceptMap addUseContext(UsageContext t) { //3 5246 if (t == null) 5247 return this; 5248 if (this.useContext == null) 5249 this.useContext = new ArrayList<UsageContext>(); 5250 this.useContext.add(t); 5251 return this; 5252 } 5253 5254 /** 5255 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 5256 */ 5257 public UsageContext getUseContextFirstRep() { 5258 if (getUseContext().isEmpty()) { 5259 addUseContext(); 5260 } 5261 return getUseContext().get(0); 5262 } 5263 5264 /** 5265 * @return {@link #jurisdiction} (A legal or geographic region in which the concept map is intended to be used.) 5266 */ 5267 public List<CodeableConcept> getJurisdiction() { 5268 if (this.jurisdiction == null) 5269 this.jurisdiction = new ArrayList<CodeableConcept>(); 5270 return this.jurisdiction; 5271 } 5272 5273 /** 5274 * @return Returns a reference to <code>this</code> for easy method chaining 5275 */ 5276 public ConceptMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 5277 this.jurisdiction = theJurisdiction; 5278 return this; 5279 } 5280 5281 public boolean hasJurisdiction() { 5282 if (this.jurisdiction == null) 5283 return false; 5284 for (CodeableConcept item : this.jurisdiction) 5285 if (!item.isEmpty()) 5286 return true; 5287 return false; 5288 } 5289 5290 public CodeableConcept addJurisdiction() { //3 5291 CodeableConcept t = new CodeableConcept(); 5292 if (this.jurisdiction == null) 5293 this.jurisdiction = new ArrayList<CodeableConcept>(); 5294 this.jurisdiction.add(t); 5295 return t; 5296 } 5297 5298 public ConceptMap addJurisdiction(CodeableConcept t) { //3 5299 if (t == null) 5300 return this; 5301 if (this.jurisdiction == null) 5302 this.jurisdiction = new ArrayList<CodeableConcept>(); 5303 this.jurisdiction.add(t); 5304 return this; 5305 } 5306 5307 /** 5308 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 5309 */ 5310 public CodeableConcept getJurisdictionFirstRep() { 5311 if (getJurisdiction().isEmpty()) { 5312 addJurisdiction(); 5313 } 5314 return getJurisdiction().get(0); 5315 } 5316 5317 /** 5318 * @return {@link #purpose} (Explanation of why this concept map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5319 */ 5320 public MarkdownType getPurposeElement() { 5321 if (this.purpose == null) 5322 if (Configuration.errorOnAutoCreate()) 5323 throw new Error("Attempt to auto-create ConceptMap.purpose"); 5324 else if (Configuration.doAutoCreate()) 5325 this.purpose = new MarkdownType(); // bb 5326 return this.purpose; 5327 } 5328 5329 public boolean hasPurposeElement() { 5330 return this.purpose != null && !this.purpose.isEmpty(); 5331 } 5332 5333 public boolean hasPurpose() { 5334 return this.purpose != null && !this.purpose.isEmpty(); 5335 } 5336 5337 /** 5338 * @param value {@link #purpose} (Explanation of why this concept map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5339 */ 5340 public ConceptMap setPurposeElement(MarkdownType value) { 5341 this.purpose = value; 5342 return this; 5343 } 5344 5345 /** 5346 * @return Explanation of why this concept map is needed and why it has been designed as it has. 5347 */ 5348 public String getPurpose() { 5349 return this.purpose == null ? null : this.purpose.getValue(); 5350 } 5351 5352 /** 5353 * @param value Explanation of why this concept map is needed and why it has been designed as it has. 5354 */ 5355 public ConceptMap setPurpose(String value) { 5356 if (Utilities.noString(value)) 5357 this.purpose = null; 5358 else { 5359 if (this.purpose == null) 5360 this.purpose = new MarkdownType(); 5361 this.purpose.setValue(value); 5362 } 5363 return this; 5364 } 5365 5366 /** 5367 * @return {@link #copyright} (A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5368 */ 5369 public MarkdownType getCopyrightElement() { 5370 if (this.copyright == null) 5371 if (Configuration.errorOnAutoCreate()) 5372 throw new Error("Attempt to auto-create ConceptMap.copyright"); 5373 else if (Configuration.doAutoCreate()) 5374 this.copyright = new MarkdownType(); // bb 5375 return this.copyright; 5376 } 5377 5378 public boolean hasCopyrightElement() { 5379 return this.copyright != null && !this.copyright.isEmpty(); 5380 } 5381 5382 public boolean hasCopyright() { 5383 return this.copyright != null && !this.copyright.isEmpty(); 5384 } 5385 5386 /** 5387 * @param value {@link #copyright} (A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5388 */ 5389 public ConceptMap setCopyrightElement(MarkdownType value) { 5390 this.copyright = value; 5391 return this; 5392 } 5393 5394 /** 5395 * @return A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map. 5396 */ 5397 public String getCopyright() { 5398 return this.copyright == null ? null : this.copyright.getValue(); 5399 } 5400 5401 /** 5402 * @param value A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map. 5403 */ 5404 public ConceptMap setCopyright(String value) { 5405 if (Utilities.noString(value)) 5406 this.copyright = null; 5407 else { 5408 if (this.copyright == null) 5409 this.copyright = new MarkdownType(); 5410 this.copyright.setValue(value); 5411 } 5412 return this; 5413 } 5414 5415 /** 5416 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 5417 */ 5418 public StringType getCopyrightLabelElement() { 5419 if (this.copyrightLabel == null) 5420 if (Configuration.errorOnAutoCreate()) 5421 throw new Error("Attempt to auto-create ConceptMap.copyrightLabel"); 5422 else if (Configuration.doAutoCreate()) 5423 this.copyrightLabel = new StringType(); // bb 5424 return this.copyrightLabel; 5425 } 5426 5427 public boolean hasCopyrightLabelElement() { 5428 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 5429 } 5430 5431 public boolean hasCopyrightLabel() { 5432 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 5433 } 5434 5435 /** 5436 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 5437 */ 5438 public ConceptMap setCopyrightLabelElement(StringType value) { 5439 this.copyrightLabel = value; 5440 return this; 5441 } 5442 5443 /** 5444 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5445 */ 5446 public String getCopyrightLabel() { 5447 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 5448 } 5449 5450 /** 5451 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5452 */ 5453 public ConceptMap setCopyrightLabel(String value) { 5454 if (Utilities.noString(value)) 5455 this.copyrightLabel = null; 5456 else { 5457 if (this.copyrightLabel == null) 5458 this.copyrightLabel = new StringType(); 5459 this.copyrightLabel.setValue(value); 5460 } 5461 return this; 5462 } 5463 5464 /** 5465 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 5466 */ 5467 public DateType getApprovalDateElement() { 5468 if (this.approvalDate == null) 5469 if (Configuration.errorOnAutoCreate()) 5470 throw new Error("Attempt to auto-create ConceptMap.approvalDate"); 5471 else if (Configuration.doAutoCreate()) 5472 this.approvalDate = new DateType(); // bb 5473 return this.approvalDate; 5474 } 5475 5476 public boolean hasApprovalDateElement() { 5477 return this.approvalDate != null && !this.approvalDate.isEmpty(); 5478 } 5479 5480 public boolean hasApprovalDate() { 5481 return this.approvalDate != null && !this.approvalDate.isEmpty(); 5482 } 5483 5484 /** 5485 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 5486 */ 5487 public ConceptMap setApprovalDateElement(DateType value) { 5488 this.approvalDate = value; 5489 return this; 5490 } 5491 5492 /** 5493 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 5494 */ 5495 public Date getApprovalDate() { 5496 return this.approvalDate == null ? null : this.approvalDate.getValue(); 5497 } 5498 5499 /** 5500 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 5501 */ 5502 public ConceptMap setApprovalDate(Date value) { 5503 if (value == null) 5504 this.approvalDate = null; 5505 else { 5506 if (this.approvalDate == null) 5507 this.approvalDate = new DateType(); 5508 this.approvalDate.setValue(value); 5509 } 5510 return this; 5511 } 5512 5513 /** 5514 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 5515 */ 5516 public DateType getLastReviewDateElement() { 5517 if (this.lastReviewDate == null) 5518 if (Configuration.errorOnAutoCreate()) 5519 throw new Error("Attempt to auto-create ConceptMap.lastReviewDate"); 5520 else if (Configuration.doAutoCreate()) 5521 this.lastReviewDate = new DateType(); // bb 5522 return this.lastReviewDate; 5523 } 5524 5525 public boolean hasLastReviewDateElement() { 5526 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 5527 } 5528 5529 public boolean hasLastReviewDate() { 5530 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 5531 } 5532 5533 /** 5534 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 5535 */ 5536 public ConceptMap setLastReviewDateElement(DateType value) { 5537 this.lastReviewDate = value; 5538 return this; 5539 } 5540 5541 /** 5542 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 5543 */ 5544 public Date getLastReviewDate() { 5545 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 5546 } 5547 5548 /** 5549 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 5550 */ 5551 public ConceptMap setLastReviewDate(Date value) { 5552 if (value == null) 5553 this.lastReviewDate = null; 5554 else { 5555 if (this.lastReviewDate == null) 5556 this.lastReviewDate = new DateType(); 5557 this.lastReviewDate.setValue(value); 5558 } 5559 return this; 5560 } 5561 5562 /** 5563 * @return {@link #effectivePeriod} (The period during which the ConceptMap content was or is planned to be in active use.) 5564 */ 5565 public Period getEffectivePeriod() { 5566 if (this.effectivePeriod == null) 5567 if (Configuration.errorOnAutoCreate()) 5568 throw new Error("Attempt to auto-create ConceptMap.effectivePeriod"); 5569 else if (Configuration.doAutoCreate()) 5570 this.effectivePeriod = new Period(); // cc 5571 return this.effectivePeriod; 5572 } 5573 5574 public boolean hasEffectivePeriod() { 5575 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 5576 } 5577 5578 /** 5579 * @param value {@link #effectivePeriod} (The period during which the ConceptMap content was or is planned to be in active use.) 5580 */ 5581 public ConceptMap setEffectivePeriod(Period value) { 5582 this.effectivePeriod = value; 5583 return this; 5584 } 5585 5586 /** 5587 * @return {@link #topic} (Descriptions related to the content of the ConceptMap. Topics provide a high-level categorization as well as keywords for the ConceptMap that can be useful for filtering and searching.) 5588 */ 5589 public List<CodeableConcept> getTopic() { 5590 if (this.topic == null) 5591 this.topic = new ArrayList<CodeableConcept>(); 5592 return this.topic; 5593 } 5594 5595 /** 5596 * @return Returns a reference to <code>this</code> for easy method chaining 5597 */ 5598 public ConceptMap setTopic(List<CodeableConcept> theTopic) { 5599 this.topic = theTopic; 5600 return this; 5601 } 5602 5603 public boolean hasTopic() { 5604 if (this.topic == null) 5605 return false; 5606 for (CodeableConcept item : this.topic) 5607 if (!item.isEmpty()) 5608 return true; 5609 return false; 5610 } 5611 5612 public CodeableConcept addTopic() { //3 5613 CodeableConcept t = new CodeableConcept(); 5614 if (this.topic == null) 5615 this.topic = new ArrayList<CodeableConcept>(); 5616 this.topic.add(t); 5617 return t; 5618 } 5619 5620 public ConceptMap addTopic(CodeableConcept t) { //3 5621 if (t == null) 5622 return this; 5623 if (this.topic == null) 5624 this.topic = new ArrayList<CodeableConcept>(); 5625 this.topic.add(t); 5626 return this; 5627 } 5628 5629 /** 5630 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 5631 */ 5632 public CodeableConcept getTopicFirstRep() { 5633 if (getTopic().isEmpty()) { 5634 addTopic(); 5635 } 5636 return getTopic().get(0); 5637 } 5638 5639 /** 5640 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the ConceptMap.) 5641 */ 5642 public List<ContactDetail> getAuthor() { 5643 if (this.author == null) 5644 this.author = new ArrayList<ContactDetail>(); 5645 return this.author; 5646 } 5647 5648 /** 5649 * @return Returns a reference to <code>this</code> for easy method chaining 5650 */ 5651 public ConceptMap setAuthor(List<ContactDetail> theAuthor) { 5652 this.author = theAuthor; 5653 return this; 5654 } 5655 5656 public boolean hasAuthor() { 5657 if (this.author == null) 5658 return false; 5659 for (ContactDetail item : this.author) 5660 if (!item.isEmpty()) 5661 return true; 5662 return false; 5663 } 5664 5665 public ContactDetail addAuthor() { //3 5666 ContactDetail t = new ContactDetail(); 5667 if (this.author == null) 5668 this.author = new ArrayList<ContactDetail>(); 5669 this.author.add(t); 5670 return t; 5671 } 5672 5673 public ConceptMap addAuthor(ContactDetail t) { //3 5674 if (t == null) 5675 return this; 5676 if (this.author == null) 5677 this.author = new ArrayList<ContactDetail>(); 5678 this.author.add(t); 5679 return this; 5680 } 5681 5682 /** 5683 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 5684 */ 5685 public ContactDetail getAuthorFirstRep() { 5686 if (getAuthor().isEmpty()) { 5687 addAuthor(); 5688 } 5689 return getAuthor().get(0); 5690 } 5691 5692 /** 5693 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the ConceptMap.) 5694 */ 5695 public List<ContactDetail> getEditor() { 5696 if (this.editor == null) 5697 this.editor = new ArrayList<ContactDetail>(); 5698 return this.editor; 5699 } 5700 5701 /** 5702 * @return Returns a reference to <code>this</code> for easy method chaining 5703 */ 5704 public ConceptMap setEditor(List<ContactDetail> theEditor) { 5705 this.editor = theEditor; 5706 return this; 5707 } 5708 5709 public boolean hasEditor() { 5710 if (this.editor == null) 5711 return false; 5712 for (ContactDetail item : this.editor) 5713 if (!item.isEmpty()) 5714 return true; 5715 return false; 5716 } 5717 5718 public ContactDetail addEditor() { //3 5719 ContactDetail t = new ContactDetail(); 5720 if (this.editor == null) 5721 this.editor = new ArrayList<ContactDetail>(); 5722 this.editor.add(t); 5723 return t; 5724 } 5725 5726 public ConceptMap addEditor(ContactDetail t) { //3 5727 if (t == null) 5728 return this; 5729 if (this.editor == null) 5730 this.editor = new ArrayList<ContactDetail>(); 5731 this.editor.add(t); 5732 return this; 5733 } 5734 5735 /** 5736 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 5737 */ 5738 public ContactDetail getEditorFirstRep() { 5739 if (getEditor().isEmpty()) { 5740 addEditor(); 5741 } 5742 return getEditor().get(0); 5743 } 5744 5745 /** 5746 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ConceptMap.) 5747 */ 5748 public List<ContactDetail> getReviewer() { 5749 if (this.reviewer == null) 5750 this.reviewer = new ArrayList<ContactDetail>(); 5751 return this.reviewer; 5752 } 5753 5754 /** 5755 * @return Returns a reference to <code>this</code> for easy method chaining 5756 */ 5757 public ConceptMap setReviewer(List<ContactDetail> theReviewer) { 5758 this.reviewer = theReviewer; 5759 return this; 5760 } 5761 5762 public boolean hasReviewer() { 5763 if (this.reviewer == null) 5764 return false; 5765 for (ContactDetail item : this.reviewer) 5766 if (!item.isEmpty()) 5767 return true; 5768 return false; 5769 } 5770 5771 public ContactDetail addReviewer() { //3 5772 ContactDetail t = new ContactDetail(); 5773 if (this.reviewer == null) 5774 this.reviewer = new ArrayList<ContactDetail>(); 5775 this.reviewer.add(t); 5776 return t; 5777 } 5778 5779 public ConceptMap addReviewer(ContactDetail t) { //3 5780 if (t == null) 5781 return this; 5782 if (this.reviewer == null) 5783 this.reviewer = new ArrayList<ContactDetail>(); 5784 this.reviewer.add(t); 5785 return this; 5786 } 5787 5788 /** 5789 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 5790 */ 5791 public ContactDetail getReviewerFirstRep() { 5792 if (getReviewer().isEmpty()) { 5793 addReviewer(); 5794 } 5795 return getReviewer().get(0); 5796 } 5797 5798 /** 5799 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the ConceptMap for use in some setting.) 5800 */ 5801 public List<ContactDetail> getEndorser() { 5802 if (this.endorser == null) 5803 this.endorser = new ArrayList<ContactDetail>(); 5804 return this.endorser; 5805 } 5806 5807 /** 5808 * @return Returns a reference to <code>this</code> for easy method chaining 5809 */ 5810 public ConceptMap setEndorser(List<ContactDetail> theEndorser) { 5811 this.endorser = theEndorser; 5812 return this; 5813 } 5814 5815 public boolean hasEndorser() { 5816 if (this.endorser == null) 5817 return false; 5818 for (ContactDetail item : this.endorser) 5819 if (!item.isEmpty()) 5820 return true; 5821 return false; 5822 } 5823 5824 public ContactDetail addEndorser() { //3 5825 ContactDetail t = new ContactDetail(); 5826 if (this.endorser == null) 5827 this.endorser = new ArrayList<ContactDetail>(); 5828 this.endorser.add(t); 5829 return t; 5830 } 5831 5832 public ConceptMap addEndorser(ContactDetail t) { //3 5833 if (t == null) 5834 return this; 5835 if (this.endorser == null) 5836 this.endorser = new ArrayList<ContactDetail>(); 5837 this.endorser.add(t); 5838 return this; 5839 } 5840 5841 /** 5842 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 5843 */ 5844 public ContactDetail getEndorserFirstRep() { 5845 if (getEndorser().isEmpty()) { 5846 addEndorser(); 5847 } 5848 return getEndorser().get(0); 5849 } 5850 5851 /** 5852 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 5853 */ 5854 public List<RelatedArtifact> getRelatedArtifact() { 5855 if (this.relatedArtifact == null) 5856 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5857 return this.relatedArtifact; 5858 } 5859 5860 /** 5861 * @return Returns a reference to <code>this</code> for easy method chaining 5862 */ 5863 public ConceptMap setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 5864 this.relatedArtifact = theRelatedArtifact; 5865 return this; 5866 } 5867 5868 public boolean hasRelatedArtifact() { 5869 if (this.relatedArtifact == null) 5870 return false; 5871 for (RelatedArtifact item : this.relatedArtifact) 5872 if (!item.isEmpty()) 5873 return true; 5874 return false; 5875 } 5876 5877 public RelatedArtifact addRelatedArtifact() { //3 5878 RelatedArtifact t = new RelatedArtifact(); 5879 if (this.relatedArtifact == null) 5880 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5881 this.relatedArtifact.add(t); 5882 return t; 5883 } 5884 5885 public ConceptMap addRelatedArtifact(RelatedArtifact t) { //3 5886 if (t == null) 5887 return this; 5888 if (this.relatedArtifact == null) 5889 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5890 this.relatedArtifact.add(t); 5891 return this; 5892 } 5893 5894 /** 5895 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 5896 */ 5897 public RelatedArtifact getRelatedArtifactFirstRep() { 5898 if (getRelatedArtifact().isEmpty()) { 5899 addRelatedArtifact(); 5900 } 5901 return getRelatedArtifact().get(0); 5902 } 5903 5904 /** 5905 * @return {@link #property} (A property defines a slot through which additional information can be provided about a map from source -> target.) 5906 */ 5907 public List<PropertyComponent> getProperty() { 5908 if (this.property == null) 5909 this.property = new ArrayList<PropertyComponent>(); 5910 return this.property; 5911 } 5912 5913 /** 5914 * @return Returns a reference to <code>this</code> for easy method chaining 5915 */ 5916 public ConceptMap setProperty(List<PropertyComponent> theProperty) { 5917 this.property = theProperty; 5918 return this; 5919 } 5920 5921 public boolean hasProperty() { 5922 if (this.property == null) 5923 return false; 5924 for (PropertyComponent item : this.property) 5925 if (!item.isEmpty()) 5926 return true; 5927 return false; 5928 } 5929 5930 public PropertyComponent addProperty() { //3 5931 PropertyComponent t = new PropertyComponent(); 5932 if (this.property == null) 5933 this.property = new ArrayList<PropertyComponent>(); 5934 this.property.add(t); 5935 return t; 5936 } 5937 5938 public ConceptMap addProperty(PropertyComponent t) { //3 5939 if (t == null) 5940 return this; 5941 if (this.property == null) 5942 this.property = new ArrayList<PropertyComponent>(); 5943 this.property.add(t); 5944 return this; 5945 } 5946 5947 /** 5948 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 5949 */ 5950 public PropertyComponent getPropertyFirstRep() { 5951 if (getProperty().isEmpty()) { 5952 addProperty(); 5953 } 5954 return getProperty().get(0); 5955 } 5956 5957 /** 5958 * @return {@link #additionalAttribute} (An additionalAttribute defines an additional data element found in the source or target data model where the data will come from or be mapped to. Some mappings are based on data in addition to the source data element, where codes in multiple fields are combined to a single field (or vice versa).) 5959 */ 5960 public List<AdditionalAttributeComponent> getAdditionalAttribute() { 5961 if (this.additionalAttribute == null) 5962 this.additionalAttribute = new ArrayList<AdditionalAttributeComponent>(); 5963 return this.additionalAttribute; 5964 } 5965 5966 /** 5967 * @return Returns a reference to <code>this</code> for easy method chaining 5968 */ 5969 public ConceptMap setAdditionalAttribute(List<AdditionalAttributeComponent> theAdditionalAttribute) { 5970 this.additionalAttribute = theAdditionalAttribute; 5971 return this; 5972 } 5973 5974 public boolean hasAdditionalAttribute() { 5975 if (this.additionalAttribute == null) 5976 return false; 5977 for (AdditionalAttributeComponent item : this.additionalAttribute) 5978 if (!item.isEmpty()) 5979 return true; 5980 return false; 5981 } 5982 5983 public AdditionalAttributeComponent addAdditionalAttribute() { //3 5984 AdditionalAttributeComponent t = new AdditionalAttributeComponent(); 5985 if (this.additionalAttribute == null) 5986 this.additionalAttribute = new ArrayList<AdditionalAttributeComponent>(); 5987 this.additionalAttribute.add(t); 5988 return t; 5989 } 5990 5991 public ConceptMap addAdditionalAttribute(AdditionalAttributeComponent t) { //3 5992 if (t == null) 5993 return this; 5994 if (this.additionalAttribute == null) 5995 this.additionalAttribute = new ArrayList<AdditionalAttributeComponent>(); 5996 this.additionalAttribute.add(t); 5997 return this; 5998 } 5999 6000 /** 6001 * @return The first repetition of repeating field {@link #additionalAttribute}, creating it if it does not already exist {3} 6002 */ 6003 public AdditionalAttributeComponent getAdditionalAttributeFirstRep() { 6004 if (getAdditionalAttribute().isEmpty()) { 6005 addAdditionalAttribute(); 6006 } 6007 return getAdditionalAttribute().get(0); 6008 } 6009 6010 /** 6011 * @return {@link #sourceScope} (Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.) 6012 */ 6013 public DataType getSourceScope() { 6014 return this.sourceScope; 6015 } 6016 6017 /** 6018 * @return {@link #sourceScope} (Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.) 6019 */ 6020 public UriType getSourceScopeUriType() throws FHIRException { 6021 if (this.sourceScope == null) 6022 this.sourceScope = new UriType(); 6023 if (!(this.sourceScope instanceof UriType)) 6024 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.sourceScope.getClass().getName()+" was encountered"); 6025 return (UriType) this.sourceScope; 6026 } 6027 6028 public boolean hasSourceScopeUriType() { 6029 return this != null && this.sourceScope instanceof UriType; 6030 } 6031 6032 /** 6033 * @return {@link #sourceScope} (Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.) 6034 */ 6035 public CanonicalType getSourceScopeCanonicalType() throws FHIRException { 6036 if (this.sourceScope == null) 6037 this.sourceScope = new CanonicalType(); 6038 if (!(this.sourceScope instanceof CanonicalType)) 6039 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.sourceScope.getClass().getName()+" was encountered"); 6040 return (CanonicalType) this.sourceScope; 6041 } 6042 6043 public boolean hasSourceScopeCanonicalType() { 6044 return this != null && this.sourceScope instanceof CanonicalType; 6045 } 6046 6047 public boolean hasSourceScope() { 6048 return this.sourceScope != null && !this.sourceScope.isEmpty(); 6049 } 6050 6051 /** 6052 * @param value {@link #sourceScope} (Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.) 6053 */ 6054 public ConceptMap setSourceScope(DataType value) { 6055 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 6056 throw new FHIRException("Not the right type for ConceptMap.sourceScope[x]: "+value.fhirType()); 6057 this.sourceScope = value; 6058 return this; 6059 } 6060 6061 /** 6062 * @return {@link #targetScope} (Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.) 6063 */ 6064 public DataType getTargetScope() { 6065 return this.targetScope; 6066 } 6067 6068 /** 6069 * @return {@link #targetScope} (Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.) 6070 */ 6071 public UriType getTargetScopeUriType() throws FHIRException { 6072 if (this.targetScope == null) 6073 this.targetScope = new UriType(); 6074 if (!(this.targetScope instanceof UriType)) 6075 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.targetScope.getClass().getName()+" was encountered"); 6076 return (UriType) this.targetScope; 6077 } 6078 6079 public boolean hasTargetScopeUriType() { 6080 return this != null && this.targetScope instanceof UriType; 6081 } 6082 6083 /** 6084 * @return {@link #targetScope} (Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.) 6085 */ 6086 public CanonicalType getTargetScopeCanonicalType() throws FHIRException { 6087 if (this.targetScope == null) 6088 this.targetScope = new CanonicalType(); 6089 if (!(this.targetScope instanceof CanonicalType)) 6090 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.targetScope.getClass().getName()+" was encountered"); 6091 return (CanonicalType) this.targetScope; 6092 } 6093 6094 public boolean hasTargetScopeCanonicalType() { 6095 return this != null && this.targetScope instanceof CanonicalType; 6096 } 6097 6098 public boolean hasTargetScope() { 6099 return this.targetScope != null && !this.targetScope.isEmpty(); 6100 } 6101 6102 /** 6103 * @param value {@link #targetScope} (Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.) 6104 */ 6105 public ConceptMap setTargetScope(DataType value) { 6106 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 6107 throw new FHIRException("Not the right type for ConceptMap.targetScope[x]: "+value.fhirType()); 6108 this.targetScope = value; 6109 return this; 6110 } 6111 6112 /** 6113 * @return {@link #group} (A group of mappings that all have the same source and target system.) 6114 */ 6115 public List<ConceptMapGroupComponent> getGroup() { 6116 if (this.group == null) 6117 this.group = new ArrayList<ConceptMapGroupComponent>(); 6118 return this.group; 6119 } 6120 6121 /** 6122 * @return Returns a reference to <code>this</code> for easy method chaining 6123 */ 6124 public ConceptMap setGroup(List<ConceptMapGroupComponent> theGroup) { 6125 this.group = theGroup; 6126 return this; 6127 } 6128 6129 public boolean hasGroup() { 6130 if (this.group == null) 6131 return false; 6132 for (ConceptMapGroupComponent item : this.group) 6133 if (!item.isEmpty()) 6134 return true; 6135 return false; 6136 } 6137 6138 public ConceptMapGroupComponent addGroup() { //3 6139 ConceptMapGroupComponent t = new ConceptMapGroupComponent(); 6140 if (this.group == null) 6141 this.group = new ArrayList<ConceptMapGroupComponent>(); 6142 this.group.add(t); 6143 return t; 6144 } 6145 6146 public ConceptMap addGroup(ConceptMapGroupComponent t) { //3 6147 if (t == null) 6148 return this; 6149 if (this.group == null) 6150 this.group = new ArrayList<ConceptMapGroupComponent>(); 6151 this.group.add(t); 6152 return this; 6153 } 6154 6155 /** 6156 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist {3} 6157 */ 6158 public ConceptMapGroupComponent getGroupFirstRep() { 6159 if (getGroup().isEmpty()) { 6160 addGroup(); 6161 } 6162 return getGroup().get(0); 6163 } 6164 6165 protected void listChildren(List<Property> children) { 6166 super.listChildren(children); 6167 children.add(new Property("url", "uri", "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.", 0, 1, url)); 6168 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6169 children.add(new Property("version", "string", "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 6170 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which ConceptMap is more current.", 0, 1, versionAlgorithm)); 6171 children.add(new Property("name", "string", "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 6172 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the concept map.", 0, 1, title)); 6173 children.add(new Property("status", "code", "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status)); 6174 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 6175 children.add(new Property("date", "dateTime", "The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 0, 1, date)); 6176 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the concept map.", 0, 1, publisher)); 6177 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 6178 children.add(new Property("description", "markdown", "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, description)); 6179 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 6180 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the concept map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 6181 children.add(new Property("purpose", "markdown", "Explanation of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose)); 6182 children.add(new Property("copyright", "markdown", "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 0, 1, copyright)); 6183 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 6184 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 6185 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 6186 children.add(new Property("effectivePeriod", "Period", "The period during which the ConceptMap content was or is planned to be in active use.", 0, 1, effectivePeriod)); 6187 children.add(new Property("topic", "CodeableConcept", "Descriptions related to the content of the ConceptMap. Topics provide a high-level categorization as well as keywords for the ConceptMap that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 6188 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the ConceptMap.", 0, java.lang.Integer.MAX_VALUE, author)); 6189 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the ConceptMap.", 0, java.lang.Integer.MAX_VALUE, editor)); 6190 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ConceptMap.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 6191 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the ConceptMap for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 6192 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 6193 children.add(new Property("property", "", "A property defines a slot through which additional information can be provided about a map from source -> target.", 0, java.lang.Integer.MAX_VALUE, property)); 6194 children.add(new Property("additionalAttribute", "", "An additionalAttribute defines an additional data element found in the source or target data model where the data will come from or be mapped to. Some mappings are based on data in addition to the source data element, where codes in multiple fields are combined to a single field (or vice versa).", 0, java.lang.Integer.MAX_VALUE, additionalAttribute)); 6195 children.add(new Property("sourceScope[x]", "uri|canonical(ValueSet)", "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.", 0, 1, sourceScope)); 6196 children.add(new Property("targetScope[x]", "uri|canonical(ValueSet)", "Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.", 0, 1, targetScope)); 6197 children.add(new Property("group", "", "A group of mappings that all have the same source and target system.", 0, java.lang.Integer.MAX_VALUE, group)); 6198 } 6199 6200 @Override 6201 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6202 switch (_hash) { 6203 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.", 0, 1, url); 6204 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 6205 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 6206 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which ConceptMap is more current.", 0, 1, versionAlgorithm); 6207 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which ConceptMap is more current.", 0, 1, versionAlgorithm); 6208 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which ConceptMap is more current.", 0, 1, versionAlgorithm); 6209 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which ConceptMap is more current.", 0, 1, versionAlgorithm); 6210 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 6211 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the concept map.", 0, 1, title); 6212 case -892481550: /*status*/ return new Property("status", "code", "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status); 6213 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 6214 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the concept map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 0, 1, date); 6215 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the concept map.", 0, 1, publisher); 6216 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 6217 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, description); 6218 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 6219 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the concept map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 6220 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose); 6221 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 0, 1, copyright); 6222 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 6223 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 6224 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 6225 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the ConceptMap content was or is planned to be in active use.", 0, 1, effectivePeriod); 6226 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptions related to the content of the ConceptMap. Topics provide a high-level categorization as well as keywords for the ConceptMap that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 6227 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the ConceptMap.", 0, java.lang.Integer.MAX_VALUE, author); 6228 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the ConceptMap.", 0, java.lang.Integer.MAX_VALUE, editor); 6229 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the ConceptMap.", 0, java.lang.Integer.MAX_VALUE, reviewer); 6230 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the ConceptMap for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 6231 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 6232 case -993141291: /*property*/ return new Property("property", "", "A property defines a slot through which additional information can be provided about a map from source -> target.", 0, java.lang.Integer.MAX_VALUE, property); 6233 case -1004532171: /*additionalAttribute*/ return new Property("additionalAttribute", "", "An additionalAttribute defines an additional data element found in the source or target data model where the data will come from or be mapped to. Some mappings are based on data in addition to the source data element, where codes in multiple fields are combined to a single field (or vice versa).", 0, java.lang.Integer.MAX_VALUE, additionalAttribute); 6234 case -1850861849: /*sourceScope[x]*/ return new Property("sourceScope[x]", "uri|canonical(ValueSet)", "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.", 0, 1, sourceScope); 6235 case -96223495: /*sourceScope*/ return new Property("sourceScope[x]", "uri|canonical(ValueSet)", "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.", 0, 1, sourceScope); 6236 case -1850867789: /*sourceScopeUri*/ return new Property("sourceScope[x]", "uri", "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.", 0, 1, sourceScope); 6237 case -1487745541: /*sourceScopeCanonical*/ return new Property("sourceScope[x]", "canonical(ValueSet)", "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings. Limits the scope of the map to source codes (ConceptMap.group.element code or valueSet) that are members of this value set.", 0, 1, sourceScope); 6238 case -2079438243: /*targetScope[x]*/ return new Property("targetScope[x]", "uri|canonical(ValueSet)", "Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.", 0, 1, targetScope); 6239 case -2096156861: /*targetScope*/ return new Property("targetScope[x]", "uri|canonical(ValueSet)", "Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.", 0, 1, targetScope); 6240 case -2079444183: /*targetScopeUri*/ return new Property("targetScope[x]", "uri", "Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.", 0, 1, targetScope); 6241 case -1851780879: /*targetScopeCanonical*/ return new Property("targetScope[x]", "canonical(ValueSet)", "Identifier for the target value set that provides important context about how the mapping choices are made. Limits the scope of the map to target codes (ConceptMap.group.element.target code or valueSet) that are members of this value set.", 0, 1, targetScope); 6242 case 98629247: /*group*/ return new Property("group", "", "A group of mappings that all have the same source and target system.", 0, java.lang.Integer.MAX_VALUE, group); 6243 default: return super.getNamedProperty(_hash, _name, _checkValid); 6244 } 6245 6246 } 6247 6248 @Override 6249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6250 switch (hash) { 6251 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 6252 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6253 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 6254 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 6255 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 6256 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 6257 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 6258 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 6259 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 6260 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 6261 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 6262 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 6263 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 6264 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 6265 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 6266 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 6267 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 6268 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 6269 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 6270 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 6271 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 6272 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 6273 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 6274 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 6275 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 6276 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 6277 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // PropertyComponent 6278 case -1004532171: /*additionalAttribute*/ return this.additionalAttribute == null ? new Base[0] : this.additionalAttribute.toArray(new Base[this.additionalAttribute.size()]); // AdditionalAttributeComponent 6279 case -96223495: /*sourceScope*/ return this.sourceScope == null ? new Base[0] : new Base[] {this.sourceScope}; // DataType 6280 case -2096156861: /*targetScope*/ return this.targetScope == null ? new Base[0] : new Base[] {this.targetScope}; // DataType 6281 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // ConceptMapGroupComponent 6282 default: return super.getProperty(hash, name, checkValid); 6283 } 6284 6285 } 6286 6287 @Override 6288 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6289 switch (hash) { 6290 case 116079: // url 6291 this.url = TypeConvertor.castToUri(value); // UriType 6292 return value; 6293 case -1618432855: // identifier 6294 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6295 return value; 6296 case 351608024: // version 6297 this.version = TypeConvertor.castToString(value); // StringType 6298 return value; 6299 case 1508158071: // versionAlgorithm 6300 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 6301 return value; 6302 case 3373707: // name 6303 this.name = TypeConvertor.castToString(value); // StringType 6304 return value; 6305 case 110371416: // title 6306 this.title = TypeConvertor.castToString(value); // StringType 6307 return value; 6308 case -892481550: // status 6309 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6310 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6311 return value; 6312 case -404562712: // experimental 6313 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6314 return value; 6315 case 3076014: // date 6316 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6317 return value; 6318 case 1447404028: // publisher 6319 this.publisher = TypeConvertor.castToString(value); // StringType 6320 return value; 6321 case 951526432: // contact 6322 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6323 return value; 6324 case -1724546052: // description 6325 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6326 return value; 6327 case -669707736: // useContext 6328 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 6329 return value; 6330 case -507075711: // jurisdiction 6331 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6332 return value; 6333 case -220463842: // purpose 6334 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6335 return value; 6336 case 1522889671: // copyright 6337 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6338 return value; 6339 case 765157229: // copyrightLabel 6340 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6341 return value; 6342 case 223539345: // approvalDate 6343 this.approvalDate = TypeConvertor.castToDate(value); // DateType 6344 return value; 6345 case -1687512484: // lastReviewDate 6346 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 6347 return value; 6348 case -403934648: // effectivePeriod 6349 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 6350 return value; 6351 case 110546223: // topic 6352 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6353 return value; 6354 case -1406328437: // author 6355 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6356 return value; 6357 case -1307827859: // editor 6358 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6359 return value; 6360 case -261190139: // reviewer 6361 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6362 return value; 6363 case 1740277666: // endorser 6364 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6365 return value; 6366 case 666807069: // relatedArtifact 6367 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 6368 return value; 6369 case -993141291: // property 6370 this.getProperty().add((PropertyComponent) value); // PropertyComponent 6371 return value; 6372 case -1004532171: // additionalAttribute 6373 this.getAdditionalAttribute().add((AdditionalAttributeComponent) value); // AdditionalAttributeComponent 6374 return value; 6375 case -96223495: // sourceScope 6376 this.sourceScope = TypeConvertor.castToType(value); // DataType 6377 return value; 6378 case -2096156861: // targetScope 6379 this.targetScope = TypeConvertor.castToType(value); // DataType 6380 return value; 6381 case 98629247: // group 6382 this.getGroup().add((ConceptMapGroupComponent) value); // ConceptMapGroupComponent 6383 return value; 6384 default: return super.setProperty(hash, name, value); 6385 } 6386 6387 } 6388 6389 @Override 6390 public Base setProperty(String name, Base value) throws FHIRException { 6391 if (name.equals("url")) { 6392 this.url = TypeConvertor.castToUri(value); // UriType 6393 } else if (name.equals("identifier")) { 6394 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6395 } else if (name.equals("version")) { 6396 this.version = TypeConvertor.castToString(value); // StringType 6397 } else if (name.equals("versionAlgorithm[x]")) { 6398 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 6399 } else if (name.equals("name")) { 6400 this.name = TypeConvertor.castToString(value); // StringType 6401 } else if (name.equals("title")) { 6402 this.title = TypeConvertor.castToString(value); // StringType 6403 } else if (name.equals("status")) { 6404 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6405 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6406 } else if (name.equals("experimental")) { 6407 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6408 } else if (name.equals("date")) { 6409 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6410 } else if (name.equals("publisher")) { 6411 this.publisher = TypeConvertor.castToString(value); // StringType 6412 } else if (name.equals("contact")) { 6413 this.getContact().add(TypeConvertor.castToContactDetail(value)); 6414 } else if (name.equals("description")) { 6415 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6416 } else if (name.equals("useContext")) { 6417 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 6418 } else if (name.equals("jurisdiction")) { 6419 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 6420 } else if (name.equals("purpose")) { 6421 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6422 } else if (name.equals("copyright")) { 6423 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6424 } else if (name.equals("copyrightLabel")) { 6425 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6426 } else if (name.equals("approvalDate")) { 6427 this.approvalDate = TypeConvertor.castToDate(value); // DateType 6428 } else if (name.equals("lastReviewDate")) { 6429 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 6430 } else if (name.equals("effectivePeriod")) { 6431 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 6432 } else if (name.equals("topic")) { 6433 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 6434 } else if (name.equals("author")) { 6435 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 6436 } else if (name.equals("editor")) { 6437 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 6438 } else if (name.equals("reviewer")) { 6439 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 6440 } else if (name.equals("endorser")) { 6441 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 6442 } else if (name.equals("relatedArtifact")) { 6443 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 6444 } else if (name.equals("property")) { 6445 this.getProperty().add((PropertyComponent) value); 6446 } else if (name.equals("additionalAttribute")) { 6447 this.getAdditionalAttribute().add((AdditionalAttributeComponent) value); 6448 } else if (name.equals("sourceScope[x]")) { 6449 this.sourceScope = TypeConvertor.castToType(value); // DataType 6450 } else if (name.equals("targetScope[x]")) { 6451 this.targetScope = TypeConvertor.castToType(value); // DataType 6452 } else if (name.equals("group")) { 6453 this.getGroup().add((ConceptMapGroupComponent) value); 6454 } else 6455 return super.setProperty(name, value); 6456 return value; 6457 } 6458 6459 @Override 6460 public void removeChild(String name, Base value) throws FHIRException { 6461 if (name.equals("url")) { 6462 this.url = null; 6463 } else if (name.equals("identifier")) { 6464 this.getIdentifier().remove(value); 6465 } else if (name.equals("version")) { 6466 this.version = null; 6467 } else if (name.equals("versionAlgorithm[x]")) { 6468 this.versionAlgorithm = null; 6469 } else if (name.equals("name")) { 6470 this.name = null; 6471 } else if (name.equals("title")) { 6472 this.title = null; 6473 } else if (name.equals("status")) { 6474 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6475 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6476 } else if (name.equals("experimental")) { 6477 this.experimental = null; 6478 } else if (name.equals("date")) { 6479 this.date = null; 6480 } else if (name.equals("publisher")) { 6481 this.publisher = null; 6482 } else if (name.equals("contact")) { 6483 this.getContact().remove(value); 6484 } else if (name.equals("description")) { 6485 this.description = null; 6486 } else if (name.equals("useContext")) { 6487 this.getUseContext().remove(value); 6488 } else if (name.equals("jurisdiction")) { 6489 this.getJurisdiction().remove(value); 6490 } else if (name.equals("purpose")) { 6491 this.purpose = null; 6492 } else if (name.equals("copyright")) { 6493 this.copyright = null; 6494 } else if (name.equals("copyrightLabel")) { 6495 this.copyrightLabel = null; 6496 } else if (name.equals("approvalDate")) { 6497 this.approvalDate = null; 6498 } else if (name.equals("lastReviewDate")) { 6499 this.lastReviewDate = null; 6500 } else if (name.equals("effectivePeriod")) { 6501 this.effectivePeriod = null; 6502 } else if (name.equals("topic")) { 6503 this.getTopic().remove(value); 6504 } else if (name.equals("author")) { 6505 this.getAuthor().remove(value); 6506 } else if (name.equals("editor")) { 6507 this.getEditor().remove(value); 6508 } else if (name.equals("reviewer")) { 6509 this.getReviewer().remove(value); 6510 } else if (name.equals("endorser")) { 6511 this.getEndorser().remove(value); 6512 } else if (name.equals("relatedArtifact")) { 6513 this.getRelatedArtifact().remove(value); 6514 } else if (name.equals("property")) { 6515 this.getProperty().remove((PropertyComponent) value); 6516 } else if (name.equals("additionalAttribute")) { 6517 this.getAdditionalAttribute().remove((AdditionalAttributeComponent) value); 6518 } else if (name.equals("sourceScope[x]")) { 6519 this.sourceScope = null; 6520 } else if (name.equals("targetScope[x]")) { 6521 this.targetScope = null; 6522 } else if (name.equals("group")) { 6523 this.getGroup().remove((ConceptMapGroupComponent) value); 6524 } else 6525 super.removeChild(name, value); 6526 6527 } 6528 6529 @Override 6530 public Base makeProperty(int hash, String name) throws FHIRException { 6531 switch (hash) { 6532 case 116079: return getUrlElement(); 6533 case -1618432855: return addIdentifier(); 6534 case 351608024: return getVersionElement(); 6535 case -115699031: return getVersionAlgorithm(); 6536 case 1508158071: return getVersionAlgorithm(); 6537 case 3373707: return getNameElement(); 6538 case 110371416: return getTitleElement(); 6539 case -892481550: return getStatusElement(); 6540 case -404562712: return getExperimentalElement(); 6541 case 3076014: return getDateElement(); 6542 case 1447404028: return getPublisherElement(); 6543 case 951526432: return addContact(); 6544 case -1724546052: return getDescriptionElement(); 6545 case -669707736: return addUseContext(); 6546 case -507075711: return addJurisdiction(); 6547 case -220463842: return getPurposeElement(); 6548 case 1522889671: return getCopyrightElement(); 6549 case 765157229: return getCopyrightLabelElement(); 6550 case 223539345: return getApprovalDateElement(); 6551 case -1687512484: return getLastReviewDateElement(); 6552 case -403934648: return getEffectivePeriod(); 6553 case 110546223: return addTopic(); 6554 case -1406328437: return addAuthor(); 6555 case -1307827859: return addEditor(); 6556 case -261190139: return addReviewer(); 6557 case 1740277666: return addEndorser(); 6558 case 666807069: return addRelatedArtifact(); 6559 case -993141291: return addProperty(); 6560 case -1004532171: return addAdditionalAttribute(); 6561 case -1850861849: return getSourceScope(); 6562 case -96223495: return getSourceScope(); 6563 case -2079438243: return getTargetScope(); 6564 case -2096156861: return getTargetScope(); 6565 case 98629247: return addGroup(); 6566 default: return super.makeProperty(hash, name); 6567 } 6568 6569 } 6570 6571 @Override 6572 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6573 switch (hash) { 6574 case 116079: /*url*/ return new String[] {"uri"}; 6575 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6576 case 351608024: /*version*/ return new String[] {"string"}; 6577 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 6578 case 3373707: /*name*/ return new String[] {"string"}; 6579 case 110371416: /*title*/ return new String[] {"string"}; 6580 case -892481550: /*status*/ return new String[] {"code"}; 6581 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6582 case 3076014: /*date*/ return new String[] {"dateTime"}; 6583 case 1447404028: /*publisher*/ return new String[] {"string"}; 6584 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 6585 case -1724546052: /*description*/ return new String[] {"markdown"}; 6586 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 6587 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 6588 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6589 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 6590 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 6591 case 223539345: /*approvalDate*/ return new String[] {"date"}; 6592 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 6593 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 6594 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 6595 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 6596 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 6597 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 6598 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 6599 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 6600 case -993141291: /*property*/ return new String[] {}; 6601 case -1004532171: /*additionalAttribute*/ return new String[] {}; 6602 case -96223495: /*sourceScope*/ return new String[] {"uri", "canonical"}; 6603 case -2096156861: /*targetScope*/ return new String[] {"uri", "canonical"}; 6604 case 98629247: /*group*/ return new String[] {}; 6605 default: return super.getTypesForProperty(hash, name); 6606 } 6607 6608 } 6609 6610 @Override 6611 public Base addChild(String name) throws FHIRException { 6612 if (name.equals("url")) { 6613 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 6614 } 6615 else if (name.equals("identifier")) { 6616 return addIdentifier(); 6617 } 6618 else if (name.equals("version")) { 6619 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.version"); 6620 } 6621 else if (name.equals("versionAlgorithmString")) { 6622 this.versionAlgorithm = new StringType(); 6623 return this.versionAlgorithm; 6624 } 6625 else if (name.equals("versionAlgorithmCoding")) { 6626 this.versionAlgorithm = new Coding(); 6627 return this.versionAlgorithm; 6628 } 6629 else if (name.equals("name")) { 6630 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.name"); 6631 } 6632 else if (name.equals("title")) { 6633 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.title"); 6634 } 6635 else if (name.equals("status")) { 6636 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.status"); 6637 } 6638 else if (name.equals("experimental")) { 6639 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.experimental"); 6640 } 6641 else if (name.equals("date")) { 6642 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.date"); 6643 } 6644 else if (name.equals("publisher")) { 6645 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.publisher"); 6646 } 6647 else if (name.equals("contact")) { 6648 return addContact(); 6649 } 6650 else if (name.equals("description")) { 6651 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.description"); 6652 } 6653 else if (name.equals("useContext")) { 6654 return addUseContext(); 6655 } 6656 else if (name.equals("jurisdiction")) { 6657 return addJurisdiction(); 6658 } 6659 else if (name.equals("purpose")) { 6660 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.purpose"); 6661 } 6662 else if (name.equals("copyright")) { 6663 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.copyright"); 6664 } 6665 else if (name.equals("copyrightLabel")) { 6666 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.copyrightLabel"); 6667 } 6668 else if (name.equals("approvalDate")) { 6669 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.approvalDate"); 6670 } 6671 else if (name.equals("lastReviewDate")) { 6672 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.lastReviewDate"); 6673 } 6674 else if (name.equals("effectivePeriod")) { 6675 this.effectivePeriod = new Period(); 6676 return this.effectivePeriod; 6677 } 6678 else if (name.equals("topic")) { 6679 return addTopic(); 6680 } 6681 else if (name.equals("author")) { 6682 return addAuthor(); 6683 } 6684 else if (name.equals("editor")) { 6685 return addEditor(); 6686 } 6687 else if (name.equals("reviewer")) { 6688 return addReviewer(); 6689 } 6690 else if (name.equals("endorser")) { 6691 return addEndorser(); 6692 } 6693 else if (name.equals("relatedArtifact")) { 6694 return addRelatedArtifact(); 6695 } 6696 else if (name.equals("property")) { 6697 return addProperty(); 6698 } 6699 else if (name.equals("additionalAttribute")) { 6700 return addAdditionalAttribute(); 6701 } 6702 else if (name.equals("sourceScopeUri")) { 6703 this.sourceScope = new UriType(); 6704 return this.sourceScope; 6705 } 6706 else if (name.equals("sourceScopeCanonical")) { 6707 this.sourceScope = new CanonicalType(); 6708 return this.sourceScope; 6709 } 6710 else if (name.equals("targetScopeUri")) { 6711 this.targetScope = new UriType(); 6712 return this.targetScope; 6713 } 6714 else if (name.equals("targetScopeCanonical")) { 6715 this.targetScope = new CanonicalType(); 6716 return this.targetScope; 6717 } 6718 else if (name.equals("group")) { 6719 return addGroup(); 6720 } 6721 else 6722 return super.addChild(name); 6723 } 6724 6725 public String fhirType() { 6726 return "ConceptMap"; 6727 6728 } 6729 6730 public ConceptMap copy() { 6731 ConceptMap dst = new ConceptMap(); 6732 copyValues(dst); 6733 return dst; 6734 } 6735 6736 public void copyValues(ConceptMap dst) { 6737 super.copyValues(dst); 6738 dst.url = url == null ? null : url.copy(); 6739 if (identifier != null) { 6740 dst.identifier = new ArrayList<Identifier>(); 6741 for (Identifier i : identifier) 6742 dst.identifier.add(i.copy()); 6743 }; 6744 dst.version = version == null ? null : version.copy(); 6745 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 6746 dst.name = name == null ? null : name.copy(); 6747 dst.title = title == null ? null : title.copy(); 6748 dst.status = status == null ? null : status.copy(); 6749 dst.experimental = experimental == null ? null : experimental.copy(); 6750 dst.date = date == null ? null : date.copy(); 6751 dst.publisher = publisher == null ? null : publisher.copy(); 6752 if (contact != null) { 6753 dst.contact = new ArrayList<ContactDetail>(); 6754 for (ContactDetail i : contact) 6755 dst.contact.add(i.copy()); 6756 }; 6757 dst.description = description == null ? null : description.copy(); 6758 if (useContext != null) { 6759 dst.useContext = new ArrayList<UsageContext>(); 6760 for (UsageContext i : useContext) 6761 dst.useContext.add(i.copy()); 6762 }; 6763 if (jurisdiction != null) { 6764 dst.jurisdiction = new ArrayList<CodeableConcept>(); 6765 for (CodeableConcept i : jurisdiction) 6766 dst.jurisdiction.add(i.copy()); 6767 }; 6768 dst.purpose = purpose == null ? null : purpose.copy(); 6769 dst.copyright = copyright == null ? null : copyright.copy(); 6770 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 6771 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 6772 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 6773 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 6774 if (topic != null) { 6775 dst.topic = new ArrayList<CodeableConcept>(); 6776 for (CodeableConcept i : topic) 6777 dst.topic.add(i.copy()); 6778 }; 6779 if (author != null) { 6780 dst.author = new ArrayList<ContactDetail>(); 6781 for (ContactDetail i : author) 6782 dst.author.add(i.copy()); 6783 }; 6784 if (editor != null) { 6785 dst.editor = new ArrayList<ContactDetail>(); 6786 for (ContactDetail i : editor) 6787 dst.editor.add(i.copy()); 6788 }; 6789 if (reviewer != null) { 6790 dst.reviewer = new ArrayList<ContactDetail>(); 6791 for (ContactDetail i : reviewer) 6792 dst.reviewer.add(i.copy()); 6793 }; 6794 if (endorser != null) { 6795 dst.endorser = new ArrayList<ContactDetail>(); 6796 for (ContactDetail i : endorser) 6797 dst.endorser.add(i.copy()); 6798 }; 6799 if (relatedArtifact != null) { 6800 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 6801 for (RelatedArtifact i : relatedArtifact) 6802 dst.relatedArtifact.add(i.copy()); 6803 }; 6804 if (property != null) { 6805 dst.property = new ArrayList<PropertyComponent>(); 6806 for (PropertyComponent i : property) 6807 dst.property.add(i.copy()); 6808 }; 6809 if (additionalAttribute != null) { 6810 dst.additionalAttribute = new ArrayList<AdditionalAttributeComponent>(); 6811 for (AdditionalAttributeComponent i : additionalAttribute) 6812 dst.additionalAttribute.add(i.copy()); 6813 }; 6814 dst.sourceScope = sourceScope == null ? null : sourceScope.copy(); 6815 dst.targetScope = targetScope == null ? null : targetScope.copy(); 6816 if (group != null) { 6817 dst.group = new ArrayList<ConceptMapGroupComponent>(); 6818 for (ConceptMapGroupComponent i : group) 6819 dst.group.add(i.copy()); 6820 }; 6821 } 6822 6823 protected ConceptMap typedCopy() { 6824 return copy(); 6825 } 6826 6827 @Override 6828 public boolean equalsDeep(Base other_) { 6829 if (!super.equalsDeep(other_)) 6830 return false; 6831 if (!(other_ instanceof ConceptMap)) 6832 return false; 6833 ConceptMap o = (ConceptMap) other_; 6834 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 6835 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 6836 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 6837 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 6838 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 6839 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 6840 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 6841 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 6842 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 6843 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(property, o.property, true) 6844 && compareDeep(additionalAttribute, o.additionalAttribute, true) && compareDeep(sourceScope, o.sourceScope, true) 6845 && compareDeep(targetScope, o.targetScope, true) && compareDeep(group, o.group, true); 6846 } 6847 6848 @Override 6849 public boolean equalsShallow(Base other_) { 6850 if (!super.equalsShallow(other_)) 6851 return false; 6852 if (!(other_ instanceof ConceptMap)) 6853 return false; 6854 ConceptMap o = (ConceptMap) other_; 6855 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 6856 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 6857 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 6858 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 6859 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 6860 ; 6861 } 6862 6863 public boolean isEmpty() { 6864 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 6865 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 6866 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, approvalDate 6867 , lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact 6868 , property, additionalAttribute, sourceScope, targetScope, group); 6869 } 6870 6871 @Override 6872 public ResourceType getResourceType() { 6873 return ResourceType.ConceptMap; 6874 } 6875 6876 /** 6877 * Search parameter: <b>context-quantity</b> 6878 * <p> 6879 * Description: <b>Multiple Resources: 6880 6881* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 6882* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 6883* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 6884* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 6885* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 6886* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 6887* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 6888* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 6889* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 6890* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 6891* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 6892* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 6893* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 6894* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 6895* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 6896* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 6897* [Library](library.html): A quantity- or range-valued use context assigned to the library 6898* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 6899* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 6900* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 6901* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 6902* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 6903* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 6904* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 6905* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 6906* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 6907* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 6908* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 6909* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 6910* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 6911</b><br> 6912 * Type: <b>quantity</b><br> 6913 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 6914 * </p> 6915 */ 6916 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 6917 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6918 /** 6919 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6920 * <p> 6921 * Description: <b>Multiple Resources: 6922 6923* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 6924* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 6925* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 6926* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 6927* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 6928* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 6929* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 6930* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 6931* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 6932* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 6933* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 6934* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 6935* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 6936* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 6937* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 6938* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 6939* [Library](library.html): A quantity- or range-valued use context assigned to the library 6940* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 6941* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 6942* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 6943* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 6944* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 6945* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 6946* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 6947* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 6948* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 6949* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 6950* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 6951* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 6952* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 6953</b><br> 6954 * Type: <b>quantity</b><br> 6955 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 6956 * </p> 6957 */ 6958 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 6959 6960 /** 6961 * Search parameter: <b>context-type-quantity</b> 6962 * <p> 6963 * Description: <b>Multiple Resources: 6964 6965* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 6966* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 6967* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 6968* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 6969* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 6970* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 6971* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 6972* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 6973* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 6974* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 6975* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 6976* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 6977* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 6978* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 6979* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 6980* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 6981* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 6982* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 6983* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 6984* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 6985* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 6986* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 6987* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 6988* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 6989* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 6990* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 6991* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 6992* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 6993* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 6994* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 6995</b><br> 6996 * Type: <b>composite</b><br> 6997 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6998 * </p> 6999 */ 7000 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 7001 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 7002 /** 7003 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 7004 * <p> 7005 * Description: <b>Multiple Resources: 7006 7007* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7008* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7009* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7010* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7011* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7012* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7013* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7014* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7015* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7016* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7017* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7018* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7019* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7020* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7021* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7022* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7023* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7024* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7025* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7026* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7027* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7028* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7029* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7030* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7031* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7032* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7033* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7034* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7035* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7036* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7037</b><br> 7038 * Type: <b>composite</b><br> 7039 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7040 * </p> 7041 */ 7042 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 7043 7044 /** 7045 * Search parameter: <b>context-type-value</b> 7046 * <p> 7047 * Description: <b>Multiple Resources: 7048 7049* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7050* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7051* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7052* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7053* [Citation](citation.html): A use context type and value assigned to the citation 7054* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7055* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7056* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7057* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7058* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7059* [Evidence](evidence.html): A use context type and value assigned to the evidence 7060* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7061* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7062* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7063* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7064* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7065* [Library](library.html): A use context type and value assigned to the library 7066* [Measure](measure.html): A use context type and value assigned to the measure 7067* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7068* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7069* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7070* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7071* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7072* [Requirements](requirements.html): A use context type and value assigned to the requirements 7073* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7074* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7075* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7076* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7077* [TestScript](testscript.html): A use context type and value assigned to the test script 7078* [ValueSet](valueset.html): A use context type and value assigned to the value set 7079</b><br> 7080 * Type: <b>composite</b><br> 7081 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7082 * </p> 7083 */ 7084 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 7085 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 7086 /** 7087 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 7088 * <p> 7089 * Description: <b>Multiple Resources: 7090 7091* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7092* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7093* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7094* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7095* [Citation](citation.html): A use context type and value assigned to the citation 7096* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7097* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7098* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7099* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7100* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7101* [Evidence](evidence.html): A use context type and value assigned to the evidence 7102* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7103* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7104* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7105* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7106* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7107* [Library](library.html): A use context type and value assigned to the library 7108* [Measure](measure.html): A use context type and value assigned to the measure 7109* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7110* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7111* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7112* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7113* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7114* [Requirements](requirements.html): A use context type and value assigned to the requirements 7115* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7116* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7117* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7118* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7119* [TestScript](testscript.html): A use context type and value assigned to the test script 7120* [ValueSet](valueset.html): A use context type and value assigned to the value set 7121</b><br> 7122 * Type: <b>composite</b><br> 7123 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7124 * </p> 7125 */ 7126 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 7127 7128 /** 7129 * Search parameter: <b>context-type</b> 7130 * <p> 7131 * Description: <b>Multiple Resources: 7132 7133* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 7134* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 7135* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 7136* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 7137* [Citation](citation.html): A type of use context assigned to the citation 7138* [CodeSystem](codesystem.html): A type of use context assigned to the code system 7139* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 7140* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 7141* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 7142* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 7143* [Evidence](evidence.html): A type of use context assigned to the evidence 7144* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 7145* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 7146* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 7147* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 7148* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 7149* [Library](library.html): A type of use context assigned to the library 7150* [Measure](measure.html): A type of use context assigned to the measure 7151* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 7152* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 7153* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 7154* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 7155* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 7156* [Requirements](requirements.html): A type of use context assigned to the requirements 7157* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 7158* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 7159* [StructureMap](structuremap.html): A type of use context assigned to the structure map 7160* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 7161* [TestScript](testscript.html): A type of use context assigned to the test script 7162* [ValueSet](valueset.html): A type of use context assigned to the value set 7163</b><br> 7164 * Type: <b>token</b><br> 7165 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 7166 * </p> 7167 */ 7168 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 7169 public static final String SP_CONTEXT_TYPE = "context-type"; 7170 /** 7171 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 7172 * <p> 7173 * Description: <b>Multiple Resources: 7174 7175* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 7176* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 7177* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 7178* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 7179* [Citation](citation.html): A type of use context assigned to the citation 7180* [CodeSystem](codesystem.html): A type of use context assigned to the code system 7181* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 7182* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 7183* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 7184* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 7185* [Evidence](evidence.html): A type of use context assigned to the evidence 7186* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 7187* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 7188* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 7189* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 7190* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 7191* [Library](library.html): A type of use context assigned to the library 7192* [Measure](measure.html): A type of use context assigned to the measure 7193* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 7194* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 7195* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 7196* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 7197* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 7198* [Requirements](requirements.html): A type of use context assigned to the requirements 7199* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 7200* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 7201* [StructureMap](structuremap.html): A type of use context assigned to the structure map 7202* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 7203* [TestScript](testscript.html): A type of use context assigned to the test script 7204* [ValueSet](valueset.html): A type of use context assigned to the value set 7205</b><br> 7206 * Type: <b>token</b><br> 7207 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 7208 * </p> 7209 */ 7210 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 7211 7212 /** 7213 * Search parameter: <b>context</b> 7214 * <p> 7215 * Description: <b>Multiple Resources: 7216 7217* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7218* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7219* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7220* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7221* [Citation](citation.html): A use context assigned to the citation 7222* [CodeSystem](codesystem.html): A use context assigned to the code system 7223* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7224* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7225* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7226* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7227* [Evidence](evidence.html): A use context assigned to the evidence 7228* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7229* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7230* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7231* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7232* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7233* [Library](library.html): A use context assigned to the library 7234* [Measure](measure.html): A use context assigned to the measure 7235* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7236* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7237* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7238* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7239* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7240* [Requirements](requirements.html): A use context assigned to the requirements 7241* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7242* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7243* [StructureMap](structuremap.html): A use context assigned to the structure map 7244* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7245* [TestScript](testscript.html): A use context assigned to the test script 7246* [ValueSet](valueset.html): A use context assigned to the value set 7247</b><br> 7248 * Type: <b>token</b><br> 7249 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7250 * </p> 7251 */ 7252 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 7253 public static final String SP_CONTEXT = "context"; 7254 /** 7255 * <b>Fluent Client</b> search parameter constant for <b>context</b> 7256 * <p> 7257 * Description: <b>Multiple Resources: 7258 7259* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7260* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7261* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7262* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7263* [Citation](citation.html): A use context assigned to the citation 7264* [CodeSystem](codesystem.html): A use context assigned to the code system 7265* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7266* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7267* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7268* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7269* [Evidence](evidence.html): A use context assigned to the evidence 7270* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7271* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7272* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7273* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7274* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7275* [Library](library.html): A use context assigned to the library 7276* [Measure](measure.html): A use context assigned to the measure 7277* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7278* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7279* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7280* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7281* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7282* [Requirements](requirements.html): A use context assigned to the requirements 7283* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7284* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7285* [StructureMap](structuremap.html): A use context assigned to the structure map 7286* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7287* [TestScript](testscript.html): A use context assigned to the test script 7288* [ValueSet](valueset.html): A use context assigned to the value set 7289</b><br> 7290 * Type: <b>token</b><br> 7291 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7292 * </p> 7293 */ 7294 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 7295 7296 /** 7297 * Search parameter: <b>date</b> 7298 * <p> 7299 * Description: <b>Multiple Resources: 7300 7301* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7302* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7303* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7304* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7305* [Citation](citation.html): The citation publication date 7306* [CodeSystem](codesystem.html): The code system publication date 7307* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7308* [ConceptMap](conceptmap.html): The concept map publication date 7309* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7310* [EventDefinition](eventdefinition.html): The event definition publication date 7311* [Evidence](evidence.html): The evidence publication date 7312* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7313* [ExampleScenario](examplescenario.html): The example scenario publication date 7314* [GraphDefinition](graphdefinition.html): The graph definition publication date 7315* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7316* [Library](library.html): The library publication date 7317* [Measure](measure.html): The measure publication date 7318* [MessageDefinition](messagedefinition.html): The message definition publication date 7319* [NamingSystem](namingsystem.html): The naming system publication date 7320* [OperationDefinition](operationdefinition.html): The operation definition publication date 7321* [PlanDefinition](plandefinition.html): The plan definition publication date 7322* [Questionnaire](questionnaire.html): The questionnaire publication date 7323* [Requirements](requirements.html): The requirements publication date 7324* [SearchParameter](searchparameter.html): The search parameter publication date 7325* [StructureDefinition](structuredefinition.html): The structure definition publication date 7326* [StructureMap](structuremap.html): The structure map publication date 7327* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7328* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7329* [TestScript](testscript.html): The test script publication date 7330* [ValueSet](valueset.html): The value set publication date 7331</b><br> 7332 * Type: <b>date</b><br> 7333 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7334 * </p> 7335 */ 7336 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 7337 public static final String SP_DATE = "date"; 7338 /** 7339 * <b>Fluent Client</b> search parameter constant for <b>date</b> 7340 * <p> 7341 * Description: <b>Multiple Resources: 7342 7343* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7344* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7345* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7346* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7347* [Citation](citation.html): The citation publication date 7348* [CodeSystem](codesystem.html): The code system publication date 7349* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7350* [ConceptMap](conceptmap.html): The concept map publication date 7351* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7352* [EventDefinition](eventdefinition.html): The event definition publication date 7353* [Evidence](evidence.html): The evidence publication date 7354* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7355* [ExampleScenario](examplescenario.html): The example scenario publication date 7356* [GraphDefinition](graphdefinition.html): The graph definition publication date 7357* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7358* [Library](library.html): The library publication date 7359* [Measure](measure.html): The measure publication date 7360* [MessageDefinition](messagedefinition.html): The message definition publication date 7361* [NamingSystem](namingsystem.html): The naming system publication date 7362* [OperationDefinition](operationdefinition.html): The operation definition publication date 7363* [PlanDefinition](plandefinition.html): The plan definition publication date 7364* [Questionnaire](questionnaire.html): The questionnaire publication date 7365* [Requirements](requirements.html): The requirements publication date 7366* [SearchParameter](searchparameter.html): The search parameter publication date 7367* [StructureDefinition](structuredefinition.html): The structure definition publication date 7368* [StructureMap](structuremap.html): The structure map publication date 7369* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7370* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7371* [TestScript](testscript.html): The test script publication date 7372* [ValueSet](valueset.html): The value set publication date 7373</b><br> 7374 * Type: <b>date</b><br> 7375 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7376 * </p> 7377 */ 7378 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 7379 7380 /** 7381 * Search parameter: <b>description</b> 7382 * <p> 7383 * Description: <b>Multiple Resources: 7384 7385* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7386* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7387* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7388* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7389* [Citation](citation.html): The description of the citation 7390* [CodeSystem](codesystem.html): The description of the code system 7391* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7392* [ConceptMap](conceptmap.html): The description of the concept map 7393* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7394* [EventDefinition](eventdefinition.html): The description of the event definition 7395* [Evidence](evidence.html): The description of the evidence 7396* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7397* [GraphDefinition](graphdefinition.html): The description of the graph definition 7398* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7399* [Library](library.html): The description of the library 7400* [Measure](measure.html): The description of the measure 7401* [MessageDefinition](messagedefinition.html): The description of the message definition 7402* [NamingSystem](namingsystem.html): The description of the naming system 7403* [OperationDefinition](operationdefinition.html): The description of the operation definition 7404* [PlanDefinition](plandefinition.html): The description of the plan definition 7405* [Questionnaire](questionnaire.html): The description of the questionnaire 7406* [Requirements](requirements.html): The description of the requirements 7407* [SearchParameter](searchparameter.html): The description of the search parameter 7408* [StructureDefinition](structuredefinition.html): The description of the structure definition 7409* [StructureMap](structuremap.html): The description of the structure map 7410* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7411* [TestScript](testscript.html): The description of the test script 7412* [ValueSet](valueset.html): The description of the value set 7413</b><br> 7414 * Type: <b>string</b><br> 7415 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7416 * </p> 7417 */ 7418 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 7419 public static final String SP_DESCRIPTION = "description"; 7420 /** 7421 * <b>Fluent Client</b> search parameter constant for <b>description</b> 7422 * <p> 7423 * Description: <b>Multiple Resources: 7424 7425* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7426* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7427* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7428* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7429* [Citation](citation.html): The description of the citation 7430* [CodeSystem](codesystem.html): The description of the code system 7431* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7432* [ConceptMap](conceptmap.html): The description of the concept map 7433* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7434* [EventDefinition](eventdefinition.html): The description of the event definition 7435* [Evidence](evidence.html): The description of the evidence 7436* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7437* [GraphDefinition](graphdefinition.html): The description of the graph definition 7438* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7439* [Library](library.html): The description of the library 7440* [Measure](measure.html): The description of the measure 7441* [MessageDefinition](messagedefinition.html): The description of the message definition 7442* [NamingSystem](namingsystem.html): The description of the naming system 7443* [OperationDefinition](operationdefinition.html): The description of the operation definition 7444* [PlanDefinition](plandefinition.html): The description of the plan definition 7445* [Questionnaire](questionnaire.html): The description of the questionnaire 7446* [Requirements](requirements.html): The description of the requirements 7447* [SearchParameter](searchparameter.html): The description of the search parameter 7448* [StructureDefinition](structuredefinition.html): The description of the structure definition 7449* [StructureMap](structuremap.html): The description of the structure map 7450* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7451* [TestScript](testscript.html): The description of the test script 7452* [ValueSet](valueset.html): The description of the value set 7453</b><br> 7454 * Type: <b>string</b><br> 7455 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7456 * </p> 7457 */ 7458 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 7459 7460 /** 7461 * Search parameter: <b>identifier</b> 7462 * <p> 7463 * Description: <b>Multiple Resources: 7464 7465* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7466* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7467* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7468* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7469* [Citation](citation.html): External identifier for the citation 7470* [CodeSystem](codesystem.html): External identifier for the code system 7471* [ConceptMap](conceptmap.html): External identifier for the concept map 7472* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7473* [EventDefinition](eventdefinition.html): External identifier for the event definition 7474* [Evidence](evidence.html): External identifier for the evidence 7475* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7476* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7477* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7478* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7479* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7480* [Library](library.html): External identifier for the library 7481* [Measure](measure.html): External identifier for the measure 7482* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7483* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7484* [NamingSystem](namingsystem.html): External identifier for the naming system 7485* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7486* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7487* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7488* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7489* [Requirements](requirements.html): External identifier for the requirements 7490* [SearchParameter](searchparameter.html): External identifier for the search parameter 7491* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7492* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7493* [StructureMap](structuremap.html): External identifier for the structure map 7494* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7495* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7496* [TestPlan](testplan.html): An identifier for the test plan 7497* [TestScript](testscript.html): External identifier for the test script 7498* [ValueSet](valueset.html): External identifier for the value set 7499</b><br> 7500 * Type: <b>token</b><br> 7501 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7502 * </p> 7503 */ 7504 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 7505 public static final String SP_IDENTIFIER = "identifier"; 7506 /** 7507 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7508 * <p> 7509 * Description: <b>Multiple Resources: 7510 7511* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7512* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7513* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7514* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7515* [Citation](citation.html): External identifier for the citation 7516* [CodeSystem](codesystem.html): External identifier for the code system 7517* [ConceptMap](conceptmap.html): External identifier for the concept map 7518* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7519* [EventDefinition](eventdefinition.html): External identifier for the event definition 7520* [Evidence](evidence.html): External identifier for the evidence 7521* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7522* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7523* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7524* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7525* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7526* [Library](library.html): External identifier for the library 7527* [Measure](measure.html): External identifier for the measure 7528* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7529* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7530* [NamingSystem](namingsystem.html): External identifier for the naming system 7531* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7532* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7533* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7534* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7535* [Requirements](requirements.html): External identifier for the requirements 7536* [SearchParameter](searchparameter.html): External identifier for the search parameter 7537* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7538* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7539* [StructureMap](structuremap.html): External identifier for the structure map 7540* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7541* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7542* [TestPlan](testplan.html): An identifier for the test plan 7543* [TestScript](testscript.html): External identifier for the test script 7544* [ValueSet](valueset.html): External identifier for the value set 7545</b><br> 7546 * Type: <b>token</b><br> 7547 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7548 * </p> 7549 */ 7550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7551 7552 /** 7553 * Search parameter: <b>jurisdiction</b> 7554 * <p> 7555 * Description: <b>Multiple Resources: 7556 7557* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7558* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7559* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7560* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7561* [Citation](citation.html): Intended jurisdiction for the citation 7562* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7563* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7564* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7565* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7566* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7567* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7568* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7569* [Library](library.html): Intended jurisdiction for the library 7570* [Measure](measure.html): Intended jurisdiction for the measure 7571* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7572* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7573* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7574* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7575* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7576* [Requirements](requirements.html): Intended jurisdiction for the requirements 7577* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7578* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7579* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7580* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7581* [TestScript](testscript.html): Intended jurisdiction for the test script 7582* [ValueSet](valueset.html): Intended jurisdiction for the value set 7583</b><br> 7584 * Type: <b>token</b><br> 7585 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7586 * </p> 7587 */ 7588 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 7589 public static final String SP_JURISDICTION = "jurisdiction"; 7590 /** 7591 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 7592 * <p> 7593 * Description: <b>Multiple Resources: 7594 7595* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7596* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7597* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7598* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7599* [Citation](citation.html): Intended jurisdiction for the citation 7600* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7601* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7602* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7603* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7604* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7605* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7606* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7607* [Library](library.html): Intended jurisdiction for the library 7608* [Measure](measure.html): Intended jurisdiction for the measure 7609* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7610* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7611* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7612* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7613* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7614* [Requirements](requirements.html): Intended jurisdiction for the requirements 7615* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7616* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7617* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7618* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7619* [TestScript](testscript.html): Intended jurisdiction for the test script 7620* [ValueSet](valueset.html): Intended jurisdiction for the value set 7621</b><br> 7622 * Type: <b>token</b><br> 7623 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7624 * </p> 7625 */ 7626 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 7627 7628 /** 7629 * Search parameter: <b>name</b> 7630 * <p> 7631 * Description: <b>Multiple Resources: 7632 7633* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7634* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7635* [Citation](citation.html): Computationally friendly name of the citation 7636* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7637* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7638* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7639* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7640* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7641* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7642* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7643* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7644* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7645* [Library](library.html): Computationally friendly name of the library 7646* [Measure](measure.html): Computationally friendly name of the measure 7647* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7648* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7649* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7650* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7651* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7652* [Requirements](requirements.html): Computationally friendly name of the requirements 7653* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7654* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7655* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7656* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7657* [TestScript](testscript.html): Computationally friendly name of the test script 7658* [ValueSet](valueset.html): Computationally friendly name of the value set 7659</b><br> 7660 * Type: <b>string</b><br> 7661 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7662 * </p> 7663 */ 7664 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 7665 public static final String SP_NAME = "name"; 7666 /** 7667 * <b>Fluent Client</b> search parameter constant for <b>name</b> 7668 * <p> 7669 * Description: <b>Multiple Resources: 7670 7671* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7672* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7673* [Citation](citation.html): Computationally friendly name of the citation 7674* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7675* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7676* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7677* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7678* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7679* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7680* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7681* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7682* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7683* [Library](library.html): Computationally friendly name of the library 7684* [Measure](measure.html): Computationally friendly name of the measure 7685* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7686* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7687* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7688* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7689* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7690* [Requirements](requirements.html): Computationally friendly name of the requirements 7691* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7692* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7693* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7694* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7695* [TestScript](testscript.html): Computationally friendly name of the test script 7696* [ValueSet](valueset.html): Computationally friendly name of the value set 7697</b><br> 7698 * Type: <b>string</b><br> 7699 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7700 * </p> 7701 */ 7702 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 7703 7704 /** 7705 * Search parameter: <b>publisher</b> 7706 * <p> 7707 * Description: <b>Multiple Resources: 7708 7709* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 7710* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 7711* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 7712* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 7713* [Citation](citation.html): Name of the publisher of the citation 7714* [CodeSystem](codesystem.html): Name of the publisher of the code system 7715* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 7716* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 7717* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 7718* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 7719* [Evidence](evidence.html): Name of the publisher of the evidence 7720* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 7721* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 7722* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 7723* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 7724* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 7725* [Library](library.html): Name of the publisher of the library 7726* [Measure](measure.html): Name of the publisher of the measure 7727* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 7728* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 7729* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 7730* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 7731* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 7732* [Requirements](requirements.html): Name of the publisher of the requirements 7733* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 7734* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 7735* [StructureMap](structuremap.html): Name of the publisher of the structure map 7736* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 7737* [TestScript](testscript.html): Name of the publisher of the test script 7738* [ValueSet](valueset.html): Name of the publisher of the value set 7739</b><br> 7740 * Type: <b>string</b><br> 7741 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 7742 * </p> 7743 */ 7744 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 7745 public static final String SP_PUBLISHER = "publisher"; 7746 /** 7747 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 7748 * <p> 7749 * Description: <b>Multiple Resources: 7750 7751* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 7752* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 7753* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 7754* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 7755* [Citation](citation.html): Name of the publisher of the citation 7756* [CodeSystem](codesystem.html): Name of the publisher of the code system 7757* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 7758* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 7759* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 7760* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 7761* [Evidence](evidence.html): Name of the publisher of the evidence 7762* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 7763* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 7764* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 7765* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 7766* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 7767* [Library](library.html): Name of the publisher of the library 7768* [Measure](measure.html): Name of the publisher of the measure 7769* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 7770* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 7771* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 7772* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 7773* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 7774* [Requirements](requirements.html): Name of the publisher of the requirements 7775* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 7776* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 7777* [StructureMap](structuremap.html): Name of the publisher of the structure map 7778* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 7779* [TestScript](testscript.html): Name of the publisher of the test script 7780* [ValueSet](valueset.html): Name of the publisher of the value set 7781</b><br> 7782 * Type: <b>string</b><br> 7783 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 7784 * </p> 7785 */ 7786 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 7787 7788 /** 7789 * Search parameter: <b>status</b> 7790 * <p> 7791 * Description: <b>Multiple Resources: 7792 7793* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7794* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7795* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7796* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7797* [Citation](citation.html): The current status of the citation 7798* [CodeSystem](codesystem.html): The current status of the code system 7799* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7800* [ConceptMap](conceptmap.html): The current status of the concept map 7801* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7802* [EventDefinition](eventdefinition.html): The current status of the event definition 7803* [Evidence](evidence.html): The current status of the evidence 7804* [EvidenceReport](evidencereport.html): The current status of the evidence report 7805* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7806* [ExampleScenario](examplescenario.html): The current status of the example scenario 7807* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7808* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7809* [Library](library.html): The current status of the library 7810* [Measure](measure.html): The current status of the measure 7811* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7812* [MessageDefinition](messagedefinition.html): The current status of the message definition 7813* [NamingSystem](namingsystem.html): The current status of the naming system 7814* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7815* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7816* [PlanDefinition](plandefinition.html): The current status of the plan definition 7817* [Questionnaire](questionnaire.html): The current status of the questionnaire 7818* [Requirements](requirements.html): The current status of the requirements 7819* [SearchParameter](searchparameter.html): The current status of the search parameter 7820* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7821* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7822* [StructureMap](structuremap.html): The current status of the structure map 7823* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7824* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7825* [TestPlan](testplan.html): The current status of the test plan 7826* [TestScript](testscript.html): The current status of the test script 7827* [ValueSet](valueset.html): The current status of the value set 7828</b><br> 7829 * Type: <b>token</b><br> 7830 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7831 * </p> 7832 */ 7833 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 7834 public static final String SP_STATUS = "status"; 7835 /** 7836 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7837 * <p> 7838 * Description: <b>Multiple Resources: 7839 7840* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7841* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7842* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7843* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7844* [Citation](citation.html): The current status of the citation 7845* [CodeSystem](codesystem.html): The current status of the code system 7846* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7847* [ConceptMap](conceptmap.html): The current status of the concept map 7848* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7849* [EventDefinition](eventdefinition.html): The current status of the event definition 7850* [Evidence](evidence.html): The current status of the evidence 7851* [EvidenceReport](evidencereport.html): The current status of the evidence report 7852* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7853* [ExampleScenario](examplescenario.html): The current status of the example scenario 7854* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7855* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7856* [Library](library.html): The current status of the library 7857* [Measure](measure.html): The current status of the measure 7858* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7859* [MessageDefinition](messagedefinition.html): The current status of the message definition 7860* [NamingSystem](namingsystem.html): The current status of the naming system 7861* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7862* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7863* [PlanDefinition](plandefinition.html): The current status of the plan definition 7864* [Questionnaire](questionnaire.html): The current status of the questionnaire 7865* [Requirements](requirements.html): The current status of the requirements 7866* [SearchParameter](searchparameter.html): The current status of the search parameter 7867* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7868* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7869* [StructureMap](structuremap.html): The current status of the structure map 7870* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7871* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7872* [TestPlan](testplan.html): The current status of the test plan 7873* [TestScript](testscript.html): The current status of the test script 7874* [ValueSet](valueset.html): The current status of the value set 7875</b><br> 7876 * Type: <b>token</b><br> 7877 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7878 * </p> 7879 */ 7880 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 7881 7882 /** 7883 * Search parameter: <b>title</b> 7884 * <p> 7885 * Description: <b>Multiple Resources: 7886 7887* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 7888* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 7889* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 7890* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 7891* [Citation](citation.html): The human-friendly name of the citation 7892* [CodeSystem](codesystem.html): The human-friendly name of the code system 7893* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 7894* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 7895* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 7896* [Evidence](evidence.html): The human-friendly name of the evidence 7897* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 7898* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 7899* [Library](library.html): The human-friendly name of the library 7900* [Measure](measure.html): The human-friendly name of the measure 7901* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 7902* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 7903* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 7904* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 7905* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 7906* [Requirements](requirements.html): The human-friendly name of the requirements 7907* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 7908* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 7909* [StructureMap](structuremap.html): The human-friendly name of the structure map 7910* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 7911* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 7912* [TestScript](testscript.html): The human-friendly name of the test script 7913* [ValueSet](valueset.html): The human-friendly name of the value set 7914</b><br> 7915 * Type: <b>string</b><br> 7916 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 7917 * </p> 7918 */ 7919 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 7920 public static final String SP_TITLE = "title"; 7921 /** 7922 * <b>Fluent Client</b> search parameter constant for <b>title</b> 7923 * <p> 7924 * Description: <b>Multiple Resources: 7925 7926* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 7927* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 7928* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 7929* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 7930* [Citation](citation.html): The human-friendly name of the citation 7931* [CodeSystem](codesystem.html): The human-friendly name of the code system 7932* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 7933* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 7934* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 7935* [Evidence](evidence.html): The human-friendly name of the evidence 7936* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 7937* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 7938* [Library](library.html): The human-friendly name of the library 7939* [Measure](measure.html): The human-friendly name of the measure 7940* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 7941* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 7942* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 7943* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 7944* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 7945* [Requirements](requirements.html): The human-friendly name of the requirements 7946* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 7947* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 7948* [StructureMap](structuremap.html): The human-friendly name of the structure map 7949* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 7950* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 7951* [TestScript](testscript.html): The human-friendly name of the test script 7952* [ValueSet](valueset.html): The human-friendly name of the value set 7953</b><br> 7954 * Type: <b>string</b><br> 7955 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 7956 * </p> 7957 */ 7958 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 7959 7960 /** 7961 * Search parameter: <b>url</b> 7962 * <p> 7963 * Description: <b>Multiple Resources: 7964 7965* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 7966* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 7967* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 7968* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 7969* [Citation](citation.html): The uri that identifies the citation 7970* [CodeSystem](codesystem.html): The uri that identifies the code system 7971* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 7972* [ConceptMap](conceptmap.html): The URI that identifies the concept map 7973* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 7974* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 7975* [Evidence](evidence.html): The uri that identifies the evidence 7976* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 7977* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 7978* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 7979* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 7980* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 7981* [Library](library.html): The uri that identifies the library 7982* [Measure](measure.html): The uri that identifies the measure 7983* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 7984* [NamingSystem](namingsystem.html): The uri that identifies the naming system 7985* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 7986* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 7987* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 7988* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 7989* [Requirements](requirements.html): The uri that identifies the requirements 7990* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 7991* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 7992* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 7993* [StructureMap](structuremap.html): The uri that identifies the structure map 7994* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 7995* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 7996* [TestPlan](testplan.html): The uri that identifies the test plan 7997* [TestScript](testscript.html): The uri that identifies the test script 7998* [ValueSet](valueset.html): The uri that identifies the value set 7999</b><br> 8000 * Type: <b>uri</b><br> 8001 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8002 * </p> 8003 */ 8004 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 8005 public static final String SP_URL = "url"; 8006 /** 8007 * <b>Fluent Client</b> search parameter constant for <b>url</b> 8008 * <p> 8009 * Description: <b>Multiple Resources: 8010 8011* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8012* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8013* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8014* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8015* [Citation](citation.html): The uri that identifies the citation 8016* [CodeSystem](codesystem.html): The uri that identifies the code system 8017* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8018* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8019* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8020* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8021* [Evidence](evidence.html): The uri that identifies the evidence 8022* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8023* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8024* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8025* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8026* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8027* [Library](library.html): The uri that identifies the library 8028* [Measure](measure.html): The uri that identifies the measure 8029* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8030* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8031* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8032* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8033* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8034* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8035* [Requirements](requirements.html): The uri that identifies the requirements 8036* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8037* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8038* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8039* [StructureMap](structuremap.html): The uri that identifies the structure map 8040* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8041* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8042* [TestPlan](testplan.html): The uri that identifies the test plan 8043* [TestScript](testscript.html): The uri that identifies the test script 8044* [ValueSet](valueset.html): The uri that identifies the value set 8045</b><br> 8046 * Type: <b>uri</b><br> 8047 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8048 * </p> 8049 */ 8050 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 8051 8052 /** 8053 * Search parameter: <b>version</b> 8054 * <p> 8055 * Description: <b>Multiple Resources: 8056 8057* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8058* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8059* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8060* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8061* [Citation](citation.html): The business version of the citation 8062* [CodeSystem](codesystem.html): The business version of the code system 8063* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8064* [ConceptMap](conceptmap.html): The business version of the concept map 8065* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8066* [EventDefinition](eventdefinition.html): The business version of the event definition 8067* [Evidence](evidence.html): The business version of the evidence 8068* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8069* [ExampleScenario](examplescenario.html): The business version of the example scenario 8070* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8071* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8072* [Library](library.html): The business version of the library 8073* [Measure](measure.html): The business version of the measure 8074* [MessageDefinition](messagedefinition.html): The business version of the message definition 8075* [NamingSystem](namingsystem.html): The business version of the naming system 8076* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8077* [PlanDefinition](plandefinition.html): The business version of the plan definition 8078* [Questionnaire](questionnaire.html): The business version of the questionnaire 8079* [Requirements](requirements.html): The business version of the requirements 8080* [SearchParameter](searchparameter.html): The business version of the search parameter 8081* [StructureDefinition](structuredefinition.html): The business version of the structure definition 8082* [StructureMap](structuremap.html): The business version of the structure map 8083* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 8084* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 8085* [TestScript](testscript.html): The business version of the test script 8086* [ValueSet](valueset.html): The business version of the value set 8087</b><br> 8088 * Type: <b>token</b><br> 8089 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 8090 * </p> 8091 */ 8092 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 8093 public static final String SP_VERSION = "version"; 8094 /** 8095 * <b>Fluent Client</b> search parameter constant for <b>version</b> 8096 * <p> 8097 * Description: <b>Multiple Resources: 8098 8099* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8100* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8101* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8102* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8103* [Citation](citation.html): The business version of the citation 8104* [CodeSystem](codesystem.html): The business version of the code system 8105* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8106* [ConceptMap](conceptmap.html): The business version of the concept map 8107* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8108* [EventDefinition](eventdefinition.html): The business version of the event definition 8109* [Evidence](evidence.html): The business version of the evidence 8110* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8111* [ExampleScenario](examplescenario.html): The business version of the example scenario 8112* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8113* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8114* [Library](library.html): The business version of the library 8115* [Measure](measure.html): The business version of the measure 8116* [MessageDefinition](messagedefinition.html): The business version of the message definition 8117* [NamingSystem](namingsystem.html): The business version of the naming system 8118* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8119* [PlanDefinition](plandefinition.html): The business version of the plan definition 8120* [Questionnaire](questionnaire.html): The business version of the questionnaire 8121* [Requirements](requirements.html): The business version of the requirements 8122* [SearchParameter](searchparameter.html): The business version of the search parameter 8123* [StructureDefinition](structuredefinition.html): The business version of the structure definition 8124* [StructureMap](structuremap.html): The business version of the structure map 8125* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 8126* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 8127* [TestScript](testscript.html): The business version of the test script 8128* [ValueSet](valueset.html): The business version of the value set 8129</b><br> 8130 * Type: <b>token</b><br> 8131 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 8132 * </p> 8133 */ 8134 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 8135 8136 /** 8137 * Search parameter: <b>mapping-property</b> 8138 * <p> 8139 * Description: <b>Other properties required for this mapping</b><br> 8140 * Type: <b>uri</b><br> 8141 * Path: <b>ConceptMap.property.uri</b><br> 8142 * </p> 8143 */ 8144 @SearchParamDefinition(name="mapping-property", path="ConceptMap.property.uri", description="Other properties required for this mapping", type="uri" ) 8145 public static final String SP_MAPPING_PROPERTY = "mapping-property"; 8146 /** 8147 * <b>Fluent Client</b> search parameter constant for <b>mapping-property</b> 8148 * <p> 8149 * Description: <b>Other properties required for this mapping</b><br> 8150 * Type: <b>uri</b><br> 8151 * Path: <b>ConceptMap.property.uri</b><br> 8152 * </p> 8153 */ 8154 public static final ca.uhn.fhir.rest.gclient.UriClientParam MAPPING_PROPERTY = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_MAPPING_PROPERTY); 8155 8156 /** 8157 * Search parameter: <b>other-map</b> 8158 * <p> 8159 * Description: <b>canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped</b><br> 8160 * Type: <b>reference</b><br> 8161 * Path: <b>ConceptMap.group.unmapped.otherMap</b><br> 8162 * </p> 8163 */ 8164 @SearchParamDefinition(name="other-map", path="ConceptMap.group.unmapped.otherMap", description="canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped", type="reference", target={ConceptMap.class } ) 8165 public static final String SP_OTHER_MAP = "other-map"; 8166 /** 8167 * <b>Fluent Client</b> search parameter constant for <b>other-map</b> 8168 * <p> 8169 * Description: <b>canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped</b><br> 8170 * Type: <b>reference</b><br> 8171 * Path: <b>ConceptMap.group.unmapped.otherMap</b><br> 8172 * </p> 8173 */ 8174 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OTHER_MAP = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OTHER_MAP); 8175 8176/** 8177 * Constant for fluent queries to be used to add include statements. Specifies 8178 * the path value of "<b>ConceptMap:other-map</b>". 8179 */ 8180 public static final ca.uhn.fhir.model.api.Include INCLUDE_OTHER_MAP = new ca.uhn.fhir.model.api.Include("ConceptMap:other-map").toLocked(); 8181 8182 /** 8183 * Search parameter: <b>source-code</b> 8184 * <p> 8185 * Description: <b>Identifies elements being mapped</b><br> 8186 * Type: <b>token</b><br> 8187 * Path: <b>ConceptMap.group.element.code</b><br> 8188 * </p> 8189 */ 8190 @SearchParamDefinition(name="source-code", path="ConceptMap.group.element.code", description="Identifies elements being mapped", type="token" ) 8191 public static final String SP_SOURCE_CODE = "source-code"; 8192 /** 8193 * <b>Fluent Client</b> search parameter constant for <b>source-code</b> 8194 * <p> 8195 * Description: <b>Identifies elements being mapped</b><br> 8196 * Type: <b>token</b><br> 8197 * Path: <b>ConceptMap.group.element.code</b><br> 8198 * </p> 8199 */ 8200 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SOURCE_CODE); 8201 8202 /** 8203 * Search parameter: <b>source-group-system</b> 8204 * <p> 8205 * Description: <b>Source system where concepts to be mapped are defined</b><br> 8206 * Type: <b>reference</b><br> 8207 * Path: <b>ConceptMap.group.source</b><br> 8208 * </p> 8209 */ 8210 @SearchParamDefinition(name="source-group-system", path="ConceptMap.group.source", description="Source system where concepts to be mapped are defined", type="reference", target={CodeSystem.class } ) 8211 public static final String SP_SOURCE_GROUP_SYSTEM = "source-group-system"; 8212 /** 8213 * <b>Fluent Client</b> search parameter constant for <b>source-group-system</b> 8214 * <p> 8215 * Description: <b>Source system where concepts to be mapped are defined</b><br> 8216 * Type: <b>reference</b><br> 8217 * Path: <b>ConceptMap.group.source</b><br> 8218 * </p> 8219 */ 8220 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_GROUP_SYSTEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE_GROUP_SYSTEM); 8221 8222/** 8223 * Constant for fluent queries to be used to add include statements. Specifies 8224 * the path value of "<b>ConceptMap:source-group-system</b>". 8225 */ 8226 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_GROUP_SYSTEM = new ca.uhn.fhir.model.api.Include("ConceptMap:source-group-system").toLocked(); 8227 8228 /** 8229 * Search parameter: <b>source-scope-uri</b> 8230 * <p> 8231 * Description: <b>The URI for the source value set that contains the concepts being mapped</b><br> 8232 * Type: <b>uri</b><br> 8233 * Path: <b>(ConceptMap.sourceScope as uri)</b><br> 8234 * </p> 8235 */ 8236 @SearchParamDefinition(name="source-scope-uri", path="(ConceptMap.sourceScope as uri)", description="The URI for the source value set that contains the concepts being mapped", type="uri" ) 8237 public static final String SP_SOURCE_SCOPE_URI = "source-scope-uri"; 8238 /** 8239 * <b>Fluent Client</b> search parameter constant for <b>source-scope-uri</b> 8240 * <p> 8241 * Description: <b>The URI for the source value set that contains the concepts being mapped</b><br> 8242 * Type: <b>uri</b><br> 8243 * Path: <b>(ConceptMap.sourceScope as uri)</b><br> 8244 * </p> 8245 */ 8246 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE_SCOPE_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SOURCE_SCOPE_URI); 8247 8248 /** 8249 * Search parameter: <b>source-scope</b> 8250 * <p> 8251 * Description: <b>The source value set that contains the concepts that are being mapped</b><br> 8252 * Type: <b>reference</b><br> 8253 * Path: <b>(ConceptMap.sourceScope as canonical)</b><br> 8254 * </p> 8255 */ 8256 @SearchParamDefinition(name="source-scope", path="(ConceptMap.sourceScope as canonical)", description="The source value set that contains the concepts that are being mapped", type="reference", target={ValueSet.class } ) 8257 public static final String SP_SOURCE_SCOPE = "source-scope"; 8258 /** 8259 * <b>Fluent Client</b> search parameter constant for <b>source-scope</b> 8260 * <p> 8261 * Description: <b>The source value set that contains the concepts that are being mapped</b><br> 8262 * Type: <b>reference</b><br> 8263 * Path: <b>(ConceptMap.sourceScope as canonical)</b><br> 8264 * </p> 8265 */ 8266 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_SCOPE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE_SCOPE); 8267 8268/** 8269 * Constant for fluent queries to be used to add include statements. Specifies 8270 * the path value of "<b>ConceptMap:source-scope</b>". 8271 */ 8272 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_SCOPE = new ca.uhn.fhir.model.api.Include("ConceptMap:source-scope").toLocked(); 8273 8274 /** 8275 * Search parameter: <b>target-code</b> 8276 * <p> 8277 * Description: <b>Code that identifies the target element</b><br> 8278 * Type: <b>token</b><br> 8279 * Path: <b>ConceptMap.group.element.target.code</b><br> 8280 * </p> 8281 */ 8282 @SearchParamDefinition(name="target-code", path="ConceptMap.group.element.target.code", description="Code that identifies the target element", type="token" ) 8283 public static final String SP_TARGET_CODE = "target-code"; 8284 /** 8285 * <b>Fluent Client</b> search parameter constant for <b>target-code</b> 8286 * <p> 8287 * Description: <b>Code that identifies the target element</b><br> 8288 * Type: <b>token</b><br> 8289 * Path: <b>ConceptMap.group.element.target.code</b><br> 8290 * </p> 8291 */ 8292 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_CODE); 8293 8294 /** 8295 * Search parameter: <b>target-group-system</b> 8296 * <p> 8297 * Description: <b>Target system that the concepts are to be mapped to</b><br> 8298 * Type: <b>reference</b><br> 8299 * Path: <b>ConceptMap.group.target</b><br> 8300 * </p> 8301 */ 8302 @SearchParamDefinition(name="target-group-system", path="ConceptMap.group.target", description="Target system that the concepts are to be mapped to", type="reference", target={CodeSystem.class } ) 8303 public static final String SP_TARGET_GROUP_SYSTEM = "target-group-system"; 8304 /** 8305 * <b>Fluent Client</b> search parameter constant for <b>target-group-system</b> 8306 * <p> 8307 * Description: <b>Target system that the concepts are to be mapped to</b><br> 8308 * Type: <b>reference</b><br> 8309 * Path: <b>ConceptMap.group.target</b><br> 8310 * </p> 8311 */ 8312 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET_GROUP_SYSTEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET_GROUP_SYSTEM); 8313 8314/** 8315 * Constant for fluent queries to be used to add include statements. Specifies 8316 * the path value of "<b>ConceptMap:target-group-system</b>". 8317 */ 8318 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET_GROUP_SYSTEM = new ca.uhn.fhir.model.api.Include("ConceptMap:target-group-system").toLocked(); 8319 8320 /** 8321 * Search parameter: <b>target-scope-uri</b> 8322 * <p> 8323 * Description: <b>The URI for the target value set that contains the concepts being mapped.</b><br> 8324 * Type: <b>uri</b><br> 8325 * Path: <b>(ConceptMap.targetScope as uri)</b><br> 8326 * </p> 8327 */ 8328 @SearchParamDefinition(name="target-scope-uri", path="(ConceptMap.targetScope as uri)", description="The URI for the target value set that contains the concepts being mapped.", type="uri" ) 8329 public static final String SP_TARGET_SCOPE_URI = "target-scope-uri"; 8330 /** 8331 * <b>Fluent Client</b> search parameter constant for <b>target-scope-uri</b> 8332 * <p> 8333 * Description: <b>The URI for the target value set that contains the concepts being mapped.</b><br> 8334 * Type: <b>uri</b><br> 8335 * Path: <b>(ConceptMap.targetScope as uri)</b><br> 8336 * </p> 8337 */ 8338 public static final ca.uhn.fhir.rest.gclient.UriClientParam TARGET_SCOPE_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_TARGET_SCOPE_URI); 8339 8340 /** 8341 * Search parameter: <b>target-scope</b> 8342 * <p> 8343 * Description: <b>The target value set which provides context for the mappings</b><br> 8344 * Type: <b>reference</b><br> 8345 * Path: <b>(ConceptMap.targetScope as canonical)</b><br> 8346 * </p> 8347 */ 8348 @SearchParamDefinition(name="target-scope", path="(ConceptMap.targetScope as canonical)", description="The target value set which provides context for the mappings", type="reference", target={ValueSet.class } ) 8349 public static final String SP_TARGET_SCOPE = "target-scope"; 8350 /** 8351 * <b>Fluent Client</b> search parameter constant for <b>target-scope</b> 8352 * <p> 8353 * Description: <b>The target value set which provides context for the mappings</b><br> 8354 * Type: <b>reference</b><br> 8355 * Path: <b>(ConceptMap.targetScope as canonical)</b><br> 8356 * </p> 8357 */ 8358 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET_SCOPE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TARGET_SCOPE); 8359 8360/** 8361 * Constant for fluent queries to be used to add include statements. Specifies 8362 * the path value of "<b>ConceptMap:target-scope</b>". 8363 */ 8364 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET_SCOPE = new ca.uhn.fhir.model.api.Include("ConceptMap:target-scope").toLocked(); 8365 8366 /** 8367 * Search parameter: <b>derived-from</b> 8368 * <p> 8369 * Description: <b>Multiple Resources: 8370 8371* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8372* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 8373* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 8374* [EventDefinition](eventdefinition.html): What resource is being referenced 8375* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8376* [Library](library.html): What resource is being referenced 8377* [Measure](measure.html): What resource is being referenced 8378* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 8379* [PlanDefinition](plandefinition.html): What resource is being referenced 8380* [ValueSet](valueset.html): A resource that the ValueSet is derived from 8381</b><br> 8382 * Type: <b>reference</b><br> 8383 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 8384 * </p> 8385 */ 8386 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 8387 public static final String SP_DERIVED_FROM = "derived-from"; 8388 /** 8389 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 8390 * <p> 8391 * Description: <b>Multiple Resources: 8392 8393* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8394* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 8395* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 8396* [EventDefinition](eventdefinition.html): What resource is being referenced 8397* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8398* [Library](library.html): What resource is being referenced 8399* [Measure](measure.html): What resource is being referenced 8400* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 8401* [PlanDefinition](plandefinition.html): What resource is being referenced 8402* [ValueSet](valueset.html): A resource that the ValueSet is derived from 8403</b><br> 8404 * Type: <b>reference</b><br> 8405 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 8406 * </p> 8407 */ 8408 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 8409 8410/** 8411 * Constant for fluent queries to be used to add include statements. Specifies 8412 * the path value of "<b>ConceptMap:derived-from</b>". 8413 */ 8414 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ConceptMap:derived-from").toLocked(); 8415 8416 /** 8417 * Search parameter: <b>effective</b> 8418 * <p> 8419 * Description: <b>Multiple Resources: 8420 8421* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 8422* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 8423* [Citation](citation.html): The time during which the citation is intended to be in use 8424* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 8425* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 8426* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 8427* [Library](library.html): The time during which the library is intended to be in use 8428* [Measure](measure.html): The time during which the measure is intended to be in use 8429* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 8430* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 8431* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 8432* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 8433</b><br> 8434 * Type: <b>date</b><br> 8435 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 8436 * </p> 8437 */ 8438 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 8439 public static final String SP_EFFECTIVE = "effective"; 8440 /** 8441 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 8442 * <p> 8443 * Description: <b>Multiple Resources: 8444 8445* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 8446* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 8447* [Citation](citation.html): The time during which the citation is intended to be in use 8448* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 8449* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 8450* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 8451* [Library](library.html): The time during which the library is intended to be in use 8452* [Measure](measure.html): The time during which the measure is intended to be in use 8453* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 8454* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 8455* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 8456* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 8457</b><br> 8458 * Type: <b>date</b><br> 8459 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 8460 * </p> 8461 */ 8462 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 8463 8464 /** 8465 * Search parameter: <b>predecessor</b> 8466 * <p> 8467 * Description: <b>Multiple Resources: 8468 8469* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8470* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 8471* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 8472* [EventDefinition](eventdefinition.html): What resource is being referenced 8473* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8474* [Library](library.html): What resource is being referenced 8475* [Measure](measure.html): What resource is being referenced 8476* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 8477* [PlanDefinition](plandefinition.html): What resource is being referenced 8478* [ValueSet](valueset.html): The predecessor of the ValueSet 8479</b><br> 8480 * Type: <b>reference</b><br> 8481 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 8482 * </p> 8483 */ 8484 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 8485 public static final String SP_PREDECESSOR = "predecessor"; 8486 /** 8487 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 8488 * <p> 8489 * Description: <b>Multiple Resources: 8490 8491* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8492* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 8493* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 8494* [EventDefinition](eventdefinition.html): What resource is being referenced 8495* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8496* [Library](library.html): What resource is being referenced 8497* [Measure](measure.html): What resource is being referenced 8498* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 8499* [PlanDefinition](plandefinition.html): What resource is being referenced 8500* [ValueSet](valueset.html): The predecessor of the ValueSet 8501</b><br> 8502 * Type: <b>reference</b><br> 8503 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 8504 * </p> 8505 */ 8506 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 8507 8508/** 8509 * Constant for fluent queries to be used to add include statements. Specifies 8510 * the path value of "<b>ConceptMap:predecessor</b>". 8511 */ 8512 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("ConceptMap:predecessor").toLocked(); 8513 8514 /** 8515 * Search parameter: <b>topic</b> 8516 * <p> 8517 * Description: <b>Multiple Resources: 8518 8519* [ActivityDefinition](activitydefinition.html): Topics associated with the module 8520* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 8521* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 8522* [EventDefinition](eventdefinition.html): Topics associated with the module 8523* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 8524* [Library](library.html): Topics associated with the module 8525* [Measure](measure.html): Topics associated with the measure 8526* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 8527* [PlanDefinition](plandefinition.html): Topics associated with the module 8528* [ValueSet](valueset.html): Topics associated with the ValueSet 8529</b><br> 8530 * Type: <b>token</b><br> 8531 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 8532 * </p> 8533 */ 8534 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 8535 public static final String SP_TOPIC = "topic"; 8536 /** 8537 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 8538 * <p> 8539 * Description: <b>Multiple Resources: 8540 8541* [ActivityDefinition](activitydefinition.html): Topics associated with the module 8542* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 8543* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 8544* [EventDefinition](eventdefinition.html): Topics associated with the module 8545* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 8546* [Library](library.html): Topics associated with the module 8547* [Measure](measure.html): Topics associated with the measure 8548* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 8549* [PlanDefinition](plandefinition.html): Topics associated with the module 8550* [ValueSet](valueset.html): Topics associated with the ValueSet 8551</b><br> 8552 * Type: <b>token</b><br> 8553 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 8554 * </p> 8555 */ 8556 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 8557 8558// Manual code (from Configuration.txt): 8559 public String getAttributeUri(String code) { 8560 if (code == null) { 8561 return null; 8562 } 8563 for (AdditionalAttributeComponent aa : getAdditionalAttribute()) { 8564 if (code.equals(aa.getCode())) { 8565 return aa.hasUri() ? aa.getUri() : code; 8566 } 8567 } 8568 return code; 8569 } 8570 8571 public String registerAttribute(String uri) { 8572 if (uri == null) { 8573 return null; 8574 } 8575 // determine a default code 8576 String t = tail(uri).replace("-", ""); 8577 if (Utilities.noString(t)) 8578 t = "code"; 8579 String code = t; 8580 int i = 0; 8581 while (alreadyExistsAsCode(code)) { 8582 i++; 8583 code = t + i; 8584 } 8585 8586 for (AdditionalAttributeComponent aa : getAdditionalAttribute()) { 8587 if (uri.equals(aa.getUri())) { 8588 if (!aa.hasCode()) { 8589 aa.setCode(code); 8590 } 8591 return aa.getCode(); 8592 } 8593 } 8594 addAdditionalAttribute().setUri(uri).setCode(code); 8595 return code; 8596 } 8597 8598 private boolean alreadyExistsAsCode(String code) { 8599 for (PropertyComponent prop : getProperty()) { 8600 if (code.equals(prop.getCode())) { 8601 return true; 8602 } 8603 } 8604 for (AdditionalAttributeComponent prop : getAdditionalAttribute()) { 8605 if (code.equals(prop.getCode())) { 8606 return true; 8607 } 8608 } 8609 return false; 8610 } 8611 8612 private String tail(String uri) { 8613 return uri.contains("/") ? uri.substring(uri.lastIndexOf("/")+1) : uri; 8614 } 8615 8616 public ConceptMapGroupComponent getGroup(String su, String tu) { 8617 for (ConceptMapGroupComponent g : getGroup()) { 8618 if (su.equals(g.getSource()) && tu.equals(g.getTarget())) { 8619 return g; 8620 } 8621 } 8622 return null; 8623 } 8624 8625 public ConceptMapGroupComponent forceGroup(String su, String tu) { 8626 for (ConceptMapGroupComponent g : getGroup()) { 8627 if (su.equals(g.getSource()) && tu.equals(g.getTarget())) { 8628 return g; 8629 } 8630 } 8631 ConceptMapGroupComponent g = addGroup(); 8632 g.setSource(su); 8633 g.setTarget(tu); 8634 return g; 8635 8636 } 8637 8638 public List<ConceptMapGroupComponent> getGroups(String su) { 8639 List<ConceptMapGroupComponent> res = new ArrayList<>(); 8640 8641 for (ConceptMapGroupComponent g : getGroup()) { 8642 if (su.equals(g.getSource())) { 8643 res.add(g); 8644 } 8645 } 8646 return res; 8647 } 8648 8649// end addition 8650 8651 8652} 8653